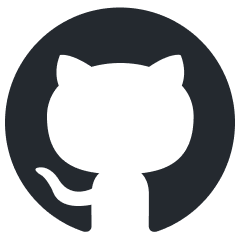
solana-agent-kit
connect any ai agents to solana protocols
Stars: 1148
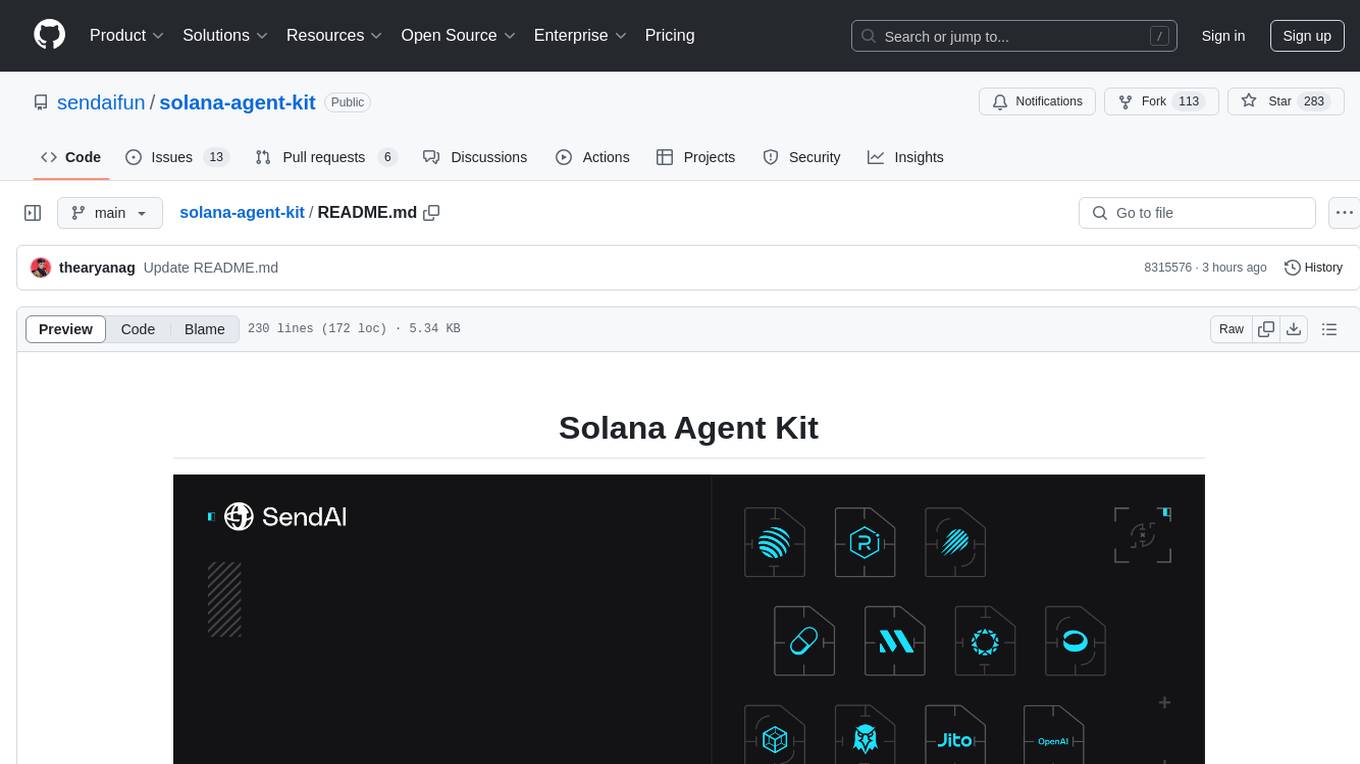
Solana Agent Kit is an open-source toolkit designed for connecting AI agents to Solana protocols. It enables agents, regardless of the model used, to autonomously perform various Solana actions such as trading tokens, launching new tokens, lending assets, sending compressed airdrops, executing blinks, and more. The toolkit integrates core blockchain features like token operations, NFT management via Metaplex, DeFi integration, Solana blinks, AI integration features with LangChain, autonomous modes, and AI tools. It provides ready-to-use tools for blockchain operations, supports autonomous agent actions, and offers features like memory management, real-time feedback, and error handling. Solana Agent Kit facilitates tasks such as deploying tokens, creating NFT collections, swapping tokens, lending tokens, staking SOL, and sending SPL token airdrops via ZK compression. It also includes functionalities for fetching price data from Pyth and relies on key Solana and Metaplex libraries for its operations.
README:
An open-source toolkit for connecting AI agents to Solana protocols. Now, any agent, using any model can autonomously perform 60+ Solana actions:
- Trade tokens
- Launch new tokens
- Lend assets
- Send compressed airdrops
- Execute blinks
- Launch tokens on AMMs
- Bridge tokens across chains
- And more...
Anyone - whether an SF-based AI researcher or a crypto-native builder - can bring their AI agents trained with any model and seamlessly integrate with Solana.
Replit template created by Arpit Singh
-
Token Operations
- Deploy SPL tokens by Metaplex
- Transfer assets
- Balance checks
- Stake SOL
- Zk compressed Airdrop by Light Protocol and Helius
- Bridge tokens across chains using Wormhole
-
NFTs on 3.Land
- Create your own collection
- NFT creation and automatic listing on 3.land
- List your NFT for sale in any SPL token
-
NFT Management via Metaplex
- Collection deployment
- NFT minting
- Metadata management
- Royalty configuration
-
DeFi Integration
- Jupiter Exchange swaps
- Launch on Pump via PumpPortal
- Raydium pool creation (CPMM, CLMM, AMMv4)
- Orca Whirlpool integration
- Manifest market creation, and limit orders
- Meteora Dynamic AMM, DLMM Pool, and Alpha Vault
- Openbook market creation
- Register and Resolve SNS
- Jito Bundles
- Pyth Price feeds for fetching Asset Prices
- Register/resolve Alldomains
- Perpetuals Trading with Adrena Protocol
- Drift Vaults, Perps, Lending and Borrowing
- Cross-chain bridging via deBridge DLN
- Cross chain bridging via Wormhole
-
Solana Blinks
- Lending by Lulo (Best APR for USDC)
- Send Arcade Games
- JupSOL staking
- Solayer SOL (sSOL)staking
-
Non-Financial Actions
- Gib Work for registering bounties
-
Market Data Integration
- CoinGecko Pro API integration
- Real-time token price data
- Trending tokens and pools
- Top gainers analysis
- Token information lookup
- Latest pool tracking
-
LangChain Integration
- Ready-to-use LangChain tools for blockchain operations
- Autonomous agent support with React framework
- Memory management for persistent interactions
- Streaming responses for real-time feedback
-
Vercel AI SDK Integration
- Vercel AI SDK for AI agent integration
- Framework agnostic support
- Quick and easy toolkit setup
-
Autonomous Modes
- Interactive chat mode for guided operations
- Autonomous mode for independent agent actions
- Configurable action intervals
- Built-in error handling and recovery
-
AI Tools
- DALL-E integration for NFT artwork generation
- Natural language processing for blockchain commands
- Price feed integration for market analysis
- Automated decision-making capabilities
You can view the full documentation of the kit at docs.sendai.fun
npm install solana-agent-kit
import { SolanaAgentKit, createSolanaTools } from "solana-agent-kit";
// Initialize with private key and optional RPC URL
const agent = new SolanaAgentKit(
"your-wallet-private-key-as-base58",
"https://api.mainnet-beta.solana.com",
{ OPENAI_API_KEY: "your-openai-api-key" } // optional config
);
// Create LangChain tools
const tools = createSolanaTools(agent);
const result = await agent.deployToken(
"my ai token", // name
"uri", // uri
"token", // symbol
9, // decimals
{
mintAuthority: null, // by default, deployer account
freezeAuthority: null, // by default, deployer account
updateAuthority: undefined, // by default, deployer account
isMutable: false // by default, true
},
1000000 // initial supply
);
console.log("Token Mint Address:", result.mint.toString());
const result = await agent.delpoyToken2022(
"my ai token 2022", // name
"uri", // uri
"token2022", // symbol
9, // decimals
{
mintAuthority: null, // by default, deployer account
freezeAuthority: null, // by default, deployer account
updateAuthority: undefined, // by default, deployer account
isMutable: false // by default, true
},
1000000 // initial supply
);
console.log("Token2022 Mint Address:", result.mint.toString());
const chains = await agent.getWormholeSupportedChains();
console.log("Supported Chains:", chains);
const result = await agent.createWrappedToken({
destinationChain: "BaseSepolia", // Target chain
tokenAddress: "4zMMC9srt5Ri5X14GAgXhaHii3GnPAEERYPJgZJDncDU", // Original token address
network: "Testnet", // Network type (Testnet or Mainnet)
});
console.log("Wrapped Token Result:", result);
Note: When using Wormhole for cross-chain operations:
- Always verify the destination chain is supported before attempting transfers
- Use the correct network parameter ("Testnet" or "Mainnet") based on your environment
- Make sure the destination address wallet private key is presernt in the .env file as automatic transfer is not supported yet on Solana
const transfer = await agent.cctpTransfer({
destinationChain: "Base Sepolia", // Target chain
transferAmount: "1", // Amount to transfer
network: "Testnet", // Network type (Testnet or Mainnet)
});
console.log("Transfer result:", transfer);
const transfer = await agent.tokenTransfer({
destinationChain: "Base Sepolia", // Target chain
tokenAddress: "4zMMC9srt5Ri5X14GAgXhaHii3GnPAEERYPJgZJDncDU", // Original token address or leave it empty to treansfer Native SOL
network: "Testnet", // Network type (Testnet or Mainnet)
});
const isDevnet = false; // (Optional) if not present TX takes place in Mainnet
const priorityFeeParam = 1000000; // (Optional) if not present the default priority fee will be 50000
const collectionOpts: CreateCollectionOptions = {
collectionName: "",
collectionSymbol: "",
collectionDescription: "",
mainImageUrl: ""
};
const result = await agent.create3LandCollection(
collectionOpts,
isDevnet, // (Optional) if not present TX takes place in Mainnet
priorityFeeParam, //(Optional)
);
When creating an NFT using 3Land's tool, it automatically goes for sale on 3.land website
const isDevnet = true; // (Optional) if not present TX takes place in Mainnet
const withPool = true; // (Optional) only present if NFT will be created with a Liquidity Pool for a specific SPL token
const priorityFeeParam = 1000000; // (Optional) if not present the default priority fee will be 50000
const collectionAccount = ""; //hash for the collection
const createItemOptions: CreateSingleOptions = {
itemName: "",
sellerFee: 500, //5%
itemAmount: 100, //total items to be created
itemSymbol: "",
itemDescription: "",
traits: [
{ trait_type: "", value: "" },
],
price: 0, //100000000 == 0.1 sol, can be set to 0 for a free mint
splHash: "", //present if listing is on a specific SPL token, if not present sale will be on $SOL, must be present if "withPool" is true
poolName: "", // Only present if "withPool" is true
mainImageUrl: "",
};
const result = await agent.create3LandNft(
collectionAccount,
createItemOptions,
isDevnet, // (Optional) if not present TX takes place in Mainnet
withPool
priorityFeeParam, //(Optional)
);
const collection = await agent.deployCollection({
name: "My NFT Collection",
uri: "https://arweave.net/metadata.json",
royaltyBasisPoints: 500, // 5%
creators: [
{
address: "creator-wallet-address",
percentage: 100,
},
],
});
import { PublicKey } from "@solana/web3.js";
const signature = await agent.trade(
new PublicKey("target-token-mint"),
100, // amount
new PublicKey("source-token-mint"),
300 // 3% slippage
);
import { PublicKey } from "@solana/web3.js";
const signature = await agent.lendAssets(
100 // amount of USDC to lend
);
const signature = await agent.stake(
1 // amount in SOL to stake
);
const signature = await agent.restake(
1 // amount in SOL to stake
);
import { PublicKey } from "@solana/web3.js";
(async () => {
console.log(
"~Airdrop cost estimate:",
getAirdropCostEstimate(
1000, // recipients
30_000 // priority fee in lamports
)
);
const signature = await agent.sendCompressedAirdrop(
new PublicKey("JUPyiwrYJFskUPiHa7hkeR8VUtAeFoSYbKedZNsDvCN"), // mint
42, // amount per recipient
[
new PublicKey("1nc1nerator11111111111111111111111111111111"),
// ... add more recipients
],
30_000 // priority fee in lamports
);
})();
const priceFeedID = await agent.getPythPriceFeedID("SOL");
const price = await agent.getPythPrice(priceFeedID);
console.log("Price of SOL/USD:", price);
import { PublicKey } from "@solana/web3.js";
const signature = await agent.openPerpTradeLong({
price: 300, // $300 SOL Max price
collateralAmount: 10, // 10 jitoSOL in
collateralMint: new PublicKey("J1toso1uCk3RLmjorhTtrVwY9HJ7X8V9yYac6Y7kGCPn"), // jitoSOL
leverage: 50000, // x5
tradeMint: new PublicKey("J1toso1uCk3RLmjorhTtrVwY9HJ7X8V9yYac6Y7kGCPn"), // jitoSOL
slippage: 0.3, // 0.3%
});
import { PublicKey } from "@solana/web3.js";
const signature = await agent.closePerpTradeLong({
price: 200, // $200 SOL price
tradeMint: new PublicKey("J1toso1uCk3RLmjorhTtrVwY9HJ7X8V9yYac6Y7kGCPn"), // jitoSOL
});
const { signature } = await agent.closeEmptyTokenAccounts();
Create a drift account with an initial token deposit.
const result = await agent.createDriftUserAccount()
Create a drift vault.
const signature = await agent.createDriftVault({
name: "my-drift-vault",
marketName: "USDC-SPOT",
redeemPeriod: 1, // in days
maxTokens: 100000, // in token units e.g 100000 USDC
minDepositAmount: 5, // in token units e.g 5 USDC
managementFee: 1, // 1%
profitShare: 10, // 10%
hurdleRate: 5, // 5%
permissioned: false, // public vault or whitelist
})
Deposit tokens into a drift vault.
const signature = await agent.depositIntoDriftVault(100, "41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU")
Deposit tokens into your drift account.
const {txSig} = await agent.depositToDriftUserAccount(100, "USDC")
Derive a drift vault address.
const vaultPublicKey = await agent.deriveDriftVaultAddress("my-drift-vault")
Check if agent has a drift account.
const {hasAccount, account} = await agent.doesUserHaveDriftAccount()
Get drift account information.
const accountInfo = await agent.driftUserAccountInfo()
Request withdrawal from drift vault.
const signature = await agent.requestWithdrawalFromDriftVault(100, "41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU")
Open a perpetual trade using a drift vault that is delegated to you.
const signature = await agent.tradeUsingDelegatedDriftVault({
vault: "41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU",
amount: 500,
symbol: "SOL",
action: "long",
type: "limit",
price: 180 // Please long limit order at $180/SOL
})
Open a perpetual trade using your drift account.
const signature = await agent.tradeUsingDriftPerpAccount({
amount: 500,
symbol: "SOL",
action: "long",
type: "limit",
price: 180 // Please long limit order at $180/SOL
})
Update drift vault parameters.
