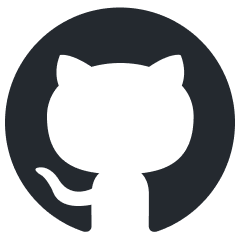
CEO
CEO is an intuitive and modular AI agent framework for task automation.
Stars: 127
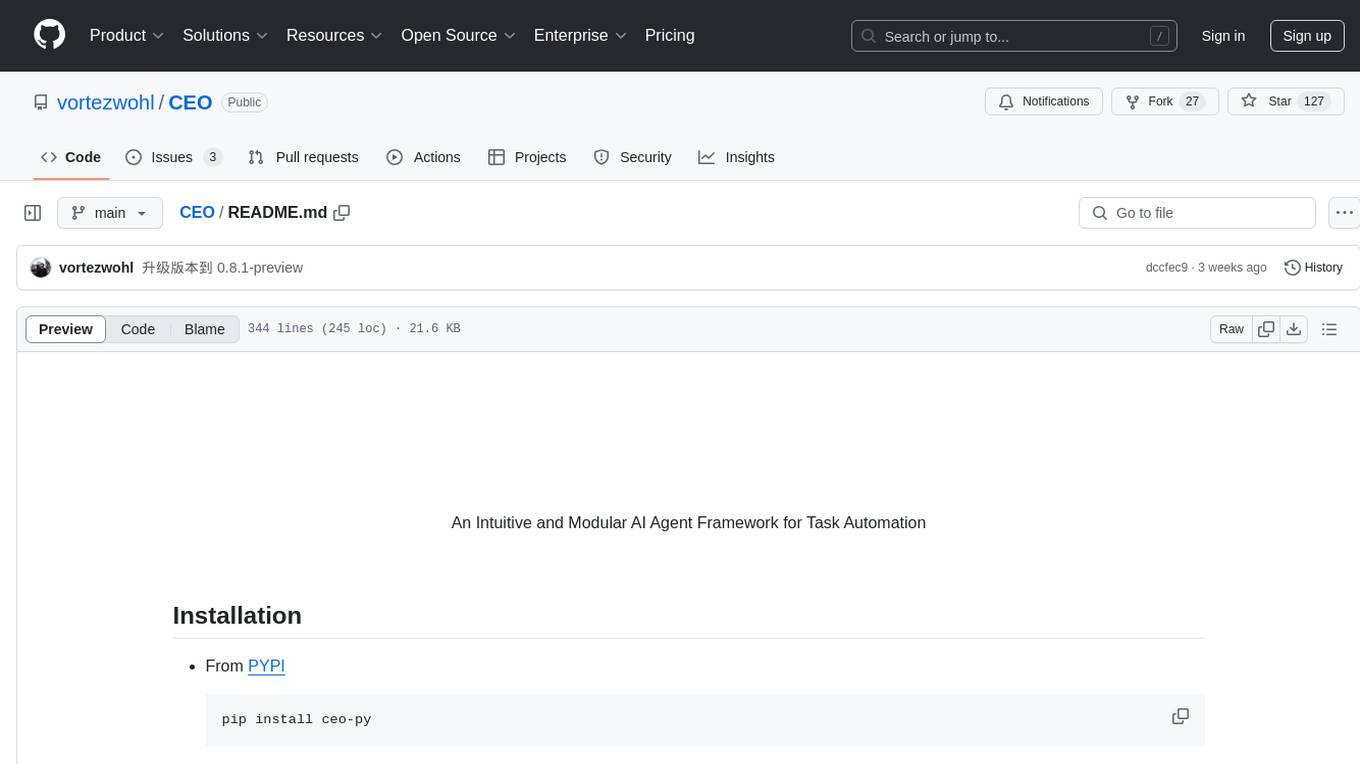
CEO is an intuitive and modular AI agent framework designed for task automation. It provides a flexible environment for building agents with specific abilities and personalities, allowing users to assign tasks and interact with the agents to automate various processes. The framework supports multi-agent collaboration scenarios and offers functionalities like instantiating agents, granting abilities, assigning queries, and executing tasks. Users can customize agent personalities and define specific abilities using decorators, making it easy to create complex automation workflows.
README:
-
From PYPI
pip install ceo-py
-
From Github
Download .whl first then run
pip install ./ceo_py-x.x.x-py3-none-any.whl
If you are incorporating the CEO
framework into your research, please remember to properly cite it to acknowledge its contribution to your work.
Если вы интегрируете фреймворк CEO
в своё исследование, пожалуйста, не забудьте правильно сослаться на него, указывая его вклад в вашу работу.
もしあなたが研究に CEO
フレームワークを組み入れているなら、その貢献を認めるために適切に引用することを忘れないでください.
如果您正在將 CEO
框架整合到您的研究中,請記得正確引用它,以聲明它對您工作的貢獻.
@software {CEO,
author = {Zihao Wu},
title = {CEO: An Intuitive and Modular AI Agent Framework for Task Automation},
publisher = {Github},
howpublished = {\url{https://github.com/vortezwohl/CEO}},
year = {2024},
date = {2024-10-25}
}
To start building your own agent, follow the steps listed.
-
set environmental variable
OPENAI_API_KEY
# .env OPENAI_API_KEY=sk-...
-
bring in SDKs from
CEO
-
Agent
lets you instantiate an agent. -
Personality
is an enumeration class used for customizing personalities of agents.-
Personality.PRUDENT
makes the agent's behavior more cautious. -
Personality.INQUISITIVE
encourages the agent to be more proactive in trying and exploring.
-
-
get_openai_model
gives you aBaseChatModel
as thought engine. -
@ability(brain: BaseChatModel)
is a decorator which lets you declare a function as anAbility
. -
@agentic(agent: Agent)
is a decorator which lets you declare a function as anAgenticAbility
.
from ceo import ( Agent, Personality, get_openai_model, ability, agentic )
-
-
declare functions as basic abilities
@ability def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.'
-
instantiate an agent
You can grant abilities to agents while instantiating them.
model = get_openai_model() agent = Agent(abilities=[calculator, write_file], brain=model, name='CEO', personality=Personality.INQUISITIVE)
-
You can also grant more abilities to agents later:
agent.grant_ability(calculator)
or
agent.grant_abilities([calculator])
-
To deprive abilities:
agent.deprive_ability(calculator)
or
agent.deprive_abilities([calculator])
You can change an agent's personality using method
change_personality(personality: Personality)
agent.change_personality(Personality.PRUDENT)
-
-
assign a query to your agent
agent.assign("Here is a sphere with radius of (1 * 9.5 / 2 * 2) cm and pi here is 3.14159, find the area and volume respectively then write the results into a file called 'result.txt'.")
-
leave the rest to your agent
response = agent.just_do_it() print(response)
ceo
also supports multi-agent collaboration scenario, declare a function as agent calling ability with@agentic(agent: Agent)
, then grant it to an agent. See example.
-
-
Find the surface area and volume of a sphere and write the results into a file.
import logging from ceo import ( Agent, Personality, get_openai_model, ability ) from sympy import simplify from dotenv import load_dotenv load_dotenv() logging.getLogger('ceo').setLevel(logging.DEBUG) @ability def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.' if __name__ == '__main__': ceo = Agent(abilities=[calculator, write_file], brain=get_openai_model(), name='CEO', personality=Personality.INQUISITIVE) ceo.assign("Here is a sphere with radius of (1 * 9.5 / 2 * 2) cm and pi here is 3.14159, find the area and volume respectively then write the results into a file called 'result.txt'.") result = ceo.just_do_it() print(result)
# result.txt Surface Area: 1134.11399 cm² Volume: 3591.36097 cm³
# stdout [DEBUG] 2024-12-03 01:56:59,298 ceo : Agent: CEO; Expected steps: 4; Query: "{"User's intention": "Calculate the radius, surface area, and volume of a sphere, then write the results to 'result.txt'."}"; [DEBUG] 2024-12-03 01:57:06,375 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 01:57:06.375661', 'agent_name': 'CEO', 'message_from_CEO': "I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to input the expression '(1 * 9.5 / 2 * 2)'. \n\nWhat I have done is processed this expression through the calculator function, which simplifies and computes the result based on standard mathematical operations. The evaluation of the expression yielded the result of 9.50000000000000."}; [DEBUG] 2024-12-03 01:57:18,717 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 01:57:18.716921', 'agent_name': 'CEO', 'message_from_CEO': "I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to calculate the expression '4 * 3.14159 * (9.5 ** 2)'. \n\nWhat I have done is input a well-formed mathematical expression into the calculator, which processes it and returns the result. The expression calculates the area of a circle with a radius of 9.5, multiplied by 4, using the value of π (pi) as approximately 3.14159.\n\nThe result of this calculation is 1134.11399000000."}; [DEBUG] 2024-12-03 01:57:57,160 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 01:57:57.160521', 'agent_name': 'CEO', 'message_from_CEO': 'I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to input the expression "(4/3) * 3.14159 * (9.5 ** 3)". \n\nWhat I have done is calculate the volume of a sphere with a radius of 9.5 using the formula for the volume of a sphere, which is \\( V = \\frac{4}{3} \\pi r^3 \\). The result of this calculation is approximately 3591.36096833333.'}; [DEBUG] 2024-12-03 01:58:09,530 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 01:58:09.530346', 'agent_name': 'CEO', 'message_from_CEO': 'I used the ability to write a file. My choice was to create a file named "result.txt" and write the content "Surface Area: 1134.11399 cm²\\nVolume: 3591.36097 cm³" into it. \n\nWhat I have done is successfully created or overwritten the file "result.txt" with the specified content. The result of this operation is that the text "Surface Area: 1134.11399 cm²\\nVolume: 3591.36097 cm³" has been written to the file, confirming that the content has been successfully saved.'}; [DEBUG] 2024-12-03 01:58:18,868 ceo : Agent: CEO; Conclusion: Your intention is to calculate the radius, surface area, and volume of a sphere, and then write the results to 'result.txt'. I have successfully achieved your intention. Here are the results: - **Radius**: 9.5 cm - **Surface Area**: 1134.11399 cm² - **Volume**: 3591.36097 cm³ Additionally, I have written the following content to 'result.txt': Surface Area: 1134.11399 cm² Volume: 3591.36097 cm³ {'success': True, 'response': "Your intention is to calculate the radius, surface area, and volume of a sphere, and then write the results to 'result.txt'. I have successfully achieved your intention.\n\nHere are the results:\n\n- **Radius**: 9.5 cm\n- **Surface Area**: 1134.11399 cm²\n- **Volume**: 3591.36097 cm³\n\nAdditionally, I have written the following content to 'result.txt':\n```\nSurface Area: 1134.11399 cm²\nVolume: 3591.36097 cm³\n```"}
-
-
-
Ask the suitable agents to find the surface area and volume of a sphere and write the results into a file.
import logging from sympy import simplify from dotenv import load_dotenv from ceo import ( Agent, Personality, get_openai_model, agentic, ability ) load_dotenv() logging.getLogger('ceo').setLevel(logging.DEBUG) model = get_openai_model() @ability def calculator(expr: str) -> float: # this function only accepts a single math expression return simplify(expr) @ability def write_file(filename: str, content: str) -> str: with open(filename, 'w', encoding='utf-8') as f: f.write(content) return f'{content} written to {filename}.' jack = Agent(abilities=[calculator], brain=model, name='Jack', personality=Personality.INQUISITIVE) tylor = Agent(abilities=[write_file], brain=model, name='Tylor', personality=Personality.PRUDENT) @agentic(jack) def agent1(): return @agentic(tylor) def agent2(): return if __name__ == '__main__': ceo = Agent(abilities=[agent1, agent2], brain=model, name='CEO', personality=Personality.INQUISITIVE) ceo.assign("Here is a sphere with radius of (1 * 9.5 / 2 * 2) cm and pi here is 3.14159, find the area and volume respectively then write the results into a file called 'result.txt'.") result = ceo.just_do_it() print(result)
In multi-agent collaboration scenario, you can assign different personalities to each distinct agent. For example, in the aforementioned script, Jack's capability is to perform calculations. I want him to try more and explore more, so Jack's personality is set to
Personality.INQUISITIVE
. On the other hand, Taylor's capability is to create and write files. For operations involving interaction with the external file system, I want him to be more cautious, so Taylor's personality is set toPersonality.PRUDENT
.# result.txt Radius: 9.5 cm Surface Area: 1134.11 cm² Volume: 3591.36 cm³
