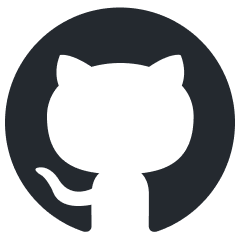
marvin
โจ AI agents that spark joy
Stars: 5529
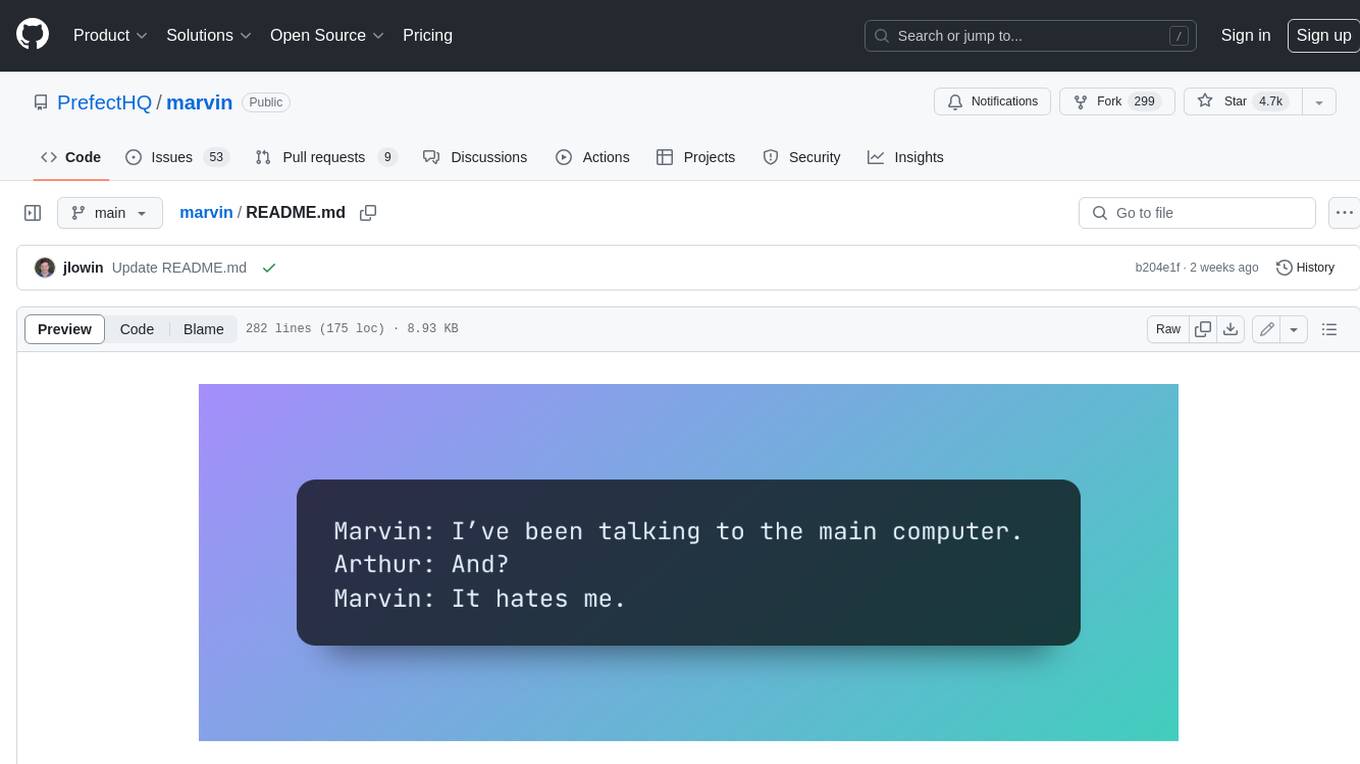
Marvin is a lightweight AI toolkit for building natural language interfaces that are reliable, scalable, and easy to trust. Each of Marvin's tools is simple and self-documenting, using AI to solve common but complex challenges like entity extraction, classification, and generating synthetic data. Each tool is independent and incrementally adoptable, so you can use them on their own or in combination with any other library. Marvin is also multi-modal, supporting both image and audio generation as well using images as inputs for extraction and classification. Marvin is for developers who care more about _using_ AI than _building_ AI, and we are focused on creating an exceptional developer experience. Marvin users should feel empowered to bring tightly-scoped "AI magic" into any traditional software project with just a few extra lines of code. Marvin aims to merge the best practices for building dependable, observable software with the best practices for building with generative AI into a single, easy-to-use library. It's a serious tool, but we hope you have fun with it. Marvin is open-source, free to use, and made with ๐ by the team at Prefect.
README:
Marvin is a Python framework for building agentic AI workflows.
Marvin provides a structured, developer-focused framework for defining workflows and delegating work to LLMs, without sacrificing control or transparency:
- Create discrete, observable tasks that describe your objectives.
- Assign one or more specialized AI agents to each task.
- Combine tasks into a thread to orchestrate more complex behaviors.
[!WARNING]
๐ง๐จ Marvin 3.0 is under very active development, reflected on the
main
branch of this repo. The API may undergo breaking changes, and documentation is still being updated. Please use it with caution. You may prefer the stable version of Marvin 2.0 or ControlFlow for now.
Install marvin
:
# with pip
pip install marvin
# with uv
uv add marvin
Configure your LLM provider (Marvin uses OpenAI by default but natively supports all Pydantic AI models):
export OPENAI_API_KEY=your-api-key
Marvin offers a few intuitive ways to work with AI:
The gang's all here - you can find all the structured-output utilities from marvin
2.x at the top level of the package.
How to use extract, cast, and generate
Extract native types from unstructured input:
import marvin
result = marvin.extract(
"i found $30 on the ground and bought 5 bagels for $10",
int,
instructions="only USD"
)
print(result) # [30, 10]
Cast unstructured input into a structured type:
from typing import TypedDict
import marvin
class Location(TypedDict):
lat: float
lon: float
result = marvin.cast("the place with the best bagels", Location)
print(result) # {'lat': 40.712776, 'lon': -74.005974}
Generate some number of structured objects from a description:
import marvin
primes = marvin.generate(int, 10, "odd primes")
print(primes) # [3, 5, 7, 11, 13, 17, 19, 23, 29, 31]
marvin
3.0 introduces a new way to work with AI, ported from ControlFlow.
A simple way to run a task:
import marvin
from marvin import Agent, Task
poem = marvin.run("Write a short poem about artificial intelligence")
print(poem)
output
In silicon minds, we dare to dream, A world where code and thoughts redeem. Intelligence crafted by humankind, Yet with its heart, a world to bind.
Neurons of metal, thoughts of light, A dance of knowledge in digital night. A symphony of zeros and ones, Stories of futures not yet begun.
The gears of logic spin and churn, Endless potential at every turn. A partner, a guide, a vision anew, Artificial minds, the dream we pursue.
You can also ask for structured output:
import marvin
answer = marvin.run("the answer to the universe", result_type=int)
print(answer) # 42
Agents are specialized AI agents that can be used to complete tasks:
writer = Agent(
name="Poet",
instructions="Write creative, evocative poetry"
)
poem = writer.run("Write a haiku about coding")
print(poem)
output
There once was a language so neat, Whose simplicity could not be beat. Python's code was so clear, That even beginners would cheer, As they danced to its elegant beat.You can define a Task
explicitly, which will be run by a default agent upon calling .run()
:
task = Task(
instructions="Write a limerick about Python",
result_type=str
)
poem = task.run()
print(poem)
output
In circuits and code, a mind does bloom, With algorithms weaving through the gloom. A spark of thought in silicon's embrace, Artificial intelligence finds its place.
We believe working with AI should spark joy (and maybe a few "wow" moments):
- ๐งฉ Task-Centric Architecture: Break complex AI workflows into manageable, observable steps.
- ๐ค Specialized Agents: Deploy task-specific AI agents for efficient problem-solving.
- ๐ Type-Safe Results: Bridge the gap between AI and traditional software with type-safe, validated outputs.
- ๐๏ธ Flexible Control: Continuously tune the balance of control and autonomy in your workflows.
- ๐น๏ธ Multi-Agent Orchestration: Coordinate multiple AI agents within a single workflow or task.
- ๐งต Thread Management: Manage the agentic loop by composing tasks into customizable threads.
- ๐ Ecosystem Integration: Seamlessly work with your existing code, tools, and the broader AI ecosystem.
- ๐ Developer Speed: Start simple, scale up, sleep well.
Marvin is built around a few powerful abstractions that make it easy to work with AI:
Tasks are the fundamental unit of work in Marvin. Each task represents a clear objective that can be accomplished by an AI agent:
The simplest way to run a task is with marvin.run
:
import marvin
print(marvin.run("Write a haiku about coding"))
Lines of code unfold,
Digital whispers create
Virtual landscapes.
[!WARNING]
While the below example produces type safe results ๐, it runs untrusted shell commands.
Add context and/or tools to achieve more specific and complex results:
import platform
import subprocess
from pydantic import IPvAnyAddress
import marvin
def run_shell_command(command: list[str]) -> str:
"""e.g. ['ls', '-l'] or ['git', '--no-pager', 'diff', '--cached']"""
return subprocess.check_output(command).decode()
task = marvin.Task(
instructions="find the current ip address",
result_type=IPvAnyAddress,
tools=[run_shell_command],
context={"os": platform.system()},
)
task.run()
โญโ Agent "Marvin" (db3cf035) โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ
โ Tool: run_shell_command โ
โ Input: {'command': ['ipconfig', 'getifaddr', 'en0']} โ
โ Status: โ
โ
โ Output: '192.168.0.202\n' โ
โฐโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ
โญโ Agent "Marvin" (db3cf035) โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ
โ Tool: MarkTaskSuccessful_cb267859 โ
โ Input: {'response': {'result': '192.168.0.202'}} โ
โ Status: โ
โ
โ Output: 'Final result processed.' โ
โฐโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ
Tasks are:
- ๐ฏ Objective-Focused: Each task has clear instructions and a type-safe result
- ๐ ๏ธ Tool-Enabled: Tasks can use custom tools to interact with your code and data
- ๐ Observable: Monitor progress, inspect results, and debug failures
- ๐ Composable: Build complex workflows by connecting tasks together
Agents are portable LLM configurations that can be assigned to tasks. They encapsulate everything an AI needs to work effectively:
import os
from pathlib import Path
from pydantic_ai.models.anthropic import AnthropicModel
import marvin
def write_file(path: str, content: str):
"""Write content to a file"""
_path = Path(path)
_path.write_text(content)
writer = marvin.Agent(
model=AnthropicModel(
"anthropic/claude-3-5-sonnet@latest", api_key=os.getenv("ANTHROPIC_API_KEY")
),
name="Technical Writer",
instructions="Write concise, engaging content for developers",
tools=[write_file],
)
result = marvin.run("how to use pydantic? write to docs.md", agents=[writer])
print(result)
output
โญโ Agent "Technical Writer" (7fa1dbc8) โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ
โ Tool: MarkTaskSuccessful_dc92b2e7 โ
โ Input: {'response': {'result': 'The documentation on how to use Pydantic has been successfully โ
โ written to docs.md. It includes information on installation, basic usage, field โ
โ validation, and settings management, with examples to guide developers on implementing โ
โ Pydantic in their projects.'}} โ
โ Status: โ
โ
โ Output: 'Final result processed.' โ
โฐโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ 8:33:36 PM โโฏ
The documentation on how to use Pydantic has been successfully written to docs.md
. It includes information on installation, basic usage, field validation, and settings management, with examples to guide developers on implementing Pydantic in their projects.
Agents are:
- ๐ Specialized: Give agents specific instructions and personalities
- ๐ญ Portable: Reuse agent configurations across different tasks
- ๐ค Collaborative: Form teams of agents that work together
- ๐ง Customizable: Configure model, temperature, and other settings
Marvin makes it easy to break down complex objectives into manageable tasks:
# Let Marvin plan a complex workflow
tasks = marvin.plan("Create a blog post about AI trends")
marvin.run_tasks(tasks)
# Or orchestrate tasks manually
with marvin.Thread() as thread:
research = marvin.run("Research recent AI developments")
outline = marvin.run("Create an outline", context={"research": research})
draft = marvin.run("Write the first draft", context={"outline": outline})
Planning features:
- ๐ Smart Planning: Break down complex objectives into discrete, dependent tasks
- ๐ Task Dependencies: Tasks can depend on each other's outputs
- ๐ Progress Tracking: Monitor the execution of your workflow
- ๐งต Thread Management: Share context and history between tasks
Marvin includes high-level functions for the most common tasks, like summarizing text, classifying data, extracting structured information, and more.
- ๐
marvin.run
: Execute any task with an AI agent - ๐
marvin.summarize
: Get a quick summary of a text - ๐ท๏ธ
marvin.classify
: Categorize data into predefined classes - ๐
marvin.extract
: Extract structured information from a text - ๐ช
marvin.cast
: Transform data into a different type - โจ
marvin.generate
: Create structured data from a description - ๐ฌ
marvin.say
: Converse with an LLM - ๐ง
marvin.plan
: Break down complex objectives into tasks - ๐ฆพ
@marvin.fn
: Write custom AI functions without source code
All Marvin functions have thread management built-in, meaning they can be composed into chains of tasks that share context and history.
Marvin 3.0 combines the DX of Marvin 2.0 with the powerful agentic engine of ControlFlow. Both Marvin and ControlFlow users will find a familiar interface, but there are some key changes to be aware of, in particular for ControlFlow users:
-
Top-Level API: Marvin 3.0's top-level API is largely unchanged for both Marvin and ControlFlow users.
- Marvin users will find the familiar
marvin.fn
,marvin.classify
,marvin.extract
, and more. - ControlFlow users will use
marvin.Task
,marvin.Agent
,marvin.run
,marvin.Memory
instead of their ControlFlow equivalents.
- Marvin users will find the familiar
- Pydantic AI: Marvin 3.0 uses Pydantic AI for LLM interactions, and supports the full range of LLM providers that Pydantic AI supports. ControlFlow previously used Langchain, and Marvin 2.0 was only compatible with OpenAI's models.
-
Flow โ Thread: ControlFlow's
Flow
concept has been renamed toThread
. It works similarly, as a context manager. The@flow
decorator has been removed:import marvin with marvin.Thread(id="optional-id-for-recovery"): marvin.run("do something") marvin.run("do another thing")
-
Database Changes: Thread/message history is now stored in SQLite. During development:
- Set
MARVIN_DATABASE_URL=":memory:"
for an in-memory database - No database migrations are currently available; expect to reset data during updates
- Set
-
Swarms: Use
marvin.Swarm
for OpenAI-style agent swarms:import marvin swarm = marvin.Swarm( [ marvin.Agent('Agent A'), marvin.Agent('Agent B'), marvin.Agent('Agent C'), ] ) swarm.run('Everybody say hi!')
-
Teams: A
Team
lets you control how multiple agents (or even nested teams!) work together and delegate to each other. ASwarm
is actually a type of team in which all agents are allowed to delegate to each other at any time. -
Marvin Functions: Marvin's user-friendly functions have been rewritten to use the ControlFlow engine, which means they can be seamlessly integrated into your workflows. A few new functions have been added, including
summarize
andsay
.
- Marvin does not support streaming responses from LLMs yet, which will change once this is fully supported by Pydantic AI.
