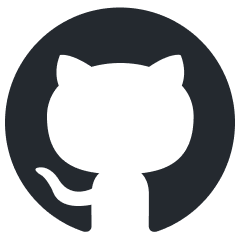
laravel-crod
Make easy & fast crud for Laravel with automatic query like AI
Stars: 110
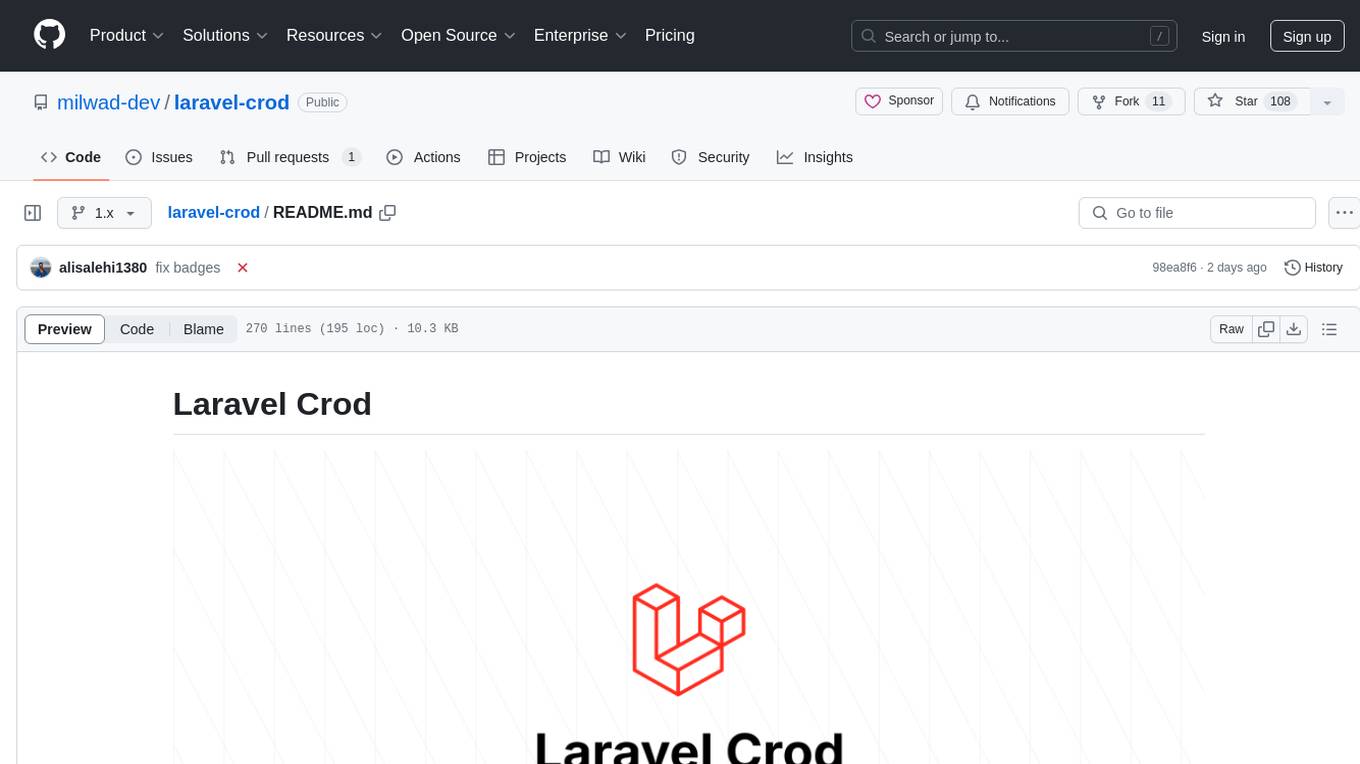
Laravel Crod is a package designed to facilitate the implementation of CRUD operations in Laravel projects. It allows users to quickly generate controllers, models, migrations, services, repositories, views, and requests with various customization options. The package simplifies tasks such as creating resource controllers, making models fillable, querying repositories and services, and generating additional files like seeders and factories. Laravel Crod aims to streamline the process of building CRUD functionalities in Laravel applications by providing a set of commands and tools for developers.
README:
Laravel crod is a package for implementing CRUD faster and easier. You can quickly create controllers, models, migrations, services, repositories, views and requests. You can make it automatically fillable for models, query for repositories and services, make resource controllers, and have a lot of options.
Docs: https://github.com/milwad-dev/laravel-crod/wiki
PHP: ^8.0
Laravel framework: ^9
doctrine/dbal: ^3.6
Crod | L7 | L8 | L9 | L10 | L11 |
---|---|---|---|---|---|
1.0 | ✅ | ✅ | ✅ | ❌ | ❌ |
1.1 | ❌ | ❌ | ✅ | ✅ | ✅ |
1.2 | ❌ | ❌ | ✅ | ✅ | ✅ |
1.3 | ❌ | ❌ | ✅ | ✅ | ✅ |
1.4 | ❌ | ❌ | ✅ | ✅ | ✅ |
composer require milwad/laravel-crod
After installation, you need to publish config files.
php artisan vendor:publish --provider="Milwad\LaravelCrod\LaravelCrodServiceProvider" --tag="laravel-crod-config"
When you install the Laravel Crod
, a series of commands will be activated for you. For see these commands, you can run below command:
php artisan
For creating crud files, you need to run the crud:make
command in your terminal:
php artisan crud:make {name}
For example
php artisan crud:make Product
When you execute this command, after creating the files, you will see a list of options that will create a series of additional files for you, which of course are optional, you can choose and if you need, it will create additional files for you such as seeder
, factory
, repository
, etc.
✅ After, you can see Laravel Crod
creates crud files such as Model
, Controller
, Form-Requests
, Migrations
etc.
If you run crud:query
command, the result is:
- Add
index
,create
,store
,edit
,update
,destroy
function to your controller - Get all migration columns and move it to your model fillable
- Add
index
,findById
,delete
functions to your repositories - Add
store
,update
functions to your services - Add resource route (SOON)
** You must run the migrate command, before crud:query
command. **
php artisan migrate
For using automatic query, you can run below command:
php artisan crud:query {table_name} {model} {--id-controller}
For example:
php artisan crud:query products Product
When you add --id-controller
option, the Laravel Crod
create crud functions without Route Model Binding in controller.
After you can see Laravel Crod
added query to service, repository, controller, model, etc.
If you are using Modular Architecture, you are able to run crud:make-module
command. This command create a new module and create the default crud files such as Model
, Controller
, Migration
, etc:
php artisan crud:make-module {module_name}
For example:
php artisan crud:make-module Product
When you execute this command, after creating the files, you will see a list of options that will create a series of additional files for you, which of course are optional, you can choose and if you need, it will create additional files for you such as seeder
, factory
, repository
, etc.
This command adds query and date to CRUD files for module.
This command is similar to crud:query
command, but this command is for module. if you have a modular you can write your module name and Laravel Crod
find it automatically.
** You must run your migration file **
php artisan crud:query-module {table_name} {model} {--id-controller}
For example:
php artisan crud:query-module products Product
OR
php artisan crud:query-module products Product --id-controller
When you add --id-controller
option, the Laravel Crod
create crud functions without Route Model Binding in controller.
After you can see Laravel Crod
added query to service, repository, controller, model, ... for your module.
You can custom file path in config file. ``````
With Laravel Crod
config, you can customize the commands, for example you want to set the route file name.
This config file exists in config/laravel-crod.php
:
<?php
return [
/*
* Repository namespace.
*
* This is a word that move into the latest name of repository file, for ex: ProductRepo.
* If this value is changed, any repos that are created will be renamed, for ex: ProductRepository.
*/
'repository_namespace' => 'Repo',
/*
* Get main controller.
*
* This is a namespace of main controller that default path is `App\Http\Controllers\Controller`.
*/
'main_controller' => 'App\Http\Controllers\Controller',
/*
* Are using PEST?
*
* If you are using PEST framework, you can change it this value to `true`.
*/
'are_using_pest' => false,
/*
* Route namespace.
*
* This is a word that move into the latest name of route file.
*/
'route_namespace' => '',
/*
* Route name.
*
* This is a word that name of route file.
*/
'route_name' => 'web',
/*
* Modules config.
*
* You can make custom modules with special folders ... .
*/
'modules' => [
'module_namespace' => 'Modules', // This value is for the name of the folder that the modules are in.
'model_path' => 'Entities', // This value is for the name of the folder that contains the module models.
'migration_path' => 'Database\Migrations', // This value is for the name of the folder that contains the module migrations.
'controller_path' => 'Http\Controllers', // This value is for the name of the folder that contains the module controllers.
'request_path' => 'Http\Requests', // This value is for the name of the folder that contains the module requests-form.
'view_path' => 'Resources\Views', // This value is for the name of the folder that contains the module views.
'service_path' => 'Services', // This value is for the name of the folder that contains the module services.
'repository_path' => 'Repositories', // This value is for the name of the folder that contains the module Repositories.
'feature_test_path' => 'Tests\Feature', // This value is for the name of the folder that contains the module feature-tests.
'unit_test_path' => 'Tests\Unit', // This value is for the name of the folder that contains the module unit-tests.
'provider_path' => 'Providers', // This value is for the name of the folder that contains the module providers.
'factory_path' => 'Database\Factories', // This value is for the name of the folder that contains the module factories.
'seeder_path' => 'Database\Seeders', // This value is for the name of the folder that contains the module seeders.
'route_path' => 'Routes', // This value is for the name of the folder that contains the module routes.
],
/*
* Queries.
*
* This is some config for add queries.
*/
'queries' => [
/*
* Except columns in fillable.
*
* This `except_columns_in_fillable` must be arrayed!
* This `except_columns_in_fillable` that remove field from $fillable in model.
*/
'except_columns_in_fillable' => [
'id', 'updated_at', 'created_at',
],
],
];
- This package is created and modified by Milwad Khosravi for Laravel >= 9 and is released under the MIT License.
Run the tests with:
vendor/bin/phpunit
composer test
composer test-coverage
This project exists thanks to all the people who contribute. CONTRIBUTING
If you've found a bug regarding security please mail [email protected] instead of using the issue tracker.
The Laravel-Crod
is a simple yet powerful package that can help you create CRUD operations for your Laravel models in just a few lines of code. By following this documentation, you should now have a better understanding of how to use the package in your Laravel project. If you have any issues or questions, please feel free to open an issue on the package's GitHub repository.
If this package is helpful for you, you can buy a coffee for me :) ❤️
- Iraninan Gateway: https://daramet.com/milwad_khosravi
- Paypal Gateway: SOON
- MetaMask Address:
0xf208a562c5a93DEf8450b656c3dbc1d0a53BDE58
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for laravel-crod
Similar Open Source Tools
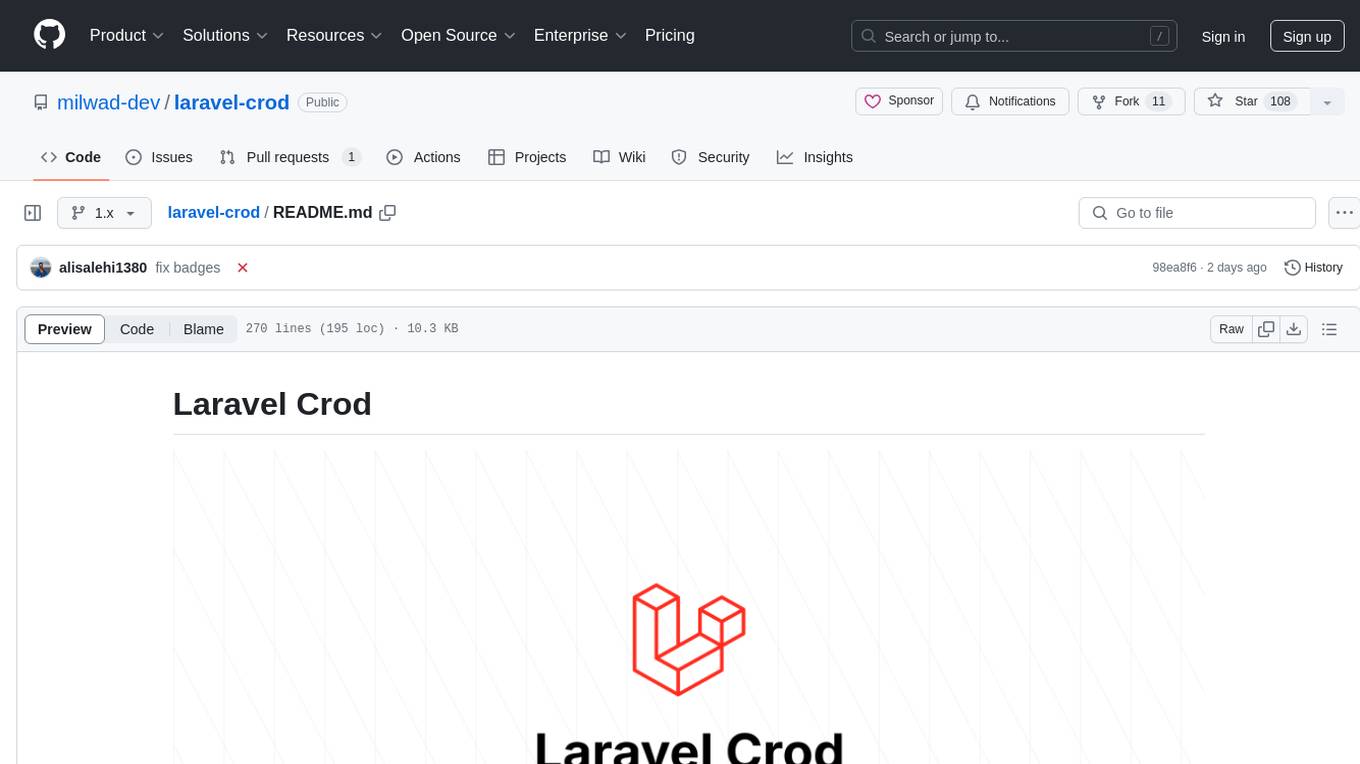
laravel-crod
Laravel Crod is a package designed to facilitate the implementation of CRUD operations in Laravel projects. It allows users to quickly generate controllers, models, migrations, services, repositories, views, and requests with various customization options. The package simplifies tasks such as creating resource controllers, making models fillable, querying repositories and services, and generating additional files like seeders and factories. Laravel Crod aims to streamline the process of building CRUD functionalities in Laravel applications by providing a set of commands and tools for developers.
