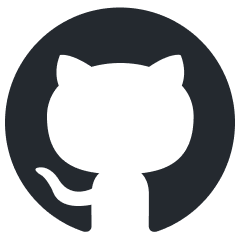
agentic
AI agent stdlib that works with any LLM and TypeScript AI SDK.
Stars: 17266
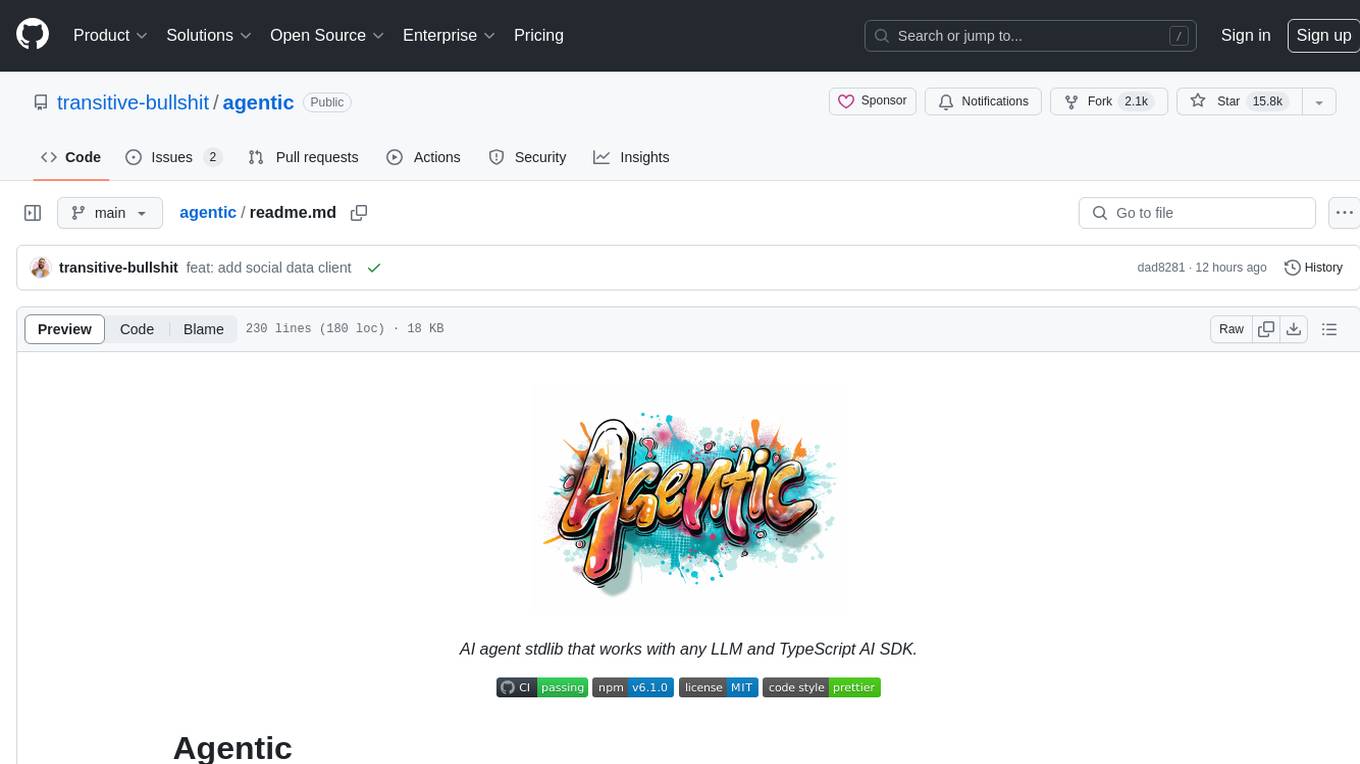
Agentic is a standard AI functions/tools library optimized for TypeScript and LLM-based apps, compatible with major AI SDKs. It offers a set of thoroughly tested AI functions that can be used with favorite AI SDKs without writing glue code. The library includes various clients for services like Bing web search, calculator, Clearbit data resolution, Dexa podcast questions, and more. It also provides compound tools like SearchAndCrawl and supports multiple AI SDKs such as OpenAI, Vercel AI SDK, LangChain, LlamaIndex, Firebase Genkit, and Dexa Dexter. The goal is to create minimal clients with strongly-typed TypeScript DX, composable AIFunctions via AIFunctionSet, and compatibility with major TS AI SDKs.
README:
AI agent stdlib that works with any LLM and TypeScript AI SDK.
Agentic is a standard library of AI functions / tools which are optimized for both normal TS-usage as well as LLM-based usage. Agentic works with all of the major TS AI SDKs (Vercel AI SDK, Mastra, LangChain, LlamaIndex, OpenAI SDK, MCP, etc).
Agentic clients like WeatherClient
can be used as normal TS classes:
import { WeatherClient } from '@agentic/stdlib'
// Requires `process.env.WEATHER_API_KEY` (free from weatherapi.com)
const weather = new WeatherClient()
const result = await weather.getCurrentWeather({
q: 'San Francisco'
})
console.log(result)
Or you can use these clients as LLM-based tools. Here's an example using Vercel's AI SDK:
// sdk-specific imports
import { openai } from '@ai-sdk/openai'
import { generateText } from 'ai'
import { createAISDKTools } from '@agentic/ai-sdk'
// sdk-agnostic imports
import { WeatherClient } from '@agentic/stdlib'
const weather = new WeatherClient()
const result = await generateText({
model: openai('gpt-4o-mini'),
// this is the key line which uses the `@agentic/ai-sdk` adapter
tools: createAISDKTools(weather),
toolChoice: 'required',
prompt: 'What is the weather in San Francisco?'
})
console.log(result.toolResults[0])
You can use our standard library of thoroughly tested AI functions with your favorite AI SDK – without having to write any glue code!
All adapters (like createAISDKTools
) accept a very flexible var args of AIFunctionLike
parameters, so you can pass as many tools as you'd like.
They also expose a .functions
property which is an AIFunctionSet
. This combination makes it really easy to mix & match different tools together.
import { SerperClient, WikipediaClient, FirecrawlClient } from '@agentic/stdlib'
import { createAIFunction } from '@agentic/core'
import { z } from 'zod'
const googleSearch = new SerperClient()
const wikipedia = new WikipediaClient()
const firecrawl = new FirecrawlClient()
const result = await generateText({
model: openai('gpt-4o-mini'),
// This example uses tools from 4 different sources. You can pass as many
// AIFunctionLike objects as you want.
tools: createAISDKTools(
googleSearch,
wikipedia,
// Pick a single function from the firecrawl client's set of AI functions
firecrawl.functions.pick('firecrawl_search'),
// Create a custom AI function (based off of Anthropic's think tool: https://www.anthropic.com/engineering/claude-think-tool)
createAIFunction({
name: 'think',
description: `Use this tool to think about something. It will not obtain new information or change the database, but just append the thought to the log. Use it when complex reasoning or some cache memory is needed.`,
inputSchema: z.object({
thought: z.string().describe('A thought to think about.')
}),
execute: ({ thought }) => thought
})
),
prompt:
'What year did Jurassic Park the movie come out, and what else happened that year?'
})
An AIFunctionLike
can be any agentic client instance, a single AIFunction
selected from the client's .functions
property (which holds an AIFunctionSet
of available AI functions), or an AI function created manually via createAIFunction
.
AIFunctionLike
and AIFunctionSet
are implementation details that you likely won't have to touch directly, but they're important because of their flexibility.
- ✅ All tools are thoroughly tested in production
- ✅ Tools work across all leading TS AI SDKs
- ✅ Tools are hand-coded and extremely minimal
- ✅ Tools have both a good manual DX and LLM DX via the
@aiFunction
decorator - ✅ Tools use native
fetch
- ✅ Tools use ky to wrap
fetch
, so HTTP options, throttling, retries, etc are easy to customize - ✅ Supports tools from any MCP server (createMcpTools(...))
- ✅ Generate new Agentic tool clients from OpenAPI specs (@agentic/openapi-to-ts)
- ✅ 100% open source && not trying to sell you anything 💯
Full docs are available at agentic.so.
Agentic adapter docs for the Vercel AI SDK
Agentic adapter docs for the Mastra AI Agent framework
Agentic adapter docs for LangChain
Agentic adapter docs for LlamaIndex
Agentic adapter docs for Genkit
Agentic adapter docs for Dexter
Agentic adapter docs for OpenAI
Agentic support in GenAIScript
Agentic adapter docs for the xsAI SDK
Service / Tool | Package | Docs | Description |
---|---|---|---|
Apollo | @agentic/apollo |
docs | B2B person and company enrichment API. |
ArXiv | @agentic/arxiv |
docs | Search for research articles. |
Bing | @agentic/bing |
docs | Bing web search. |
Brave Search | @agentic/brave-search |
docs | Brave web search and local places search. |
Calculator | @agentic/calculator |
docs | Basic calculator for simple mathematical expressions. |
Clearbit | @agentic/clearbit |
docs | Resolving and enriching people and company data. |
Dexa | @agentic/dexa |
docs | Answers questions from the world's best podcasters. |
Diffbot | @agentic/diffbot |
docs | Web page classification and scraping; person and company data enrichment. |
DuckDuckGo | @agentic/duck-duck-go |
docs | Privacy-focused web search API. |
E2B | @agentic/e2b |
docs | Hosted Python code interpreter sandbox which is really useful for data analysis, flexible code execution, and advanced reasoning on-the-fly. |
Exa | @agentic/exa |
docs | Web search tailored for LLMs. |
Firecrawl | @agentic/firecrawl |
docs | Website scraping and structured data extraction. |
Google Custom Search | @agentic/google-custom-search |
docs | Official Google Custom Search API. |
Gravatar | @agentic/gravatar |
docs | Gravatar profile API. |
HackerNews | @agentic/hacker-news |
docs | Official HackerNews API. |
Hunter | @agentic/hunter |
docs | Email finder, verifier, and enrichment. |
Jina | @agentic/jina |
docs | URL scraper and web search. |
LeadMagic | @agentic/leadmagic |
docs | B2B person, company, and email enrichment API. |
Midjourney | @agentic/midjourney |
docs | Unofficial Midjourney client for generative images. |
McpTools | @agentic/mcp |
docs | Model Context Protocol (MCP) client, supporting any MCP server. Use createMcpTools to spawn or connect to an MCP server. |
Notion | @agentic/notion |
docs | Official Notion API for accessing pages, databases, and content. |
Novu | @agentic/novu |
docs | Sending notifications (email, SMS, in-app, push, etc). |
Open Meteo | @agentic/open-meteo |
docs | Free weather API (no API key required). |
People Data Labs | @agentic/people-data-labs |
docs | People & company data (WIP). |
Perigon | @agentic/perigon |
docs | Real-time news API and web content data from 140,000+ sources. Structured and enriched by AI, primed for LLMs. |
Polygon | @agentic/polygon |
docs | Stock market and company financial data. |
PredictLeads | @agentic/predict-leads |
docs | In-depth company data including signals like fundraising events, hiring news, product launches, technologies used, etc. |
Proxycurl | @agentic/proxycurl |
docs | People and company data from LinkedIn & Crunchbase. |
RocketReach | @agentic/rocketreach |
docs | B2B person and company enrichment API. |
Searxng | @agentic/searxng |
docs | OSS meta search engine capable of searching across many providers like Reddit, Google, Brave, Arxiv, Genius, IMDB, Rotten Tomatoes, Wikidata, Wolfram Alpha, YouTube, GitHub, etc. |
SerpAPI | @agentic/serpapi |
docs | Lightweight wrapper around SerpAPI for Google search. |
Serper | @agentic/serper |
docs | Lightweight wrapper around Serper for Google search. |
Slack | @agentic/slack |
docs | Send and receive Slack messages. |
SocialData | @agentic/social-data |
docs | Unofficial Twitter / X client (readonly) which is much cheaper than the official Twitter API. |
Tavily | @agentic/tavily |
docs | Web search API tailored for LLMs. |
Twilio | @agentic/twilio |
docs | Twilio conversation API to send and receive SMS messages. |
@agentic/twitter |
docs | Basic Twitter API methods for fetching users, tweets, and searching recent tweets. Includes support for plan-aware rate-limiting. Uses Nango for OAuth support. | |
Weather | @agentic/weather |
docs | Basic access to current weather data based on location. |
Wikidata | @agentic/wikidata |
docs | Basic Wikidata client. |
Wikipedia | @agentic/wikipedia |
docs | Wikipedia page search and summaries. |
Wolfram Alpha | @agentic/wolfram-alpha |
docs | Wolfram Alpha LLM API client for answering computational, mathematical, and scientific questions. |
ZoomInfo | @agentic/zoominfo |
docs | Powerful B2B person and company data enrichment. |
[!NOTE] Missing a tool or want to add your own tool to this list? If you have an OpenAPI v3 spec for your tool's API, we make it extremely easy to add support using our @agentic/openapi-to-ts CLI. Otherwise, feel free to open an issue to discuss or submit a PR.
For more details on tool usage, see the docs.
- Travis Fischer
- David Zhang
- Philipp Burckhardt
- And all of these amazing OSS contributors:
MIT © Travis Fischer
To stay up to date or learn more, follow @transitive_bs on Twitter.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for agentic
Similar Open Source Tools
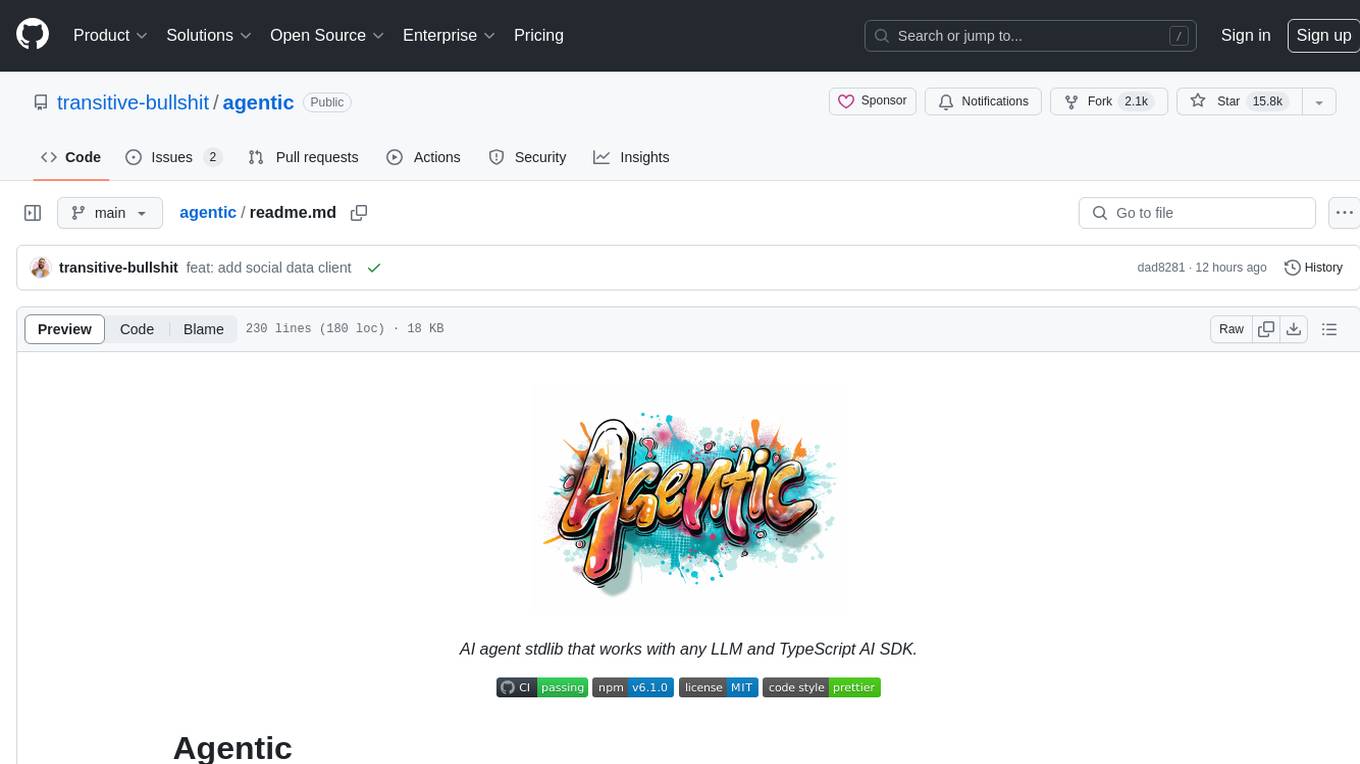
agentic
Agentic is a standard AI functions/tools library optimized for TypeScript and LLM-based apps, compatible with major AI SDKs. It offers a set of thoroughly tested AI functions that can be used with favorite AI SDKs without writing glue code. The library includes various clients for services like Bing web search, calculator, Clearbit data resolution, Dexa podcast questions, and more. It also provides compound tools like SearchAndCrawl and supports multiple AI SDKs such as OpenAI, Vercel AI SDK, LangChain, LlamaIndex, Firebase Genkit, and Dexa Dexter. The goal is to create minimal clients with strongly-typed TypeScript DX, composable AIFunctions via AIFunctionSet, and compatibility with major TS AI SDKs.
