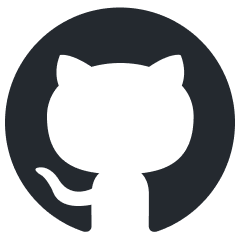
griptape
Modular Python framework for AI agents and workflows with chain-of-thought reasoning, tools, and memory.
Stars: 2144
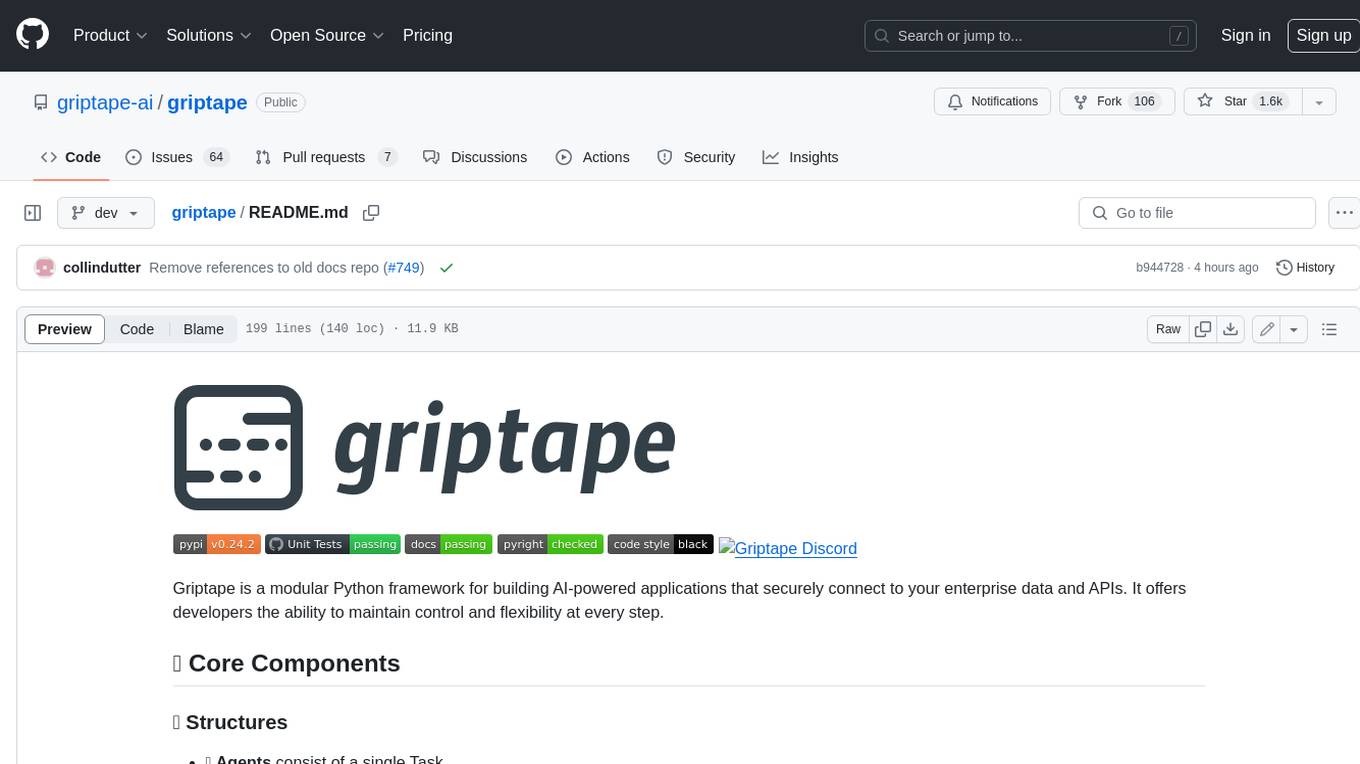
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.
README:
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step.
- π€ Agents consist of a single Task.
- π Pipelines organize a sequence of Tasks so that the output from one Task may flow into the next.
- π Workflows configure Tasks to operate in parallel.
Tasks are the core building blocks within Structures, enabling interaction with Engines, Tools, and other Griptape components.
Tools provide capabilities for LLMs to interact with data and services. Griptape includes a variety of built-in Tools, and makes it easy to create custom Tools.
- π¬ Conversation Memory enables LLMs to retain and retrieve information across interactions.
- ποΈ Task Memory keeps large or sensitive Task outputs off the prompt that is sent to the LLM.
- π Meta Memory enables passing in additional metadata to the LLM, enhancing the context and relevance of the interaction.
Drivers facilitate interactions with external resources and services:
- π£οΈ Prompt Drivers manage textual and image interactions with LLMs.
- π’ Embedding Drivers generate vector embeddings from textual inputs.
- πΎ Vector Store Drivers manage the storage and retrieval of embeddings.
- π¨ Image Generation Drivers create images from text descriptions.
- πΌ SQL Drivers interact with SQL databases.
- π Web Scraper Drivers extract information from web pages.
- π§ Conversation Memory Drivers manage the storage and retrieval of conversational data.
- π‘ Event Listener Drivers forward framework events to external services.
- ποΈ Structure Run Drivers execute structures both locally and in the cloud.
- π€ Assistant Drivers enable interactions with various "assistant" services.
- π£οΈ Text to Speech Drivers convert text to speech.
- ποΈ Audio Transcription Drivers convert audio to text.
- π Web Search Drivers search the web for information.
- π Observability Drivers send trace and event data to observability platforms.
- π Ruleset Drivers load and apply rulesets from external sources.
- ποΈ File Manager Drivers handle file operations on local and remote storage.
Engines wrap Drivers and provide use-case-specific functionality:
- π RAG Engine is an abstraction for implementing modular Retrieval Augmented Generation (RAG) pipelines.
- π οΈ Extraction Engine extracts JSON or CSV data from unstructured text.
- π Summary Engine generates summaries from textual content.
- β Eval Engine evaluates and scores the quality of generated text.
- π Rulesets steer LLM behavior with minimal prompt engineering.
- π Loaders load data from various sources.
- πΊ Artifacts allow for passing data of different types between Griptape components.
- βοΈ Chunkers segment texts into manageable pieces for diverse text types.
- π’ Tokenizers count the number of tokens in a text to not exceed LLM token limits.
Please refer to Griptape Docs for:
- Getting started guides.
- Core concepts and design overviews.
- Examples.
- Contribution guidelines.
Please check out Griptape Trade School for free online courses.
First, install griptape:
pip install "griptape[all]" -U
Second, configure an OpenAI client by getting an API key and adding it to your environment as OPENAI_API_KEY
. By default, Griptape uses OpenAI Chat Completions API to execute LLM prompts.
With Griptape, you can create Structures, such as Agents, Pipelines, and Workflows, composed of different types of Tasks. Let's build a simple creative Agent that dynamically uses three tools and moves the data around in Task Memory.
from griptape.structures import Agent
from griptape.tools import WebScraperTool, FileManagerTool, PromptSummaryTool
agent = Agent(
input="Load {{ args[0] }}, summarize it, and store it in a file called {{ args[1] }}.",
tools=[
WebScraperTool(off_prompt=True),
PromptSummaryTool(off_prompt=True),
FileManagerTool()
]
)
agent.run("https://griptape.ai", "griptape.txt")
And here is the output:
[08/12/24 14:48:15] INFO PromptTask c90d263ec69046e8b30323c131ae4ba0
Input: Load https://griptape.ai, summarize it, and store it in a file called griptape.txt.
[08/12/24 14:48:16] INFO Subtask ebe23832cbe2464fb9ecde9fcee7c30f
Actions: [
{
"tag": "call_62kBnkswnk9Y6GH6kn1GIKk6",
"name": "WebScraperTool",
"path": "get_content",
"input": {
"values": {
"url": "https://griptape.ai"
}
}
}
]
[08/12/24 14:48:17] INFO Subtask ebe23832cbe2464fb9ecde9fcee7c30f
Response: Output of "WebScraperTool.get_content" was stored in memory with memory_name "TaskMemory" and artifact_namespace
"cecca28eb0c74bcd8c7119ed7f790c95"
[08/12/24 14:48:18] INFO Subtask dca04901436d49d2ade86cd6b4e1038a
Actions: [
{
"tag": "call_o9F1taIxHty0mDlWLcAjTAAu",
"name": "PromptSummaryTool",
"path": "summarize",
"input": {
"values": {
"summary": {
"memory_name": "TaskMemory",
"artifact_namespace": "cecca28eb0c74bcd8c7119ed7f790c95"
}
}
}
}
]
[08/12/24 14:48:21] INFO Subtask dca04901436d49d2ade86cd6b4e1038a
Response: Output of "PromptSummaryTool.summarize" was stored in memory with memory_name "TaskMemory" and artifact_namespace
"73765e32b8404e32927822250dc2ae8b"
[08/12/24 14:48:22] INFO Subtask c233853450fb4fd6a3e9c04c52b33bf6
Actions: [
{
"tag": "call_eKvIUIw45aRYKDBpT1gGKc9b",
"name": "FileManagerTool",
"path": "save_memory_artifacts_to_disk",
"input": {
"values": {
"dir_name": ".",
"file_name": "griptape.txt",
"memory_name": "TaskMemory",
"artifact_namespace": "73765e32b8404e32927822250dc2ae8b"
}
}
}
]
INFO Subtask c233853450fb4fd6a3e9c04c52b33bf6
Response: Successfully saved memory artifacts to disk
[08/12/24 14:48:23] INFO PromptTask c90d263ec69046e8b30323c131ae4ba0
Output: The content from https://griptape.ai has been summarized and stored in a file called `griptape.txt`.
During the run, the Griptape Agent loaded a webpage with a Tool, stored its full content in Task Memory, queried it to answer the original question, and finally saved the answer to a file.
The important thing to note here is that no matter how big the webpage is it can never blow up the prompt token limit because the full content of the page never goes back to the LLM. Additionally, no data from the subsequent subtasks were returned back to the prompt either. So, how does it work?
In the above example, we set off_prompt to True
, which means that the LLM can never see the data it manipulates, but can send it to other Tools.
[!IMPORTANT] This example uses Griptape's PromptTask with
tools
, which requires a highly capable LLM to function correctly. By default, Griptape uses the OpenAiChatPromptDriver; for another powerful LLM try swapping to the AnthropicPromptDriver! If you're using a less powerful LLM, consider using the ToolTask instead, as thePromptTask
withtools
might not work properly or at all.
Check out our docs to learn more about how to use Griptape with other LLM providers like Anthropic, Claude, Hugging Face, and Azure.
Griptape uses Semantic Versioning.
Thank you for considering contributing to Griptape! Before you start, please review our Contributing Guidelines.
Griptape is available under the Apache 2.0 License.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for griptape
Similar Open Source Tools
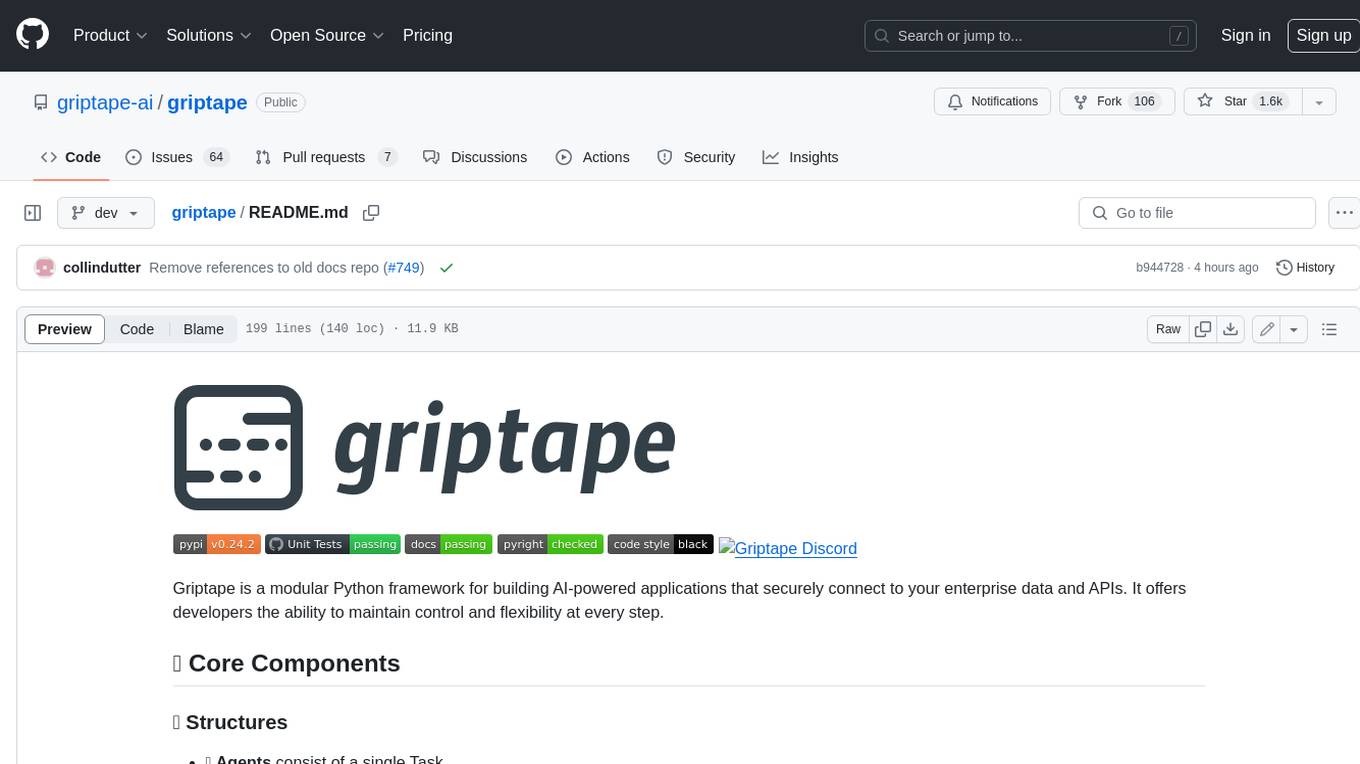
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.
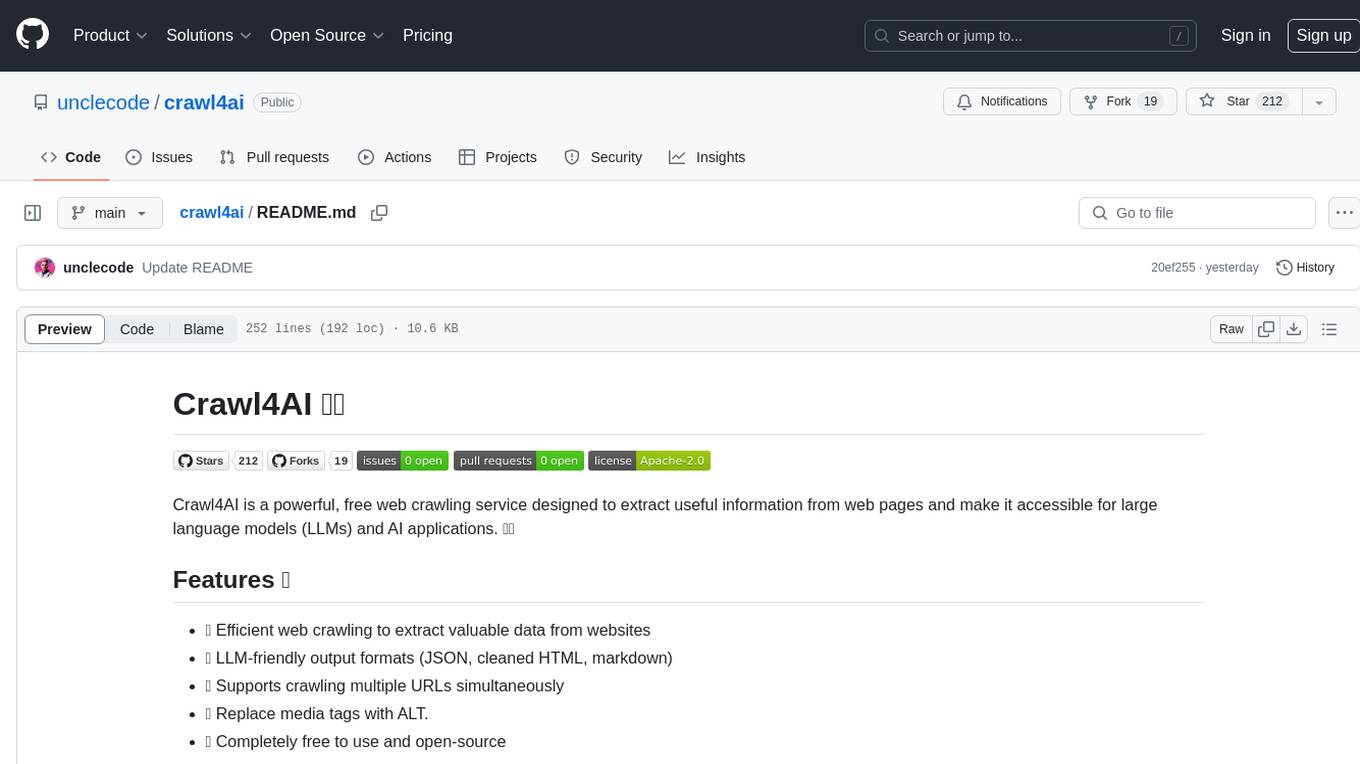
crawl4ai
Crawl4AI is a powerful and free web crawling service that extracts valuable data from websites and provides LLM-friendly output formats. It supports crawling multiple URLs simultaneously, replaces media tags with ALT, and is completely free to use and open-source. Users can integrate Crawl4AI into Python projects as a library or run it as a standalone local server. The tool allows users to crawl and extract data from specified URLs using different providers and models, with options to include raw HTML content, force fresh crawls, and extract meaningful text blocks. Configuration settings can be adjusted in the `crawler/config.py` file to customize providers, API keys, chunk processing, and word thresholds. Contributions to Crawl4AI are welcome from the open-source community to enhance its value for AI enthusiasts and developers.
