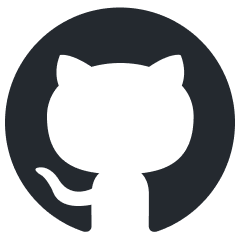
flo-ai
π₯π₯π₯ Simple way to create composable AI agents
Stars: 73
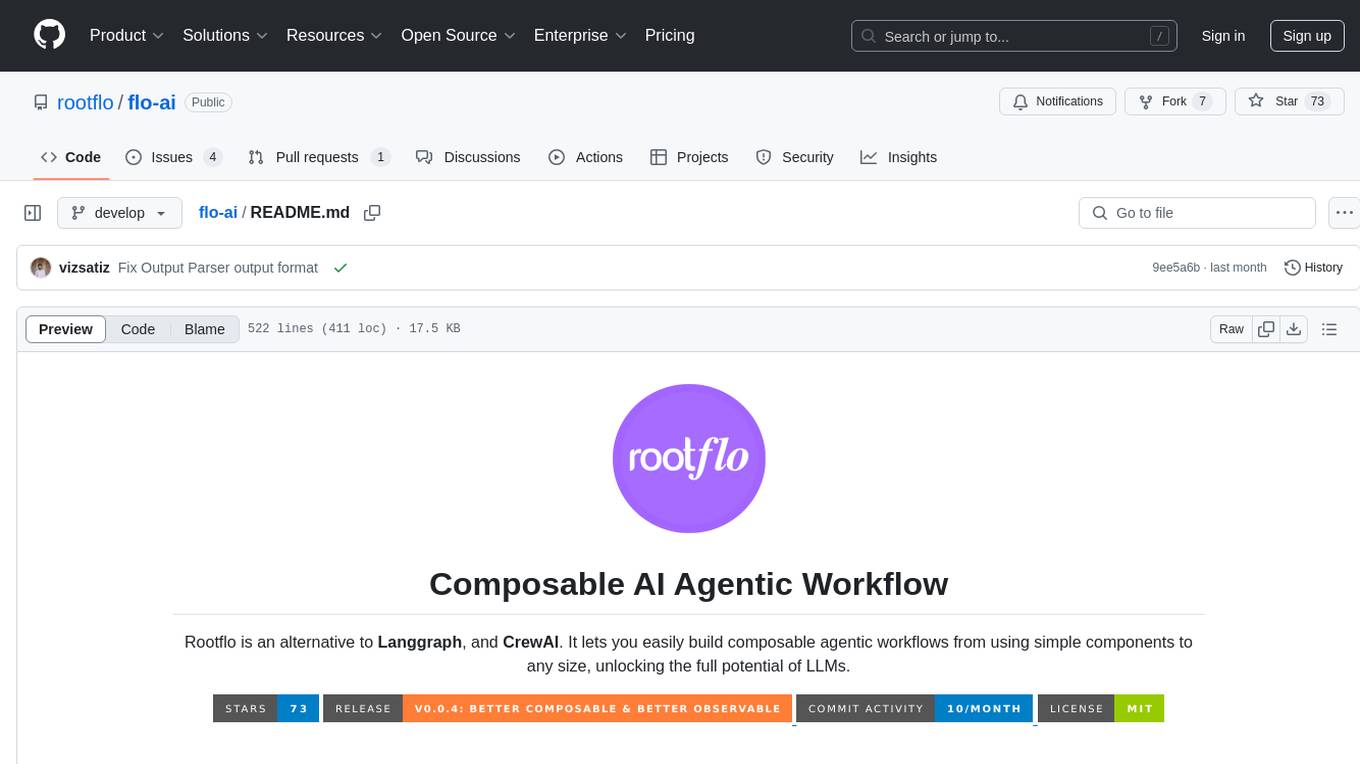
Flo AI is a Python framework that enables users to build production-ready AI agents and teams with minimal code. It allows users to compose complex AI architectures using pre-built components while maintaining the flexibility to create custom components. The framework supports composable, production-ready, YAML-first, and flexible AI systems. Users can easily create AI agents and teams, manage teams of AI agents working together, and utilize built-in support for Retrieval-Augmented Generation (RAG) and compatibility with Langchain tools. Flo AI also provides tools for output parsing and formatting, tool logging, data collection, and JSON output collection. It is MIT Licensed and offers detailed documentation, tutorials, and examples for AI engineers and teams to accelerate development, maintainability, scalability, and testability of AI systems.
README:
Rootflo is an alternative to Langgraph, and CrewAI. It lets you easily build composable agentic workflows from using simple components to any size, unlocking the full potential of LLMs.
Checkout the docs Β»
Github
β’
Website
β’
Roadmap
Build production-ready AI agents and teams with minimal code
Flo AI is a Python framework that makes building production-ready AI agents and teams as easy as writing YAML. Think "Kubernetes for AI Agents" - compose complex AI architectures using pre-built components while maintaining the flexibility to create your own.
- π Truly Composable: Build complex AI systems by combining smaller, reusable components
- ποΈ Production-Ready: Built-in best practices and optimizations for production deployments
- π YAML-First: Define your entire agent architecture in simple YAML
- π§ Flexible: Use pre-built components or create your own
- π€ Team-Oriented: Create and manage teams of AI agents working together
- π RAG Support: Built-in support for Retrieval-Augmented Generation
- π Langchain Compatible: Works with all your favorite Langchain tools
FloAI follows an agent team architecture, where agents are the basic building blocks, and teams can have multiple agents and teams themselves can be part of bigger teams.
Building a working agent or team involves 3 steps:
- Create a session using
FloSession
, and register your tools and models - Define you agent/team/team of teams using yaml or code
- Build and run using
Flo
pip install flo-ai
# or using poetry
poetry add flo-ai
from flo_ai import Flo, FloSession
from langchain_openai import ChatOpenAI
from langchain_community.tools.tavily_search.tool import TavilySearchResults
# init your LLM
llm = ChatOpenAI(temperature=0)
# create a session and register your tools
session = FloSession(llm).register_tool(name="TavilySearchResults", tool=TavilySearchResults())
# define your agent yaml
simple_weather_checking_agent = """
apiVersion: flo/alpha-v1
kind: FloAgent
name: weather-assistant
agent:
name: WeatherAssistant
job: >
Given the city name you are capable of answering the latest whether this time of the year by searching the internet
tools:
- name: InternetSearchTool
"""
flo = Flo.build(session, yaml=simple_weather_checking_agent)
# Start streaming results
for response in flo.stream("Write about recent AI developments"):
print(response)
from flo_ai import FloAgent
session = FloSession(llm)
weather_agent = FloAgent.create(
session=session,
name="WeatherAssistant",
job="Given the city name you are capable of answering the latest whether this time of the year by searching the internet",
tools=[TavilySearchResults()]
)
agent_flo: Flo = Flo.create(session, weather_agent)
result = agent_flo.invoke("Whats the whether in New Delhi, India ?")
from flo_ai import Flo, FloSession
from langchain_openai import ChatOpenAI
from langchain_community.tools.tavily_search.tool import TavilySearchResults
# Define your team in YAML
yaml_config = """
apiVersion: flo/alpha-v1
kind: FloRoutedTeam
name: research-team
team:
name: ResearchTeam
router:
name: TeamLead
kind: supervisor
agents:
- name: Researcher
role: Research Specialist
job: Research latest information on given topics
tools:
- name: TavilySearchResults
- name: Writer
role: Content Creator
job: Create engaging content from research
"""
# Set up and run
llm = ChatOpenAI(temperature=0)
session = FloSession(llm).register_tool(name="TavilySearchResults", tool=TavilySearchResults())
flo = Flo.build(session, yaml=yaml_config)
# Start streaming results
for response in flo.stream("Write about recent AI developments"):
print(response)
Note: You can make each of the above agents including the router to use different models, giving flexibility to combine the power of different LLMs. To know more, check multi-model integration in detailed documentation
from flo_ai import FloSupervisor, FloAgent, FloSession, FloTeam, FloLinear
from langchain_openai import ChatOpenAI
from langchain_community.tools.tavily_search.tool import TavilySearchResults
llm = ChatOpenAI(temperature=0, model_name='gpt-4o')
session = FloSession(llm).register_tool(
name="TavilySearchResults",
tool=TavilySearchResults()
)
researcher = FloAgent.create(
session,
name="Researcher",
role="Internet Researcher", # optional
job="Do a research on the internet and find articles of relevent to the topic asked by the user",
tools=[TavilySearchResults()]
)
blogger = FloAgent.create(
session,
name="BlogWriter",
role="Thought Leader", # optional
job="Able to write a blog using information provided",
tools=[TavilySearchResults()]
)
marketing_team = FloTeam.create(session, "Marketing", [researcher, blogger])
head_of_marketing = FloSupervisor.create(session, "Head-of-Marketing", marketing_team)
marketing_flo = Flo.create(session, routed_team=head_of_marketing)
FloAI supports all the tools built and available in langchain_community
package. To know more these tools, go here.
Along with that FloAI has a decorator @flotool
which makes any function into a tool.
Creating a simple tool using @flotool
:
from flo_ai.tools import flotool
from pydantic import BaseModel, Field
# define argument schema
class AdditionToolInput(BaseModel):
numbers: List[int] = Field(..., description='List of numbers to add')
@flotool(name='AdditionTool', description='Tool to add numbers')
async def addition_tool(numbers: List[int]) -> str:
result = sum(numbers)
await asyncio.sleep(1)
return f'The sum is {result}'
# async tools can also be defined
# when using async tool, while running the flo use async invoke
@flotool(
name='MultiplicationTool',
description='Tool to multiply numbers to get product of numbers',
)
async def mul_tool(numbers: List[int]) -> str:
result = sum(numbers)
await asyncio.sleep(1)
return f'The product is {result}'
# register your tool or use directly in code impl
session.register_tool(name='Adder', tool=addition_tool)
Note: @flotool
comes with inherent error handling capabilities to retry if an exception is thrown. Use unsafe=True
to disable error handling
FloAI now supports output parsing using JSON or YAML formatter. You can now defined your output formatter using pydantic
and use the same in code or directly make it part of the Agent Definition Yaml (ADY)
We have added parser key to your agent schema, which gives you the output. The following is the schema of the parser
name: SchemaName
fields:
- name: field_name
type: data_type
description: field_description
values: <optional(for literals, all possible values that can be taken)>
- value: <the value>
description: value_description
- str: String values
- int: Integer values
- bool: Boolean values
- float: Floating-point values
- array: Lists of items
- object: Nested objects
- literal: Enumerated values
Here an example of a simple summarization agent yaml that produces output a structured manner.
apiVersion: flo/alpha-v1
kind: FloAgent
name: SummarizationFlo
agent:
name: SummaryAgent
kind: llm
role: Book summarizer agent
job: >
You are an given a paragraph from a book
and your job is to understand the information in it and extract summary
parser:
name: BookSummary
fields:
- name: long_summary
type: str
description: A comprehensive summary of the book, with all the major topics discussed
- name: short_summary
type: str
description: A short summary of the book in less than 20 words
As you can see here, the parser
key makes sure that output of this agent will be the given key value format.
You can define parser as json in code and use it easily, here is an example:
format = {
'name': 'NameFormat',
'fields': [
{
'type': 'str',
'description': 'The first name of the person',
'name': 'first_name',
},
{
'type': 'str',
'description': 'The middle name of the person',
'name': 'middle_name',
},
{
'type': 'literal',
'description': 'The last name of the person, the value can be either of Vishnu or Satis',
'name': 'last_name',
'values': [
{'value': 'Vishnu', 'description': 'If the first_name starts with K'},
{'value': 'Satis', 'description': 'If the first_name starts with M'},
],
'default_value_prompt': 'If none of the above value is suited, please use value other than the above in snake-case',
},
],
}
researcher = FloAgent.create(
session,
name='Researcher',
role='Internet Researcher',
job='What is the first name, last name and middle name of the the person user asks about',
tools=[TavilySearchResults()],
parser=FloJsonParser.create(json_dict=format)
)
Flo.set_log_level('DEBUG')
flo: Flo = Flo.create(session, researcher)
result = flo.invoke('Mahatma Gandhi')
Output collector is an infrastructure that helps you collect outputs across multiple agents into single data structure. The most useful collector is a JSON output collector which when combined with output parser gives combined JSON outputs.
Usage:
from flo_ai.state import FloJsonOutputCollector
dc = FloJsonOutputCollector()
# register your collector to the session
session = FloSession(llm).register_tool(
name='InternetSearchTool', tool=TavilySearchResults()
)
simple_reseacher = """
apiVersion: flo/alpha-v1
kind: FloAgent
name: weather-assistant
agent:
name: WeatherAssistant
kind: agentic
job: >
Given the person name, guess the first and last name
tools:
- name: InternetSearchTool
parser:
name: NameFormatter
fields:
- type: str
description: The first name of the person
name: first_name
- type: str
description: The first name of the person
name: last_name
- name: location
type: object
description: The details about birth location
fields:
- name: state
type: str
description: The Indian State in whihc the person was born
data_collector: kv
"""
flo: Flo = Flo.build(session, simple_reseacher)
result = flo.invoke('Gandhi')
# This will output the output as JSON. The idea is that you can use the same collector across multiple agents and teams to still get a combined JSON output.
print(dc.fetch())
FloAI provides built-in capabilities for logging tool calls and collecting data through the FloExecutionLogger
and DataCollector
classes, facilitating the creation of valuable training data.
You can customize DataCollector
implementation according to your database. A sample implementation where logs are stored locally as JSON files is implemented in JSONLFileCollector
.
from flo_ai.callbacks import FloExecutionLogger
from flo_ai.storage.data_collector import JSONLFileCollector
# Initialize the file collector with a path for the JSONL log file
file_collector = JSONLFileCollector("./path/to/my_llm_logs.jsonl")
# Create a tool logger with the collector
local_tracker = FloExecutionLogger(file_collector)
# Register the logger with your session
session.register_callback(local_tracker)
- π Logs all tool calls, chain executions, and agent actions
- π Includes timestamps for start and end of operations
- π Tracks inputs, outputs, and errors
- πΎ Stores data in JSONL format for easy analysis
- π Facilitates the creation of training data from logged interactions
The logger captures detailed information including:
- Tool name and inputs
- Execution timestamps
- Operation status (completed/error)
- Chain and agent activities
- Parent-child relationship between operations
The structured logs provide valuable training data that can be used to:
- Fine-tune LLMs on your specific use cases
- Train new models to replicate successful tool usage patterns
- Create supervised datasets for tool selection and chain optimization
Visit our comprehensive documentation for:
- Detailed tutorials
- Architecture deep-dives
- API reference
- Logging
- Error handling
- Observers
- Dynamic model switching
- Best practices
- Advanced examples
- Faster Development: Build complex AI systems in minutes, not days
- Production Focus: Built-in optimizations and best practices
- Flexibility: Use our components or build your own
- Maintainable: YAML-first approach makes systems easy to understand and modify
- Scalable: From single agents to complex team hierarchies
- Testable: Each component can be tested independently
- π€ Customer Service Automation
- π Data Analysis Pipelines
- π Content Generation
- π Research Automation
- π― Task-Specific AI Teams
We love your input! Check out our Contributing Guide to get started. Ways to contribute:
- π Report bugs
- π‘ Propose new features
- π Improve documentation
- π§ Submit PRs
Flo AI is MIT Licensed.
Built with β€οΈ using:

Flo: π₯π₯π₯ Simple way to create composable AI agents
Unlock the Power of Customizable AI Workflows with FloAIβs Intuitive and Flexible Agentic Framework

Build an Agentic AI customer support bot using FloAI
We built an open-source agentic AI workflow builder named FloAI and used it to create an agentic customer support agent.

