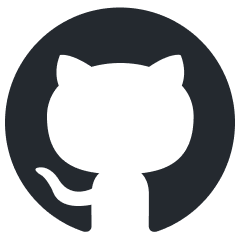
geti-sdk
Software Development Kit (SDK) for the Intel® Geti™ platform for Computer Vision AI model training.
Stars: 74
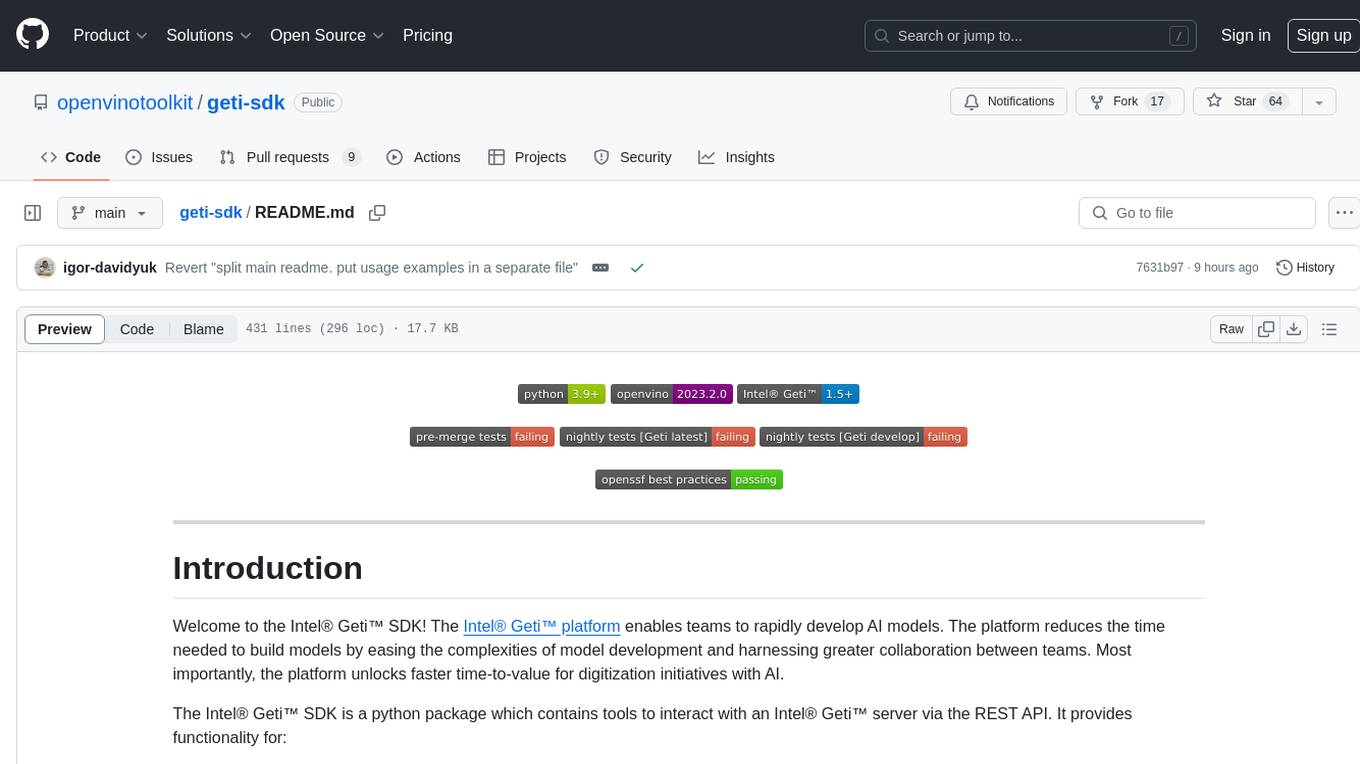
The Intel® Geti™ SDK is a python package that enables teams to rapidly develop AI models by easing the complexities of model development and enhancing collaboration between teams. It provides tools to interact with an Intel® Geti™ server via the REST API, allowing for project creation, downloading, uploading, deploying for local inference with OpenVINO, setting project and model configuration, launching and monitoring training jobs, and media upload and prediction. The SDK also includes tutorial-style Jupyter notebooks demonstrating its usage.
README:
Welcome to the Intel® Geti™ SDK! The Intel® Geti™ platform enables teams to rapidly develop AI models. The platform reduces the time needed to build models by easing the complexities of model development and harnessing greater collaboration between teams. Most importantly, the platform unlocks faster time-to-value for digitization initiatives with AI.
The Intel® Geti™ SDK is a python package which contains tools to interact with an Intel® Geti™ server via the REST API. It provides functionality for:
- Project creation from annotated datasets on disk
- Project downloading (images, videos, configuration, annotations, predictions and models)
- Project creation and upload from a previous download
- Deploying a project for local inference with OpenVINO
- Getting and setting project and model configuration
- Launching and monitoring training jobs
- Media upload and prediction
This repository also contains a set of (tutorial style) Jupyter notebooks that demonstrate how to use the SDK. We highly recommend checking them out to get a feeling for use cases for the package.
Using an environment manager such as miniforge or venv to create a new Python environment before installing the Intel® Geti™ SDK and its requirements is highly recommended.
NOTE: If you have installed multiple versions of Python, use
py -3.9 venv -m <env_name>
when creating your virtual environment to specify a supported version (in this case 3.9). Once you activate the virtual environment <venv_path>/Scripts/activate, make sure to upgrade pip to the latest versionpython -m pip install --upgrade pip wheel setuptools
.
Make sure to set up your environment using one of the supported Python versions for your operating system, as indicated in the table below.
Python <= 3.8 | Python 3.9 | Python 3.10 | Python 3.11 | Python 3.12 | |
---|---|---|---|---|---|
Linux | ❌ | ✔️ | ✔️ | ✔️ | ❌ |
Windows | ❌ | ✔️ | ✔️ | ✔️ | ❌ |
MacOS | ❌ | ✔️ | ✔️ | ✔️ | ❌ |
Once you have created and activated a new environment, follow the steps below to install the package.
Use pip install geti-sdk
to install the SDK from the Python Package Index (PyPI). To
install a specific version (for instance v1.5.0), use the command
pip install geti-sdk==1.5.0
-
Download or clone the repository and navigate to the root directory of the repo in your terminal.
-
Base installation Within this directory, install the SDK using
pip install .
This command will install the package and its base dependencies in your environment. -
Notebooks installation (Optional) If you want to be able to run the notebooks, make sure to install the extra requirements using
pip install .[notebooks]
This will install both the SDK and all other dependencies needed to run the notebooks in your environment -
Development installation (Optional) If you plan on running the tests or want to build the documentation, you can install the package extra requirements by doing for example
pip install -e .[dev]
The valid options for the extra requirements are
[dev, docs, notebooks]
, corresponding to the following functionality:-
dev
Install requirements to run the test suite on your local machine -
notebooks
Install requirements to run the Juypter notebooks in thenotebooks
folder in this repository. -
docs
Install requirements to build the documentation for the SDK from source on your machine
-
The SDK contains example code in various forms to help you get familiar with the package.
-
Code examples are short snippets that demonstrate how to perform several common tasks. This also shows how to configure the SDK to connect to your Intel® Geti™ server.
-
Jupyter notebooks are tutorial style notebooks that cover pretty much the full SDK functionality. These are the recommended way to get started with the SDK.
-
Example scripts are more extensive scripts that cover more advanced usage than the code examples, have a look at these if you don't like Jupyter.
The package provides a main class Geti
that can be used for the following use cases
To establish a connection between the SDK running on your local machine, and the
Intel® Geti™ platform running on a remote server, the Geti
class needs to know the
hostname or IP address for the server and it needs to have some form of authentication.
Instantiating the Geti
class will establish the connection and perform authentication.
-
Personal Access Token
The recommended authentication method is the 'Personal Access Token'. The token can be obtained by following the steps below:
- Open the Intel® Geti™ user interface in your browser
- Click on the
User
menu, in the top right corner of the page. The menu is accessible from any page inside the Intel® Geti™ interface. - In the dropdown menu that follows, click on
Personal access token
, as shown in the image below. - In the screen that follows, go through the steps to create a token.
- Make sure to copy the token value!
Once you created a personal access token, it can be passed to the
Geti
class as follows:from geti_sdk import Geti geti = Geti( host="https://your_server_hostname_or_ip_address", token="your_personal_access_token" )
-
User Credentials
NOTE: For optimal security, using the token method outlined above is recommended.
In addition to the token, your username and password can also be used to connect to the server. They can be passed as follows:
from geti_sdk import Geti geti = Geti( host="https://your_server_hostname_or_ip_address", username="dummy_user", password="dummy_password" )
Here,
"dummy_user"
and"dummy_password"
should be replaced by your username and password for the Geti server. -
SSL certificate validation
By default, the SDK verifies the SSL certificate of your server before establishing a connection over HTTPS. If the certificate can't be validated, this will results in an error and the SDK will not be able to connect to the server.
However, this may not be appropriate or desirable in all cases, for instance if your Geti server does not have a certificate because you are running it in a private network environment. In that case, certificate validation can be disabled by passing
verify_certificate=False
to theGeti
constructor. Please only disable certificate validation in a secure environment!
-
Project download The following python snippet is a minimal example of how to download a project using
Geti
:from geti_sdk import Geti geti = Geti( host="https://your_server_hostname_or_ip_address", token="your_personal_access_token" ) geti.download_project_data(project_name="dummy_project")
Here, it is assumed that the project with name 'dummy_project' exists on the cluster. The
Geti
instance will create a folder named 'dummy_project' in your current working directory, and download the project parameters, images, videos, annotations, predictions and the active model for the project (including optimized models derived from it) to that folder.The method takes the following optional parameters:
-
target_folder
-- Can be specified to change the directory to which the project data is saved. -
include_predictions
-- Set to True to download the predictions for all images and videos in the project. Set to False to not download any predictions. -
include_active_model
-- Set to True to download the active model for the project, and any optimized models derived from it. If set to False, no models are downloaded. False by default.
NOTE: During project downloading the Geti SDK stores data on local disk. If necessary, please apply additional security control to protect downloaded files (e.g., enforce access control, delete sensitive data securely).
-
-
Project upload The following python snippet is a minimal example of how to re-create a project on an Intel® Geti™ server using the data from a previously downloaded project:
from geti_sdk import Geti geti = Geti( host="https://your_server_hostname_or_ip_address", token="your_personal_access_token" ) geti.upload_project_data(target_folder="dummy_project")
The parameter
target_folder
must be a valid path to the directory holding the project data. If you want to create the project using a different name than the original project, you can pass an additional parameterproject_name
to the upload method.
The Geti
instance can be used to either back-up a project (by downloading it and later
uploading it again to the same cluster), or to migrate a project to a different cluster
(download it, and upload it to the target cluster).
To up- or download all projects from a cluster, simply use the
geti.download_all_projects
and geti.upload_all_projects
methods instead of
the single project methods in the code snippets above.
The following code snippet shows how to create a deployment for local inference with OpenVINO:
import cv2
from geti_sdk import Geti
geti = Geti(
host="https://your_server_hostname_or_ip_address", token="your_personal_access_token"
)
# Download the model data and create a `Deployment`
deployment = geti.deploy_project(project_name="dummy_project")
# Load the inference models for all tasks in the project, for CPU inference
deployment.load_inference_models(device='CPU')
# Run inference
dummy_image = cv2.imread('dummy_image.png')
prediction = deployment.infer(image=dummy_image)
# Save the deployment to disk
deployment.save(path_to_folder="dummy_project")
The deployment.infer
method takes a numpy image as input.
The deployment.save
method will save the deployment to the folder named
'dummy_project', on the local disk. The deployment can be reloaded again later using
Deployment.from_folder('dummy_project')
.
The examples
folder contains example scripts, showing various use cases for the package. They can
be run by navigating to the examples
directory in your terminal, and simply running
the scripts like any other python script.
In addition, the notebooks
folder contains Jupyter notebooks with example use cases for the geti_sdk
. To run
the notebooks, make sure that the requirements for the notebooks are installed in your
Python environment. If you have not installed these when you were installing the SDK,
you can install them at any time using
pip install -r requirements/requirements-notebooks.txt
Once the notebook requirements are installed, navigate to the notebooks
directory in
your terminal. Then, launch JupyterLab by typing jupyter lab
. This should open your
browser and take you to the JupyterLab landing page, with the SDK notebooks open (see
the screenshot below).
NOTE: Both the example scripts and the notebooks require access to a server running the Intel® Geti™ platform.
The Geti
class provides the following methods:
-
download_project_data
-- Downloads a project by project name (Geti-SDK representation), returns an interactive object. -
upload_project_data
-- Uploads project (Geti-SDK representation) from a folder. -
download_all_projects
-- Downloads all projects found on the server. -
upload_all_projects
-- Uploads all projects found in a specified folder to the server. -
export_project
-- Exports a project to an archive on disk. This method is useful for creating a backup of a project, or for migrating a project to a different cluster. -
import_project
-- Imports a project from an archive on disk. This method is useful for restoring a project from a backup, or for migrating a project to a different cluster. -
export_dataset
-- Exports a dataset to an archive on disk. This method is useful for creating a backup of a dataset, or for migrating a dataset to a different cluster. -
import_dataset
-- Imports a dataset from an archive on disk. A new project will be created for the dataset. This method is useful for restoring a project from a dataset backup, or for migrating a dataset to a different cluster. -
upload_and_predict_image
-- Uploads a single image to an existing project on the server, and requests a prediction for that image. Optionally, the prediction can be visualized as an overlay on the image. -
upload_and_predict_video
-- Uploads a single video to an existing project on the server, and requests predictions for the frames in the video. As with upload_and_predict_image, the predictions can be visualized on the frames. The parameterframe_stride
can be used to control which frames are extracted for prediction. -
upload_and_predict_media_folder
-- Uploads all media (images and videos) from a folder on local disk to an existing project on the server, and download predictions for all uploaded media. -
deploy_project
-- Downloads the active model for all tasks in the project as an OpenVINO inference model. The resultingDeployment
can be used to run inference for the project on a local machine. Pipeline inference is also supported. -
create_project_single_task_from_dataset
-- Creates a single task project on the server, potentially using labels and uploading annotations from an external dataset. -
create_task_chain_project_from_dataset
-- Creates a task chain project on the server, potentially using labels and uploading annotations from an external dataset.
For further details regarding these methods, please refer to the method documentation, the code snippets, and example scripts provided in this repo.
Please visit the full documentation for a complete API reference.
The Dockerfile can be used to run the package without having to install python on your machine.
First build the docker image
docker build -t geti-sdk .
then run it using,
docker run --rm -ti -v $(pwd):/app geti-sdk:latest /bin/bash
-
Creating projects. You can pass a variable
project_type
to control what kind of tasks will be created in the project pipeline. For example, if you want to create a single task segmentation project, you'd passproject_type='segmentation'
. For a detection -> segmentation task chain, you can passproject_type=detection_to_segmentation
. Please see the scripts in theexamples
folder for examples on how to do this. -
Creating datasets and retrieving dataset statistics.
-
Uploading images, videos, annotations for images and video frames and configurations to a project.
-
Downloading images, videos, annotations, models and predictions for all images and videos/video frames in a project. Also downloading the full project configuration is supported.
-
Setting configuration for a project, like turning auto train on/off and setting number of iterations for all tasks.
-
Deploying a project to load OpenVINO inference models for all tasks in the pipeline, and running the full pipeline inference on a local machine.
-
Creating and restoring a backup of an existing project, using the code snippets provided above. Only annotations, media and configurations are backed up, models are not.
-
Launching and monitoring training jobs is straightforward with the
TrainingClient
. Please refer to the notebook007_train_project
for instructions. -
Authorization via Personal Access Token is available for both On-Prem and SaaS users.
-
Fetching the active dataset
-
Triggering (post-training) model optimization for model quantization and changing models precision.
