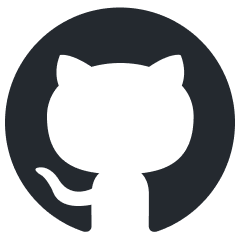
acte
A framework to build GUI-like Agent Tools, enhancement to Function Calling of LLM AI.
Stars: 113
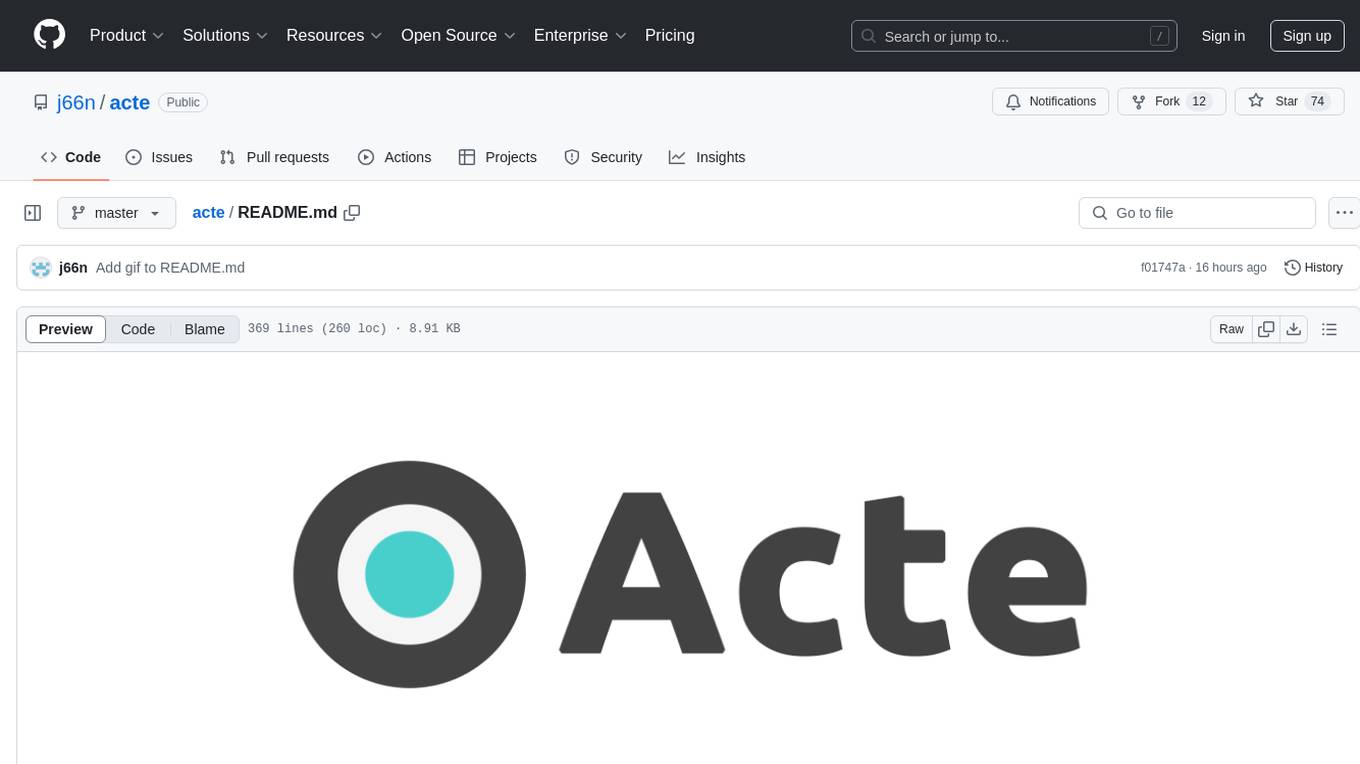
Acte is a framework designed to build GUI-like tools for AI Agents. It aims to address the issues of cognitive load and freedom degrees when interacting with multiple APIs in complex scenarios. By providing a graphical user interface (GUI) for Agents, Acte helps reduce cognitive load and constraints interaction, similar to how humans interact with computers through GUIs. The tool offers APIs for starting new sessions, executing actions, and displaying screens, accessible via HTTP requests or the SessionManager class.
README:
Acte is a framework to build GUI-like tools for AI Agents.
The current tool solution, as known as Function Calling, is based on API calling, like weather API, Google API, etc. It has two main problems in complex scenarios:
- Multiple APIs increase the cognitive load for AI Agents, potentially leading to reasoning errors, especially when the APIs have a strict calling order.
- Directly calling APIs is a way with too much freedom degrees, lacking constraints, potentially resulting in data errors.
The way Agents interact with APIs reminds me of how human interacts with computers in old days. A guy faces a black and thick screen and types the keyboard, while looking up commands in a manual. Not just Agent, we also faces these two problems.
Then a technique that surprised Steve Jobs comes up: Graphical User Interface (GUI).
Since then, most of us no longer directly interact with command lines (a kind of API). We interact with API through GUI. GUI generally solved these two problems by constraining interaction to reduce cognitive load and degrees of freedom.
This is why Agents also need GUI-like tools. I prefer calling it Agentic User Interface (AUI).
pip install acte
from acte import Component, v
from acte.chatbot import OpenaiChatbot
from acte.server import Server
from acte.session import SessionManager
class HelloWorld(Component):
def view(self) -> None:
v.text("Hello World")
server = Server(
session_manager=SessionManager(HelloWorld, debug=True),
chatbot=OpenaiChatbot( # default model is gpt-4o
api_key="YOUR OPENAI API KEY",
)
)
if __name__ == '__main__':
server.run()
-
Copy the code to a Python file, and set OpenAI Api Key.
-
Run the code, and visit the playground: http://127.0.0.1:8000.
-
Input "Hi, please new a session, and tell what you see." Then, the Agent will interact with Screen, and give you a response.
Note: You can also interact with Screen to debug your app.
from acte import Component, v
from acte.chatbot import OpenaiChatbot
from acte.server import Server
from acte.session import SessionManager
from acte.state.signal import Signal
class Counter(Component):
def __init__(self) -> None:
self._n = Signal(0)
def view(self) -> None:
v.text("This is a counter.")
with v.div():
v.text(lambda: f"Current Value: {self._n.value}")
# _n is Signal, so you need to use self._n.value in lambda
v.button("add", on_press=self._add)
async def _add(self) -> None:
await self._n.set(self._n.value + 1)
server = Server(
session_manager=SessionManager(Counter, debug=True),
chatbot=OpenaiChatbot(
api_key="YOUR OPENAI API KEY",
)
)
if __name__ == '__main__':
server.run()
-
Agent can press the button in Screen. The number on the right-top of the button is Interactive ID. Agent interacts with Screen by pointing to ID.
-
Interaction's result will show in Screen. You can click "View"s in Dialog to check the Screen status in each step.
from typing import Callable, Awaitable
from acte.chatbot import OpenaiChatbot
from acte.schema import IntSchema
from acte.server import Server
from acte.session import SessionManager
from acte import Component, v, Prop, as_prop
from acte.state.signal import Signal
class MenuItem(Component):
def __init__(
self,
name: Prop[str], # Prop = Ref[T] | T
price: Prop[float],
quantity: Prop[int],
on_quantity_change: Callable[[str, float, int], Awaitable[None]]
) -> None:
self._name = as_prop(name) # as_prop is to convert T to Ref[T]
self._price = as_prop(price)
self._quantity = as_prop(quantity)
self._on_quantity_change = on_quantity_change
def view(self) -> None:
with v.div():
v.text(lambda: f"{self._name.value}: ${self._price.value}")
v.input("quantity", self._quantity, self._on_set, schema=IntSchema())
async def _on_set(self, value: str) -> None:
await self._on_quantity_change(
self._name.value,
self._price.value,
0 if value == '' else int(value)
)
class Menu(Component):
def __init__(self) -> None:
self._menu = {
"Pizza": {"price": 10.0, "quantity": Signal(0)}, # Signal is a kind of Ref, but can be set
"Coke": {"price": 2.0, "quantity": Signal(0)},
}
def view(self) -> None:
v.text("Super Restaurant Menu")
for key, value in self._menu.items():
v.component(
MenuItem(
name=key,
price=value['price'],
quantity=value['quantity'],
on_quantity_change=self._on_quantity_change,
)
)
v.text(self.total)
v.button("checkout", on_press=self._checkout)
def total(self) -> str:
total = 0
for key, value in self._menu.items():
total += value['price'] * value['quantity'].value
return f"Total: ${total}"
async def _on_quantity_change(self, name: str, price: float, quantity: int) -> None:
await self._menu[name]['quantity'].set(quantity)
async def _checkout(self) -> None:
total = self.total()
for value in self._menu.values():
await value['quantity'].set(0)
print(f"Checkout: {total}")
server = Server(
session_manager=SessionManager(Menu, debug=True),
chatbot=OpenaiChatbot(
system_message="You are restaurant assistant to help customers to order food through App. "
"You should confirm before Checkout",
api_key="YOUR OPENAI API KEY",
)
)
if __name__ == '__main__':
server.run()
-
Input fields also have their own Interactive ID. Agent can take multiple actions in one calling.
-
You can define the backend logic after Agent press the button, such as make a request.
Acte Tool has 3 APIs: new_session
, execute
, and display
,
which can be accessed by HTTP request or SessionManager
Start a new App session, then display the session's latest screen.
POST /session
from acte.session import SessionManager
sm = SessionManager(...)
sm.new_session()
{
"session_id": str,
"screen": str,
}
Execute one or more action(s), then display the session's latest screen.
POST /execute
json:
{
"session_id": "str",
"actions": [
{
"target_id": "str",
"action_type": "str",
"value": "str | None",
},
]
}
from acte.session import SessionManager
sm = SessionManager(...)
sm.execute(
{
"session_id": str,
"actions": [
{
"target_id": str, # interactive id
"action_type": str, # one of ["press", "fill"]
"value": str | None, # required when action_type is "fill"
},
...
]
}
)
{
"session_id": str,
"screen": str,
}
Display the session's latest screen.
POST /display
json:
{
"session_id": "str",
}
from acte.session import SessionManager
sm = SessionManager(...)
sm.execute(
{
"session_id": str,
}
)
{
"session_id": str,
"screen": str,
}
-
[ ] Full Document
-
[ ] Test Code
Note: The project is in Alpha stage. The API may change frequently.
The project is licensed under the terms of the MIT license.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for acte
Similar Open Source Tools
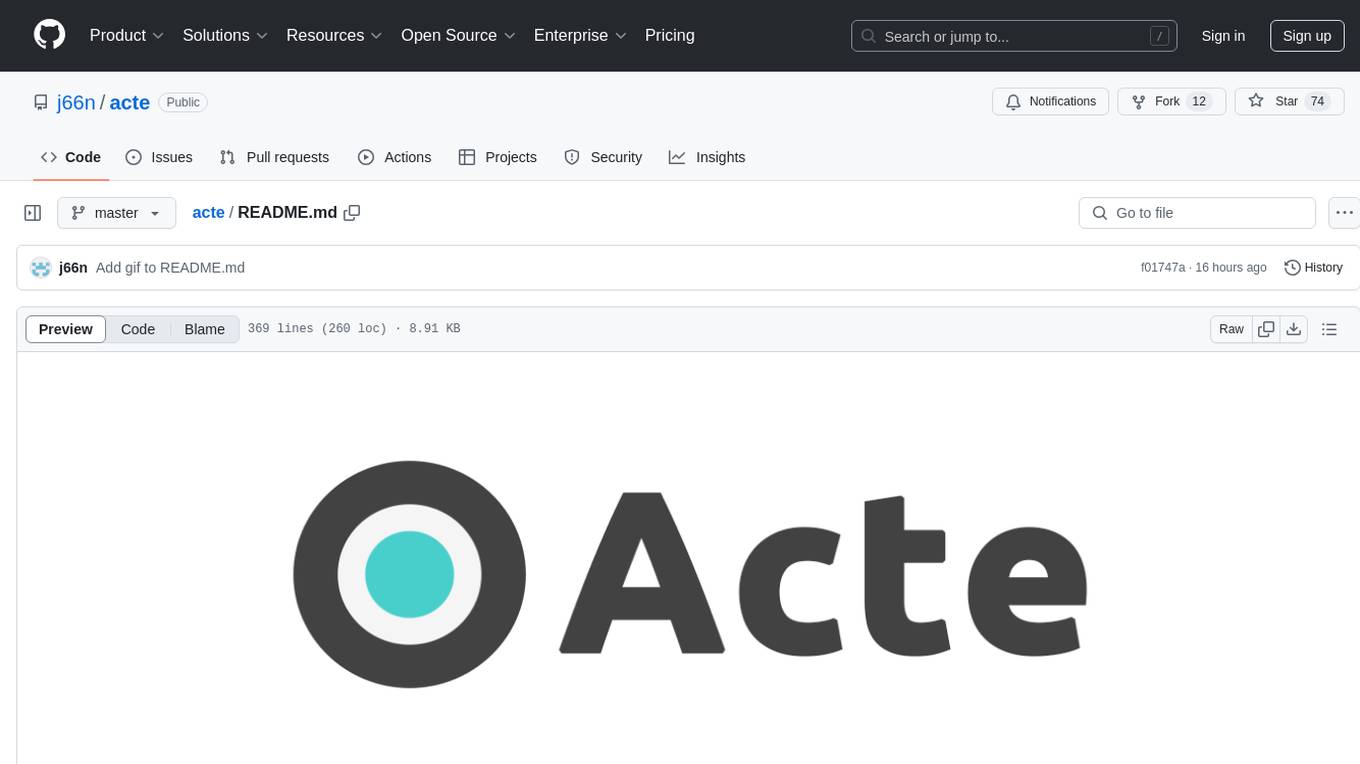
acte
Acte is a framework designed to build GUI-like tools for AI Agents. It aims to address the issues of cognitive load and freedom degrees when interacting with multiple APIs in complex scenarios. By providing a graphical user interface (GUI) for Agents, Acte helps reduce cognitive load and constraints interaction, similar to how humans interact with computers through GUIs. The tool offers APIs for starting new sessions, executing actions, and displaying screens, accessible via HTTP requests or the SessionManager class.
