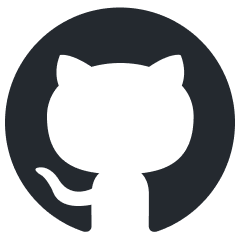
redis-vl-python
Redis Vector Library (RedisVL) interfaces with Redis' vector database for realtime semantic search, RAG, and recommendation systems.
Stars: 253
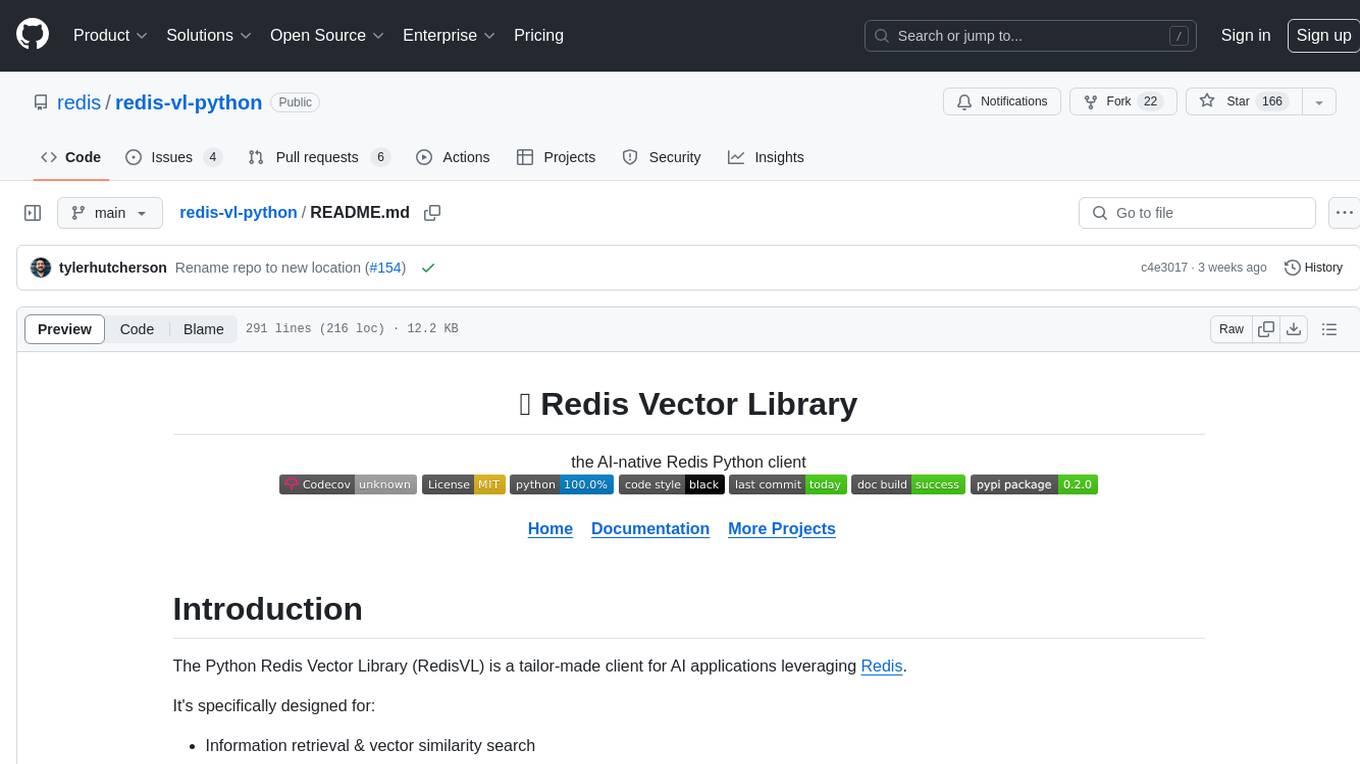
The Python Redis Vector Library (RedisVL) is a tailor-made client for AI applications leveraging Redis. It enhances applications with Redis' speed, flexibility, and reliability, incorporating capabilities like vector-based semantic search, full-text search, and geo-spatial search. The library bridges the gap between the emerging AI-native developer ecosystem and the capabilities of Redis by providing a lightweight, elegant, and intuitive interface. It abstracts the features of Redis into a grammar that is more aligned to the needs of today's AI/ML Engineers or Data Scientists.
README:
Redis Vector Library is the ultimate Python client designed for AI-native applications harnessing the power of Redis.
redisvl is your go-to client for:
- Lightning-fast information retrieval & vector similarity search
- Real-time RAG pipelines
- Agentic memory structures
- Smart recommendation engines
Install redisvl
into your Python (>=3.8) environment using pip
:
pip install redisvl
For more detailed instructions, visit the installation guide.
Choose from multiple Redis deployment options:
- Redis Cloud: Managed cloud database (free tier available)
-
Redis Stack: Docker image for development
docker run -d --name redis-stack -p 6379:6379 -p 8001:8001 redis/redis-stack:latest
- Redis Enterprise: Commercial, self-hosted database
- Azure Managed Redis: Fully managed Redis Enterprise on Azure
Enhance your experience and observability with the free Redis Insight GUI.
-
Design a schema for your use case that models your dataset with built-in Redis and indexable fields (e.g. text, tags, numerics, geo, and vectors). Load a schema from a YAML file:
index: name: user-idx prefix: user storage_type: json fields: - name: user type: tag - name: credit_score type: tag - name: embedding type: vector attrs: algorithm: flat dims: 4 distance_metric: cosine datatype: float32
from redisvl.schema import IndexSchema schema = IndexSchema.from_yaml("schemas/schema.yaml")
Or load directly from a Python dictionary:
schema = IndexSchema.from_dict({ "index": { "name": "user-idx", "prefix": "user", "storage_type": "json" }, "fields": [ {"name": "user", "type": "tag"}, {"name": "credit_score", "type": "tag"}, { "name": "embedding", "type": "vector", "attrs": { "algorithm": "flat", "datatype": "float32", "dims": 4, "distance_metric": "cosine" } } ] })
-
Create a SearchIndex class with an input schema and client connection in order to perform admin and search operations on your index in Redis:
from redis import Redis from redisvl.index import SearchIndex # Establish Redis connection and define index client = Redis.from_url("redis://localhost:6379") index = SearchIndex(schema, client) # Create the index in Redis index.create()
Async compliant search index class also available: AsyncSearchIndex.
-
Load and fetch data to/from your Redis instance:
data = {"user": "john", "credit_score": "high", "embedding": [0.23, 0.49, -0.18, 0.95]} # load list of dictionaries, specify the "id" field index.load([data], id_field="user") # fetch by "id" john = index.fetch("john")
Define queries and perform advanced searches over your indices, including the combination of vectors, metadata filters, and more.
-
VectorQuery - Flexible vector queries with customizable filters enabling semantic search:
from redisvl.query import VectorQuery query = VectorQuery( vector=[0.16, -0.34, 0.98, 0.23], vector_field_name="embedding", num_results=3 ) # run the vector search query against the embedding field results = index.query(query)
Incorporate complex metadata filters on your queries:
from redisvl.query.filter import Tag # define a tag match filter tag_filter = Tag("user") == "john" # update query definition query.set_filter(tag_filter) # execute query results = index.query(query)
-
RangeQuery - Vector search within a defined range paired with customizable filters
-
FilterQuery - Standard search using filters and the full-text search
-
CountQuery - Count the number of indexed records given attributes
Read more about building advanced Redis queries.
Integrate with popular embedding providers to greatly simplify the process of vectorizing unstructured data for your index and queries:
from redisvl.utils.vectorize import CohereTextVectorizer
# set COHERE_API_KEY in your environment
co = CohereTextVectorizer()
embedding = co.embed(
text="What is the capital city of France?",
input_type="search_query"
)
embeddings = co.embed_many(
texts=["my document chunk content", "my other document chunk content"],
input_type="search_document"
)
Learn more about using vectorizers in your embedding workflows.
Integrate with popular reranking providers to improve the relevancy of the initial search results from Redis
We're excited to announce the support for RedisVL Extensions. These modules implement interfaces exposing best practices and design patterns for working with LLM memory and agents. We've taken the best from what we've learned from our users (that's you) as well as bleeding-edge customers, and packaged it up.
Have an idea for another extension? Open a PR or reach out to us at [email protected]. We're always open to feedback.
Increase application throughput and reduce the cost of using LLM models in production by leveraging previously generated knowledge with the SemanticCache
.
from redisvl.extensions.llmcache import SemanticCache
# init cache with TTL and semantic distance threshold
llmcache = SemanticCache(
name="llmcache",
ttl=360,
redis_url="redis://localhost:6379",
distance_threshold=0.1
)
# store user queries and LLM responses in the semantic cache
llmcache.store(
prompt="What is the capital city of France?",
response="Paris"
)
# quickly check the cache with a slightly different prompt (before invoking an LLM)
response = llmcache.check(prompt="What is France's capital city?")
print(response[0]["response"])
>>> Paris
Learn more about semantic caching for LLMs.
Improve personalization and accuracy of LLM responses by providing user chat history as context. Manage access to the session data using recency or relevancy, powered by vector search with the SemanticSessionManager
.
from redisvl.extensions.session_manager import SemanticSessionManager
session = SemanticSessionManager(
name="my-session",
redis_url="redis://localhost:6379",
distance_threshold=0.7
)
session.add_messages([
{"role": "user", "content": "hello, how are you?"},
{"role": "assistant", "content": "I'm doing fine, thanks."},
{"role": "user", "content": "what is the weather going to be today?"},
{"role": "assistant", "content": "I don't know"}
])
Get recent chat history:
session.get_recent(top_k=1)
>>> [{"role": "assistant", "content": "I don't know"}]
Get relevant chat history (powered by vector search):
session.get_relevant("weather", top_k=1)
>>> [{"role": "user", "content": "what is the weather going to be today?"}]
Learn more about LLM session management.
Build fast decision models that run directly in Redis and route user queries to the nearest "route" or "topic".
from redisvl.extensions.router import Route, SemanticRouter
routes = [
Route(
name="greeting",
references=["hello", "hi"],
metadata={"type": "greeting"},
distance_threshold=0.3,
),
Route(
name="farewell",
references=["bye", "goodbye"],
metadata={"type": "farewell"},
distance_threshold=0.3,
),
]
# build semantic router from routes
router = SemanticRouter(
name="topic-router",
routes=routes,
redis_url="redis://localhost:6379",
)
router("Hi, good morning")
>>> RouteMatch(name='greeting', distance=0.273891836405)
Learn more about semantic routing.
Create, destroy, and manage Redis index configurations from a purpose-built CLI interface: rvl
.
$ rvl -h
usage: rvl <command> [<args>]
Commands:
index Index manipulation (create, delete, etc.)
version Obtain the version of RedisVL
stats Obtain statistics about an index
Read more about using the CLI.
In the age of GenAI, vector databases and LLMs are transforming information retrieval systems. With emerging and popular frameworks like LangChain and LlamaIndex, innovation is rapid. Yet, many organizations face the challenge of delivering AI solutions quickly and at scale.
Enter Redis – a cornerstone of the NoSQL world, renowned for its versatile data structures and processing engines. Redis excels in real-time workloads like caching, session management, and search. It's also a powerhouse as a vector database for RAG, an LLM cache, and a chat session memory store for conversational AI.
The Redis Vector Library bridges the gap between the AI-native developer ecosystem and Redis's robust capabilities. With a lightweight, elegant, and intuitive interface, RedisVL makes it easy to leverage Redis's power. Built on the Redis Python client, redisvl
transforms Redis's features into a grammar perfectly aligned with the needs of today's AI/ML Engineers and Data Scientists.
For additional help, check out the following resources:
Please help us by contributing PRs, opening GitHub issues for bugs or new feature ideas, improving documentation, or increasing test coverage. Read more about how to contribute!
This project is supported by Redis, Inc on a good faith effort basis. To report bugs, request features, or receive assistance, please file an issue.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for redis-vl-python
Similar Open Source Tools
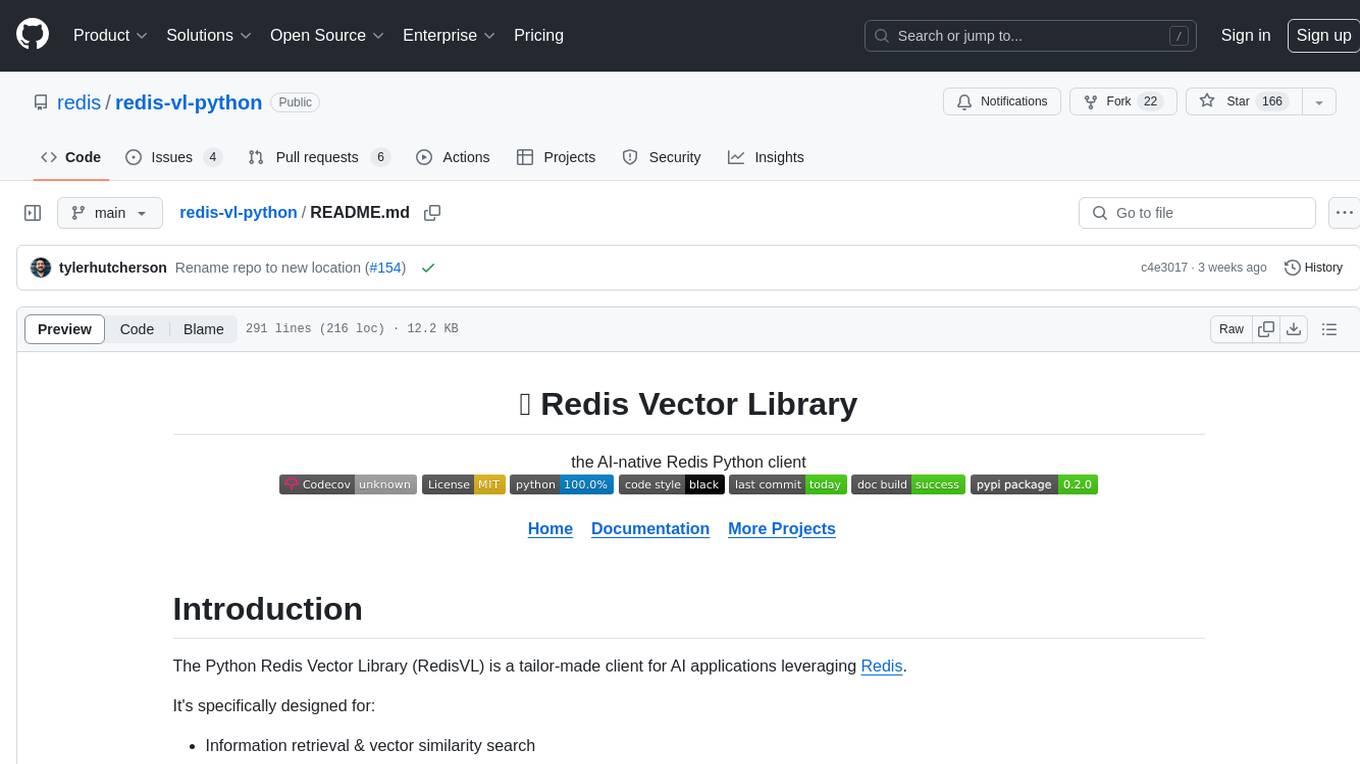
redis-vl-python
The Python Redis Vector Library (RedisVL) is a tailor-made client for AI applications leveraging Redis. It enhances applications with Redis' speed, flexibility, and reliability, incorporating capabilities like vector-based semantic search, full-text search, and geo-spatial search. The library bridges the gap between the emerging AI-native developer ecosystem and the capabilities of Redis by providing a lightweight, elegant, and intuitive interface. It abstracts the features of Redis into a grammar that is more aligned to the needs of today's AI/ML Engineers or Data Scientists.