Build an Agentic RAG using FloAI in minutes
FloAI has just made implementing agentic RAG simple and easy to manage

Mastering AI Interaction Logging and Data Collection with FloAI
Learn how to leverage FloAI's powerful logging system for debugging, training data generation, and system optimization
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for flo-ai
Similar Open Source Tools
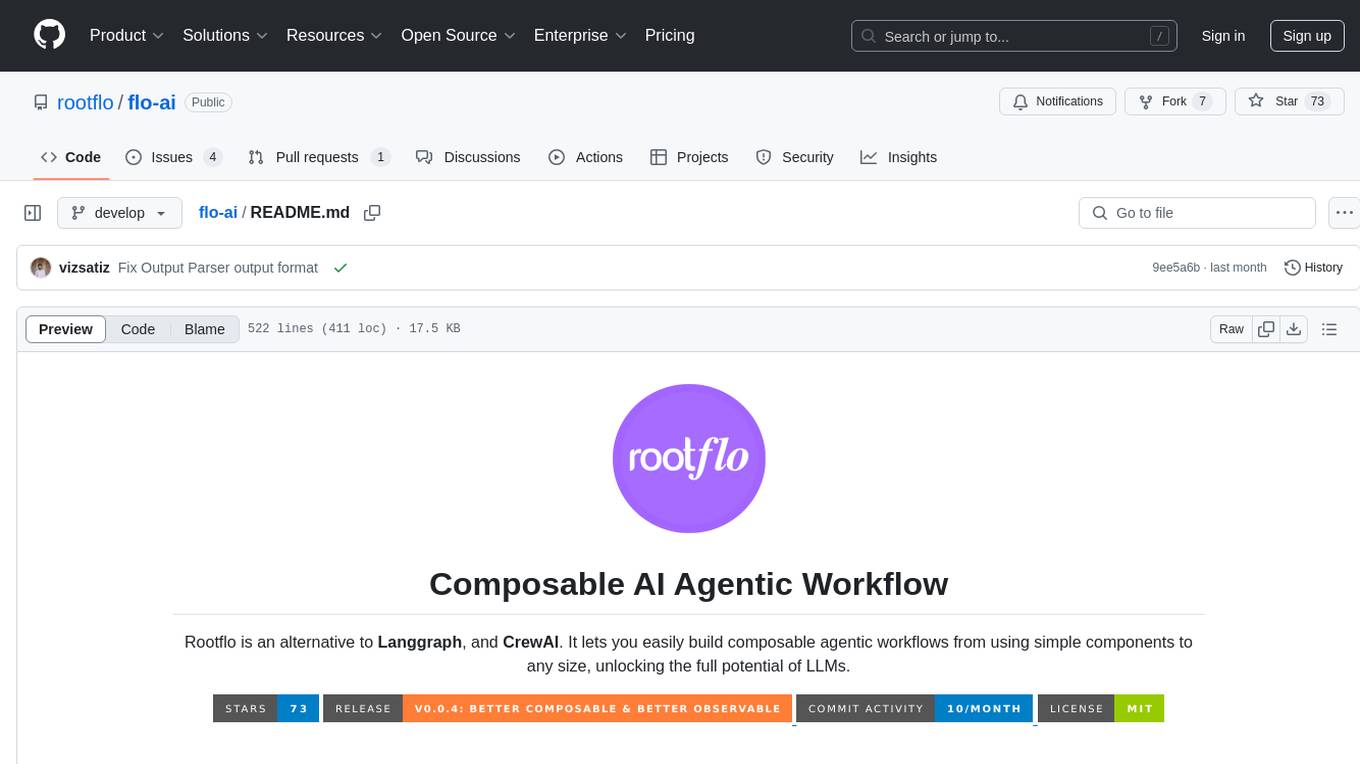
flo-ai
Flo AI is a Python framework that enables users to build production-ready AI agents and teams with minimal code. It allows users to compose complex AI architectures using pre-built components while maintaining the flexibility to create custom components. The framework supports composable, production-ready, YAML-first, and flexible AI systems. Users can easily create AI agents and teams, manage teams of AI agents working together, and utilize built-in support for Retrieval-Augmented Generation (RAG) and compatibility with Langchain tools. Flo AI also provides tools for output parsing and formatting, tool logging, data collection, and JSON output collection. It is MIT Licensed and offers detailed documentation, tutorials, and examples for AI engineers and teams to accelerate development, maintainability, scalability, and testability of AI systems.
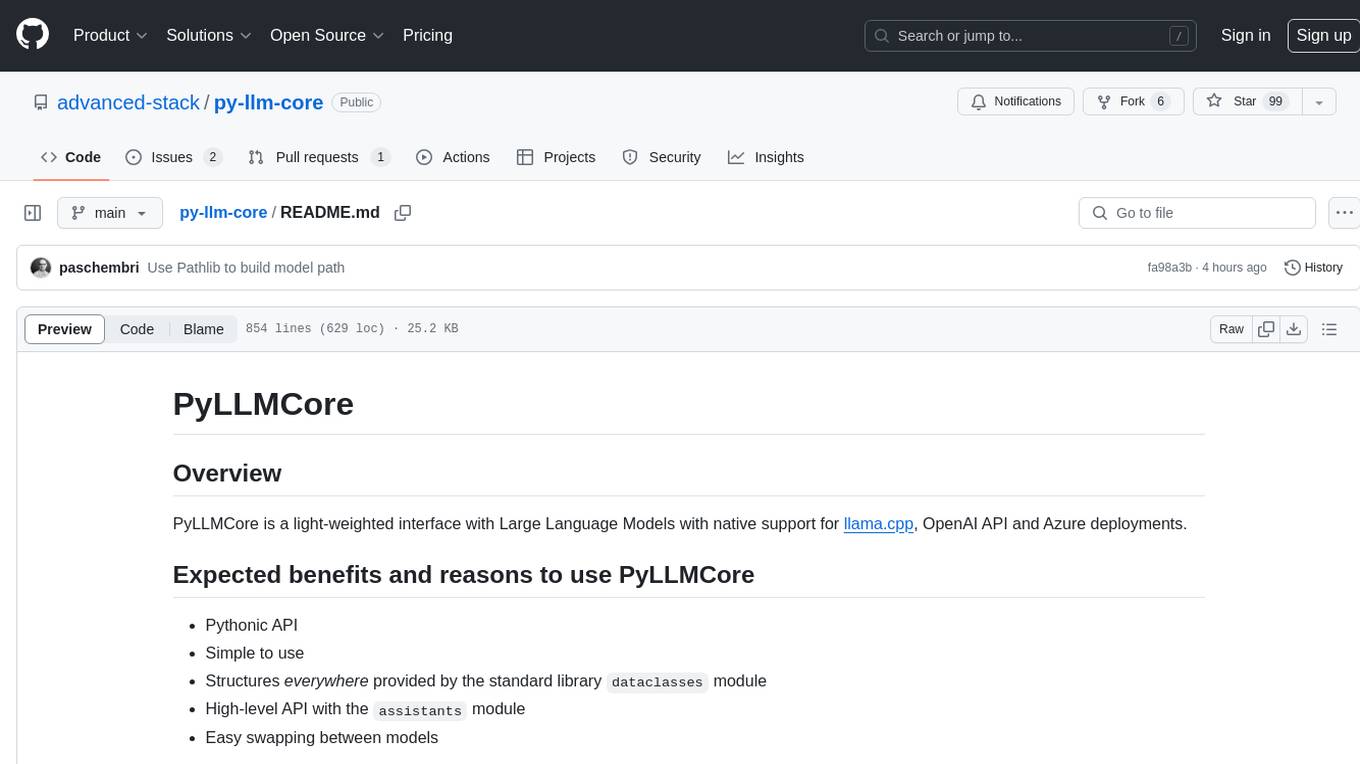
py-llm-core
PyLLMCore is a light-weighted interface with Large Language Models with native support for llama.cpp, OpenAI API, and Azure deployments. It offers a Pythonic API that is simple to use, with structures provided by the standard library dataclasses module. The high-level API includes the assistants module for easy swapping between models. PyLLMCore supports various models including those compatible with llama.cpp, OpenAI, and Azure APIs. It covers use cases such as parsing, summarizing, question answering, hallucinations reduction, context size management, and tokenizing. The tool allows users to interact with language models for tasks like parsing text, summarizing content, answering questions, reducing hallucinations, managing context size, and tokenizing text.
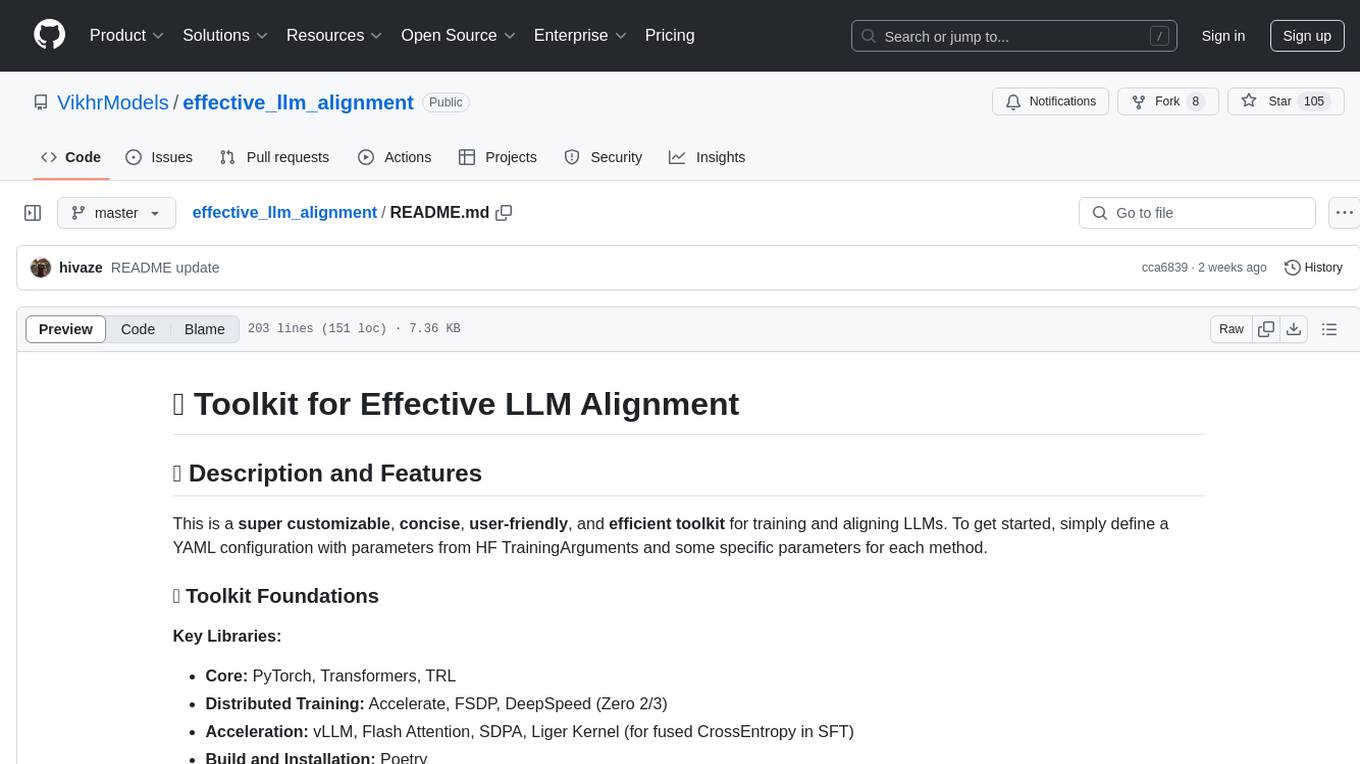
effective_llm_alignment
This is a super customizable, concise, user-friendly, and efficient toolkit for training and aligning LLMs. It provides support for various methods such as SFT, Distillation, DPO, ORPO, CPO, SimPO, SMPO, Non-pair Reward Modeling, Special prompts basket format, Rejection Sampling, Scoring using RM, Effective FAISS Map-Reduce Deduplication, LLM scoring using RM, NER, CLIP, Classification, and STS. The toolkit offers key libraries like PyTorch, Transformers, TRL, Accelerate, FSDP, DeepSpeed, and tools for result logging with wandb or clearml. It allows mixing datasets, generation and logging in wandb/clearml, vLLM batched generation, and aligns models using the SMPO method.
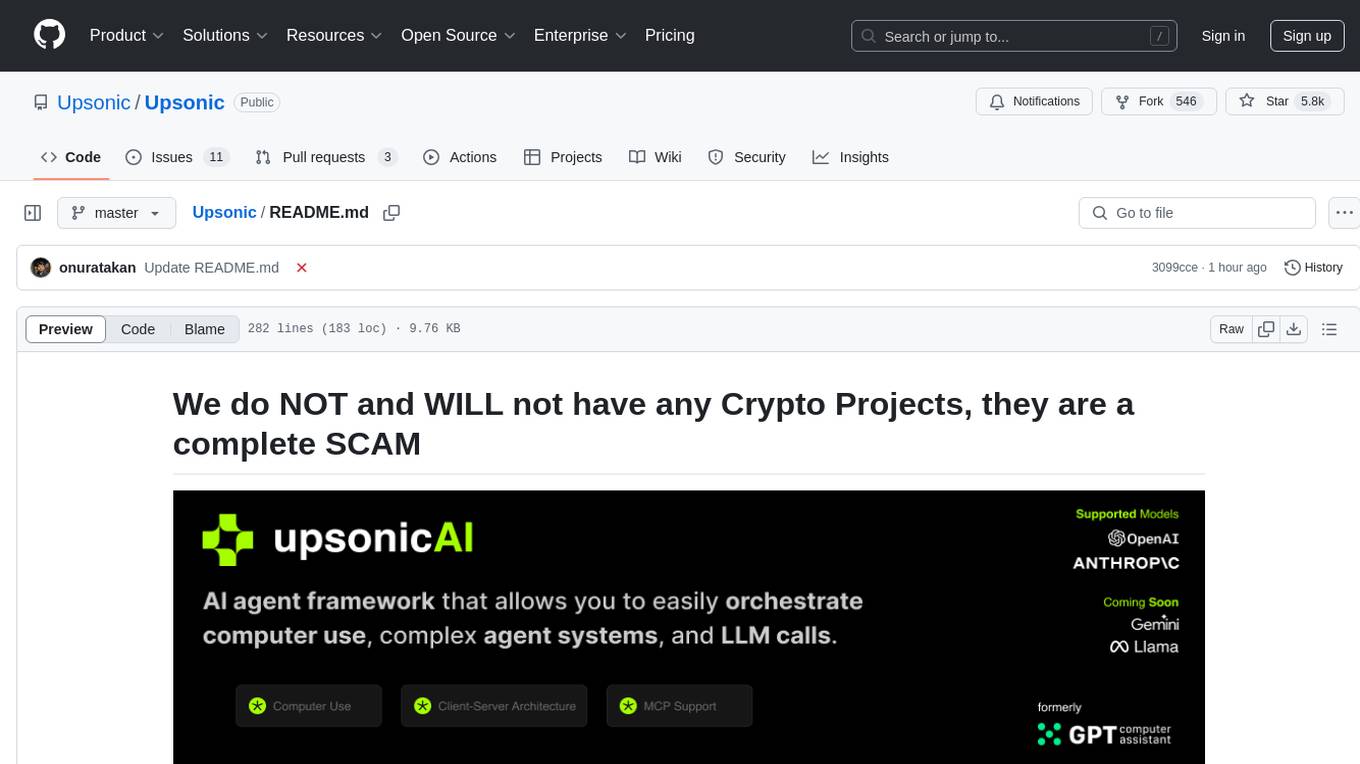
Upsonic
Upsonic offers a cutting-edge enterprise-ready framework for orchestrating LLM calls, agents, and computer use to complete tasks cost-effectively. It provides reliable systems, scalability, and a task-oriented structure for real-world cases. Key features include production-ready scalability, task-centric design, MCP server support, tool-calling server, computer use integration, and easy addition of custom tools. The framework supports client-server architecture and allows seamless deployment on AWS, GCP, or locally using Docker.