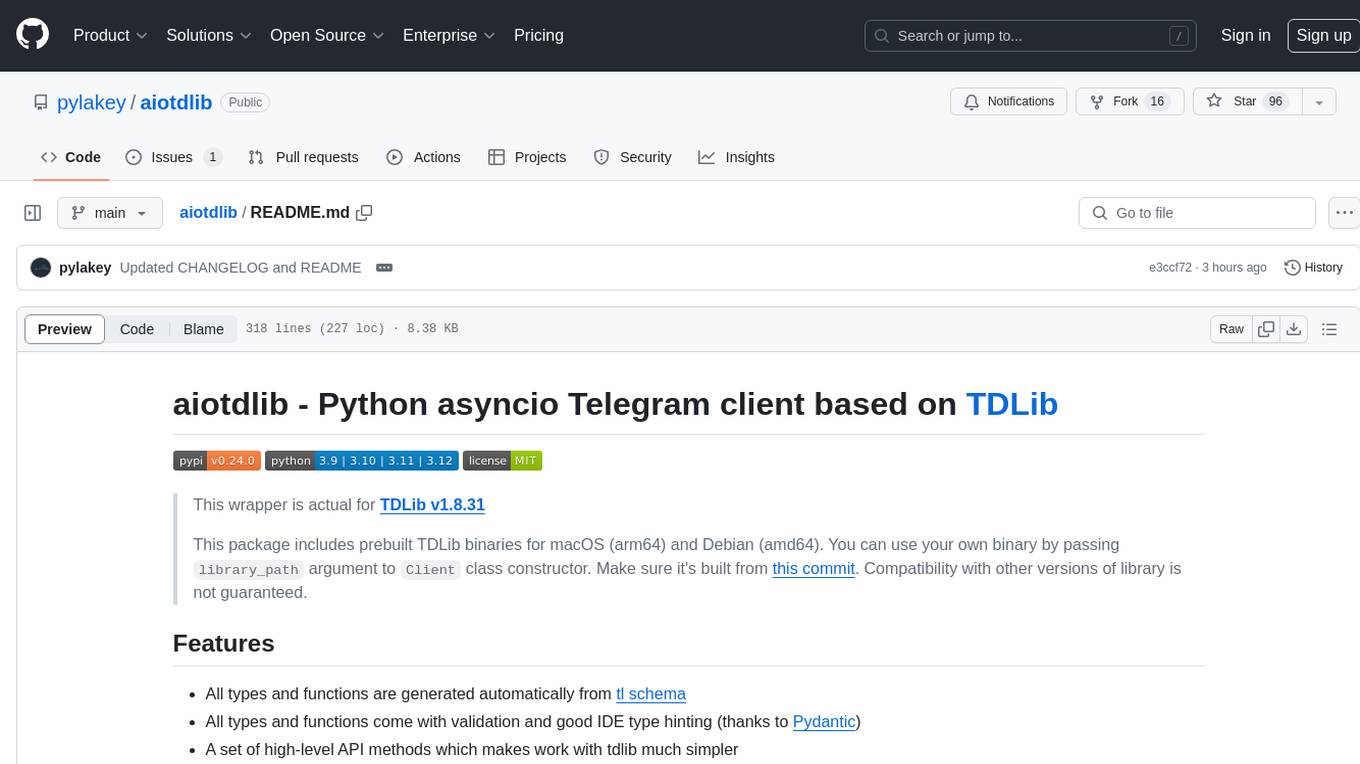
aiotdlib
aiotdlib is a Python asyncio Telegram client based on TDLib. It provides automatic generation of types and functions from tl schema, validation, good IDE type hinting, and high-level API methods for simpler work with tdlib. The package includes prebuilt TDLib binaries for macOS (arm64) and Debian Bullseye (amd64). Users can use their own binary by passing `library_path` argument to `Client` class constructor. Compatibility with other versions of the library is not guaranteed. The tool requires Python 3.9+ and users need to get their `api_id` and `api_hash` from Telegram docs for installation and usage.
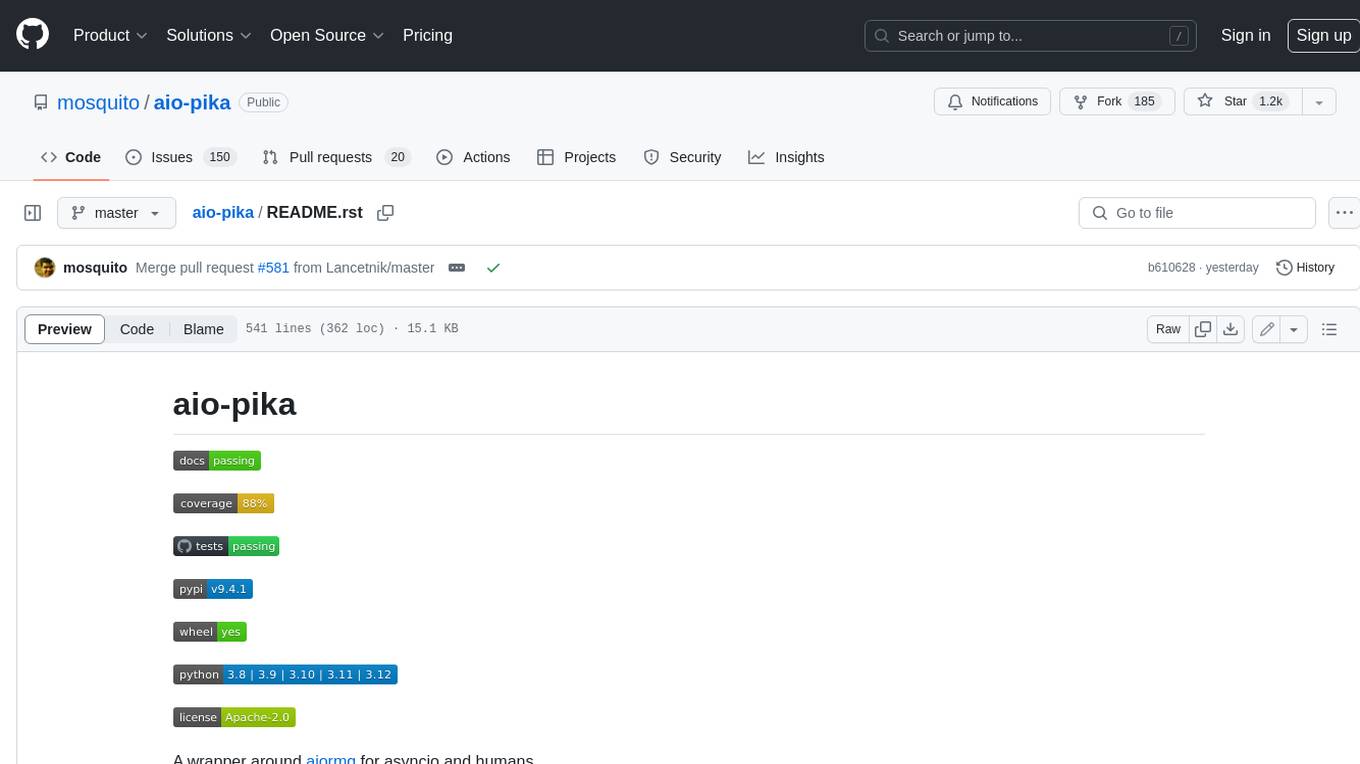
aio-pika
Aio-pika is a wrapper around aiormq for asyncio and humans. It provides a completely asynchronous API, object-oriented API, transparent auto-reconnects with complete state recovery, Python 3.7+ compatibility, transparent publisher confirms support, transactions support, and complete type-hints coverage.
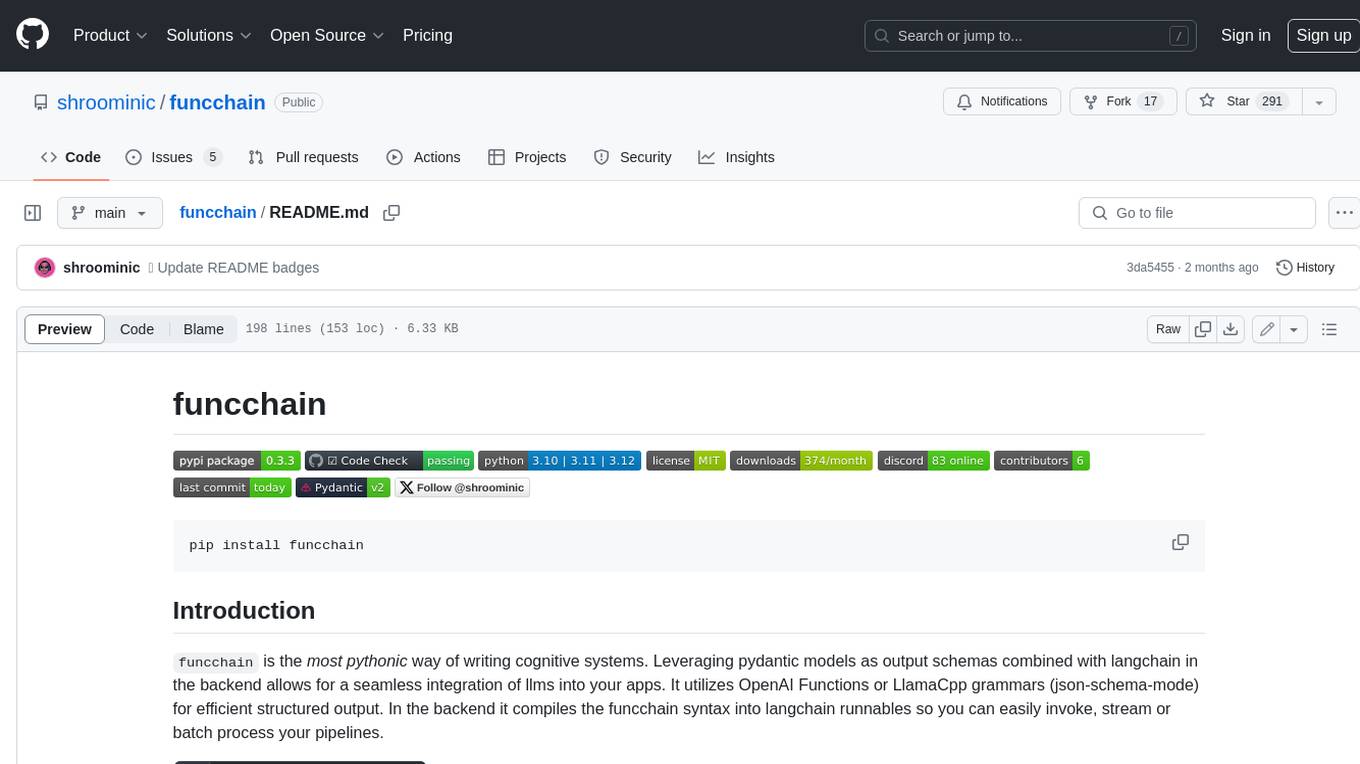
funcchain
Funcchain is a Python library that allows you to easily write cognitive systems by leveraging Pydantic models as output schemas and LangChain in the backend. It provides a seamless integration of LLMs into your apps, utilizing OpenAI Functions or LlamaCpp grammars (json-schema-mode) for efficient structured output. Funcchain compiles the Funcchain syntax into LangChain runnables, enabling you to invoke, stream, or batch process your pipelines effortlessly.