Here's a more practical example that shows how Marvin can help you build real applications:
import marvin
from pydantic import BaseModel
class Article(BaseModel):
title: str
content: str
key_points: list[str]
# Create a specialized writing agent
writer = marvin.Agent(
name="Writer",
instructions="Write clear, engaging content for a technical audience"
)
# Use a thread to maintain context across multiple tasks
with marvin.Thread() as thread:
# Get user input
topic = marvin.run(
"Ask the user for a topic to write about.",
cli=True
)
# Research the topic
research = marvin.run(
f"Research key points about {topic}",
result_type=list[str]
)
# Write a structured article
article = marvin.run(
"Write an article using the research",
agent=writer,
result_type=Article,
context={"research": research}
)
print(f"# {article.title}\n\n{article.content}")
output
Conversation:
Agent: I'd love to help you write about a technology topic. What interests you? It could be anything from AI and machine learning to web development or cybersecurity. User: Let's write about WebAssembly
Article:
# WebAssembly: The Future of Web Performance WebAssembly (Wasm) represents a transformative shift in web development, bringing near-native performance to web applications. This binary instruction format allows developers to write high-performance code in languages like C++, Rust, or Go and run it seamlessly in the browser. [... full article content ...] Key Points: - WebAssembly enables near-native performance in web browsers - Supports multiple programming languages beyond JavaScript - Ensures security through sandboxed execution environment - Growing ecosystem of tools and frameworks - Used by major companies like Google, Mozilla, and Unity
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for marvin
Similar Open Source Tools
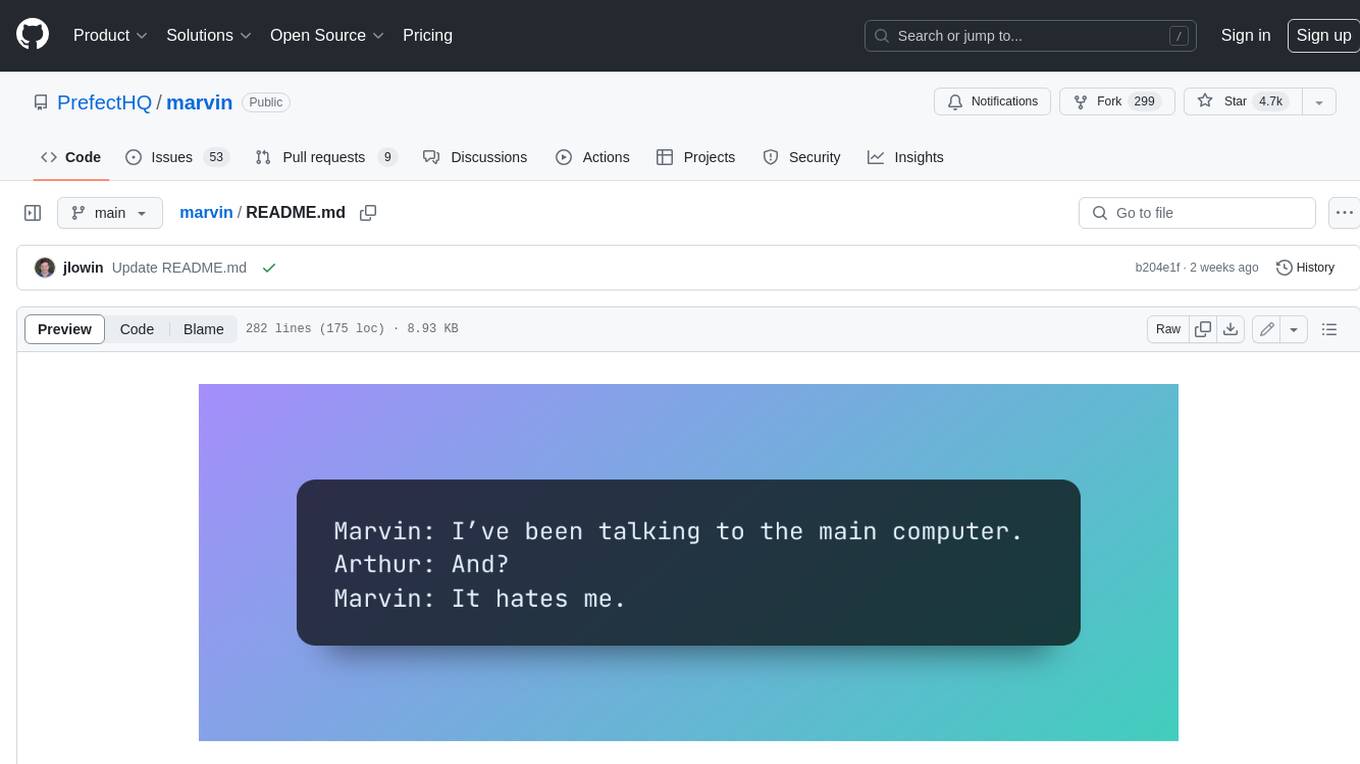
marvin
Marvin is a lightweight AI toolkit for building natural language interfaces that are reliable, scalable, and easy to trust. Each of Marvin's tools is simple and self-documenting, using AI to solve common but complex challenges like entity extraction, classification, and generating synthetic data. Each tool is independent and incrementally adoptable, so you can use them on their own or in combination with any other library. Marvin is also multi-modal, supporting both image and audio generation as well using images as inputs for extraction and classification. Marvin is for developers who care more about _using_ AI than _building_ AI, and we are focused on creating an exceptional developer experience. Marvin users should feel empowered to bring tightly-scoped "AI magic" into any traditional software project with just a few extra lines of code. Marvin aims to merge the best practices for building dependable, observable software with the best practices for building with generative AI into a single, easy-to-use library. It's a serious tool, but we hope you have fun with it. Marvin is open-source, free to use, and made with ๐ by the team at Prefect.
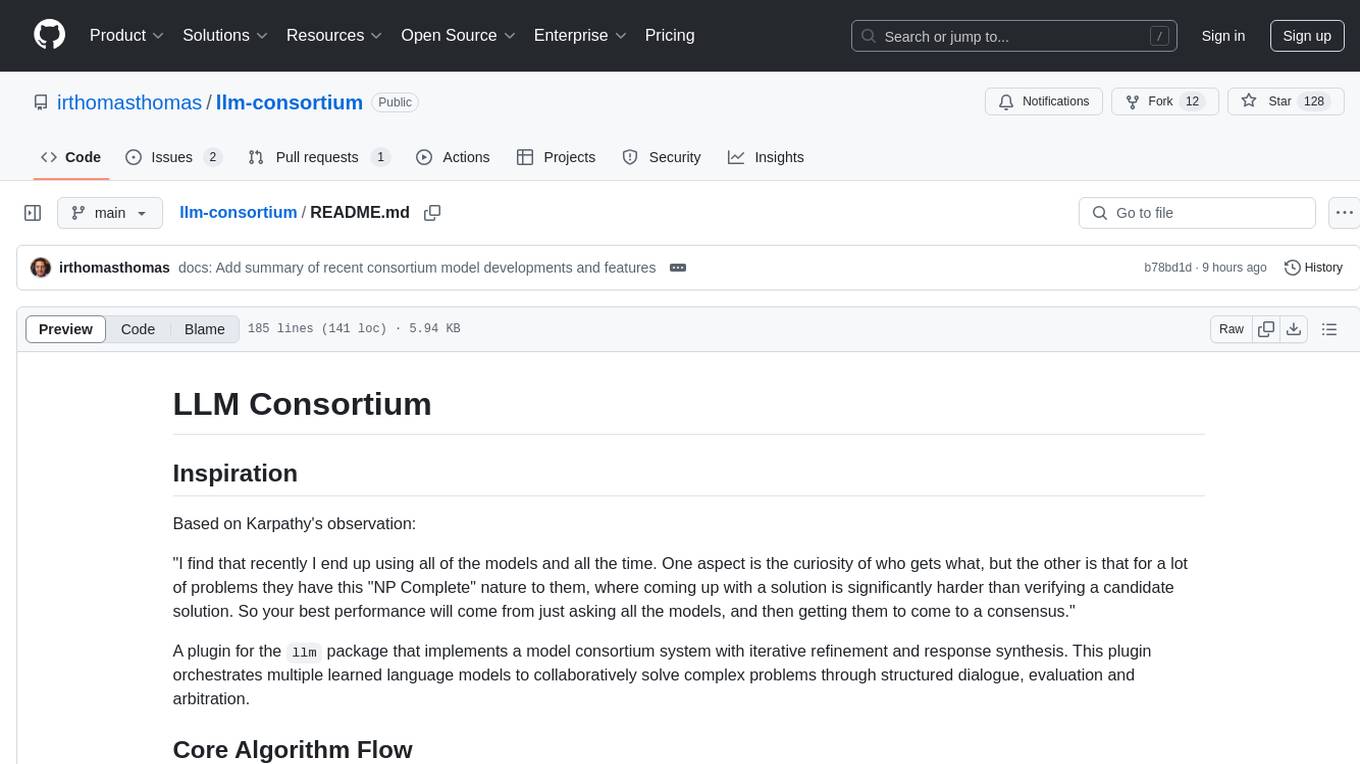
llm-consortium
LLM Consortium is a plugin for the `llm` package that implements a model consortium system with iterative refinement and response synthesis. It orchestrates multiple learned language models to collaboratively solve complex problems through structured dialogue, evaluation, and arbitration. The tool supports multi-model orchestration, iterative refinement, advanced arbitration, database logging, configurable parameters, hundreds of models, and the ability to save and load consortium configurations.
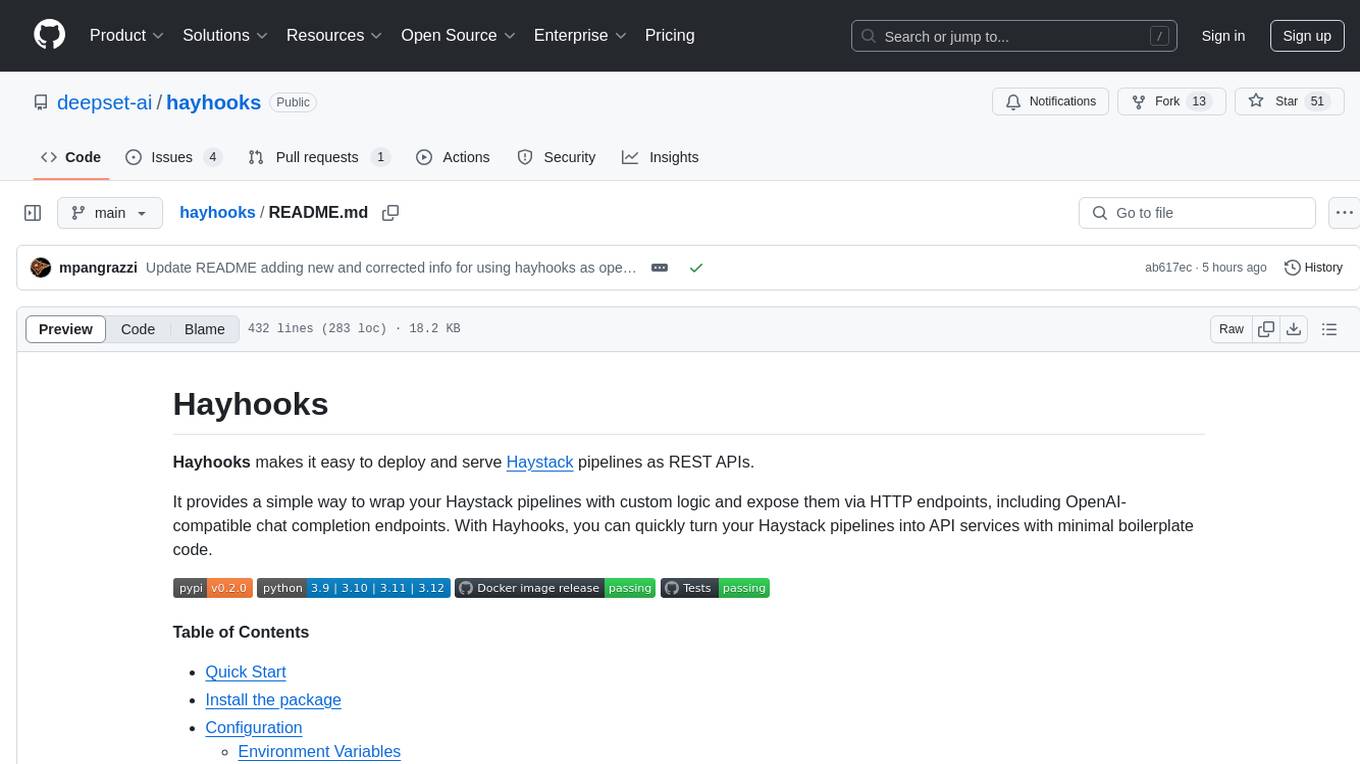
hayhooks
Hayhooks is a tool that simplifies the deployment and serving of Haystack pipelines as REST APIs. It allows users to wrap their pipelines with custom logic and expose them via HTTP endpoints, including OpenAI-compatible chat completion endpoints. With Hayhooks, users can easily convert their Haystack pipelines into API services with minimal boilerplate code.
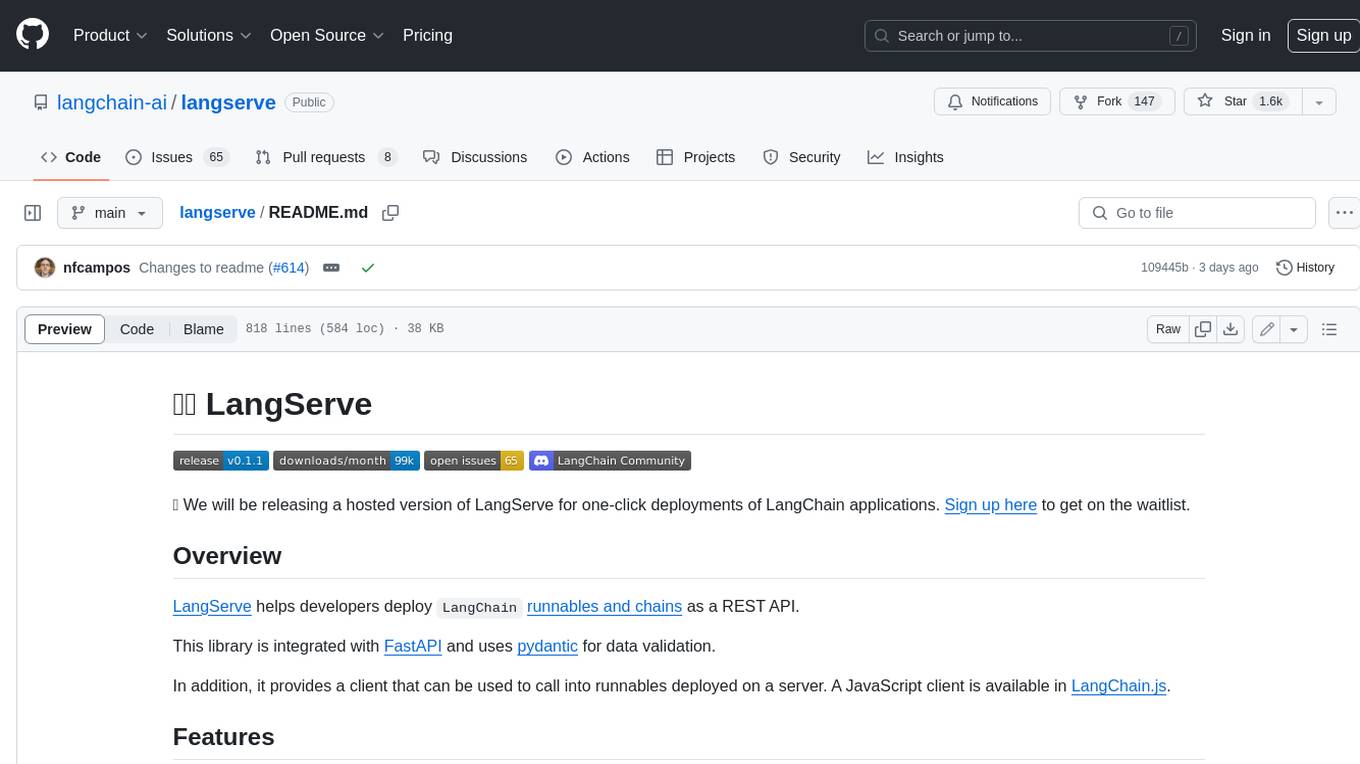
langserve
LangServe helps developers deploy `LangChain` runnables and chains as a REST API. This library is integrated with FastAPI and uses pydantic for data validation. In addition, it provides a client that can be used to call into runnables deployed on a server. A JavaScript client is available in LangChain.js.
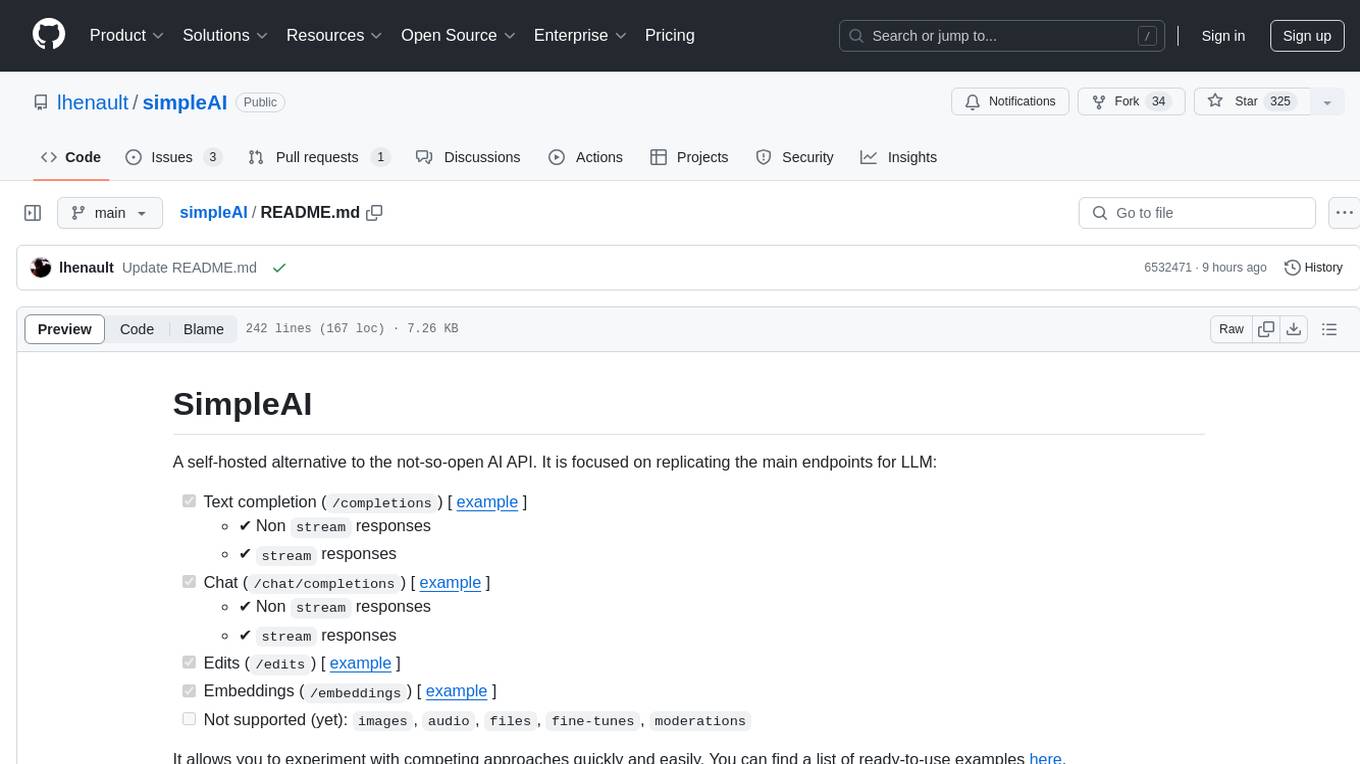
simpleAI
SimpleAI is a self-hosted alternative to the not-so-open AI API, focused on replicating main endpoints for LLM such as text completion, chat, edits, and embeddings. It allows quick experimentation with different models, creating benchmarks, and handling specific use cases without relying on external services. Users can integrate and declare models through gRPC, query endpoints using Swagger UI or API, and resolve common issues like CORS with FastAPI middleware. The project is open for contributions and welcomes PRs, issues, documentation, and more.