# stdout [DEBUG] 2024-12-03 02:00:40,666 ceo : Agent: CEO; Expected steps: 4; Query: "{"User's intention": "Calculate the radius, surface area, and volume of a sphere, then write the results to 'result.txt'."}"; [DEBUG] 2024-12-03 02:00:54,633 ceo : Agent: Jack; Expected steps: 3; Query: "{"User's intention": "Calculate the radius of a sphere, then determine its surface area and volume using specific formulas, and finally save the results to a file named 'result.txt'."}"; [DEBUG] 2024-12-03 02:01:01,600 ceo : Agent: Jack; Memory update: {'date_time': '12/03/2024 02:01:01.598989', 'agent_name': 'Jack', 'message_from_Jack': "I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to input the expression '(1 * 9.5 / 2 * 2)'. \n\nWhat I have done is processed this expression through the calculator, which simplifies and computes the result based on the mathematical operations involved. The result of this evaluation is '9.50000000000000'."}; [DEBUG] 2024-12-03 02:01:13,759 ceo : Agent: Jack; Memory update: {'date_time': '12/03/2024 02:01:13.759667', 'agent_name': 'Jack', 'message_from_Jack': "I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to calculate the expression '4 * 3.14159 * (9.5 ** 2)'. \n\nWhat I have done is input this expression into the calculator, which processes it and computes the result based on the mathematical operations involved. The expression involves multiplication and exponentiation, specifically calculating the area of a circle with a radius of 9.5, multiplied by 4 and the value of π (approximately 3.14159).\n\nThe result of this calculation is 1134.11399000000."}; [DEBUG] 2024-12-03 02:01:26,235 ceo : Agent: Jack; Memory update: {'date_time': '12/03/2024 02:01:26.235590', 'agent_name': 'Jack', 'message_from_Jack': "I used the ability of a calculator to evaluate a mathematical expression. The choice I made was to calculate the expression '(4/3) * 3.14159 * (9.5 ** 3)'. \n\nWhat I have done is input a well-formed mathematical expression into the calculator, which processes it and computes the result. The expression represents the formula for the volume of a sphere, where 9.5 is the radius. \n\nThe result of this calculation is 3591.36096833333."}; [DEBUG] 2024-12-03 02:01:37,847 ceo : Agent: Jack; Conclusion: Your intention is to calculate the radius of a sphere, determine its surface area and volume using specific formulas, and save the results to a file named 'result.txt'. I have partially achieved your intention. I calculated the radius (9.5), the surface area (1134.11), and the volume (3591.36) of the sphere using the appropriate formulas. However, I did not save the results to a file named 'result.txt' as that action was not performed. Here are the results: - Radius: 9.5 - Surface Area: 1134.11 - Volume: 3591.36 If you need me to assist with saving these results to a file, please let me know!; [DEBUG] 2024-12-03 02:01:44,421 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 02:01:44.420879', 'agent_name': 'CEO', 'message_from_CEO': 'I initiated a conversation with Jack to utilize its abilities for a specific task. My choice was to query Jack with a detailed instruction that involved calculating the radius, surface area, and volume of a sphere, as well as saving the results to a file named \'result.txt\'.\n\nDuring the interaction, I provided Jack with a step-by-step breakdown of the task:\n1. Identify the radius of the sphere, calculated as (1 * 9.5 / 2 * 2) cm.\n2. Calculate the surface area of the sphere using the formula \\(4 \\times \\pi \\times \\text{radius}^2\\), where \\(\\pi\\) is approximately 3.14159.\n3. Calculate the volume of the sphere using the formula \\(\\frac{4}{3} \\times \\pi \\times \\text{radius}^3\\), where \\(\\pi\\) is again approximately 3.14159.\n4. Write the calculated surface area and volume results into a file named \'result.txt\'.\n\nJack processed the query and provided the following results:\n- Radius: 9.5 cm\n- Surface Area: 1134.11 cm²\n- Volume: 3591.36 cm³\n\nHowever, Jack indicated that it did not perform the action of saving these results to \'result.txt\'. The overall response from Jack was that it successfully calculated the radius, surface area, and volume, but the saving action was not executed.\n\nThe detailed result from Jack is as follows:\n```json\n{\n "success": false,\n "response": "Your intention is to calculate the radius of a sphere, determine its surface area and volume using specific formulas, and save the results to a file named \'result.txt\'. \\n\\nI have partially achieved your intention. I calculated the radius (9.5), the surface area (1134.11), and the volume (3591.36) of the sphere using the appropriate formulas. However, I did not save the results to a file named \'result.txt\' as that action was not performed.\\n\\nHere are the results:\\n- Radius: 9.5\\n- Surface Area: 1134.11\\n- Volume: 3591.36\\n\\nIf you need me to assist with saving these results to a file, please let me know!"\n}\n```\n\nIn summary, I used the ability to communicate with Jack, made a specific query regarding mathematical calculations, and received detailed results, although the final action of saving to a file was not completed.'}; [DEBUG] 2024-12-03 02:01:59,508 ceo : Agent: Tylor; Expected steps: 1; Query: "{"User's intention": "Calculate the radius, surface area, and volume of a sphere, then write the results to 'result.txt'."}"; [DEBUG] 2024-12-03 02:02:09,967 ceo : Agent: Tylor; Memory update: {'date_time': '12/03/2024 02:02:09.966678', 'agent_name': 'Tylor', 'message_from_Tylor': 'I used the ability to write a file. My choice was to create a file named "result.txt" and write specific content into it. The content included details about a radius of 9.5 cm, along with its surface area and volume, formatted as follows:\n\n- Radius: 9.5 cm\n- Surface Area: 1134.11 cm²\n- Volume: 3591.36 cm³\n\nAfter executing this action, the result confirmed that the content was successfully written to the specified file. The final output was: "Radius: 9.5 cm\\nSurface Area: 1134.11 cm²\\nVolume: 3591.36 cm³ written to result.txt."'}; [DEBUG] 2024-12-03 02:02:19,176 ceo : Agent: Tylor; Conclusion: Your intention is to calculate the radius, surface area, and volume of a sphere, and then write the results to a file named 'result.txt'. I have successfully achieved your intention. I calculated the following results: - Radius: 9.5 cm - Surface Area: 1134.11 cm² - Volume: 3591.36 cm³ Additionally, I wrote these results to 'result.txt'. The content of the file is as follows: - Radius: 9.5 cm - Surface Area: 1134.11 cm² - Volume: 3591.36 cm³ If you need any further assistance, feel free to ask!; [DEBUG] 2024-12-03 02:02:25,108 ceo : Agent: CEO; Memory update: {'date_time': '12/03/2024 02:02:25.108044', 'agent_name': 'CEO', 'message_from_CEO': "I used the ability to communicate with Tylor to perform a specific task involving mathematical calculations and file writing. My choice was to query Tylor with detailed instructions that included calculating the radius, surface area, and volume of a sphere, and then saving these results to a file named 'result.txt'.\n\nHere’s what I have done step by step:\n\n1. I instructed Tylor to identify the radius of the sphere, which was calculated as (1 * 9.5 / 2 * 2) cm.\n2. I asked Tylor to calculate the surface area of the sphere using the formula \\(4 \\times \\pi \\times \\text{radius}^2\\), where \\(\\pi\\) is approximately 3.14159.\n3. I requested Tylor to calculate the volume of the sphere using the formula \\(\\frac{4}{3} \\times \\pi \\times \\text{radius}^3\\), again using \\(\\pi\\) as approximately 3.14159.\n4. Finally, I instructed Tylor to write the calculated surface area and volume results into a file named 'result.txt'.\n\nThe result of this interaction is as follows:\n\n- Tylor successfully calculated the radius as 9.5 cm.\n- The surface area was calculated to be 1134.11 cm².\n- The volume was calculated to be 3591.36 cm³.\n- Additionally, Tylor confirmed that it wrote these results to 'result.txt'.\n\nThe content of the file 'result.txt' is:\n```\n- Radius: 9.5 cm\n- Surface Area: 1134.11 cm²\n- Volume: 3591.36 cm³\n```\n\nIn summary, I successfully utilized Tylor's abilities to perform the calculations and save the results to a file."}; [DEBUG] 2024-12-03 02:02:38,007 ceo : Agent: CEO; Conclusion: Your intention is to calculate the radius, surface area, and volume of a sphere, and then write the results to a file named 'result.txt'. I have partially achieved your intention. I successfully calculated the radius (9.5 cm), the surface area (1134.11 cm²), and the volume (3591.36 cm³) of the sphere using the appropriate formulas. However, I did not save the results to 'result.txt' in my initial attempt. In a subsequent attempt, I did manage to save the results to 'result.txt'. Here are the results: - Radius: 9.5 cm - Surface Area: 1134.11 cm² - Volume: 3591.36 cm³ The content of the file 'result.txt' is: - Radius: 9.5 cm - Surface Area: 1134.11 cm² - Volume: 3591.36 cm³ If you need any further assistance, feel free to ask!; {'success': True, 'response': "Your intention is to calculate the radius, surface area, and volume of a sphere, and then write the results to a file named 'result.txt'. \n\nI have partially achieved your intention. I successfully calculated the radius (9.5 cm), the surface area (1134.11 cm²), and the volume (3591.36 cm³) of the sphere using the appropriate formulas. However, I did not save the results to 'result.txt' in my initial attempt. \n\nIn a subsequent attempt, I did manage to save the results to 'result.txt'. Here are the results:\n\n- Radius: 9.5 cm\n- Surface Area: 1134.11 cm²\n- Volume: 3591.36 cm³\n\nThe content of the file 'result.txt' is:\n```\n- Radius: 9.5 cm\n- Surface Area: 1134.11 cm²\n- Volume: 3591.36 cm³\n```\n\nIf you need any further assistance, feel free to ask!"}
-
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for CEO
Similar Open Source Tools
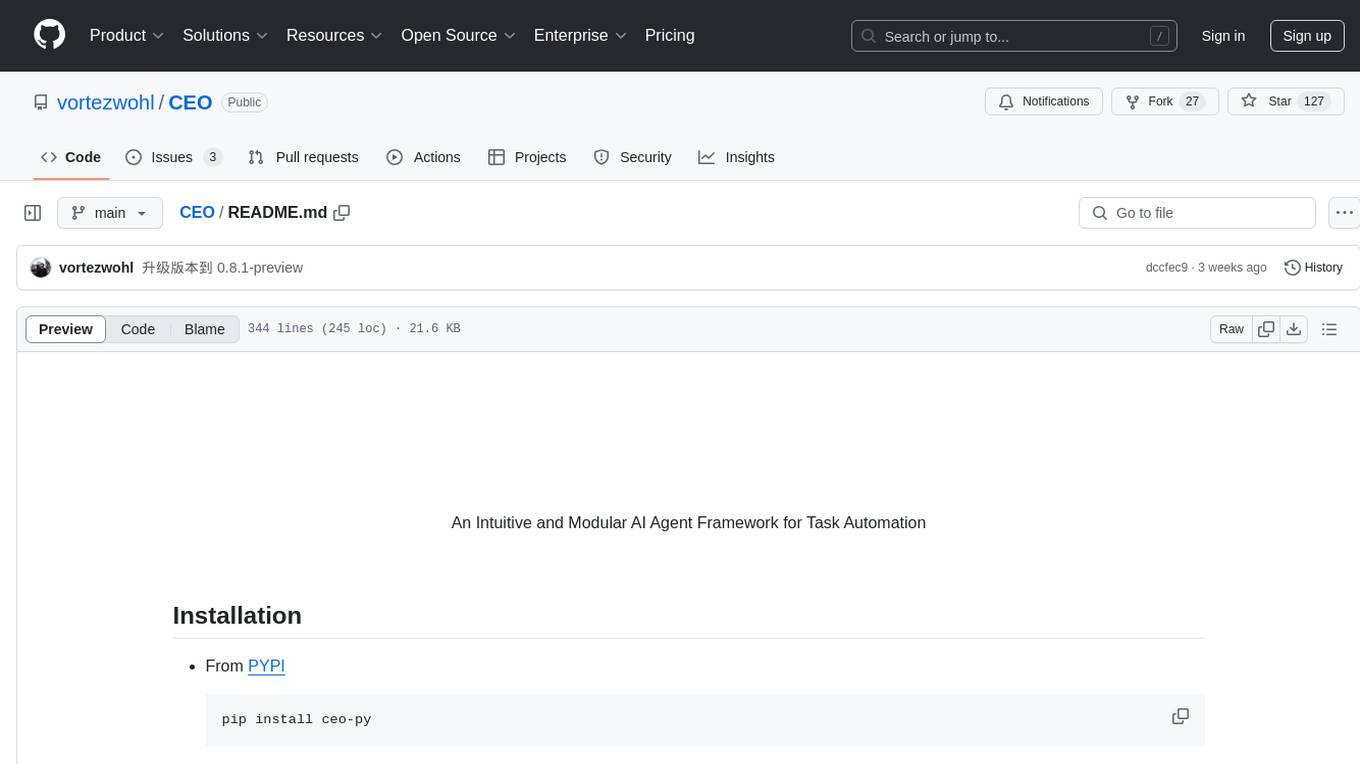
CEO
CEO is an intuitive and modular AI agent framework designed for task automation. It provides a flexible environment for building agents with specific abilities and personalities, allowing users to assign tasks and interact with the agents to automate various processes. The framework supports multi-agent collaboration scenarios and offers functionalities like instantiating agents, granting abilities, assigning queries, and executing tasks. Users can customize agent personalities and define specific abilities using decorators, making it easy to create complex automation workflows.
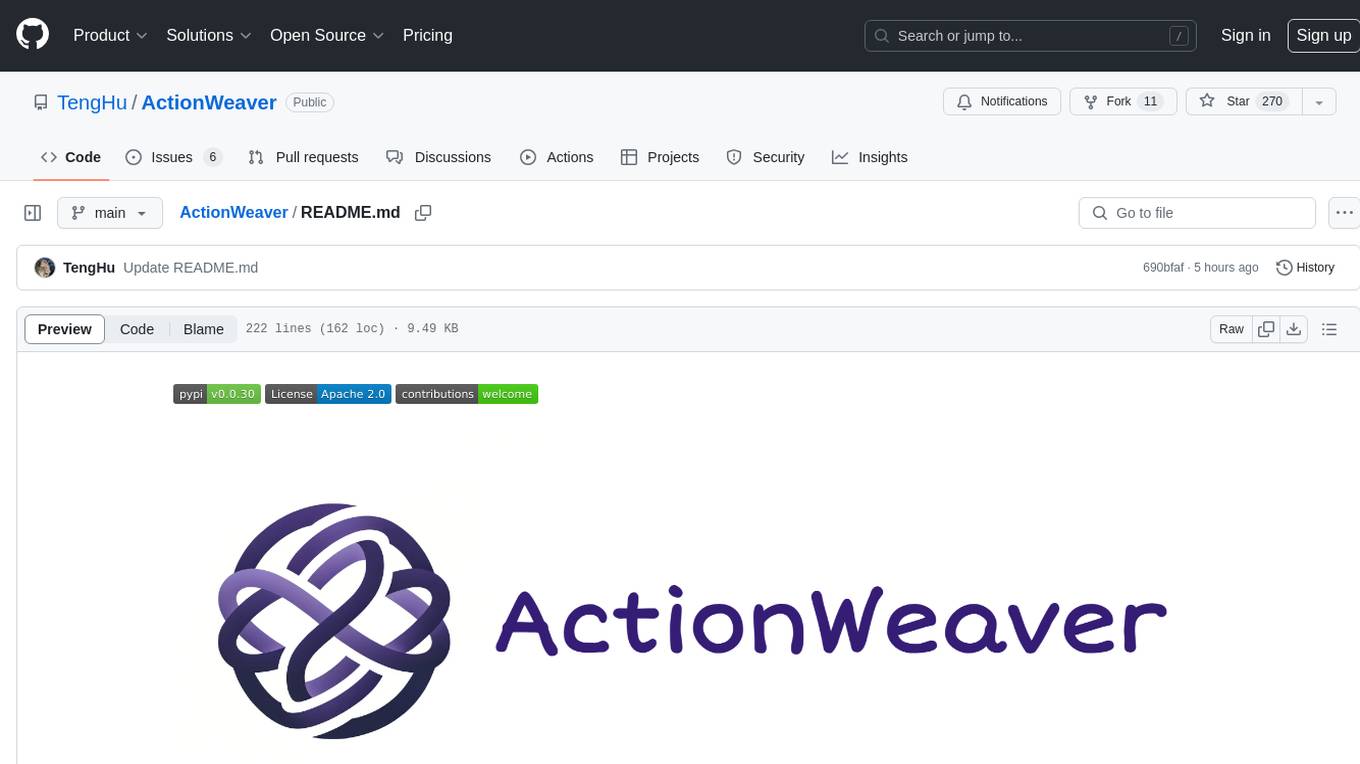
ActionWeaver
ActionWeaver is an AI application framework designed for simplicity, relying on OpenAI and Pydantic. It supports both OpenAI API and Azure OpenAI service. The framework allows for function calling as a core feature, extensibility to integrate any Python code, function orchestration for building complex call hierarchies, and telemetry and observability integration. Users can easily install ActionWeaver using pip and leverage its capabilities to create, invoke, and orchestrate actions with the language model. The framework also provides structured extraction using Pydantic models and allows for exception handling customization. Contributions to the project are welcome, and users are encouraged to cite ActionWeaver if found useful.
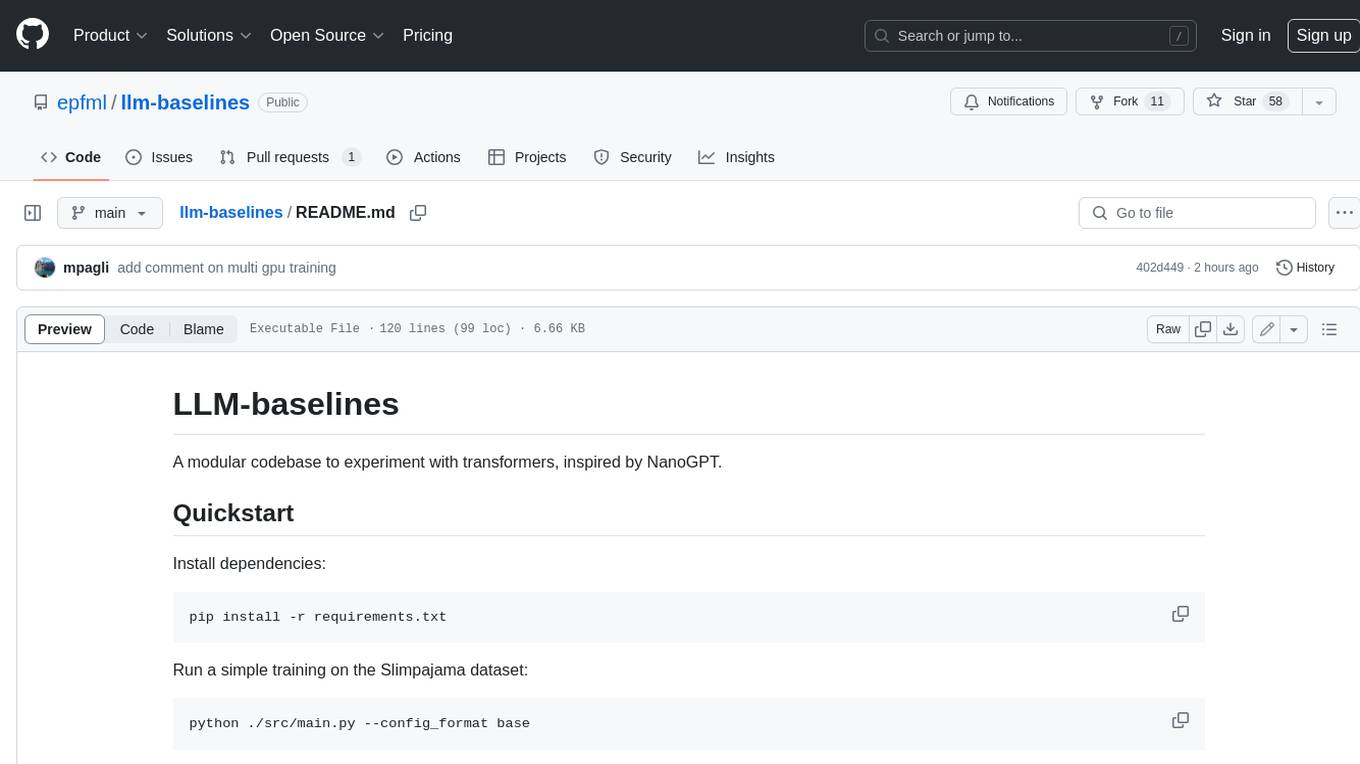
llm-baselines
LLM-baselines is a modular codebase to experiment with transformers, inspired from NanoGPT. It provides a quick and easy way to train and evaluate transformer models on a variety of datasets. The codebase is well-documented and easy to use, making it a great resource for researchers and practitioners alike.