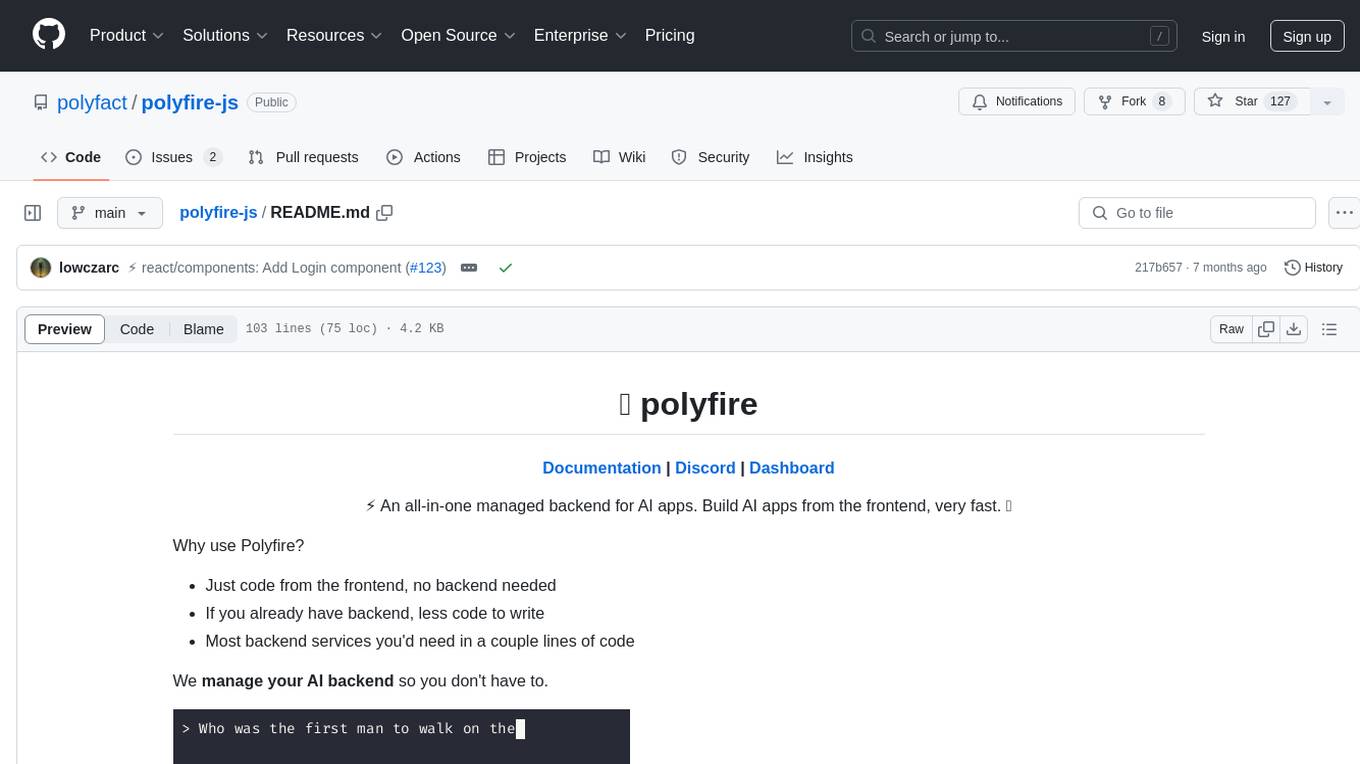
polyfire-js
Polyfire is an all-in-one managed backend for AI apps that allows users to build AI apps directly from the frontend, eliminating the need for a separate backend. It simplifies the process by providing most backend services in just a few lines of code. With Polyfire, users can easily create chatbots, transcribe audio files to text, generate simple text, create a long-term memory, and generate images with Dall-E. The tool also offers starter guides and tutorials to help users get started quickly and efficiently.
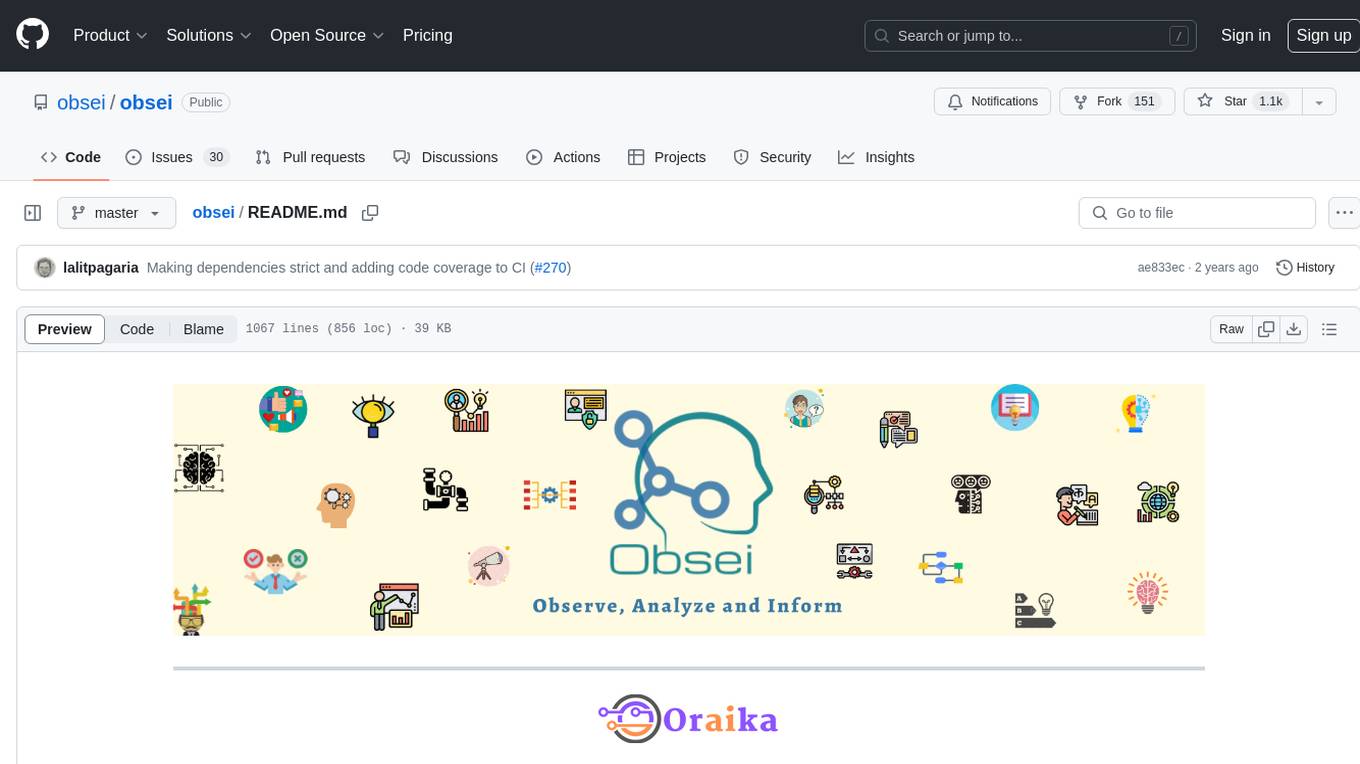
obsei
Obsei is an open-source, low-code, AI powered automation tool that consists of an Observer to collect unstructured data from various sources, an Analyzer to analyze the collected data with various AI tasks, and an Informer to send analyzed data to various destinations. The tool is suitable for scheduled jobs or serverless applications as all Observers can store their state in databases. Obsei is still in alpha stage, so caution is advised when using it in production. The tool can be used for social listening, alerting/notification, automatic customer issue creation, extraction of deeper insights from feedbacks, market research, dataset creation for various AI tasks, and more based on creativity.
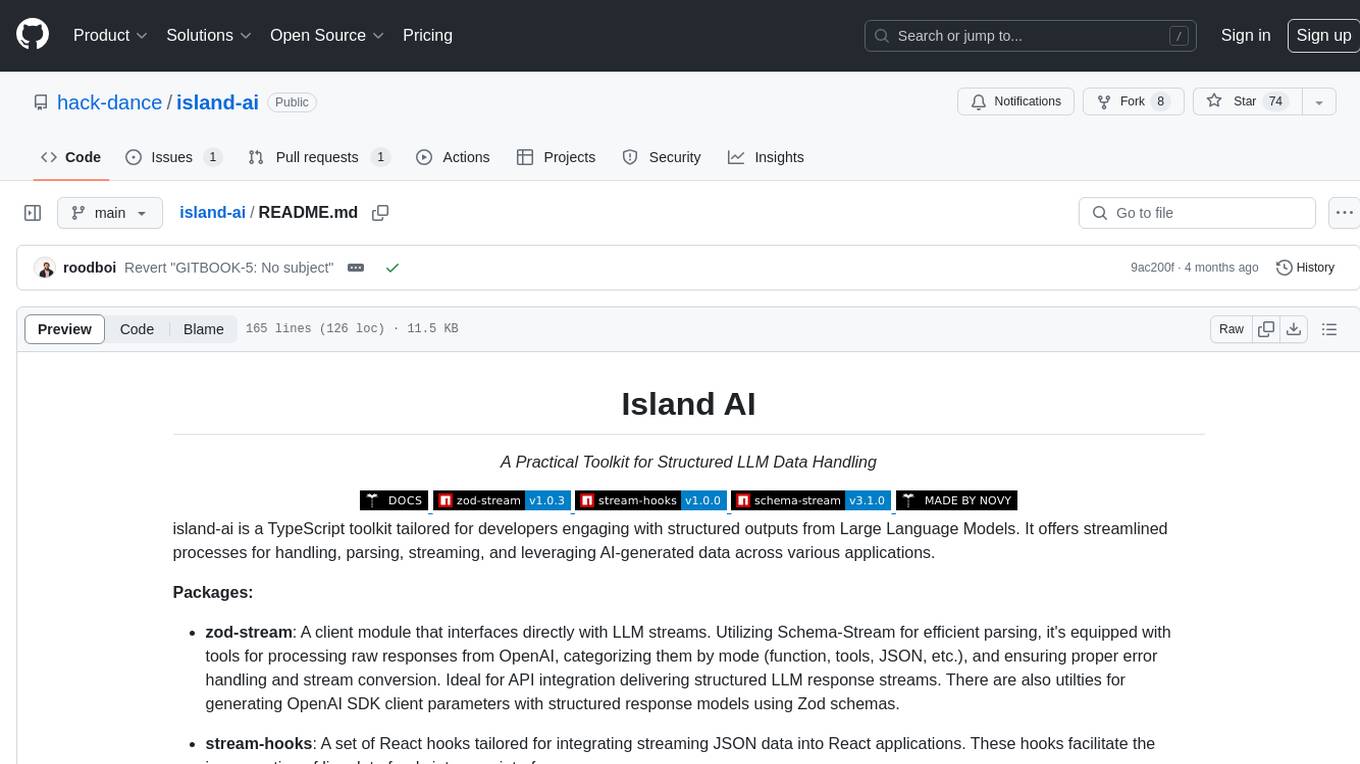
island-ai
island-ai is a TypeScript toolkit tailored for developers engaging with structured outputs from Large Language Models. It offers streamlined processes for handling, parsing, streaming, and leveraging AI-generated data across various applications. The toolkit includes packages like zod-stream for interfacing with LLM streams, stream-hooks for integrating streaming JSON data into React applications, and schema-stream for JSON streaming parsing based on Zod schemas. Additionally, related packages like @instructor-ai/instructor-js focus on data validation and retry mechanisms, enhancing the reliability of data processing workflows.
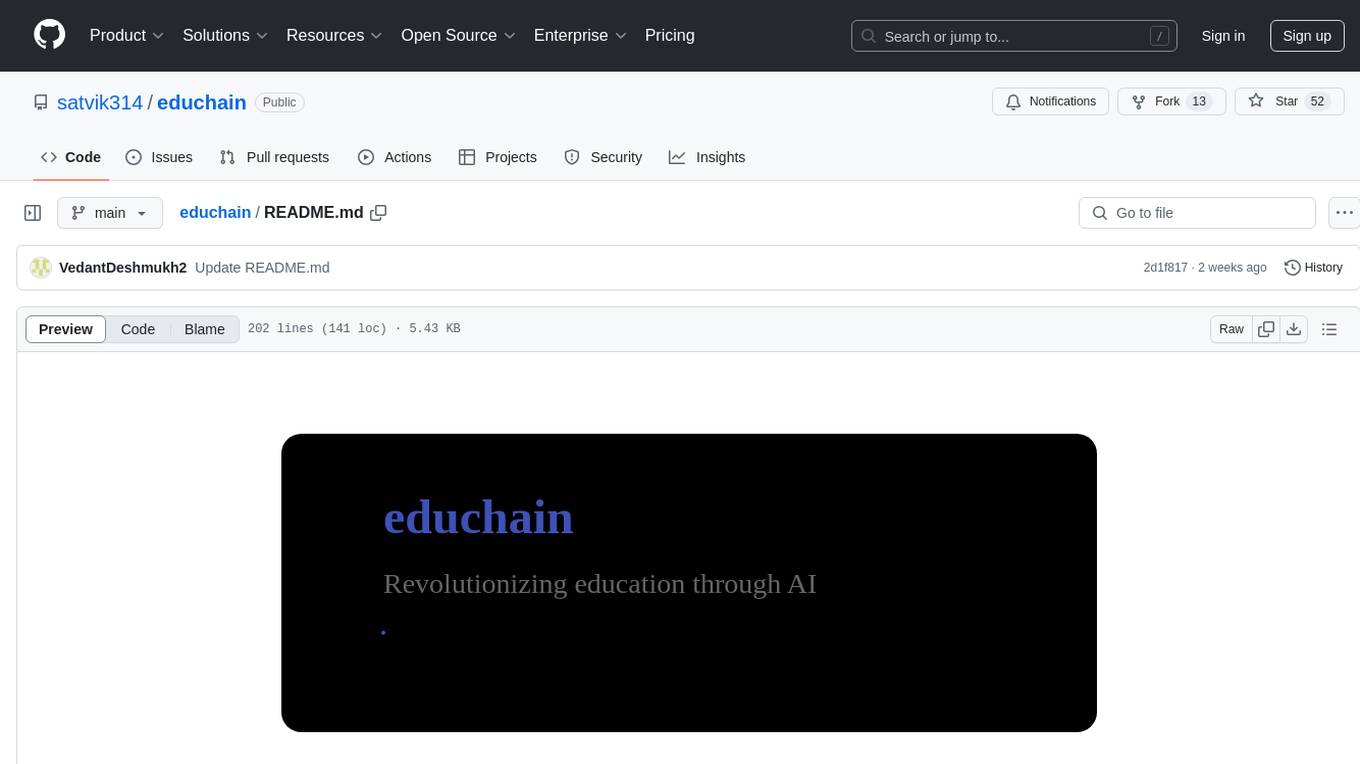
educhain
Educhain is a powerful Python package that leverages Generative AI to create engaging and personalized educational content. It enables users to generate multiple-choice questions, create lesson plans, and support various LLM models. Users can export questions to JSON, PDF, and CSV formats, customize prompt templates, and generate questions from text, PDF, URL files, youtube videos, and images. Educhain outperforms traditional methods in content generation speed and quality. It offers advanced configuration options and has a roadmap for future enhancements, including integration with popular Learning Management Systems and a mobile app for content generation on-the-go.