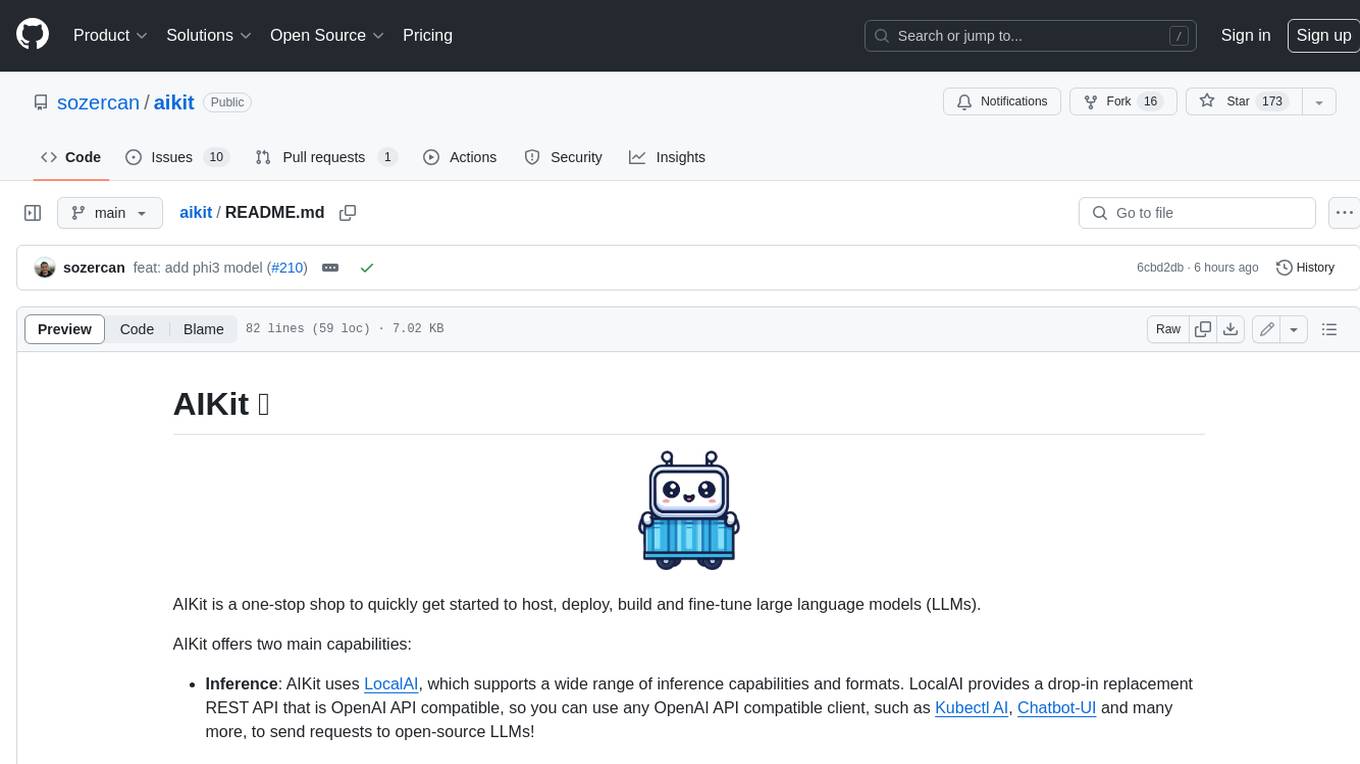
aikit
AIKit is a one-stop shop to quickly get started to host, deploy, build and fine-tune large language models (LLMs). AIKit offers two main capabilities: Inference: AIKit uses LocalAI, which supports a wide range of inference capabilities and formats. LocalAI provides a drop-in replacement REST API that is OpenAI API compatible, so you can use any OpenAI API compatible client, such as Kubectl AI, Chatbot-UI and many more, to send requests to open-source LLMs! Fine Tuning: AIKit offers an extensible fine tuning interface. It supports Unsloth for fast, memory efficient, and easy fine-tuning experience.
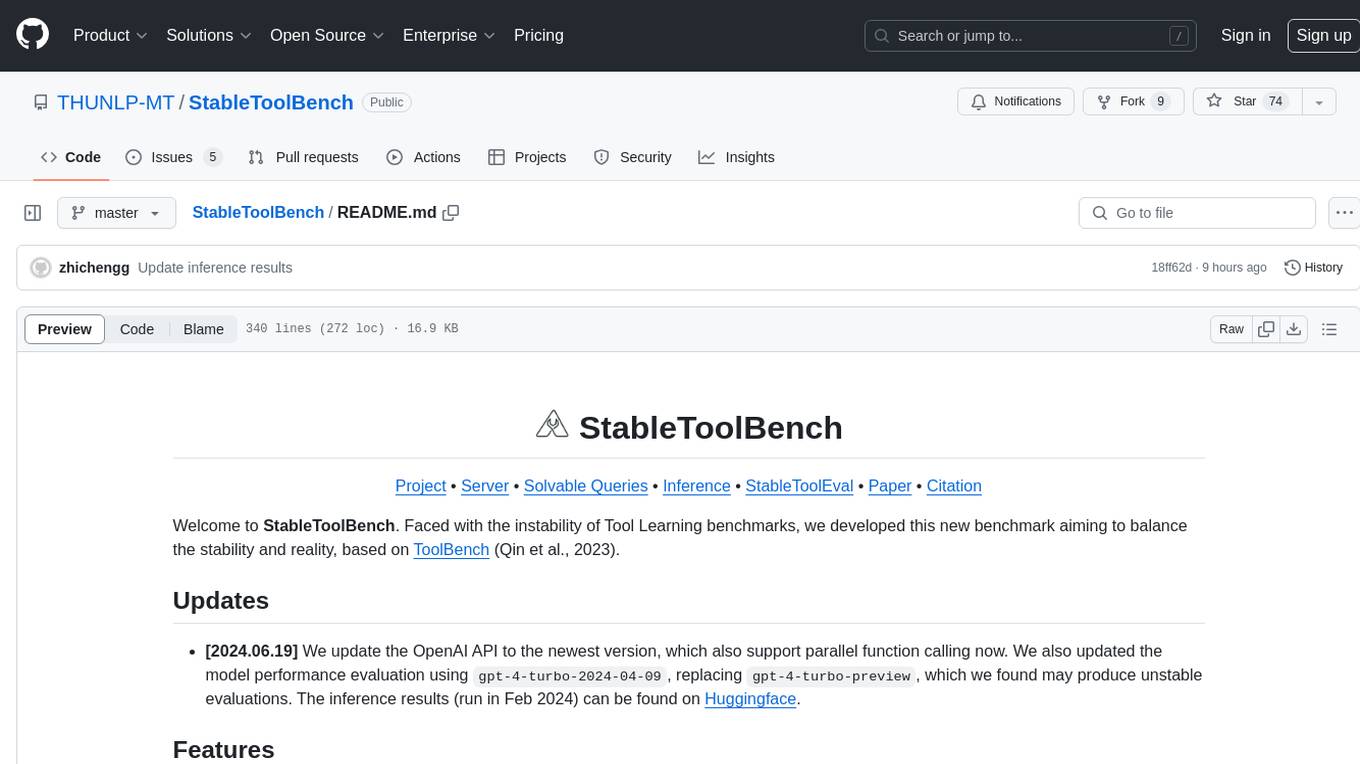
StableToolBench
StableToolBench is a new benchmark developed to address the instability of Tool Learning benchmarks. It aims to balance stability and reality by introducing features like Virtual API System, Solvable Queries, and Stable Evaluation System. The benchmark ensures consistency through a caching system and API simulators, filters queries based on solvability using LLMs, and evaluates model performance using GPT-4 with metrics like Solvable Pass Rate and Solvable Win Rate.
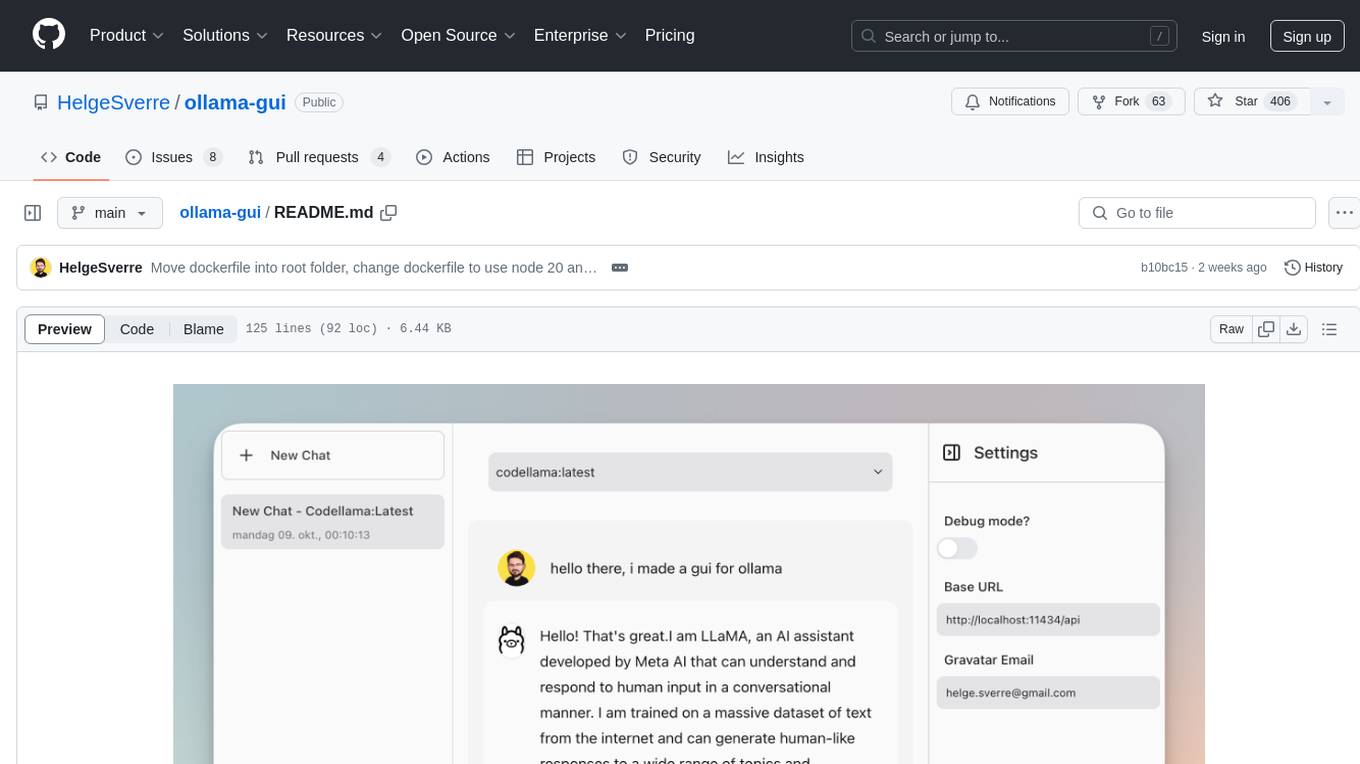
ollama-gui
Ollama GUI is a web interface for ollama.ai, a tool that enables running Large Language Models (LLMs) on your local machine. It provides a user-friendly platform for chatting with LLMs and accessing various models for text generation. Users can easily interact with different models, manage chat history, and explore available models through the web interface. The tool is built with Vue.js, Vite, and Tailwind CSS, offering a modern and responsive design for seamless user experience.
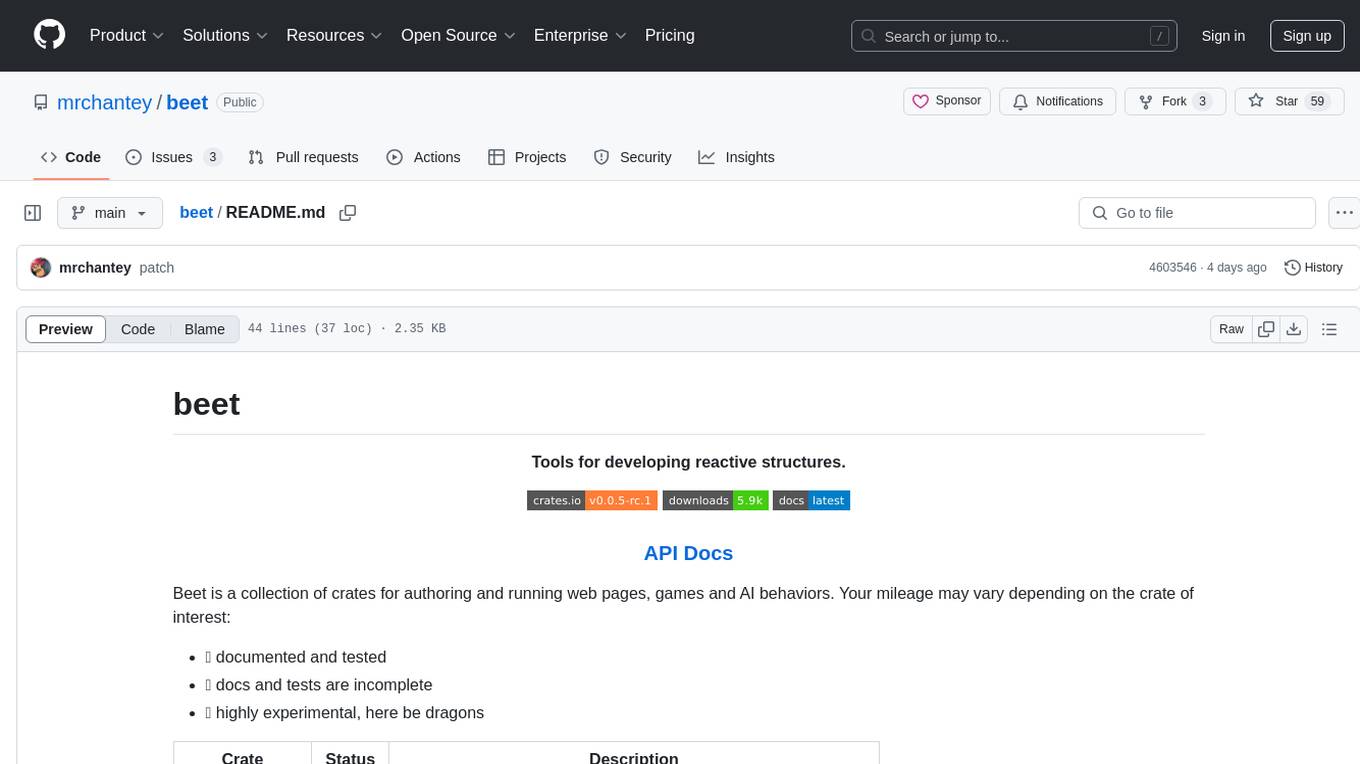
beet
Beet is a collection of crates for authoring and running web pages, games and AI behaviors. It includes crates like `beet_flow` for scenes-as-control-flow bevy library, `beet_spatial` for spatial behaviors, `beet_ml` for machine learning, `beet_sim` for simulation tooling, `beet_rsx` for authoring tools for html and bevy, and `beet_router` for file-based router for web docs. The `beet` crate acts as a base crate that re-exports sub-crates based on feature flags, similar to the `bevy` crate structure.