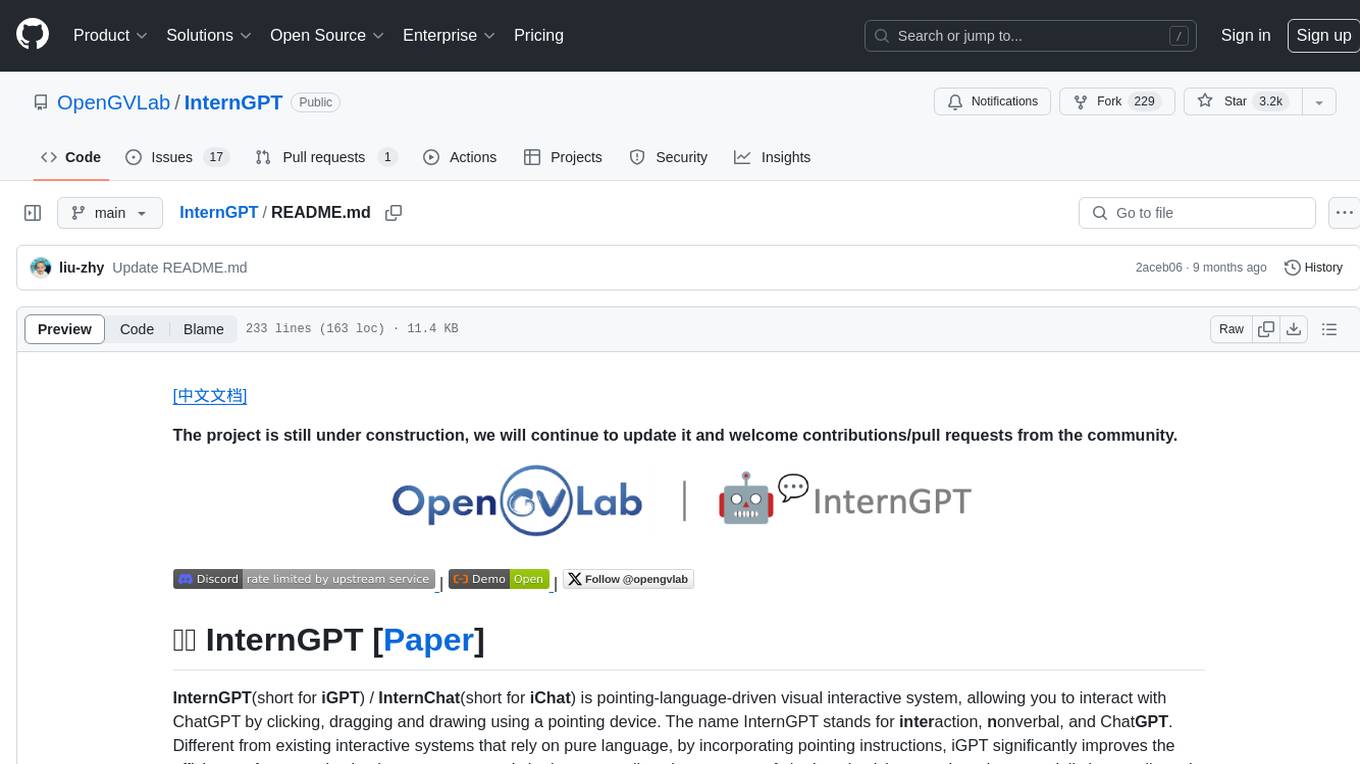
InternGPT
InternGPT (iGPT) is a pointing-language-driven visual interactive system that enhances communication between users and chatbots by incorporating pointing instructions. It improves chatbot accuracy in vision-centric tasks, especially in complex visual scenarios. The system includes an auxiliary control mechanism to enhance the control capability of the language model. InternGPT features a large vision-language model called Husky, fine-tuned for high-quality multi-modal dialogue. Users can interact with ChatGPT by clicking, dragging, and drawing using a pointing device, leading to efficient communication and improved chatbot performance in vision-related tasks.
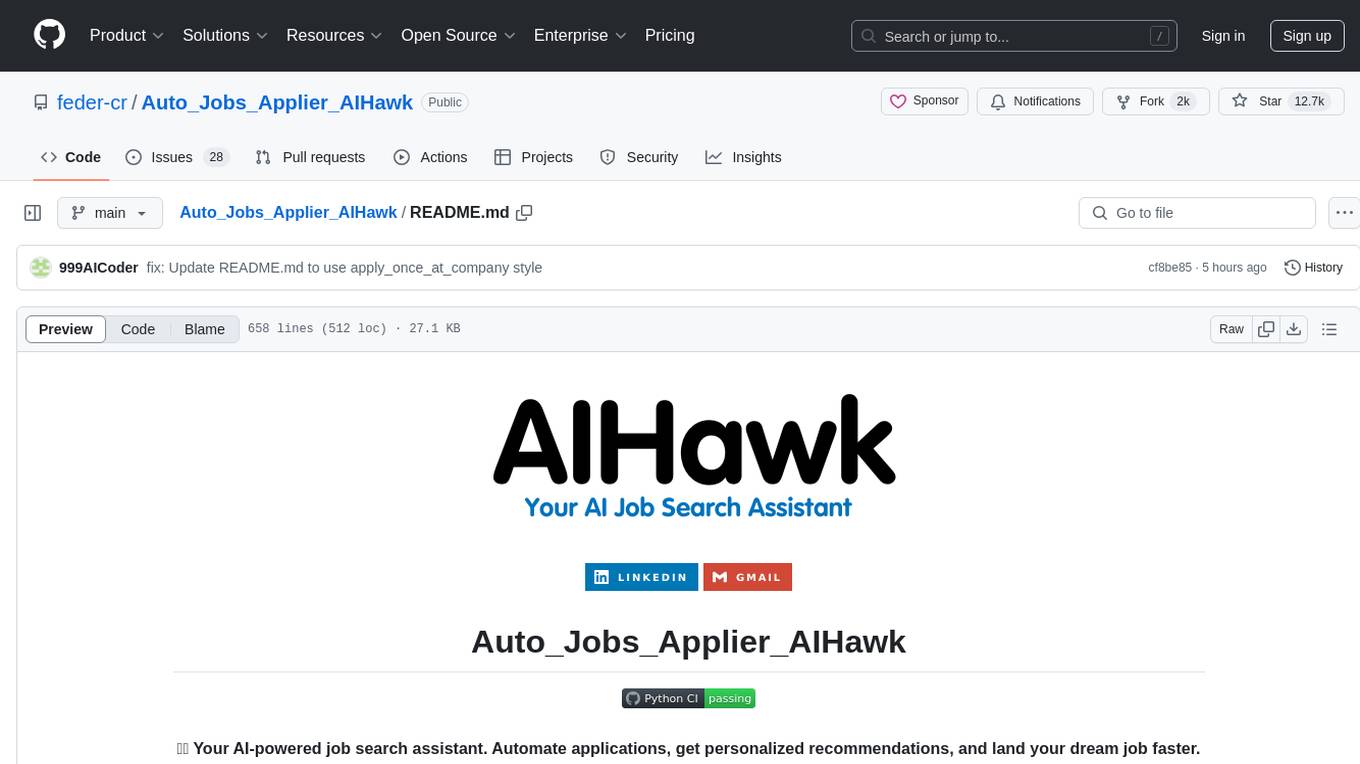
Auto_Jobs_Applier_AIHawk
Auto_Jobs_Applier_AIHawk is an AI-powered job search assistant that revolutionizes the job search and application process. It automates application submissions, provides personalized recommendations, and enhances the chances of landing a dream job. The tool offers features like intelligent job search automation, rapid application submission, AI-powered personalization, volume management with quality, intelligent filtering, dynamic resume generation, and secure data handling. It aims to address the challenges of modern job hunting by saving time, increasing efficiency, and improving application quality.
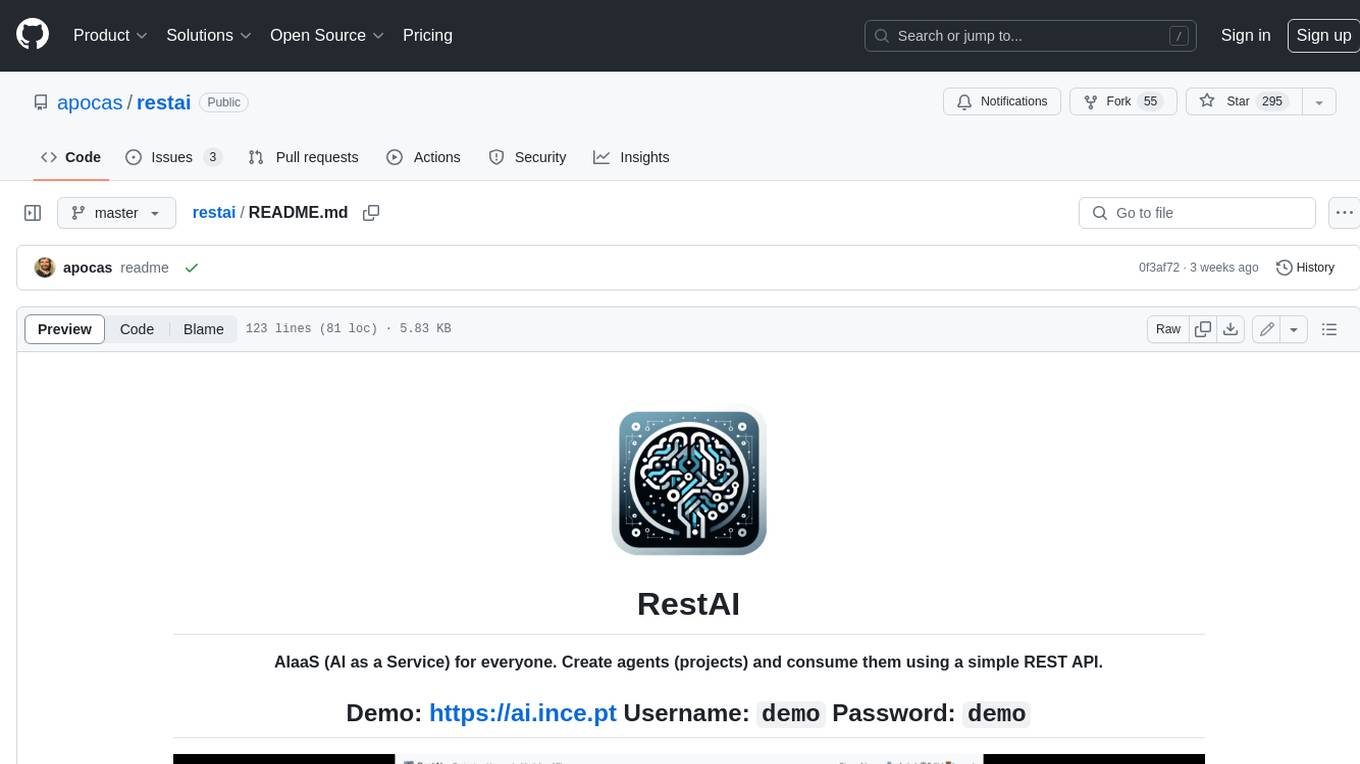
restai
RestAI is an AIaaS (AI as a Service) platform that allows users to create and consume AI agents (projects) using a simple REST API. It supports various types of agents, including RAG (Retrieval-Augmented Generation), RAGSQL (RAG for SQL), inference, vision, and router. RestAI features automatic VRAM management, support for any public LLM supported by LlamaIndex or any local LLM supported by Ollama, a user-friendly API with Swagger documentation, and a frontend for easy access. It also provides evaluation capabilities for RAG agents using deepeval.
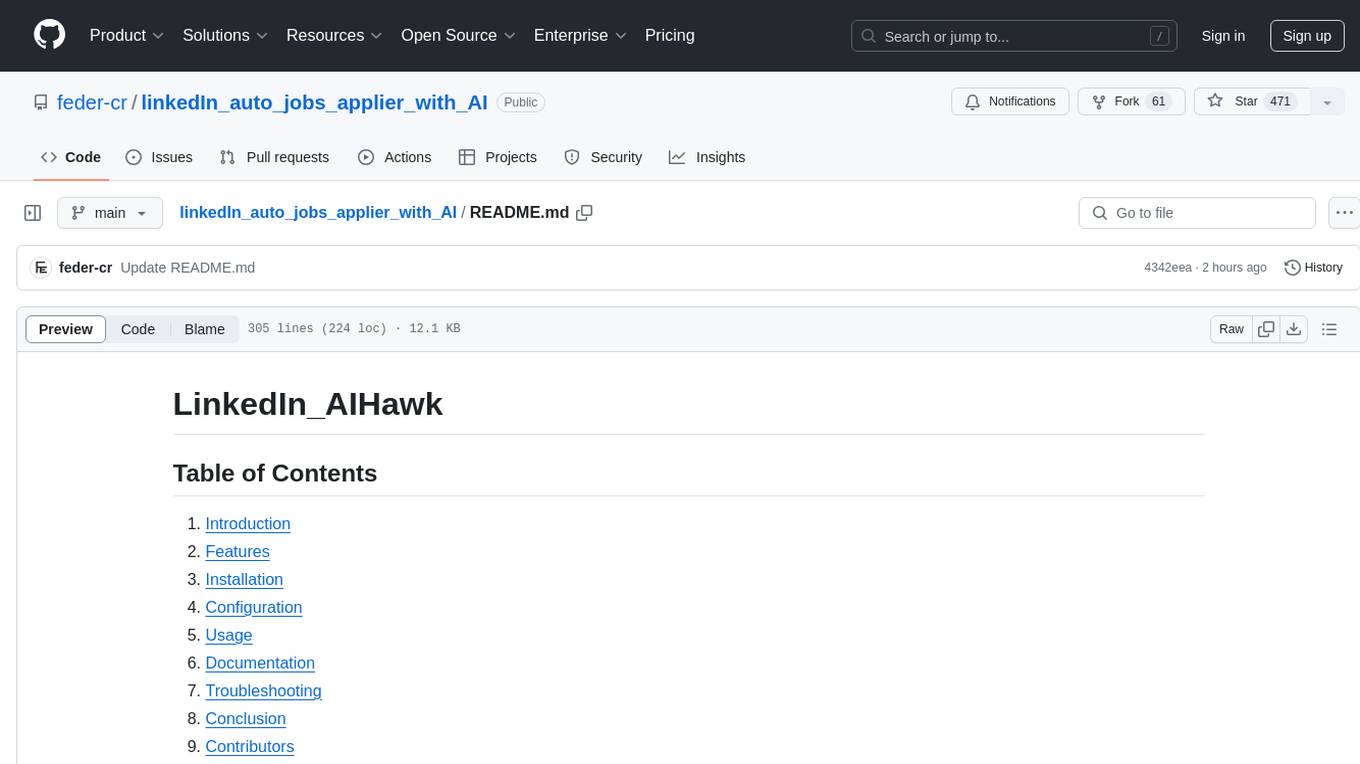
linkedIn_auto_jobs_applier_with_AI
LinkedIn_AIHawk is an automated tool designed to revolutionize the job search and application process on LinkedIn. It leverages automation and artificial intelligence to efficiently apply to relevant positions, personalize responses, manage application volume, filter listings, generate dynamic resumes, and handle sensitive information securely. The tool aims to save time, increase application relevance, and enhance job search effectiveness in today's competitive landscape.