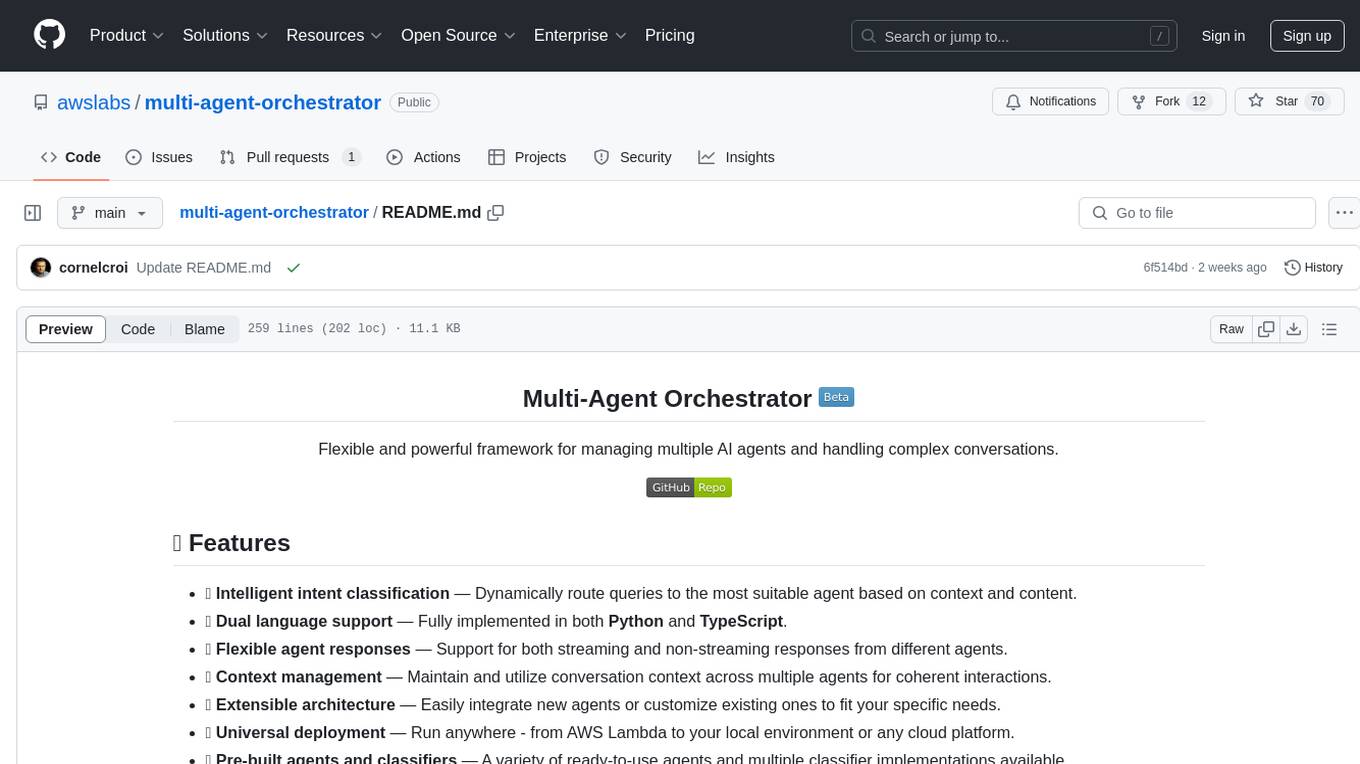
multi-agent-orchestrator
Multi-Agent Orchestrator is a flexible and powerful framework for managing multiple AI agents and handling complex conversations. It intelligently routes queries to the most suitable agent based on context and content, supports dual language implementation in Python and TypeScript, offers flexible agent responses, context management across agents, extensible architecture for customization, universal deployment options, and pre-built agents and classifiers. It is suitable for various applications, from simple chatbots to sophisticated AI systems, accommodating diverse requirements and scaling efficiently.
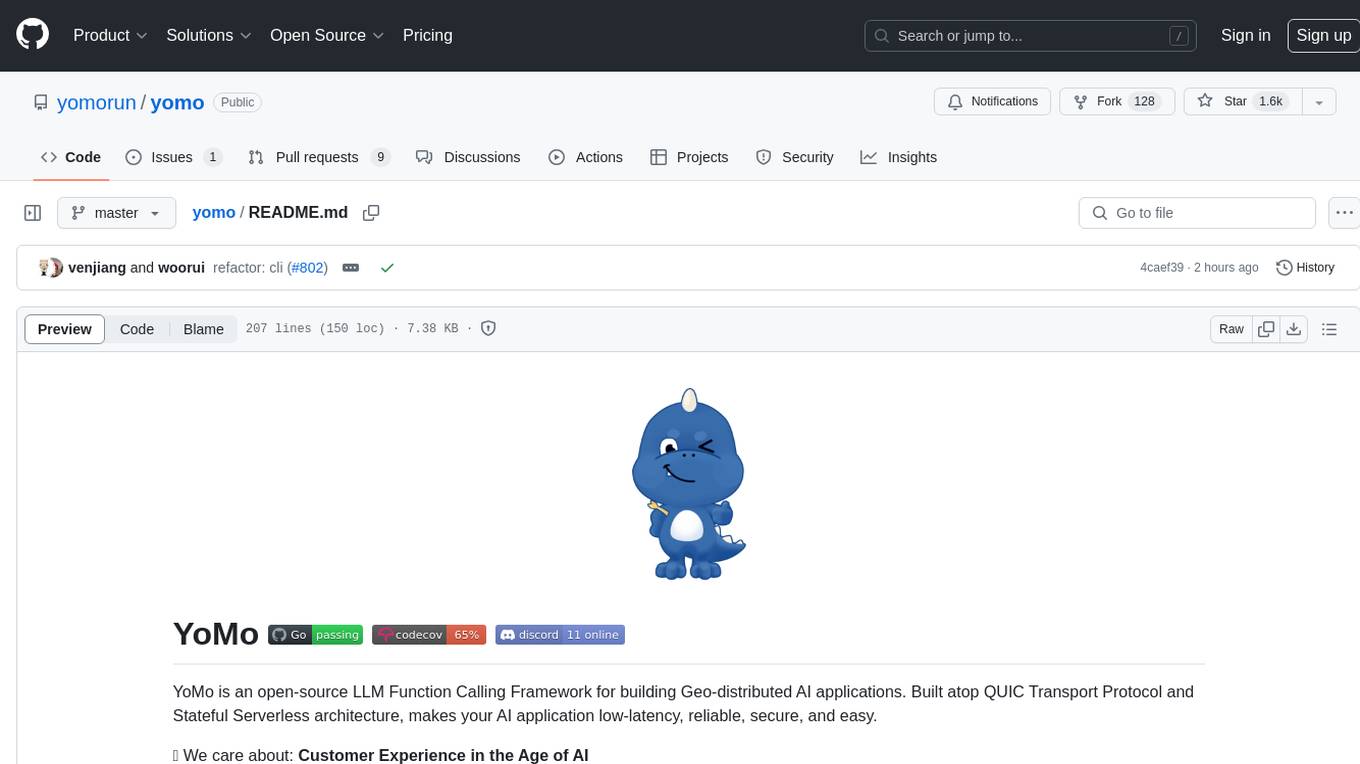
yomo
YoMo is an open-source LLM Function Calling Framework for building Geo-distributed AI applications. It is built atop QUIC Transport Protocol and Stateful Serverless architecture, making AI applications low-latency, reliable, secure, and easy. The framework focuses on providing low-latency, secure, stateful serverless functions that can be distributed geographically to bring AI inference closer to end users. It offers features such as low-latency communication, security with TLS v1.3, stateful serverless functions for faster GPU processing, geo-distributed architecture, and a faster-than-real-time codec called Y3. YoMo enables developers to create and deploy stateful serverless functions for AI inference in a distributed manner, ensuring quick responses to user queries from various locations worldwide.
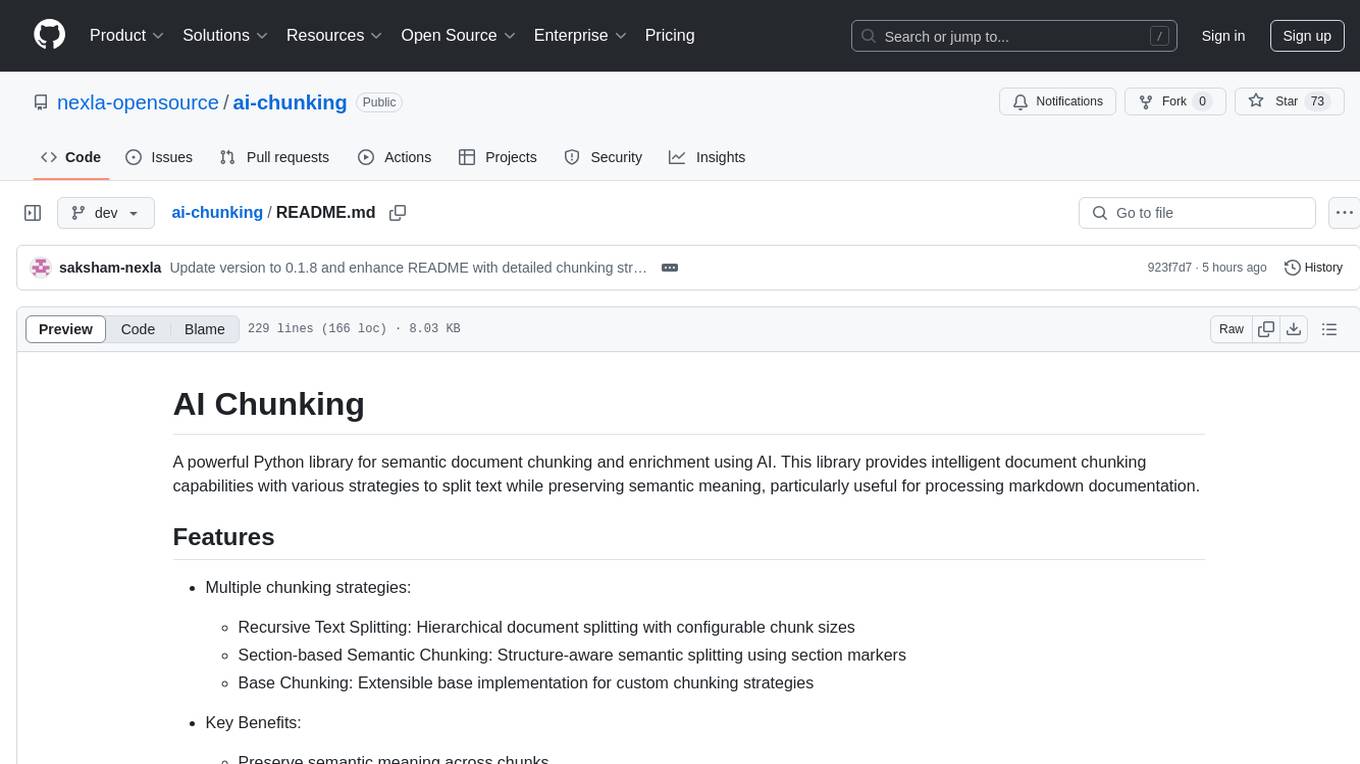
ai-chunking
AI Chunking is a powerful Python library for semantic document chunking and enrichment using AI. It provides intelligent document chunking capabilities with various strategies to split text while preserving semantic meaning, particularly useful for processing markdown documentation. The library offers multiple chunking strategies such as Recursive Text Splitting, Section-based Semantic Chunking, and Base Chunking. Users can configure chunk sizes, overlap, and support various text formats. The tool is easy to extend with custom chunking strategies, making it versatile for different document processing needs.
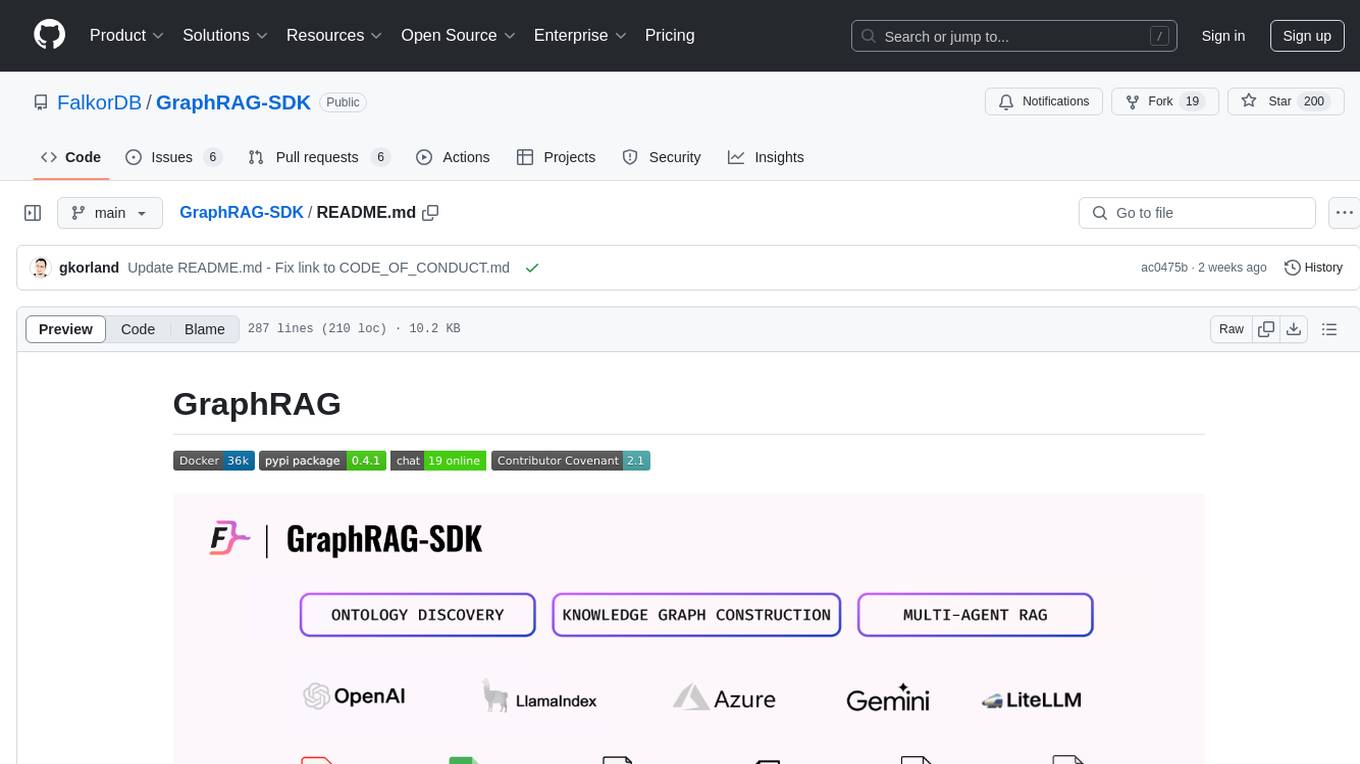
GraphRAG-SDK
Build fast and accurate GenAI applications with GraphRAG SDK, a specialized toolkit for building Graph Retrieval-Augmented Generation (GraphRAG) systems. It integrates knowledge graphs, ontology management, and state-of-the-art LLMs to deliver accurate, efficient, and customizable RAG workflows. The SDK simplifies the development process by automating ontology creation, knowledge graph agent creation, and query handling, enabling users to interact and query their knowledge graphs effectively. It supports multi-agent systems and orchestrates agents specialized in different domains. The SDK is optimized for FalkorDB, ensuring high performance and scalability for large-scale applications. By leveraging knowledge graphs, it enables semantic relationships and ontology-driven queries that go beyond standard vector similarity, enhancing retrieval-augmented generation capabilities.
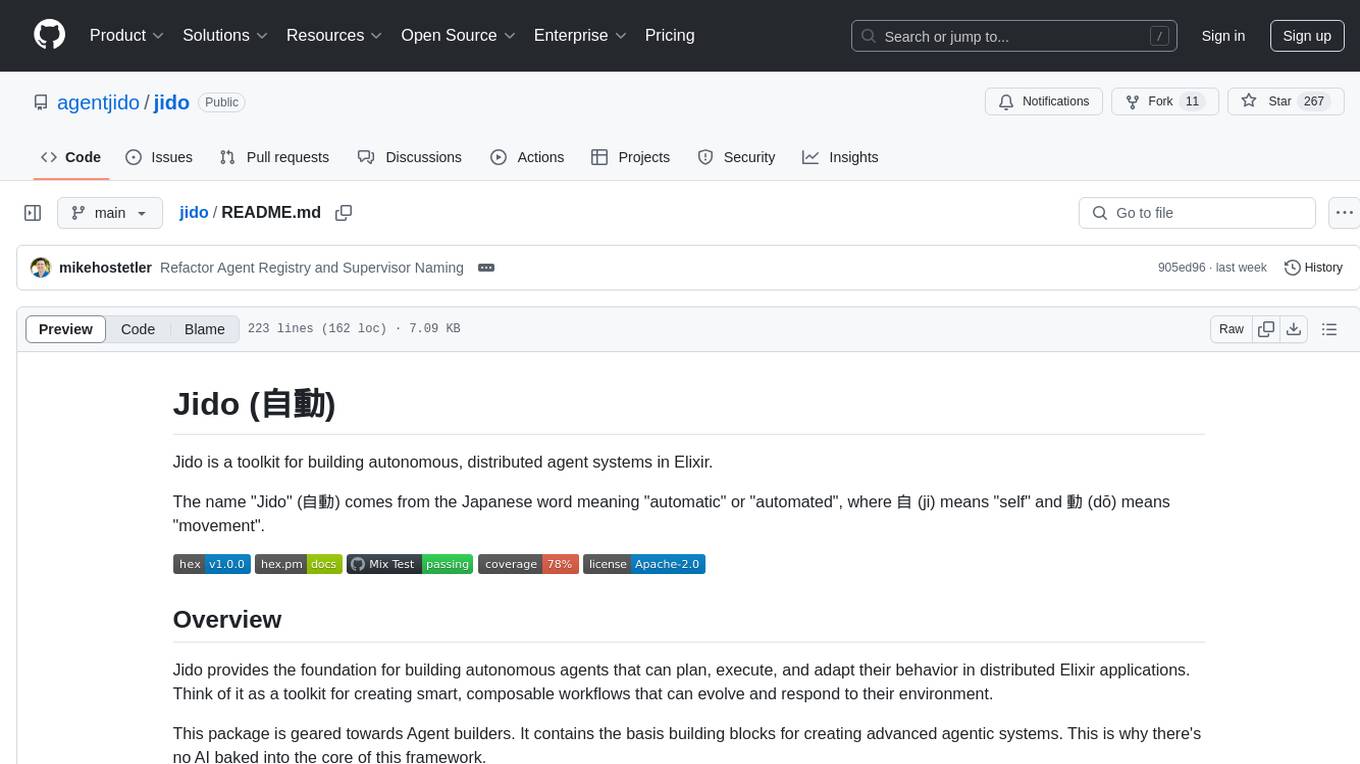
jido
Jido is a toolkit for building autonomous, distributed agent systems in Elixir. It provides the foundation for creating smart, composable workflows that can evolve and respond to their environment. Geared towards Agent builders, it contains core state primitives, composable actions, agent data structures, real-time sensors, signal system, skills, and testing tools. Jido is designed for multi-node Elixir clusters and offers rich helpers for unit and property-based testing.