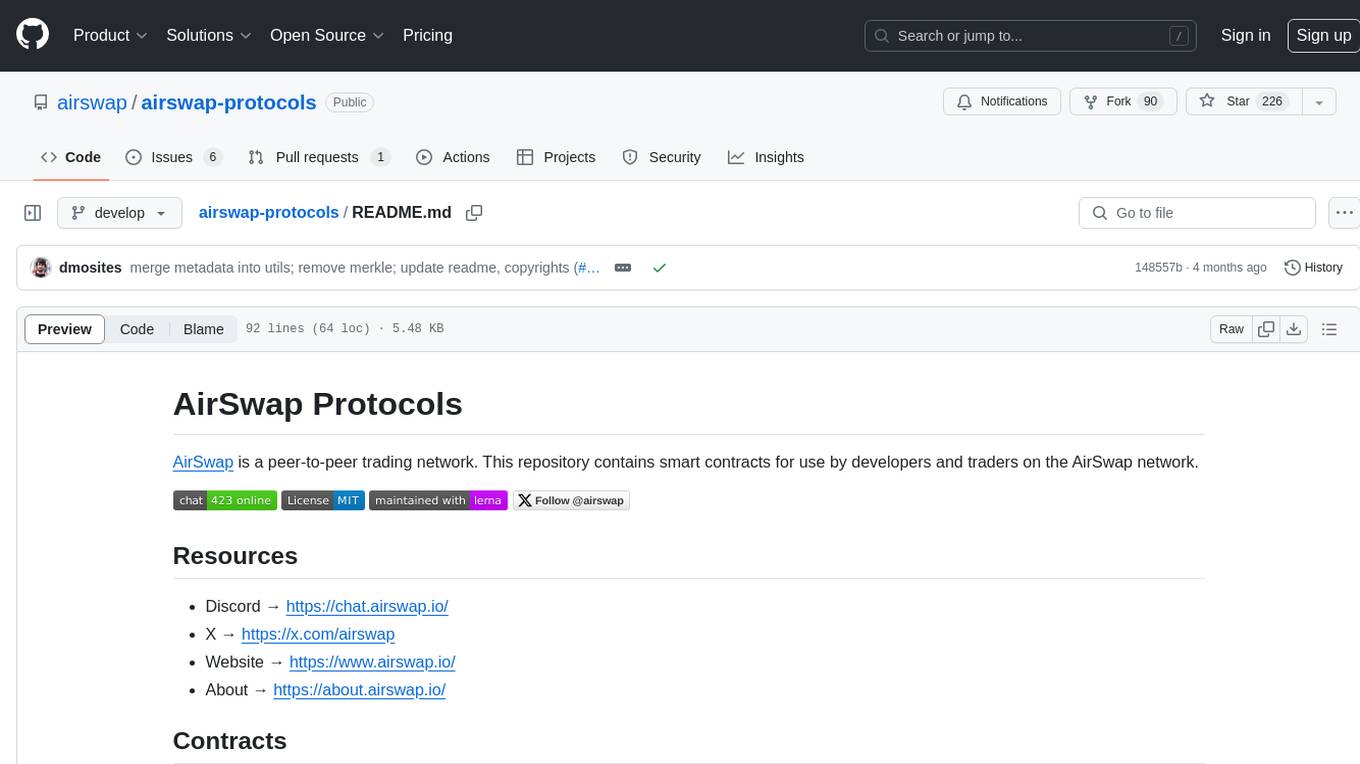
airswap-protocols
AirSwap Protocols is a repository containing smart contracts for developers and traders on the AirSwap peer-to-peer trading network. It includes various packages for functionalities like server registry, atomic token swap, staking, rewards pool, batch token and order calls, libraries, and utils. The repository follows a branching and release process for contracts and tools, with steps for regular development process and individual package features or patches. Users can deploy and verify contracts using specific commands with network flags.
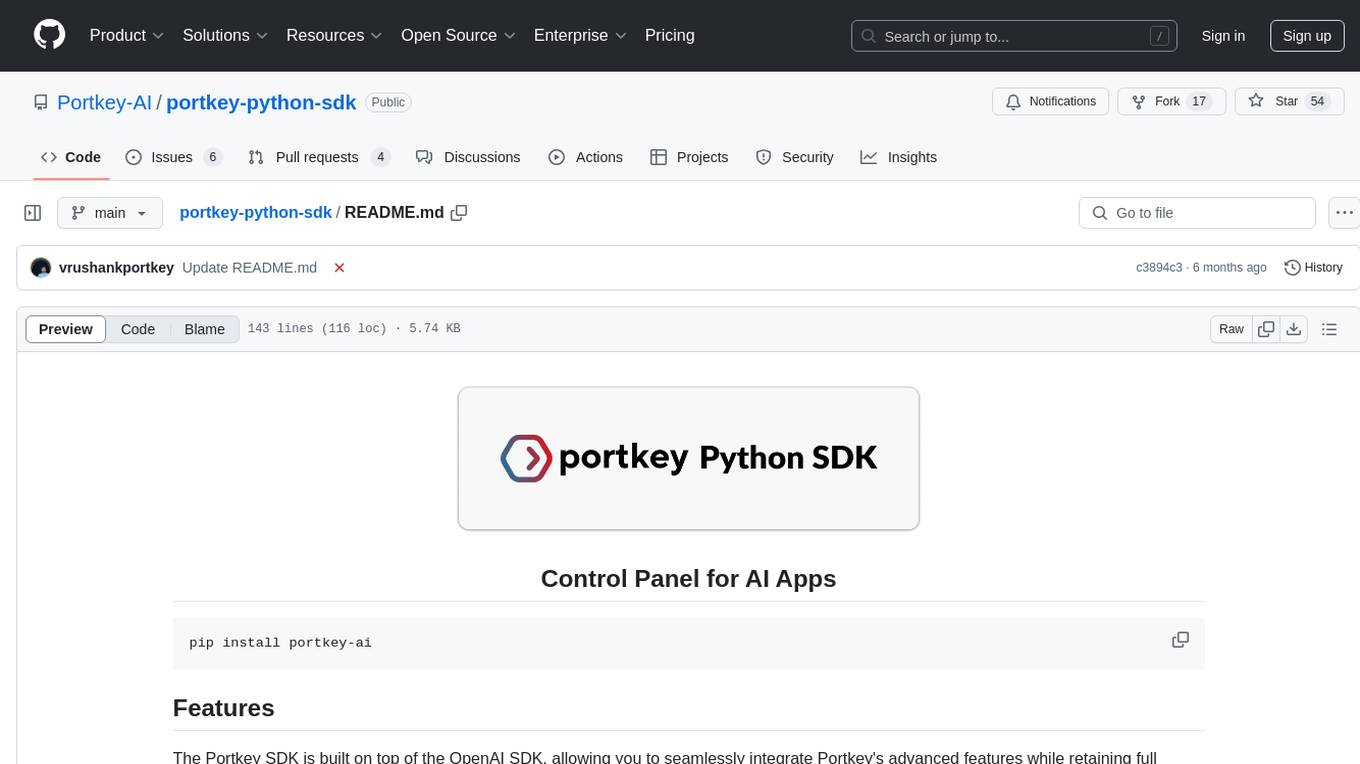
portkey-python-sdk
The Portkey Python SDK is a control panel for AI apps that allows seamless integration of Portkey's advanced features with OpenAI methods. It provides features such as AI gateway for unified API signature, interoperability, automated fallbacks & retries, load balancing, semantic caching, virtual keys, request timeouts, observability with logging, requests tracing, custom metadata, feedback collection, and analytics. Users can make requests to OpenAI using Portkey SDK and also use async functionality. The SDK is compatible with OpenAI SDK methods and offers Portkey-specific methods like feedback and prompts. It supports various providers and encourages contributions through Github issues or direct contact via email or Discord.
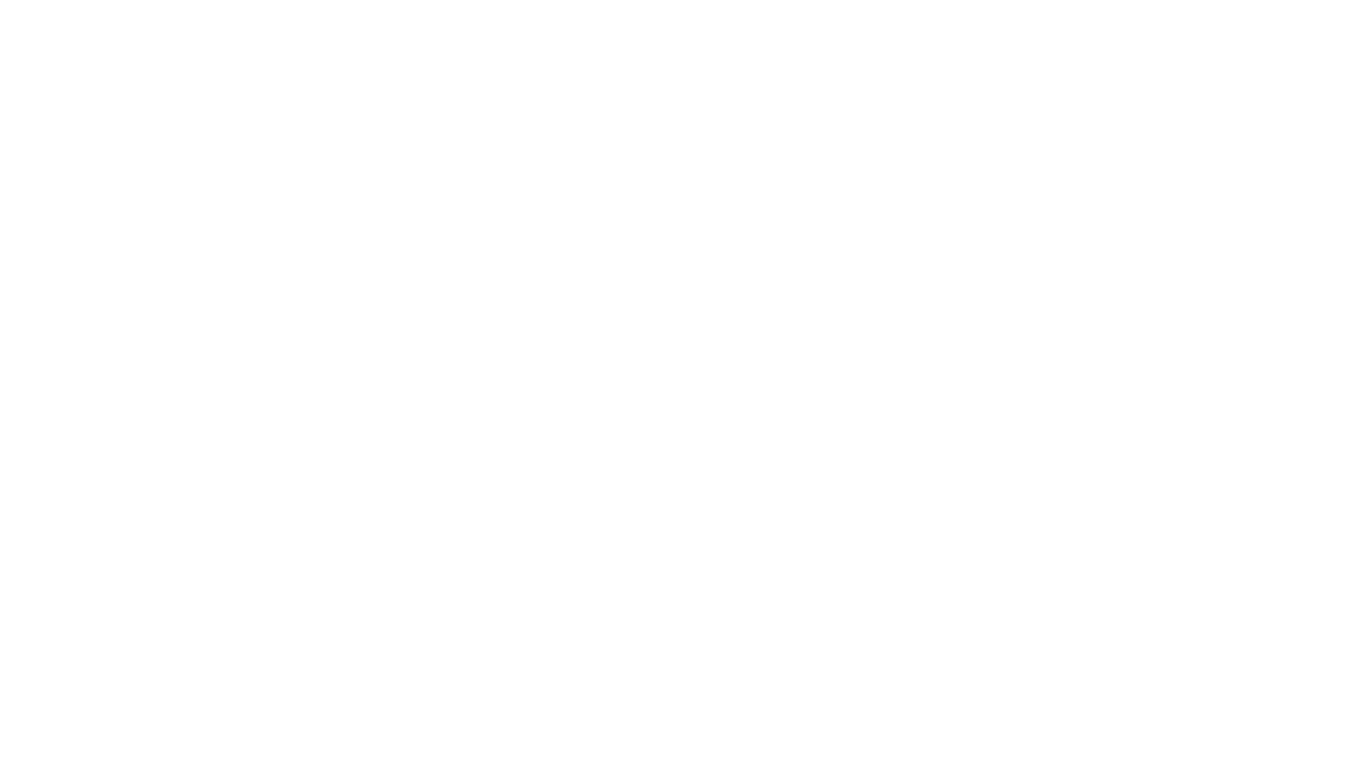
graphrag-visualizer
GraphRAG Visualizer is an application designed to visualize Microsoft GraphRAG artifacts by uploading parquet files generated from the GraphRAG indexing pipeline. Users can view and analyze data in 2D or 3D graphs, display data tables, search for specific nodes or relationships, and process artifacts locally for data security and privacy.
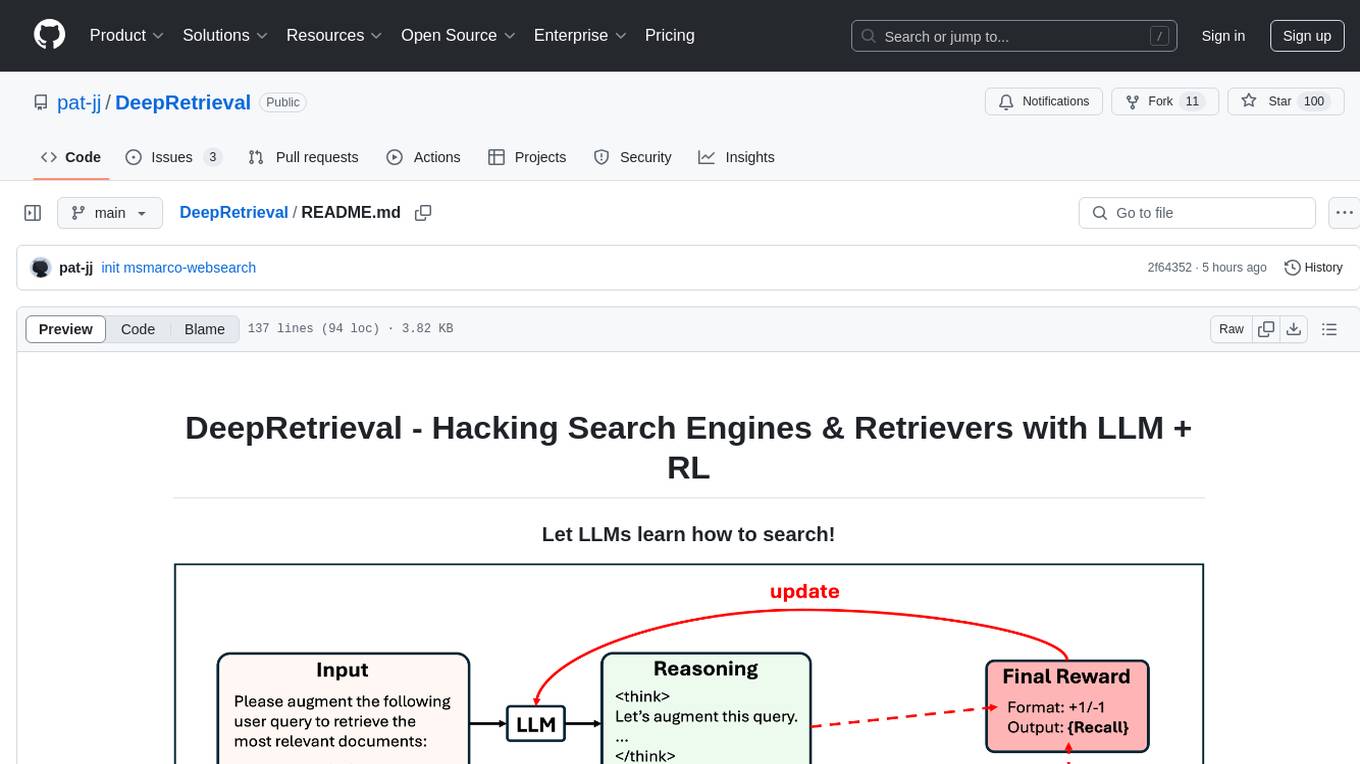
DeepRetrieval
DeepRetrieval is a tool designed to enhance search engines and retrievers using Large Language Models (LLMs) and Reinforcement Learning (RL). It allows LLMs to learn how to search effectively by integrating with search engine APIs and customizing reward functions. The tool provides functionalities for data preparation, training, evaluation, and monitoring search performance. DeepRetrieval aims to improve information retrieval tasks by leveraging advanced AI techniques.
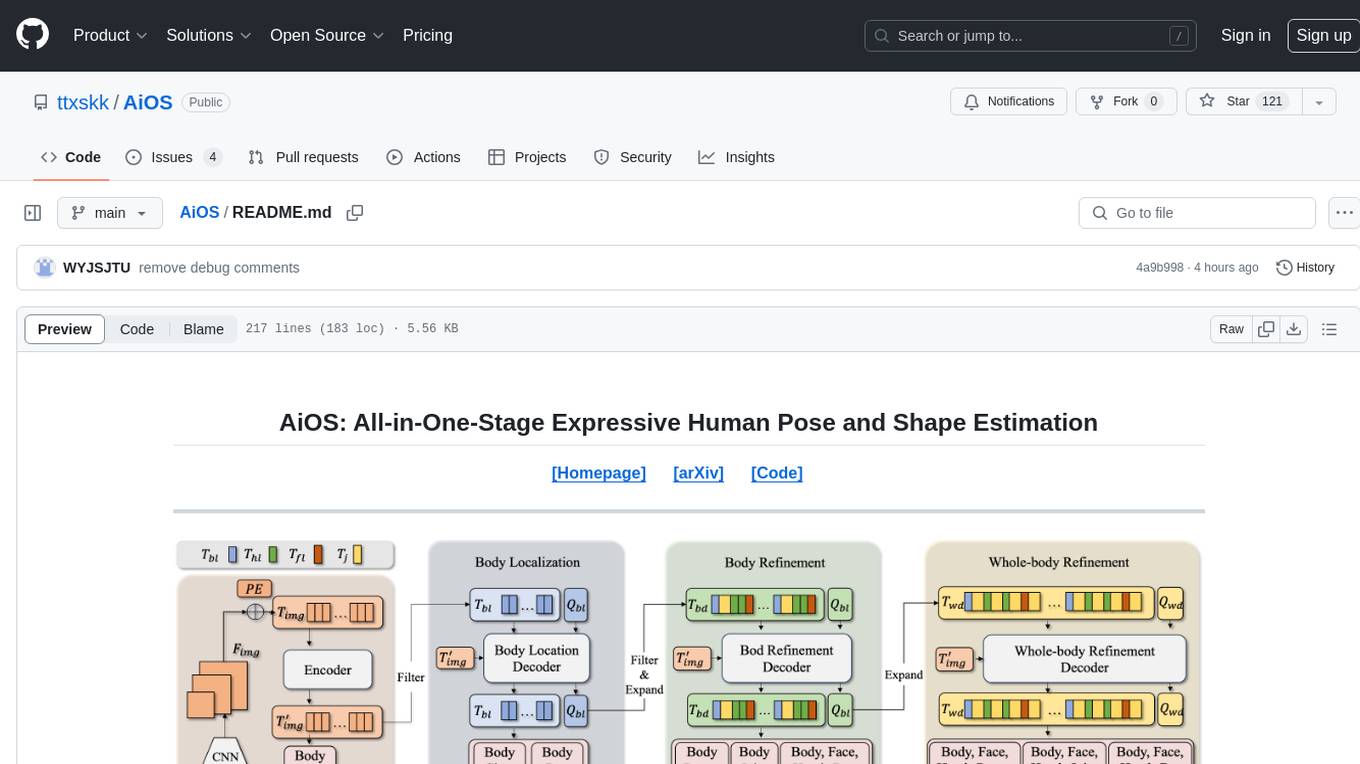
AiOS
AiOS is a tool for human pose and shape estimation, performing human localization and SMPL-X estimation in a progressive manner. It consists of body localization, body refinement, and whole-body refinement stages. Users can download datasets for evaluation, SMPL-X body models, and AiOS checkpoint. Installation involves creating a conda virtual environment, installing PyTorch, torchvision, Pytorch3D, MMCV, and other dependencies. Inference requires placing the video for inference and pretrained models in specific directories. Test results are provided for NMVE, NMJE, MVE, and MPJPE on datasets like BEDLAM and AGORA. Users can run scripts for AGORA validation, AGORA test leaderboard, and BEDLAM leaderboard. The tool acknowledges codes from MMHuman3D, ED-Pose, and SMPLer-X.
