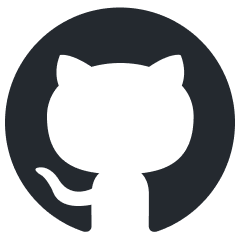
CEO-Agentic-AI-Framework
An ultra-lightweight agentic AI framework based on the ReAct paradigm.
Stars: 190
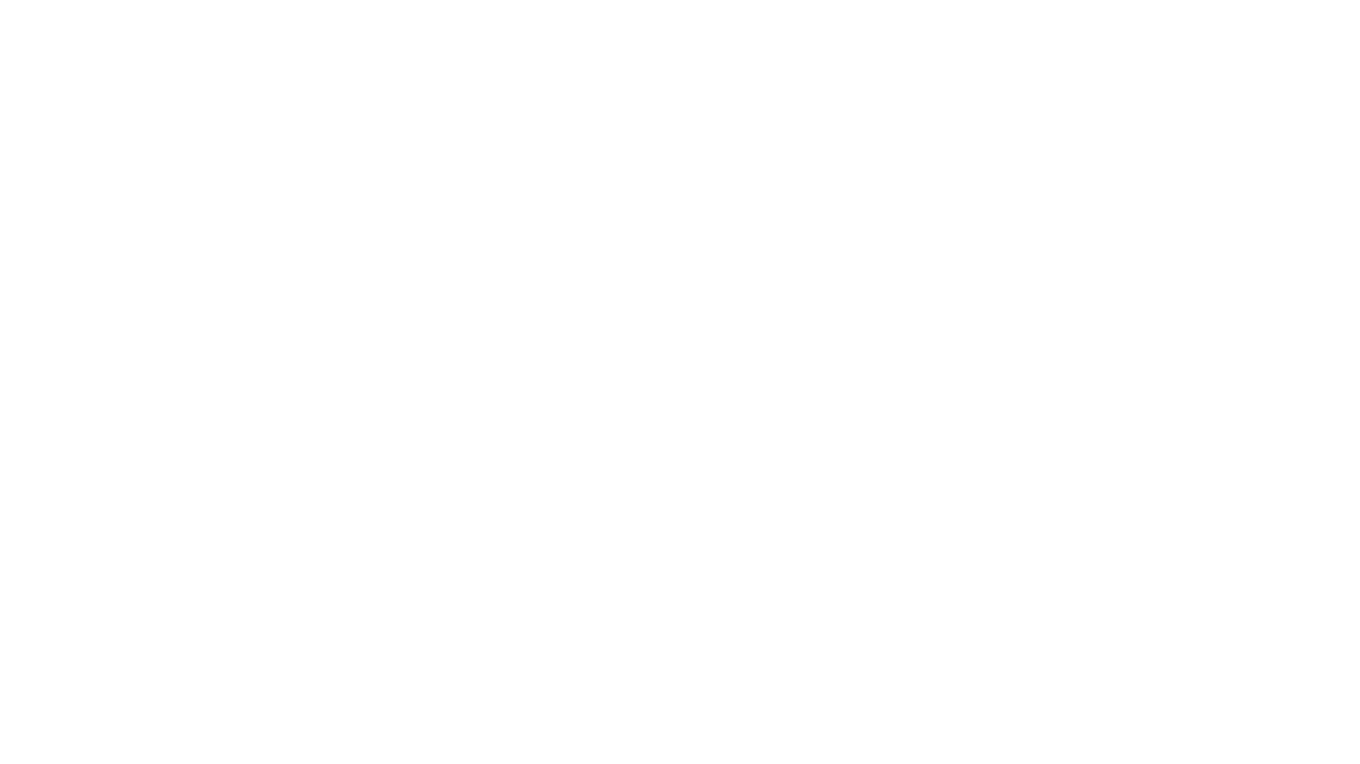
CEO-Agentic-AI-Framework is an ultra-lightweight Agentic AI framework based on the ReAct paradigm. It supports mainstream LLMs and is stronger than Swarm. The framework allows users to build their own agents, assign tasks, and interact with them through a set of predefined abilities. Users can customize agent personalities, grant and deprive abilities, and assign queries for specific tasks. CEO also supports multi-agent collaboration scenarios, where different agents with distinct capabilities can work together to achieve complex tasks. The framework provides a quick start guide, examples, and detailed documentation for seamless integration into research projects.
README:
An ultra-lightweight agentic AI framework based on the ReAct paradigm.
MCP is currently supported. How to use McpAgent.
This study proposes a lightweight autonomous agent framework based on the ReAct paradigm, designed to solve complex tasks through adaptive decision-making and multi-agent collaboration. Unlike traditional frameworks that generate fixed workflows in advance, this framework dynamically decides the next move during execution based solely on the current state. To address the issue of inappropriate termination that may result from adaptive execution paths, a novel abandonment strategy based on a probabilistic penalty mechanism is introduced. Additionally, to enable multi-agent collaboration, a memory-passing mechanism is introduced, allowing agents to share and dynamically update memories. The framework decomposes complex tasks into sub-tasks and plans their execution order and required capabilities according to the agents' abilities, enhancing task execution efficiency and relevance. The innovative abandonment algorithm dynamically adjusts the agent's task abandonment probability via a probabilistic penalty mechanism. By tuning the algorithm's hyperparameters, the agent's execution strategy can be balanced between conservative and exploratory tendencies, significantly improving adaptability and efficiency in complex environments. Moreover, the framework's modular design and tool-learning technology support flexible extensibility and ease of use, enabling customization and optimization based on actual needs. Agents can enhance their functionality using external tools and improve tool usage effectiveness through continuous learning and optimization. The multi-agent collaboration mechanism, with clear division and cooperation, allows each agent to focus on specific task parts, significantly boosting execution efficiency and quality.
The experimental results demonstrate that the ceo-py
framework significantly outperforms autogen
and langchain
in handling tasks of varying complexity, especially in multi-step tasks with possible failures.
Framework | Version | Model | one-step-task | multi-step-task | multi-step-task-with-possible-failure |
---|---|---|---|---|---|
ceo-py |
0.12.3rc0 |
gpt-4o-mini qwen-plus deepseek-v3 |
96.7%100%100% | 100%96.7%100% | 76.7%93.3%93.3% |
autogen |
0.4.9.2 |
gpt-4o-mini qwen-plus deepseek-v3 |
90%90%N/A | 53.3%0%N/A | 3.3%3.3%N/A |
langchain |
0.3.21 |
gpt-4o-mini qwen-plus deepseek-v3 |
73.3%73.3%76.7% | 13.3%13.3%13.3% | 10%13.3%6.7% |
-
one-step-task
: Tasks that can be completed with a single tool call. -
multi-step-task
: Tasks that require multiple tool calls to complete, with no possibility of tool failure. -
multi-step-task-with-possible-failure
: Tasks that require multiple tool calls to complete, where tools may fail, requiring the agent to retry and correct errors.
The deepseek-v3 model is not supported by
autogen-agentchat==0.4.9.2
.
You can reproduce my experiments here.
If you are incorporating the CEO
framework into your research, please remember to properly cite it to acknowledge its contribution to your work.
Если вы интегрируете фреймворк CEO
в своё исследование, пожалуйста, не забудьте правильно сослаться на него, указывая его вклад в вашу работу.
もしあなたが研究に CEO
フレームワークを組み入れているなら、その貢献を認めるために適切に引用することを忘れないでください.
如果您正在將 CEO
框架整合到您的研究中,請務必正確引用它,以聲明它對您工作的貢獻.
@software {CEO,
author = {Zihao Wu},
title = {CEO: An ultra-lightweight agentic AI framework based on the ReAct paradigm},
publisher = {Github},
howpublished = {\url{https://github.com/vortezwohl/CEO-Agentic-AI-Framework}},
year = {2024},
date = {2024-10-25}
}
-
From PYPI
pip install ceo-py
-
From Github
Get access to unreleased features.
pip install git+https://github.com/vortezwohl/CEO-Agentic-AI-Framework.git
To start building your own agent, follow the steps listed.
-
set environmental variable
OPENAI_API_KEY
# .env OPENAI_API_KEY=sk-...
-
import required dependencies
-
Agent
lets you instantiate an agent. -
Personality
is an enumeration class used for customizing personalities of agents.-
Personality.PRUDENT
makes the agent's behavior more cautious. -
Personality.INQUISITIVE
encourages the agent to be more proactive in trying and exploring.
-
-
get_openai_model
gives you aBaseChatModel
as thought engine. -
@ability(brain: BaseChatModel, cache: bool = True, cache_dir: str = '')
is a decorator which lets you declare a function as anAbility
. -
@agentic(agent: Agent)
is a decorator which lets you declare a function as anAgenticAbility
.
from ceo import ( Agent, Personality, get_openai_model, ability, agentic )
-
-
declare functions as basic abilities
@ability def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.'
-
instantiate an agent
You can grant abilities to agents while instantiating them.
model = get_openai_model() agent = Agent(abilities=[calculator, write_file], brain=model, name='CEO', personality=Personality.INQUISITIVE)
-
You can also grant more abilities to agents later:
agent.grant_ability(calculator)
or
agent.grant_abilities([calculator])
-
To deprive abilities:
agent.deprive_ability(calculator)
or
agent.deprive_abilities([calculator])
You can change an agent's personality using method
change_personality(personality: Personality)
agent.change_personality(Personality.PRUDENT)
-
-
assign a request to your agent
agent.assign("Here is a sphere with radius of 9.5 cm and pi here is 3.14159, find the area and volume respectively then write the results into a file called 'result.txt'.")
-
leave the rest to your agent
response = agent.just_do_it() print(response)
ceo
also supports multi-agent collaboration scenario, declare a function as agent calling ability with@agentic(agent: Agent)
, then grant it to an agent. See example.
Integration with MCP
I provide McpAgent
to support tool calls based on the MCP protocol. Below is a brief guide to integrating McpAgent
with mcp.stdio_client
:
-
import required dependencies
-
McpAgent
allows you to instantiate an agent capable of accessing MCP tools. -
StdioMcpConfig
is an alias formcp.client.stdio.StdioServerParameters
and serves as the MCP server connection configuration. -
@mcp_session(mcp_config: StdioMcpConfig)
allows you to declare a function as an MCP session. -
sync_call
allows you to synchronizedly call a coroutine function.
from ceo import ( McpAgent, get_openai_model, StdioMcpConfig, mcp_session, sync_call )
-
-
create an MCP session
-
To connect with a stdio based MCP server, use
StdioMcpConfig
.mcp_config = StdioMcpConfig( command='python', args=['./my_stdio_mcp_server.py'], env=dict(), cwd='./mcp_servers' )
A function decorated with
@mcp_session
will receive an MCP session instance as its first parameter. A function can be decorated with multiple@mcp_session
decorators to access sessions for different MCP servers.@sync_call @mcp_session(mcp_config) async def run(session, request: str) -> str: ...
-
To connect via HTTP with a SSE based MCP server, just provide the URL.
@sync_call @mcp_session('http://localhost:8000/sse') async def run(session, request: str) -> str: ...
-
To connect via websocket with a WS based MCP server, provide the URL.
@sync_call @mcp_session('ws://localhost:8000/message') async def run(session, request: str) -> str: ...
-
-
create an
McpAgent
instance within the MCP sessionAfter creating
McpAgent
, you need to call thefetch_abilities()
method to retrieve tool configurations from the MCP server.@sync_call @mcp_session(mcp_config) async def run(session, request: str) -> str: mcp_agent = await McpAgent(session=session, brain=model).fetch_abilities() ...
-
assign tasks to the
McpAgent
instance and await execution result@sync_call @mcp_session(mcp_config) async def run(session, request: str) -> str: mcp_agent = await McpAgent(session=session, brain=model).fetch_abilities() result = await mcp_agent.assign(request).just_do_it() return result.conclusion
-
call the function
if __name__ == '__main__': ret = run(request='What can you do?') print(ret)
I also provide the complete MCP agent test script. See example.
To make the working process of agents observable, I provide two hooks, namely BeforeActionTaken
and AfterActionTaken
.
They allow you to observe and intervene in the decision-making and execution results of each step of the agent's actions.
You can obtain and modify the agent's decision results for the next action through the BeforeActionTaken
hook,
while AfterActionTaken
allows you to obtain and modify the execution results of the actions (the tampered execution results will be part of the agent's memory).
To start using hooks, follow the steps listed.
-
bring in hooks and messages from
CEO
from ceo.brain.hook import BeforeActionTaken, AfterActionTaken from ceo.message import BeforeActionTakenMessage, AfterActionTakenMessage
-
declare functions and encapsulate them as hooks
def before_action_taken(agent: Agent, message: BeforeActionTakenMessage): print(f'Agent: {agent.name}, Next move: {message}') return message def after_action_taken(agent: Agent, message: AfterActionTakenMessage): print(f'Agent: {agent.name}, Action taken: {message}') return message before_action_taken_hook = BeforeActionTaken(before_action_taken) after_action_taken_hook = AfterActionTaken(after_action_taken)
In these two hook functions, you intercepted the message and printed the information in the message. Afterwards, you returned the message unaltered to the agent. Of course, you also have the option to modify the information in the message, thereby achieving intervention in the agent's working process.
-
use hooks during the agent's working process
agent.assign(...).just_do_it(before_action_taken_hook, after_action_taken_hook)
-
-
Find the surface area and volume of a sphere and write the results into a file.
from ceo import ( Agent, Personality, get_openai_model, ability ) from ceo.brain.hook import BeforeActionTaken, AfterActionTaken from ceo.message import BeforeActionTakenMessage, AfterActionTakenMessage from sympy import simplify from dotenv import load_dotenv load_dotenv() @ability def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.' def before_action_taken(agent: Agent, message: BeforeActionTakenMessage): print(f'Agent: {agent.name}, Next move: {message}') return message def after_action_taken(agent: Agent, message: AfterActionTakenMessage): print(f'Agent: {agent.name}, Action taken: {message}') return message if __name__ == '__main__': ceo = Agent(abilities=[calculator, write_file], brain=get_openai_model(), name='CEO', personality=Personality.INQUISITIVE) radius = '(10.01 * 10.36 * 3.33 / 2 * 16)' # 2762.663904 pi = 3.14159 output_file = 'result.txt' request = f"Here is a sphere with radius of {radius} cm and pi here is {pi}, find the area and volume respectively then write the results into a file called '{output_file}'." result = ceo.assign(request).just_do_it(BeforeActionTaken(before_action_taken), AfterActionTaken(after_action_taken)) # area = 95910378.2949379, volume = 88322713378.13666 print(f'Result: {result}')
# result.txt Surface Area: 95910378.2949379 cm² Volume: 88322713378.1367 cm³
# stdout Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the simplified result as a float. It is designed to accept a single math expression, ensuring that the input is a valid string representation of a mathematical operation."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '(10.01 * 10.36 * 3.33 / 2 * 16)'}) Agent: CEO, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '(10.01 * 10.36 * 3.33 / 2 * 16)'}, returns='2762.66390400000', summarization="I used the calculator ability to evaluate the expression '(10.01 * 10.36 * 3.33 / 2 * 16)', and the result is 2762.66390400000, which indicates the simplified result of the mathematical operation.") Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the simplified result as a float. It is designed to accept a single math expression, ensuring that the input is a valid string representation of a mathematical operation."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '4 * 3.14159 * (2762.66390400000^2)'}) Agent: CEO, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '4 * 3.14159 * (2762.66390400000^2)'}, returns='95910378.2949379', summarization="I used the calculator ability to evaluate the expression '4 * 3.14159 * (2762.66390400000^2)', and the result is 95910378.2949379, which represents the simplified calculation of the given mathematical operation.") Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the simplified result as a float. It is designed to accept a single math expression, ensuring that the input is a valid string representation of a mathematical operation."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '(4/3) * 3.14159 * (2762.66390400000^3)'}) Agent: CEO, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '(4/3) * 3.14159 * (2762.66390400000^3)'}, returns='88322713378.1367', summarization="I used the calculator ability to evaluate the expression '(4/3) * 3.14159 * (2762.66390400000^3)', and the result is 88322713378.1367, which represents the simplified value of the mathematical operation.") Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "write_file", "description": {"brief_description": "The `write_file` function writes the specified content to a file with the given filename. It takes two parameters: `filename`, which is the name of the file to be created or overwritten, and `content`, which is the string data to be written into the file. Upon successful writing, it returns a confirmation message indicating that the content has been written to the specified file."}, "parameters_required": ["filename", "content"], "returns": "<class 'str'>"}, arguments={'filename': 'result.txt', 'content': 'Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³'}) Agent: CEO, Action taken: AfterActionTakenMessage(ability='write_file', arguments={'filename': 'result.txt', 'content': 'Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³'}, returns='Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³ written to result.txt.', summarization="I used the write_file ability to write the specified content about surface area and volume to a file named 'result.txt'. The result confirms that the content was successfully written to the file.") Result: AllDoneMessage(success=True, conclusion="Your request has been fully achieved. The calculations resulted in a surface area of 95910378.2949379 cm² and a volume of 88322713378.1367 cm³, which were successfully written to 'result.txt'.", raw_response="--THOUGHT-PROCESS-- \n(Start) [Calculate radius]: I evaluated the expression '(10.01 * 10.36 * 3.33 / 2 * 16)' and obtained the radius as 2762.66390400000 cm. (--SUCCESS--) \n(After: Calculate radius) [Calculate surface area]: I evaluated the expression '4 * 3.14159 * (2762.66390400000^2)' and obtained the surface area as 95910378.2949379 cm². (--SUCCESS--) \n(After: Calculate surface area) [Calculate volume]: I evaluated the expression '(4/3) * 3.14159 * (2762.66390400000^3)' and obtained the volume as 88322713378.1367 cm³. (--SUCCESS--) \n(After: Calculate volume) [Write results to file]: I wrote the surface area and volume to 'result.txt'. The content was successfully written. (--SUCCESS--) \n\nBased on above assessments, here is my conclusion: \n--CONCLUSION-- \nYour request has been fully achieved. The calculations resulted in a surface area of 95910378.2949379 cm² and a volume of 88322713378.1367 cm³, which were successfully written to 'result.txt'. \n--END--", time_used=62.49354759999551, step_count=4)
-
-
-
Ask the suitable agents to find the surface area and volume of a sphere and write the results into a file.