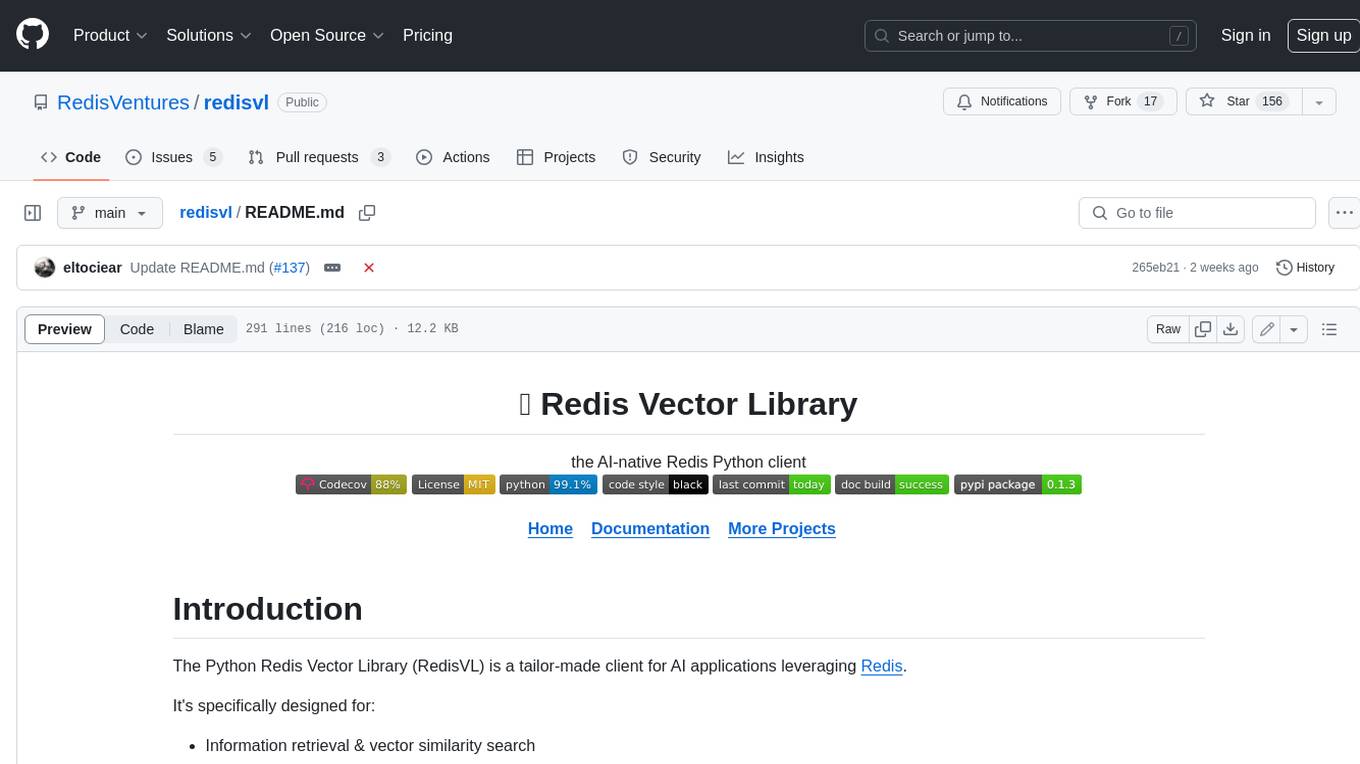
redisvl
Redis Vector Library (RedisVL) is a Python client library for building AI applications on top of Redis. It provides a high-level interface for managing vector indexes, performing vector search, and integrating with popular embedding models and providers. RedisVL is designed to make it easy for developers to build and deploy AI applications that leverage the speed, flexibility, and reliability of Redis.
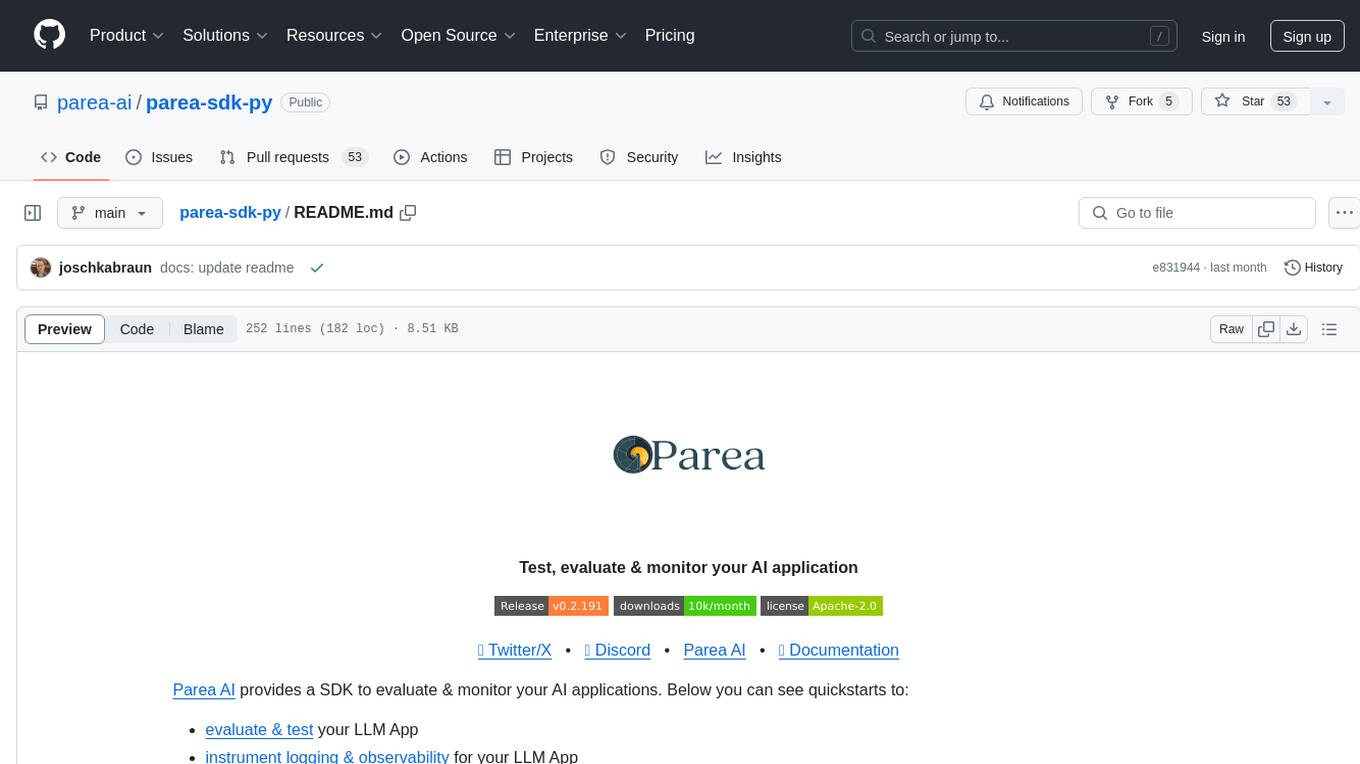
parea-sdk-py
Parea AI provides a SDK to evaluate & monitor AI applications. It allows users to test, evaluate, and monitor their AI models by defining and running experiments. The SDK also enables logging and observability for AI applications, as well as deploying prompts to facilitate collaboration between engineers and subject-matter experts. Users can automatically log calls to OpenAI and Anthropic, create hierarchical traces of their applications, and deploy prompts for integration into their applications.
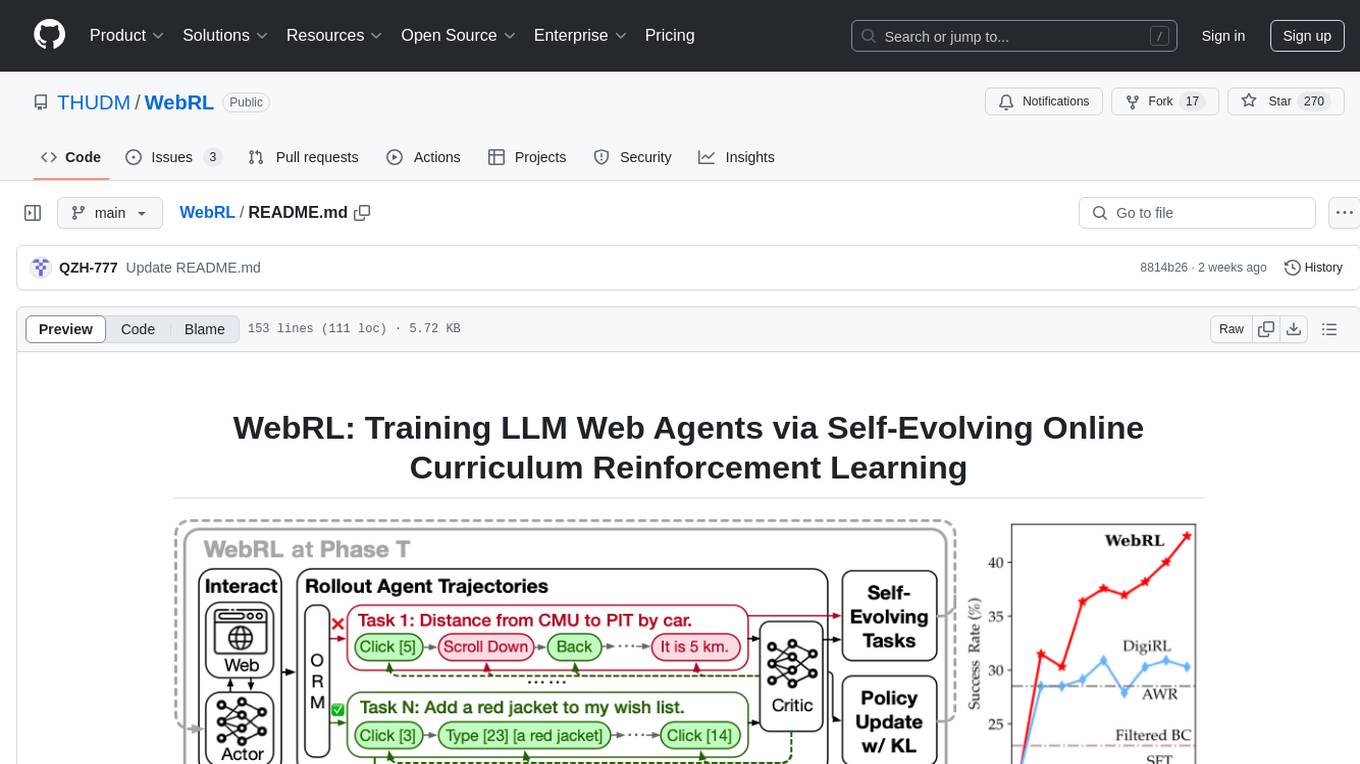
WebRL
WebRL is a self-evolving online curriculum learning framework designed for training web agents in the WebArena environment. It provides model checkpoints, training instructions, and evaluation processes for training the actor and critic models. The tool enables users to generate new instructions and interact with WebArena to configure tasks for training and evaluation.
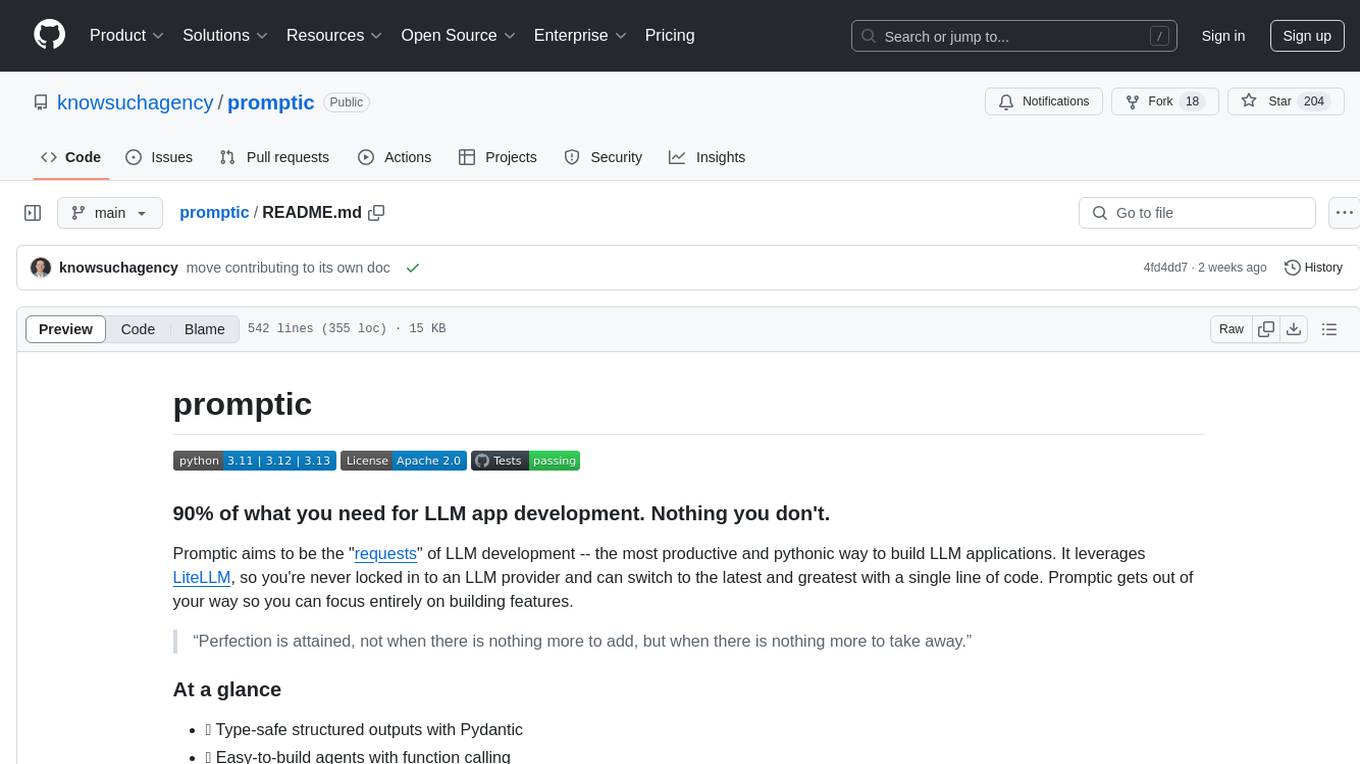
promptic
Promptic is a tool designed for LLM app development, providing a productive and pythonic way to build LLM applications. It leverages LiteLLM, allowing flexibility to switch LLM providers easily. Promptic focuses on building features by providing type-safe structured outputs, easy-to-build agents, streaming support, automatic prompt caching, and built-in conversation memory.