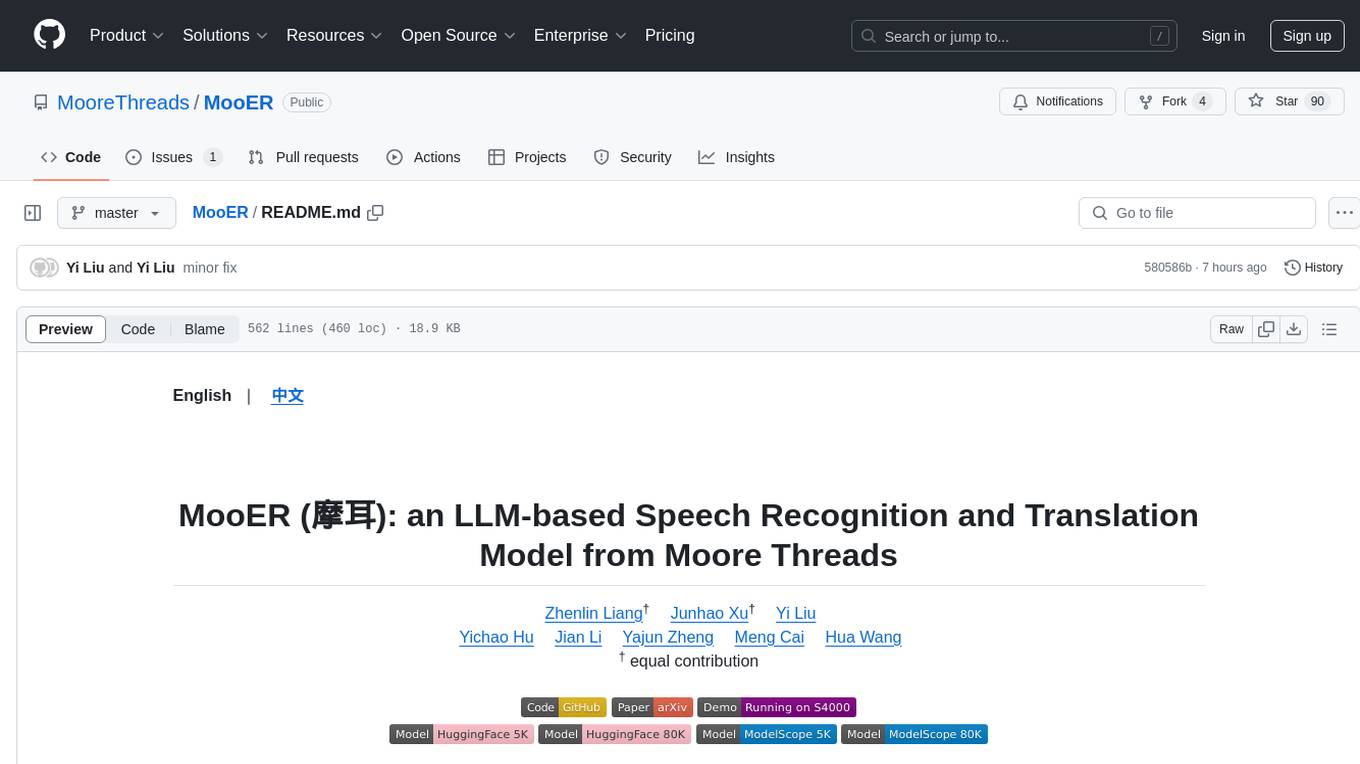
MooER
MooER (摩耳) is an LLM-based speech recognition and translation model developed by Moore Threads. It allows users to transcribe speech into text (ASR) and translate speech into other languages (AST) in an end-to-end manner. The model was trained using 5K hours of data and is now also available with an 80K hours version. MooER is the first LLM-based speech model trained and inferred using domestic GPUs. The repository includes pretrained models, inference code, and a Gradio demo for a better user experience.
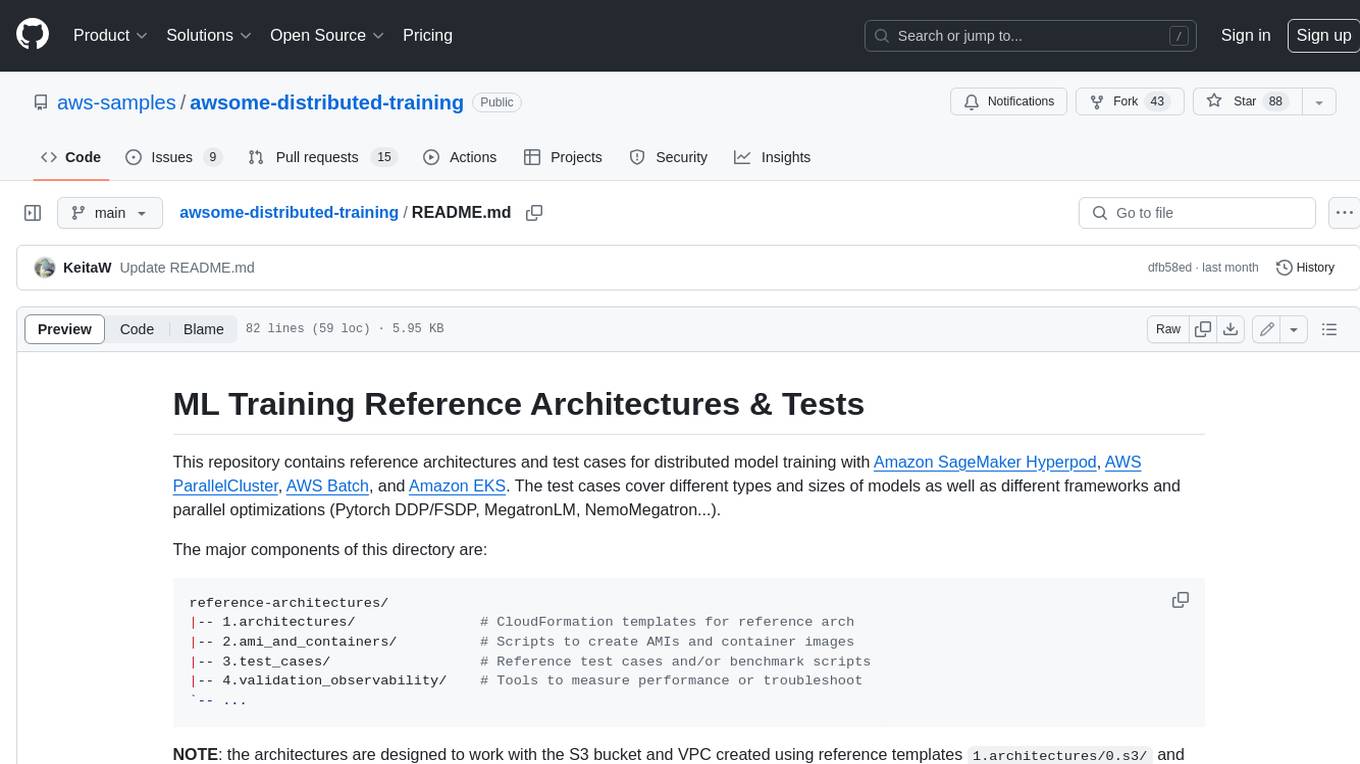
awsome-distributed-training
This repository contains reference architectures and test cases for distributed model training with Amazon SageMaker Hyperpod, AWS ParallelCluster, AWS Batch, and Amazon EKS. The test cases cover different types and sizes of models as well as different frameworks and parallel optimizations (Pytorch DDP/FSDP, MegatronLM, NemoMegatron...).
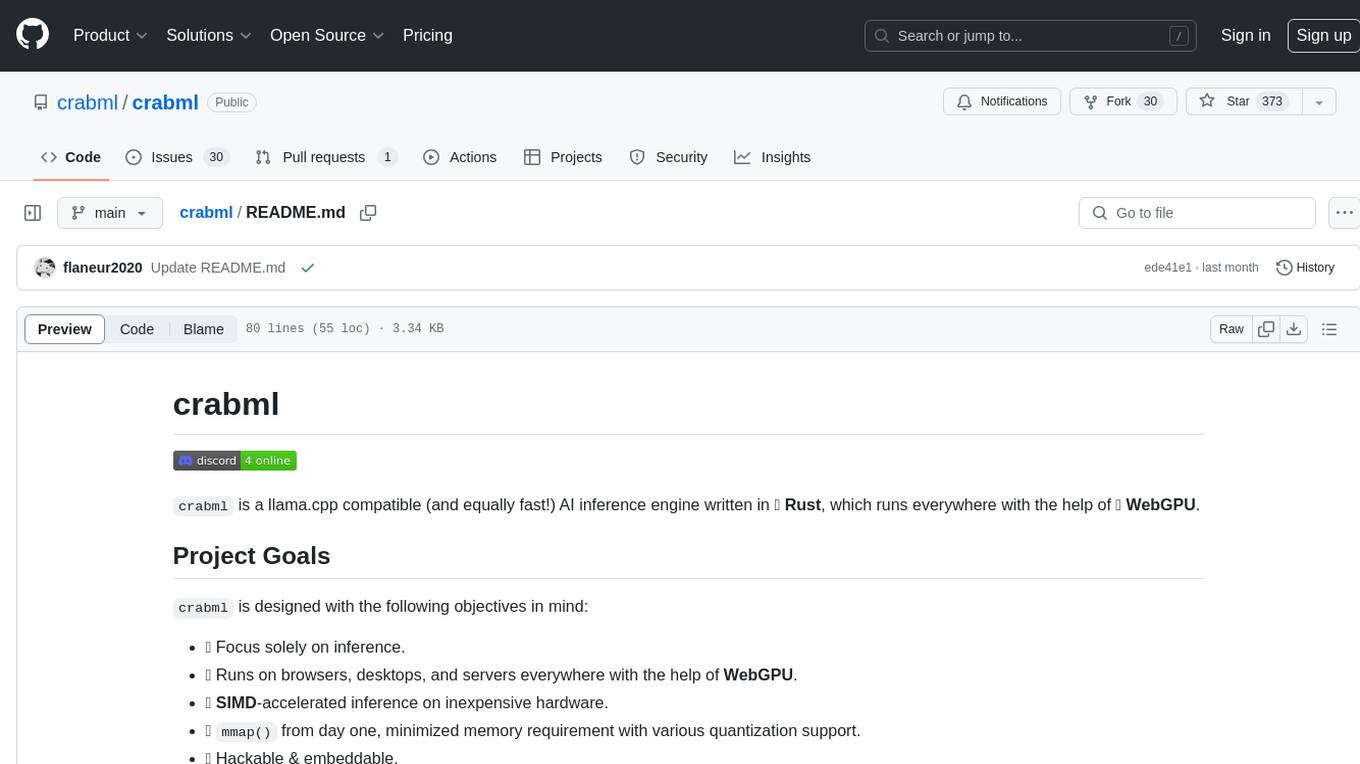
crabml
Crabml is a llama.cpp compatible AI inference engine written in Rust, designed for efficient inference on various platforms with WebGPU support. It focuses on running inference tasks with SIMD acceleration and minimal memory requirements, supporting multiple models and quantization methods. The project is hackable, embeddable, and aims to provide high-performance AI inference capabilities.
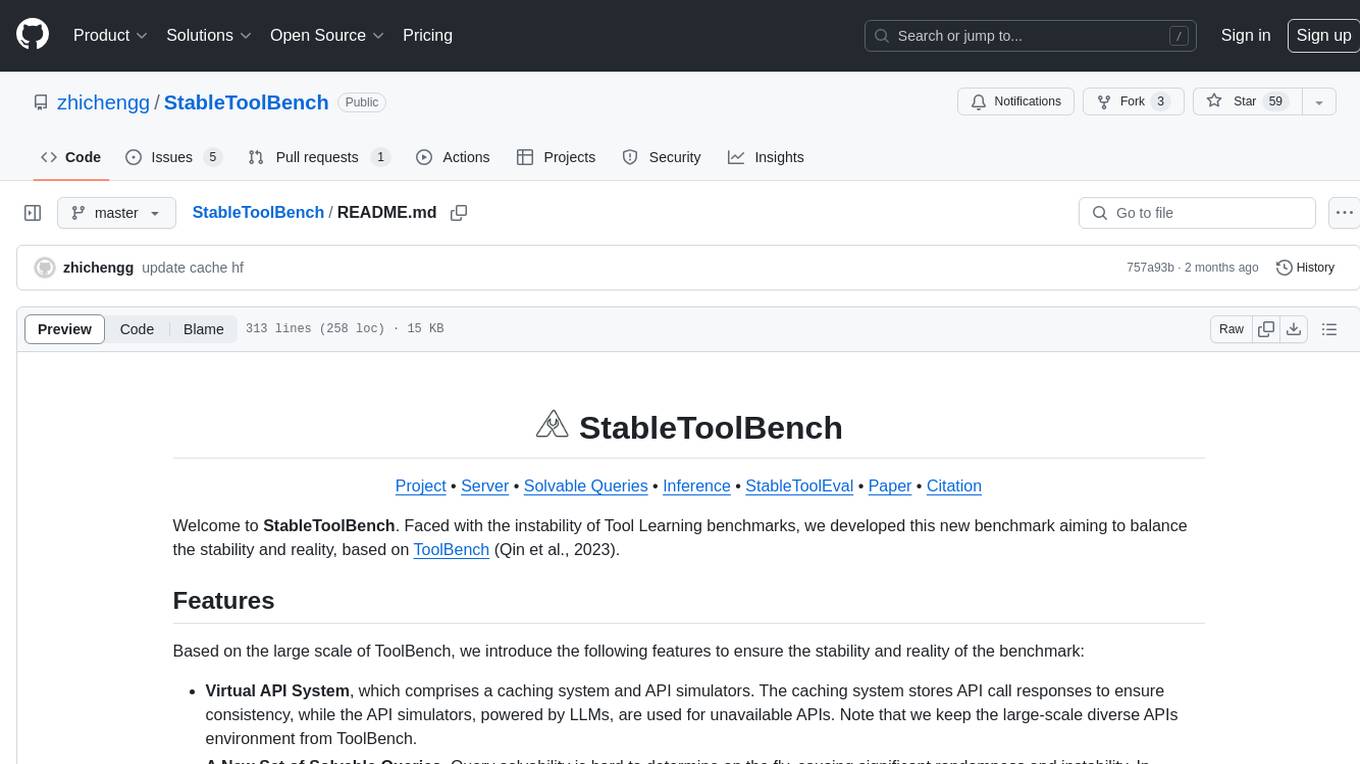
StableToolBench
StableToolBench is a new benchmark developed to address the instability of Tool Learning benchmarks. It aims to balance stability and reality by introducing features such as a Virtual API System with caching and API simulators, a new set of solvable queries determined by LLMs, and a Stable Evaluation System using GPT-4. The Virtual API Server can be set up either by building from source or using a prebuilt Docker image. Users can test the server using provided scripts and evaluate models with Solvable Pass Rate and Solvable Win Rate metrics. The tool also includes model experiments results comparing different models' performance.