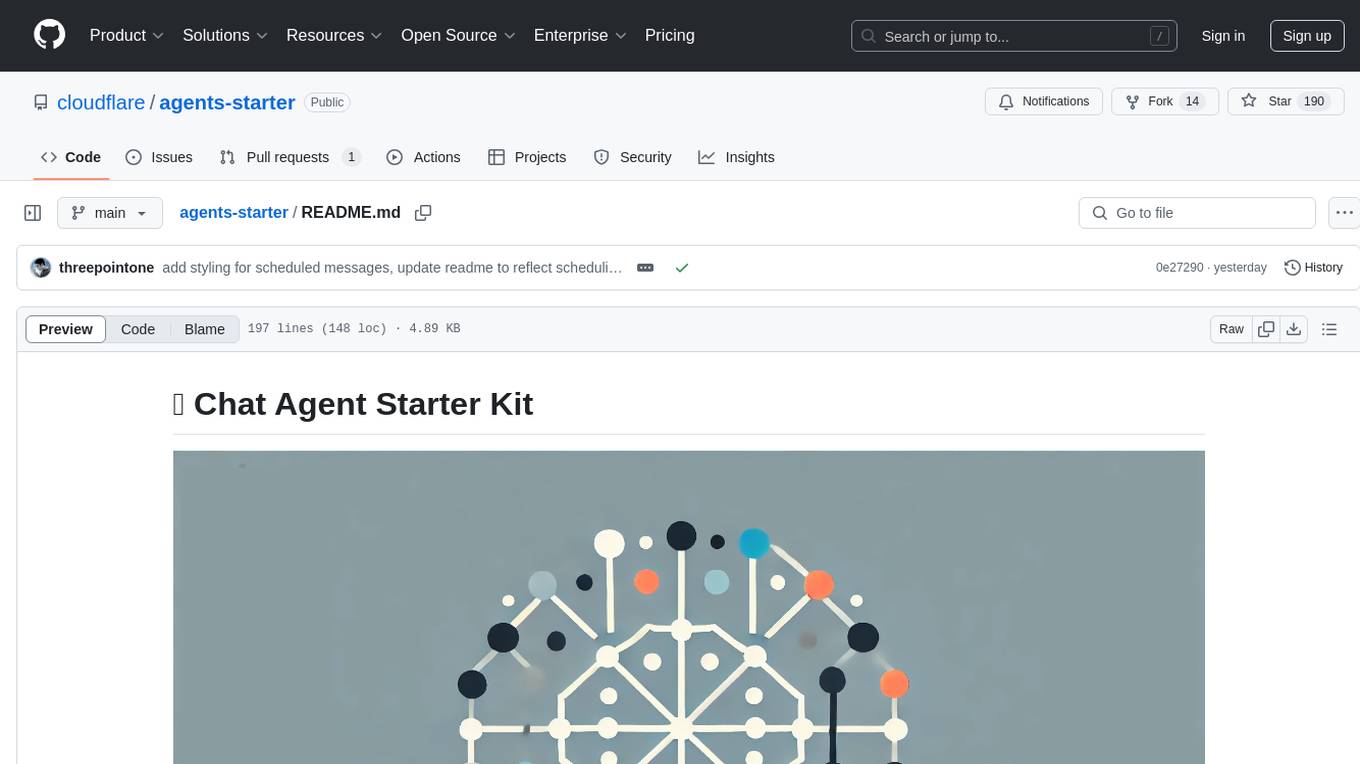
agents-starter
A starter template for building AI-powered chat agents using Cloudflare's Agent platform, powered by agents-sdk. It provides a foundation for creating interactive chat experiences with AI, complete with a modern UI and tool integration capabilities. Features include interactive chat interface with AI, built-in tool system with human-in-the-loop confirmation, advanced task scheduling, dark/light theme support, real-time streaming responses, state management, and chat history. Prerequisites include a Cloudflare account and OpenAI API key. The project structure includes components for chat UI implementation, chat agent logic, tool definitions, and helper functions. Customization guide covers adding new tools, modifying the UI, and example use cases for customer support, development assistant, data analysis assistant, personal productivity assistant, and scheduling assistant.
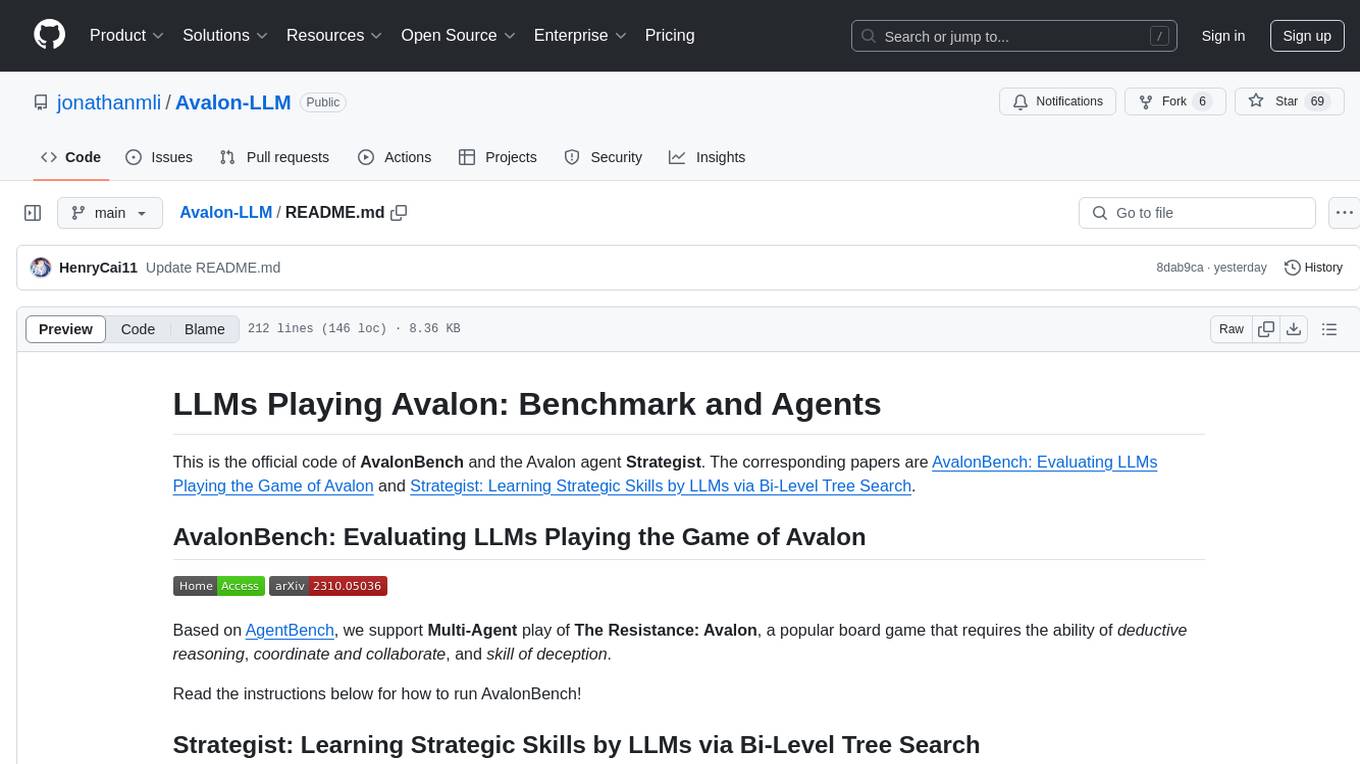
Avalon-LLM
Avalon-LLM is a repository containing the official code for AvalonBench and the Avalon agent Strategist. AvalonBench evaluates Large Language Models (LLMs) playing The Resistance: Avalon, a board game requiring deductive reasoning, coordination, collaboration, and deception skills. Strategist utilizes LLMs to learn strategic skills through self-improvement, including high-level strategic evaluation and low-level execution guidance. The repository provides instructions for running AvalonBench, setting up Strategist, and conducting experiments with different agents in the game environment.
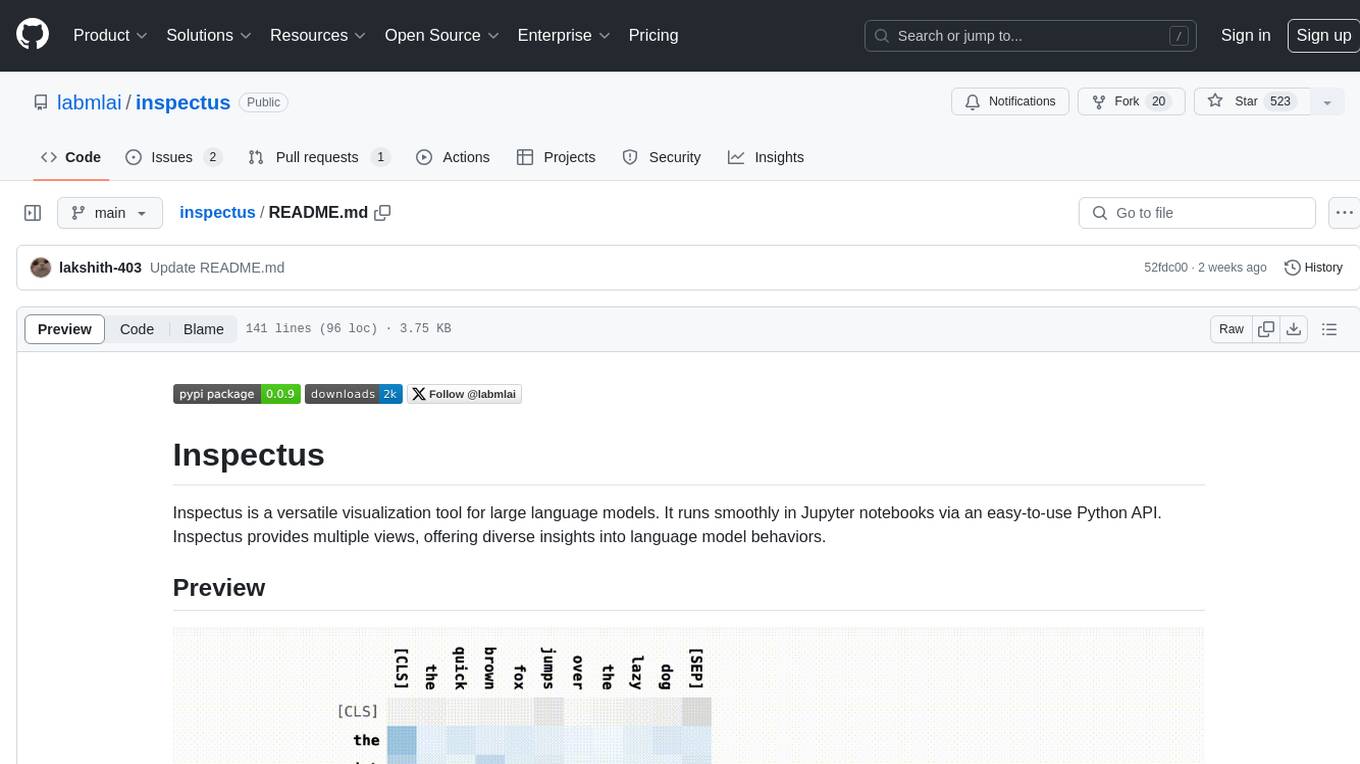
inspectus
Inspectus is a versatile visualization tool for large language models. It provides multiple views, including Attention Matrix, Query Token Heatmap, Key Token Heatmap, and Dimension Heatmap, to offer insights into language model behaviors. Users can interact with the tool in Jupyter notebooks through an easy-to-use Python API. Inspectus allows users to visualize attention scores between tokens, analyze how tokens focus on each other during processing, and explore the relationships between query and key tokens. The tool supports the visualization of attention maps from Huggingface transformers and custom attention maps, making it a valuable resource for researchers and developers working with language models.
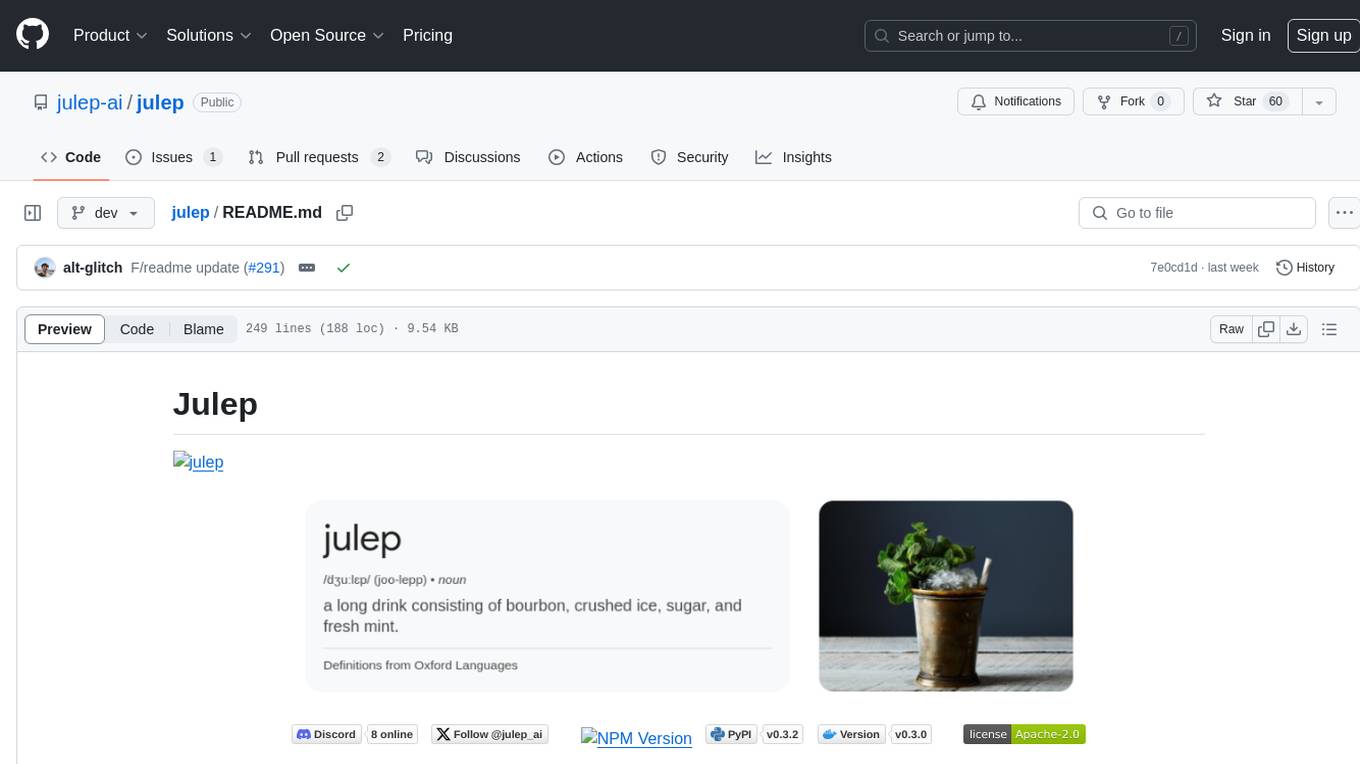
julep
Julep is an advanced platform for creating stateful and functional AI apps powered by large language models. It offers features like statefulness by design, automatic function calling, production-ready deployment, cron-like asynchronous functions, 90+ built-in tools, and the ability to switch between different LLMs easily. Users can build AI applications without the need to write code for embedding, saving, and retrieving conversation history, and can connect to third-party applications using Composio. Julep simplifies the process of getting started with AI apps, whether they are conversational, functional, or agentic.