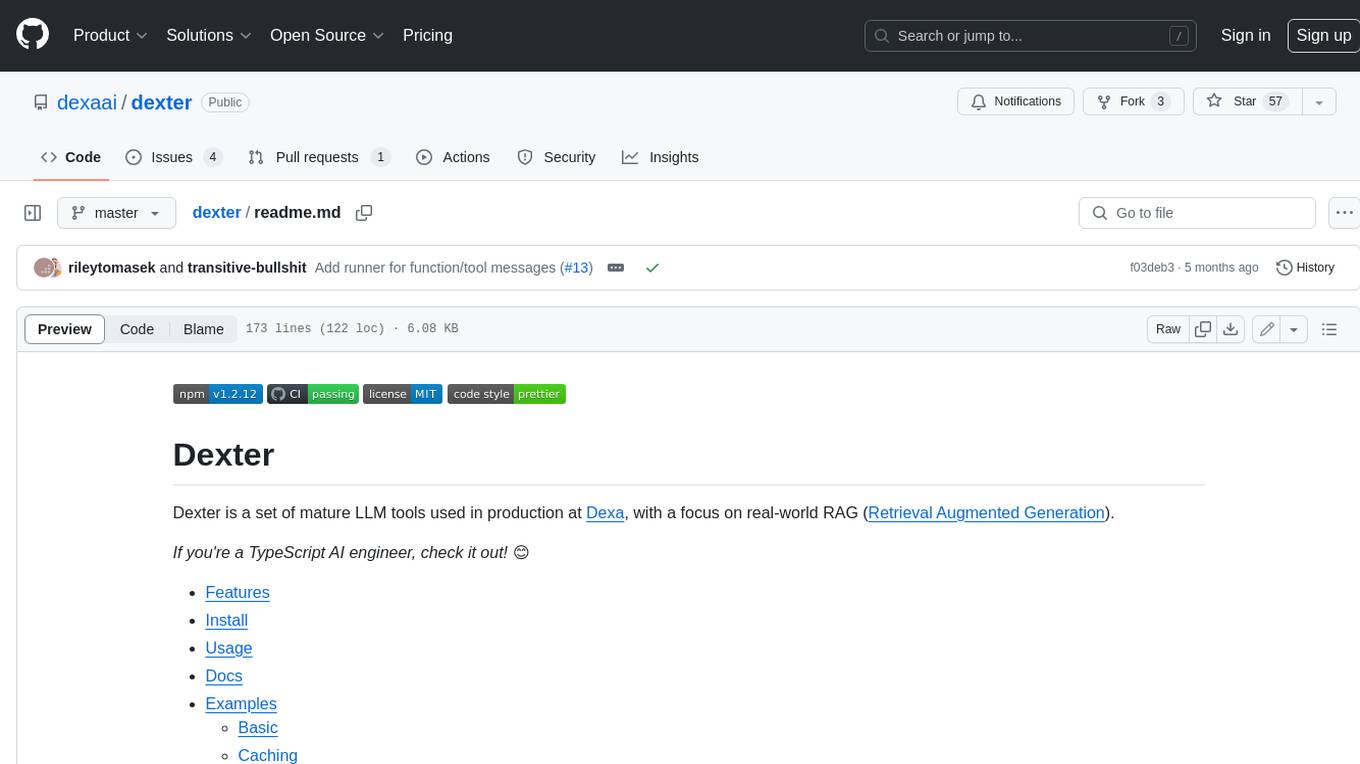
dexter
Dexter is a set of mature LLM tools used in production at Dexa, with a focus on real-world RAG (Retrieval Augmented Generation). It is a production-quality RAG that is extremely fast and minimal, and handles caching, throttling, and batching for ingesting large datasets. It also supports optional hybrid search with SPLADE embeddings, and is a minimal TS package with full typing that uses `fetch` everywhere and supports Node.js 18+, Deno, Cloudflare Workers, Vercel edge functions, etc. Dexter has full docs and includes examples for basic usage, caching, Redis caching, AI function, AI runner, and chatbot.
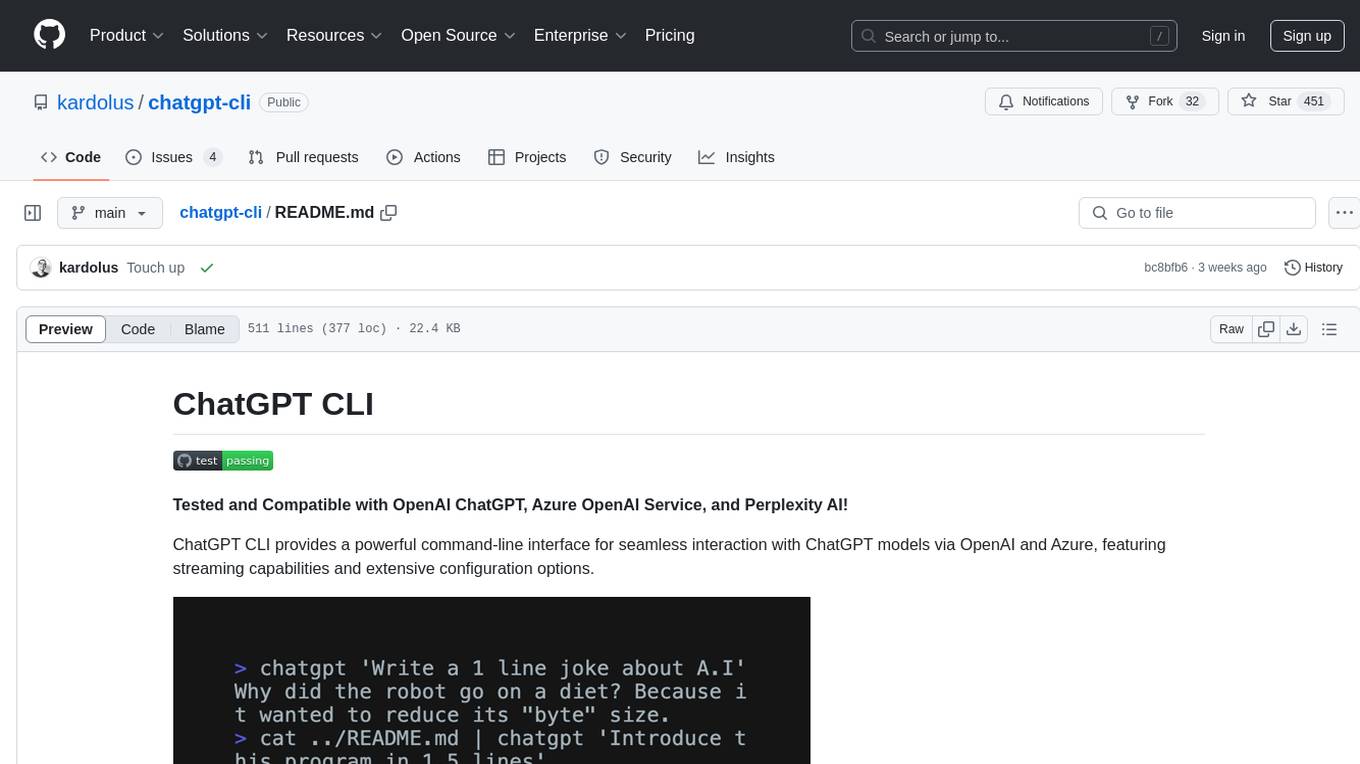
chatgpt-cli
ChatGPT CLI provides a powerful command-line interface for seamless interaction with ChatGPT models via OpenAI and Azure. It features streaming capabilities, extensive configuration options, and supports various modes like streaming, query, and interactive mode. Users can manage thread-based context, sliding window history, and provide custom context from any source. The CLI also offers model and thread listing, advanced configuration options, and supports GPT-4, GPT-3.5-turbo, and Perplexity's models. Installation is available via Homebrew or direct download, and users can configure settings through default values, a config.yaml file, or environment variables.
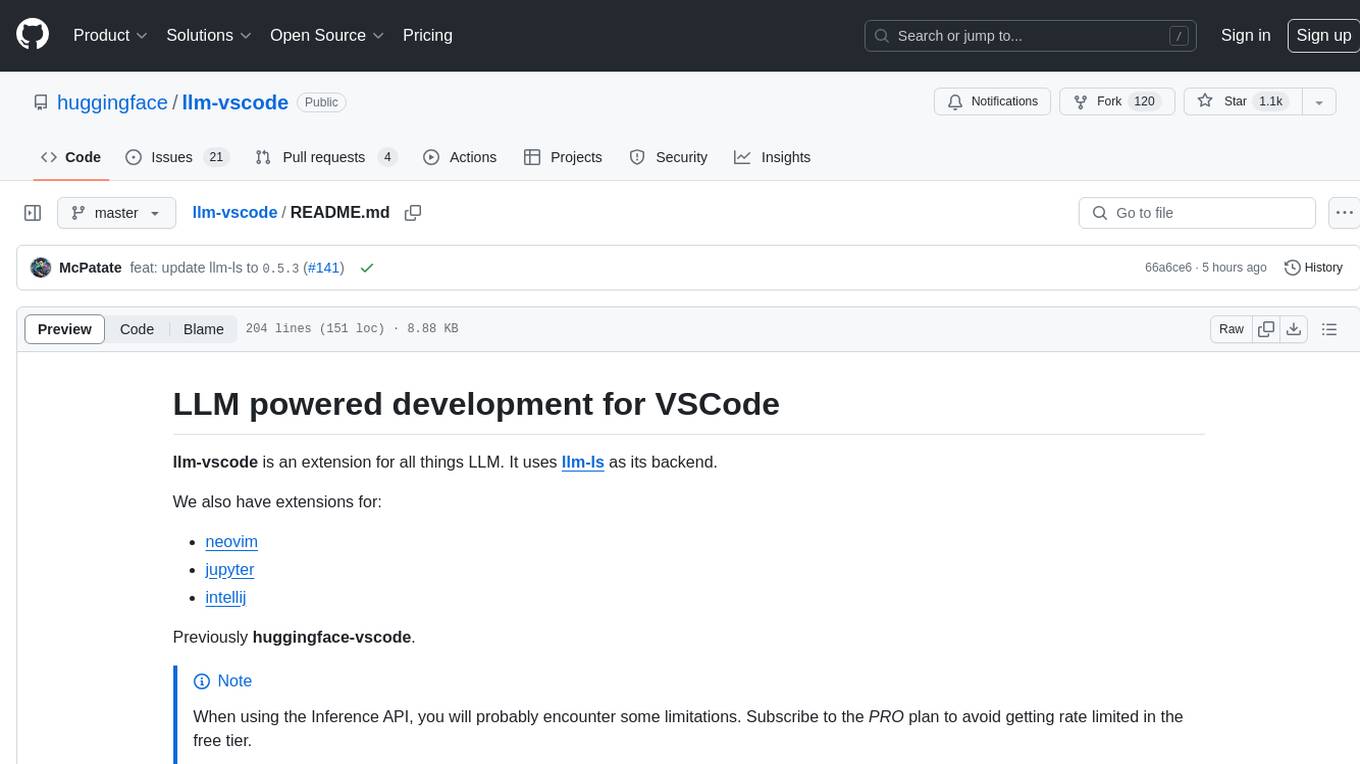
llm-vscode
llm-vscode is an extension designed for all things LLM, utilizing llm-ls as its backend. It offers features such as code completion with 'ghost-text' suggestions, the ability to choose models for code generation via HTTP requests, ensuring prompt size fits within the context window, and code attribution checks. Users can configure the backend, suggestion behavior, keybindings, llm-ls settings, and tokenization options. Additionally, the extension supports testing models like Code Llama 13B, Phind/Phind-CodeLlama-34B-v2, and WizardLM/WizardCoder-Python-34B-V1.0. Development involves cloning llm-ls, building it, and setting up the llm-vscode extension for use.
