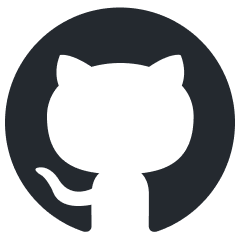
amadeus-node
Node library for the Amadeus Self-Service travel APIs
Stars: 186
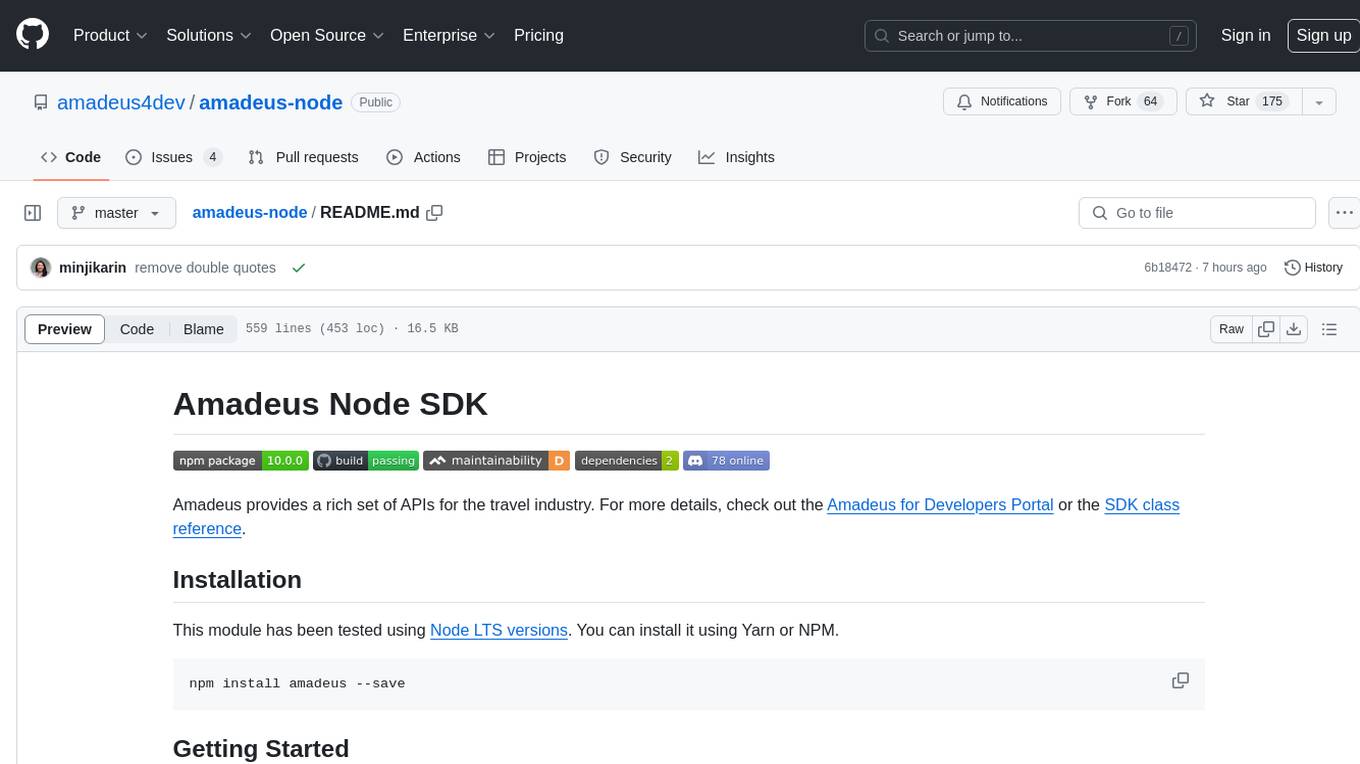
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
README:
Amadeus provides a rich set of APIs for the travel industry. For more details, check out the Amadeus for Developers Portal or the SDK class reference.
This module has been tested using Node LTS versions. You can install it using Yarn or NPM.
npm install amadeus --save
To make your first API call, you will need to register for an Amadeus Developer Account and set up your first application.
var Amadeus = require('amadeus');
var amadeus = new Amadeus({
clientId: 'REPLACE_BY_YOUR_API_KEY',
clientSecret: 'REPLACE_BY_YOUR_API_SECRET'
});
amadeus.shopping.flightOffersSearch.get({
originLocationCode: 'SYD',
destinationLocationCode: 'BKK',
departureDate: '2022-06-01',
adults: '2'
}).then(function(response){
console.log(response.data);
}).catch(function(responseError){
console.log(responseError.code);
});
You can find all the endpoints in self-contained code examples.
The client can be initialized directly.
// Initialize using parameters
var amadeus = new Amadeus({
clientId: 'REPLACE_BY_YOUR_API_KEY',
clientSecret: 'REPLACE_BY_YOUR_API_SECRET'
});
Alternatively, it can be initialized without any parameters if the environment variables AMADEUS_CLIENT_ID
and AMADEUS_CLIENT_SECRET
are present.
var amadeus = new Amadeus();
Your credentials can be found on the Amadeus dashboard.
By default, the SDK environment is set to test
environment. To switch to a production
(pay-as-you-go) environment, please switch the hostname as follows:
var amadeus = new Amadeus({
hostname: 'production'
});
Amadeus has a large set of APIs, and our documentation is here to get you started today. Head over to our reference documentation for in-depth information about every SDK method, its arguments and return types.
This library conveniently maps every API path to a similar path. For example, GET /v2/reference-data/urls/checkin-links?airlineCode=BA
would be:
amadeus.referenceData.urls.checkinLinks.get({ airlineCode: 'BA' });
Similarly, to select a resource by ID, you can pass in the ID to the singular path. For example, GET /v1/shopping/hotelOffers/123/
would be:
amadeus.shopping.hotelOffer('123').get(...);
You can make any arbitrary GET
API call directly with the .client.get
method as well:
amadeus.client.get('/v2/reference-data/urls/checkin-links', { airlineCode: 'BA' });
Or, with a POST
using .client.post
method:
amadeus.client.post('/v1/shopping/flight-offers/pricing', JSON.stringify({ data }));
Every API call returns a Promise
that either resolves or rejects.
Every resolved API call returns a Response
object containing a body
attribute with the raw response. If the API call contained a JSON response, it will parse the JSON into the result
attribute. If this data contains a data
key, that will be made available in data
attribute.
For a failed API call, it returns a ResponseError
object containing the (parsed or unparsed) response, the request, and an error code.
amadeus.referenceData.urls.checkinLinks.get({
airlineCode: 'BA'
}).then(function(response){
console.log(response.body); //=> The raw body
console.log(response.result); //=> The fully parsed result
console.log(response.data); //=> The data attribute taken from the result
}).catch(function(error){
console.log(error.response); //=> The response object with (un)parsed data
console.log(error.response.request); //=> The details of the request made
console.log(error.code); //=> A unique error code to identify the type of error
});
If an API endpoint supports pagination, the other pages are available under the .next
, .previous
, .last
and .first
methods.
amadeus.referenceData.locations.get({
keyword: 'LON',
subType: 'AIRPORT,CITY'
}).then(function(response){
console.log(response.data); // first page
return amadeus.next(response);
}).then(function(nextResponse){
console.log(nextResponse.data); // second page
});
If a page is not available, the response will resolve to null
.
The SDK makes it easy to add your own logger that is compatible with the default console
.
var amadeus = new Amadeus({
clientId: 'REPLACE_BY_YOUR_API_KEY',
clientSecret: 'REPLACE_BY_YOUR_API_SECRET',
logger: new MyConsole()
});
Additionally, to enable more verbose logging, you can set the appropriate level on your own logger. The easiest way would be to enable debugging via a parameter during initialization, or using the AMADEUS_LOG_LEVEL
environment variable. The available options are silent
(default), warn
, and debug
.
var amadeus = new Amadeus({
clientId: 'REPLACE_BY_YOUR_API_KEY',
clientSecret: 'REPLACE_BY_YOUR_API_SECRET',
logLevel: 'debug'
});
//Airport Routes
amadeus.airport.directDestinations.get({
departureAirportCode: 'CDG',
})
//Airline Routes
//find all destinations served by a given airline
amadeus.airline.destinations.get({
airlineCode: 'BA',
})
// Flight Inspiration Search
amadeus.shopping.flightDestinations.get({
origin : 'MAD'
})
// Flight Cheapest Date Search
amadeus.shopping.flightDates.get({
origin : 'MAD',
destination : 'MUC'
})
// Flight Offers Search GET
amadeus.shopping.flightOffersSearch.get({
originLocationCode: 'SYD',
destinationLocationCode: 'BKK',
departureDate: '2022-11-01',
adults: '2'
})
// Flight Offers Search POST
// A full example can be found at https://github.com/amadeus4dev/amadeus-code-examples
amadeus.shopping.flightOffersSearch.post(JSON.stringify(body))
// Flight Offers Price
amadeus.shopping.flightOffersSearch.get({
originLocationCode: 'SYD',
destinationLocationCode: 'BKK',
departureDate: '2022-11-01',
adults: '1'
}).then(function(response){
return amadeus.shopping.flightOffers.pricing.post(
JSON.stringify({
'data': {
'type': 'flight-offers-pricing',
'flightOffers': [response.data[0]]
}
})
)
}).then(function(response){
console.log(response.data);
}).catch(function(responseError){
console.log(responseError);
});
// Flight Offers Price with additional parameters
// for example: check additional baggage options
amadeus.shopping.flightOffers.pricing.post(JSON.stringify(body),{include: 'bags'})
// Flight Create Orders
// To book the flight-offer(s) returned by the Flight Offers Price
// and create a flight-order with travelers' information.