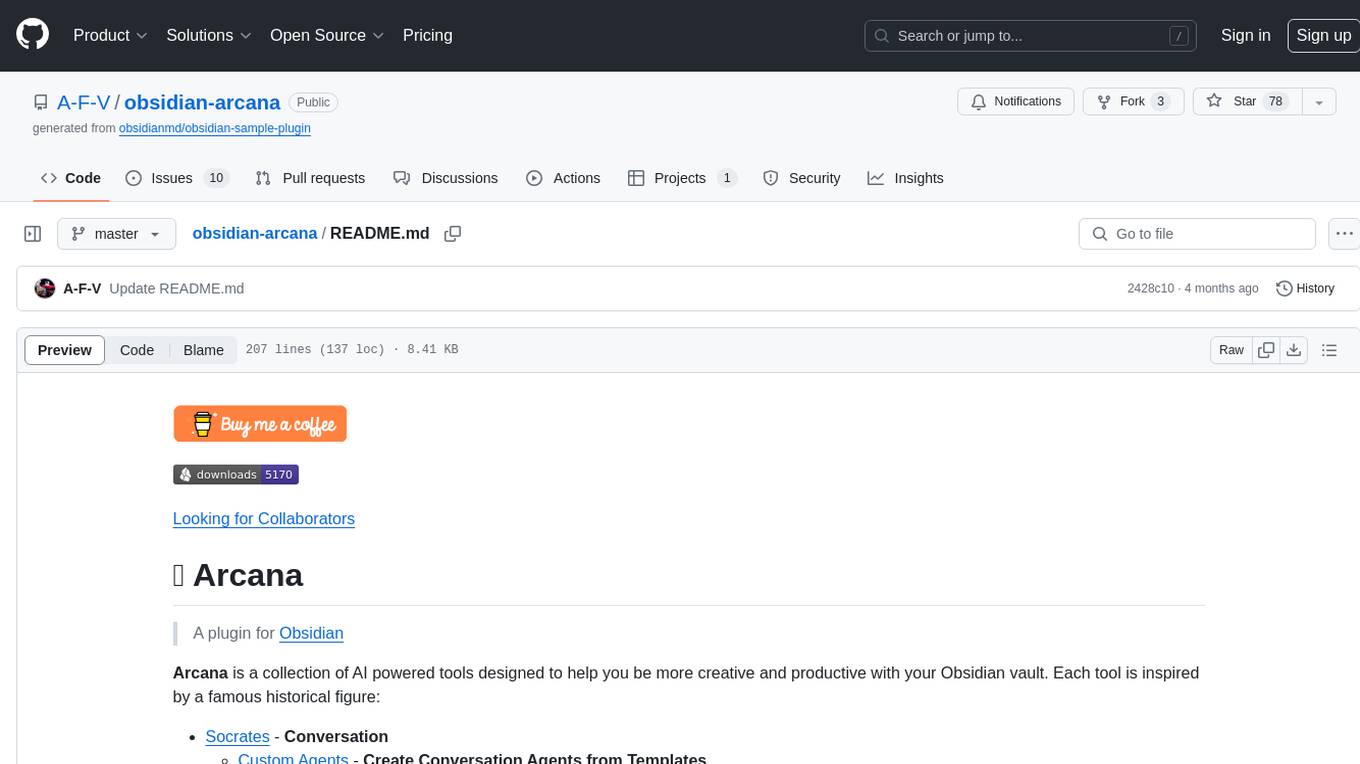
obsidian-arcana
Arcana is a plugin for Obsidian that offers a collection of AI-powered tools inspired by famous historical figures to enhance creativity and productivity. It includes tools for conversation, text-to-speech transcription, speech-to-text replies, metadata markup, text generation, file moving, flashcard generation, auto tagging, and note naming. Users can interact with these tools using the command palette and sidebar views, with an OpenAI API key required for usage. The plugin aims to assist users in various note-taking and knowledge management tasks within the Obsidian vault environment.
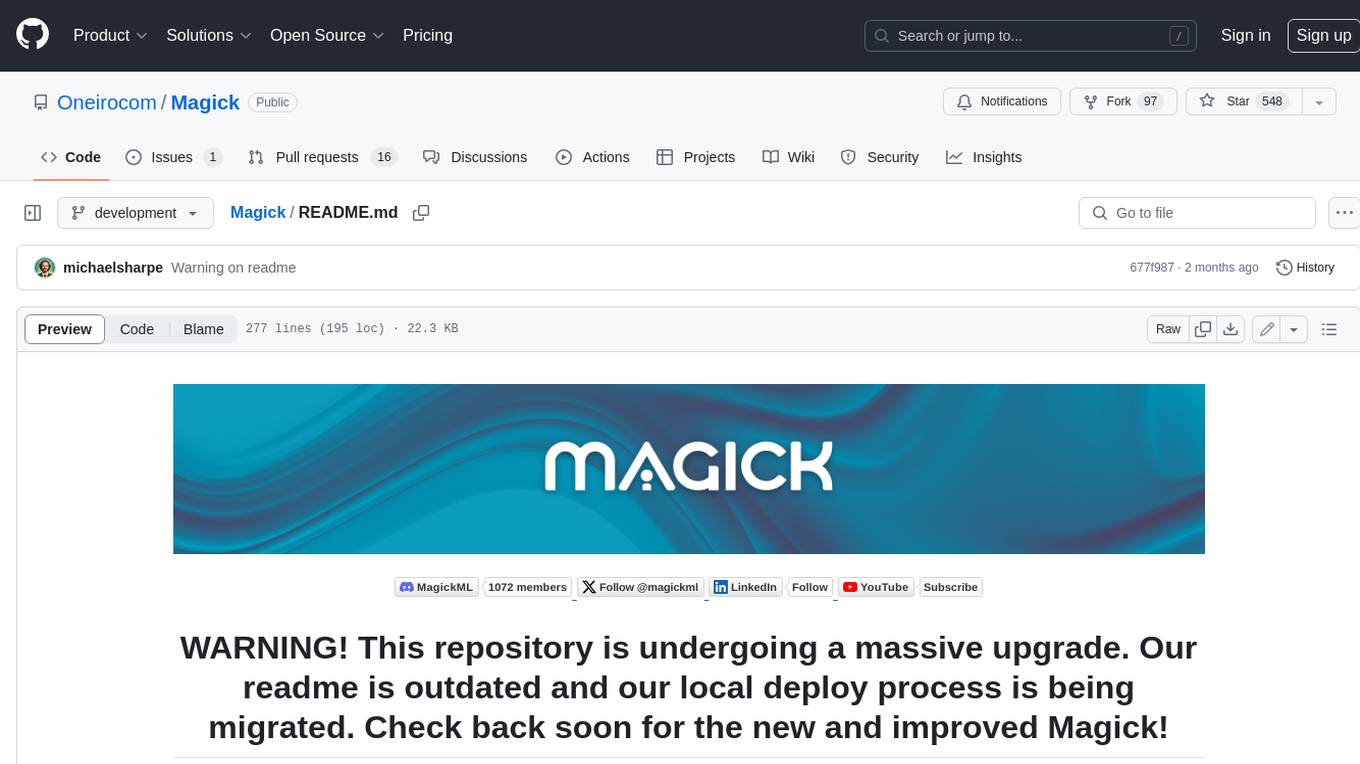
Magick
Magick is a groundbreaking visual AIDE (Artificial Intelligence Development Environment) for no-code data pipelines and multimodal agents. Magick can connect to other services and comes with nodes and templates well-suited for intelligent agents, chatbots, complex reasoning systems and realistic characters.
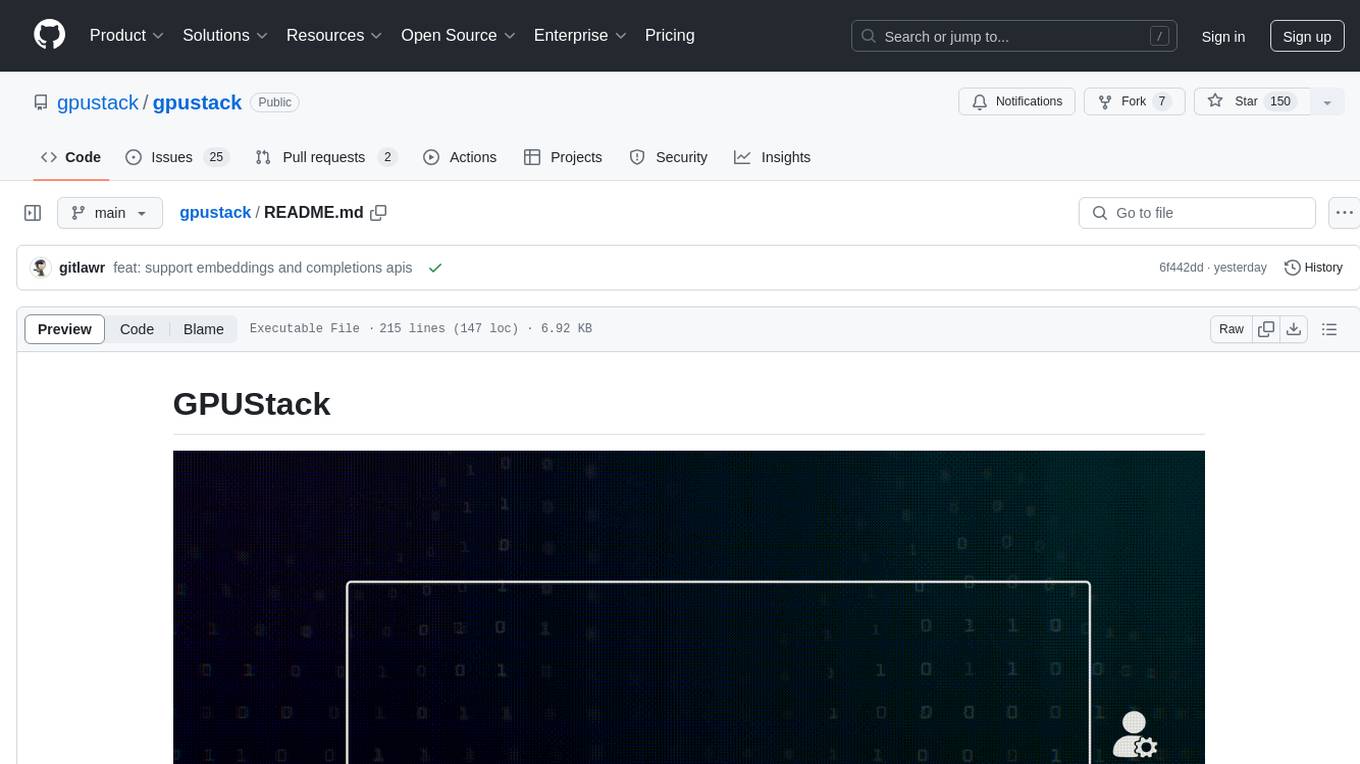
gpustack
GPUStack is an open-source GPU cluster manager designed for running large language models (LLMs). It supports a wide variety of hardware, scales with GPU inventory, offers lightweight Python package with minimal dependencies, provides OpenAI-compatible APIs, simplifies user and API key management, enables GPU metrics monitoring, and facilitates token usage and rate metrics tracking. The tool is suitable for managing GPU clusters efficiently and effectively.
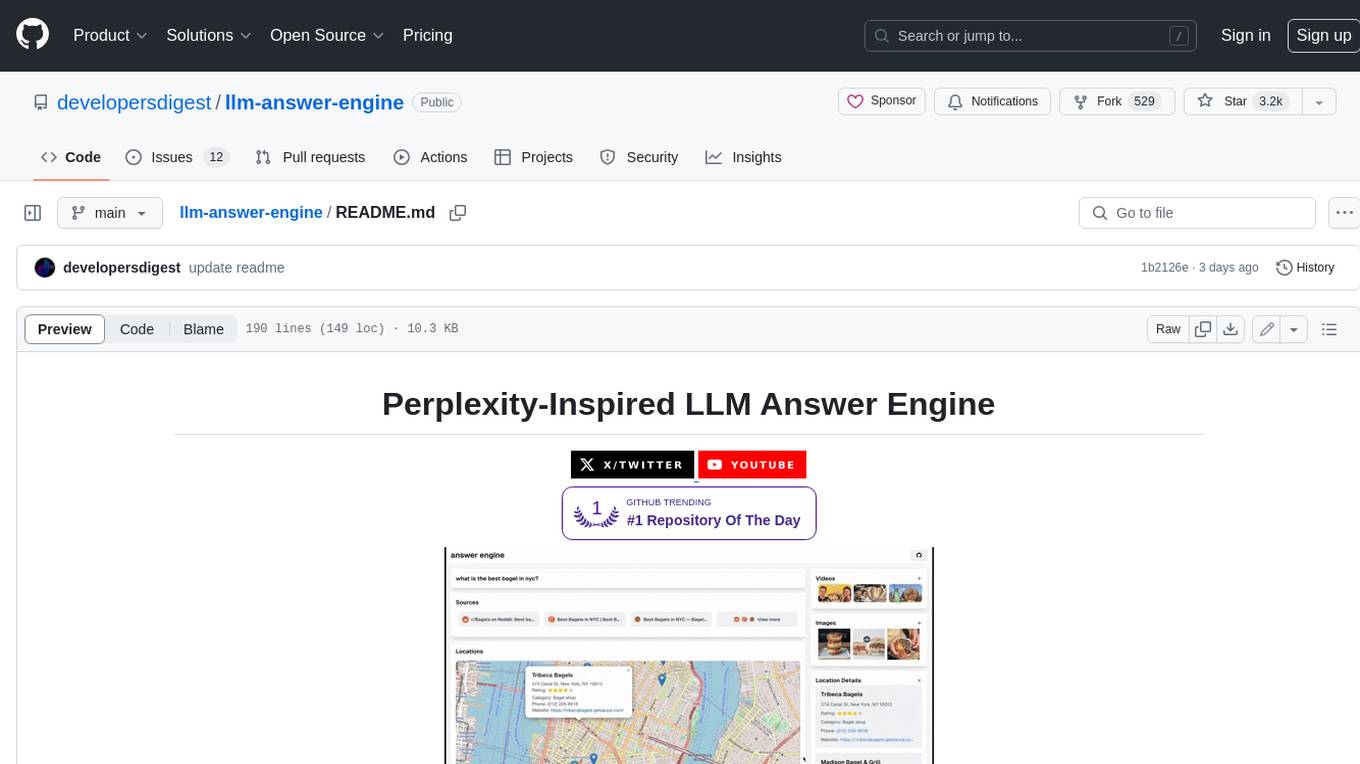
llm-answer-engine
This repository contains the code and instructions needed to build a sophisticated answer engine that leverages the capabilities of Groq, Mistral AI's Mixtral, Langchain.JS, Brave Search, Serper API, and OpenAI. Designed to efficiently return sources, answers, images, videos, and follow-up questions based on user queries, this project is an ideal starting point for developers interested in natural language processing and search technologies.