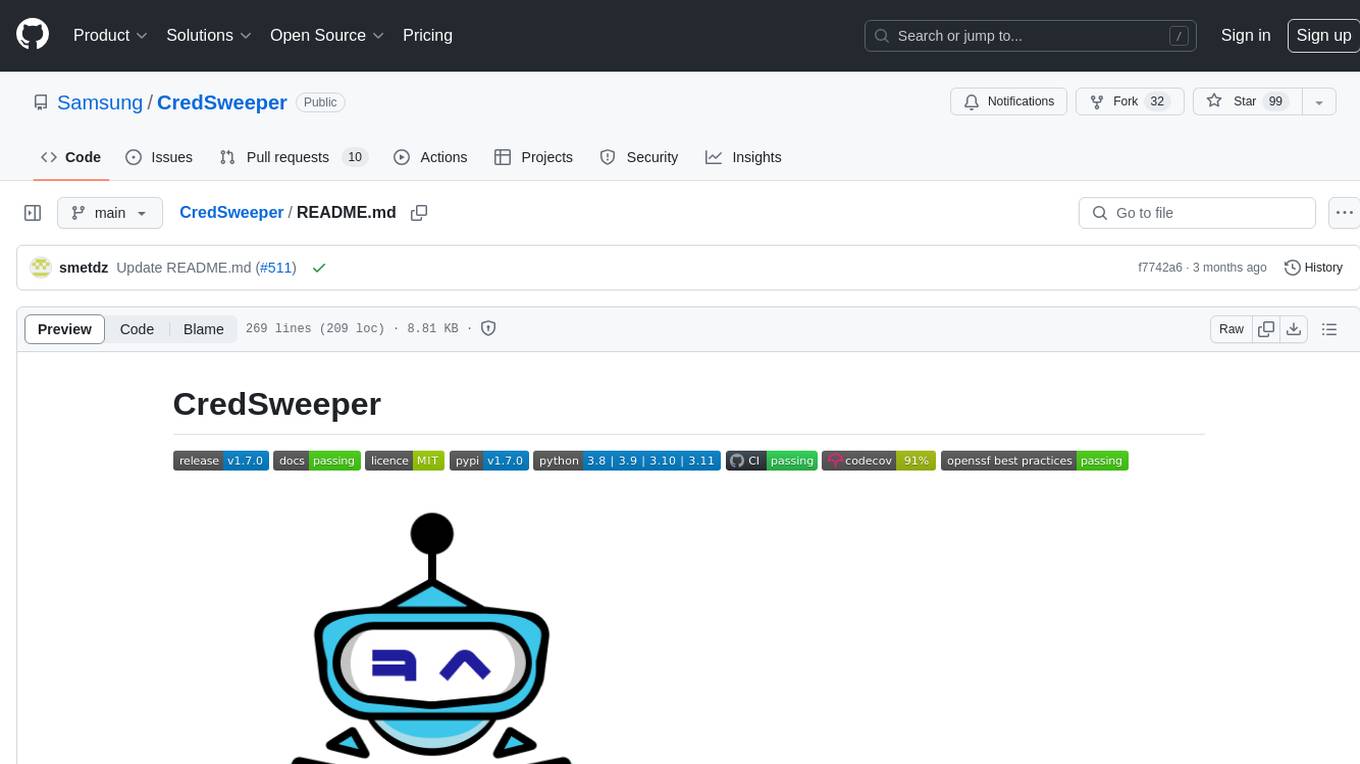
CredSweeper
CredSweeper is a tool designed to detect credentials like tokens, passwords, and API keys in directories or files. It helps users identify potential exposure of sensitive information by scanning lines, filtering, and utilizing an AI model. The tool reports lines containing possible credentials, their location, and the expected type of credential.
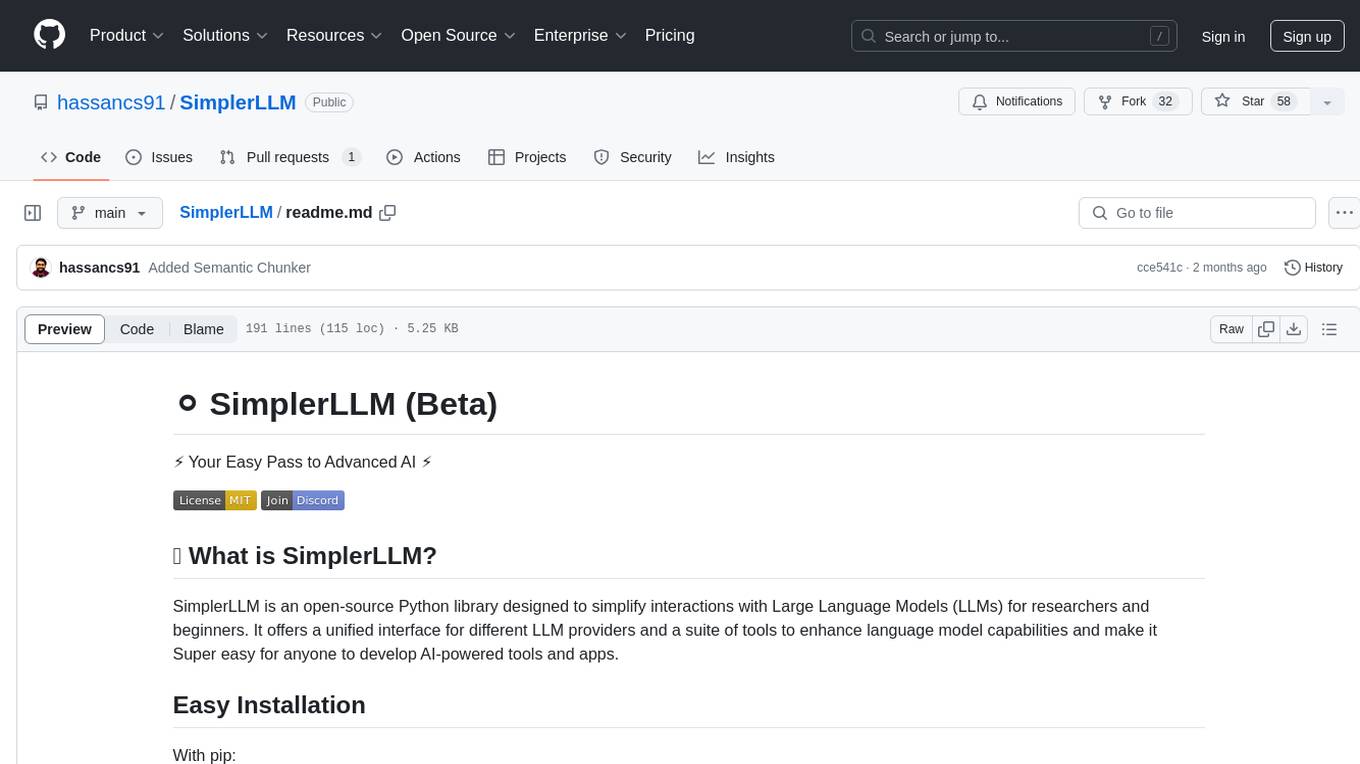
SimplerLLM
SimplerLLM is an open-source Python library that simplifies interactions with Large Language Models (LLMs) for researchers and beginners. It provides a unified interface for different LLM providers, tools for enhancing language model capabilities, and easy development of AI-powered tools and apps. The library offers features like unified LLM interface, generic text loader, RapidAPI connector, SERP integration, prompt template builder, and more. Users can easily set up environment variables, create LLM instances, use tools like SERP, generic text loader, calling RapidAPI APIs, and prompt template builder. Additionally, the library includes chunking functions to split texts into manageable chunks based on different criteria. Future updates will bring more tools, interactions with local LLMs, prompt optimization, response evaluation, GPT Trainer, document chunker, advanced document loader, integration with more providers, Simple RAG with SimplerVectors, integration with vector databases, agent builder, and LLM server.
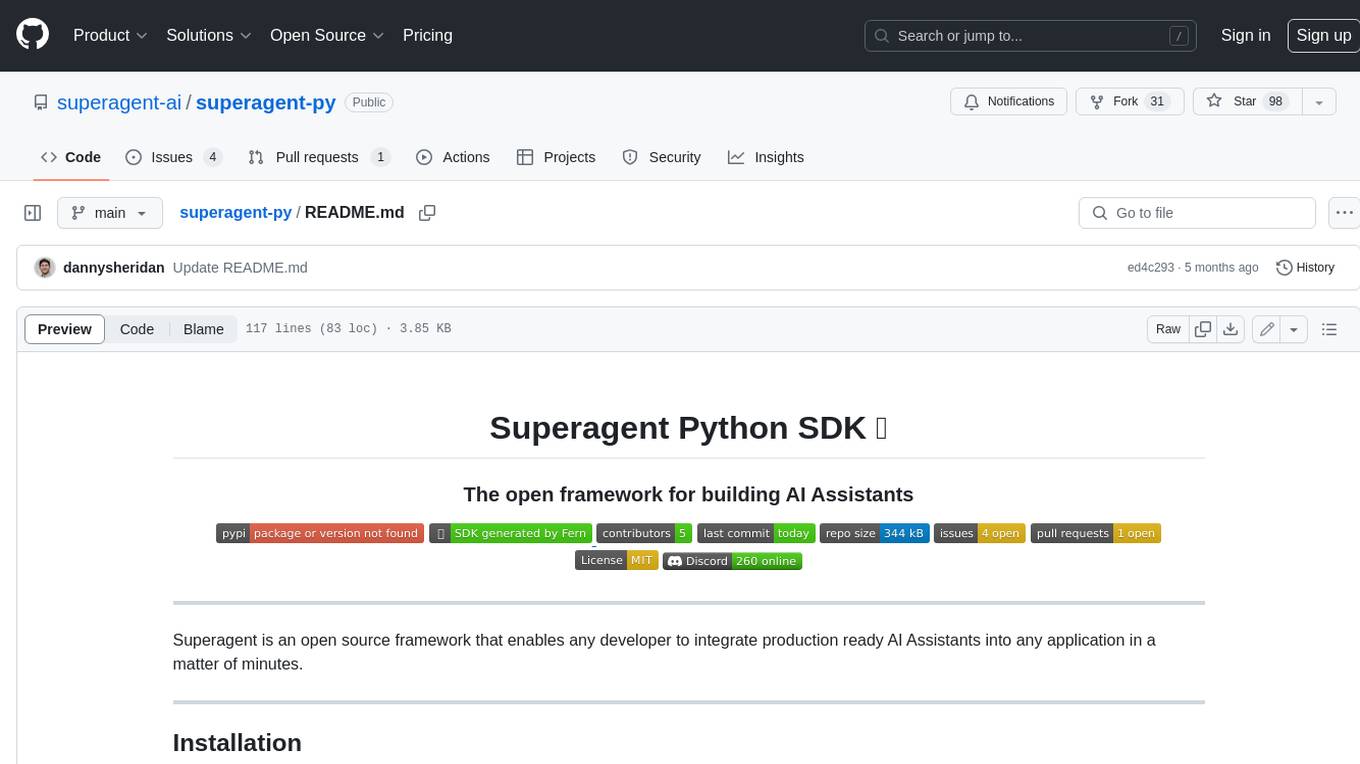
superagent-py
Superagent is an open-source framework that enables developers to integrate production-ready AI assistants into any application quickly and easily. It provides a Python SDK for interacting with the Superagent API, allowing developers to create, manage, and invoke AI agents. The SDK simplifies the process of building AI-powered applications, making it accessible to developers of all skill levels.