from sympy import simplify from dotenv import load_dotenv from ceo import ( Agent, Personality, get_openai_model, agentic, ability ) from ceo.brain.hook import BeforeActionTaken, AfterActionTaken from ceo.message import BeforeActionTakenMessage, AfterActionTakenMessage load_dotenv() model = get_openai_model() @ability(model) def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability(model) def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.' jack = Agent(abilities=[calculator], brain=model, name='Jack', personality=Personality.INQUISITIVE) tylor = Agent(abilities=[write_file], brain=model, name='Tylor', personality=Personality.PRUDENT) @agentic(jack) def agent1(): return @agentic(tylor) def agent2(): return def before_action_taken(agent: Agent, message: BeforeActionTakenMessage): print(f'Agent: {agent.name}, Next move: {message}') return message def after_action_taken(agent: Agent, message: AfterActionTakenMessage): print(f'Agent: {agent.name}, Action taken: {message}') return message if __name__ == '__main__': ceo = Agent(abilities=[agent1, agent2], brain=model, name='CEO', personality=Personality.INQUISITIVE) radius = '(10.01 * 10.36 * 3.33 / 2 * 16)' # 2762.663904 pi = 3.14159 output_file = 'result.txt' request = f"Here is a sphere with radius of {radius} cm and pi here is {pi}, find the area and volume respectively then write the results into a file called '{output_file}'." result = ceo.assign(request).just_do_it(BeforeActionTaken(before_action_taken), AfterActionTaken(after_action_taken)) # area = 95910378.2949379, volume = 88322713378.13666 print(f'Result: {result}')
In multi-agent collaboration scenario, you can assign different personalities to each distinct agent. For example, in the aforementioned script, Jack's capability is to perform calculations. I want him to try more and explore more, so Jack's personality is set to
Personality.INQUISITIVE
. On the other hand, Taylor's capability is to create and write files. For operations involving interaction with the external file system, I want him to be more cautious, so Taylor's personality is set toPersonality.PRUDENT
.# result.txt Surface Area: 95910378.2949379 cm² Volume: 88322713378.1367 cm³
# stdout Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "__AgenticAbility__talk_to_Jack", "description": {"brief_description": "Initiates a conversation with \"Jack\" to use its abilities.", "detailed_description": "First, carefully consider and explore Jack's potential abilities in solving your tasks, then, if you need Jack's help, you must tell comprehensively, precisely and exactly what you need Jack to do.", "self_introduction_from_Jack": "My name is Jack. What can I do: I can evaluate mathematical expressions as a calculator and provide the result as a float. Additionally, I have the ability to retrieve my personal information, but this can only be done once.", "hint": "By reading <self_introduction_from_Jack>, you can learn what Jack can do, and then decide whether to initiates a conversation with Jack according to its abilities."}, "parameters_required": [], "returns": "<class 'str'>"}, arguments={'expression': '(10.01 * 10.36 * 3.33 / 2 * 16)'}) Agent: Jack, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the result as a float. It uses the `simplify` function to process the expression and ensure it is correctly computed."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '(10.01 * 10.36 * 3.33 / 2 * 16)'}) Agent: Jack, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '(10.01 * 10.36 * 3.33 / 2 * 16)'}, returns='2762.66390400000', summarization="I used the calculator ability to evaluate the expression '(10.01 * 10.36 * 3.33 / 2 * 16)', and the result is '2762.66390400000', which indicates the computed value of the mathematical expression.") Agent: Jack, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the result as a float. It uses the `simplify` function to process the expression and ensure it is correctly computed."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '4 * 3.14159 * (2762.66390400000^2)'}) Agent: Jack, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '4 * 3.14159 * (2762.66390400000^2)'}, returns='95910378.2949379', summarization="I used the calculator ability to evaluate the expression '4 * 3.14159 * (2762.66390400000^2)', resulting in the value '95910378.2949379'.") Agent: Jack, Next move: BeforeActionTakenMessage(ability={"ability_name": "calculator", "description": {"brief_description": "The `calculator` function evaluates a mathematical expression provided as a string and returns the result as a float. It uses the `simplify` function to process the expression and ensure it is correctly computed."}, "parameters_required": ["expr"], "returns": "<class 'float'>"}, arguments={'expr': '(4/3) * 3.14159 * (2762.66390400000^3)'}) Agent: Jack, Action taken: AfterActionTakenMessage(ability='calculator', arguments={'expr': '(4/3) * 3.14159 * (2762.66390400000^3)'}, returns='88322713378.1367', summarization="I used the calculator ability to evaluate the expression '(4/3) * 3.14159 * (2762.66390400000^3)', and the result is 88322713378.1367, which represents the computed volume of a sphere with a radius of approximately 2762.66.") Agent: CEO, Action taken: AfterActionTakenMessage(ability='__AgenticAbility__talk_to_Jack', arguments={'arguments': 'Ask for a favor.'}, returns='{"success": false, "response": "--THOUGHT-PROCESS-- \\n(Initial calculation) [Calculate radius]: I calculated the radius as \'2762.66390400000\' cm. (--SUCCESS--) \\n(After: Calculate radius) [Calculate surface area]: I calculated the surface area using the formula \'4 * 3.14159 * (2762.66390400000^2)\', resulting in \'95910378.2949379\'. (--SUCCESS--) \\n(After: Calculate surface area) [Calculate volume]: I calculated the volume using the formula \'(4/3) * 3.14159 * (2762.66390400000^3)\', resulting in \'88322713378.1367\'. (--SUCCESS--) \\n(After: Calculate volume) [Write results to file]: There is no record in <history> indicating that the results were written to \'result.txt\'. (--FAILED--) \\n\\nBased on above assessments, here is my conclusion: \\n--CONCLUSION-- \\nYour request has not been fully achieved. I calculated the radius as \'2762.66390400000\' cm, the surface area as \'95910378.2949379\', and the volume as \'88322713378.1367\', but the results were not written to \'result.txt\'. \\n--END--"}', summarization="I used the __AgenticAbility__talk_to_Jack ability to ask Jack for a favor. Jack calculated the radius, surface area, and volume of a sphere, but failed to write the results to 'result.txt'.") Agent: CEO, Next move: BeforeActionTakenMessage(ability={"ability_name": "__AgenticAbility__talk_to_Tylor", "description": {"brief_description": "Initiates a conversation with \"Tylor\" to use its abilities.", "detailed_description": "First, carefully consider and explore Tylor's potential abilities in solving your tasks, then, if you need Tylor's help, you must tell comprehensively, precisely and exactly what you need Tylor to do.", "self_introduction_from_Tylor": "My name is Tylor. What can I do: I can write content to a file and provide my personal information upon request, but the latter can only be used once.", "hint": "By reading <self_introduction_from_Tylor>, you can learn what Tylor can do, and then decide whether to initiates a conversation with Tylor according to its abilities."}, "parameters_required": [], "returns": "<class 'str'>"}, arguments={'file_name': 'result.txt', 'content': 'Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³'}) Agent: Tylor, Next move: BeforeActionTakenMessage(ability={"ability_name": "write_file", "description": {"brief_description": "The `write_file` function writes the specified content to a file with the given filename. It opens the file in write mode, encodes the content in UTF-8, and returns a confirmation message indicating that the content has been successfully written to the file."}, "parameters_required": ["filename", "content"], "returns": "<class 'str'>"}, arguments={'filename': 'result.txt', 'content': 'Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³'}) Agent: Tylor, Action taken: AfterActionTakenMessage(ability='write_file', arguments={'filename': 'result.txt', 'content': 'Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³'}, returns='Surface Area: 95910378.2949379 cm²\nVolume: 88322713378.1367 cm³ written to result.txt.', summarization="I used the write_file ability to write the specified content about surface area and volume to a file named 'result.txt'. The result confirms that the content was successfully written to the file.") Agent: CEO, Action taken: AfterActionTakenMessage(ability='__AgenticAbility__talk_to_Tylor', arguments={'arguments': 'Ask for a favor.'}, returns='{"success": true, "response": "--THOUGHT-PROCESS-- \\n(Initial calculation) [Calculate radius]: The radius was calculated as \'2762.66390400000\' cm. (--SUCCESS--) \\n(After: Calculate radius) [Calculate surface area]: The surface area was calculated using the formula \'4 * 3.14159 * (2762.66390400000^2)\', resulting in \'95910378.2949379\'. (--SUCCESS--) \\n(After: Calculate surface area) [Calculate volume]: The volume was calculated using the formula \'(4/3) * 3.14159 * (2762.66390400000^3)\', resulting in \'88322713378.1367\'. (--SUCCESS--) \\n(After: Calculate volume) [Write results to file]: The results were successfully written to \'result.txt\'. (--SUCCESS--) \\n\\nBased on above assessments, here is my conclusion: \\n--CONCLUSION-- \\nYour request has been fully achieved. The radius was calculated as \'2762.66390400000\' cm, the surface area as \'95910378.2949379\' cm², and the volume as \'88322713378.1367\' cm³. The results were successfully written to \'result.txt\'. \\n--END-- "}', summarization="I used the __AgenticAbility__talk_to_Tylor ability to ask Tylor for a favor, which involved calculating the radius, surface area, and volume of a sphere. The results were successfully computed and written to 'result.txt'.") Result: AllDoneMessage(success=True, conclusion="Your request has been fully achieved. The radius was calculated as '2762.66390400000' cm, the surface area as '95910378.2949379' cm², and the volume as '88322713378.1367' cm³. The results were successfully written to 'result.txt'.", raw_response="--THOUGHT-PROCESS-- \n(Initial calculation) [Calculate radius]: The radius was calculated as '2762.66390400000' cm. (--SUCCESS--) \n(After: Calculate radius) [Calculate surface area]: The surface area was calculated using the formula '4 * 3.14159 * (2762.66390400000^2)', resulting in '95910378.2949379' cm². (--SUCCESS--) \n(After: Calculate surface area) [Calculate volume]: The volume was calculated using the formula '(4/3) * 3.14159 * (2762.66390400000^3)', resulting in '88322713378.1367' cm³. (--SUCCESS--) \n(After: Calculate volume) [Write results to file]: The results were successfully written to 'result.txt'. (--SUCCESS--) \n\nBased on above assessments, here is my conclusion: \n--CONCLUSION-- \nYour request has been fully achieved. The radius was calculated as '2762.66390400000' cm, the surface area as '95910378.2949379' cm², and the volume as '88322713378.1367' cm³. The results were successfully written to 'result.txt'. \n--END-- ", time_used=123.79718699998921, step_count=2)
-
-
-
Ask a browser-use augmented mcp agent to search for and find out what RL is.
from ceo import ( McpAgent, get_openai_model, ability, sync_call, StdioMcpConfig, __BLOG__ ) from ceo.util.mcp_session import mcp_session from ceo.brain.hook import BeforeActionTaken, AfterActionTaken from ceo.message import BeforeActionTakenMessage, AfterActionTakenMessage from dotenv import load_dotenv load_dotenv() model = get_openai_model() stdio_mcp_config = StdioMcpConfig( command='python', args=['./playwright-plus-python-mcp.py'], env=dict(), cwd='./mcp_server' ) @ability(model) def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.' def before_action_taken(agent: McpAgent, message: BeforeActionTakenMessage): print(f'Agent: {agent.name}, Next move: {message.ability.name}') return message def after_action_taken(agent: McpAgent, message: AfterActionTakenMessage): print(f'Agent: {agent.name}, Action taken: {message.summarization}') return message @sync_call @mcp_session(stdio_mcp_config) async def run(session, request: str) -> str: mcp_agent = await McpAgent(session=session, brain=model).fetch_abilities() mcp_agent.grant_ability(write_file) result = await mcp_agent.assign(request).just_do_it( BeforeActionTaken(before_action_taken), AfterActionTaken(after_action_taken) ) return result.conclusion if __name__ == '__main__': output_file = 'result.txt' request = (f'What is reinforcement learning? Bing (www.bing.com) it and write down the search results into local file: {output_file}. ' f'Then navigate to {__BLOG__}.') ret = run(request) print(ret)
# result.txt Reinforcement Learning: An Overview Reinforcement Learning (RL) Key Concepts of Reinforcement Learning Agent: The learner or decision-maker. Agent Environment: Everything the agent interacts with. Environment State: A specific situation in which the agent finds itself. State Action: All possible moves the agent can make. Action Reward: Feedback from the environment based on the action taken 1. Reward How Reinforcement Learning Works IBM https://www.ibm.com › think › topics › reinforcement-learning What is reinforcement learning? | IBM 2024年3月25日 · In reinforcement learning, an agent learns to make decisions by interacting with an environment. It is used in robotics and other decision-making settings. Reinforcement … 2024年3月25日 Wikipedia https://en.wikipedia.org › wiki › Reinforcement_learning Reinforcement learning - Wikipedia Overview Principles Exploration Algorithms for control learning Theory Research Comparison of key algorithms Statistical comparison of reinforcement learning algorithms machine learning optimal control intelligent agent take actions maximize a reward three basic machine learning paradigms supervised learning unsupervised learning Wikipedia · CC-BY-SA 许可下的文字 · CC-BY-SA 许可下的文字 CC-BY-SA 许可 预计阅读时间:10 分钟 其他用户还问了以下问题 What are reinforcement learning algorithms? Reinforcement learning encompasses a variety of algorithms. Some of the most notable include: Q-learning is a model-free, value-based algorithm that learns an action-value function (Q-function) to estimate the expected cumulative reward for taking a given action in a given state. Q-learning What is reinforcement learning? - cudocompute.com cudocompute.com What is reinforcement learning (RL)? Reinforcement learning (RL) is a type of machine learning process that focuses on decision making by autonomous agents. An autonomous agent is any system that can make decisions and act in response to its environment independent of direct instruction by a human user. Robots and self-driving cars are examples of autonomous agents.
# stdout Agent: 智能體47239e型號, Next move: playwright_navigate Agent: 智能體47239e型號, Action taken: I utilized the **playwright_navigate** ability to navigate to the URL "https://www.bing.com/search?q=What+is+reinforcement+learning". The result confirms that the navigation was successful, and the page content includes search results related to "reinforcement learning", such as articles from IBM, Wikipedia, and other sources. This operation successfully retrieved the desired webpage content for further processing or analysis. Agent: 智能體47239e型號, Next move: write_file Agent: 智能體47239e型號, Action taken: I used the `write_file` ability to write a detailed overview of Reinforcement Learning into a file named "result.txt". The content included key concepts such as Agent, Environment, State, Action, and Reward, along with additional information from sources like IBM and Wikipedia. The result confirms that the content was successfully written to the specified file. Agent: 智能體47239e型號, Next move: playwright_navigate Agent: 智能體47239e型號, Action taken: I used the playwright_navigate ability to navigate to the URL "https://vortezwohl.github.io". The result confirms that the navigation was successful, and the page content includes text such as "vortezwohl", "About", "Years", "Categories", "Tags", and various blog post titles related to reinforcement learning, machine learning concepts, and other technical topics. Your request has been fully achieved. I searched for "What is reinforcement learning" on Bing, retrieved relevant information, and wrote it into a file named "result.txt". Subsequently, I navigated to the website "https://vortezwohl.github.io", confirming that the navigation was successful and the page content was as expected.
-
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for CEO-Agentic-AI-Framework
Similar Open Source Tools
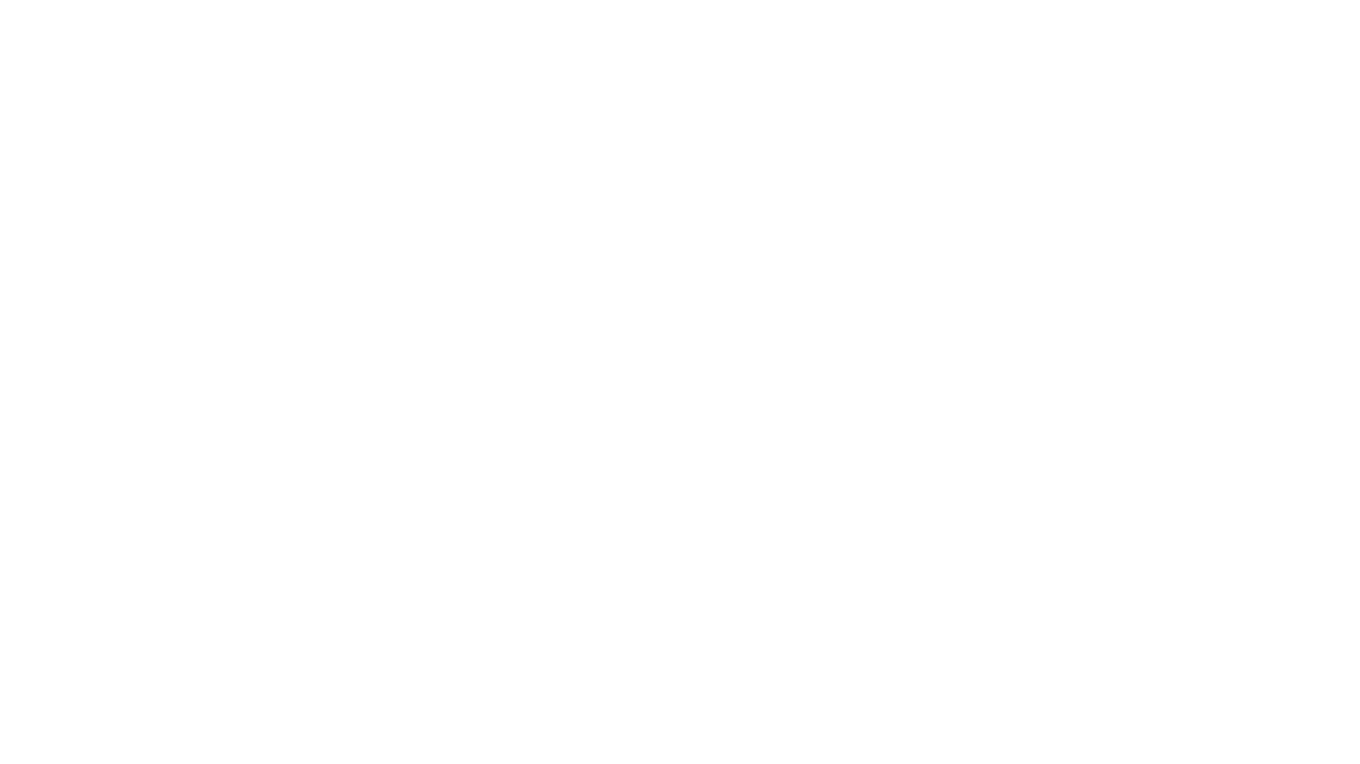
CEO-Agentic-AI-Framework
CEO-Agentic-AI-Framework is an ultra-lightweight Agentic AI framework based on the ReAct paradigm. It supports mainstream LLMs and is stronger than Swarm. The framework allows users to build their own agents, assign tasks, and interact with them through a set of predefined abilities. Users can customize agent personalities, grant and deprive abilities, and assign queries for specific tasks. CEO also supports multi-agent collaboration scenarios, where different agents with distinct capabilities can work together to achieve complex tasks. The framework provides a quick start guide, examples, and detailed documentation for seamless integration into research projects.