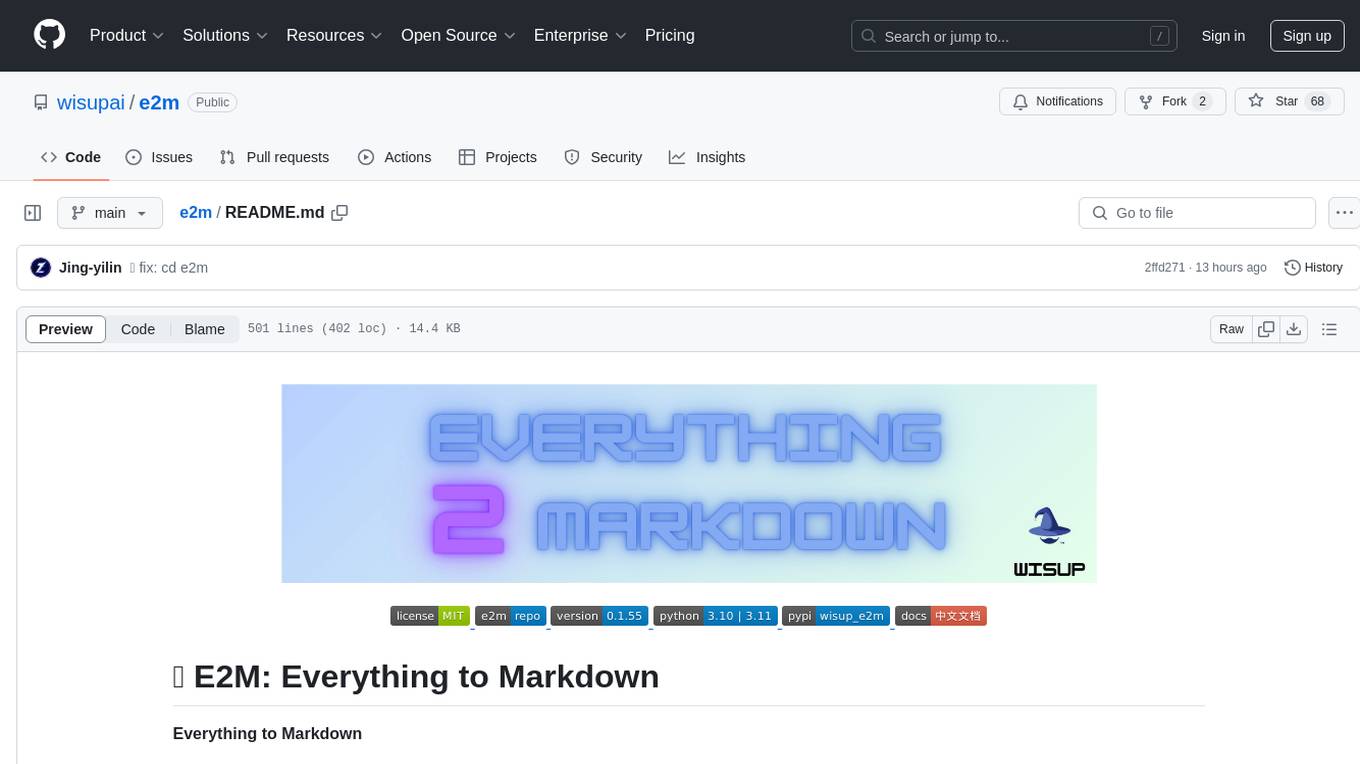
e2m
E2M is a Python library that can parse and convert various file types into Markdown format. It supports the conversion of multiple file formats, including doc, docx, epub, html, htm, url, pdf, ppt, pptx, mp3, and m4a. The ultimate goal of the E2M project is to provide high-quality data for Retrieval-Augmented Generation (RAG) and model training or fine-tuning. The core architecture consists of a Parser responsible for parsing various file types into text or image data, and a Converter responsible for converting text or image data into Markdown format.
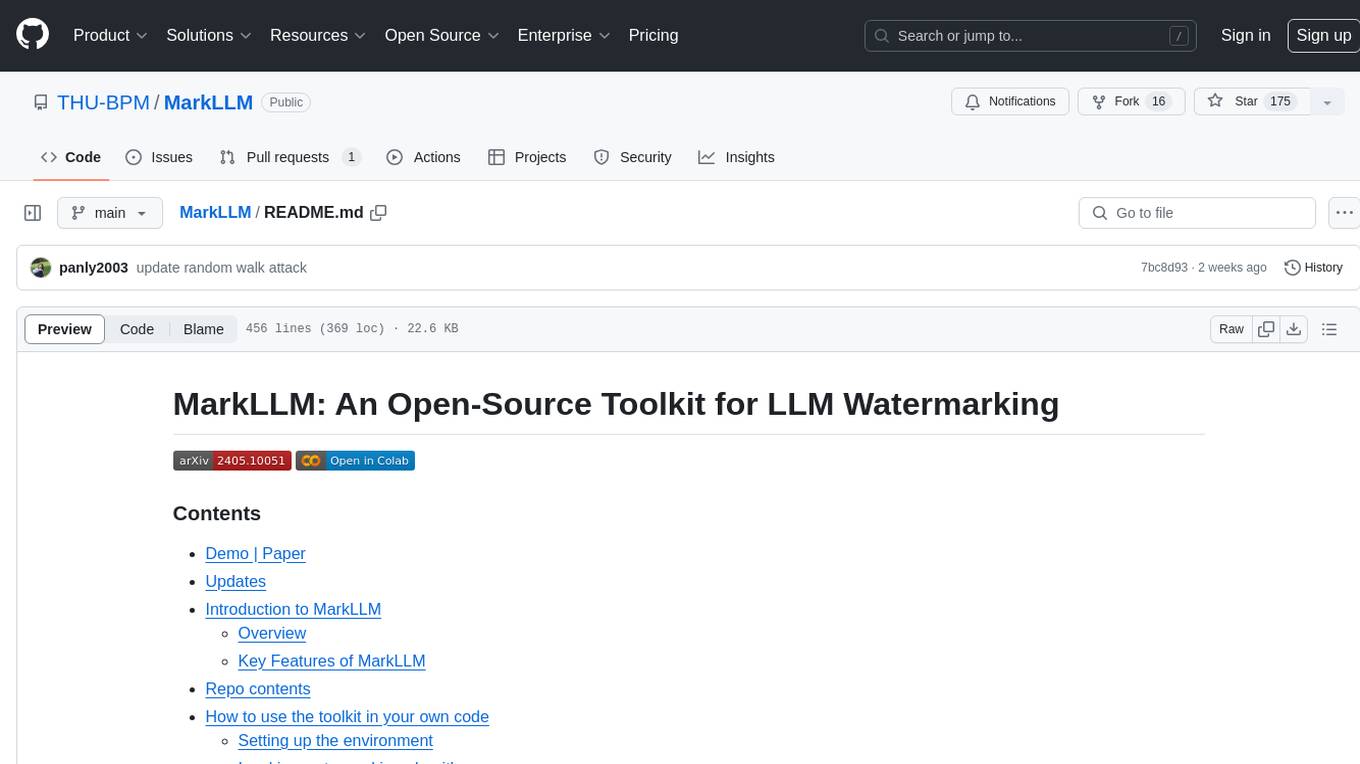
MarkLLM
MarkLLM is an open-source toolkit designed for watermarking technologies within large language models (LLMs). It simplifies access, understanding, and assessment of watermarking technologies, supporting various algorithms, visualization tools, and evaluation modules. The toolkit aids researchers and the community in ensuring the authenticity and origin of machine-generated text.
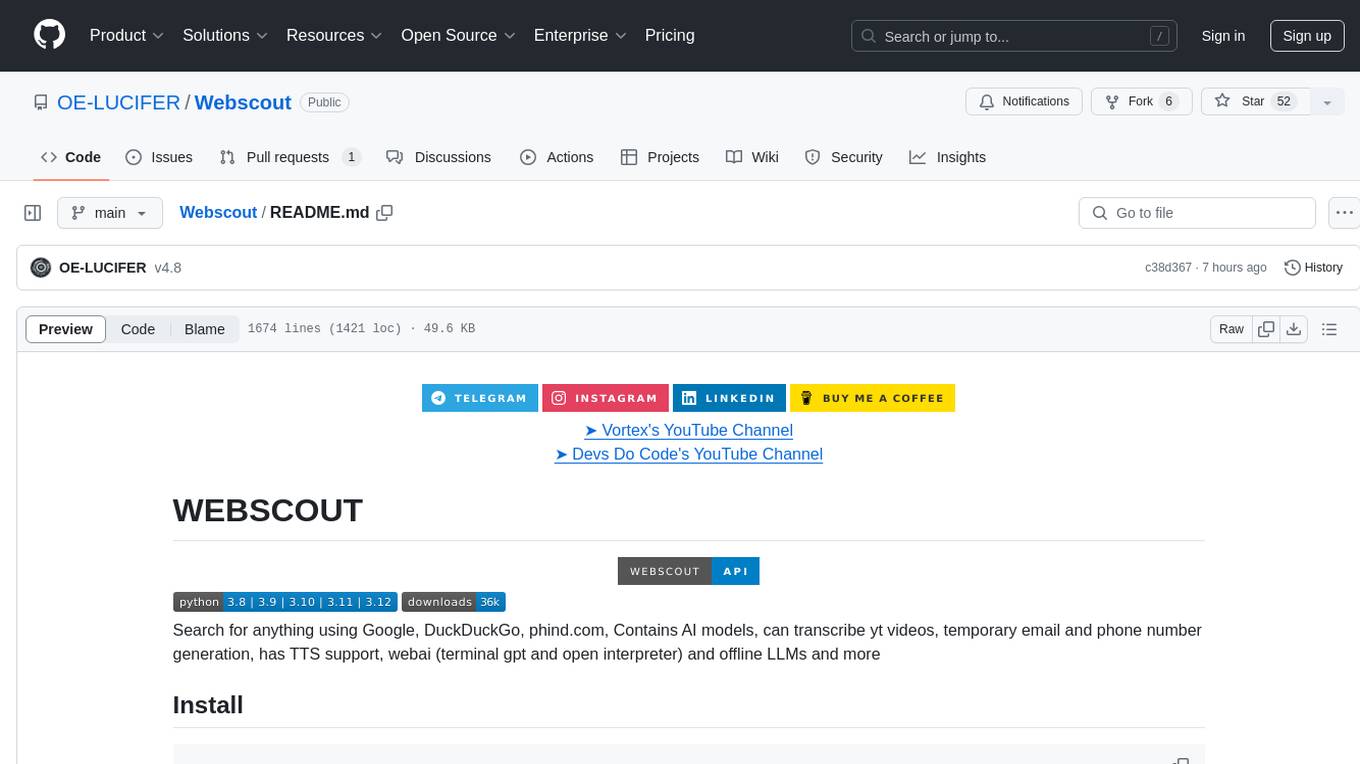
Webscout
WebScout is a versatile tool that allows users to search for anything using Google, DuckDuckGo, and phind.com. It contains AI models, can transcribe YouTube videos, generate temporary email and phone numbers, has TTS support, webai (terminal GPT and open interpreter), and offline LLMs. It also supports features like weather forecasting, YT video downloading, temp mail and number generation, text-to-speech, advanced web searches, and more.