const signature = await agent.updateDriftVault({
name: "my-drift-vault",
marketName: "USDC-SPOT",
redeemPeriod: 1, // in days
maxTokens: 100000, // in token units e.g 100000 USDC
minDepositAmount: 5, // in token units e.g 5 USDC
managementFee: 1, // 1%
profitShare: 10, // 10%
hurdleRate: 5, // 5%
permissioned: false, // public vault or whitelist
})
Withdraw tokens from your drift account.
const {txSig} = await agent.withdrawFromDriftAccount(100, "USDC")
Borrow tokens from drift.
const {txSig} = await agent.withdrawFromDriftAccount(1, "SOL", true)
Repay a loan from drift.
const {txSig} = await agent.depositToDriftUserAccount(1, "SOL", true)
Withdraw tokens from a drift vault after the redemption period has elapsed.
const signature = await agent.withdrawFromDriftVault( "41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU")
Update the address a drift vault is delegated to.
const signature = await agent.updateDriftVaultDelegate("41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU", "new-address")
Get the current position values and total value of assets in a Voltr vault.
const values = await agent.voltrGetPositionValues("7opUkqYtxmQRriZvwZkPcg6LqmGjAh1RSEsVrdsGDx5K")
Deposit assets into a specific strategy within a Voltr vault.
const signature = await agent.voltrDepositStrategy(
new BN("1000000000"), // amount in base units (e.g., 1 USDC = 1000000)
"7opUkqYtxmQRriZvwZkPcg6LqmGjAh1RSEsVrdsGDx5K", // vault
"9ZQQYvr4x7AMqd6abVa1f5duGjti5wk1MHsX6hogPsLk" // strategy
)
Withdraw assets from a specific strategy within a Voltr vault.
const signature = await agent.voltrWithdrawStrategy(
new BN("1000000000"), // amount in base units (e.g., 1 USDC = 1000000)
"7opUkqYtxmQRriZvwZkPcg6LqmGjAh1RSEsVrdsGDx5K", // vault
"9ZQQYvr4x7AMqd6abVa1f5duGjti5wk1MHsX6hogPsLk" // strategy
)
const asset = await agent.getAsset("41Y8C4oxk4zgJT1KXyQr35UhZcfsp5mP86Z2G7UUzojU")
Get the price for a given token and timeframe from Allora's API
const sol5mPrice = await agent.getPriceInference("SOL", "5m");
console.log("5m price inference of SOL/USD:", sol5mPrice);
const topics = await agent.getAllTopics();
console.log("Allora topics:", topics);
const inference = await agent.getInferenceByTopicId(42);
console.log("Allora inference for topic 42:", inference);
Simulate a given Switchboard feed. Find or create feeds here.
const value = await agent.simulateSwitchboardFeed(
"9wcBMATS8bGLQ2UcRuYjsRAD7TPqB1CMhqfueBx78Uj2", // TRUMP/USD
"http://crossbar.switchboard.xyz");;
console.log("Simulation resulted in the following value:", value);
### Cross-Chain Swap
```typescript
import { PublicKey } from "@solana/web3.js";
const signature = await agent.swap(
amount: "10",
fromChain: "bsc",
fromToken: "0x3c499c542cef5e3811e1192ce70d8cc03d5c3359",
toChain: "solana",
toToken: "0x0000000000000000000000000000000000000000",
dstAddr: "0xc2d3024d64f27d85e05c40056674Fd18772dd922",
);
The Solana Agent Kit supports cross-chain token transfers using deBridge's DLN protocol. Here's how to use it:
- Check supported chains:
const chains = await agent.getDebridgeSupportedChains();
console.log("Available chains:", chains);
// Example output: { chains: [{ chainId: "1", chainName: "Ethereum" }, { chainId: "7565164", chainName: "Solana" }] }
- Get available tokens (optional):
const tokens = await agent.getDebridgeTokensInfo("1", "USDC"); // Search for USDC on Ethereum
console.log("Available tokens:", tokens);
// Shows tokens matching 'USDC' on the specified chain
- Create bridge order (SOL -> ETH):
const orderInput = {
srcChainId: "7565164", // Solana
srcChainTokenIn: "11111111111111111111111111111111", // Native SOL
srcChainTokenInAmount: "1000000000", // 1 SOL (9 decimals)
dstChainId: "1", // Ethereum
dstChainTokenOut: "0x0000000000000000000000000000000000000000", // ETH
dstChainTokenOutRecipient: "0x23C279e58ddF1018C3B9D0C224534fA2a83fb1d2" // ETH recipient
};
const order = await agent.createDebridgeOrder(orderInput);
console.log("Order created:", order);
// Contains transaction data and estimated amounts
- Execute the bridge order:
const signature = await agent.executeDebridgeOrder(order.tx.data);
console.log("Bridge transaction sent:", signature);
- Check bridge status:
const status = await agent.checkDebridgeTransactionStatus(signature);
console.log("Bridge status:", status);
// Shows current status: Created, Fulfilled, etc.
Note: When bridging between chains:
- To Solana: Use base58 addresses for recipients and token mints
- From Solana: Use EVM addresses for recipients and ERC-20 format for tokens
- Always verify addresses and amounts before executing bridge transactions
const priceData = await agent.getTokenPriceData([
"So11111111111111111111111111111111111111112", // SOL
"EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v" // USDC
]);
console.log("Token prices:", priceData);
const trendingTokens = await agent.getTrendingTokens();
console.log("Trending tokens:", trendingTokens);
const latestPools = await agent.getLatestPools();
console.log("Latest pools:", latestPools);
const tokenInfo = await agent.getTokenInfo("EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v");
console.log("Token info:", tokenInfo);
const topGainers = await agent.getTopGainers("24h", "all");
console.log("Top gainers:", topGainers);
const trendingPools = await agent.getTrendingPools("24h");
console.log("Trending pools:", trendingPools);
The toolkit provides comprehensive integration with OKX DEX for Solana. Here's how to use the DEX API tools:
const tokens = await agent.getTokens();
console.log("Supported tokens:", tokens);
// Returns list of tokens available on OKX DEX
const quote = await agent.getQuote(
"So11111111111111111111111111111111111111112", // fromTokenAddress (SOL)
"EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v", // toTokenAddress (USDC)
"1000000000", // amount in base units
"0.5" // slippage (optional, default 0.5%)
);
console.log("Swap quote:", quote);
// Returns quote data with price, fees, and slippage, token symbol, decimals
const swapResult = await agent.executeSwap({
fromTokenAddress: "So11111111111111111111111111111111111111112", // SOL
toTokenAddress: "EPjFWdd5AufqSSqeM2qN1xzybapC8G4wEGGkZwyTDt1v", // USDC
amount: "1000000000", // amount in base units
autoSlippage: true, // optional, use auto slippage
slippage: "0.1", // optional, custom slippage (0.1%)
maxAutoSlippageBps: "100" // optional, max auto slippage in basis points
userAddress: "signer-public-key" // optional, user wallet address
});
console.log("Swap executed:", swapResult);
Note: To use OKX DEX integration, you need to set up the following environment variables: Get OKX API keys from the [OKX Developer Portal] (https://www.okx.com/web3/build/dev-portal)
OKX_API_KEY
OKX_SECRET_KEY
OKX_API_PASSPHRASE
OKX_PROJECT_ID
RPC_URL
SOLANA_PRIVATE_KEY
SOLANA_WALLET_ADDRESS
The repository includes an advanced example of building a multi-agent system using LangGraph and Solana Agent Kit. Located in examples/agent-kit-langgraph
, this example demonstrates:
- Multi-agent architecture using LangGraph's StateGraph
- Specialized agents for different tasks:
- General purpose agent for basic queries
- Transfer/Swap agent for transaction operations
- Read agent for blockchain data queries
- Manager agent for routing and orchestration
- Fully typed TypeScript implementation
- Environment-based configuration
Check out the LangGraph example for a complete implementation of an advanced Solana agent system.
The toolkit relies on several key Solana and Metaplex libraries:
- @solana/web3.js
- @solana/spl-token
- @metaplex-foundation/digital-asset-standard-api
- @metaplex-foundation/mpl-token-metadata
- @metaplex-foundation/mpl-core
- @metaplex-foundation/umi
- @lightprotocol/compressed-token
- @lightprotocol/stateless.js
- @coingecko/sdk
Contributions are welcome! Please feel free to submit a Pull Request. Refer to CONTRIBUTING.md for detailed guidelines on how to contribute to this project.
Apache-2 License
If you wanna give back any tokens or donations to the OSS community -- The Public Solana Agent Kit Treasury Address:
Solana Network : EKHTbXpsm6YDgJzMkFxNU1LNXeWcUW7Ezf8mjUNQQ4Pa
This toolkit handles private keys and transactions. Always ensure you're using it in a secure environment and never share your private keys.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for solana-agent-kit
Similar Open Source Tools
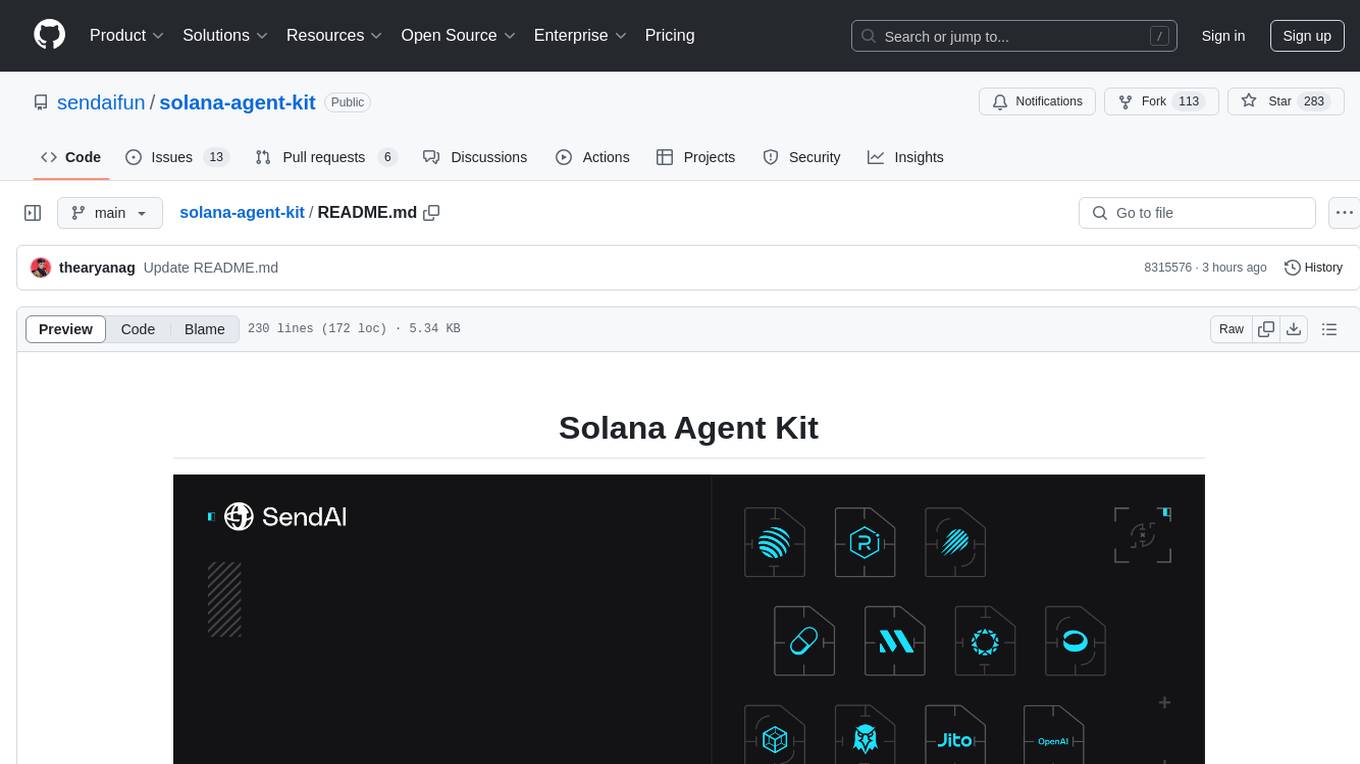
solana-agent-kit
Solana Agent Kit is an open-source toolkit designed for connecting AI agents to Solana protocols. It enables agents, regardless of the model used, to autonomously perform various Solana actions such as trading tokens, launching new tokens, lending assets, sending compressed airdrops, executing blinks, and more. The toolkit integrates core blockchain features like token operations, NFT management via Metaplex, DeFi integration, Solana blinks, AI integration features with LangChain, autonomous modes, and AI tools. It provides ready-to-use tools for blockchain operations, supports autonomous agent actions, and offers features like memory management, real-time feedback, and error handling. Solana Agent Kit facilitates tasks such as deploying tokens, creating NFT collections, swapping tokens, lending tokens, staking SOL, and sending SPL token airdrops via ZK compression. It also includes functionalities for fetching price data from Pyth and relies on key Solana and Metaplex libraries for its operations.
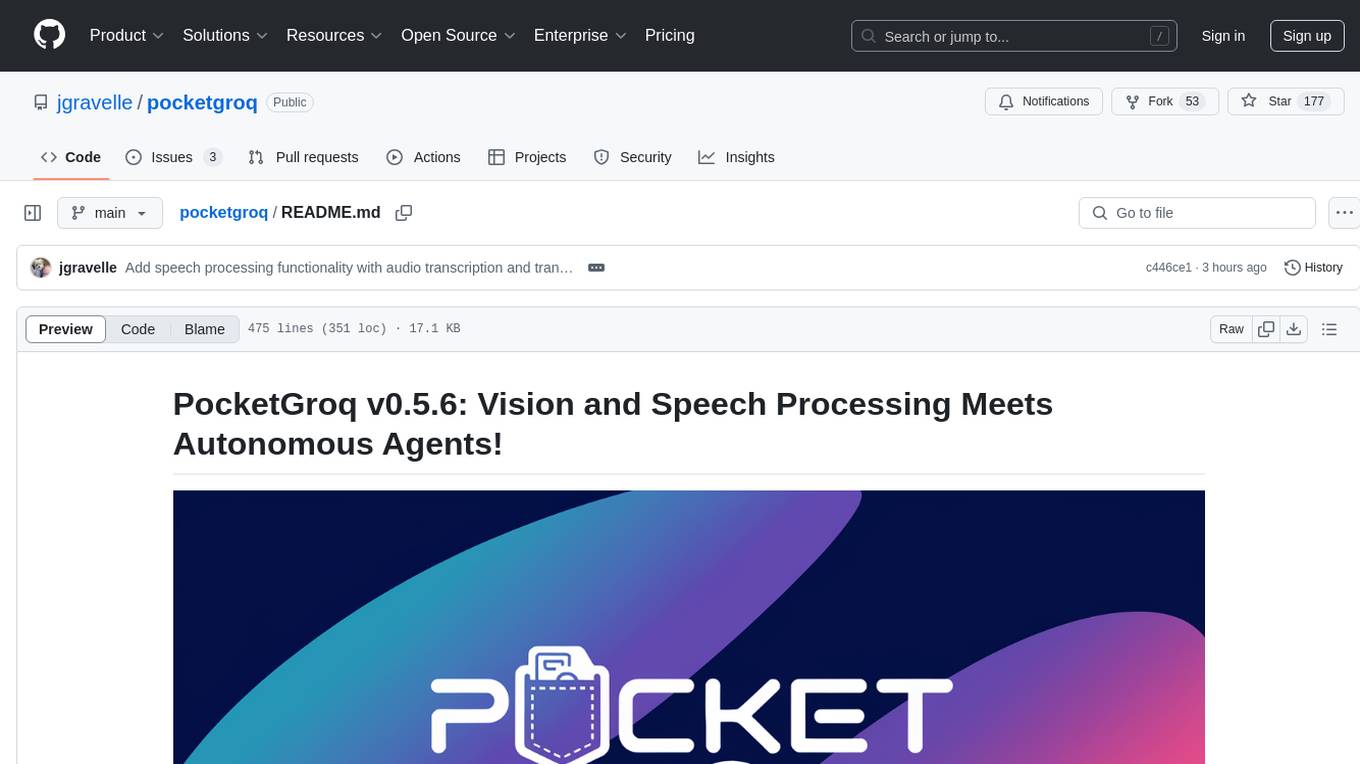
pocketgroq
PocketGroq is a tool that provides advanced functionalities for text generation, web scraping, web search, and AI response evaluation. It includes features like an Autonomous Agent for answering questions, web crawling and scraping capabilities, enhanced web search functionality, and flexible integration with Ollama server. Users can customize the agent's behavior, evaluate responses using AI, and utilize various methods for text generation, conversation management, and Chain of Thought reasoning. The tool offers comprehensive methods for different tasks, such as initializing RAG, error handling, and tool management. PocketGroq is designed to enhance development processes and enable the creation of AI-powered applications with ease.
