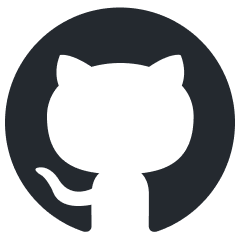
amadeus-java
Java library for the Amadeus Self-Service travel APIs
Stars: 87
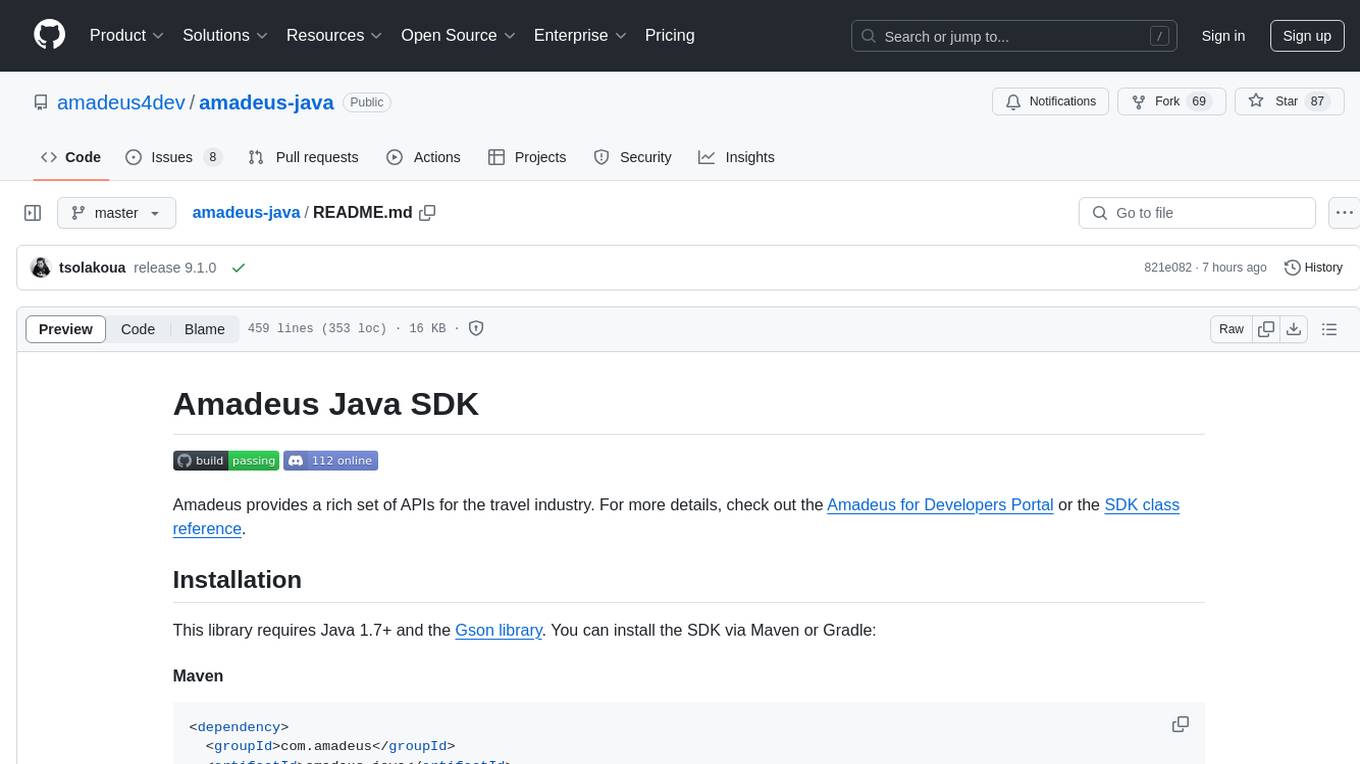
Amadeus Java SDK provides a rich set of APIs for the travel industry, allowing developers to access various functionalities such as flight search, booking, airport information, and more. The SDK simplifies interaction with the Amadeus API by providing self-contained code examples and detailed documentation. Developers can easily make API calls, handle responses, and utilize features like pagination and logging. The SDK supports various endpoints for tasks like flight search, booking management, airport information retrieval, and travel analytics. It also offers functionalities for hotel search, booking, and sentiment analysis. Overall, the Amadeus Java SDK is a comprehensive tool for integrating Amadeus APIs into Java applications.
README:
Amadeus provides a rich set of APIs for the travel industry. For more details, check out the Amadeus for Developers Portal or the SDK class reference.
This library requires Java 1.7+ and the Gson library. You can install the SDK via Maven or Gradle:
<dependency>
<groupId>com.amadeus</groupId>
<artifactId>amadeus-java</artifactId>
<version>9.1.0</version>
</dependency>
compile "com.amadeus:amadeus-java:9.1.0"
To make your first API call, you will need to register for an Amadeus Developer Account and set up your first application.
import com.amadeus.Amadeus;
import com.amadeus.Params;
import com.amadeus.exceptions.ResponseException;
import com.amadeus.referenceData.Locations;
import com.amadeus.resources.Location;
public class AmadeusExample {
public static void main(String[] args) throws ResponseException {
Amadeus amadeus = Amadeus
.builder("REPLACE_BY_YOUR_API_KEY", "REPLACE_BY_YOUR_API_SECRET")
.build();
Location[] locations = amadeus.referenceData.locations.get(Params
.with("keyword", "LON")
.and("subType", Locations.ANY));
System.out.println(locations);
}
}
You can find all the endpoints in self-contained code examples.
The client can be initialized directly:
//Initialize using parameters
Amadeus amadeus = Amadeus
.builder("REPLACE_BY_YOUR_API_KEY", "REPLACE_BY_YOUR_API_SECRET")
.build();
Alternatively, it can be initialized without any parameters if the environment variables AMADEUS_CLIENT_ID
and AMADEUS_CLIENT_SECRET
are present.
Amadeus amadeus = Amadeus
.builder(System.getenv())
.build();
Your credentials can be found on the Amadeus dashboard.
By default the SDK is set to test
environment. To switch to a production
(pay-as-you-go) environment, please switch the hostname as follows:
Amadeus amadeus = Amadeus
.builder(System.getenv())
.setHostname("production")
.build();
Amadeus has a large set of APIs, and our documentation is here to get you started. Head over to our reference documentation for in-depth information about every SDK method, its arguments and return types.
This library conveniently maps every API path to a similar path. For example, GET /v2/reference-data/urls/checkin-links?airlineCode=BA
would be:
amadeus.referenceData.urls.checkinLinks.get(Params.with("airlineCode", "BA"));
Similarly, to select a resource by ID, you can pass in the ID to the singular path. For example, GET v1/reference-data/locations/pois
would be:
amadeus.referenceData.locations.pointOfInterest("9CB40CB5D0").get();
You can make any arbitrary API call as well directly with the .get
method.
Keep in mind, this returns a raw Resource
.
Response response = amadeus.get("/v2/reference-data/urls/checkin-links", Params.with("airlineCode", "BA"));
response.getResult();
Or, with POST method:
Response response = amadeus.post("/v1/shopping/availability/flight-availabilities", body);
Every successful API call returns a Resource
object. The Resource
object has the raw response body (in string format) available:
Location[] locations = amadeus.referenceData.locations.get(Params
.with("keyword", "LON")
.and("subType", Locations.ANY));
// The raw response, as a string
locations[0].getResponse().getBody();
If an API endpoint supports pagination, the other pages are available under the
.next
, .previous
, .last
and .first
methods.
Location[] locations = amadeus.referenceData.locations.get(Params
.with("keyword", "LON")
.and("subType", Locations.ANY));
// Fetches the next page
Location[] locations = (Location[]) amadeus.next(locations[0]);
If a page is not available, the method will return null
.
The SDK makes it easy to add your own logger.
import java.util.logging.Logger;
// Assumes the current class is called MyLogger
private final static Logger LOGGER = Logger.getLogger(MyLogger.class.getName());
...
Amadeus amadeus = Amadeus
.builder("REPLACE_BY_YOUR_API_KEY", "REPLACE_BY_YOUR_API_SECRET")
.setLogger(LOGGER)
.build();
)
Additionally, to enable more verbose logging, you can set the appropriate level on your own logger. The easiest way would be to enable debugging via a parameter during initialization, or using the AMADEUS_LOG_LEVEL
environment variable.
Amadeus amadeus = Amadeus
.builder("REPLACE_BY_YOUR_API_KEY", "REPLACE_BY_YOUR_API_SECRET")
.setLogLevel("debug") // or warn
.build();
// Flight Inspiration Search
FlightDestination[] flightDestinations = amadeus.shopping.flightDestinations.get(Params
.with("origin", "MAD"));
// Flight Cheapest Date Search
FlightDate[] flightDates = amadeus.shopping.flightDates.get(Params
.with("origin", "MAD")
.and("destination", "MUC"));
// Flight Offers Search v2 GET
FlightOfferSearch[] flightOffersSearches = amadeus.shopping.flightOffersSearch.get(
Params.with("originLocationCode", "SYD")
.and("destinationLocationCode", "BKK")
.and("departureDate", "2023-11-01")
.and("returnDate", "2023-11-08")
.and("adults", 2)
.and("max", 3));
// Flight Offers Search v2 POST
// body can be a String version of your JSON or a JsonObject
FlightOfferSearch[] flightOffersSearches = amadeus.shopping.flightOffersSearch.post(body);
// Flight Order Management
// The flightOrderID comes from the Flight Create Orders (in test environment it's temporary)
// Retrieve a flight order
FlightOrder order = amadeus.booking.flightOrder("eJzTd9f3NjIJdzUGAAp%2fAiY=").get();
// Cancel a flight order
Response order = amadeus.booking.flightOrder("eJzTd9f3NjIJdzUGAAp%2fAiY=").delete();
// Flight Offers price
FlightPrice[] flightPricing = amadeus.shopping.flightOffersSearch.pricing.post(
body,
Params.with("include", "other-services")
.and("forceClass", "false"));
// Flight Choice Prediction
// Note that the example calls 2 APIs: Flight Offers Search & Flight Choice Prediction
FlightOfferSearch[] flightOffers = amadeus.shopping.flightOffersSearch.get(
Params.with("originLocationCode", "NYC")
.and("destinationLocationCode", "MAD")
.and("departureDate", "2024-04-01")
.and("returnDate", "2024-04-08")
.and("adults", 1));
// Using a JSonObject
JsonObject result = flightOffers[0].getResponse().getResult();
FlightOfferSearch[] flightOffersPrediction = amadeus.shopping.flightOffers.prediction.post(result);
// Using a String
String body = flightOffers[0].getResponse().getBody();
FlightOfferSearch[] flightOffersPrediction = amadeus.shopping.flightOffers.prediction.post(body);
// Flight Check-in Links
CheckinLink[] checkinLinks = amadeus.referenceData.urls.checkinLinks.get(Params
.with("airlineCode", "BA"));
// Airline Code LookUp
Airline[] airlines = amadeus.referenceData.airlines.get(Params
.with("airlineCodes", "BA"));
// Airport & City Search (autocomplete)
// Find all the cities and airports starting by the keyword 'LON'
Location[] locations = amadeus.referenceData.locations.get(Params
.with("keyword", "LON")
.and("subType", Locations.ANY));
// Get a specific city or airport based on its id
Location location = amadeus.referenceData
.location("ALHR").get();
// Airport Nearest Relevant (for London)
Location[] locations = amadeus.referenceData.locations.airports.get(Params
.with("latitude", 0.1278)
.and("longitude", 51.5074));
// City Search
City[] cities = amadeus.referenceData.locations.cities.get(Params
.with("keyword","PARIS"));
// Flight Most Booked Destinations
AirTraffic[] airTraffics = amadeus.travel.analytics.airTraffic.booked.get(Params
.with("originCityCode", "MAD")
.and("period", "2017-08"));
// Flight Most Traveled Destinations
AirTraffic[] airTraffics = amadeus.travel.analytics.airTraffic.traveled.get(Params
.with("originCityCode", "MAD")
.and("period", "2017-01"));
// Flight Busiest Traveling Period
Period[] busiestPeriods = amadeus.travel.analytics.airTraffic.busiestPeriod.get(Params
.with("cityCode", "MAD")
.and("period", "2017")
.and("direction", BusiestPeriod.ARRIVING));
// Points of Interest
// What are the popular places in Barcelona (based a geo location and a radius)
PointOfInterest[] pointsOfInterest = amadeus.referenceData.locations.pointsOfInterest.get(Params
.with("latitude", "41.39715")
.and("longitude", "2.160873"));
// What are the popular places in Barcelona? (based on a square)
PointOfInterest[] pointsOfInterest = amadeus.referenceData.locations.pointsOfInterest.bySquare.get(Params
.with("north", "41.397158")
.and("west", "2.160873")
.and("south", "41.394582")
.and("east", "2.177181"));
// Returns a single Point of Interest from a given id
PointOfInterest pointOfInterest = amadeus.referenceData.locations.pointOfInterest("9CB40CB5D0").get();
// Tours and Activities
// What are the popular activities in Barcelona (based a geo location and a radius)
Activity[] activities = amadeus.shopping.activities.get(Params
.with("latitude", "41.39715")
.and("longitude", "2.160873"));
// What are the popular activities in Barcelona? (based on a square)
Activity[] activities = amadeus.shopping.activities.bySquare.get(Params
.with("north", "41.397158")
.and("west", "2.160873")
.and("south", "41.394582")
.and("east", "2.177181"));
// Returns a single activity from a given id
Activity activity = amadeus.shopping.activity("4615").get();
// What's the likelihood flights from this airport will leave on time?
Prediction AirportOnTime = amadeus.airport.predictions.onTime.get(Params
.with("airportCode", "NCE")
.and("date", "2024-04-01"));
// What's the likelihood of a given flight to be delayed?
Prediction[] flightDelay = amadeus.travel.predictions.flightDelay.get(Params
.with("originLocationCode", "NCE")
.and("destinationLocationCode", "IST")
.and("departureDate", "2020-08-01")
.and("departureTime", "18:20:00")
.and("arrivalDate", "2020-08-01")
.and("arrivalTime", "22:15:00")
.and("aircraftCode", "321")
.and("carrierCode", "TK")
.and("flightNumber", "1816")
.and("duration", "PT31H10M"));
// Flight Create Orders to book a flight
// Using a JSonObject or String
FlightOrder createdOrder = amadeus.booking.flightOrders.post(body);
// Using a JsonObject for flight offer and Traveler[] as traveler information
// see example at src/main/java/examples/flight/createorders/FlightCreateOrders.java
FlightOrder createdOrder = amadeus.booking.flightOrders.post(flightOffersSearches, travelerArray);
// What is the the seat map of a given flight?