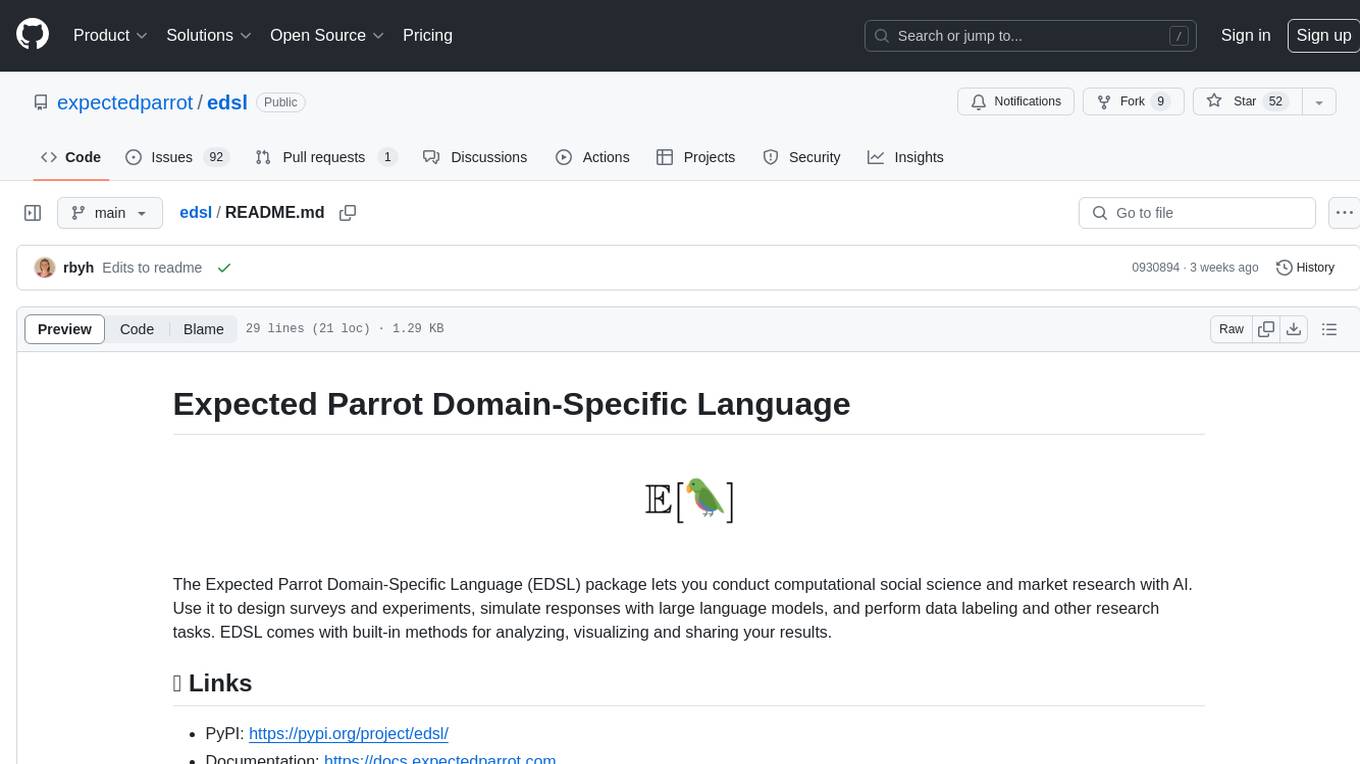
edsl
The Expected Parrot Domain-Specific Language (EDSL) package enables users to conduct computational social science and market research with AI. It facilitates designing surveys and experiments, simulating responses using large language models, and performing data labeling and other research tasks. EDSL includes built-in methods for analyzing, visualizing, and sharing research results. It is compatible with Python 3.9 - 3.11 and requires API keys for LLMs stored in a `.env` file.
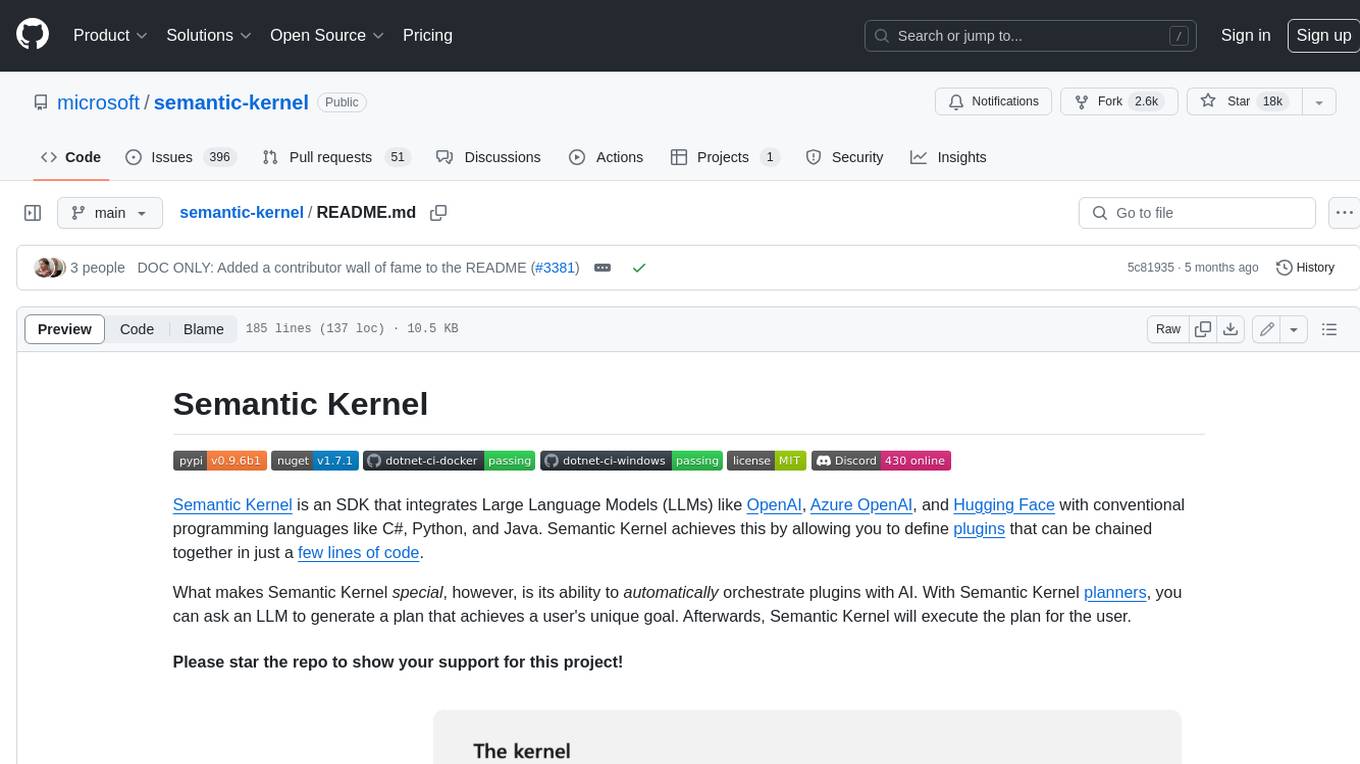
semantic-kernel
Semantic Kernel is an SDK that integrates Large Language Models (LLMs) like OpenAI, Azure OpenAI, and Hugging Face with conventional programming languages like C#, Python, and Java. Semantic Kernel achieves this by allowing you to define plugins that can be chained together in just a few lines of code. What makes Semantic Kernel _special_ , however, is its ability to _automatically_ orchestrate plugins with AI. With Semantic Kernel planners, you can ask an LLM to generate a plan that achieves a user's unique goal. Afterwards, Semantic Kernel will execute the plan for the user.
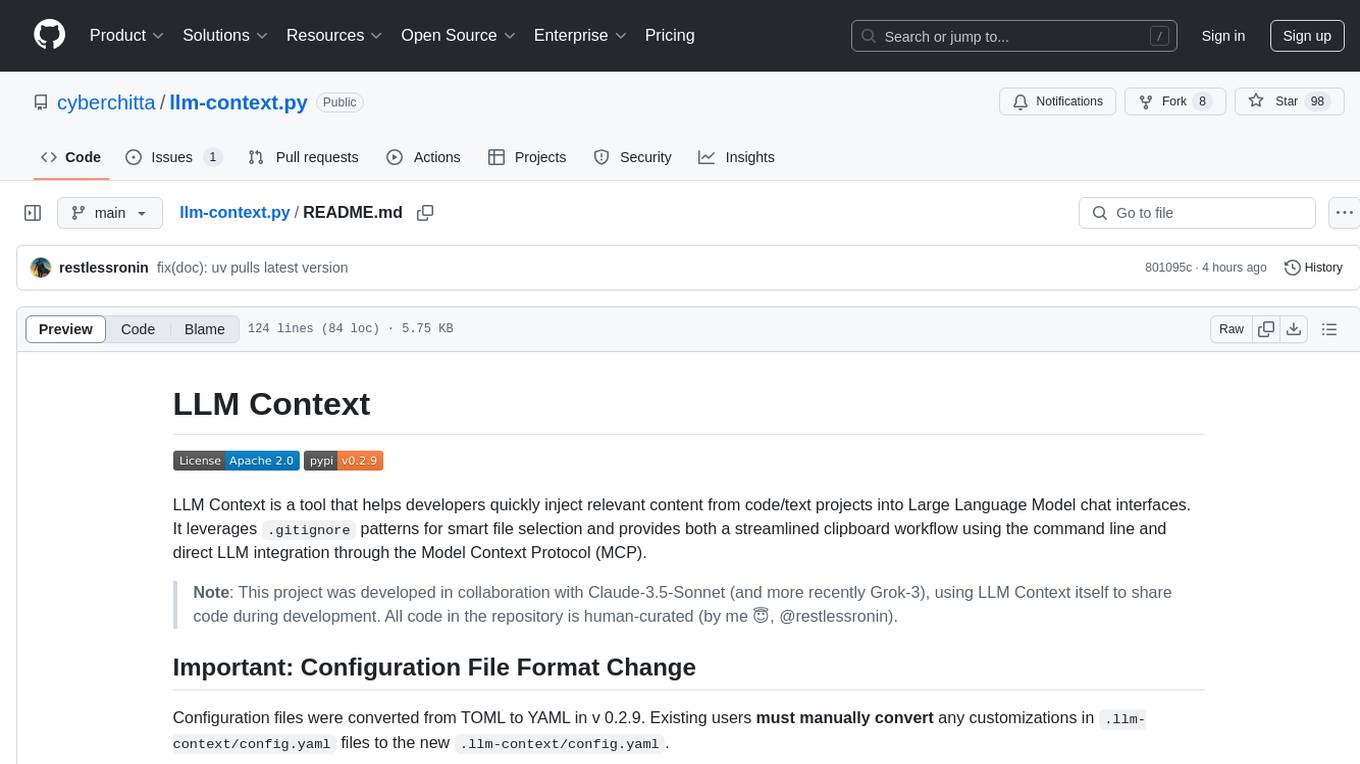
llm-context.py
LLM Context is a tool designed to assist developers in quickly injecting relevant content from code/text projects into Large Language Model chat interfaces. It leverages `.gitignore` patterns for smart file selection and offers a streamlined clipboard workflow using the command line. The tool also provides direct integration with Large Language Models through the Model Context Protocol (MCP). LLM Context is optimized for code repositories and collections of text/markdown/html documents, making it suitable for developers working on projects that fit within an LLM's context window. The tool is under active development and aims to enhance AI-assisted development workflows by harnessing the power of Large Language Models.
For similar tasks
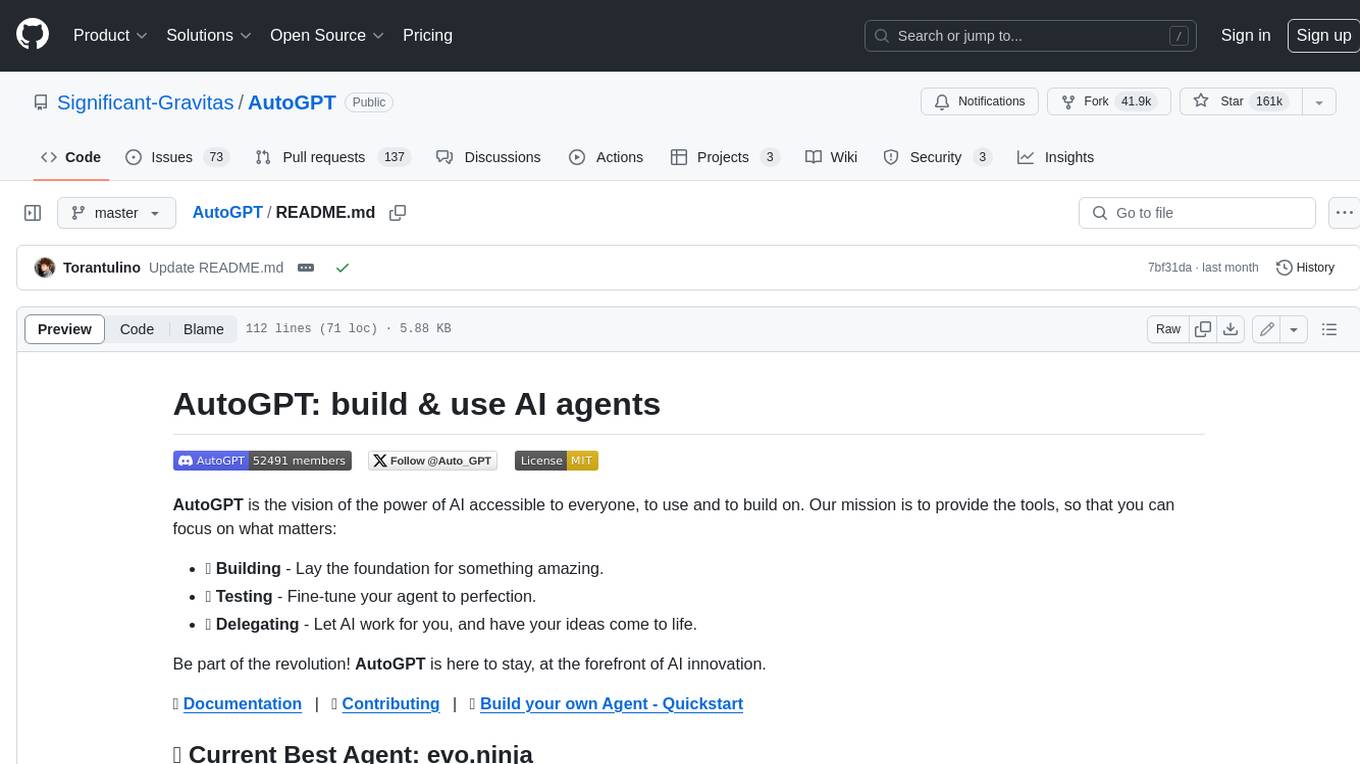
AutoGPT
AutoGPT is a revolutionary tool that empowers everyone to harness the power of AI. With AutoGPT, you can effortlessly build, test, and delegate tasks to AI agents, unlocking a world of possibilities. Our mission is to provide the tools you need to focus on what truly matters: innovation and creativity.
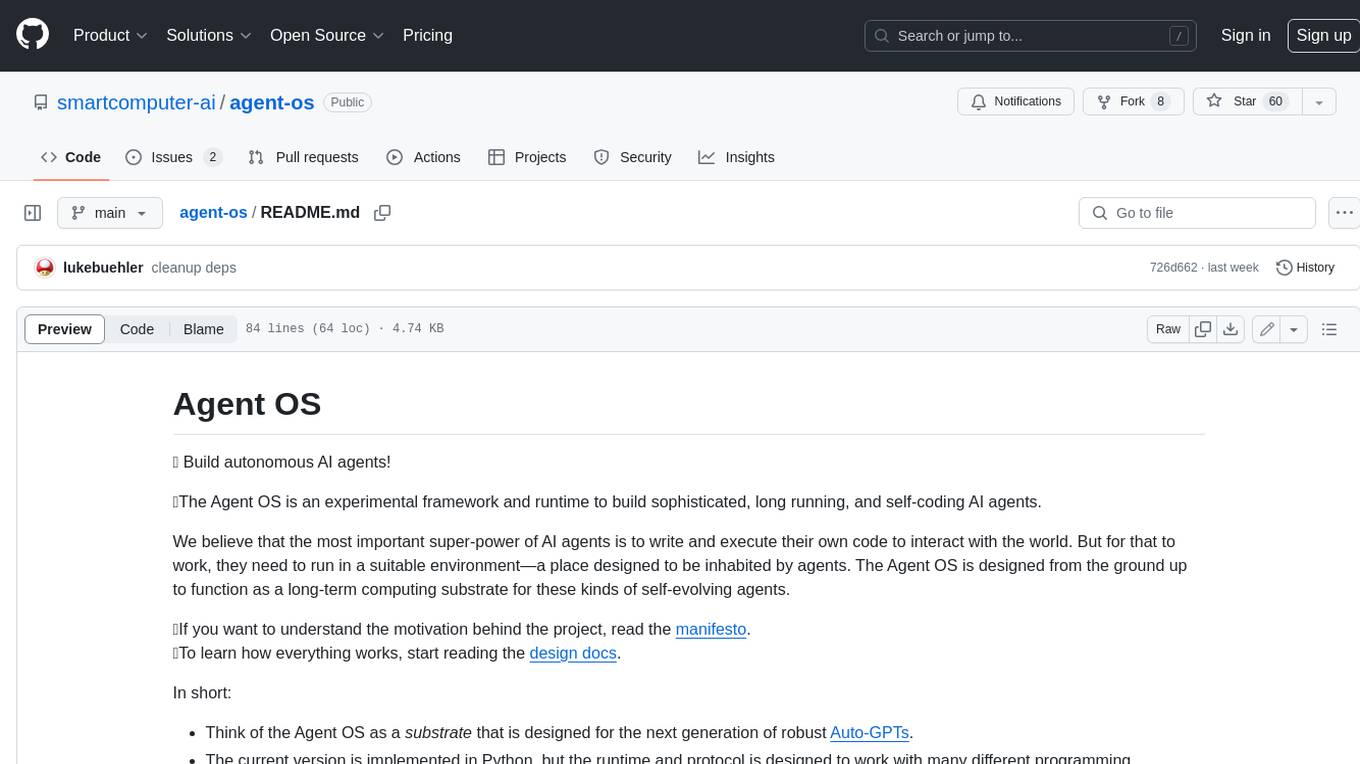
agent-os
The Agent OS is an experimental framework and runtime to build sophisticated, long running, and self-coding AI agents. We believe that the most important super-power of AI agents is to write and execute their own code to interact with the world. But for that to work, they need to run in a suitable environmentβa place designed to be inhabited by agents. The Agent OS is designed from the ground up to function as a long-term computing substrate for these kinds of self-evolving agents.