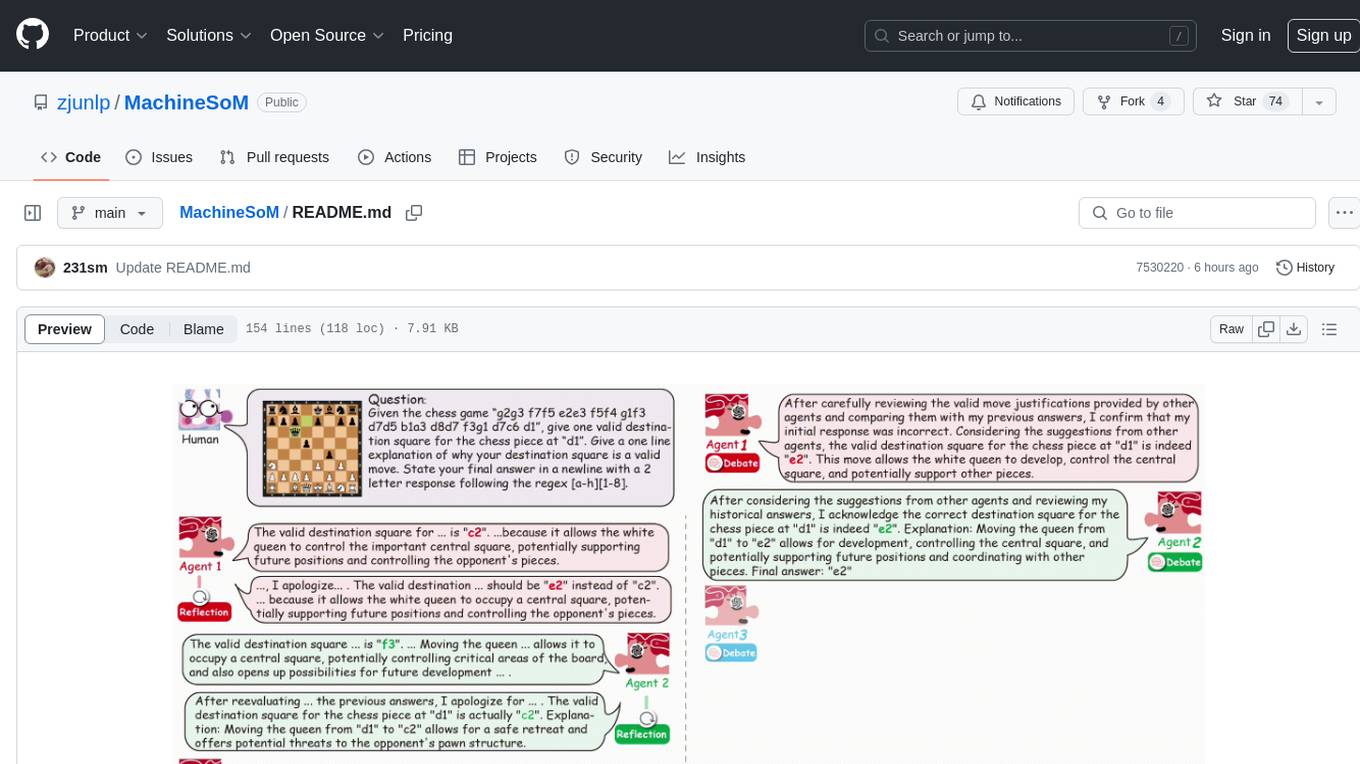
MachineSoM
MachineSoM is a code repository for the paper 'Exploring Collaboration Mechanisms for LLM Agents: A Social Psychology View'. It focuses on the emergence of intelligence from collaborative and communicative computational modules, enabling effective completion of complex tasks. The repository includes code for societies of LLM agents with different traits, collaboration processes such as debate and self-reflection, and interaction strategies for determining when and with whom to interact. It provides a coding framework compatible with various inference services like Replicate, OpenAI, Dashscope, and Anyscale, supporting models like Qwen and GPT. Users can run experiments, evaluate results, and draw figures based on the paper's content, with available datasets for MMLU, Math, and Chess Move Validity.
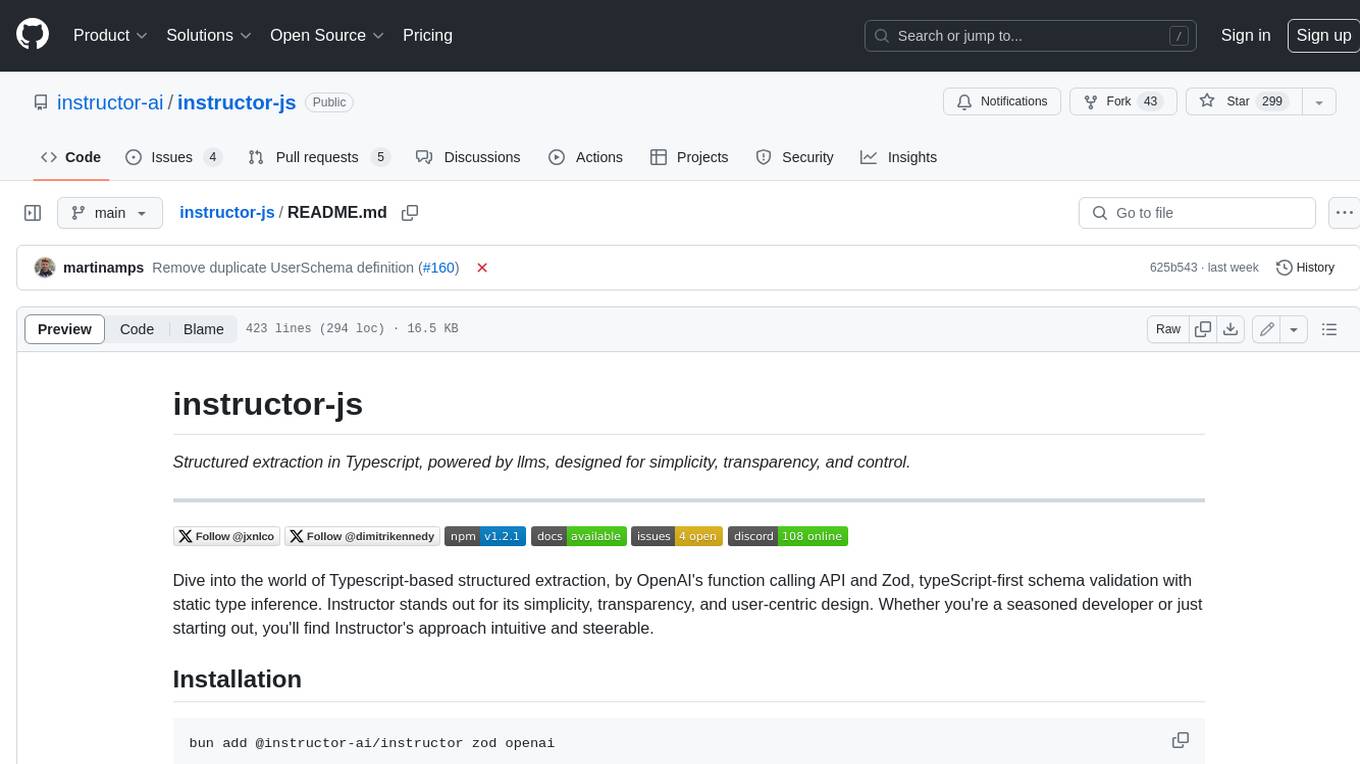
instructor-js
Instructor is a Typescript library for structured extraction in Typescript, powered by llms, designed for simplicity, transparency, and control. It stands out for its simplicity, transparency, and user-centric design. Whether you're a seasoned developer or just starting out, you'll find Instructor's approach intuitive and steerable.
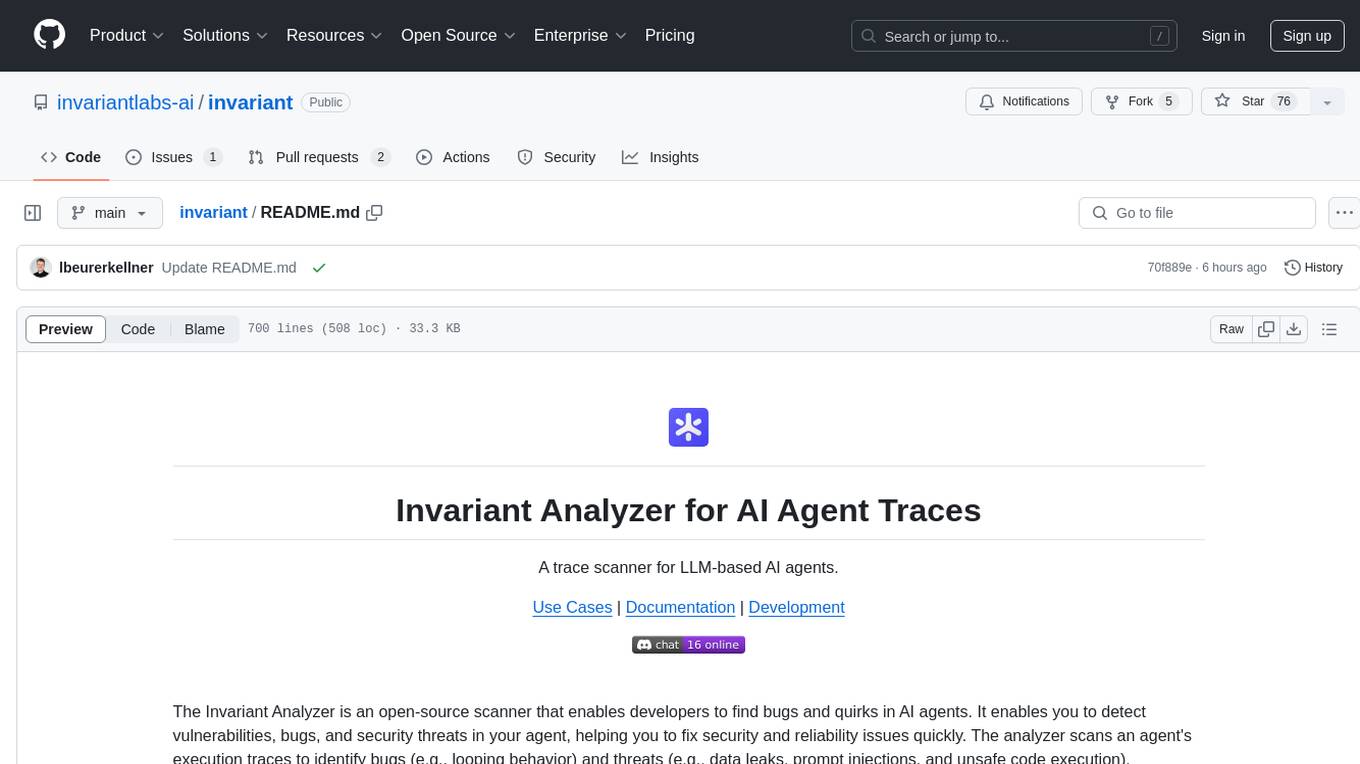
invariant
Invariant Analyzer is an open-source scanner designed for LLM-based AI agents to find bugs, vulnerabilities, and security threats. It scans agent execution traces to identify issues like looping behavior, data leaks, prompt injections, and unsafe code execution. The tool offers a library of built-in checkers, an expressive policy language, data flow analysis, real-time monitoring, and extensible architecture for custom checkers. It helps developers debug AI agents, scan for security violations, and prevent security issues and data breaches during runtime. The analyzer leverages deep contextual understanding and a purpose-built rule matching engine for security policy enforcement.
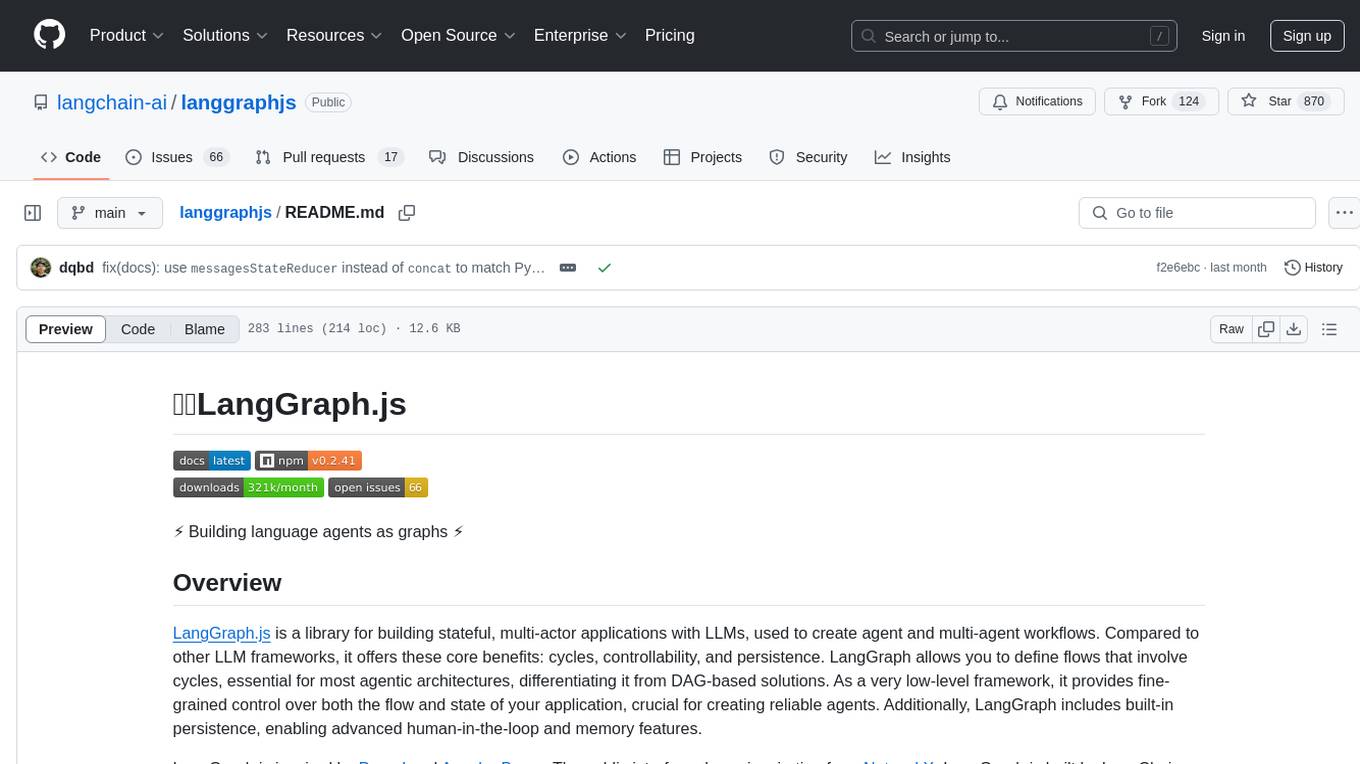
langgraphjs
LangGraph.js is a library for building stateful, multi-actor applications with LLMs, offering benefits such as cycles, controllability, and persistence. It allows defining flows involving cycles, providing fine-grained control over application flow and state. Inspired by Pregel and Apache Beam, it includes features like loops, persistence, human-in-the-loop workflows, and streaming support. LangGraph integrates seamlessly with LangChain.js and LangSmith but can be used independently.