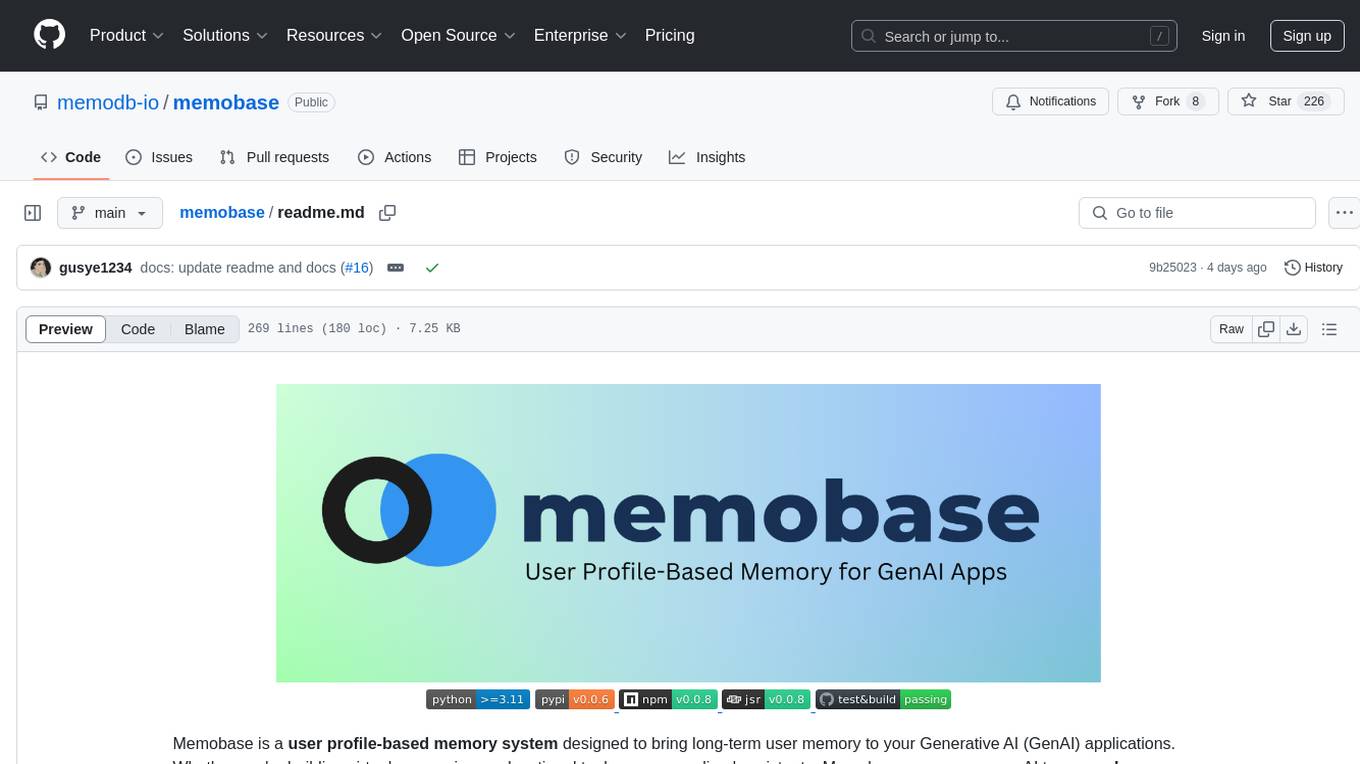
memobase
Memobase is a user profile-based memory system designed to enhance Generative AI applications by enabling them to remember, understand, and evolve with users. It provides structured user profiles, scalable profiling, easy integration with existing LLM stacks, batch processing for speed, and is production-ready. Users can manage users, insert data, get memory profiles, and track user preferences and behaviors. Memobase is ideal for applications that require user analysis, tracking, and personalized interactions.
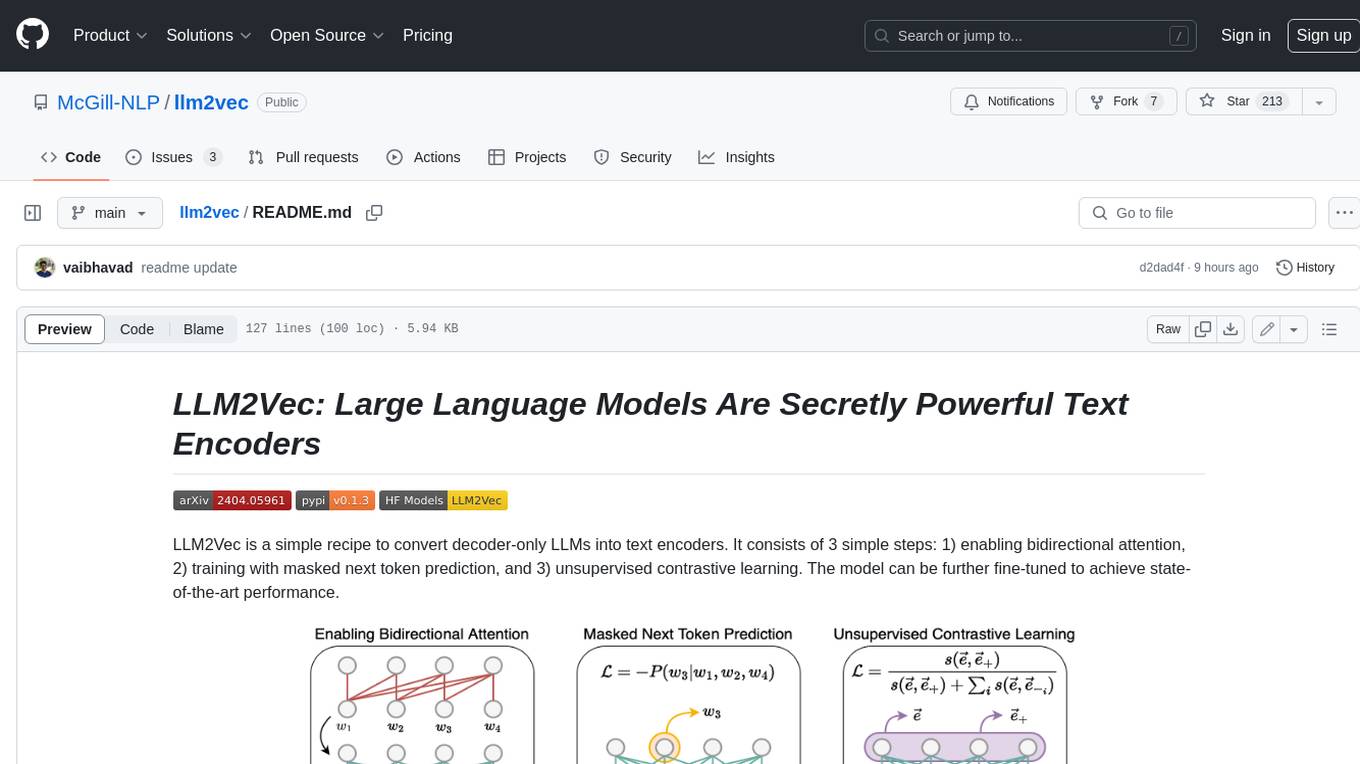
llm2vec
LLM2Vec is a simple recipe to convert decoder-only LLMs into text encoders. It consists of 3 simple steps: 1) enabling bidirectional attention, 2) training with masked next token prediction, and 3) unsupervised contrastive learning. The model can be further fine-tuned to achieve state-of-the-art performance.
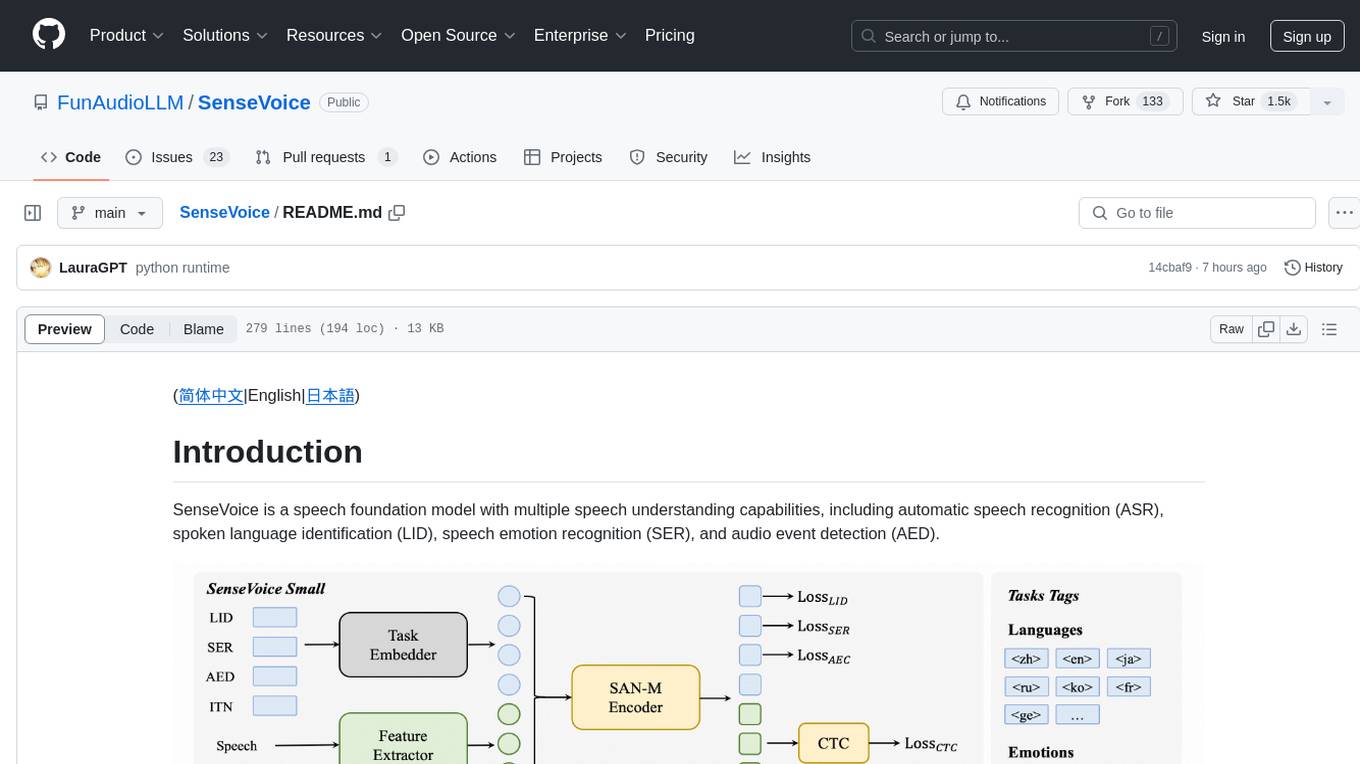
SenseVoice
SenseVoice is a speech foundation model focusing on high-accuracy multilingual speech recognition, speech emotion recognition, and audio event detection. Trained with over 400,000 hours of data, it supports more than 50 languages and excels in emotion recognition and sound event detection. The model offers efficient inference with low latency and convenient finetuning scripts. It can be deployed for service with support for multiple client-side languages. SenseVoice-Small model is open-sourced and provides capabilities for Mandarin, Cantonese, English, Japanese, and Korean. The tool also includes features for natural speech generation and fundamental speech recognition tasks.
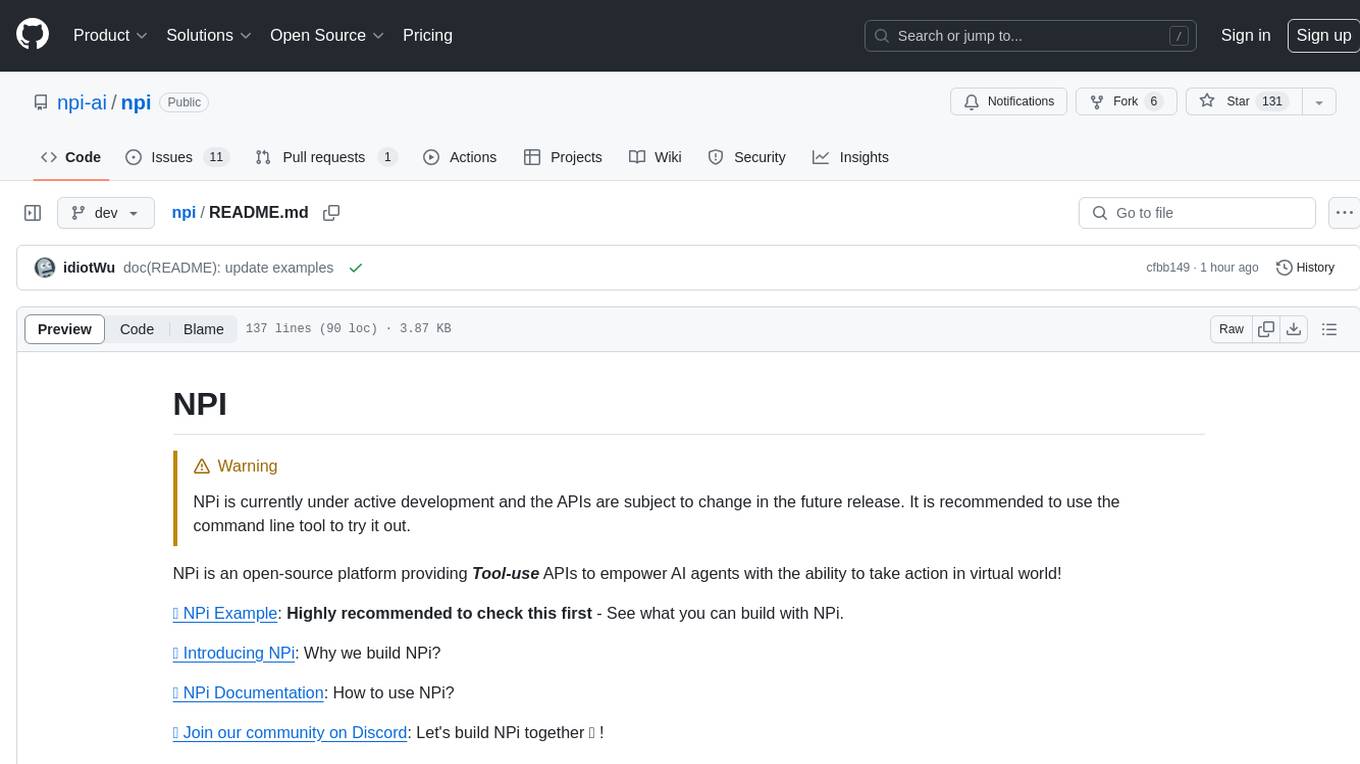
npi
NPi is an open-source platform providing Tool-use APIs to empower AI agents with the ability to take action in the virtual world. It is currently under active development, and the APIs are subject to change in future releases. NPi offers a command line tool for installation and setup, along with a GitHub app for easy access to repositories. The platform also includes a Python SDK and examples like Calendar Negotiator and Twitter Crawler. Join the NPi community on Discord to contribute to the development and explore the roadmap for future enhancements.