-
Running model tests
-
Benchmarking models to measure inference throughput on different hardware. It allows for quick and easy comparison of inference framerates for different model architectures and precision levels for the specified project.
- Model upload
- Prediction upload
- Importing datasets to an existing project: For this, you can use the import functionality from the Intel® Geti™ user interface instead.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for geti-sdk
Similar Open Source Tools
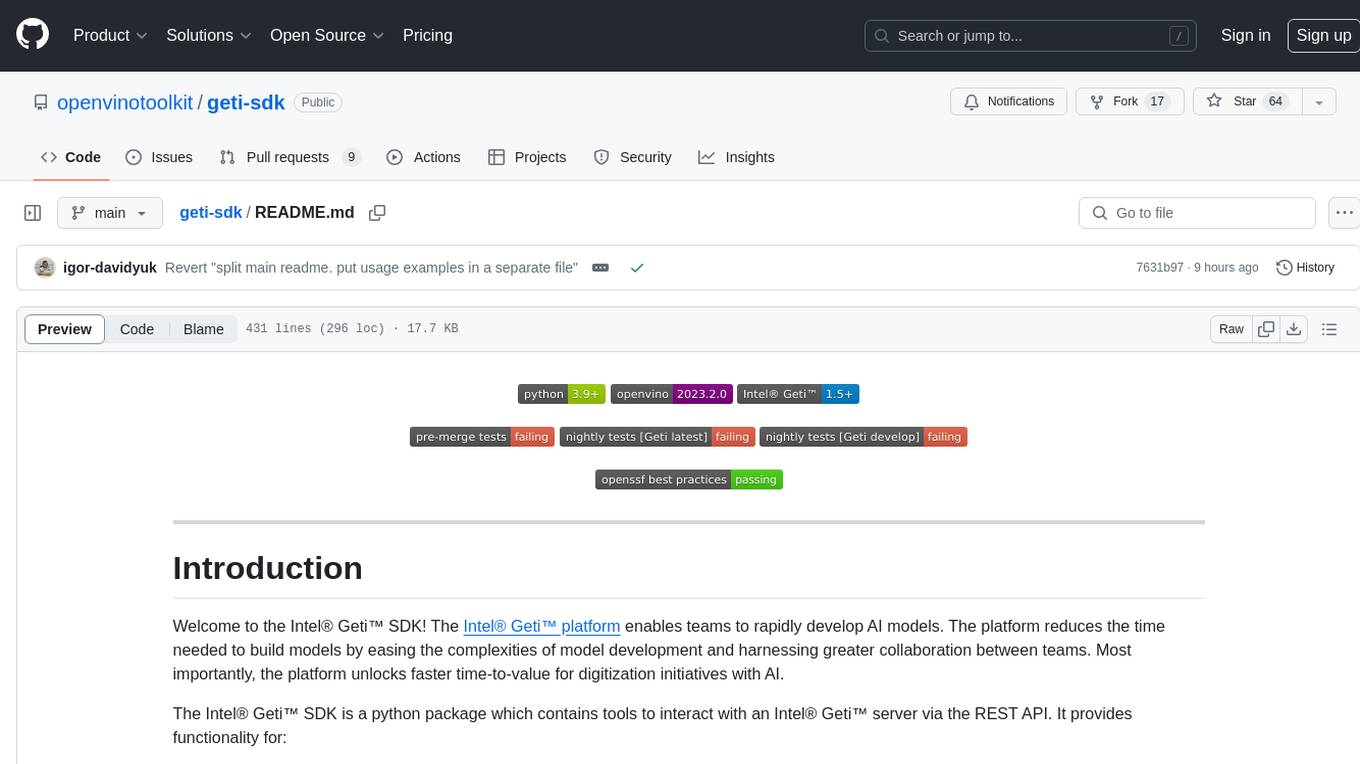
geti-sdk
The Intel® Geti™ SDK is a python package that enables teams to rapidly develop AI models by easing the complexities of model development and enhancing collaboration between teams. It provides tools to interact with an Intel® Geti™ server via the REST API, allowing for project creation, downloading, uploading, deploying for local inference with OpenVINO, setting project and model configuration, launching and monitoring training jobs, and media upload and prediction. The SDK also includes tutorial-style Jupyter notebooks demonstrating its usage.
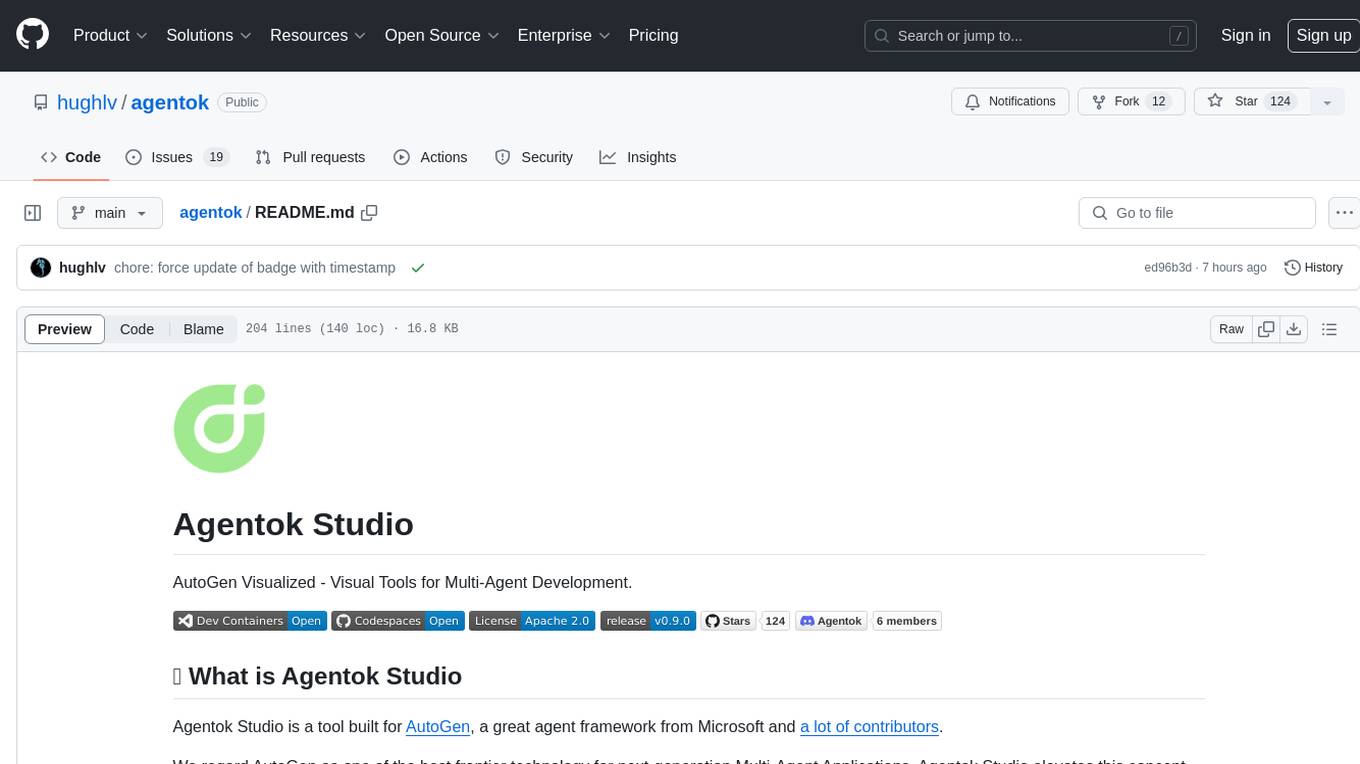
agentok
Agentok Studio is a visual tool built for AutoGen, a cutting-edge agent framework from Microsoft and various contributors. It offers intuitive visual tools to simplify the construction and management of complex agent-based workflows. Users can create workflows visually as graphs, chat with agents, and share flow templates. The tool is designed to streamline the development process for creators and developers working on next-generation Multi-Agent Applications.
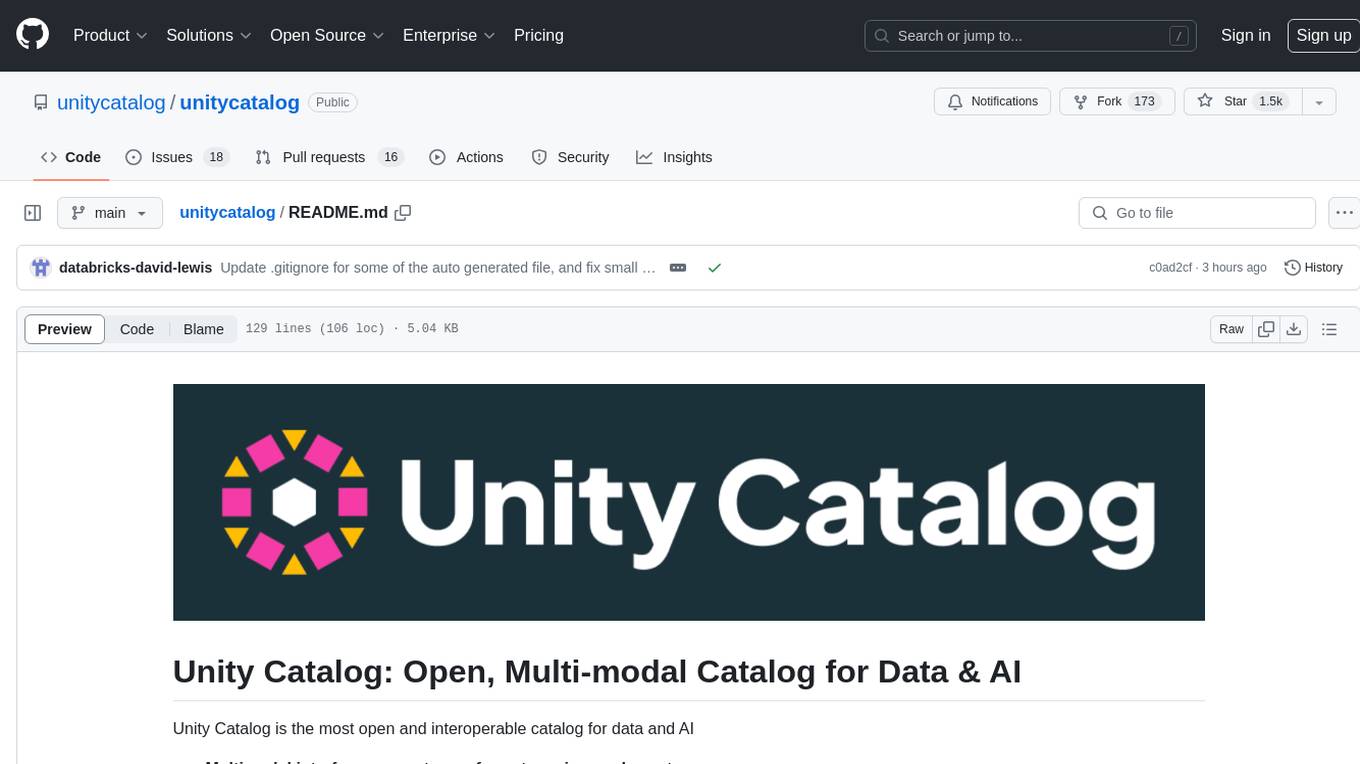
unitycatalog
Unity Catalog is an open and interoperable catalog for data and AI, supporting multi-format tables, unstructured data, and AI assets. It offers plugin support for extensibility and interoperates with Delta Sharing protocol. The catalog is fully open with OpenAPI spec and OSS implementation, providing unified governance for data and AI with asset-level access control enforced through REST APIs.
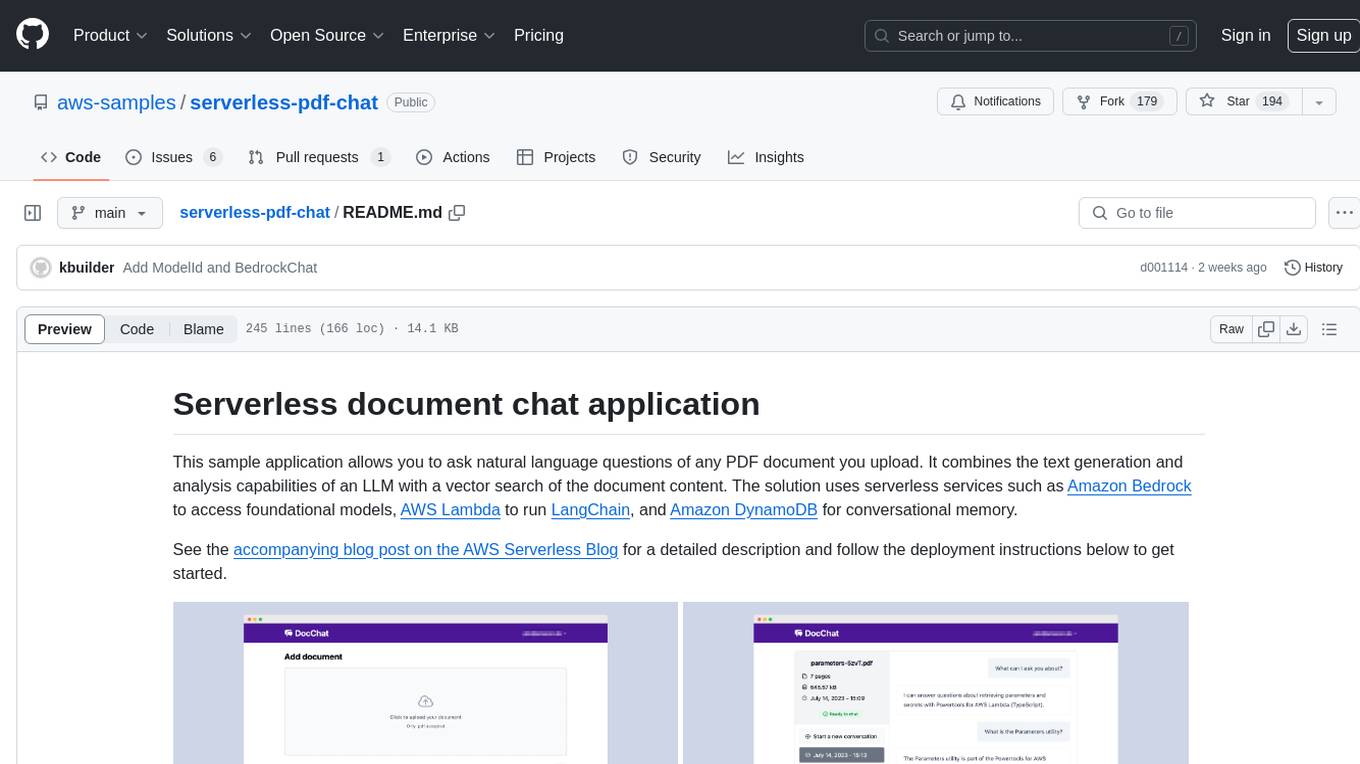
serverless-pdf-chat
The serverless-pdf-chat repository contains a sample application that allows users to ask natural language questions of any PDF document they upload. It leverages serverless services like Amazon Bedrock, AWS Lambda, and Amazon DynamoDB to provide text generation and analysis capabilities. The application architecture involves uploading a PDF document to an S3 bucket, extracting metadata, converting text to vectors, and using a LangChain to search for information related to user prompts. The application is not intended for production use and serves as a demonstration and educational tool.
