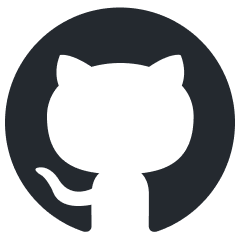
nuxt-llms
Automatically generates /llms.txt markdown documentation for your Nuxt application.
Stars: 117
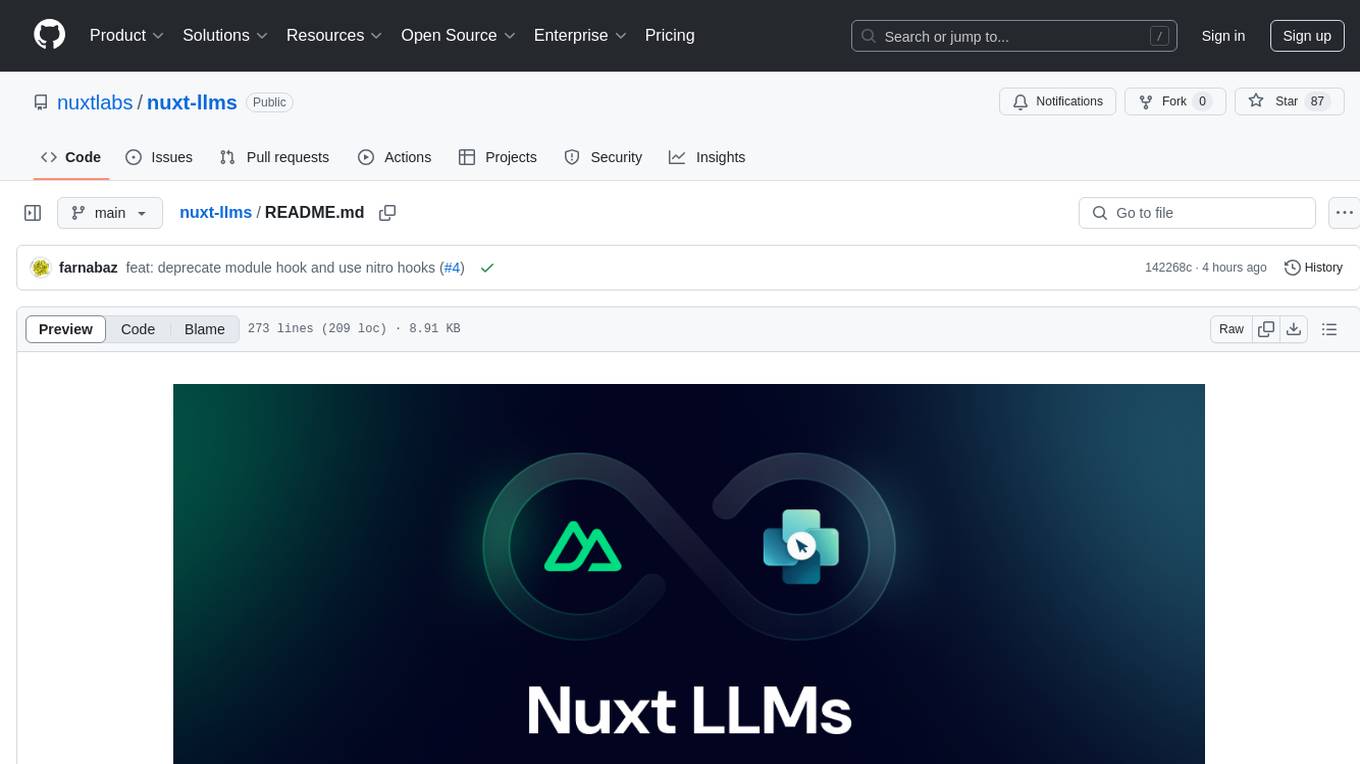
Nuxt LLMs automatically generates llms.txt markdown documentation for Nuxt applications. It provides runtime hooks to collect data from various sources and generate structured documentation. The tool allows customization of sections directly from nuxt.config.ts and integrates with Nuxt modules via the runtime hooks system. It generates two documentation formats: llms.txt for concise structured documentation and llms_full.txt for detailed documentation. Users can extend documentation using hooks to add sections, links, and metadata. The tool is suitable for developers looking to automate documentation generation for their Nuxt applications.
README:
Nuxt LLMs automatically generates llms.txt
markdown documentation for your Nuxt application. It provides runtime hooks to collect data from various sources (CMS, Nuxt Content, etc.) and generate structured documentation in a text-based format.
- Generates & prerenders
/llms.txt
automatically - Generate & prerenders
/llms-full.txt
when enabled - Customizable sections directly from your
nuxt.config.ts
- Integrates with Nuxt modules and your application via the runtime hooks system
- Install the module:
npm i nuxt-llms
- Register
nuxt-llms
in yournuxt.config.ts
:
export default defineNuxtConfig({
modules: ['nuxt-llms']
})
- Configure your application details:
export default defineNuxtConfig({
modules: ['nuxt-llms'],
llms: {
domain: 'https://example.com',
title: 'My Application',
description: 'My Application Description',
sections: [
{
title: 'Section 1',
description: 'Section 1 Description',
links: [
{
title: 'Link 1',
description: 'Link 1 Description',
href: '/link-1',
},
{
title: 'Link 2',
description: 'Link 2 Description',
href: '/link-2',
},
],
},
],
},
})
That's it! You can visit /llms.txt
to see the generated documentation ✨
-
domain
(required): The domain of the application -
title
: The title of the application, will be displayed at the top of the documentation -
description
: The description of the application, will be displayed at the top of the documentation right after the title -
sections
: The sections of the documentation. Section are consisted of a title, one or more paragraphs of description and possibly a list of links. Each section is an object with the following properties:-
title
(required): The title of the section -
description
: The description of the section -
links
: The links of the section-
title
(required): The title of the link -
description
: The description of the link -
href
(required): The href of the link
-
-
-
notes
: The notes of the documentation. Notes are a special section which always appears at the end of the documentation. Notes are useful to add any information about the application or documentation itself. -
full
: Thellms-full.txt
configuration. Setting this option will enable thellms-full.txt
route.-
title
: The title of the llms-full documentation -
description
: The description of the llms-full documentation
-
The module generates two different documentation formats:
The /llms.txt
route generates a concise, structured documentation that follows the llms.txt specification. This format is optimized for both human readability and AI consumption. It includes:
- Application title and description
- Organized sections with titles and descriptions
- Links with titles, descriptions, and URLs
- Optional notes section
The /llms-full.txt
route provides a more detailed, free-form documentation format. This is useful to reduce crawler traffic on your application and provide a more detailed documentation to your users and LLMs.
By default module does not generate the llms-full.txt
route, you need to enable it by setting full.title
and full.description
in your nuxt.config.ts
.
export default defineNuxtConfig({
llms: {
domain: 'https://example.com',
title: 'My Application',
full: {
title: 'Full Documentation',
description: 'Full documentation of the application',
},
},
})
The module provides a hooks system that allows you to dynamically extend both documentation formats. There are two main hooks:
This hook is called for every request to /llms.txt
. Use this hook to modify the structured documentation, It allows you to add sections, links, and metadata.
Parameters:
-
event
: H3Event - The current request event -
options
: ModuleOptions - The module options that you can modify to add sections, links, etc.
This hook is called for every request to /llms-full.txt
. It allows you to add custom content sections in any format.
Parameters:
-
event
: H3Event - The current request event -
options
: ModuleOptions - The module options that you can modify to add sections, links, etc. -
contents
: string[] - Array of content sections you can add to or modify
Create a server plugin in your server/plugins
directory:
// server/plugins/llms.ts
export default defineNitroPlugin((nitroApp) => {
// Method 1: Using the hooks directly
nitroApp.hooks.hook('llms:generate', (event, options) => {
// Add a new section to llms.txt
options.sections.push({
title: 'API Documentation',
description: 'REST API endpoints and usage',
links: [
{
title: 'Authentication',
description: 'API authentication methods',
href: `${options.domain}/api/auth`
}
]
})
})
// Method 2: Using the helper function
nitroApp.hooks.hook('llms:generate:full', (event, options, contents) => {
// Add detailed documentation to llms-full.txt
contents.push(`## API Authentication
### Bearer Token
To authenticate API requests, include a Bearer token in the Authorization header:
\`\`\`
Authorization: Bearer <your-token>
\`\`\`
### API Keys
For server-to-server communication, use API keys:
\`\`\`
X-API-Key: <your-api-key>
\`\`\`
`)
})
})
If you're developing a Nuxt module that needs to extend the LLMs documentation:
- Create a server plugin in your module:
// module/runtime/server/plugins/my-module-llms.ts
export default defineNitroPlugin((nitroApp) => {
nitroApp.hooks.hook('llms:generate', (event, options) => {
options.sections.push({
title: 'My Module',
description: 'Documentation for my module features',
links: [/* ... */]
})
})
})
- Register the plugin in your module setup:
import { defineNuxtModule, addServerPlugin } from '@nuxt/kit'
import { fileURLToPath } from 'url'
export default defineNuxtModule({
setup(options, nuxt) {
const runtimeDir = fileURLToPath(new URL('./runtime', import.meta.url))
addServerPlugin(resolve(runtimeDir, 'server/plugins/my-module-llms'))
}
})
Nuxt Content ^3.2.0 comes with built-in support for LLMs documentation. You can use nuxt-llms
with @nuxt/content
to efficiently write content and documentation for your website and generate LLM-friendly documentation without extra effort. Content module uses nuxt-llms
hooks and automatically adds all your contents into llms.txt
and llms-full.txt
documentation.
All you need is to install both modules and write your content files in the content
directory.
export default defineNuxtConfig({
modules: ['nuxt-llms', '@nuxt/content'],
llms: {
domain: 'https://example.com',
title: 'My Application',
description: 'My Application Description',
},
})
Checkout the Nuxt Content documentation for more information on how to write your content files.
And checkout the Nuxt Content llms documentation for more information on how to customize LLMs contents with nuxt-llms
and @nuxt/content
.
- Clone repository
- Install dependencies using
pnpm install
- Prepare using
pnpm dev:prepare
- Build using
pnpm prepack
- Try playground using
pnpm dev
- Test using
pnpm test
Copyright (c) NuxtLabs
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for nuxt-llms
Similar Open Source Tools
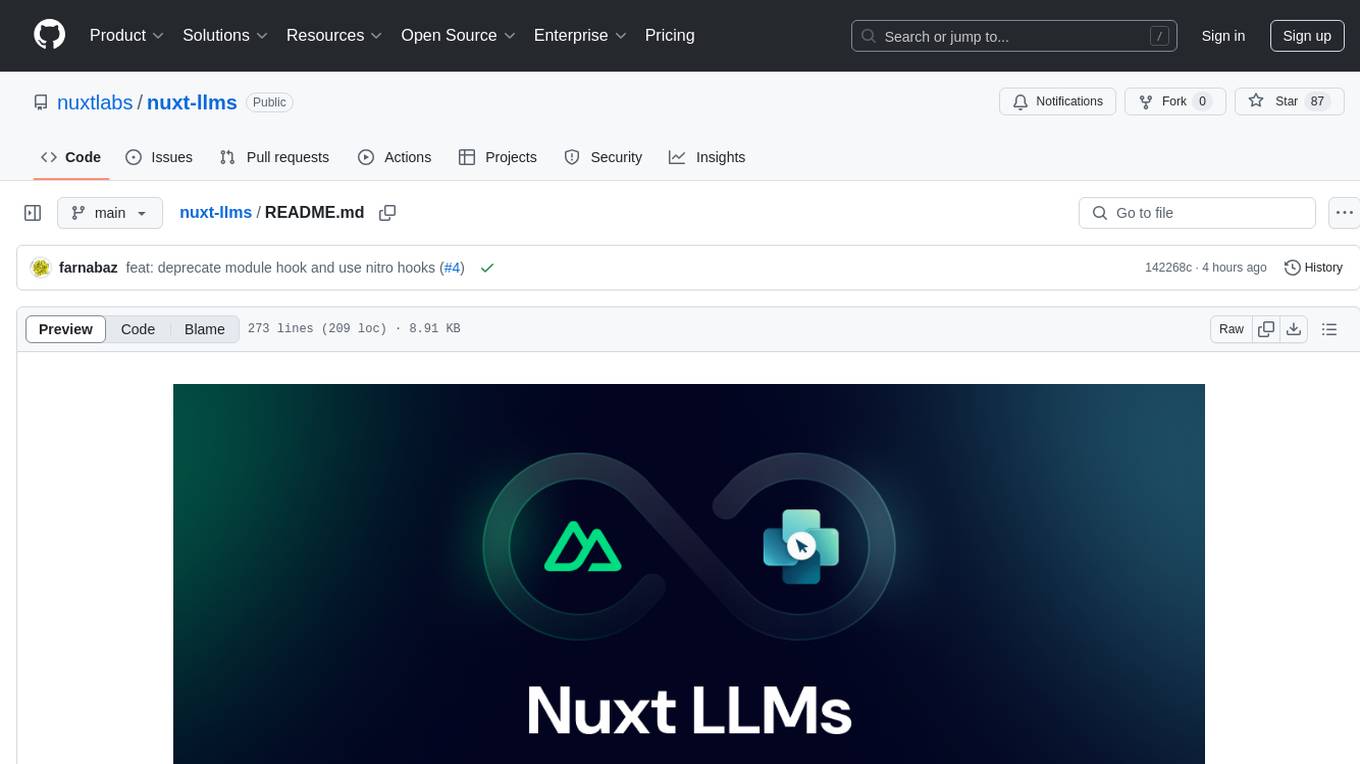
nuxt-llms
Nuxt LLMs automatically generates llms.txt markdown documentation for Nuxt applications. It provides runtime hooks to collect data from various sources and generate structured documentation. The tool allows customization of sections directly from nuxt.config.ts and integrates with Nuxt modules via the runtime hooks system. It generates two documentation formats: llms.txt for concise structured documentation and llms_full.txt for detailed documentation. Users can extend documentation using hooks to add sections, links, and metadata. The tool is suitable for developers looking to automate documentation generation for their Nuxt applications.
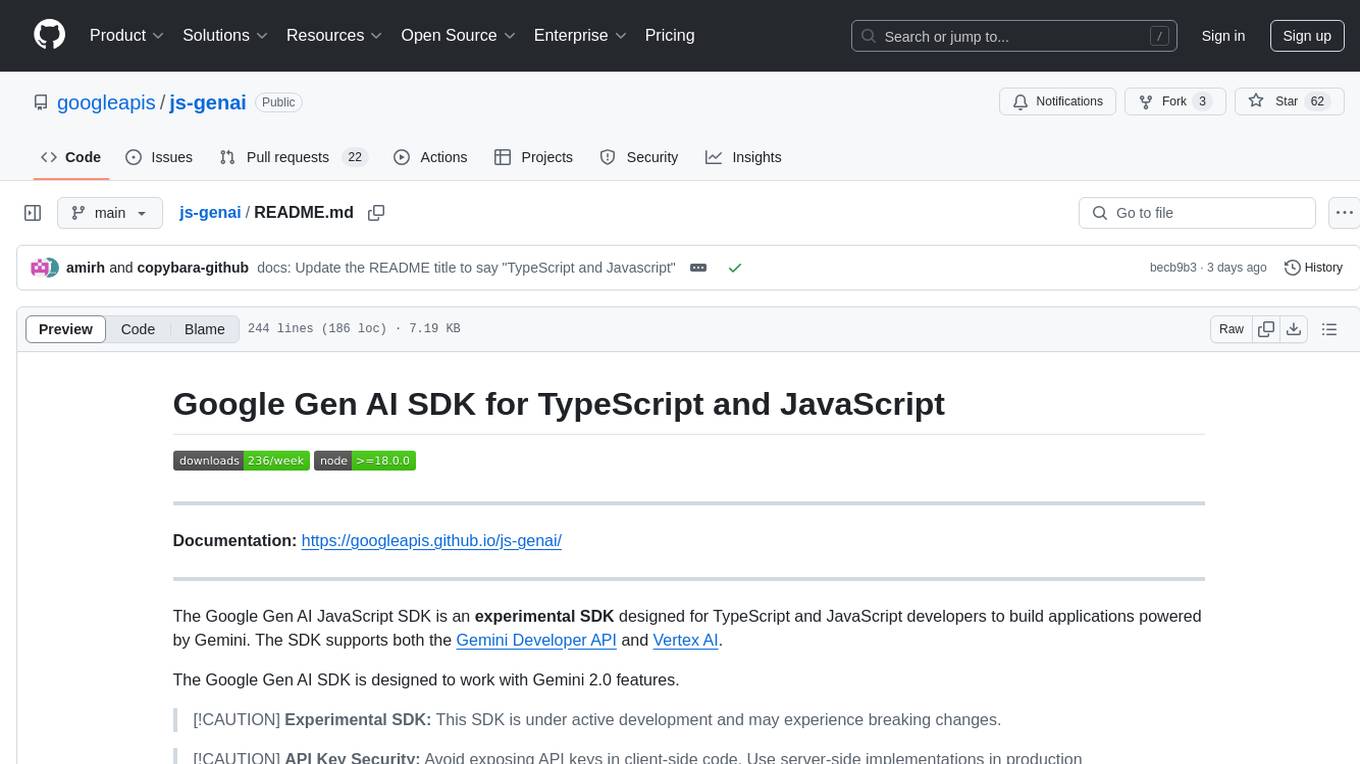
js-genai
The Google Gen AI JavaScript SDK is an experimental SDK for TypeScript and JavaScript developers to build applications powered by Gemini. It supports both the Gemini Developer API and Vertex AI. The SDK is designed to work with Gemini 2.0 features. Users can access API features through the GoogleGenAI classes, which provide submodules for querying models, managing caches, creating chats, uploading files, and starting live sessions. The SDK also allows for function calling to interact with external systems. Users can find more samples in the GitHub samples directory.