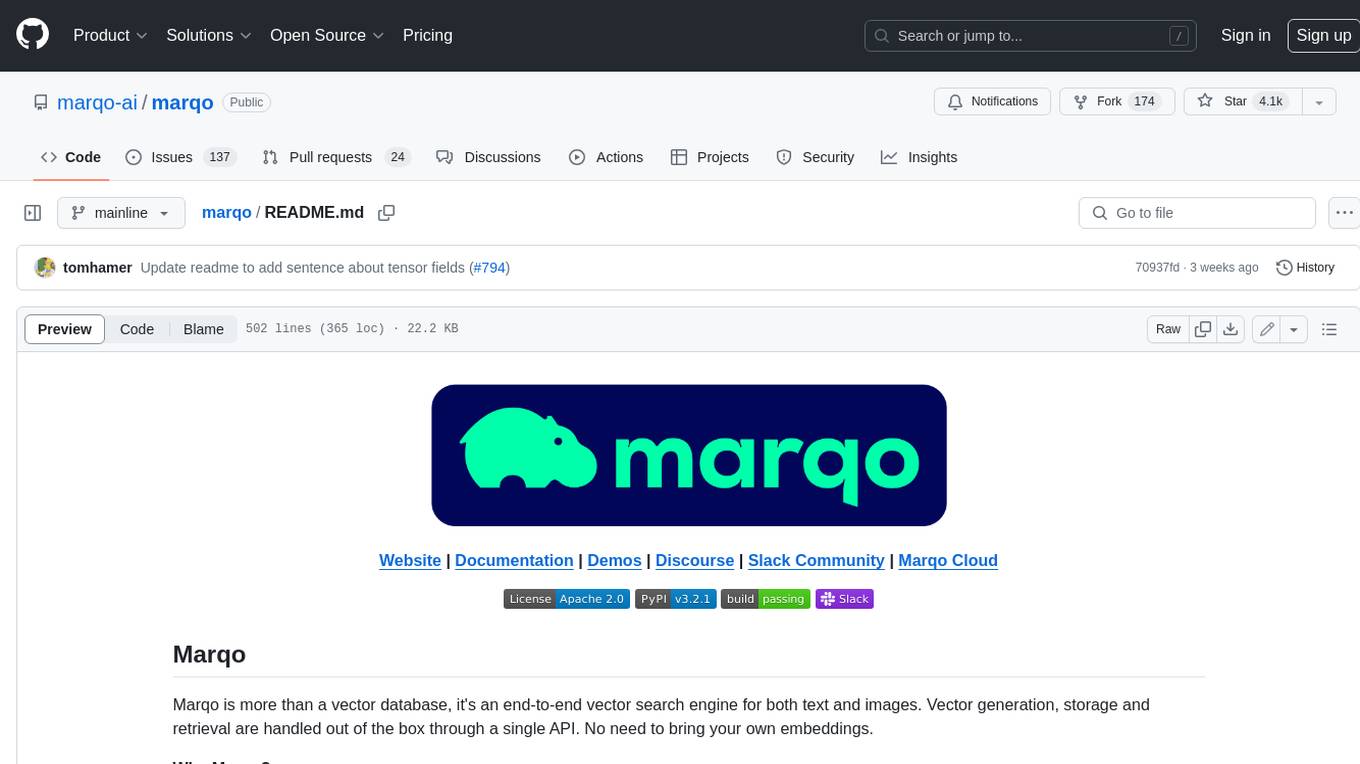
marqo
Marqo is more than a vector database, it's an end-to-end vector search engine for both text and images. Vector generation, storage and retrieval are handled out of the box through a single API. No need to bring your own embeddings.
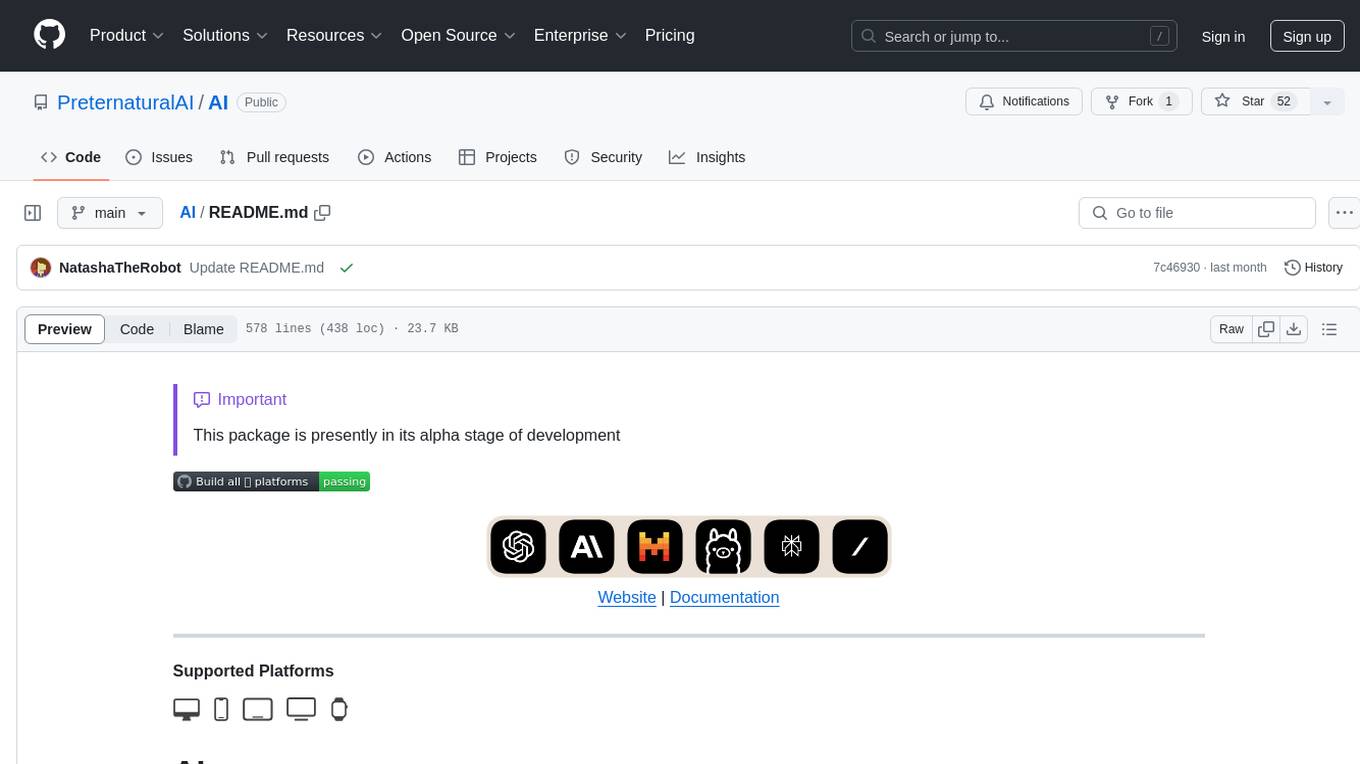
AI
AI is an open-source Swift framework for interfacing with generative AI. It provides functionalities for text completions, image-to-text vision, function calling, DALLE-3 image generation, audio transcription and generation, and text embeddings. The framework supports multiple AI models from providers like OpenAI, Anthropic, Mistral, Groq, and ElevenLabs. Users can easily integrate AI capabilities into their Swift projects using AI framework.
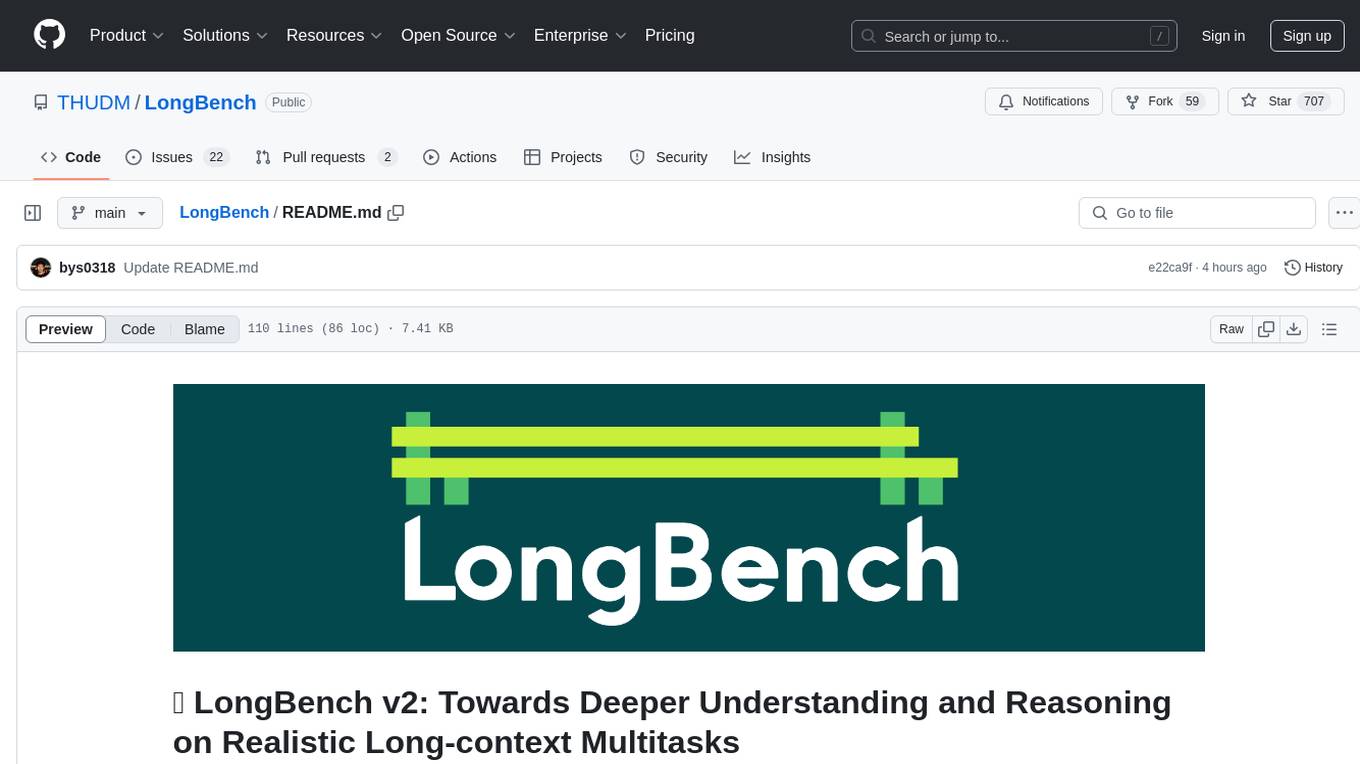
LongBench
LongBench v2 is a benchmark designed to assess the ability of large language models (LLMs) to handle long-context problems requiring deep understanding and reasoning across various real-world multitasks. It consists of 503 challenging multiple-choice questions with contexts ranging from 8k to 2M words, covering six major task categories. The dataset is collected from nearly 100 highly educated individuals with diverse professional backgrounds and is designed to be challenging even for human experts. The evaluation results highlight the importance of enhanced reasoning ability and scaling inference-time compute to tackle the long-context challenges in LongBench v2.
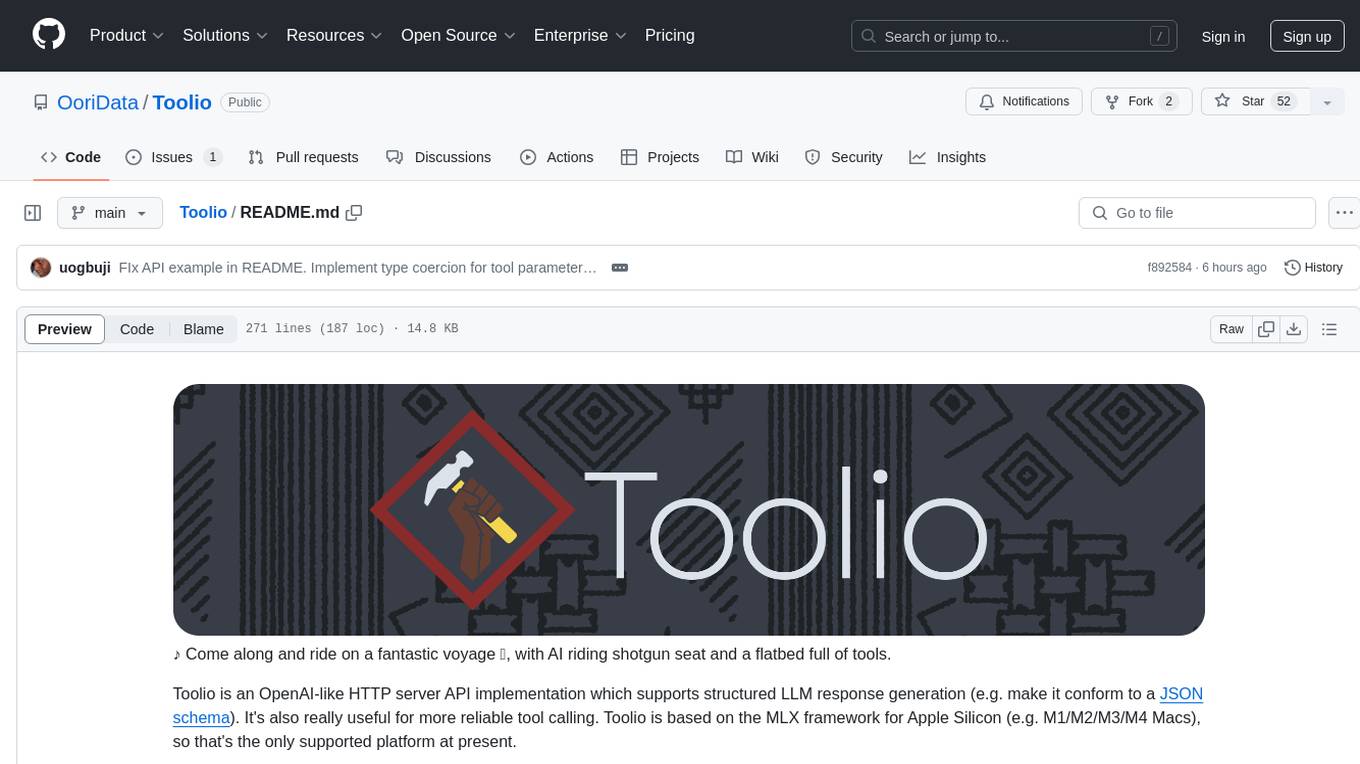
Toolio
Toolio is an OpenAI-like HTTP server API implementation that supports structured LLM response generation, making it conform to a JSON schema. It is useful for reliable tool calling and agentic workflows based on schema-driven output. Toolio is based on the MLX framework for Apple Silicon, specifically M1/M2/M3/M4 Macs. It allows users to host MLX-format LLMs for structured output queries and provides a command line client for easier usage of tools. The tool also supports multiple tool calls and the creation of custom tools for specific tasks.