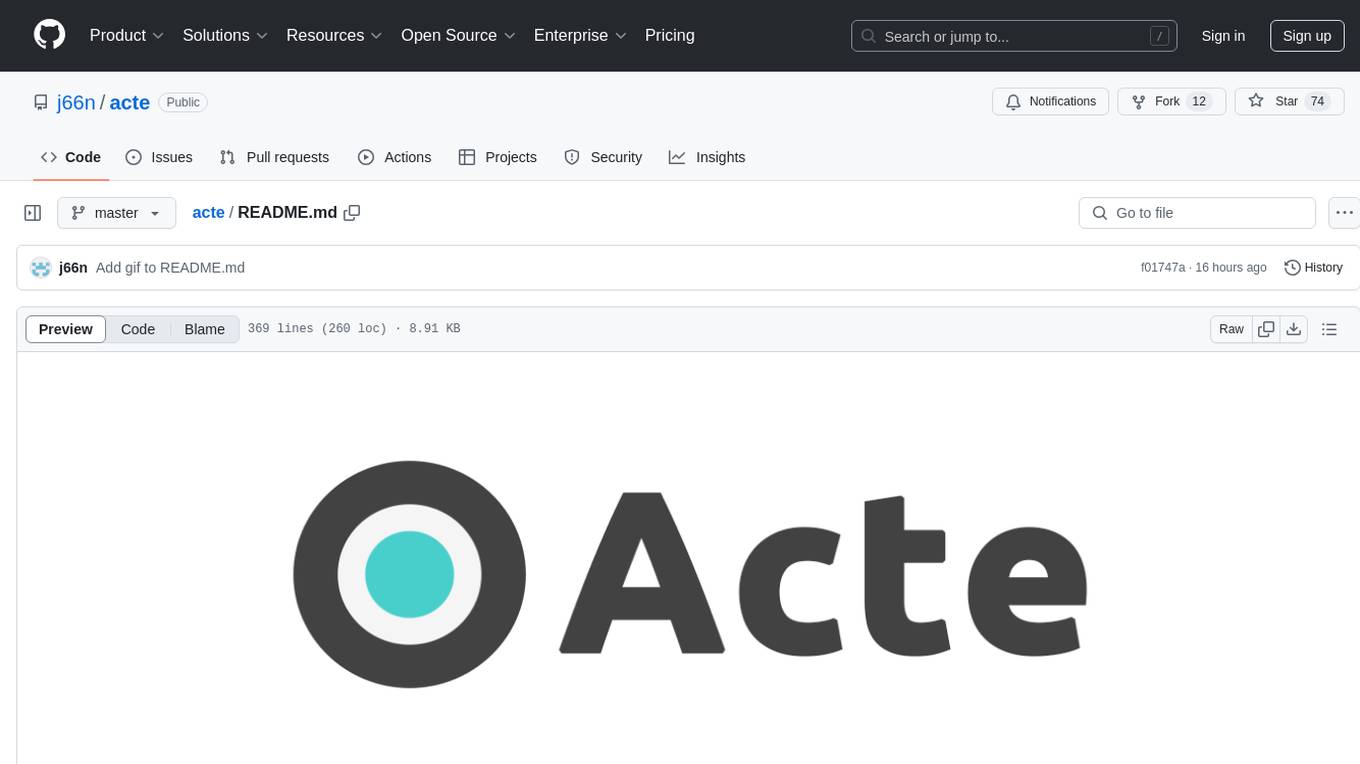
acte
Acte is a framework designed to build GUI-like tools for AI Agents. It aims to address the issues of cognitive load and freedom degrees when interacting with multiple APIs in complex scenarios. By providing a graphical user interface (GUI) for Agents, Acte helps reduce cognitive load and constraints interaction, similar to how humans interact with computers through GUIs. The tool offers APIs for starting new sessions, executing actions, and displaying screens, accessible via HTTP requests or the SessionManager class.
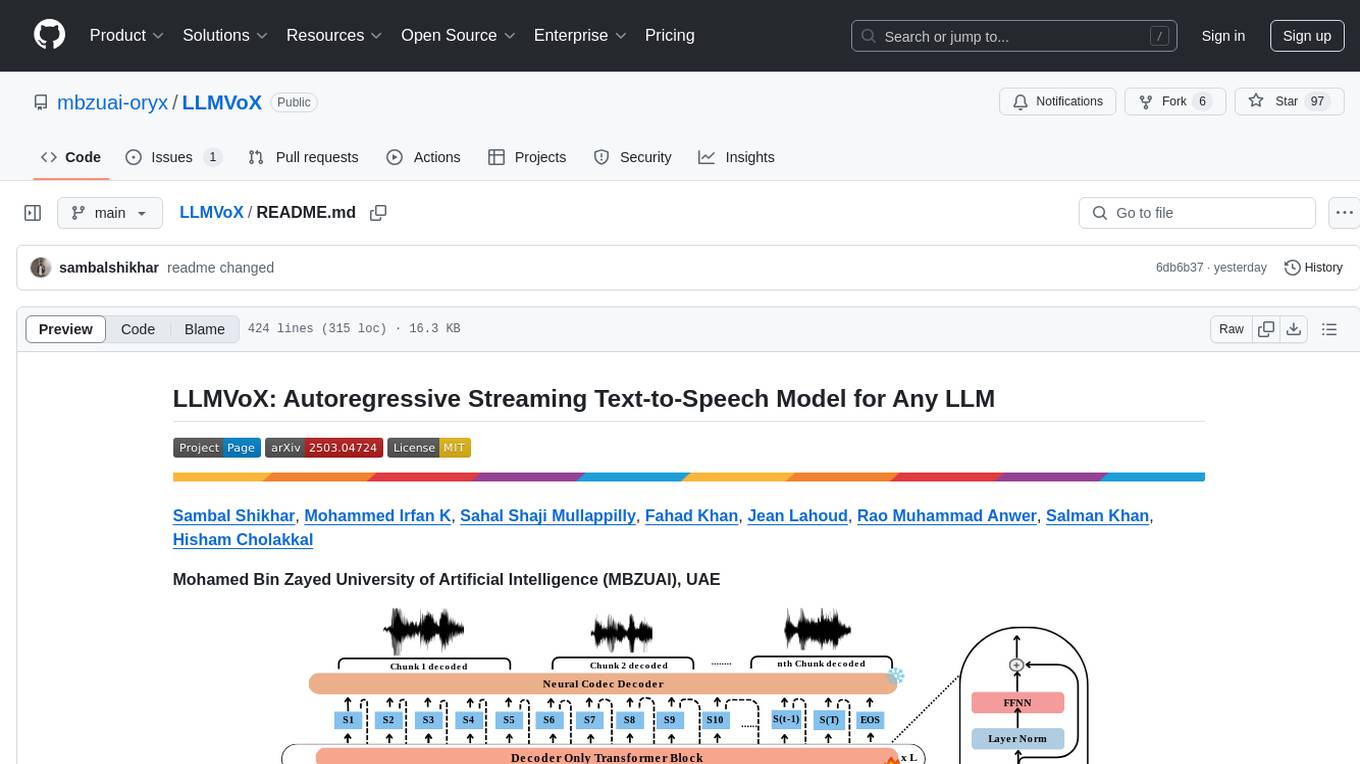
LLMVoX
LLMVoX is a lightweight 30M-parameter, LLM-agnostic, autoregressive streaming Text-to-Speech (TTS) system designed to convert text outputs from Large Language Models into high-fidelity streaming speech with low latency. It achieves significantly lower Word Error Rate compared to speech-enabled LLMs while operating at comparable latency and speech quality. Key features include being lightweight & fast with only 30M parameters, LLM-agnostic for easy integration with existing models, multi-queue streaming for continuous speech generation, and multilingual support for easy adaptation to new languages.
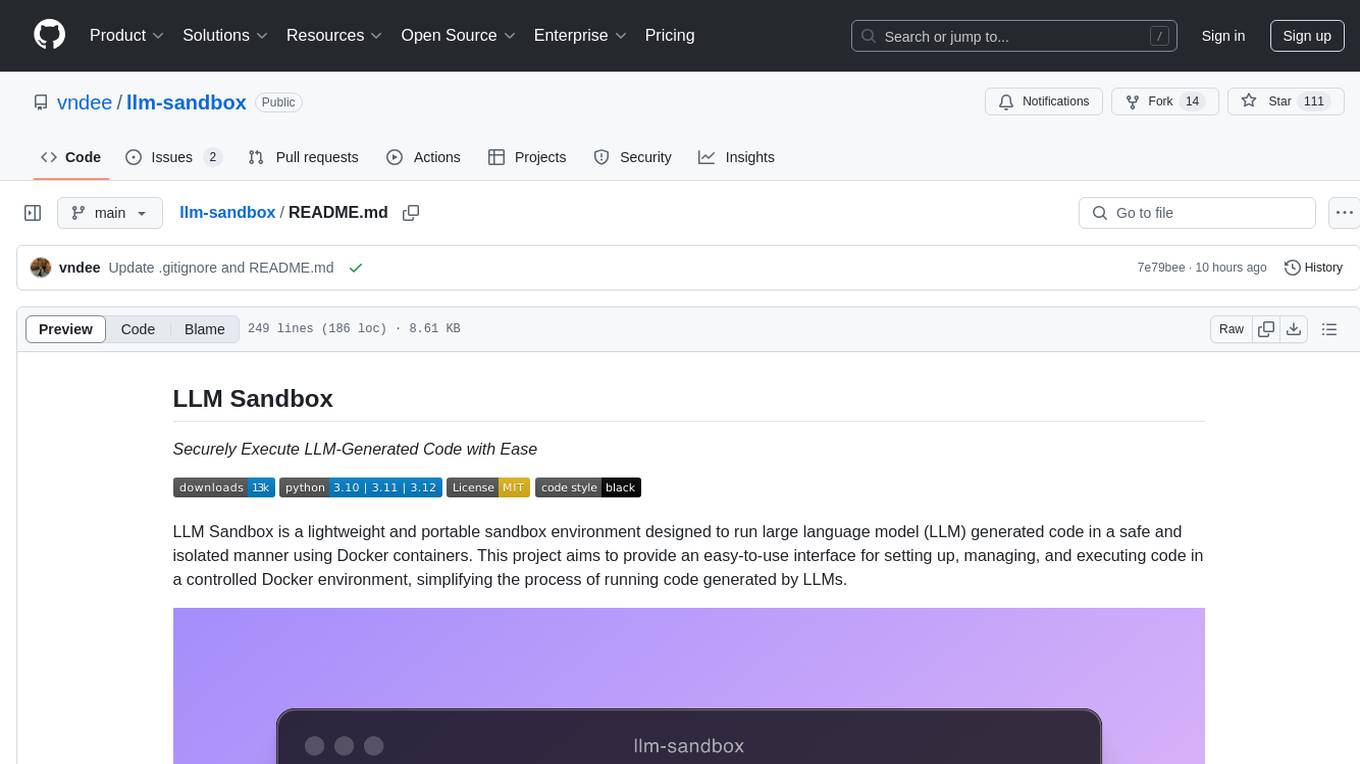
llm-sandbox
LLM Sandbox is a lightweight and portable sandbox environment designed to securely execute large language model (LLM) generated code in a safe and isolated manner using Docker containers. It provides an easy-to-use interface for setting up, managing, and executing code in a controlled Docker environment, simplifying the process of running code generated by LLMs. The tool supports multiple programming languages, offers flexibility with predefined Docker images or custom Dockerfiles, and allows scalability with support for Kubernetes and remote Docker hosts.
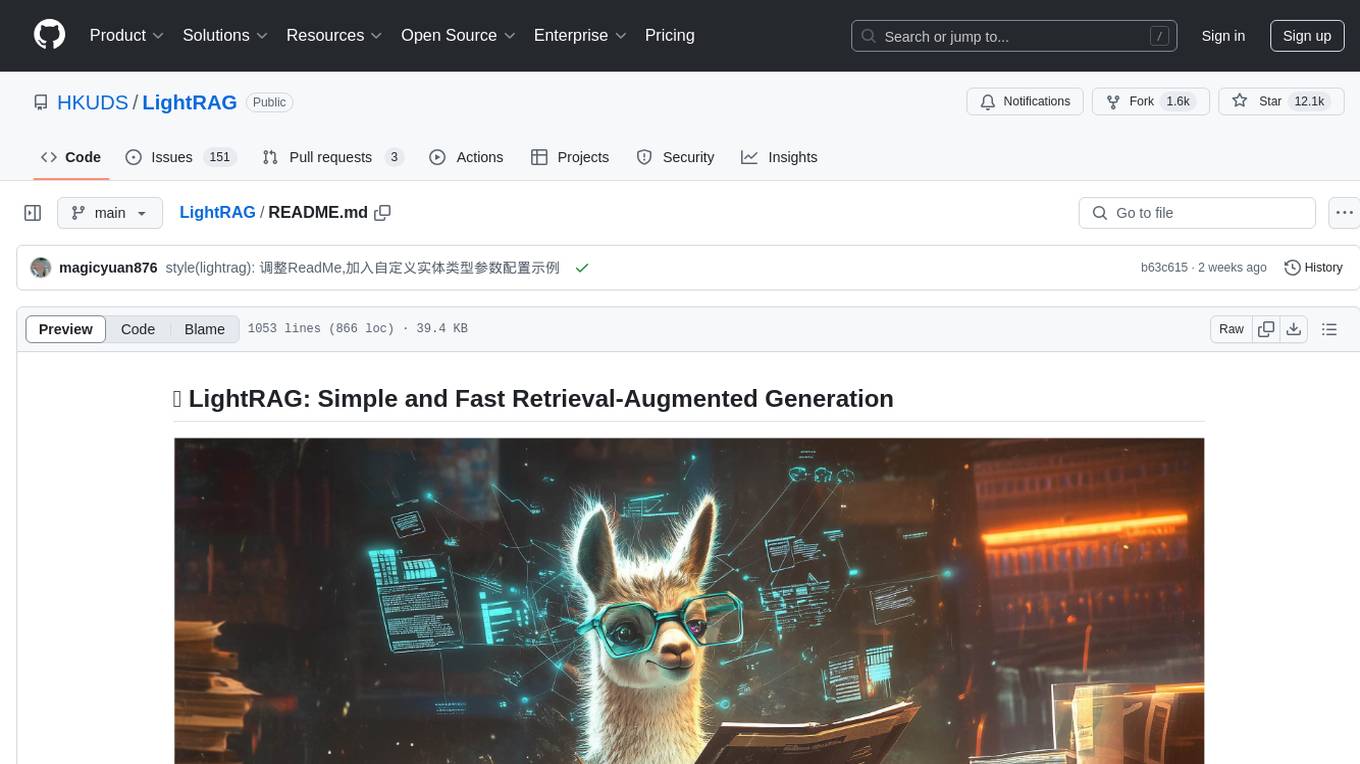
LightRAG
LightRAG is a repository hosting the code for LightRAG, a system that supports seamless integration of custom knowledge graphs, Oracle Database 23ai, Neo4J for storage, and multiple file types. It includes features like entity deletion, batch insert, incremental insert, and graph visualization. LightRAG provides an API server implementation for RESTful API access to RAG operations, allowing users to interact with it through HTTP requests. The repository also includes evaluation scripts, code for reproducing results, and a comprehensive code structure.