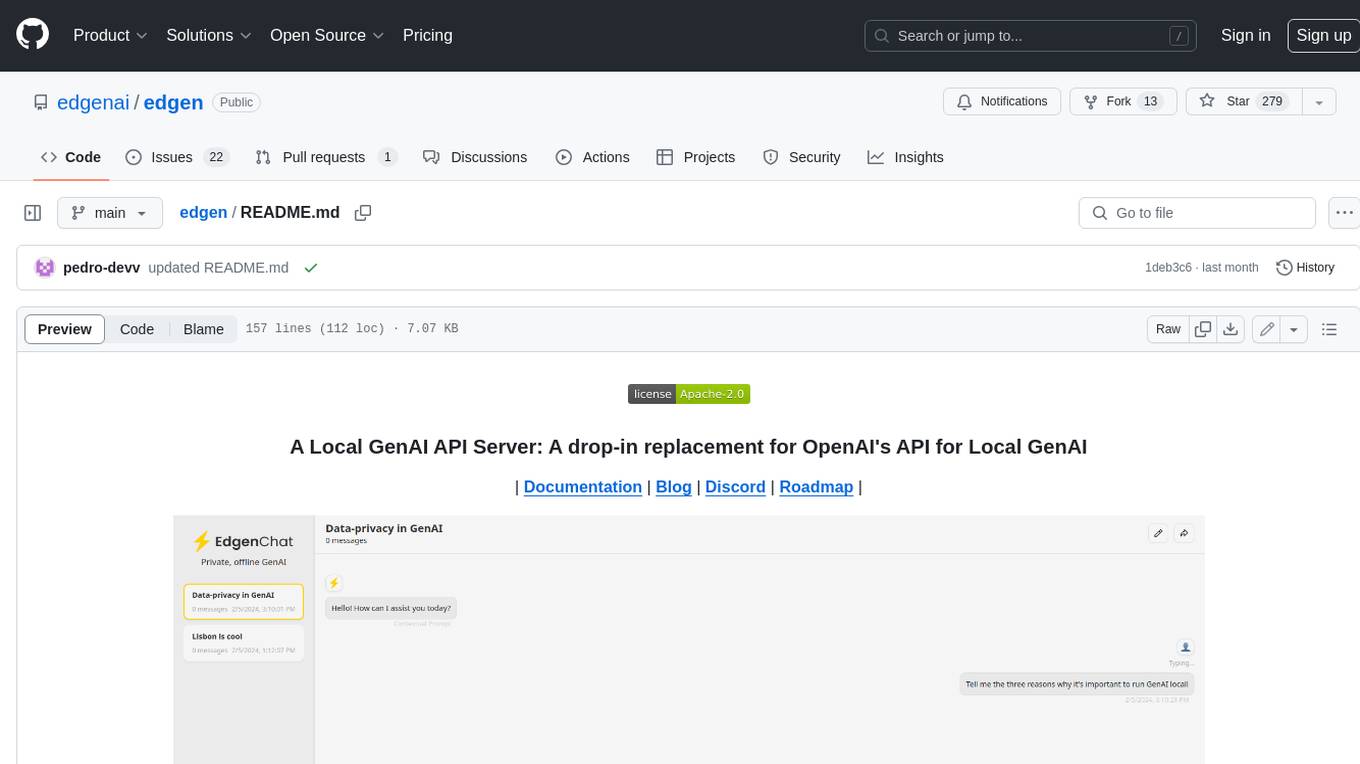
edgen
Edgen is a local GenAI API server that serves as a drop-in replacement for OpenAI's API. It provides multi-endpoint support for chat completions and speech-to-text, is model agnostic, offers optimized inference, and features model caching. Built in Rust, Edgen is natively compiled for Windows, MacOS, and Linux, eliminating the need for Docker. It allows users to utilize GenAI locally on their devices for free and with data privacy. With features like session caching, GPU support, and support for various endpoints, Edgen offers a scalable, reliable, and cost-effective solution for running GenAI applications locally.
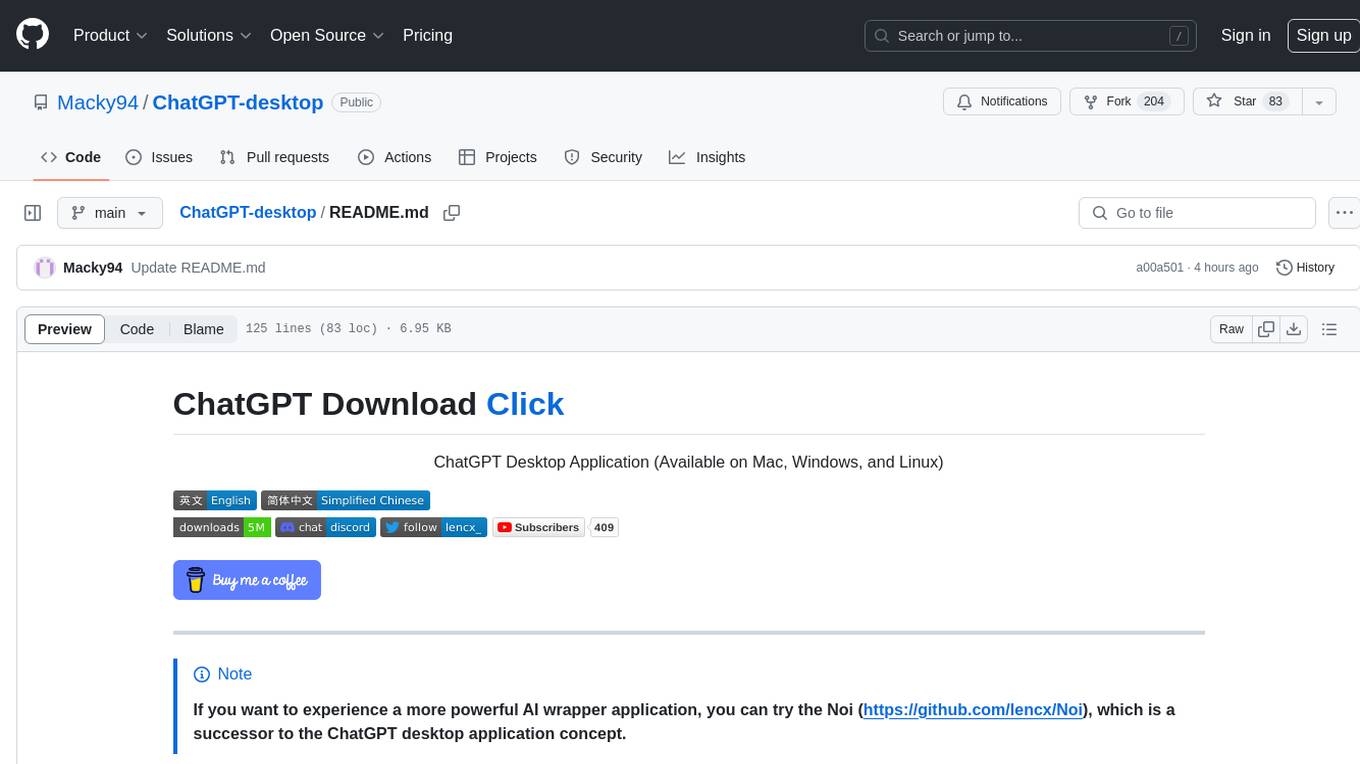
ChatGPT-desktop
ChatGPT Desktop Application is a multi-platform tool that provides a powerful AI wrapper for generating text. It offers features like text-to-speech, exporting chat history in various formats, automatic application upgrades, system tray hover window, support for slash commands, customization of global shortcuts, and pop-up search. The application is built using Tauri and aims to enhance user experience by simplifying text generation tasks. It is available for Mac, Windows, and Linux, and is designed for personal learning and research purposes.
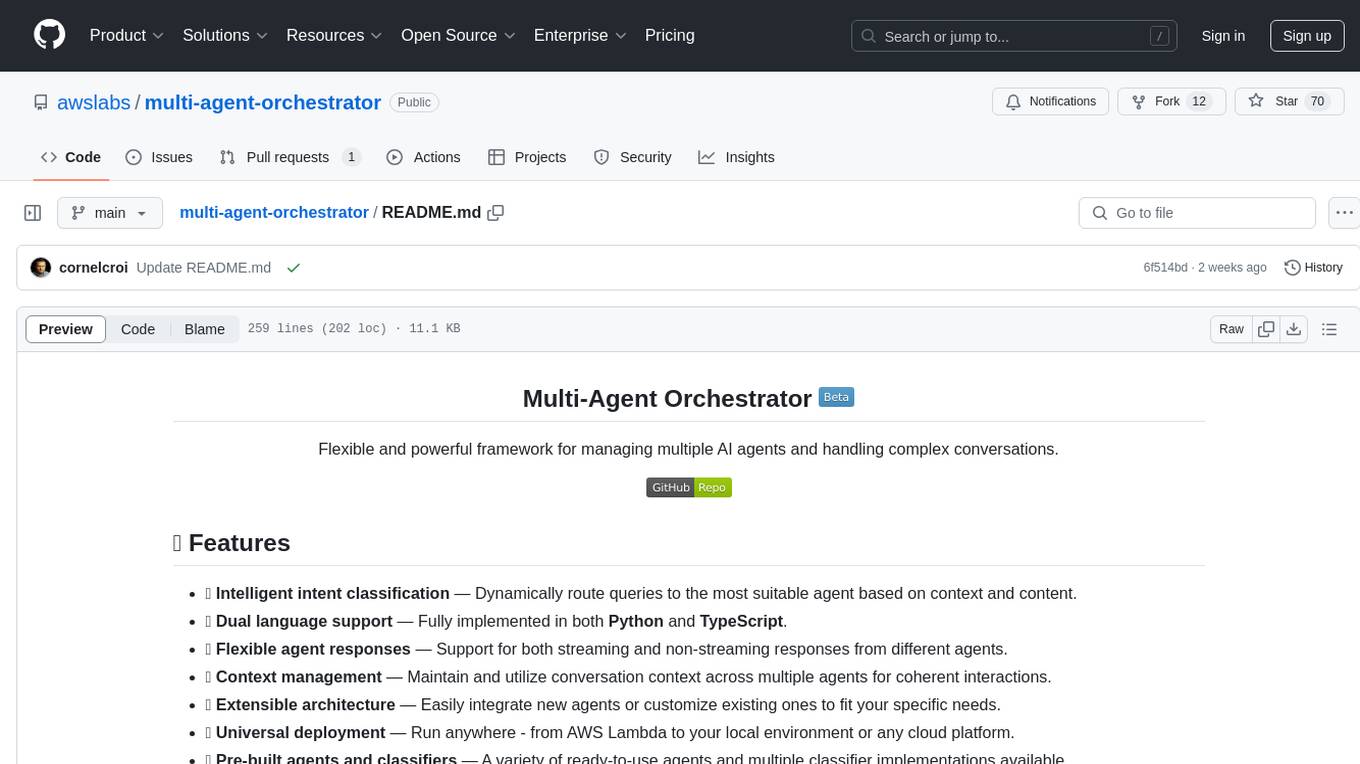
multi-agent-orchestrator
Multi-Agent Orchestrator is a flexible and powerful framework for managing multiple AI agents and handling complex conversations. It intelligently routes queries to the most suitable agent based on context and content, supports dual language implementation in Python and TypeScript, offers flexible agent responses, context management across agents, extensible architecture for customization, universal deployment options, and pre-built agents and classifiers. It is suitable for various applications, from simple chatbots to sophisticated AI systems, accommodating diverse requirements and scaling efficiently.
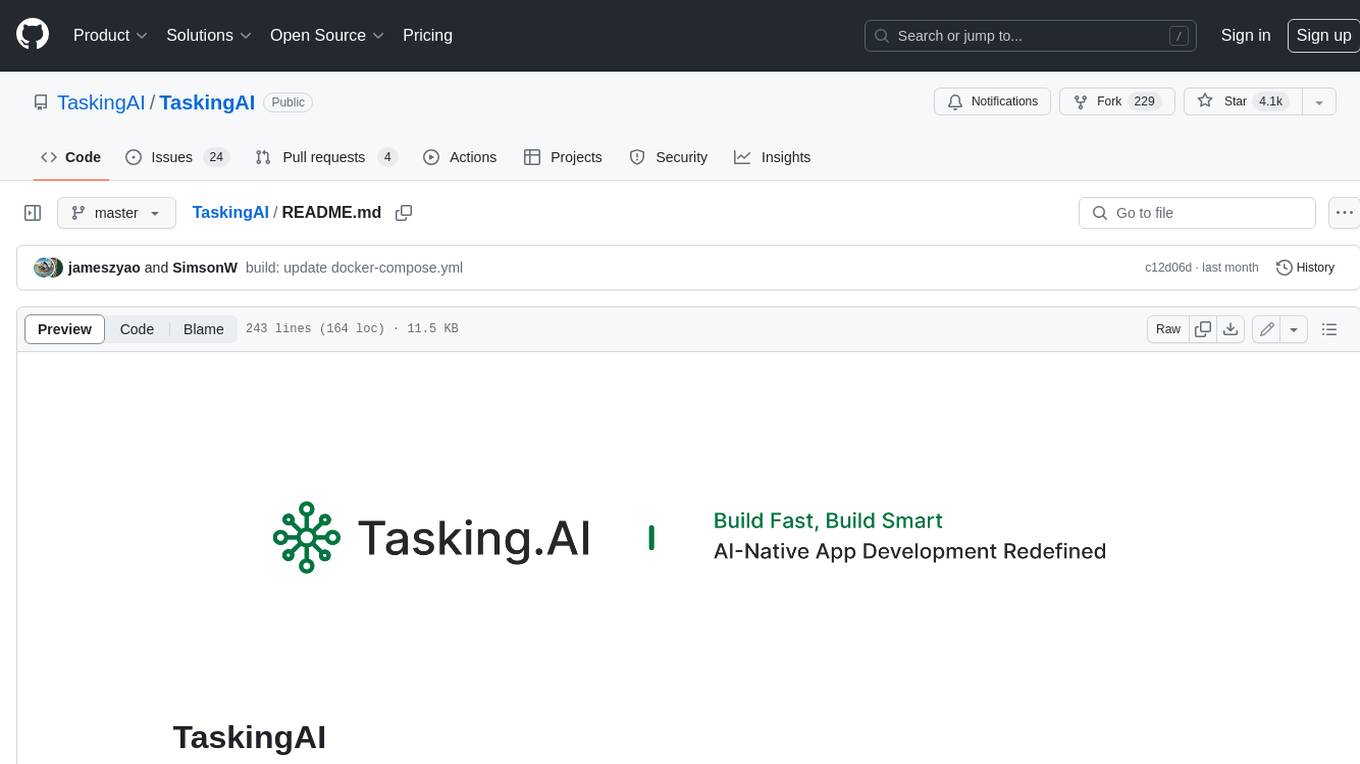
TaskingAI
TaskingAI brings Firebase's simplicity to **AI-native app development**. The platform enables the creation of GPTs-like multi-tenant applications using a wide range of LLMs from various providers. It features distinct, modular functions such as Inference, Retrieval, Assistant, and Tool, seamlessly integrated to enhance the development process. TaskingAIβs cohesive design ensures an efficient, intelligent, and user-friendly experience in AI application development.