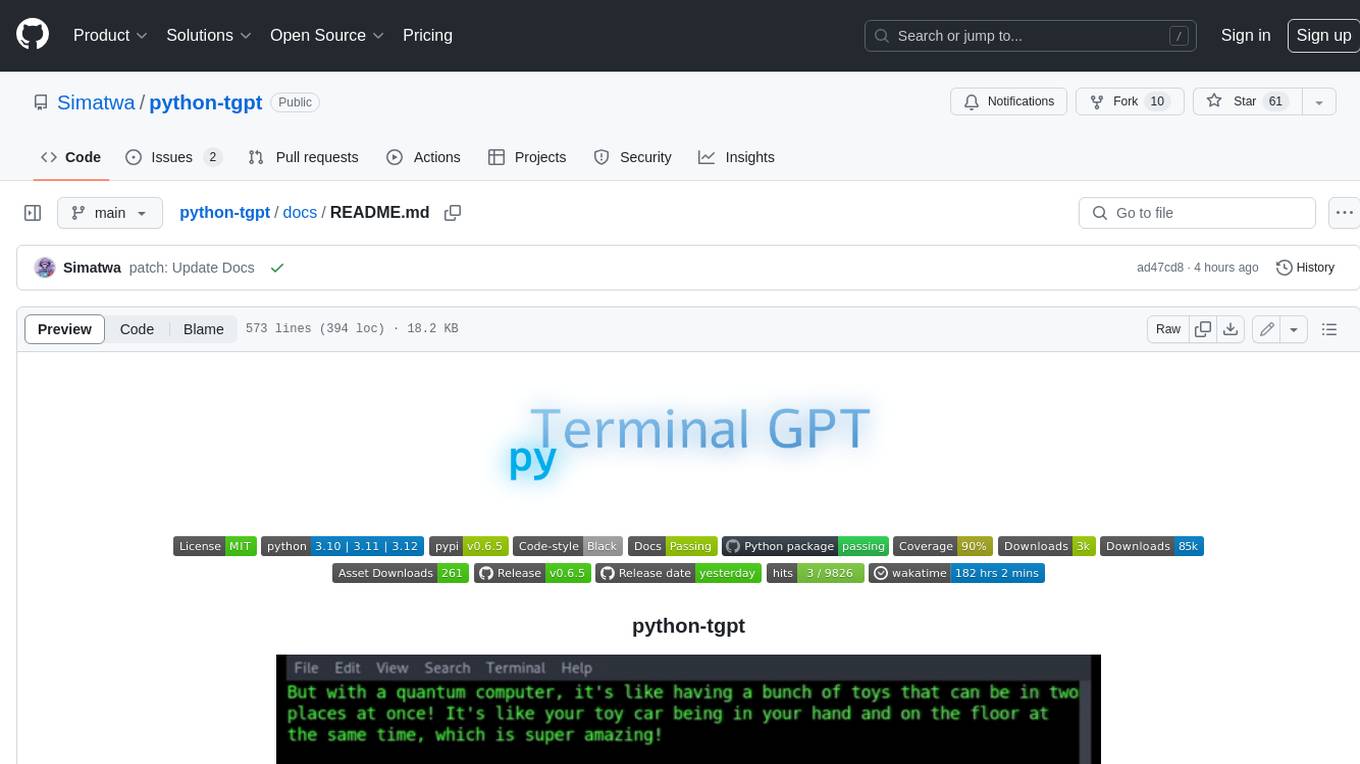
python-tgpt
Python-tgpt is a Python package that enables seamless interaction with over 45 free LLM providers without requiring an API key. It also provides image generation capabilities. The name _python-tgpt_ draws inspiration from its parent project tgpt, which operates on Golang. Through this Python adaptation, users can effortlessly engage with a number of free LLMs available, fostering a smoother AI interaction experience.
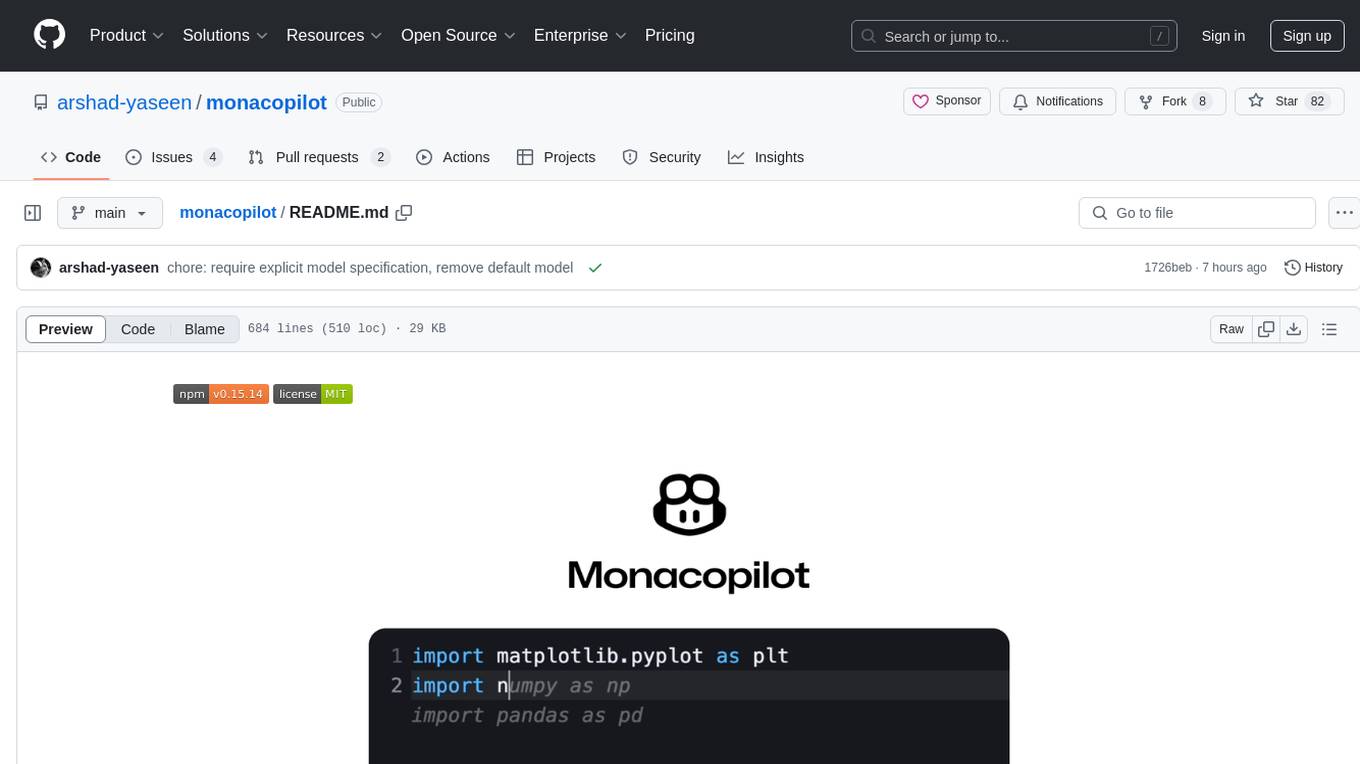
monacopilot
Monacopilot is a powerful and customizable AI auto-completion plugin for the Monaco Editor. It supports multiple AI providers such as Anthropic, OpenAI, Groq, and Google, providing real-time code completions with an efficient caching system. The plugin offers context-aware suggestions, customizable completion behavior, and framework agnostic features. Users can also customize the model support and trigger completions manually. Monacopilot is designed to enhance coding productivity by providing accurate and contextually appropriate completions in daily spoken language.
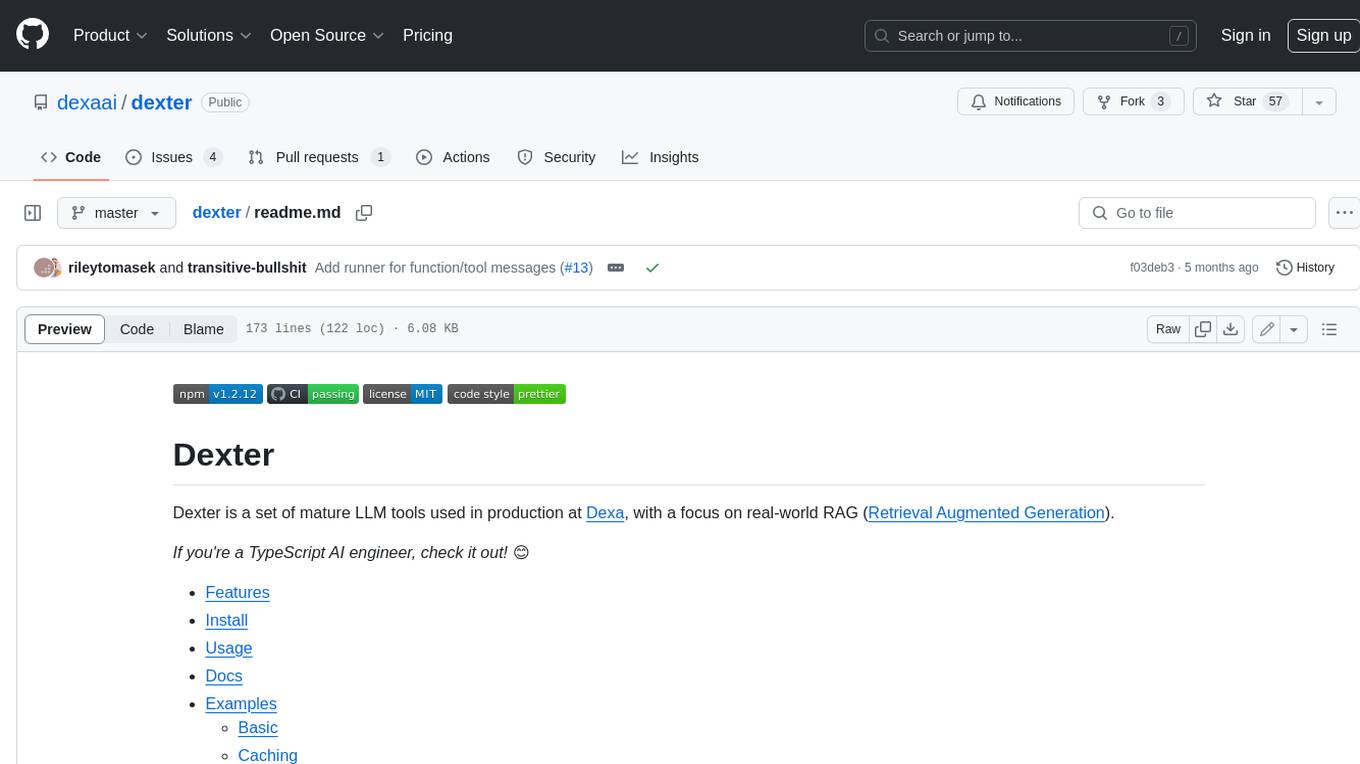
dexter
Dexter is a set of mature LLM tools used in production at Dexa, with a focus on real-world RAG (Retrieval Augmented Generation). It is a production-quality RAG that is extremely fast and minimal, and handles caching, throttling, and batching for ingesting large datasets. It also supports optional hybrid search with SPLADE embeddings, and is a minimal TS package with full typing that uses `fetch` everywhere and supports Node.js 18+, Deno, Cloudflare Workers, Vercel edge functions, etc. Dexter has full docs and includes examples for basic usage, caching, Redis caching, AI function, AI runner, and chatbot.
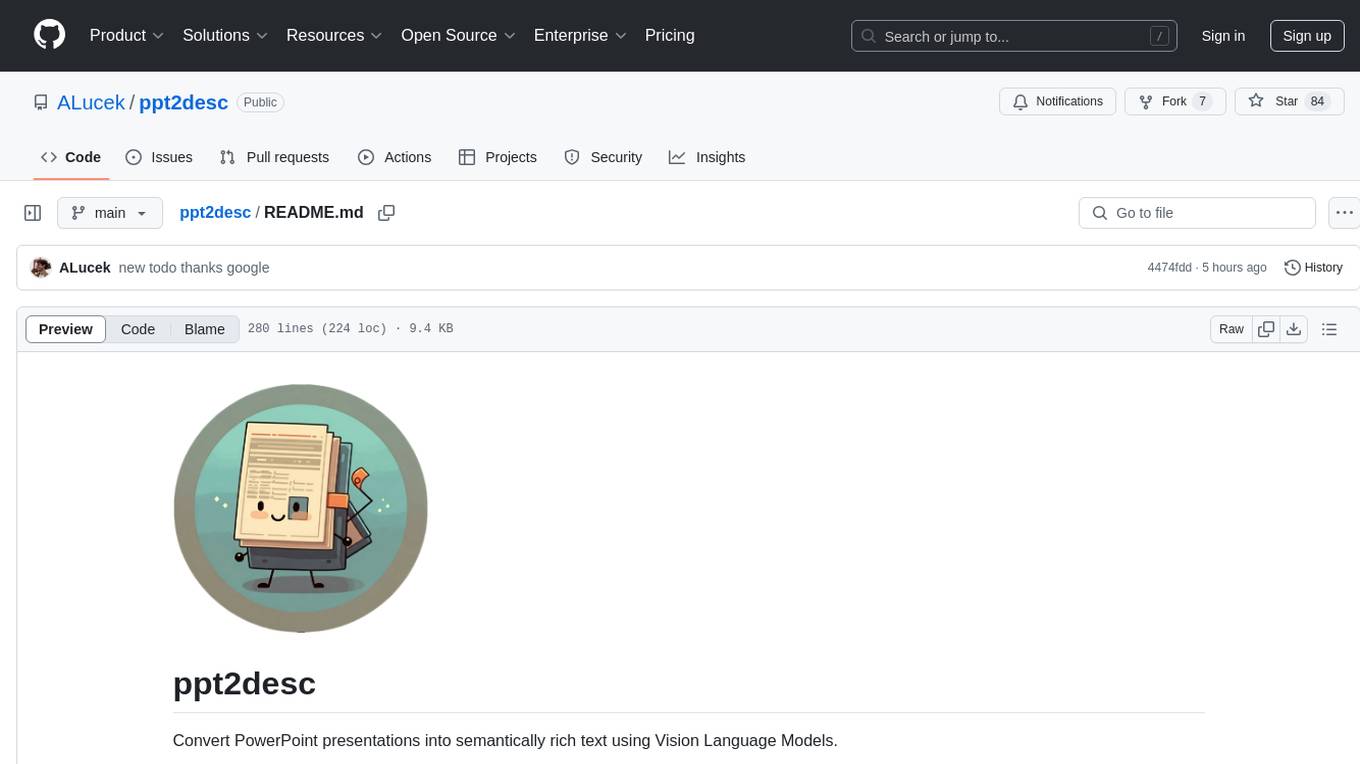
ppt2desc
ppt2desc is a command-line tool that converts PowerPoint presentations into detailed textual descriptions using vision language models. It interprets and describes visual elements, capturing the full semantic meaning of each slide in a machine-readable format. The tool supports various model providers and offers features like converting PPT/PPTX files to semantic descriptions, processing individual files or directories, visual elements interpretation, rate limiting for API calls, customizable prompts, and JSON output format for easy integration.