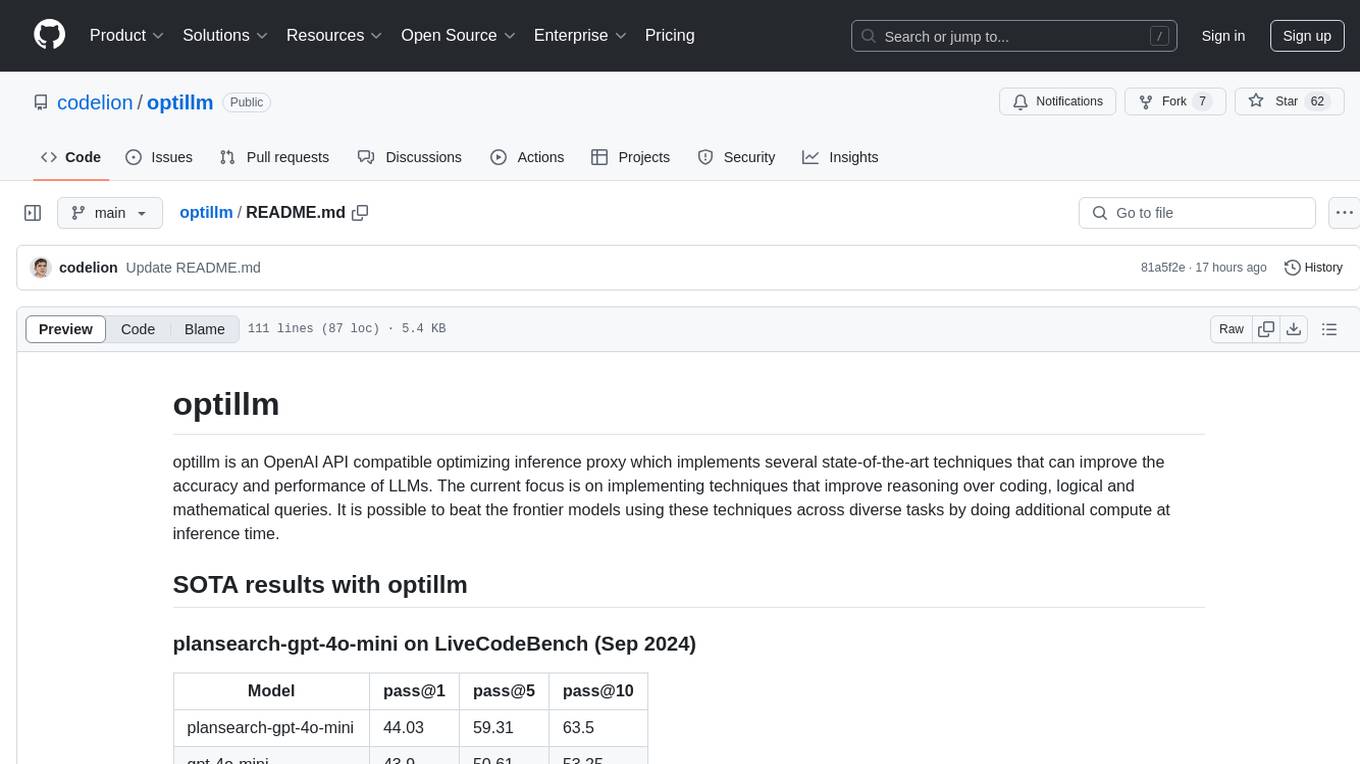
optillm
optillm is an OpenAI API compatible optimizing inference proxy implementing state-of-the-art techniques to enhance accuracy and performance of LLMs, focusing on reasoning over coding, logical, and mathematical queries. By leveraging additional compute at inference time, it surpasses frontier models across diverse tasks.
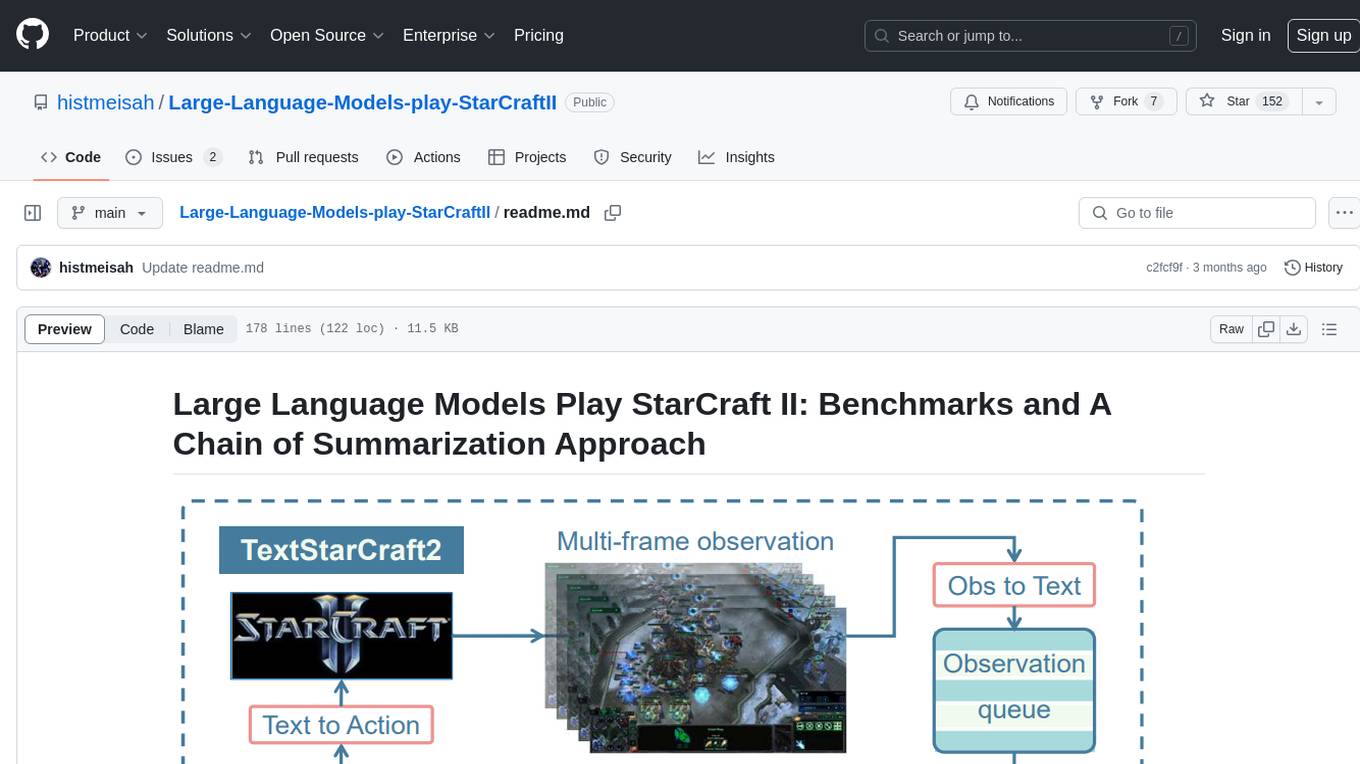
Large-Language-Models-play-StarCraftII
Large Language Models Play StarCraft II is a project that explores the capabilities of large language models (LLMs) in playing the game StarCraft II. The project introduces TextStarCraft II, a textual environment for the game, and a Chain of Summarization method for analyzing game information and making strategic decisions. Through experiments, the project demonstrates that LLM agents can defeat the built-in AI at a challenging difficulty level. The project provides benchmarks and a summarization approach to enhance strategic planning and interpretability in StarCraft II gameplay.
For similar tasks
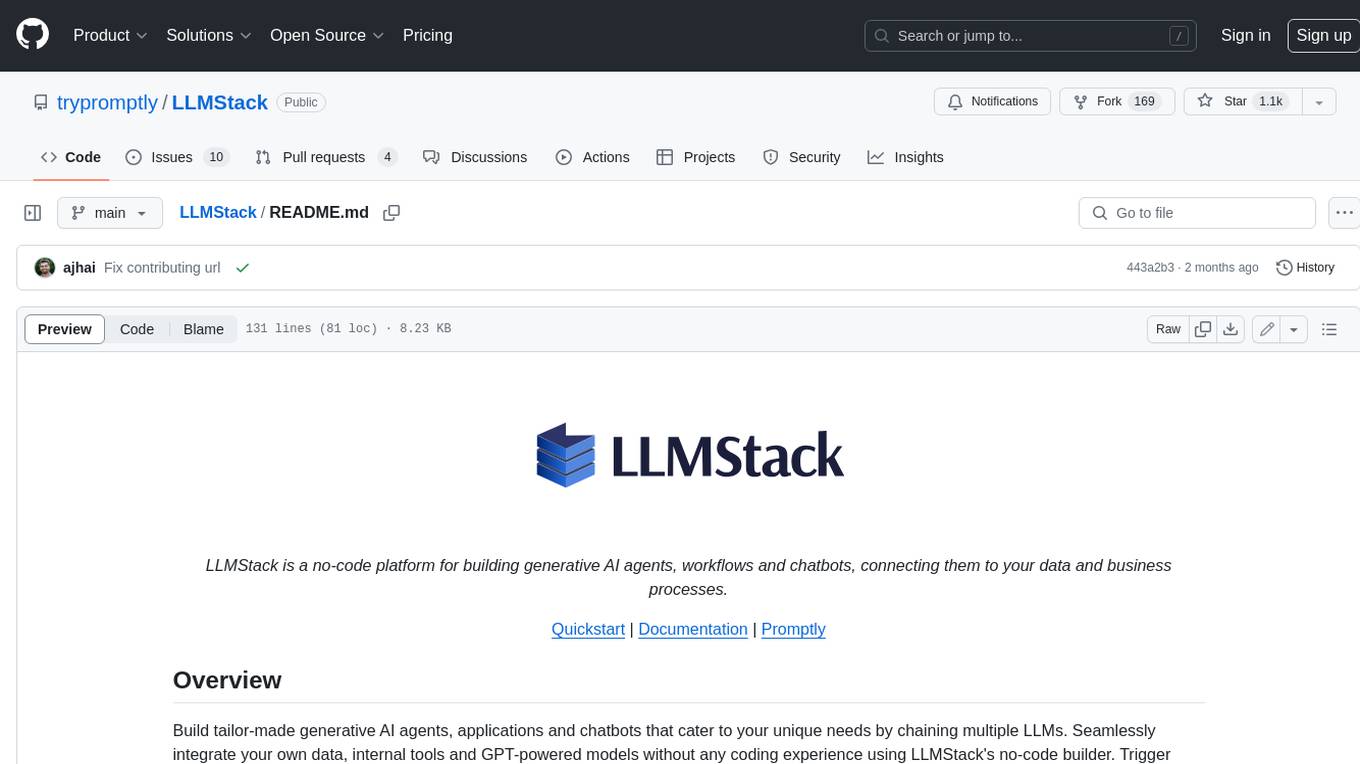
LLMStack
LLMStack is a no-code platform for building generative AI agents, workflows, and chatbots. It allows users to connect their own data, internal tools, and GPT-powered models without any coding experience. LLMStack can be deployed to the cloud or on-premise and can be accessed via HTTP API or triggered from Slack or Discord.
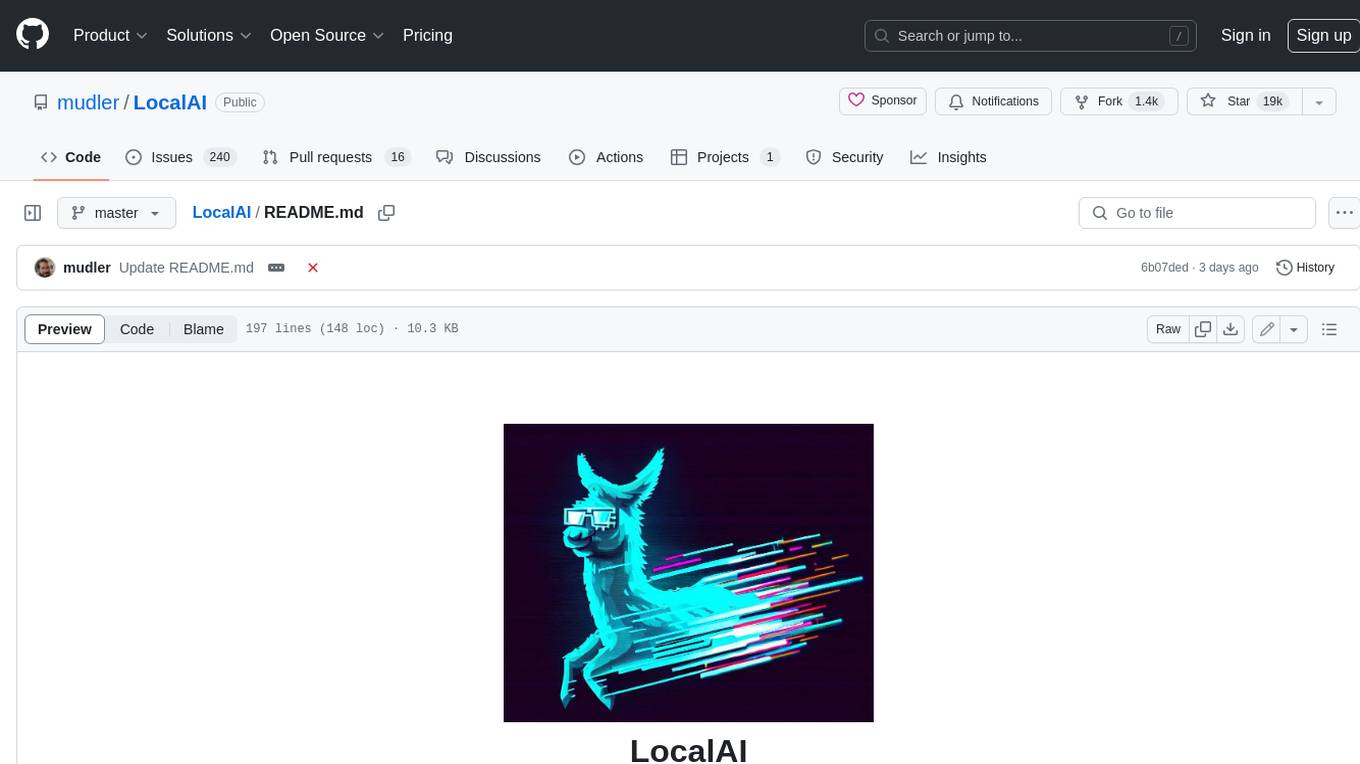
LocalAI
LocalAI is a free and open-source OpenAI alternative that acts as a drop-in replacement REST API compatible with OpenAI (Elevenlabs, Anthropic, etc.) API specifications for local AI inferencing. It allows users to run LLMs, generate images, audio, and more locally or on-premises with consumer-grade hardware, supporting multiple model families and not requiring a GPU. LocalAI offers features such as text generation with GPTs, text-to-audio, audio-to-text transcription, image generation with stable diffusion, OpenAI functions, embeddings generation for vector databases, constrained grammars, downloading models directly from Huggingface, and a Vision API. It provides a detailed step-by-step introduction in its Getting Started guide and supports community integrations such as custom containers, WebUIs, model galleries, and various bots for Discord, Slack, and Telegram. LocalAI also offers resources like an LLM fine-tuning guide, instructions for local building and Kubernetes installation, projects integrating LocalAI, and a how-tos section curated by the community. It encourages users to cite the repository when utilizing it in downstream projects and acknowledges the contributions of various software from the community.