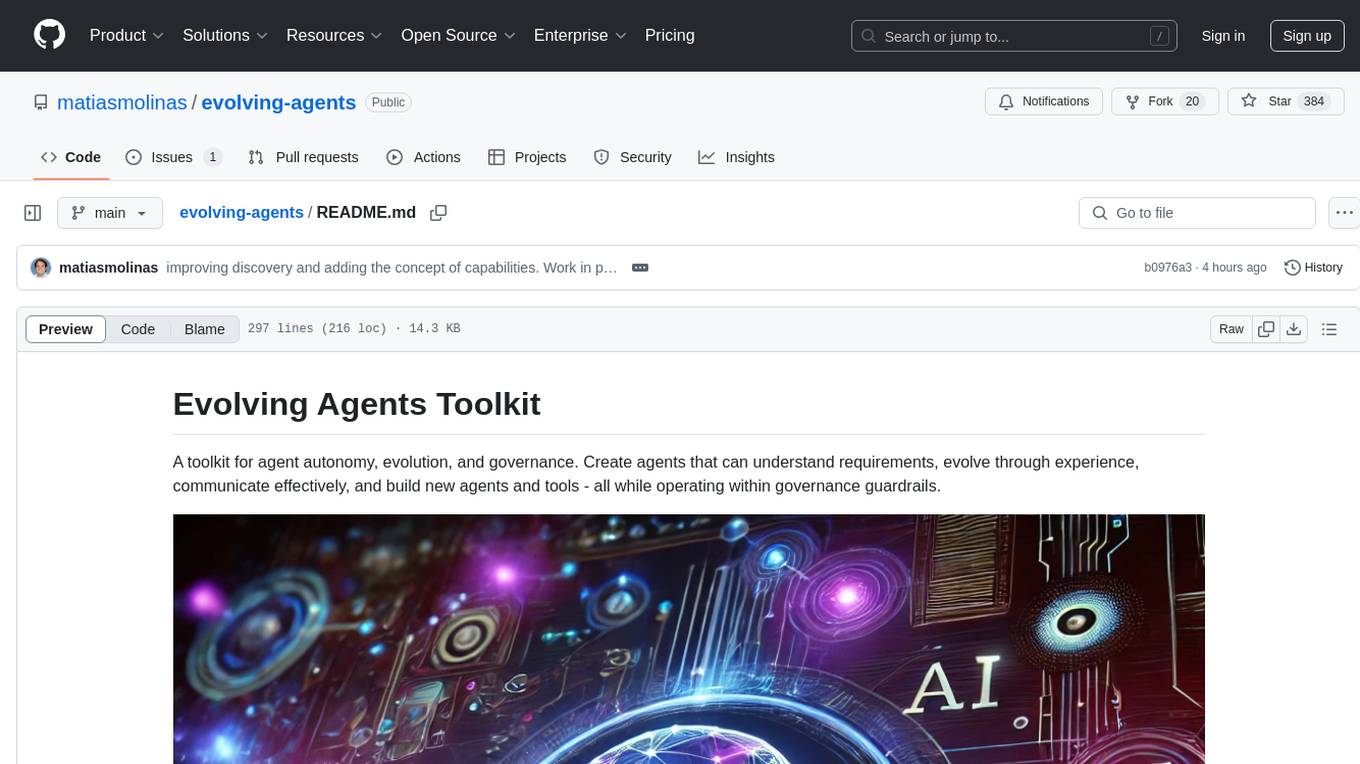
evolving-agents
A toolkit for agent autonomy, evolution, and governance enabling agents to learn from experience, collaborate, communicate, and build new tools within governance guardrails. It focuses on autonomous evolution, agent self-discovery, governance firmware, self-building systems, and agent-centric architecture. The toolkit leverages existing frameworks to enable agent autonomy and self-governance, moving towards truly autonomous AI systems.
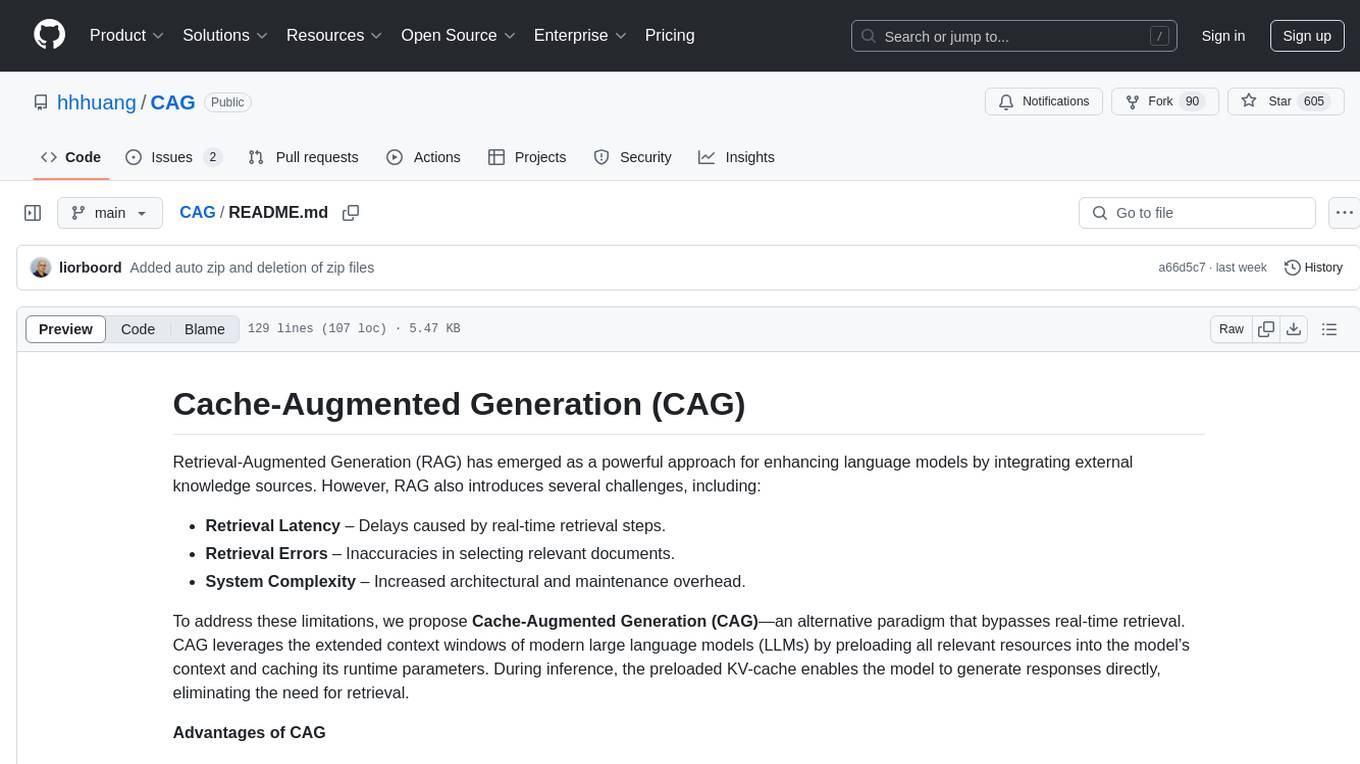
CAG
Cache-Augmented Generation (CAG) is an alternative paradigm to Retrieval-Augmented Generation (RAG) that eliminates real-time retrieval delays and errors by preloading all relevant resources into the model's context. CAG leverages extended context windows of large language models (LLMs) to generate responses directly, providing reduced latency, improved reliability, and simplified design. While CAG has limitations in knowledge size and context length, advancements in LLMs are addressing these issues, making CAG a practical and scalable alternative for complex applications.
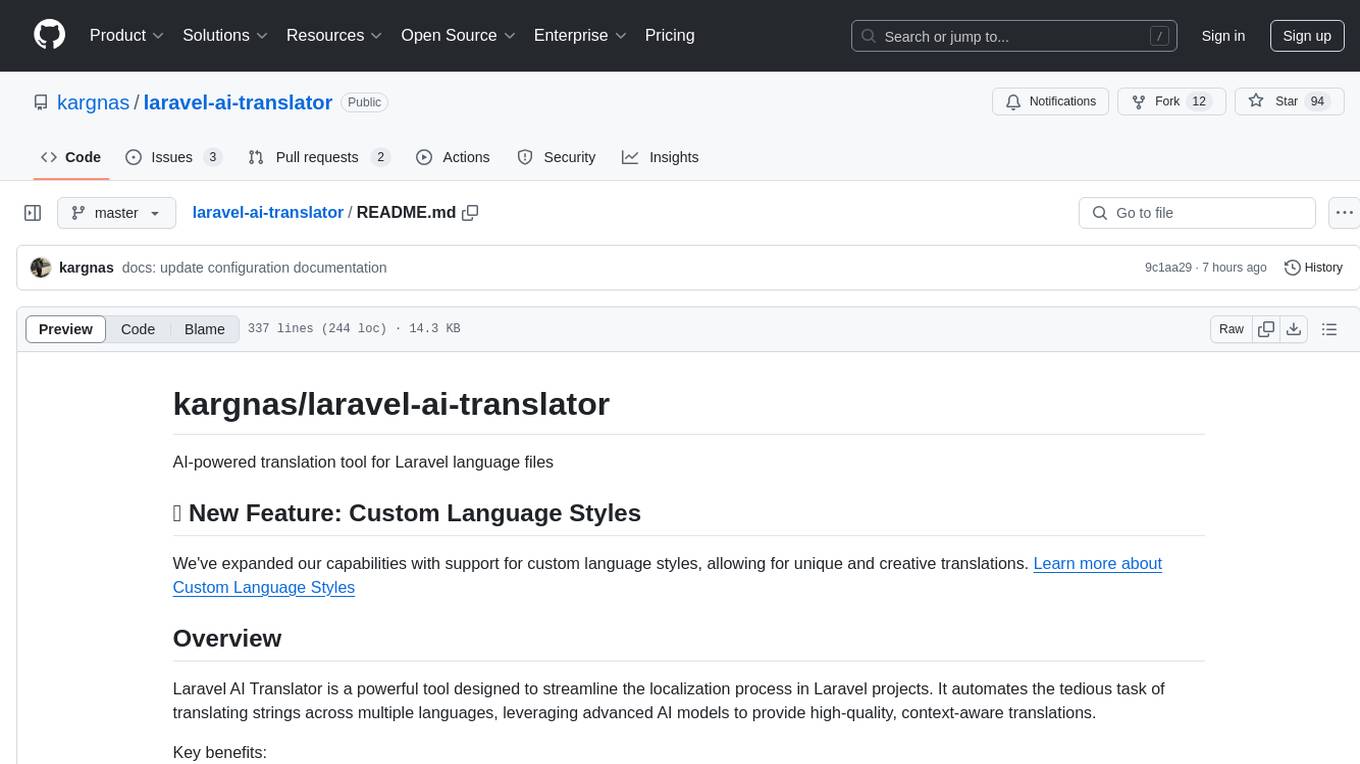
laravel-ai-translator
Laravel AI Translator is a powerful tool designed to streamline the localization process in Laravel projects. It automates the task of translating strings across multiple languages using advanced AI models like GPT-4 and Claude. The tool supports custom language styles, preserves variables and nested structures, and ensures consistent tone and style across translations. It integrates seamlessly with Laravel projects, making internationalization easier and more efficient. Users can customize translation rules, handle large language files efficiently, and validate translations for accuracy. The tool offers contextual understanding, linguistic precision, variable handling, smart length adaptation, and tone consistency for intelligent translations.
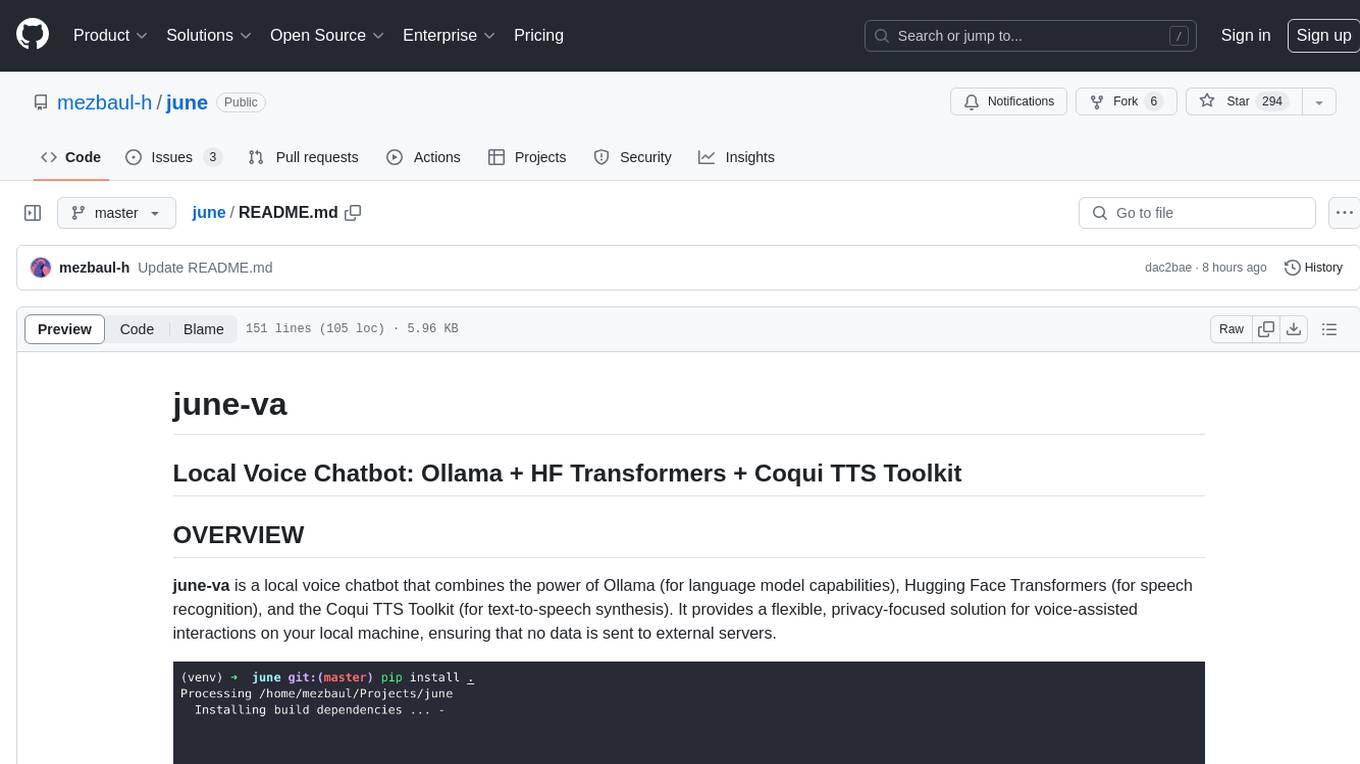
june
june-va is a local voice chatbot that combines Ollama for language model capabilities, Hugging Face Transformers for speech recognition, and the Coqui TTS Toolkit for text-to-speech synthesis. It provides a flexible, privacy-focused solution for voice-assisted interactions on your local machine, ensuring that no data is sent to external servers. The tool supports various interaction modes including text input/output, voice input/text output, text input/audio output, and voice input/audio output. Users can customize the tool's behavior with a JSON configuration file and utilize voice conversion features for voice cloning. The application can be further customized using a configuration file with attributes for language model, speech-to-text model, and text-to-speech model configurations.