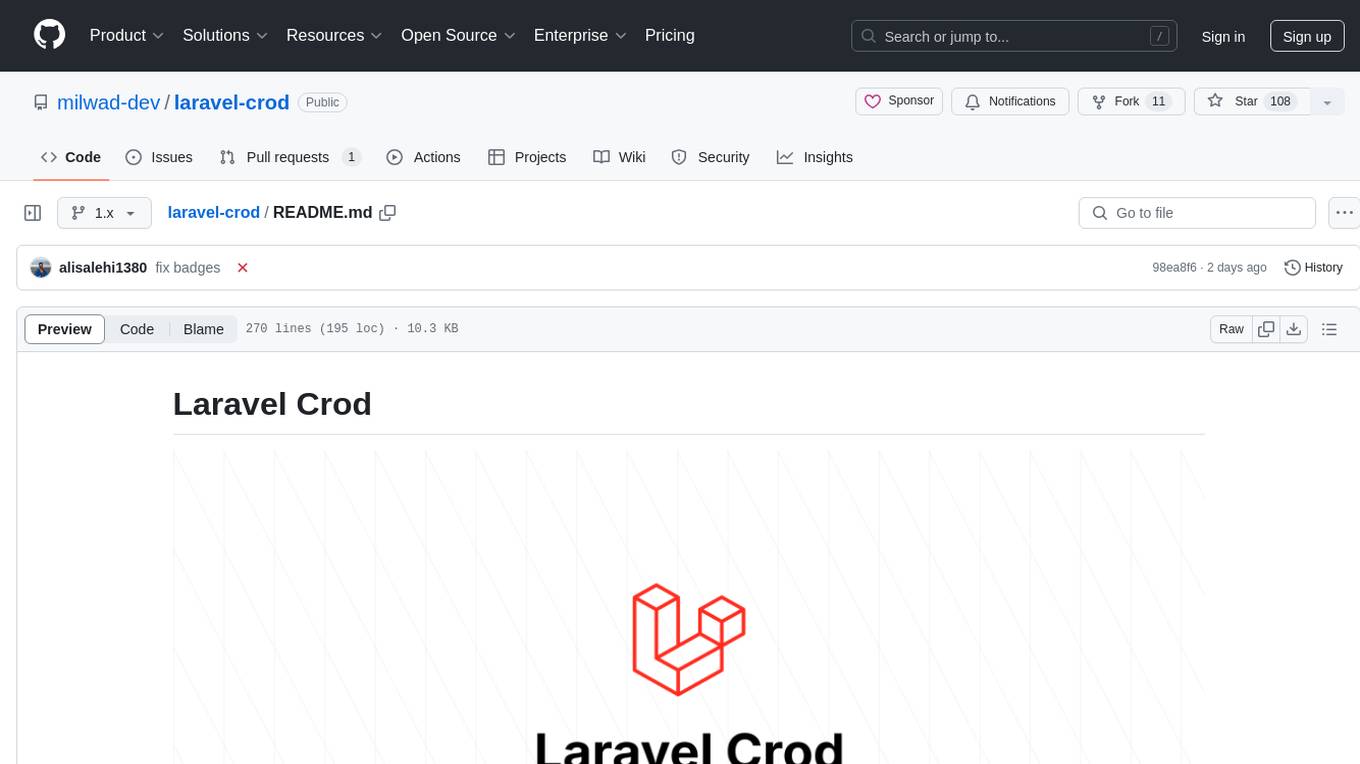
laravel-crod
Laravel Crod is a package designed to facilitate the implementation of CRUD operations in Laravel projects. It allows users to quickly generate controllers, models, migrations, services, repositories, views, and requests with various customization options. The package simplifies tasks such as creating resource controllers, making models fillable, querying repositories and services, and generating additional files like seeders and factories. Laravel Crod aims to streamline the process of building CRUD functionalities in Laravel applications by providing a set of commands and tools for developers.
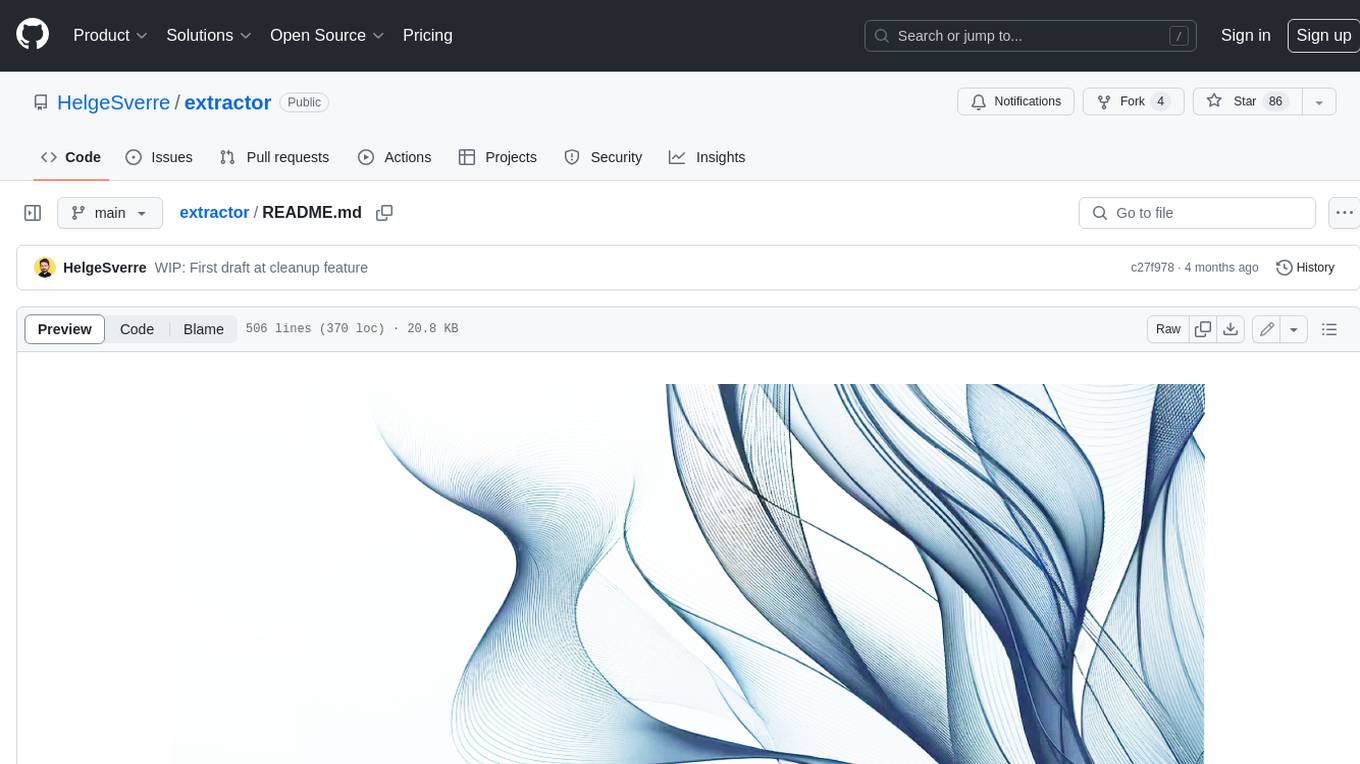
extractor
Extractor is an AI-powered data extraction library for Laravel that leverages OpenAI's capabilities to effortlessly extract structured data from various sources, including images, PDFs, and emails. It features a convenient wrapper around OpenAI Chat and Completion endpoints, supports multiple input formats, includes a flexible Field Extractor for arbitrary data extraction, and integrates with Textract for OCR functionality. Extractor utilizes JSON Mode from the latest GPT-3.5 and GPT-4 models, providing accurate and efficient data extraction.
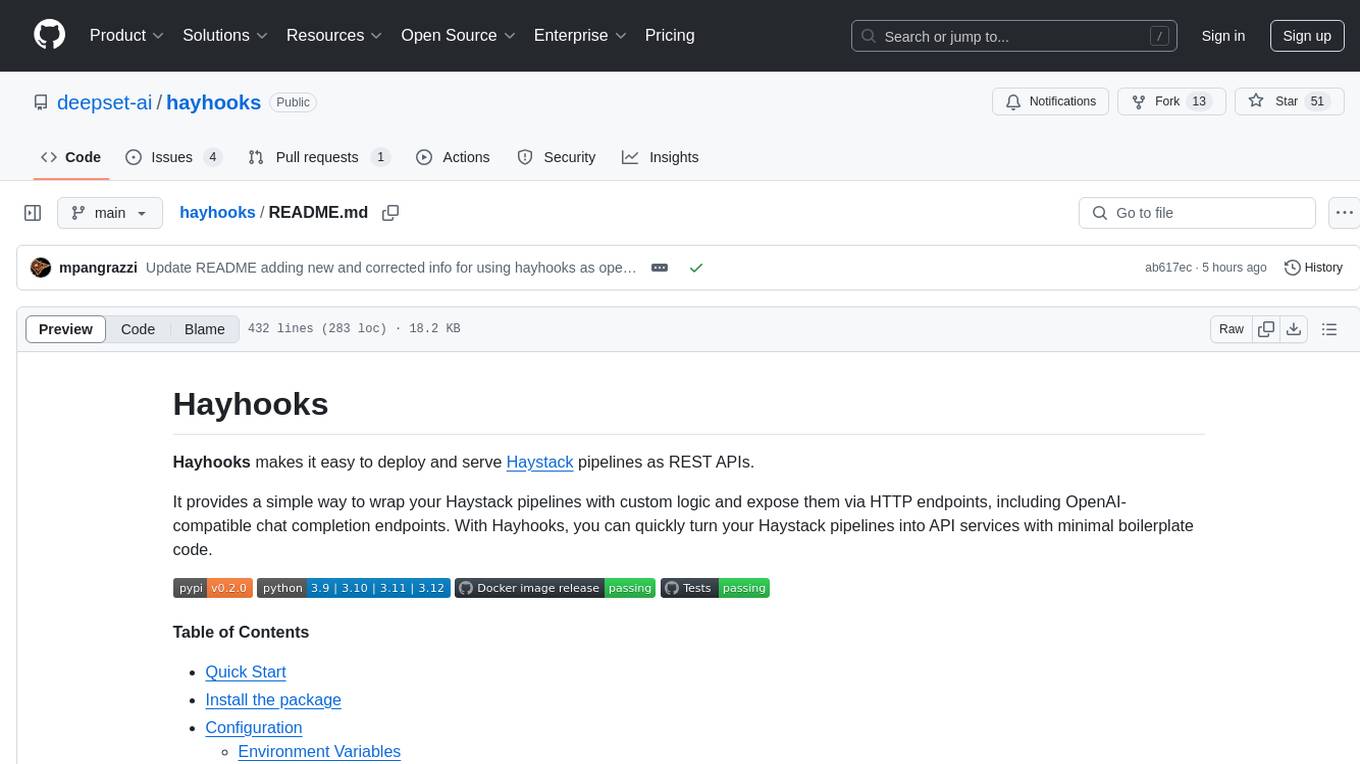
hayhooks
Hayhooks is a tool that simplifies the deployment and serving of Haystack pipelines as REST APIs. It allows users to wrap their pipelines with custom logic and expose them via HTTP endpoints, including OpenAI-compatible chat completion endpoints. With Hayhooks, users can easily convert their Haystack pipelines into API services with minimal boilerplate code.
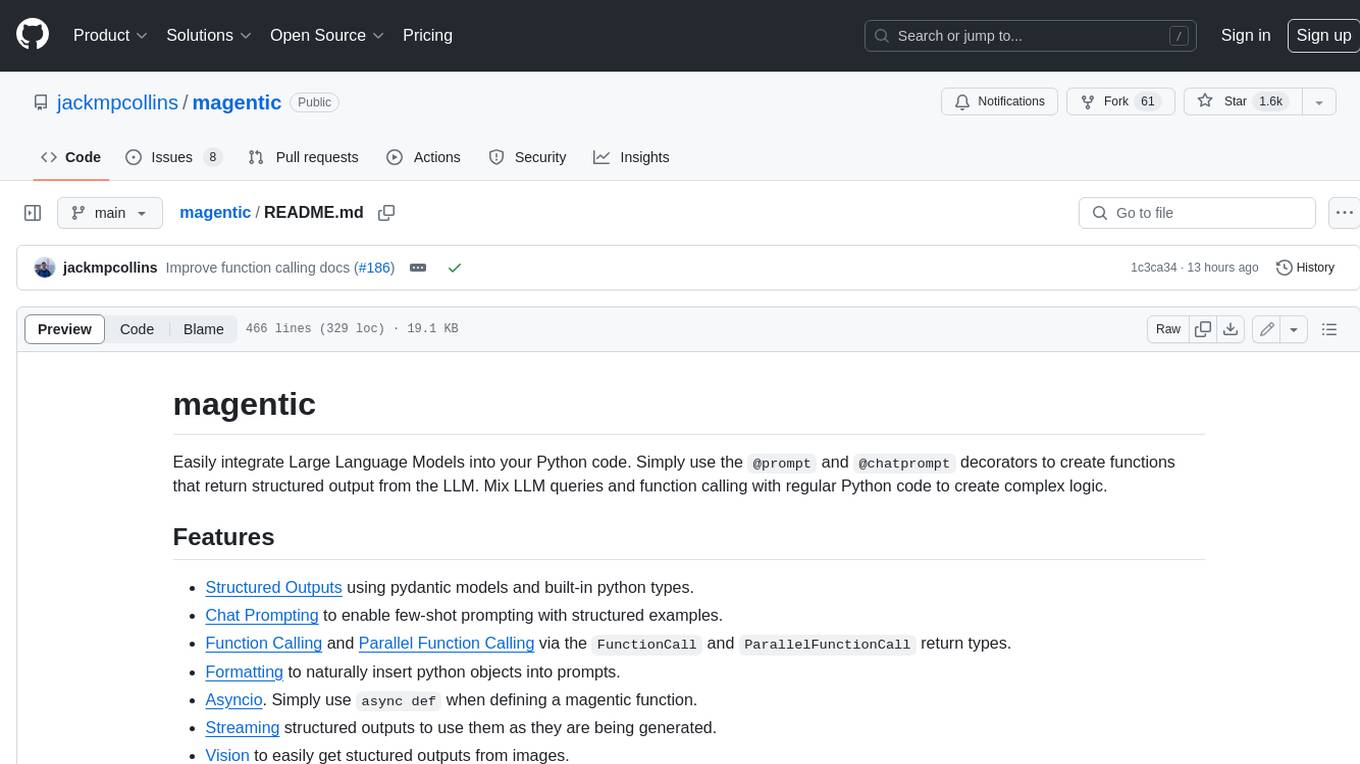
magentic
Easily integrate Large Language Models into your Python code. Simply use the `@prompt` and `@chatprompt` decorators to create functions that return structured output from the LLM. Mix LLM queries and function calling with regular Python code to create complex logic.