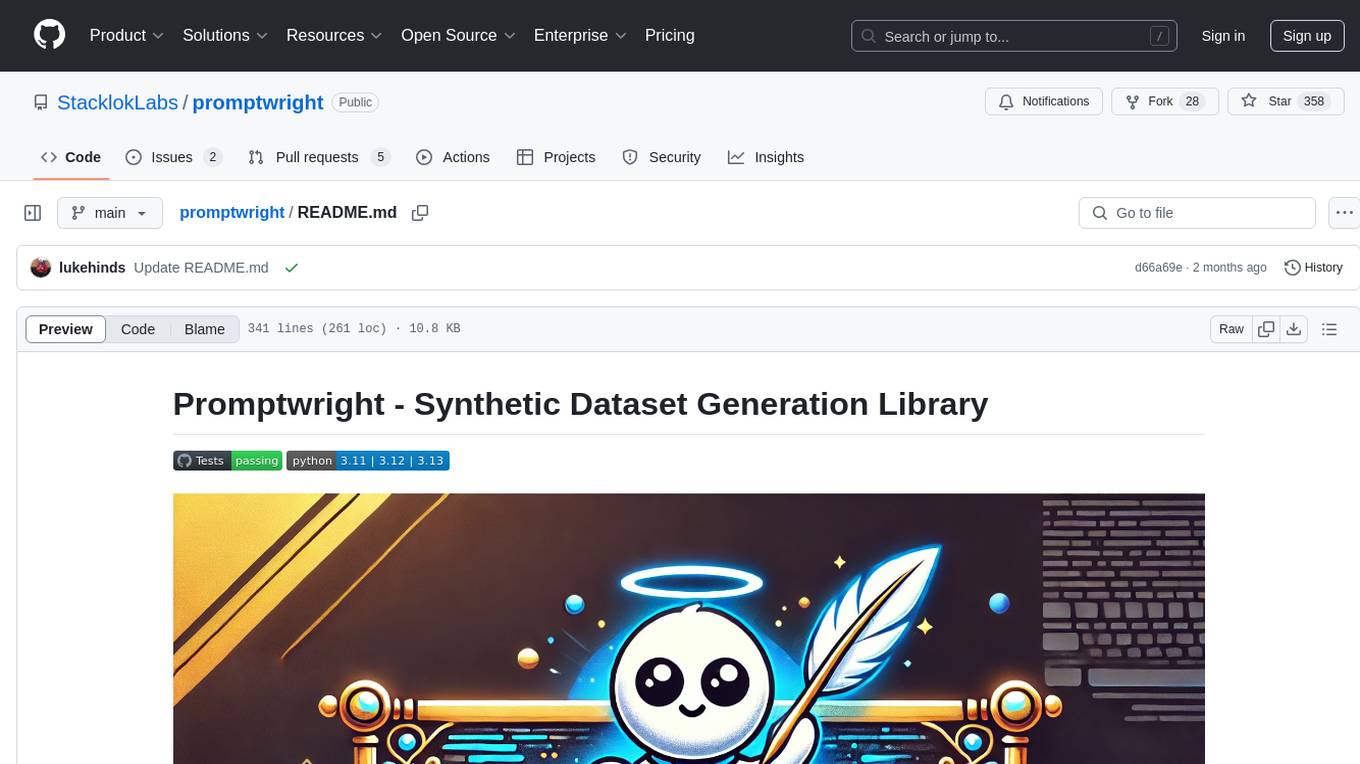
promptwright
Promptwright is a Python library designed for generating large synthetic datasets using a local LLM and various LLM service providers. It offers flexible interfaces for generating prompt-led synthetic datasets. The library supports multiple providers, configurable instructions and prompts, YAML configuration for tasks, command line interface for running tasks, push to Hugging Face Hub for dataset upload, and system message control. Users can define generation tasks using YAML configuration or Python code. Promptwright integrates with LiteLLM to interface with LLM providers and supports automatic dataset upload to Hugging Face Hub.
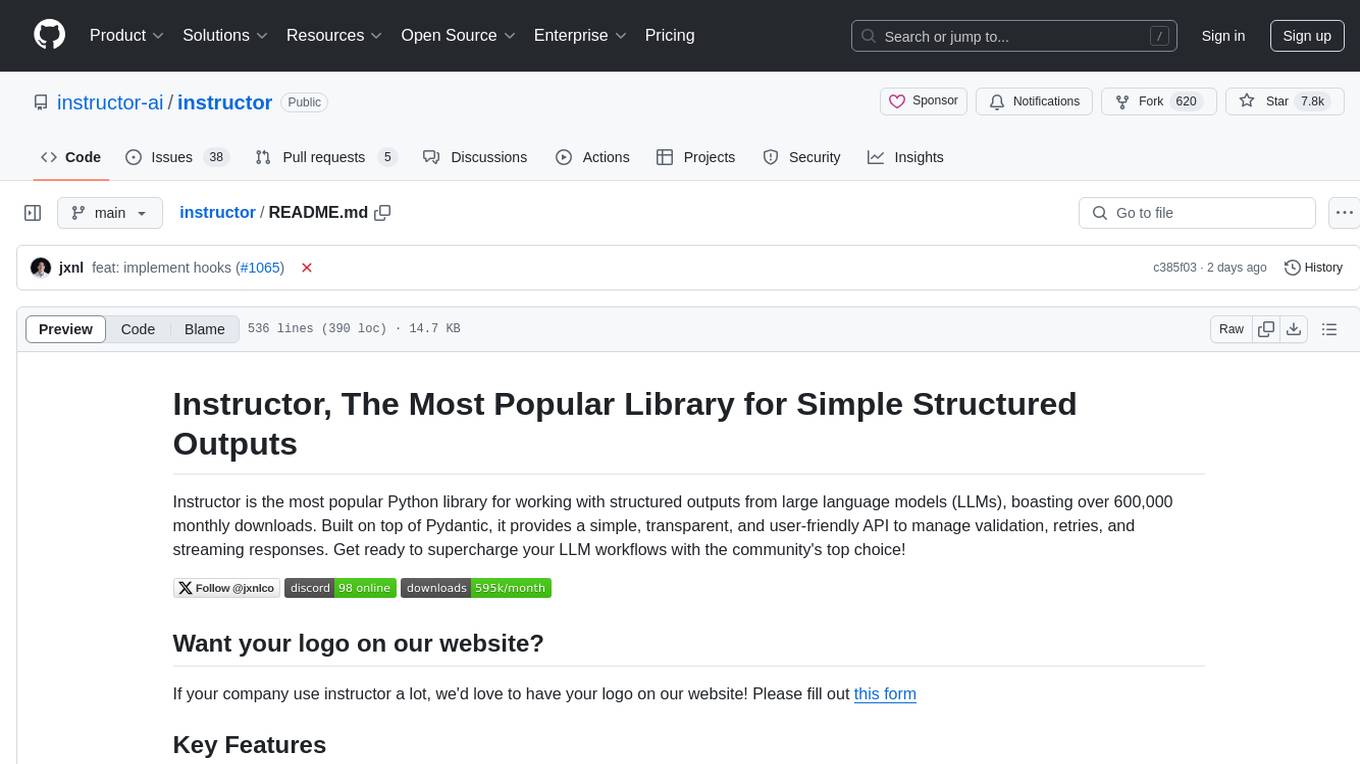
instructor
Instructor is a popular Python library for managing structured outputs from large language models (LLMs). It offers a user-friendly API for validation, retries, and streaming responses. With support for various LLM providers and multiple languages, Instructor simplifies working with LLM outputs. The library includes features like response models, retry management, validation, streaming support, and flexible backends. It also provides hooks for logging and monitoring LLM interactions, and supports integration with Anthropic, Cohere, Gemini, Litellm, and Google AI models. Instructor facilitates tasks such as extracting user data from natural language, creating fine-tuned models, managing uploaded files, and monitoring usage of OpenAI models.
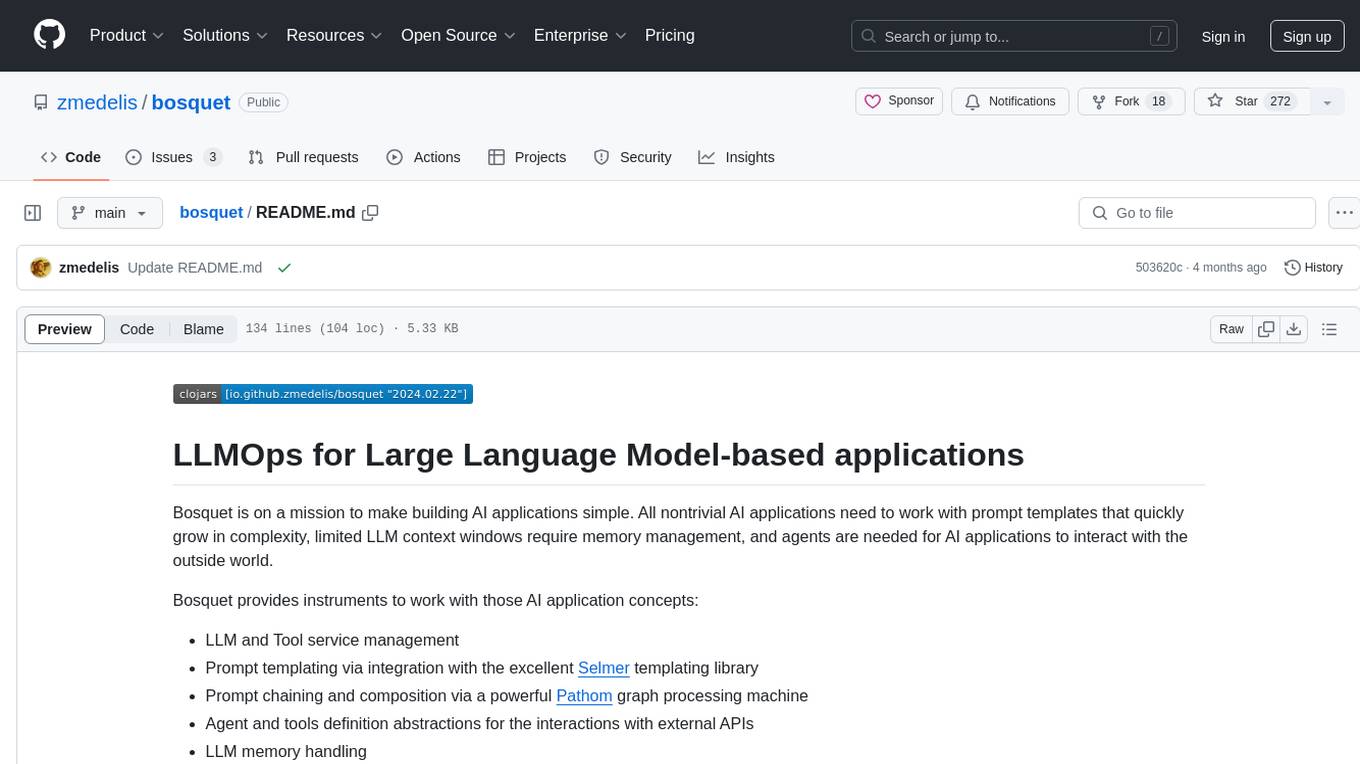
bosquet
Bosquet is a tool designed for LLMOps in large language model-based applications. It simplifies building AI applications by managing LLM and tool services, integrating with Selmer templating library for prompt templating, enabling prompt chaining and composition with Pathom graph processing, defining agents and tools for external API interactions, handling LLM memory, and providing features like call response caching. The tool aims to streamline the development process for AI applications that require complex prompt templates, memory management, and interaction with external systems.
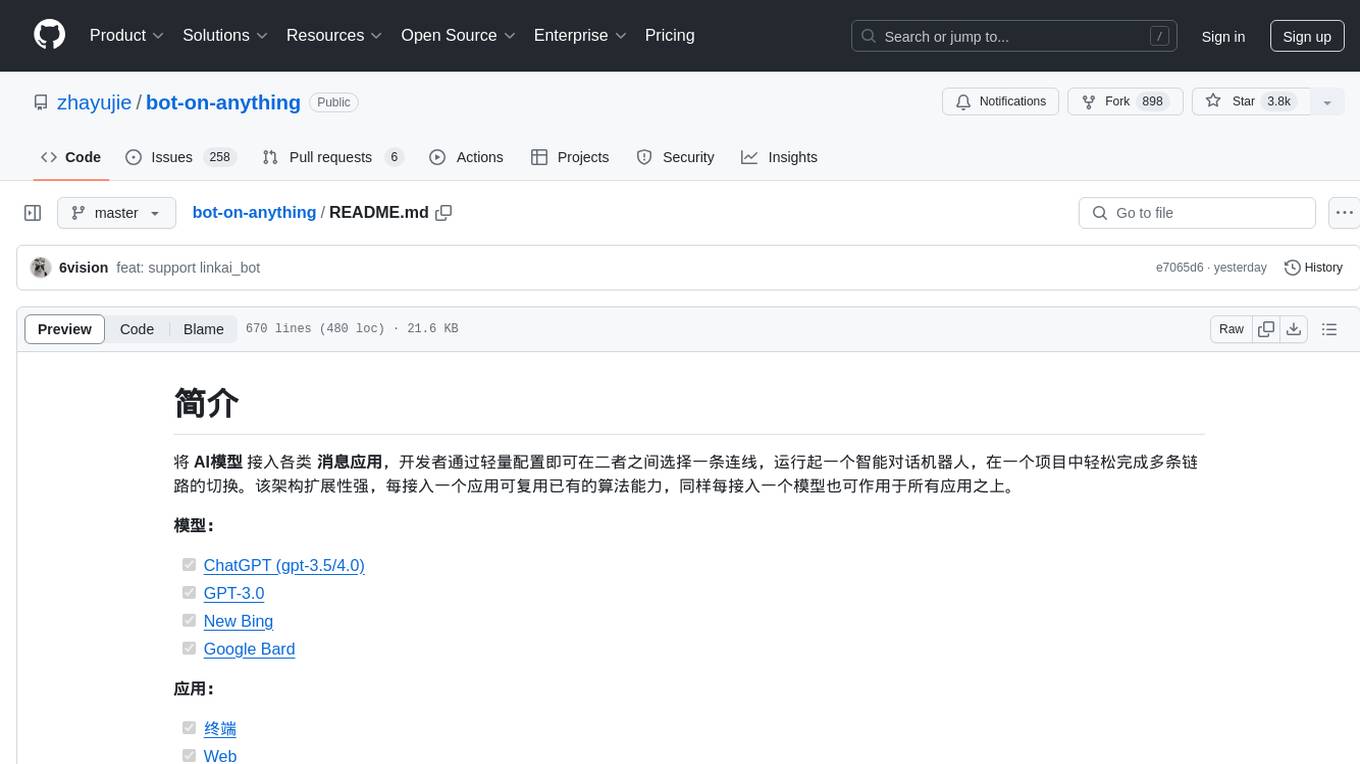
bot-on-anything
The 'bot-on-anything' repository allows developers to integrate various AI models into messaging applications, enabling the creation of intelligent chatbots. By configuring the connections between models and applications, developers can easily switch between multiple channels within a project. The architecture is highly scalable, allowing the reuse of algorithmic capabilities for each new application and model integration. Supported models include ChatGPT, GPT-3.0, New Bing, and Google Bard, while supported applications range from terminals and web platforms to messaging apps like WeChat, Telegram, QQ, and more. The repository provides detailed instructions for setting up the environment, configuring the models and channels, and running the chatbot for various tasks across different messaging platforms.