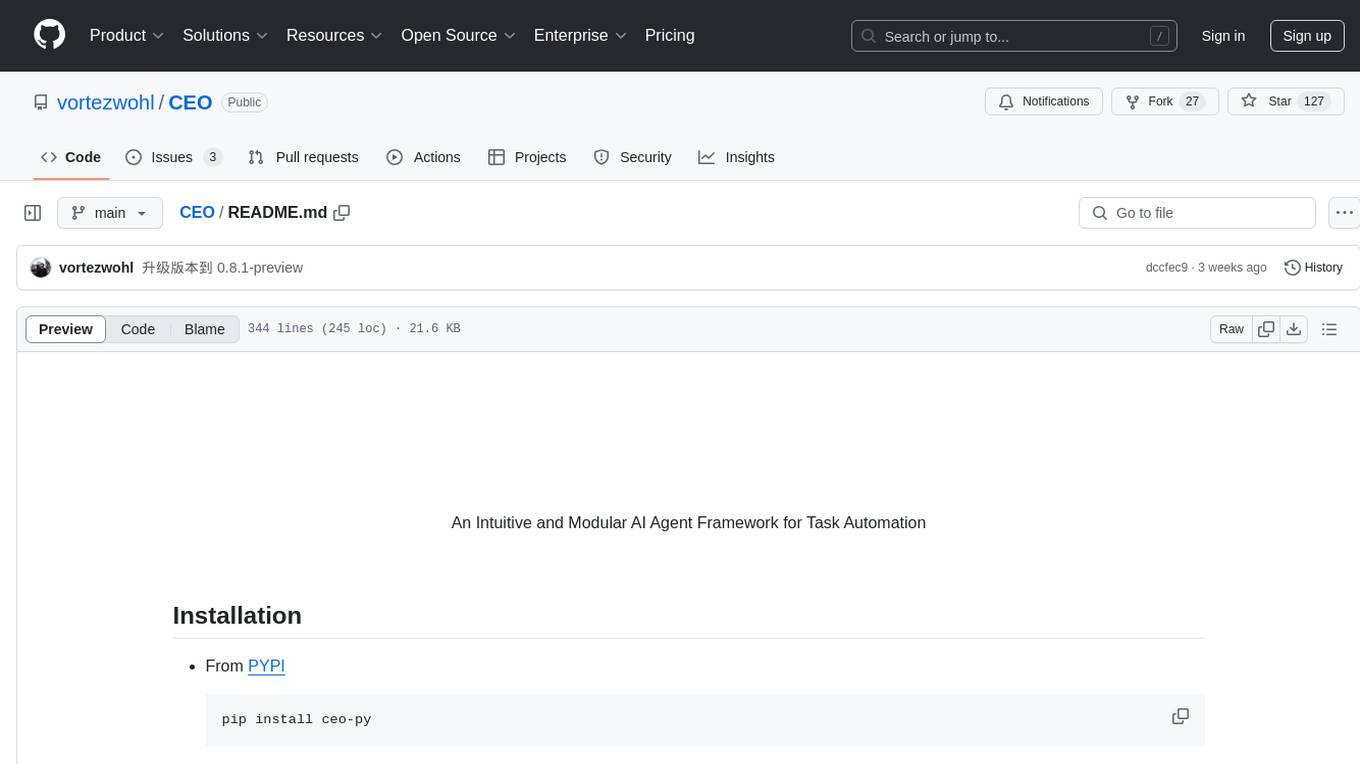
CEO
CEO is an intuitive and modular AI agent framework designed for task automation. It provides a flexible environment for building agents with specific abilities and personalities, allowing users to assign tasks and interact with the agents to automate various processes. The framework supports multi-agent collaboration scenarios and offers functionalities like instantiating agents, granting abilities, assigning queries, and executing tasks. Users can customize agent personalities and define specific abilities using decorators, making it easy to create complex automation workflows.
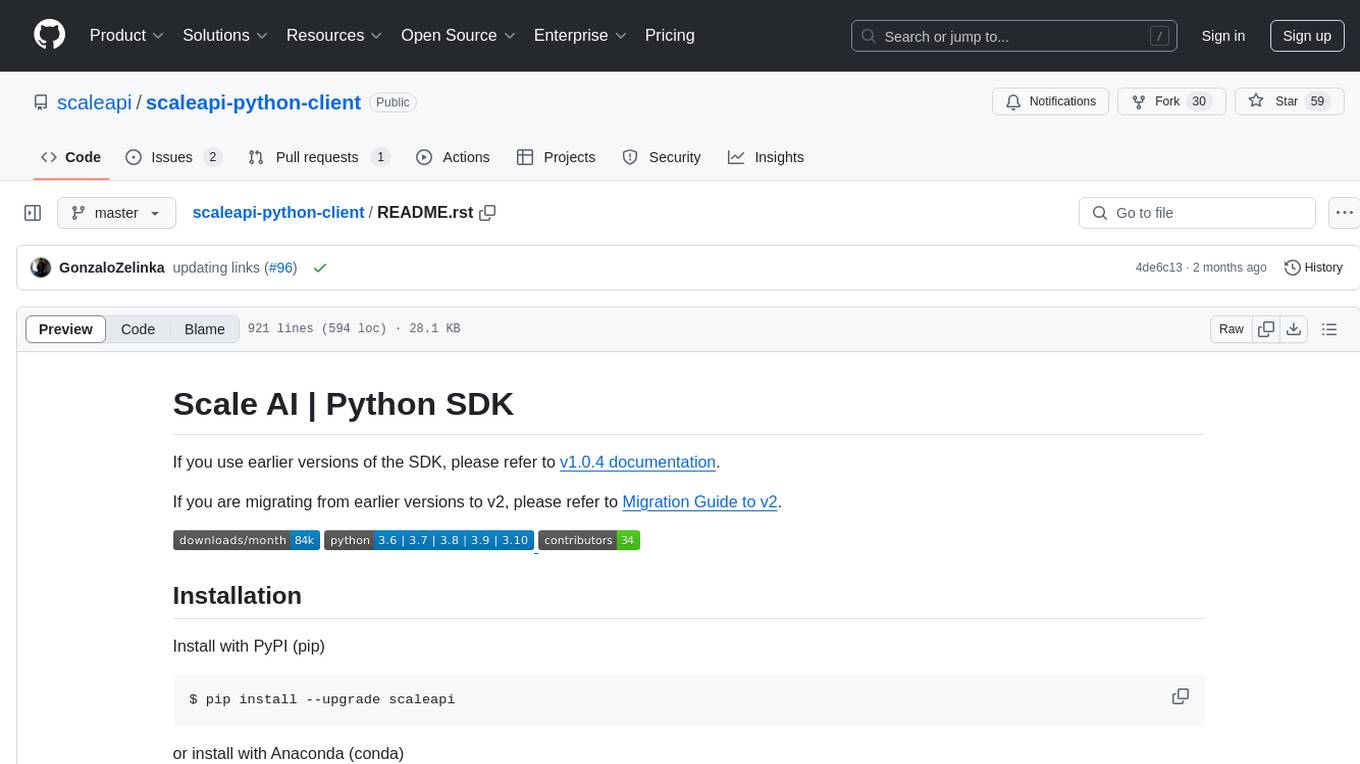
scaleapi-python-client
The Scale AI Python SDK is a tool that provides a Python interface for interacting with the Scale API. It allows users to easily create tasks, manage projects, upload files, and work with evaluation tasks, training tasks, and Studio assignments. The SDK handles error handling and provides detailed documentation for each method. Users can also manage teammates, project groups, and batches within the Scale Studio environment. The SDK supports various functionalities such as creating tasks, retrieving tasks, canceling tasks, auditing tasks, updating task attributes, managing files, managing team members, and working with evaluation and training tasks.
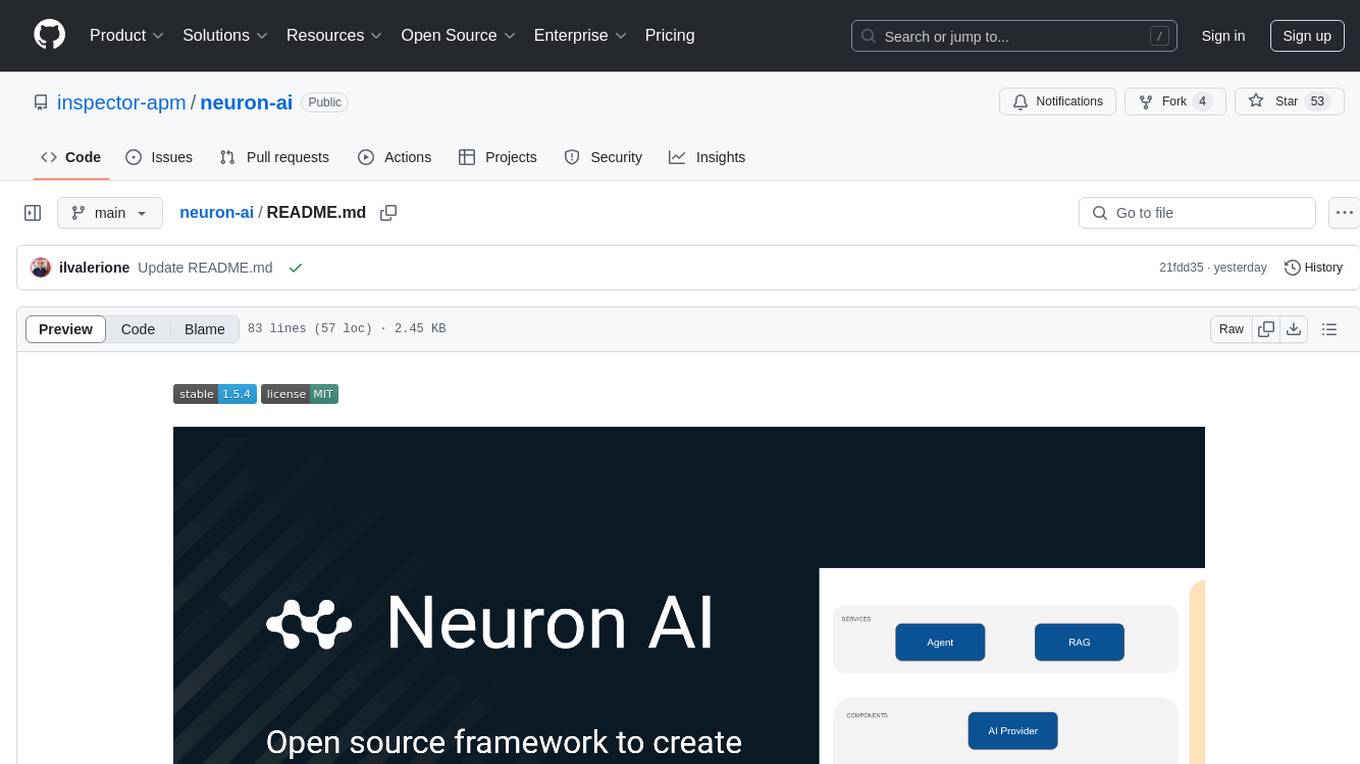
neuron-ai
Neuron AI is a PHP framework that provides an Agent class for creating fully functional agents to perform tasks like analyzing text for SEO optimization. The framework manages advanced mechanisms such as memory, tools, and function calls. Users can extend the Agent class to create custom agents and interact with them to get responses based on the underlying LLM. Neuron AI aims to simplify the development of AI-powered applications by offering a structured framework with documentation and guidelines for contributions under the MIT license.
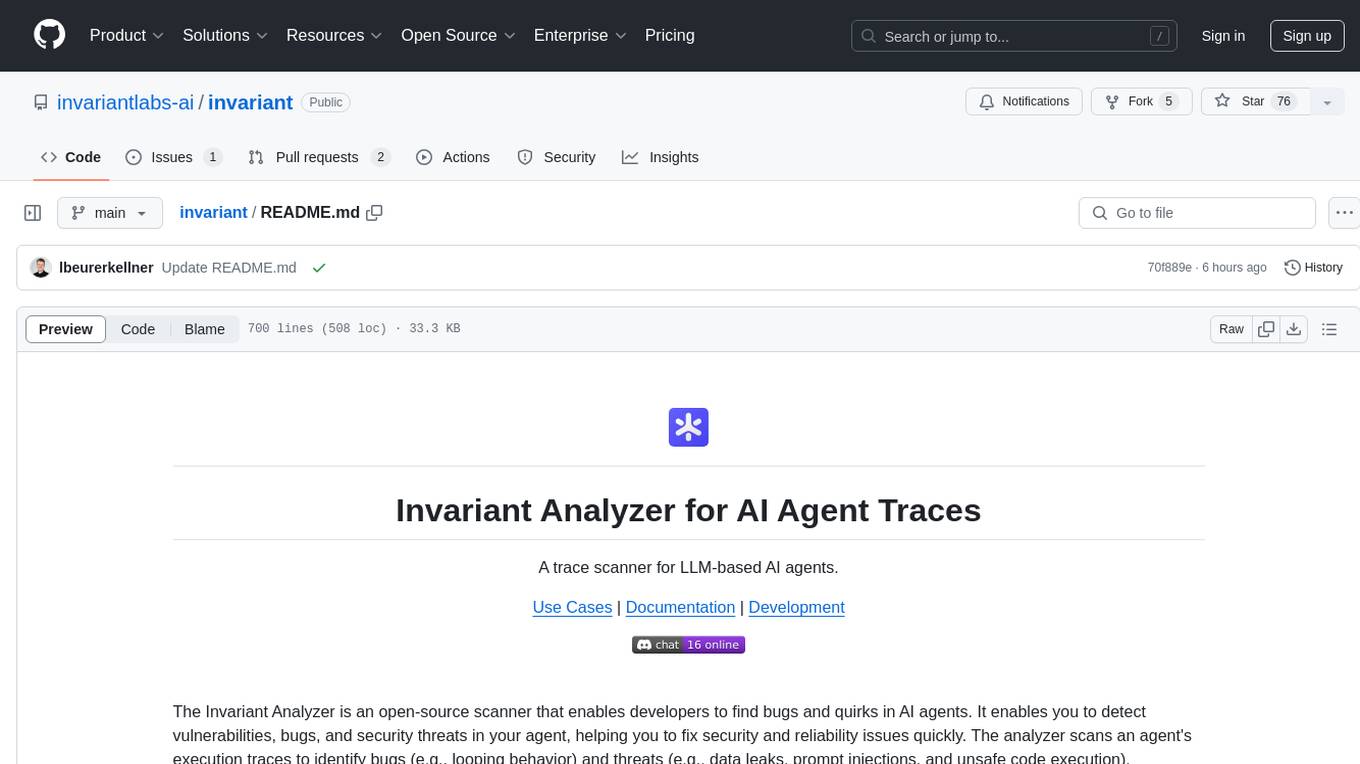
invariant
Invariant Analyzer is an open-source scanner designed for LLM-based AI agents to find bugs, vulnerabilities, and security threats. It scans agent execution traces to identify issues like looping behavior, data leaks, prompt injections, and unsafe code execution. The tool offers a library of built-in checkers, an expressive policy language, data flow analysis, real-time monitoring, and extensible architecture for custom checkers. It helps developers debug AI agents, scan for security violations, and prevent security issues and data breaches during runtime. The analyzer leverages deep contextual understanding and a purpose-built rule matching engine for security policy enforcement.
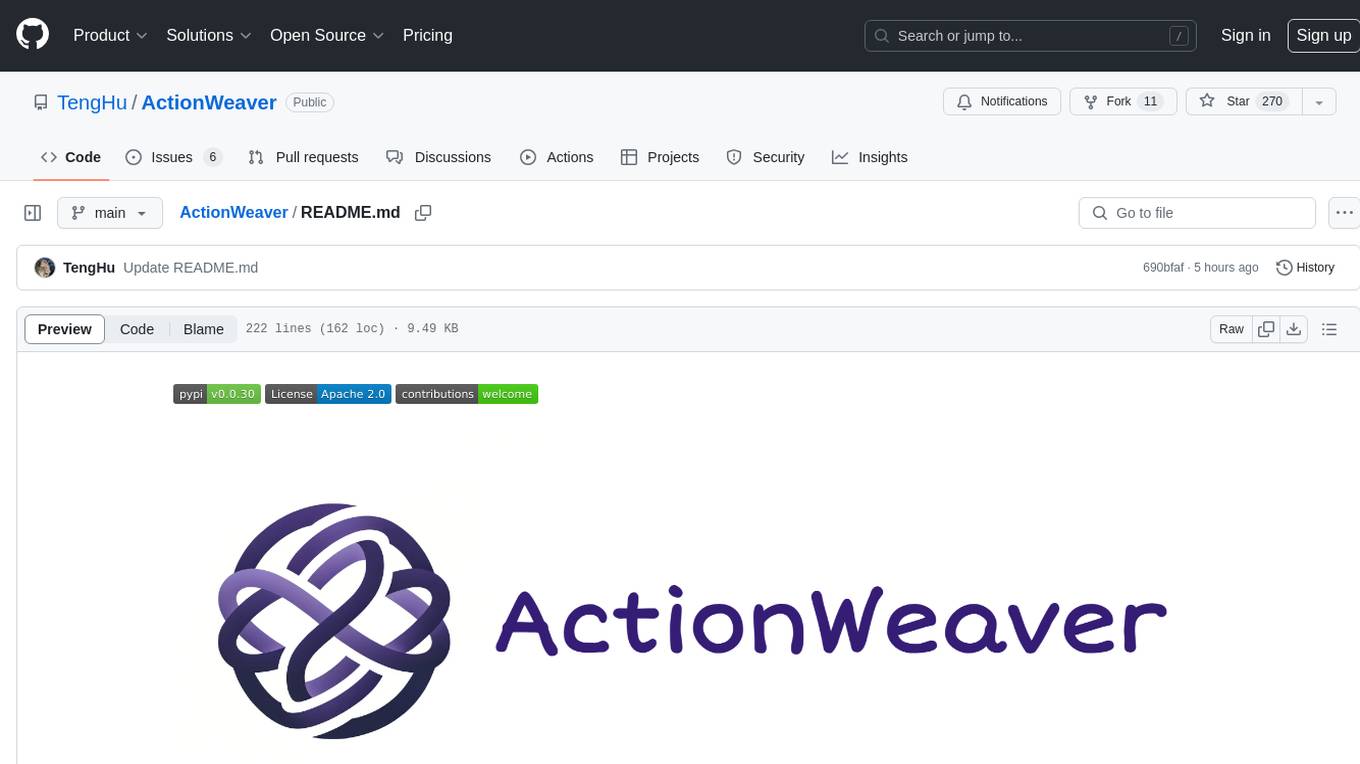
ActionWeaver
ActionWeaver is an AI application framework designed for simplicity, relying on OpenAI and Pydantic. It supports both OpenAI API and Azure OpenAI service. The framework allows for function calling as a core feature, extensibility to integrate any Python code, function orchestration for building complex call hierarchies, and telemetry and observability integration. Users can easily install ActionWeaver using pip and leverage its capabilities to create, invoke, and orchestrate actions with the language model. The framework also provides structured extraction using Pydantic models and allows for exception handling customization. Contributions to the project are welcome, and users are encouraged to cite ActionWeaver if found useful.