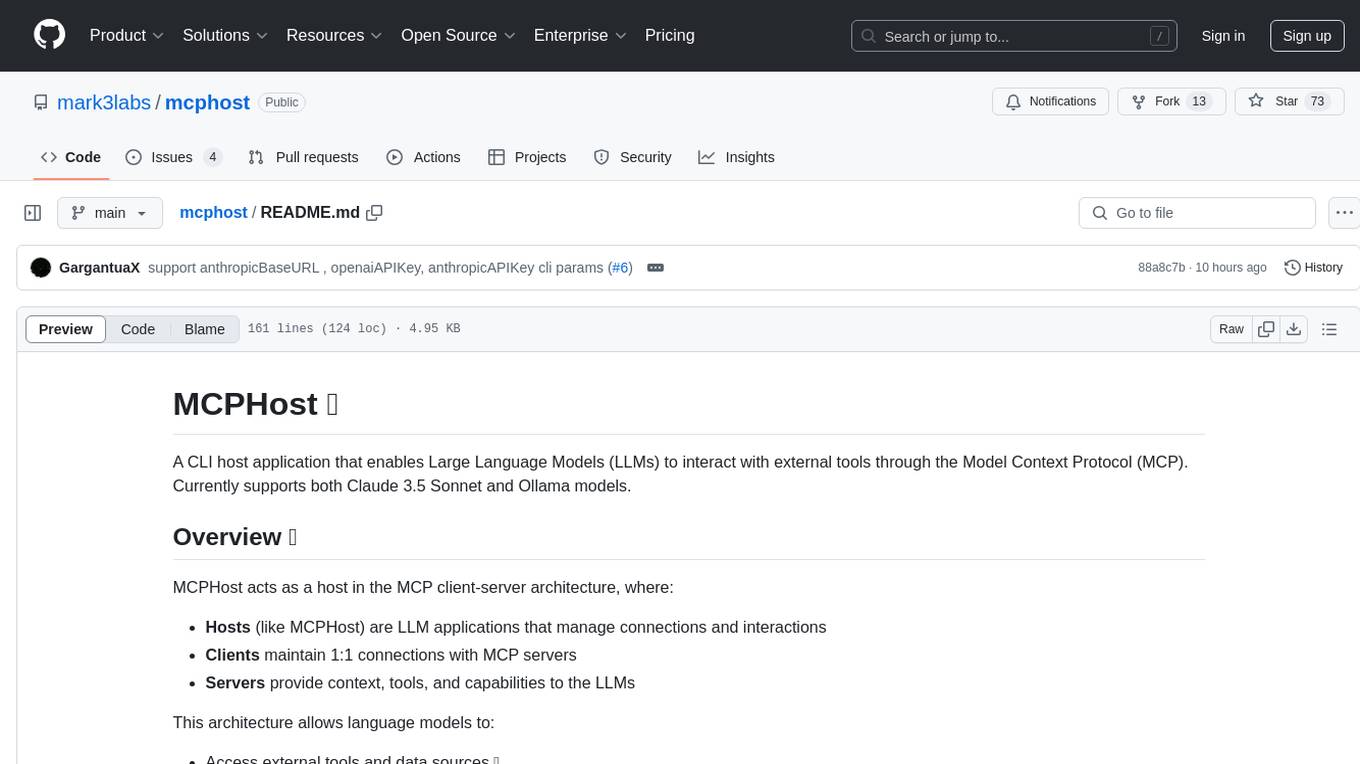
mcphost
MCPHost is a CLI host application that enables Large Language Models (LLMs) to interact with external tools through the Model Context Protocol (MCP). It acts as a host in the MCP client-server architecture, allowing language models to access external tools and data sources, maintain consistent context across interactions, and execute commands safely. The tool supports interactive conversations with Claude 3.5 Sonnet and Ollama models, multiple concurrent MCP servers, dynamic tool discovery and integration, configurable server locations and arguments, and a consistent command interface across model types.
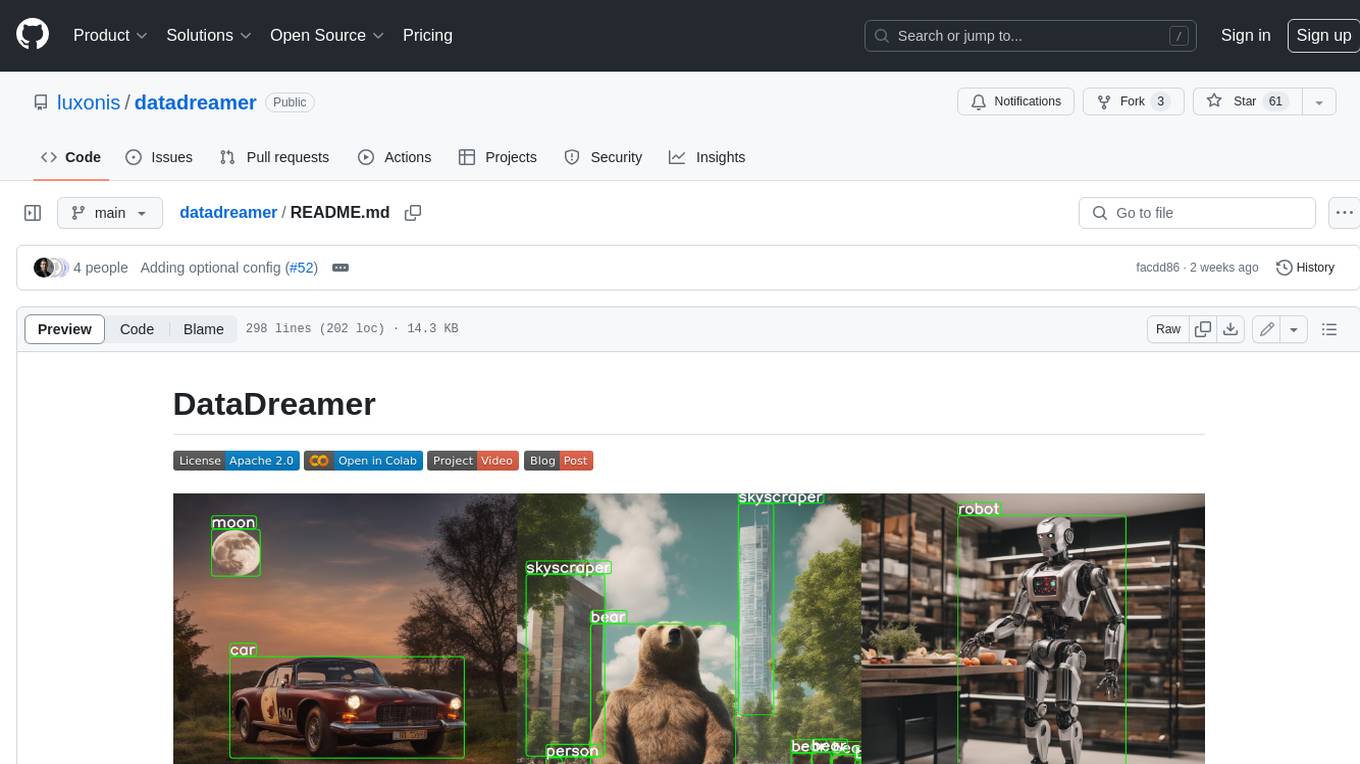
datadreamer
DataDreamer is an advanced toolkit designed to facilitate the development of edge AI models by enabling synthetic data generation, knowledge extraction from pre-trained models, and creation of efficient and potent models. It eliminates the need for extensive datasets by generating synthetic datasets, leverages latent knowledge from pre-trained models, and focuses on creating compact models suitable for integration into any device and performance for specialized tasks. The toolkit offers features like prompt generation, image generation, dataset annotation, and tools for training small-scale neural networks for edge deployment. It provides hardware requirements, usage instructions, available models, and limitations to consider while using the library.
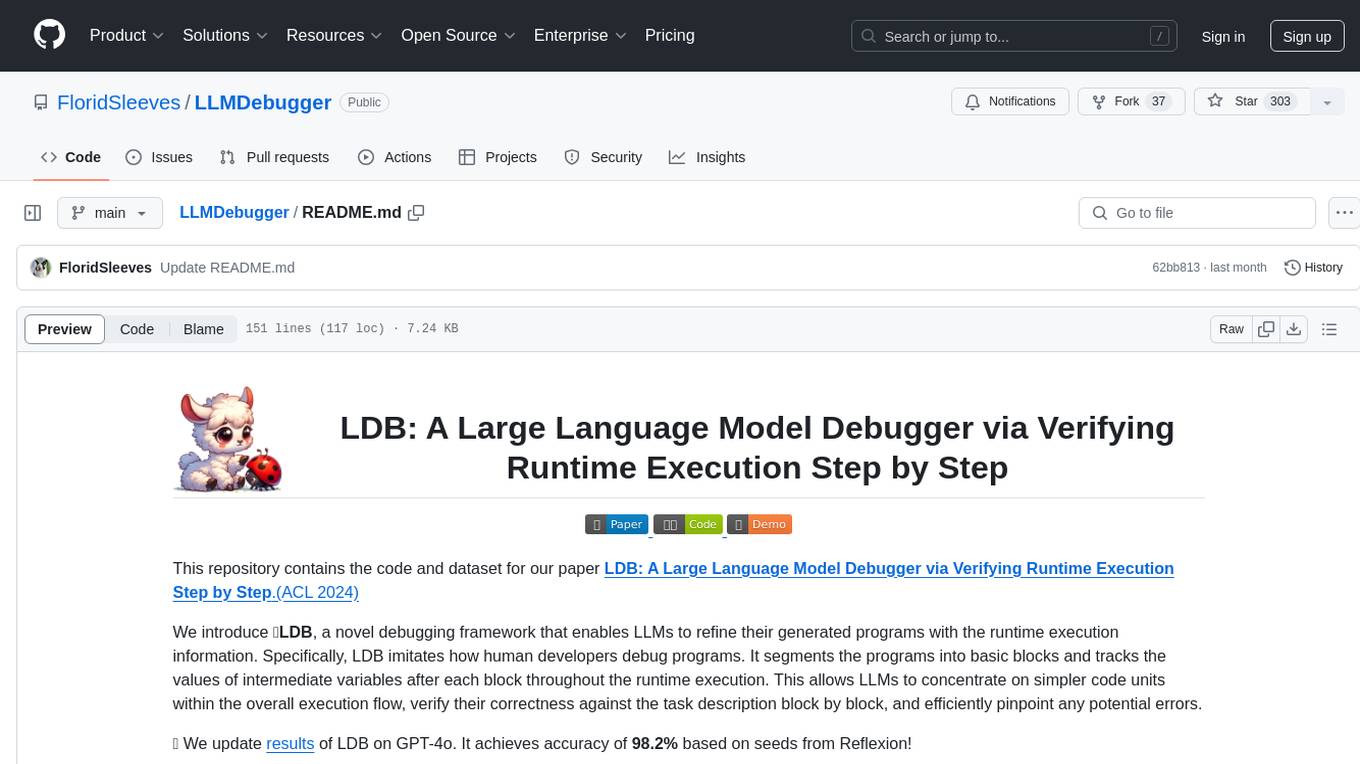
LLMDebugger
This repository contains the code and dataset for LDB, a novel debugging framework that enables Large Language Models (LLMs) to refine their generated programs by tracking the values of intermediate variables throughout the runtime execution. LDB segments programs into basic blocks, allowing LLMs to concentrate on simpler code units, verify correctness block by block, and pinpoint errors efficiently. The tool provides APIs for debugging and generating code with debugging messages, mimicking how human developers debug programs.
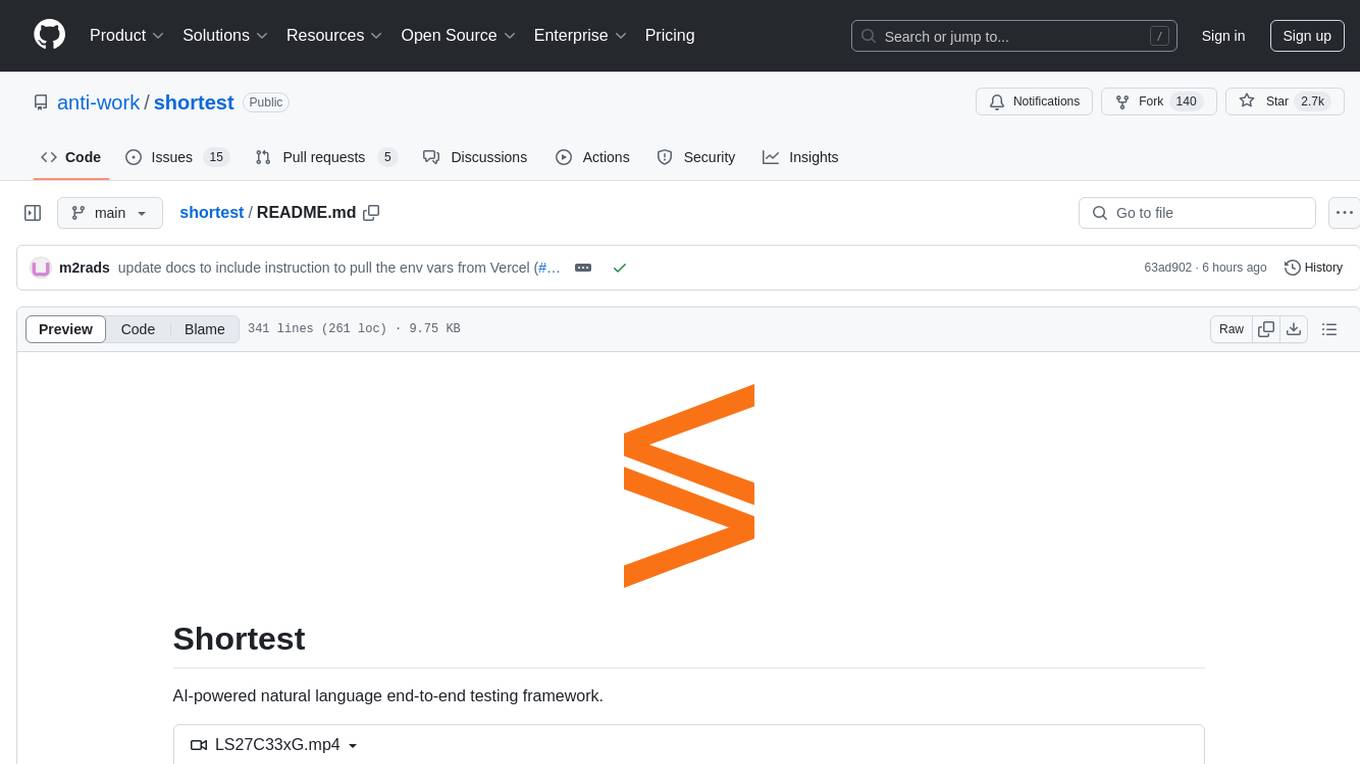
shortest
Shortest is an AI-powered natural language end-to-end testing framework built on Playwright. It provides a seamless testing experience by allowing users to write tests in natural language and execute them using Anthropic Claude API. The framework also offers GitHub integration with 2FA support, making it suitable for testing web applications with complex authentication flows. Shortest simplifies the testing process by enabling users to run tests locally or in CI/CD pipelines, ensuring the reliability and efficiency of web applications.
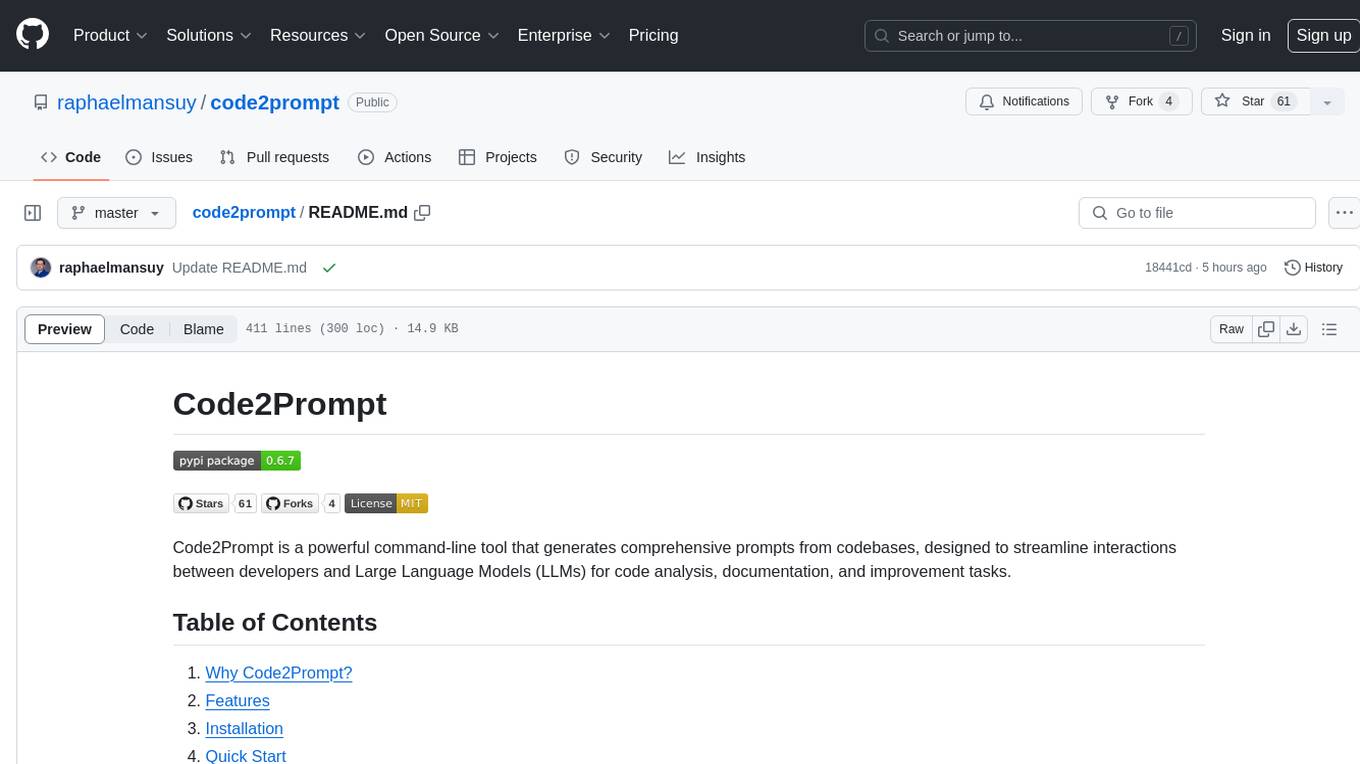
code2prompt
Code2Prompt is a powerful command-line tool that generates comprehensive prompts from codebases, designed to streamline interactions between developers and Large Language Models (LLMs) for code analysis, documentation, and improvement tasks. It bridges the gap between codebases and LLMs by converting projects into AI-friendly prompts, enabling users to leverage AI for various software development tasks. The tool offers features like holistic codebase representation, intelligent source tree generation, customizable prompt templates, smart token management, Gitignore integration, flexible file handling, clipboard-ready output, multiple output options, and enhanced code readability.