// A full example can be found at https://git.io/JtnYo
amadeus.booking.flightOrders.post(
JSON.stringify({
'type': 'flight-order',
'flightOffers': [priced-offers],
'travelers': []
})
)
// Retrieve flight order with ID 'XXX'. This ID comes from the
// Flight Create Orders API, which is a temporary ID in test environment.
amadeus.booking.flightOrder('XXX').get()
// Cancel flight order with ID 'XXX'. This ID comes from the
// Flight Create Orders API, which is a temporary ID in test environment.
amadeus.booking.flightOrder('XXX').delete()
// Flight SeatMap Display
// To retrieve the seat map of each flight included
// in flight offers for MAD-NYC flight on 2021-08-01
amadeus.shopping.flightOffersSearch.get({
originLocationCode: 'SYD',
destinationLocationCode: 'BKK',
departureDate: '2022-11-01',
adults: '1'
}).then(function(response){
return amadeus.shopping.seatmaps.post(
JSON.stringify({
'data': [response.data[0]]
})
);
}).then(function(response){
console.log(response.data);
}).catch(function(responseError){
console.log(responseError);
});
// To retrieve the seat map for flight order with ID 'XXX'
amadeus.shopping.seatmaps.get({
'flight-orderId': 'XXX'
});
// Flight Availabilities Search
amadeus.shopping.availability.flightAvailabilities.post(JSON.stringify((body));
// Branded Fares Upsell
amadeus.shopping.flightOffers.upselling.post(JSON.stringify(body));
// Flight Choice Prediction
amadeus.shopping.flightOffersSearch.get({
originLocationCode: 'SYD',
destinationLocationCode: 'BKK',
departureDate: '2022-11-01',
adults: '2'
}).then(function(response){
return amadeus.shopping.flightOffers.prediction.post(
JSON.stringify(response)
);
}).then(function(response){
console.log(response.data);
}).catch(function(responseError){
console.log(responseError);
});
// Flight Checkin Links
amadeus.referenceData.urls.checkinLinks.get({
airlineCode : 'BA'
})
// Airline Code Lookup
amadeus.referenceData.airlines.get({
airlineCodes : 'U2'
})
// Airports and City Search (autocomplete)
// Find all the cities and airports starting by 'LON'
amadeus.referenceData.locations.get({
keyword : 'LON',
subType : Amadeus.location.any
})
// Get a specific city or airport based on its id
amadeus.referenceData.location('ALHR').get()
// Airport Nearest Relevant Airport (for London)
amadeus.referenceData.locations.airports.get({
longitude : 0.1278,
latitude : 51.5074
})
// Flight Most Booked Destinations
amadeus.travel.analytics.airTraffic.booked.get({
originCityCode : 'MAD',
period : '2017-08'
}
// Flight Most Traveled Destinations
amadeus.travel.analytics.airTraffic.traveled.get({
originCityCode : 'MAD',
period : '2017-01'
})
// Flight Busiest Traveling Period
amadeus.travel.analytics.airTraffic.busiestPeriod.get({
cityCode: 'MAD',
period: '2017',
direction: Amadeus.direction.arriving
})
// City Search API
// finds cities that match a specific word or string of letters.
// Return a list of cities matching a keyword 'Paris'
amadeus.referenceData.locations.cities.get({
keyword: 'Paris'
})
//Hotel Name Autocomplete API
//Autocomplete a hotel search field
amadeus.referenceData.locations.hotel.get({
keyword: 'PARI',
subType: 'HOTEL_GDS'
})
//Hotel List API
//Get list of hotels by city code
amadeus.referenceData.locations.hotels.byCity.get({
cityCode: 'PAR'
})
//Get List of hotels by Geocode
amadeus.referenceData.locations.hotels.byGeocode.get({
latitude: 48.83152,
longitude: 2.24691
})
//Get List of hotels by hotelIds
amadeus.referenceData.locations.hotels.byHotels.get({
hotelIds: 'ACPAR245'
})
// Hotel Search API V3
// Get list of available offers in specific hotels by hotel ids
amadeus.shopping.hotelOffersSearch.get({
hotelIds: 'RTPAR001',
adults: '2'
})
// Check offer conditions of a specific offer id
amadeus.shopping.hotelOfferSearch('XXX').get()
// Hotel Booking API v2
amadeus.booking.hotelOrders.post(
JSON.stringfy({
'data': {
'type': 'hotel-order',
'guests': [],
'travelAgent': {},
'roomAssociations': [],
'payment': {}
}})
)
// Hotel Booking API v1
amadeus.booking.hotelBookings.post(
JSON.stringify({
'data': {
'offerId': 'XXXX',
'guests': [],
'payments': [],
'rooms': []
}})
)
// On-Demand Flight Status
// What's the current status of my flight?
amadeus.schedule.flights.get({
carrierCode: 'AZ',
flightNumber: '319',
scheduledDepartureDate: '2021-03-13'
})
// Points of Interest
// What are the popular places in Barcelona (based a geo location and a radius)
amadeus.referenceData.locations.pointsOfInterest.get({
latitude : 41.397158,
longitude : 2.160873
})
// What are the popular places in Barcelona? (based on a square)
amadeus.referenceData.locations.pointsOfInterest.bySquare.get({
north: 41.397158,
west: 2.160873,