SeatMap[] seatmap = amadeus.shopping.seatMaps.get(Params
.with("flight-orderId", "eJzTd9f3NjIJdzUGAAp%2fAiY="));
// What is the the seat map of a given flight?
// The body can be a String version of your JSON or a JsonObject
SeatMap[] seatmap = amadeus.shopping.seatMaps.post(body);
// Trip Purpose Prediction
Prediction tripPurpose = amadeus.travel.predictions.tripPurpose.get(Params
.with("originLocationCode", "NYC")
.and("destinationLocationCode", "MAD")
.and("departureDate", "2024-04-01")
.and("returnDate", "2024-04-08"));
// Travel Recommendations
Location destinations = amadeus.referenceData.recommendedLocations.get(Params
.with("cityCodes", "PAR")
.and("travelerCountryCode", "FR"));
// On Demand Flight Status
DatedFlight[] flightStatus = amadeus.schedule.flights.get(Params
.with("carrierCode", "AZ")
.and("flightNumber", "319")
.and("scheduledDepartureDate", "2024-03-13"));
// Flight Price Analysis
ItineraryPriceMetric[] metrics = amadeus.analytics.itineraryPriceMetrics.get(Params
.with("originIataCode", "MAD")
.and("destinationIataCode", "CDG")
.and("departureDate", "2024-03-21"));
// Airport Routes
Destination[] directDestinations = amadeus.airport.directDestinations.get(Params
.with("departureAirportCode","MAD")
.and("max","2"));
// Flight Availabilites Search POST
// body can be a String version of your JSON or a JsonObject
FlightAvailability[] flightAvailabilities
= amadeus.shopping.availability.flightAvailabilities.post(body);
// Location Score GET
ScoredLocation[] scoredLocations
= amadeus.location.analytics.categoryRatedAreas.get(Params
.with("latitude", "41.397158")
.and("longitude", "2.160873"));
// Branded Fares Upsell Post
// body can be a String version of your JSON or a JsonObject
FlightOfferSearch[] upsellFlightOffers
= amadeus.shopping.flightOffers.upselling.post(body);
// Hotel List
// Get list of hotels by hotel id
Hotel[] hotels = amadeus.referenceData.locations.hotels.byHotels.get(Params
.with("hotelIds", "ADPAR001"));
// Get list of hotels by city code
Hotel[] hotels = amadeus.referenceData.locations.hotels.byCity.get(Params
.with("cityCode", "PAR"));
// Get list of hotels by a geocode
Hotel[] hotels = amadeus.referenceData.locations.hotels.byGeocode.get(Params
.with("longitude", 2.160873)
.and("latitude", 41.397158));
// Hotel autocomplete names
Hotel[] result = amadeus.referenceData.locations.hotel.get(Params
.with("keyword", "PARI")
.and("subType", "HOTEL_GDS")
.and("countryCode", "FR")
.and("lang", "EN")
.and("max", "20"));
// Hotel Offers Search API v3
// Get multiple hotel offers
HotelOfferSearch[] offers = amadeus.shopping.hotelOffersSearch.get(Params
.with("hotelIds", "MCLONGHM")
.and("adults", 1)
.and("checkInDate", "2023-11-22")
.and("roomQuantity", 1)
.and("paymentPolicy", "NONE")
.and("bestRateOnly", true));
// Get hotel offer pricing by offer id
HotelOfferSearch offer = amadeus.shopping.hotelOfferSearch("QF3MNOBDQ8").get();
// Hotel Booking
// The body can be a String version of your JSON or a JsonObject
HotelBooking[] hotel = amadeus.booking.hotelBookings.post(body);
// Hotel Booking v2
HotelOrder hotel = amadeus.booking.hotelOrders.post(body);
// Hotel Ratings / Sentiments
HotelSentiment[] hotelSentiments = amadeus.ereputation.hotelSentiments.get(Params.with("hotelIds", "ELONMFS,ADNYCCTB"));
// Airline Routes
// Get airline destinations
Destination[] destinations = amadeus.airline.destinations.get(Params
.with("airlineCode", "BA")
.and("max", 2));
// Transfer Search
TransferOffersPost[] transfers = amadeus.shopping.transferOffers.post(body);
// Transfer Booking
TransferOrder transfers = amadeus.ordering.transferOrders.post(body, params);
// Transfer Management
TransferCancellation transfers = amadeus.ordering.transferOrder("123456").transfers.cancellation.post(params);
Want to contribute? Read our Contributors Guide for guidance on installing and running this code in a development environment.
This library is released under the MIT License.
You can find us on StackOverflow or join our developer community on Discord.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for amadeus-java
Similar Open Source Tools
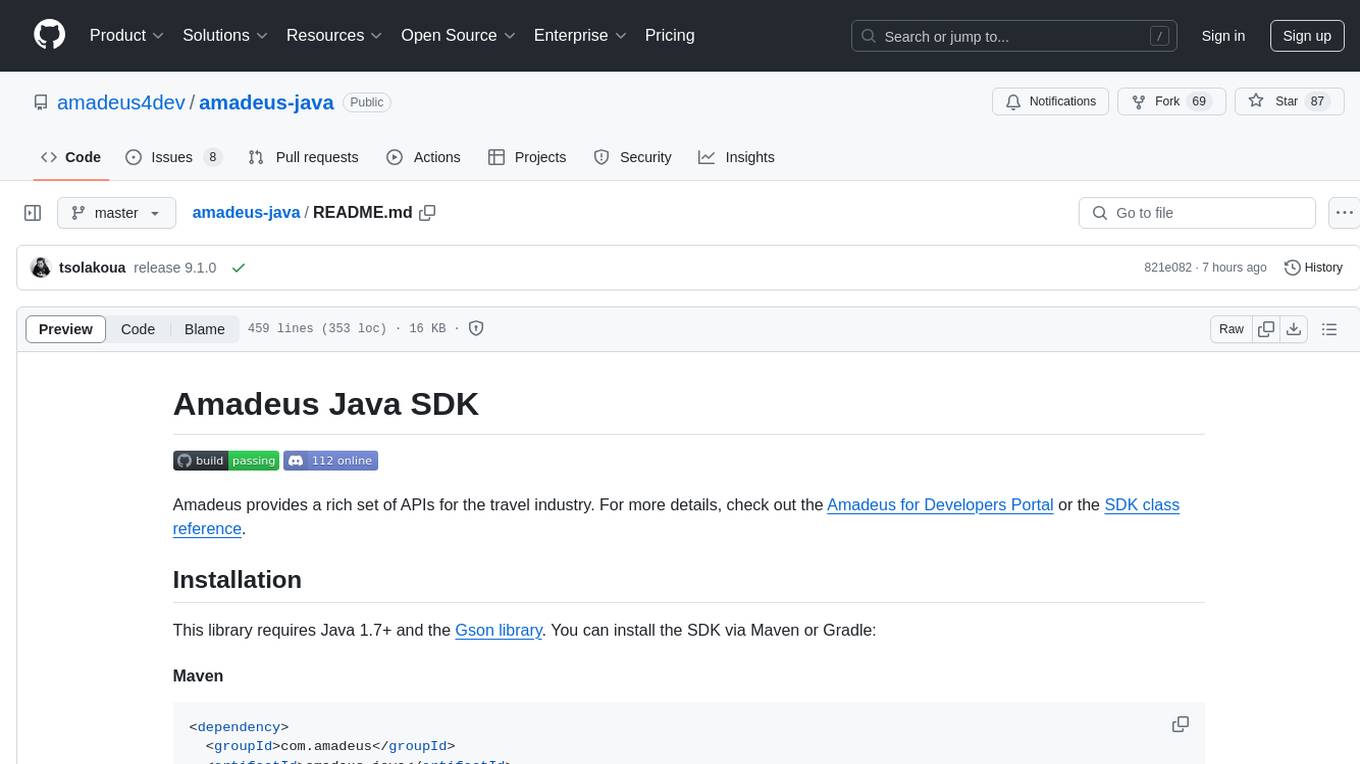
amadeus-java
Amadeus Java SDK provides a rich set of APIs for the travel industry, allowing developers to access various functionalities such as flight search, booking, airport information, and more. The SDK simplifies interaction with the Amadeus API by providing self-contained code examples and detailed documentation. Developers can easily make API calls, handle responses, and utilize features like pagination and logging. The SDK supports various endpoints for tasks like flight search, booking management, airport information retrieval, and travel analytics. It also offers functionalities for hotel search, booking, and sentiment analysis. Overall, the Amadeus Java SDK is a comprehensive tool for integrating Amadeus APIs into Java applications.
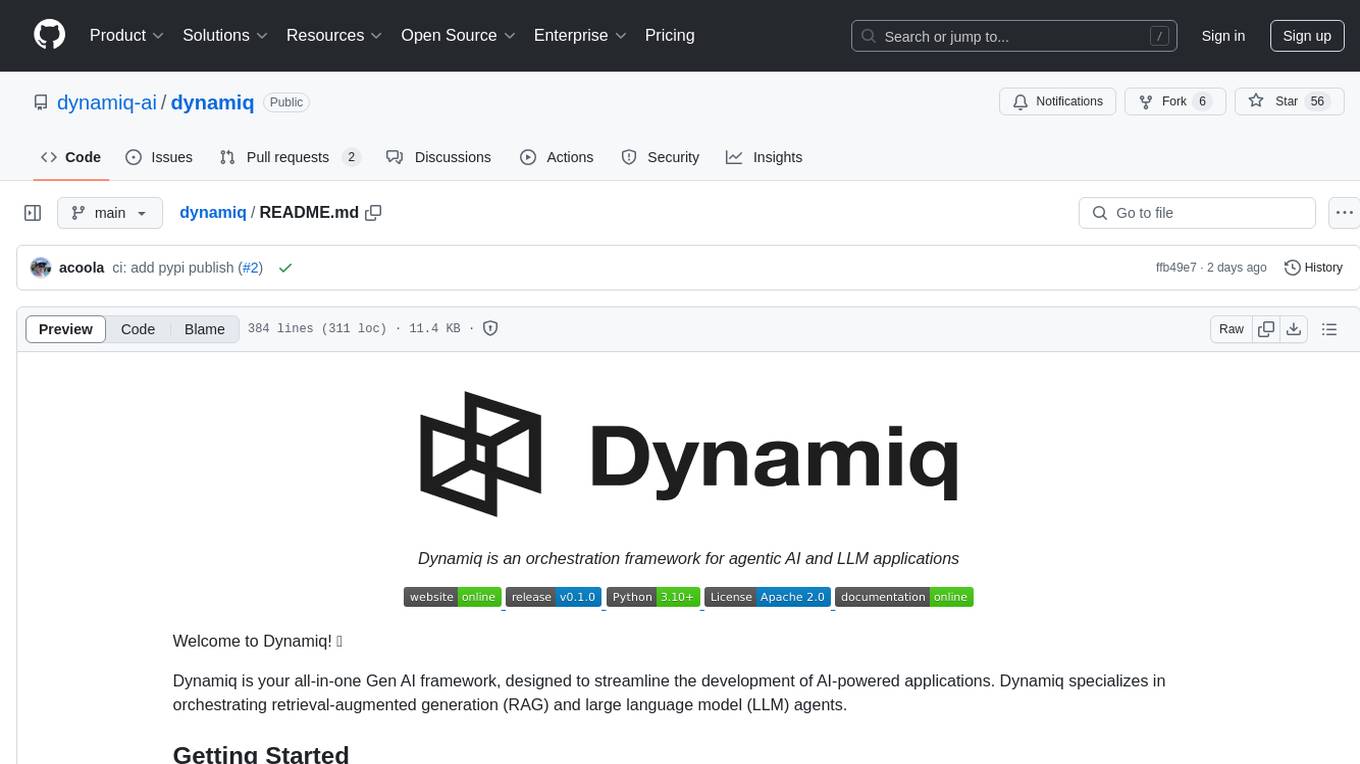
dynamiq
Dynamiq is an orchestration framework designed to streamline the development of AI-powered applications, specializing in orchestrating retrieval-augmented generation (RAG) and large language model (LLM) agents. It provides an all-in-one Gen AI framework for agentic AI and LLM applications, offering tools for multi-agent orchestration, document indexing, and retrieval flows. With Dynamiq, users can easily build and deploy AI solutions for various tasks.