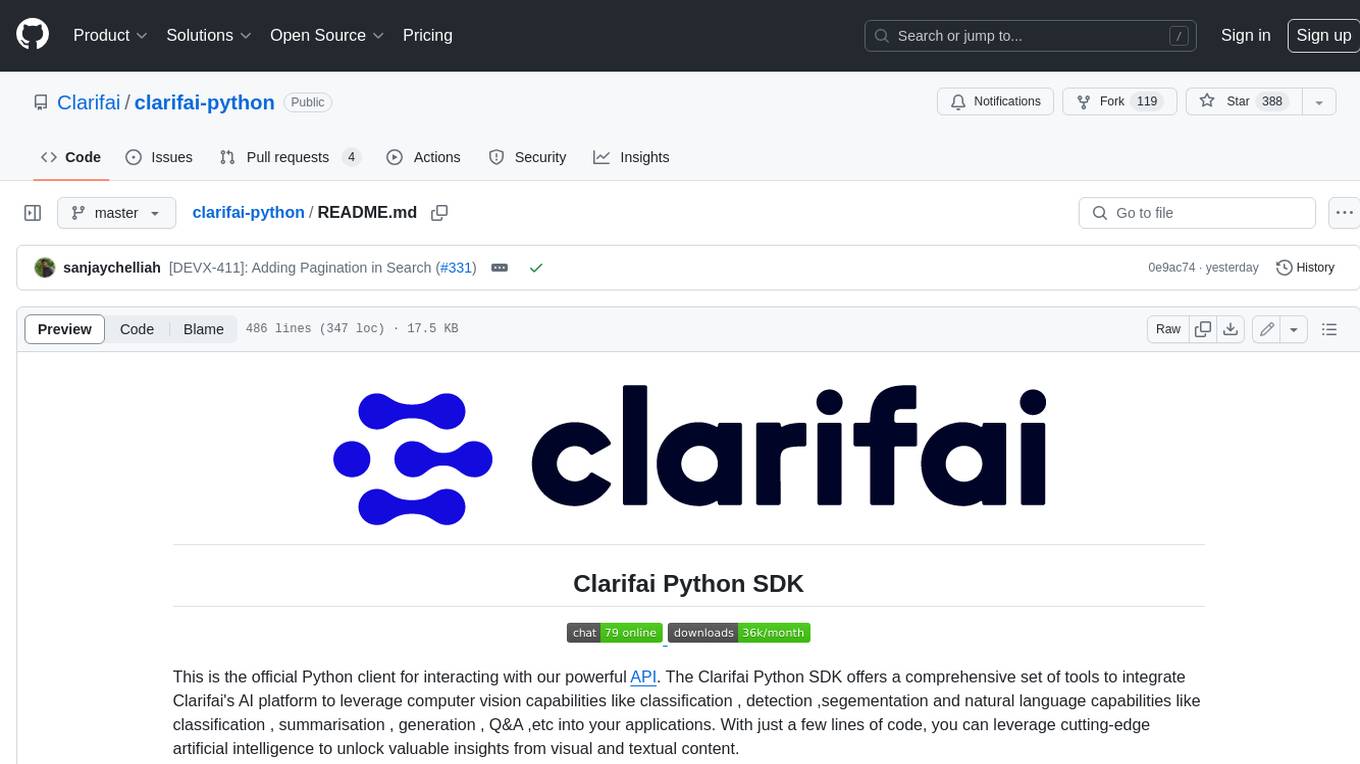
clarifai-python
The Clarifai Python SDK offers a comprehensive set of tools to integrate Clarifai's AI platform to leverage computer vision capabilities like classification , detection ,segementation and natural language capabilities like classification , summarisation , generation , Q&A ,etc into your applications. With just a few lines of code, you can leverage cutting-edge artificial intelligence to unlock valuable insights from visual and textual content.
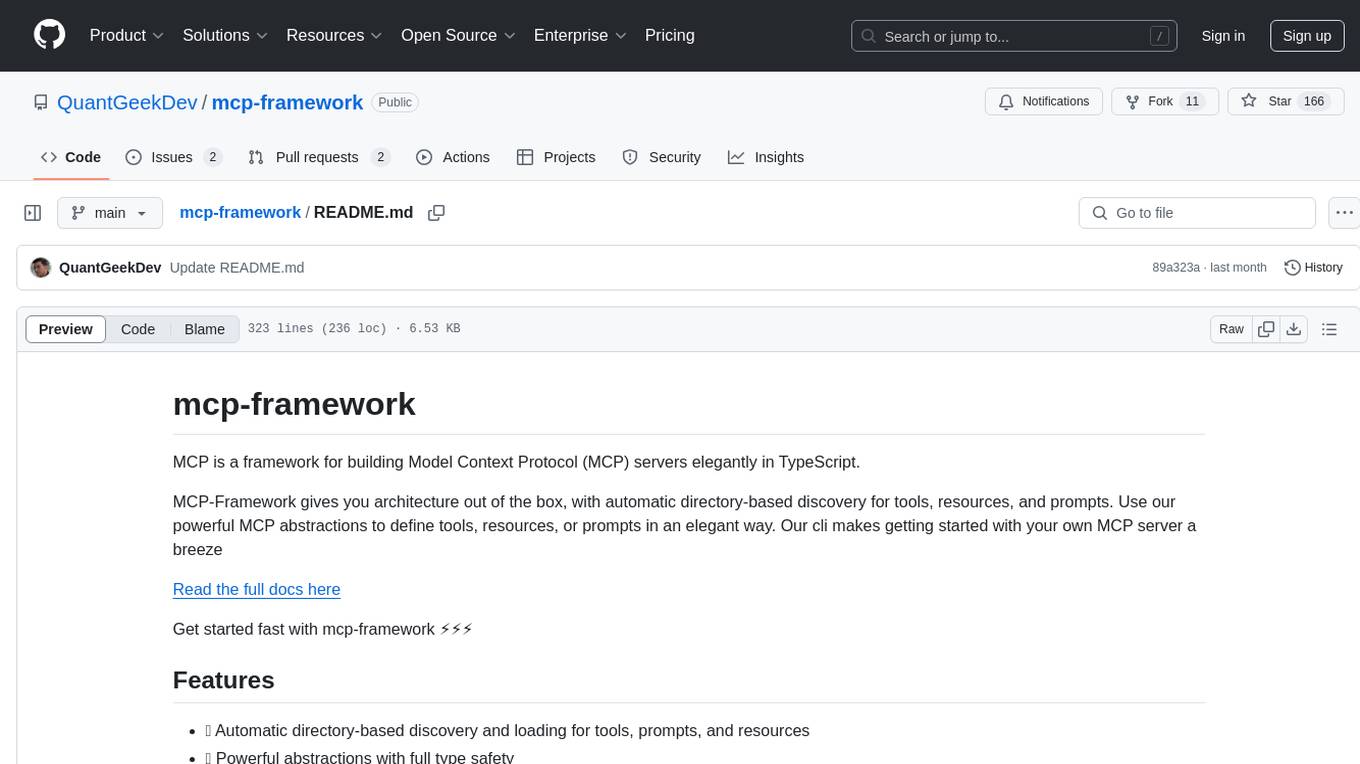
mcp-framework
MCP-Framework is a TypeScript framework for building Model Context Protocol (MCP) servers with automatic directory-based discovery for tools, resources, and prompts. It provides powerful abstractions, simple server setup, and a CLI for rapid development and project scaffolding.
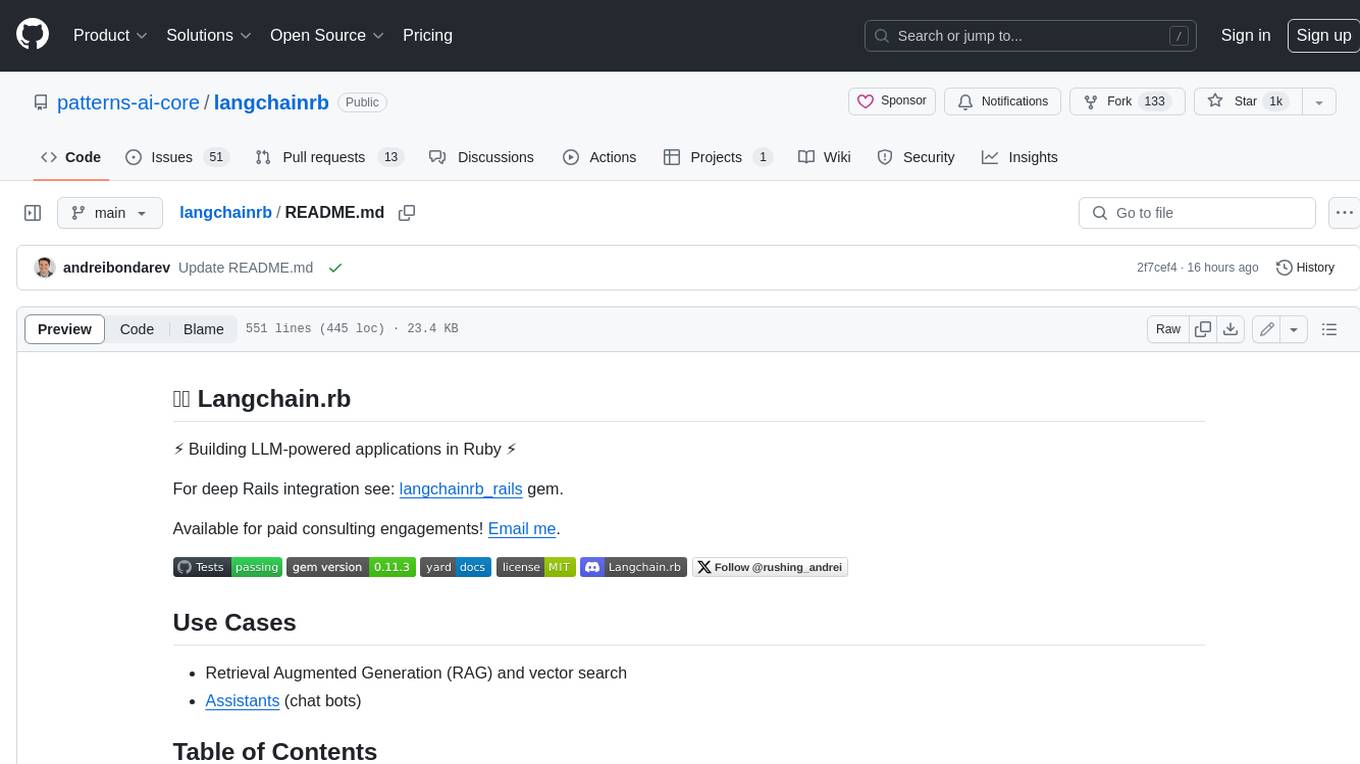
langchainrb
Langchain.rb is a Ruby library that makes it easy to build LLM-powered applications. It provides a unified interface to a variety of LLMs, vector search databases, and other tools, making it easy to build and deploy RAG (Retrieval Augmented Generation) systems and assistants. Langchain.rb is open source and available under the MIT License.
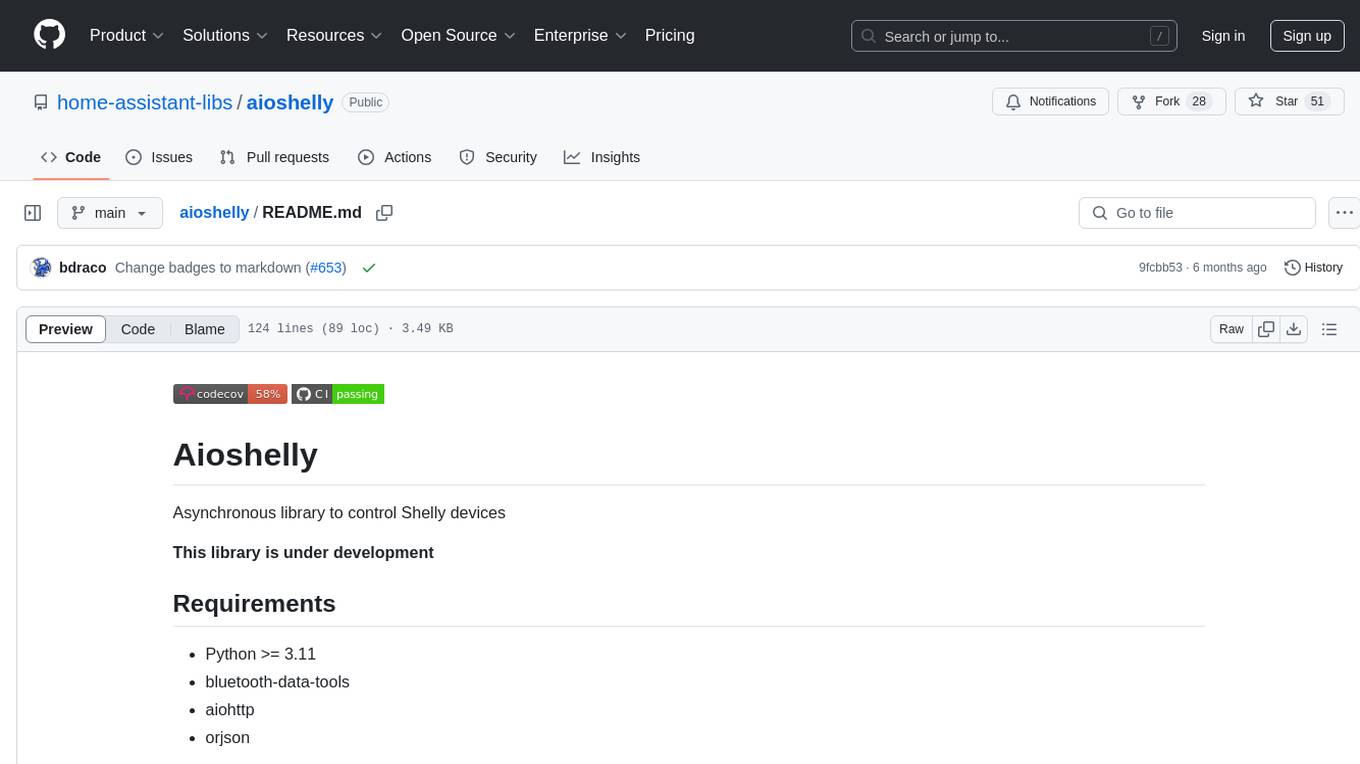
aioshelly
Aioshelly is an asynchronous library designed to control Shelly devices. It is currently under development and requires Python version 3.11 or higher, along with dependencies like bluetooth-data-tools, aiohttp, and orjson. The library provides examples for interacting with Gen1 devices using CoAP protocol and Gen2/Gen3 devices using RPC and WebSocket protocols. Users can easily connect to Shelly devices, retrieve status information, and perform various actions through the provided APIs. The repository also includes example scripts for quick testing and usage guidelines for contributors to maintain consistency with the Shelly API.