south: 41.394582,
east: 2.177181
})
// Extract the information about point of interest with ID '9CB40CB5D0'
amadeus.referenceData.locations.pointOfInterest('9CB40CB5D0').get()
// Location Score
amadeus.location.analytics.categoryRatedAreas.get({
latitude : 41.397158,
longitude : 2.160873
})
// Tours and Activities
// What are the best tours and activities in Barcelona?
amadeus.shopping.activities.get({
latitude: 41.397158,
longitude: 2.160873
})
// What are the best tours and activities in Barcelona? (based on a Square)
amadeus.shopping.activities.bySquare.get({
north: 41.397158,
west: 2.160873,
south: 41.394582,
east: 2.177181
})
// Extract the information about an activity with ID '56777'
amadeus.shopping.activity('56777').get()
// Hotel Ratings
// Get Sentiment Analysis of reviews about Holiday Inn Paris Notre Dame.
amadeus.eReputation.hotelSentiments.get({
hotelIds: 'XKPARC12'
})
// Trip Purpose Prediction
// Forecast traveler purpose, Business or Leisure, together with the probability in the context of search & shopping.
amadeus.travel.predictions.tripPurpose.get({
originLocationCode: 'NYC',
destinationLocationCode: 'MAD',
departureDate: '2021-04-01',
returnDate: '2021-04-08'
})
// Flight Delay Prediction
// This machine learning API is based on a prediction model that takes the input of the user - time, carrier, airport and aircraft information;
// and predict the segment where the flight is likely to lay.
amadeus.travel.predictions.flightDelay.get({
originLocationCode: 'BRU',
destinationLocationCode: 'FRA',
departureDate: '2020-01-14',
departureTime: '11:05:00',
arrivalDate: '2020-01-14',
arrivalTime: '12:10:00',
aircraftCode: '32A',
carrierCode: 'LH',
flightNumber: '1009',
duration: 'PT1H05M'
})
// Airport On-time Performance
// Get the percentage of on-time flight departures from JFK
amadeus.airport.predictions.onTime.get({
airportCode: 'JFK',
date: '2022-18-01'
})
// Travel Recommendations
amadeus.referenceData.recommendedLocations.get({
cityCodes: 'PAR',
travelerCountryCode: 'FR'
})
// Flight Price Analysis
amadeus.analytics.itineraryPriceMetrics.get({
originIataCode: 'MAD',
destinationIataCode: 'CDG',
departureDate: '2022-03-13',
})
//Cars & Transfers APIs
// Transfer Search API: Search Transfer
amadeus.shopping.transferOffers.post(JSON.stringify(body));
// Transfer Book API: Book a transfer based on the offer id
amadeus.ordering.transferOrders.post(JSON.stringify(body),offerId='2094123123');
// Transfer Management API: Cancel a transfer based on the order id & confirmation number
amadeus.ordering.transferOrder('XXX').transfers.cancellation.post(JSON.stringify({}), confirmNbr='12345');
Want to contribute? Read our Contributors Guide for guidance on installing and running this code in a development environment.
This library is released under the MIT License.
You can find us on StackOverflow or join our developer community on Discord.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for amadeus-node
Similar Open Source Tools
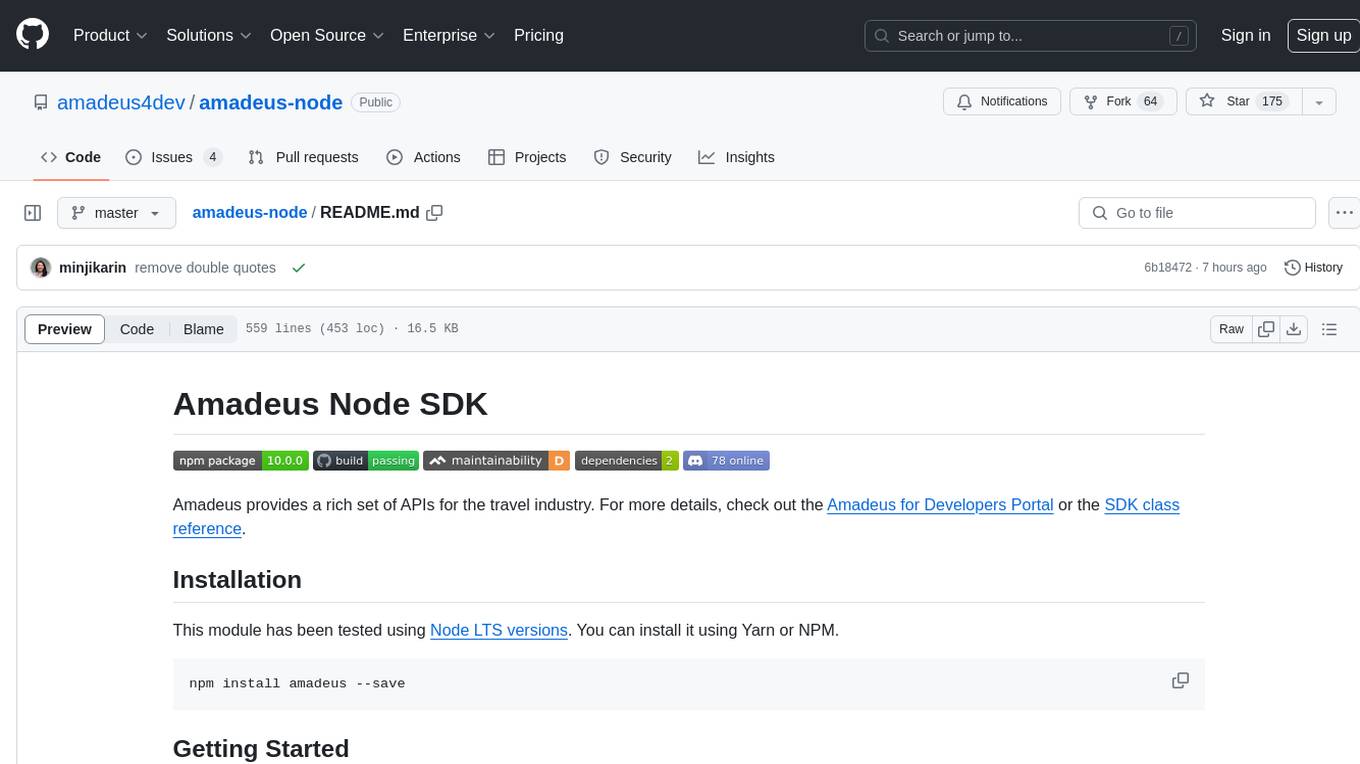
amadeus-node
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
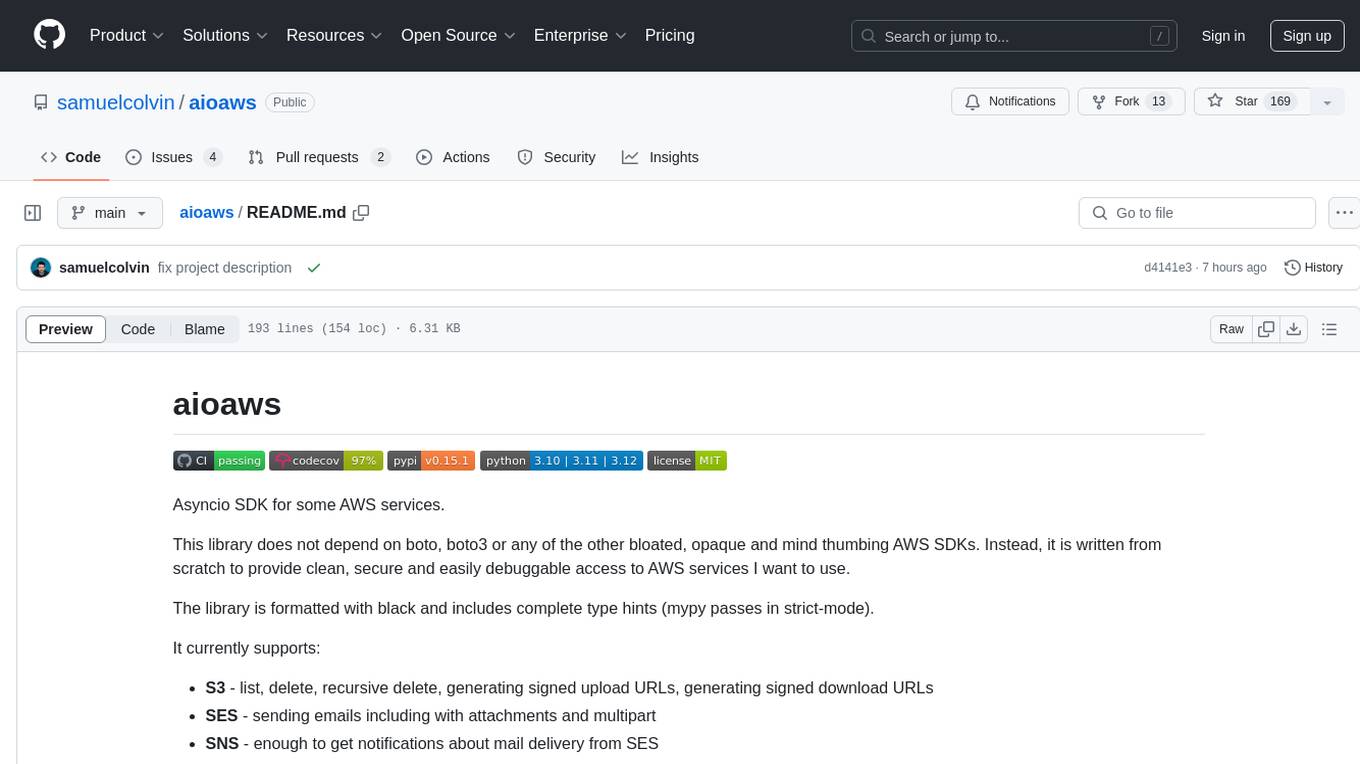
aioaws
Aioaws is an asyncio SDK for some AWS services, providing clean, secure, and easily debuggable access to services like S3, SES, and SNS. It is written from scratch without dependencies on boto or boto3, formatted with black, and includes complete type hints. The library supports various functionalities such as listing, deleting, and generating signed URLs for S3 files, sending emails with attachments and multipart content via SES, and receiving notifications about mail delivery from SES. It also offers AWS Signature Version 4 authentication and has minimal dependencies like aiofiles, cryptography, httpx, and pydantic.
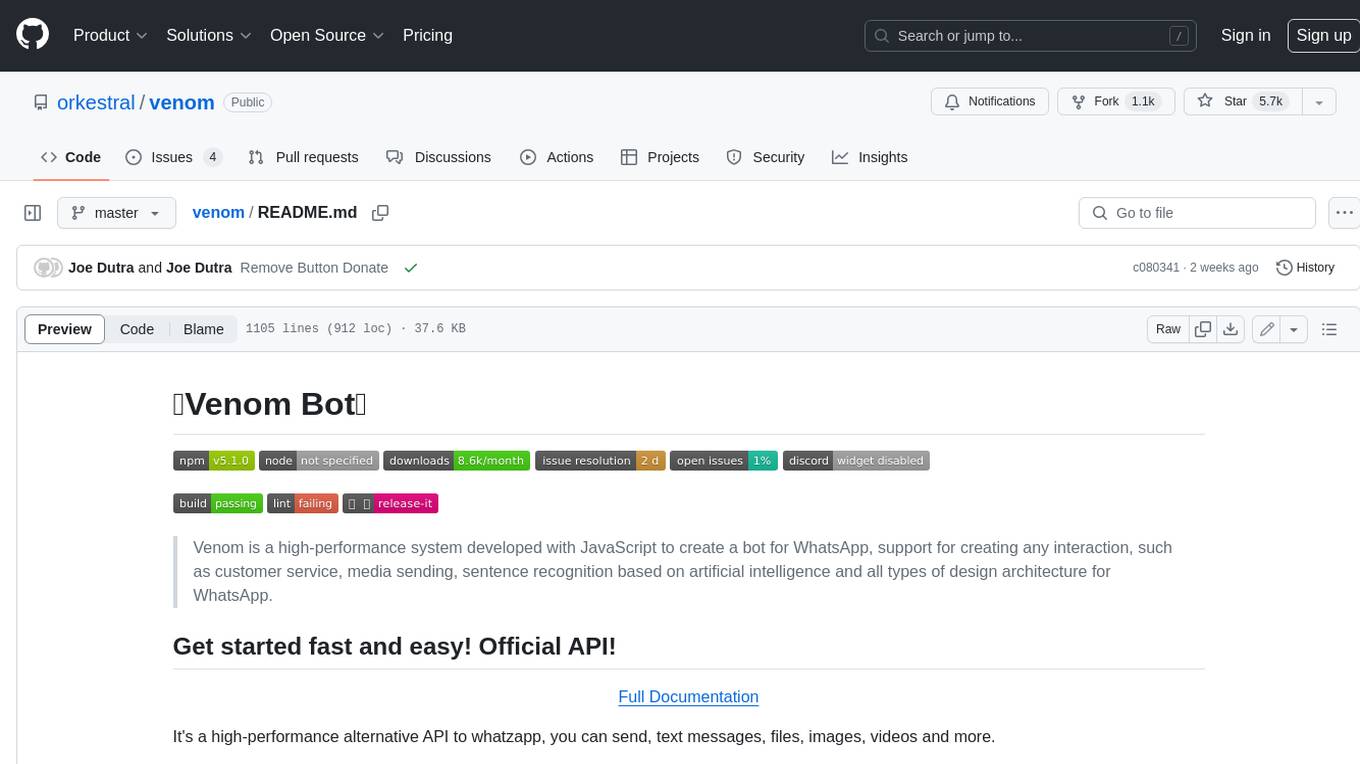
venom
Venom is a high-performance system developed with JavaScript to create a bot for WhatsApp, support for creating any interaction, such as customer service, media sending, sentence recognition based on artificial intelligence and all types of design architecture for WhatsApp.
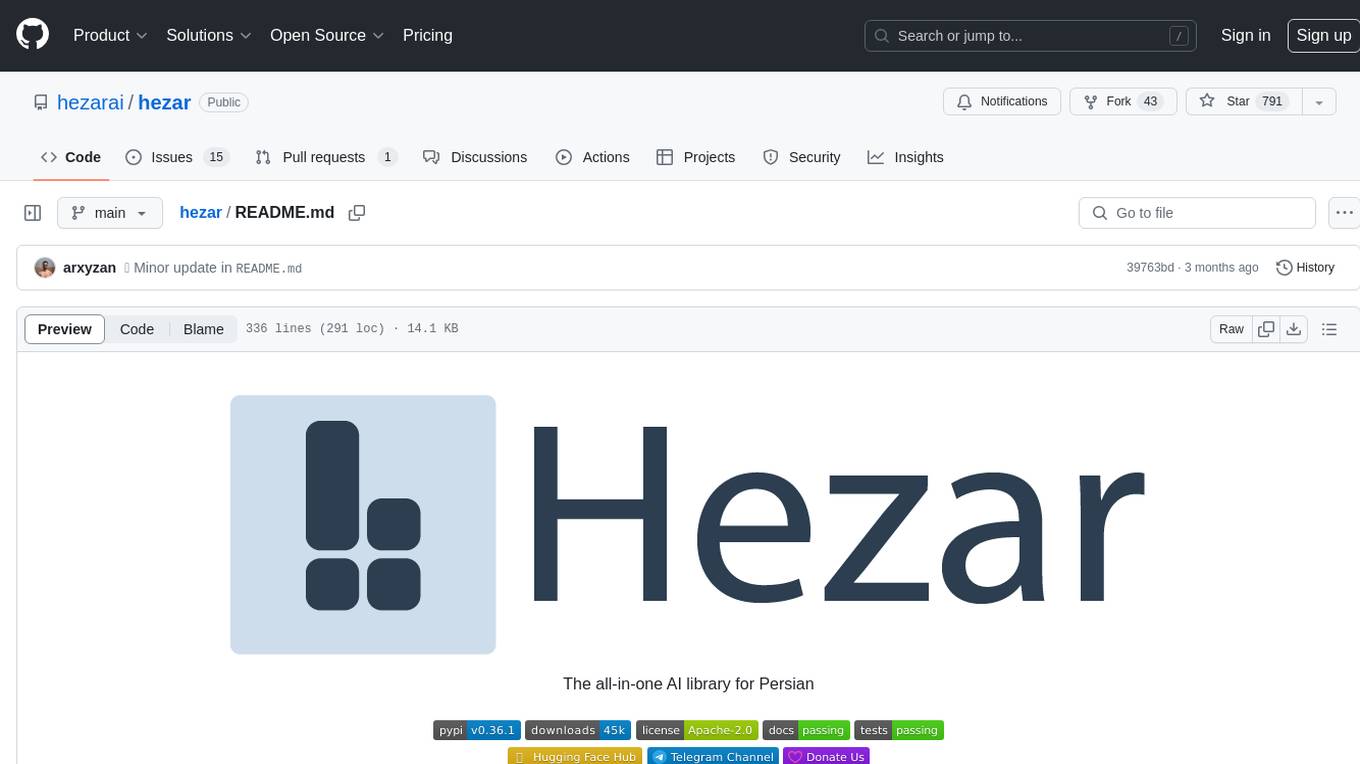
hezar
Hezar is an all-in-one AI library designed specifically for the Persian community. It brings together various AI models and tools, making it easy to use AI with just a few lines of code. The library seamlessly integrates with Hugging Face Hub, offering a developer-friendly interface and task-based model interface. In addition to models, Hezar provides tools like word embeddings, tokenizers, feature extractors, and more. It also includes supplementary ML tools for deployment, benchmarking, and optimization.