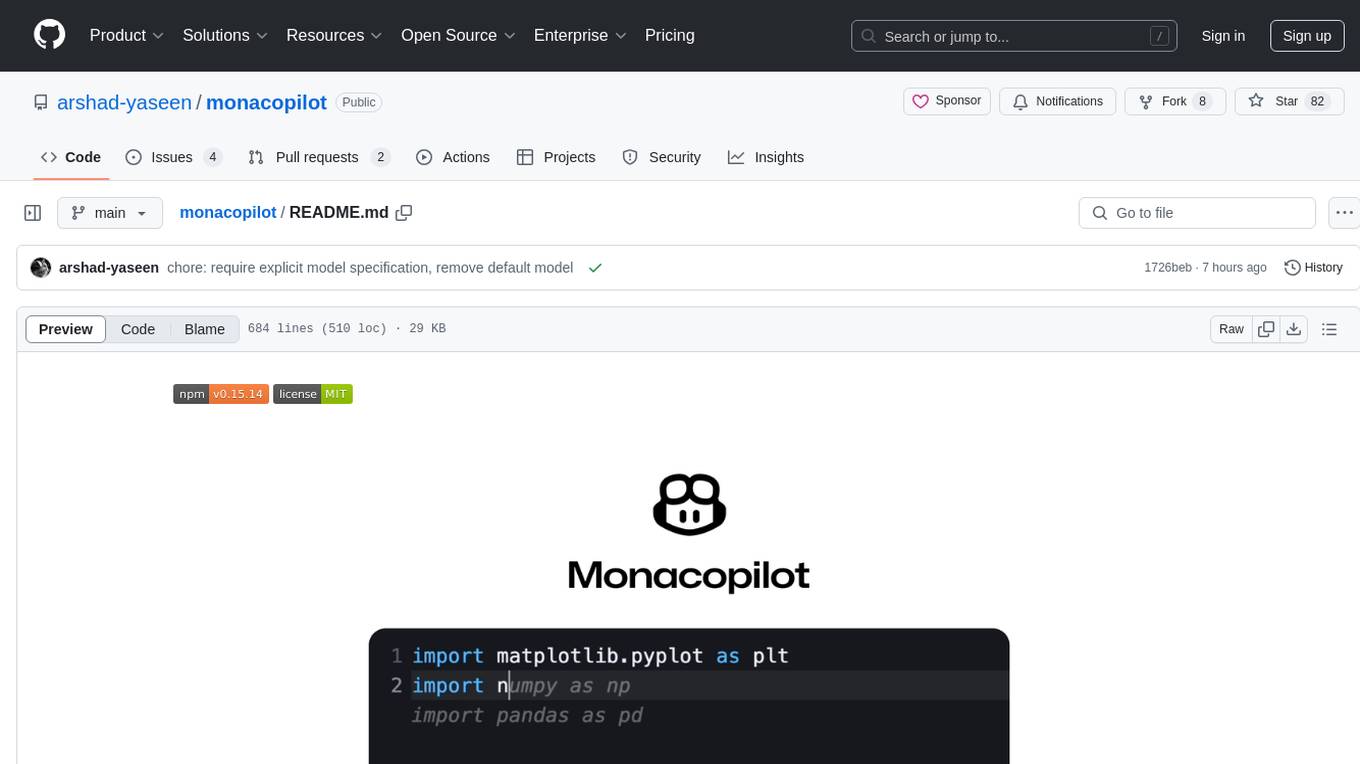
monacopilot
Monacopilot is a powerful and customizable AI auto-completion plugin for the Monaco Editor. It supports multiple AI providers such as Anthropic, OpenAI, Groq, and Google, providing real-time code completions with an efficient caching system. The plugin offers context-aware suggestions, customizable completion behavior, and framework agnostic features. Users can also customize the model support and trigger completions manually. Monacopilot is designed to enhance coding productivity by providing accurate and contextually appropriate completions in daily spoken language.
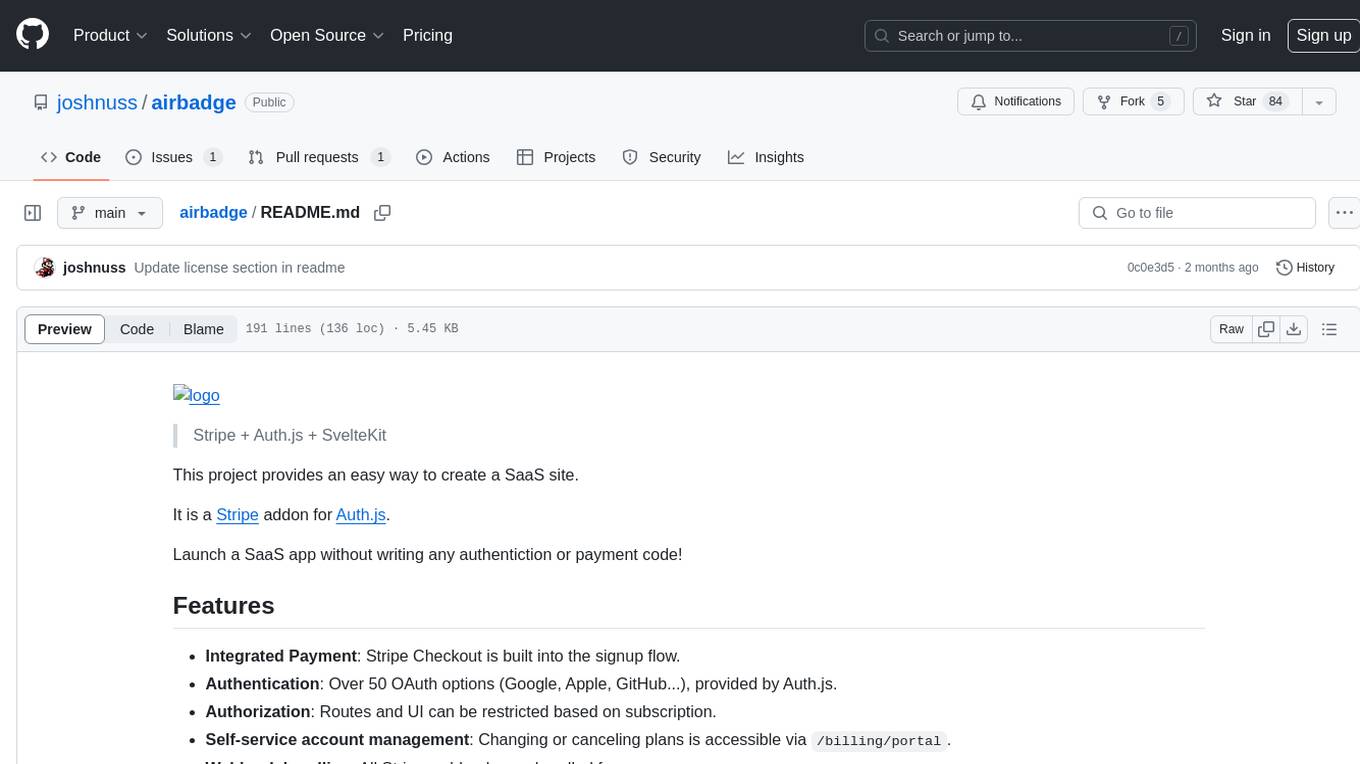
airbadge
Airbadge is a Stripe addon for Auth.js that provides an easy way to create a SaaS site without writing any authentication or payment code. It integrates Stripe Checkout into the signup flow, offers over 50 OAuth options for authentication, allows route and UI restriction based on subscription, enables self-service account management, handles all Stripe webhooks, supports trials and free plans, includes subscription and plan data in the session, and is open source with a BSL license. The project also provides components for conditional UI display based on subscription status and helper functions to restrict route access. Additionally, it offers a billing endpoint with various routes for billing operations. Setup involves installing @airbadge/sveltekit, setting up a database provider for Auth.js, adding environment variables, configuring authentication and billing options, and forwarding Stripe events to localhost.
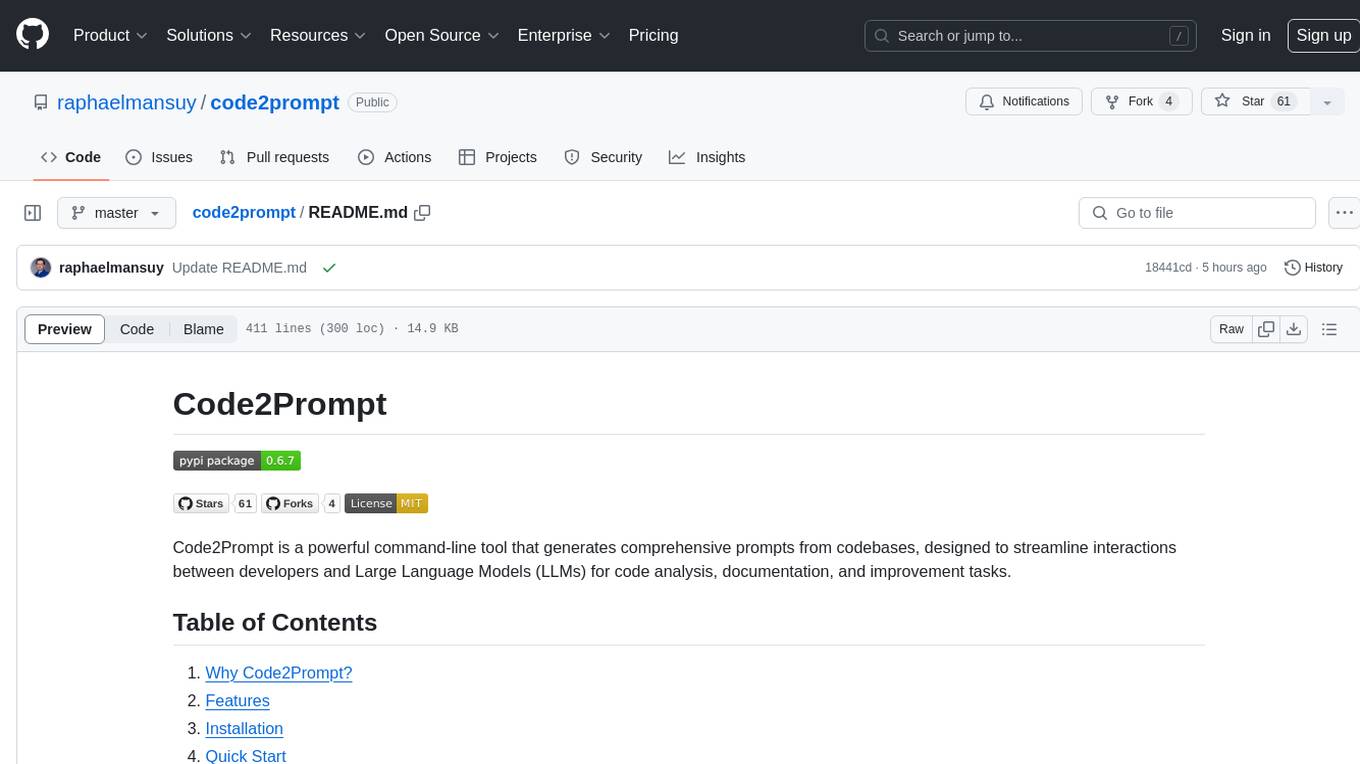
code2prompt
Code2Prompt is a powerful command-line tool that generates comprehensive prompts from codebases, designed to streamline interactions between developers and Large Language Models (LLMs) for code analysis, documentation, and improvement tasks. It bridges the gap between codebases and LLMs by converting projects into AI-friendly prompts, enabling users to leverage AI for various software development tasks. The tool offers features like holistic codebase representation, intelligent source tree generation, customizable prompt templates, smart token management, Gitignore integration, flexible file handling, clipboard-ready output, multiple output options, and enhanced code readability.
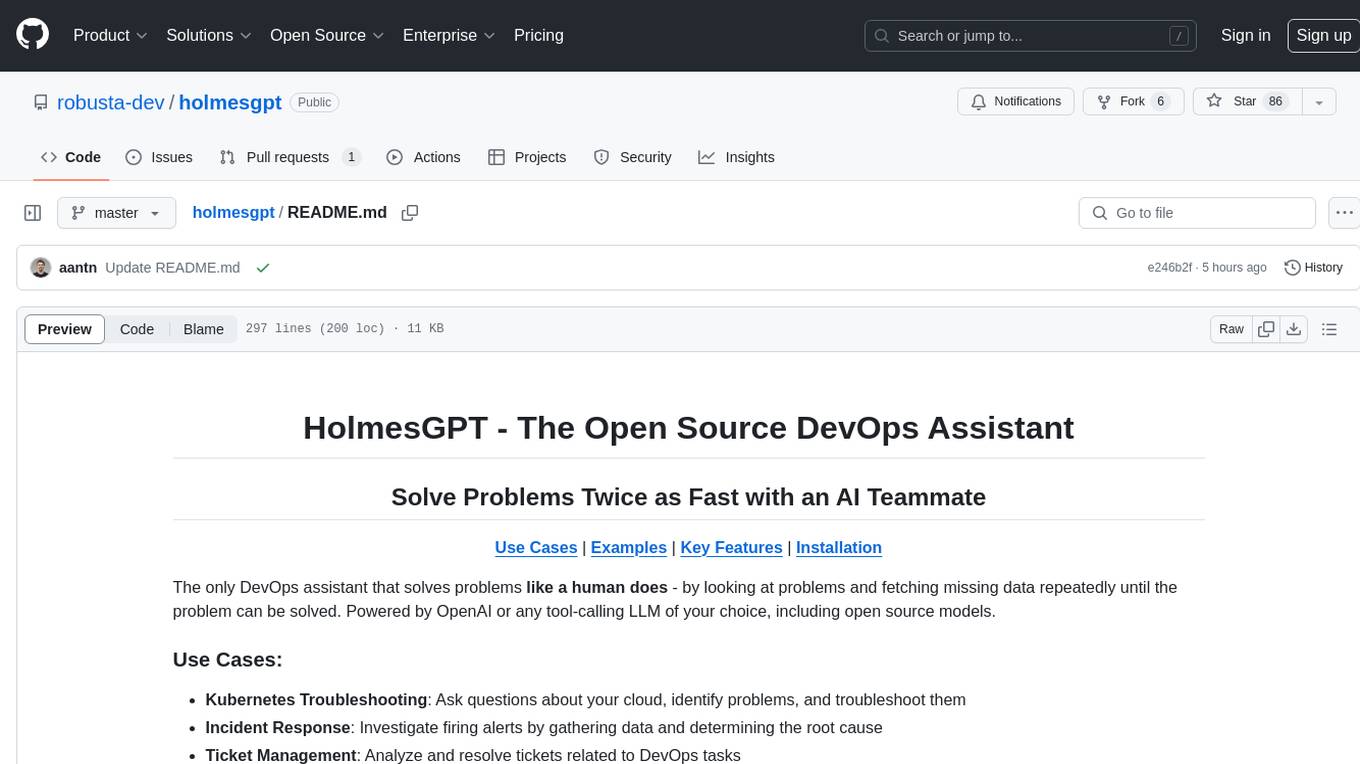
holmesgpt
HolmesGPT is an open-source DevOps assistant powered by OpenAI or any tool-calling LLM of your choice. It helps in troubleshooting Kubernetes, incident response, ticket management, automated investigation, and runbook automation in plain English. The tool connects to existing observability data, is compliance-friendly, provides transparent results, supports extensible data sources, runbook automation, and integrates with existing workflows. Users can install HolmesGPT using Brew, prebuilt Docker container, Python Poetry, or Docker. The tool requires an API key for functioning and supports OpenAI, Azure AI, and self-hosted LLMs.