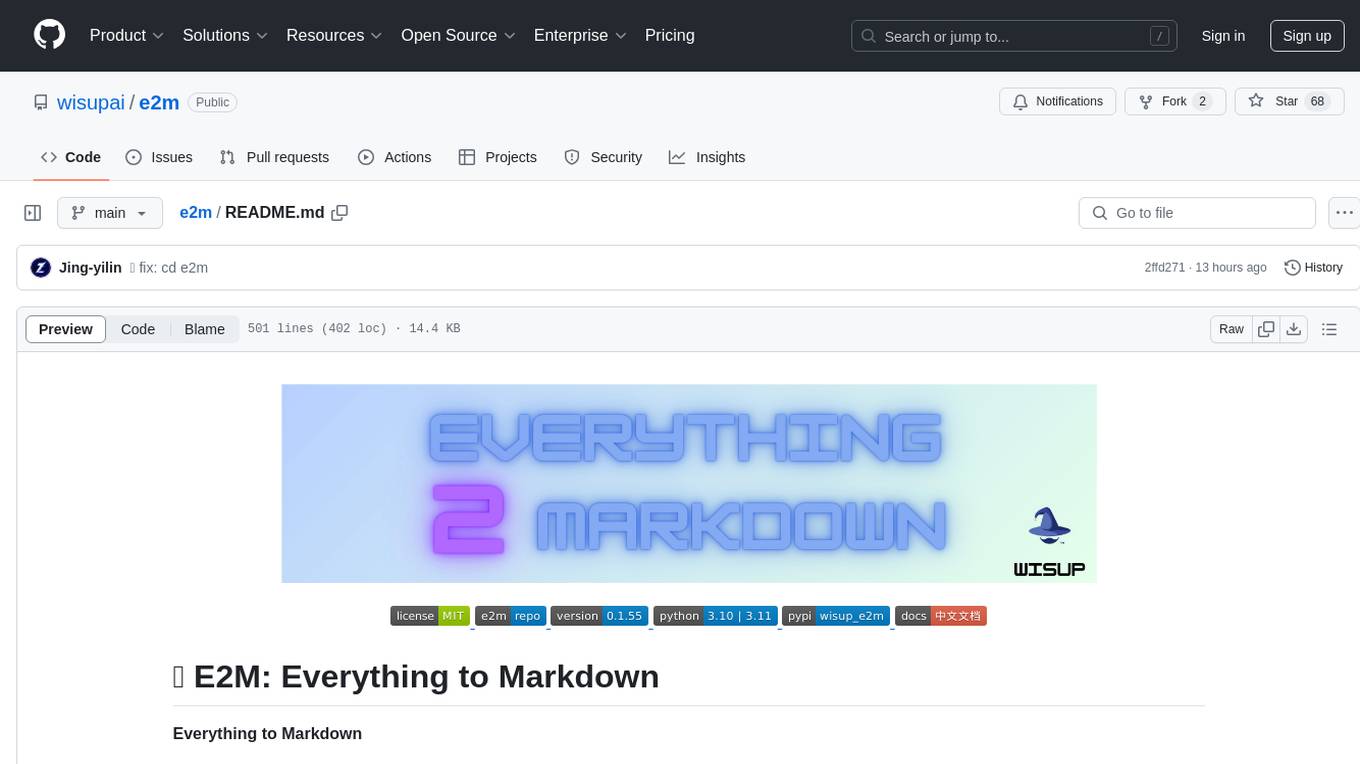
e2m
E2M is a Python library that can parse and convert various file types into Markdown format. It supports the conversion of multiple file formats, including doc, docx, epub, html, htm, url, pdf, ppt, pptx, mp3, and m4a. The ultimate goal of the E2M project is to provide high-quality data for Retrieval-Augmented Generation (RAG) and model training or fine-tuning. The core architecture consists of a Parser responsible for parsing various file types into text or image data, and a Converter responsible for converting text or image data into Markdown format.
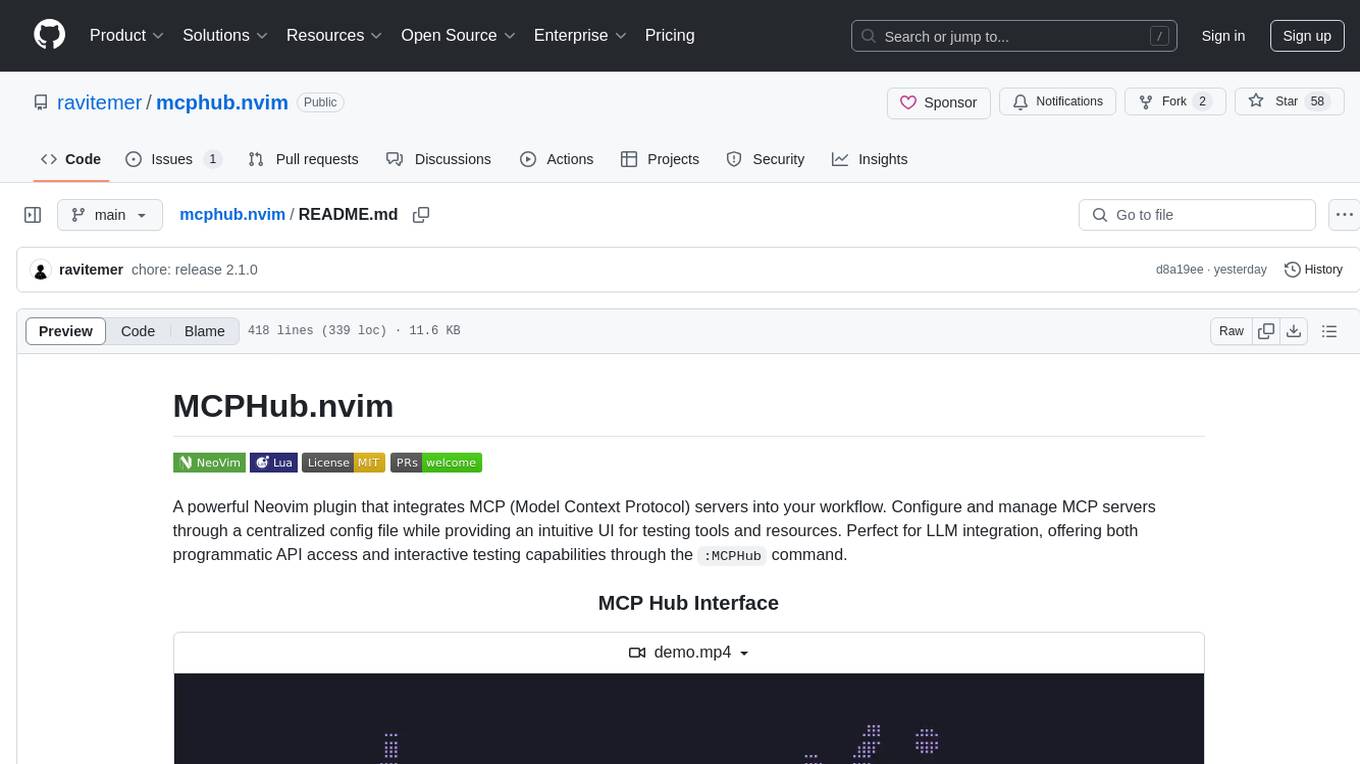
mcphub.nvim
MCPHub.nvim is a powerful Neovim plugin that integrates MCP (Model Context Protocol) servers into your workflow. It offers a centralized config file for managing servers and tools, with an intuitive UI for testing resources. Ideal for LLM integration, it provides programmatic API access and interactive testing through the `:MCPHub` command.
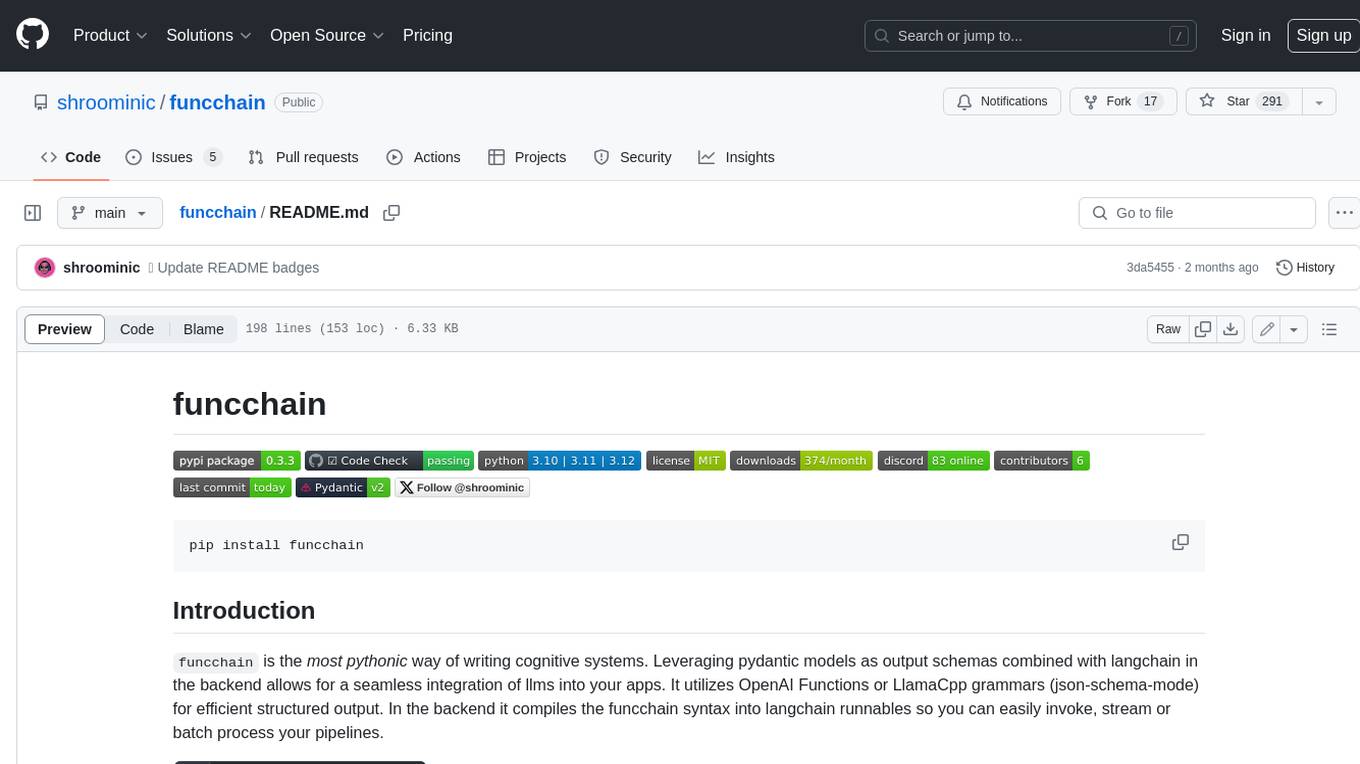
funcchain
Funcchain is a Python library that allows you to easily write cognitive systems by leveraging Pydantic models as output schemas and LangChain in the backend. It provides a seamless integration of LLMs into your apps, utilizing OpenAI Functions or LlamaCpp grammars (json-schema-mode) for efficient structured output. Funcchain compiles the Funcchain syntax into LangChain runnables, enabling you to invoke, stream, or batch process your pipelines effortlessly.
For similar tasks
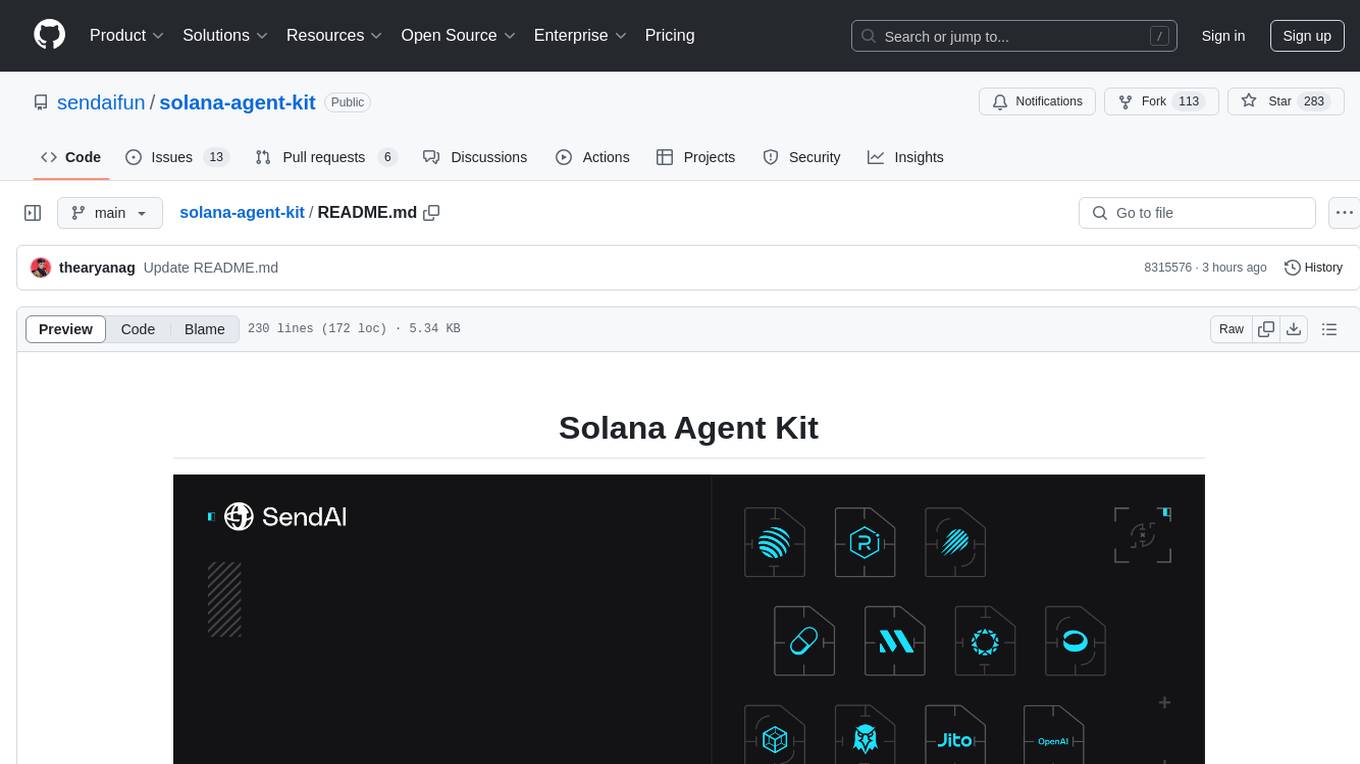
solana-agent-kit
Solana Agent Kit is an open-source toolkit designed for connecting AI agents to Solana protocols. It enables agents, regardless of the model used, to autonomously perform various Solana actions such as trading tokens, launching new tokens, lending assets, sending compressed airdrops, executing blinks, and more. The toolkit integrates core blockchain features like token operations, NFT management via Metaplex, DeFi integration, Solana blinks, AI integration features with LangChain, autonomous modes, and AI tools. It provides ready-to-use tools for blockchain operations, supports autonomous agent actions, and offers features like memory management, real-time feedback, and error handling. Solana Agent Kit facilitates tasks such as deploying tokens, creating NFT collections, swapping tokens, lending tokens, staking SOL, and sending SPL token airdrops via ZK compression. It also includes functionalities for fetching price data from Pyth and relies on key Solana and Metaplex libraries for its operations.