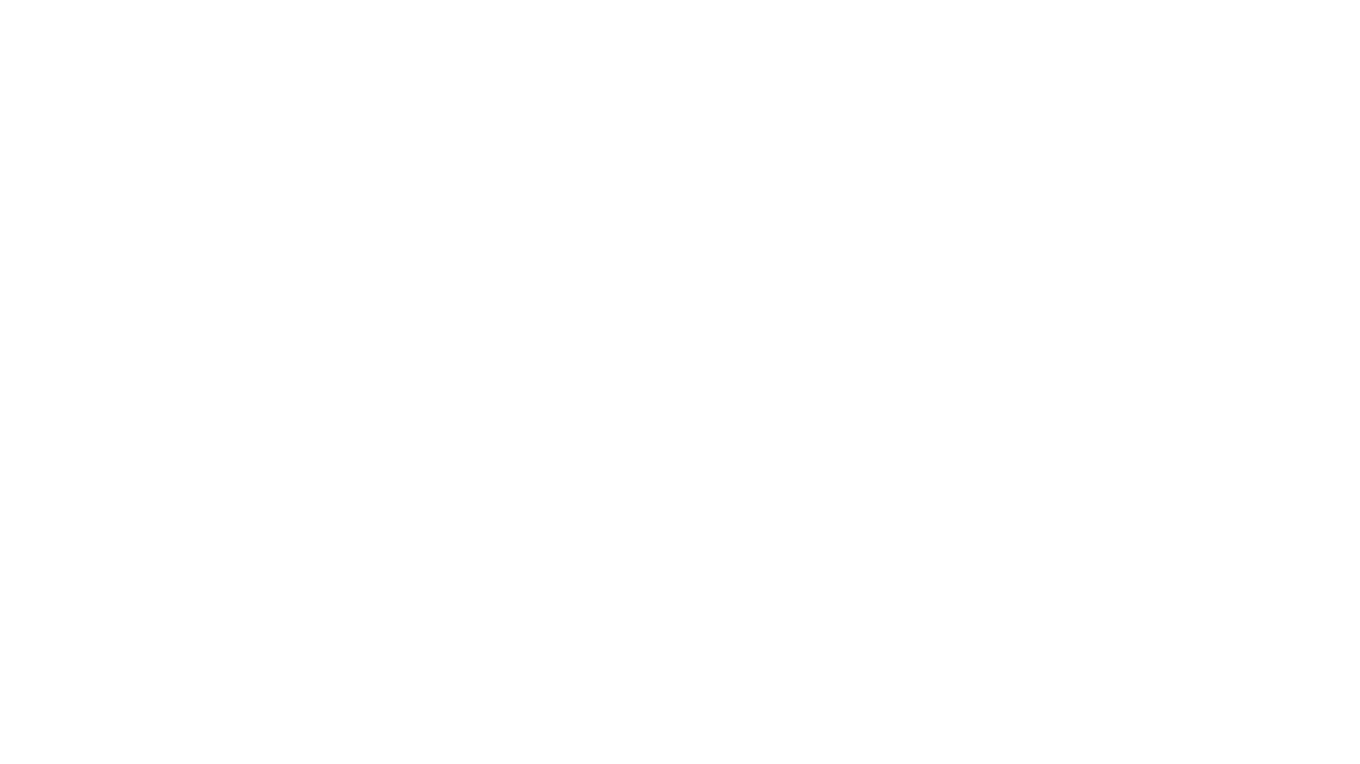
lagent
Lagent is a lightweight open-source framework that allows users to efficiently build large language model(LLM)-based agents. It also provides some typical tools to augment LLM. The overview of our framework is shown below:
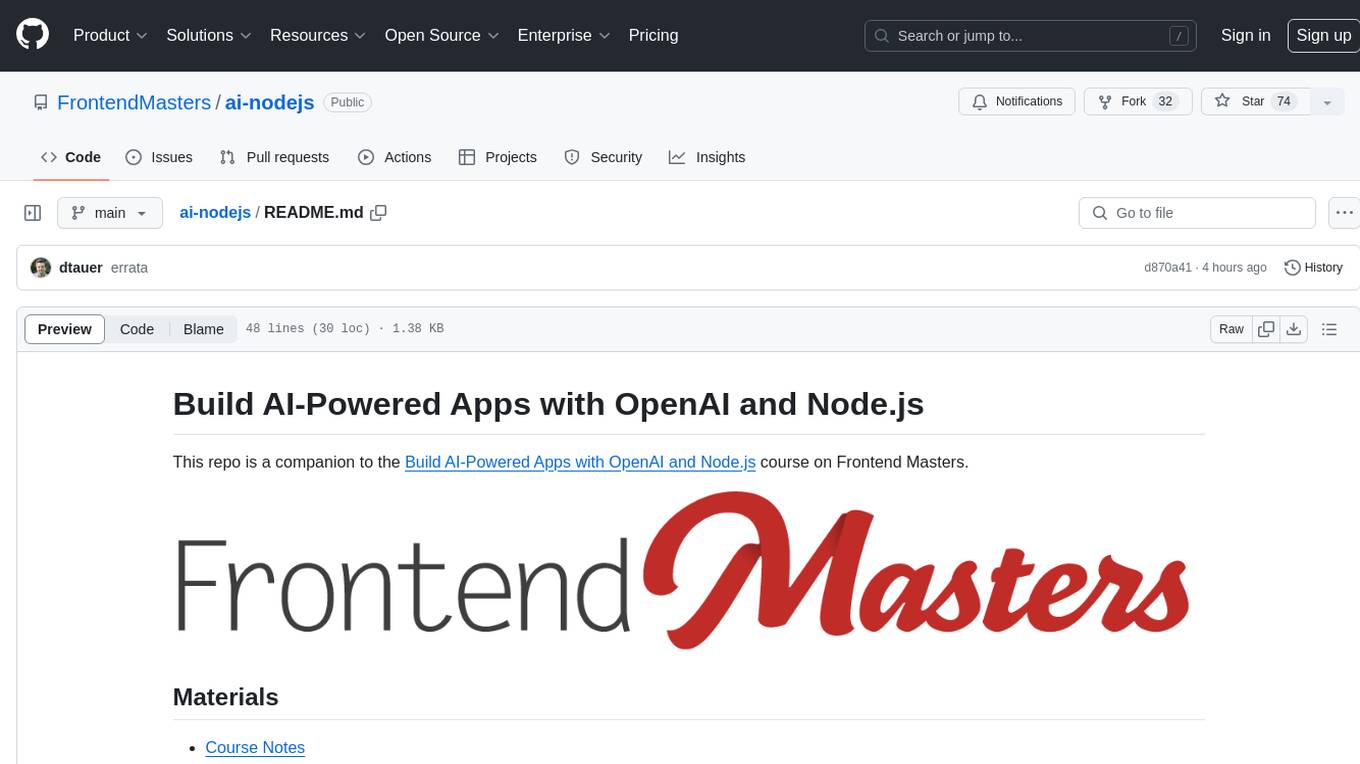
ai-nodejs
This repository serves as a companion to the Build AI-Powered Apps with OpenAI and Node.js course on Frontend Masters. It includes course notes and provides alternative approaches for deprecated Langchain methods by installing the Langchain community module and importing loaders for document processing from PDFs and YouTube videos.
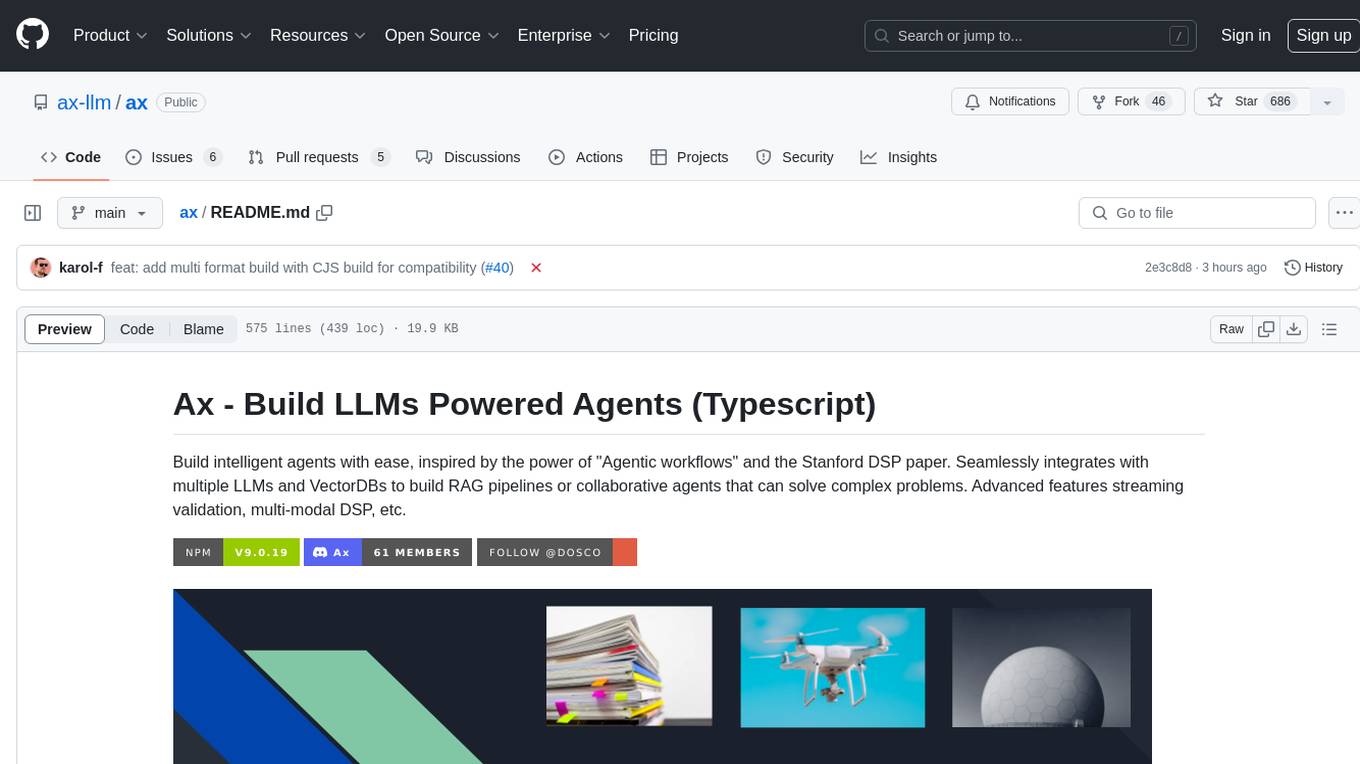
ax
Ax is a Typescript library that allows users to build intelligent agents inspired by agentic workflows and the Stanford DSP paper. It seamlessly integrates with multiple Large Language Models (LLMs) and VectorDBs to create RAG pipelines or collaborative agents capable of solving complex problems. The library offers advanced features such as streaming validation, multi-modal DSP, and automatic prompt tuning using optimizers. Users can easily convert documents of any format to text, perform smart chunking, embedding, and querying, and ensure output validation while streaming. Ax is production-ready, written in Typescript, and has zero dependencies.