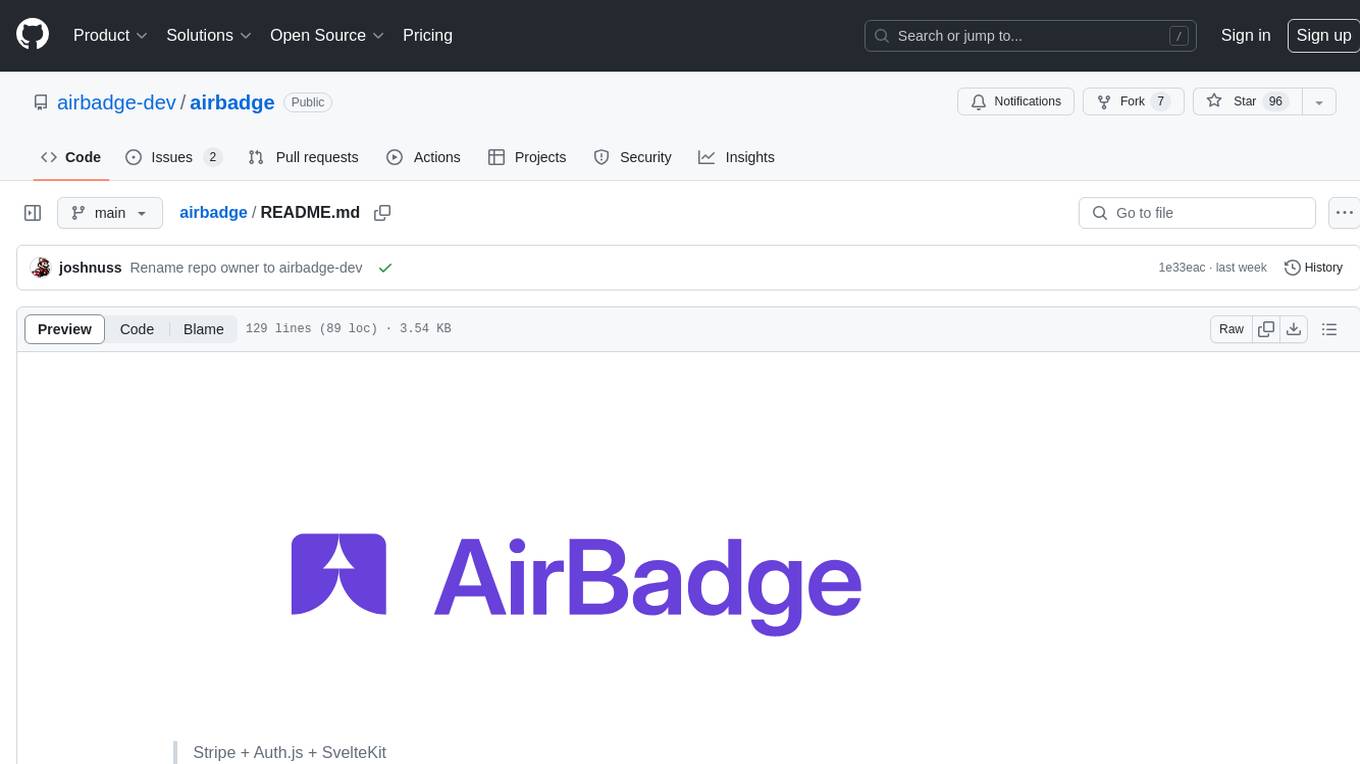
airbadge
Airbadge is a Stripe addon for Auth.js that simplifies the process of creating a SaaS site by integrating payment, authentication, gating, self-service account management, webhook handling, trials & free plans, session data, and more. It allows users to launch a SaaS app without writing any authentication or payment code. The project is open source and free to use with optional paid features under the BSL License.
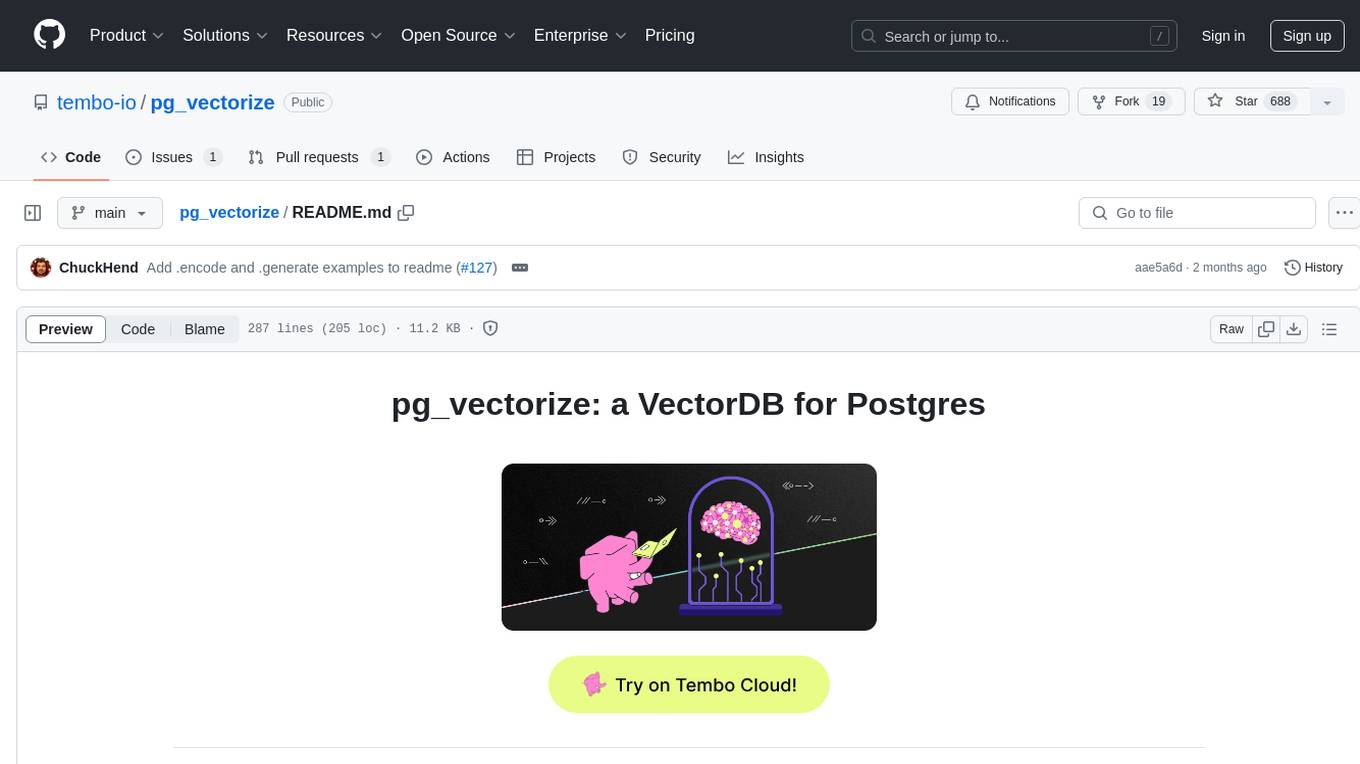
pg_vectorize
pg_vectorize is a Postgres extension that automates text to embeddings transformation, enabling vector search and LLM applications with minimal function calls. It integrates with popular LLMs, provides workflows for vector search and RAG, and automates Postgres triggers for updating embeddings. The tool is part of the VectorDB Stack on Tembo Cloud, offering high-level APIs for easy initialization and search.
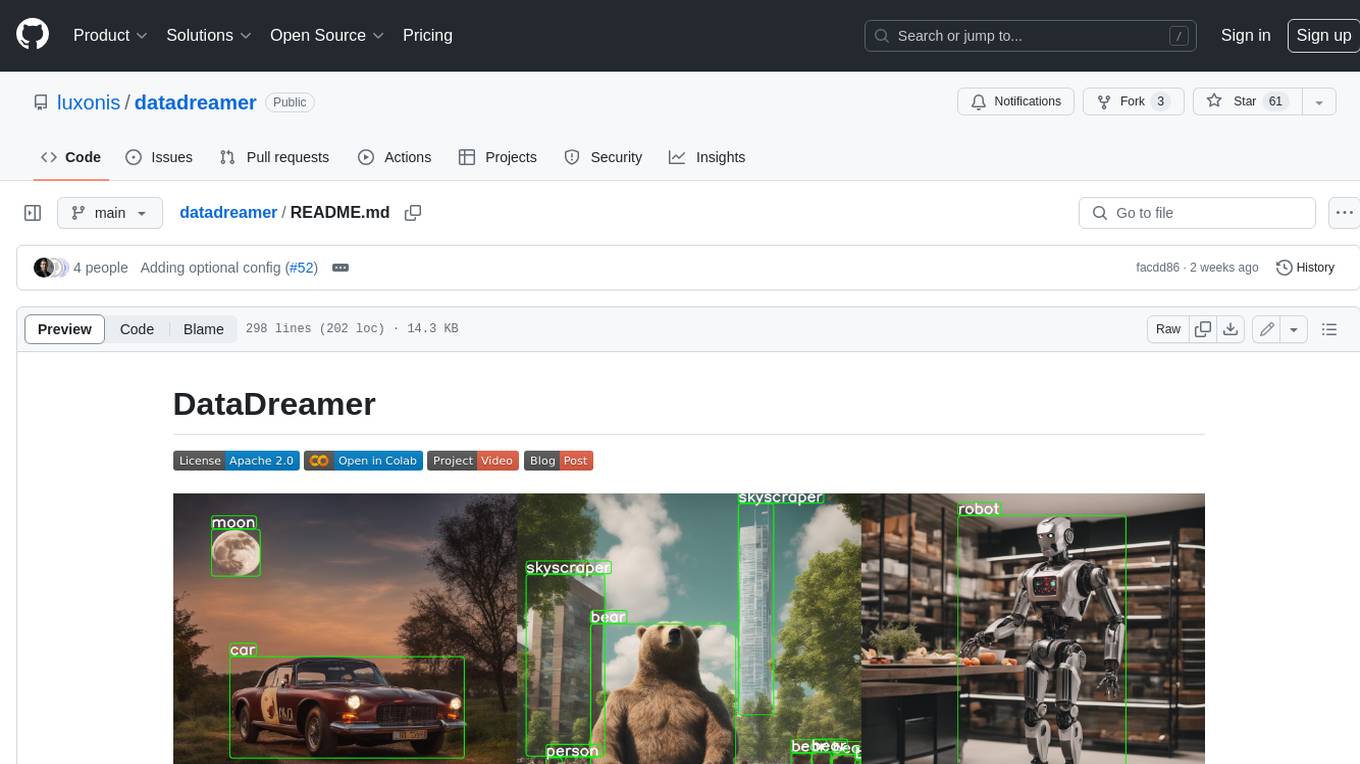
datadreamer
DataDreamer is an advanced toolkit designed to facilitate the development of edge AI models by enabling synthetic data generation, knowledge extraction from pre-trained models, and creation of efficient and potent models. It eliminates the need for extensive datasets by generating synthetic datasets, leverages latent knowledge from pre-trained models, and focuses on creating compact models suitable for integration into any device and performance for specialized tasks. The toolkit offers features like prompt generation, image generation, dataset annotation, and tools for training small-scale neural networks for edge deployment. It provides hardware requirements, usage instructions, available models, and limitations to consider while using the library.
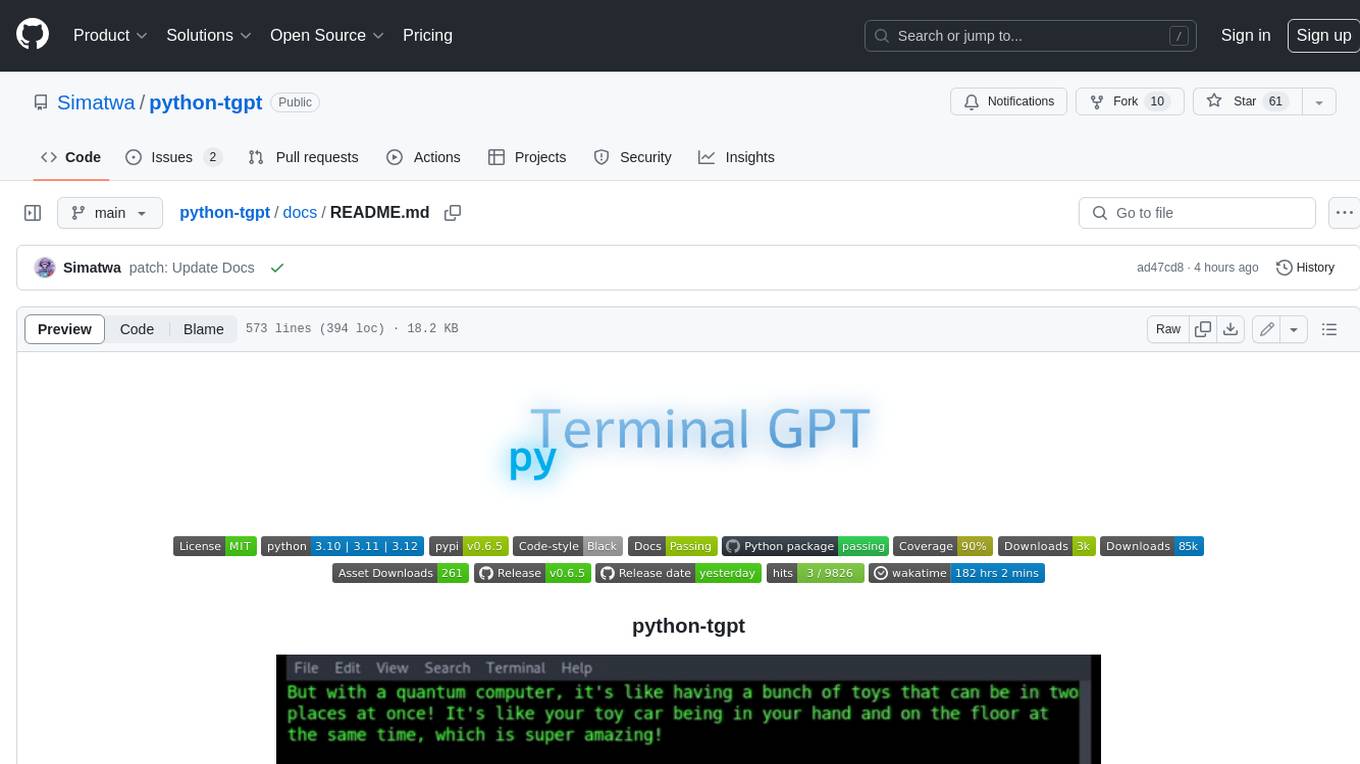
python-tgpt
Python-tgpt is a Python package that enables seamless interaction with over 45 free LLM providers without requiring an API key. It also provides image generation capabilities. The name _python-tgpt_ draws inspiration from its parent project tgpt, which operates on Golang. Through this Python adaptation, users can effortlessly engage with a number of free LLMs available, fostering a smoother AI interaction experience.