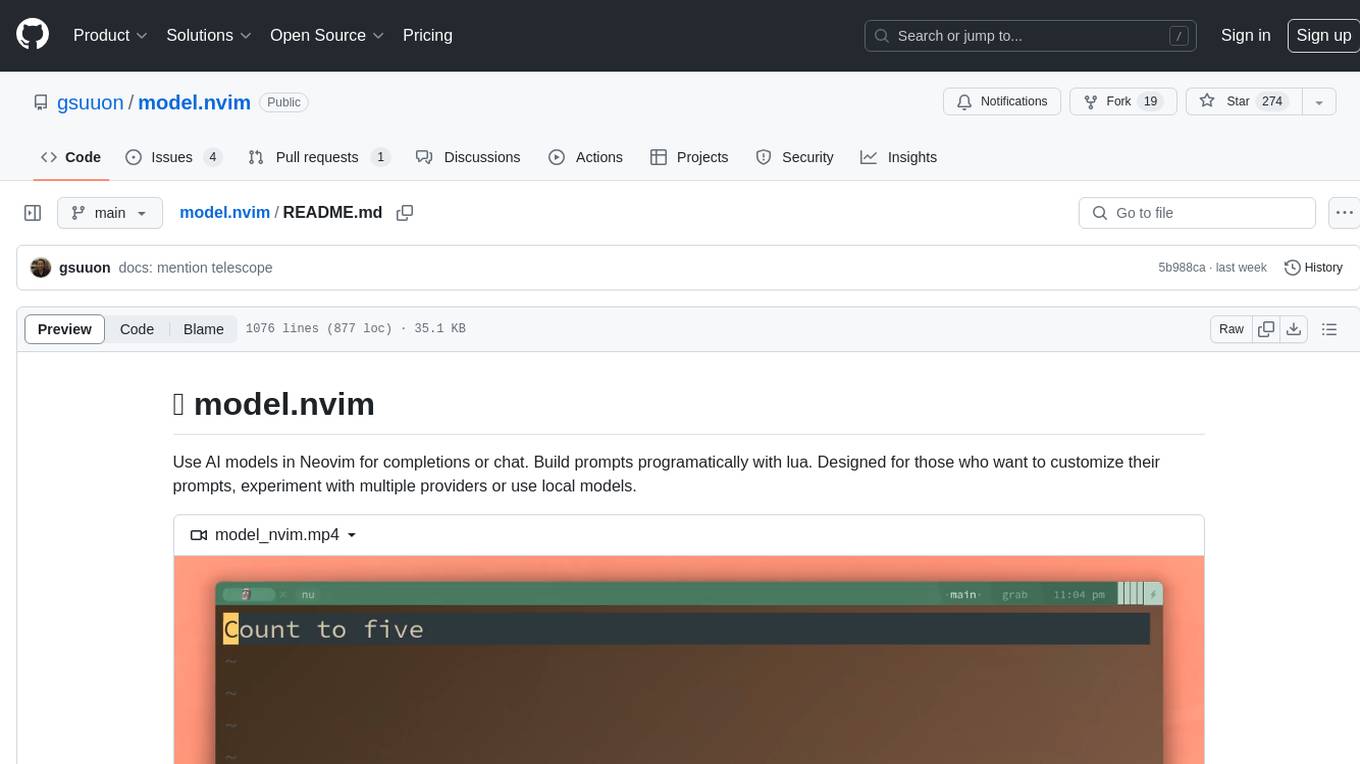
model.nvim
model.nvim is a tool designed for Neovim users who want to utilize AI models for completions or chat within their text editor. It allows users to build prompts programmatically with Lua, customize prompts, experiment with multiple providers, and use both hosted and local models. The tool supports features like provider agnosticism, programmatic prompts in Lua, async and multistep prompts, streaming completions, and chat functionality in 'mchat' filetype buffer. Users can customize prompts, manage responses, and context, and utilize various providers like OpenAI ChatGPT, Google PaLM, llama.cpp, ollama, and more. The tool also supports treesitter highlights and folds for chat buffers.
For similar tasks
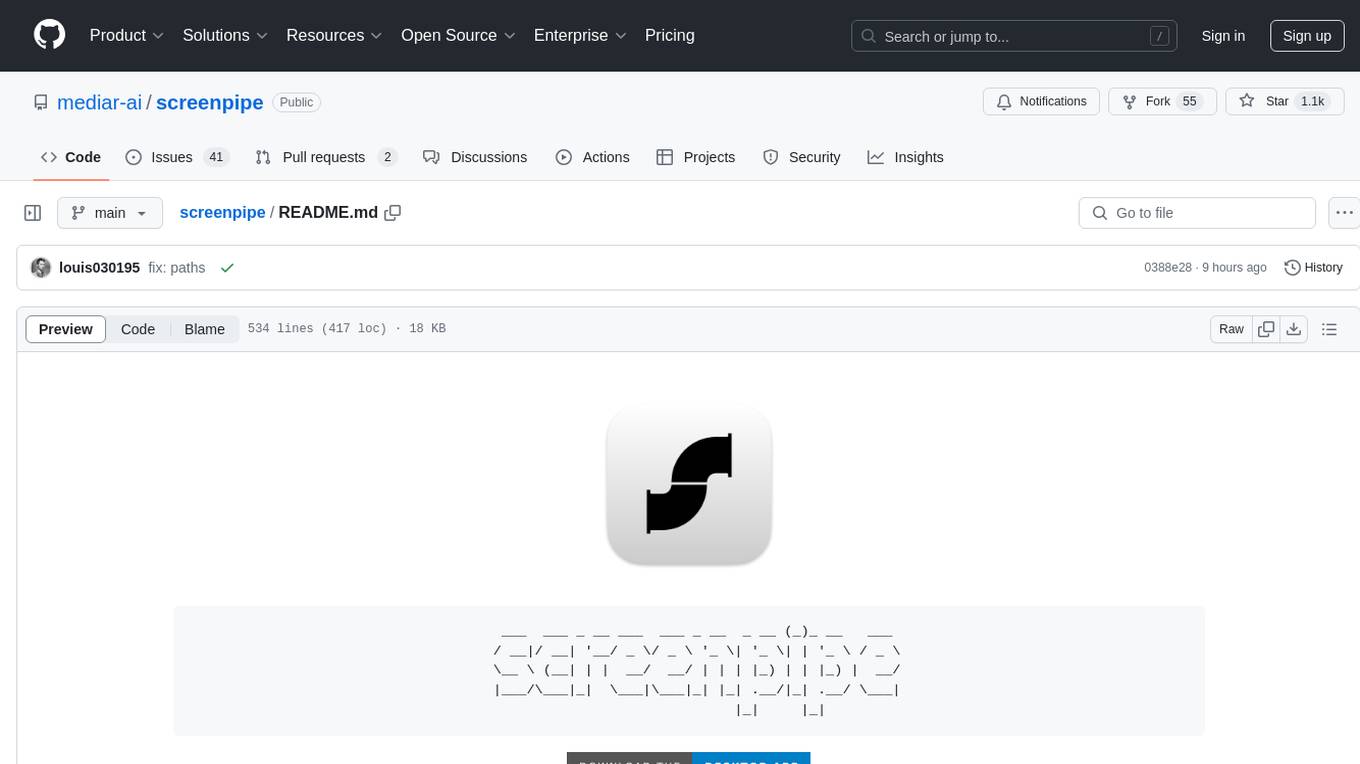
screenpipe
24/7 Screen & Audio Capture Library to build personalized AI powered by what you've seen, said, or heard. Works with Ollama. Alternative to Rewind.ai. Open. Secure. You own your data. Rust. We are shipping daily, make suggestions, post bugs, give feedback. Building a reliable stream of audio and screenshot data, simplifying life for developers by solving non-trivial problems. Multiple installation options available. Experimental tool with various integrations and features for screen and audio capture, OCR, STT, and more. Open source project focused on enabling tooling & infrastructure for a wide range of applications.
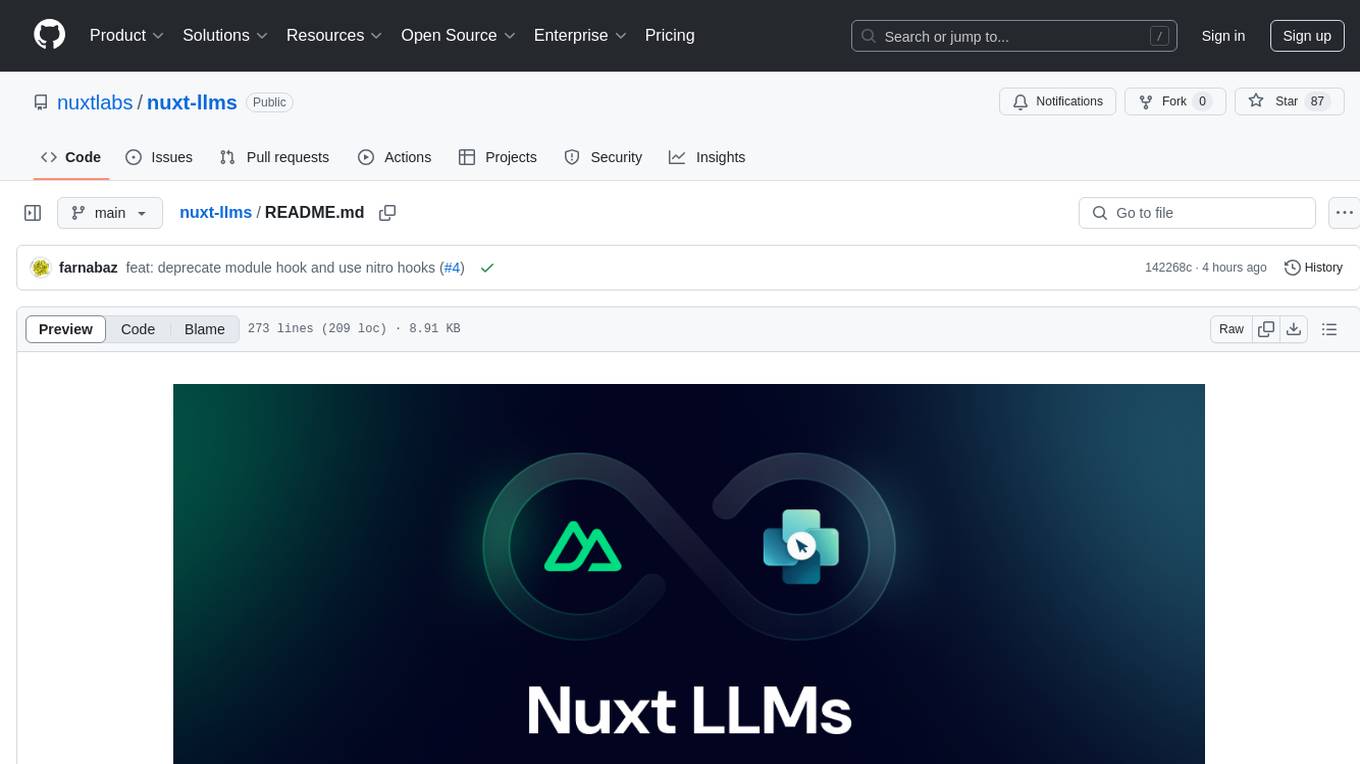
nuxt-llms
Nuxt LLMs automatically generates llms.txt markdown documentation for Nuxt applications. It provides runtime hooks to collect data from various sources and generate structured documentation. The tool allows customization of sections directly from nuxt.config.ts and integrates with Nuxt modules via the runtime hooks system. It generates two documentation formats: llms.txt for concise structured documentation and llms_full.txt for detailed documentation. Users can extend documentation using hooks to add sections, links, and metadata. The tool is suitable for developers looking to automate documentation generation for their Nuxt applications.
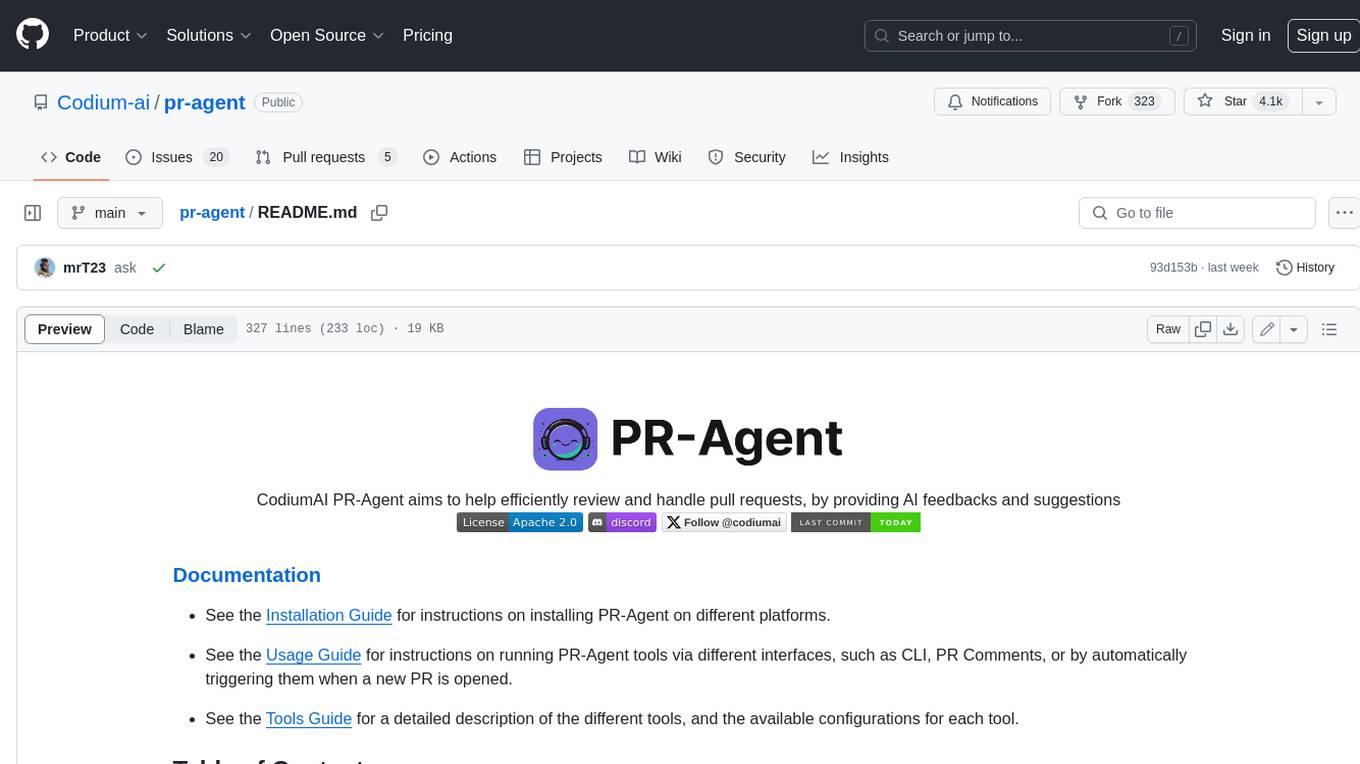
pr-agent
PR-Agent is a tool that helps to efficiently review and handle pull requests by providing AI feedbacks and suggestions. It supports various commands such as generating PR descriptions, providing code suggestions, answering questions about the PR, and updating the CHANGELOG.md file. PR-Agent can be used via CLI, GitHub Action, GitHub App, Docker, and supports multiple git providers and models. It emphasizes real-life practical usage, with each tool having a single GPT-4 call for quick and affordable responses. The PR Compression strategy enables effective handling of both short and long PRs, while the JSON prompting strategy allows for modular and customizable tools. PR-Agent Pro, the hosted version by CodiumAI, provides additional benefits such as full management, improved privacy, priority support, and extra features.