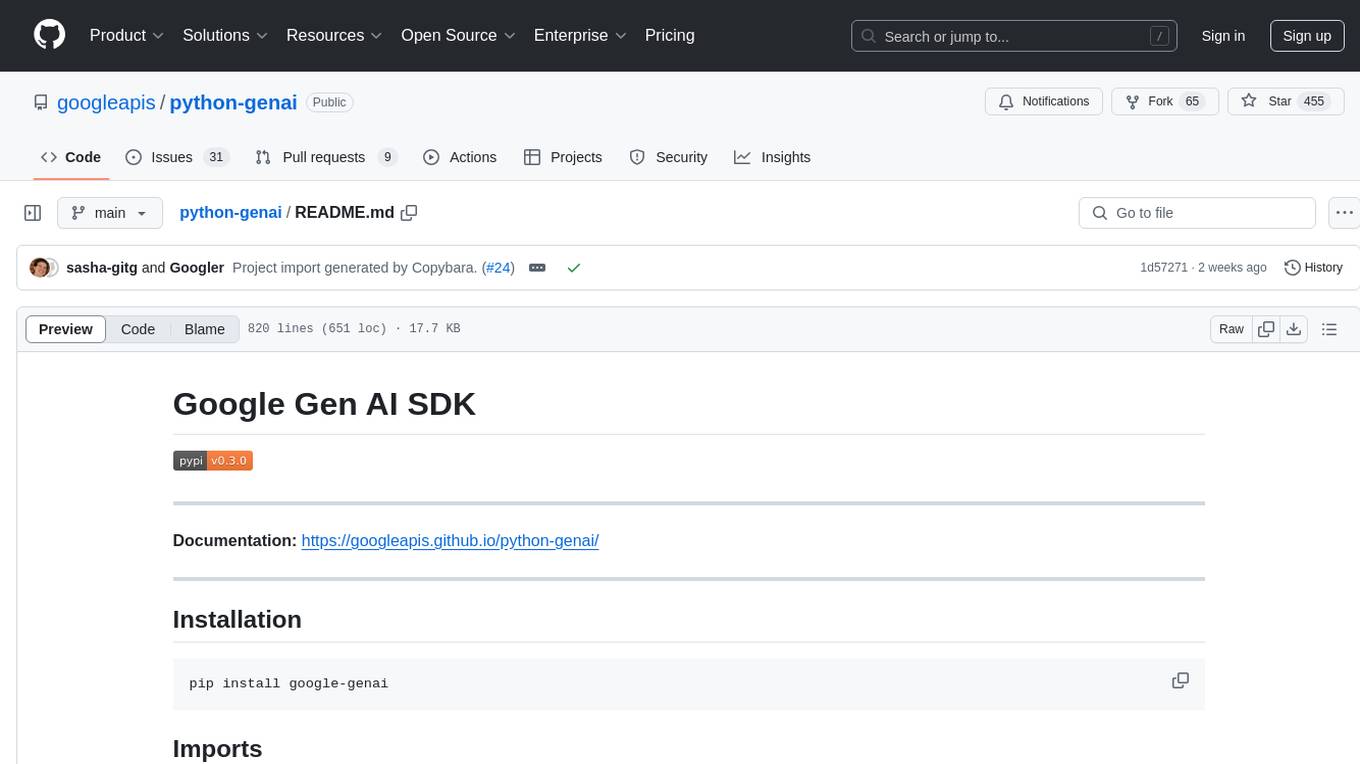
python-genai
The Google Gen AI SDK is a Python library that provides access to Google AI and Vertex AI services. It allows users to create clients for different services, work with parameter types, models, generate content, call functions, handle JSON response schemas, stream text and image content, perform async operations, count and compute tokens, embed content, generate and upscale images, edit images, work with files, create and get cached content, tune models, distill models, perform batch predictions, and more. The SDK supports various features like automatic function support, manual function declaration, JSON response schema support, streaming for text and image content, async methods, tuning job APIs, distillation, batch prediction, and more.
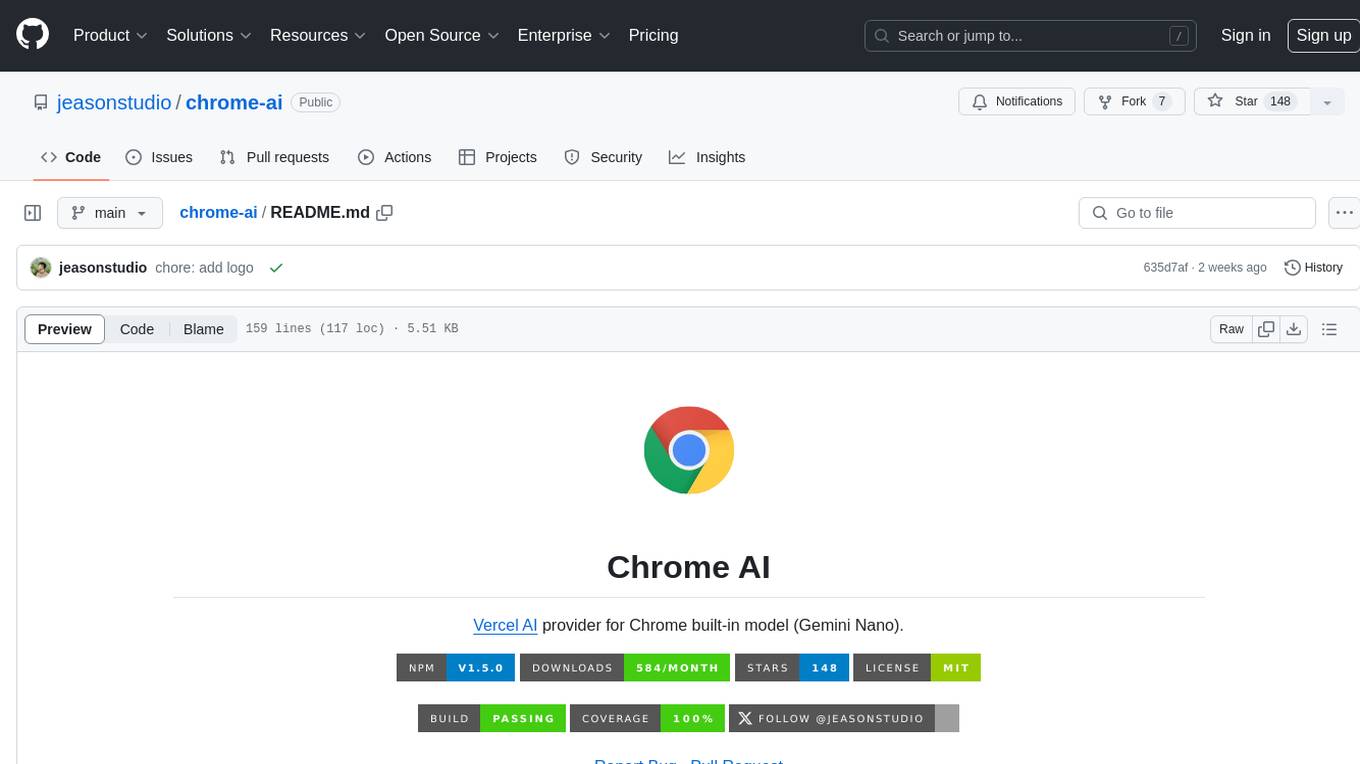
chrome-ai
Chrome AI is a Vercel AI provider for Chrome's built-in model (Gemini Nano). It allows users to create language models using Chrome's AI capabilities. The tool is under development and may contain errors and frequent changes. Users can install the ChromeAI provider module and use it to generate text, stream text, and generate objects. To enable AI in Chrome, users need to have Chrome version 127 or greater and turn on specific flags. The tool is designed for developers and researchers interested in experimenting with Chrome's built-in AI features.
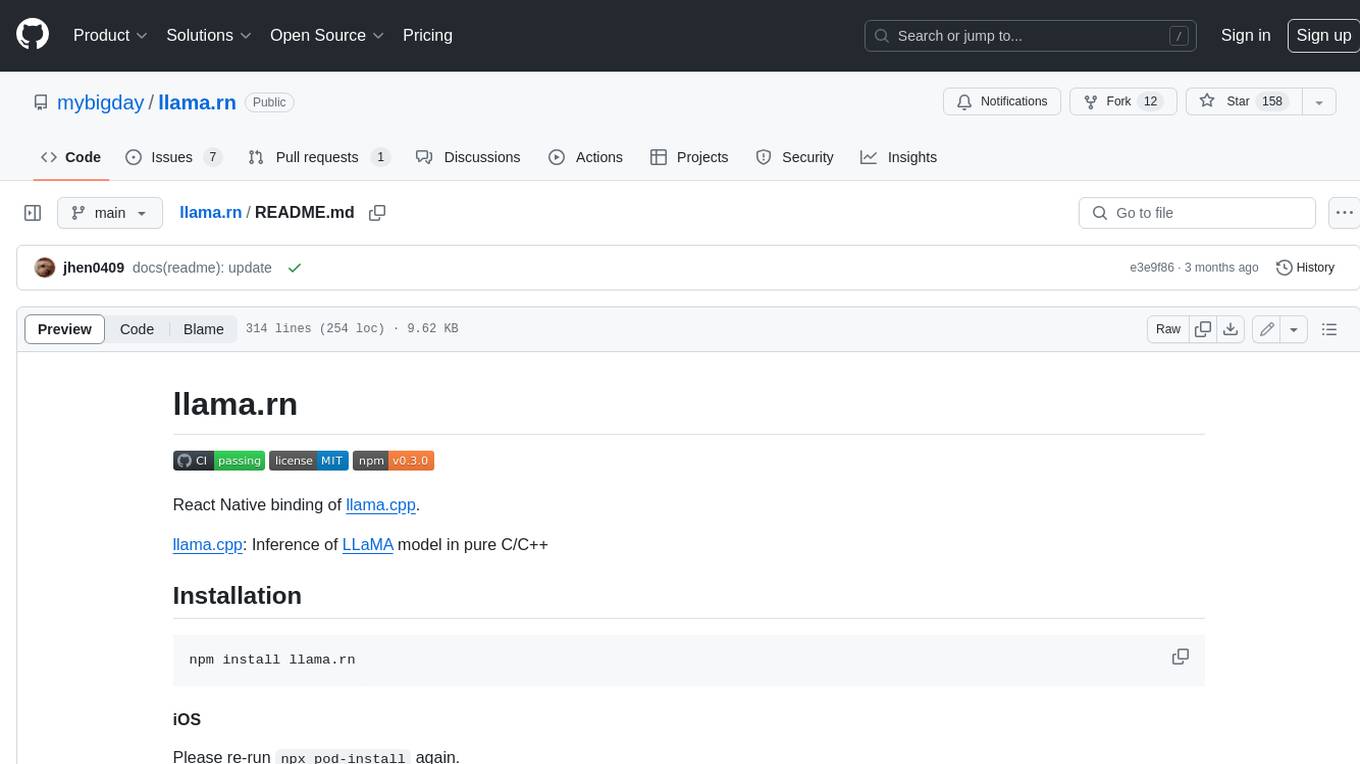
llama.rn
React Native binding of llama.cpp, which is an inference of LLaMA model in pure C/C++. This tool allows you to use the LLaMA model in your React Native applications for various tasks such as text completion, tokenization, detokenization, and embedding. It provides a convenient interface to interact with the LLaMA model and supports features like grammar sampling and mocking for testing purposes.