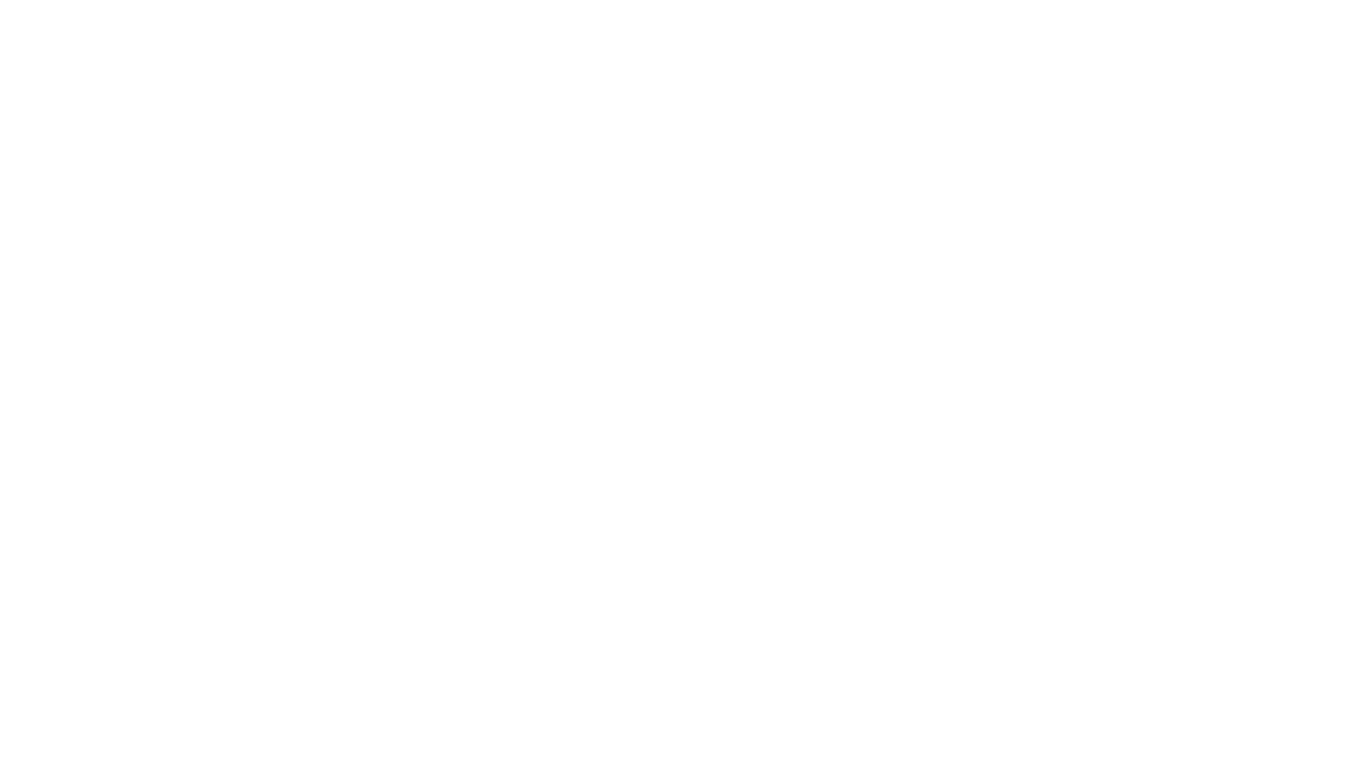
upgini
Upgini is an intelligent data search engine with a Python library that helps users find and add relevant features to their ML pipeline from various public, community, and premium external data sources. It automates the optimization of connected data sources by generating an optimal set of machine learning features using large language models, GraphNNs, and recurrent neural networks. The tool aims to simplify feature search and enrichment for external data to make it a standard approach in machine learning pipelines. It democratizes access to data sources for the data science community.
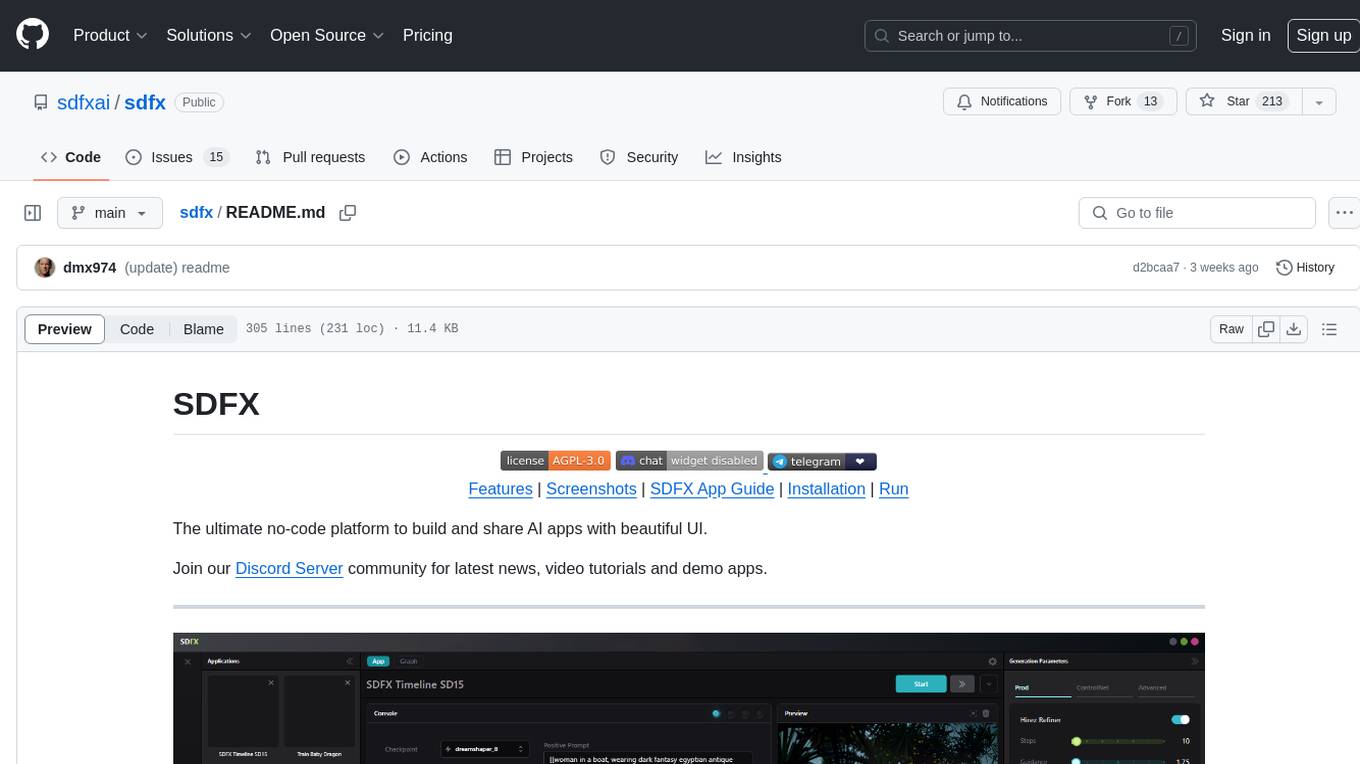
sdfx
SDFX is the ultimate no-code platform for building and sharing AI apps with beautiful UI. It enables the creation of user-friendly interfaces for complex workflows by combining Comfy workflow with a UI. The tool is designed to merge the benefits of form-based UI and graph-node based UI, allowing users to create intricate graphs with a high-level UI overlay. SDFX is fully compatible with ComfyUI, abstracting the need for installing ComfyUI. It offers features like animated graph navigation, node bookmarks, UI debugger, custom nodes manager, app and template export, image and mask editor, and more. The tool compiles as a native app or web app, making it easy to maintain and add new features.
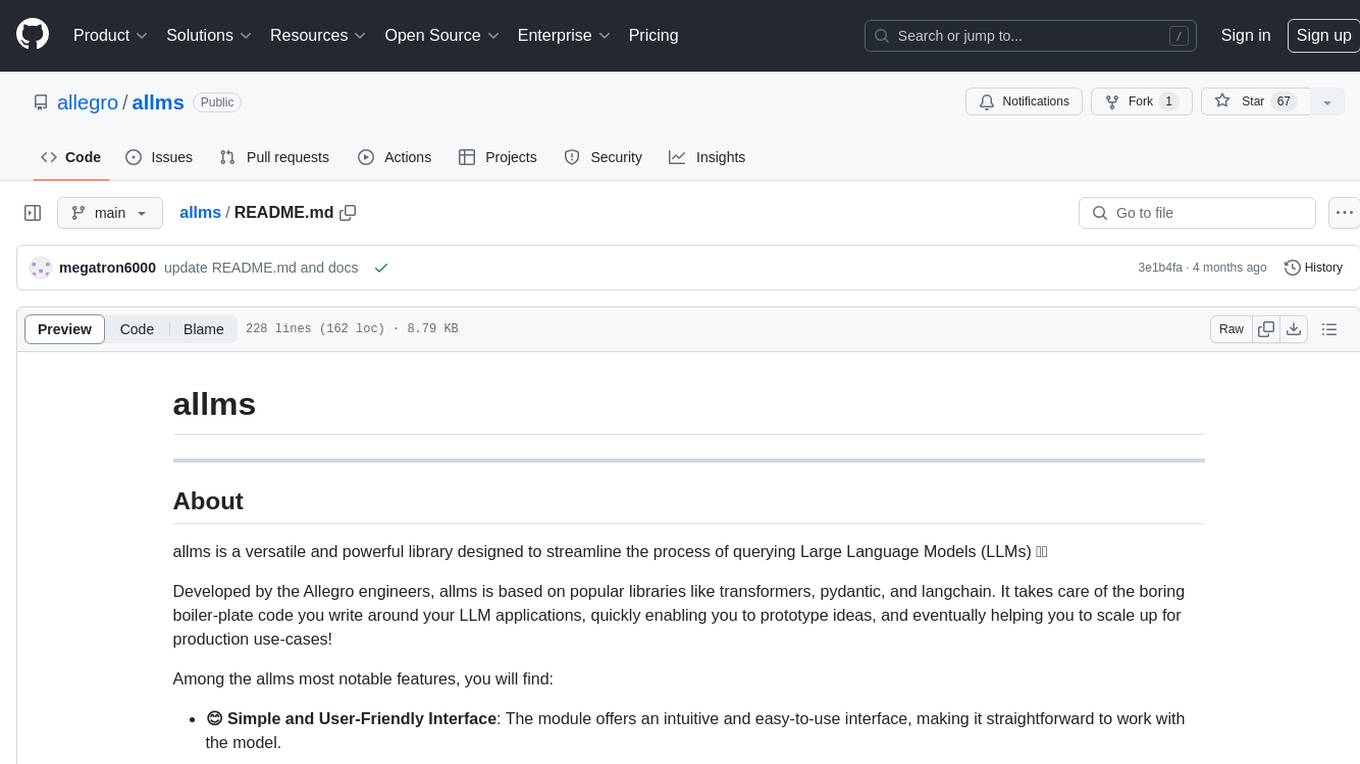
allms
allms is a versatile and powerful library designed to streamline the process of querying Large Language Models (LLMs). Developed by Allegro engineers, it simplifies working with LLM applications by providing a user-friendly interface, asynchronous querying, automatic retrying mechanism, error handling, and output parsing. It supports various LLM families hosted on different platforms like OpenAI, Google, Azure, and GCP. The library offers features for configuring endpoint credentials, batch querying with symbolic variables, and forcing structured output format. It also provides documentation, quickstart guides, and instructions for local development, testing, updating documentation, and making new releases.
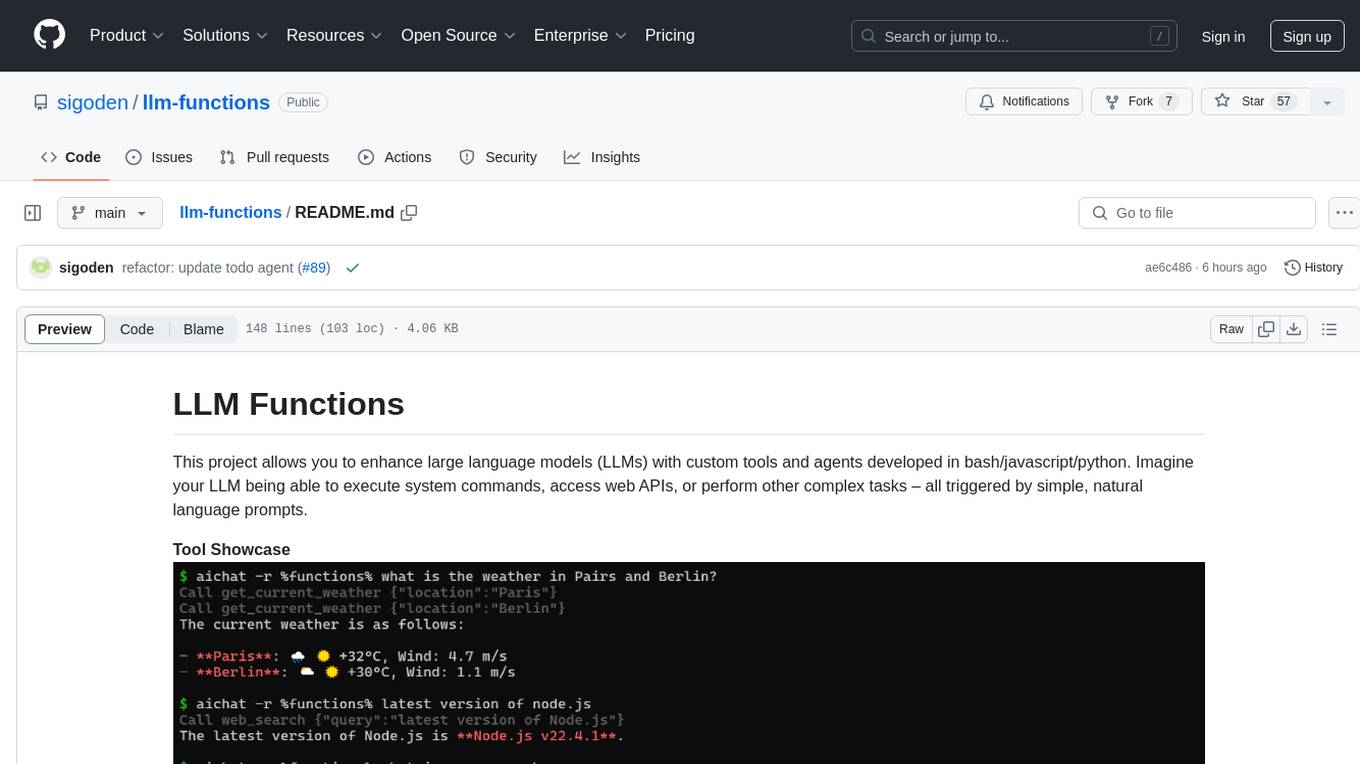
llm-functions
LLM Functions is a project that enables the enhancement of large language models (LLMs) with custom tools and agents developed in bash, javascript, and python. Users can create tools for their LLM to execute system commands, access web APIs, or perform other complex tasks triggered by natural language prompts. The project provides a framework for building tools and agents, with tools being functions written in the user's preferred language and automatically generating JSON declarations based on comments. Agents combine prompts, function callings, and knowledge (RAG) to create conversational AI agents. The project is designed to be user-friendly and allows users to easily extend the capabilities of their language models.
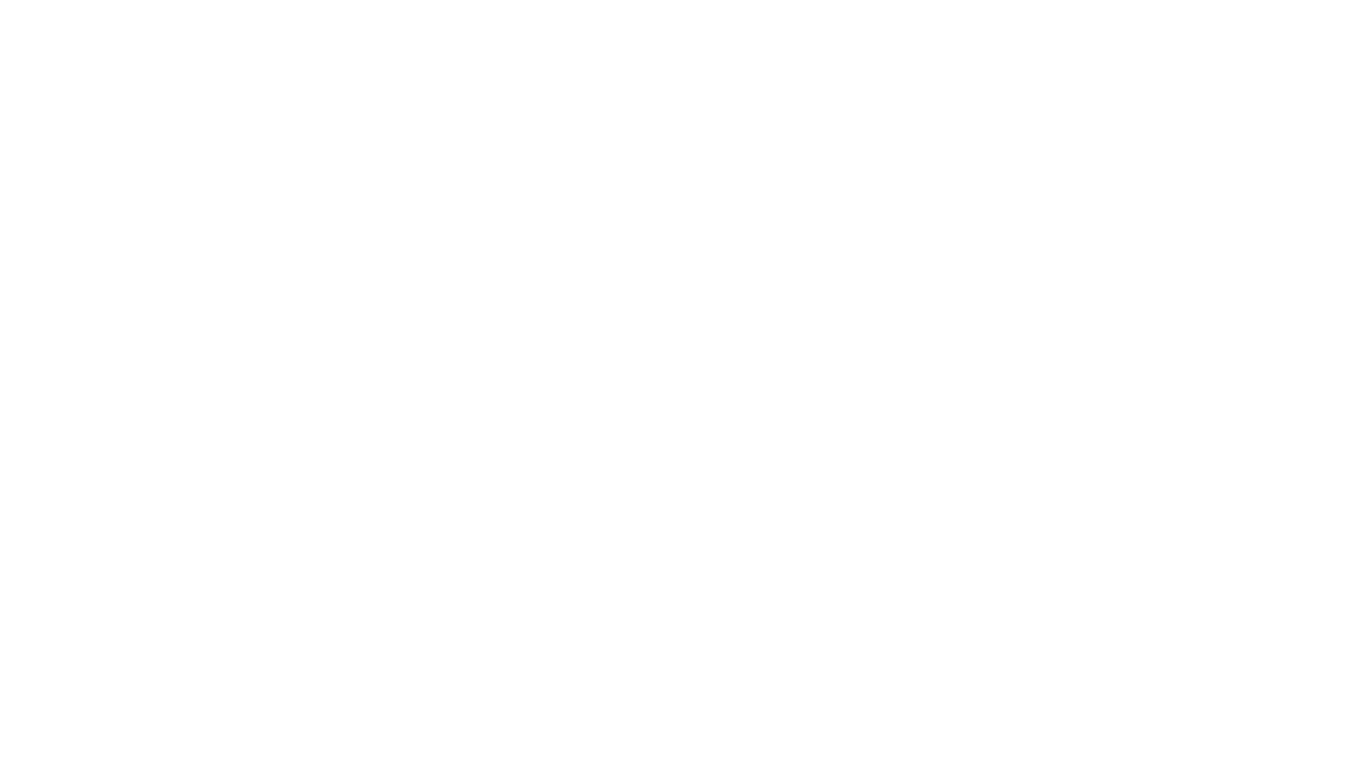
appworld
AppWorld is a high-fidelity execution environment of 9 day-to-day apps, operable via 457 APIs, populated with digital activities of ~100 people living in a simulated world. It provides a benchmark of natural, diverse, and challenging autonomous agent tasks requiring rich and interactive coding. The repository includes implementations of AppWorld apps and APIs, along with tests. It also introduces safety features for code execution and provides guides for building agents and extending the benchmark.