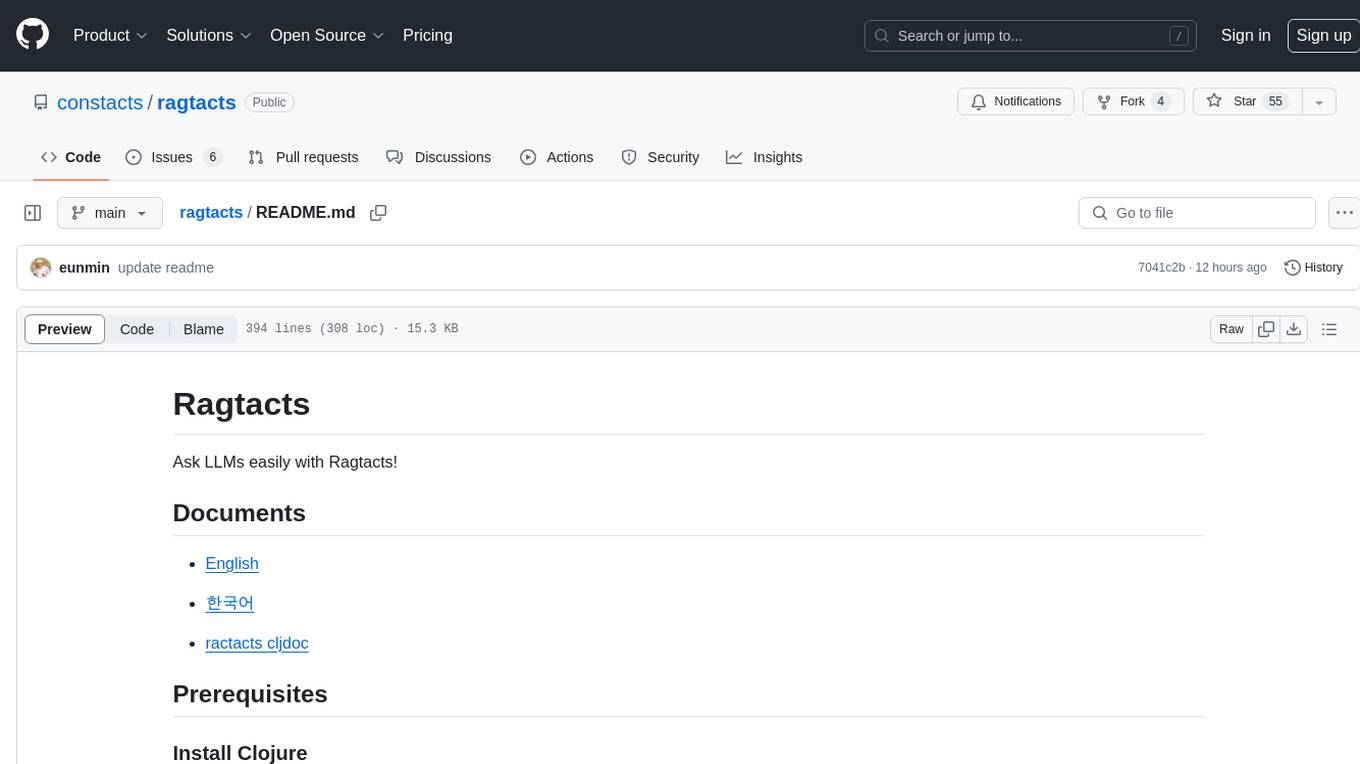
ragtacts
Ragtacts is a Clojure library that allows users to easily interact with Large Language Models (LLMs) such as OpenAI's GPT-4. Users can ask questions to LLMs, create question templates, call Clojure functions in natural language, and utilize vector databases for more accurate answers. Ragtacts also supports RAG (Retrieval-Augmented Generation) method for enhancing LLM output by incorporating external data. Users can use Ragtacts as a CLI tool, API server, or through a RAG Playground for interactive querying.
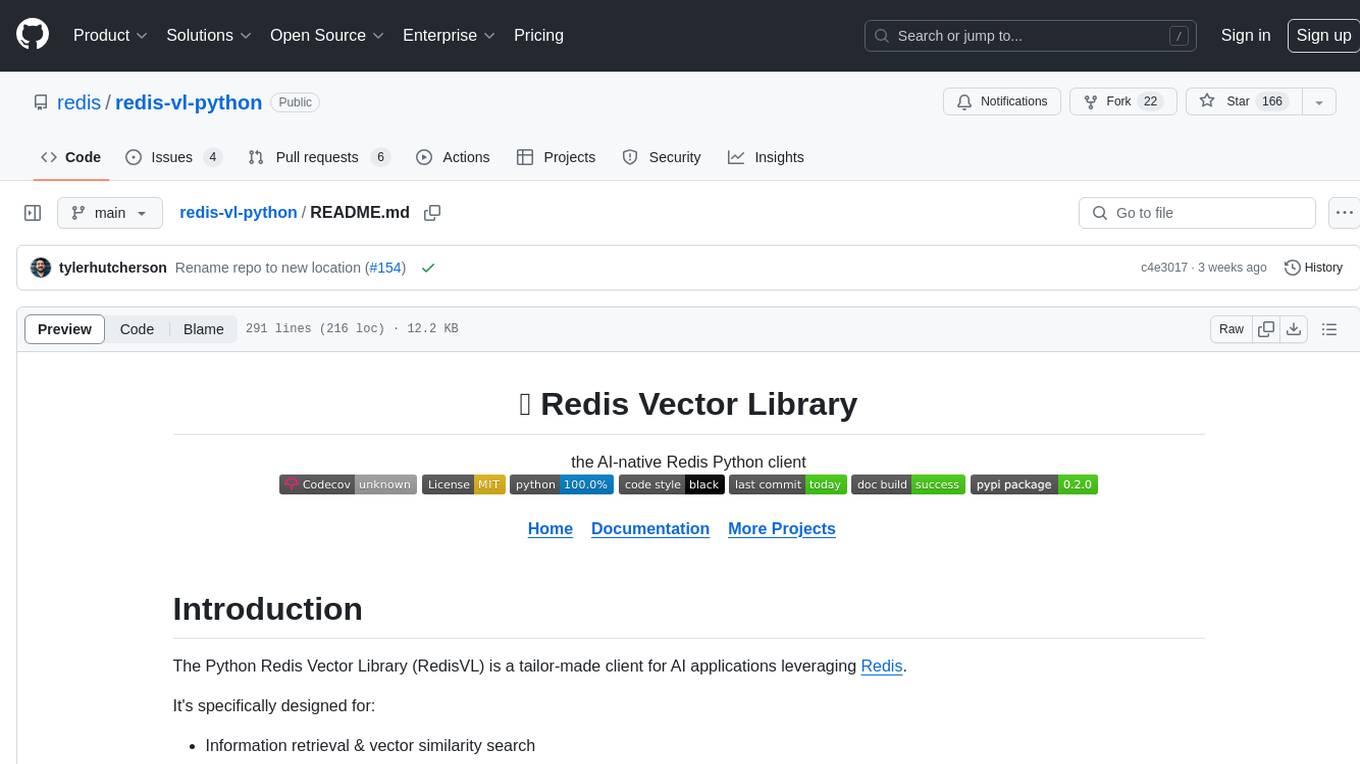
redis-vl-python
The Python Redis Vector Library (RedisVL) is a tailor-made client for AI applications leveraging Redis. It enhances applications with Redis' speed, flexibility, and reliability, incorporating capabilities like vector-based semantic search, full-text search, and geo-spatial search. The library bridges the gap between the emerging AI-native developer ecosystem and the capabilities of Redis by providing a lightweight, elegant, and intuitive interface. It abstracts the features of Redis into a grammar that is more aligned to the needs of today's AI/ML Engineers or Data Scientists.
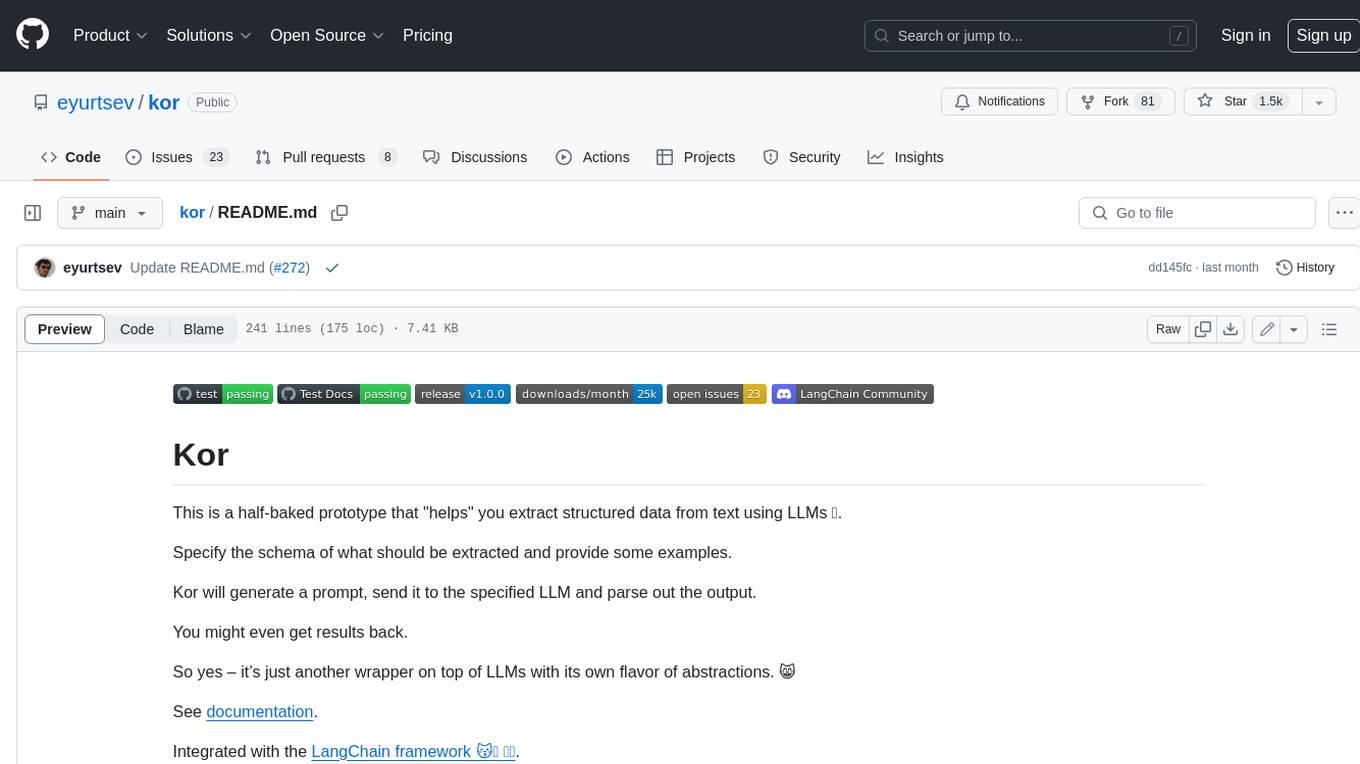
kor
Kor is a prototype tool designed to help users extract structured data from text using Language Models (LLMs). It generates prompts, sends them to specified LLMs, and parses the output. The tool works with the parsing approach and is integrated with the LangChain framework. Kor is compatible with pydantic v2 and v1, and schema is typed checked using pydantic. It is primarily used for extracting information from text based on provided reference examples and schema documentation. Kor is designed to work with all good-enough LLMs regardless of their support for function/tool calling or JSON modes.
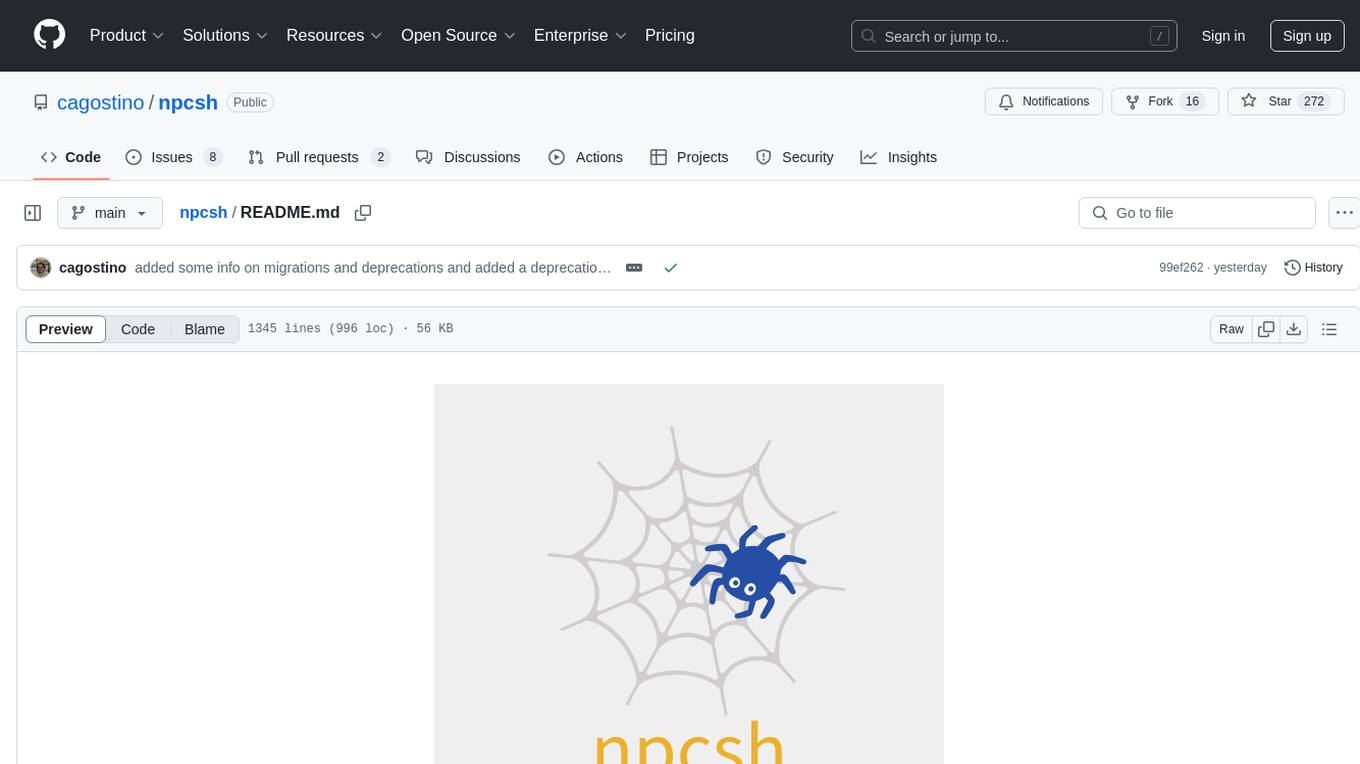
npcsh
`npcsh` is a python-based command-line tool designed to integrate Large Language Models (LLMs) and Agents into one's daily workflow by making them available and easily configurable through the command line shell. It leverages the power of LLMs to understand natural language commands and questions, execute tasks, answer queries, and provide relevant information from local files and the web. Users can also build their own tools and call them like macros from the shell. `npcsh` allows users to take advantage of agents (i.e. NPCs) through a managed system, tailoring NPCs to specific tasks and workflows. The tool is extensible with Python, providing useful functions for interacting with LLMs, including explicit coverage for popular providers like ollama, anthropic, openai, gemini, deepseek, and openai-like providers. Users can set up a flask server to expose their NPC team for use as a backend service, run SQL models defined in their project, execute assembly lines, and verify the integrity of their NPC team's interrelations. Users can execute bash commands directly, use favorite command-line tools like VIM, Emacs, ipython, sqlite3, git, pipe the output of these commands to LLMs, or pass LLM results to bash commands.
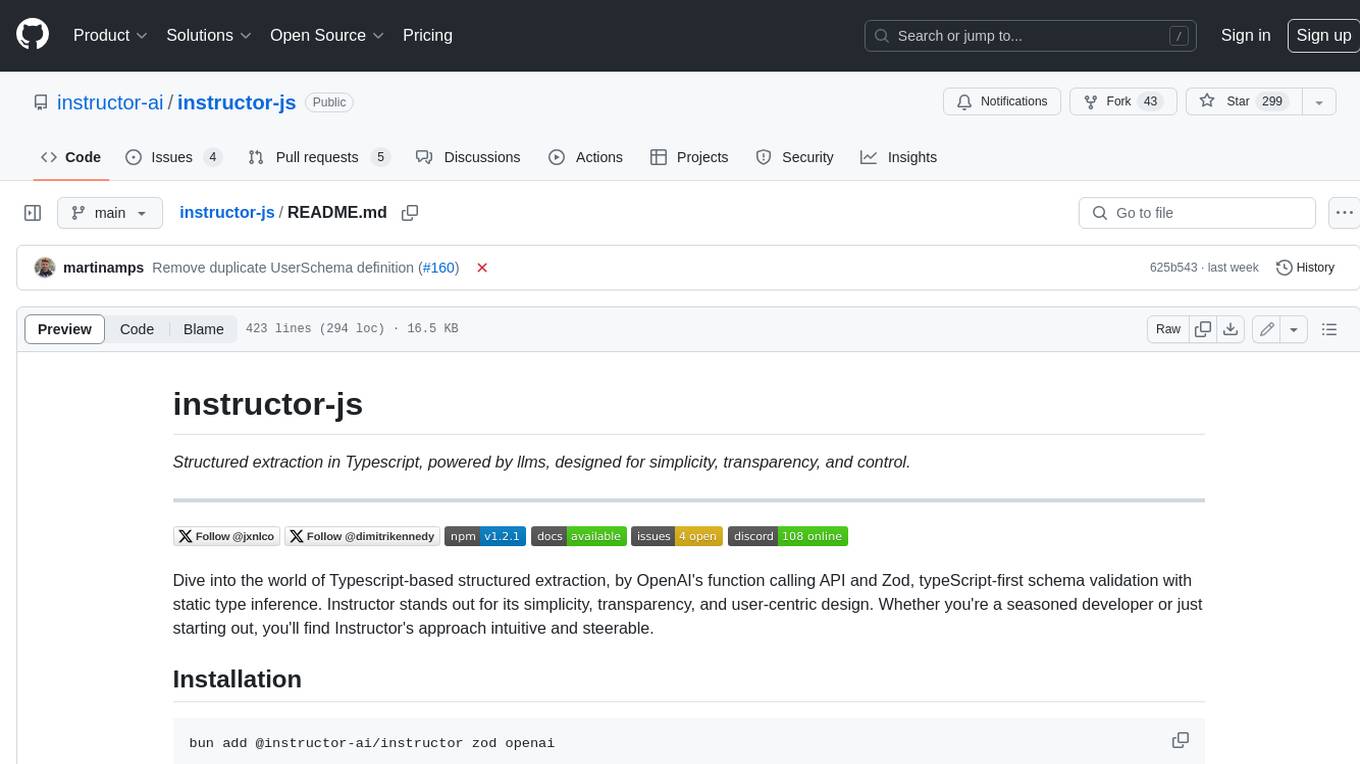
instructor-js
Instructor is a Typescript library for structured extraction in Typescript, powered by llms, designed for simplicity, transparency, and control. It stands out for its simplicity, transparency, and user-centric design. Whether you're a seasoned developer or just starting out, you'll find Instructor's approach intuitive and steerable.