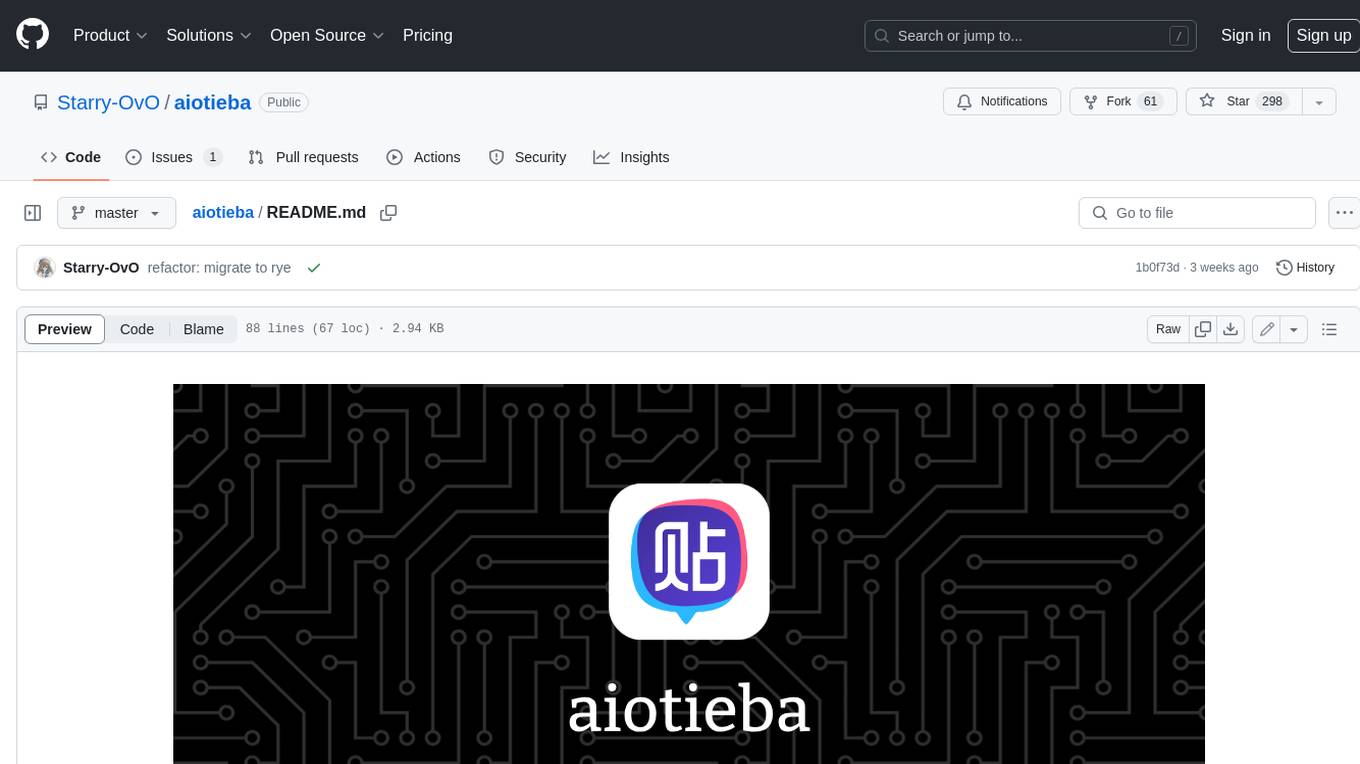
aiotieba
Aiotieba is an asynchronous Python library for interacting with the Tieba API. It provides a comprehensive set of features for working with Tieba, including support for authentication, thread and post management, and image and file uploading. Aiotieba is well-documented and easy to use, making it a great choice for developers who want to build applications that interact with Tieba.
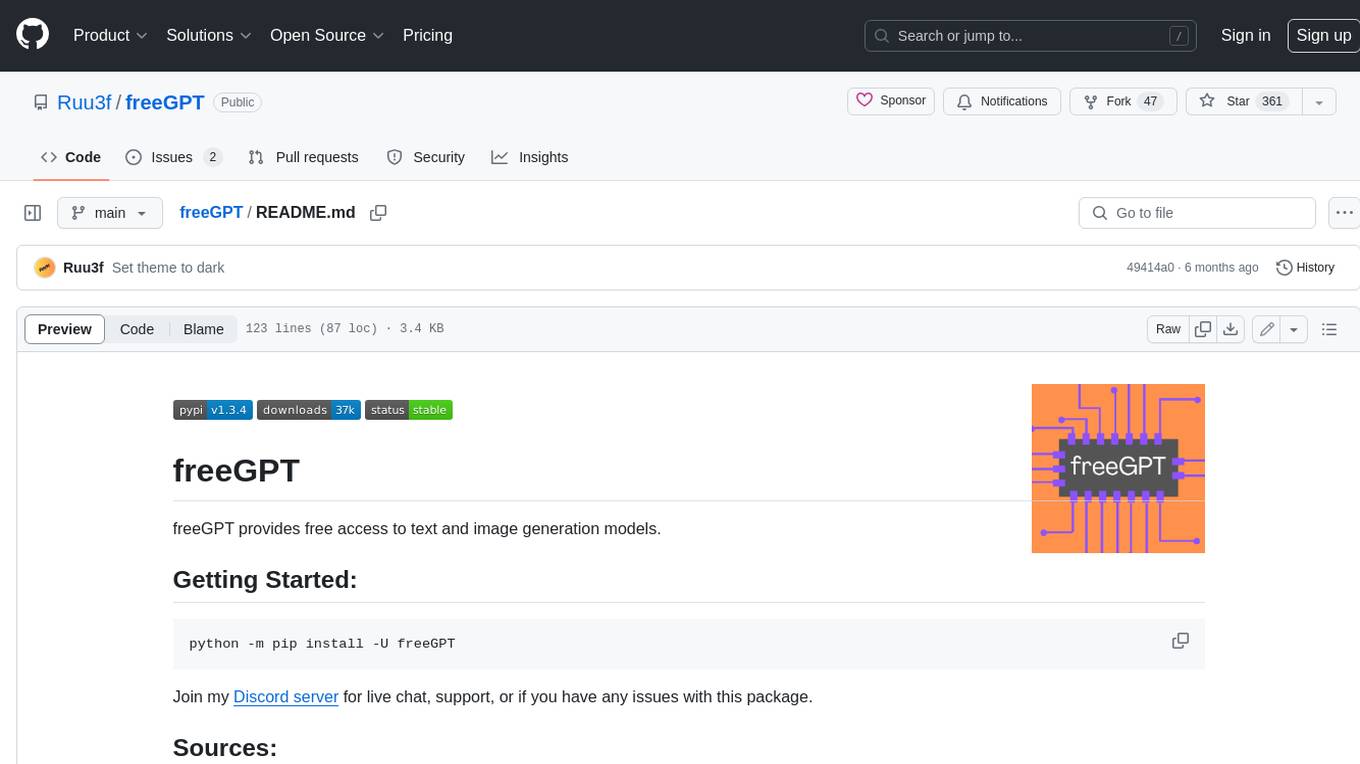
freeGPT
freeGPT provides free access to text and image generation models. It supports various models, including gpt3, gpt4, alpaca_7b, falcon_40b, prodia, and pollinations. The tool offers both asynchronous and non-asynchronous interfaces for text completion and image generation. It also features an interactive Discord bot that provides access to all the models in the repository. The tool is easy to use and can be integrated into various applications.
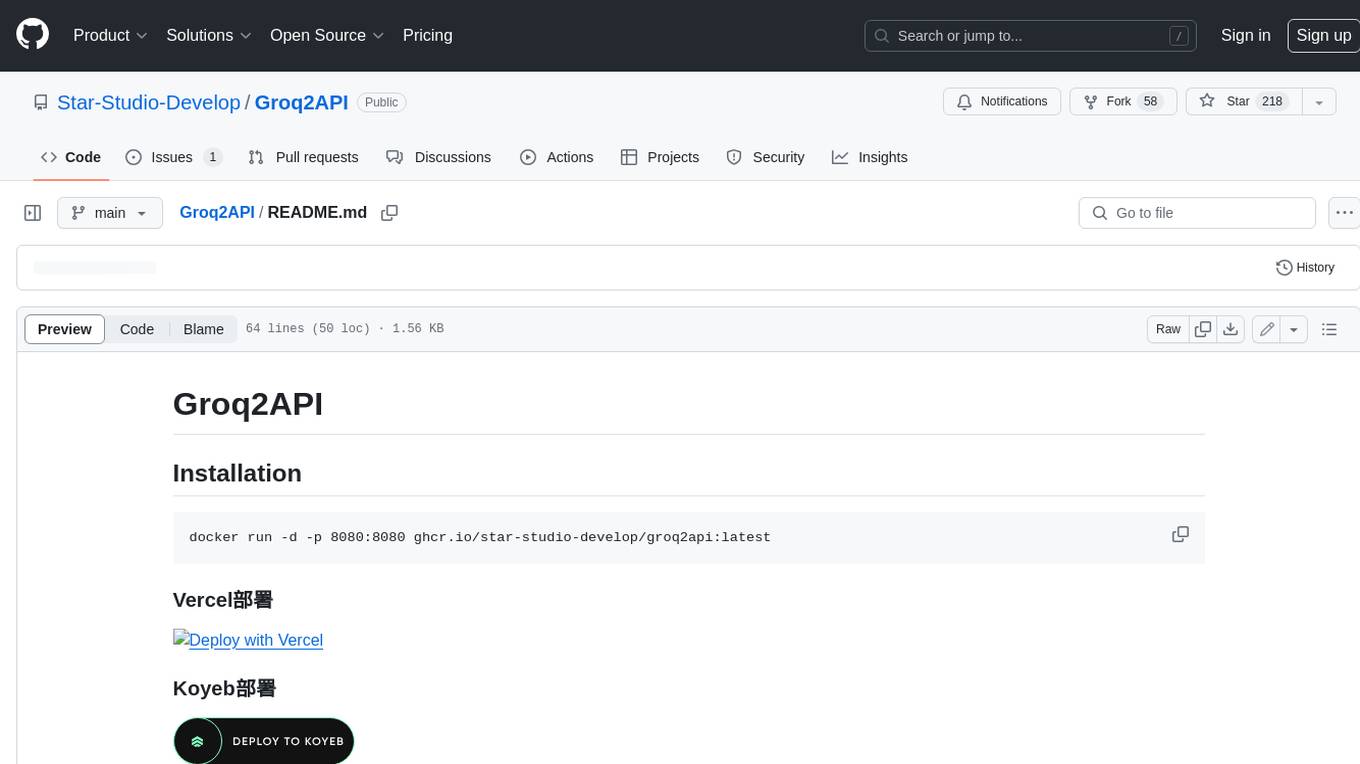
Groq2API
Groq2API is a REST API wrapper around the Groq2 model, a large language model trained by Google. The API allows you to send text prompts to the model and receive generated text responses. The API is easy to use and can be integrated into a variety of applications.
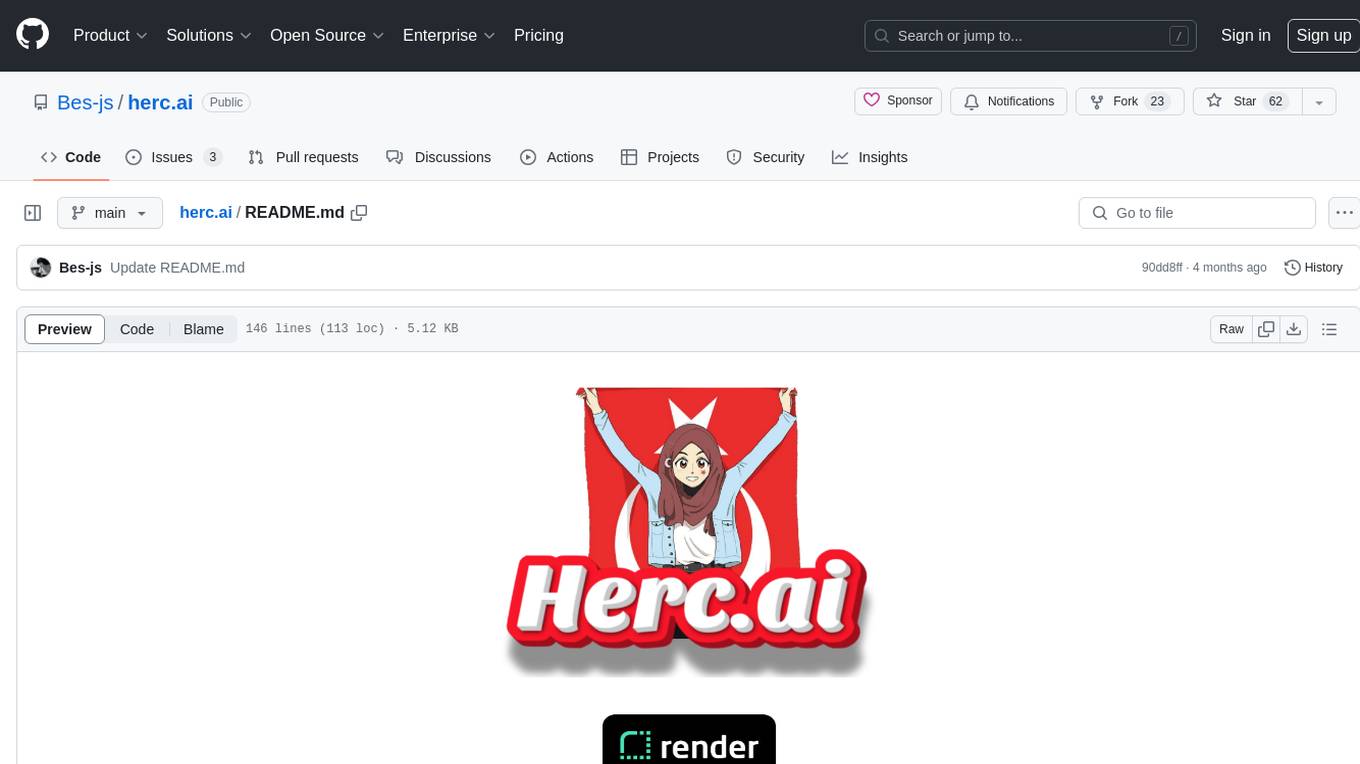
herc.ai
Herc.ai is a powerful library for interacting with the Herc.ai API. It offers free access to users and supports all languages. Users can benefit from Herc.ai's features unlimitedly with a one-time subscription and API key. The tool provides functionalities for question answering and text-to-image generation, with support for various models and customization options. Herc.ai can be easily integrated into CLI, CommonJS, TypeScript, and supports beta models for advanced usage. Developed by FiveSoBes and Luppux Development.