For similar tasks
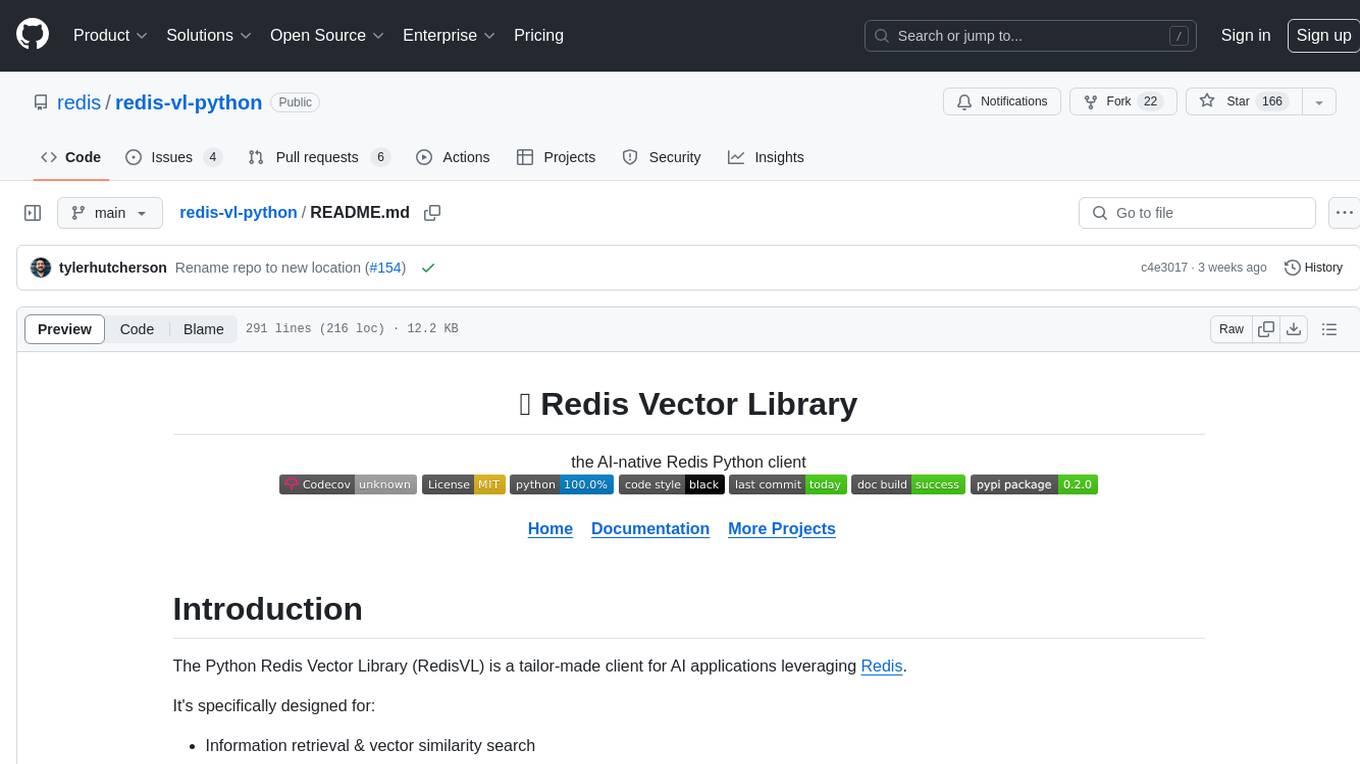
redis-vl-python
The Python Redis Vector Library (RedisVL) is a tailor-made client for AI applications leveraging Redis. It enhances applications with Redis' speed, flexibility, and reliability, incorporating capabilities like vector-based semantic search, full-text search, and geo-spatial search. The library bridges the gap between the emerging AI-native developer ecosystem and the capabilities of Redis by providing a lightweight, elegant, and intuitive interface. It abstracts the features of Redis into a grammar that is more aligned to the needs of today's AI/ML Engineers or Data Scientists.
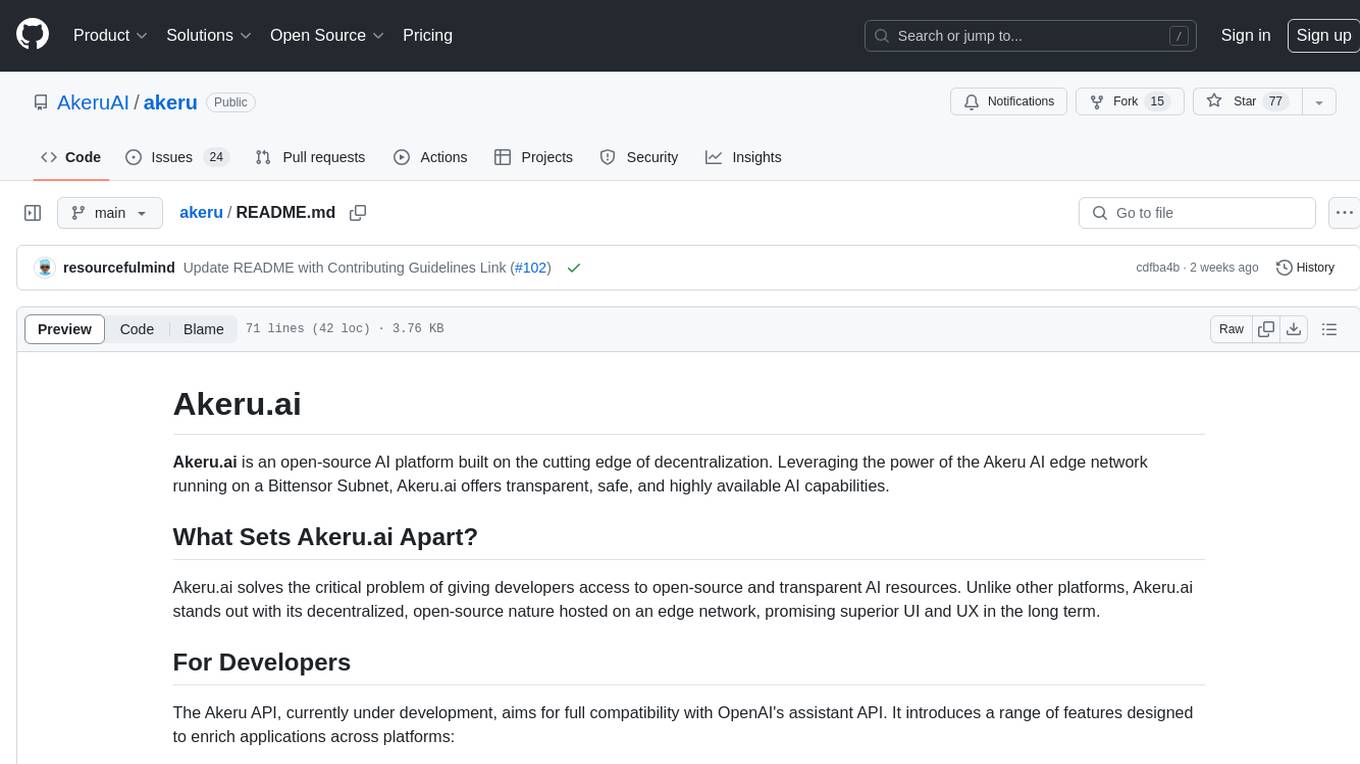
akeru
Akeru.ai is an open-source AI platform leveraging the power of decentralization. It offers transparent, safe, and highly available AI capabilities. The platform aims to give developers access to open-source and transparent AI resources through its decentralized nature hosted on an edge network. Akeru API introduces features like retrieval, function calling, conversation management, custom instructions, data input optimization, user privacy, testing and iteration, and comprehensive documentation. It is ideal for creating AI agents and enhancing web and mobile applications with advanced AI capabilities. The platform runs on a Bittensor Subnet design that aims to democratize AI technology and promote an equitable AI future. Akeru.ai embraces decentralization challenges to ensure a decentralized and equitable AI ecosystem with security features like watermarking and network pings. The API architecture integrates with technologies like Bun, Redis, and Elysia for a robust, scalable solution.
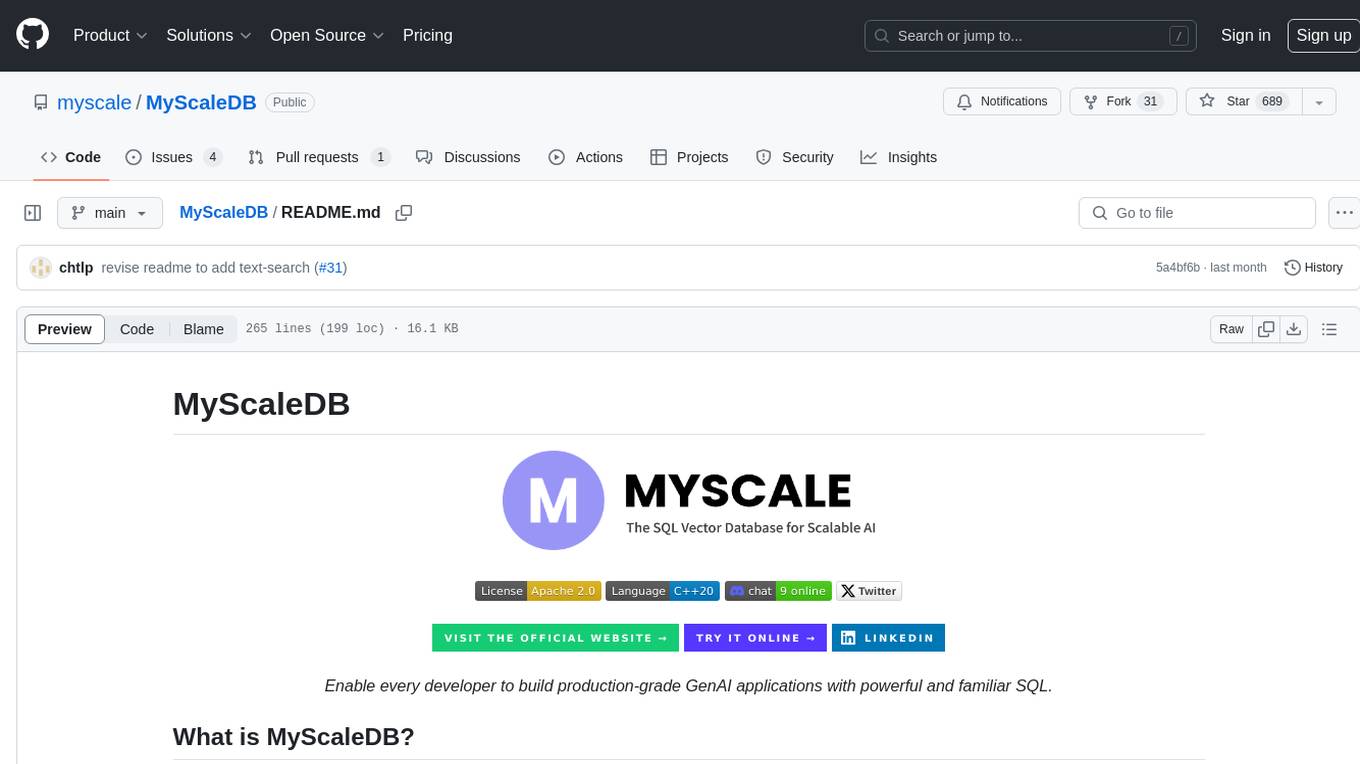
MyScaleDB
MyScaleDB is a SQL vector database optimized for AI applications, enabling developers to manage and process massive volumes of data efficiently. It offers fast and powerful vector search, filtered search, and SQL-vector join queries, making it fully SQL-compatible. MyScaleDB provides unmatched performance and scalability by leveraging cutting-edge OLAP database architecture and advanced vector algorithms. It is production-ready for AI applications, supporting structured data, text, vector, JSON, geospatial, and time-series data. MyScale Cloud offers fully-managed MyScaleDB with premium features on billion-scale data, making it cost-effective and simpler to use compared to specialized vector databases. Built on top of ClickHouse, MyScaleDB combines structured and vector search efficiently, ensuring high accuracy and performance in filtered search operations.