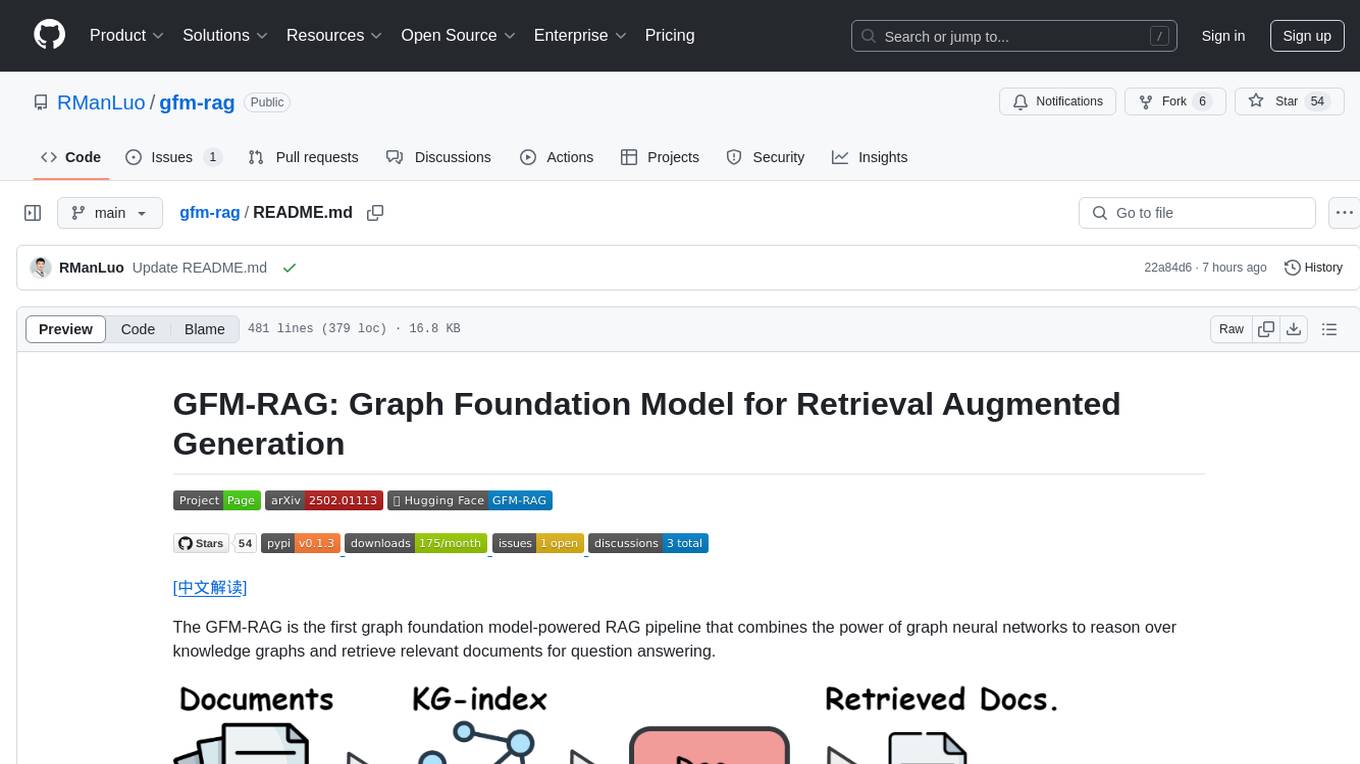
gfm-rag
The GFM-RAG is a graph foundation model-powered pipeline that combines graph neural networks to reason over knowledge graphs and retrieve relevant documents for question answering. It features a knowledge graph index, efficiency in multi-hop reasoning, generalizability to unseen datasets, transferability for fine-tuning, compatibility with agent-based frameworks, and interpretability of reasoning paths. The tool can be used for conducting retrieval and question answering tasks using pre-trained models or fine-tuning on custom datasets.
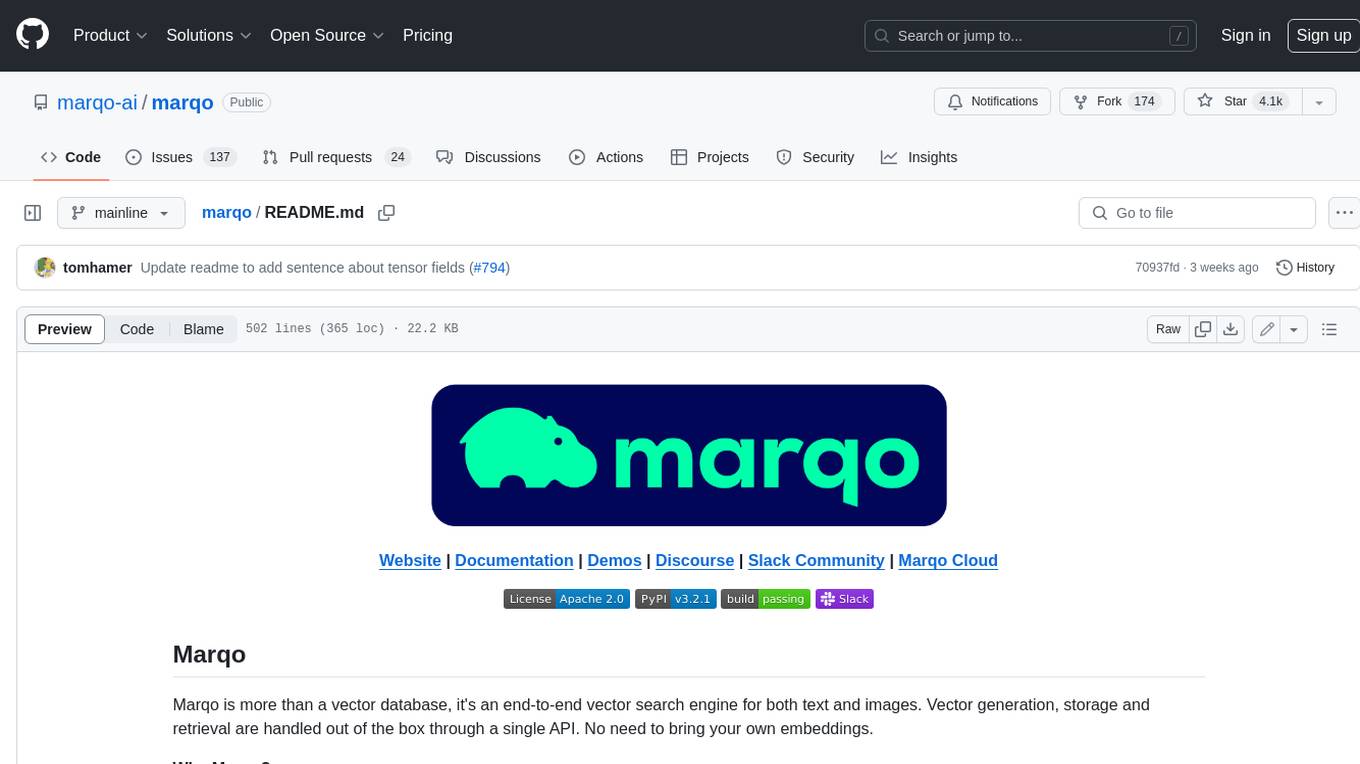
marqo
Marqo is more than a vector database, it's an end-to-end vector search engine for both text and images. Vector generation, storage and retrieval are handled out of the box through a single API. No need to bring your own embeddings.
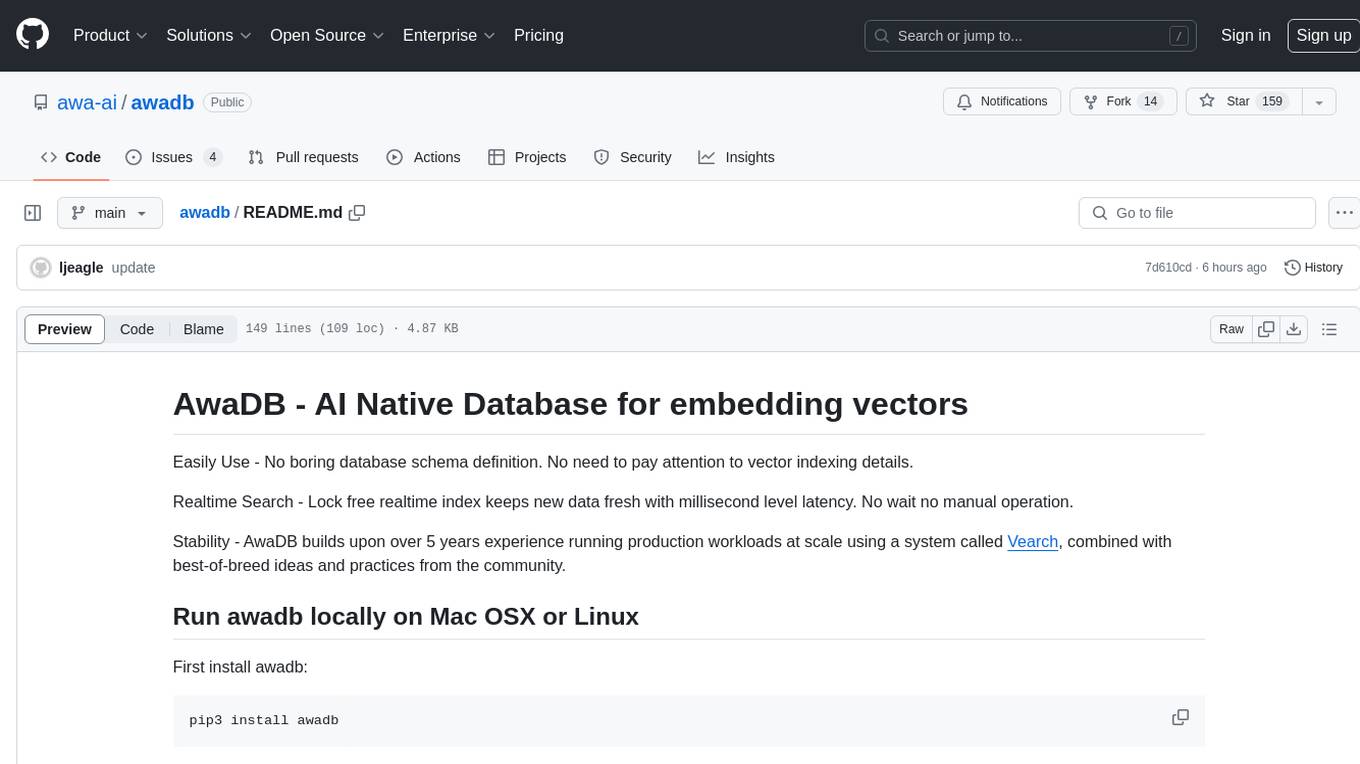
awadb
AwaDB is an AI native database designed for embedding vectors. It simplifies database usage by eliminating the need for schema definition and manual indexing. The system ensures real-time search capabilities with millisecond-level latency. Built on 5 years of production experience with Vearch, AwaDB incorporates best practices from the community to offer stability and efficiency. Users can easily add and search for embedded sentences using the provided client libraries or RESTful API.
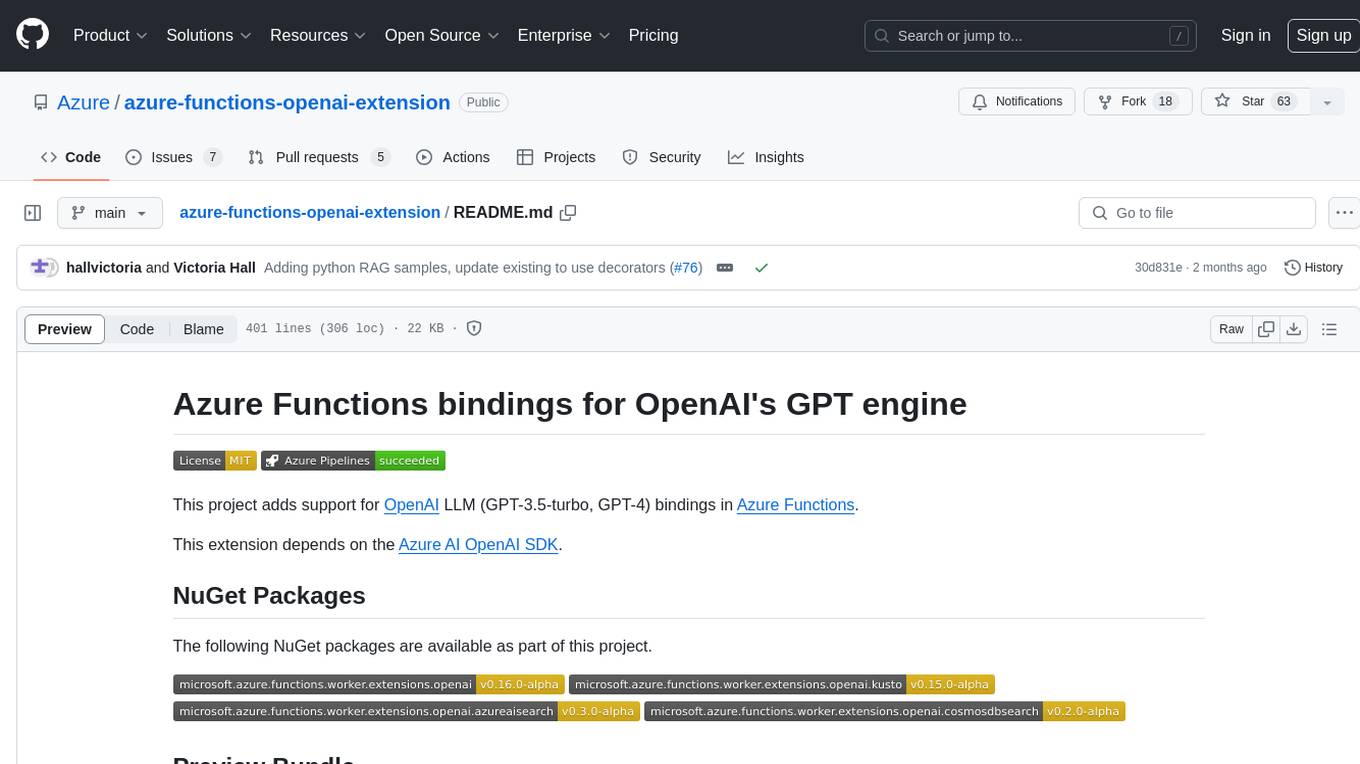
azure-functions-openai-extension
Azure Functions OpenAI Extension is a project that adds support for OpenAI LLM (GPT-3.5-turbo, GPT-4) bindings in Azure Functions. It provides NuGet packages for various functionalities like text completions, chat completions, assistants, embeddings generators, and semantic search. The project requires .NET 6 SDK or greater, Azure Functions Core Tools v4.x, and specific settings in Azure Function or local settings for development. It offers features like text completions, chat completion, assistants with custom skills, embeddings generators for text relatedness, and semantic search using vector databases. The project also includes examples in C# and Python for different functionalities.
For similar tasks
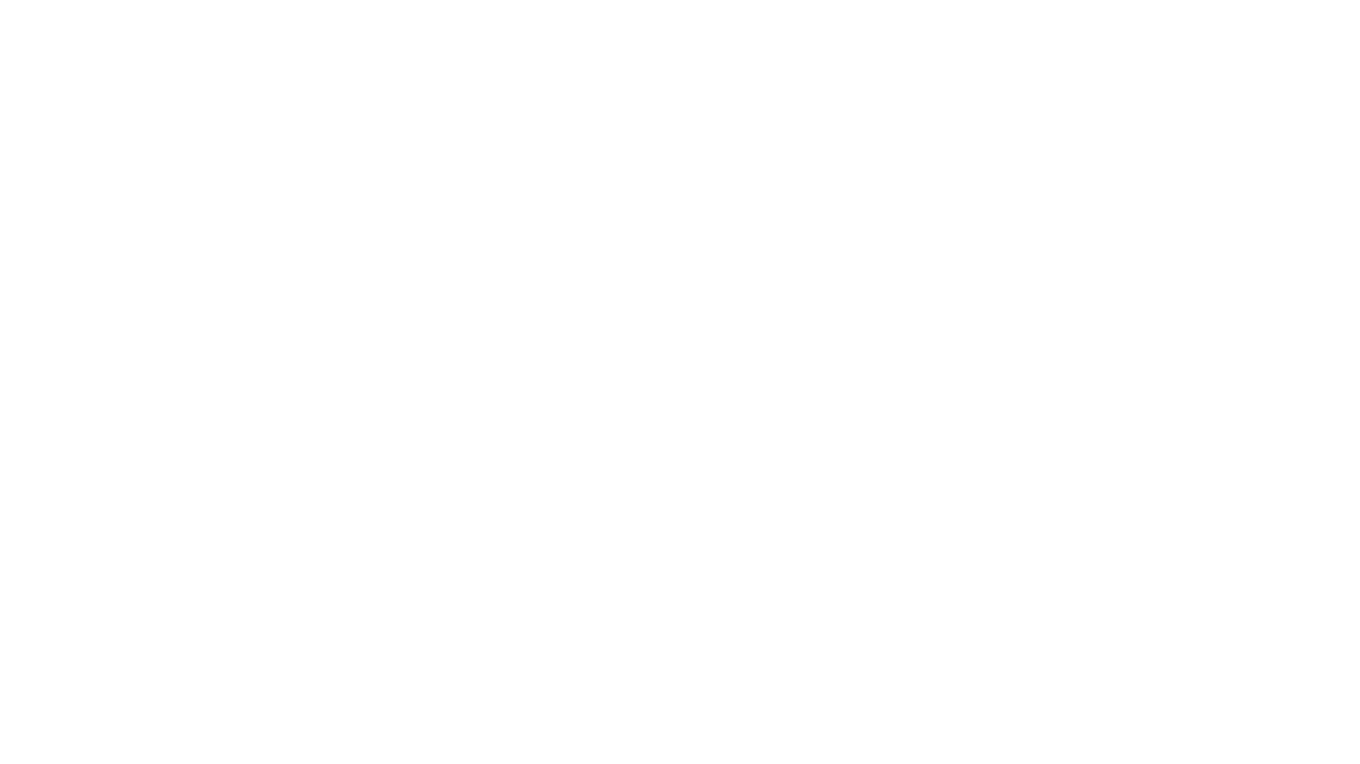
CEO-Agentic-AI-Framework
CEO-Agentic-AI-Framework is an ultra-lightweight Agentic AI framework based on the ReAct paradigm. It supports mainstream LLMs and is stronger than Swarm. The framework allows users to build their own agents, assign tasks, and interact with them through a set of predefined abilities. Users can customize agent personalities, grant and deprive abilities, and assign queries for specific tasks. CEO also supports multi-agent collaboration scenarios, where different agents with distinct capabilities can work together to achieve complex tasks. The framework provides a quick start guide, examples, and detailed documentation for seamless integration into research projects.