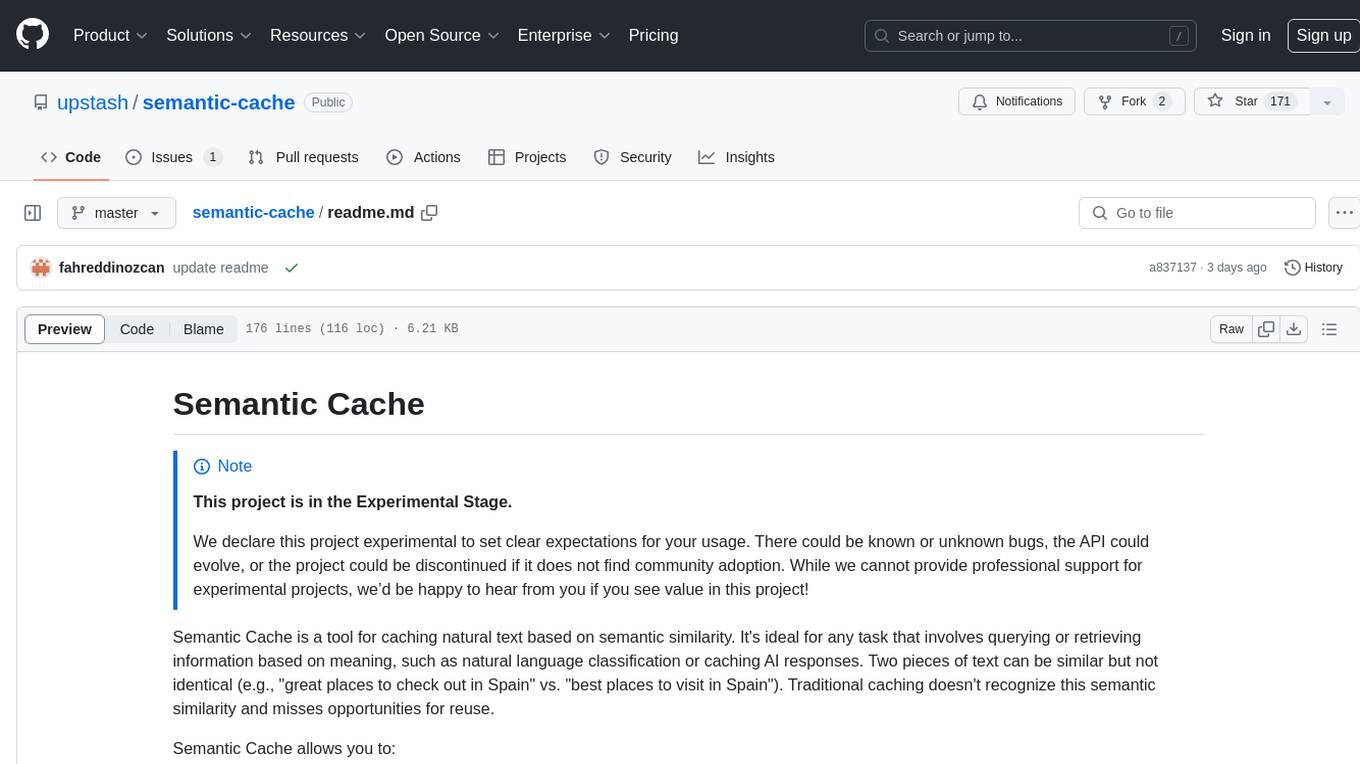
semantic-cache
Semantic Cache is a tool for caching natural text based on semantic similarity. It allows for classifying text into categories, caching AI responses, and reducing API latency by responding to similar queries with cached values. The tool stores cache entries by meaning, handles synonyms, supports multiple languages, understands complex queries, and offers easy integration with Node.js applications. Users can set a custom proximity threshold for filtering results. The tool is ideal for tasks involving querying or retrieving information based on meaning, such as natural language classification or caching AI responses.
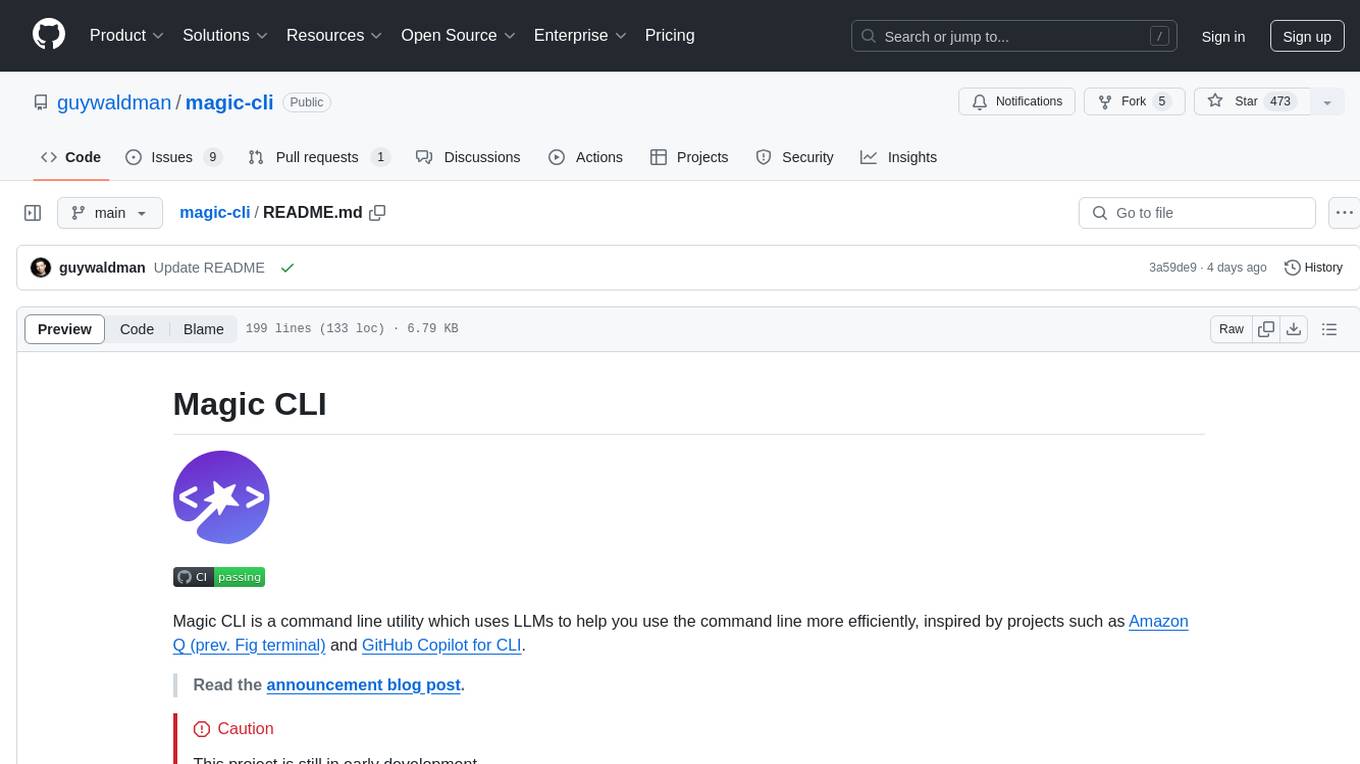
magic-cli
Magic CLI is a command line utility that leverages Large Language Models (LLMs) to enhance command line efficiency. It is inspired by projects like Amazon Q and GitHub Copilot for CLI. The tool allows users to suggest commands, search across command history, and generate commands for specific tasks using local or remote LLM providers. Magic CLI also provides configuration options for LLM selection and response generation. The project is still in early development, so users should expect breaking changes and bugs.
For similar tasks
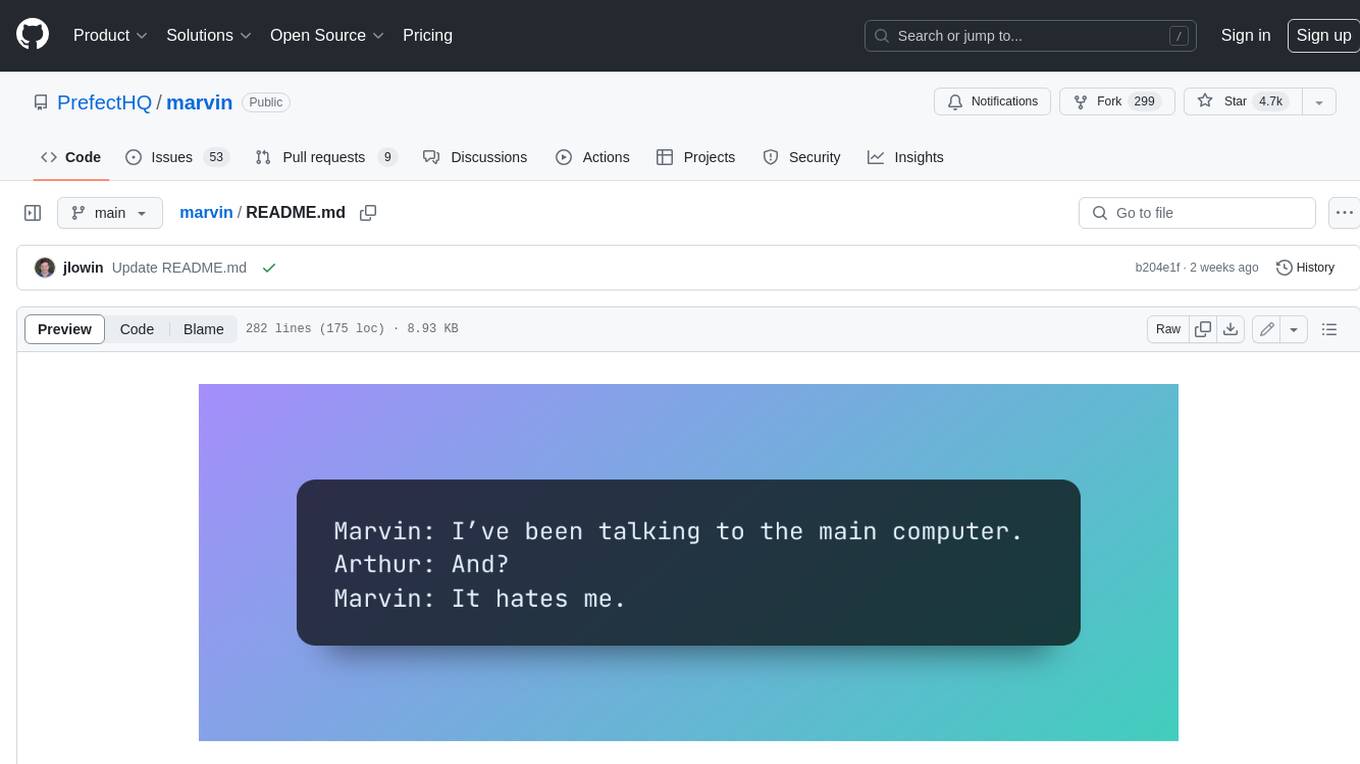
marvin
Marvin is a lightweight AI toolkit for building natural language interfaces that are reliable, scalable, and easy to trust. Each of Marvin's tools is simple and self-documenting, using AI to solve common but complex challenges like entity extraction, classification, and generating synthetic data. Each tool is independent and incrementally adoptable, so you can use them on their own or in combination with any other library. Marvin is also multi-modal, supporting both image and audio generation as well using images as inputs for extraction and classification. Marvin is for developers who care more about _using_ AI than _building_ AI, and we are focused on creating an exceptional developer experience. Marvin users should feel empowered to bring tightly-scoped "AI magic" into any traditional software project with just a few extra lines of code. Marvin aims to merge the best practices for building dependable, observable software with the best practices for building with generative AI into a single, easy-to-use library. It's a serious tool, but we hope you have fun with it. Marvin is open-source, free to use, and made with ๐ by the team at Prefect.
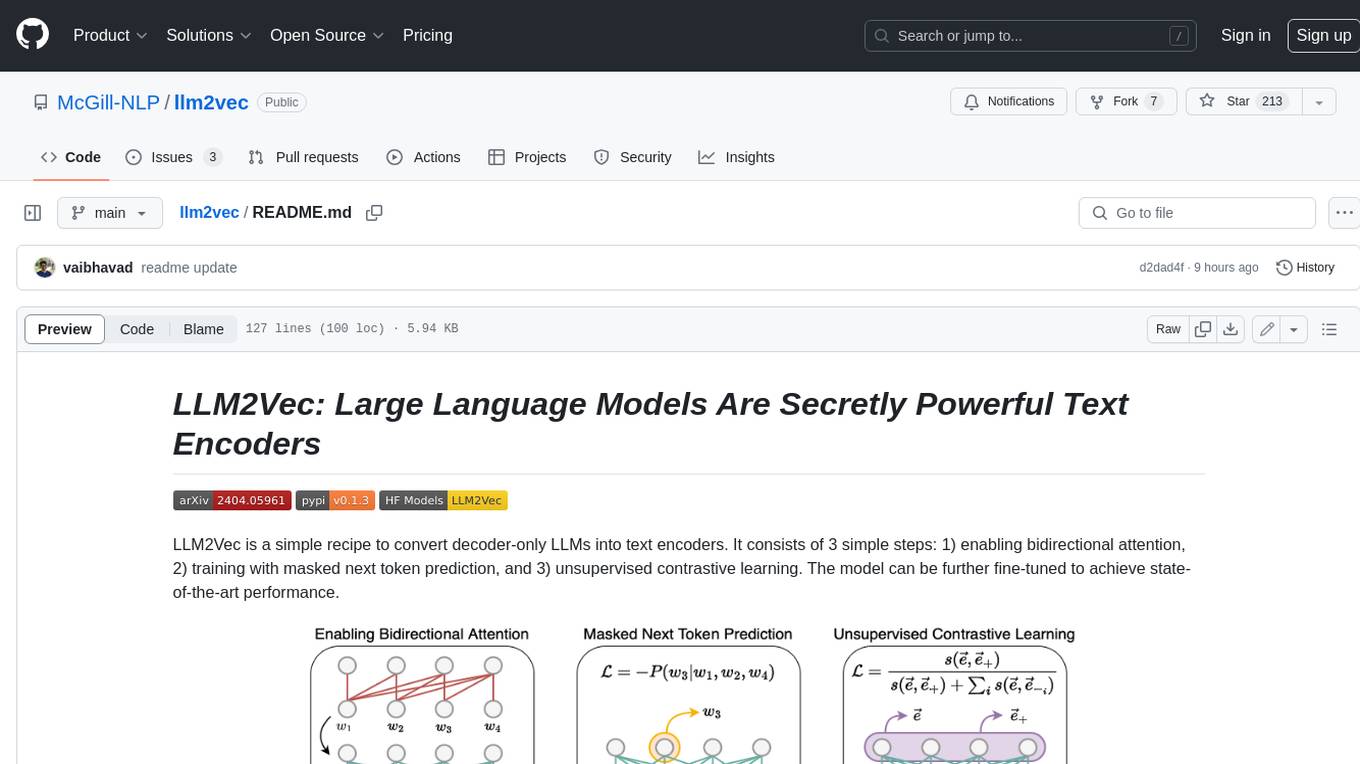
llm2vec
LLM2Vec is a simple recipe to convert decoder-only LLMs into text encoders. It consists of 3 simple steps: 1) enabling bidirectional attention, 2) training with masked next token prediction, and 3) unsupervised contrastive learning. The model can be further fine-tuned to achieve state-of-the-art performance.