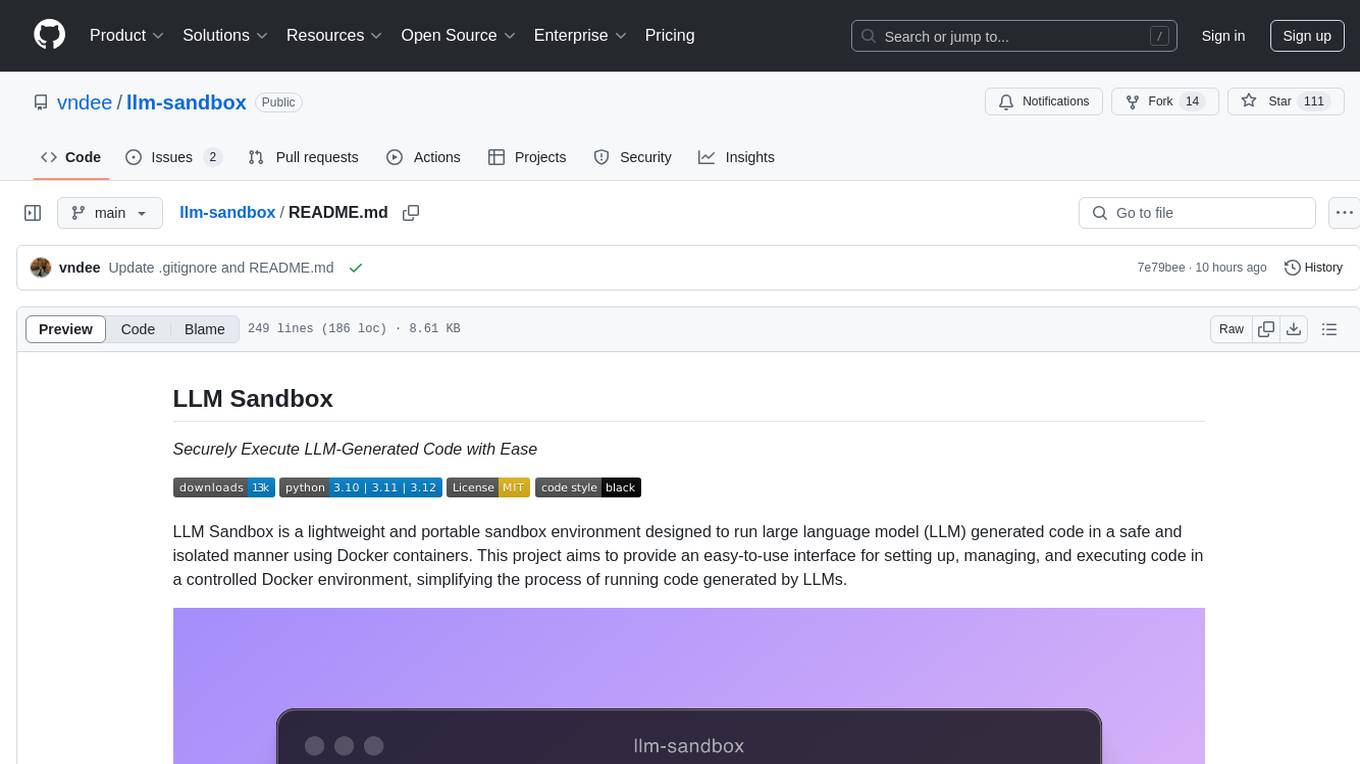
llm-sandbox
LLM Sandbox is a lightweight and portable sandbox environment designed to securely execute large language model (LLM) generated code in a safe and isolated manner using Docker containers. It provides an easy-to-use interface for setting up, managing, and executing code in a controlled Docker environment, simplifying the process of running code generated by LLMs. The tool supports multiple programming languages, offers flexibility with predefined Docker images or custom Dockerfiles, and allows scalability with support for Kubernetes and remote Docker hosts.
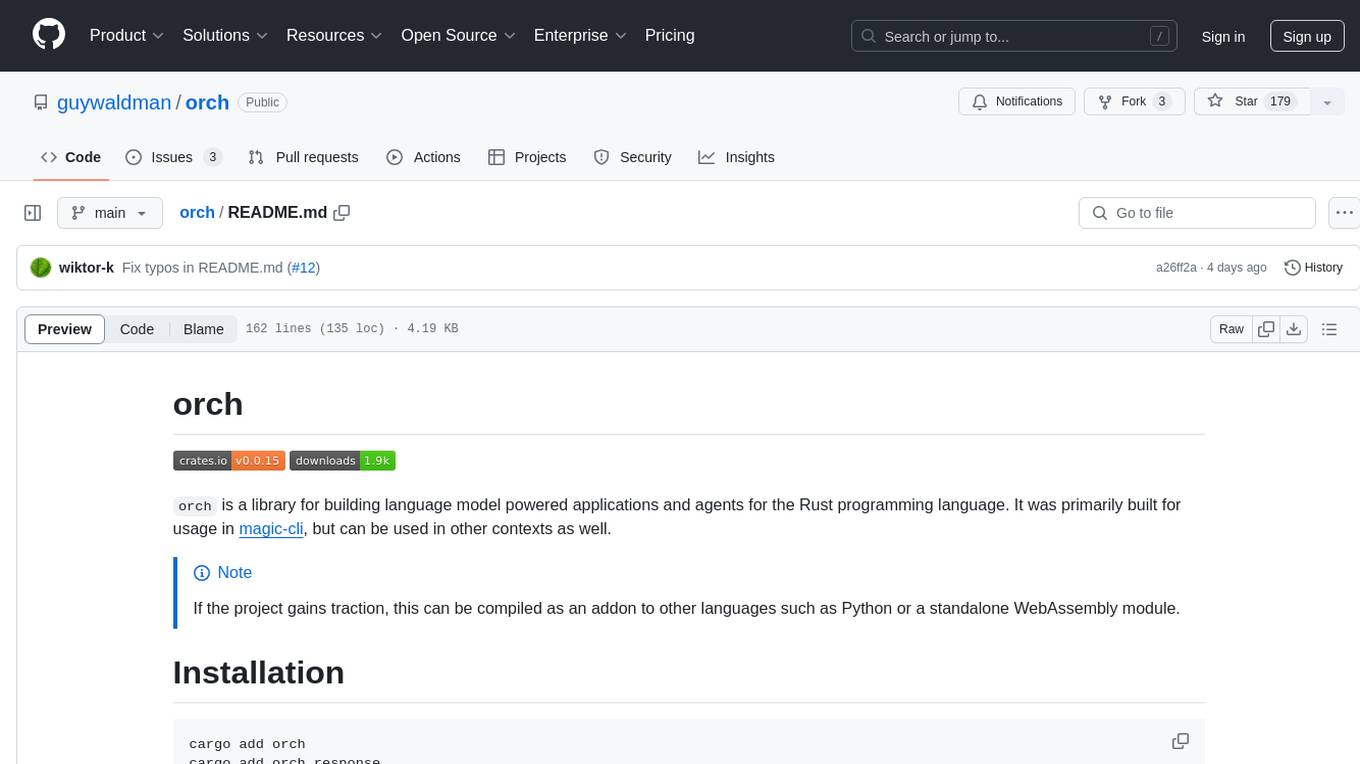
orch
orch is a library for building language model powered applications and agents for the Rust programming language. It can be used for tasks such as text generation, streaming text generation, structured data generation, and embedding generation. The library provides functionalities for executing various language model tasks and can be integrated into different applications and contexts. It offers flexibility for developers to create language model-powered features and applications in Rust.
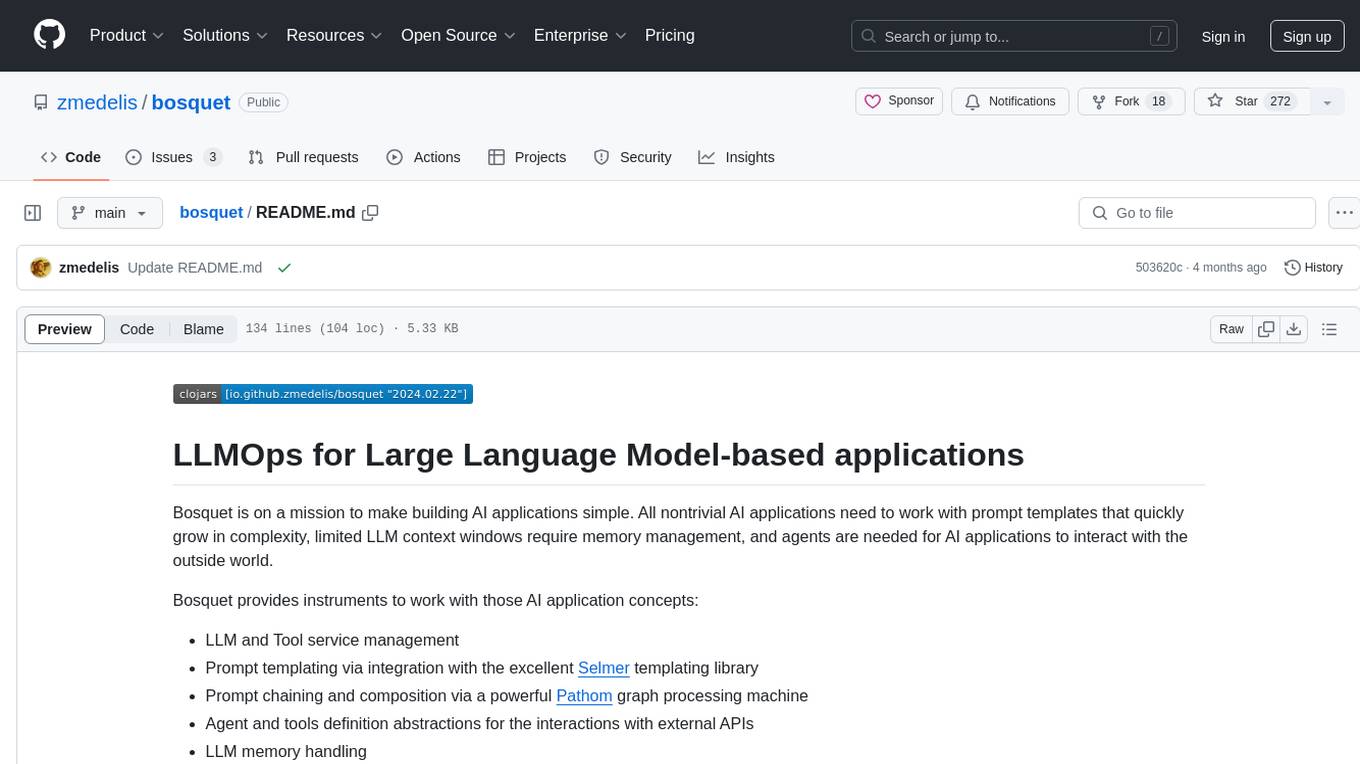
bosquet
Bosquet is a tool designed for LLMOps in large language model-based applications. It simplifies building AI applications by managing LLM and tool services, integrating with Selmer templating library for prompt templating, enabling prompt chaining and composition with Pathom graph processing, defining agents and tools for external API interactions, handling LLM memory, and providing features like call response caching. The tool aims to streamline the development process for AI applications that require complex prompt templates, memory management, and interaction with external systems.
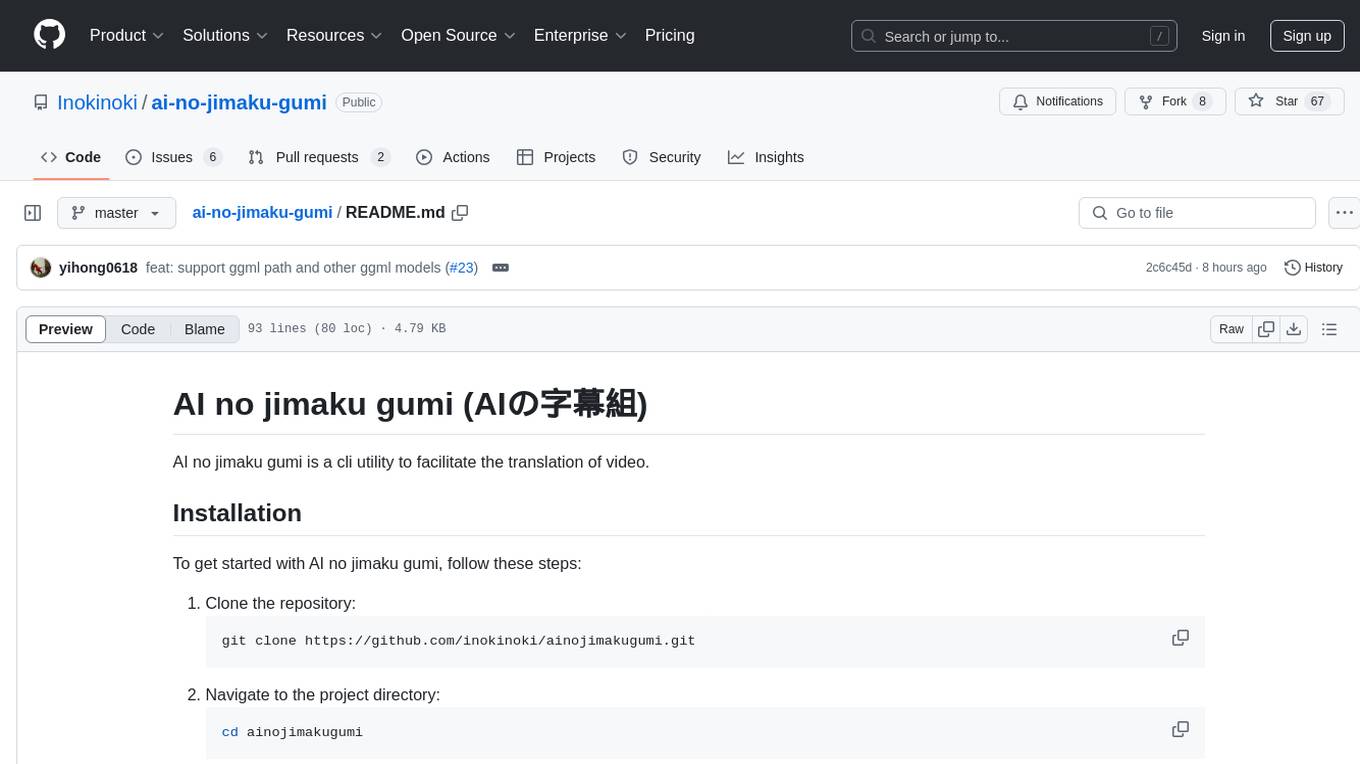
ai-no-jimaku-gumi
AI no jimaku gumi is a command-line utility designed to assist in video translation. It supports translating subtitles using AI models and provides options for different translation and subtitle sources. Users can easily set up the tool by following the installation steps and use it to translate videos to different languages with customizable settings. The tool currently supports DeepL and llm translation backends and SRT subtitle export. It aims to simplify the process of adding subtitles to videos by leveraging AI technology.