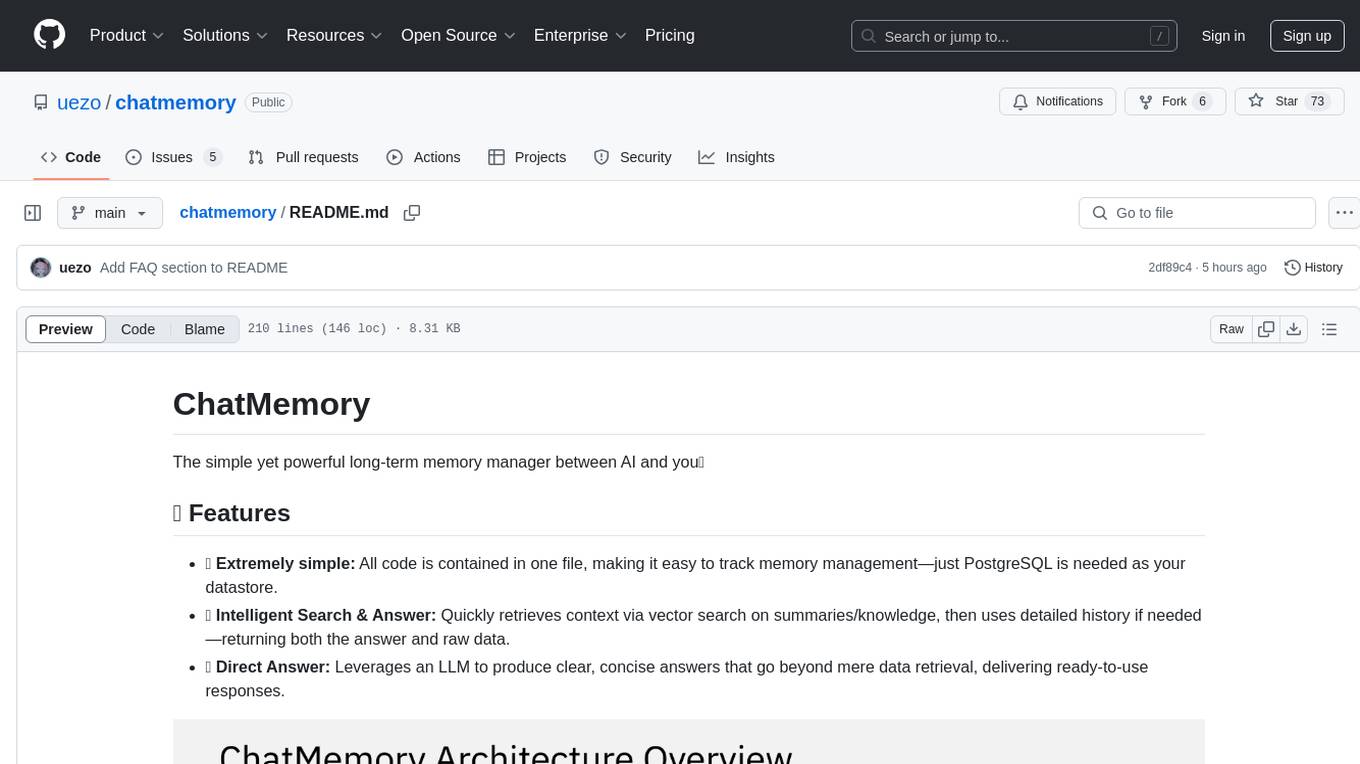
chatmemory
ChatMemory is a simple yet powerful long-term memory manager that facilitates communication between AI and users. It organizes conversation data into history, summary, and knowledge entities, enabling quick retrieval of context and generation of clear, concise answers. The tool leverages vector search on summaries/knowledge and detailed history to provide accurate responses. It balances speed and accuracy by using lightweight retrieval and fallback detailed search mechanisms, ensuring efficient memory management and response generation beyond mere data retrieval.
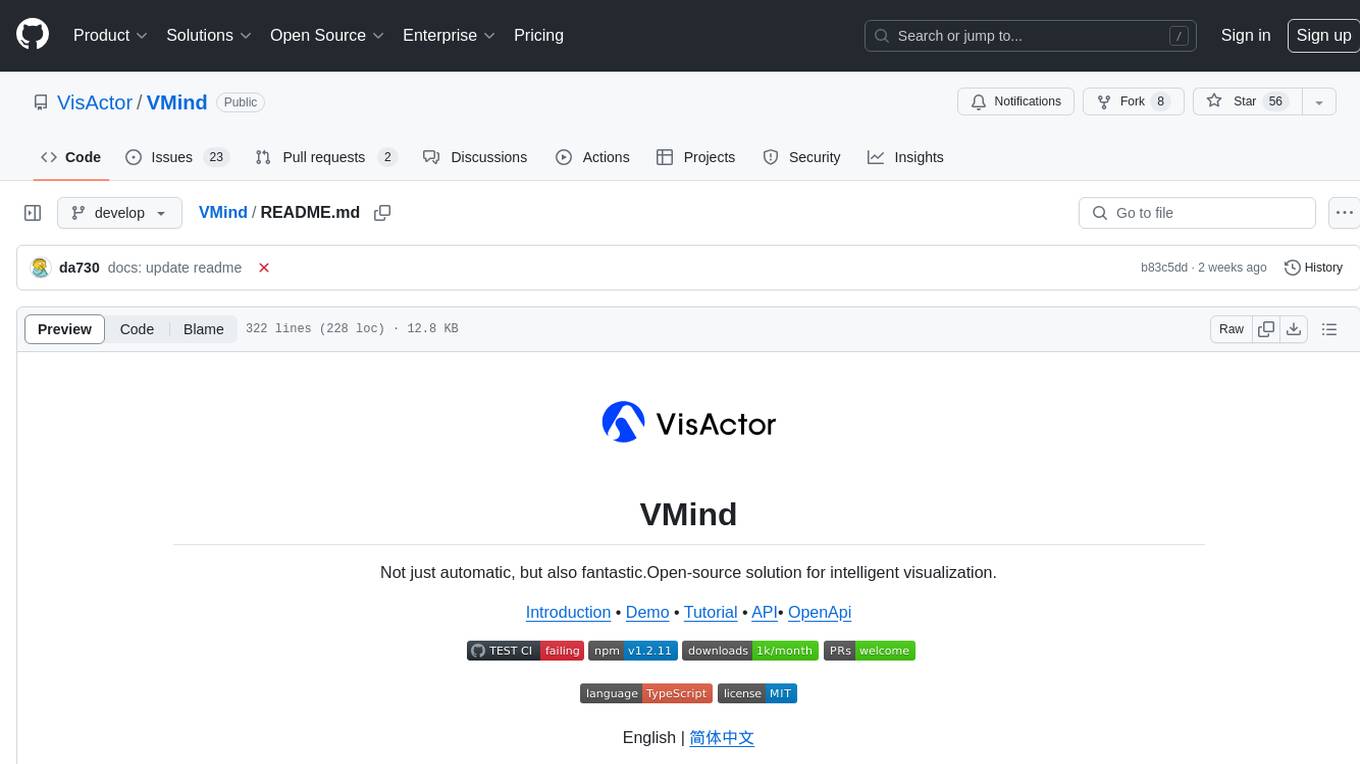
VMind
VMind is an open-source solution for intelligent visualization, providing an intelligent chart component based on LLM by VisActor. It allows users to create chart narrative works with natural language interaction, edit charts through dialogue, and export narratives as videos or GIFs. The tool is easy to use, scalable, supports various chart types, and offers one-click export functionality. Users can customize chart styles, specify themes, and aggregate data using LLM models. VMind aims to enhance efficiency in creating data visualization works through dialogue-based editing and natural language interaction.
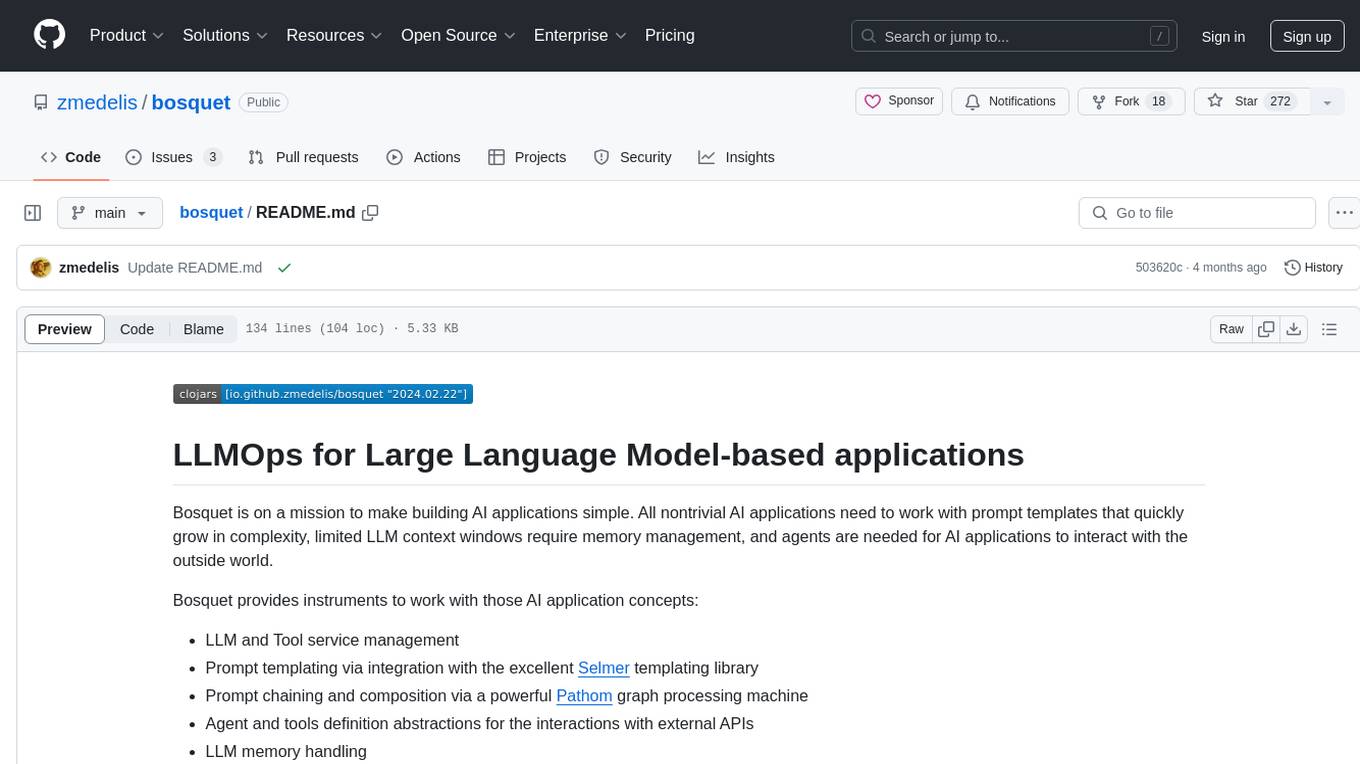
bosquet
Bosquet is a tool designed for LLMOps in large language model-based applications. It simplifies building AI applications by managing LLM and tool services, integrating with Selmer templating library for prompt templating, enabling prompt chaining and composition with Pathom graph processing, defining agents and tools for external API interactions, handling LLM memory, and providing features like call response caching. The tool aims to streamline the development process for AI applications that require complex prompt templates, memory management, and interaction with external systems.
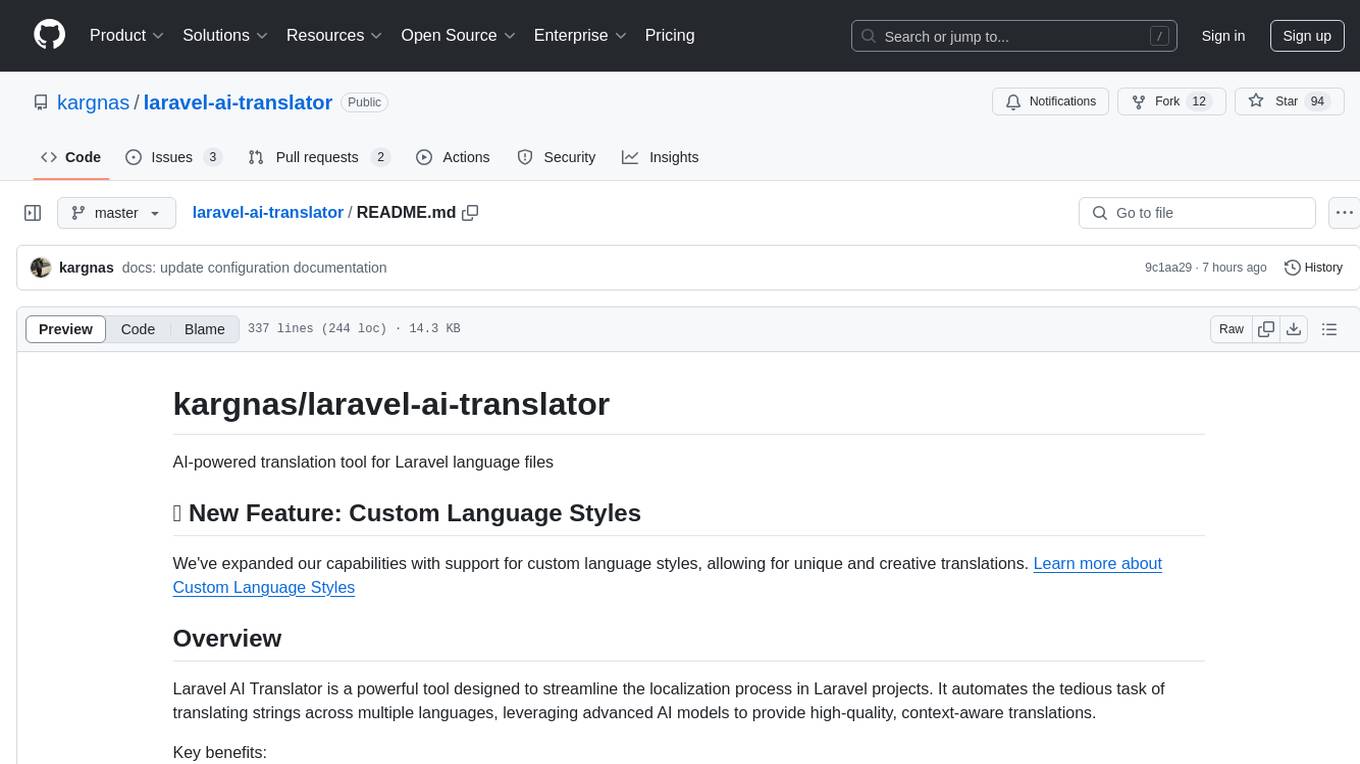
laravel-ai-translator
Laravel AI Translator is a powerful tool designed to streamline the localization process in Laravel projects. It automates the task of translating strings across multiple languages using advanced AI models like GPT-4 and Claude. The tool supports custom language styles, preserves variables and nested structures, and ensures consistent tone and style across translations. It integrates seamlessly with Laravel projects, making internationalization easier and more efficient. Users can customize translation rules, handle large language files efficiently, and validate translations for accuracy. The tool offers contextual understanding, linguistic precision, variable handling, smart length adaptation, and tone consistency for intelligent translations.