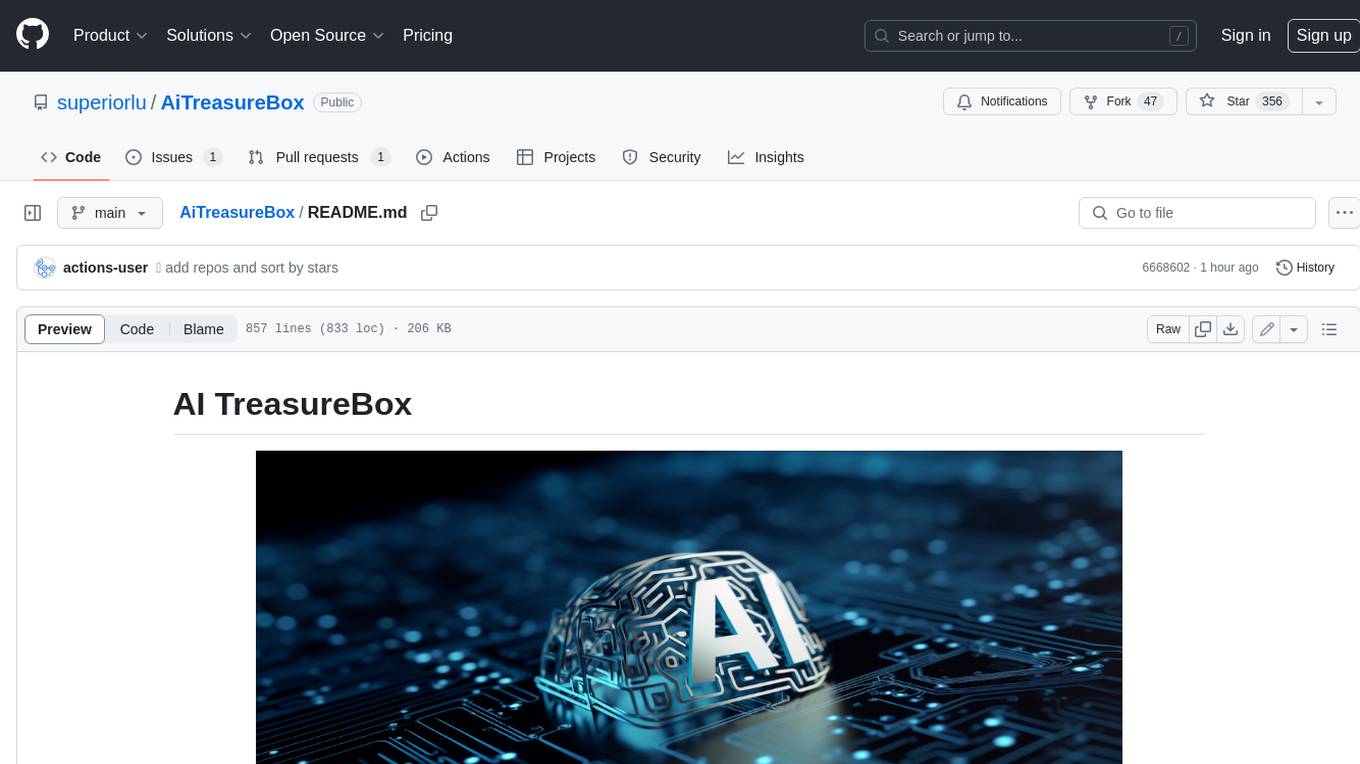
AiTreasureBox
AiTreasureBox is a versatile AI tool that provides a collection of pre-trained models and algorithms for various machine learning tasks. It simplifies the process of implementing AI solutions by offering ready-to-use components that can be easily integrated into projects. With AiTreasureBox, users can quickly prototype and deploy AI applications without the need for extensive knowledge in machine learning or deep learning. The tool covers a wide range of tasks such as image classification, text generation, sentiment analysis, object detection, and more. It is designed to be user-friendly and accessible to both beginners and experienced developers, making AI development more efficient and accessible to a wider audience.
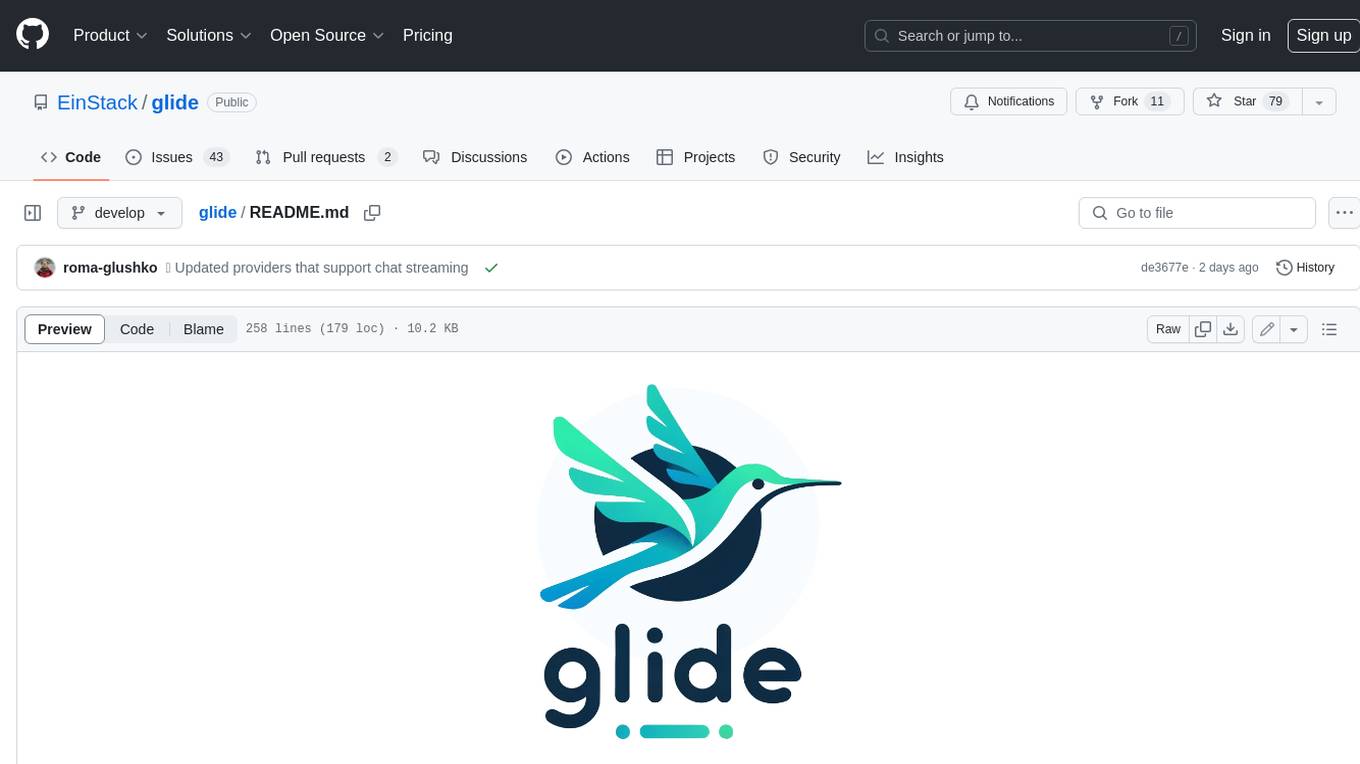
glide
Glide is a cloud-native LLM gateway that provides a unified REST API for accessing various large language models (LLMs) from different providers. It handles LLMOps tasks such as model failover, caching, key management, and more, making it easy to integrate LLMs into applications. Glide supports popular LLM providers like OpenAI, Anthropic, Azure OpenAI, AWS Bedrock (Titan), Cohere, Google Gemini, OctoML, and Ollama. It offers high availability, performance, and observability, and provides SDKs for Python and NodeJS to simplify integration.
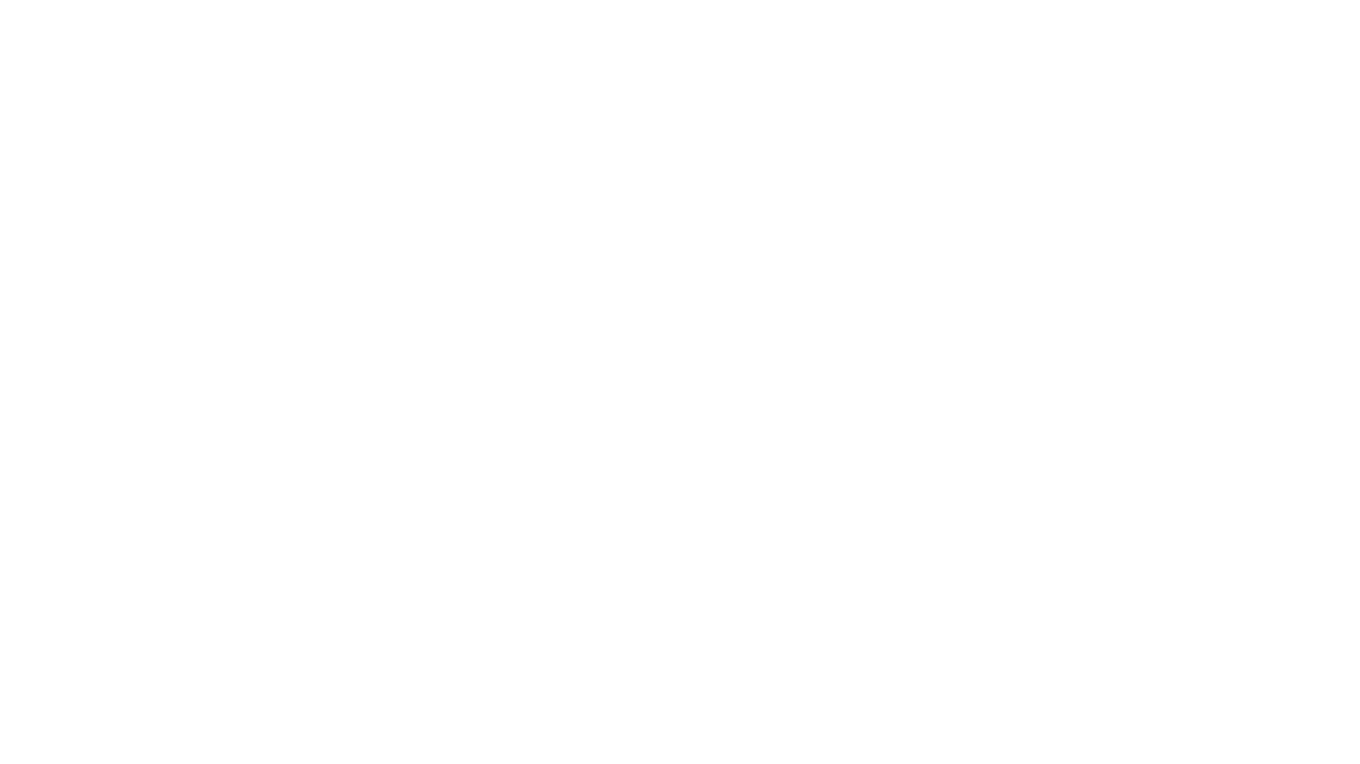
jupyter-ai
Jupyter AI connects generative AI with Jupyter notebooks. It provides a user-friendly and powerful way to explore generative AI models in notebooks and improve your productivity in JupyterLab and the Jupyter Notebook. Specifically, Jupyter AI offers: * An `%%ai` magic that turns the Jupyter notebook into a reproducible generative AI playground. This works anywhere the IPython kernel runs (JupyterLab, Jupyter Notebook, Google Colab, Kaggle, VSCode, etc.). * A native chat UI in JupyterLab that enables you to work with generative AI as a conversational assistant. * Support for a wide range of generative model providers, including AI21, Anthropic, AWS, Cohere, Gemini, Hugging Face, NVIDIA, and OpenAI. * Local model support through GPT4All, enabling use of generative AI models on consumer grade machines with ease and privacy.
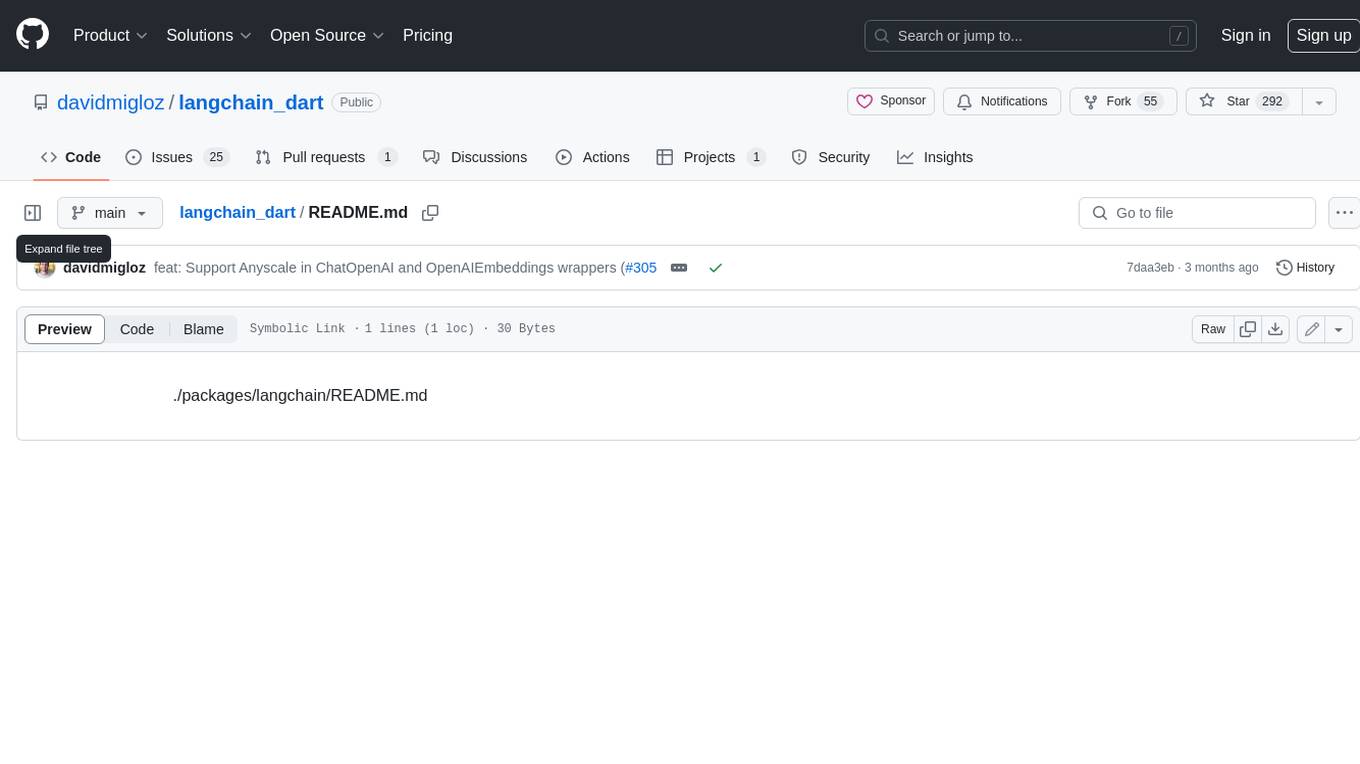
langchain_dart
LangChain.dart is a Dart port of the popular LangChain Python framework created by Harrison Chase. LangChain provides a set of ready-to-use components for working with language models and a standard interface for chaining them together to formulate more advanced use cases (e.g. chatbots, Q&A with RAG, agents, summarization, extraction, etc.). The components can be grouped into a few core modules: * **Model I/O:** LangChain offers a unified API for interacting with various LLM providers (e.g. OpenAI, Google, Mistral, Ollama, etc.), allowing developers to switch between them with ease. Additionally, it provides tools for managing model inputs (prompt templates and example selectors) and parsing the resulting model outputs (output parsers). * **Retrieval:** assists in loading user data (via document loaders), transforming it (with text splitters), extracting its meaning (using embedding models), storing (in vector stores) and retrieving it (through retrievers) so that it can be used to ground the model's responses (i.e. Retrieval-Augmented Generation or RAG). * **Agents:** "bots" that leverage LLMs to make informed decisions about which available tools (such as web search, calculators, database lookup, etc.) to use to accomplish the designated task. The different components can be composed together using the LangChain Expression Language (LCEL).