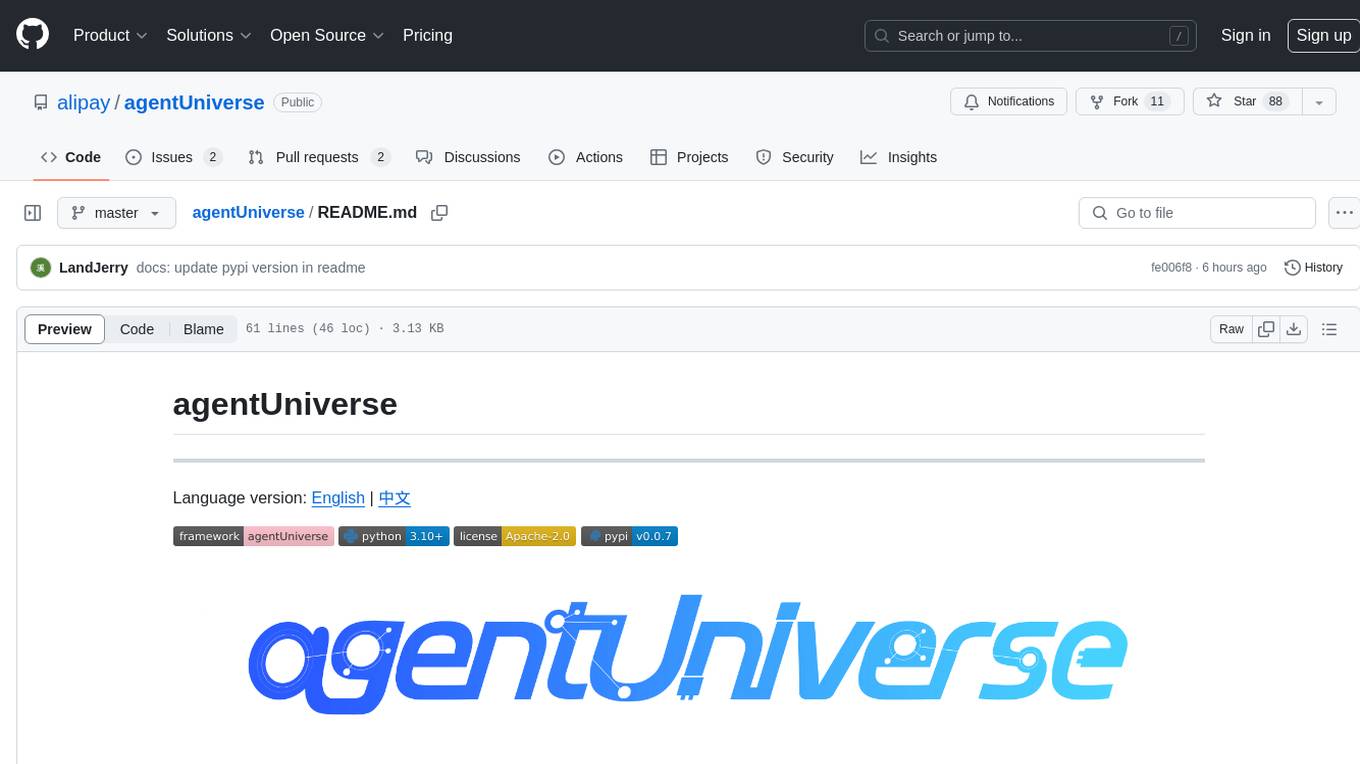
agentUniverse
agentUniverse is a framework for developing applications powered by multi-agent based on large language model. It provides essential components for building single agent and multi-agent collaboration mechanism for customizing collaboration patterns. Developers can easily construct multi-agent applications and share pattern practices from different fields. The framework includes pre-installed collaboration patterns like PEER and DOE for complex task breakdown and data-intensive tasks.
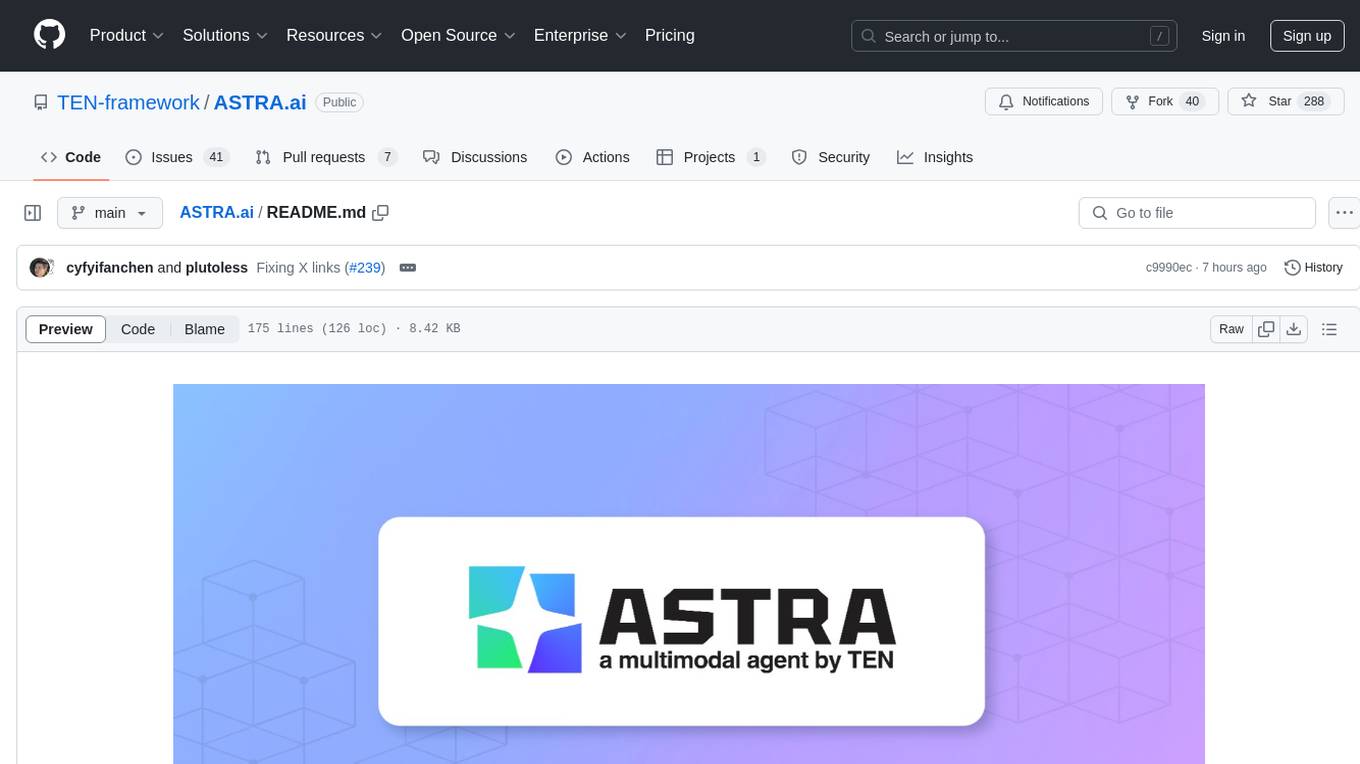
ASTRA.ai
Astra.ai is a multimodal agent powered by TEN, showcasing its capabilities in speech, vision, and reasoning through RAG from local documentation. It provides a platform for developing AI agents with features like RTC transportation, extension store, workflow builder, and local deployment. Users can build and test agents locally using Docker and Node.js, with prerequisites including Agora App ID, Azure's speech-to-text and text-to-speech API keys, and OpenAI API key. The platform offers advanced customization options through config files and API keys setup, enabling users to create and deploy their AI agents for various tasks.
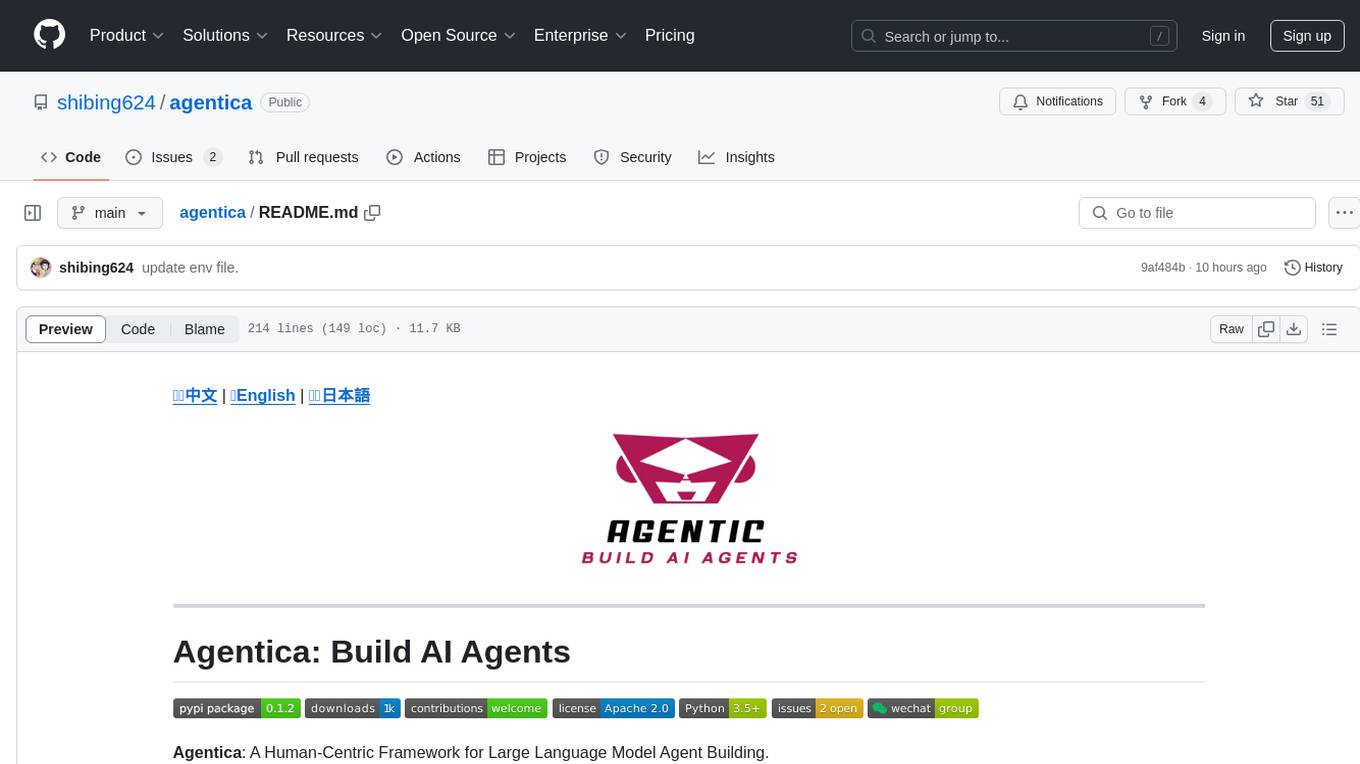
agentica
Agentica is a human-centric framework for building large language model agents. It provides functionalities for planning, memory management, tool usage, and supports features like reflection, planning and execution, RAG, multi-agent, multi-role, and workflow. The tool allows users to quickly code and orchestrate agents, customize prompts, and make API calls to various services. It supports API calls to OpenAI, Azure, Deepseek, Moonshot, Claude, Ollama, and Together. Agentica aims to simplify the process of building AI agents by providing a user-friendly interface and a range of functionalities for agent development.
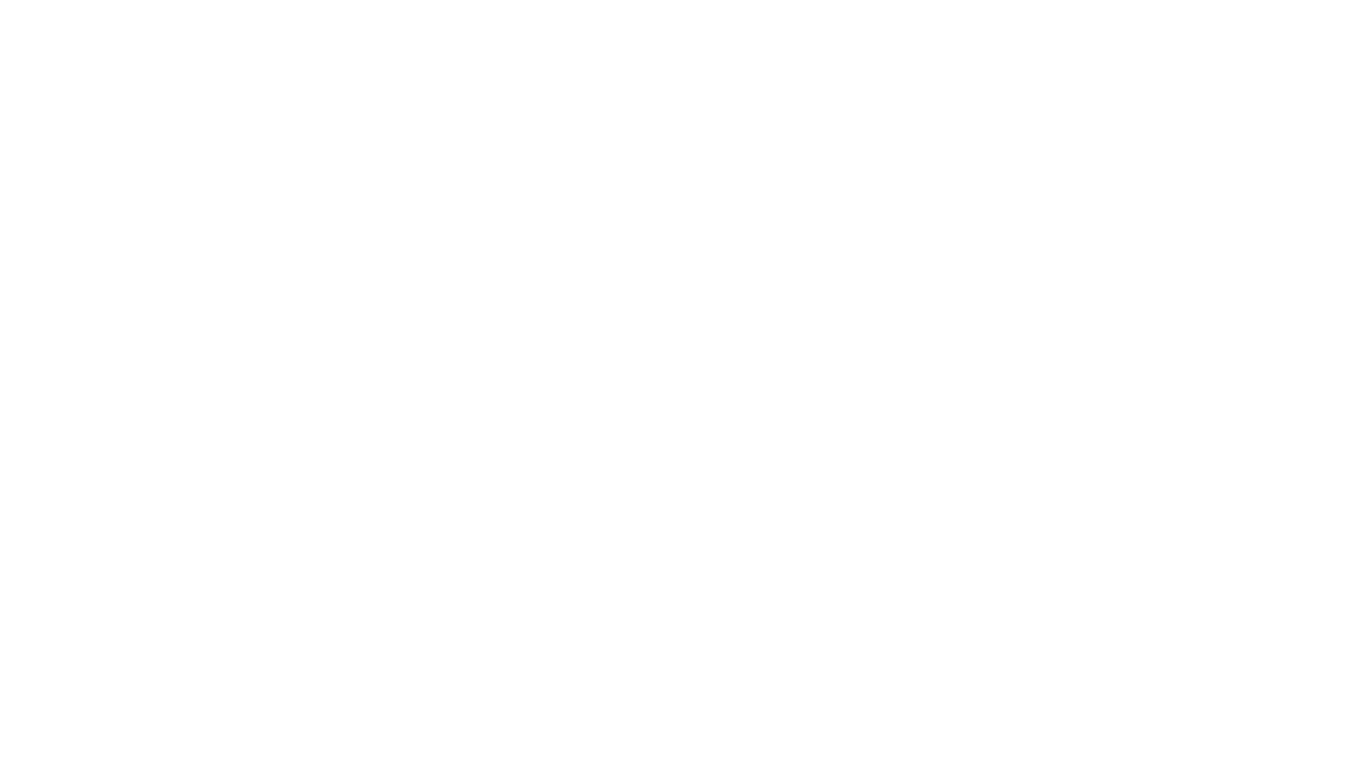
appworld
AppWorld is a high-fidelity execution environment of 9 day-to-day apps, operable via 457 APIs, populated with digital activities of ~100 people living in a simulated world. It provides a benchmark of natural, diverse, and challenging autonomous agent tasks requiring rich and interactive coding. The repository includes implementations of AppWorld apps and APIs, along with tests. It also introduces safety features for code execution and provides guides for building agents and extending the benchmark.
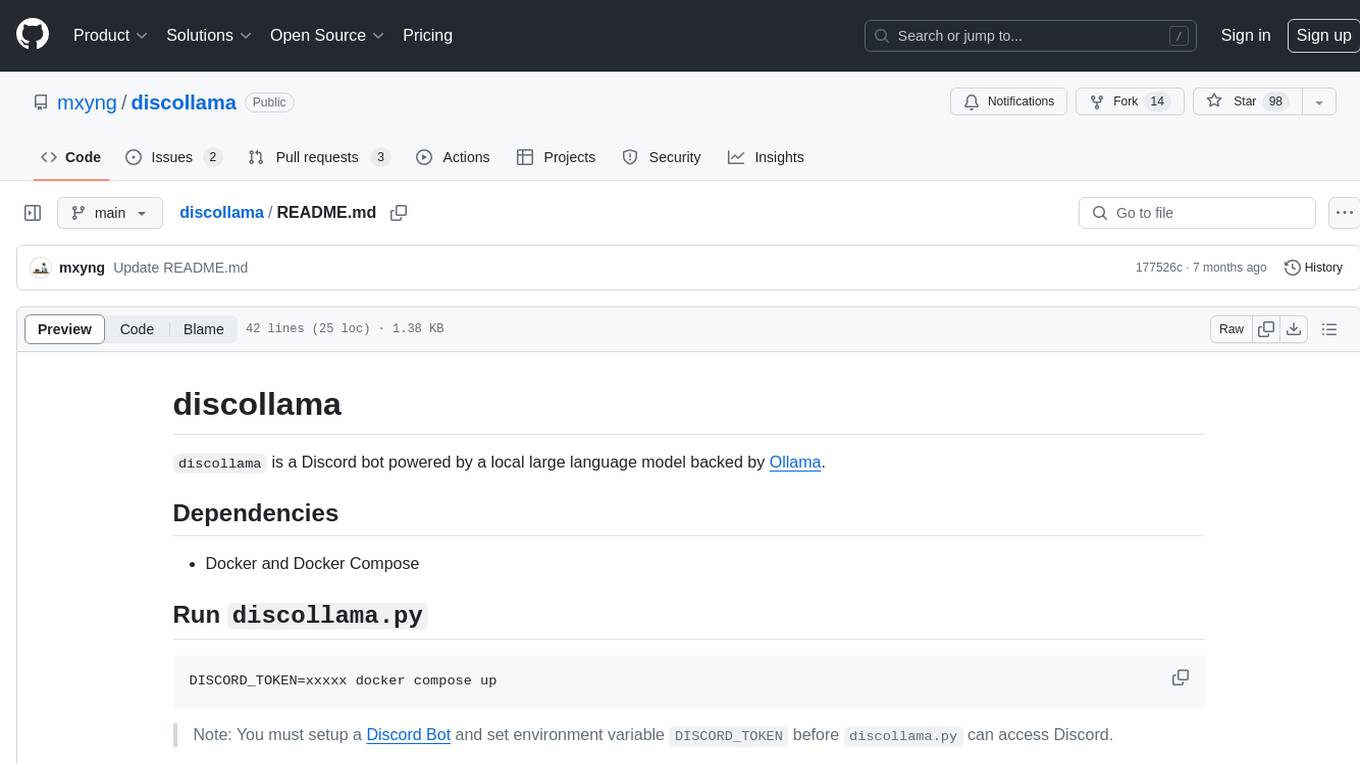
discollama
Discollama is a Discord bot powered by a local large language model backed by Ollama. It allows users to interact with the bot in Discord by mentioning it in a message to start a new conversation or in a reply to a previous response to continue an ongoing conversation. The bot requires Docker and Docker Compose to run, and users need to set up a Discord Bot and environment variable DISCORD_TOKEN before using discollama.py. Additionally, an Ollama server is needed, and users can customize the bot's personality by creating a custom model using Modelfile and running 'ollama create'.