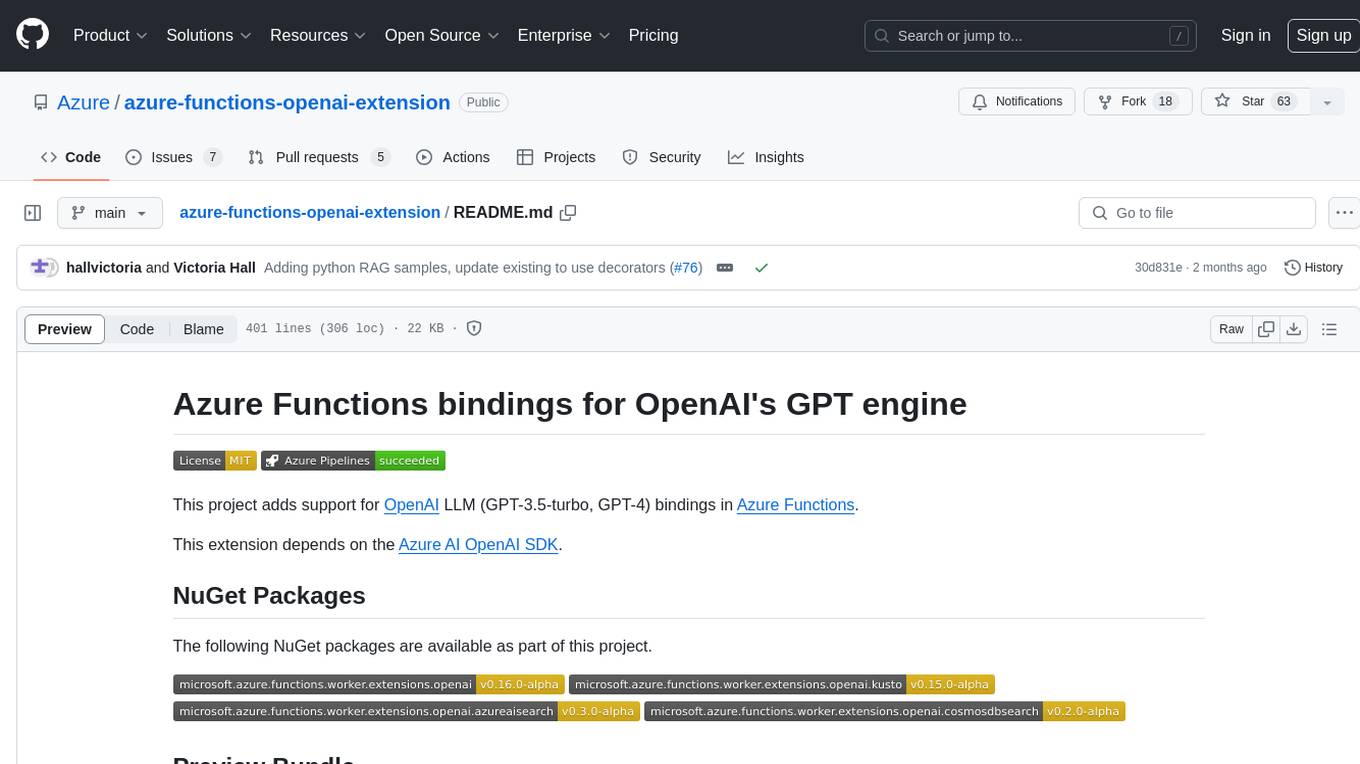
azure-functions-openai-extension
Azure Functions OpenAI Extension is a project that adds support for OpenAI LLM (GPT-3.5-turbo, GPT-4) bindings in Azure Functions. It provides NuGet packages for various functionalities like text completions, chat completions, assistants, embeddings generators, and semantic search. The project requires .NET 6 SDK or greater, Azure Functions Core Tools v4.x, and specific settings in Azure Function or local settings for development. It offers features like text completions, chat completion, assistants with custom skills, embeddings generators for text relatedness, and semantic search using vector databases. The project also includes examples in C# and Python for different functionalities.
For similar tasks
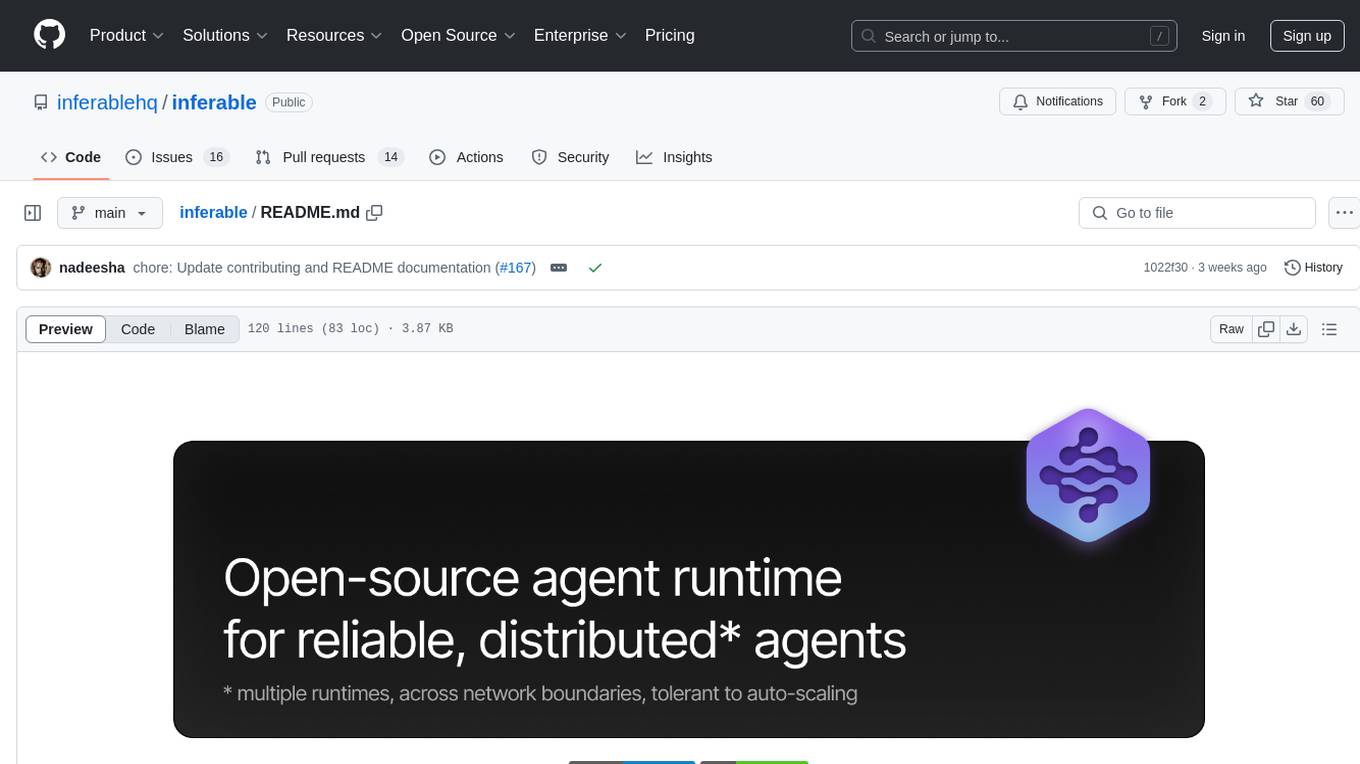
inferable
Inferable is an open source platform that helps users build reliable LLM-powered agentic automations at scale. It offers a managed agent runtime, durable tool calling, zero network configuration, multiple language support, and is fully open source under the MIT license. Users can define functions, register them with Inferable, and create runs that utilize these functions to automate tasks. The platform supports Node.js/TypeScript, Go, .NET, and React, and provides SDKs, core services, and bootstrap templates for various languages.
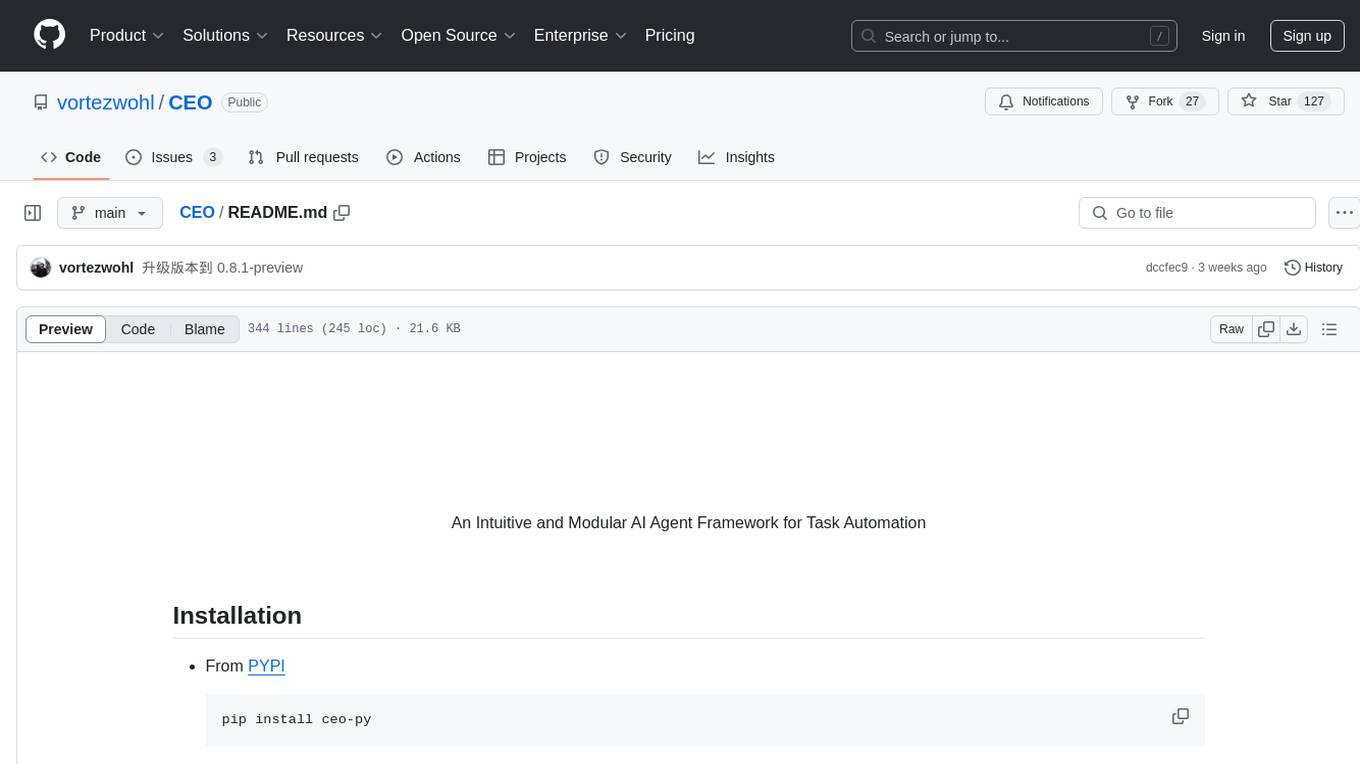
CEO
CEO is an intuitive and modular AI agent framework designed for task automation. It provides a flexible environment for building agents with specific abilities and personalities, allowing users to assign tasks and interact with the agents to automate various processes. The framework supports multi-agent collaboration scenarios and offers functionalities like instantiating agents, granting abilities, assigning queries, and executing tasks. Users can customize agent personalities and define specific abilities using decorators, making it easy to create complex automation workflows.
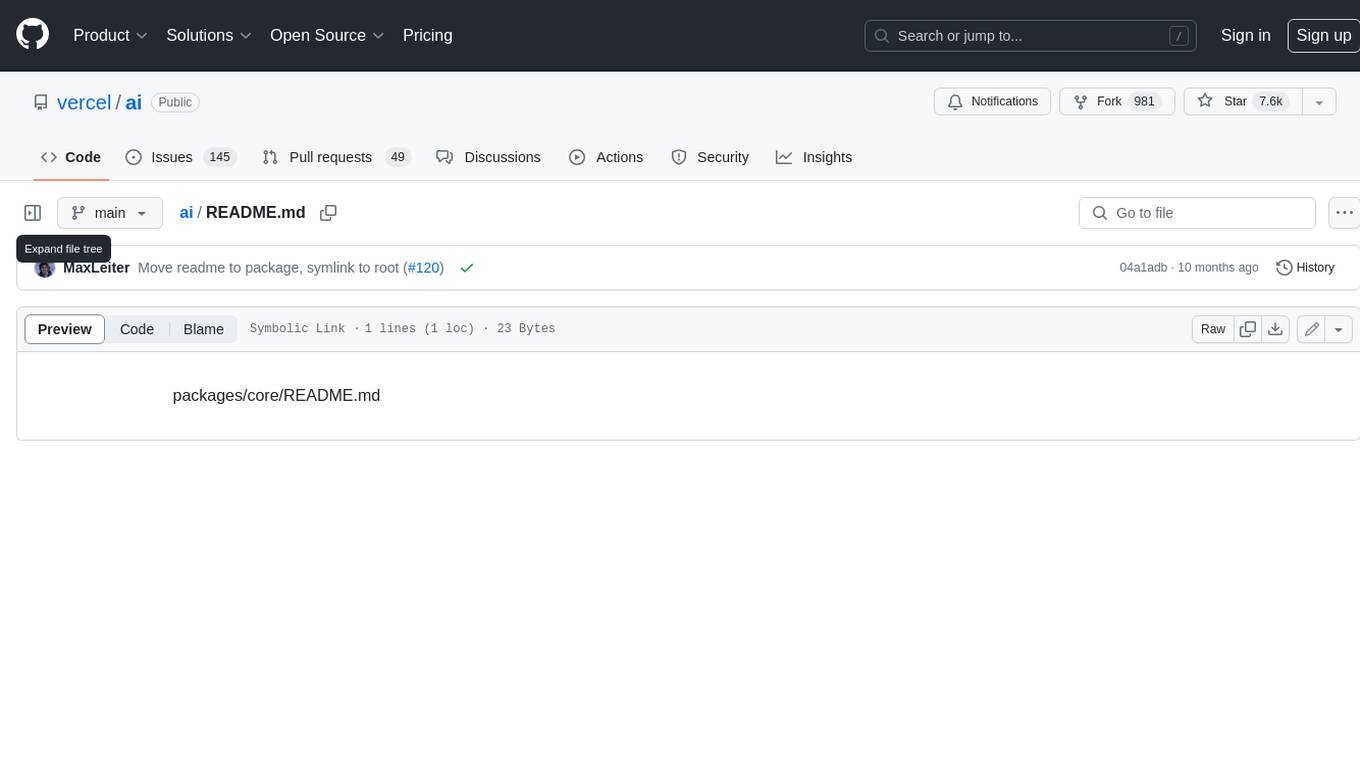
ai
The Vercel AI SDK is a library for building AI-powered streaming text and chat UIs. It provides React, Svelte, Vue, and Solid helpers for streaming text responses and building chat and completion UIs. The SDK also includes a React Server Components API for streaming Generative UI and first-class support for various AI providers such as OpenAI, Anthropic, Mistral, Perplexity, AWS Bedrock, Azure, Google Gemini, Hugging Face, Fireworks, Cohere, LangChain, Replicate, Ollama, and more. Additionally, it offers Node.js, Serverless, and Edge Runtime support, as well as lifecycle callbacks for saving completed streaming responses to a database in the same request.