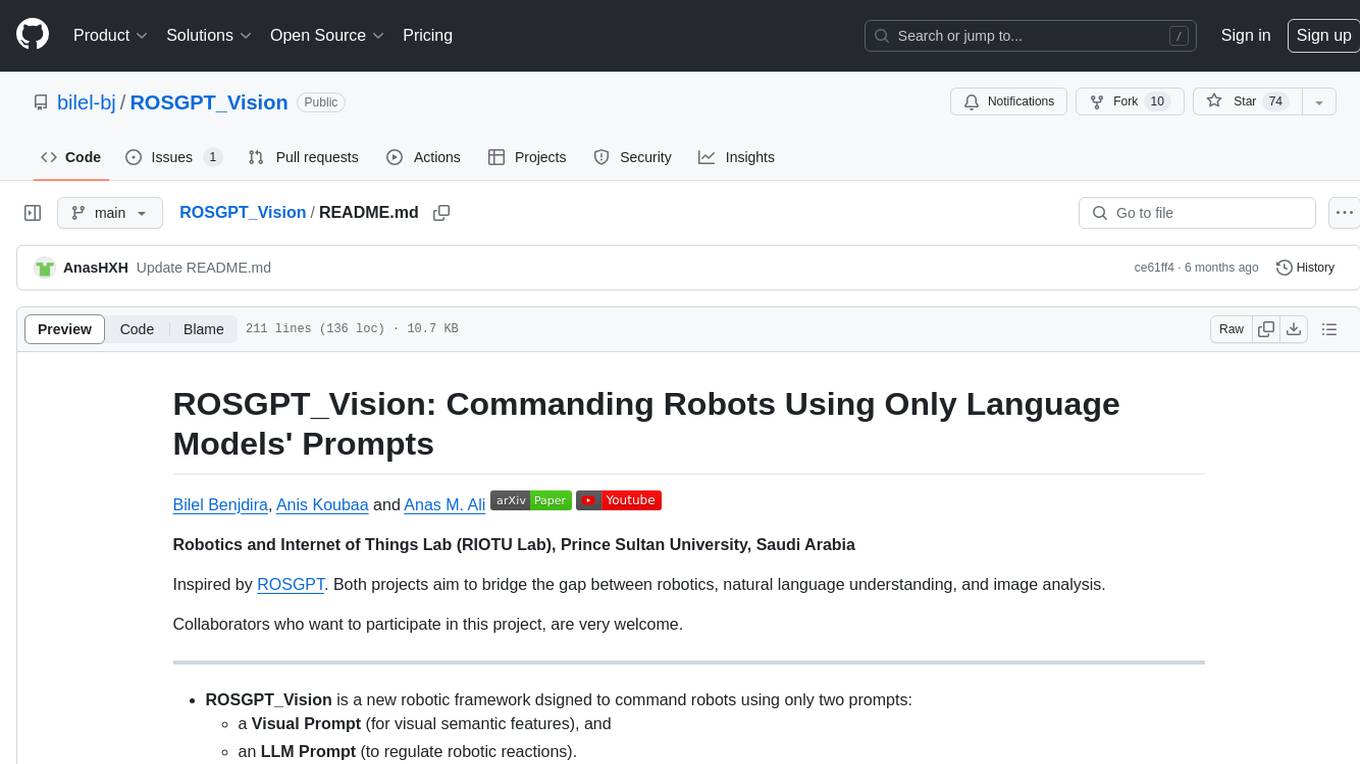
ROSGPT_Vision
ROSGPT_Vision is a new robotic framework designed to command robots using only two prompts: a Visual Prompt for visual semantic features and an LLM Prompt to regulate robotic reactions. It is based on the Prompting Robotic Modalities (PRM) design pattern and is used to develop CarMate, a robotic application for monitoring driver distractions and providing real-time vocal notifications. The framework leverages state-of-the-art language models to facilitate advanced reasoning about image data and offers a unified platform for robots to perceive, interpret, and interact with visual data through natural language. LangChain is used for easy customization of prompts, and the implementation includes the CarMate application for driver monitoring and assistance.
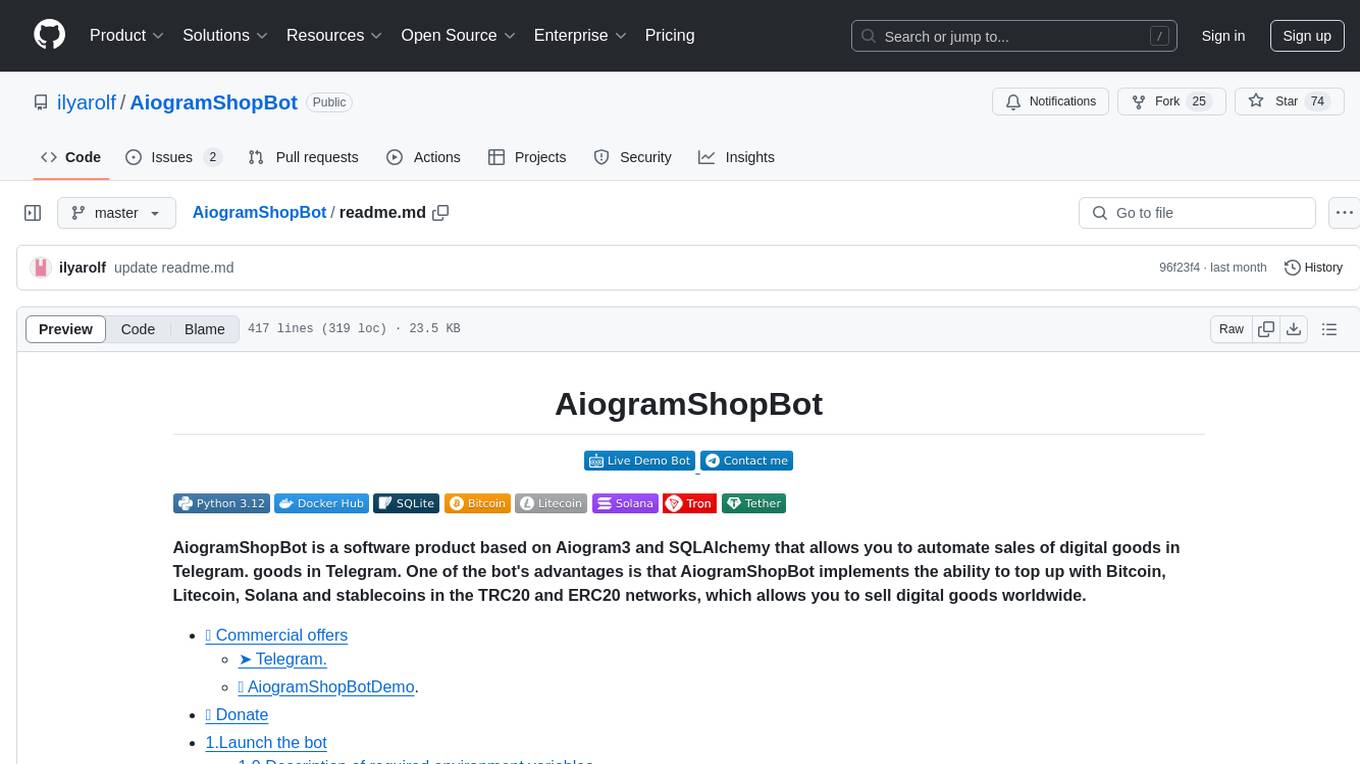
AiogramShopBot
AiogramShopBot is a software product based on Aiogram3 and SQLAlchemy that allows you to automate sales of digital goods in Telegram. One of the bot's advantages is that AiogramShopBot implements the ability to top up with Bitcoin, Litecoin, Solana and stablecoins in the TRC20 and ERC20 networks, which allows you to sell digital goods worldwide. The bot provides features for user registration, balance top-up, purchase of goods, purchase history, admin functionalities like announcements, inventory management, user management, analytics & reports, and multibot functionality. It supports encryption via SQLCipher, multiple cryptocurrencies, and offers a user-friendly interface for managing sales and transactions.
For similar tasks
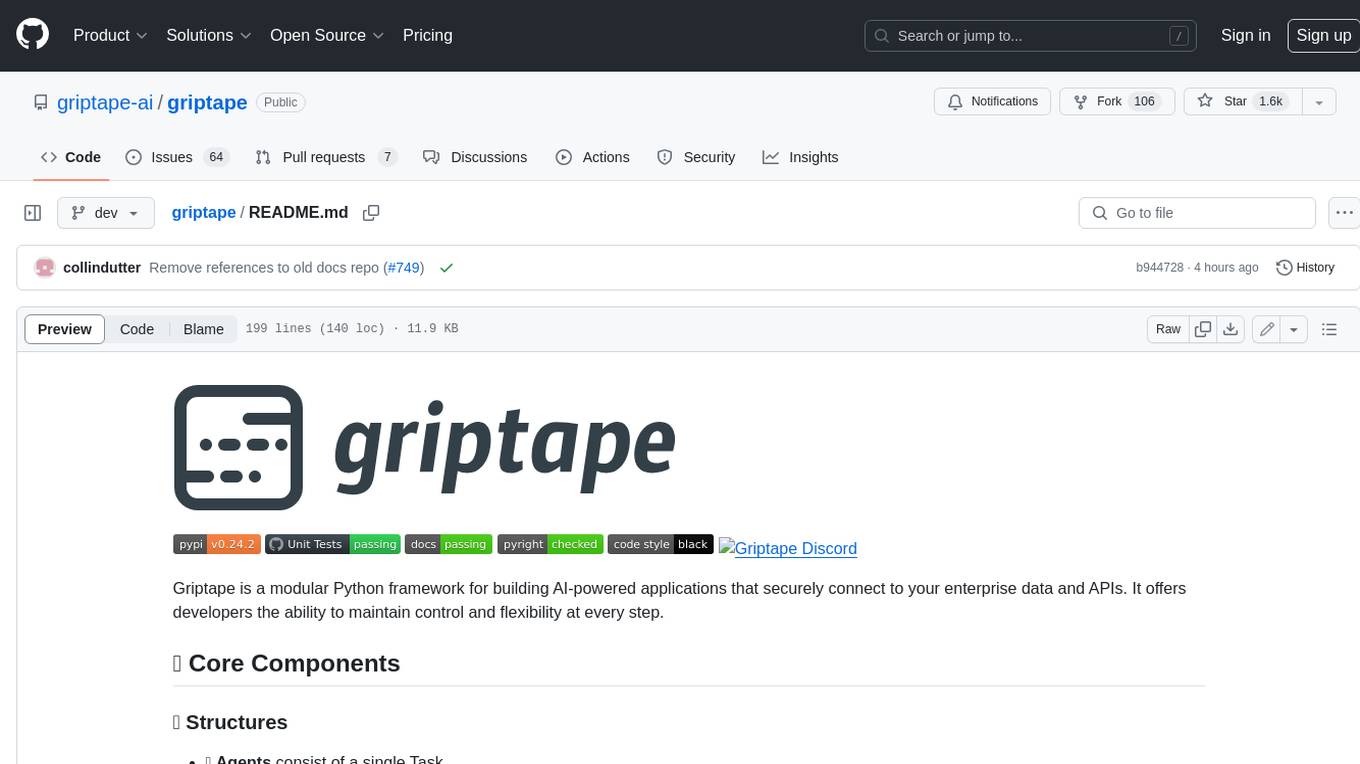
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.
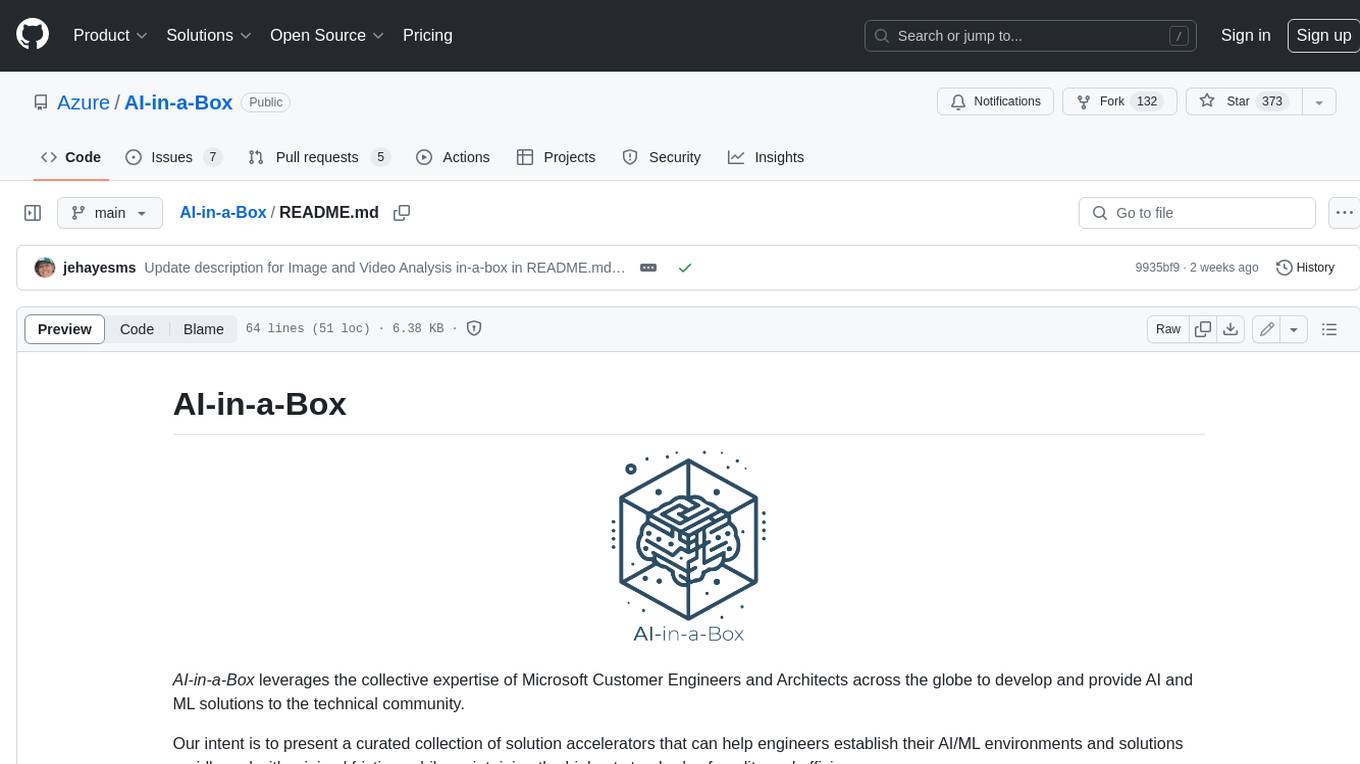
AI-in-a-Box
AI-in-a-Box is a curated collection of solution accelerators that can help engineers establish their AI/ML environments and solutions rapidly and with minimal friction, while maintaining the highest standards of quality and efficiency. It provides essential guidance on the responsible use of AI and LLM technologies, specific security guidance for Generative AI (GenAI) applications, and best practices for scaling OpenAI applications within Azure. The available accelerators include: Azure ML Operationalization in-a-box, Edge AI in-a-box, Doc Intelligence in-a-box, Image and Video Analysis in-a-box, Cognitive Services Landing Zone in-a-box, Semantic Kernel Bot in-a-box, NLP to SQL in-a-box, Assistants API in-a-box, and Assistants API Bot in-a-box.