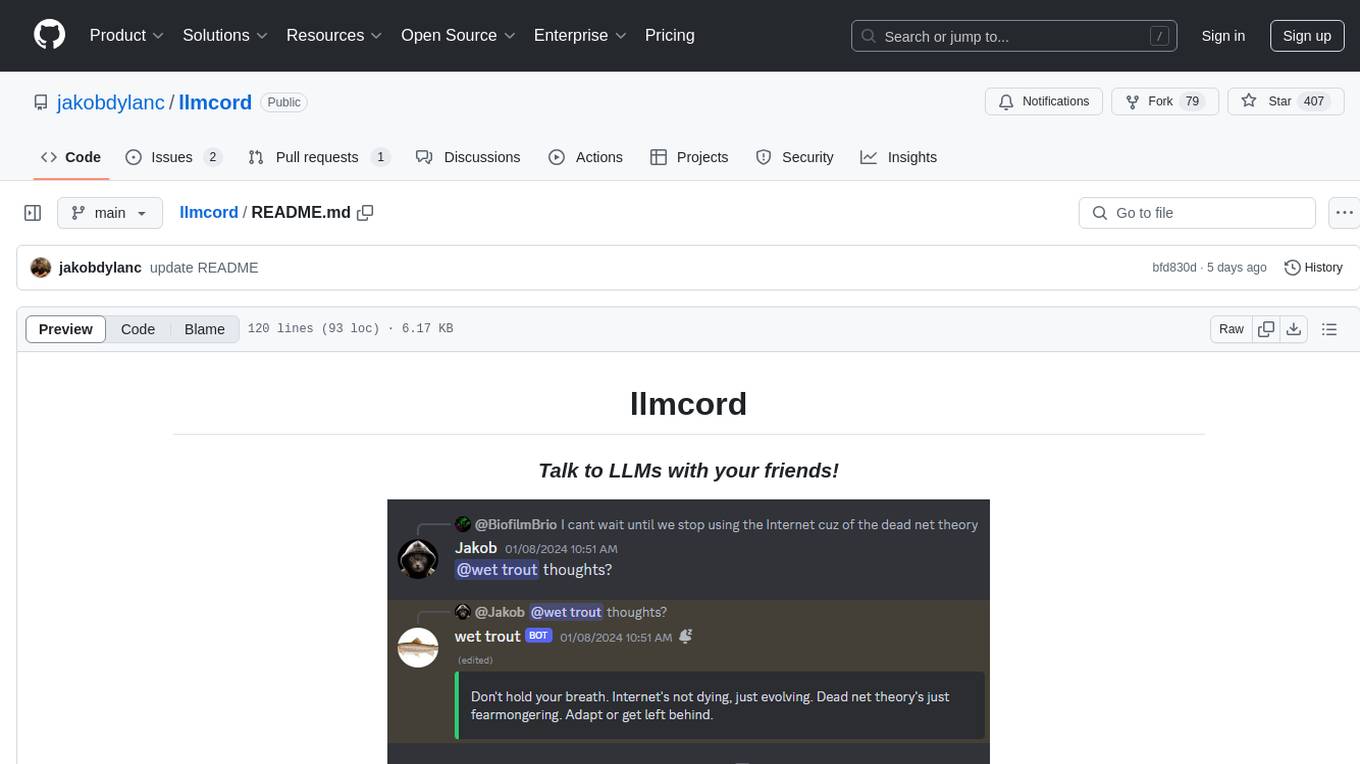
llmcord
llmcord is a Discord bot that transforms Discord into a collaborative LLM frontend, allowing users to interact with various LLM models. It features a reply-based chat system that enables branching conversations, supports remote and local LLM models, allows image and text file attachments, offers customizable personality settings, and provides streamed responses. The bot is fully asynchronous, efficient in managing message data, and offers hot reloading config. With just one Python file and around 200 lines of code, llmcord provides a seamless experience for engaging with LLMs on Discord.
For similar jobs
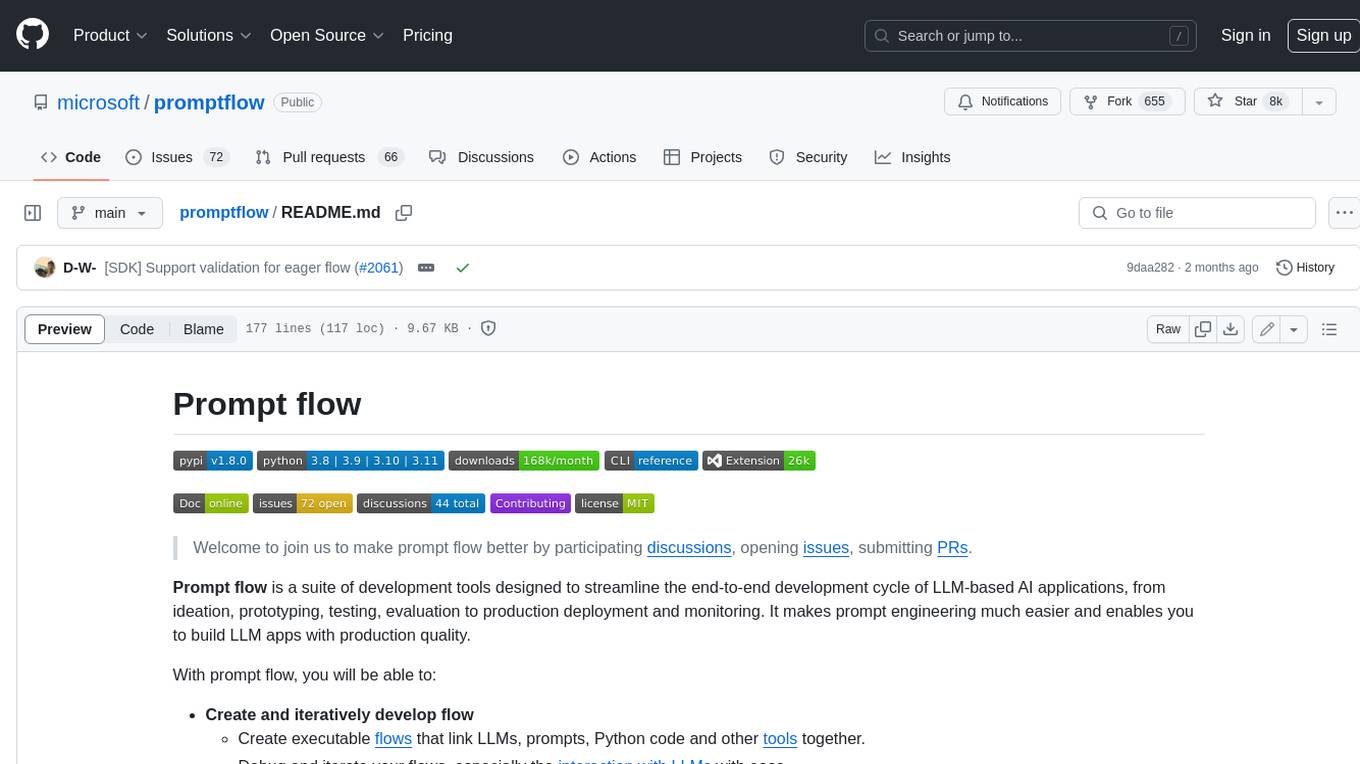
promptflow
**Prompt flow** is a suite of development tools designed to streamline the end-to-end development cycle of LLM-based AI applications, from ideation, prototyping, testing, evaluation to production deployment and monitoring. It makes prompt engineering much easier and enables you to build LLM apps with production quality.
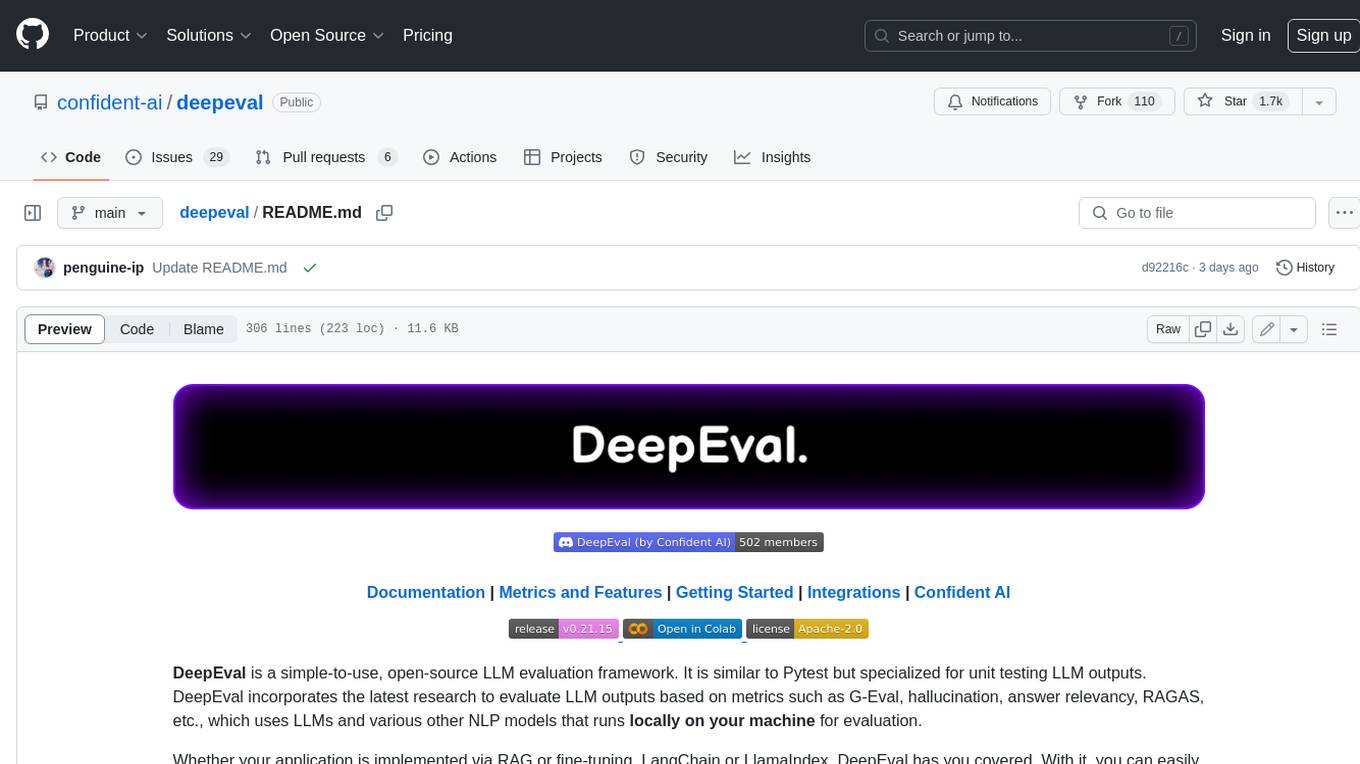
deepeval
DeepEval is a simple-to-use, open-source LLM evaluation framework specialized for unit testing LLM outputs. It incorporates various metrics such as G-Eval, hallucination, answer relevancy, RAGAS, etc., and runs locally on your machine for evaluation. It provides a wide range of ready-to-use evaluation metrics, allows for creating custom metrics, integrates with any CI/CD environment, and enables benchmarking LLMs on popular benchmarks. DeepEval is designed for evaluating RAG and fine-tuning applications, helping users optimize hyperparameters, prevent prompt drifting, and transition from OpenAI to hosting their own Llama2 with confidence.
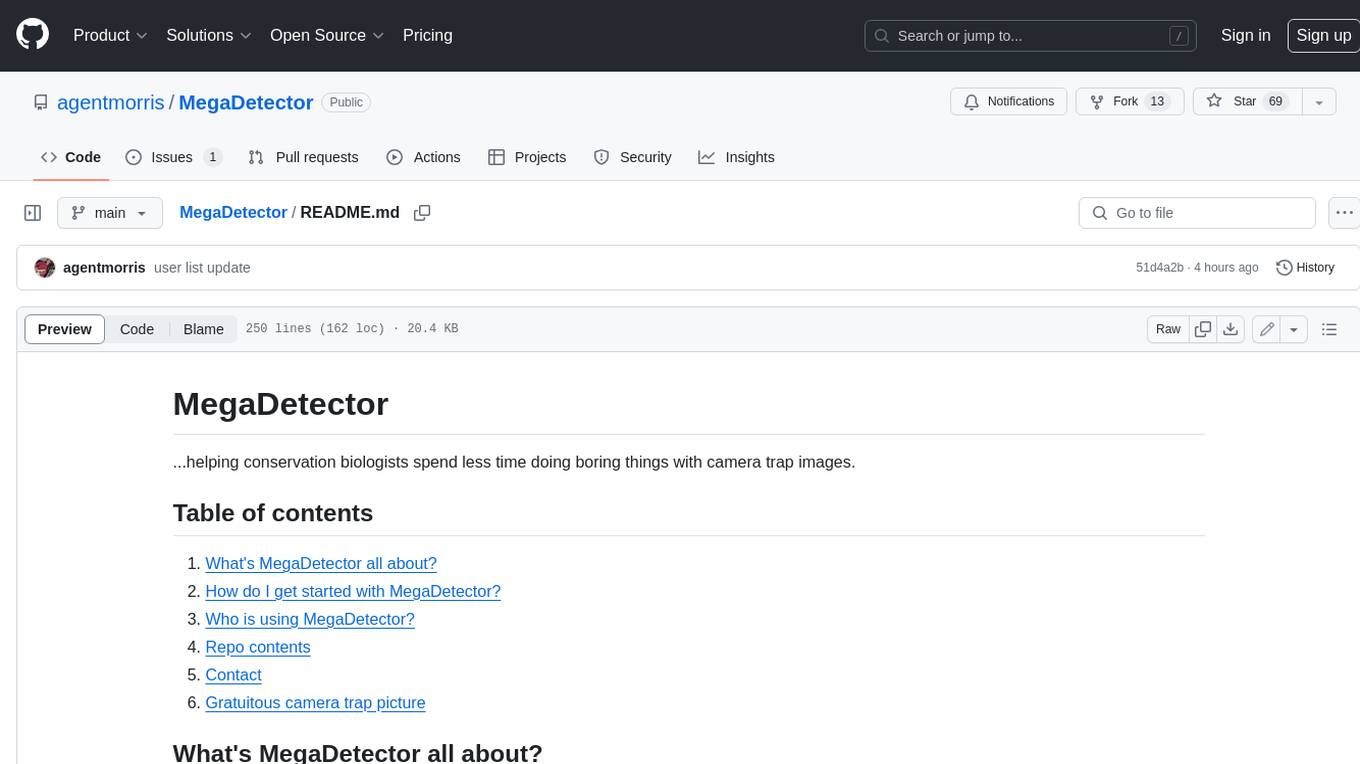
MegaDetector
MegaDetector is an AI model that identifies animals, people, and vehicles in camera trap images (which also makes it useful for eliminating blank images). This model is trained on several million images from a variety of ecosystems. MegaDetector is just one of many tools that aims to make conservation biologists more efficient with AI. If you want to learn about other ways to use AI to accelerate camera trap workflows, check out our of the field, affectionately titled "Everything I know about machine learning and camera traps".