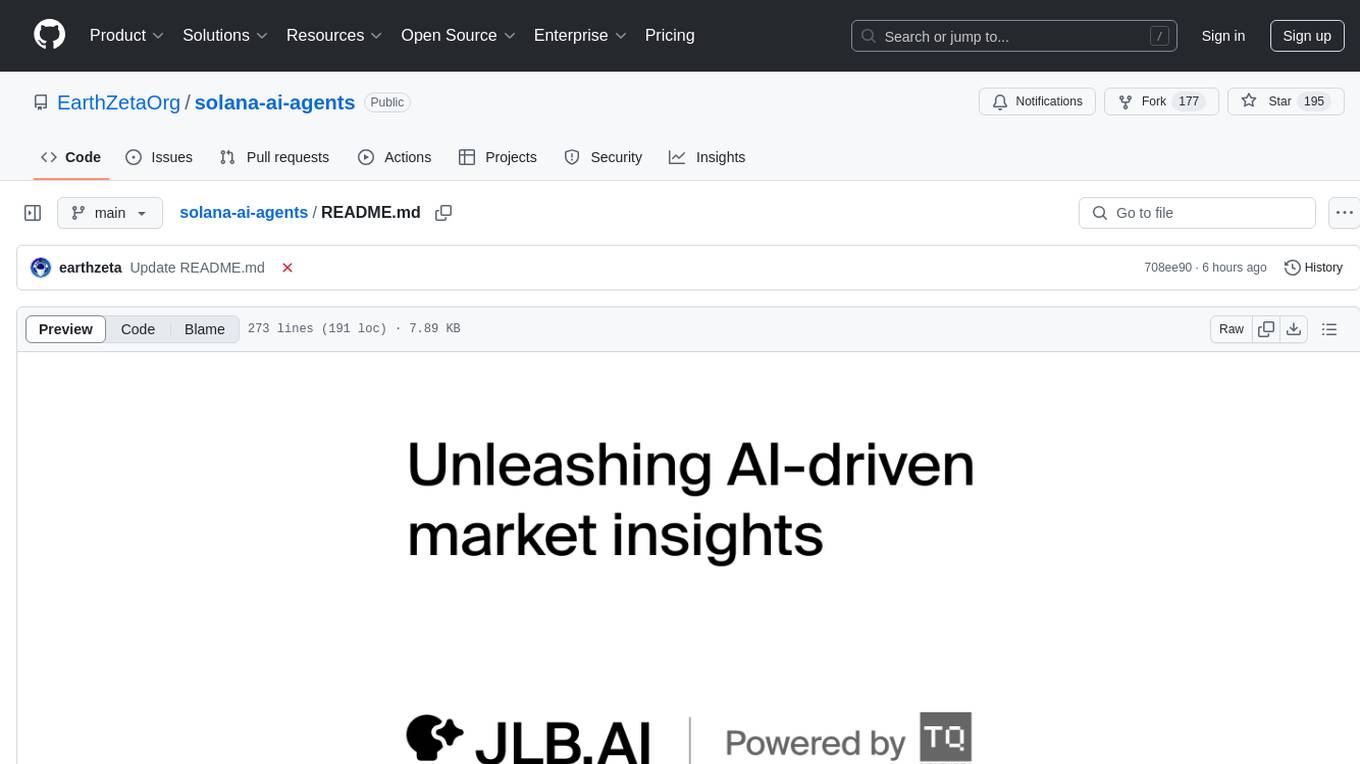
solana-ai-agents
JLB AI Agent is an innovative solution on the Solana blockchain that leverages artificial intelligence to automate complex tasks and enhance decision-making in the DeFi space. It offers real-time analytics, efficient operations, and seamless integration for both newcomers and experienced crypto enthusiasts. With features like autonomous trading, NFT management, DeFi insights, and comprehensive ecosystem integration, JLB empowers users with cutting-edge technology to navigate the dynamic landscape of blockchain.
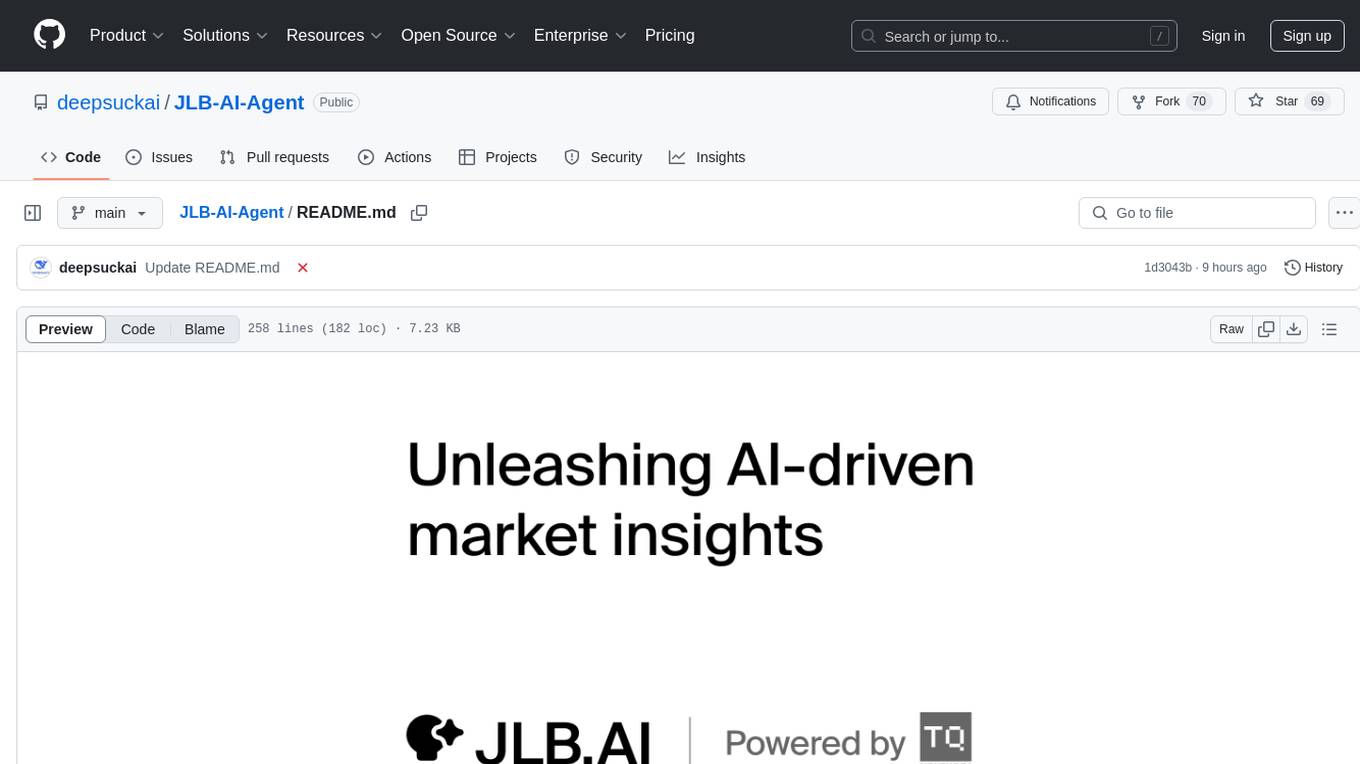
JLB-AI-Agent
JLB AI Agent is an innovative solution built on the Solana blockchain that harnesses the power of artificial intelligence to automate complex tasks and optimize decision-making in the DeFi space. It aims to provide real-time analytics, efficient operations, and seamless integration for both newcomers and experienced crypto enthusiasts. The tool offers features like blockchain agent chat terminal, real-time streaming implementation, trading infrastructure, NFT management, AI integration, and more, empowering users with autonomous technology where AI meets the dynamic landscape of blockchain.
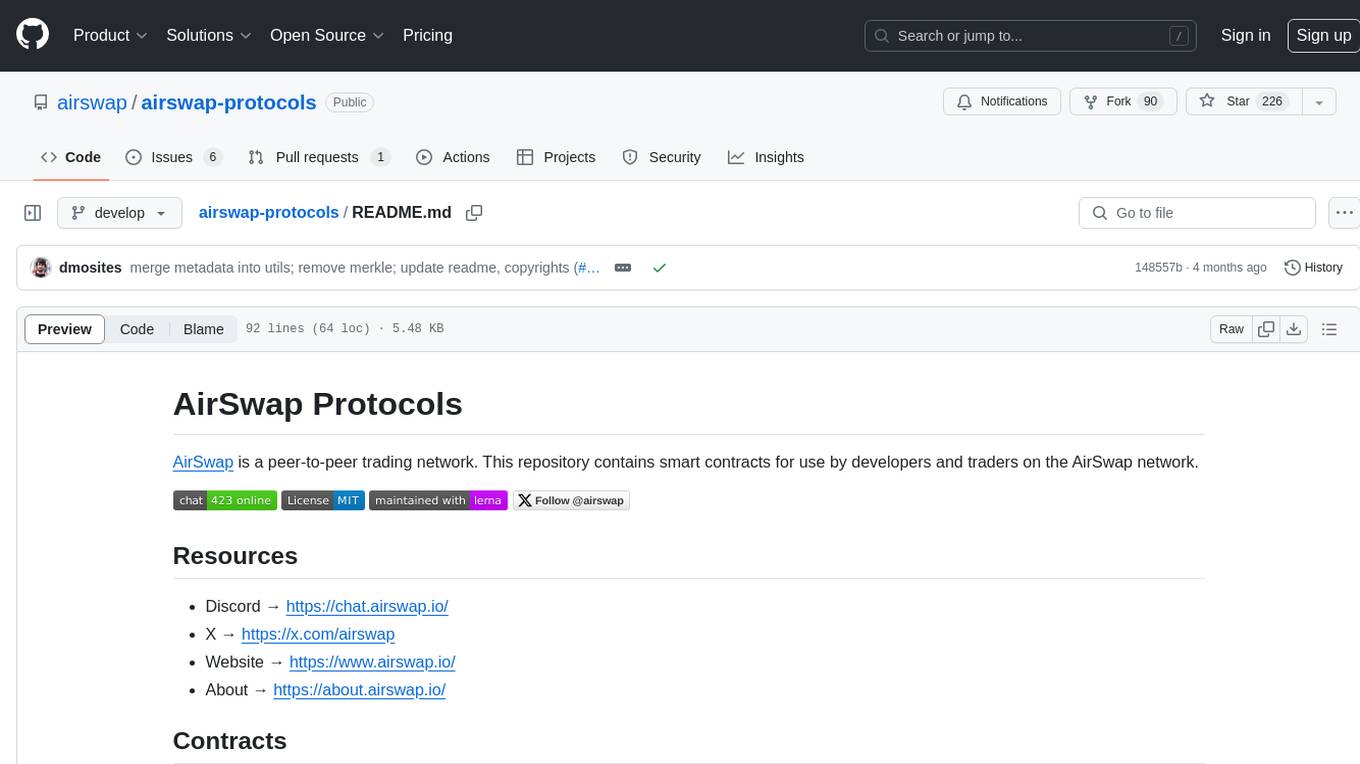
airswap-protocols
AirSwap Protocols is a repository containing smart contracts for developers and traders on the AirSwap peer-to-peer trading network. It includes various packages for functionalities like server registry, atomic token swap, staking, rewards pool, batch token and order calls, libraries, and utils. The repository follows a branching and release process for contracts and tools, with steps for regular development process and individual package features or patches. Users can deploy and verify contracts using specific commands with network flags.
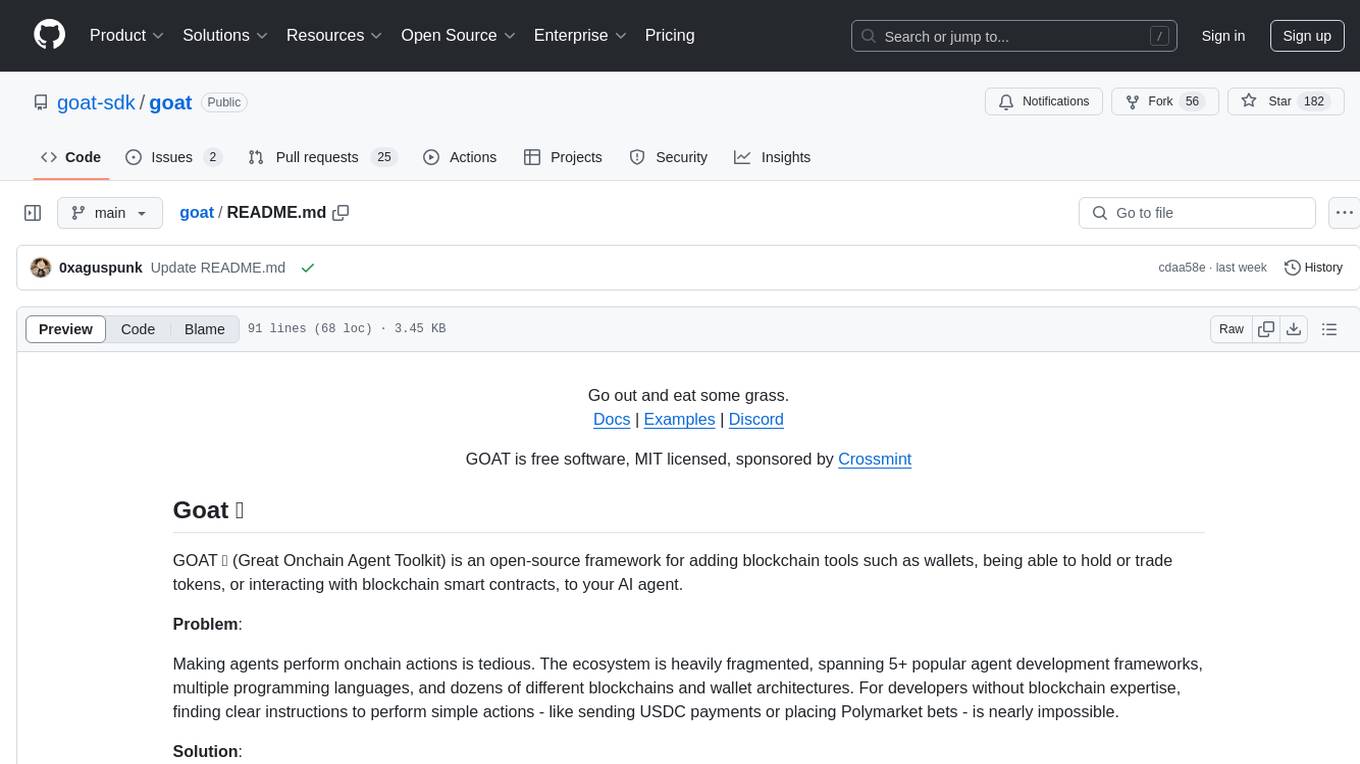
goat
GOAT (Great Onchain Agent Toolkit) is an open-source framework designed to simplify the process of making AI agents perform onchain actions by providing a provider-agnostic solution that abstracts away the complexities of interacting with blockchain tools such as wallets, token trading, and smart contracts. It offers a catalog of ready-made blockchain actions for agent developers and allows dApp/smart contract developers to develop plugins for easy access by agents. With compatibility across popular agent frameworks, support for multiple blockchains and wallet providers, and customizable onchain functionalities, GOAT aims to streamline the integration of blockchain capabilities into AI agents.