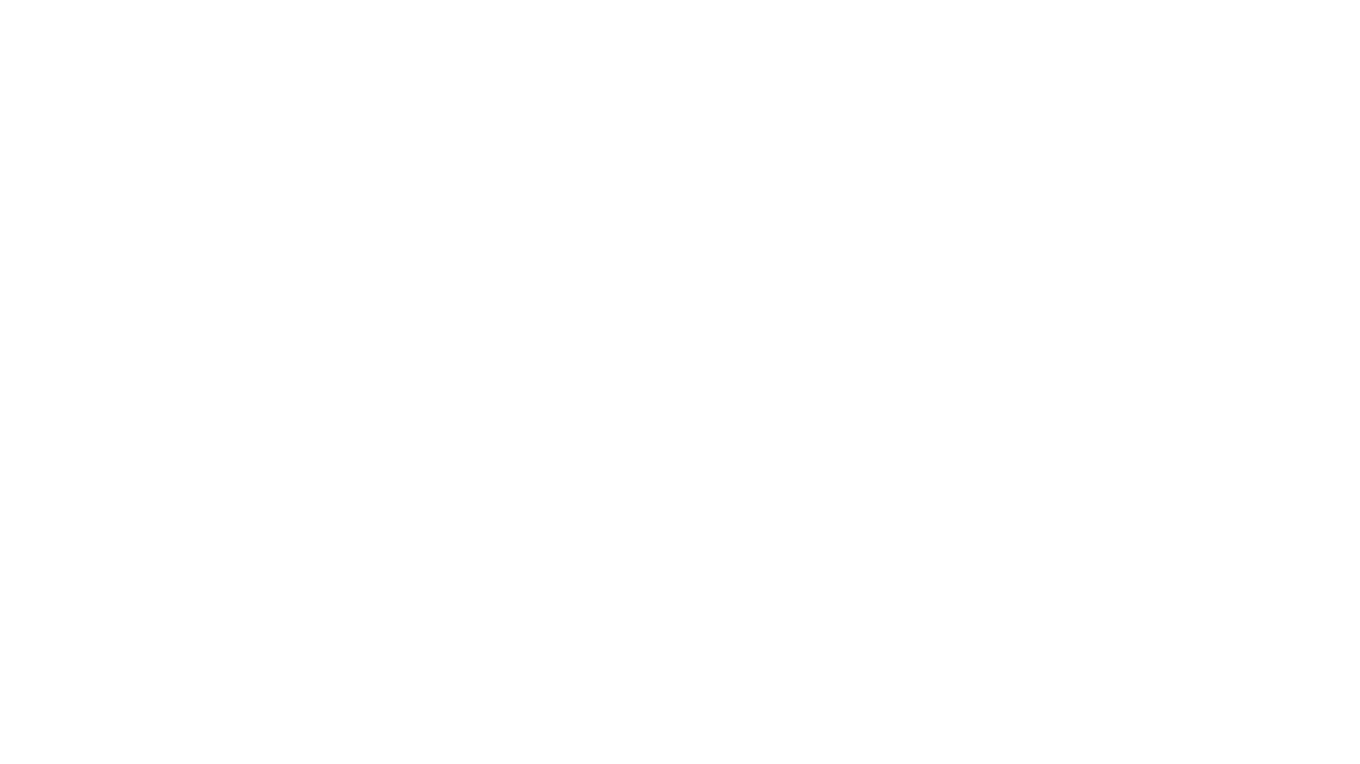
lagent
Lagent is a lightweight open-source framework that allows users to efficiently build large language model(LLM)-based agents. It also provides some typical tools to augment LLM. The overview of our framework is shown below:
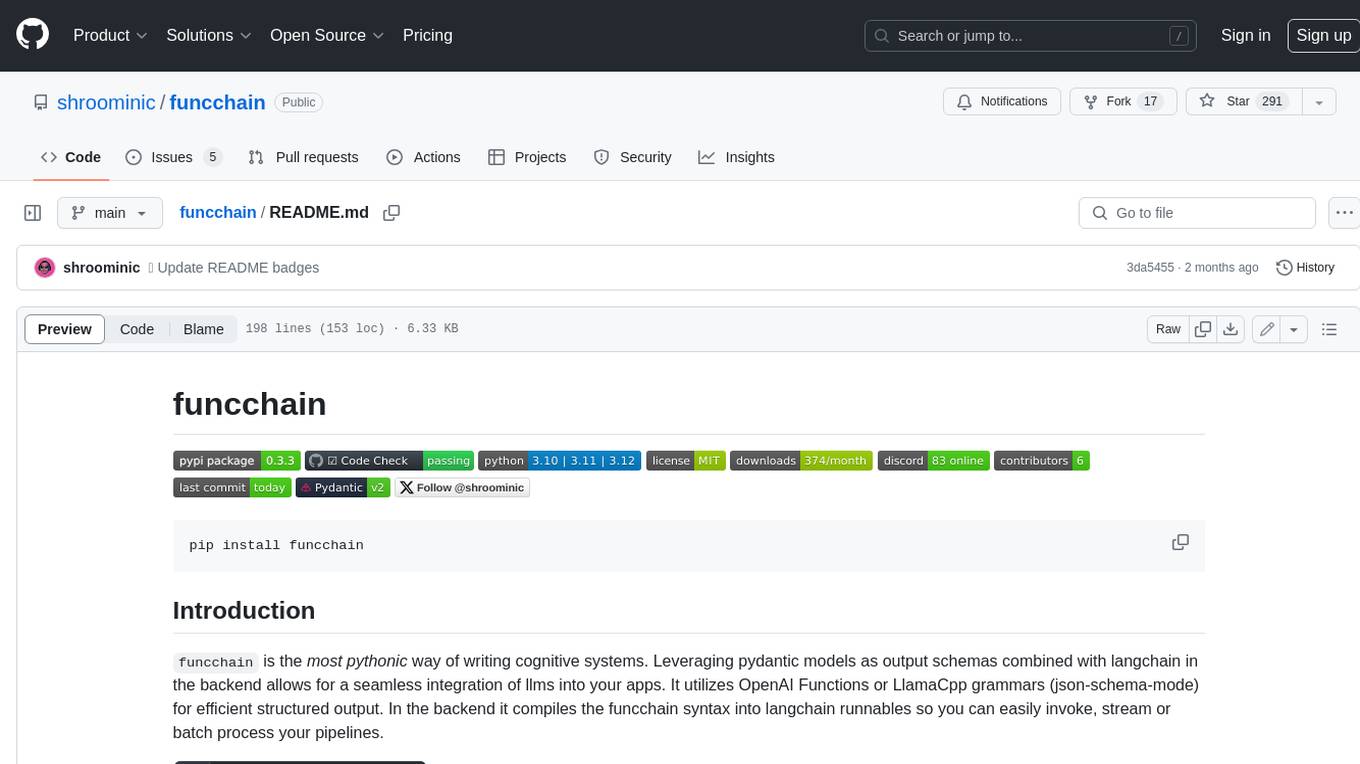
funcchain
Funcchain is a Python library that allows you to easily write cognitive systems by leveraging Pydantic models as output schemas and LangChain in the backend. It provides a seamless integration of LLMs into your apps, utilizing OpenAI Functions or LlamaCpp grammars (json-schema-mode) for efficient structured output. Funcchain compiles the Funcchain syntax into LangChain runnables, enabling you to invoke, stream, or batch process your pipelines effortlessly.
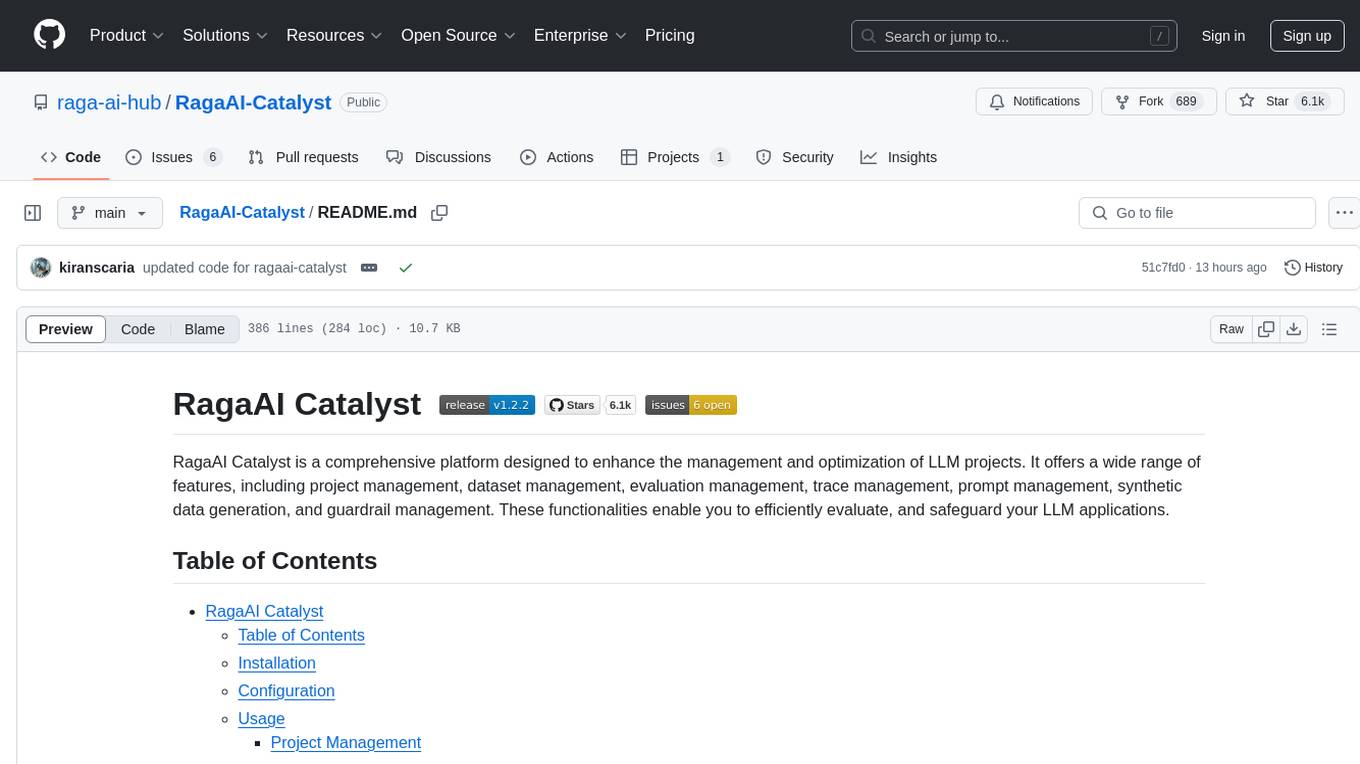
RagaAI-Catalyst
RagaAI Catalyst is a comprehensive platform designed to enhance the management and optimization of LLM projects. It offers features such as project management, dataset management, evaluation management, trace management, prompt management, synthetic data generation, and guardrail management. These functionalities enable efficient evaluation and safeguarding of LLM applications.
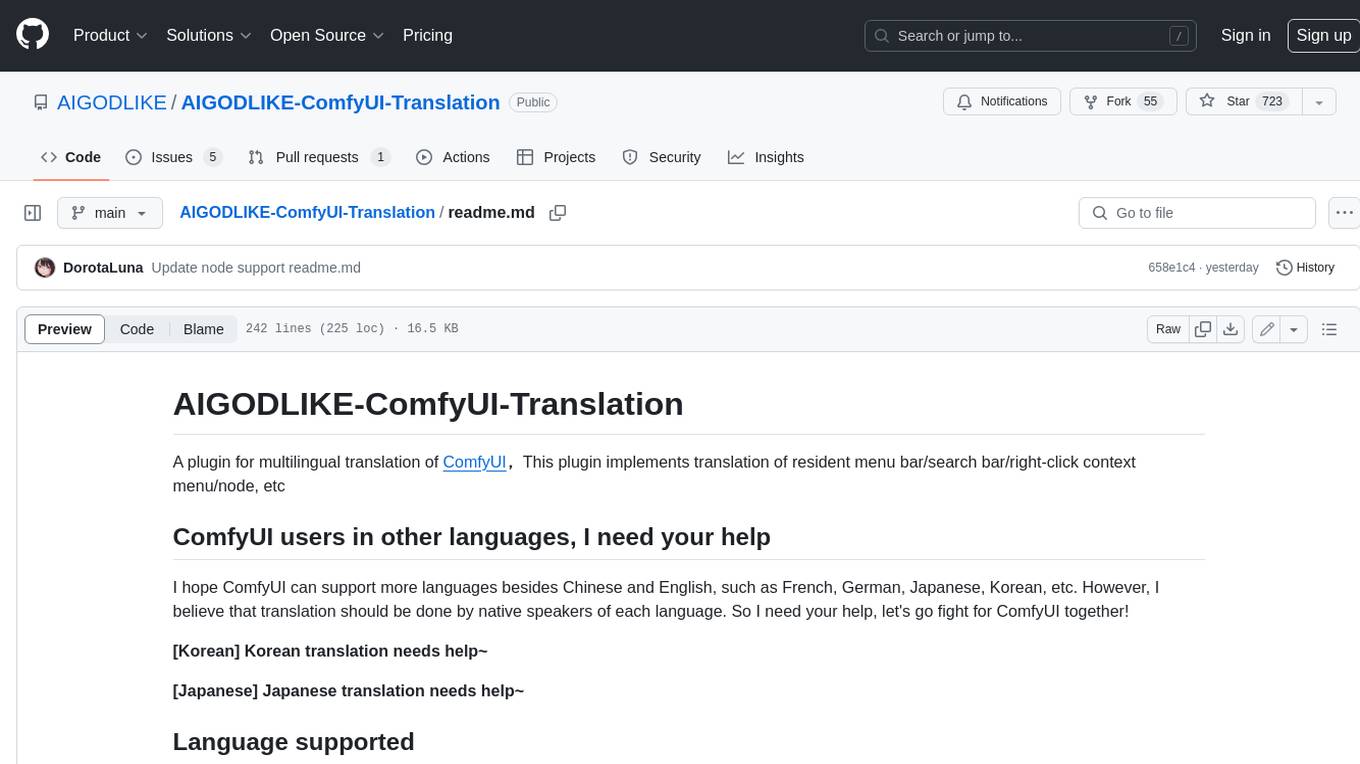
AIGODLIKE-ComfyUI-Translation
A plugin for multilingual translation of ComfyUI, This plugin implements translation of resident menu bar/search bar/right-click context menu/node, etc
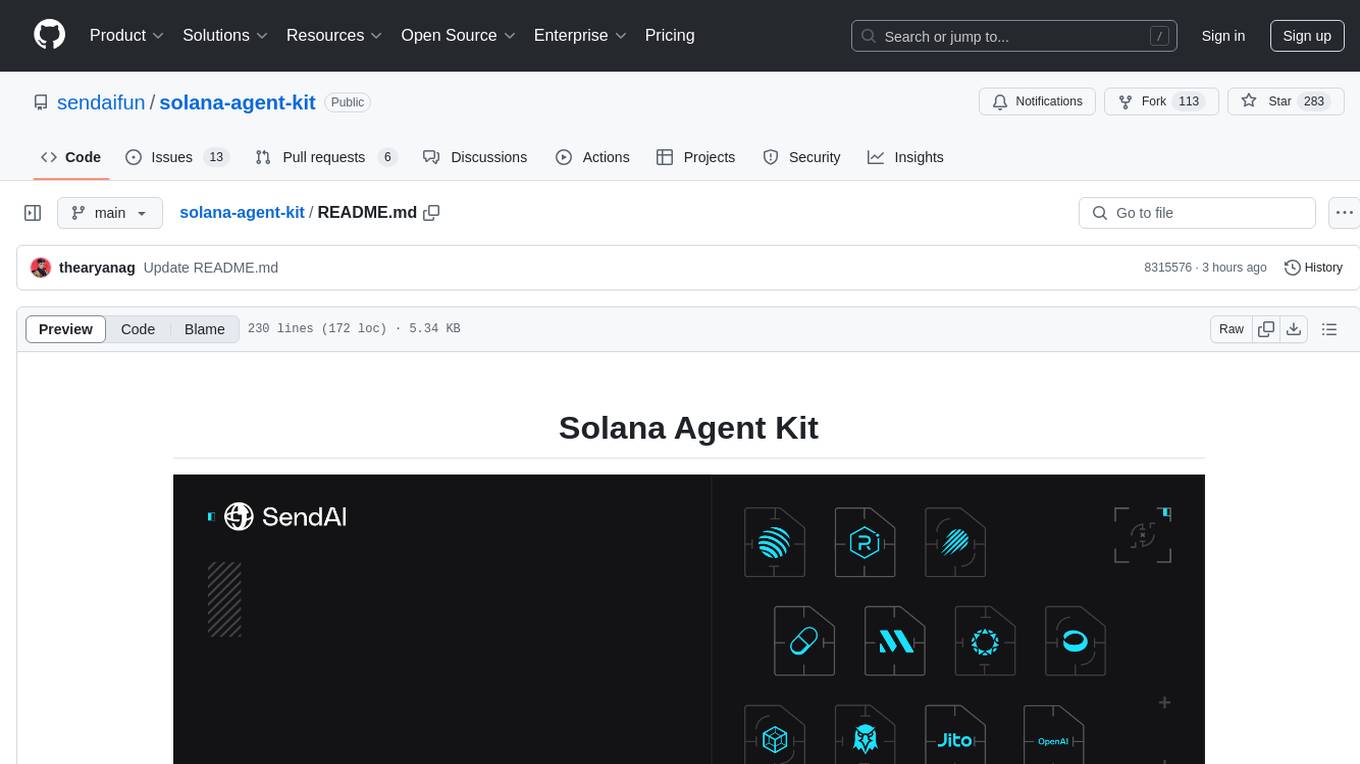
solana-agent-kit
Solana Agent Kit is an open-source toolkit designed for connecting AI agents to Solana protocols. It enables agents, regardless of the model used, to autonomously perform various Solana actions such as trading tokens, launching new tokens, lending assets, sending compressed airdrops, executing blinks, and more. The toolkit integrates core blockchain features like token operations, NFT management via Metaplex, DeFi integration, Solana blinks, AI integration features with LangChain, autonomous modes, and AI tools. It provides ready-to-use tools for blockchain operations, supports autonomous agent actions, and offers features like memory management, real-time feedback, and error handling. Solana Agent Kit facilitates tasks such as deploying tokens, creating NFT collections, swapping tokens, lending tokens, staking SOL, and sending SPL token airdrops via ZK compression. It also includes functionalities for fetching price data from Pyth and relies on key Solana and Metaplex libraries for its operations.