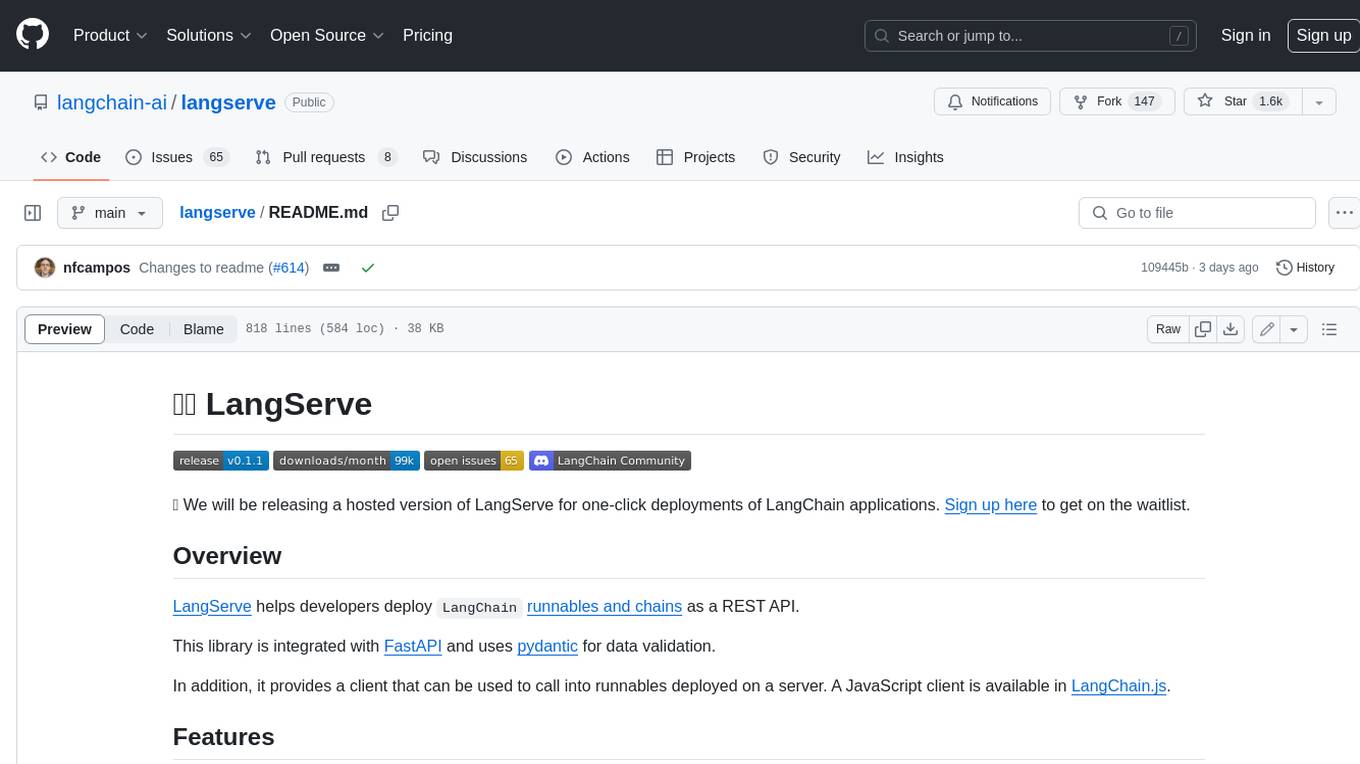
langserve
LangServe helps developers deploy `LangChain` runnables and chains as a REST API. This library is integrated with FastAPI and uses pydantic for data validation. In addition, it provides a client that can be used to call into runnables deployed on a server. A JavaScript client is available in LangChain.js.
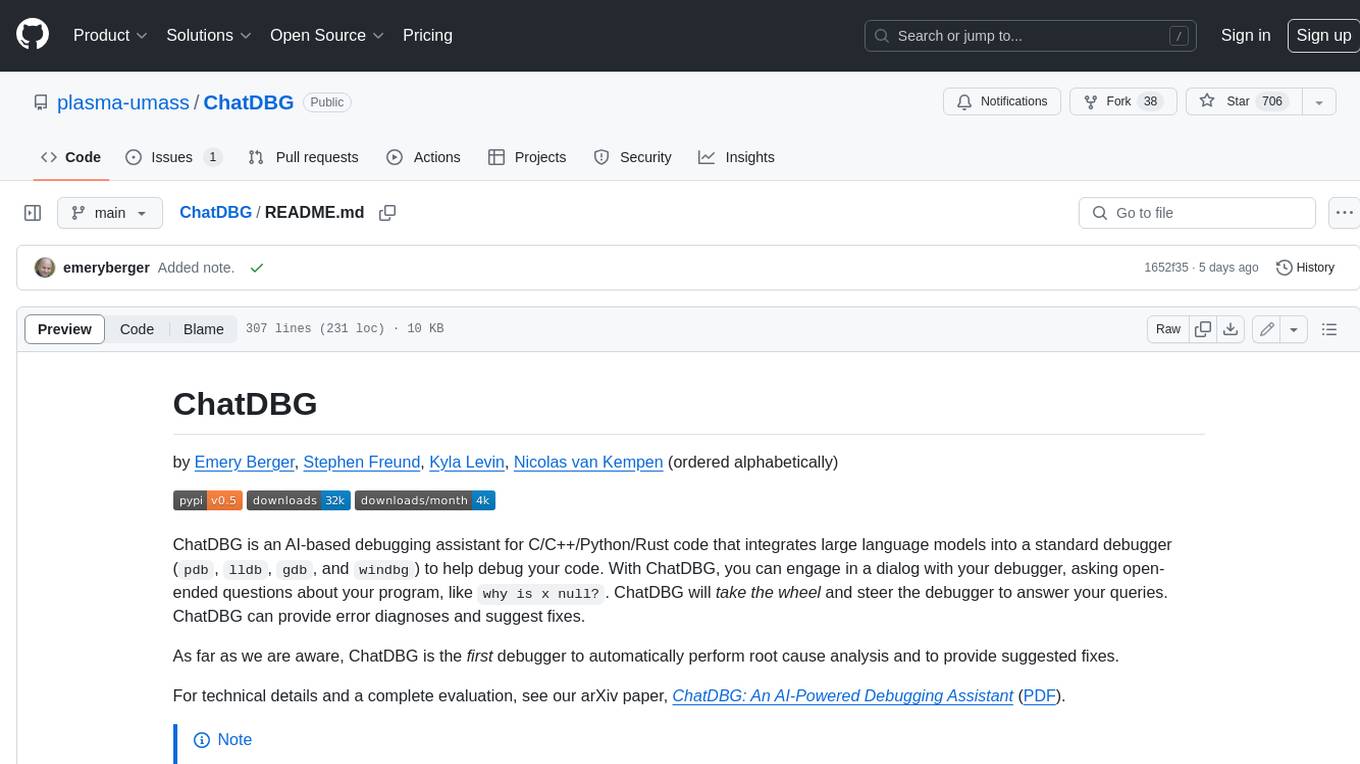
ChatDBG
ChatDBG is an AI-based debugging assistant for C/C++/Python/Rust code that integrates large language models into a standard debugger (`pdb`, `lldb`, `gdb`, and `windbg`) to help debug your code. With ChatDBG, you can engage in a dialog with your debugger, asking open-ended questions about your program, like `why is x null?`. ChatDBG will _take the wheel_ and steer the debugger to answer your queries. ChatDBG can provide error diagnoses and suggest fixes. As far as we are aware, ChatDBG is the _first_ debugger to automatically perform root cause analysis and to provide suggested fixes.
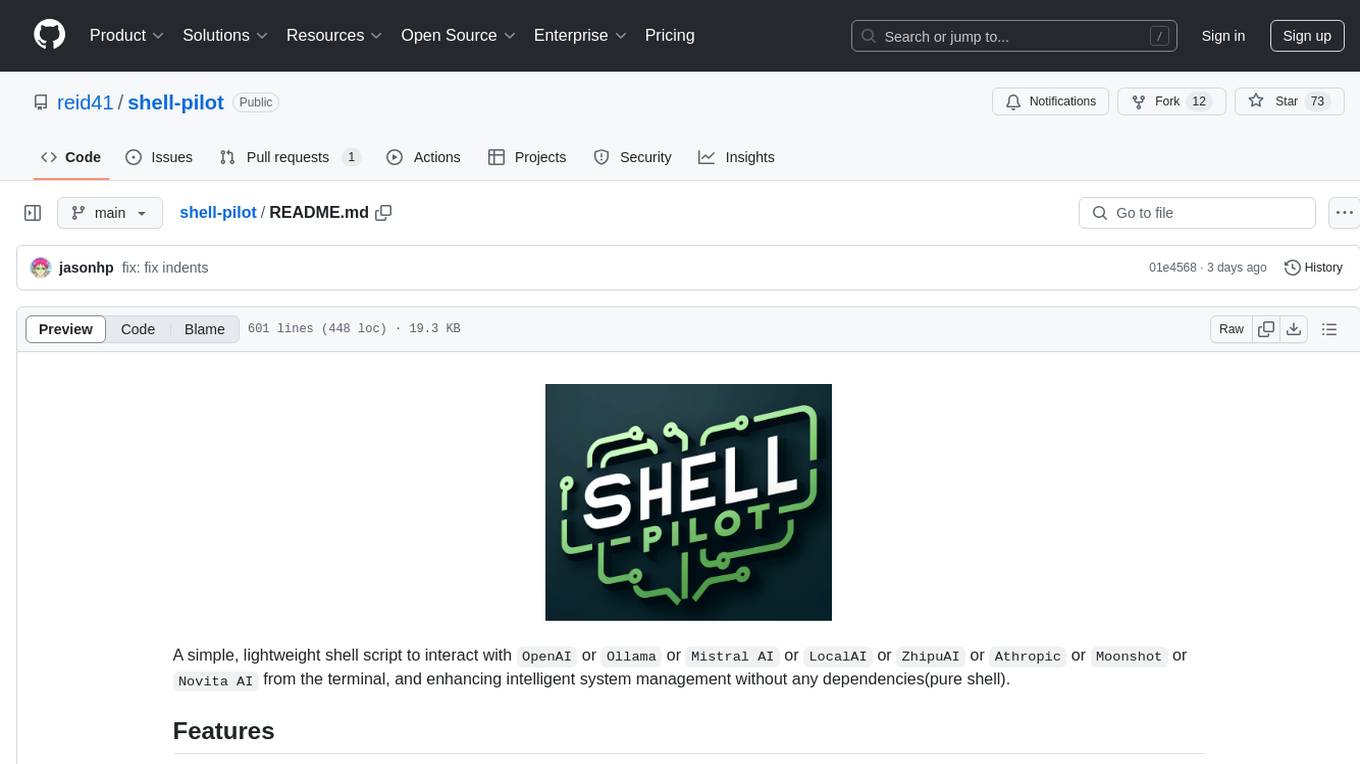
shell-pilot
Shell-pilot is a simple, lightweight shell script designed to interact with various AI models such as OpenAI, Ollama, Mistral AI, LocalAI, ZhipuAI, Anthropic, Moonshot, and Novita AI from the terminal. It enhances intelligent system management without any dependencies, offering features like setting up a local LLM repository, using official models and APIs, viewing history and session persistence, passing input prompts with pipe/redirector, listing available models, setting request parameters, generating and running commands in the terminal, easy configuration setup, system package version checking, and managing system aliases.