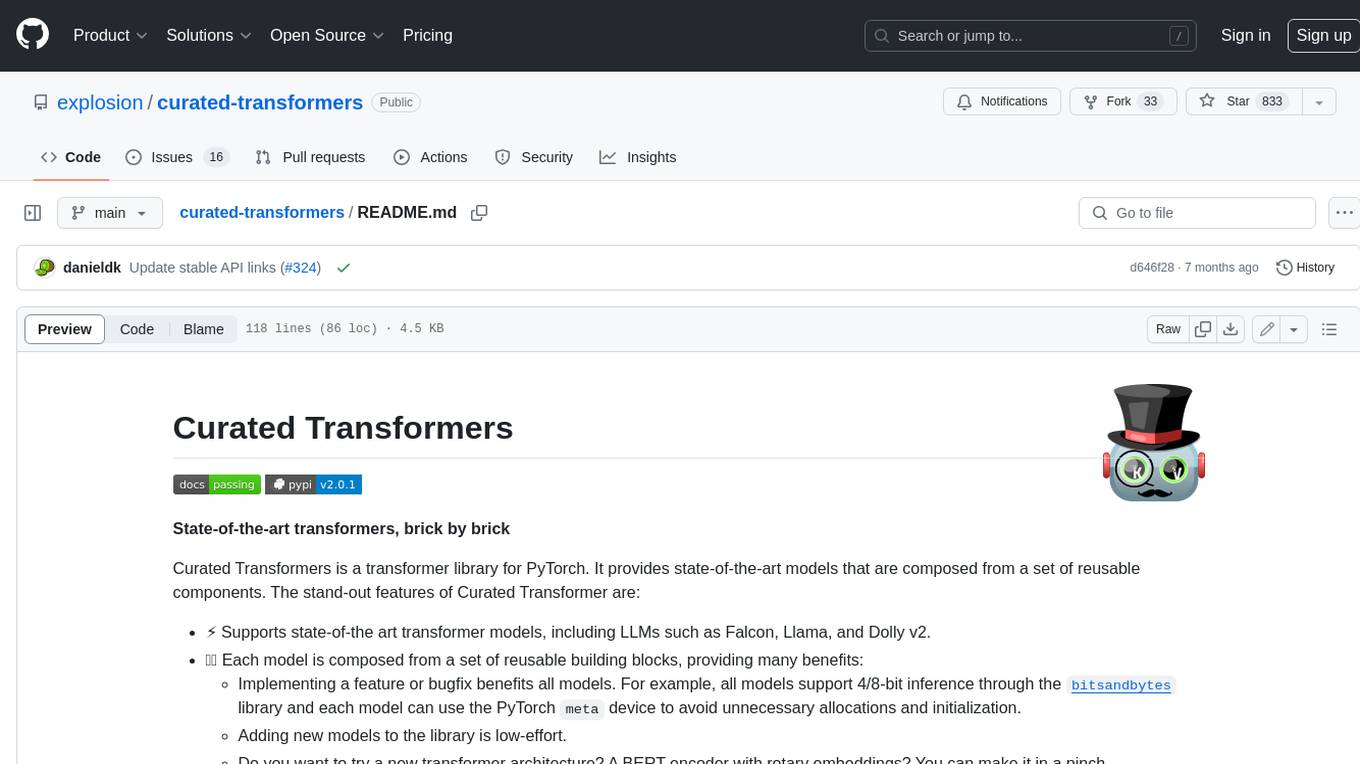
curated-transformers
Curated Transformers is a transformer library for PyTorch that provides state-of-the-art models composed of reusable components. It supports various transformer architectures, including encoders like ALBERT, BERT, and RoBERTa, and decoders like Falcon, Llama, and MPT. The library emphasizes consistent type annotations, minimal dependencies, and ease of use for education and research. It has been production-tested by Explosion and will be the default transformer implementation in spaCy 3.7.
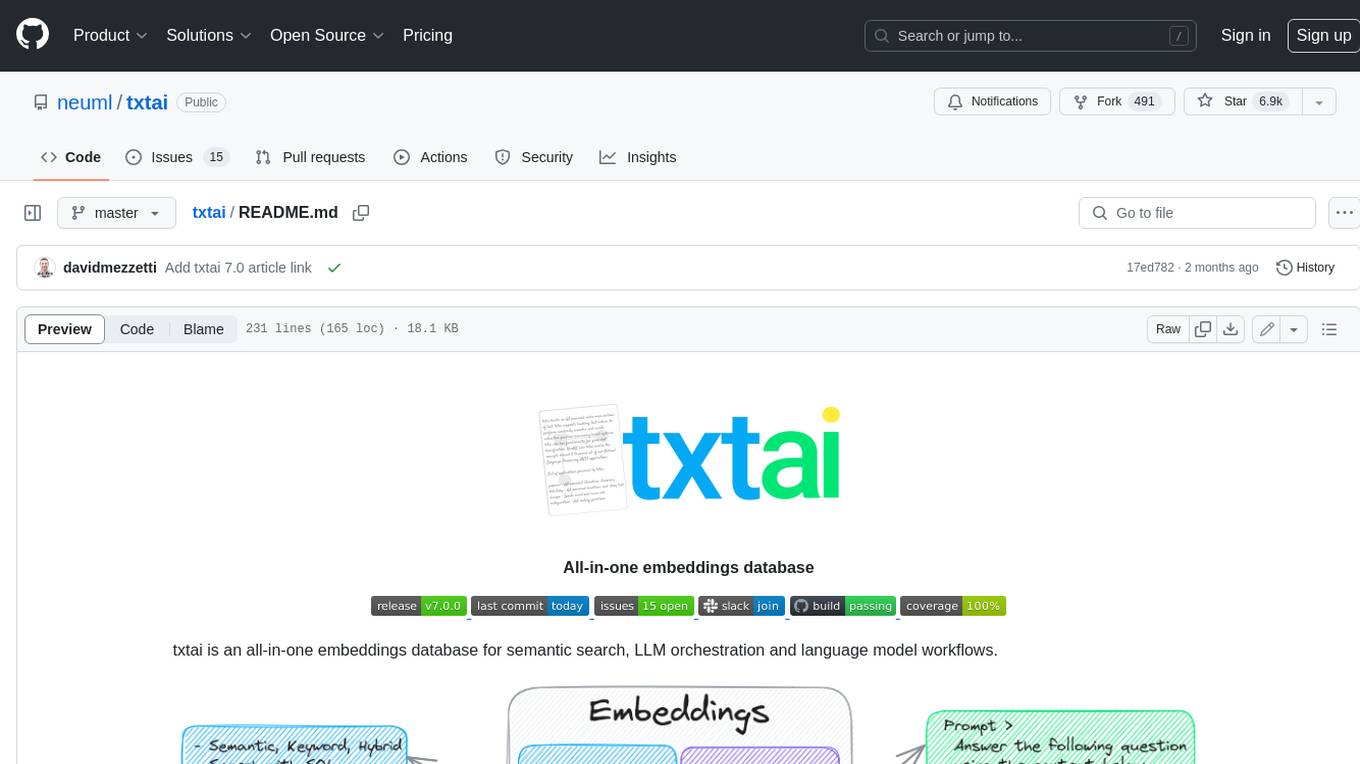
txtai
Txtai is an all-in-one embeddings database for semantic search, LLM orchestration, and language model workflows. It combines vector indexes, graph networks, and relational databases to enable vector search with SQL, topic modeling, retrieval augmented generation, and more. Txtai can stand alone or serve as a knowledge source for large language models (LLMs). Key features include vector search with SQL, object storage, topic modeling, graph analysis, multimodal indexing, embedding creation for various data types, pipelines powered by language models, workflows to connect pipelines, and support for Python, JavaScript, Java, Rust, and Go. Txtai is open-source under the Apache 2.0 license.
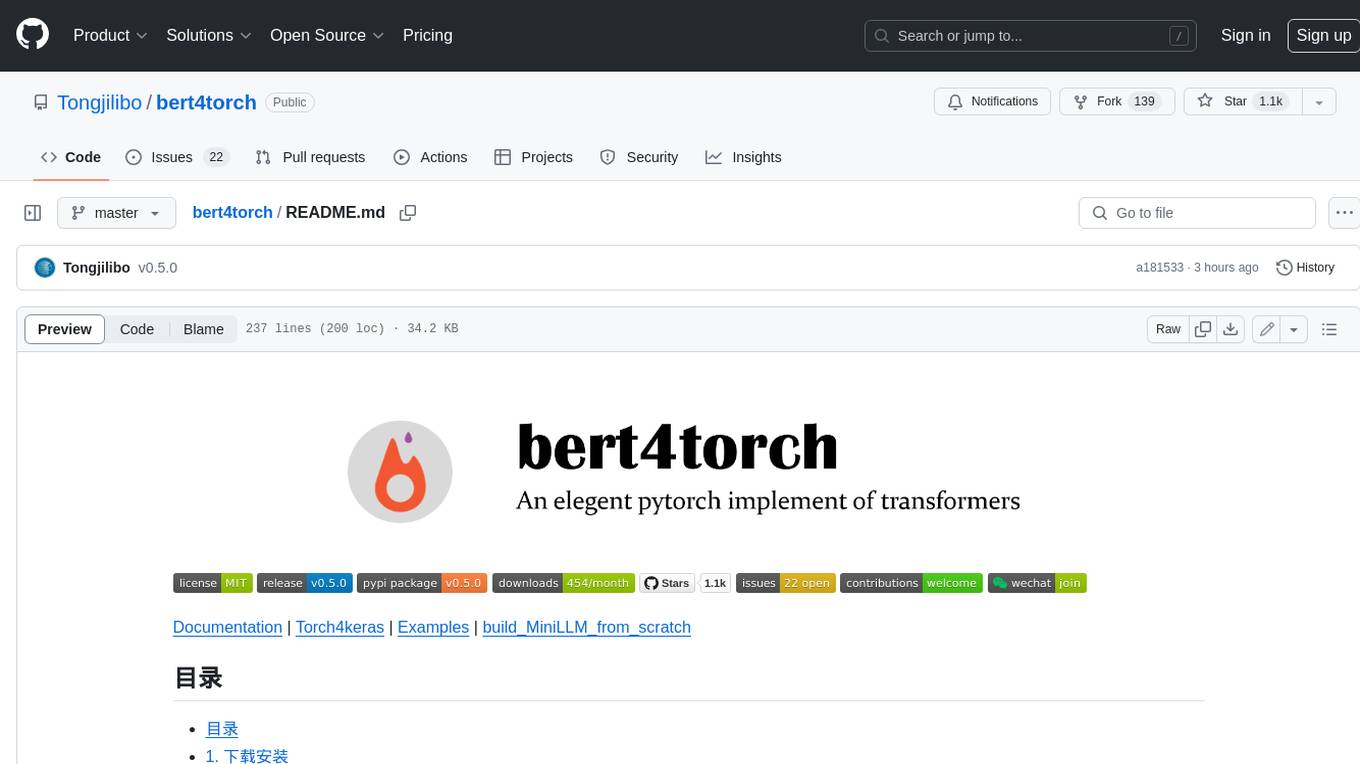
bert4torch
**bert4torch** is a high-level framework for training and deploying transformer models in PyTorch. It provides a simple and efficient API for building, training, and evaluating transformer models, and supports a wide range of pre-trained models, including BERT, RoBERTa, ALBERT, XLNet, and GPT-2. bert4torch also includes a number of useful features, such as data loading, tokenization, and model evaluation. It is a powerful and versatile tool for natural language processing tasks.
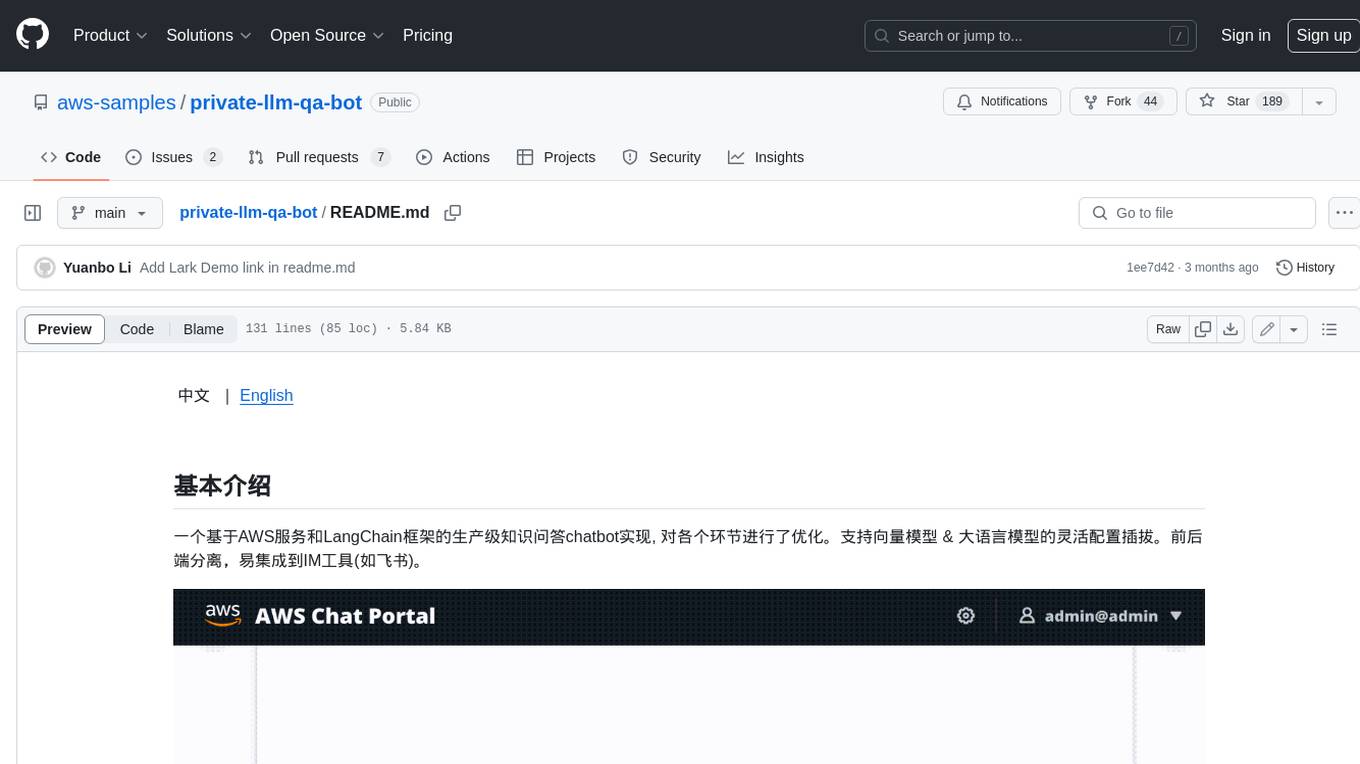
private-llm-qa-bot
This is a production-grade knowledge Q&A chatbot implementation based on AWS services and the LangChain framework, with optimizations at various stages. It supports flexible configuration and plugging of vector models and large language models. The front and back ends are separated, making it easy to integrate with IM tools (such as Feishu).
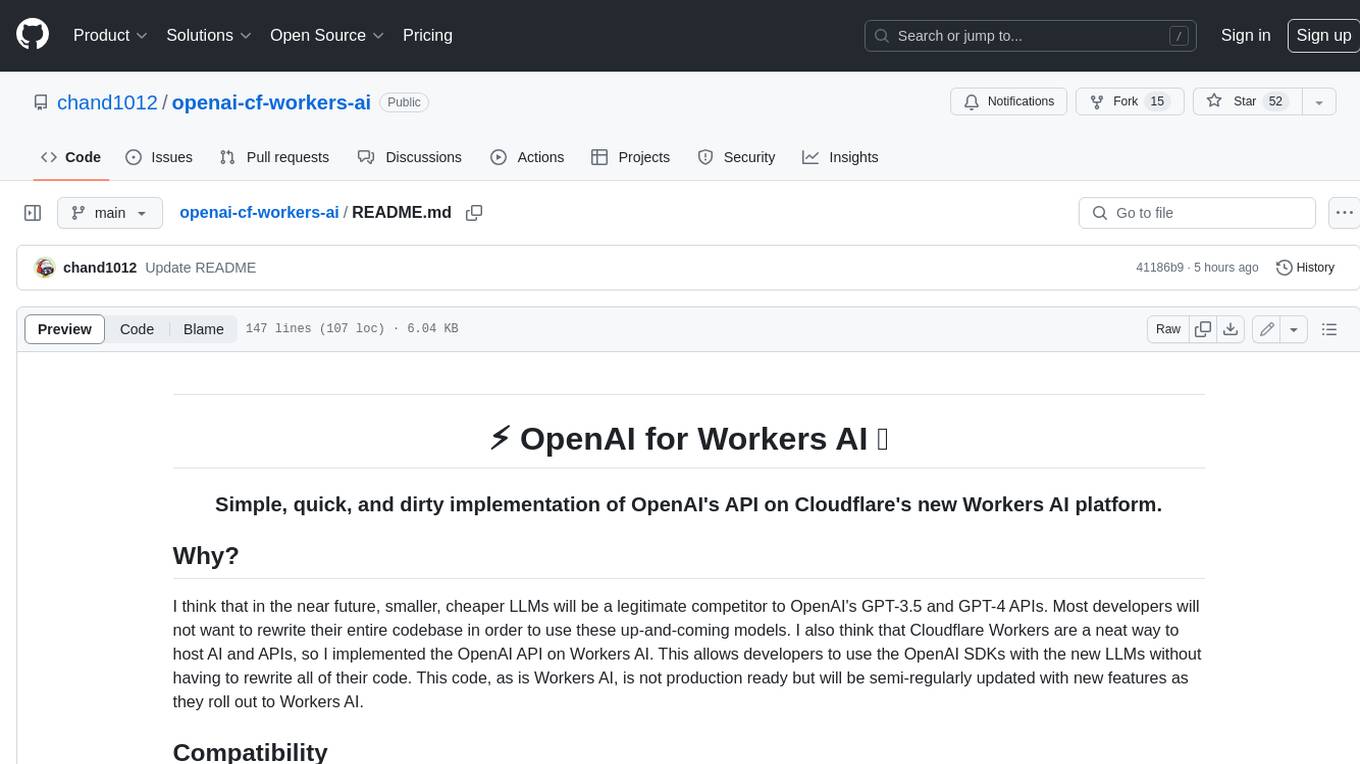
openai-cf-workers-ai
OpenAI for Workers AI is a simple, quick, and dirty implementation of OpenAI's API on Cloudflare's new Workers AI platform. It allows developers to use the OpenAI SDKs with the new LLMs without having to rewrite all of their code. The API currently supports completions, chat completions, audio transcription, embeddings, audio translation, and image generation. It is not production ready but will be semi-regularly updated with new features as they roll out to Workers AI.