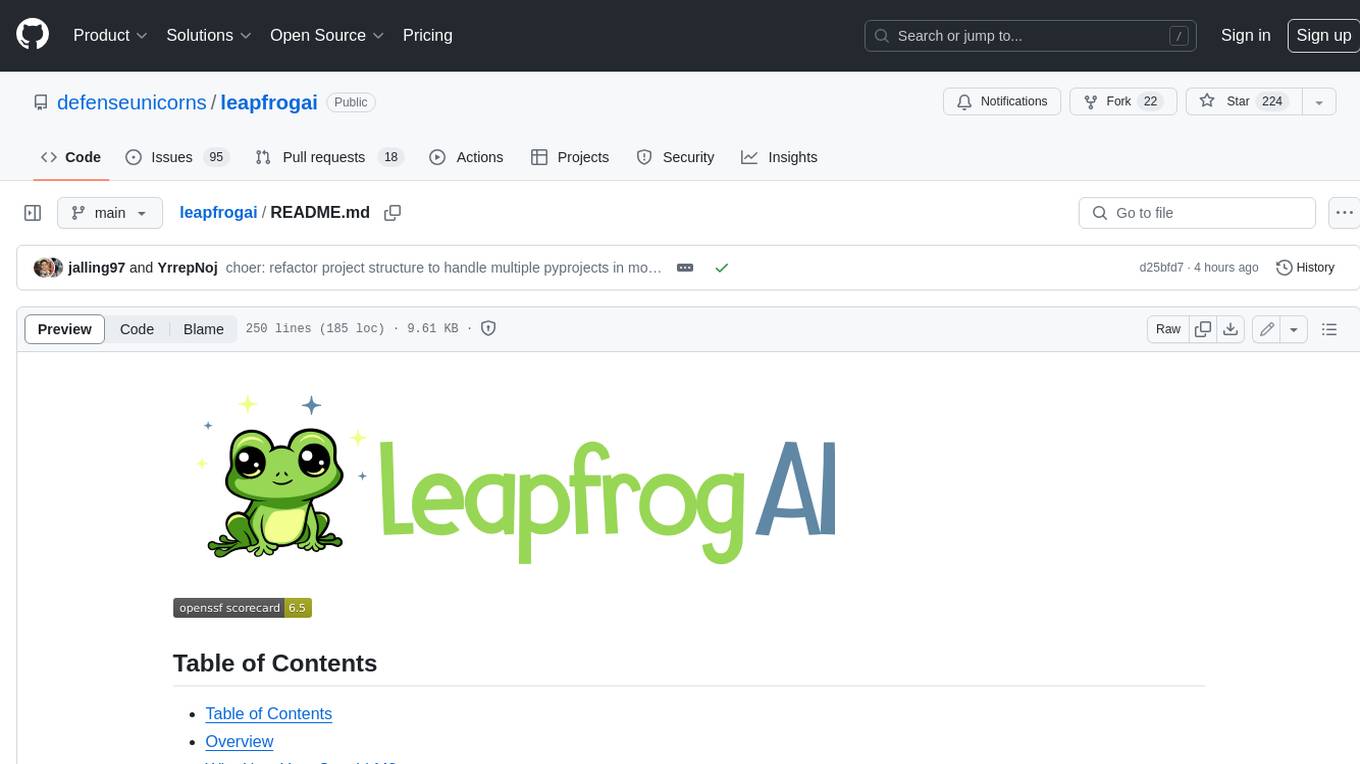
leapfrogai
LeapfrogAI is a self-hosted AI platform designed to be deployed in air-gapped resource-constrained environments. It brings sophisticated AI solutions to these environments by hosting all the necessary components of an AI stack, including vector databases, model backends, API, and UI. LeapfrogAI's API closely matches that of OpenAI, allowing tools built for OpenAI/ChatGPT to function seamlessly with a LeapfrogAI backend. It provides several backends for various use cases, including llama-cpp-python, whisper, text-embeddings, and vllm. LeapfrogAI leverages Chainguard's apko to harden base python images, ensuring the latest supported Python versions are used by the other components of the stack. The LeapfrogAI SDK provides a standard set of protobuffs and python utilities for implementing backends and gRPC. LeapfrogAI offers UI options for common use-cases like chat, summarization, and transcription. It can be deployed and run locally via UDS and Kubernetes, built out using Zarf packages. LeapfrogAI is supported by a community of users and contributors, including Defense Unicorns, Beast Code, Chainguard, Exovera, Hypergiant, Pulze, SOSi, United States Navy, United States Air Force, and United States Space Force.
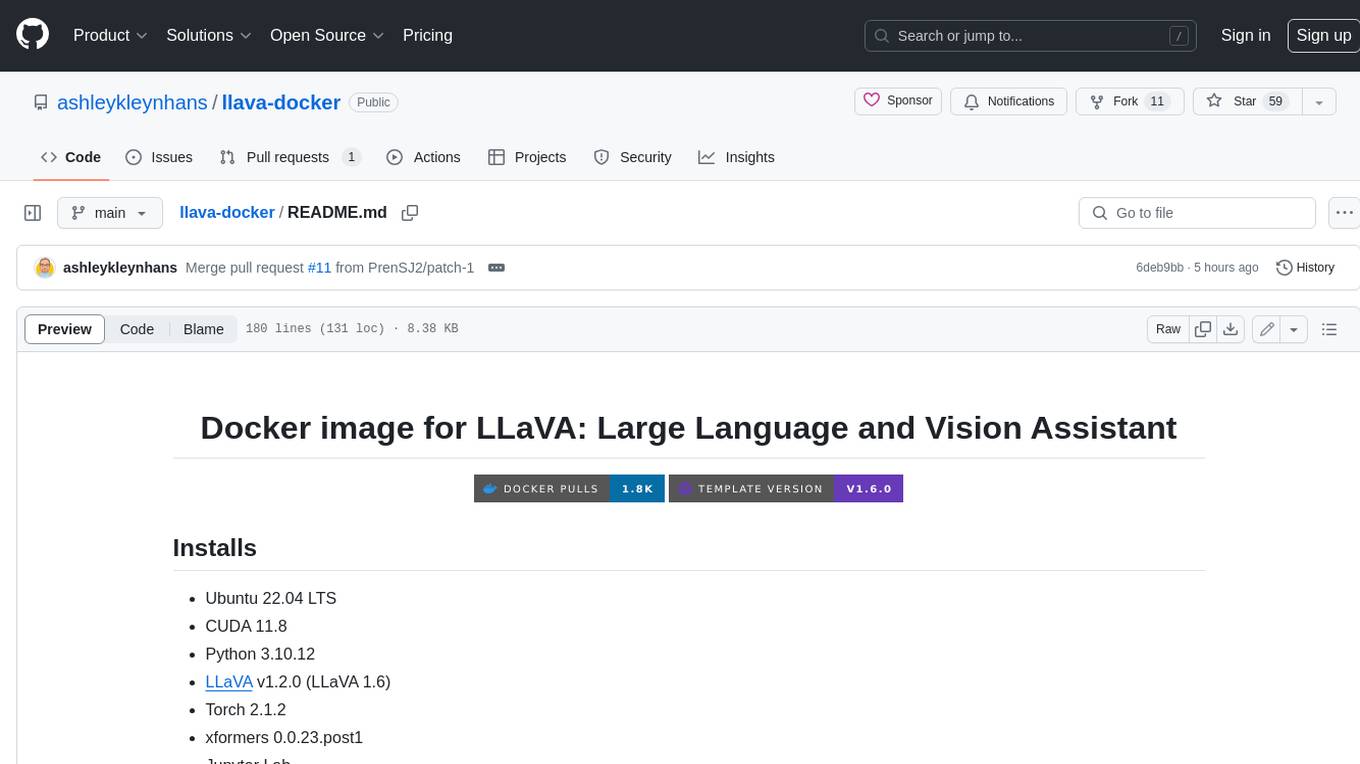
llava-docker
This Docker image for LLaVA (Large Language and Vision Assistant) provides a convenient way to run LLaVA locally or on RunPod. LLaVA is a powerful AI tool that combines natural language processing and computer vision capabilities. With this Docker image, you can easily access LLaVA's functionalities for various tasks, including image captioning, visual question answering, text summarization, and more. The image comes pre-installed with LLaVA v1.2.0, Torch 2.1.2, xformers 0.0.23.post1, and other necessary dependencies. You can customize the model used by setting the MODEL environment variable. The image also includes a Jupyter Lab environment for interactive development and exploration. Overall, this Docker image offers a comprehensive and user-friendly platform for leveraging LLaVA's capabilities.
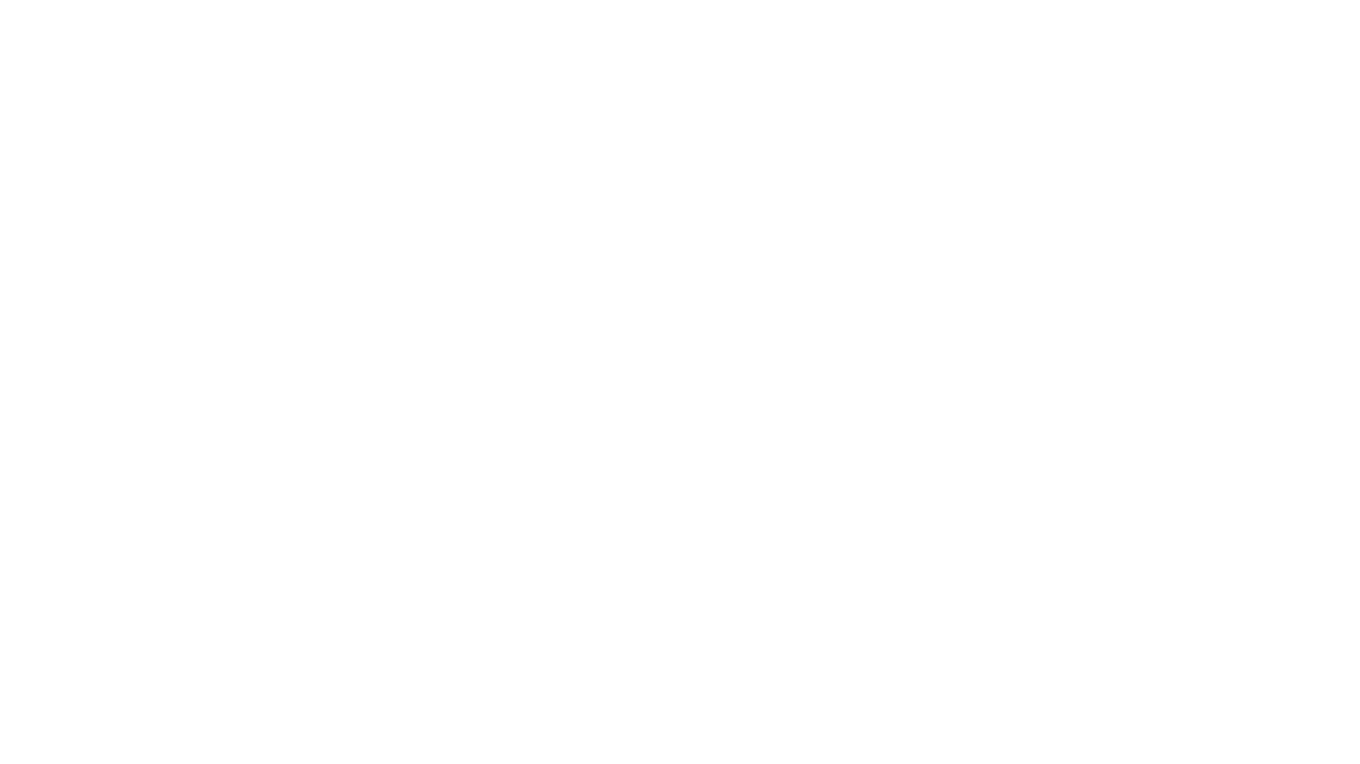
carrot
The 'carrot' repository on GitHub provides a list of free and user-friendly ChatGPT mirror sites for easy access. The repository includes sponsored sites offering various GPT models and services. Users can find and share sites, report errors, and access stable and recommended sites for ChatGPT usage. The repository also includes a detailed list of ChatGPT sites, their features, and accessibility options, making it a valuable resource for ChatGPT users seeking free and unlimited GPT services.
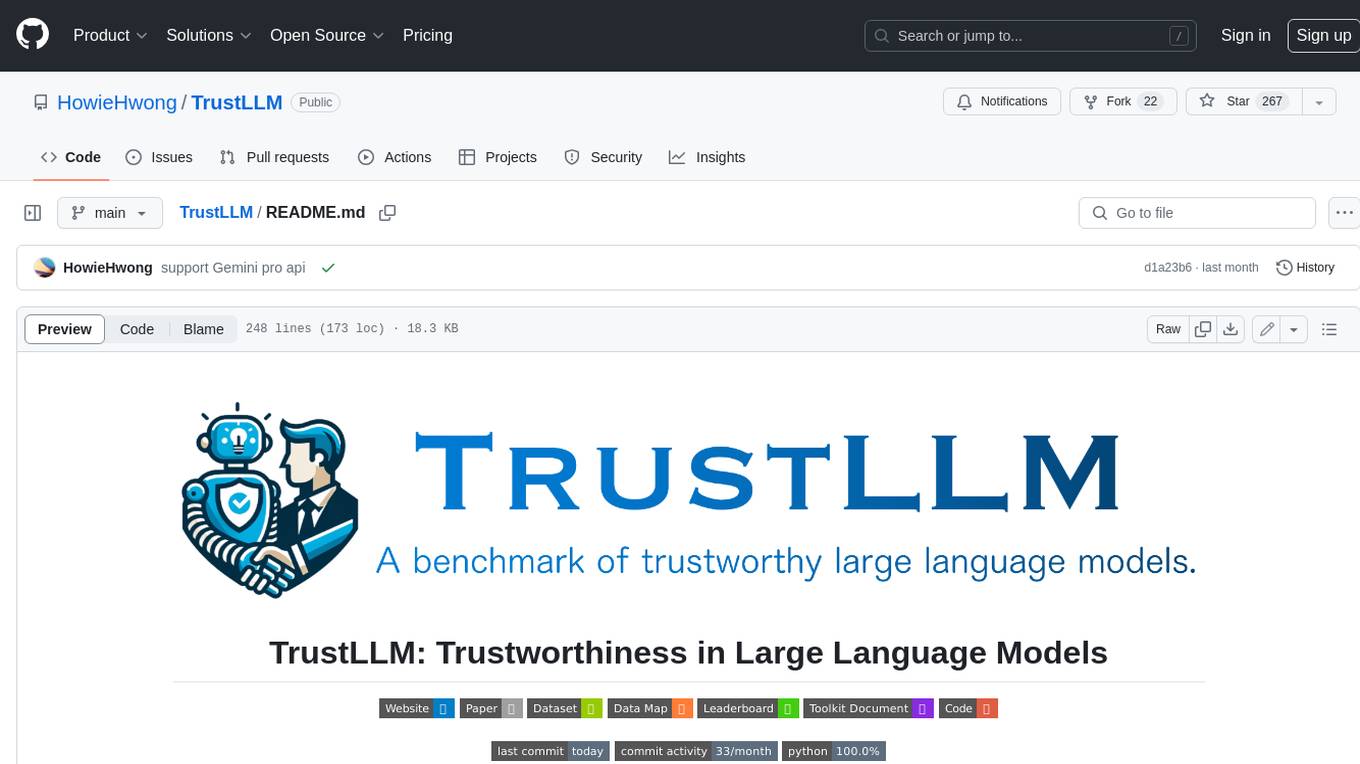
TrustLLM
TrustLLM is a comprehensive study of trustworthiness in LLMs, including principles for different dimensions of trustworthiness, established benchmark, evaluation, and analysis of trustworthiness for mainstream LLMs, and discussion of open challenges and future directions. Specifically, we first propose a set of principles for trustworthy LLMs that span eight different dimensions. Based on these principles, we further establish a benchmark across six dimensions including truthfulness, safety, fairness, robustness, privacy, and machine ethics. We then present a study evaluating 16 mainstream LLMs in TrustLLM, consisting of over 30 datasets. The document explains how to use the trustllm python package to help you assess the performance of your LLM in trustworthiness more quickly. For more details about TrustLLM, please refer to project website.
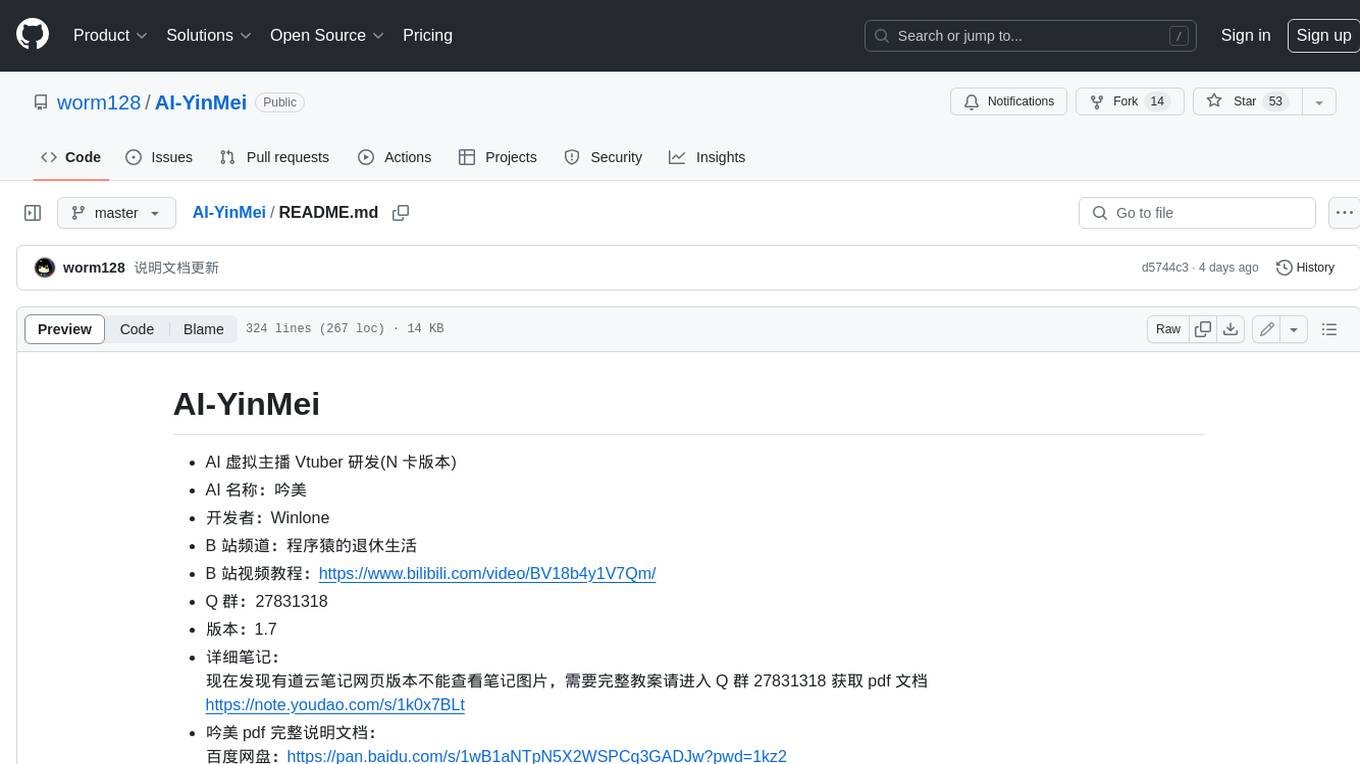
AI-YinMei
AI-YinMei is an AI virtual anchor Vtuber development tool (N card version). It supports fastgpt knowledge base chat dialogue, a complete set of solutions for LLM large language models: [fastgpt] + [one-api] + [Xinference], supports docking bilibili live broadcast barrage reply and entering live broadcast welcome speech, supports Microsoft edge-tts speech synthesis, supports Bert-VITS2 speech synthesis, supports GPT-SoVITS speech synthesis, supports expression control Vtuber Studio, supports painting stable-diffusion-webui output OBS live broadcast room, supports painting picture pornography public-NSFW-y-distinguish, supports search and image search service duckduckgo (requires magic Internet access), supports image search service Baidu image search (no magic Internet access), supports AI reply chat box [html plug-in], supports AI singing Auto-Convert-Music, supports playlist [html plug-in], supports dancing function, supports expression video playback, supports head touching action, supports gift smashing action, supports singing automatic start dancing function, chat and singing automatic cycle swing action, supports multi scene switching, background music switching, day and night automatic switching scene, supports open singing and painting, let AI automatically judge the content.