For similar tasks
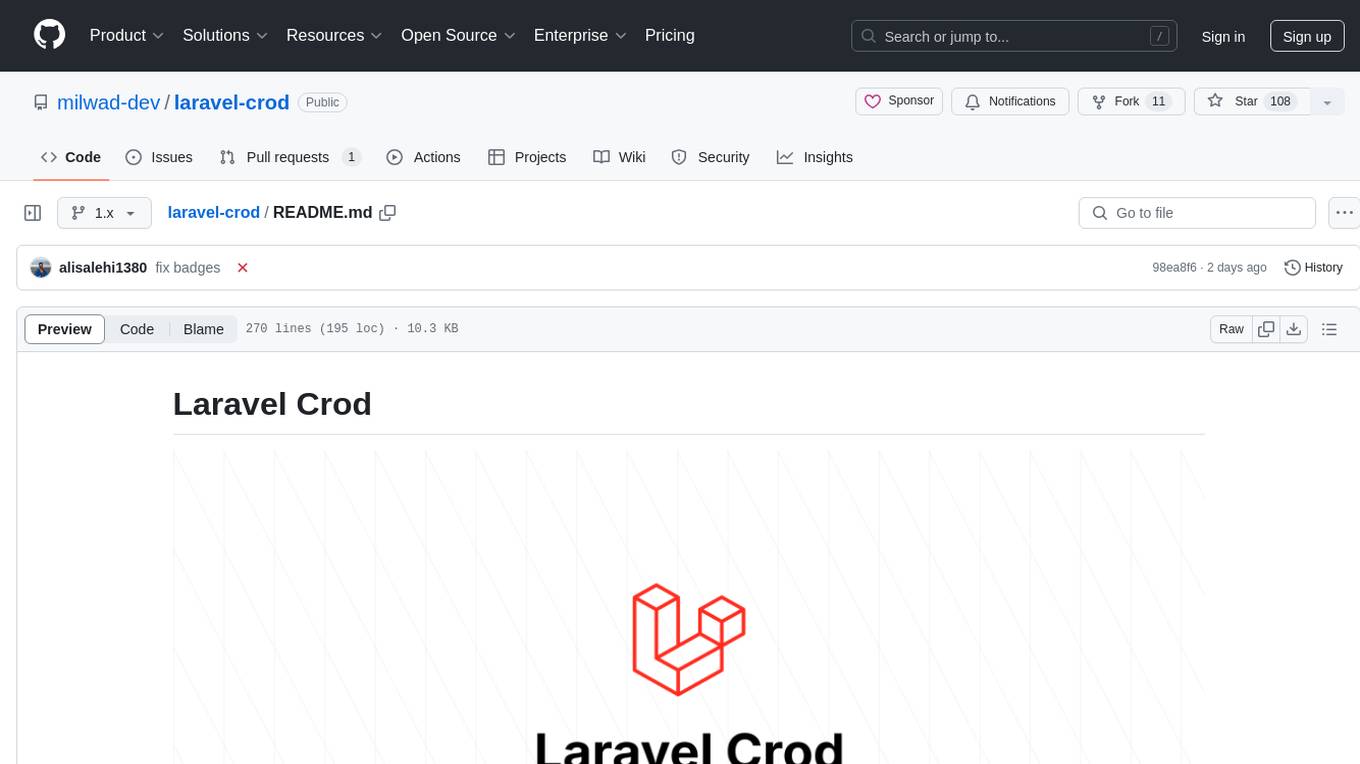
laravel-crod
Laravel Crod is a package designed to facilitate the implementation of CRUD operations in Laravel projects. It allows users to quickly generate controllers, models, migrations, services, repositories, views, and requests with various customization options. The package simplifies tasks such as creating resource controllers, making models fillable, querying repositories and services, and generating additional files like seeders and factories. Laravel Crod aims to streamline the process of building CRUD functionalities in Laravel applications by providing a set of commands and tools for developers.
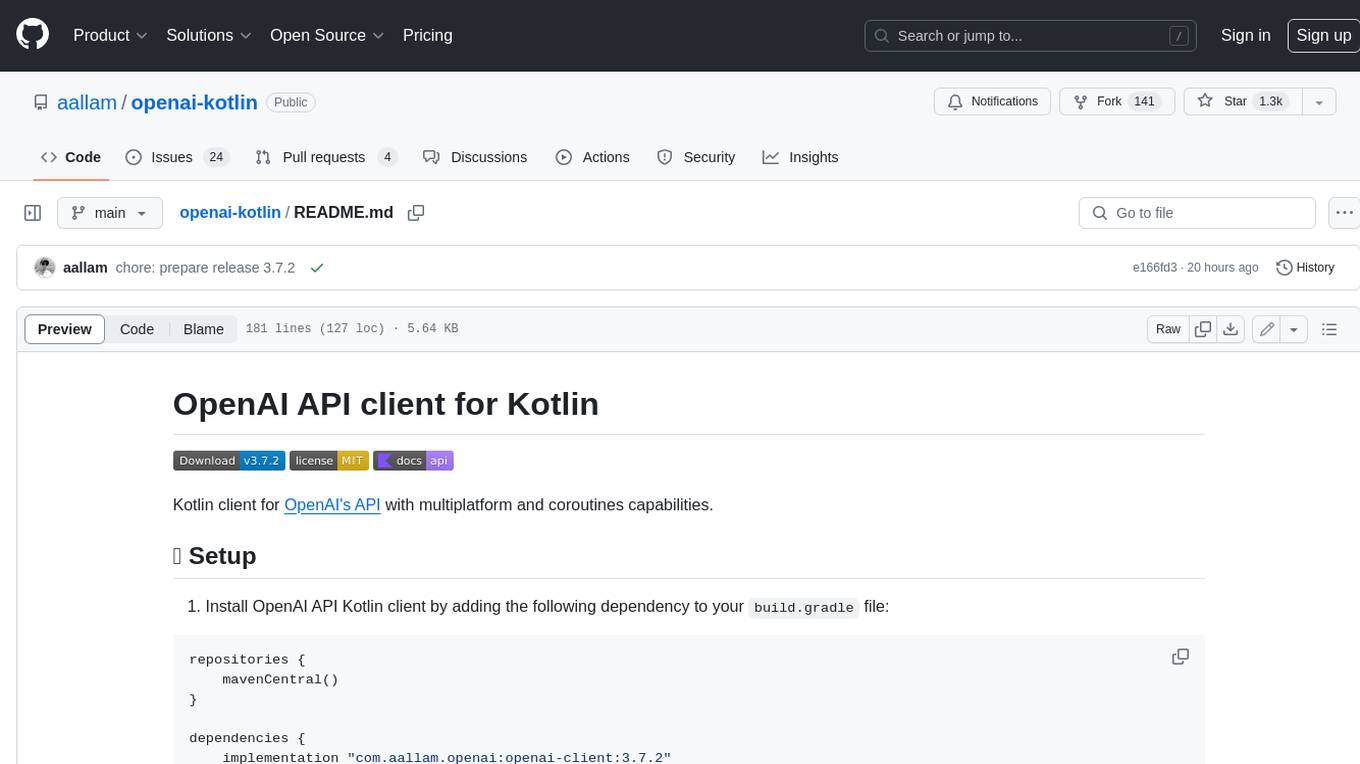
openai-kotlin
OpenAI Kotlin API client is a Kotlin client for OpenAI's API with multiplatform and coroutines capabilities. It allows users to interact with OpenAI's API using Kotlin programming language. The client supports various features such as models, chat, images, embeddings, files, fine-tuning, moderations, audio, assistants, threads, messages, and runs. It also provides guides on getting started, chat & function call, file source guide, and assistants. Sample apps are available for reference, and troubleshooting guides are provided for common issues. The project is open-source and licensed under the MIT license, allowing contributions from the community.
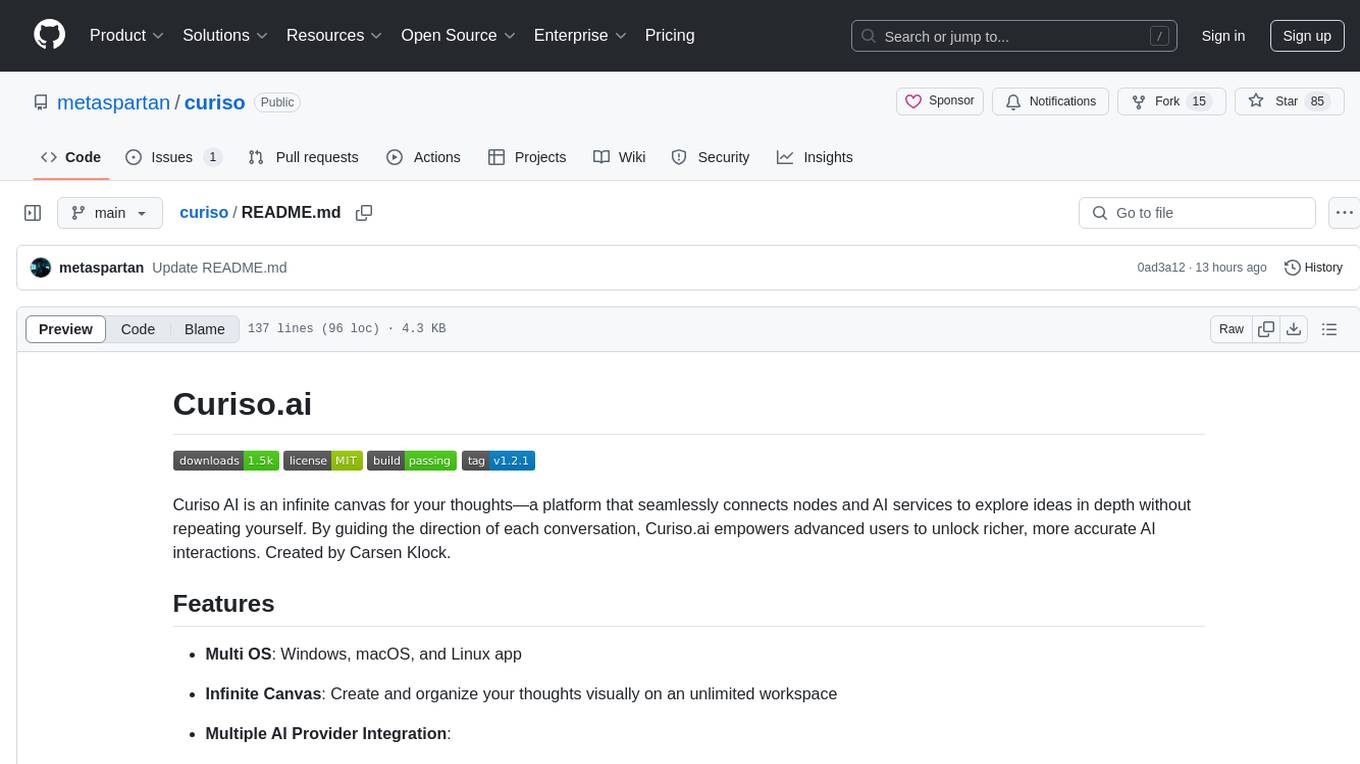
curiso
Curiso AI is an infinite canvas platform that connects nodes and AI services to explore ideas without repetition. It empowers advanced users to unlock richer AI interactions. Features include multi OS support, infinite canvas, multiple AI provider integration, local AI inference provider integration, custom model support, model metrics, RAG support, local Transformers.js embedding models, inference parameters customization, multiple boards, vision model support, customizable interface, node-based conversations, and secure local encrypted storage. Curiso also offers a Solana token for exclusive access to premium features and enhanced AI capabilities.
For similar jobs
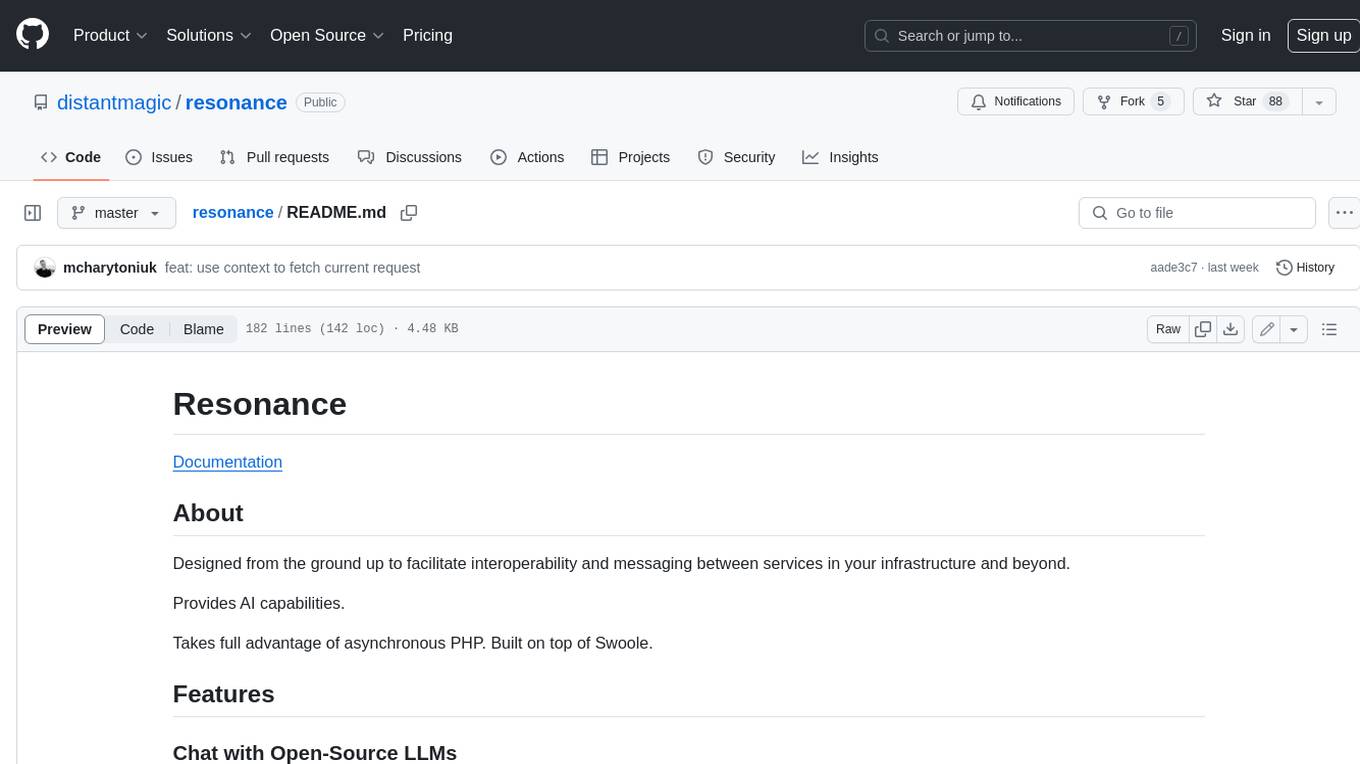
resonance
Resonance is a framework designed to facilitate interoperability and messaging between services in your infrastructure and beyond. It provides AI capabilities and takes full advantage of asynchronous PHP, built on top of Swoole. With Resonance, you can: * Chat with Open-Source LLMs: Create prompt controllers to directly answer user's prompts. LLM takes care of determining user's intention, so you can focus on taking appropriate action. * Asynchronous Where it Matters: Respond asynchronously to incoming RPC or WebSocket messages (or both combined) with little overhead. You can set up all the asynchronous features using attributes. No elaborate configuration is needed. * Simple Things Remain Simple: Writing HTTP controllers is similar to how it's done in the synchronous code. Controllers have new exciting features that take advantage of the asynchronous environment. * Consistency is Key: You can keep the same approach to writing software no matter the size of your project. There are no growing central configuration files or service dependencies registries. Every relation between code modules is local to those modules. * Promises in PHP: Resonance provides a partial implementation of Promise/A+ spec to handle various asynchronous tasks. * GraphQL Out of the Box: You can build elaborate GraphQL schemas by using just the PHP attributes. Resonance takes care of reusing SQL queries and optimizing the resources' usage. All fields can be resolved asynchronously.