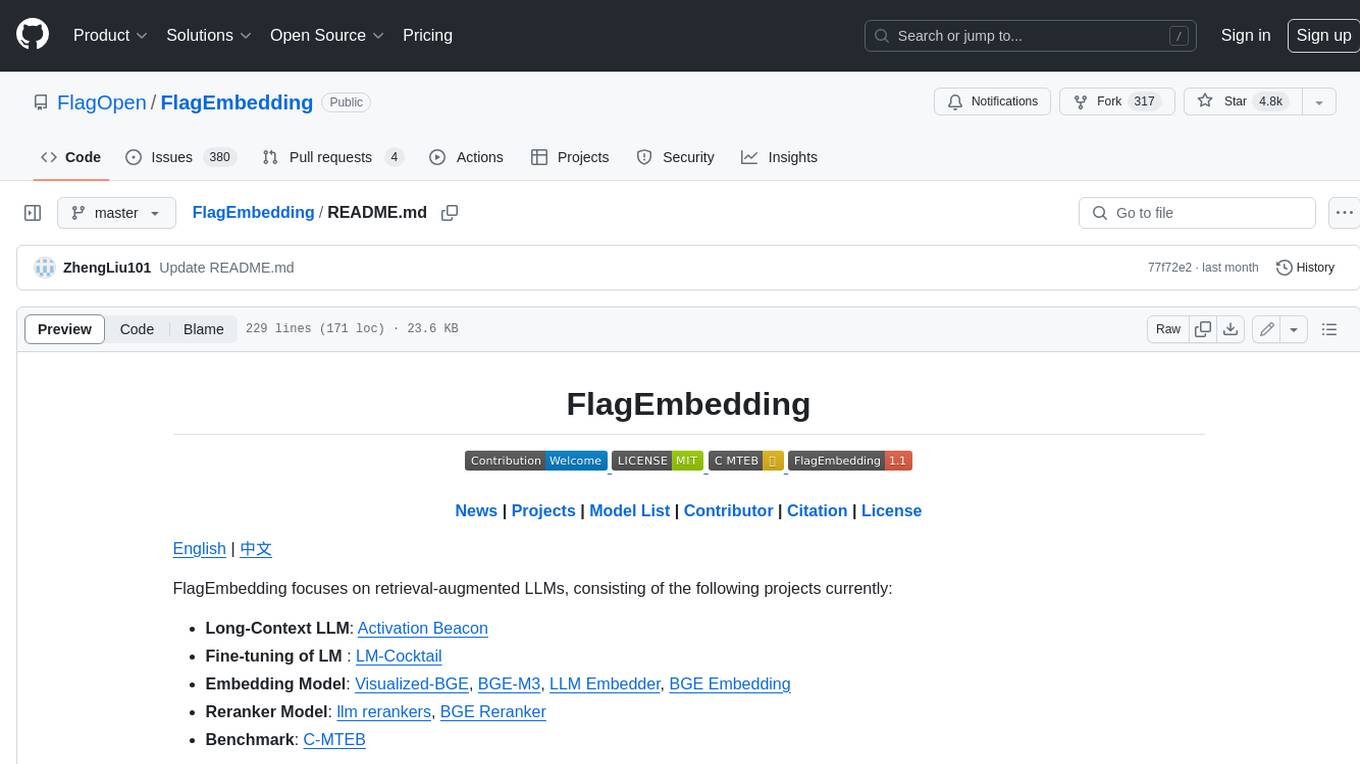
FlagEmbedding
FlagEmbedding focuses on retrieval-augmented LLMs, consisting of the following projects currently: * **Long-Context LLM** : Activation Beacon * **Fine-tuning of LM** : LM-Cocktail * **Embedding Model** : Visualized-BGE, BGE-M3, LLM Embedder, BGE Embedding * **Reranker Model** : llm rerankers, BGE Reranker * **Benchmark** : C-MTEB
For similar jobs
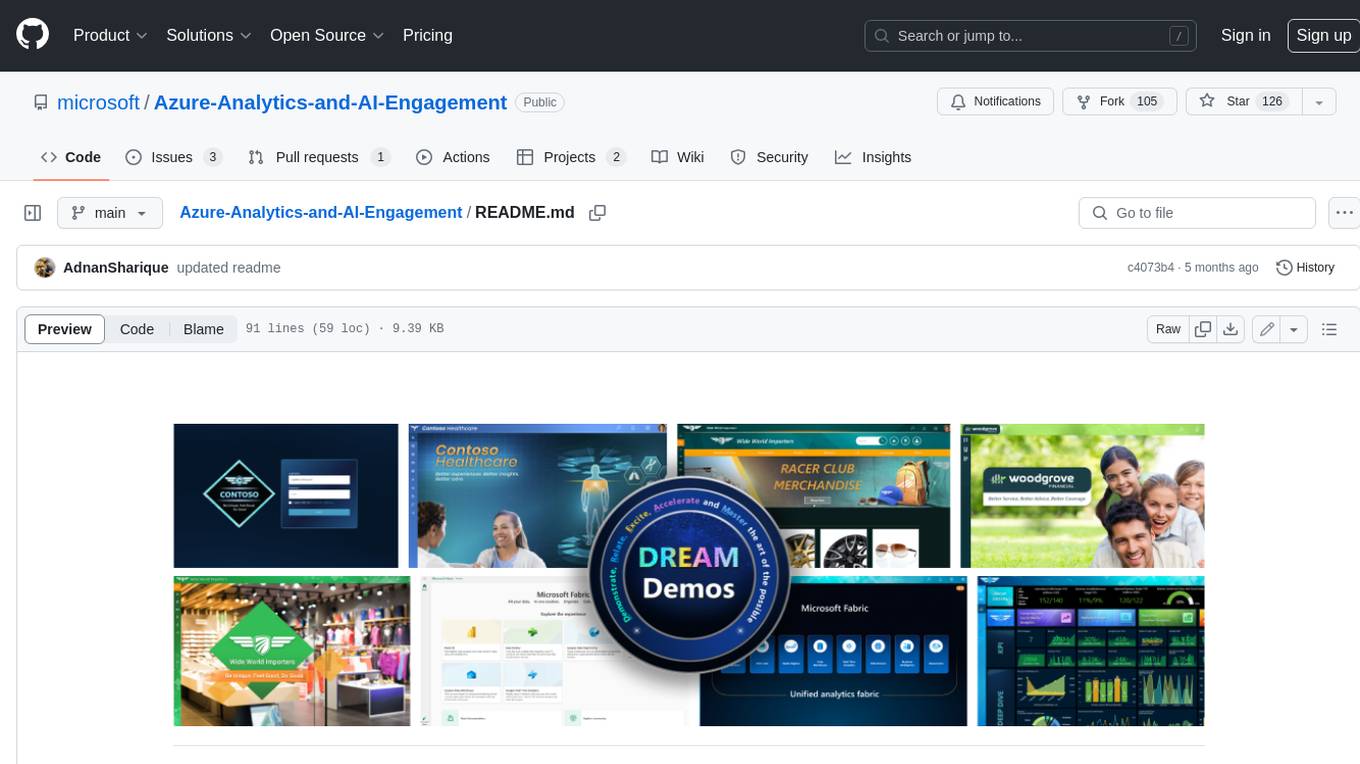
Azure-Analytics-and-AI-Engagement
The Azure-Analytics-and-AI-Engagement repository provides packaged Industry Scenario DREAM Demos with ARM templates (Containing a demo web application, Power BI reports, Synapse resources, AML Notebooks etc.) that can be deployed in a customerโs subscription using the CAPE tool within a matter of few hours. Partners can also deploy DREAM Demos in their own subscriptions using DPoC.
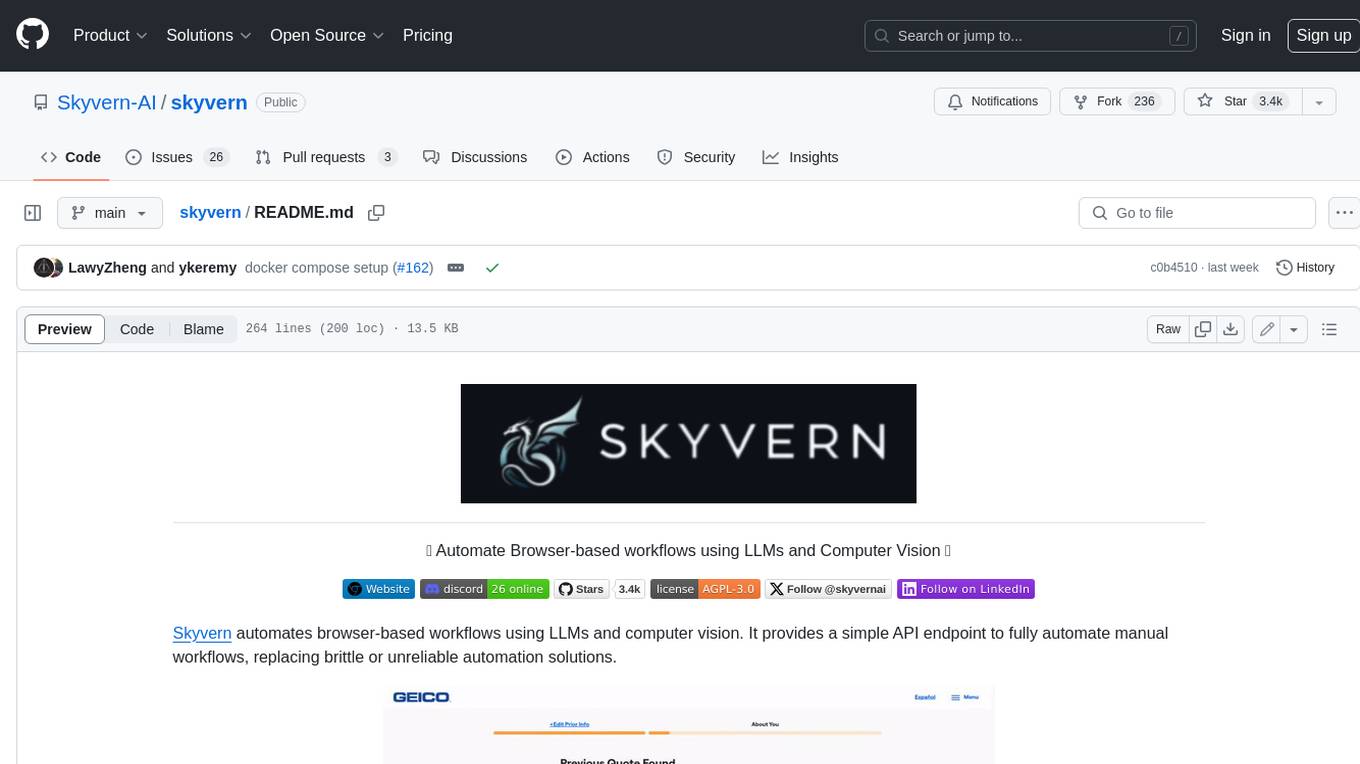
skyvern
Skyvern automates browser-based workflows using LLMs and computer vision. It provides a simple API endpoint to fully automate manual workflows, replacing brittle or unreliable automation solutions. Traditional approaches to browser automations required writing custom scripts for websites, often relying on DOM parsing and XPath-based interactions which would break whenever the website layouts changed. Instead of only relying on code-defined XPath interactions, Skyvern adds computer vision and LLMs to the mix to parse items in the viewport in real-time, create a plan for interaction and interact with them. This approach gives us a few advantages: 1. Skyvern can operate on websites itโs never seen before, as itโs able to map visual elements to actions necessary to complete a workflow, without any customized code 2. Skyvern is resistant to website layout changes, as there are no pre-determined XPaths or other selectors our system is looking for while trying to navigate 3. Skyvern leverages LLMs to reason through interactions to ensure we can cover complex situations. Examples include: 1. If you wanted to get an auto insurance quote from Geico, the answer to a common question โWere you eligible to drive at 18?โ could be inferred from the driver receiving their license at age 16 2. If you were doing competitor analysis, itโs understanding that an Arnold Palmer 22 oz can at 7/11 is almost definitely the same product as a 23 oz can at Gopuff (even though the sizes are slightly different, which could be a rounding error!) Want to see examples of Skyvern in action? Jump to #real-world-examples-of- skyvern
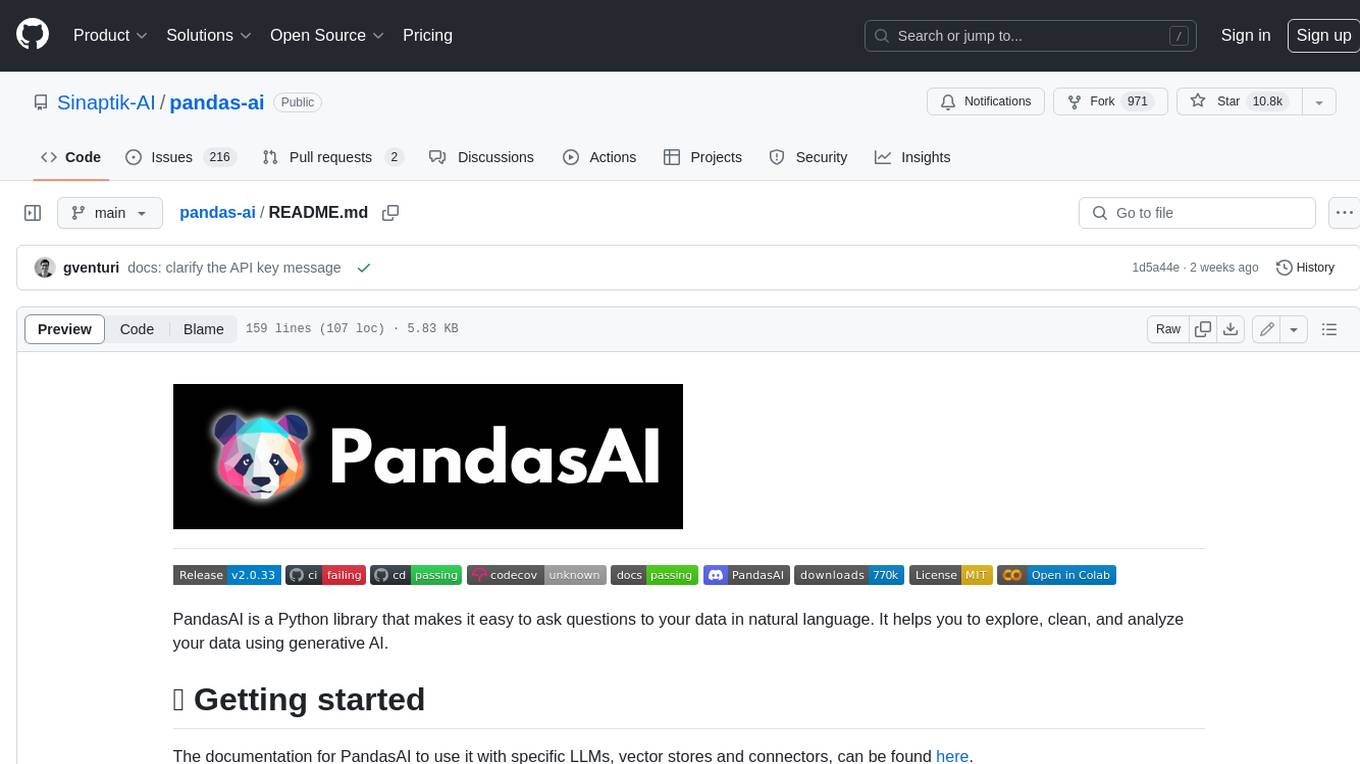
pandas-ai
PandasAI is a Python library that makes it easy to ask questions to your data in natural language. It helps you to explore, clean, and analyze your data using generative AI.