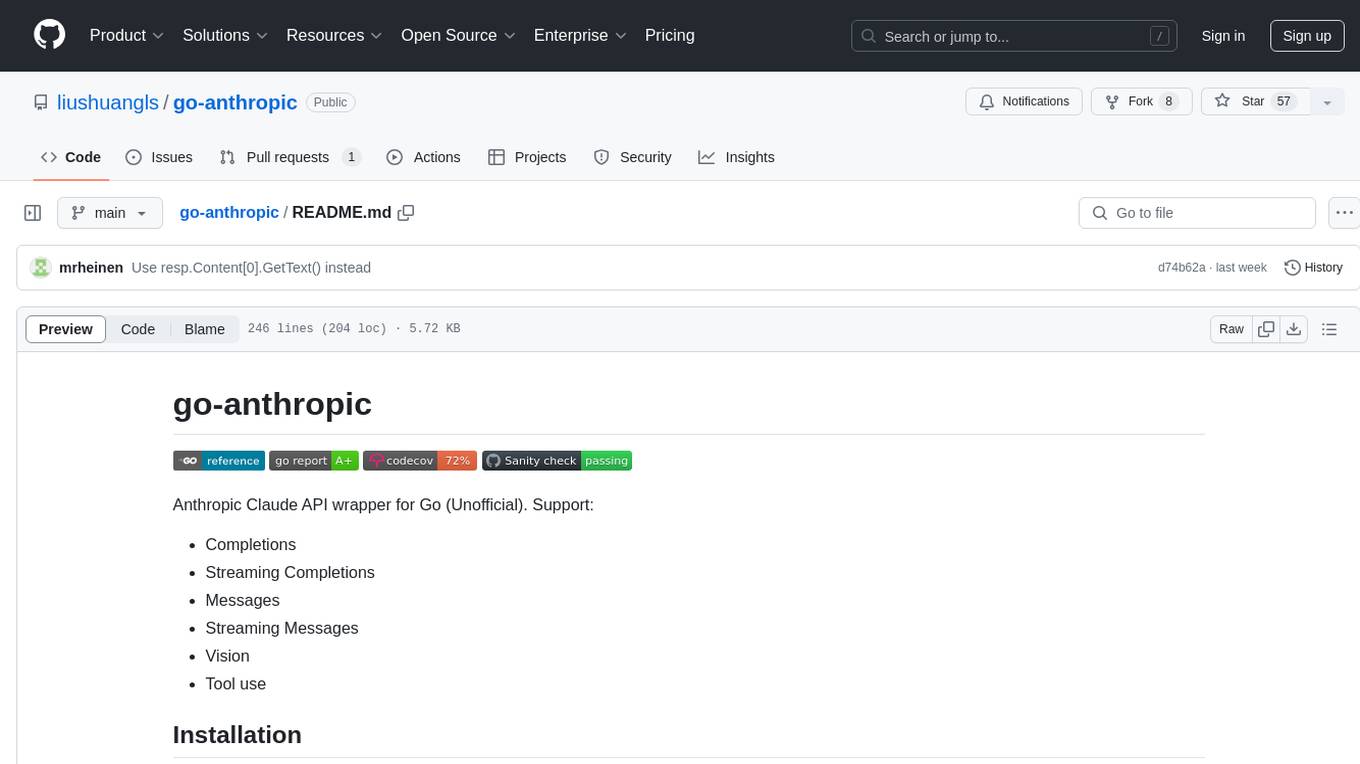
go-anthropic
Go-anthropic is an unofficial API wrapper for Anthropic Claude in Go. It supports completions, streaming completions, messages, streaming messages, vision, and tool use. Users can interact with the Anthropic Claude API to generate text completions, analyze messages, process images, and utilize specific tools for various tasks.
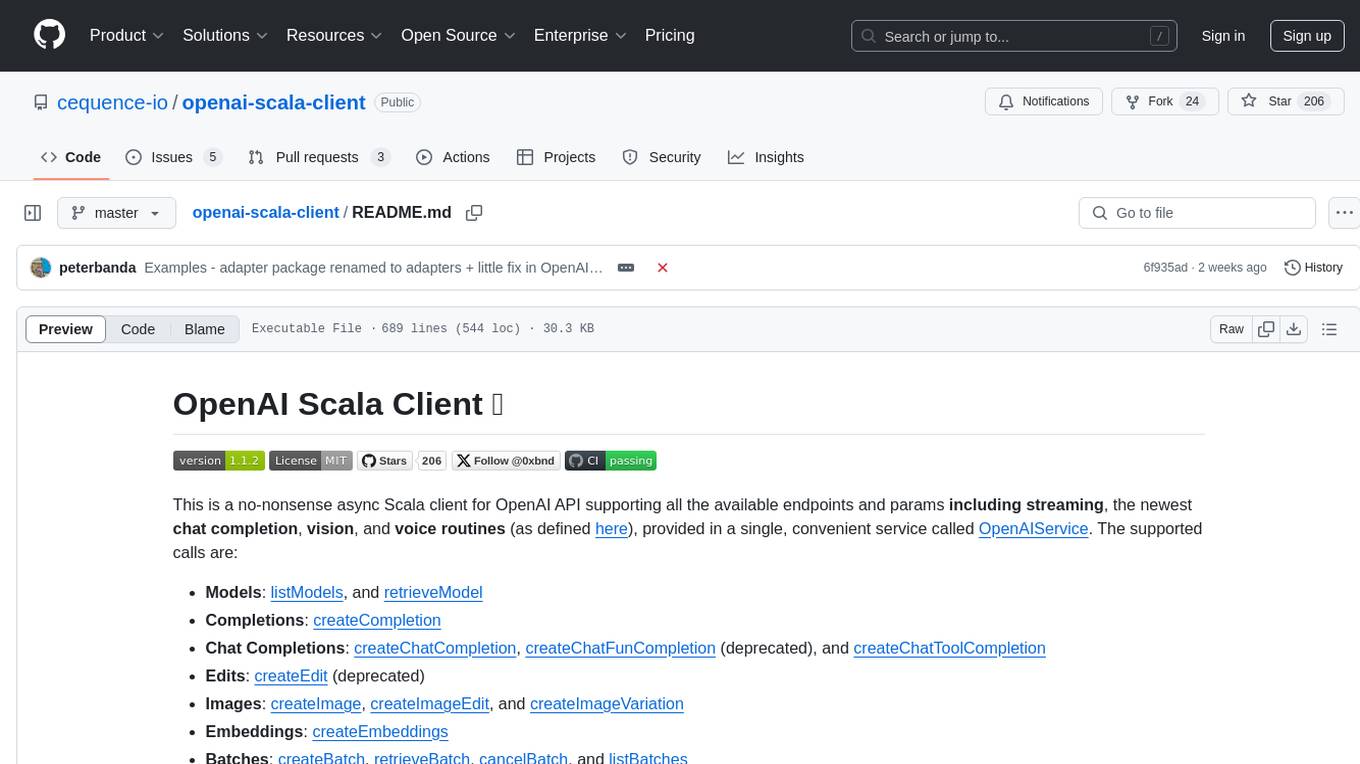
openai-scala-client
This is a no-nonsense async Scala client for OpenAI API supporting all the available endpoints and params including streaming, chat completion, vision, and voice routines. It provides a single service called OpenAIService that supports various calls such as Models, Completions, Chat Completions, Edits, Images, Embeddings, Batches, Audio, Files, Fine-tunes, Moderations, Assistants, Threads, Thread Messages, Runs, Run Steps, Vector Stores, Vector Store Files, and Vector Store File Batches. The library aims to be self-contained with minimal dependencies and supports API-compatible providers like Azure OpenAI, Azure AI, Anthropic, Google Vertex AI, Groq, Grok, Fireworks AI, OctoAI, TogetherAI, Cerebras, Mistral, Deepseek, Ollama, FastChat, and more.
For similar tasks
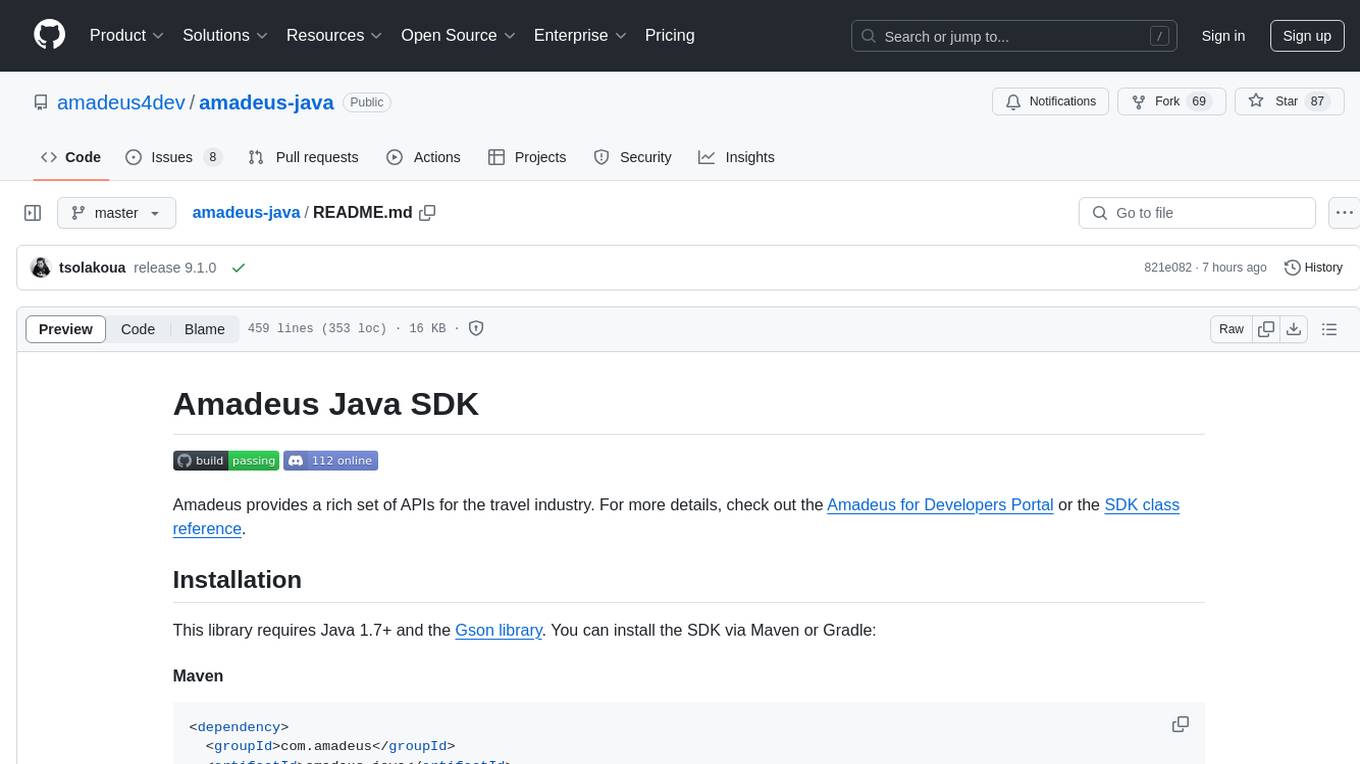
amadeus-java
Amadeus Java SDK provides a rich set of APIs for the travel industry, allowing developers to access various functionalities such as flight search, booking, airport information, and more. The SDK simplifies interaction with the Amadeus API by providing self-contained code examples and detailed documentation. Developers can easily make API calls, handle responses, and utilize features like pagination and logging. The SDK supports various endpoints for tasks like flight search, booking management, airport information retrieval, and travel analytics. It also offers functionalities for hotel search, booking, and sentiment analysis. Overall, the Amadeus Java SDK is a comprehensive tool for integrating Amadeus APIs into Java applications.