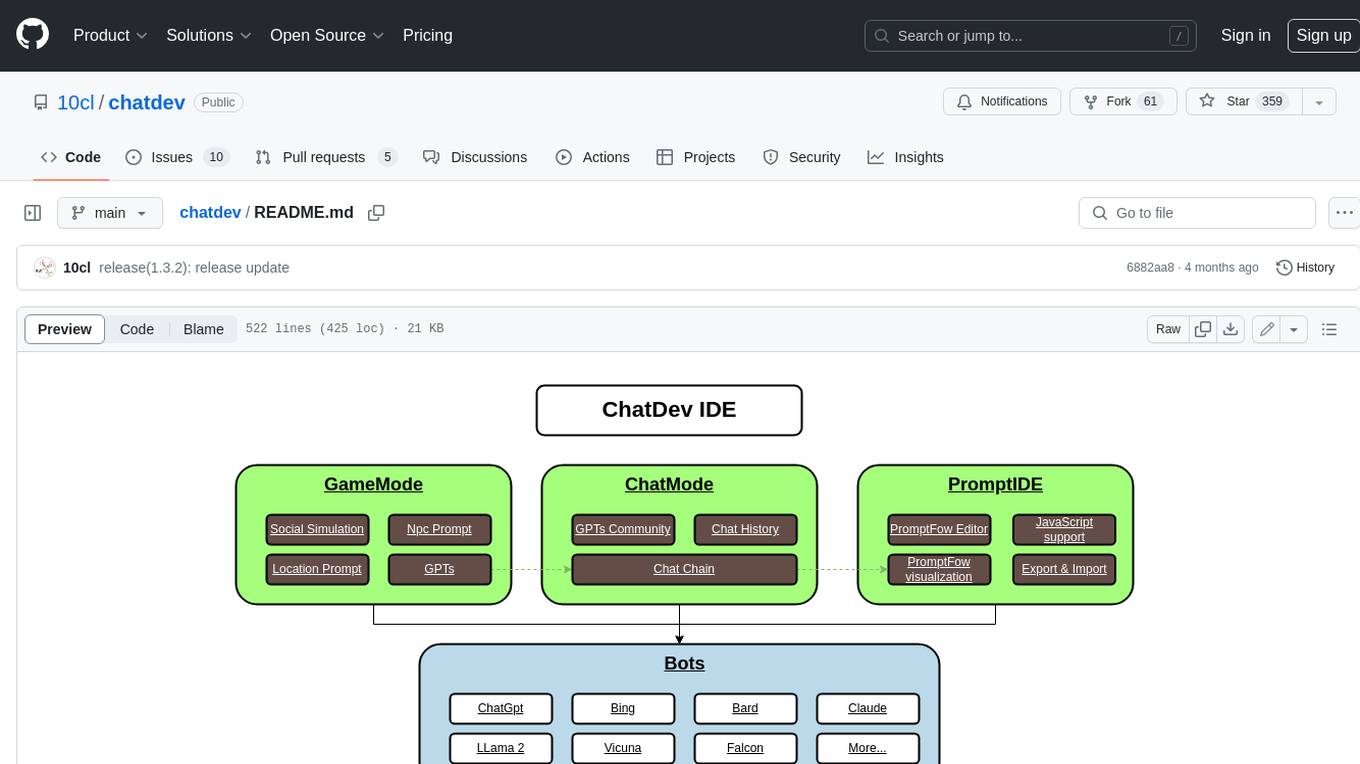
chatdev
ChatDev IDE is a tool for building your AI agent, Whether it's NPCs in games or powerful agent tools, you can design what you want for this platform. It accelerates prompt engineering through **JavaScript Support** that allows implementing complex prompting techniques.
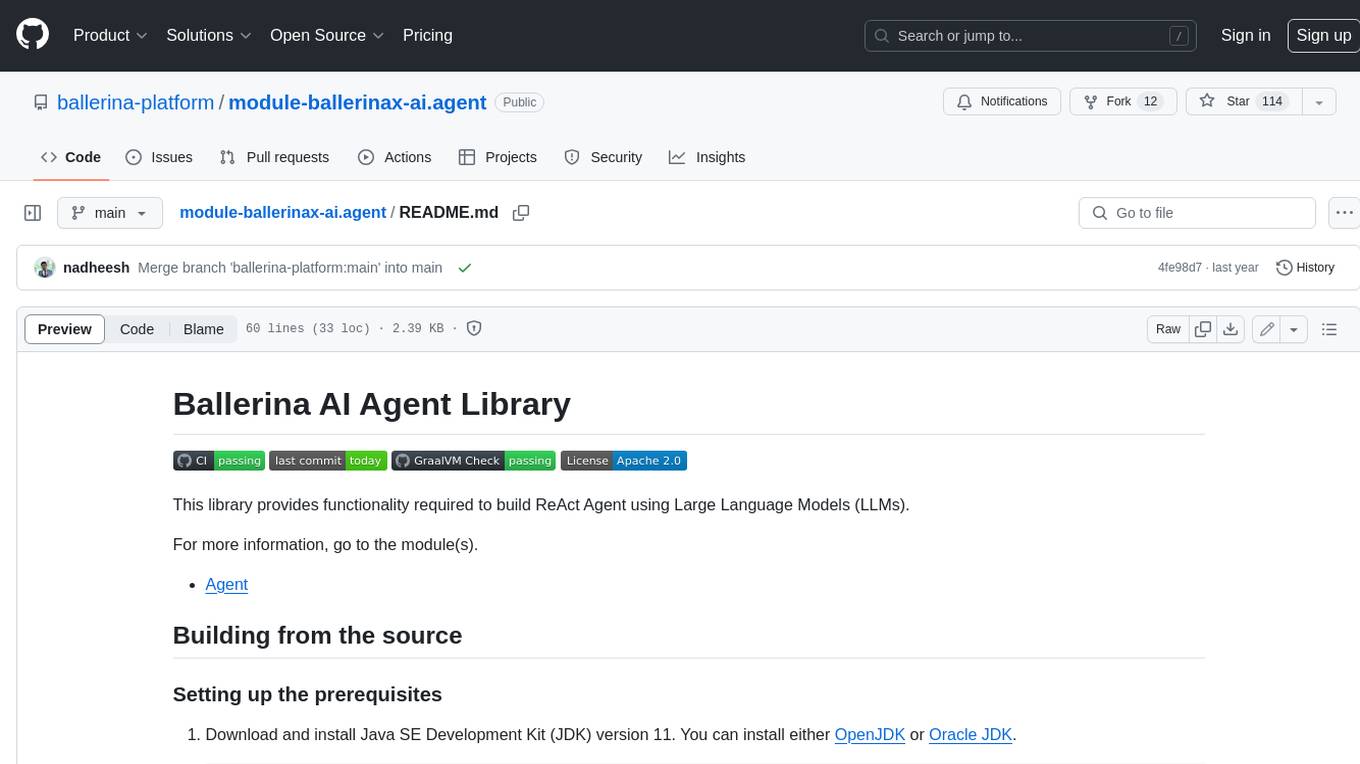
module-ballerinax-ai.agent
This library provides functionality required to build ReAct Agent using Large Language Models (LLMs).
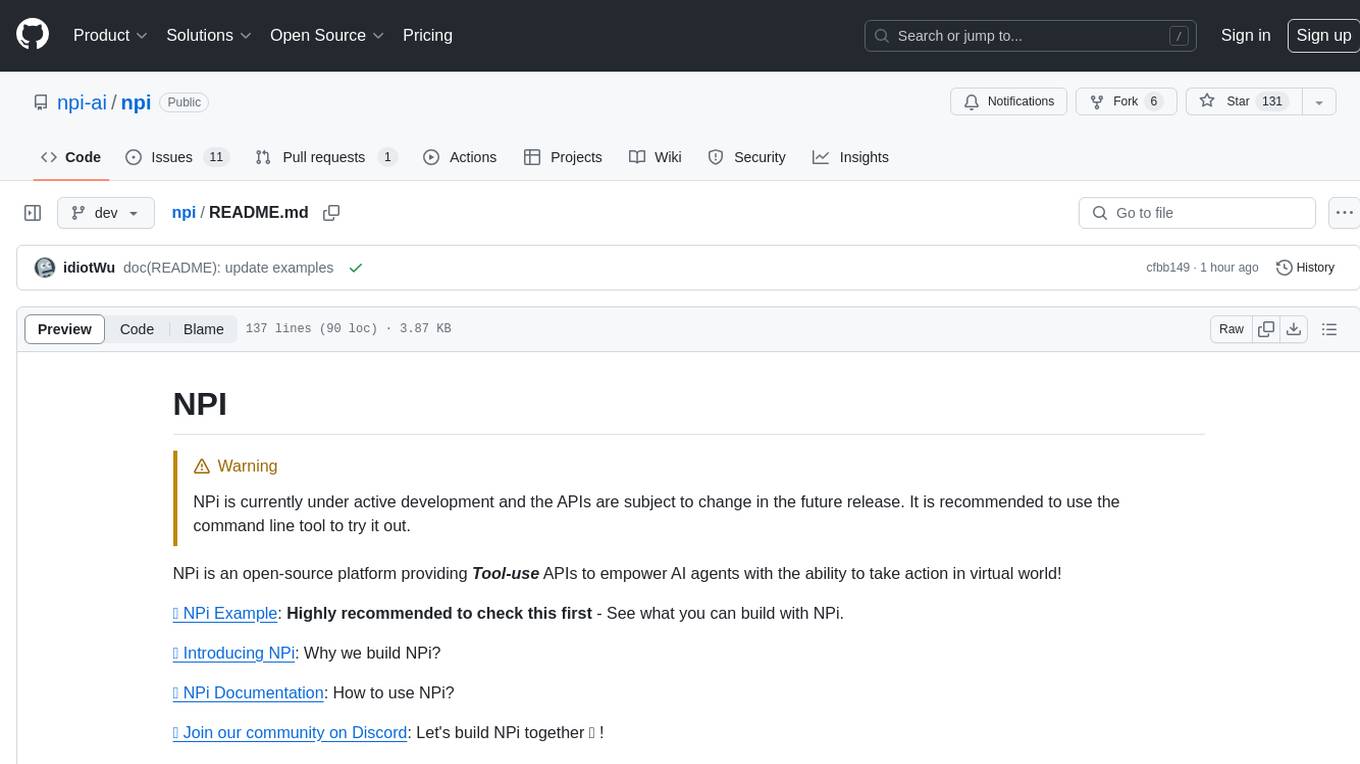
npi
NPi is an open-source platform providing Tool-use APIs to empower AI agents with the ability to take action in the virtual world. It is currently under active development, and the APIs are subject to change in future releases. NPi offers a command line tool for installation and setup, along with a GitHub app for easy access to repositories. The platform also includes a Python SDK and examples like Calendar Negotiator and Twitter Crawler. Join the NPi community on Discord to contribute to the development and explore the roadmap for future enhancements.
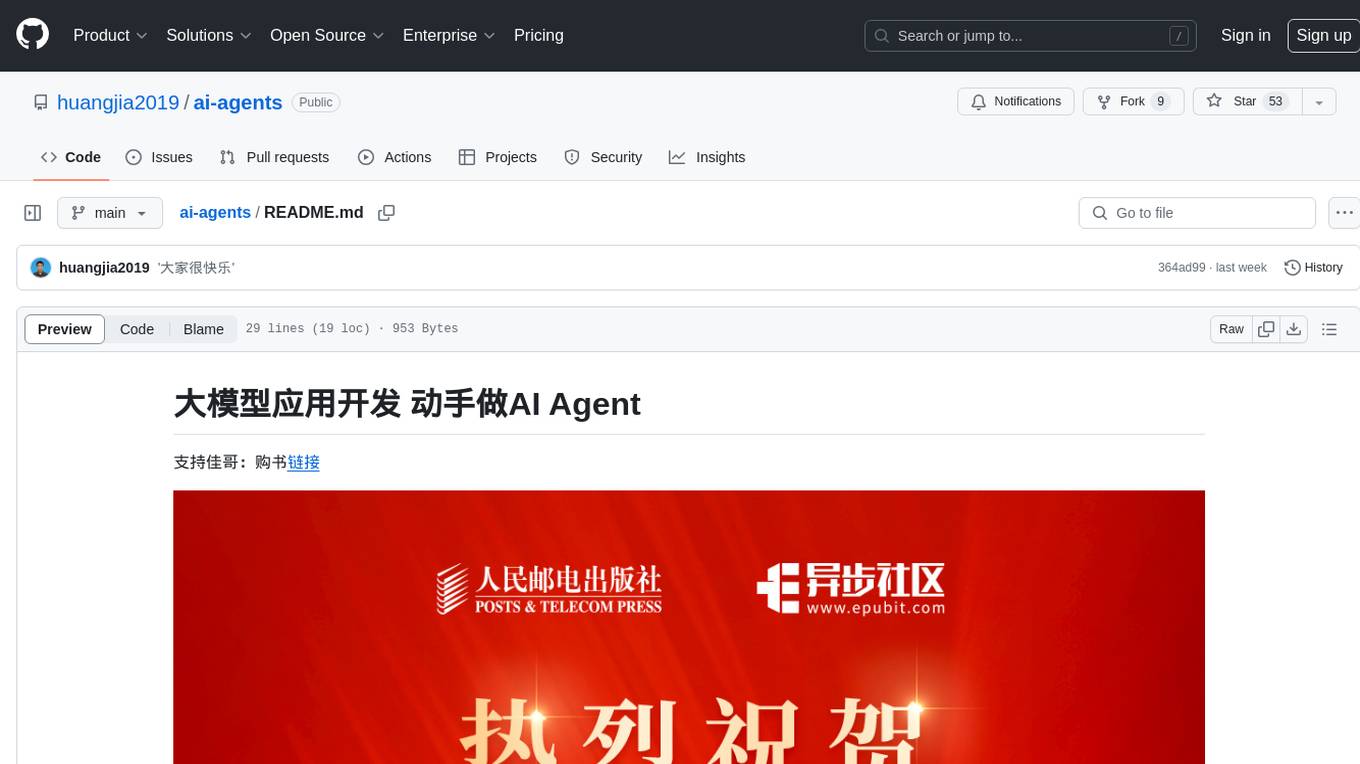
ai-agents
The 'ai-agents' repository is a collection of books and resources focused on developing AI agents, including topics such as GPT models, building AI agents from scratch, machine learning theory and practice, and basic methods and tools for data analysis. The repository provides detailed explanations and guidance for individuals interested in learning about and working with AI agents.