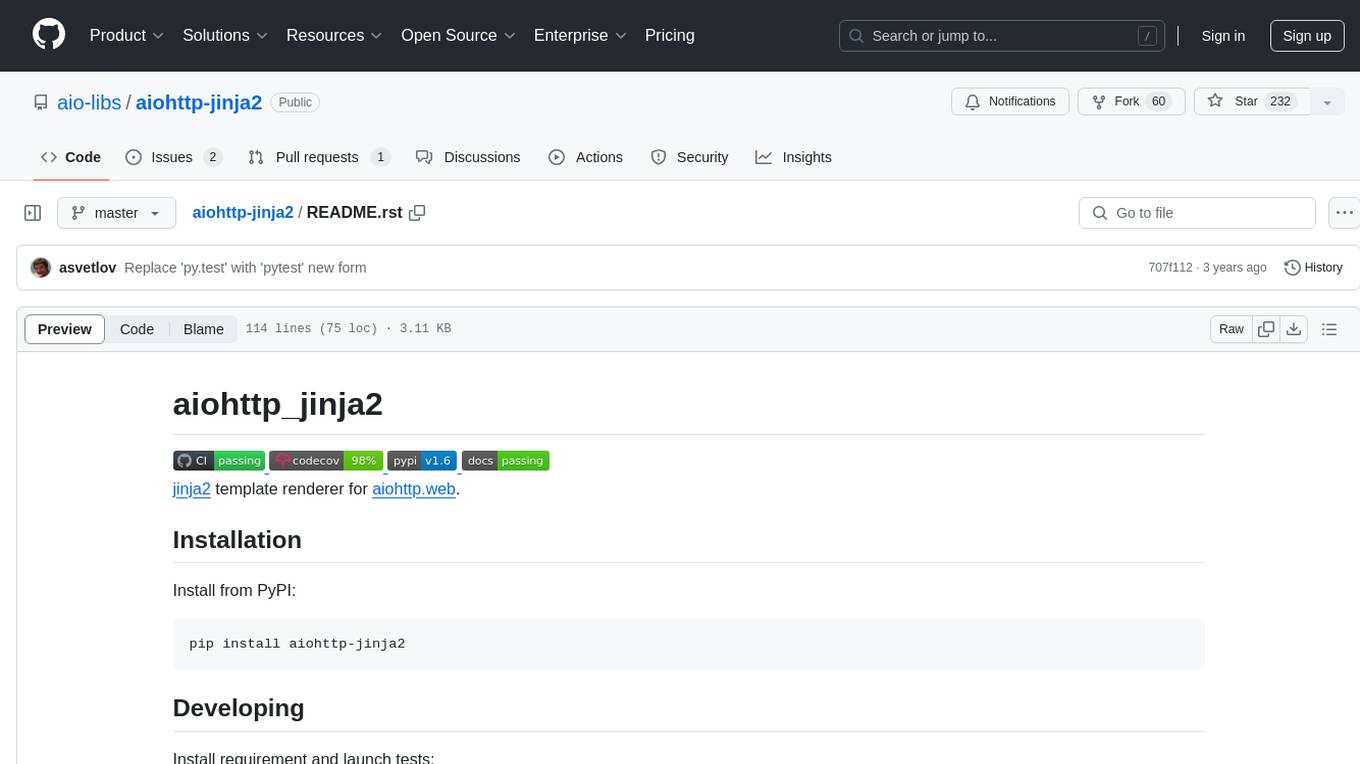
aiohttp-jinja2
aiohttp_jinja2 is a Jinja2 template renderer for aiohttp.web, allowing users to render templates in web applications built with aiohttp. It provides a convenient way to set up Jinja2 environment, use template engine in web handlers, and perform complex processing like setting response headers. The tool simplifies the process of rendering HTML text based on templates and passing context data to templates for dynamic content generation.
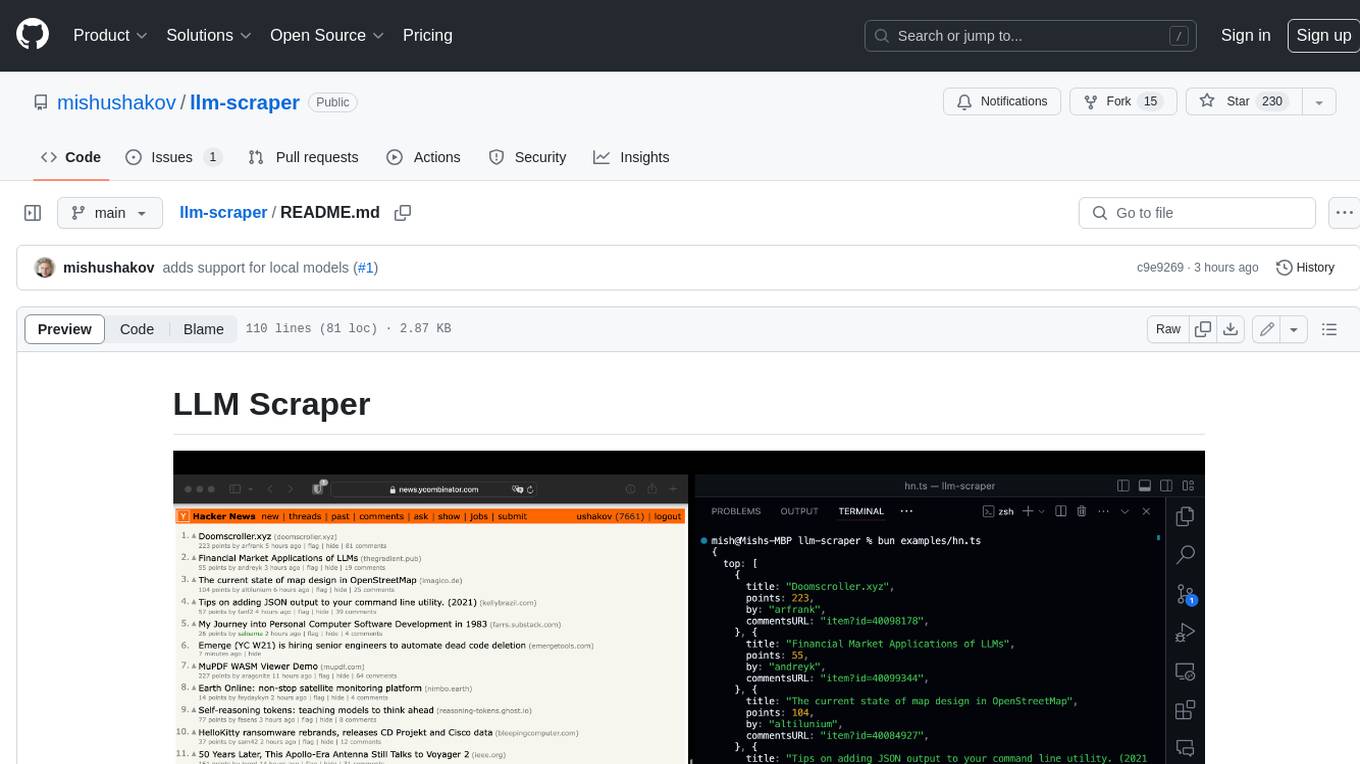
llm-scraper
LLM Scraper is a TypeScript library that allows you to convert any webpages into structured data using LLMs. It supports Local (GGUF), OpenAI, Groq chat models, and schemas defined with Zod. With full type-safety in TypeScript and based on the Playwright framework, it offers streaming when crawling multiple pages and supports four input modes: html, markdown, text, and image.