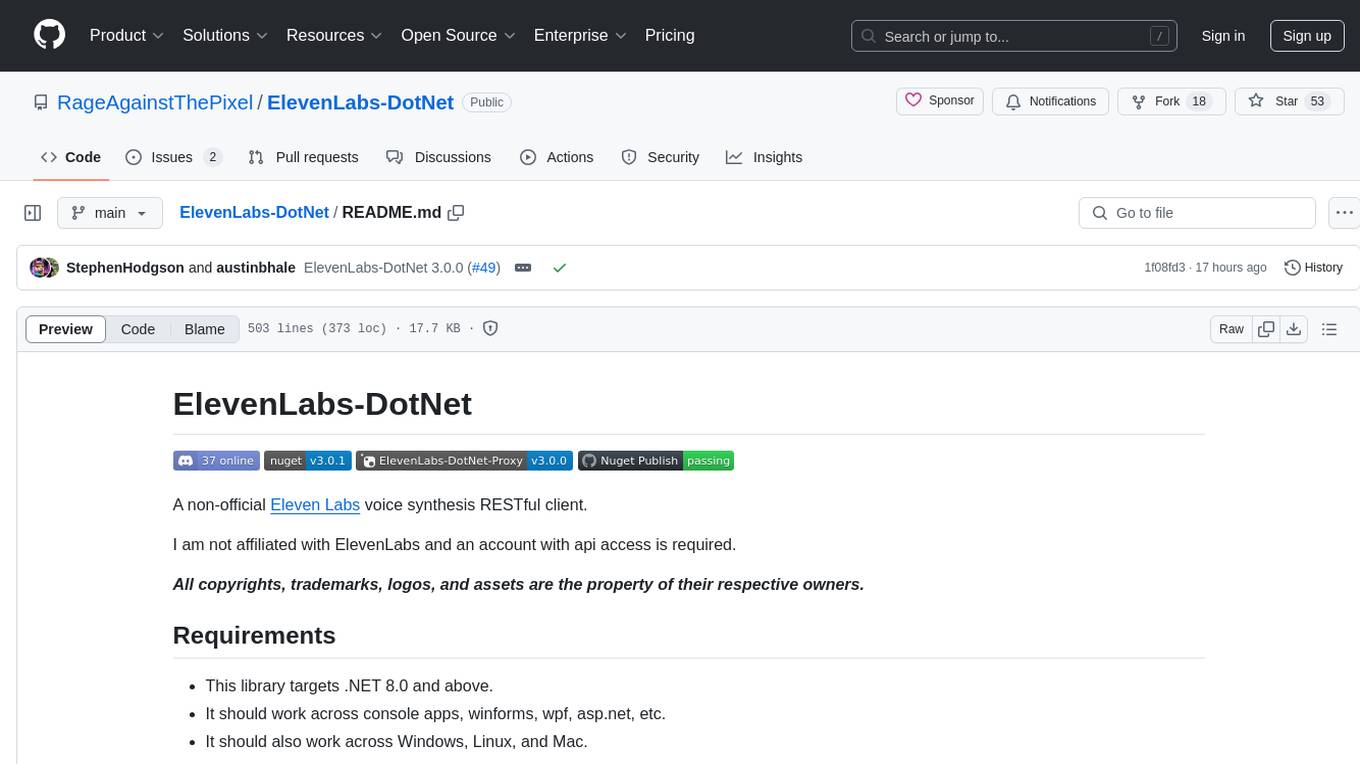
ElevenLabs-DotNet
ElevenLabs-DotNet is a non-official Eleven Labs voice synthesis RESTful client that allows users to convert text to speech. The library targets .NET 8.0 and above, working across various platforms like console apps, winforms, wpf, and asp.net, and across Windows, Linux, and Mac. Users can authenticate using API keys directly, from a configuration file, or system environment variables. The tool provides functionalities for text to speech conversion, streaming text to speech, accessing voices, dubbing audio or video files, generating sound effects, managing history of synthesized audio clips, and accessing user information and subscription status.
For similar tasks
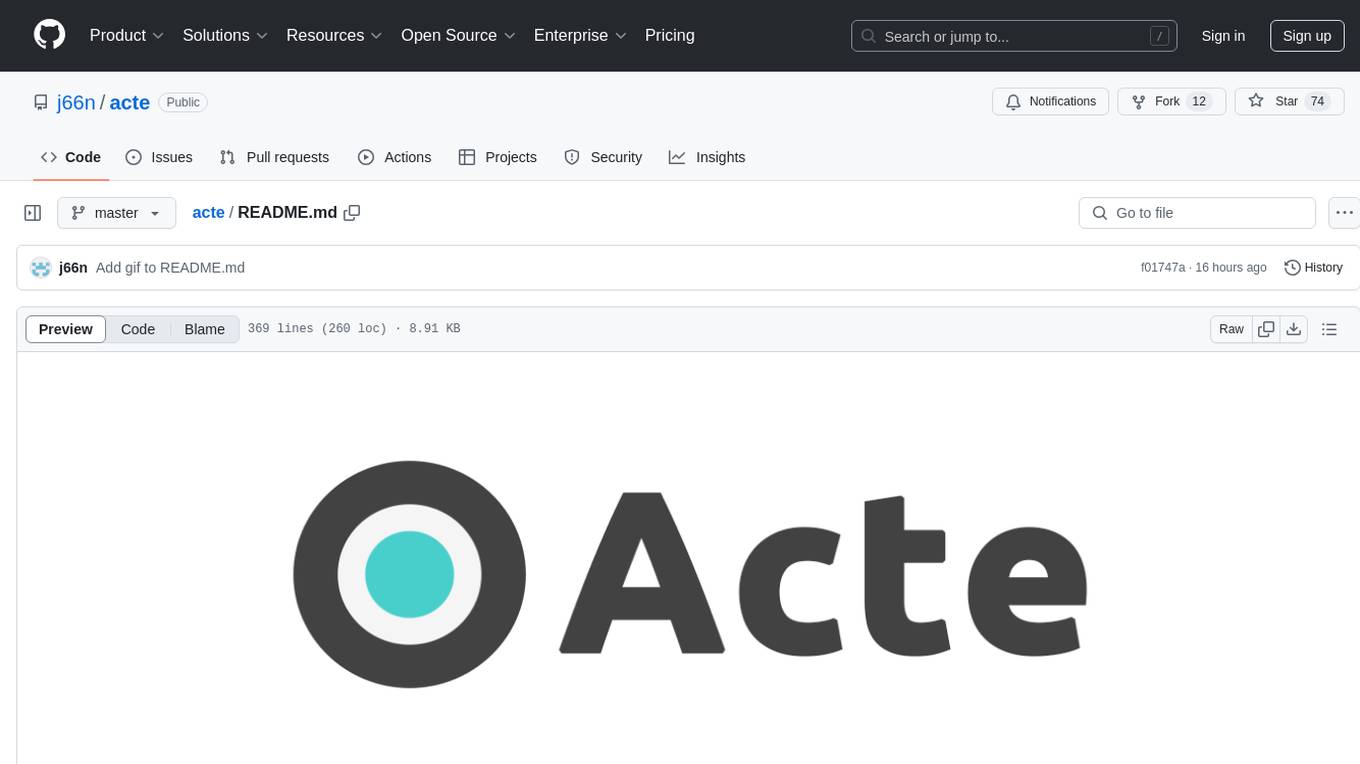
acte
Acte is a framework designed to build GUI-like tools for AI Agents. It aims to address the issues of cognitive load and freedom degrees when interacting with multiple APIs in complex scenarios. By providing a graphical user interface (GUI) for Agents, Acte helps reduce cognitive load and constraints interaction, similar to how humans interact with computers through GUIs. The tool offers APIs for starting new sessions, executing actions, and displaying screens, accessible via HTTP requests or the SessionManager class.
For similar jobs
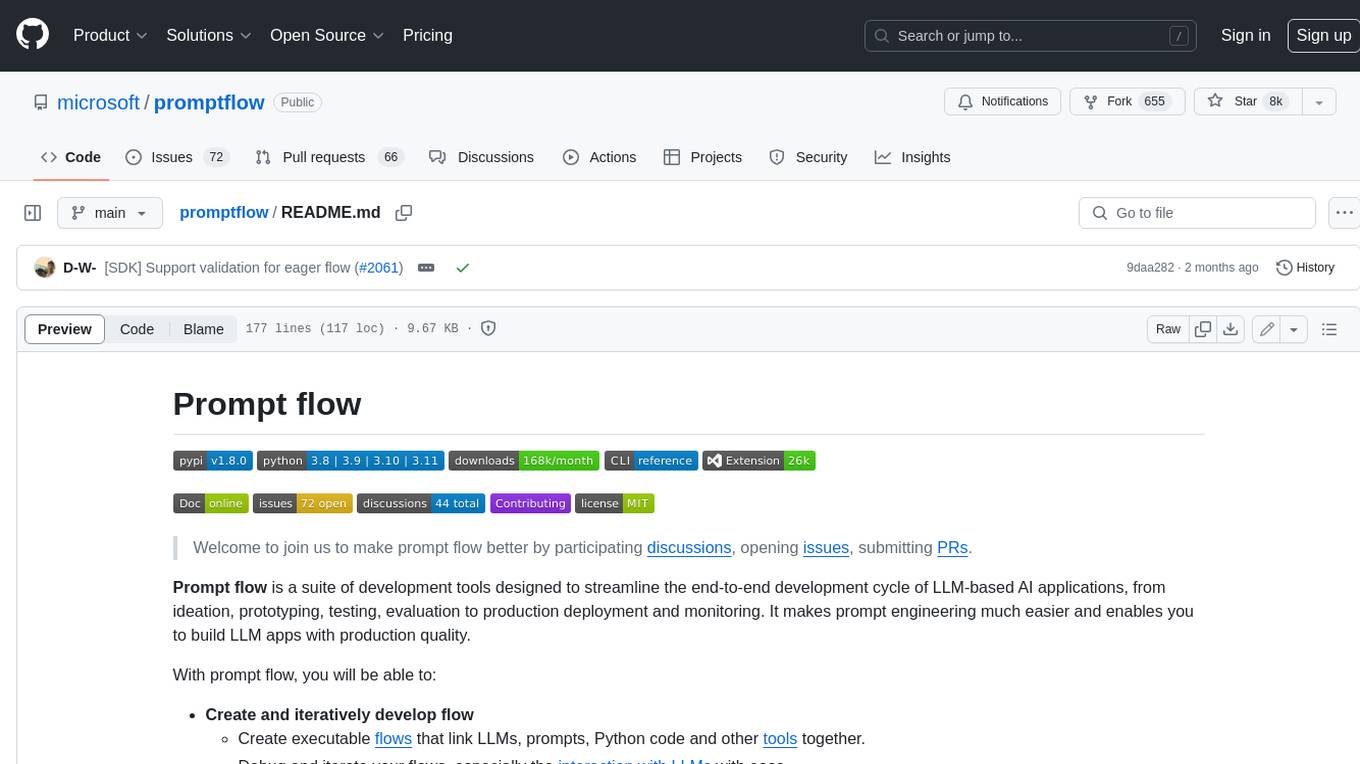
promptflow
**Prompt flow** is a suite of development tools designed to streamline the end-to-end development cycle of LLM-based AI applications, from ideation, prototyping, testing, evaluation to production deployment and monitoring. It makes prompt engineering much easier and enables you to build LLM apps with production quality.