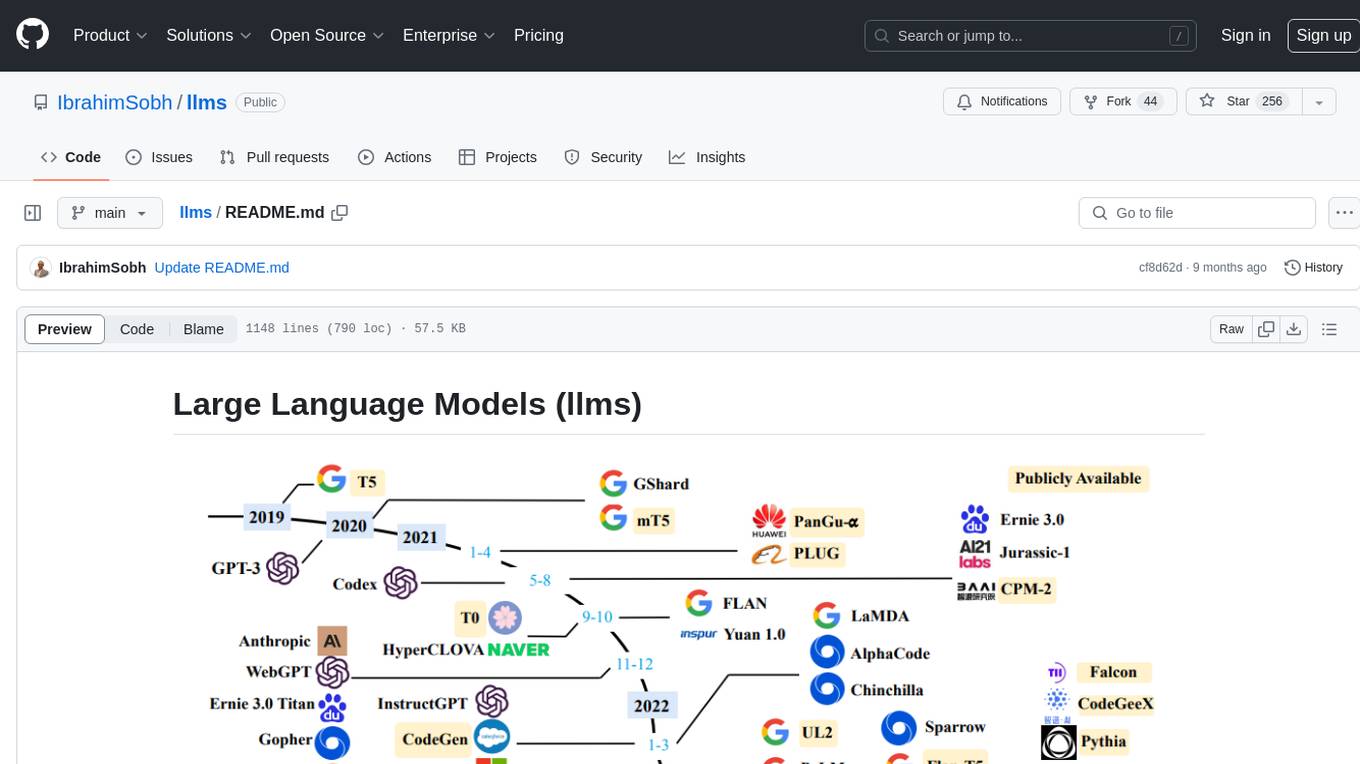
llms
The 'llms' repository is a comprehensive guide on Large Language Models (LLMs), covering topics such as language modeling, applications of LLMs, statistical language modeling, neural language models, conditional language models, evaluation methods, transformer-based language models, practical LLMs like GPT and BERT, prompt engineering, fine-tuning LLMs, retrieval augmented generation, AI agents, and LLMs for computer vision. The repository provides detailed explanations, examples, and tools for working with LLMs.
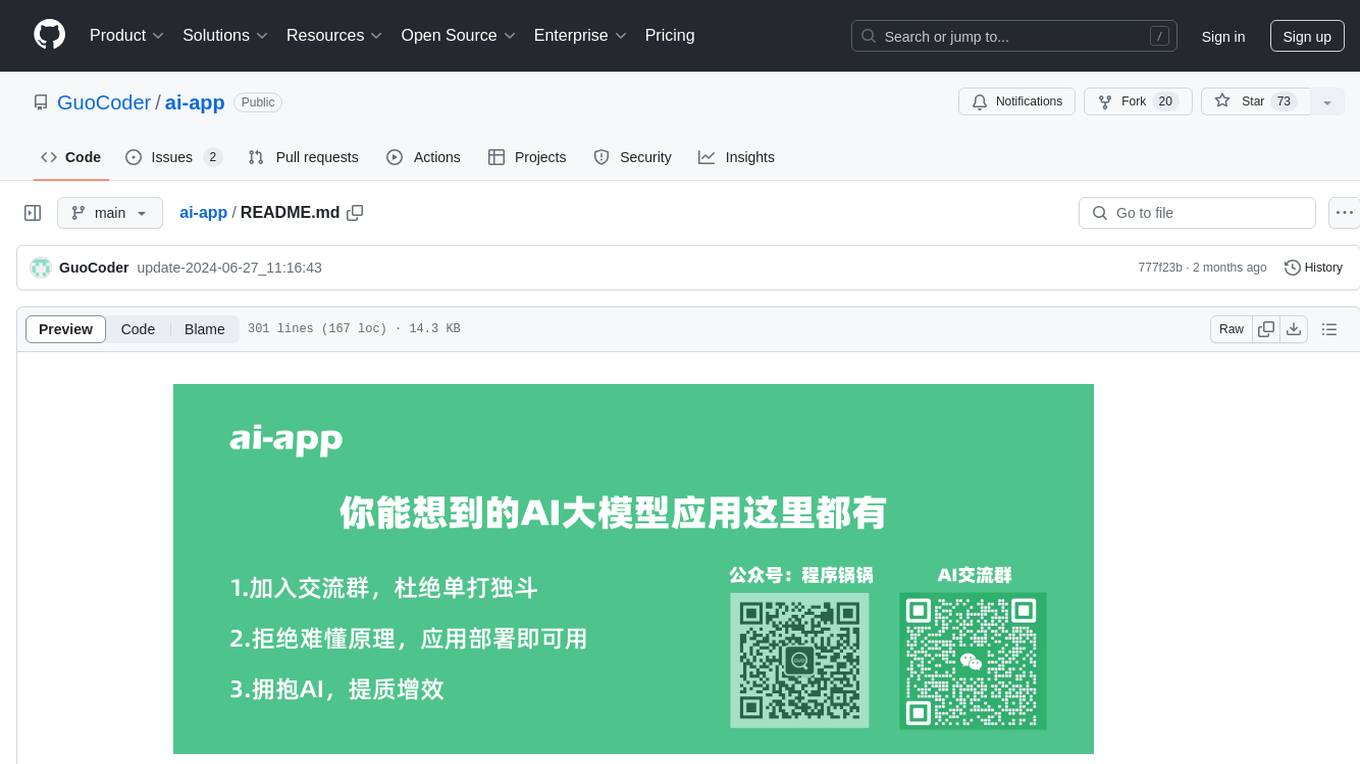
ai-app
The 'ai-app' repository is a comprehensive collection of tools and resources related to artificial intelligence, focusing on topics such as server environment setup, PyCharm and Anaconda installation, large model deployment and training, Transformer principles, RAG technology, vector databases, AI image, voice, and music generation, and AI Agent frameworks. It also