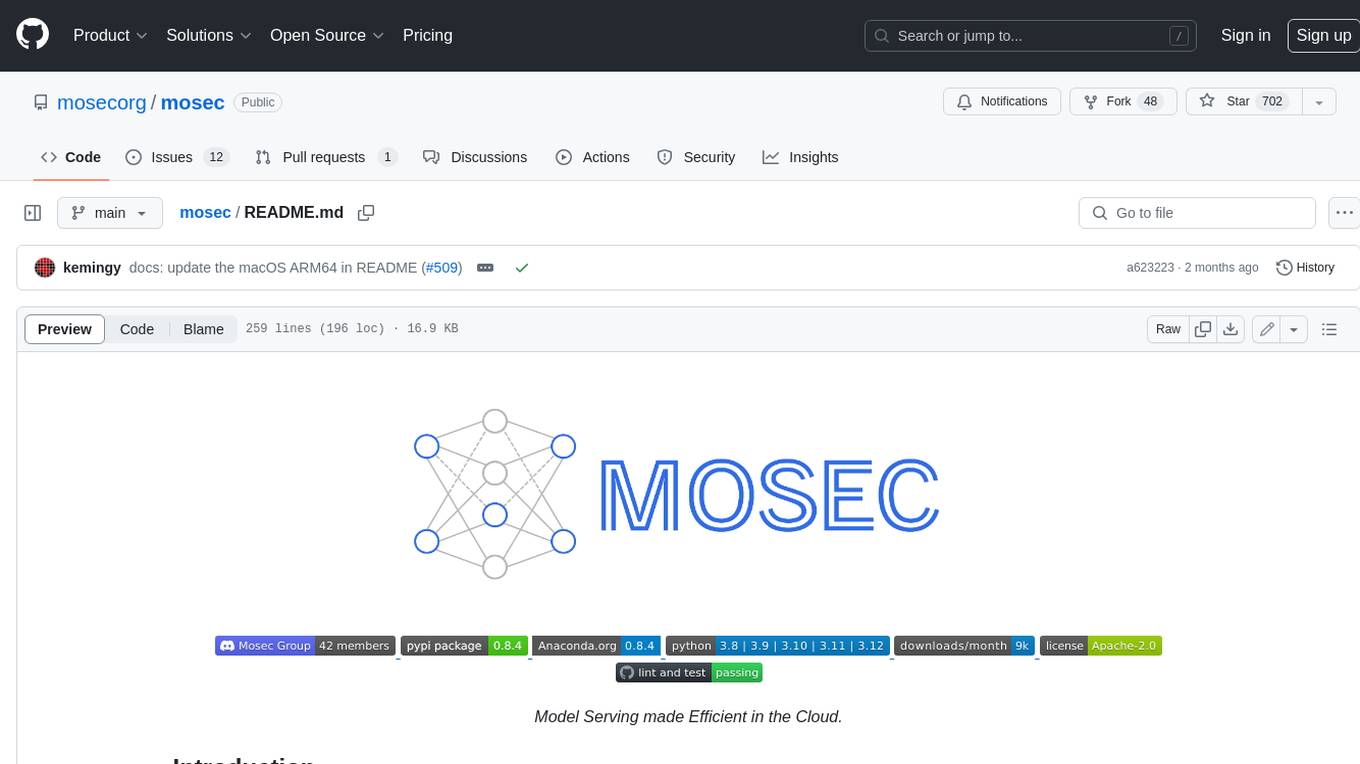
mosec
Mosec is a high-performance and flexible model serving framework for building ML model-enabled backend and microservices. It bridges the gap between any machine learning models you just trained and the efficient online service API. * **Highly performant** : web layer and task coordination built with Rust 🦀, which offers blazing speed in addition to efficient CPU utilization powered by async I/O * **Ease of use** : user interface purely in Python 🐍, by which users can serve their models in an ML framework-agnostic manner using the same code as they do for offline testing * **Dynamic batching** : aggregate requests from different users for batched inference and distribute results back * **Pipelined stages** : spawn multiple processes for pipelined stages to handle CPU/GPU/IO mixed workloads * **Cloud friendly** : designed to run in the cloud, with the model warmup, graceful shutdown, and Prometheus monitoring metrics, easily managed by Kubernetes or any container orchestration systems * **Do one thing well** : focus on the online serving part, users can pay attention to the model optimization and business logic
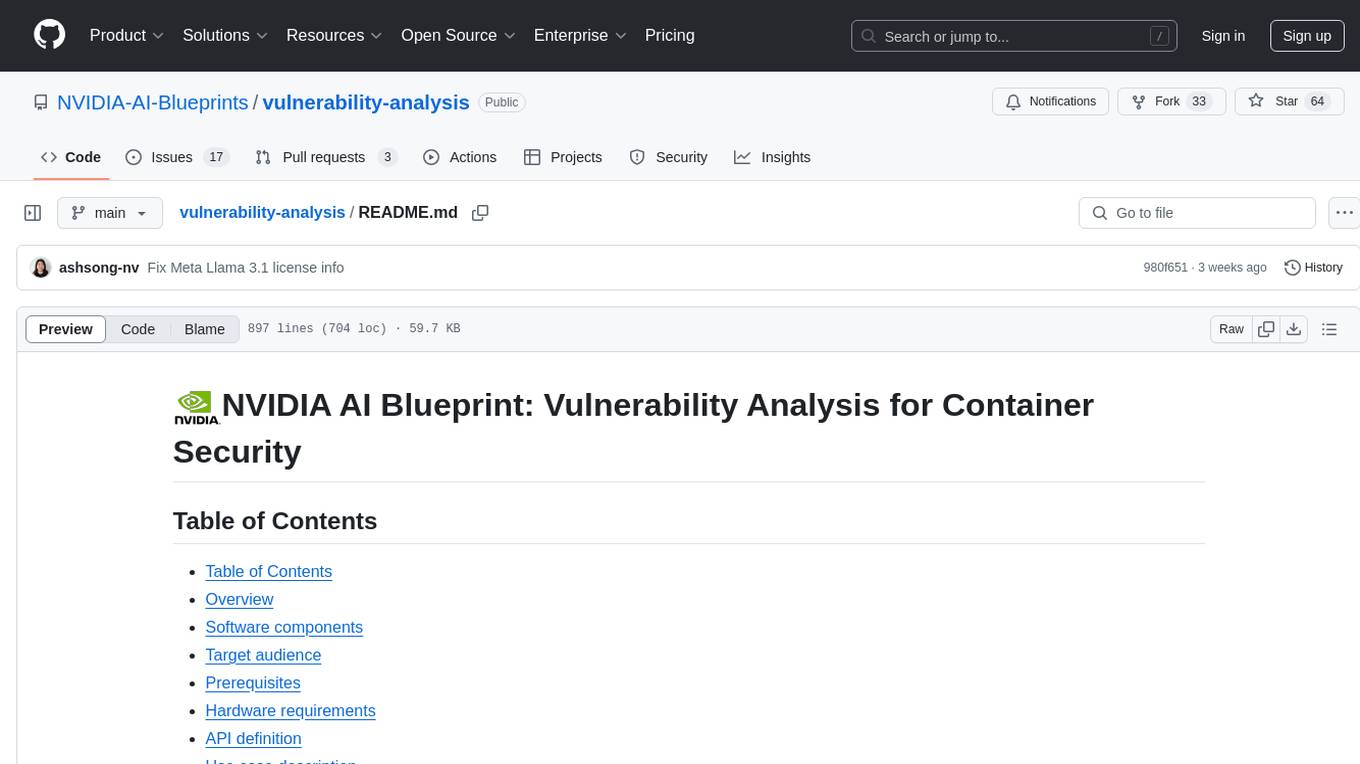
vulnerability-analysis
The NVIDIA AI Blueprint for Vulnerability Analysis for Container Security showcases accelerated analysis on common vulnerabilities and exposures (CVE) at an enterprise scale, reducing mitigation time from days to seconds. It enables security analysts to determine software package vulnerabilities using large language models (LLMs) and retrieval-augmented generation (RAG). The blueprint is designed for security analysts, IT engineers, and AI practitioners in cybersecurity. It requires NVAIE developer license and API keys for vulnerability databases, search engines, and LLM model services. Hardware requirements include L40 GPU for pipeline operation and optional LLM NIM and Embedding NIM. The workflow involves LLM pipeline for CVE impact analysis, utilizing LLM planner, agent, and summarization nodes. The blueprint uses NVIDIA NIM microservices and Morpheus Cybersecurity AI SDK for vulnerability analysis.
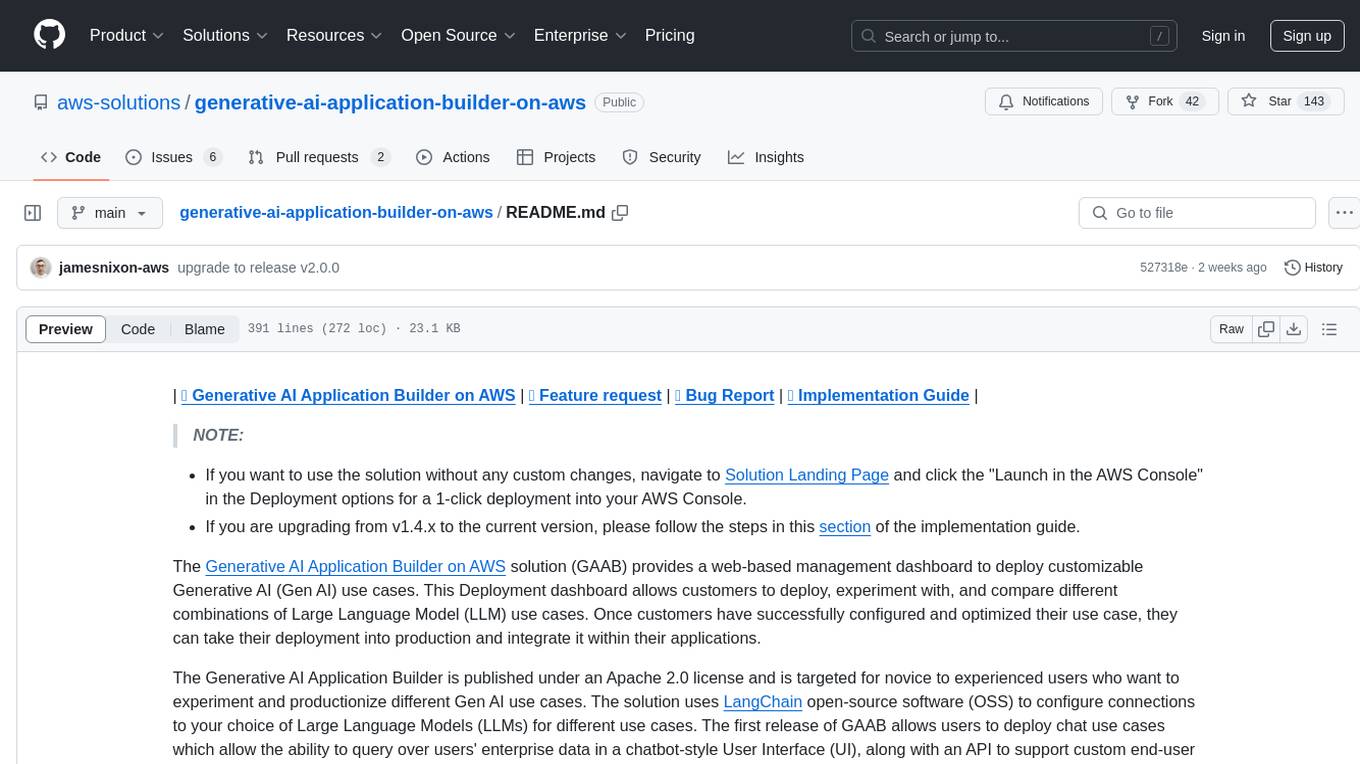
generative-ai-application-builder-on-aws
The Generative AI Application Builder on AWS (GAAB) is a solution that provides a web-based management dashboard for deploying customizable Generative AI (Gen AI) use cases. Users can experiment with and compare different combinations of Large Language Model (LLM) use cases, configure and optimize their use cases, and integrate them into their applications for production. The solution is targeted at novice to experienced users who want to experiment and productionize different Gen AI use cases. It uses LangChain open-source software to configure connections to Large Language Models (LLMs) for various use cases, with the ability to deploy chat use cases that allow querying over users' enterprise data in a chatbot-style User Interface (UI) and support custom end-user implementations through an API.
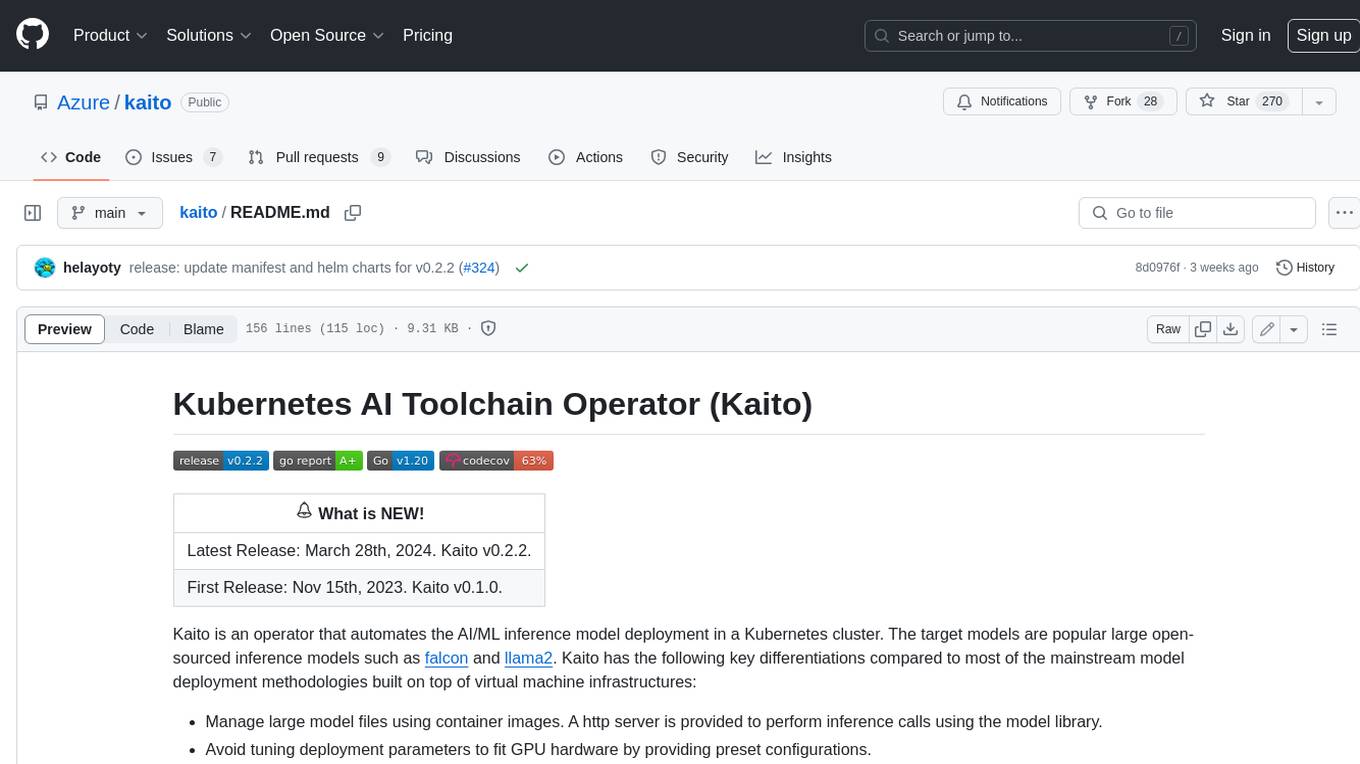
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
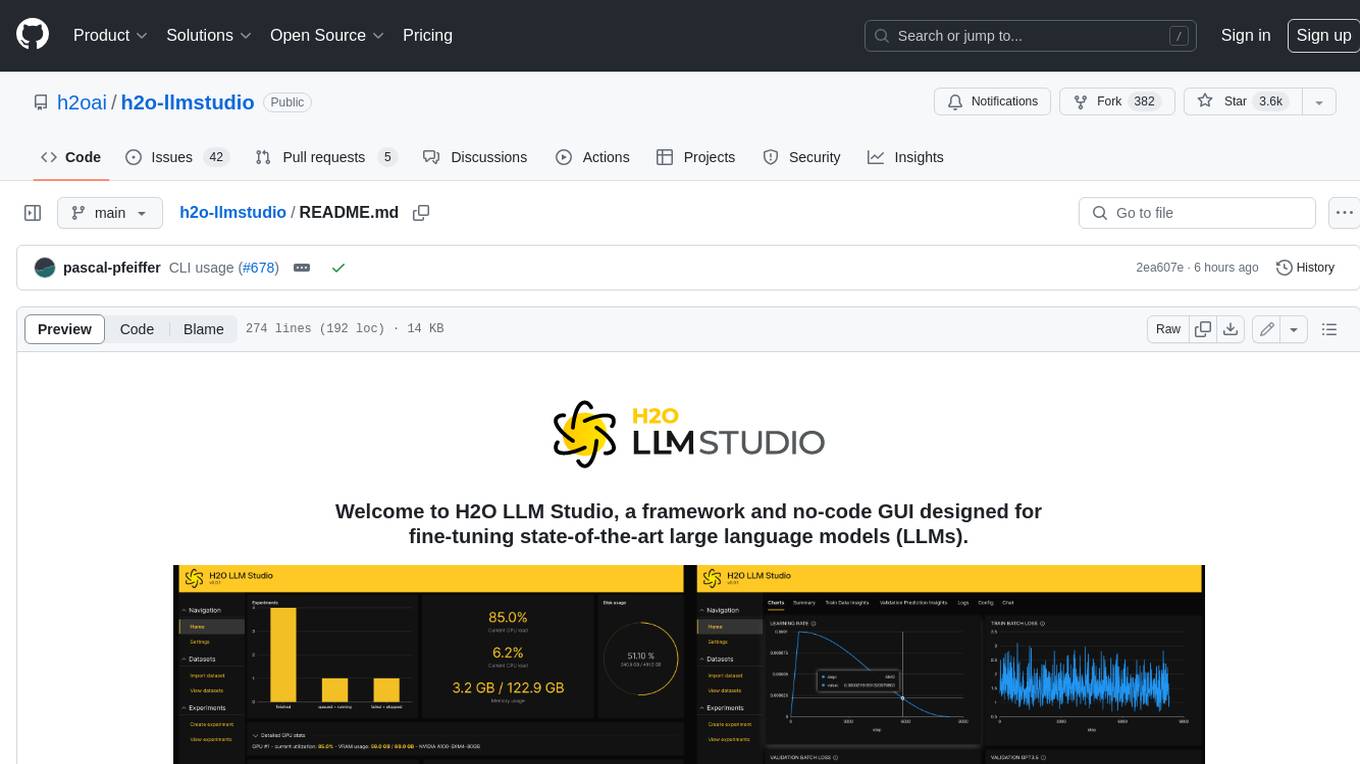
h2o-llmstudio
H2O LLM Studio is a framework and no-code GUI designed for fine-tuning state-of-the-art large language models (LLMs). With H2O LLM Studio, you can easily and effectively fine-tune LLMs without the need for any coding experience. The GUI is specially designed for large language models, and you can finetune any LLM using a large variety of hyperparameters. You can also use recent finetuning techniques such as Low-Rank Adaptation (LoRA) and 8-bit model training with a low memory footprint. Additionally, you can use Reinforcement Learning (RL) to finetune your model (experimental), use advanced evaluation metrics to judge generated answers by the model, track and compare your model performance visually, and easily export your model to the Hugging Face Hub and share it with the community.