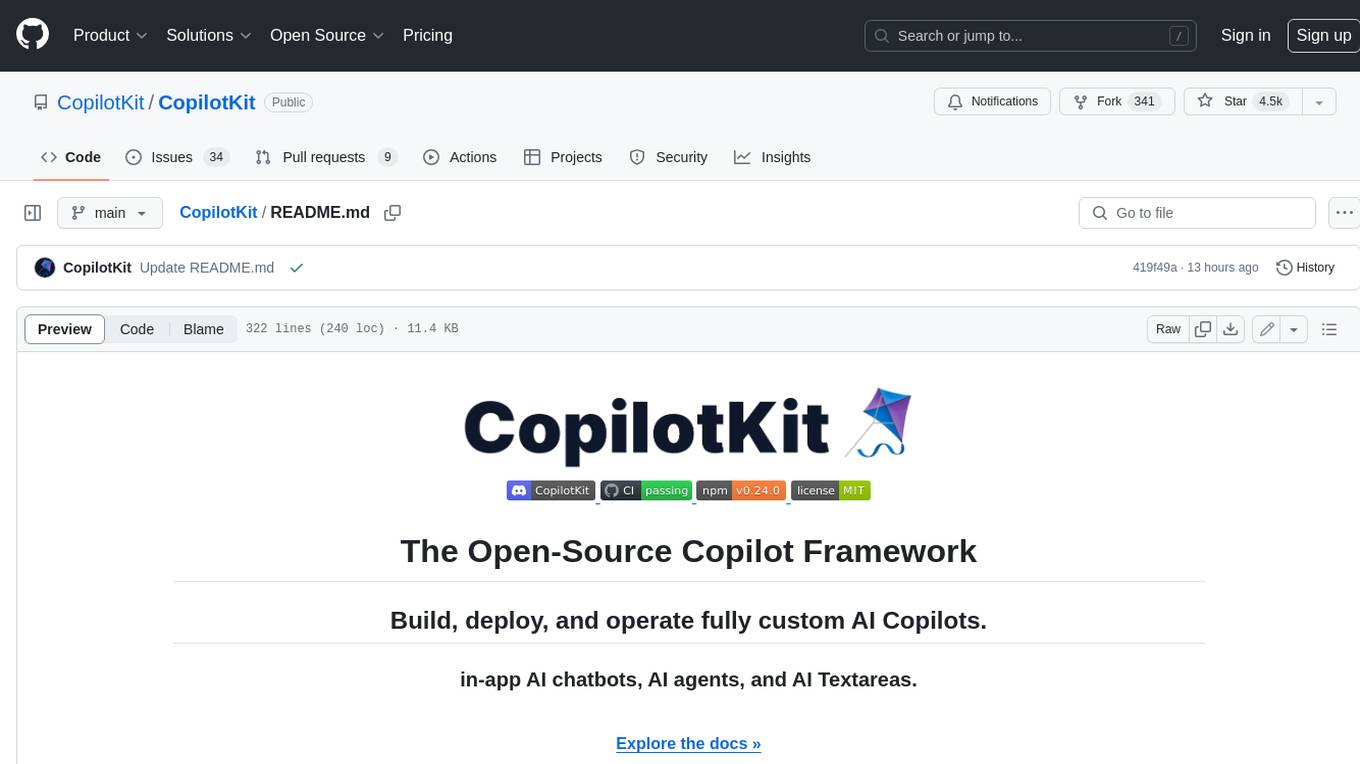
CopilotKit
CopilotKit is an open-source framework for building, deploying, and operating fully custom AI Copilots, including in-app AI chatbots, AI agents, and AI Textareas. It provides a set of components and entry points that allow developers to easily integrate AI capabilities into their applications. CopilotKit is designed to be flexible and extensible, so developers can tailor it to their specific needs. It supports a variety of use cases, including providing app-aware AI chatbots that can interact with the application state and take action, drop-in replacements for textareas with AI-assisted text generation, and in-app agents that can access real-time application context and take action within the application.
For similar jobs
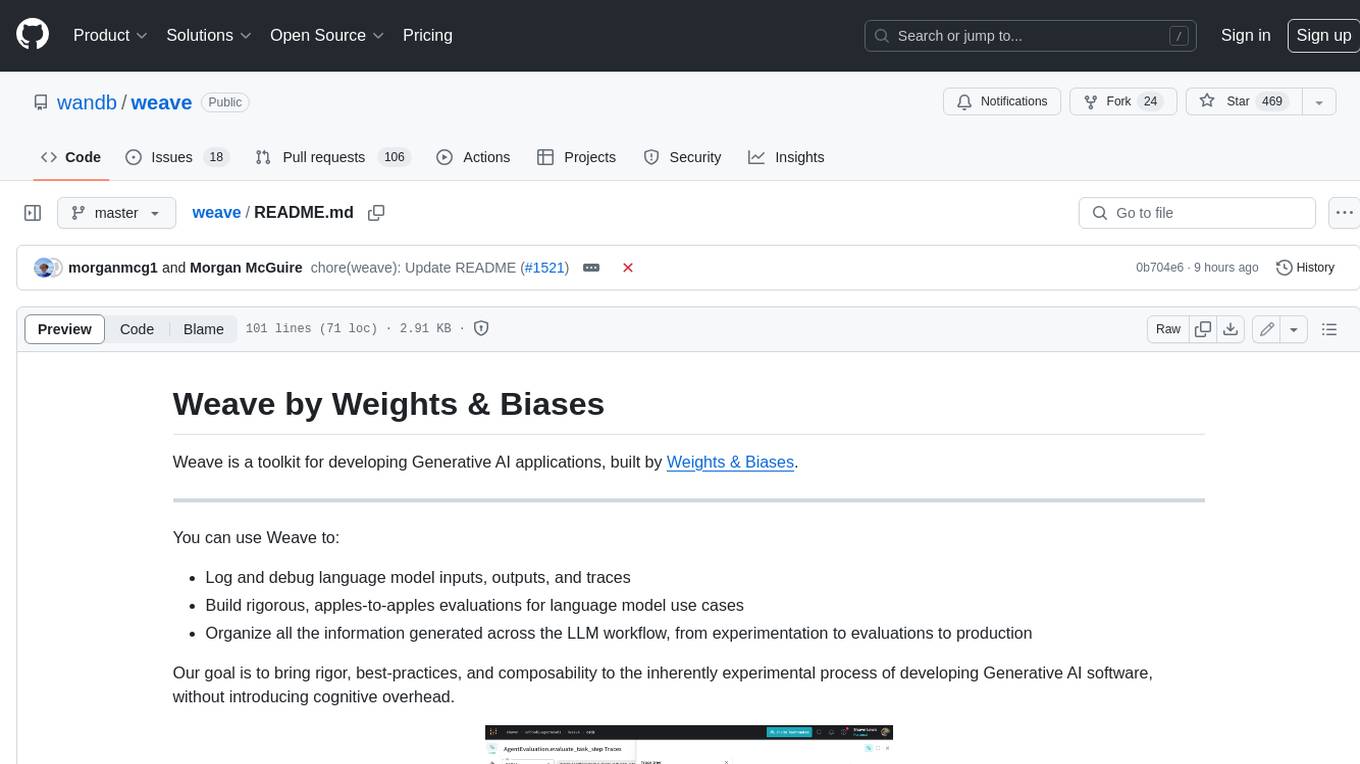
weave
Weave is a toolkit for developing Generative AI applications, built by Weights & Biases. With Weave, you can log and debug language model inputs, outputs, and traces; build rigorous, apples-to-apples evaluations for language model use cases; and organize all the information generated across the LLM workflow, from experimentation to evaluations to production. Weave aims to bring rigor, best-practices, and composability to the inherently experimental process of developing Generative AI software, without introducing cognitive overhead.
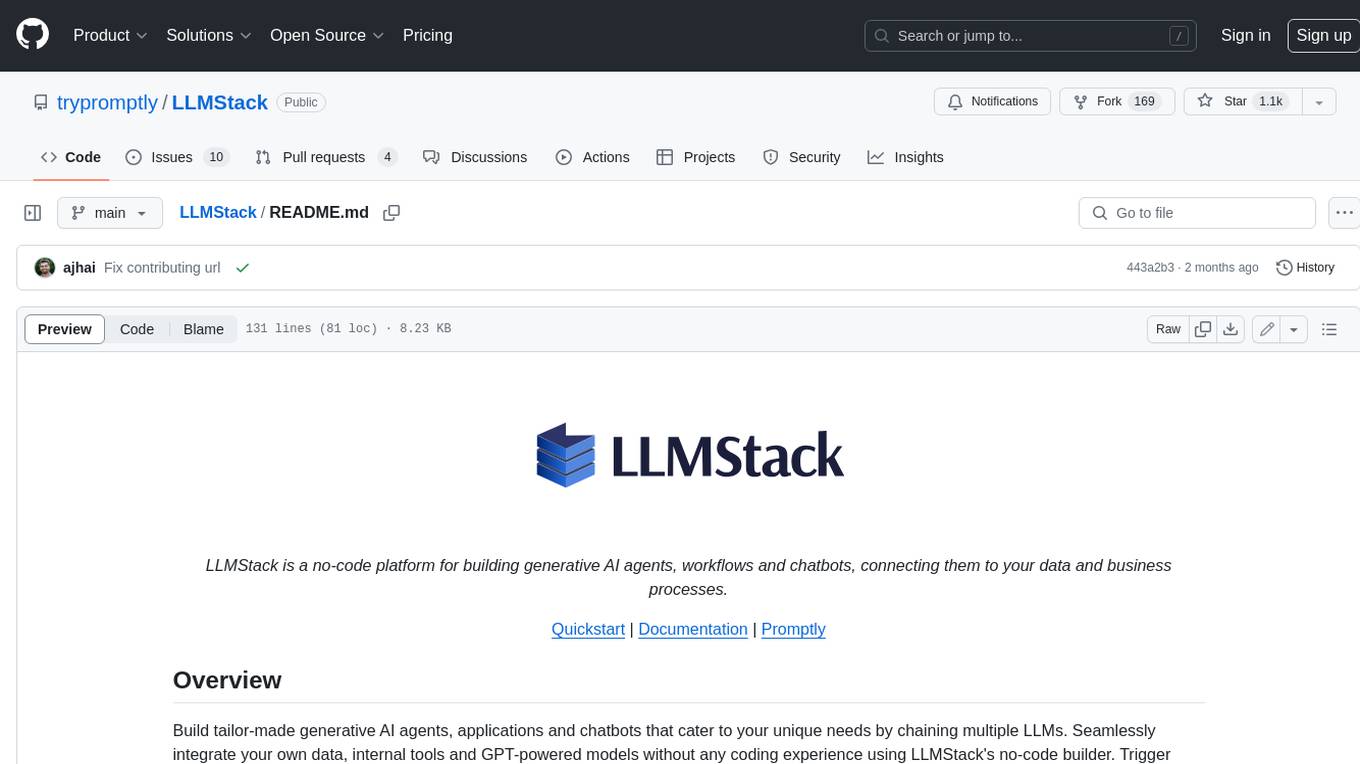
LLMStack
LLMStack is a no-code platform for building generative AI agents, workflows, and chatbots. It allows users to connect their own data, internal tools, and GPT-powered models without any coding experience. LLMStack can be deployed to the cloud or on-premise and can be accessed via HTTP API or triggered from Slack or Discord.
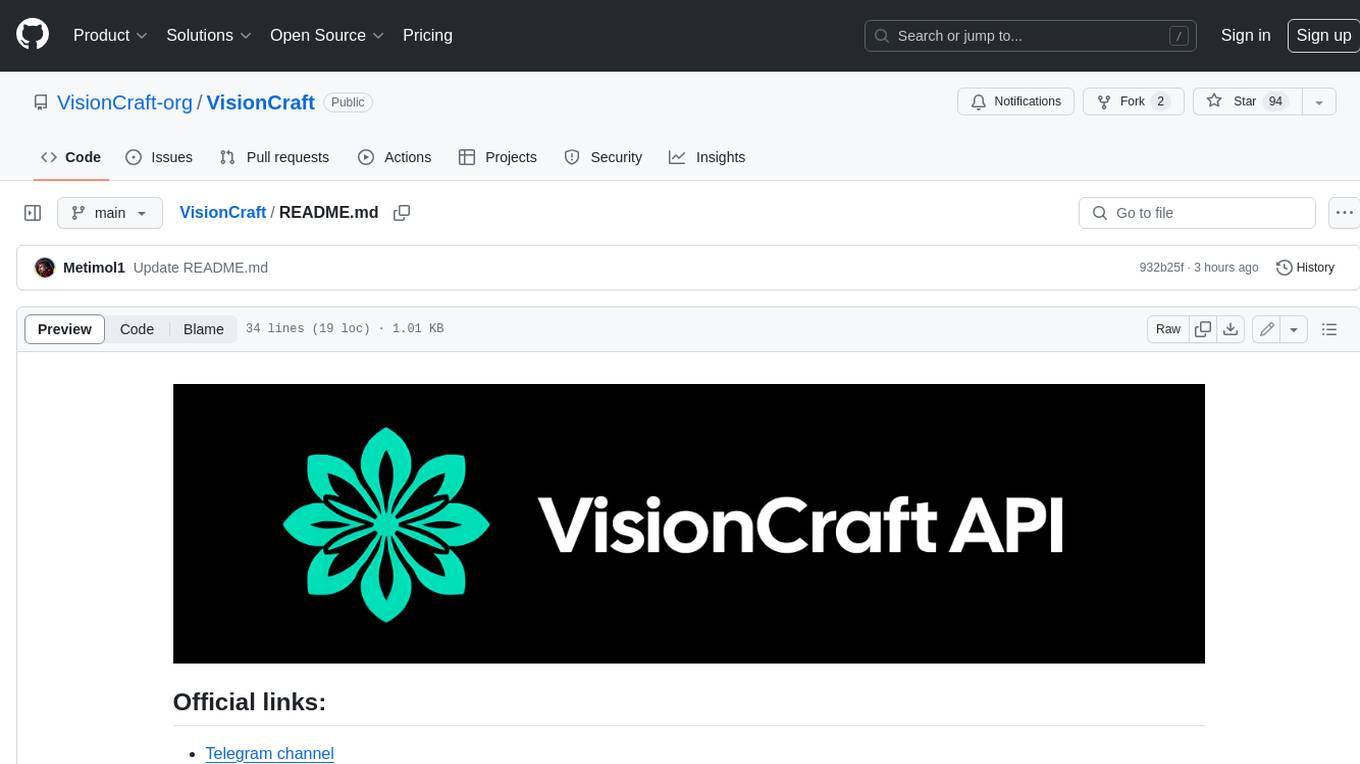
VisionCraft
The VisionCraft API is a free API for using over 100 different AI models. From images to sound.