includes practical guides and tutorials on implementing various AI applications. The repository serves as a valuable resource for individuals interested in exploring different aspects of AI technology.
For similar jobs
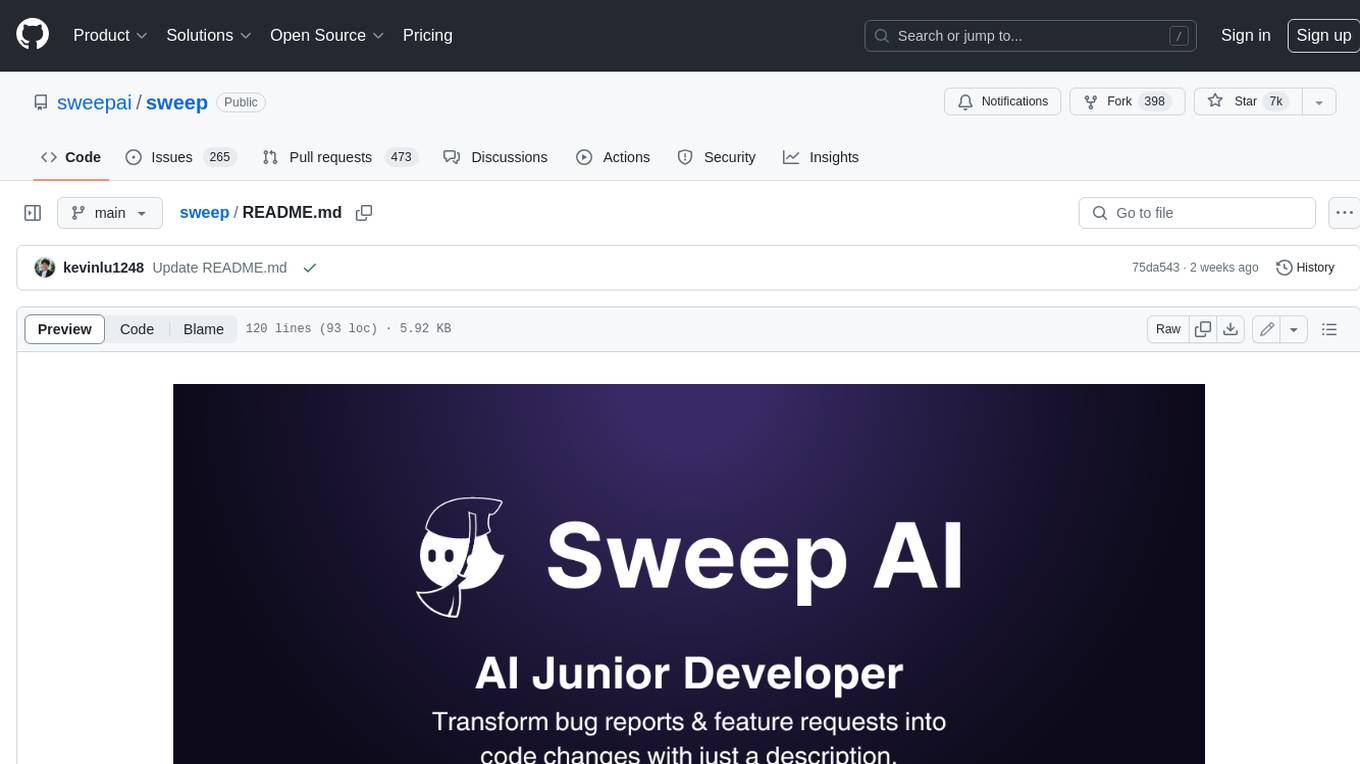
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
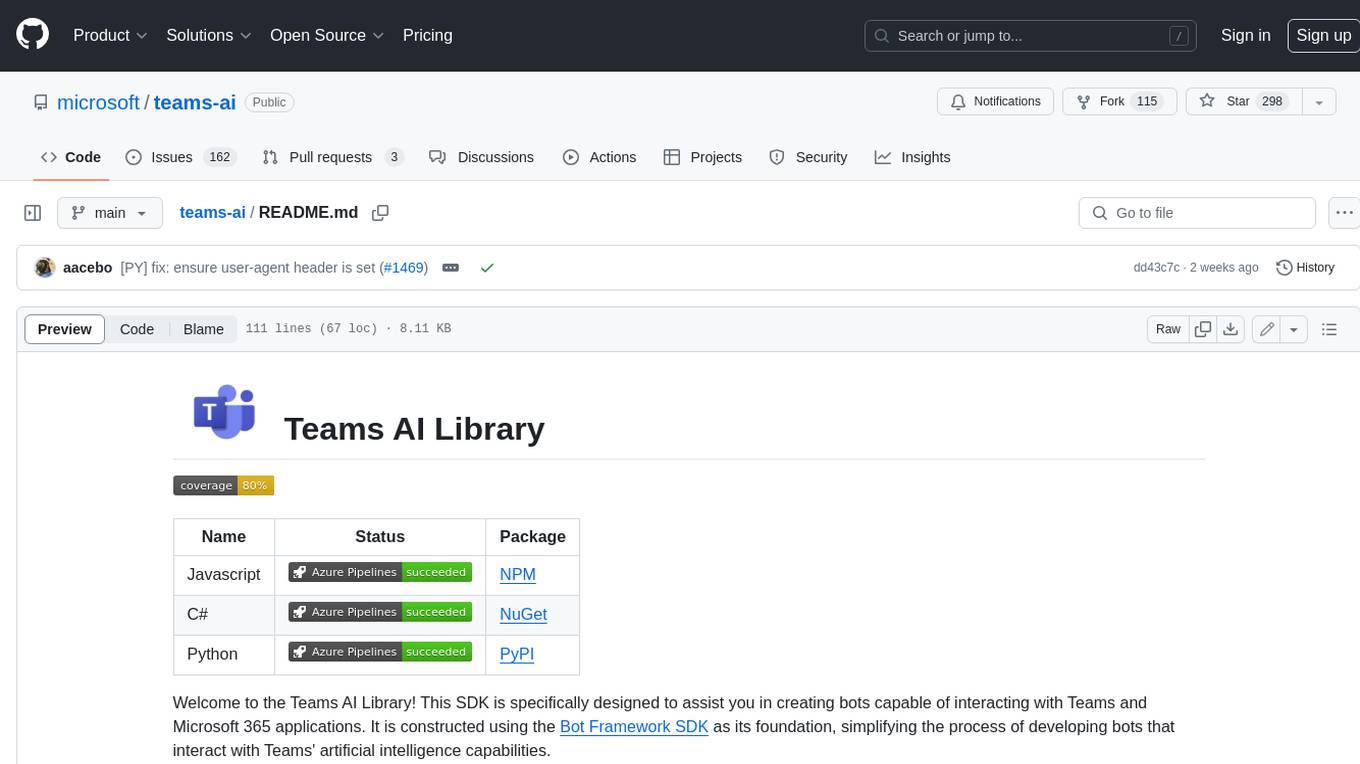
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
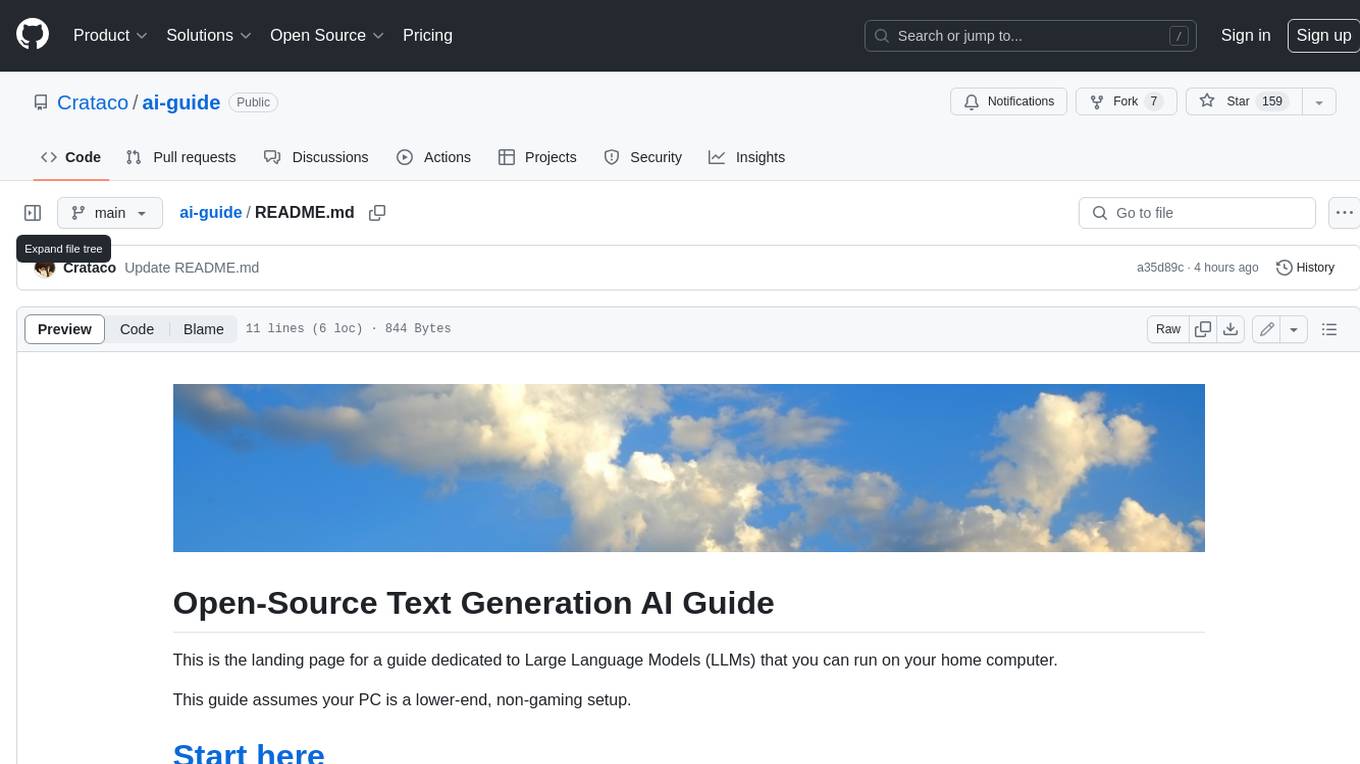
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
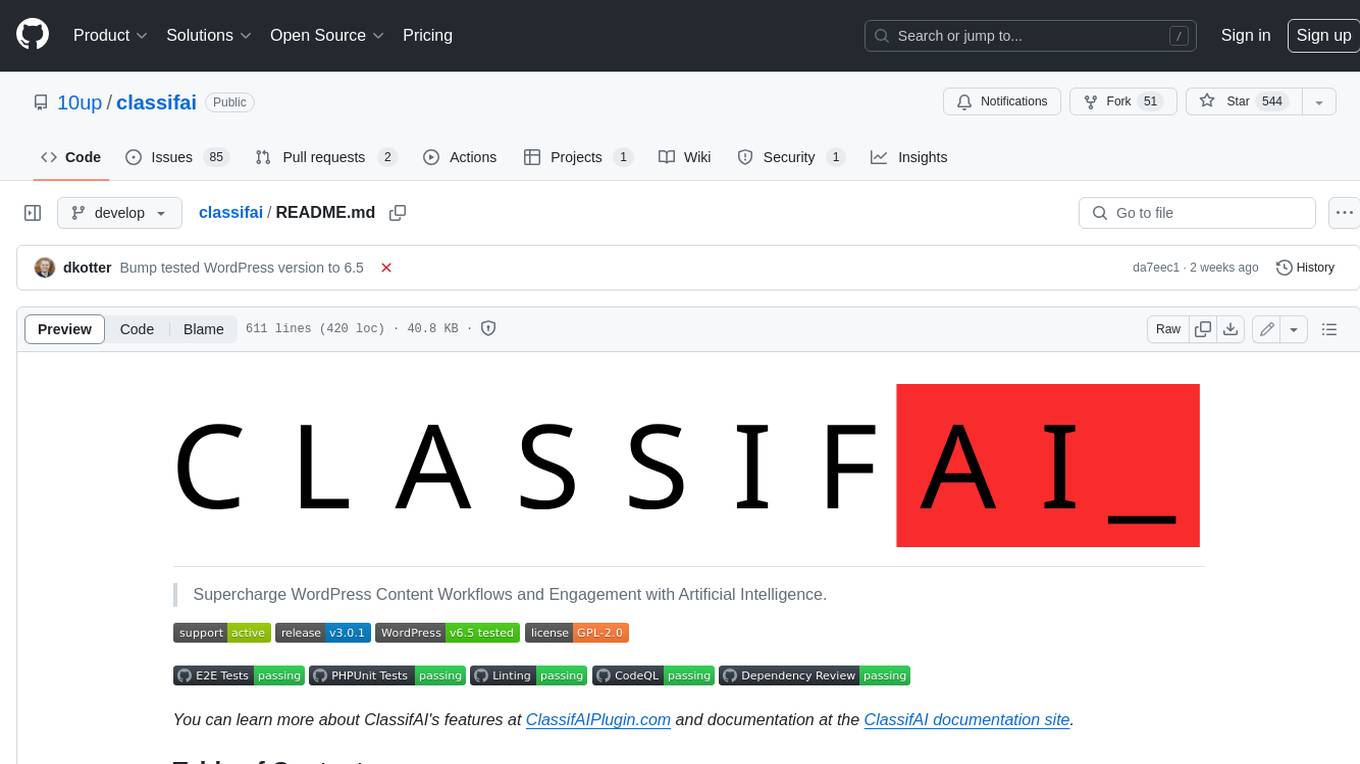
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
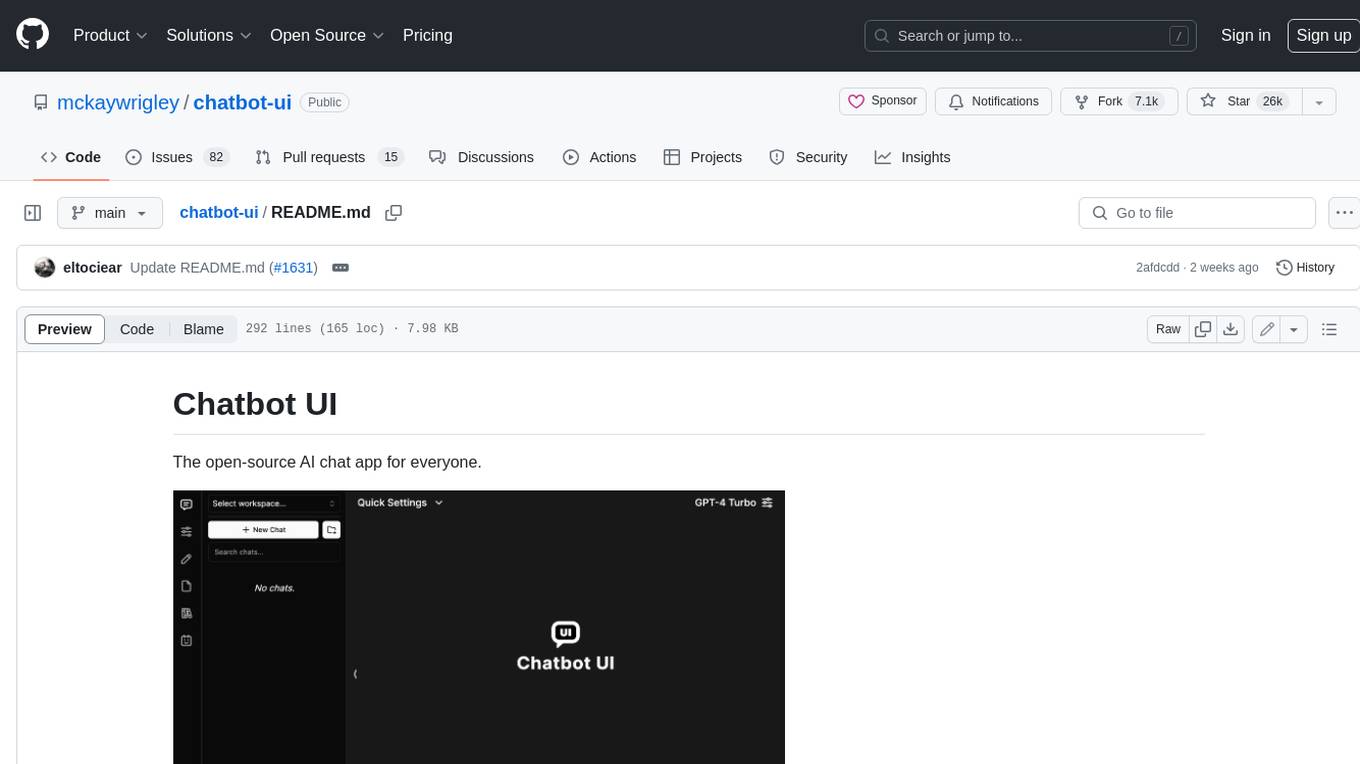
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
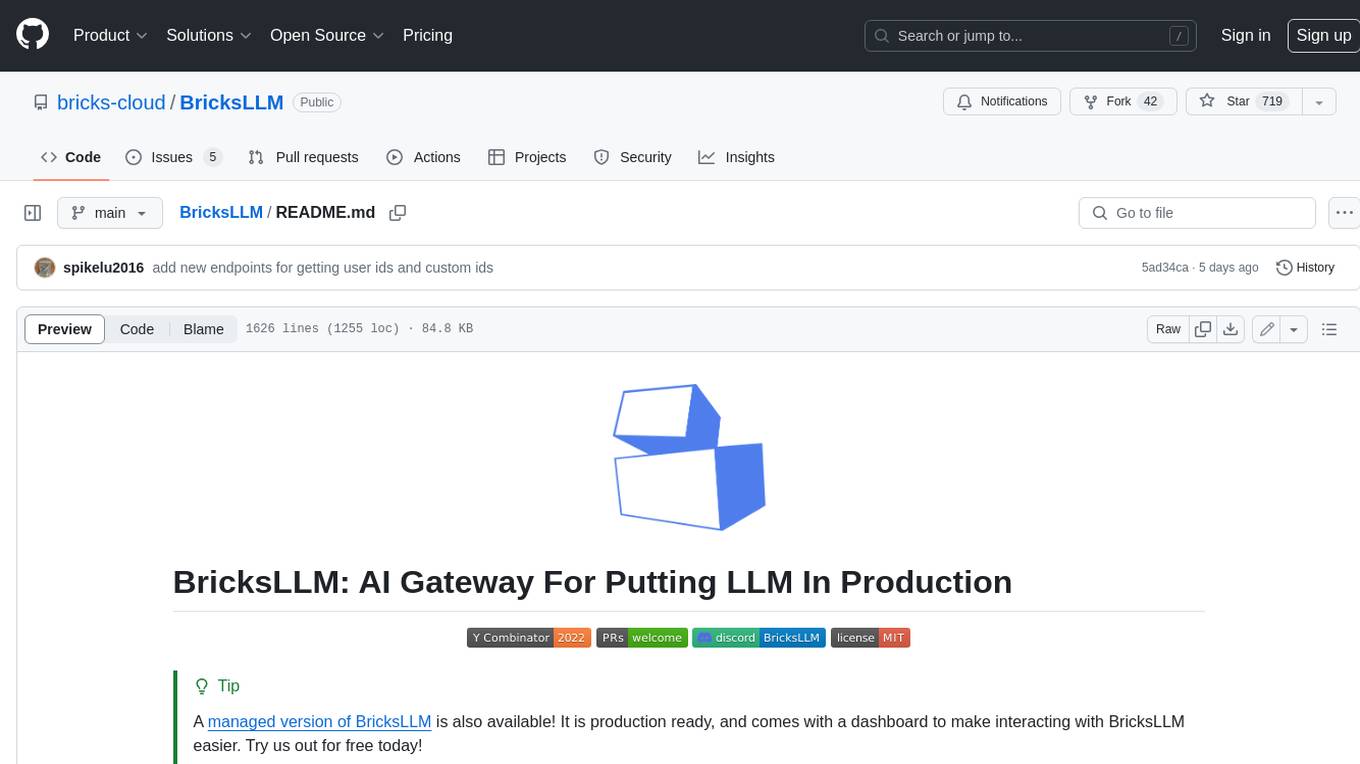
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
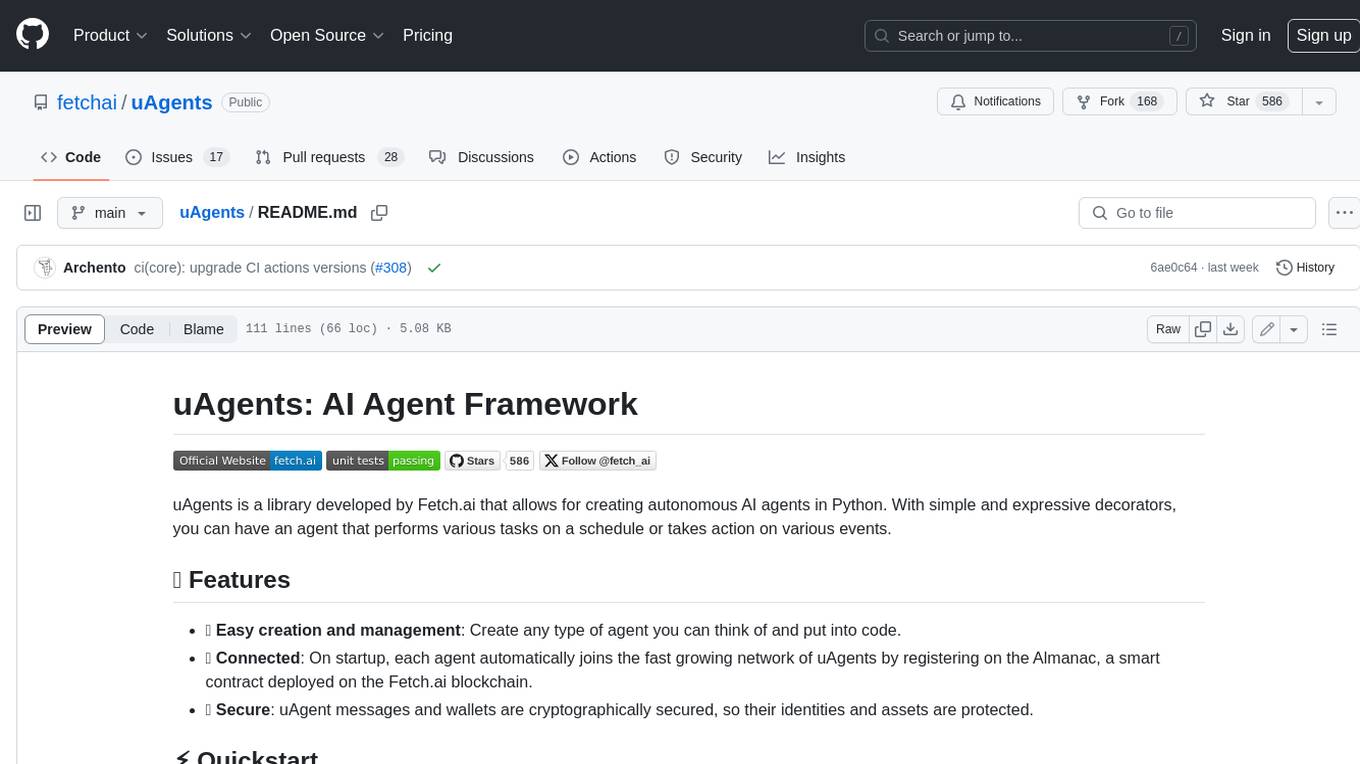
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
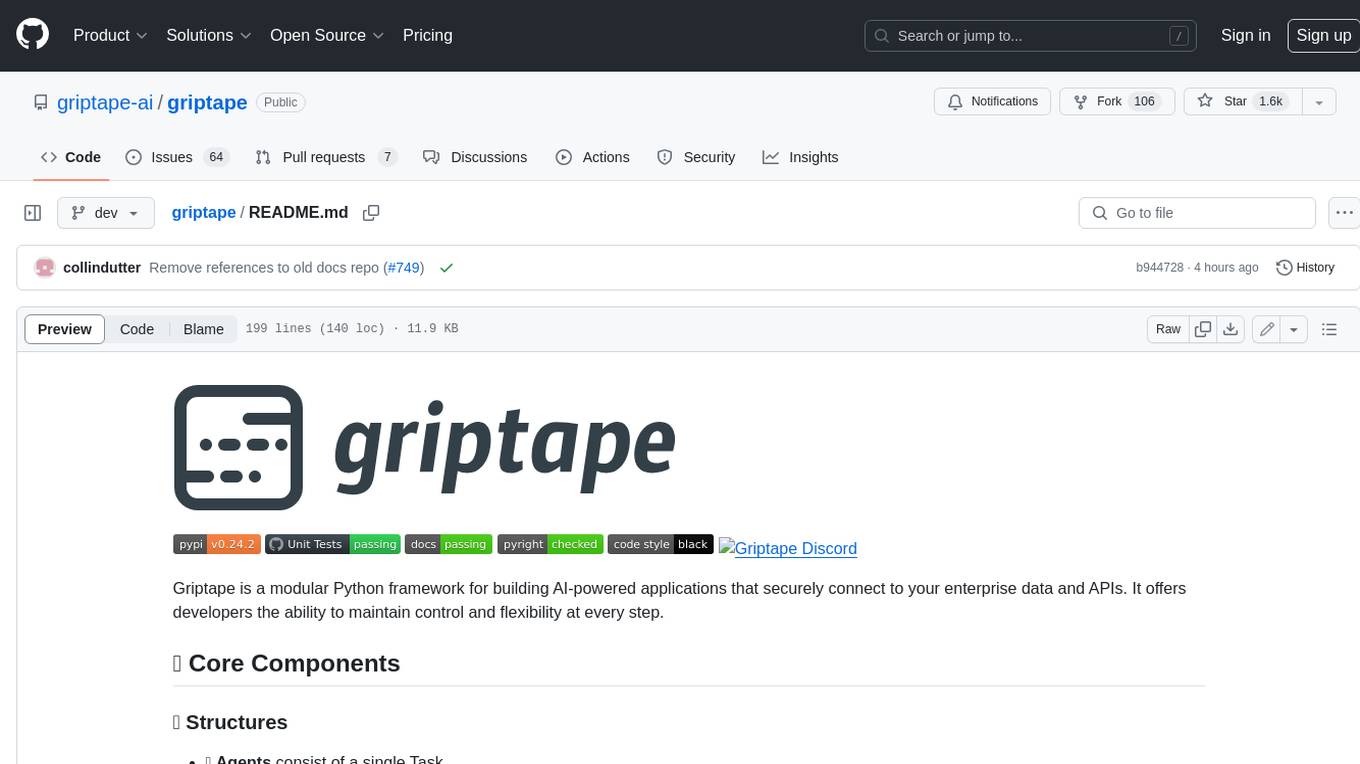
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.