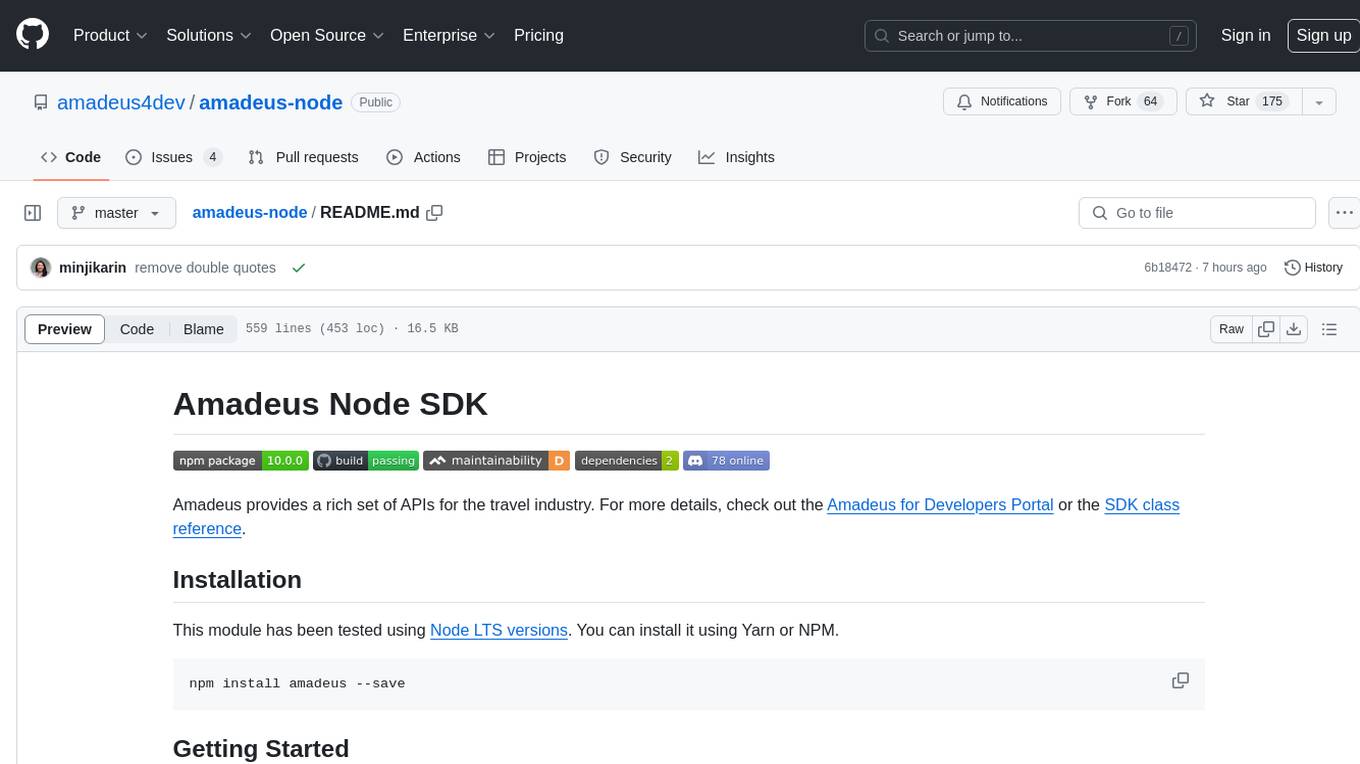
amadeus-node
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
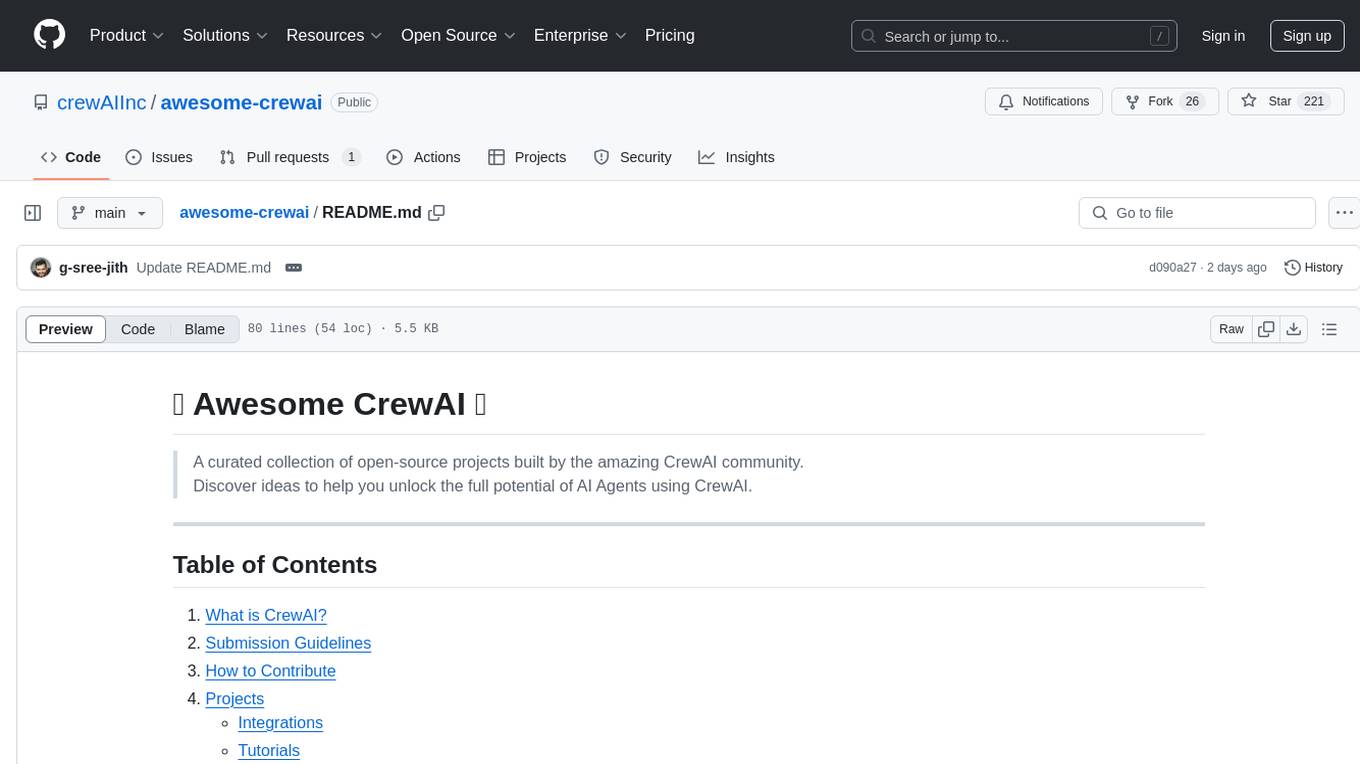
awesome-crewai
Awesome CrewAI is a curated collection of open-source projects built by the CrewAI community, aimed at unlocking the full potential of AI agents for supercharging business processes and decision-making. It includes integrations, tutorials, and tools that showcase the capabilities of CrewAI in various domains.
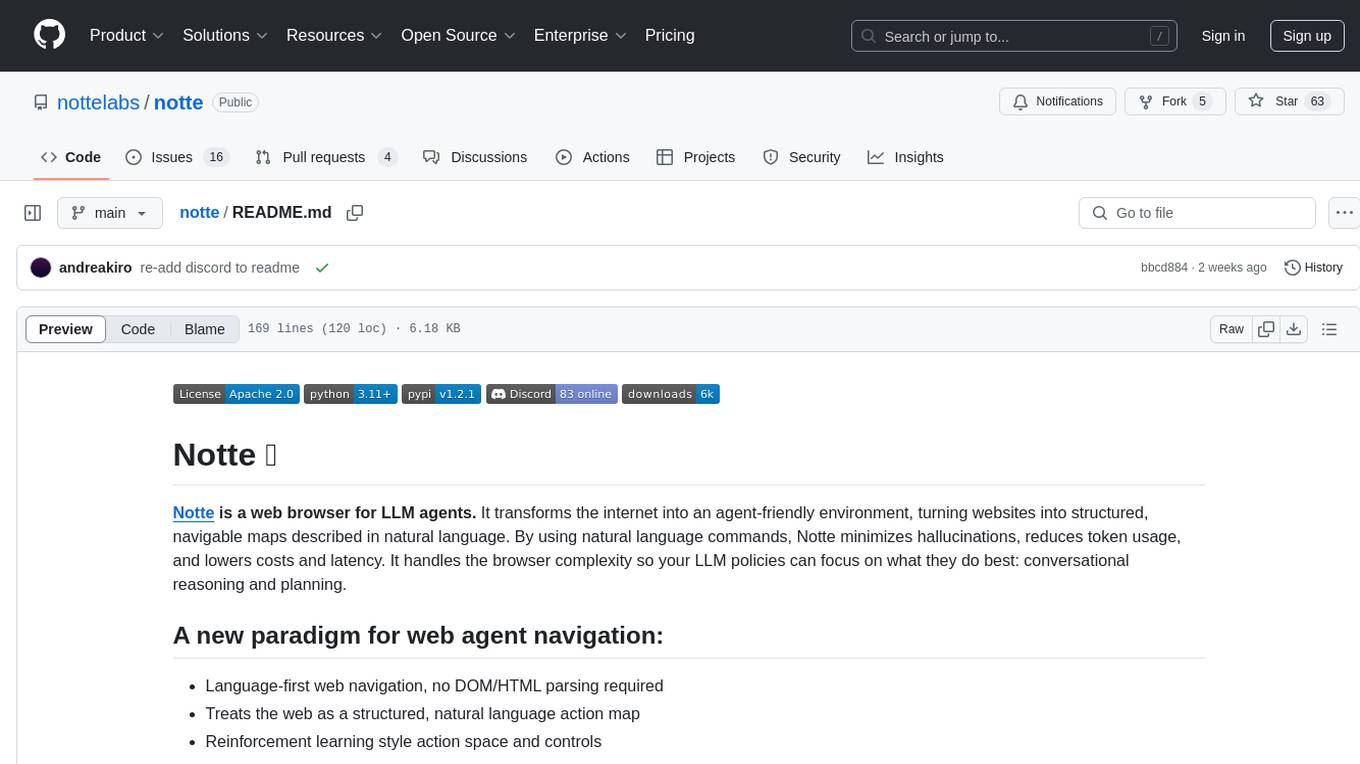
notte
Notte is a web browser designed specifically for LLM agents, providing a language-first web navigation experience without the need for DOM/HTML parsing. It transforms websites into structured, navigable maps described in natural language, enabling users to interact with the web using natural language commands. By simplifying browser complexity, Notte allows LLM policies to focus on conversational reasoning and planning, reducing token usage, costs, and latency. The tool supports various language model providers and offers a reinforcement learning style action space and controls for full navigation control.
For similar jobs
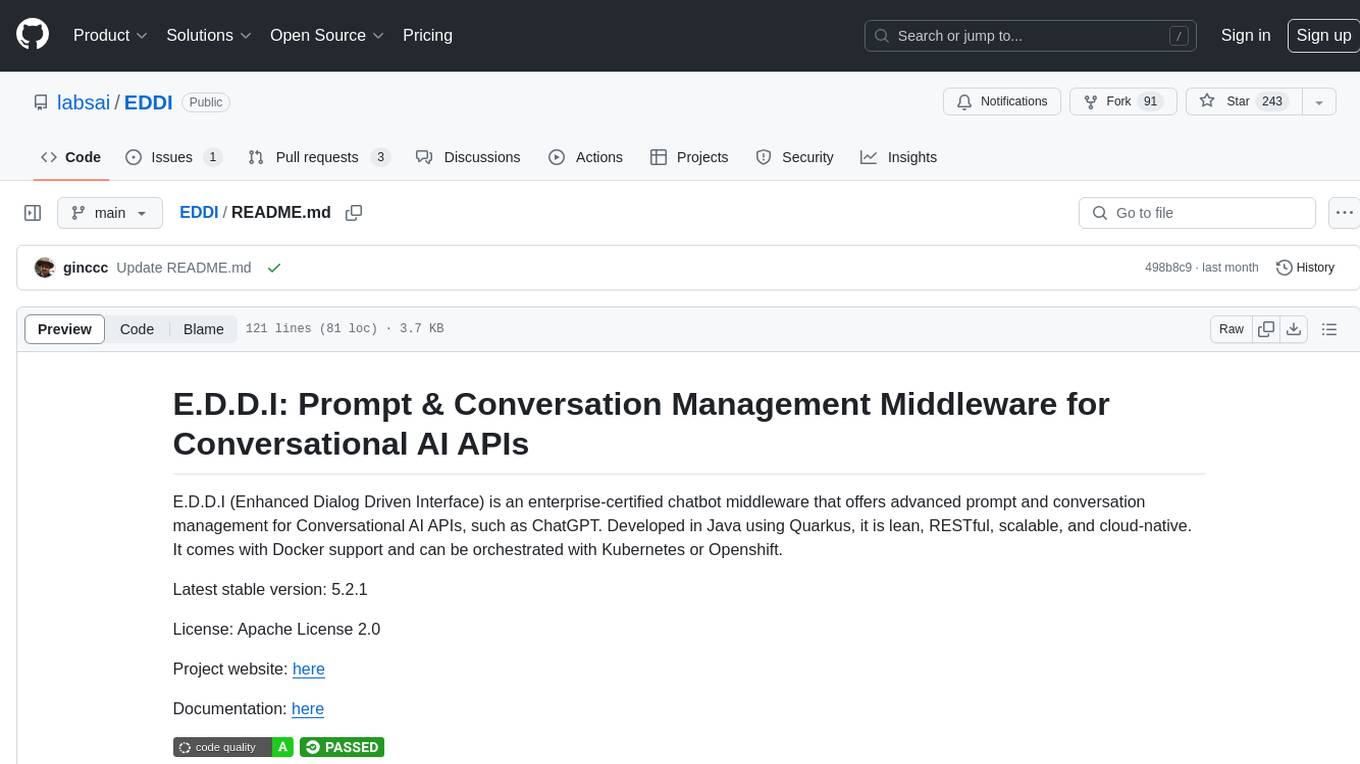
EDDI
E.D.D.I (Enhanced Dialog Driven Interface) is an enterprise-certified chatbot middleware that offers advanced prompt and conversation management for Conversational AI APIs. Developed in Java using Quarkus, it is lean, RESTful, scalable, and cloud-native. E.D.D.I is highly scalable and designed to efficiently manage conversations in AI-driven applications, with seamless API integration capabilities. Notable features include configurable NLP and Behavior rules, support for multiple chatbots running concurrently, and integration with MongoDB, OAuth 2.0, and HTML/CSS/JavaScript for UI. The project requires Java 21, Maven 3.8.4, and MongoDB >= 5.0 to run. It can be built as a Docker image and deployed using Docker or Kubernetes, with additional support for integration testing and monitoring through Prometheus and Kubernetes endpoints.