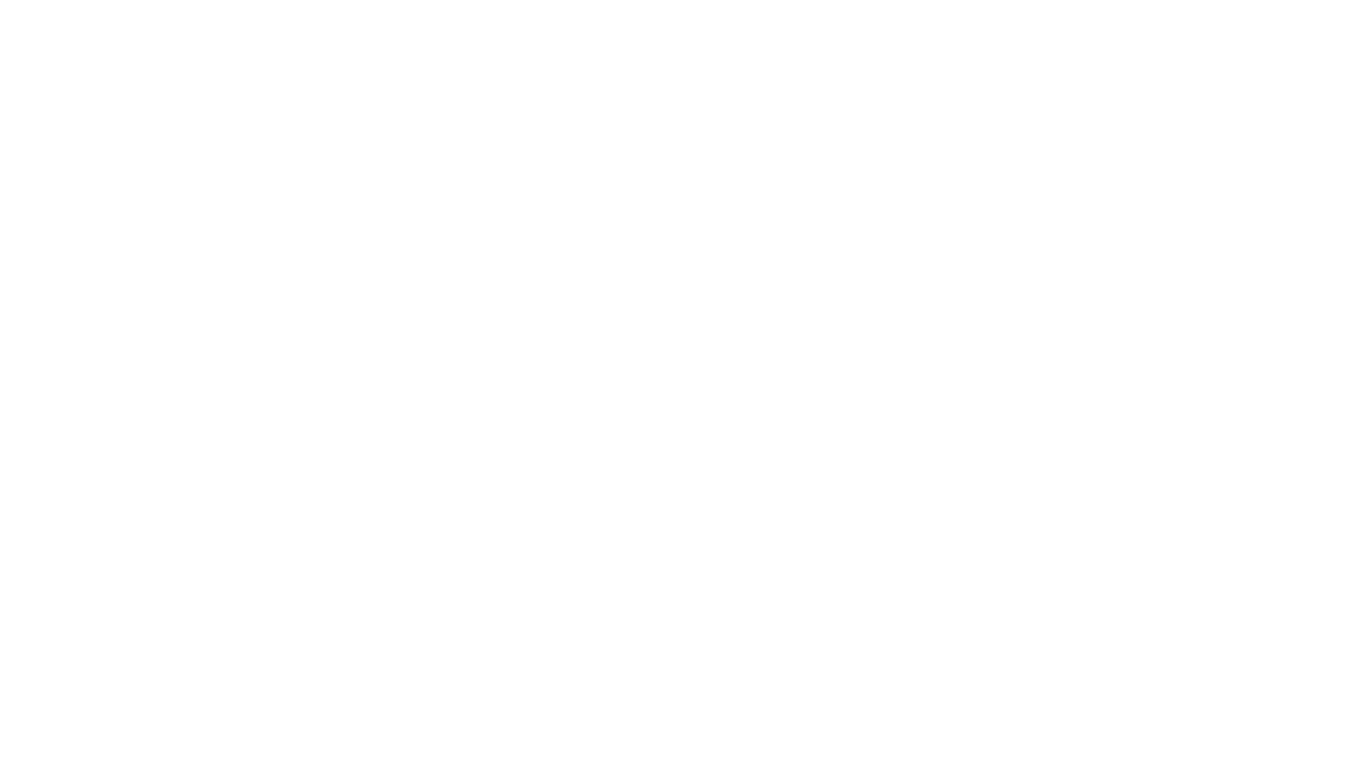
infinity
Infinity is an AI-native database designed for LLM applications, providing incredibly fast full-text and vector search capabilities. It supports a wide range of data types, including vectors, full-text, and structured data, and offers a fused search feature that combines multiple embeddings and full text. Infinity is easy to use, with an intuitive Python API and a single-binary architecture that simplifies deployment. It achieves high performance, with 0.1 milliseconds query latency on million-scale vector datasets and up to 15K QPS.
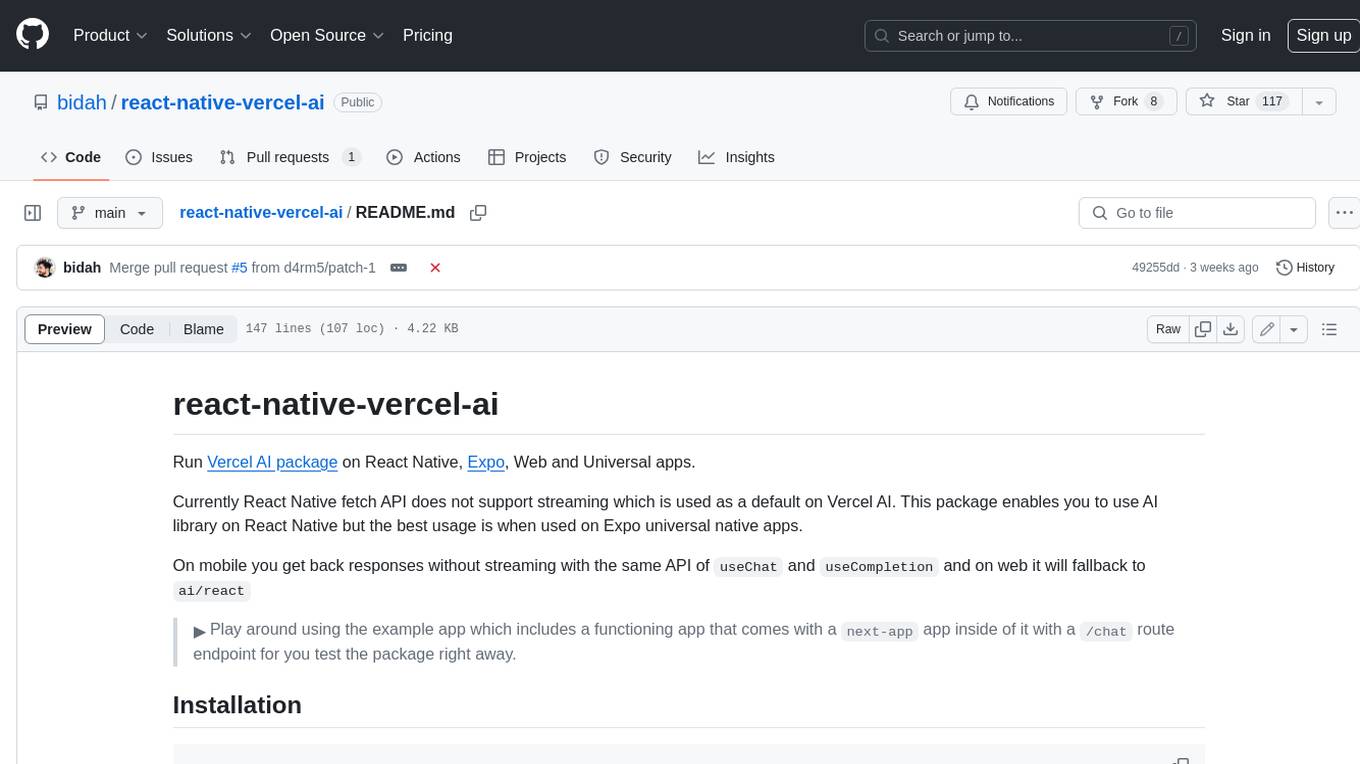
react-native-vercel-ai
Run Vercel AI package on React Native, Expo, Web and Universal apps. Currently React Native fetch API does not support streaming which is used as a default on Vercel AI. This package enables you to use AI library on React Native but the best usage is when used on Expo universal