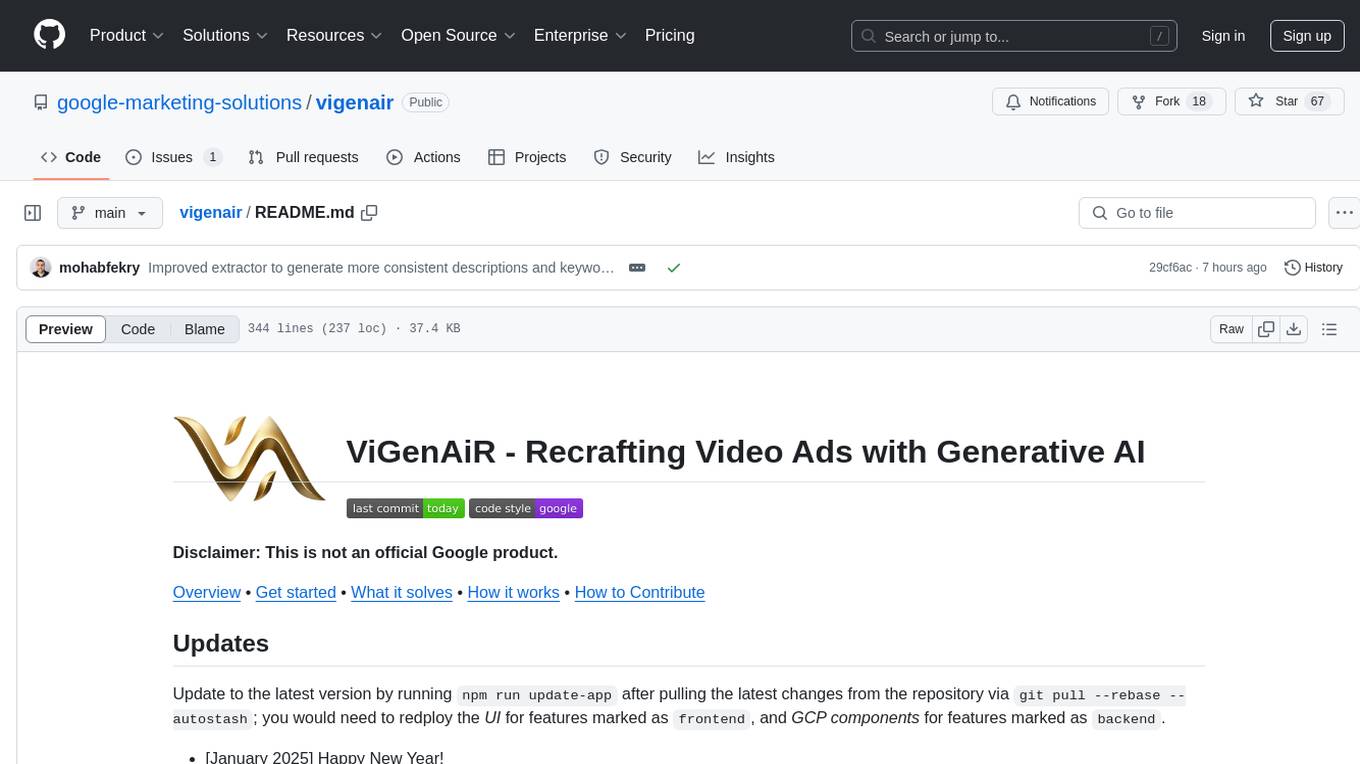
vigenair
ViGenAiR is a tool that harnesses the power of Generative AI models on Google Cloud Platform to automatically transform long-form Video Ads into shorter variants, targeting different audiences. It generates video, image, and text assets for Demand Gen and YouTube video campaigns. Users can steer the model towards generating desired videos, conduct A/B testing, and benefit from various creative features. The tool offers benefits like diverse inventory, compelling video ads, creative excellence, user control, and performance insights. ViGenAiR works by analyzing video content, splitting it into coherent segments, and generating variants following Google's best practices for effective ads.
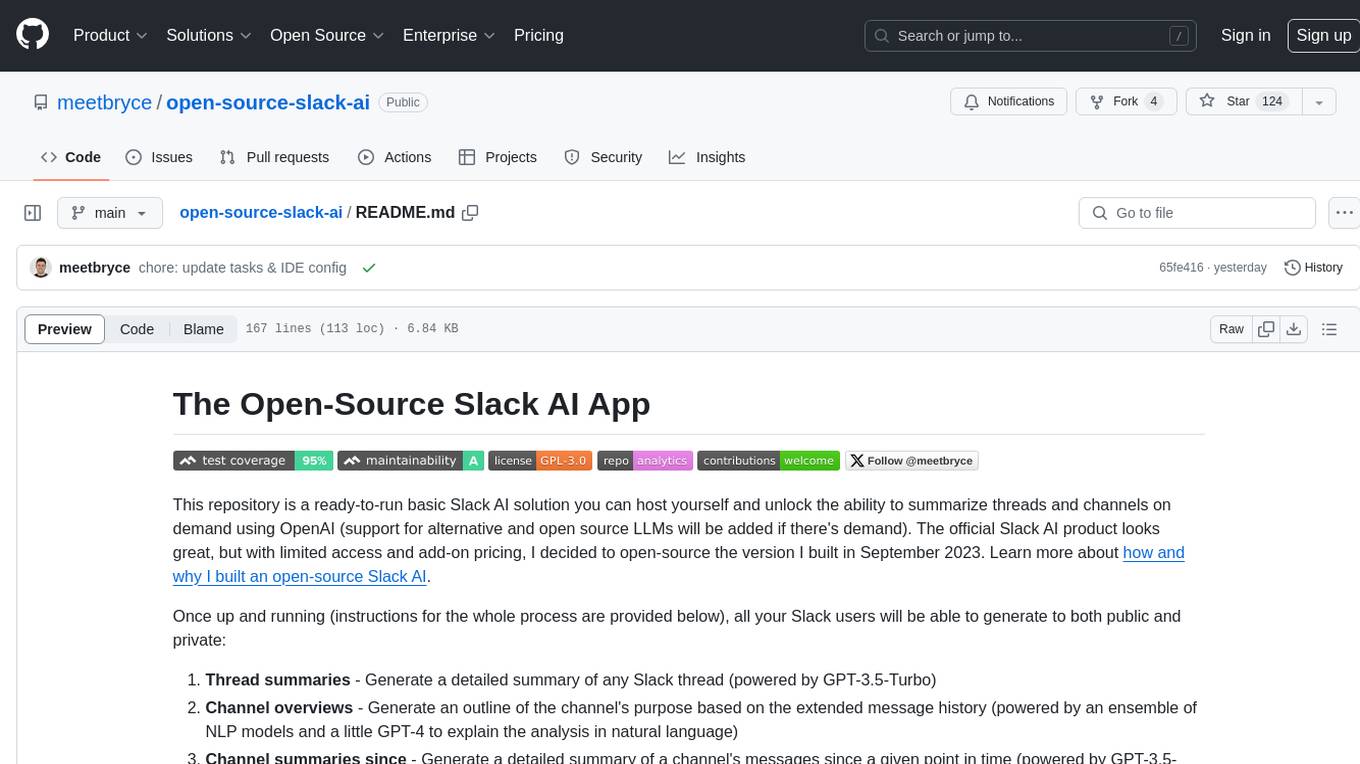
open-source-slack-ai
This repository provides a ready-to-run basic Slack AI solution that allows users to summarize threads and channels using OpenAI. Users can generate thread summaries, channel overviews, channel summaries since a specific time, and full channel summaries. The tool is powered by GPT-3.5-Turbo and an ensemble of NLP models. It requires Python 3.8 or higher, an OpenAI API key, Slack App with associated API tokens, Poetry package manager, and ngrok for local development. Users can customize channel and thread summaries, run tests with coverage using pytest, and contribute to the project for future enhancements.
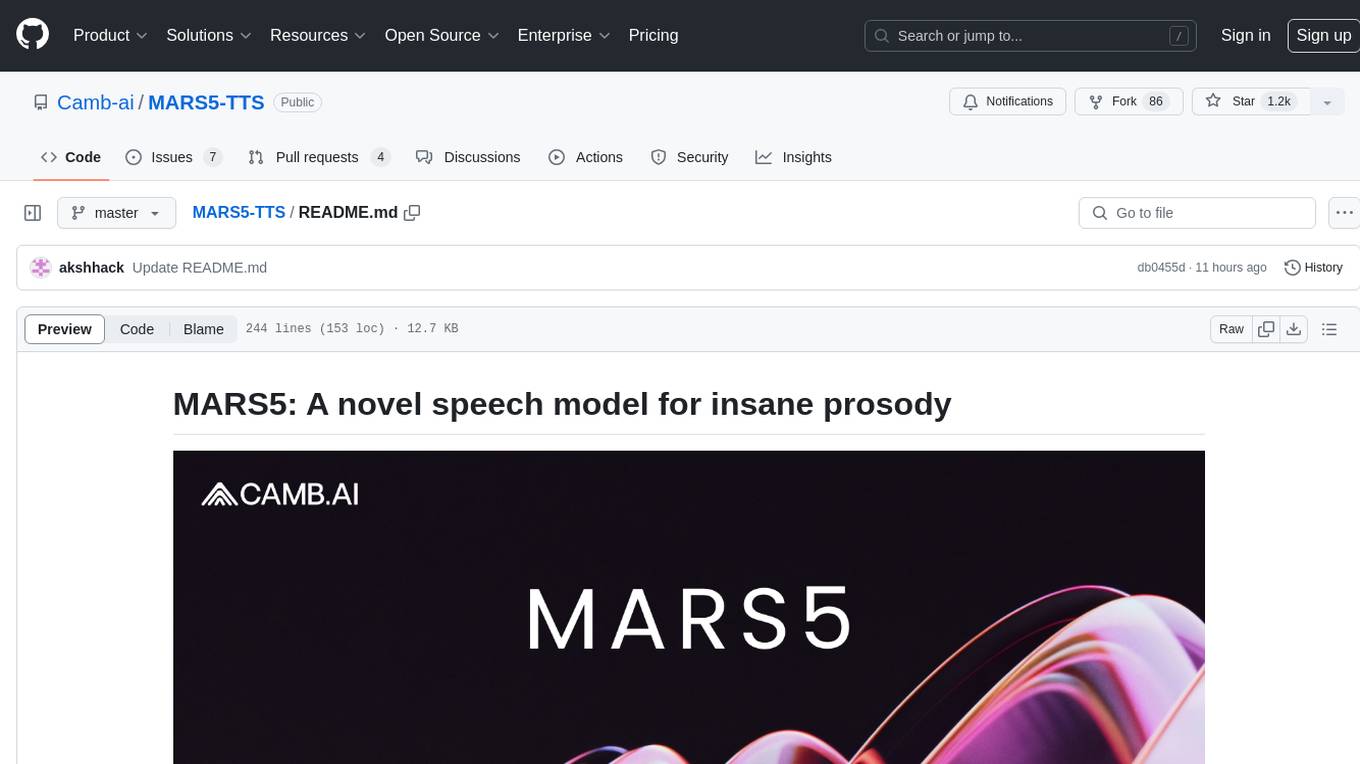
MARS5-TTS
MARS5 is a novel English speech model (TTS) developed by CAMB.AI, featuring a two-stage AR-NAR pipeline with a unique NAR component. The model can generate speech for various scenarios like sports commentary and anime with just 5 seconds of audio and a text snippet. It allows steering prosody using punctuation and capitalization in the transcript. Speaker identity is specified using an audio reference file, enabling 'deep clone' for improved quality. The model can be used via torch.hub or HuggingFace, supporting both shallow and deep cloning for inference. Checkpoints are provided for AR and NAR models, with hardware requirements of 750M+450M params on GPU. Contributions to improve model stability, performance, and reference audio selection are welcome.