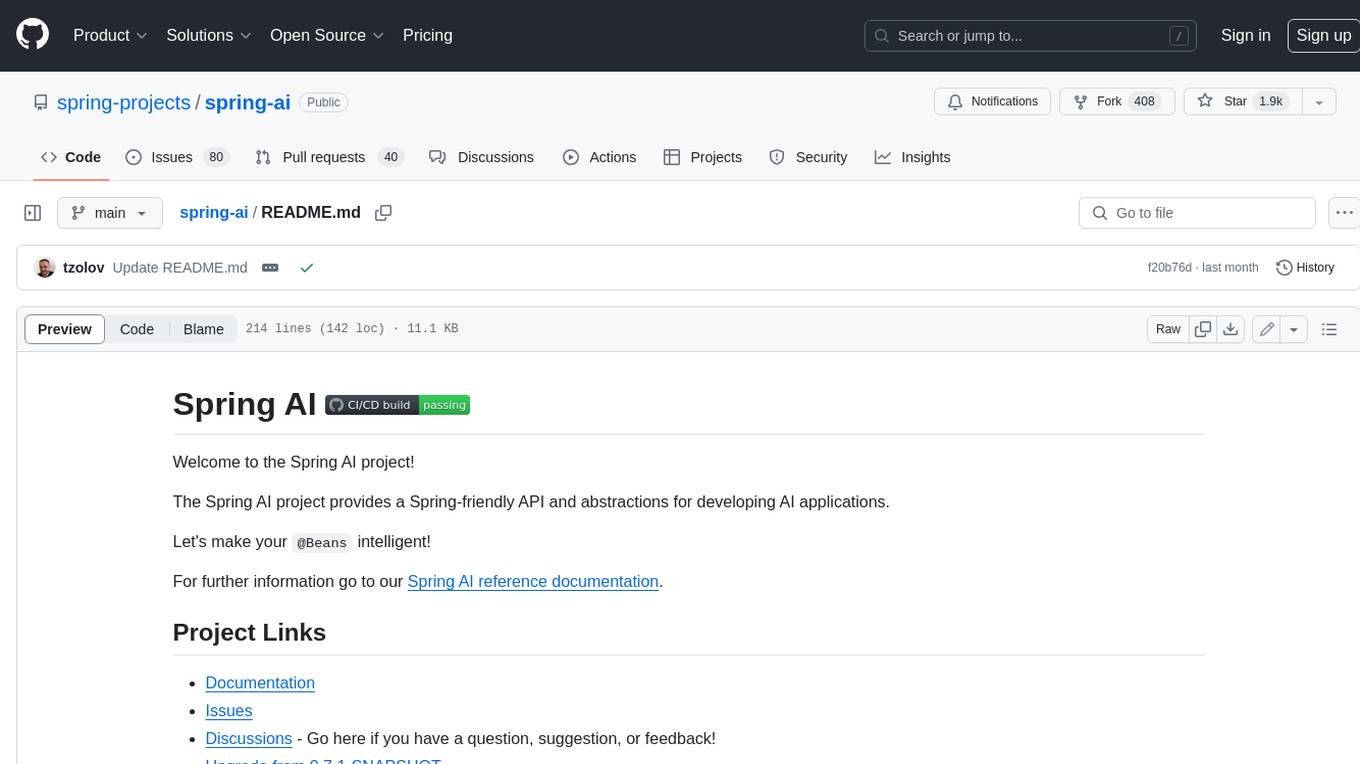
spring-ai
The Spring AI project provides a Spring-friendly API and abstractions for developing AI applications. It offers a portable client API for interacting with generative AI models, enabling developers to easily swap out implementations and access various models like OpenAI, Azure OpenAI, and HuggingFace. Spring AI also supports prompt engineering, providing classes and interfaces for creating and parsing prompts, as well as incorporating proprietary data into generative AI without retraining the model. This is achieved through Retrieval Augmented Generation (RAG), which involves extracting, transforming, and loading data into a vector database for use by AI models. Spring AI's VectorStore abstraction allows for seamless transitions between different vector database implementations.
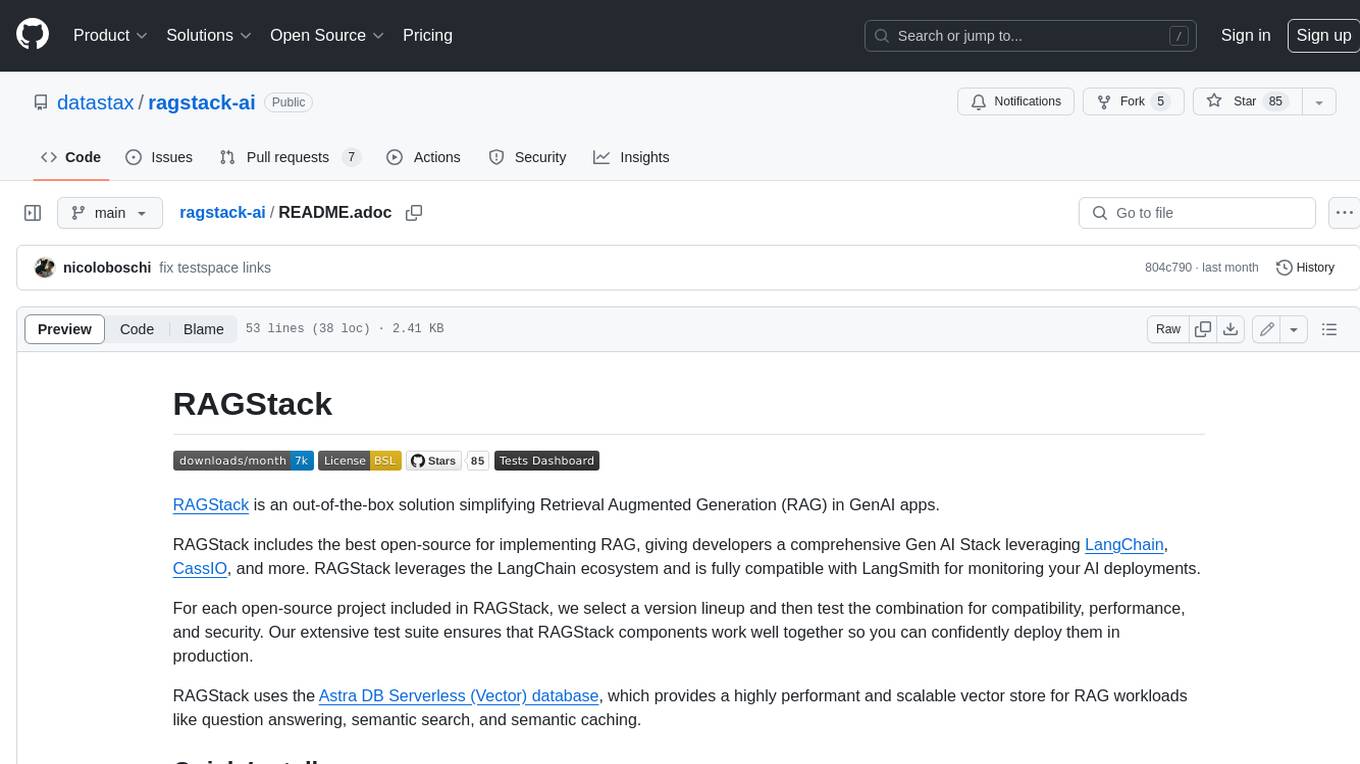
ragstack-ai
RAGStack is an out-of-the-box solution simplifying Retrieval Augmented Generation (RAG) in GenAI apps. RAGStack includes the best open-source for implementing RAG, giving developers a comprehensive Gen AI Stack leveraging LangChain, CassIO, and more. RAGStack leverages the LangChain ecosystem and is fully compatible with LangSmith for monitoring your AI deployments.
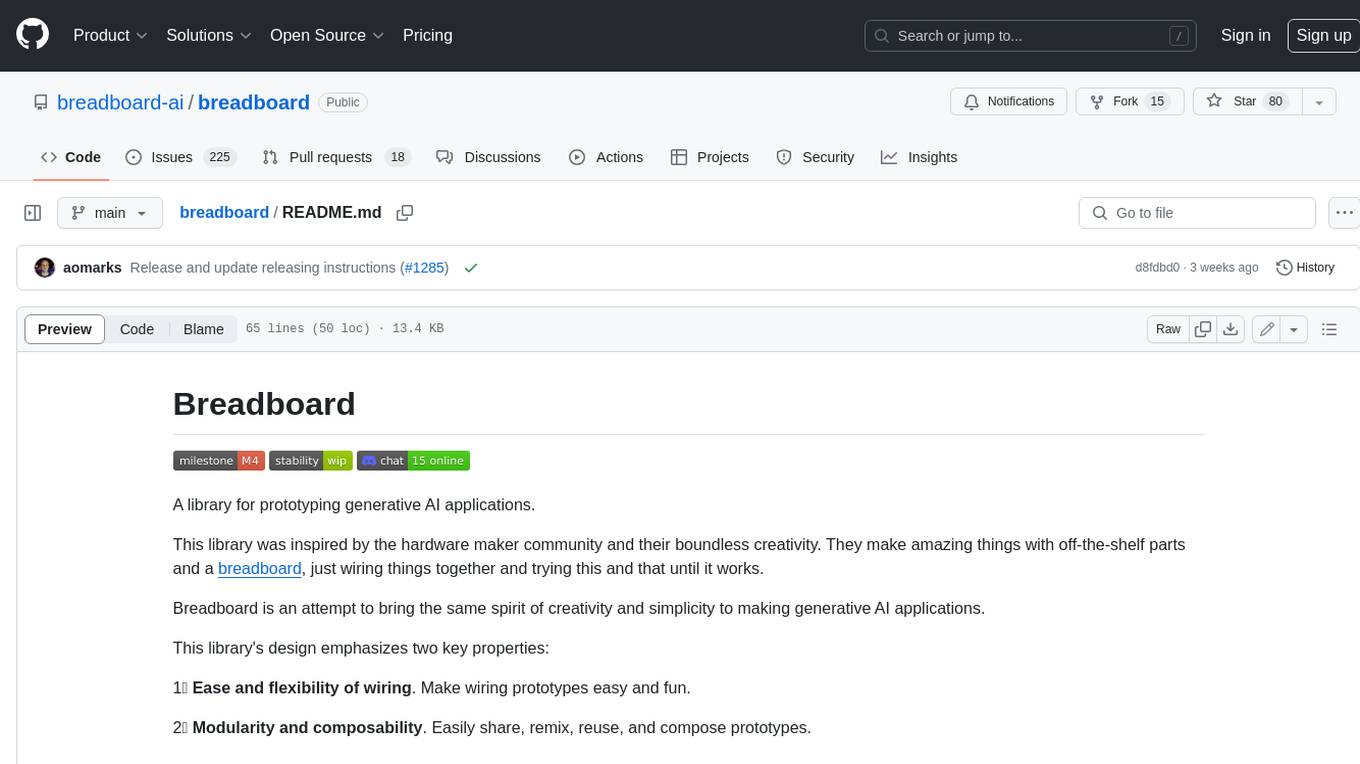
breadboard
Breadboard is a library for prototyping generative AI applications. It is inspired by the hardware maker community and their boundless creativity. Breadboard makes it easy to wire prototypes and share, remix, reuse, and compose them. The library emphasizes ease and flexibility of wiring, as well as modularity and composability.
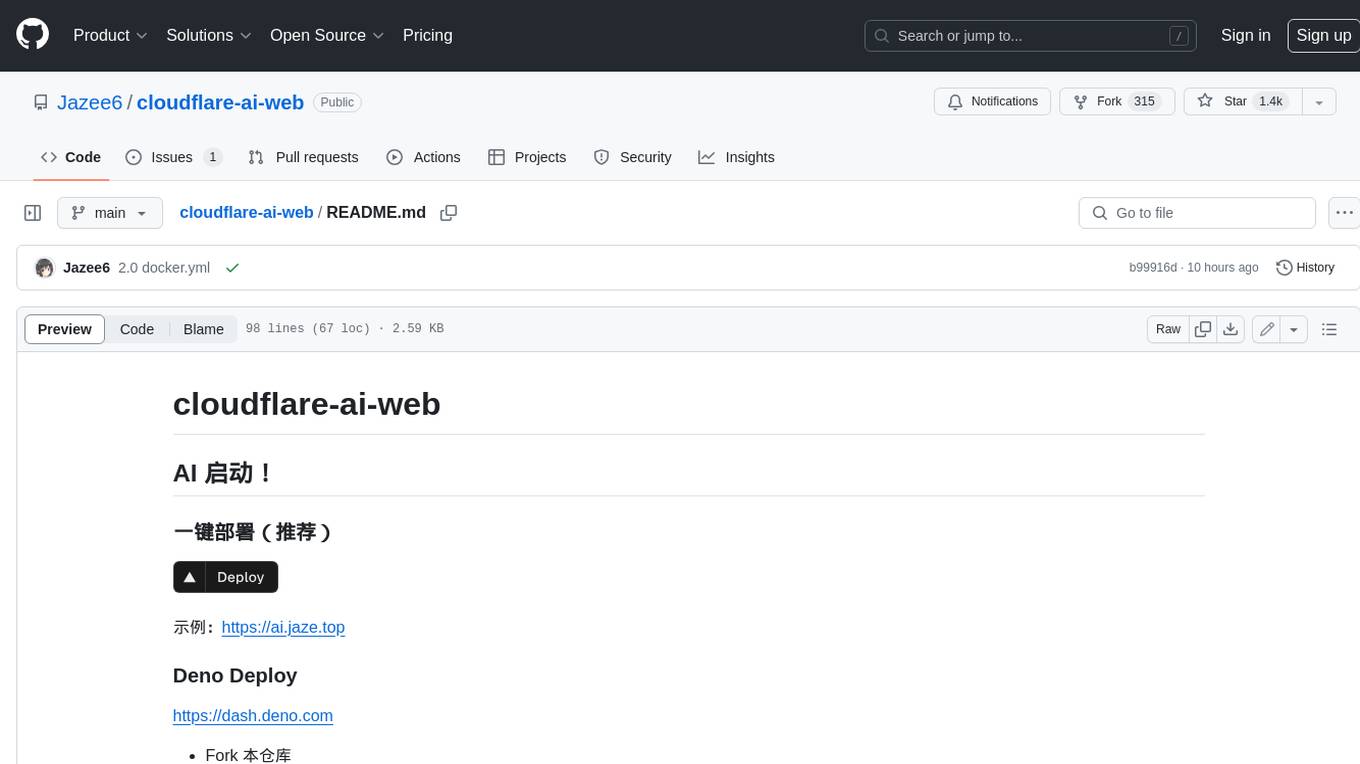
cloudflare-ai-web
Cloudflare-ai-web is a lightweight and easy-to-use tool that allows you to quickly deploy a multi-modal AI platform using Cloudflare Workers AI. It supports serverless deployment, password protection, and local storage of chat logs. With a size of only ~638 kB gzip, it is a great option for building AI-powered applications without the need for a dedicated server.
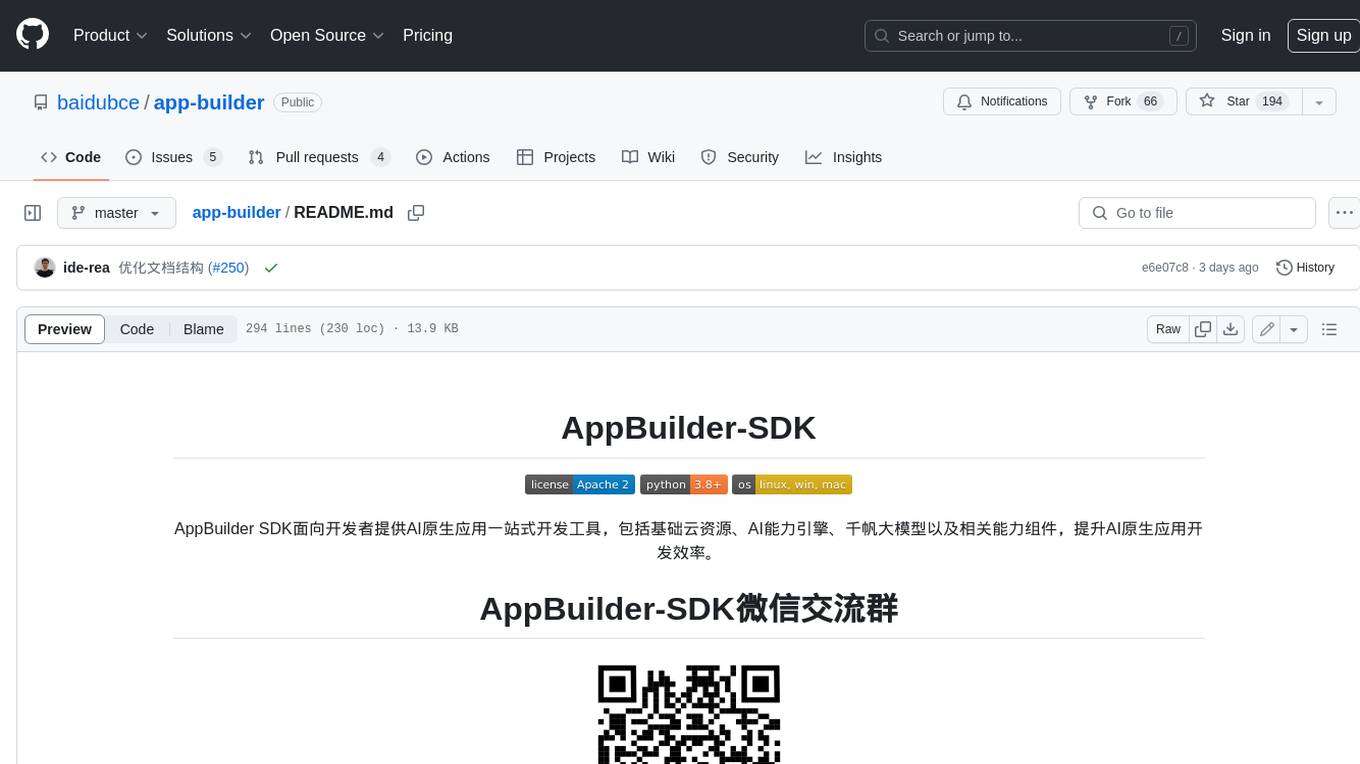
app-builder
AppBuilder SDK is a one-stop development tool for AI native applications, providing basic cloud resources, AI capability engine, Qianfan large model, and related capability components to improve the development efficiency of AI native applications.