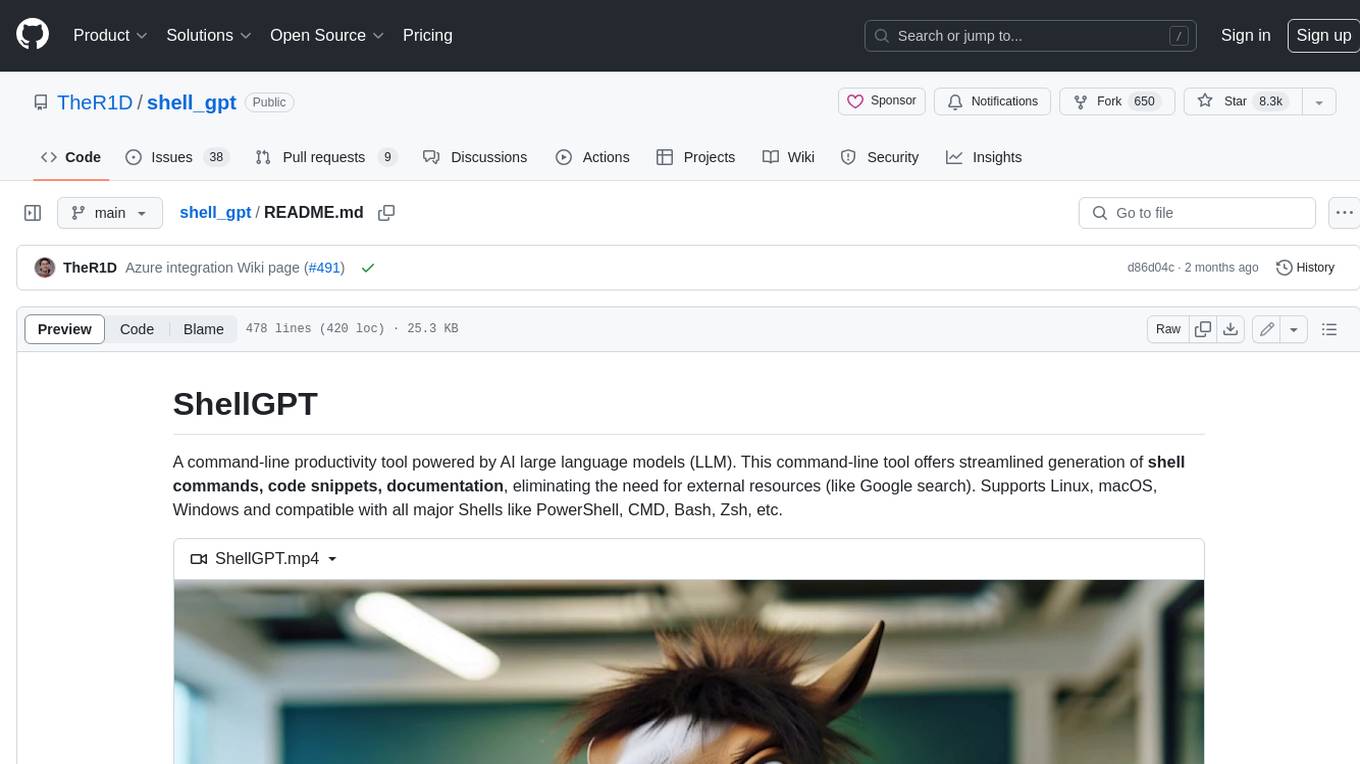
shell_gpt
ShellGPT is a command-line productivity tool powered by AI large language models (LLMs). This command-line tool offers streamlined generation of shell commands, code snippets, documentation, eliminating the need for external resources (like Google search). Supports Linux, macOS, Windows and compatible with all major Shells like PowerShell, CMD, Bash, Zsh, etc.
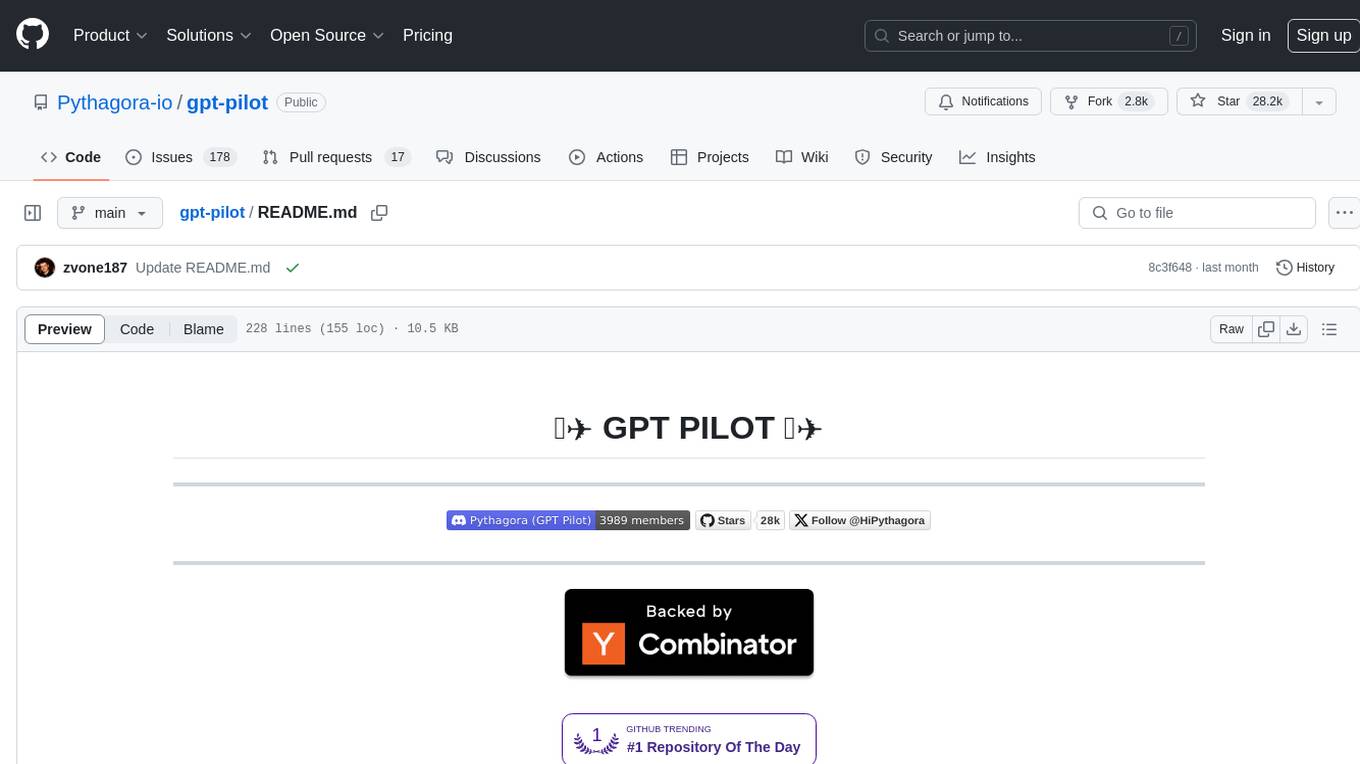
gpt-pilot
GPT Pilot is a core technology for the Pythagora VS Code extension, aiming to provide the first real AI developer companion. It goes beyond autocomplete, helping with writing full features, debugging, issue discussions, and reviews. The tool utilizes LLMs to generate production-ready apps, with developers overseeing the implementation. GPT Pilot works step by step like a developer, debugging issues as they arise. It can work at any scale, filtering out code to show only relevant parts to the AI during tasks. Contributions are welcome, with debugging and telemetry being key areas of focus for improvement.
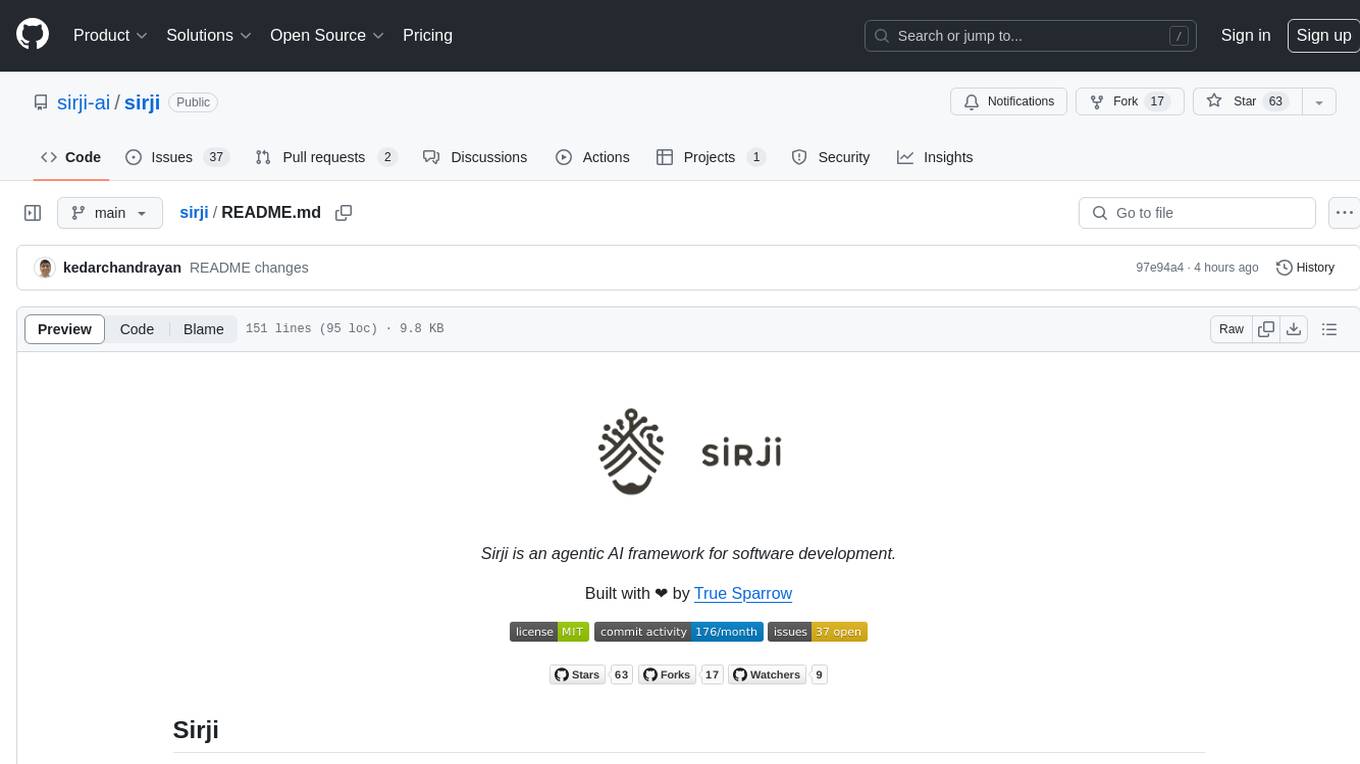
sirji
Sirji is an agentic AI framework for software development where various AI agents collaborate via a messaging protocol to solve software problems. It uses standard or user-generated recipes to list tasks and tips for problem-solving. Agents in Sirji are modular AI components that perform specific tasks based on custom pseudo code. The framework is currently implemented as a Visual Studio Code extension, providing an interactive chat interface for problem submission and feedback. Sirji sets up local or remote development environments by installing dependencies and executing generated code.
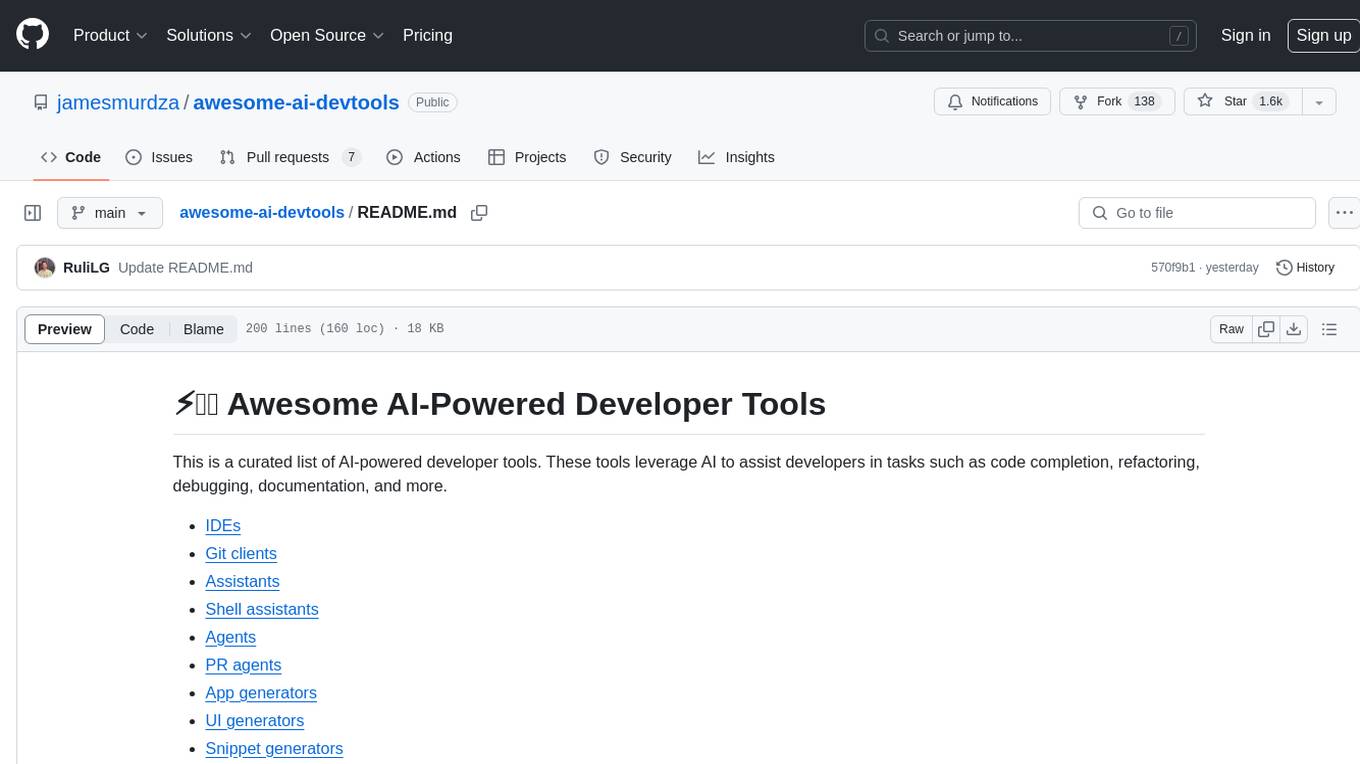
awesome-ai-devtools
Awesome AI-Powered Developer Tools is a curated list of AI-powered developer tools that leverage AI to assist developers in tasks such as code completion, refactoring, debugging, documentation, and more. The repository includes a wide range of tools, from IDEs and Git clients to assistants, agents, app generators, UI generators, snippet generators, documentation tools, code generation tools, agent platforms, OpenAI plugins, search tools, and testing tools. These tools are designed to enhance developer productivity and streamline various development tasks by integrating AI capabilities.