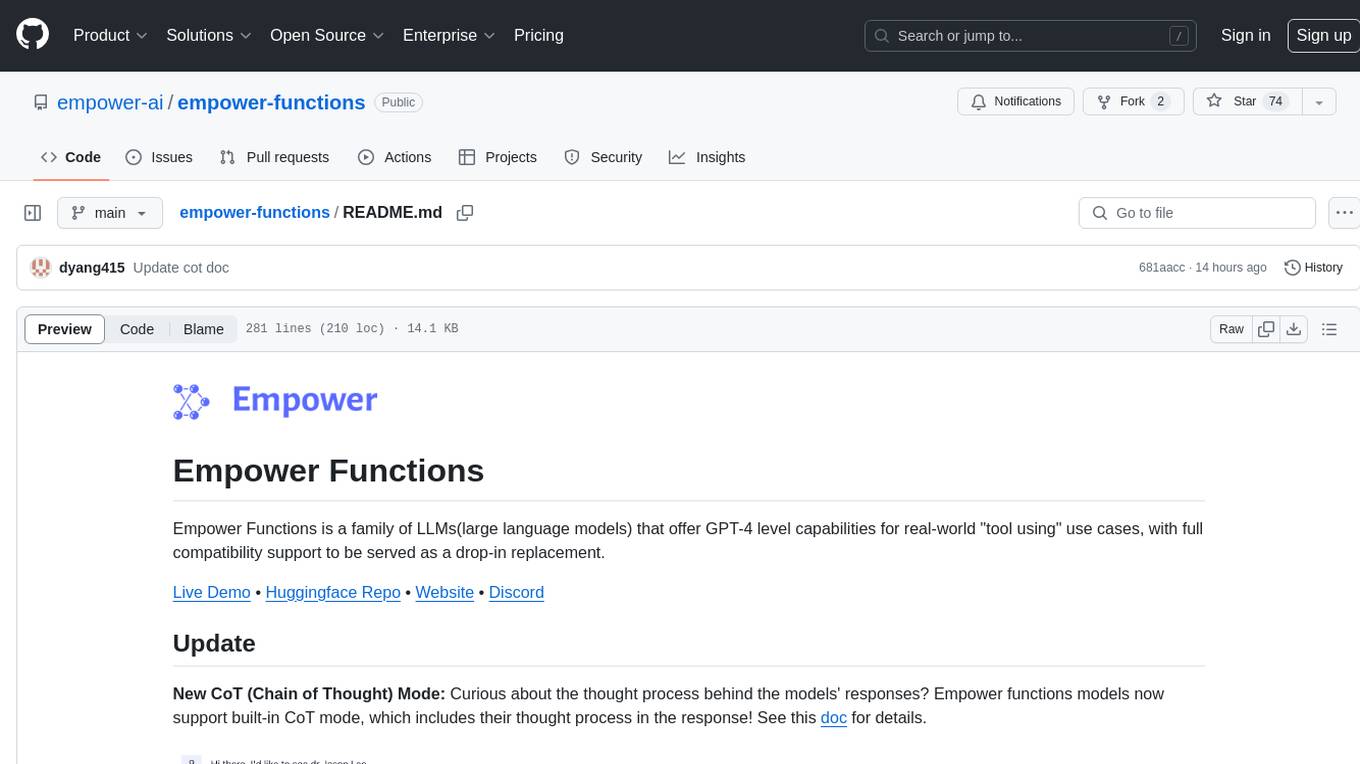
empower-functions
Empower Functions is a family of large language models (LLMs) that provide GPT-4 level capabilities for real-world 'tool using' use cases. These models offer compatibility support to be used as drop-in replacements, enabling interactions with external APIs by recognizing when a function needs to be called and generating JSON containing necessary arguments based on user inputs. This capability is crucial for building conversational agents and applications that convert natural language into API calls, facilitating tasks such as weather inquiries, data extraction, and interactions with knowledge bases. The models can handle multi-turn conversations, choose between tools or standard dialogue, ask for clarification on missing parameters, integrate responses with tool outputs in a streaming fashion, and efficiently execute multiple functions either in parallel or sequentially with dependencies.
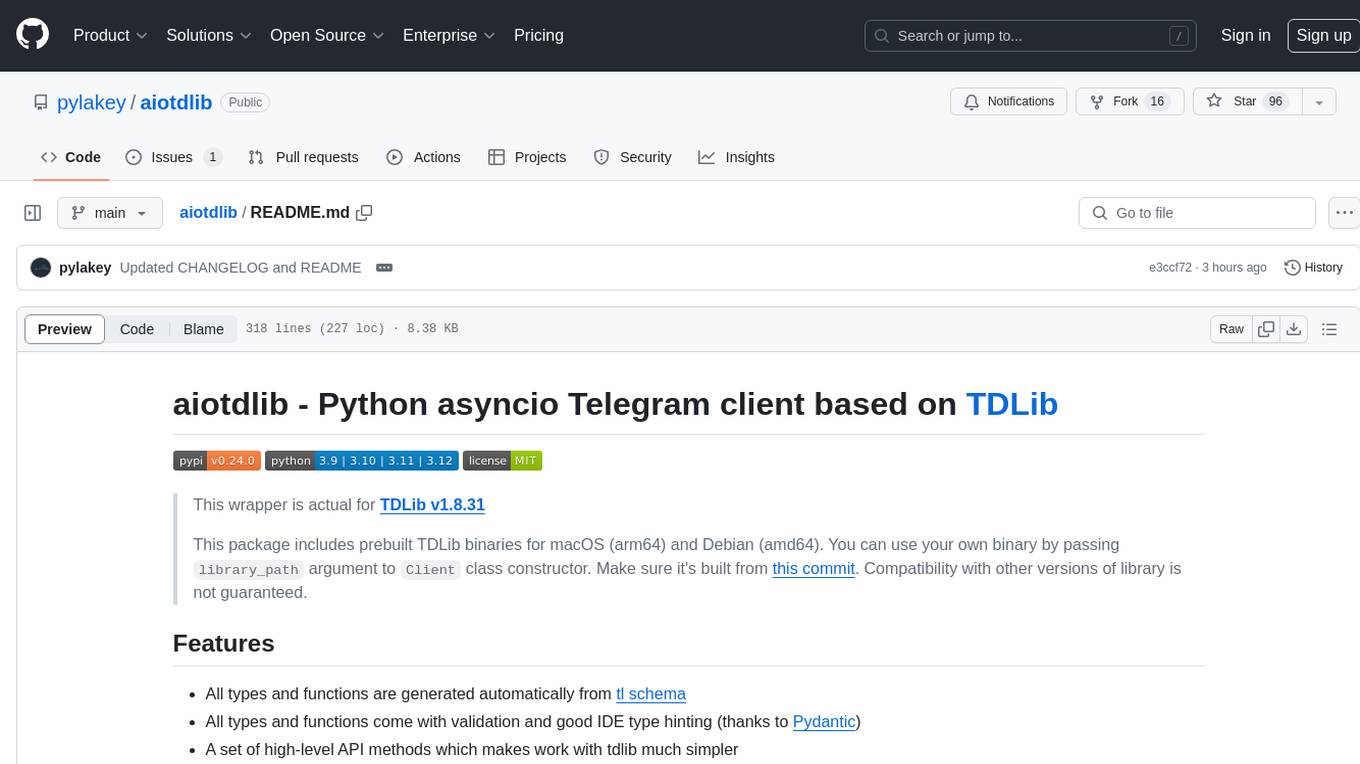
aiotdlib
aiotdlib is a Python asyncio Telegram client based on TDLib. It provides automatic generation of types and functions from tl schema, validation, good IDE type hinting, and high-level API methods for simpler work with tdlib. The package includes prebuilt TDLib binaries for macOS (arm64) and Debian Bullseye (amd64). Users can use their own binary by passing `library_path` argument to `Client` class constructor. Compatibility with other versions of the library is not guaranteed. The tool requires Python 3.9+ and users need to get their `api_id` and `api_hash` from Telegram docs for installation and usage.
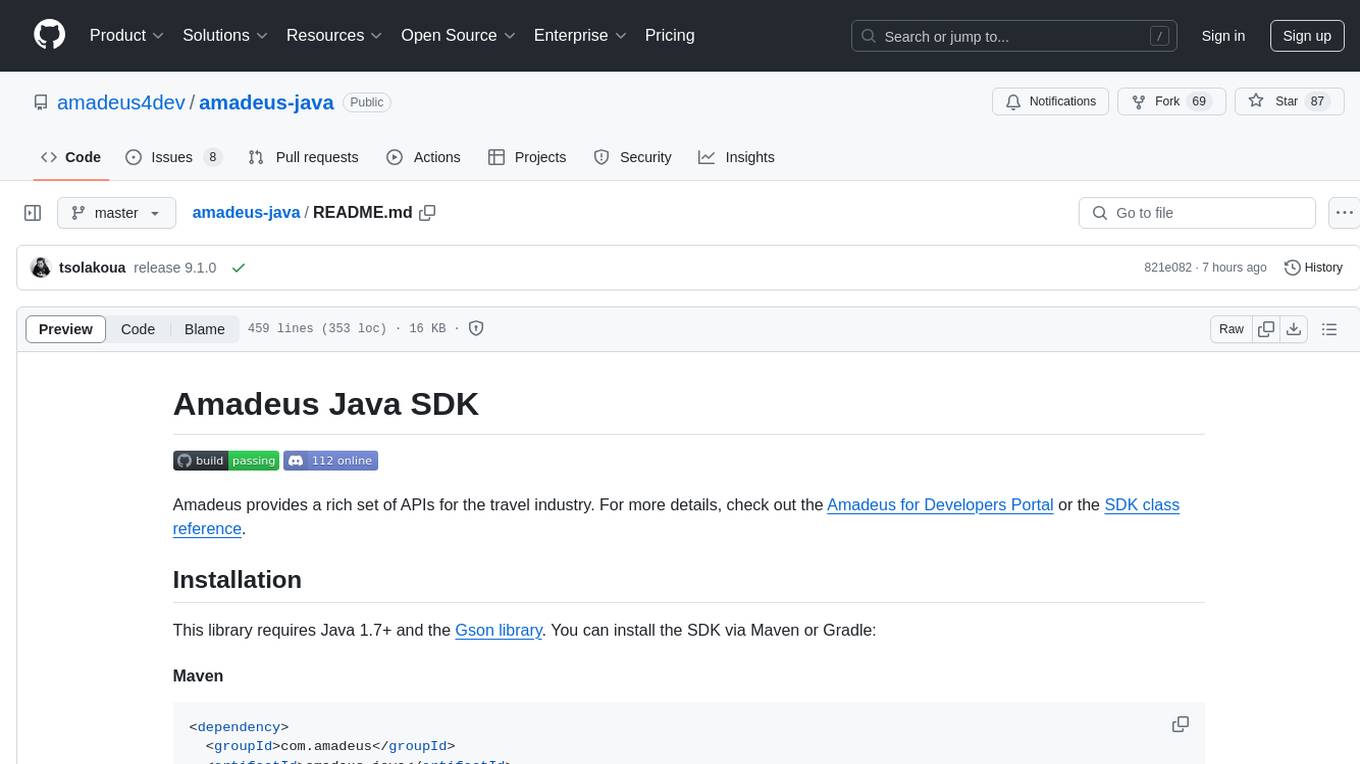
amadeus-java
Amadeus Java SDK provides a rich set of APIs for the travel industry, allowing developers to access various functionalities such as flight search, booking, airport information, and more. The SDK simplifies interaction with the Amadeus API by providing self-contained code examples and detailed documentation. Developers can easily make API calls, handle responses, and utilize features like pagination and logging. The SDK supports various endpoints for tasks like flight search, booking management, airport information retrieval, and travel analytics. It also offers functionalities for hotel search, booking, and sentiment analysis. Overall, the Amadeus Java SDK is a comprehensive tool for integrating Amadeus APIs into Java applications.