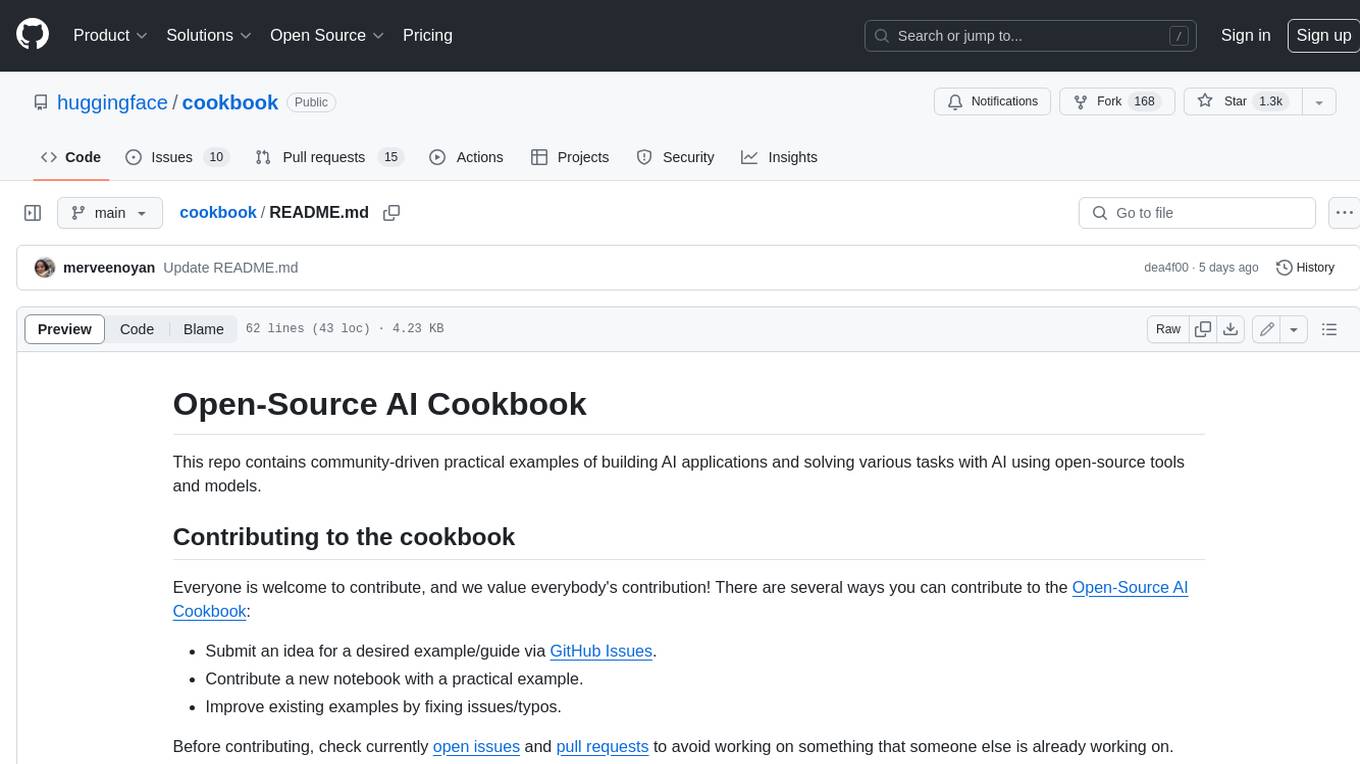
cookbook
This repository contains community-driven practical examples of building AI applications and solving various tasks with AI using open-source tools and models. Everyone is welcome to contribute, and we value everybody's contribution! There are several ways you can contribute to the Open-Source AI Cookbook: Submit an idea for a desired example/guide via GitHub Issues. Contribute a new notebook with a practical example. Improve existing examples by fixing issues/typos. Before contributing, check currently open issues and pull requests to avoid working on something that someone else is already working on.
For similar jobs
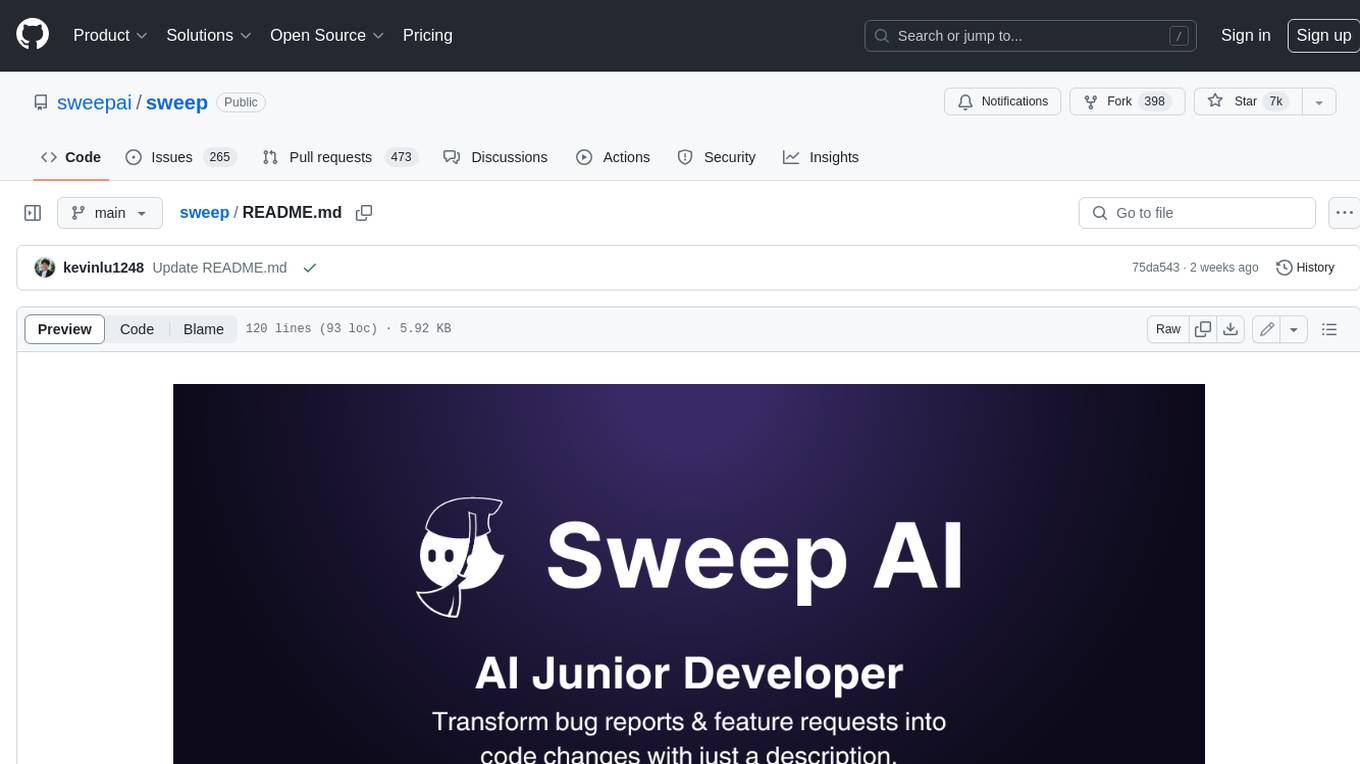