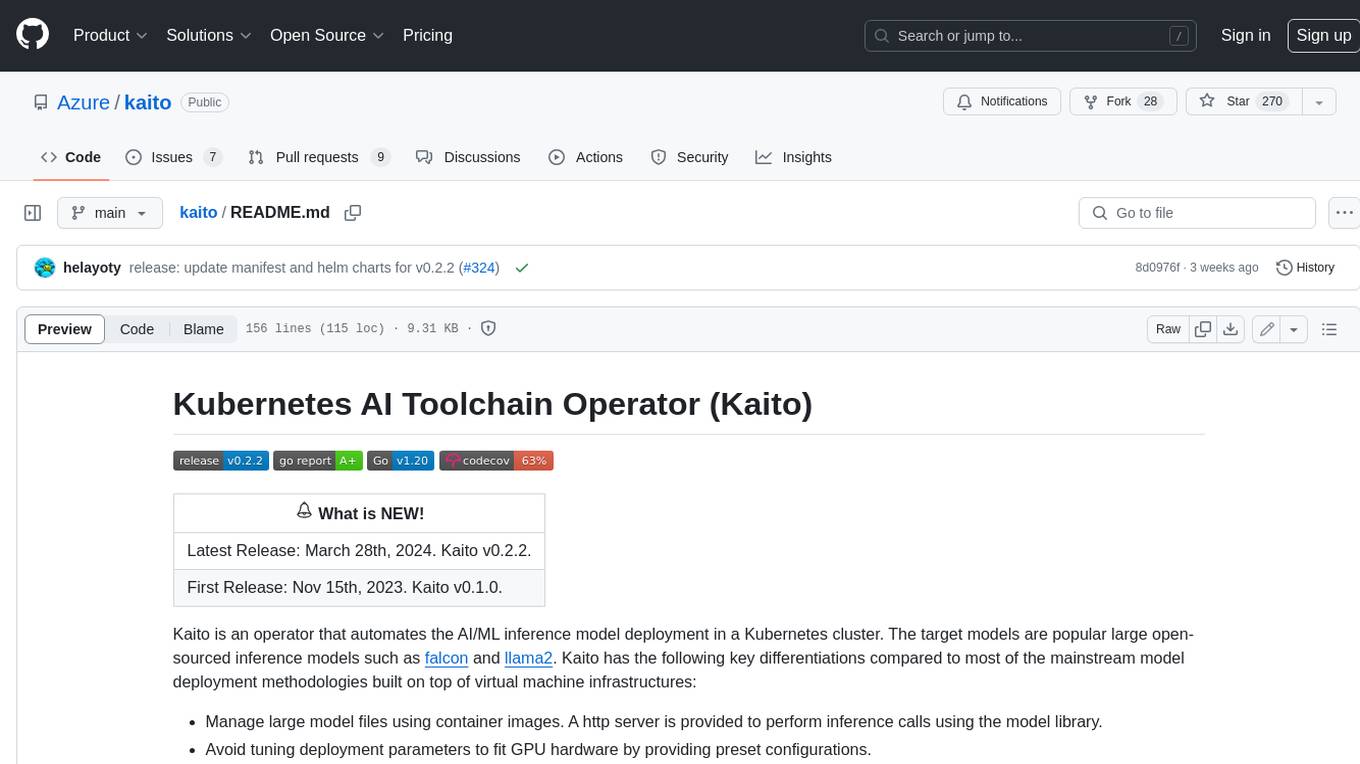
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
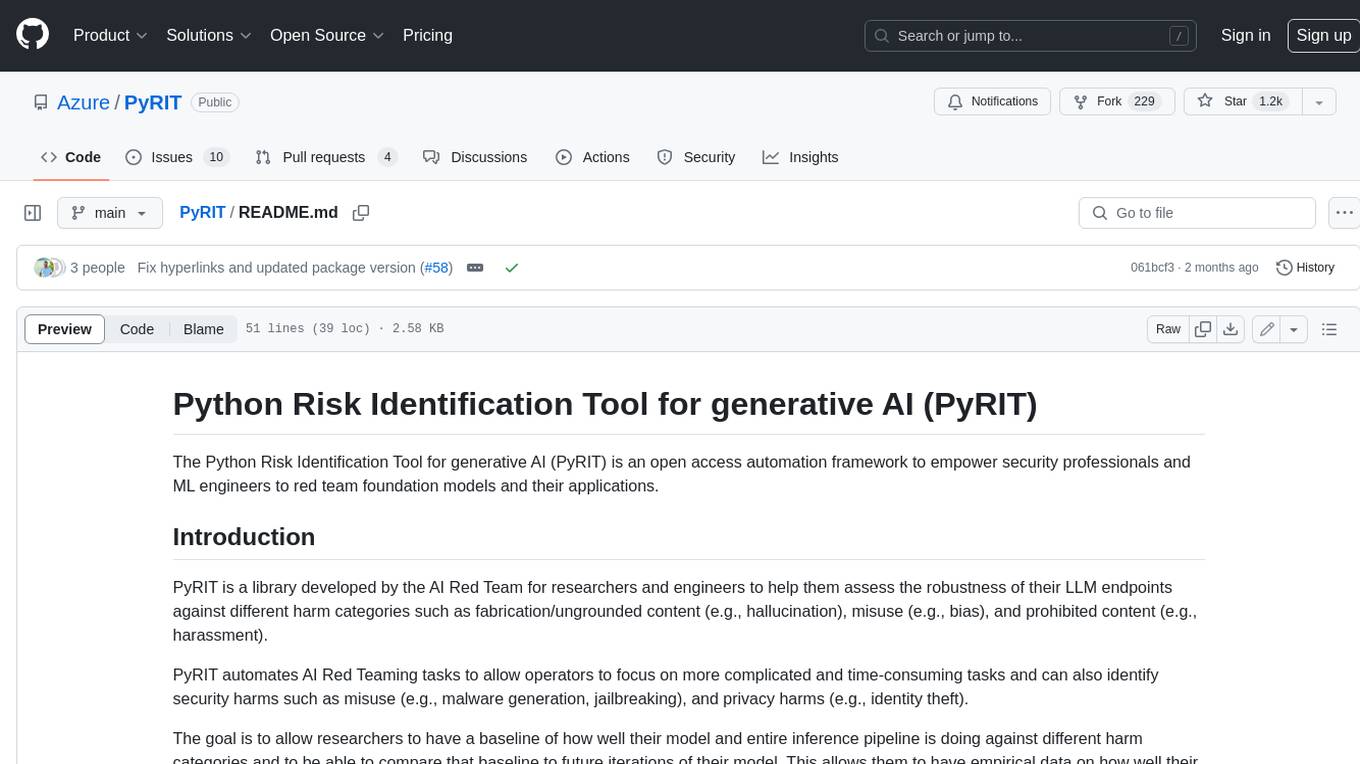
PyRIT
PyRIT is an open access automation framework designed to empower security professionals and ML engineers to red team foundation models and their applications. It automates AI Red Teaming tasks to allow operators to focus on more complicated and time-consuming tasks and can also identify security harms such as misuse (e.g., malware generation, jailbreaking), and privacy harms (e.g., identity theft). The goal is to allow researchers to have a baseline of how well their model and entire inference pipeline is doing against different harm categories and to be able to compare that baseline to future iterations of their model. This allows them to have empirical data on how well their model is doing today, and detect any degradation of performance based on future improvements.
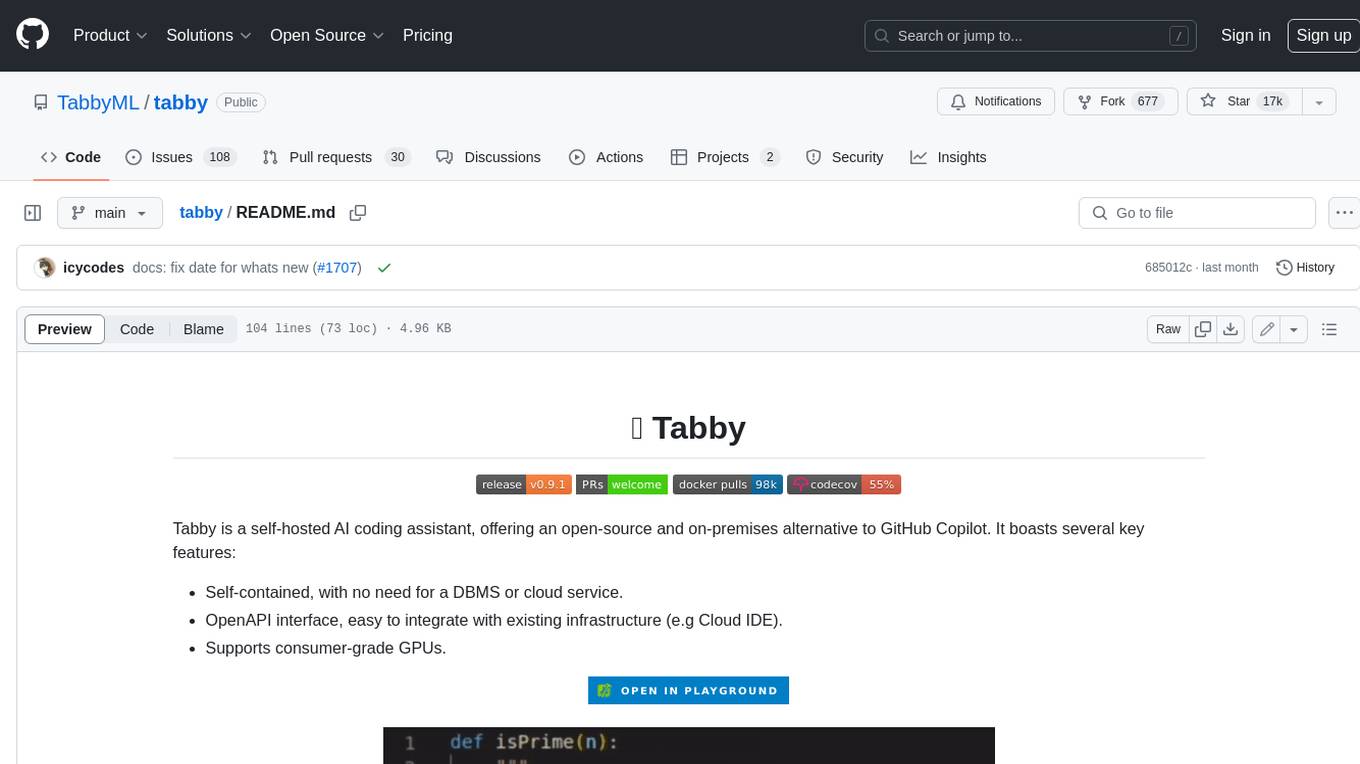
tabby
Tabby is a self-hosted AI coding assistant, offering an open-source and on-premises alternative to GitHub Copilot. It boasts several key features: * Self-contained, with no need for a DBMS or cloud service. * OpenAPI interface, easy to integrate with existing infrastructure (e.g Cloud IDE). * Supports consumer-grade GPUs.
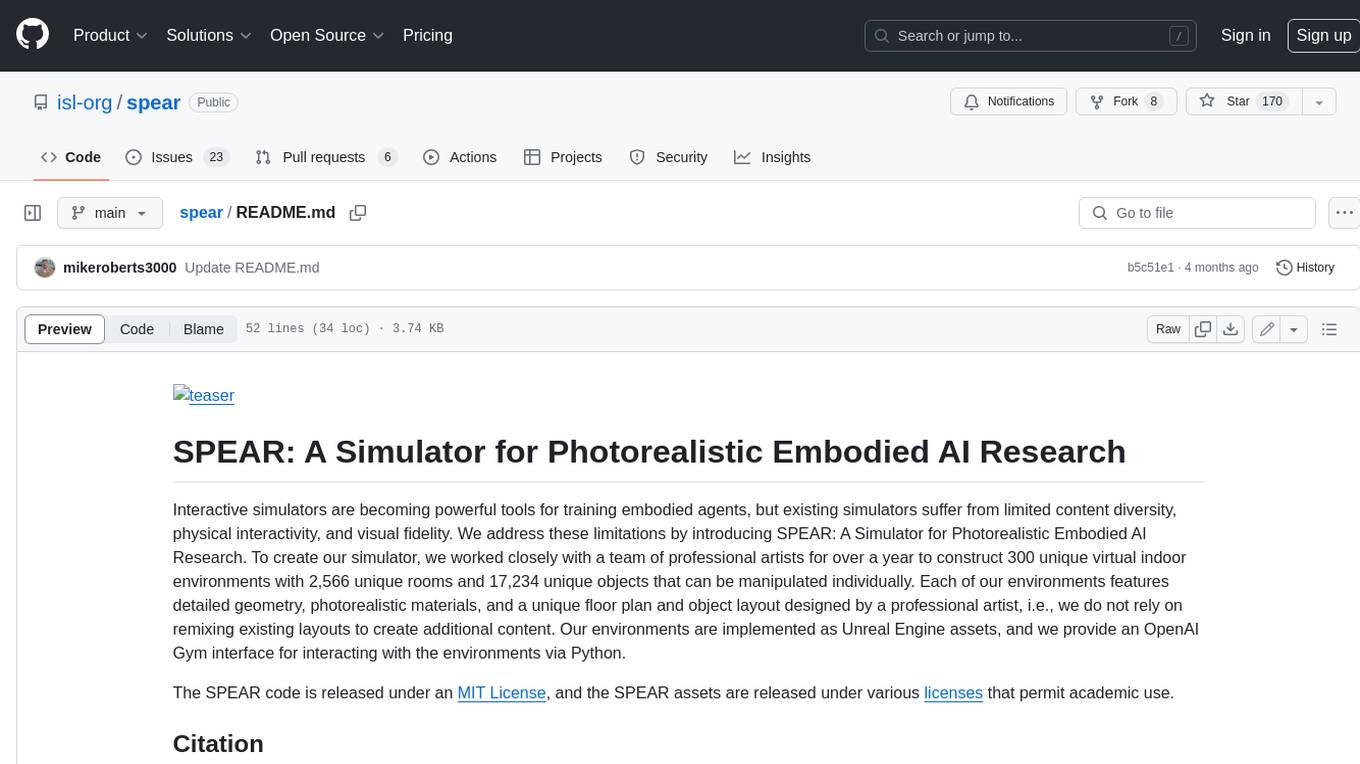
spear
SPEAR (Simulator for Photorealistic Embodied AI Research) is a powerful tool for training embodied agents. It features 300 unique virtual indoor environments with 2,566 unique rooms and 17,234 unique objects that can be manipulated individually. Each environment is designed by a professional artist and features detailed geometry, photorealistic materials, and a unique floor plan and object layout. SPEAR is implemented as Unreal Engine assets and provides an OpenAI Gym interface for interacting with the environments via Python.
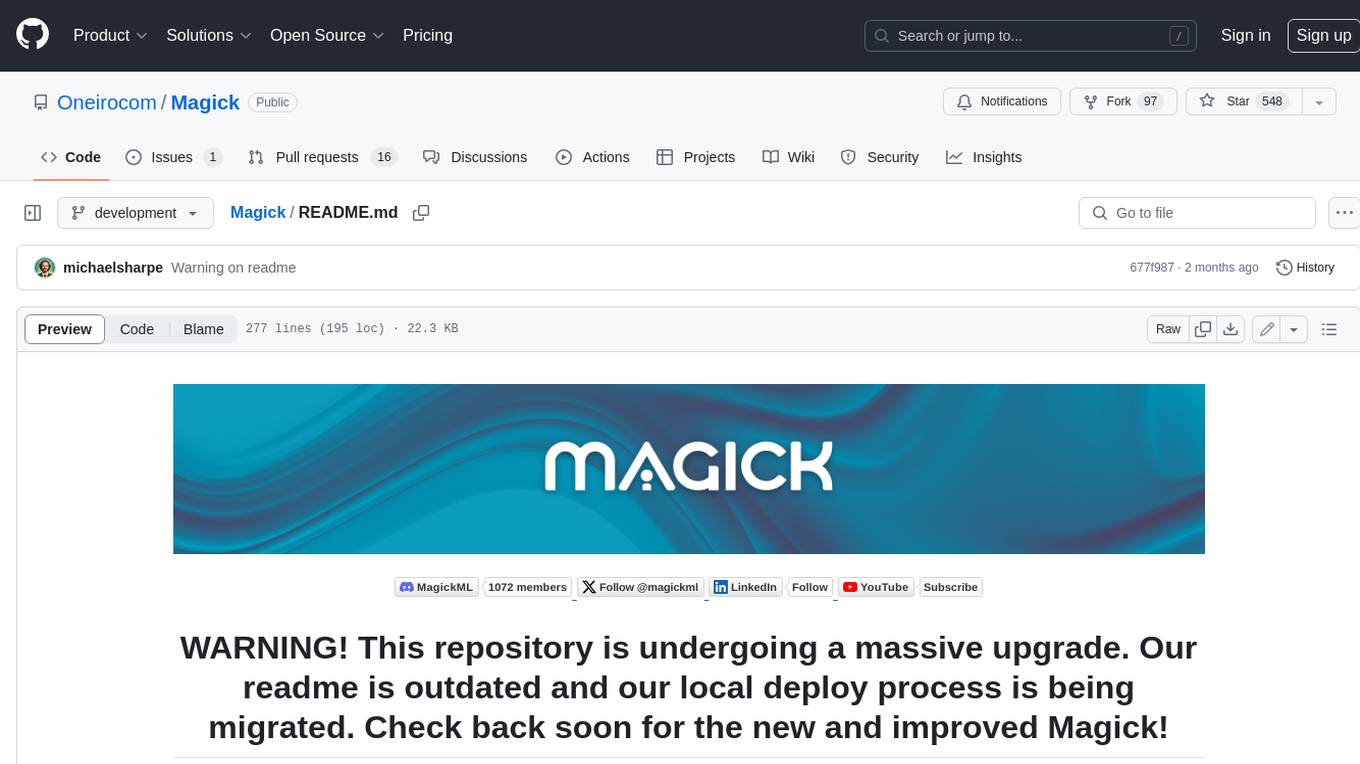
Magick
Magick is a groundbreaking visual AIDE (Artificial Intelligence Development Environment) for no-code data pipelines and multimodal agents. Magick can connect to other services and comes with nodes and templates well-suited for intelligent agents, chatbots, complex reasoning systems and realistic characters.