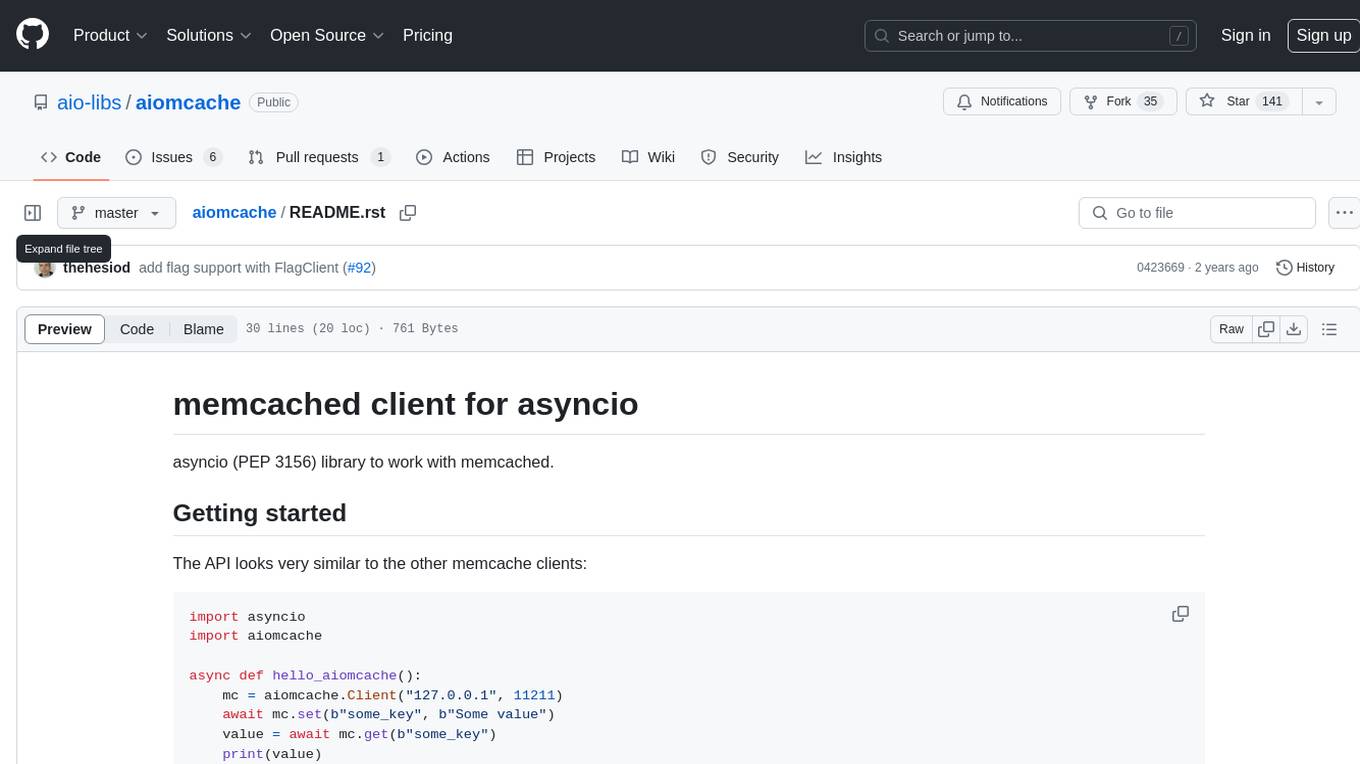
aiomcache
aiomcache is a Python library that provides an asyncio (PEP 3156) interface to work with memcached. It allows users to interact with memcached servers asynchronously, making it suitable for high-performance applications that require non-blocking I/O operations. The library offers similar functionality to other memcache clients and includes features like setting and getting values, multi-get operations, and deleting keys. Version 0.8 introduces the `FlagClient` class, which enables users to register callbacks for setting or processing flags, providing additional flexibility and customization options for working with memcached servers.
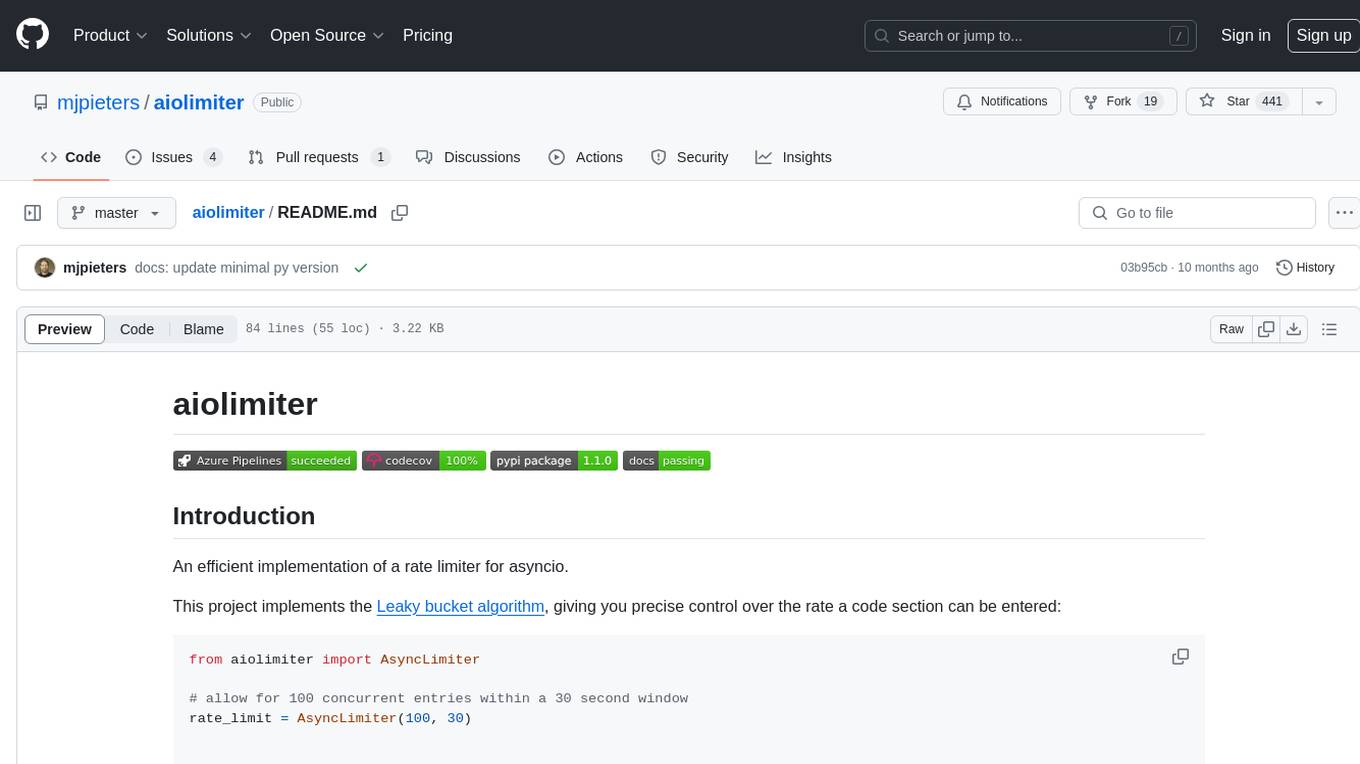
aiolimiter
An efficient implementation of a rate limiter for asyncio using the Leaky bucket algorithm, providing precise control over the rate a code section can be entered. It allows for limiting the number of concurrent entries within a specified time window, ensuring that a section of code is executed a maximum number of times in that period.
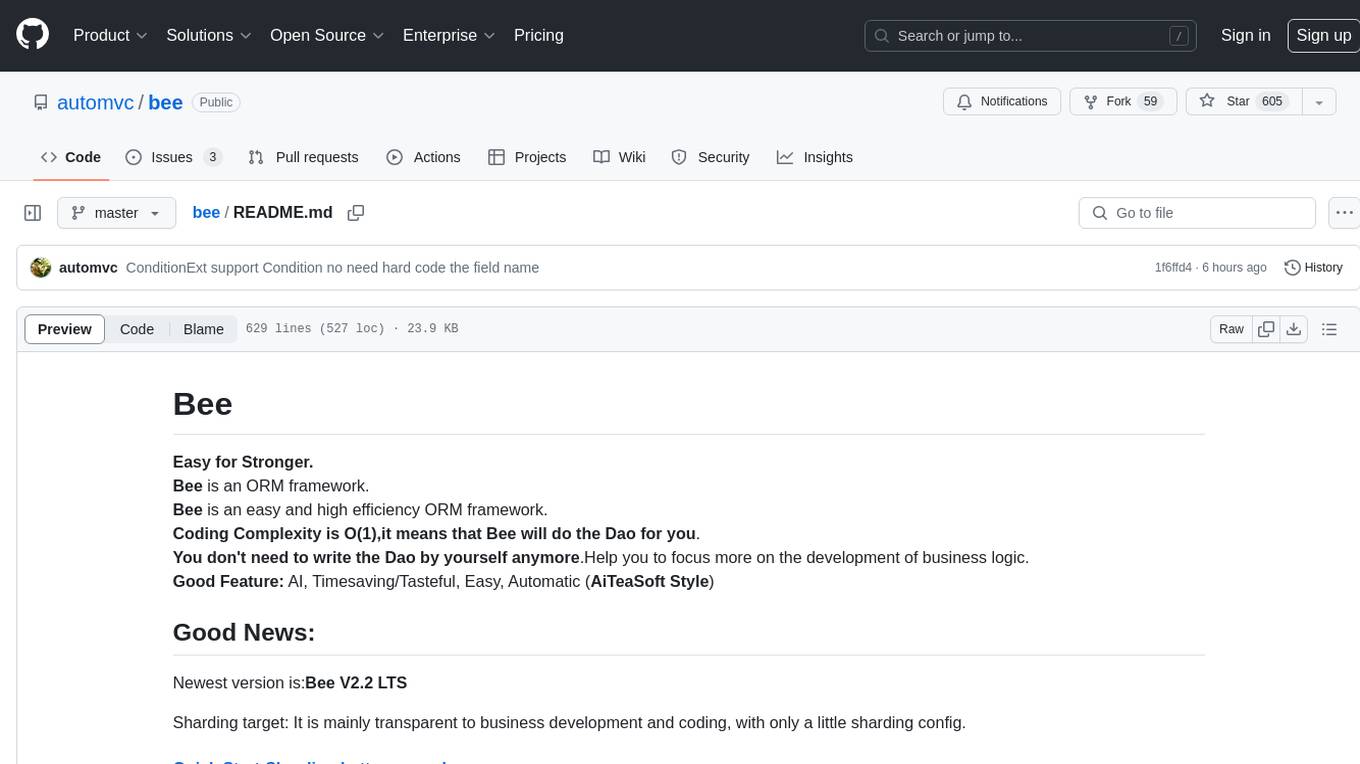
bee
Bee is an easy and high efficiency ORM framework that simplifies database operations by providing a simple interface and eliminating the need to write separate DAO code. It supports various features such as automatic filtering of properties, partial field queries, native statement pagination, JSON format results, sharding, multiple database support, and more. Bee also offers powerful functionalities like dynamic query conditions, transactions, complex queries, MongoDB ORM, cache management, and additional tools for generating distributed primary keys, reading Excel files, and more. The newest versions introduce enhancements like placeholder precompilation, default date sharding, ElasticSearch ORM support, and improved query capabilities.
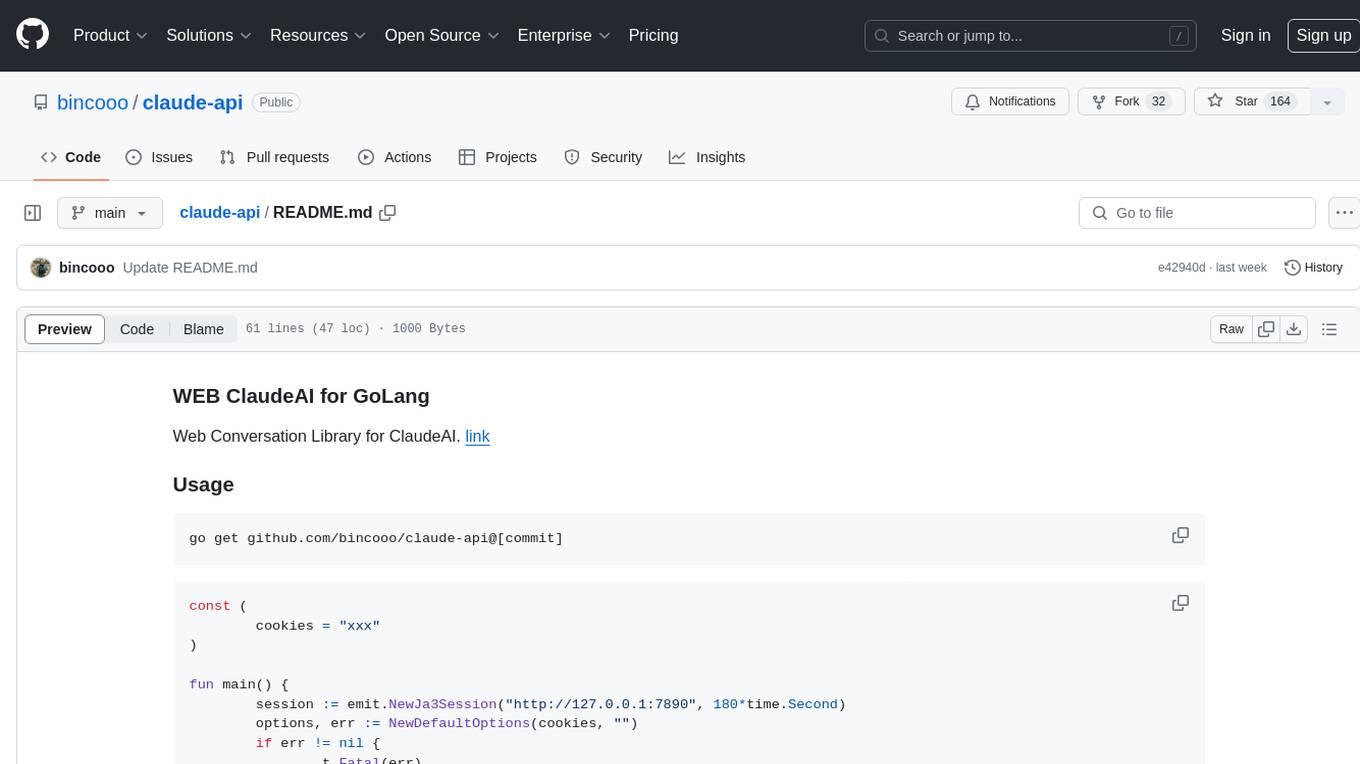
claude-api
claude-api is a web conversation library for ClaudeAI implemented in GoLang. It provides functionalities to interact with ClaudeAI for web-based conversations. Users can easily integrate this library into their Go projects to enable chatbot capabilities and handle conversations with ClaudeAI. The library includes features for sending messages, receiving responses, and managing chat sessions, making it a valuable tool for developers looking to incorporate AI-powered chatbots into their applications.