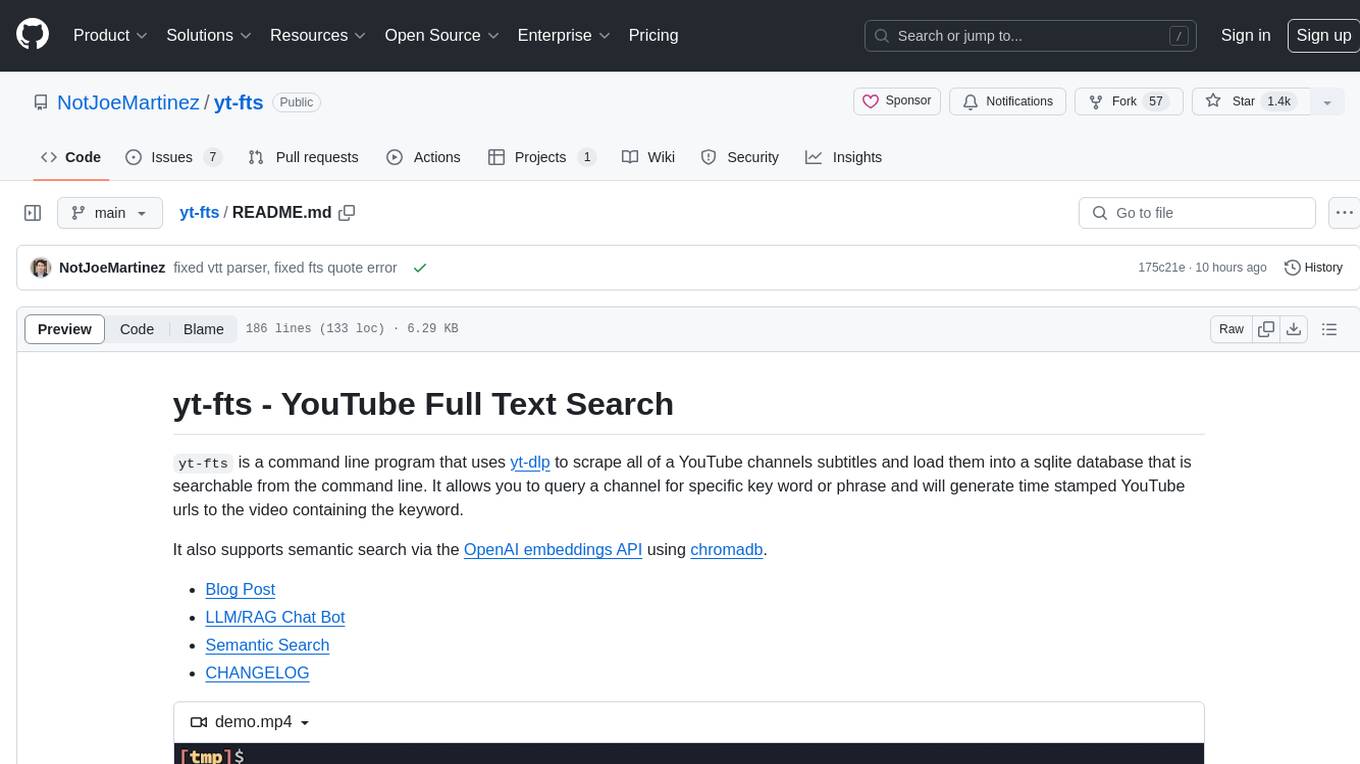
yt-fts
yt-fts is a command line program that uses yt-dlp to scrape all of a YouTube channels subtitles and load them into a sqlite database for full text search. It allows users to query a channel for specific keywords or phrases and generates time stamped YouTube URLs to the videos containing the keyword. Additionally, it supports semantic search via the OpenAI embeddings API using chromadb.
For similar jobs
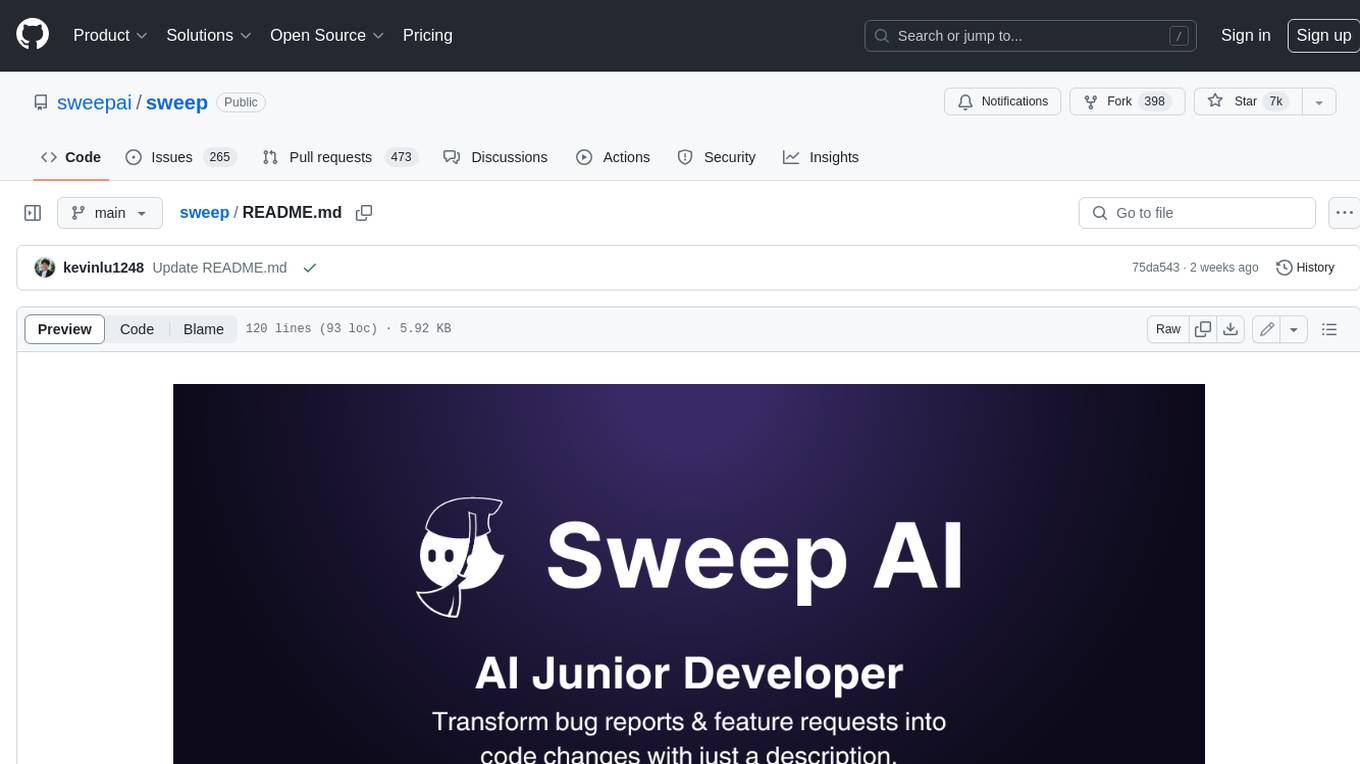
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
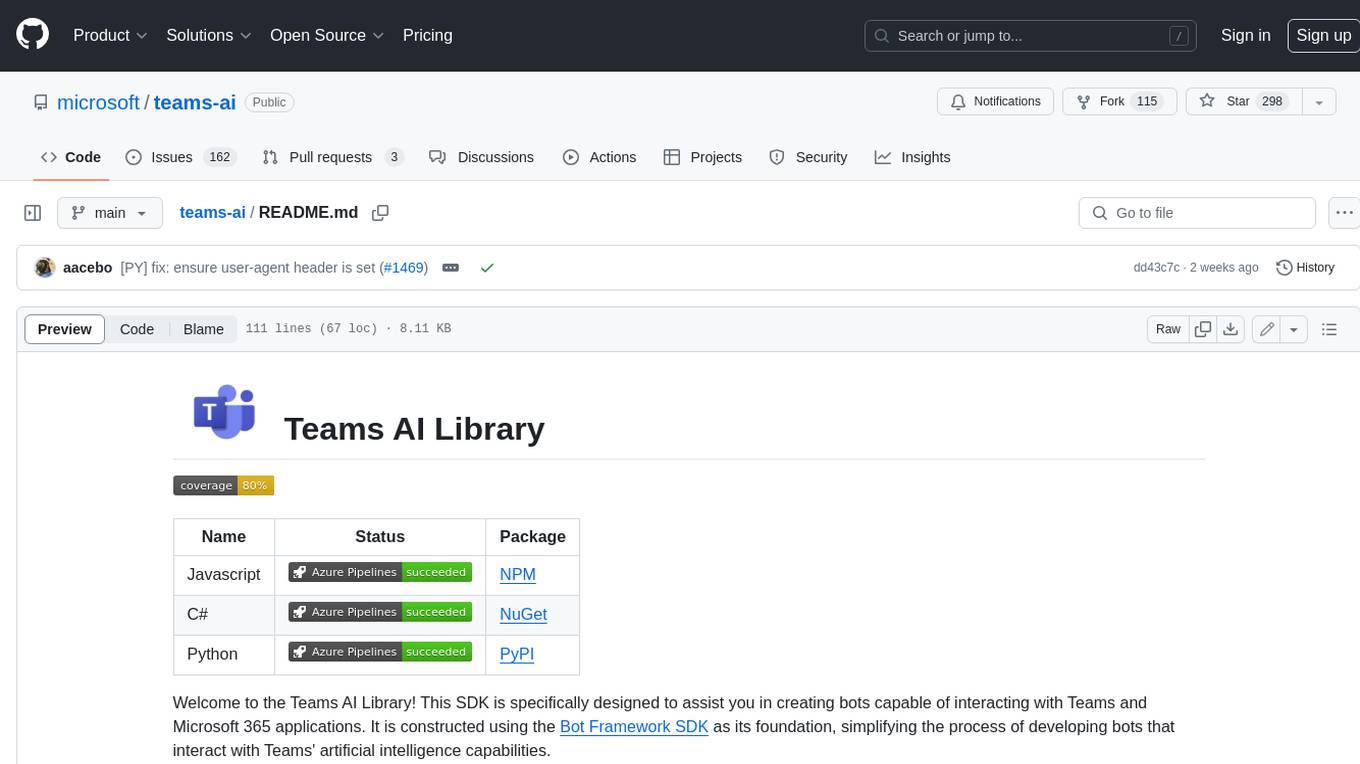