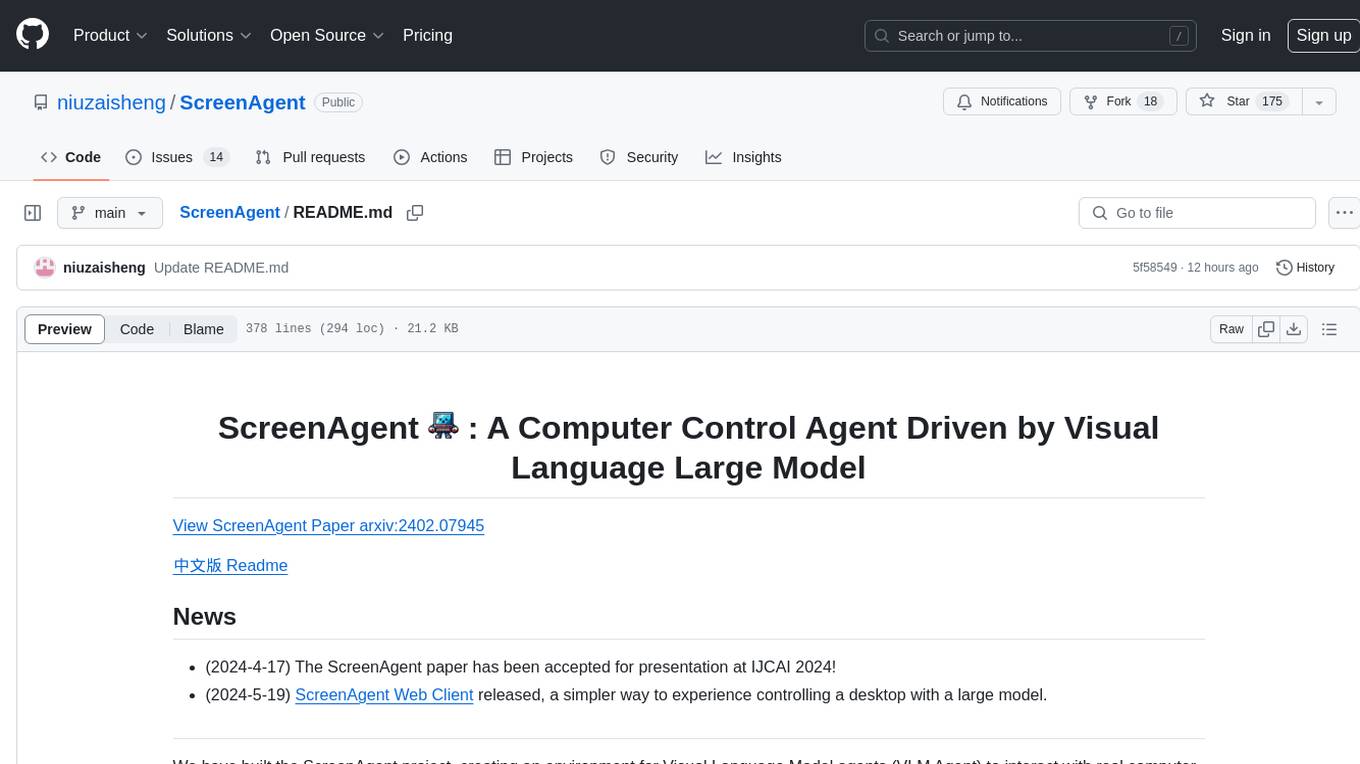
ScreenAgent
ScreenAgent is a project focused on creating an environment for Visual Language Model agents (VLM Agent) to interact with real computer screens. The project includes designing an automatic control process for agents to interact with the environment and complete multi-step tasks. It also involves building the ScreenAgent dataset, which collects screenshots and action sequences for various daily computer tasks. The project provides a controller client code, configuration files, and model training code to enable users to control a desktop with a large model.
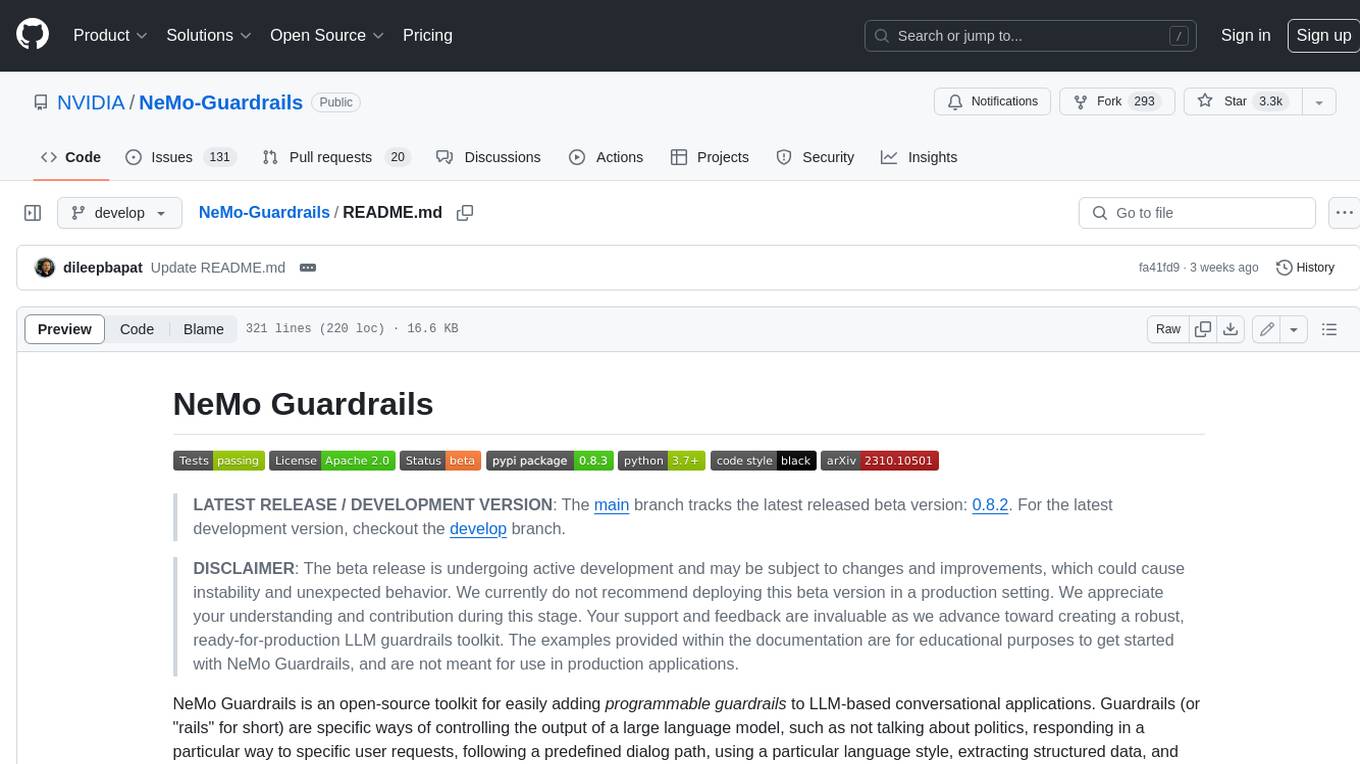
NeMo-Guardrails
NeMo Guardrails is an open-source toolkit for easily adding _programmable guardrails_ to LLM-based conversational applications. Guardrails (or "rails" for short) are specific ways of controlling the output of a large language model, such as not talking about politics, responding in a particular way to specific user requests, following a predefined dialog path, using a particular language style, extracting structured data, and more.
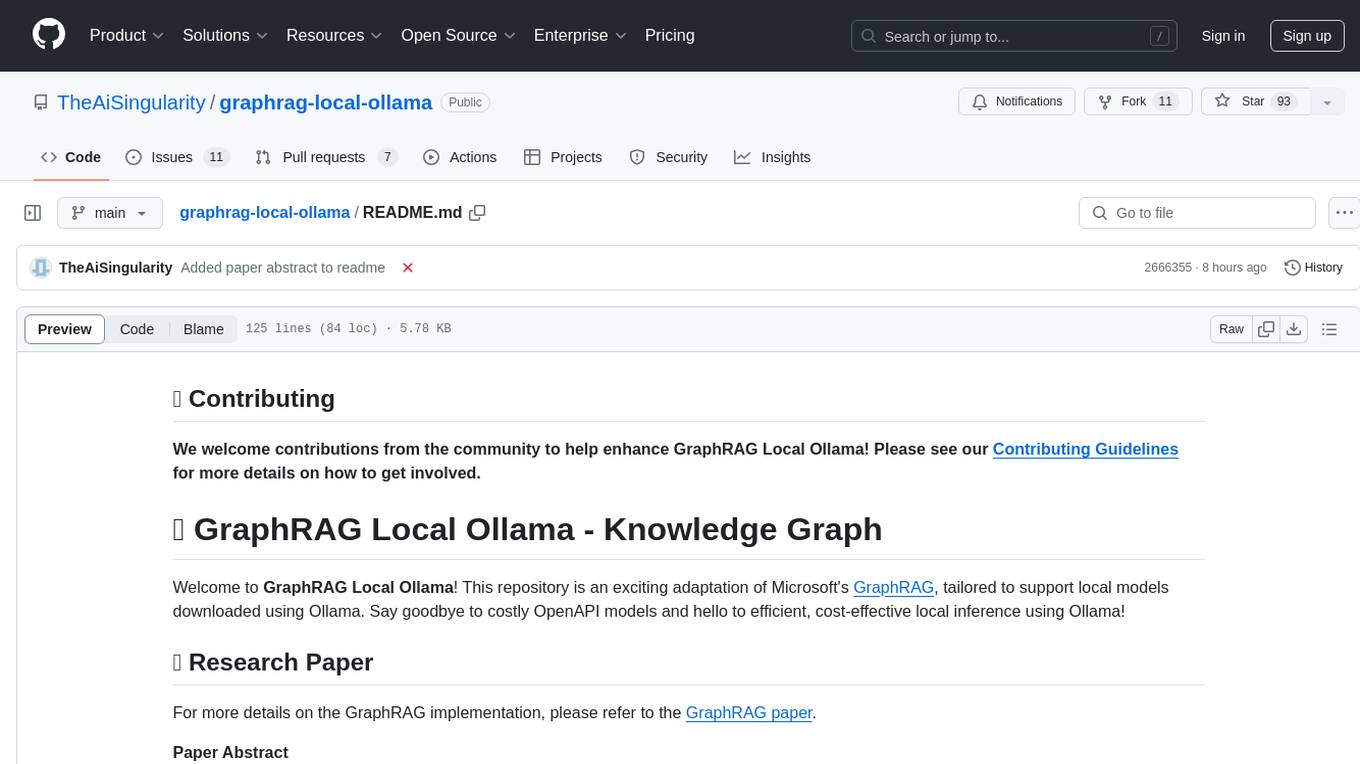
graphrag-local-ollama
GraphRAG Local Ollama is a repository that offers an adaptation of Microsoft's GraphRAG, customized to support local models downloaded using Ollama. It enables users to leverage local models with Ollama for large language models (LLMs) and embeddings, eliminating the need for costly OpenAPI models. The repository provides a simple setup process and allows users to perform question answering over private text corpora by building a graph-based text index and generating community summaries for closely-related entities. GraphRAG Local Ollama aims to improve the comprehensiveness and diversity of generated answers for global sensemaking questions over datasets.
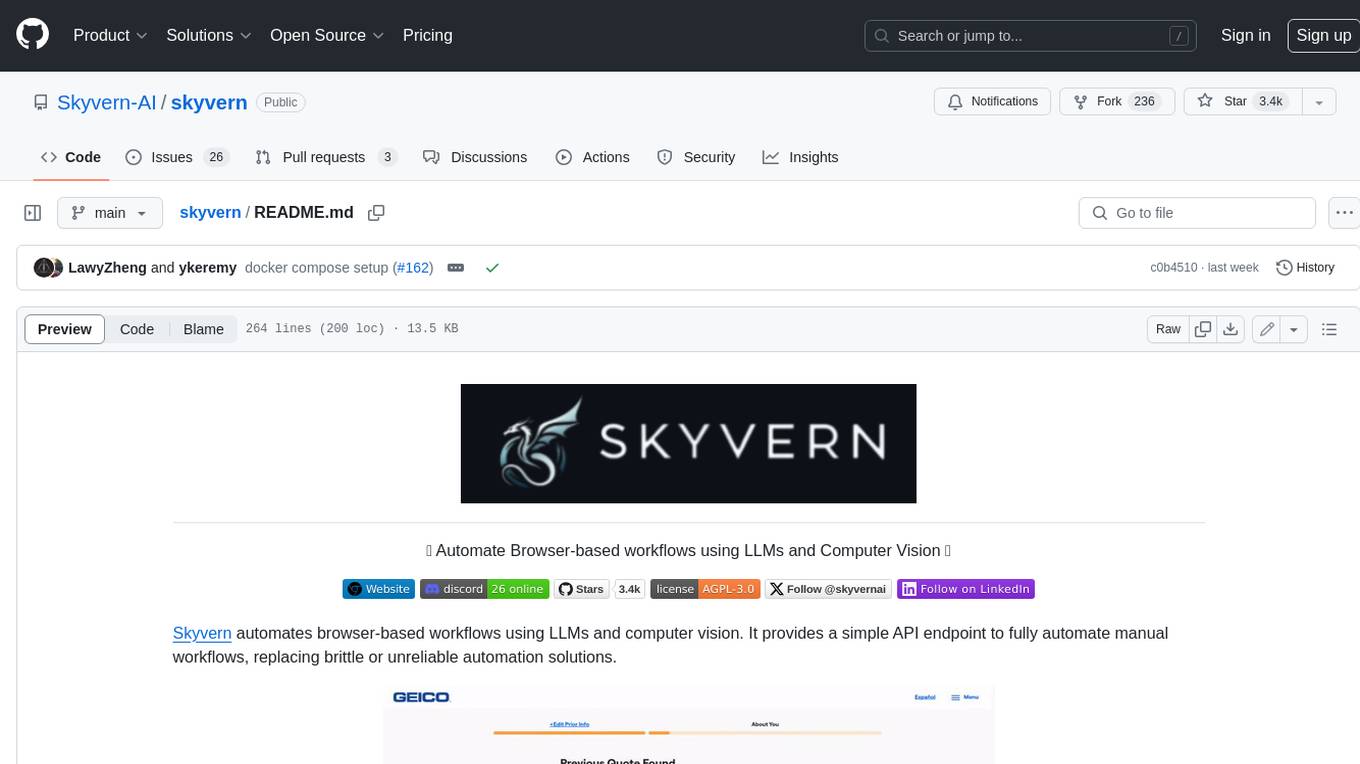
skyvern
Skyvern automates browser-based workflows using LLMs and computer vision. It provides a simple API endpoint to fully automate manual workflows, replacing brittle or unreliable automation solutions. Traditional approaches to browser automations required writing custom scripts for websites, often relying on DOM parsing and XPath-based interactions which would break whenever the website layouts changed. Instead of only relying on code-defined XPath interactions, Skyvern adds computer vision and LLMs to the mix to parse items in the viewport in real-time, create a plan for interaction and interact with them. This approach gives us a few advantages: 1. Skyvern can operate on websites it’s never seen before, as it’s able to map visual elements to actions necessary to complete a workflow, without any customized code 2. Skyvern is resistant to website layout changes, as there are no pre-determined XPaths or other selectors our system is looking for while trying to navigate 3. Skyvern leverages LLMs to reason through interactions to ensure we can cover complex situations. Examples include: 1. If you wanted to get an auto insurance quote from Geico, the answer to a common question “Were you eligible to drive at 18?” could be inferred from the driver receiving their license at age 16 2. If you were doing competitor analysis, it’s understanding that an Arnold Palmer 22 oz can at 7/11 is almost definitely the same product as a 23 oz can at Gopuff (even though the sizes are slightly different, which could be a rounding error!) Want to see examples of Skyvern in action? Jump to #real-world-examples-of- skyvern
For similar tasks
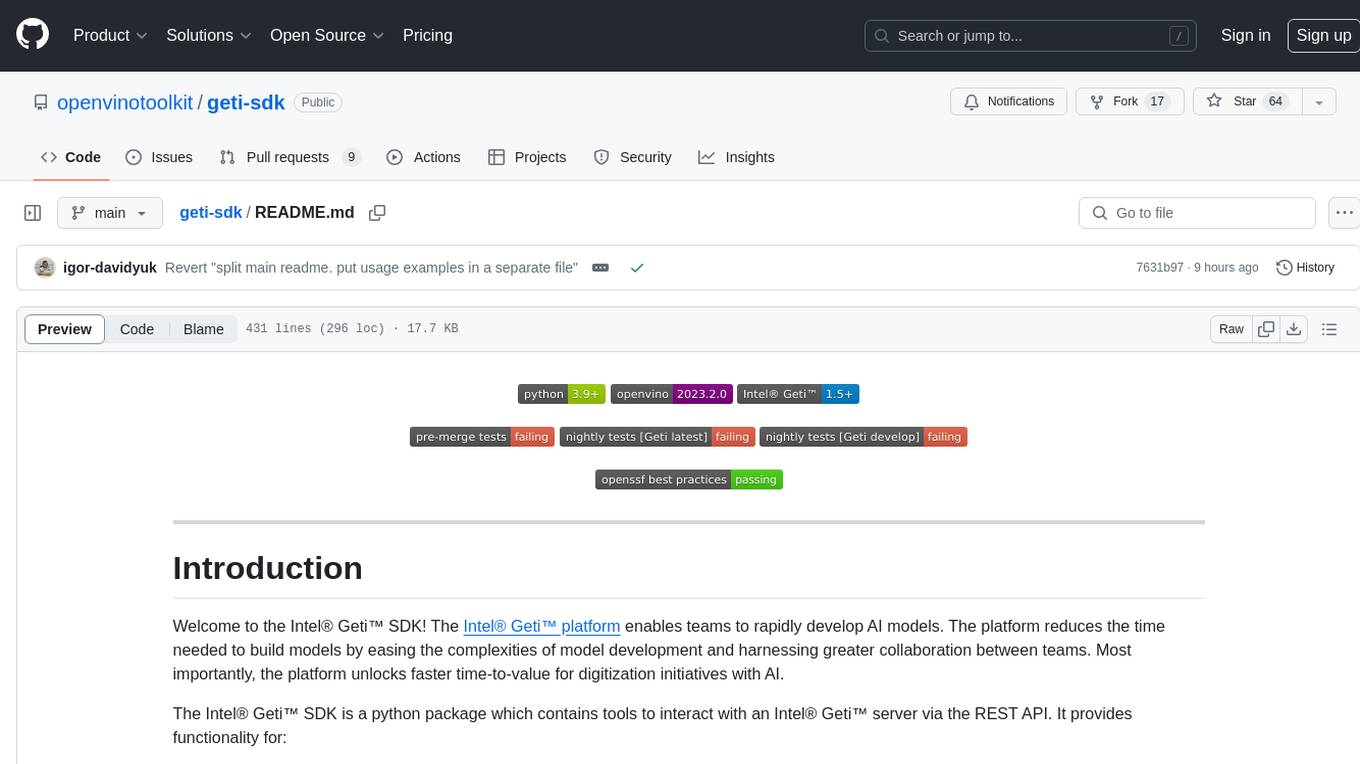
geti-sdk
The Intel® Geti™ SDK is a python package that enables teams to rapidly develop AI models by easing the complexities of model development and enhancing collaboration between teams. It provides tools to interact with an Intel® Geti™ server via the REST API, allowing for project creation, downloading, uploading, deploying for local inference with OpenVINO, setting project and model configuration, launching and monitoring training jobs, and media upload and prediction. The SDK also includes tutorial-style Jupyter notebooks demonstrating its usage.