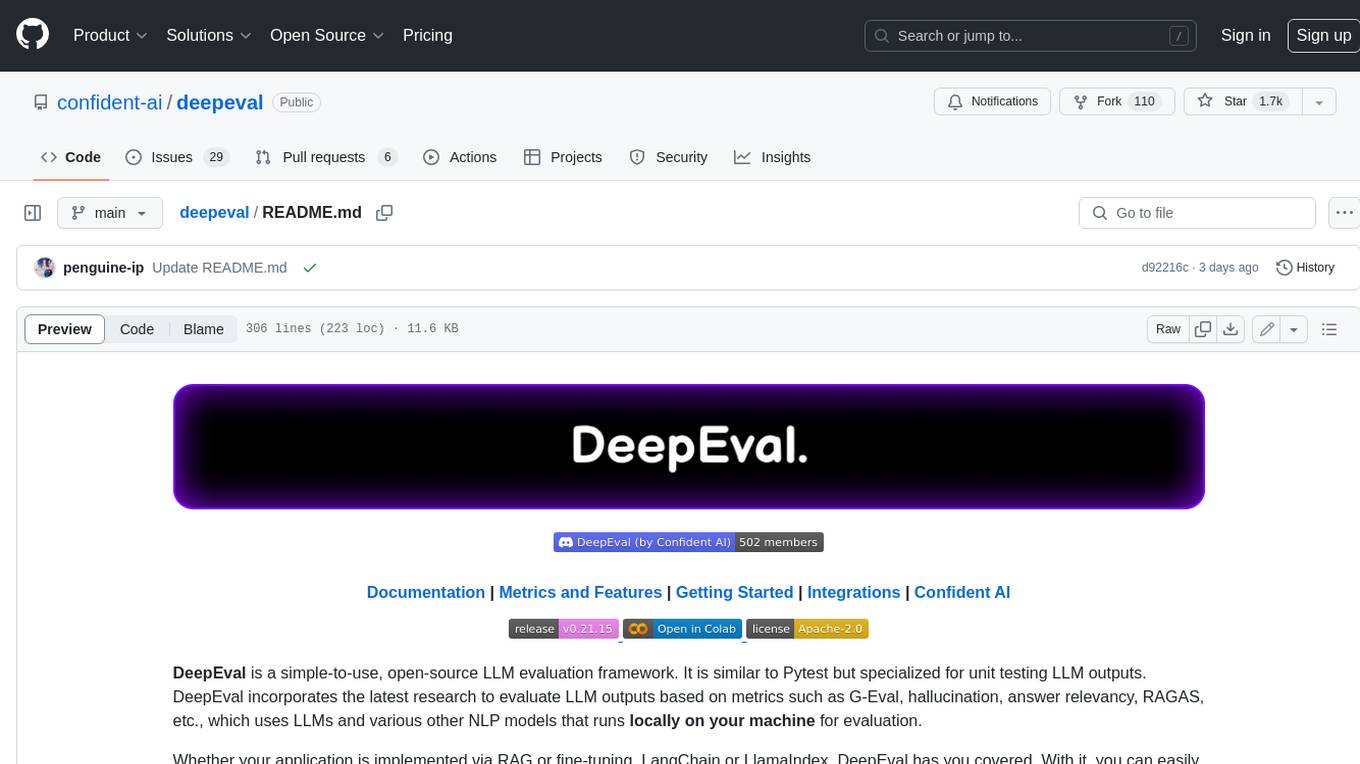
deepeval
DeepEval is a simple-to-use, open-source LLM evaluation framework specialized for unit testing LLM outputs. It incorporates various metrics such as G-Eval, hallucination, answer relevancy, RAGAS, etc., and runs locally on your machine for evaluation. It provides a wide range of ready-to-use evaluation metrics, allows for creating custom metrics, integrates with any CI/CD environment, and enables benchmarking LLMs on popular benchmarks. DeepEval is designed for evaluating RAG and fine-tuning applications, helping users optimize hyperparameters, prevent prompt drifting, and transition from OpenAI to hosting their own Llama2 with confidence.
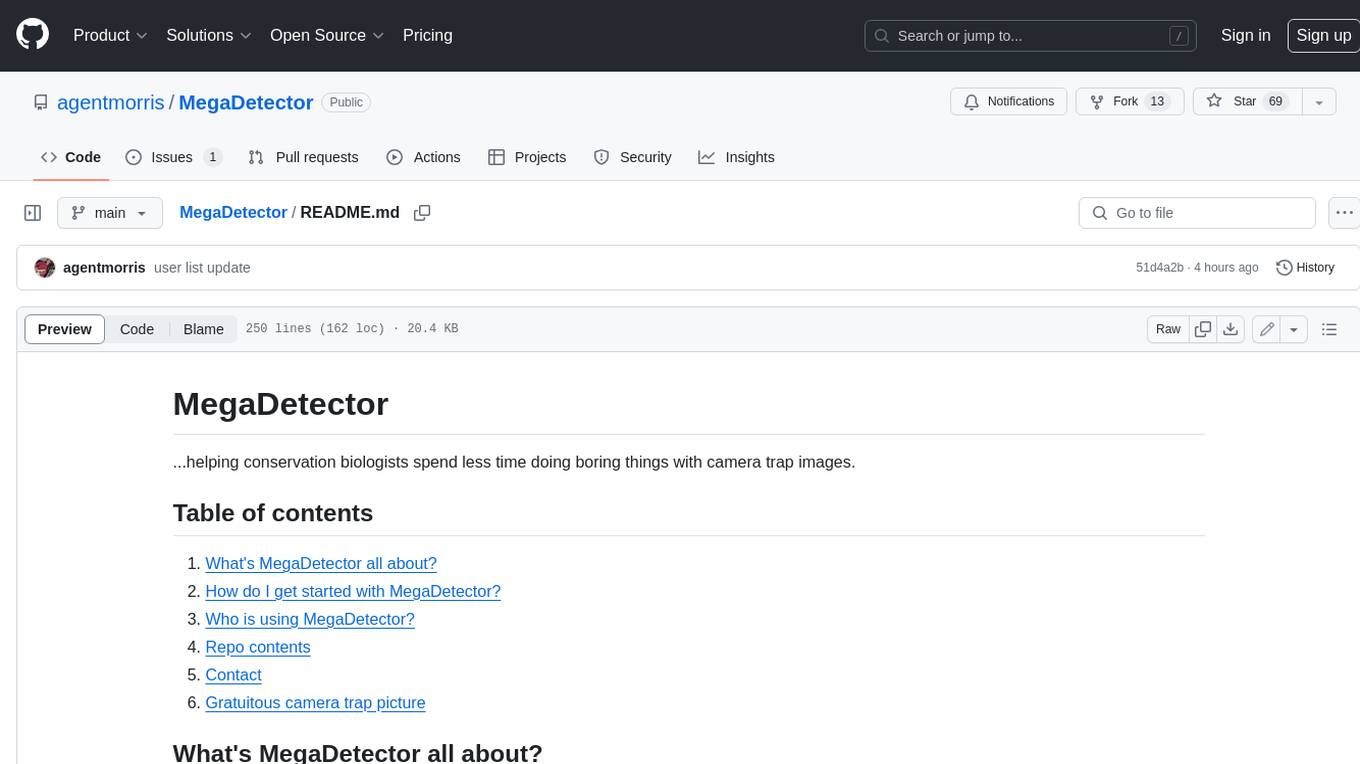
MegaDetector
MegaDetector is an AI model that identifies animals, people, and vehicles in camera trap images (which also makes it useful for eliminating blank images). This model is trained on several million images from a variety of ecosystems. MegaDetector is just one of many tools that aims to make conservation biologists more efficient with AI. If you want to learn about other ways to use AI to accelerate camera trap workflows, check out our of the field, affectionately titled "Everything I know about machine learning and camera traps".
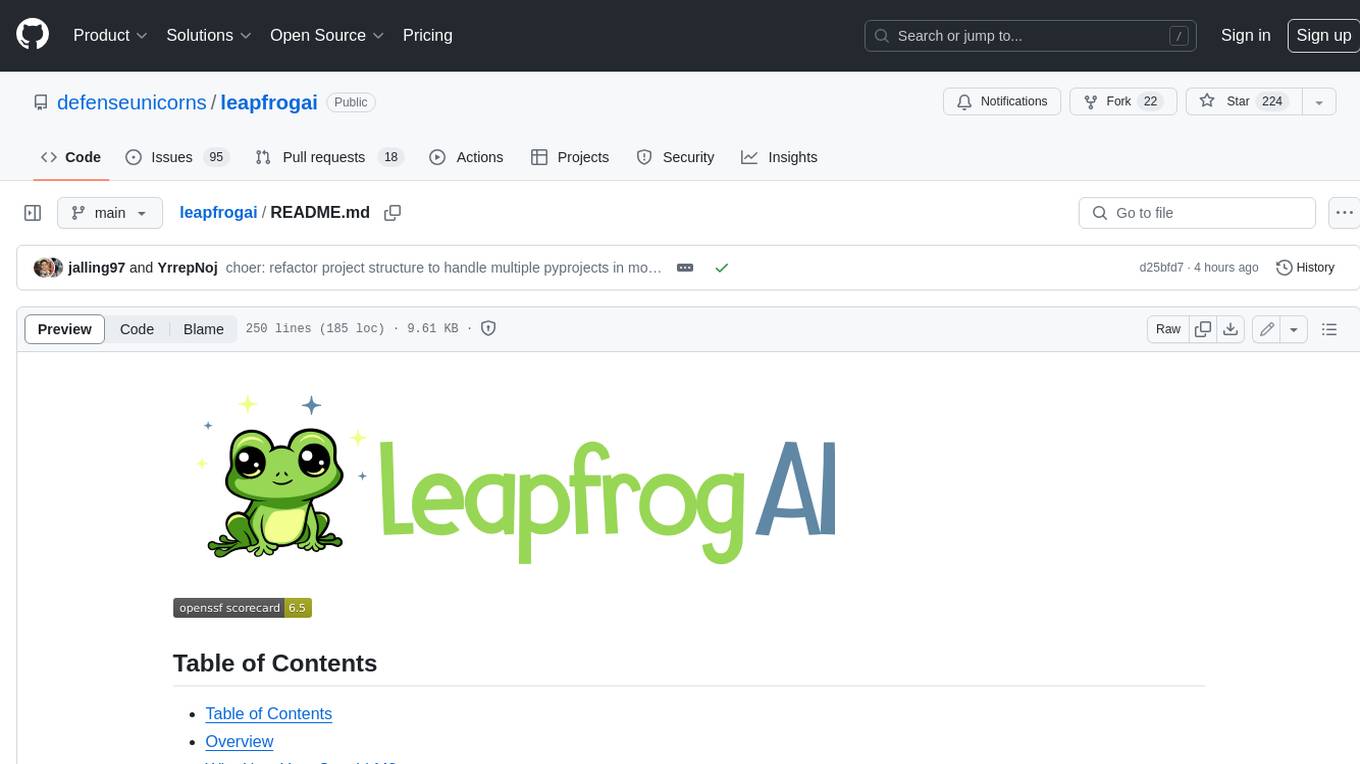
leapfrogai
LeapfrogAI is a self-hosted AI platform designed to be deployed in air-gapped resource-constrained environments. It brings sophisticated AI solutions to these environments by hosting all the necessary components of an AI stack, including vector databases, model backends, API, and UI. LeapfrogAI's API closely matches that of OpenAI, allowing tools built for OpenAI/ChatGPT to function seamlessly with a LeapfrogAI backend. It provides several backends for various use cases, including llama-cpp-python, whisper, text-embeddings, and vllm. LeapfrogAI leverages Chainguard's apko to harden base python images, ensuring the latest supported Python versions are used by the other components of the stack. The LeapfrogAI SDK provides a standard set of protobuffs and python utilities for implementing backends and gRPC. LeapfrogAI offers UI options for common use-cases like chat, summarization, and transcription. It can be deployed and run locally via UDS and Kubernetes, built out using Zarf packages. LeapfrogAI is supported by a community of users and contributors, including Defense Unicorns, Beast Code, Chainguard, Exovera, Hypergiant, Pulze, SOSi, United States Navy, United States Air Force, and United States Space Force.
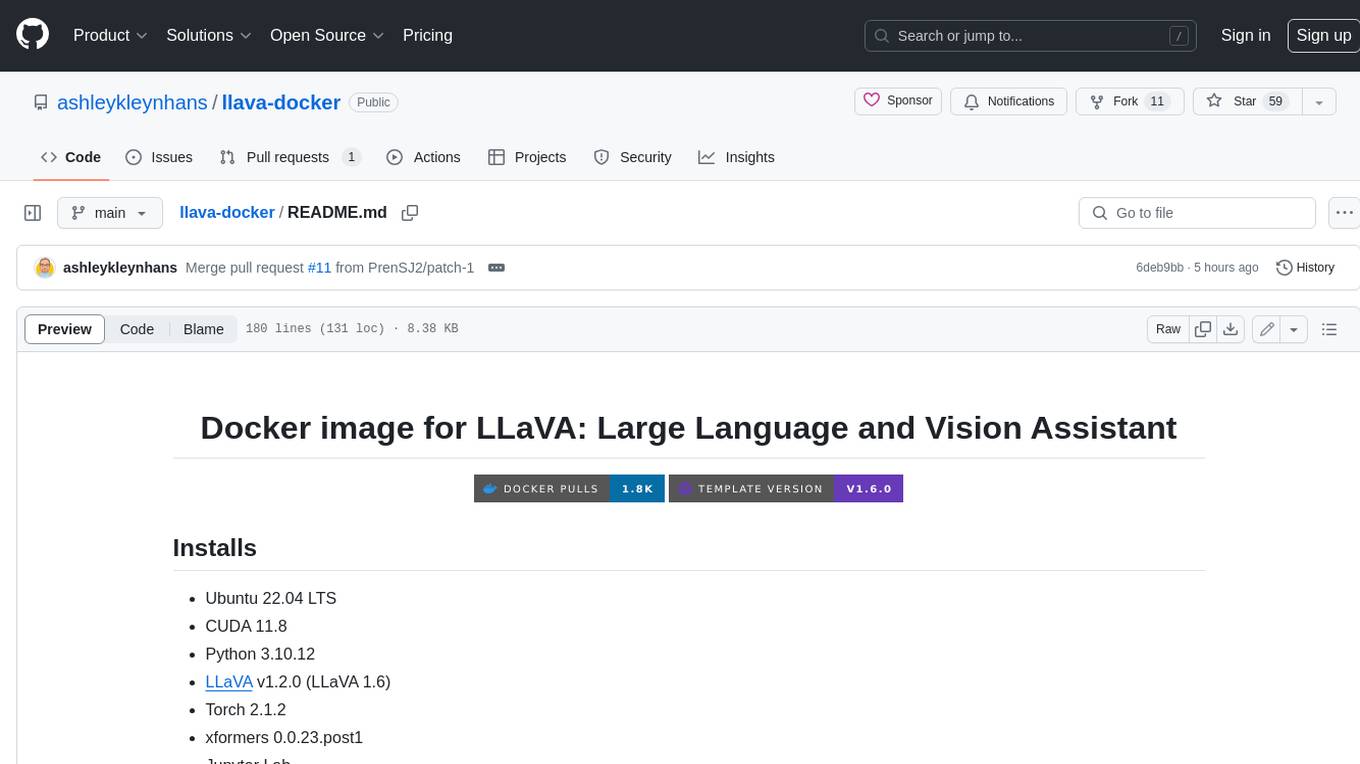
llava-docker
This Docker image for LLaVA (Large Language and Vision Assistant) provides a convenient way to run LLaVA locally or on RunPod. LLaVA is a powerful AI tool that combines natural language processing and computer vision capabilities. With this Docker image, you can easily access LLaVA's functionalities for various tasks, including image captioning, visual question answering, text summarization, and more. The image comes pre-installed with LLaVA v1.2.0, Torch 2.1.2, xformers 0.0.23.post1, and other necessary dependencies. You can customize the model used by setting the MODEL environment variable. The image also includes a Jupyter Lab environment for interactive development and exploration. Overall, this Docker image offers a comprehensive and user-friendly platform for leveraging LLaVA's capabilities.