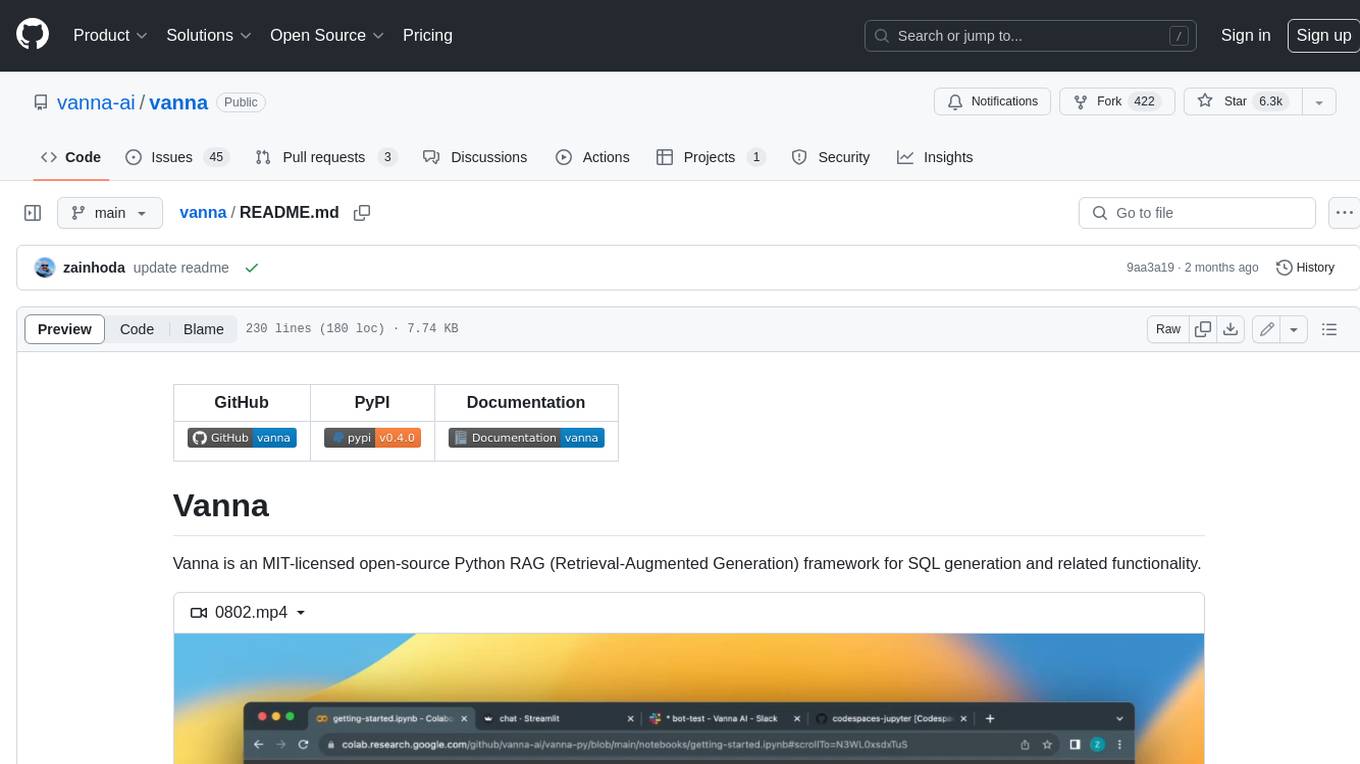
vanna
Vanna is an open-source Python framework for SQL generation and related functionality. It uses Retrieval-Augmented Generation (RAG) to train a model on your data, which can then be used to ask questions and get back SQL queries. Vanna is designed to be portable across different LLMs and vector databases, and it supports any SQL database. It is also secure and private, as your database contents are never sent to the LLM or the vector database.
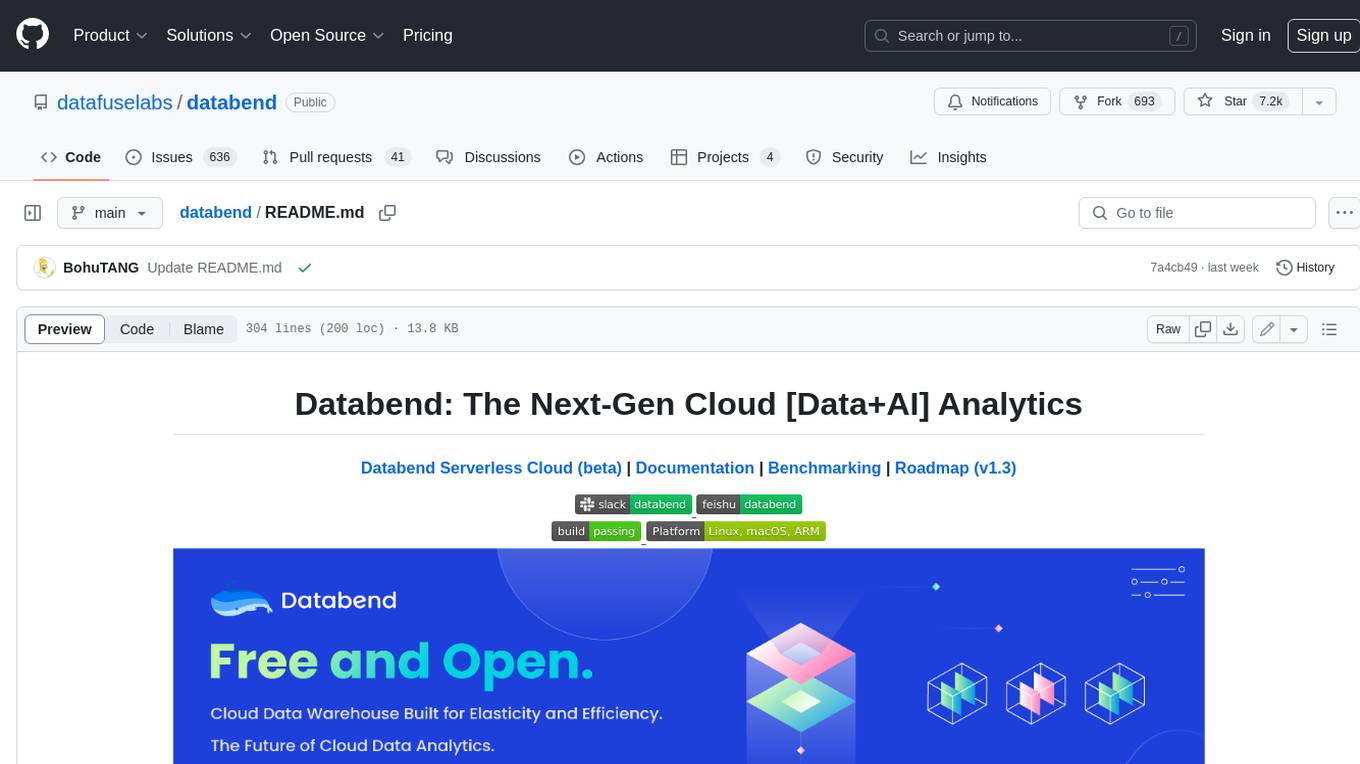
databend
Databend is an open-source cloud data warehouse that serves as a cost-effective alternative to Snowflake. With its focus on fast query execution and data ingestion, it's designed for complex analysis of the world's largest datasets.
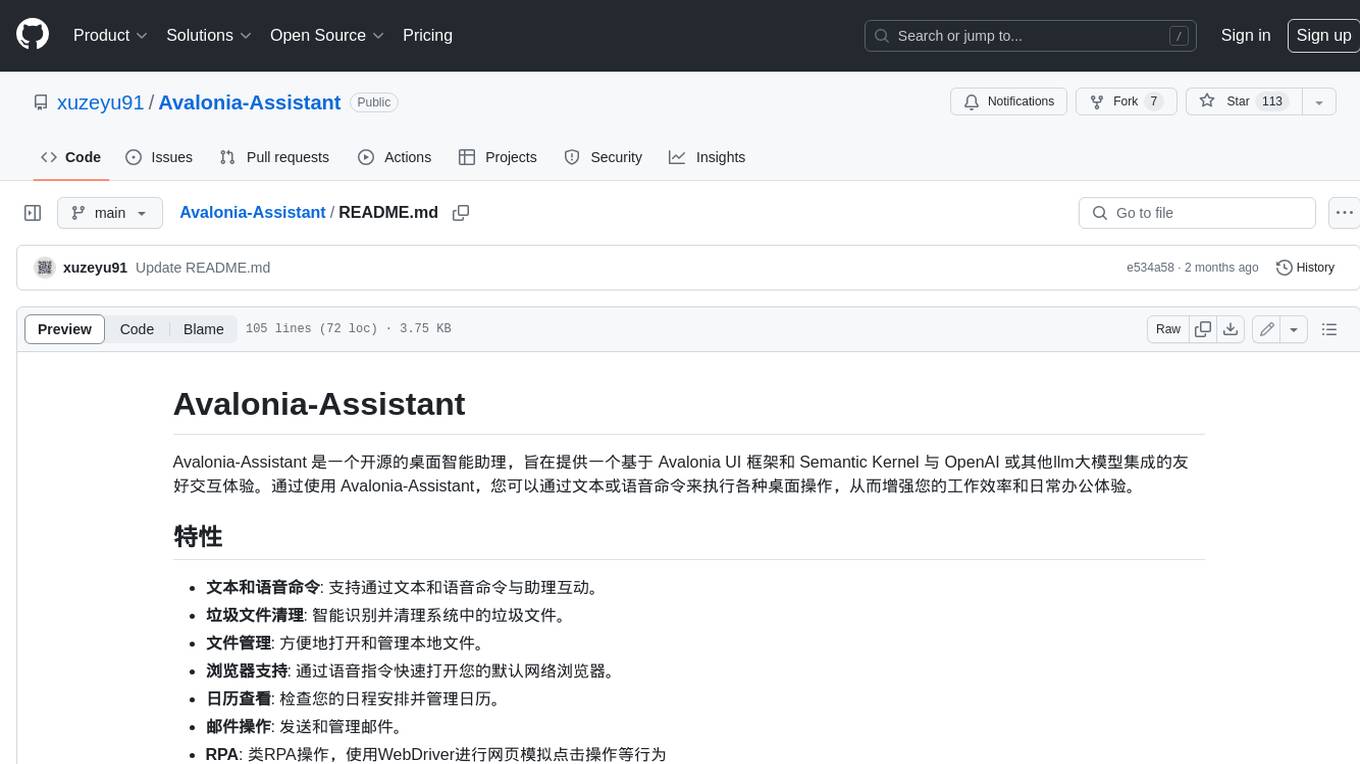
Avalonia-Assistant
Avalonia-Assistant is an open-source desktop intelligent assistant that aims to provide a user-friendly interactive experience based on the Avalonia UI framework and the integration of Semantic Kernel with OpenAI or other large LLM models. By utilizing Avalonia-Assistant, you can perform various desktop operations through text or voice commands, enhancing your productivity and daily office experience.
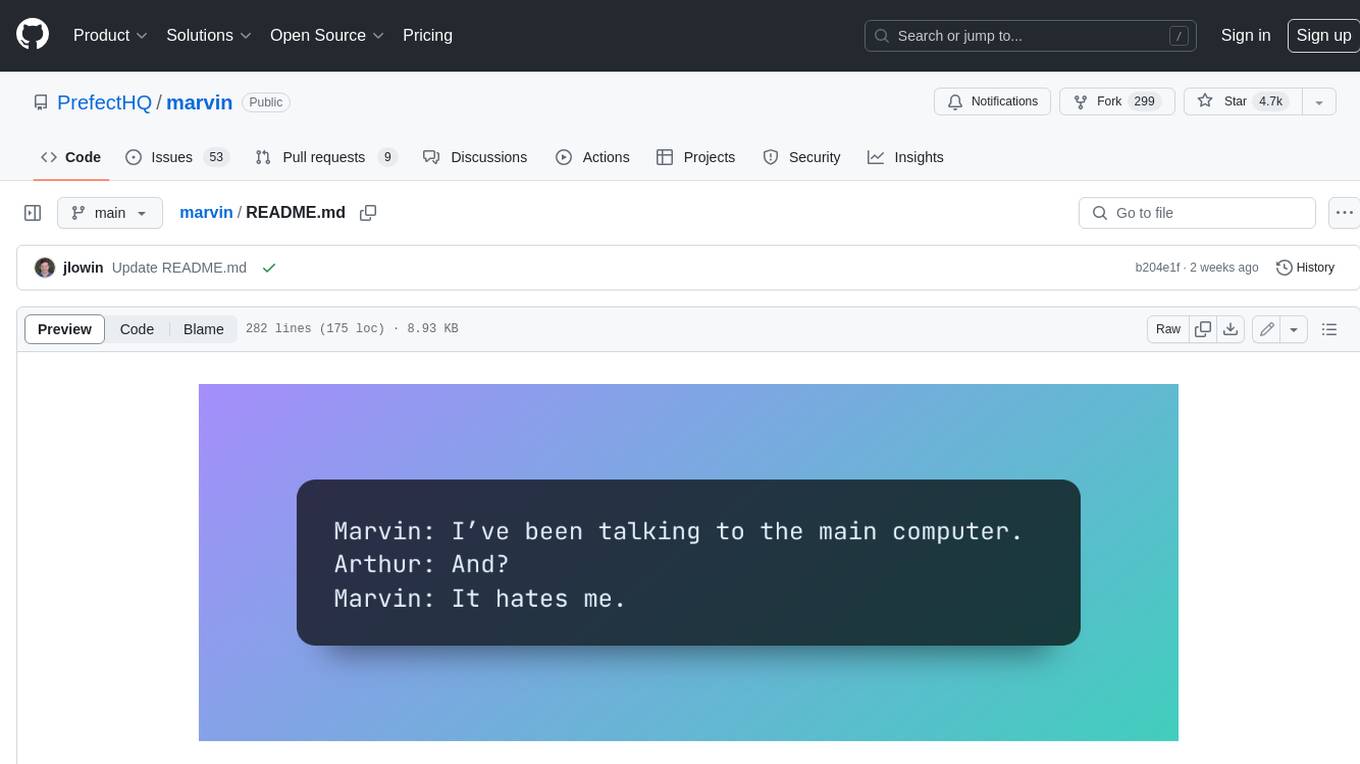
marvin
Marvin is a lightweight AI toolkit for building natural language interfaces that are reliable, scalable, and easy to trust. Each of Marvin's tools is simple and self-documenting, using AI to solve common but complex challenges like entity extraction, classification, and generating synthetic data. Each tool is independent and incrementally adoptable, so you can use them on their own or in combination with any other library. Marvin is also multi-modal, supporting both image and audio generation as well using images as inputs for extraction and classification. Marvin is for developers who care more about _using_ AI than _building_ AI, and we are focused on creating an exceptional developer experience. Marvin users should feel empowered to bring tightly-scoped "AI magic" into any traditional software project with just a few extra lines of code. Marvin aims to merge the best practices for building dependable, observable software with the best practices for building with generative AI into a single, easy-to-use library. It's a serious tool, but we hope you have fun with it. Marvin is open-source, free to use, and made with ๐ by the team at Prefect.
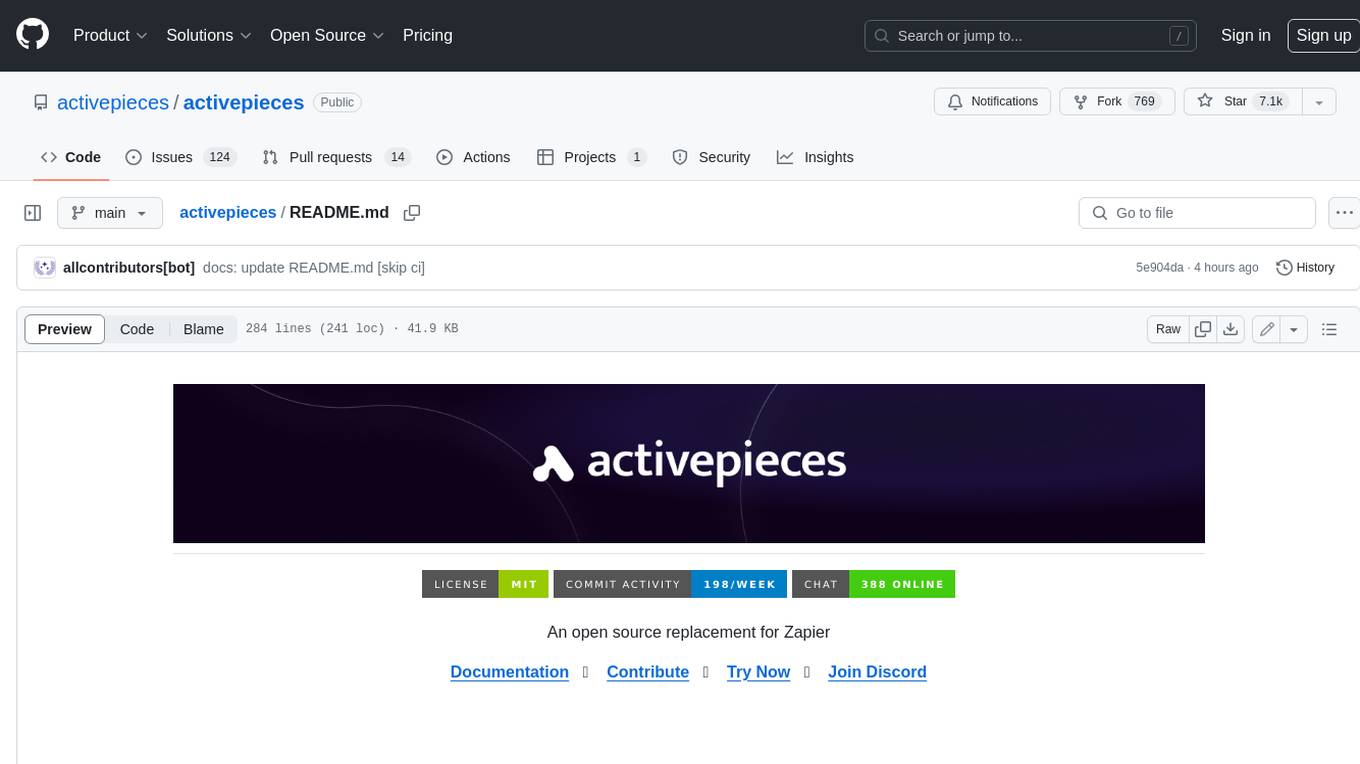
activepieces
Activepieces is an open source replacement for Zapier, designed to be extensible through a type-safe pieces framework written in Typescript. It features a user-friendly Workflow Builder with support for Branches, Loops, and Drag and Drop. Activepieces integrates with Google Sheets, OpenAI, Discord, and RSS, along with 80+ other integrations. The list of supported integrations continues to grow rapidly, thanks to valuable contributions from the community. Activepieces is an open ecosystem; all piece source code is available in the repository, and they are versioned and published directly to npmjs.com upon contributions. If you cannot find a specific piece on the pieces roadmap, please submit a request by visiting the following link: Request Piece Alternatively, if you are a developer, you can quickly build your own piece using our TypeScript framework. For guidance, please refer to the following guide: Contributor's Guide