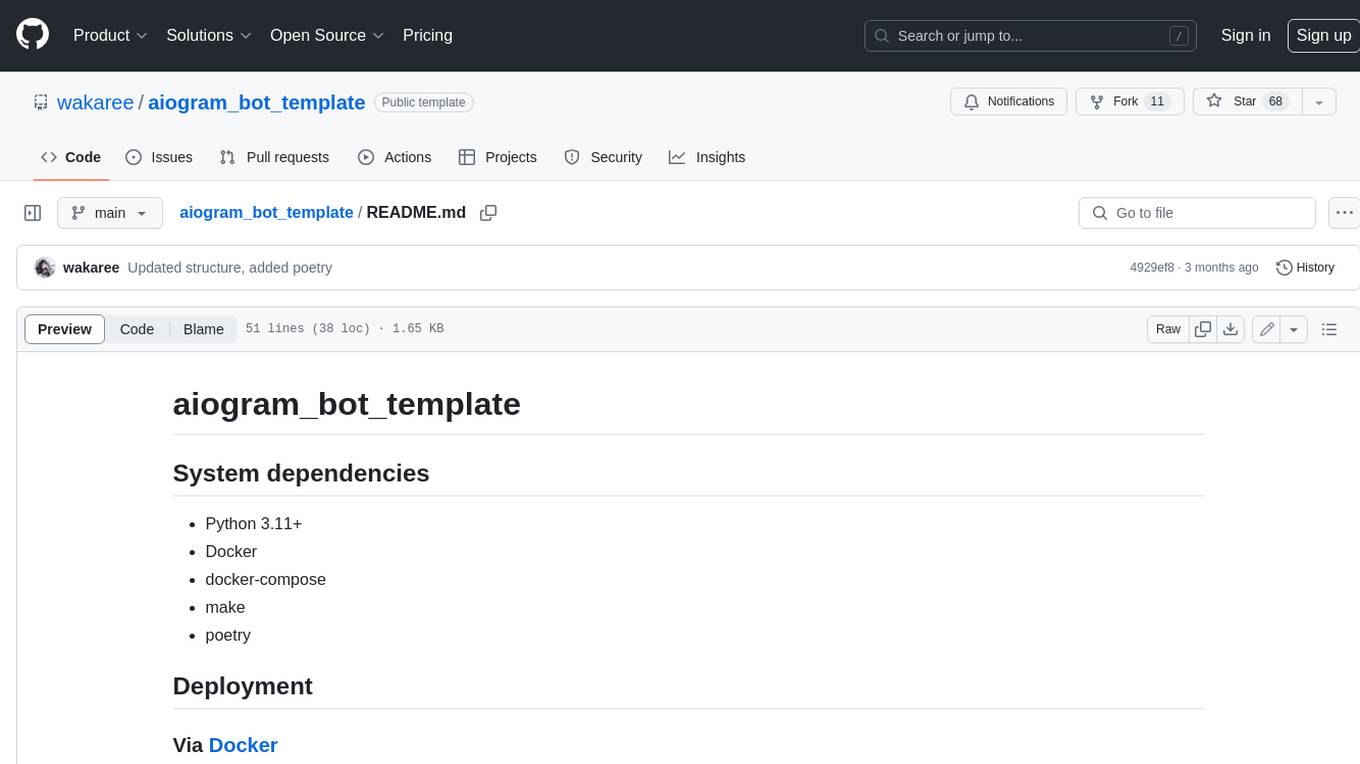
aiogram_bot_template
Aiogram bot template is a boilerplate for creating Telegram bots using Aiogram framework. It provides a solid foundation for building robust and scalable bots with a focus on code organization, database integration, and localization.
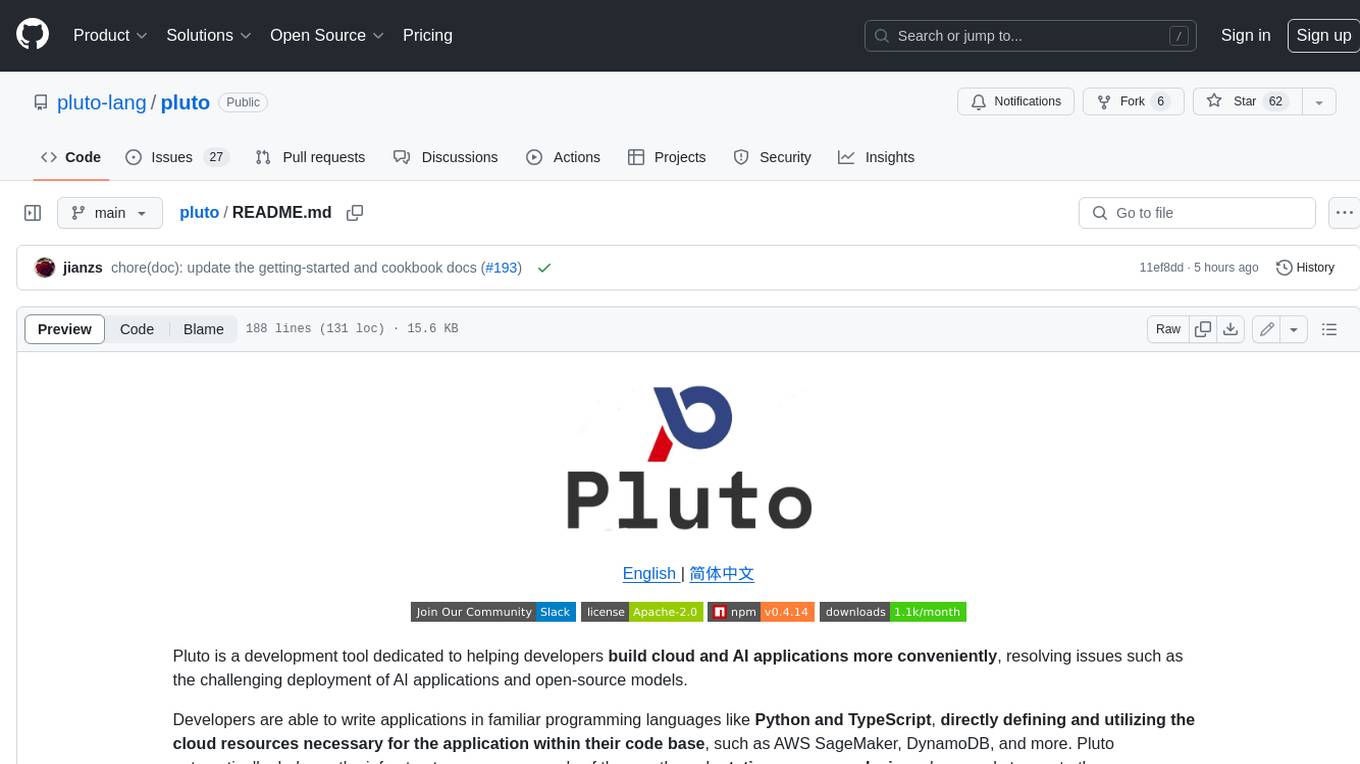
pluto
Pluto is a development tool dedicated to helping developers **build cloud and AI applications more conveniently** , resolving issues such as the challenging deployment of AI applications and open-source models. Developers are able to write applications in familiar programming languages like **Python and TypeScript** , **directly defining and utilizing the cloud resources necessary for the application within their code base** , such as AWS SageMaker, DynamoDB, and more. Pluto automatically deduces the infrastructure resource needs of the app through **static program analysis** and proceeds to create these resources on the specified cloud platform, **simplifying the resources creation and application deployment process**.
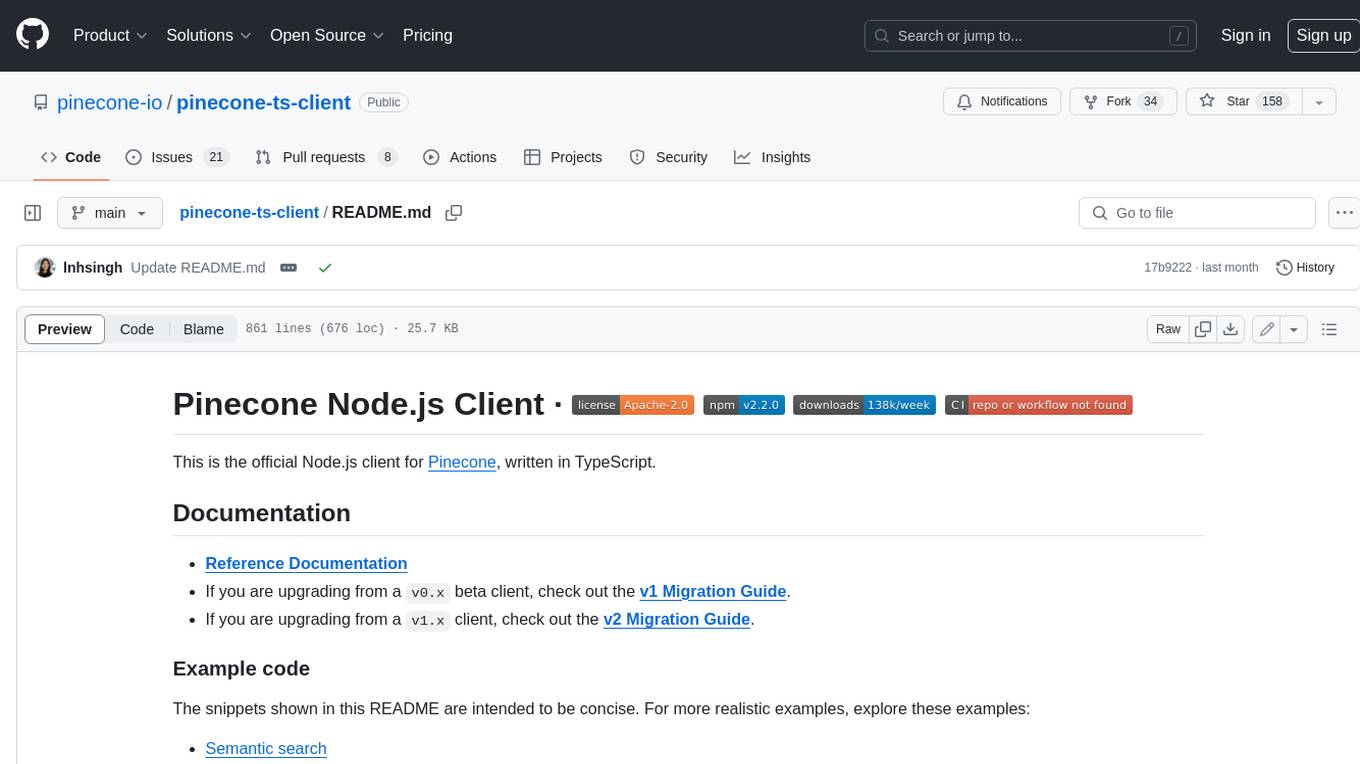
pinecone-ts-client
The official Node.js client for Pinecone, written in TypeScript. This client library provides a high-level interface for interacting with the Pinecone vector database service. With this client, you can create and manage indexes, upsert and query vector data, and perform other operations related to vector search and retrieval. The client is designed to be easy to use and provides a consistent and idiomatic experience for Node.js developers. It supports all the features and functionality of the Pinecone API, making it a comprehensive solution for building vector-powered applications in Node.js.