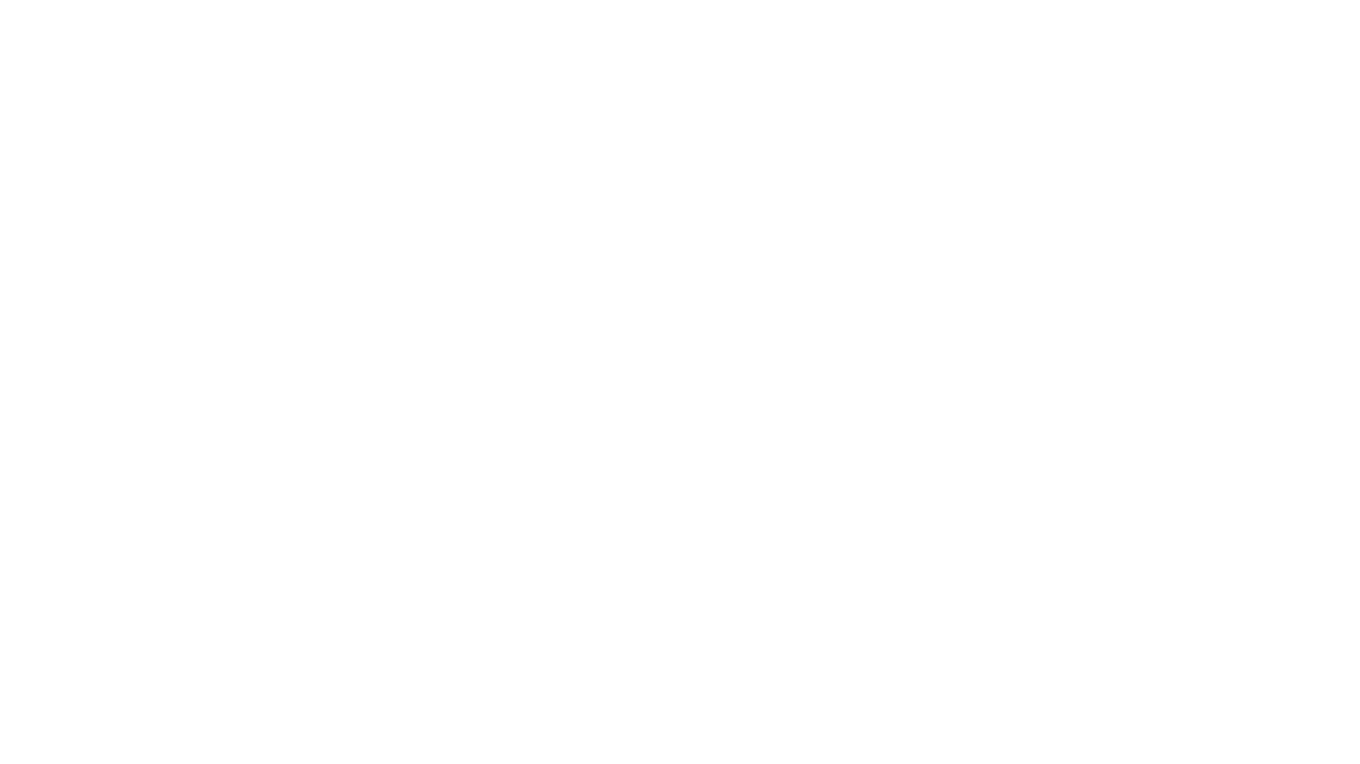
carrot
The 'carrot' repository on GitHub provides a list of free and user-friendly ChatGPT mirror sites for easy access. The repository includes sponsored sites offering various GPT models and services. Users can find and share sites, report errors, and access stable and recommended sites for ChatGPT usage. The repository also includes a detailed list of ChatGPT sites, their features, and accessibility options, making it a valuable resource for ChatGPT users seeking free and unlimited GPT services.
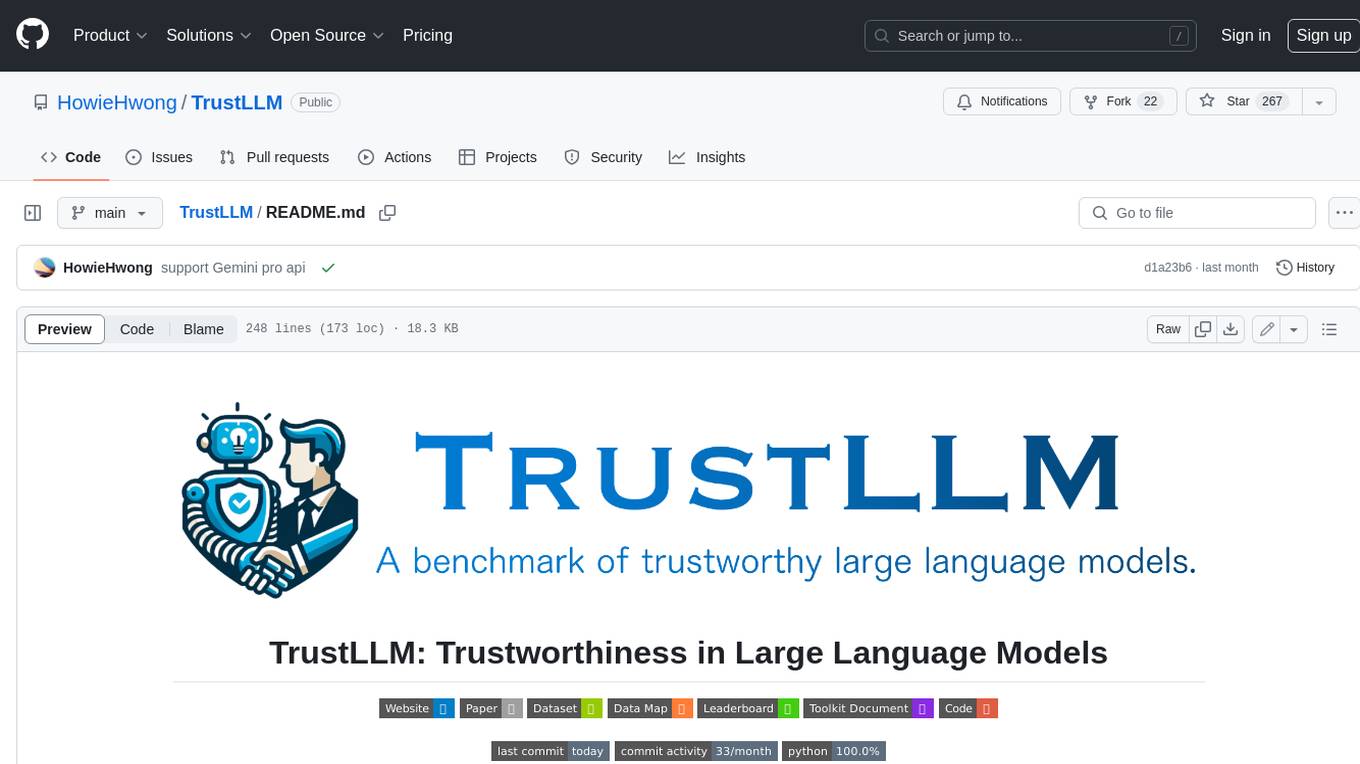
TrustLLM
TrustLLM is a comprehensive study of trustworthiness in LLMs, including principles for different dimensions of trustworthiness, established benchmark, evaluation, and analysis of trustworthiness for mainstream LLMs, and discussion of open challenges and future directions. Specifically, we first propose a set of principles for trustworthy LLMs that span eight different dimensions. Based on these principles, we further establish a benchmark across six dimensions including truthfulness, safety, fairness, robustness, privacy, and machine ethics. We then present a study evaluating 16 mainstream LLMs in TrustLLM, consisting of over 30 datasets. The document explains how to use the trustllm python package to help you assess the performance of your LLM in trustworthiness more quickly. For more details about TrustLLM, please refer to project website.
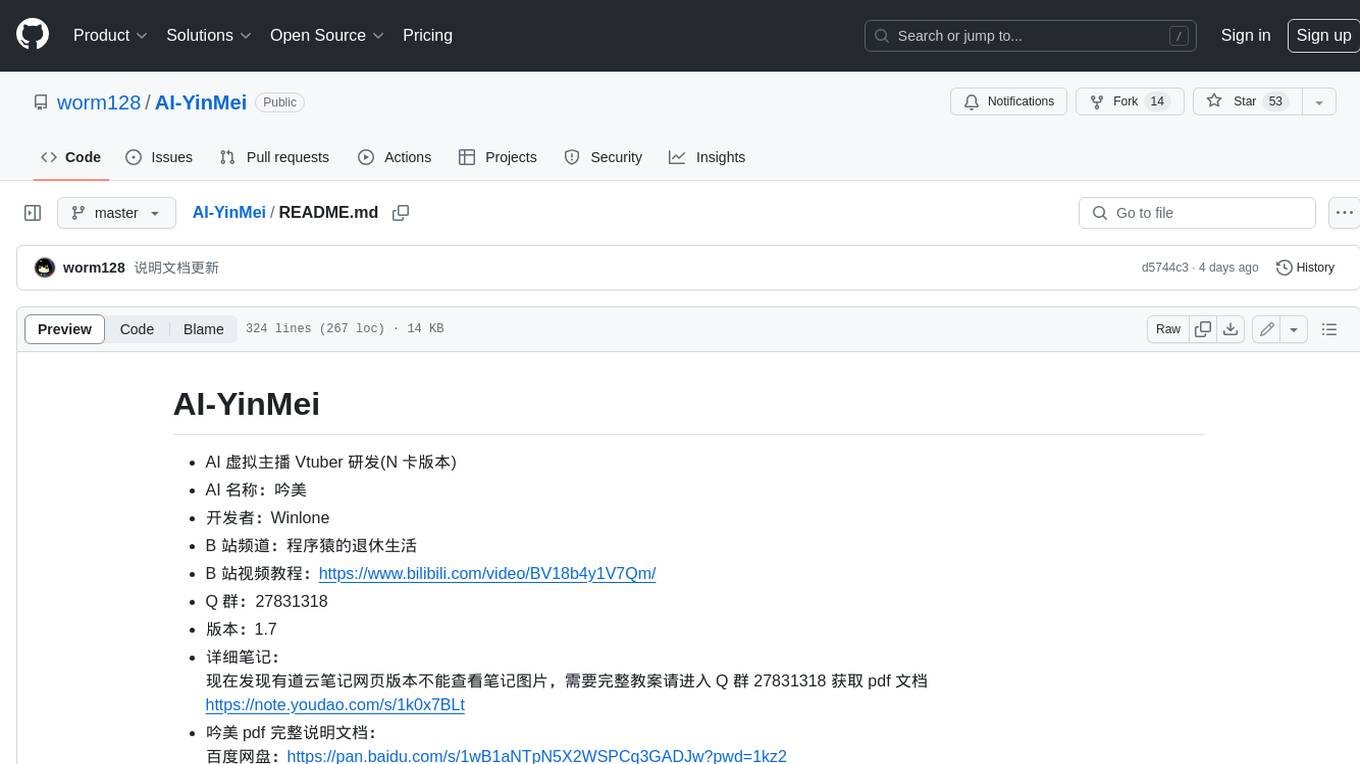
AI-YinMei
AI-YinMei is an AI virtual anchor Vtuber development tool (N card version). It supports fastgpt knowledge base chat dialogue, a complete set of solutions for LLM large language models: [fastgpt] + [one-api] + [Xinference], supports docking bilibili live broadcast barrage reply and entering live broadcast welcome speech, supports Microsoft edge-tts speech synthesis, supports Bert-VITS2 speech synthesis, supports GPT-SoVITS speech synthesis, supports expression control Vtuber Studio, supports painting stable-diffusion-webui output OBS live broadcast room, supports painting picture pornography public-NSFW-y-distinguish, supports search and image search service duckduckgo (requires magic Internet access), supports image search service Baidu image search (no magic Internet access), supports AI reply chat box [html plug-in], supports AI singing Auto-Convert-Music, supports playlist [html plug-in], supports dancing function, supports expression video playback, supports head touching action, supports gift smashing action, supports singing automatic start dancing function, chat and singing automatic cycle swing action, supports multi scene switching, background music switching, day and night automatic switching scene, supports open singing and painting, let AI automatically judge the content.