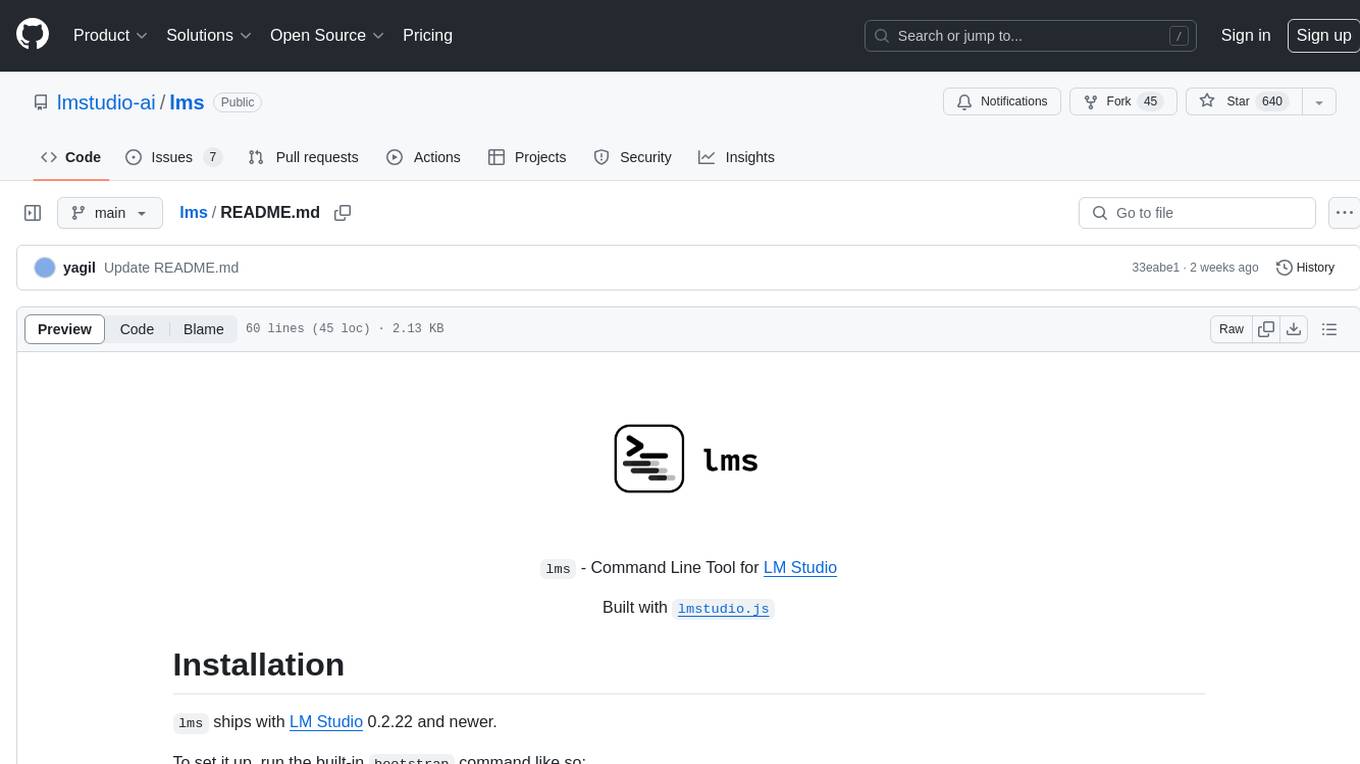
lms
The `lms` Command Line Tool for LM Studio is a powerful tool built with `lmstudio.js` that allows users to interact with LM Studio functionalities through the command line interface. It provides a wide range of commands for managing models, starting and stopping servers, creating projects, and streaming logs. Users can easily bootstrap the tool and access detailed information about each subcommand. The tool is designed to enhance the user experience and streamline workflows when working with LM Studio.
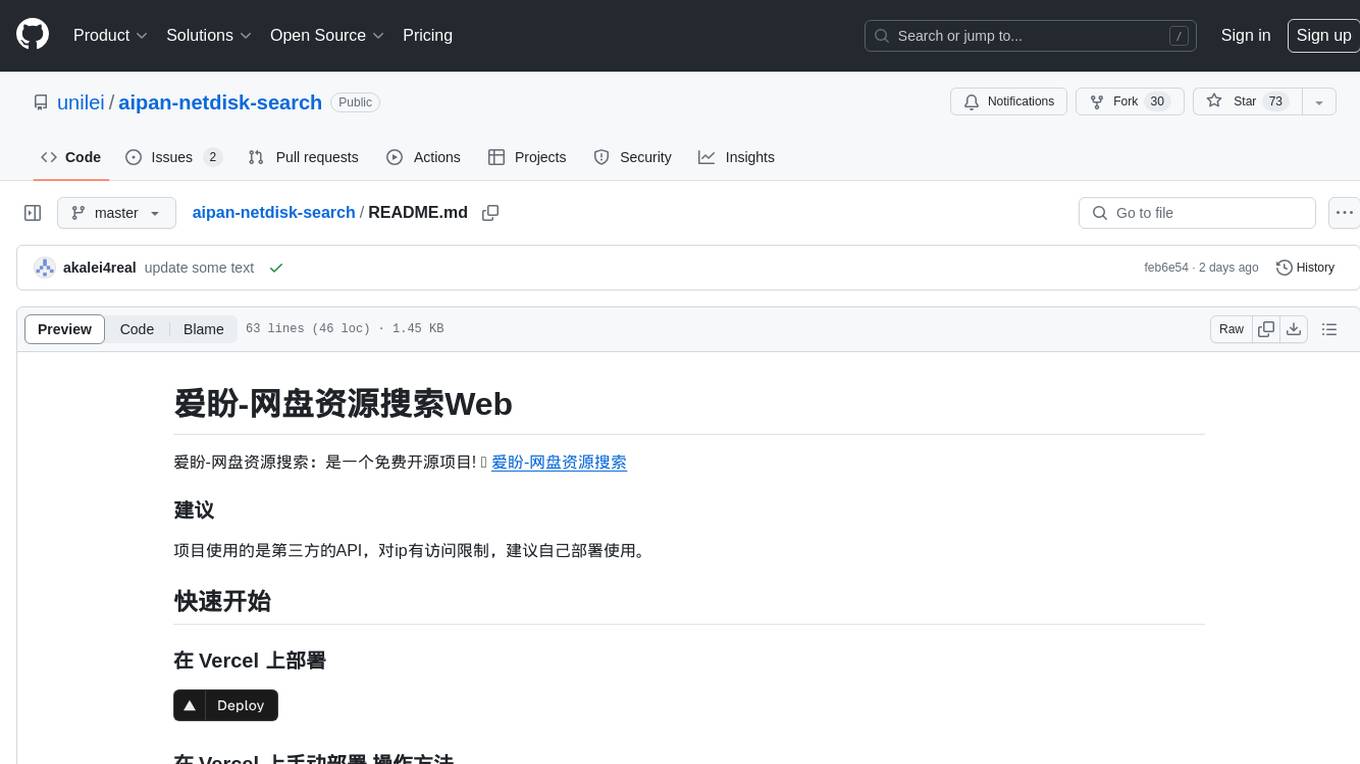
aipan-netdisk-search
Aipan-Netdisk-Search is a free and open-source web project for searching netdisk resources. It utilizes third-party APIs with IP access restrictions, suggesting self-deployment. The project can be easily deployed on Vercel and provides instructions for manual deployment. Users can clone the project, install dependencies, run it in the browser, and access it at localhost:3001. The project also includes documentation for deploying on personal servers using NUXT.JS. Additionally, there are options for donations and communication via WeChat.
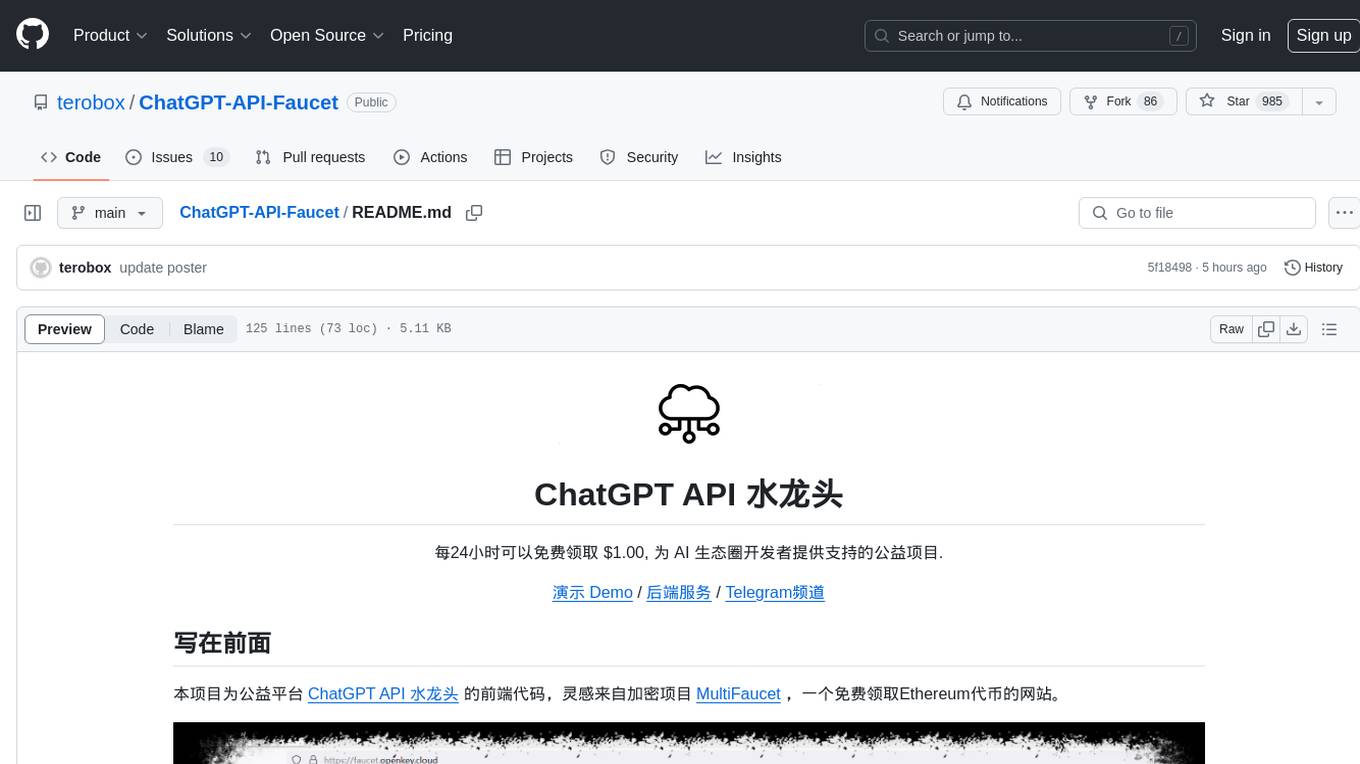
ChatGPT-API-Faucet
ChatGPT API Faucet is a frontend project for the public platform ChatGPT API Faucet, inspired by the crypto project MultiFaucet. It allows developers in the AI ecosystem to claim $1.00 for free every 24 hours. The program is developed using the Next.js framework and React library, with key components like _app.tsx for initializing pages, index.tsx for main modifications, and Layout.tsx for defining layout components. Users can deploy the project by installing dependencies, building the project, starting the project, configuring reverse proxies or using port:IP access, and running a development server. The tool also supports token balance queries and is related to projects like one-api, ChatGPT-Cost-Calculator, and Poe.Monster. It is licensed under the MIT license.