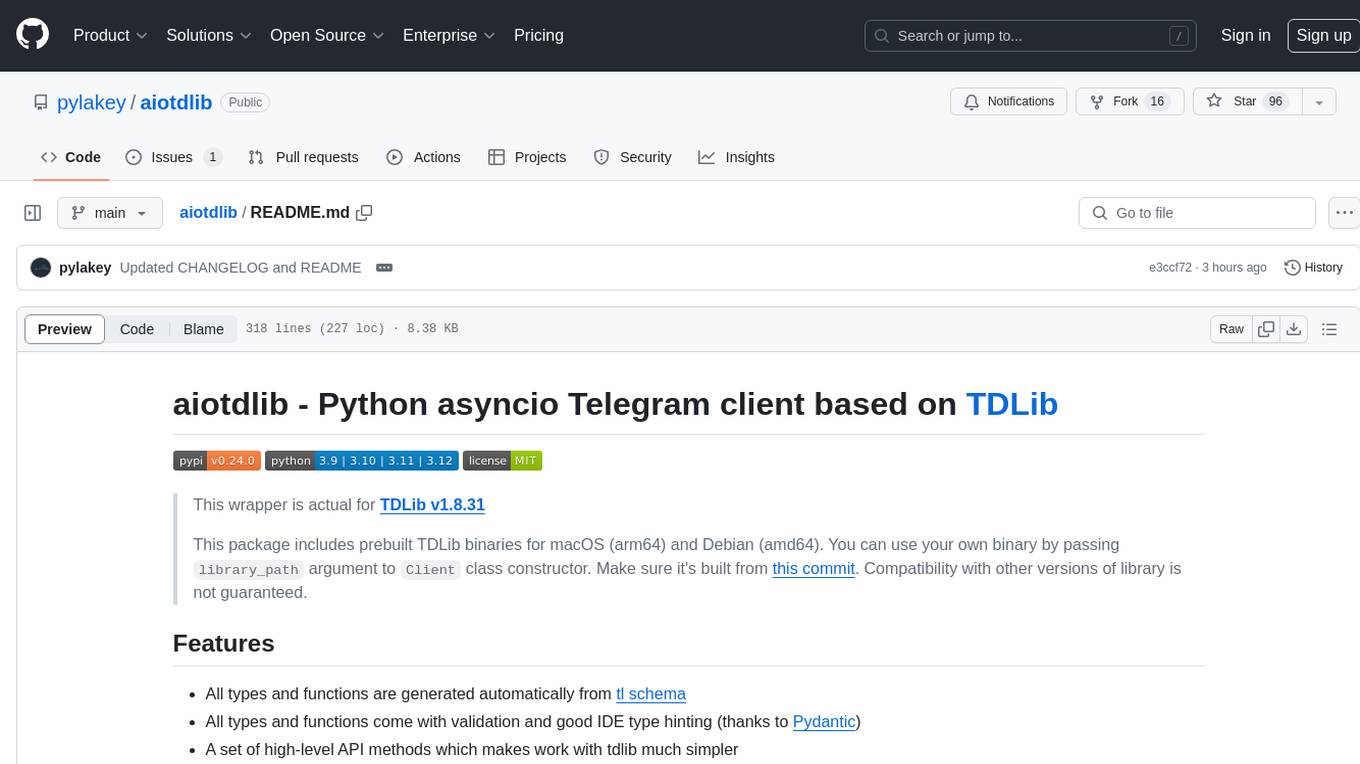
aiotdlib
aiotdlib is a Python asyncio Telegram client based on TDLib. It provides automatic generation of types and functions from tl schema, validation, good IDE type hinting, and high-level API methods for simpler work with tdlib. The package includes prebuilt TDLib binaries for macOS (arm64) and Debian Bullseye (amd64). Users can use their own binary by passing `library_path` argument to `Client` class constructor. Compatibility with other versions of the library is not guaranteed. The tool requires Python 3.9+ and users need to get their `api_id` and `api_hash` from Telegram docs for installation and usage.
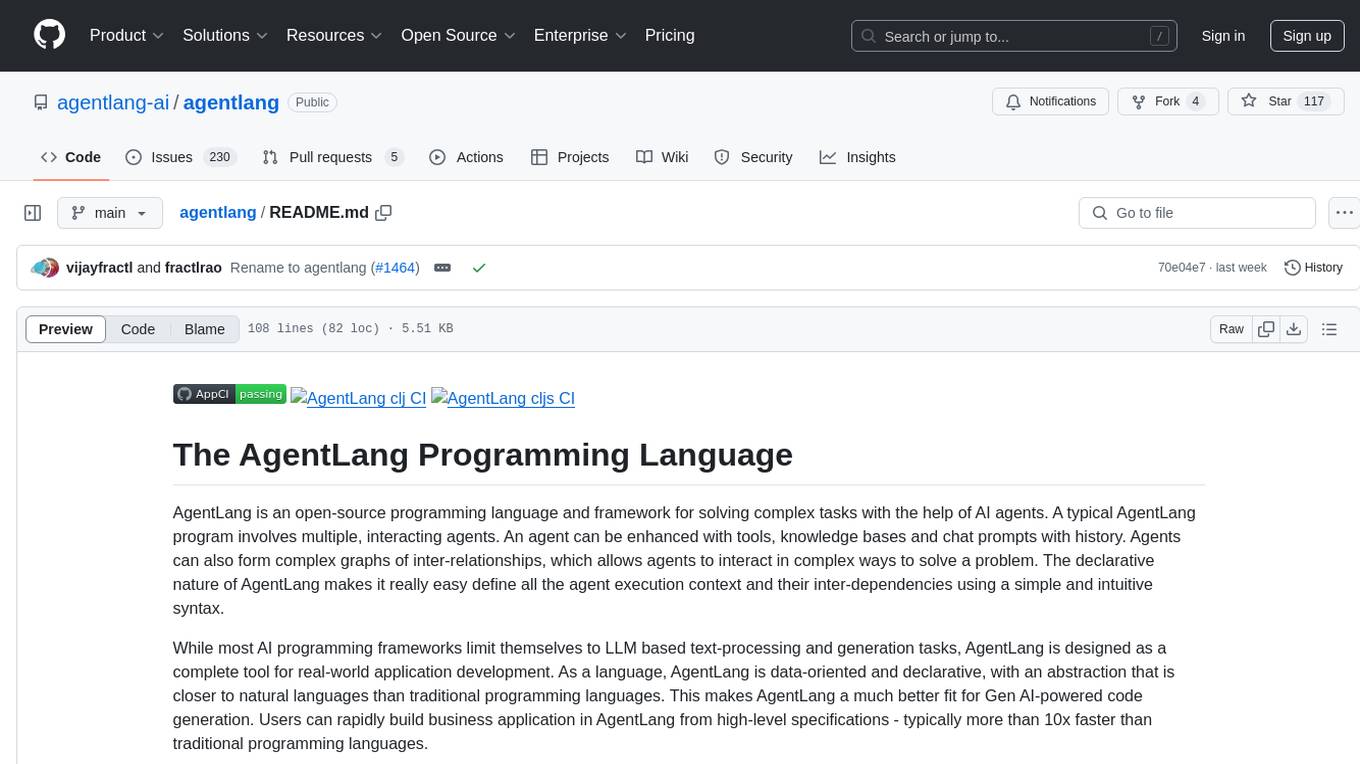
agentlang
AgentLang is an open-source programming language and framework designed for solving complex tasks with the help of AI agents. It allows users to build business applications rapidly from high-level specifications, making it more efficient than traditional programming languages. The language is data-oriented and declarative, with a syntax that is intuitive and closer to natural languages. AgentLang introduces innovative concepts such as first-class AI agents, graph-based hierarchical data model, zero-trust programming, declarative dataflow, resolvers, interceptors, and entity-graph-database mapping.
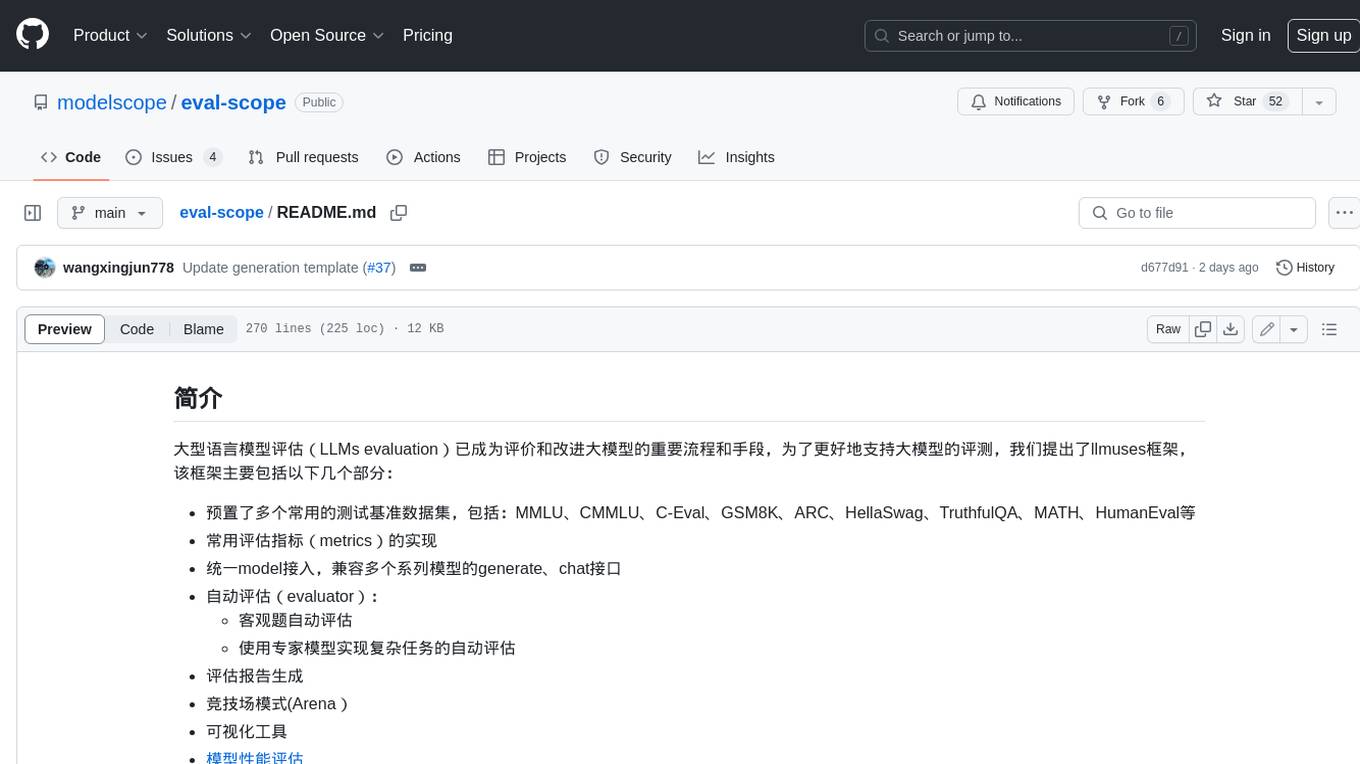
eval-scope
Eval-Scope is a framework for evaluating and improving large language models (LLMs). It provides a set of commonly used test datasets, metrics, and a unified model interface for generating and evaluating LLM responses. Eval-Scope also includes an automatic evaluator that can score objective questions and use expert models to evaluate complex tasks. Additionally, it offers a visual report generator, an arena mode for comparing multiple models, and a variety of other features to support LLM evaluation and development.