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
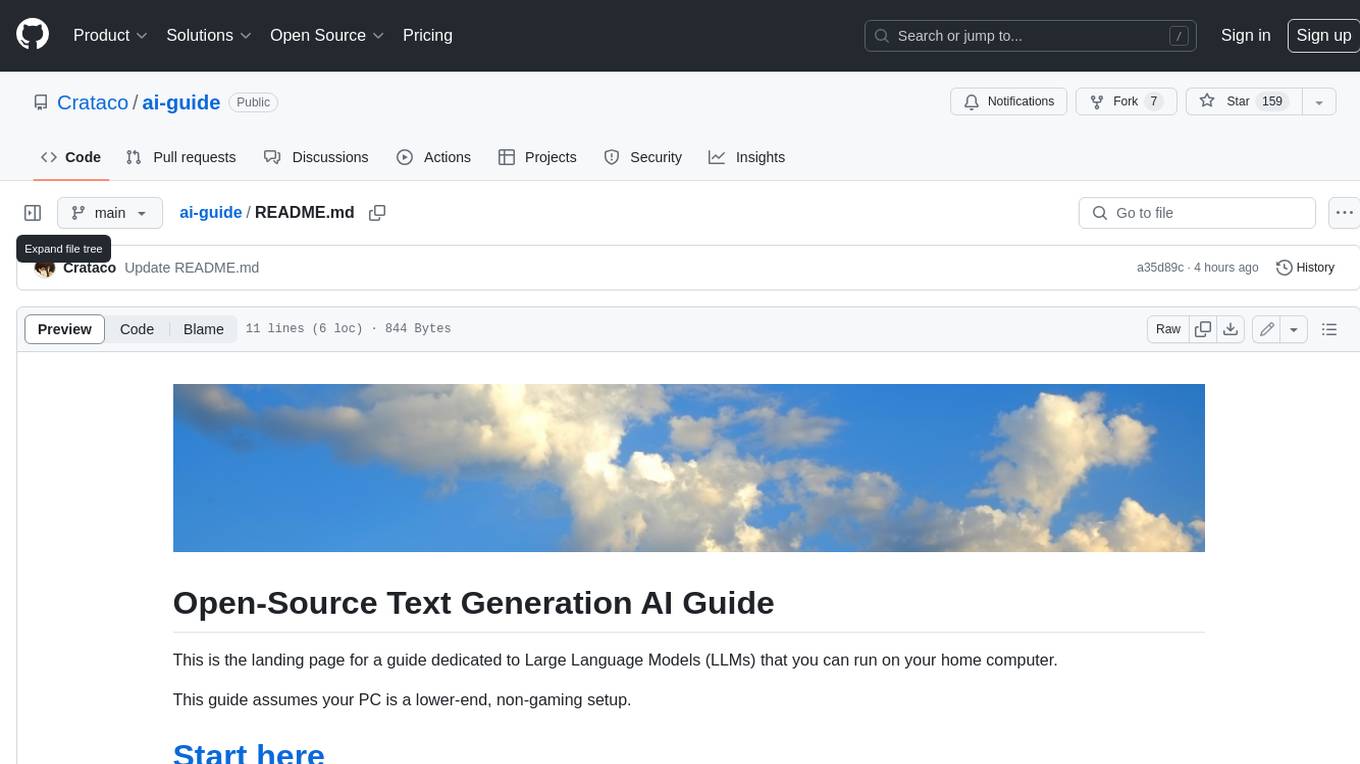
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
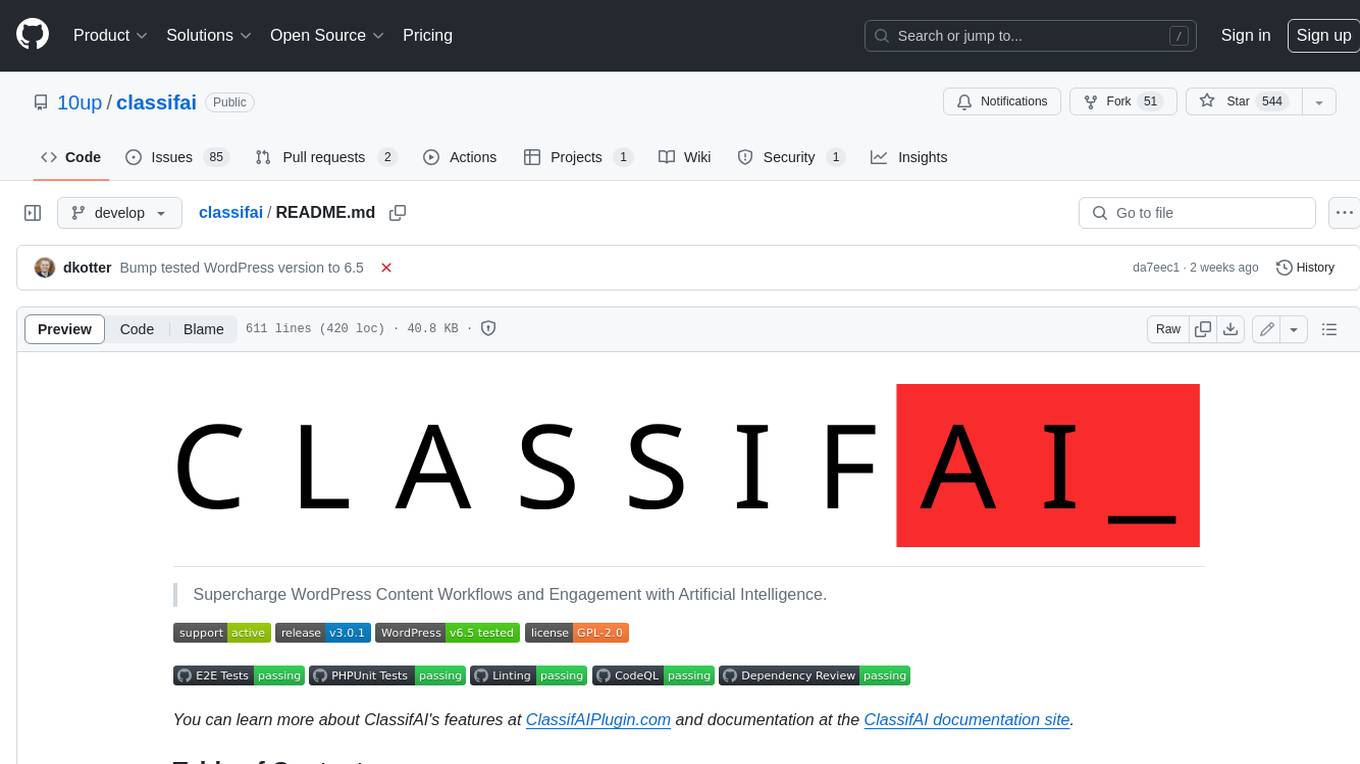
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
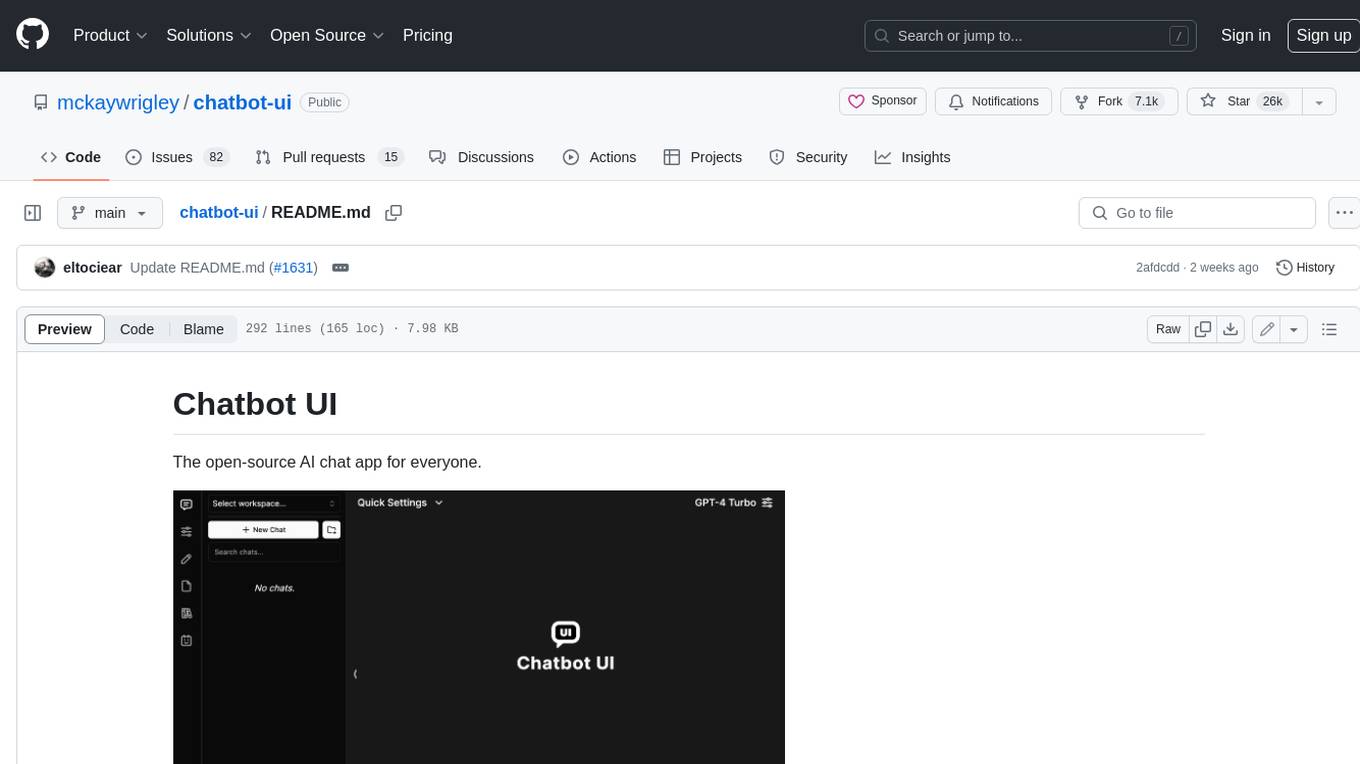
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
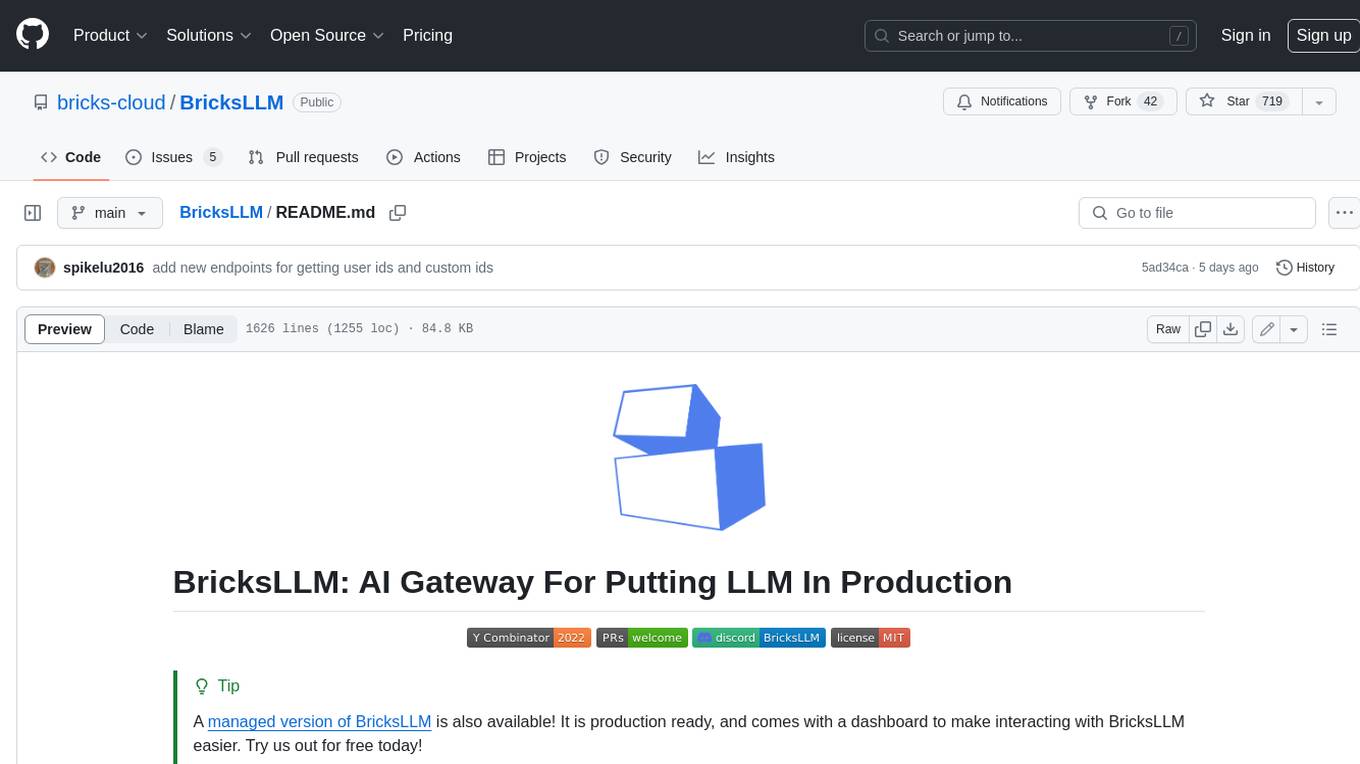
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
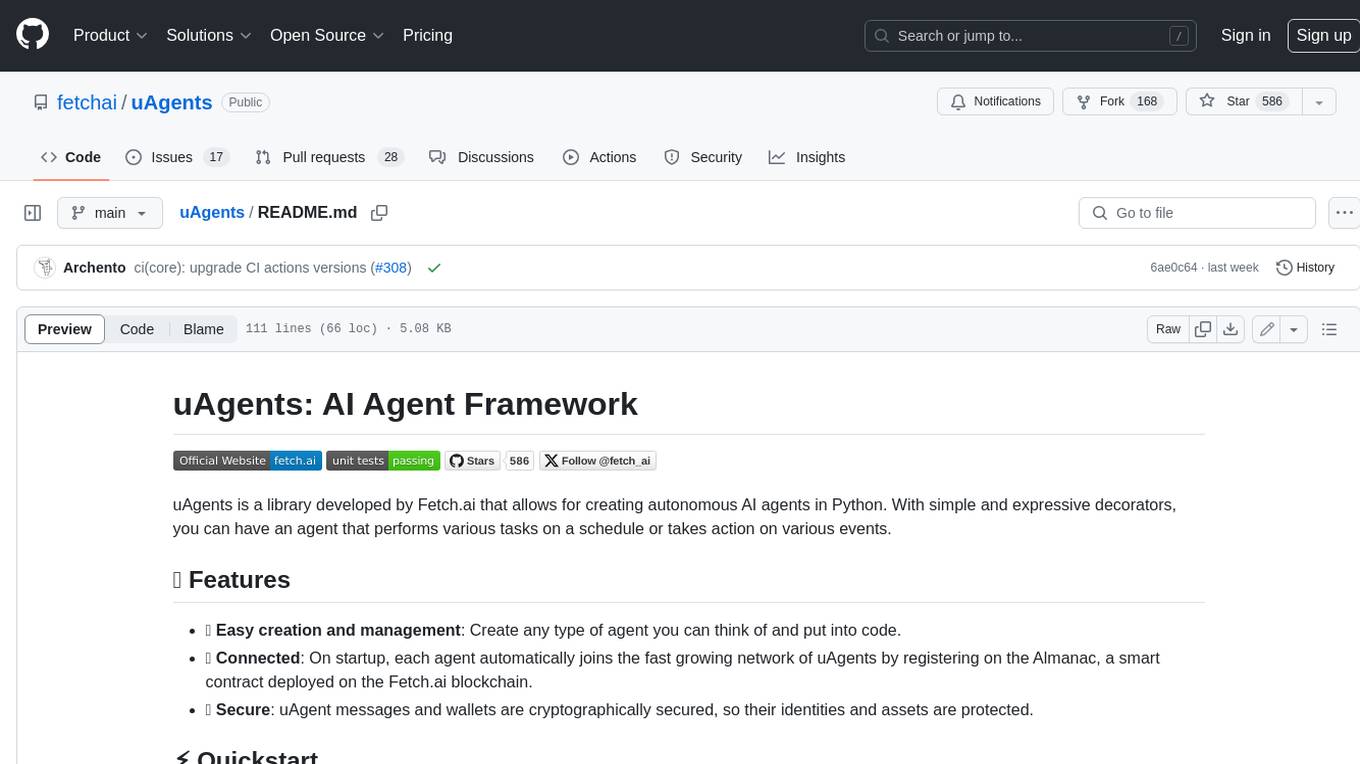
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
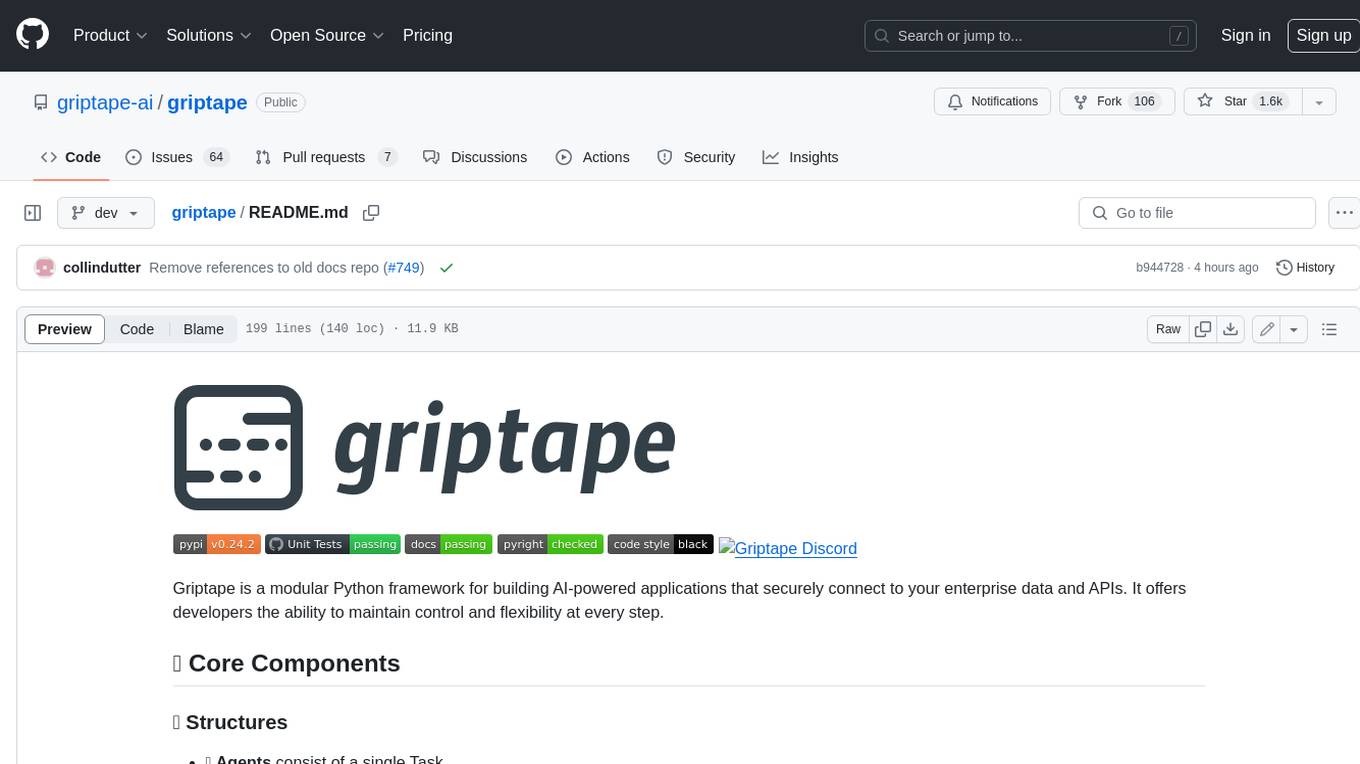
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.