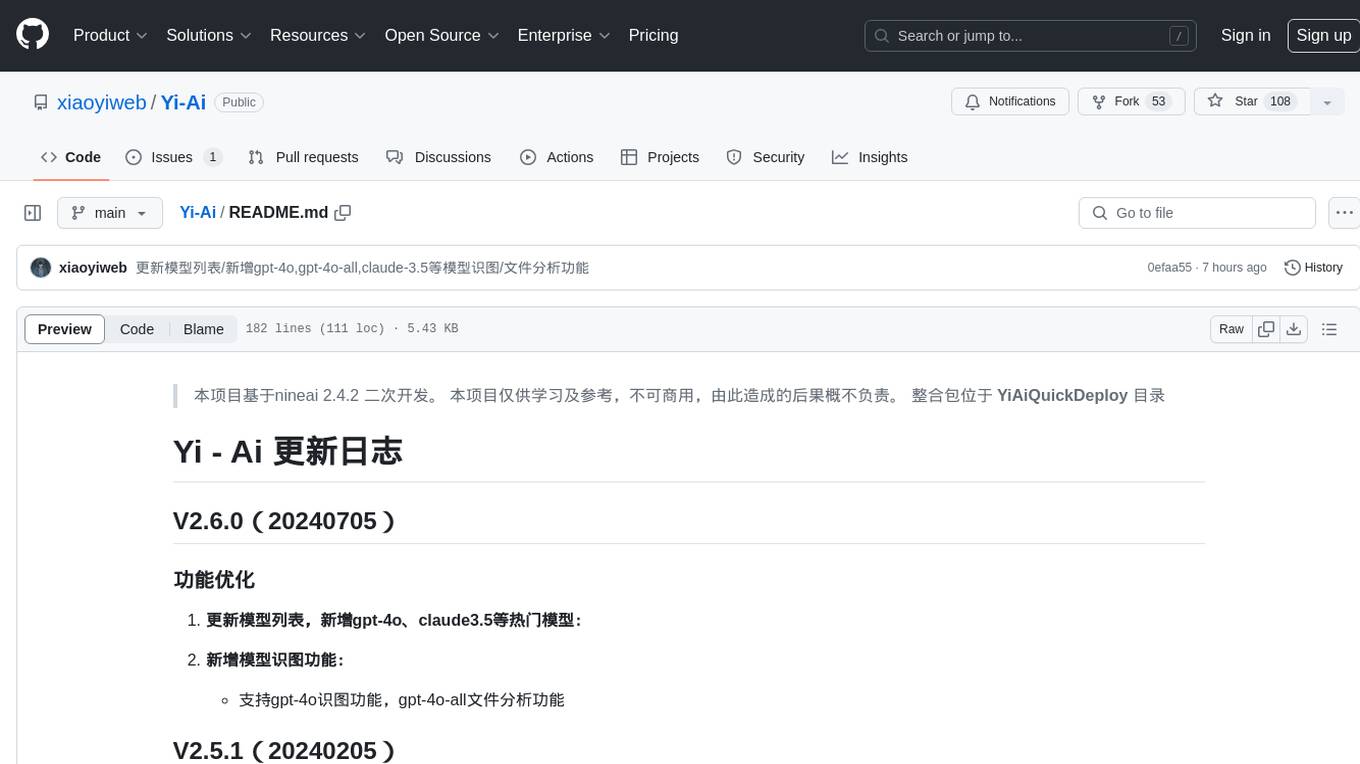
Yi-Ai
Yi-Ai is a project based on the development of nineai 2.4.2. It is for learning and reference purposes only, not for commercial use. The project includes updates to popular models like gpt-4o and claude3.5, as well as new features such as model image recognition. It also supports various functionalities like model sorting, file type extensions, and bug fixes. The project provides deployment tutorials for both integrated and compiled packages, with instructions for environment setup, configuration, dependency installation, and project startup. Additionally, it offers a management platform with different access levels and emphasizes the importance of following the steps for proper system operation.
For similar jobs
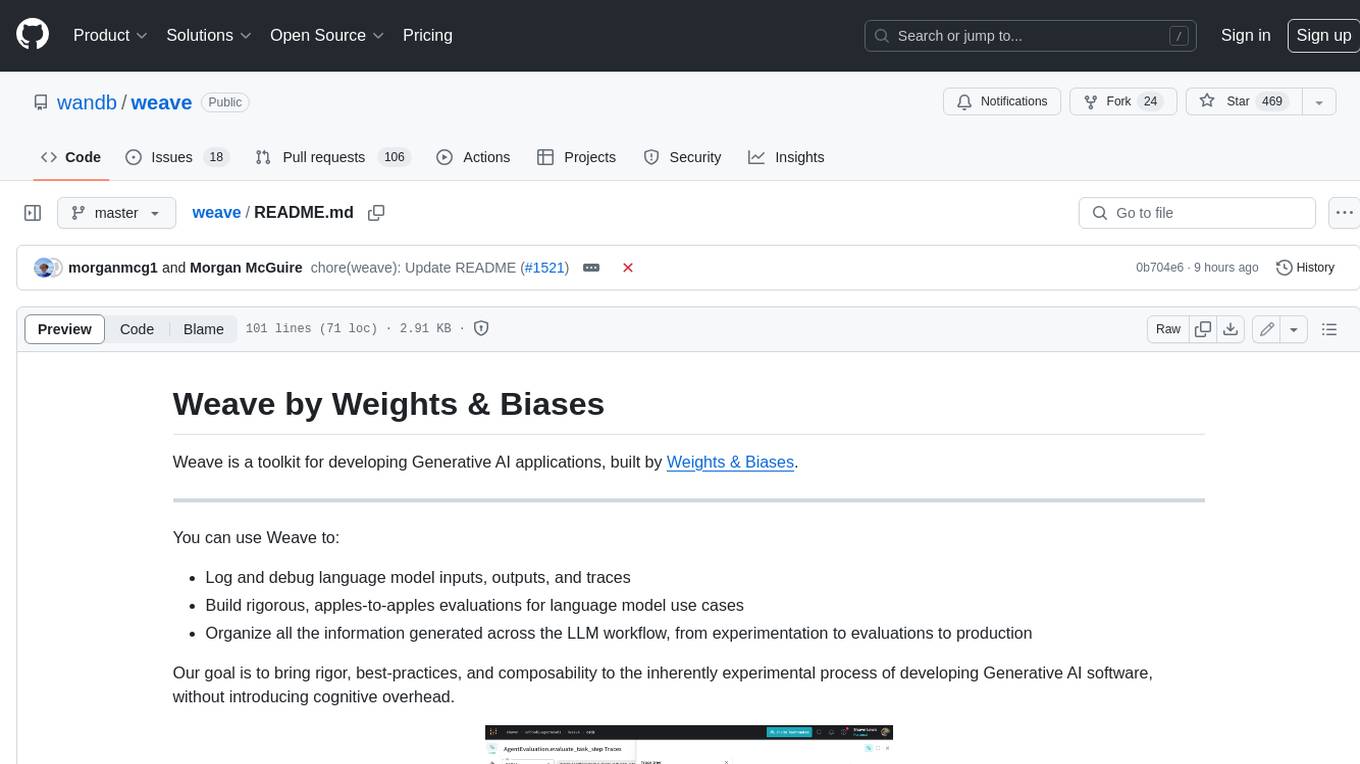
weave
Weave is a toolkit for developing Generative AI applications, built by Weights & Biases. With Weave, you can log and debug language model inputs, outputs, and traces; build rigorous, apples-to-apples evaluations for language model use cases; and organize all the information generated across the LLM workflow, from experimentation to evaluations to production. Weave aims to bring rigor, best-practices, and composability to the inherently experimental process of developing Generative AI software, without introducing cognitive overhead.
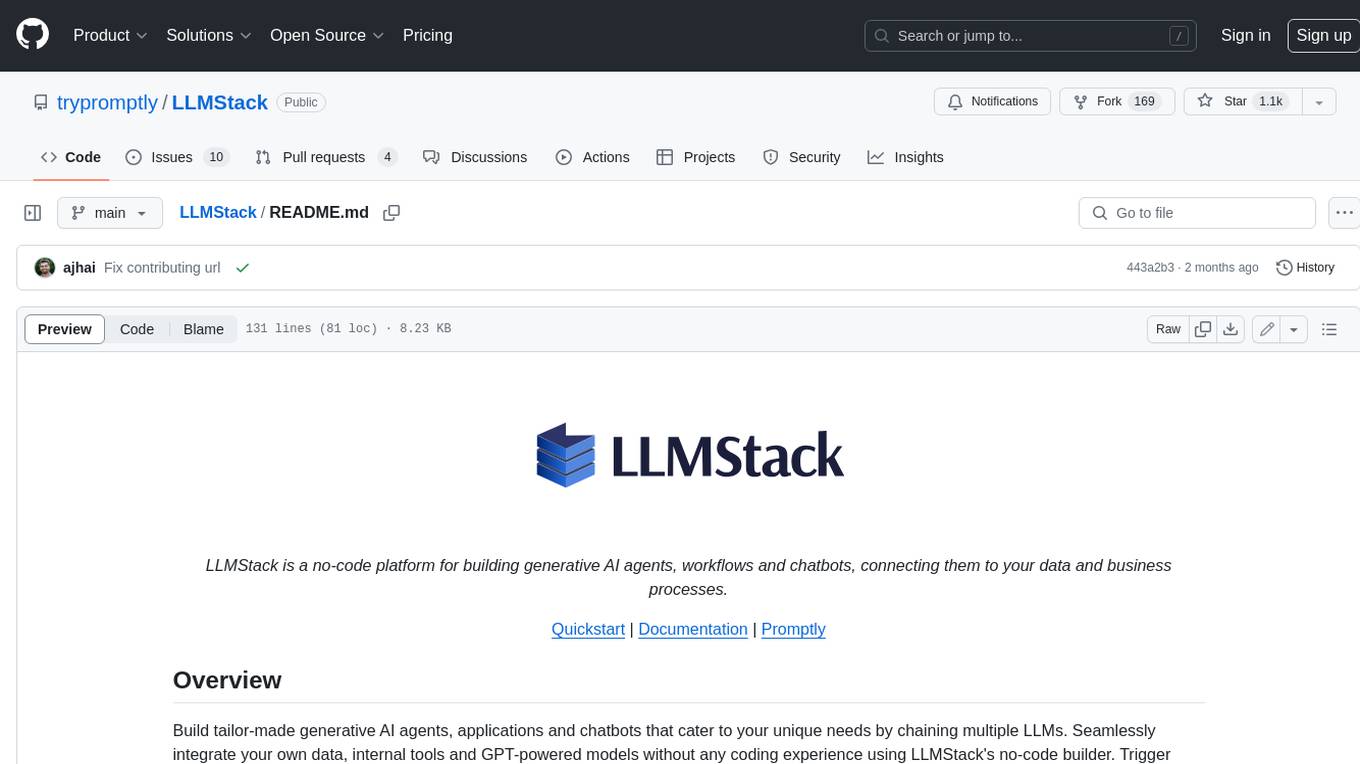
LLMStack
LLMStack is a no-code platform for building generative AI agents, workflows, and chatbots. It allows users to connect their own data, internal tools, and GPT-powered models without any coding experience. LLMStack can be deployed to the cloud or on-premise and can be accessed via HTTP API or triggered from Slack or Discord.
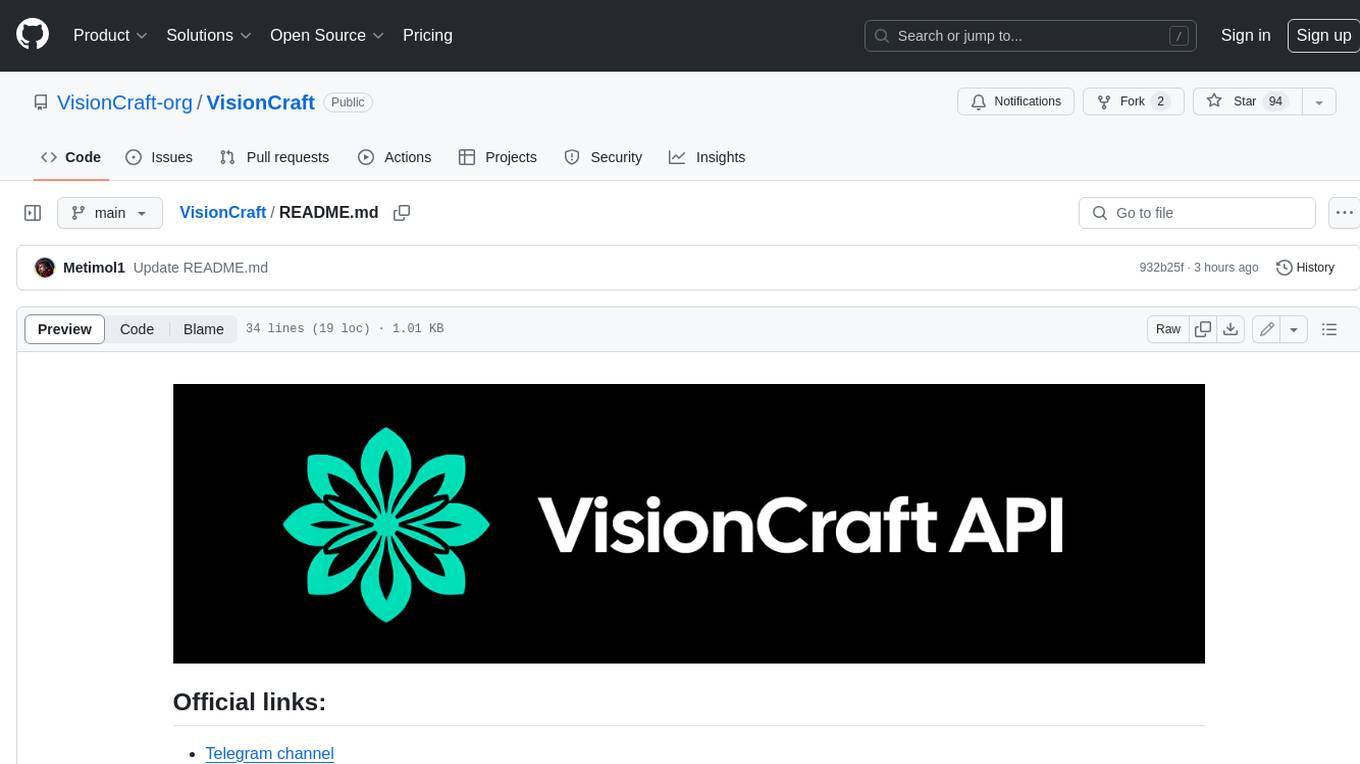
VisionCraft
The VisionCraft API is a free API for using over 100 different AI models. From images to sound.