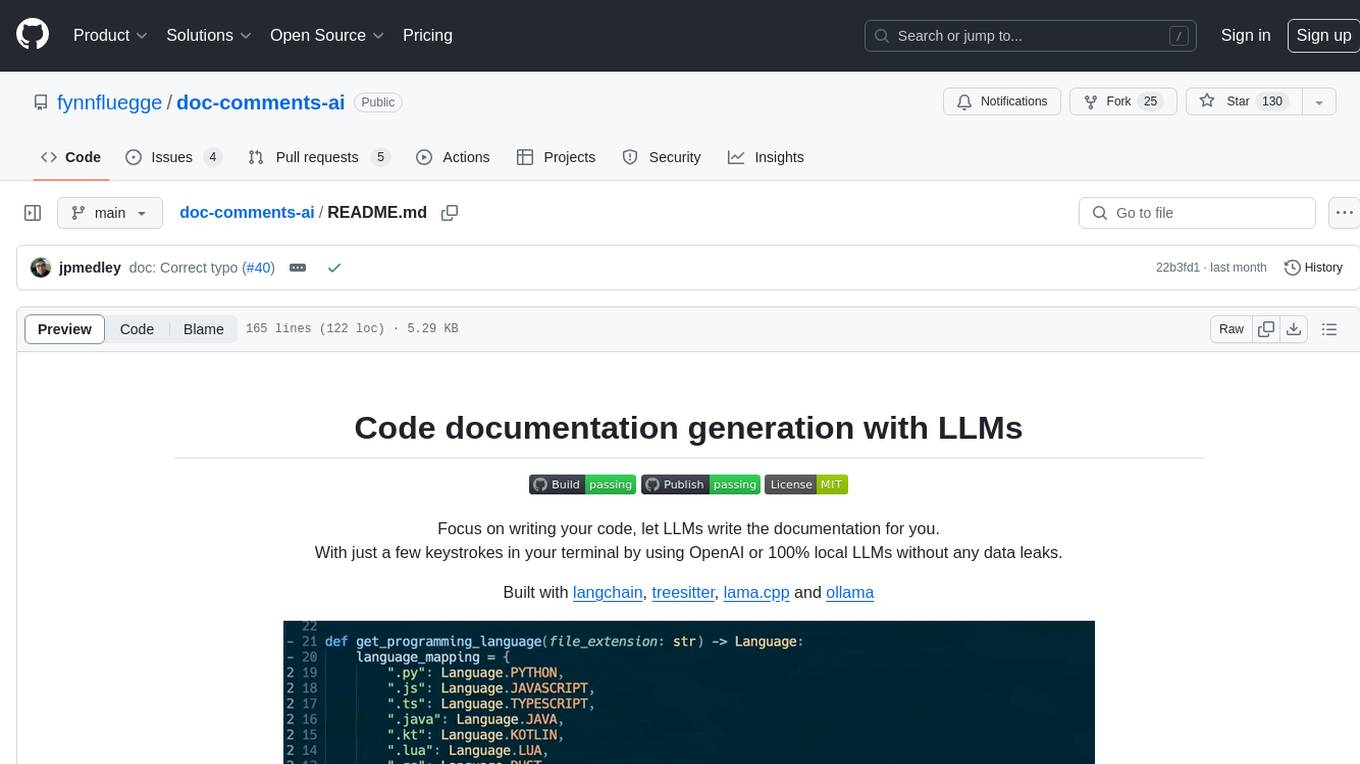
doc-comments-ai
doc-comments-ai is a tool designed to automatically generate code documentation using language models. It allows users to easily create documentation comment blocks for methods in various programming languages such as Python, Typescript, Javascript, Java, Rust, and more. The tool supports both OpenAI and local LLMs, ensuring data privacy and security. Users can generate documentation comments for methods in files, inline comments in method bodies, and choose from different models like GPT-3.5-Turbo, GPT-4, and Azure OpenAI. Additionally, the tool provides support for Treesitter integration and offers guidance on selecting the appropriate model for comprehensive documentation needs.
For similar jobs
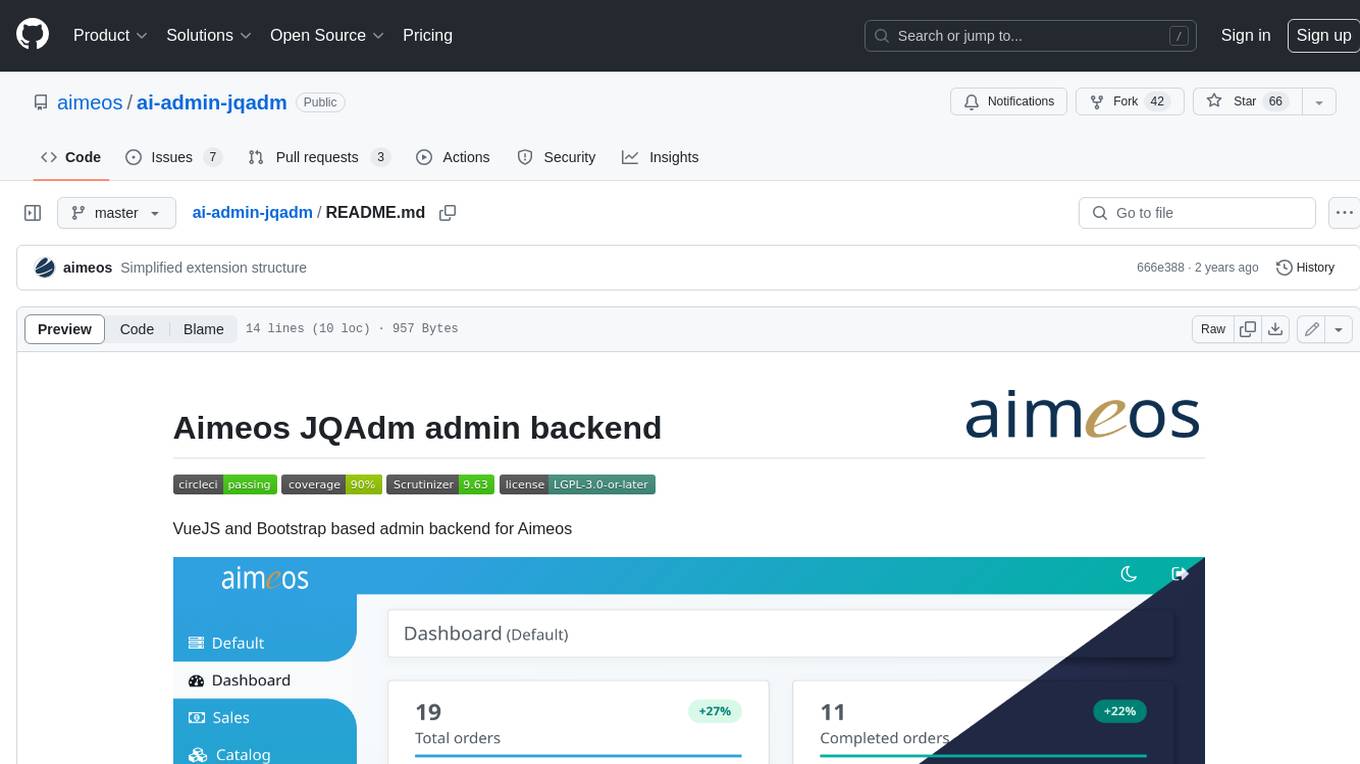
ai-admin-jqadm
Aimeos JQAdm is a VueJS and Bootstrap based admin backend for Aimeos. It provides a user-friendly interface for managing your Aimeos e-commerce website. With Aimeos JQAdm, you can easily add, edit, and delete products, categories, orders, and customers. You can also manage your website's settings, such as payment methods, shipping methods, and taxes. Aimeos JQAdm is a powerful and easy-to-use tool that can help you manage your Aimeos website more efficiently.
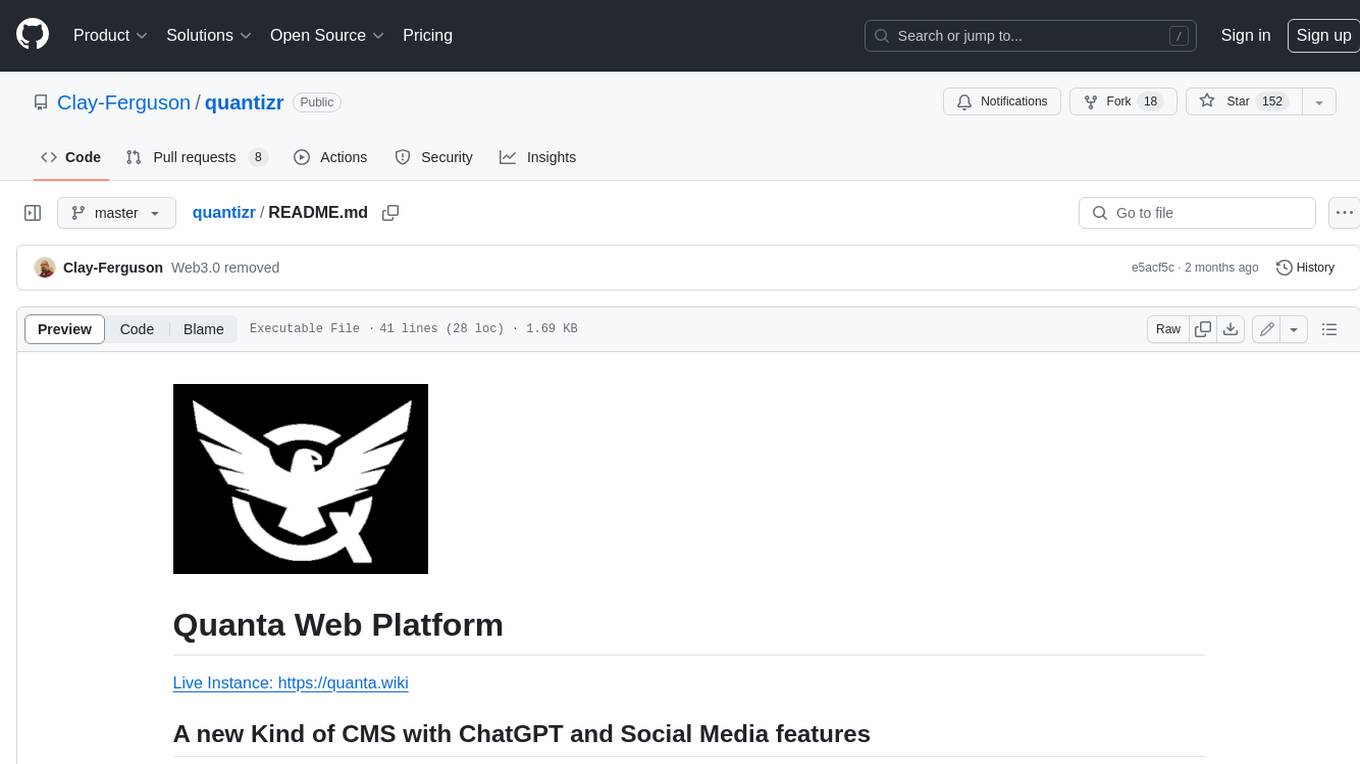
quantizr
Quanta is a new kind of Content Management platform, with powerful features including: Wikis & micro-blogging, ChatGPT Question Answering, Document collaboration and publishing, PDF Generation, Secure messaging with (E2E Encryption), Video/audio recording & sharing, File sharing, Podcatcher (RSS Reader), and many other features related to managing hierarchical content.
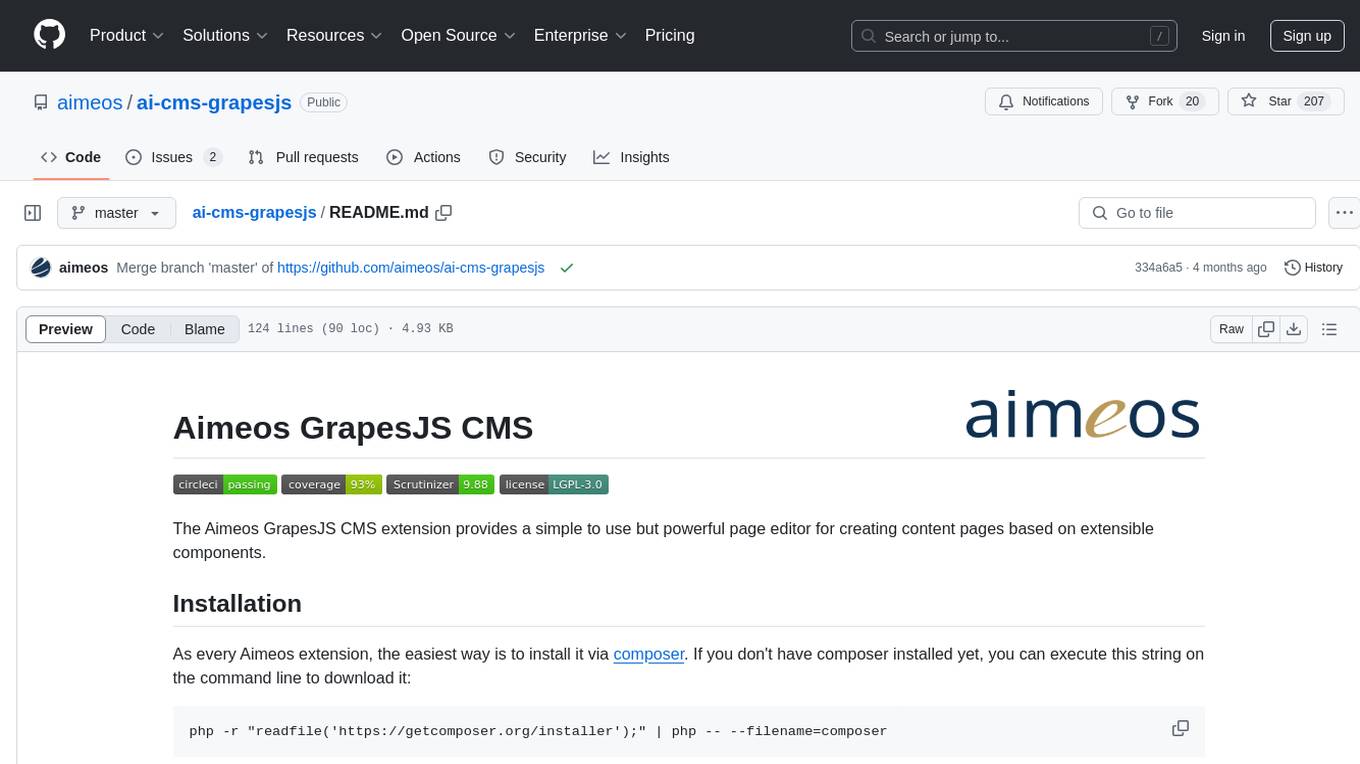
ai-cms-grapesjs
The Aimeos GrapesJS CMS extension provides a simple to use but powerful page editor for creating content pages based on extensible components. It integrates seamlessly with Laravel applications and allows users to easily manage and display CMS content. The tool also supports Google reCAPTCHA v3 for enhanced security. Users can create and customize pages with various components and manage multi-language setups effortlessly. The extension simplifies the process of creating and managing content pages, making it ideal for developers and businesses looking to enhance their website's content management capabilities.
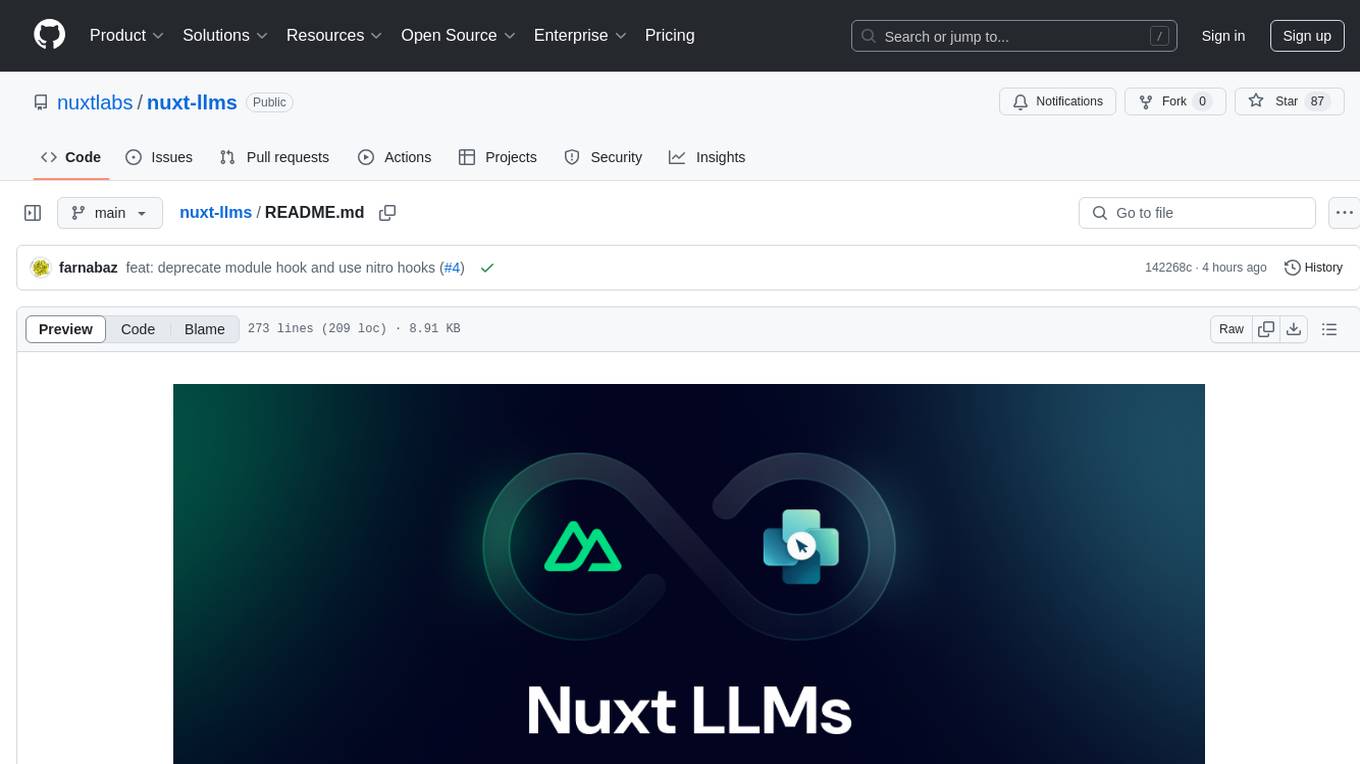
nuxt-llms
Nuxt LLMs automatically generates llms.txt markdown documentation for Nuxt applications. It provides runtime hooks to collect data from various sources and generate structured documentation. The tool allows customization of sections directly from nuxt.config.ts and integrates with Nuxt modules via the runtime hooks system. It generates two documentation formats: llms.txt for concise structured documentation and llms_full.txt for detailed documentation. Users can extend documentation using hooks to add sections, links, and metadata. The tool is suitable for developers looking to automate documentation generation for their Nuxt applications.