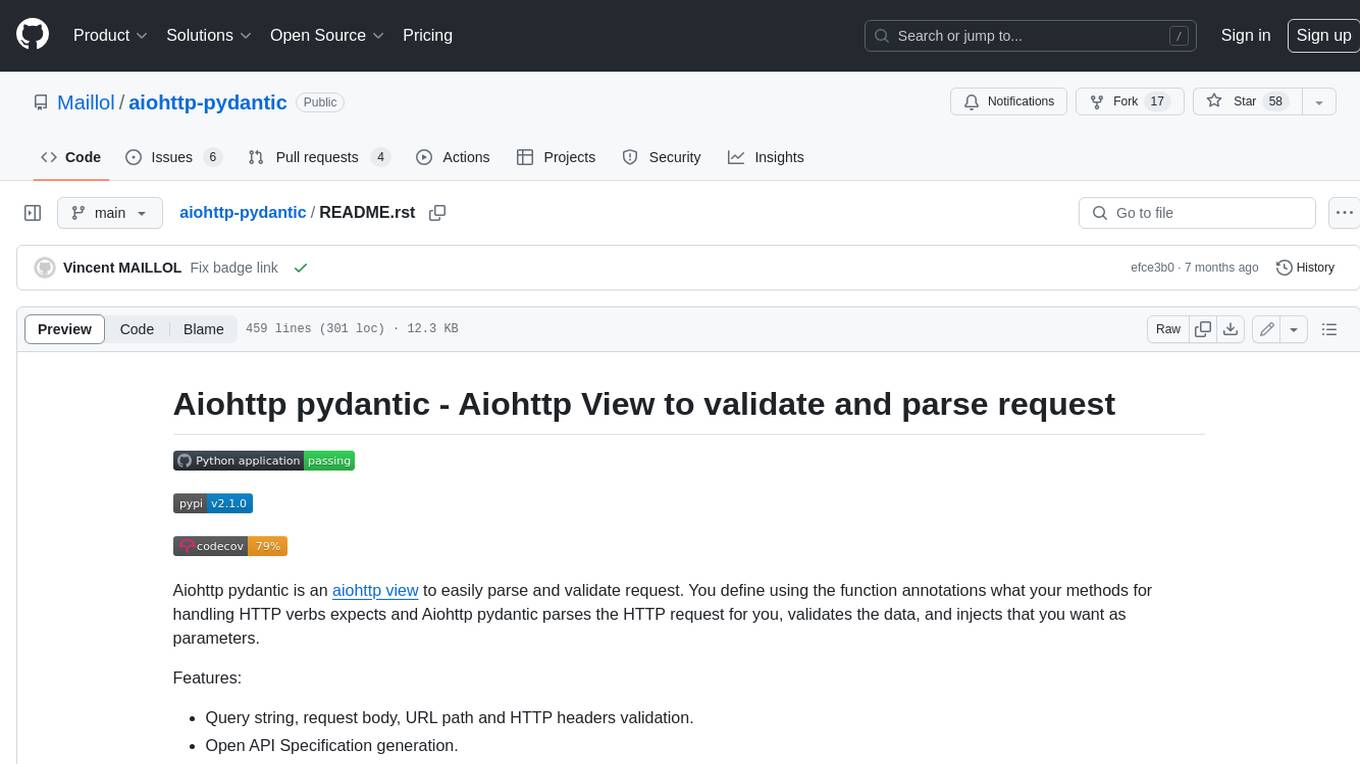
aiohttp-pydantic
Aiohttp pydantic is an aiohttp view to easily parse and validate requests. You define using function annotations what your methods for handling HTTP verbs expect, and Aiohttp pydantic parses the HTTP request for you, validates the data, and injects the parameters you want. It provides features like query string, request body, URL path, and HTTP headers validation, as well as Open API Specification generation.
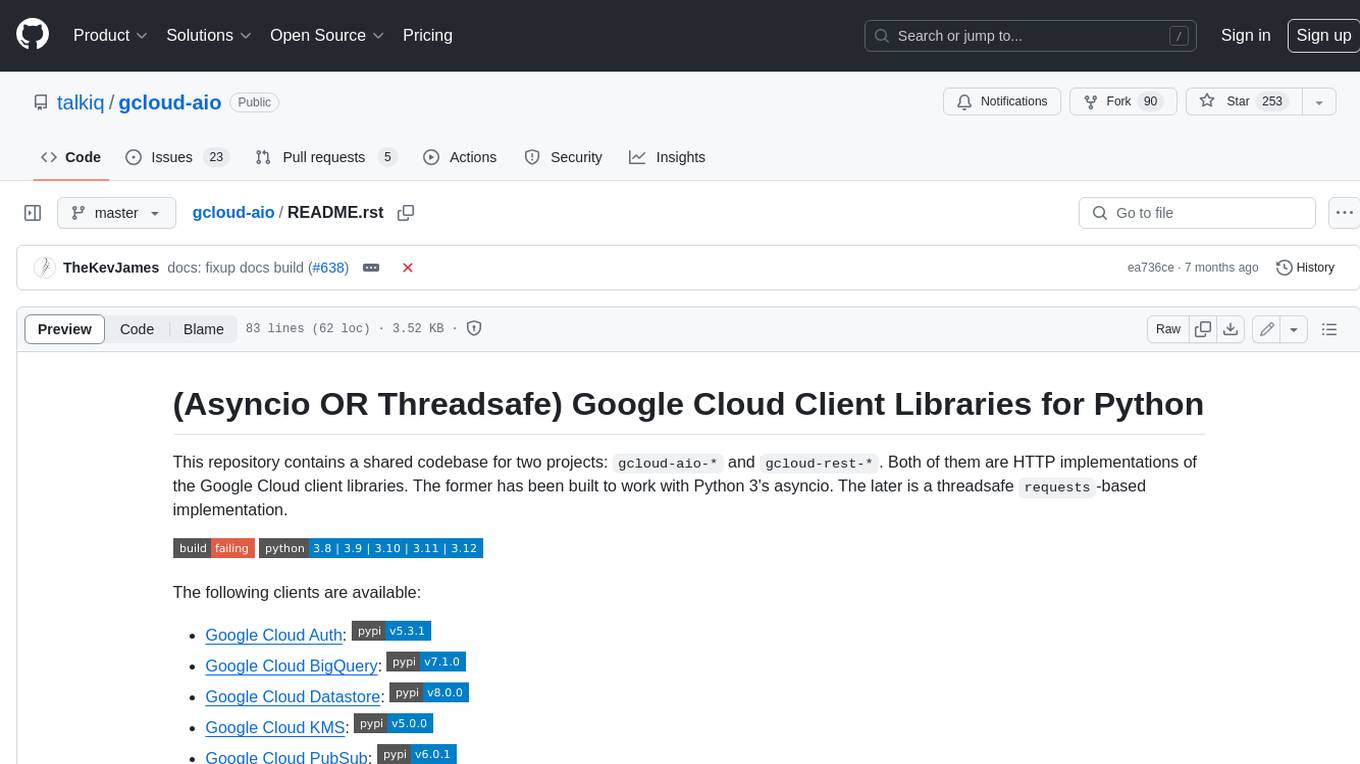
gcloud-aio
This repository contains shared codebase for two projects: gcloud-aio and gcloud-rest. gcloud-aio is built for Python 3's asyncio, while gcloud-rest is a threadsafe requests-based implementation. It provides clients for Google Cloud services like Auth, BigQuery, Datastore, KMS, PubSub, Storage, and Task Queue. Users can install the library using pip and refer to the documentation for usage details. Developers can contribute to the project by following the contribution guide.
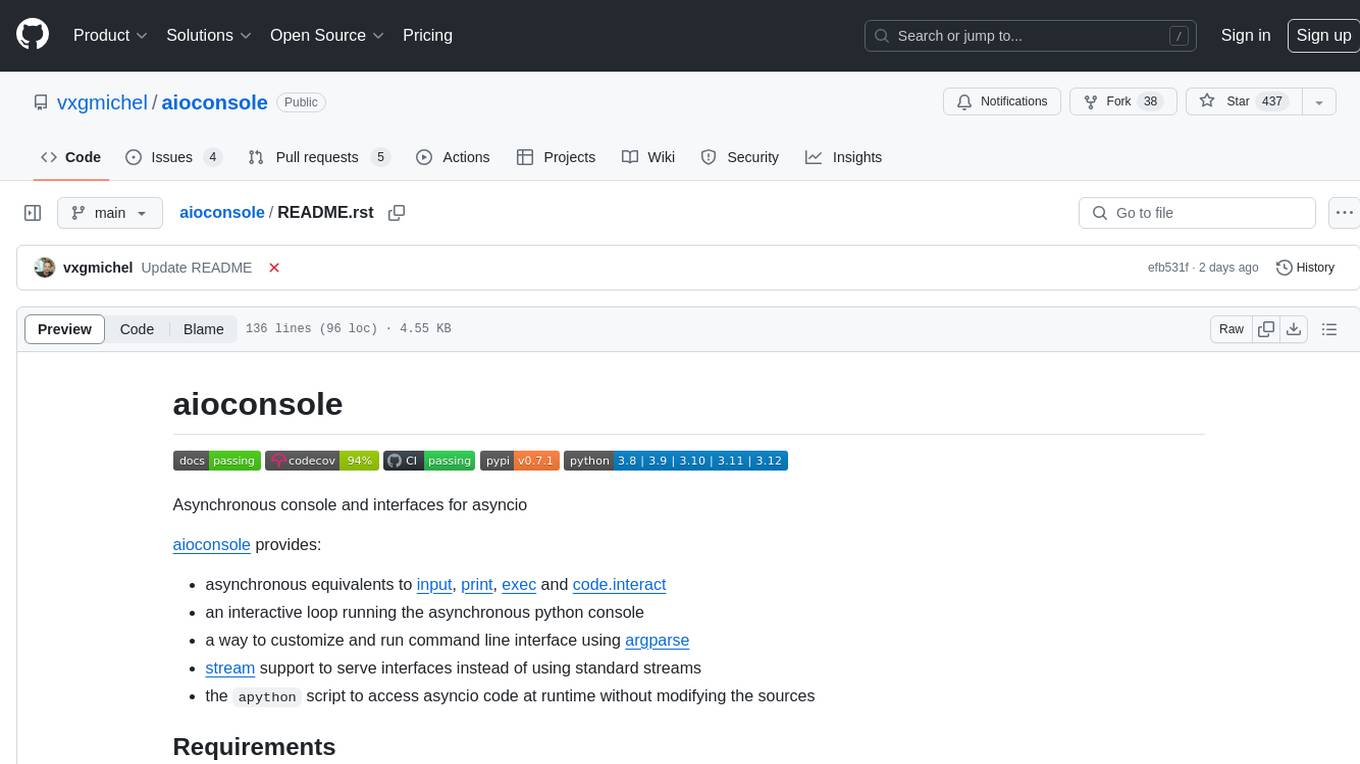
aioconsole
aioconsole is a Python package that provides asynchronous console and interfaces for asyncio. It offers asynchronous equivalents to input, print, exec, and code.interact, an interactive loop running the asynchronous Python console, customization and running of command line interfaces using argparse, stream support to serve interfaces instead of using standard streams, and the apython script to access asyncio code at runtime without modifying the sources. The package requires Python version 3.8 or higher and can be installed from PyPI or GitHub. It allows users to run Python files or modules with a modified asyncio policy, replacing the default event loop with an interactive loop. aioconsole is useful for scenarios where users need to interact with asyncio code in a console environment.
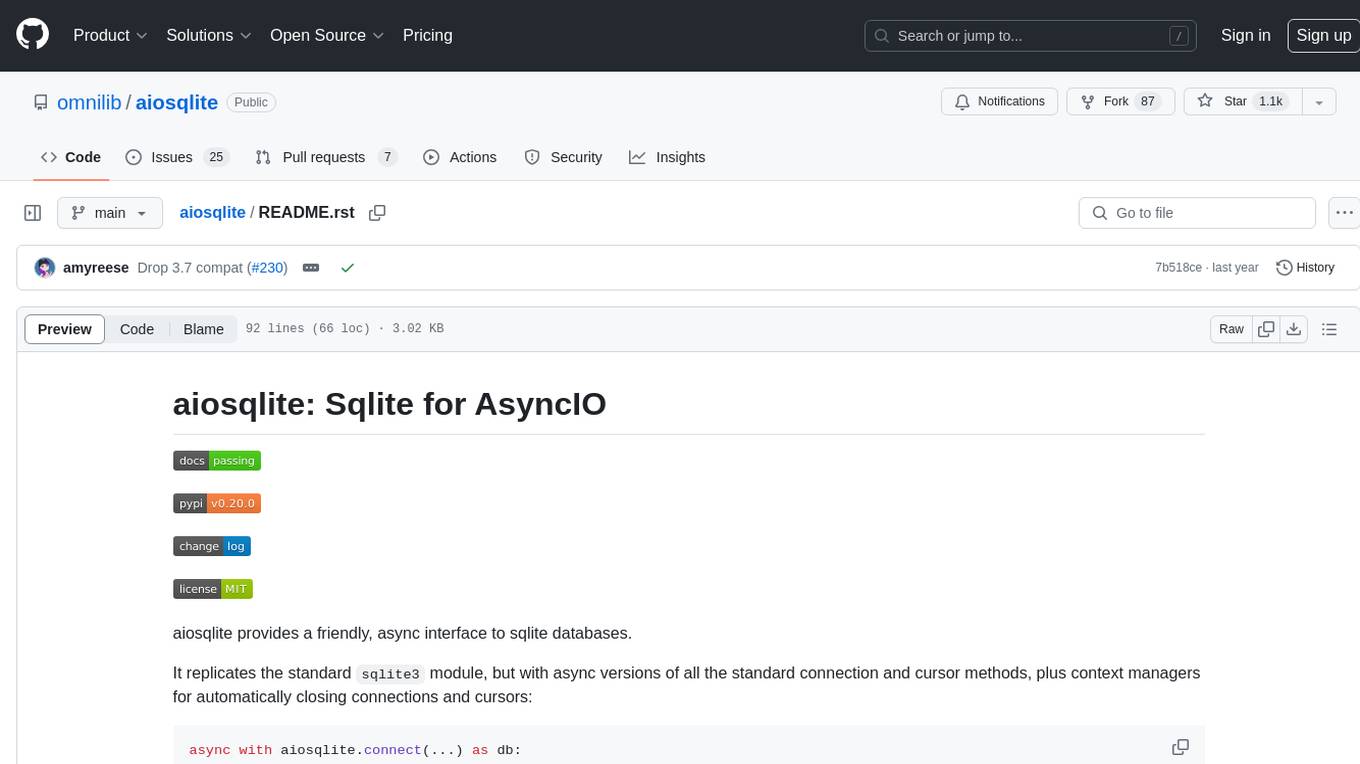
aiosqlite
aiosqlite is a Python library that provides a friendly, async interface to SQLite databases. It replicates the standard sqlite3 module but with async versions of all the standard connection and cursor methods, along with context managers for automatically closing connections and cursors. It allows interaction with SQLite databases on the main AsyncIO event loop without blocking execution of other coroutines while waiting for queries or data fetches. The library also replicates most of the advanced features of sqlite3, such as row factories and total changes tracking.