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
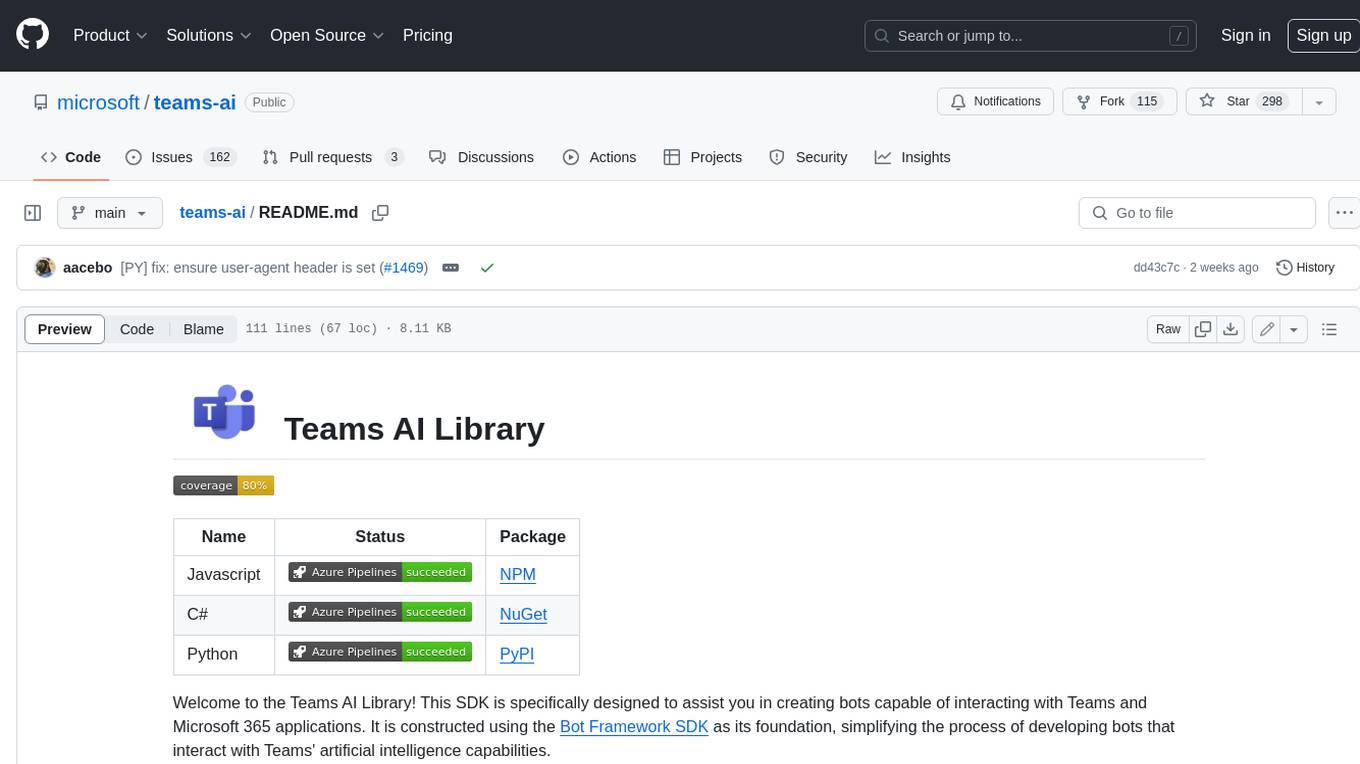
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
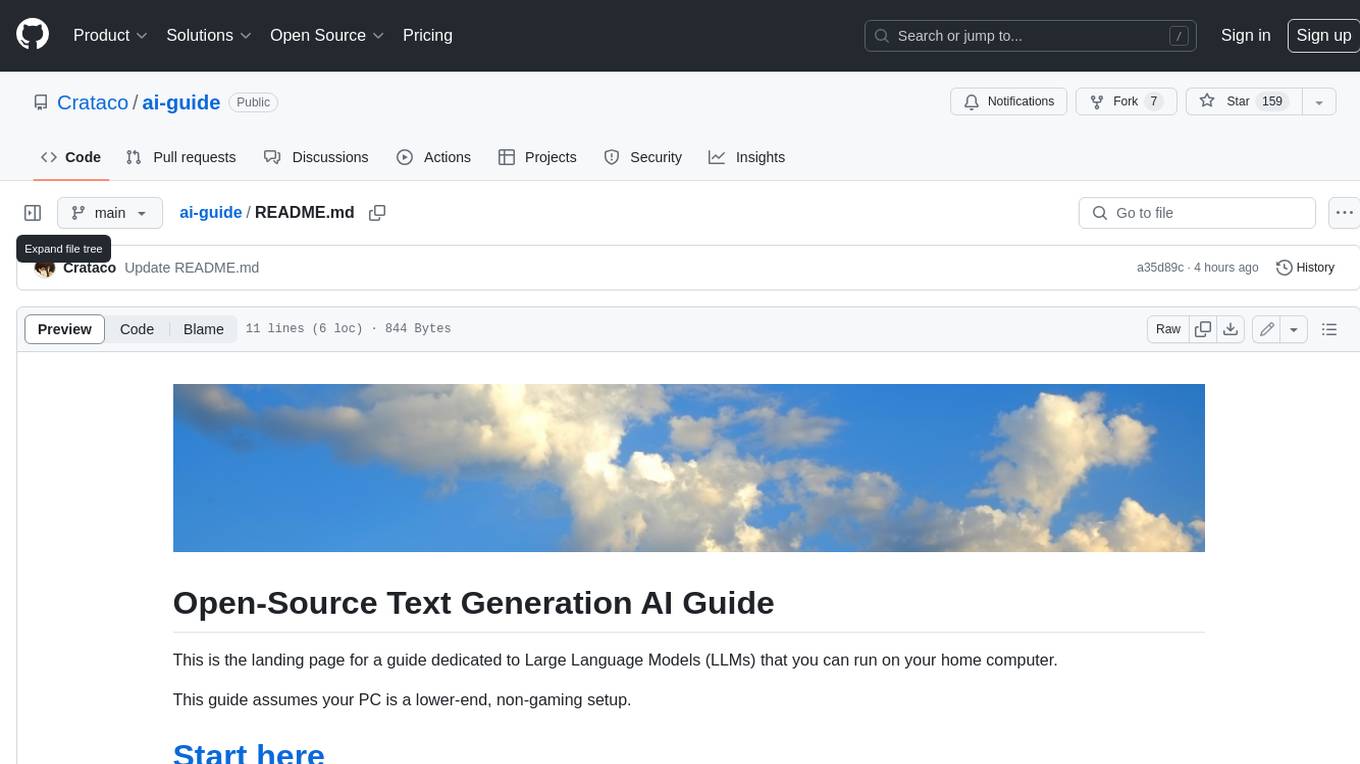
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
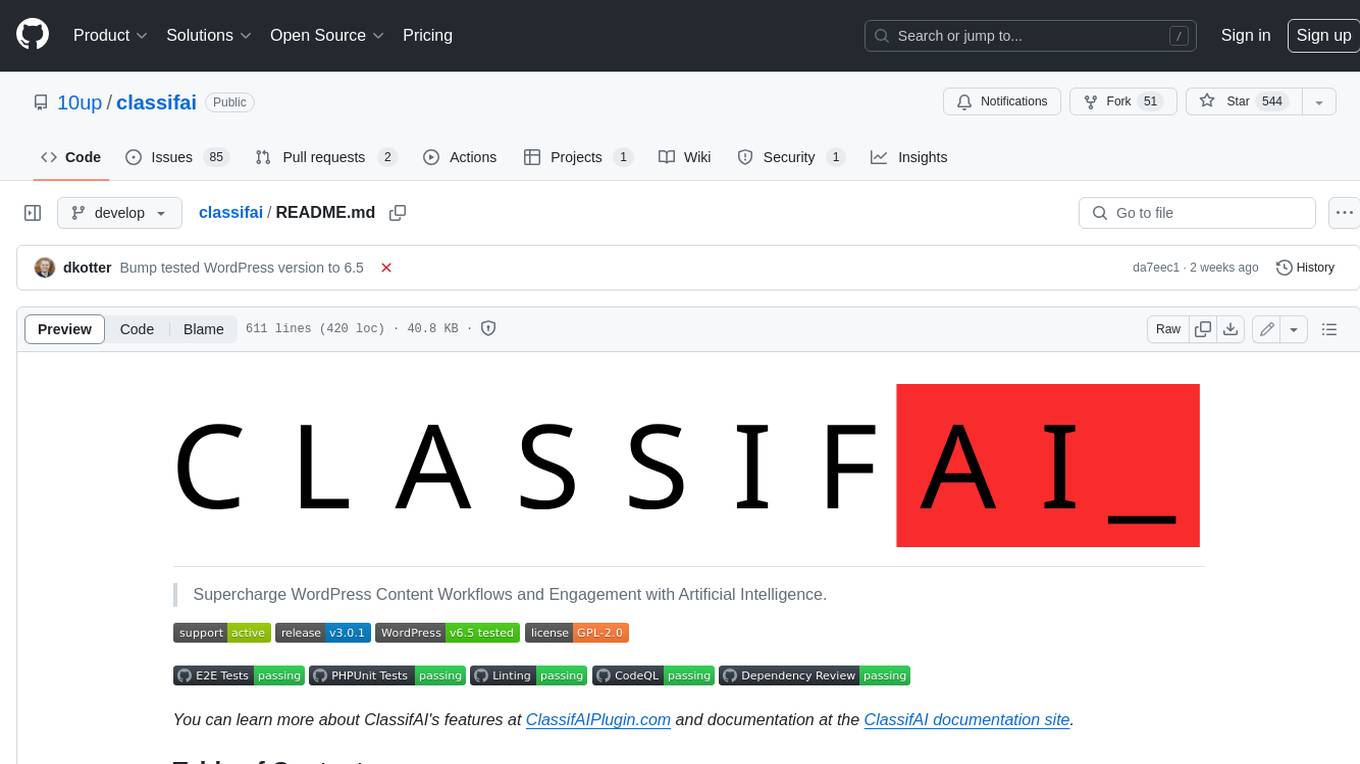
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
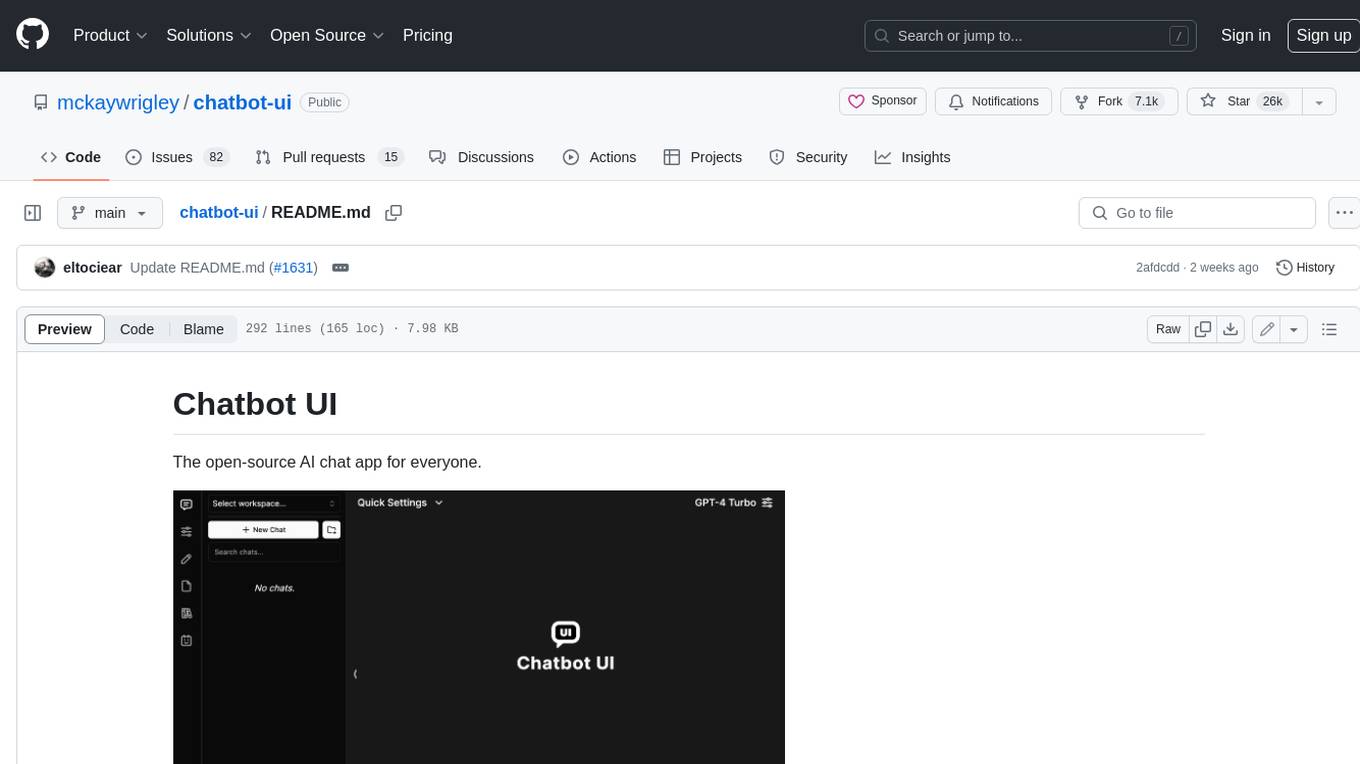
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
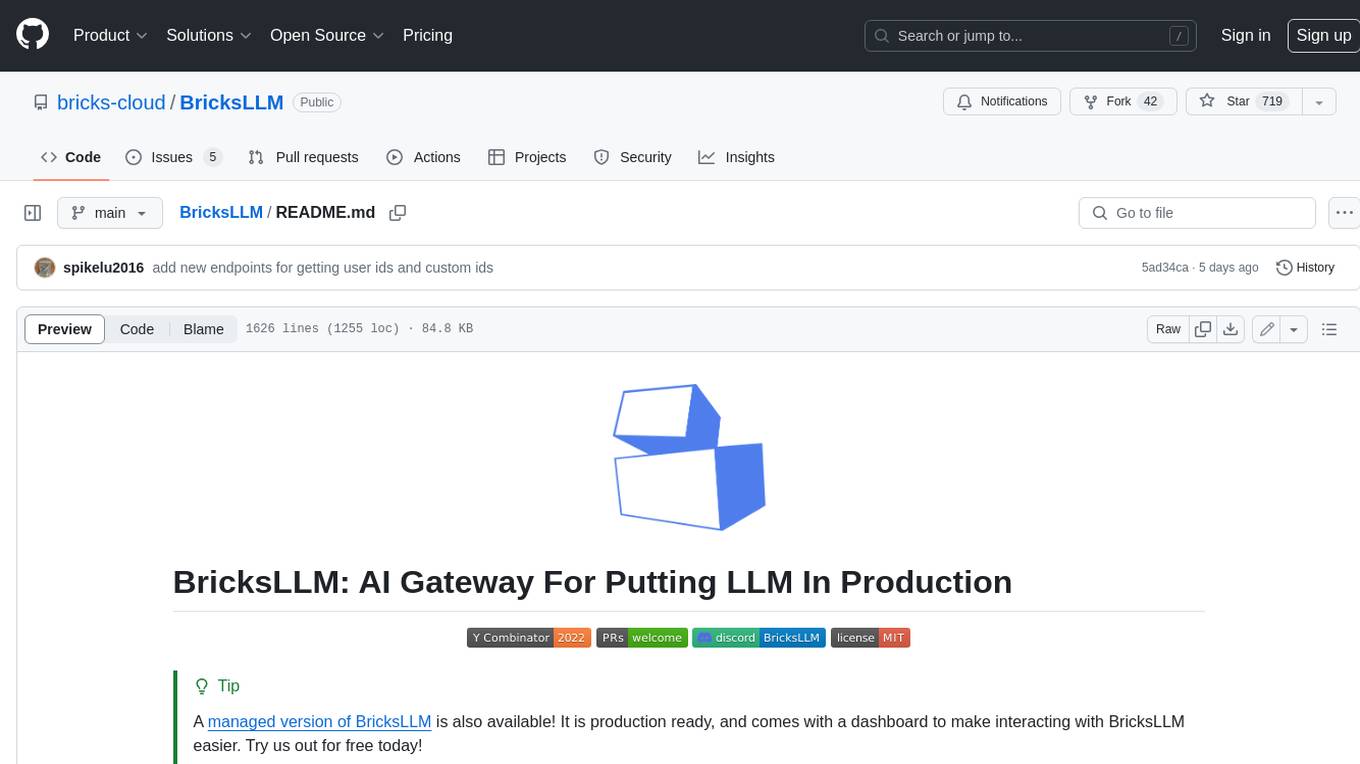
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
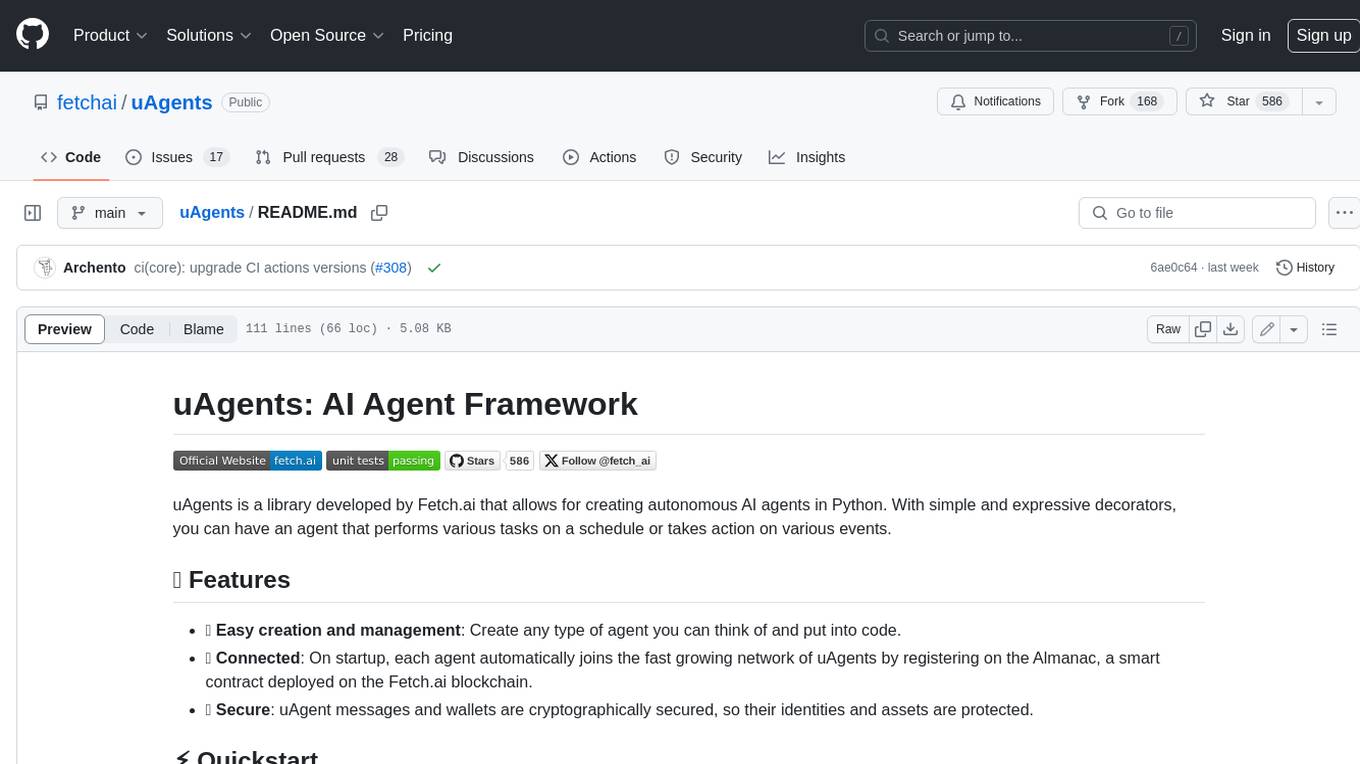
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
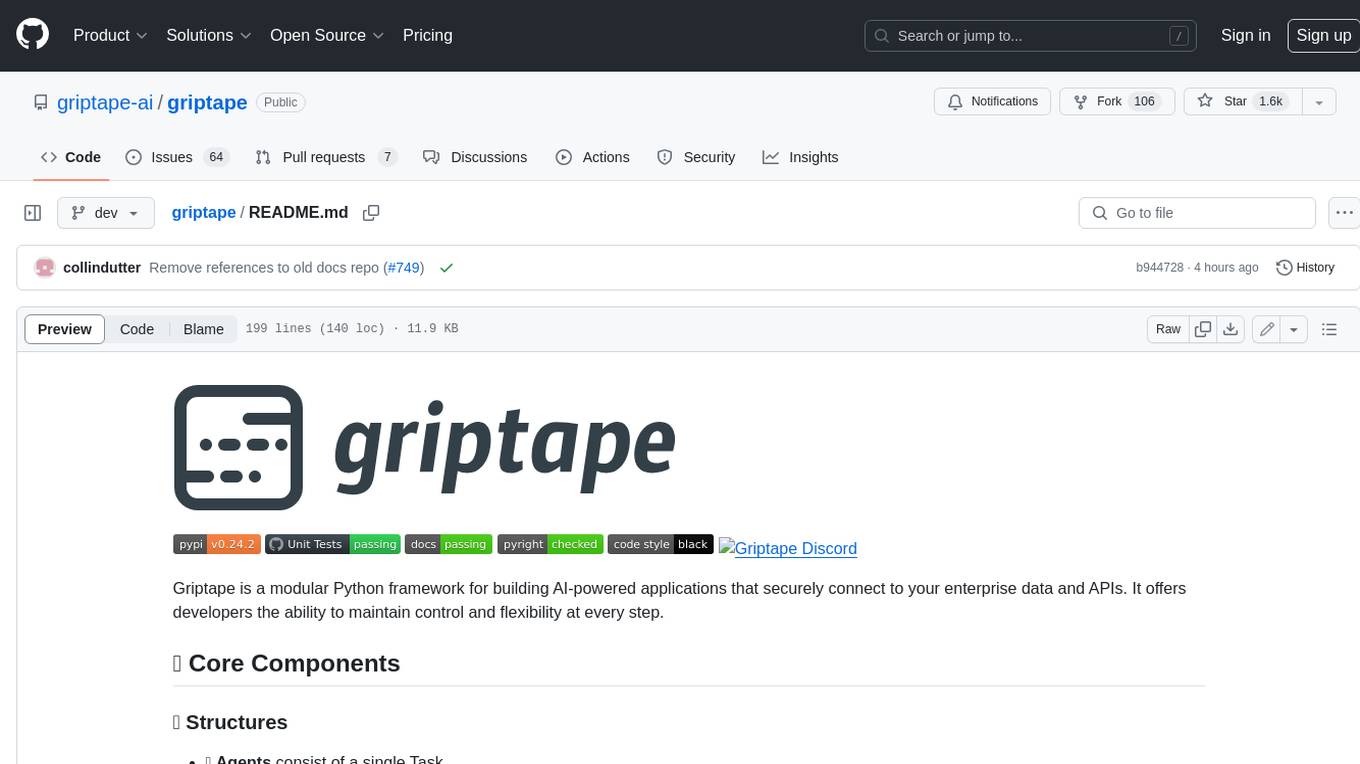
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.