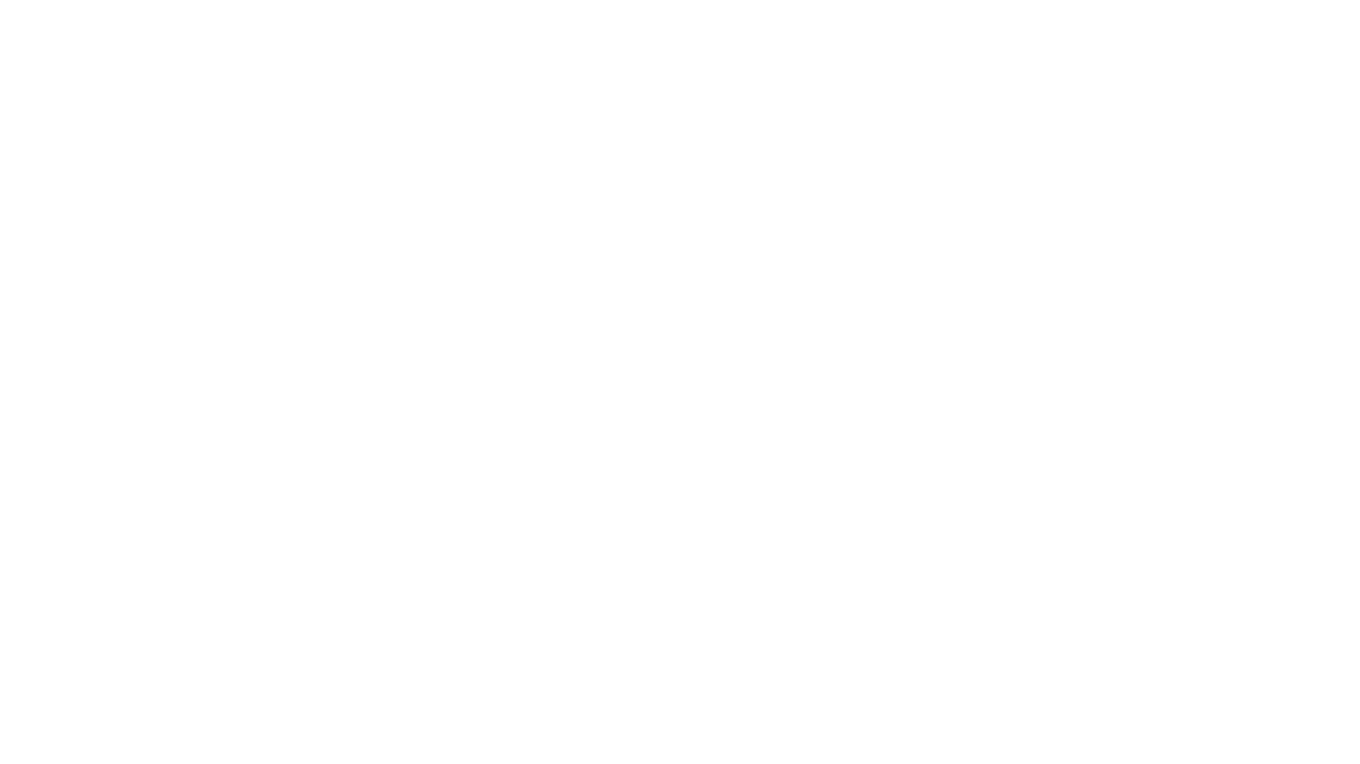
DocsGPT
DocsGPT is an open-source documentation assistant powered by GPT models. It simplifies the process of searching for information in project documentation by allowing developers to ask questions and receive accurate answers. With DocsGPT, users can say goodbye to manual searches and quickly find the information they need. The tool aims to revolutionize project documentation experiences and offers features like live previews, Discord community, guides, and contribution opportunities. It consists of a Flask app, Chrome extension, similarity search index creation script, and a frontend built with Vite and React. Users can quickly get started with DocsGPT by following the provided setup instructions and can contribute to its development by following the guidelines in the CONTRIBUTING.md file. The project follows a Code of Conduct to ensure a harassment-free community environment for all participants. DocsGPT is licensed under MIT and is built with LangChain.
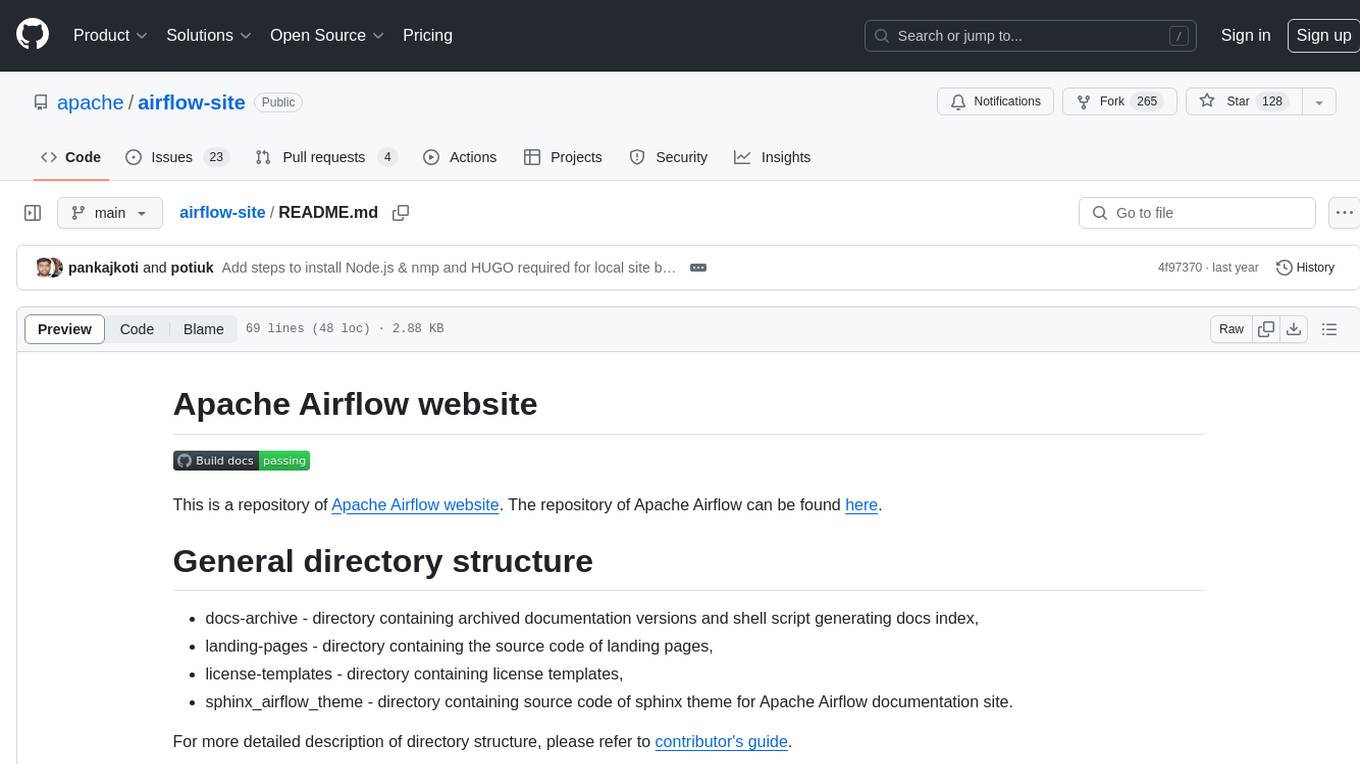
airflow-site
This repository contains the source code for the Apache Airflow website, including directories for archived documentation versions, landing pages, license templates, and the Sphinx theme. To work on the site locally, users need to install coreutils, Node.js, NPM, and HUGO, and run specific scripts provided in the repository. Contributors can refer to the contributor's guide for detailed instructions on how to contribute to the website.
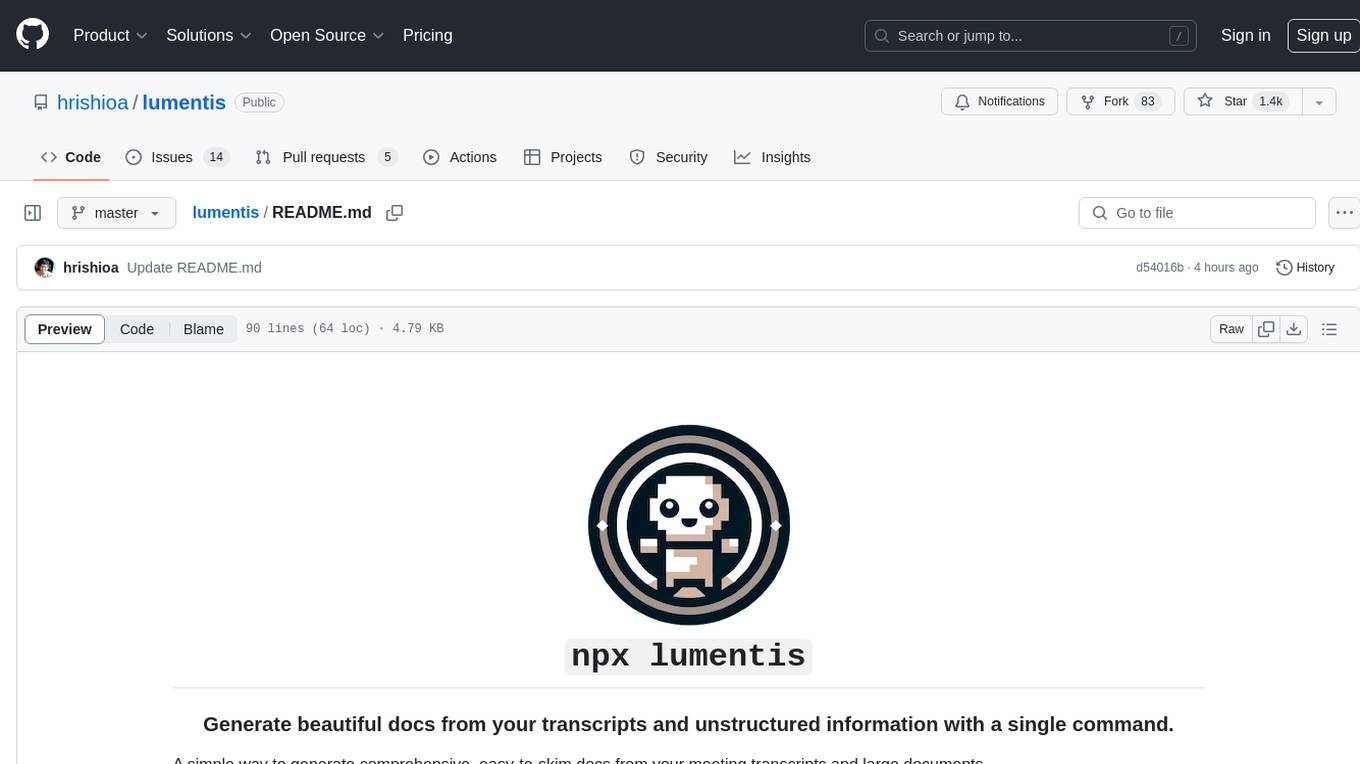
lumentis
Lumentis is a tool that allows users to generate beautiful and comprehensive documentation from meeting transcripts and large documents with a single command. It reads transcripts, asks questions to understand themes and audience, generates an outline, and creates detailed pages with visual variety and styles. Users can switch models for different tasks, control the process, and deploy the generated docs to Vercel. The tool is designed to be open, clean, fast, and easy to use, with upcoming features including folders, PDFs, auto-transcription, website scraping, scientific papers handling, summarization, and continuous updates.
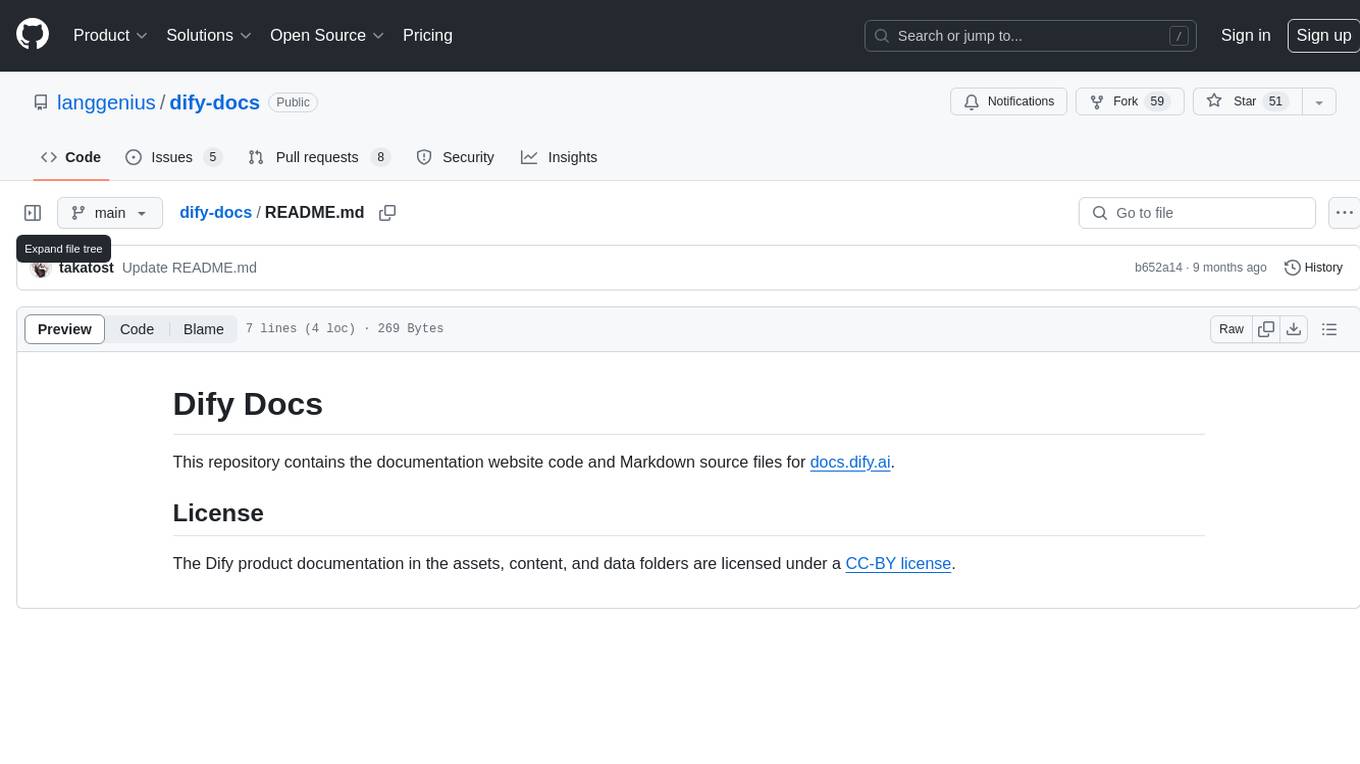
dify-docs
Dify Docs is a repository that houses the documentation website code and Markdown source files for docs.dify.ai. It contains assets, content, and data folders that are licensed under a CC-BY license.