For similar jobs
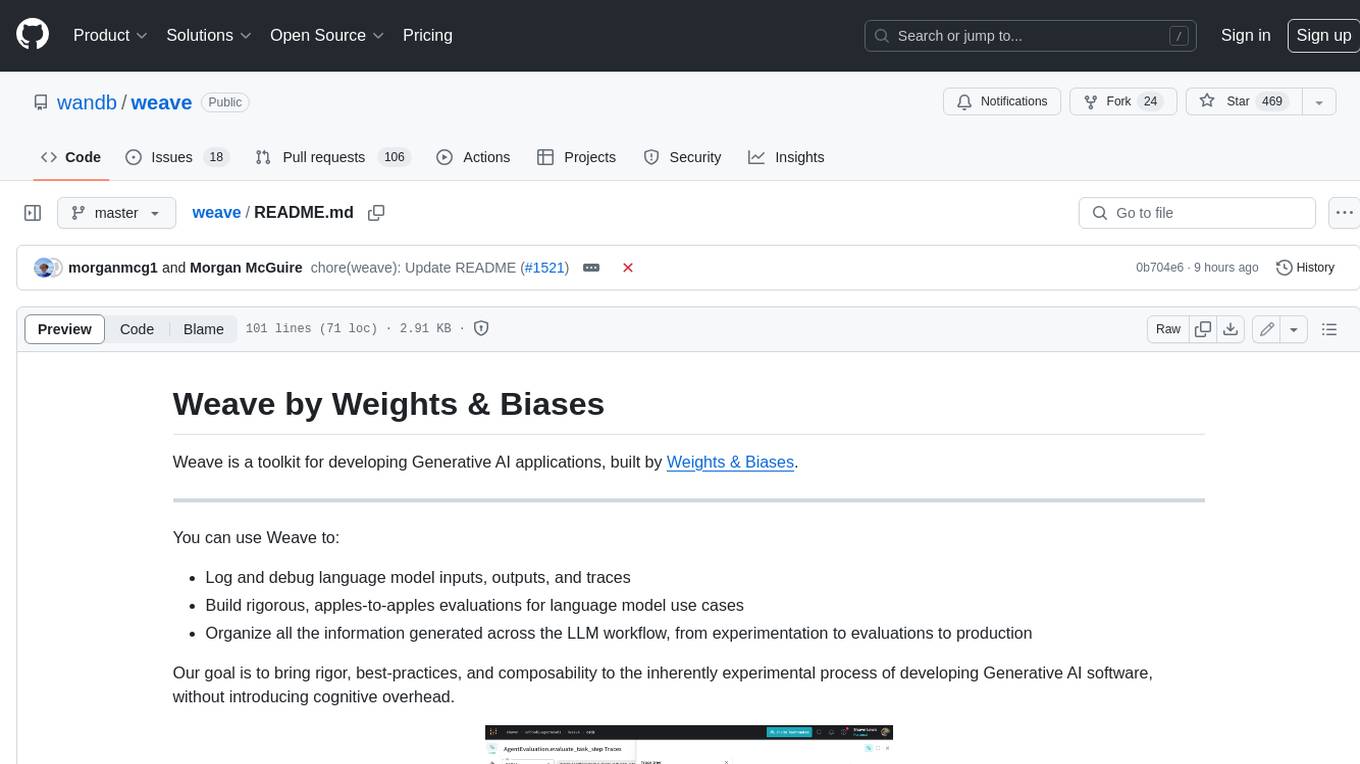
weave
Weave is a toolkit for developing Generative AI applications, built by Weights & Biases. With Weave, you can log and debug language model inputs, outputs, and traces; build rigorous, apples-to-apples evaluations for language model use cases; and organize all the information generated across the LLM workflow, from experimentation to evaluations to production. Weave aims to bring rigor, best-practices, and composability to the inherently experimental process of developing Generative AI software, without introducing cognitive overhead.
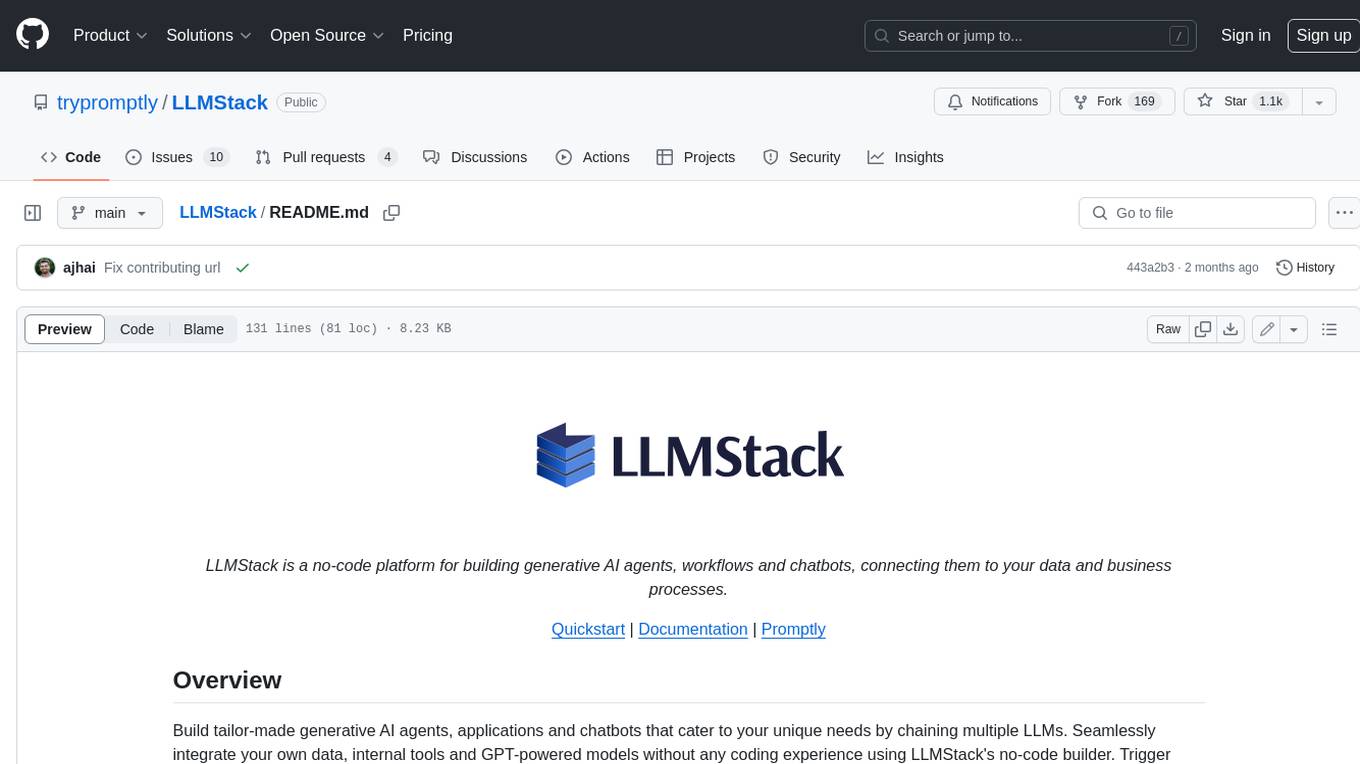
LLMStack
LLMStack is a no-code platform for building generative AI agents, workflows, and chatbots. It allows users to connect their own data, internal tools, and GPT-powered models without any coding experience. LLMStack can be deployed to the cloud or on-premise and can be accessed via HTTP API or triggered from Slack or Discord.
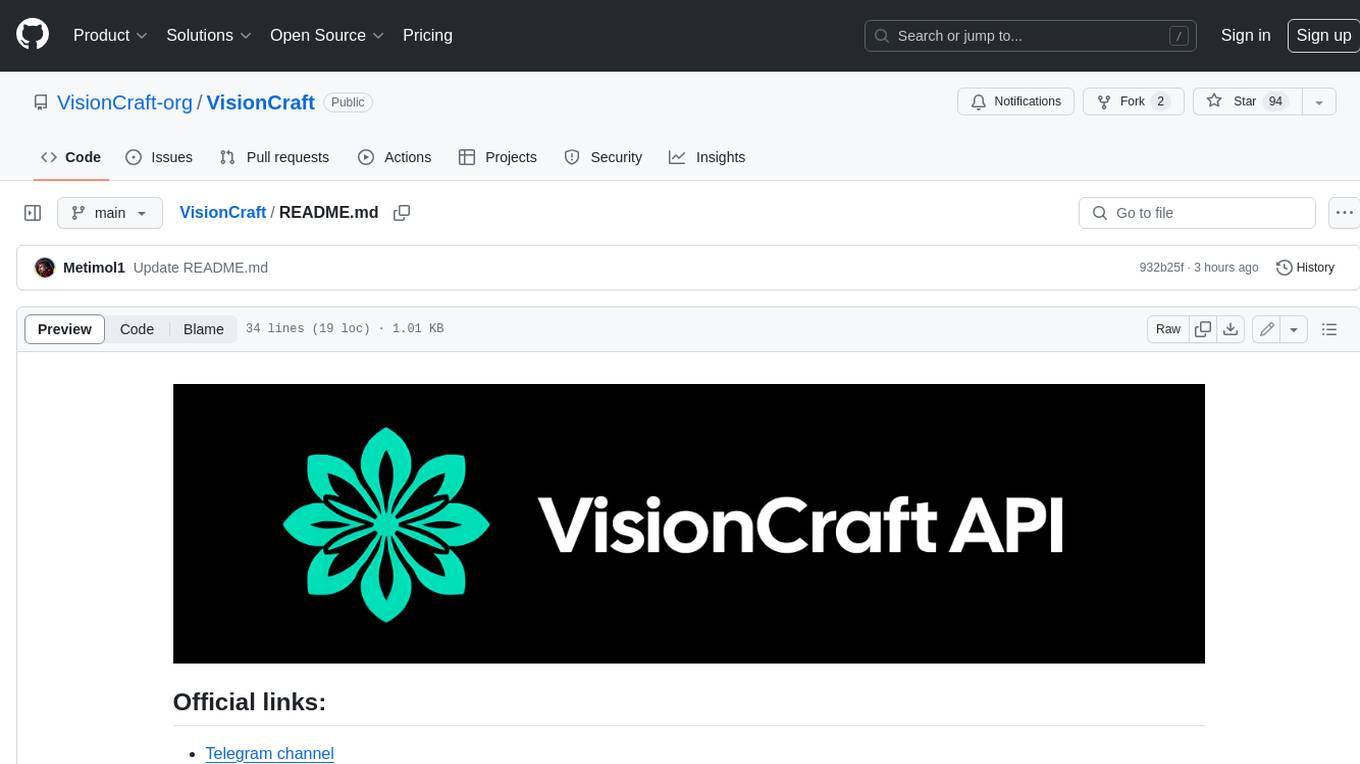
VisionCraft
The VisionCraft API is a free API for using over 100 different AI models. From images to sound.