native apps. On mobile you get back responses without streaming with the same API of `useChat` and `useCompletion` and on web it will fallback to `ai/react`
For similar jobs
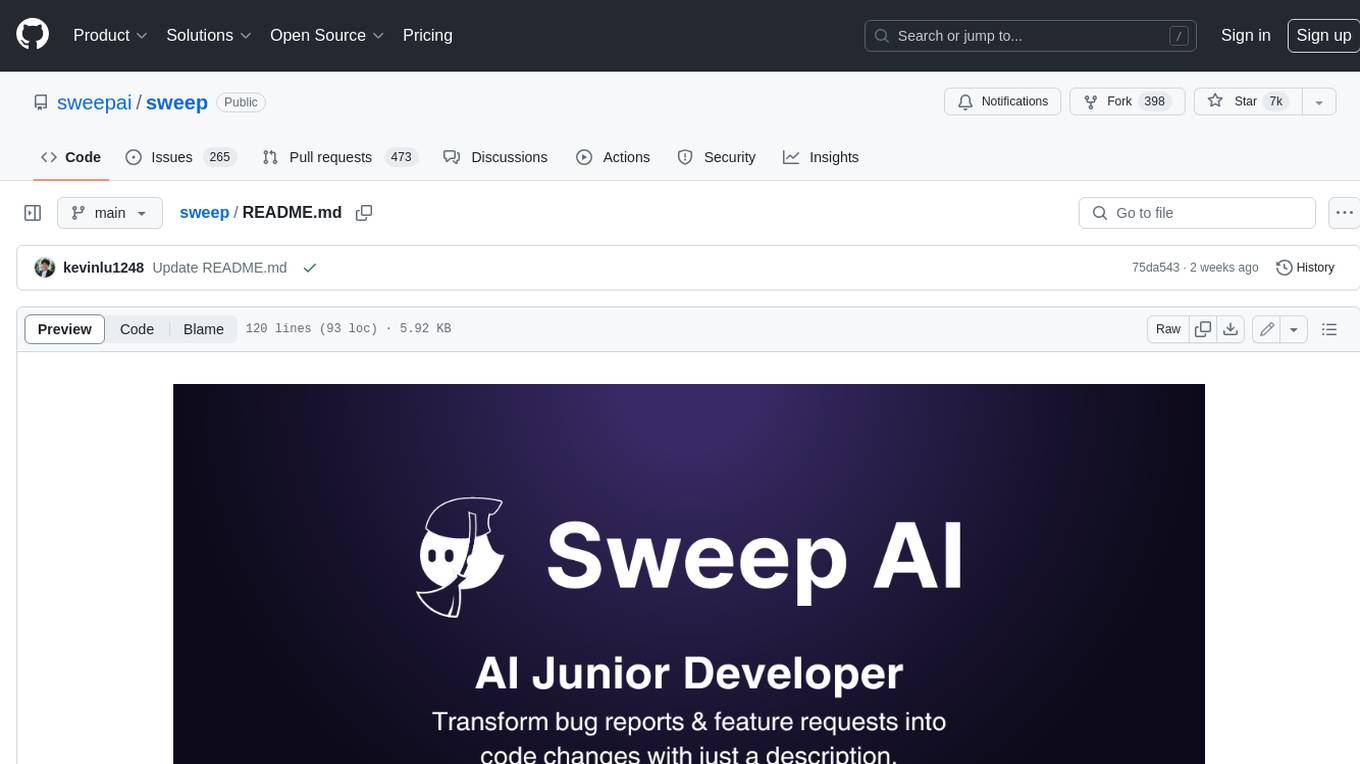
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
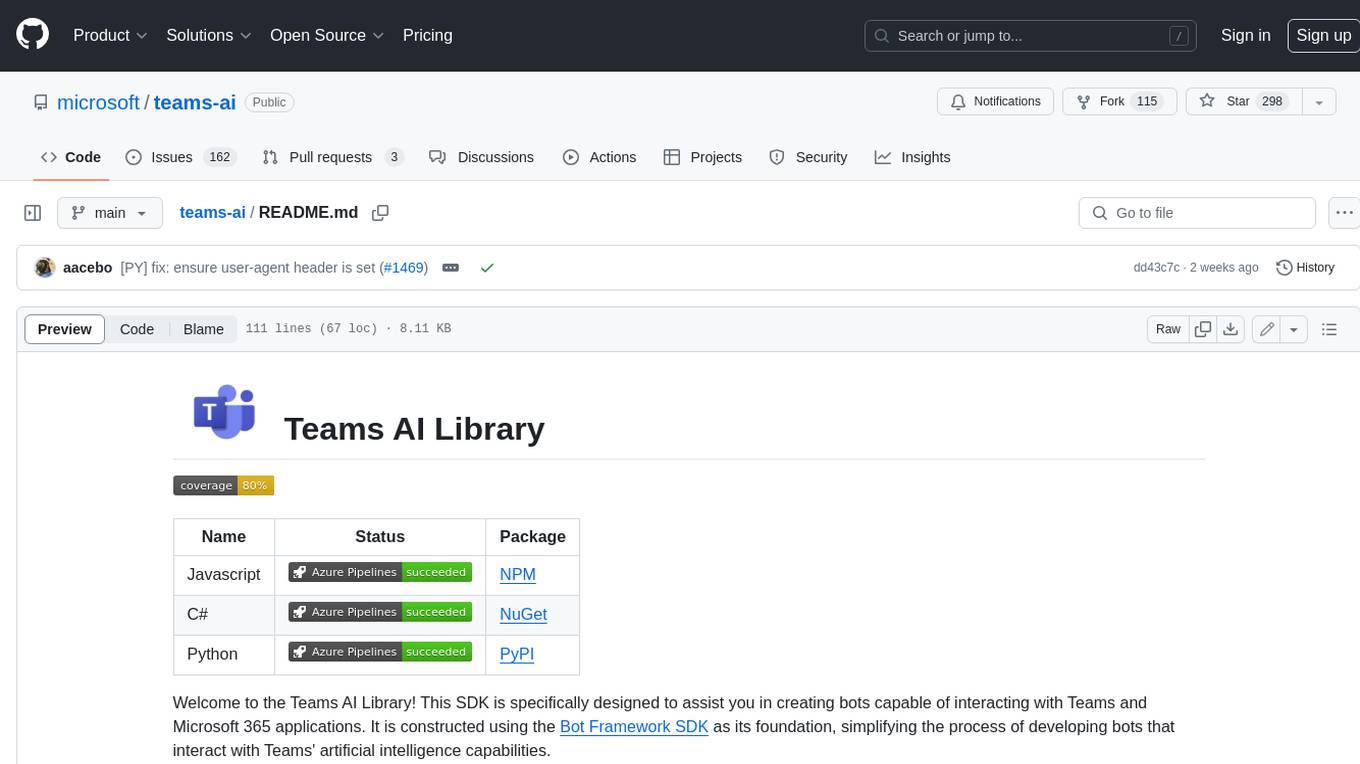
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
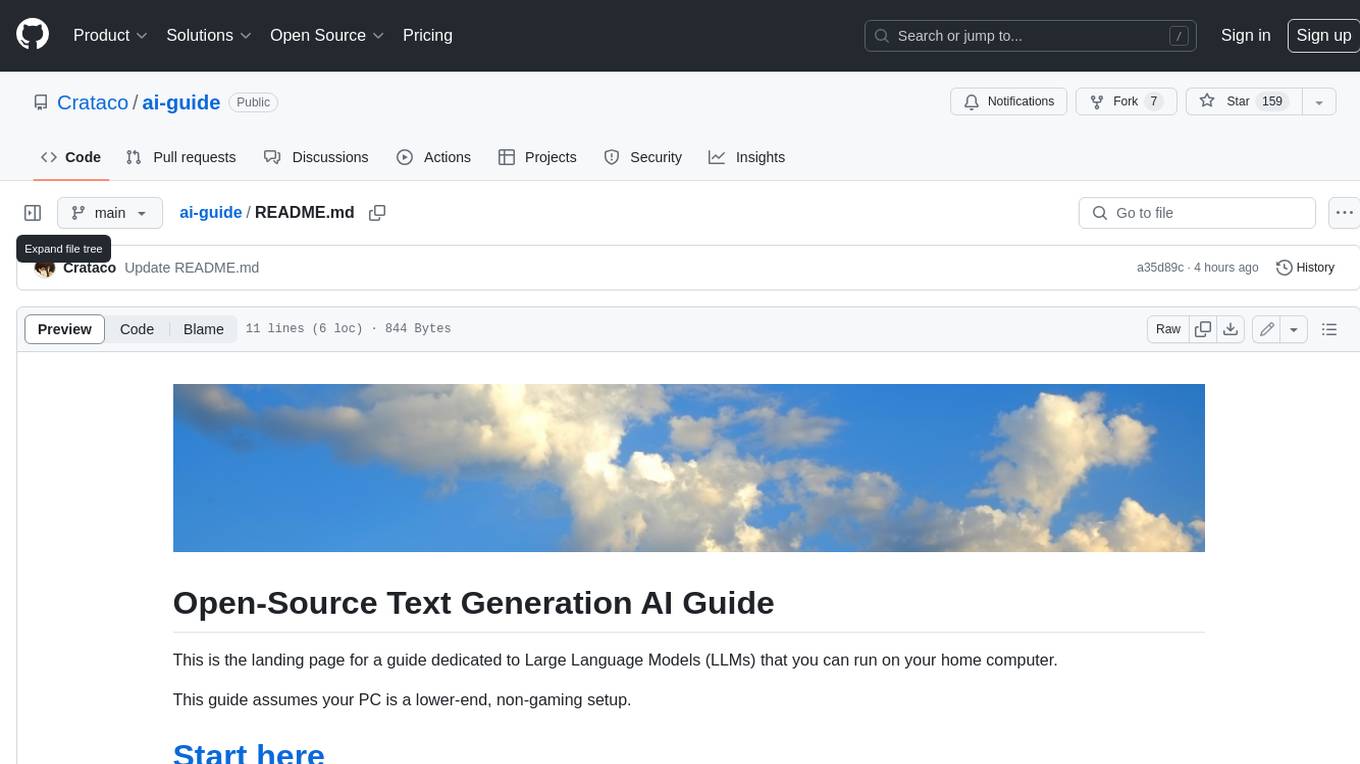
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
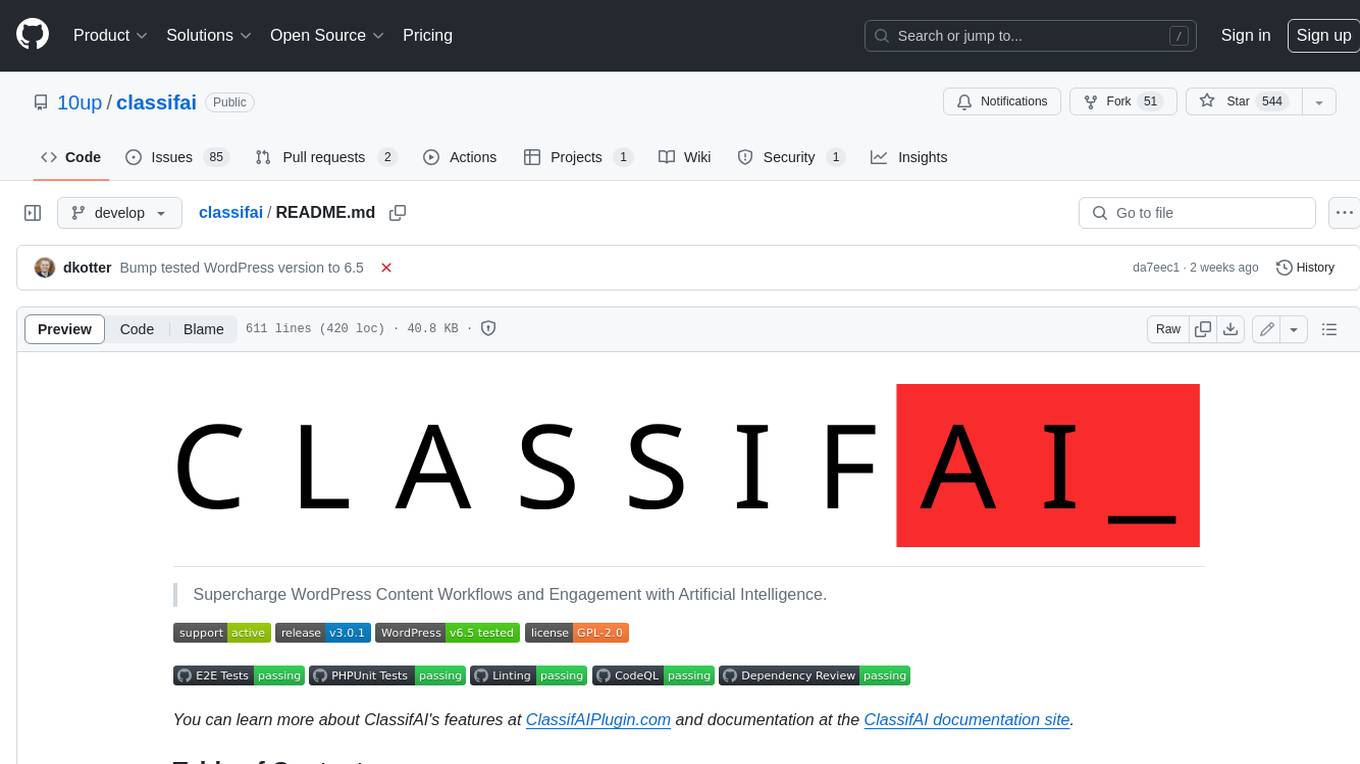
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
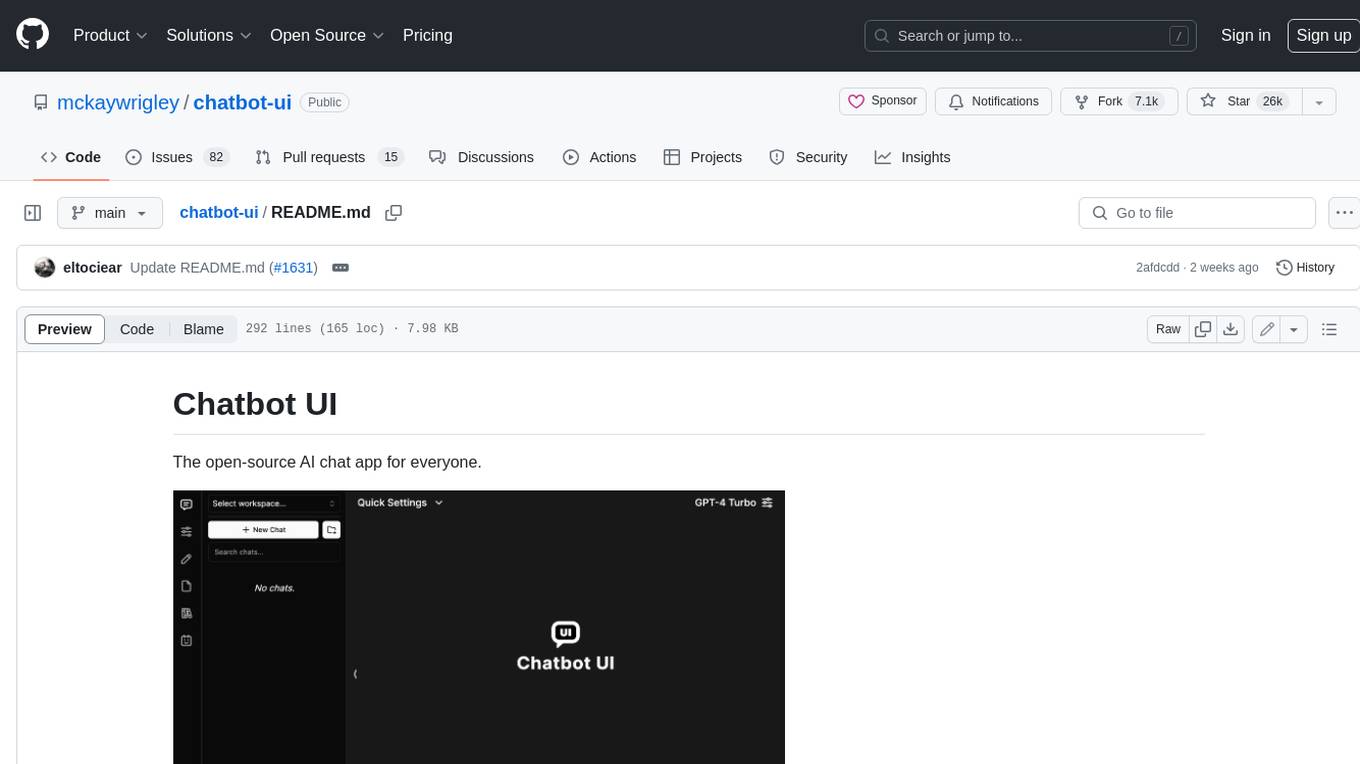
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
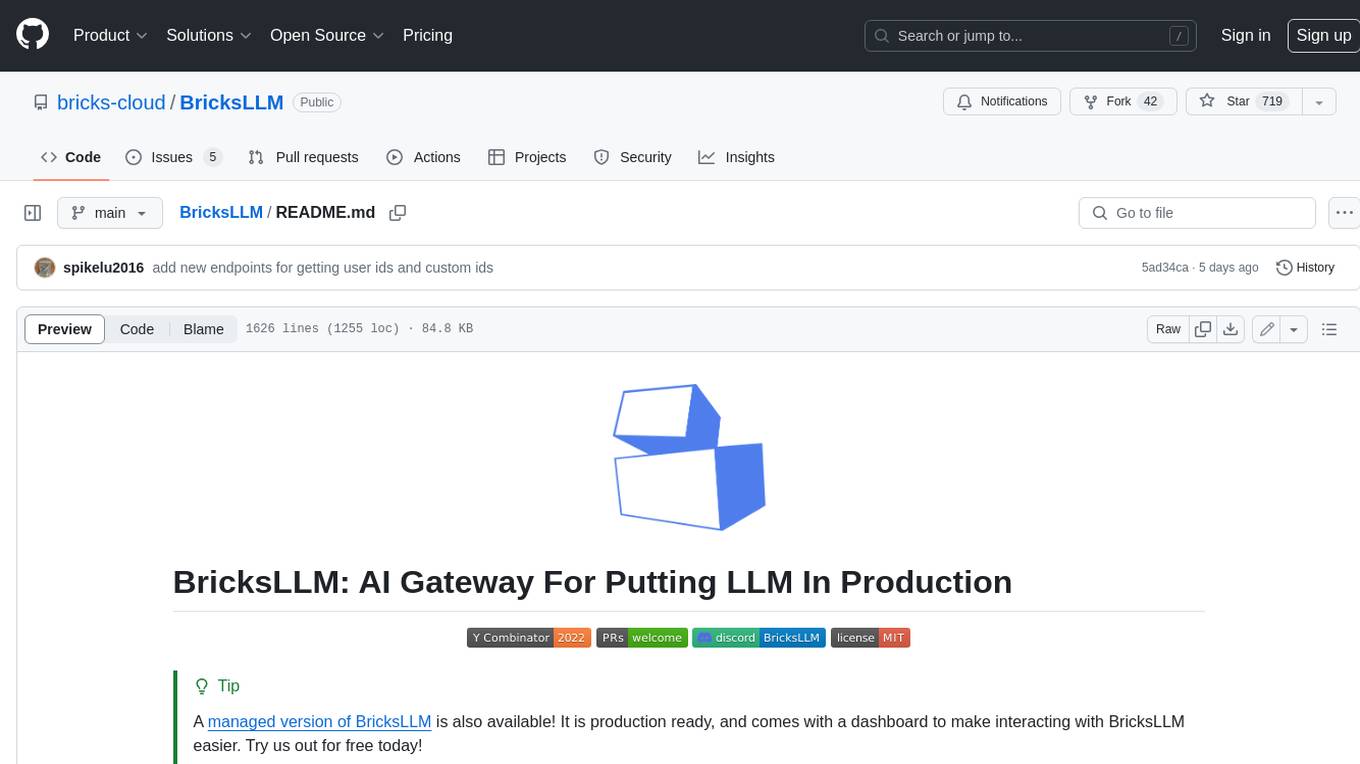
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
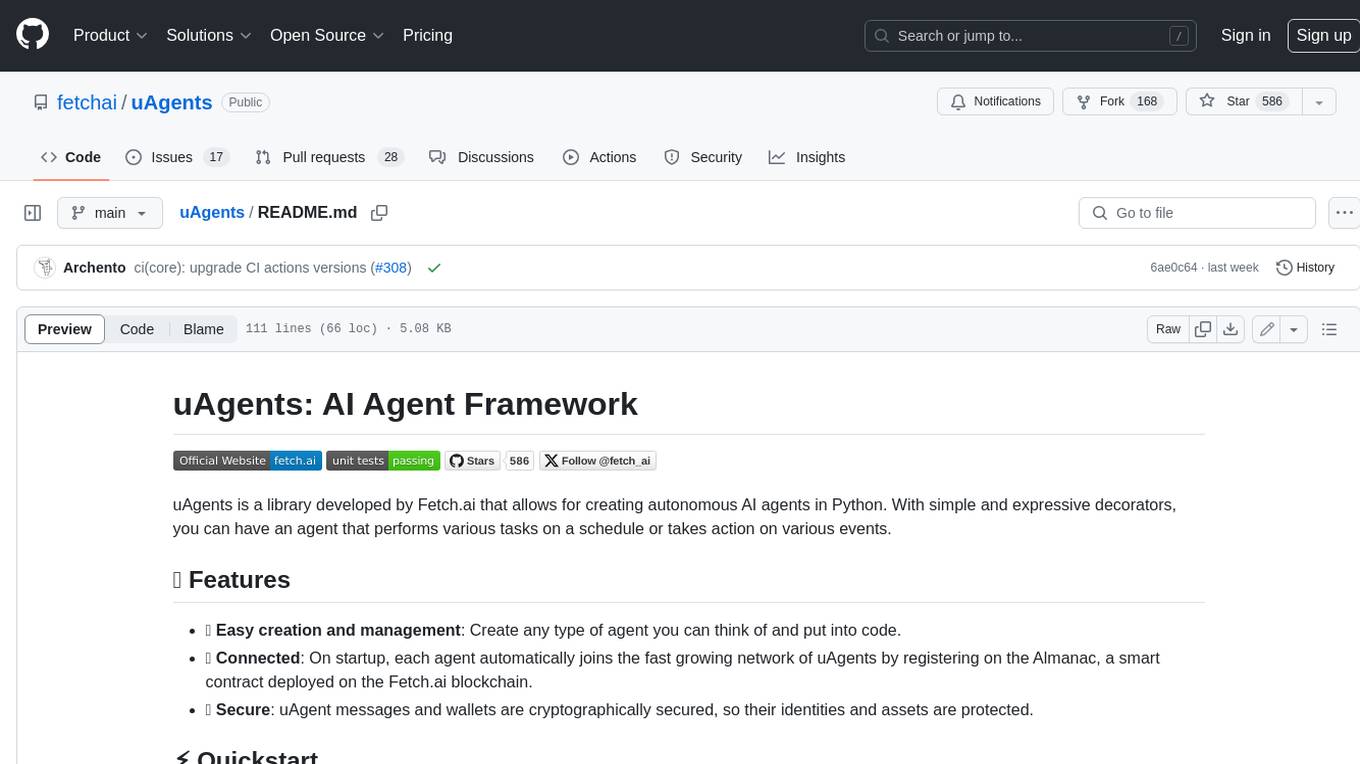
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
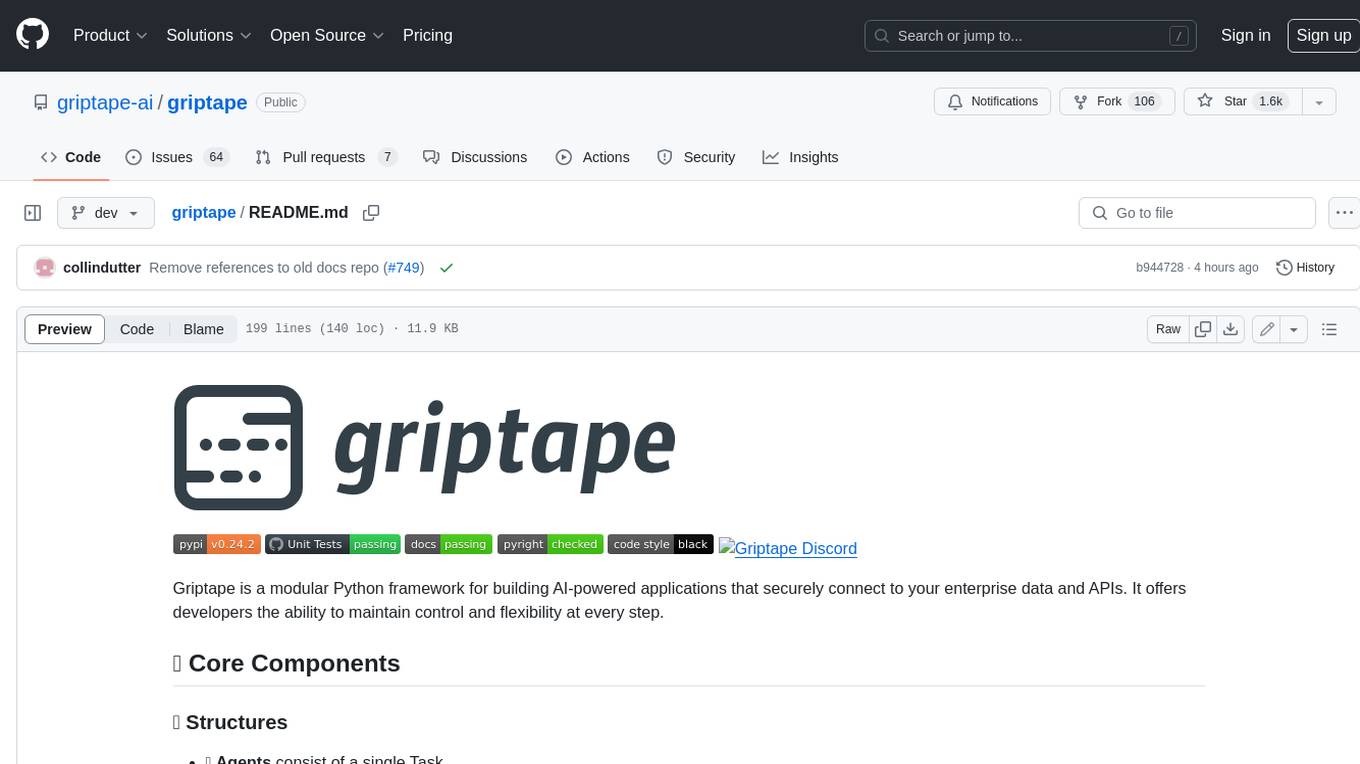
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.