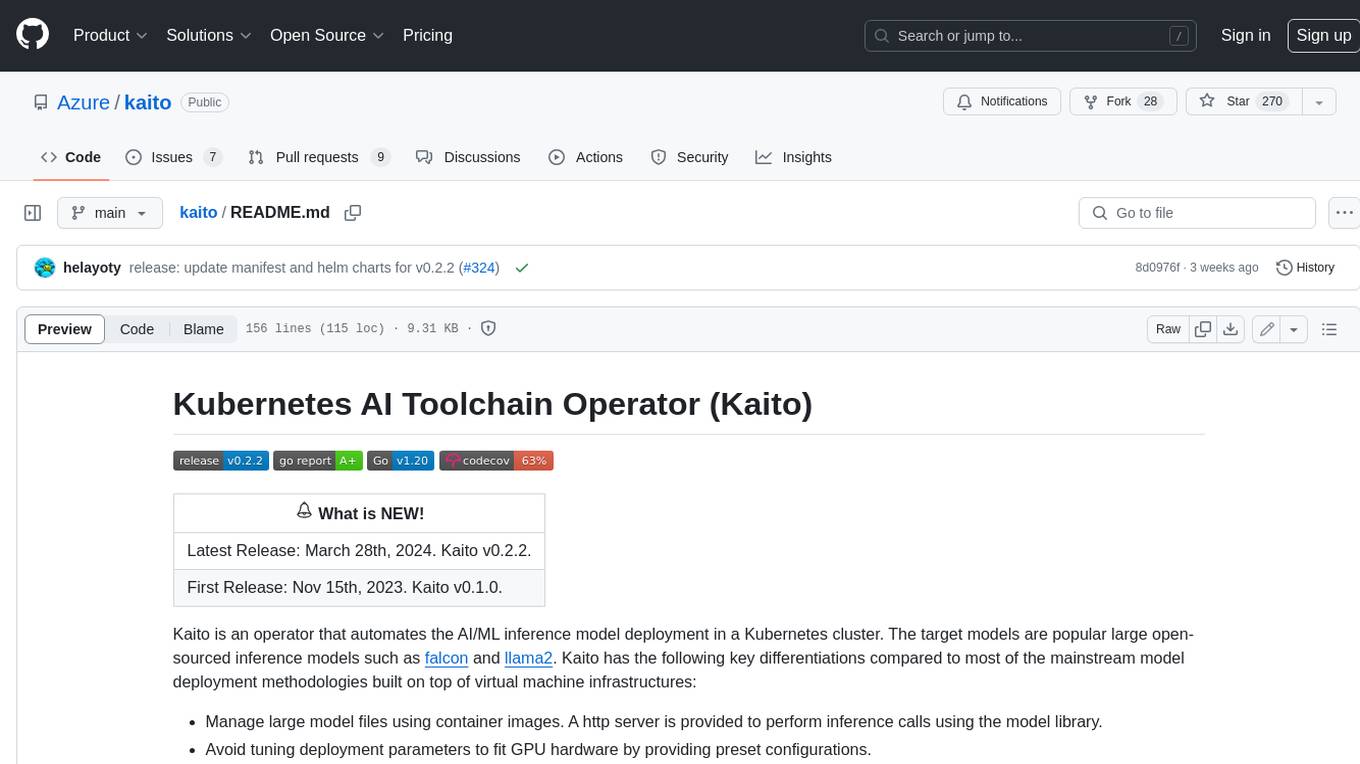
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
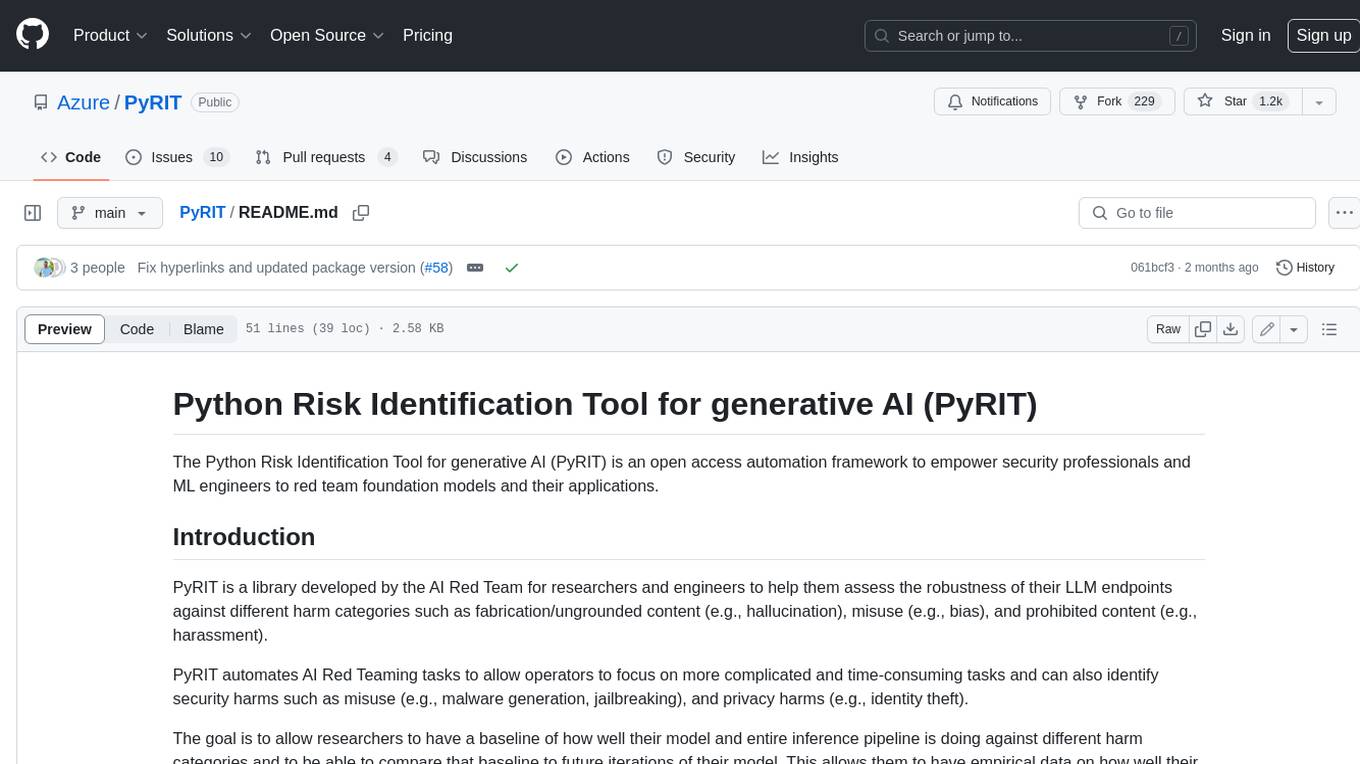
PyRIT
PyRIT is an open access automation framework designed to empower security professionals and ML engineers to red team foundation models and their applications. It automates AI Red Teaming tasks to allow operators to focus on more complicated and time-consuming tasks and can also identify security harms such as misuse (e.g., malware generation, jailbreaking), and privacy harms (e.g., identity theft). The goal is to allow researchers to have a baseline of how well their model and entire inference pipeline is doing against different harm categories and to be able to compare that baseline to future iterations of their model. This allows them to have empirical data on how well their model is doing today, and detect any degradation of performance based on future improvements.
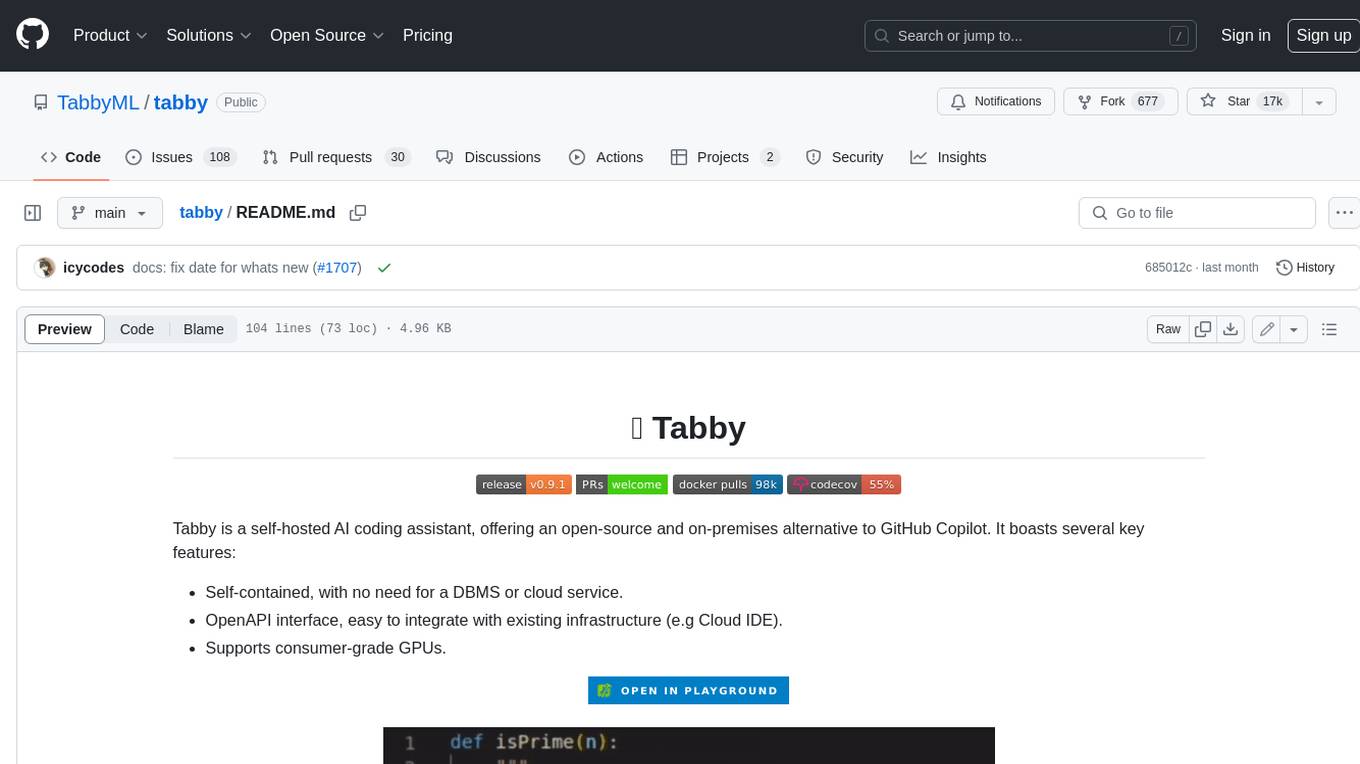
tabby
Tabby is a self-hosted AI coding assistant, offering an open-source and on-premises alternative to GitHub Copilot. It boasts several key features: * Self-contained, with no need for a DBMS or cloud service. * OpenAPI interface, easy to integrate with existing infrastructure (e.g Cloud IDE). * Supports consumer-grade GPUs.
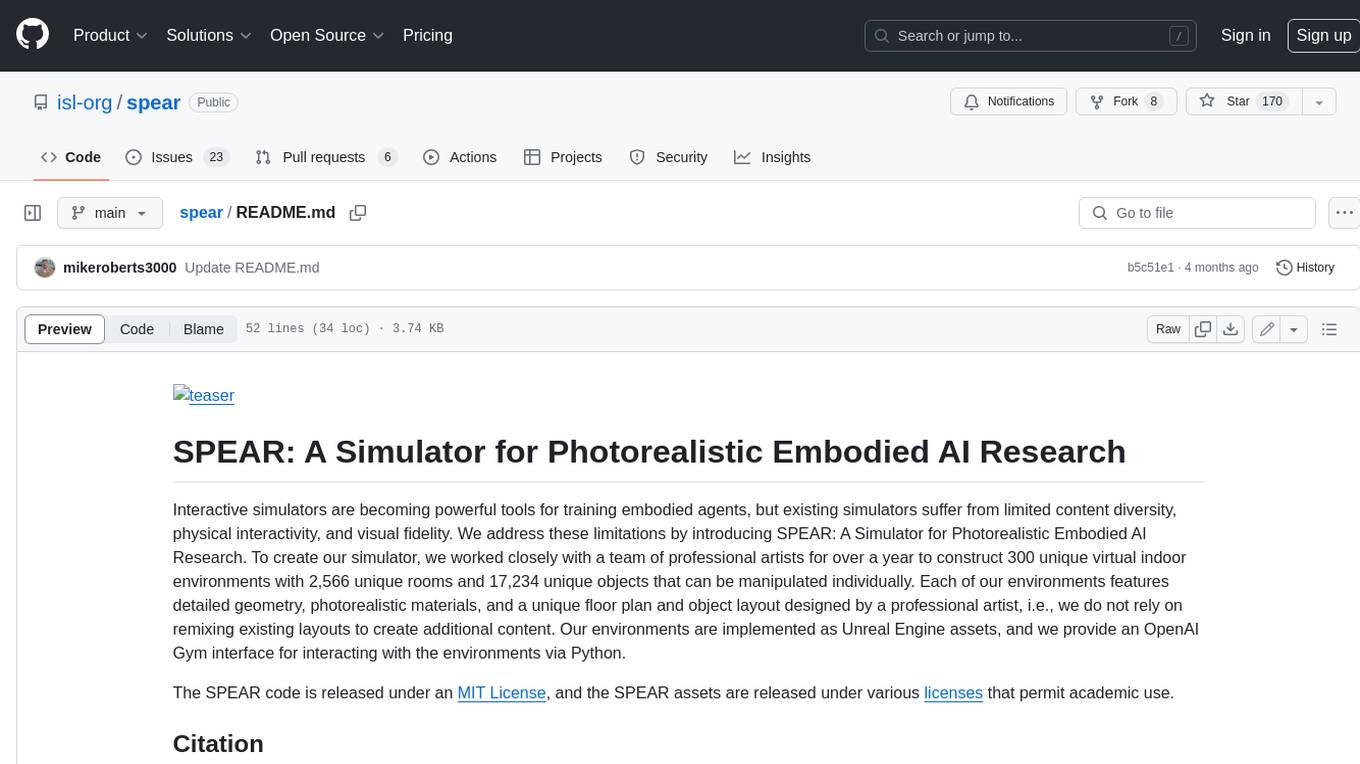
spear
SPEAR (Simulator for Photorealistic Embodied AI Research) is a powerful tool for training embodied agents. It features 300 unique virtual indoor environments with 2,566 unique rooms and 17,234 unique objects that can be manipulated individually. Each environment is designed by a professional artist and features detailed geometry, photorealistic materials, and a unique floor plan and object layout. SPEAR is implemented as Unreal Engine assets and provides an OpenAI Gym interface for interacting with the environments via Python.
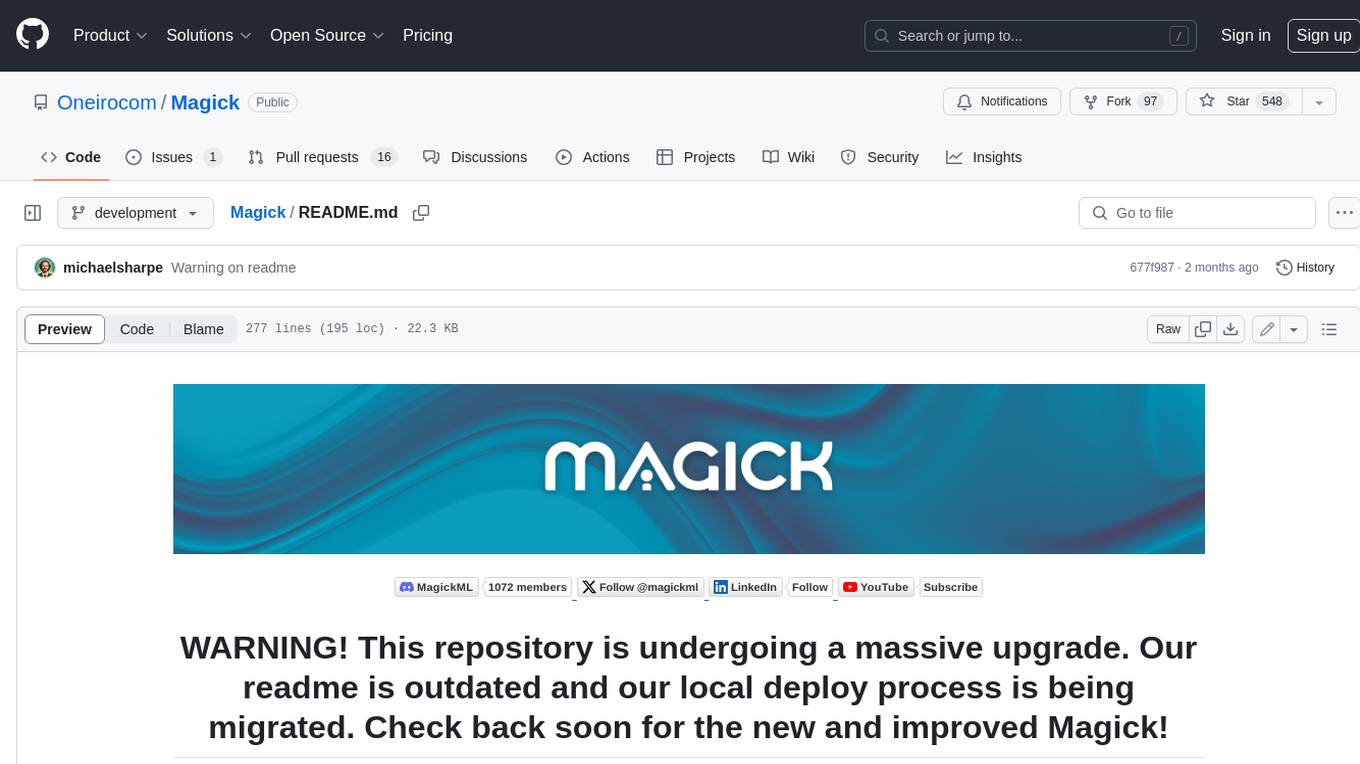
Magick
Magick is a groundbreaking visual AIDE (Artificial Intelligence Development Environment) for no-code data pipelines and multimodal agents. Magick can connect to other services and comes with nodes and templates well-suited for intelligent agents, chatbots, complex reasoning systems and realistic characters.