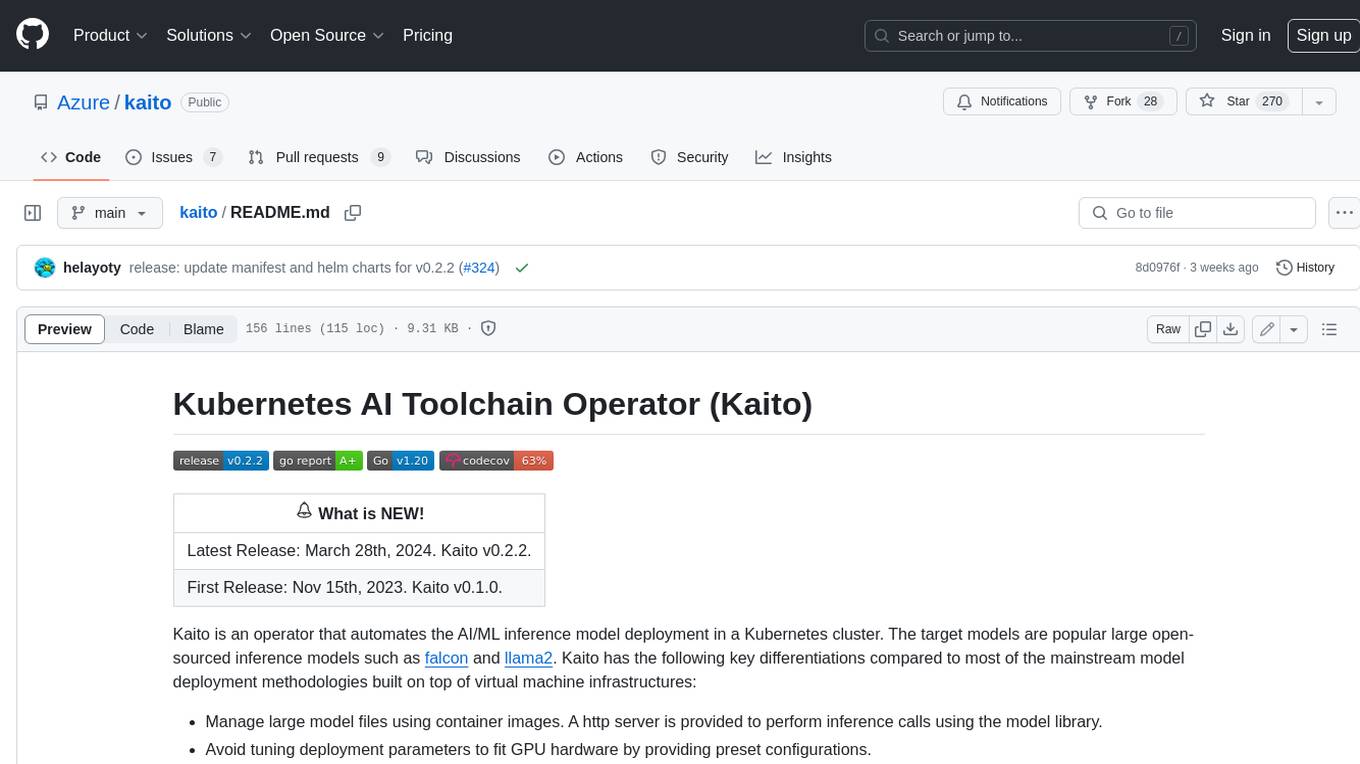
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
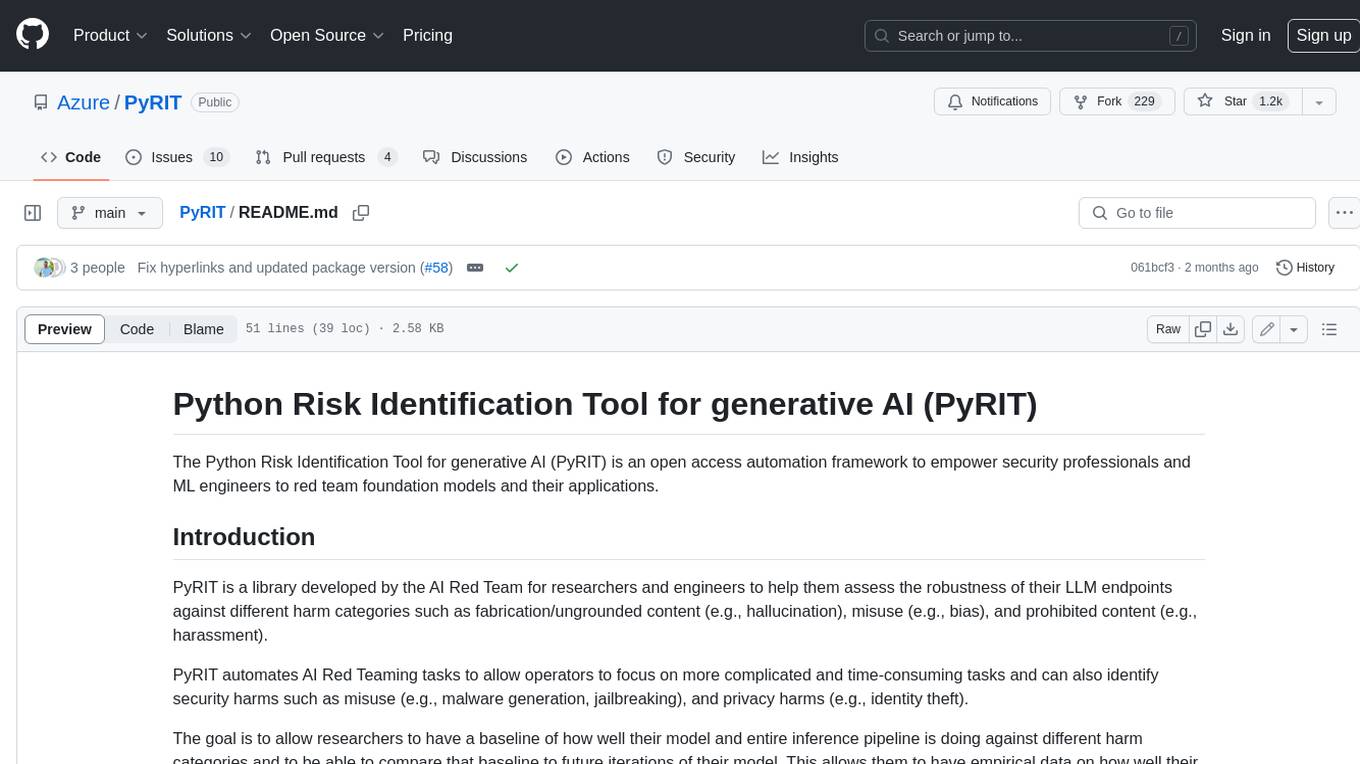
PyRIT
PyRIT is an open access automation framework designed to empower security professionals and ML engineers to red team foundation models and their applications. It automates AI Red Teaming tasks to allow operators to focus on more complicated and time-consuming tasks and can also identify security harms such as misuse (e.g., malware generation, jailbreaking), and privacy harms (e.g., identity theft). The goal is to allow researchers to have a baseline of how well their model and entire inference pipeline is doing against different harm categories and to be able to compare that baseline to future iterations of their model. This allows them to have empirical data on how well their model is doing today, and detect any degradation of performance based on future improvements.
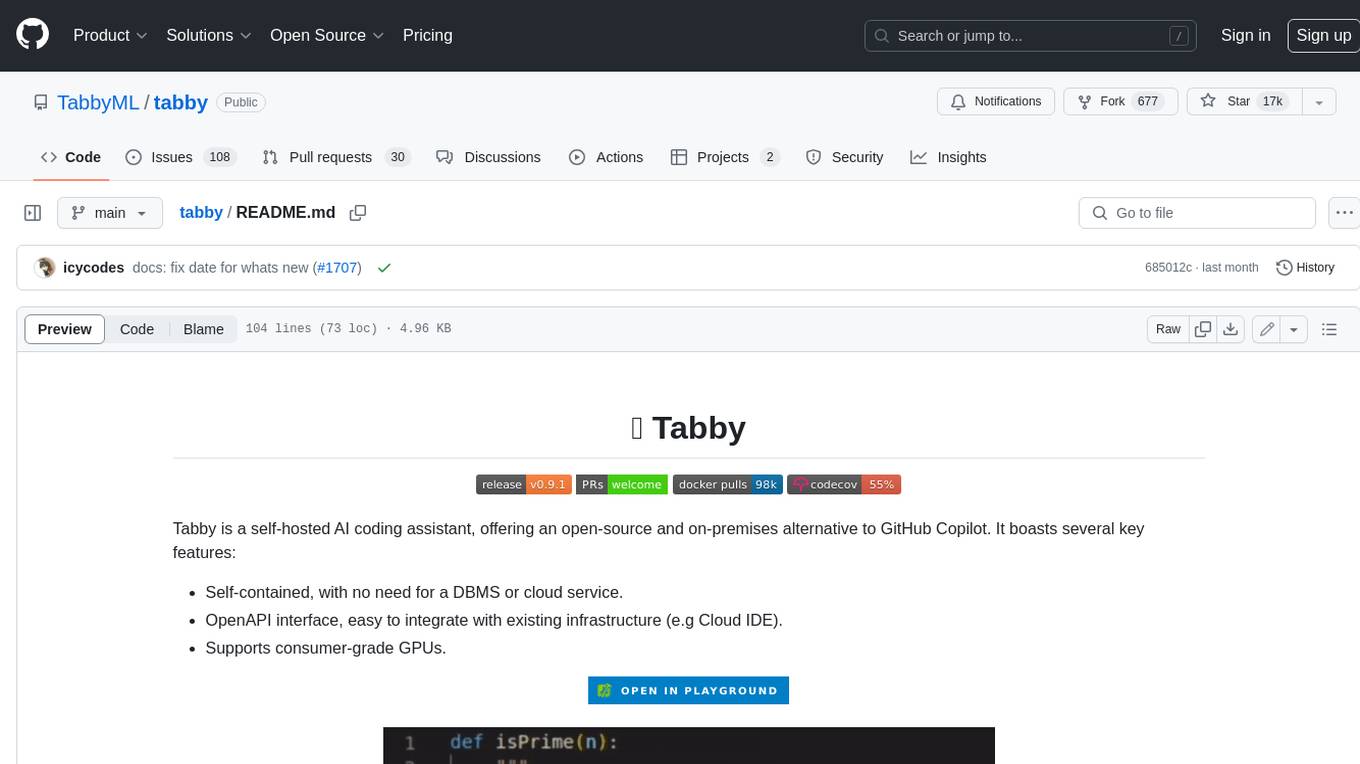
tabby
Tabby is a self-hosted AI coding assistant, offering an open-source and on-premises alternative to GitHub Copilot. It boasts several key features: * Self-contained, with no need for a DBMS or cloud service. * OpenAPI interface, easy to integrate with existing infrastructure (e.g Cloud IDE). * Supports consumer-grade GPUs.
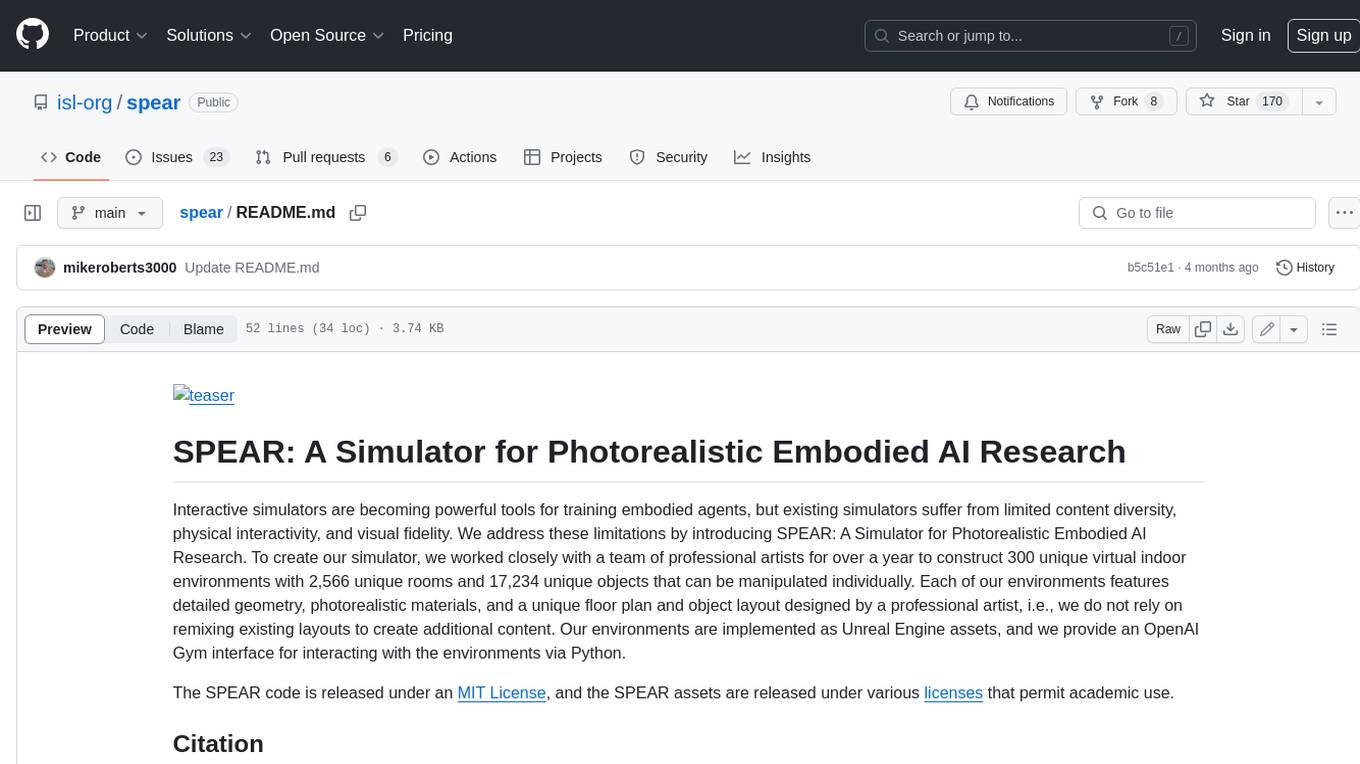
spear
SPEAR (Simulator for Photorealistic Embodied AI Research) is a powerful tool for training embodied agents. It features 300 unique virtual indoor environments with 2,566 unique rooms and 17,234 unique objects that can be manipulated individually. Each environment is designed by a professional artist and features detailed geometry, photorealistic materials, and a unique floor plan and object layout. SPEAR is implemented as Unreal Engine assets and provides an OpenAI Gym interface for interacting with the environments via Python.
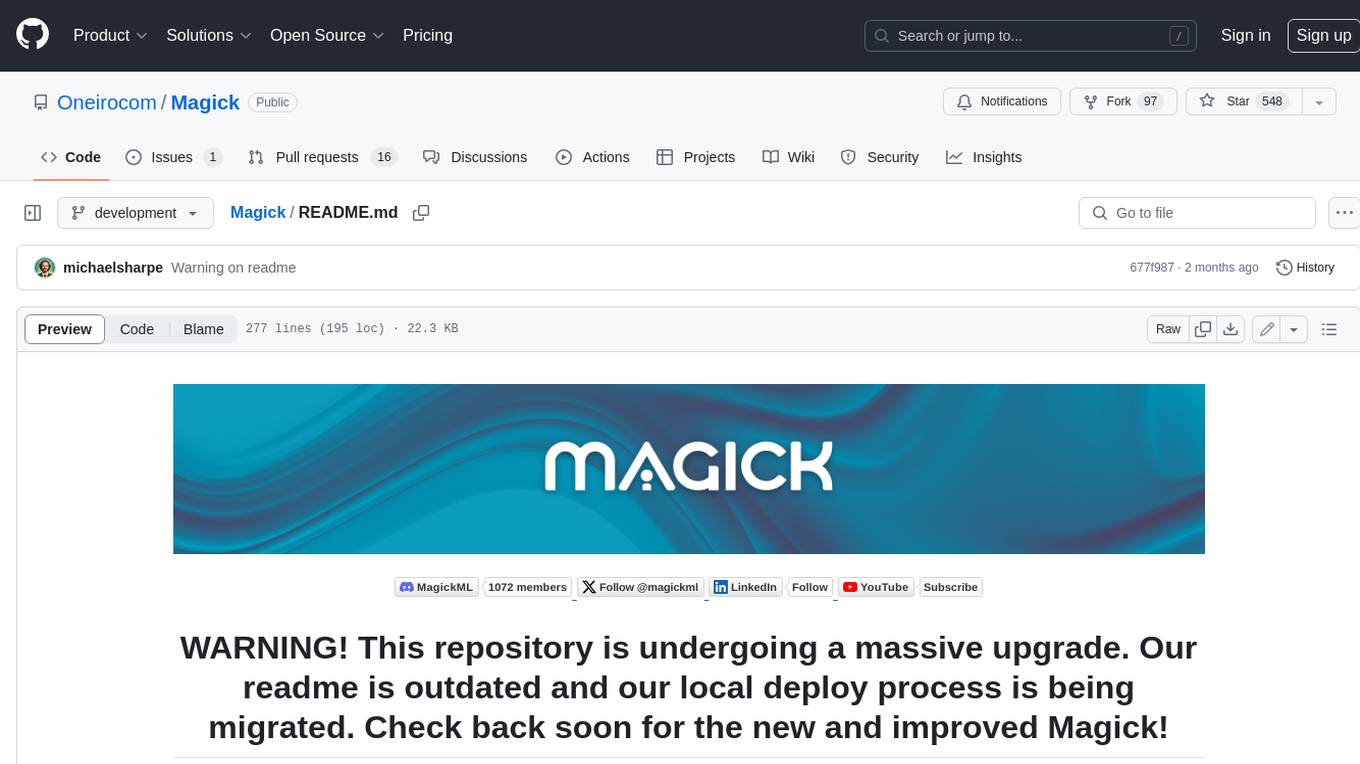
Magick
Magick is a groundbreaking visual AIDE (Artificial Intelligence Development Environment) for no-code data pipelines and multimodal agents. Magick can connect to other services and comes with nodes and templates well-suited for intelligent agents, chatbots, complex reasoning systems and realistic characters.