For similar tasks
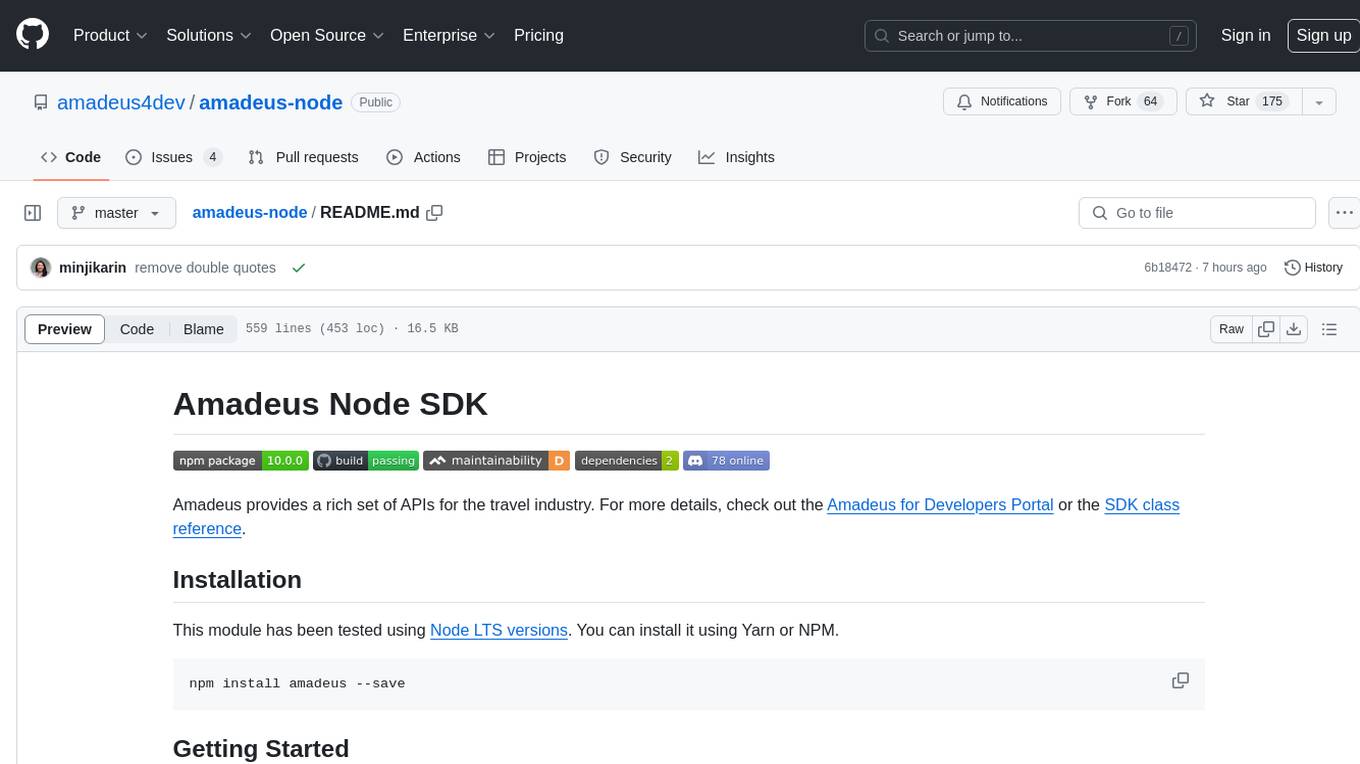
amadeus-node
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
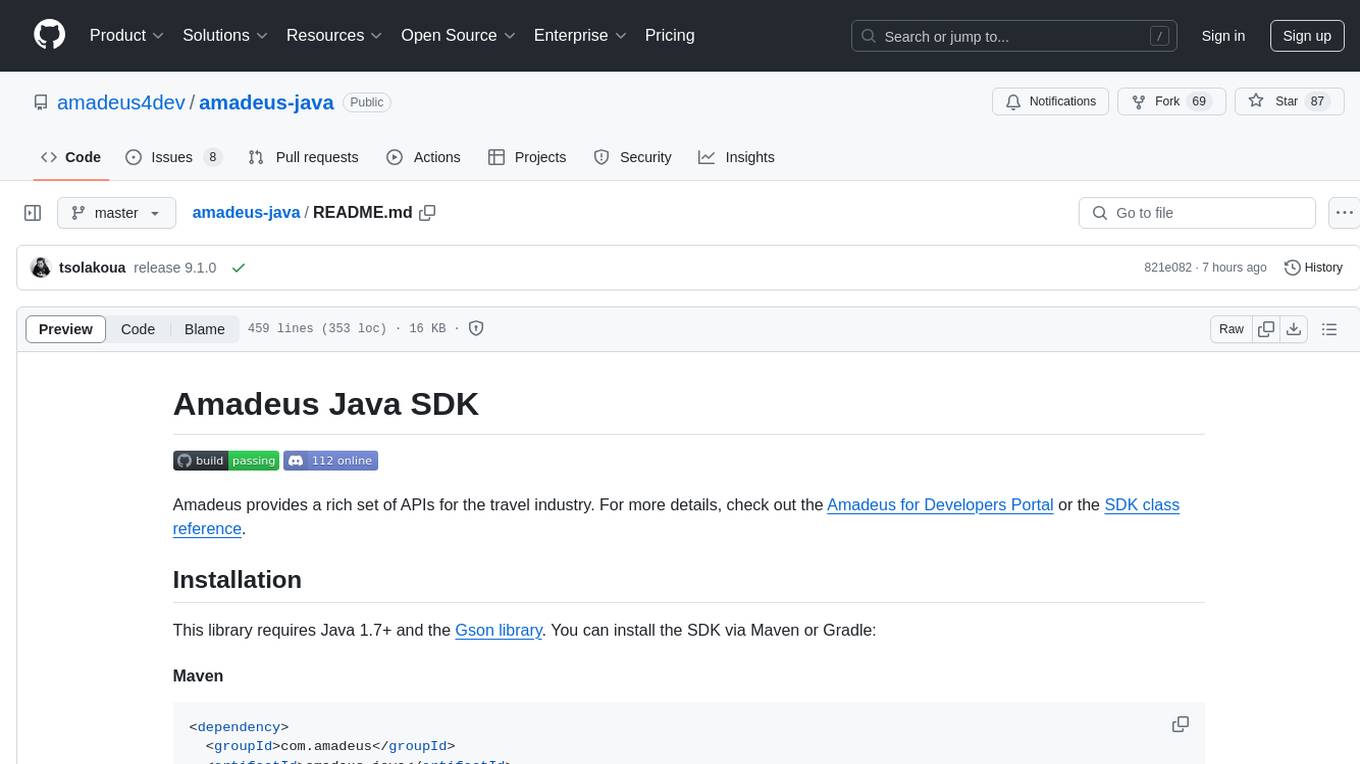
amadeus-java
Amadeus Java SDK provides a rich set of APIs for the travel industry, allowing developers to access various functionalities such as flight search, booking, airport information, and more. The SDK simplifies interaction with the Amadeus API by providing self-contained code examples and detailed documentation. Developers can easily make API calls, handle responses, and utilize features like pagination and logging. The SDK supports various endpoints for tasks like flight search, booking management, airport information retrieval, and travel analytics. It also offers functionalities for hotel search, booking, and sentiment analysis. Overall, the Amadeus Java SDK is a comprehensive tool for integrating Amadeus APIs into Java applications.
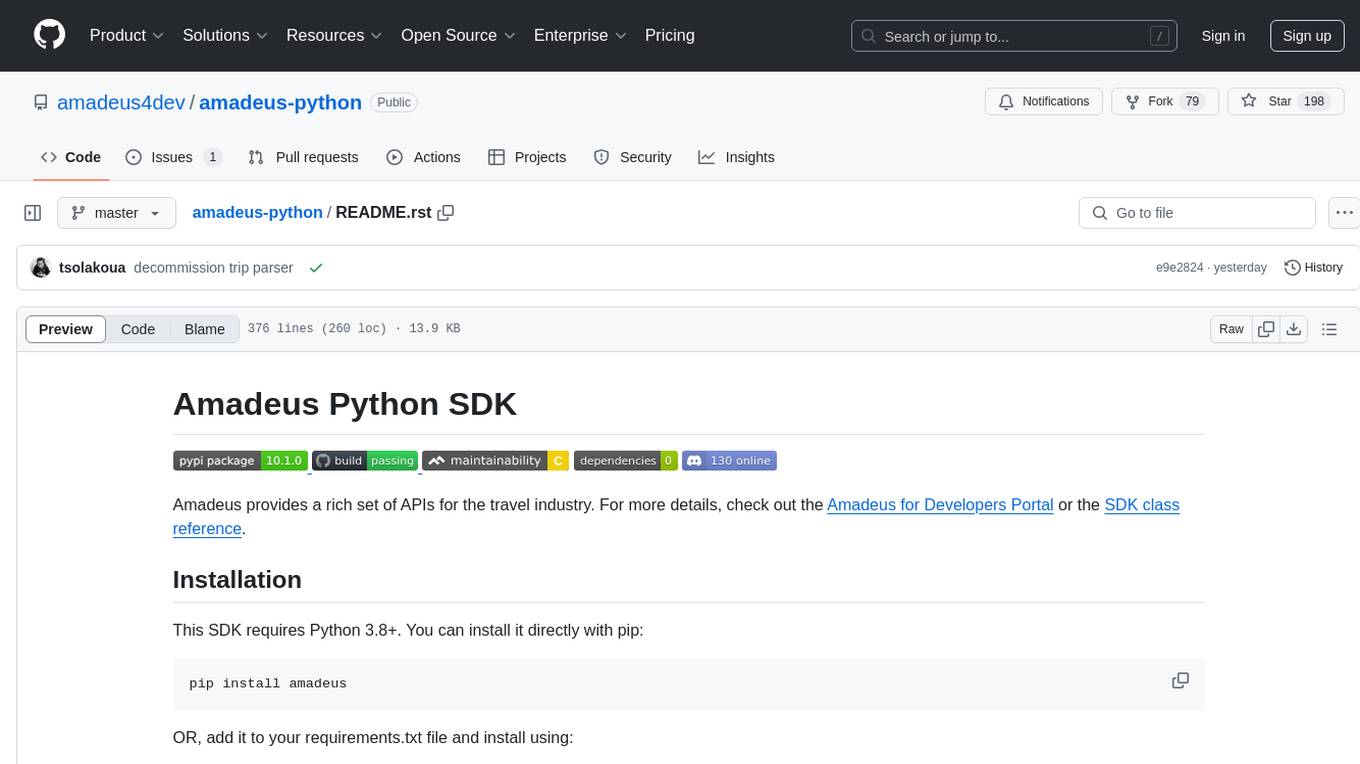
amadeus-python
Amadeus Python SDK provides a rich set of APIs for the travel industry. It allows users to make API calls for various travel-related tasks such as flight offers search, hotel bookings, trip purpose prediction, flight delay prediction, airport on-time performance, travel recommendations, and more. The SDK conveniently maps API paths to similar paths, making it easy to interact with the Amadeus APIs. Users can initialize the client with their API key and secret, make API calls, handle responses, and enable logging for debugging purposes. The SDK documentation includes detailed information about each SDK method, arguments, and return types.
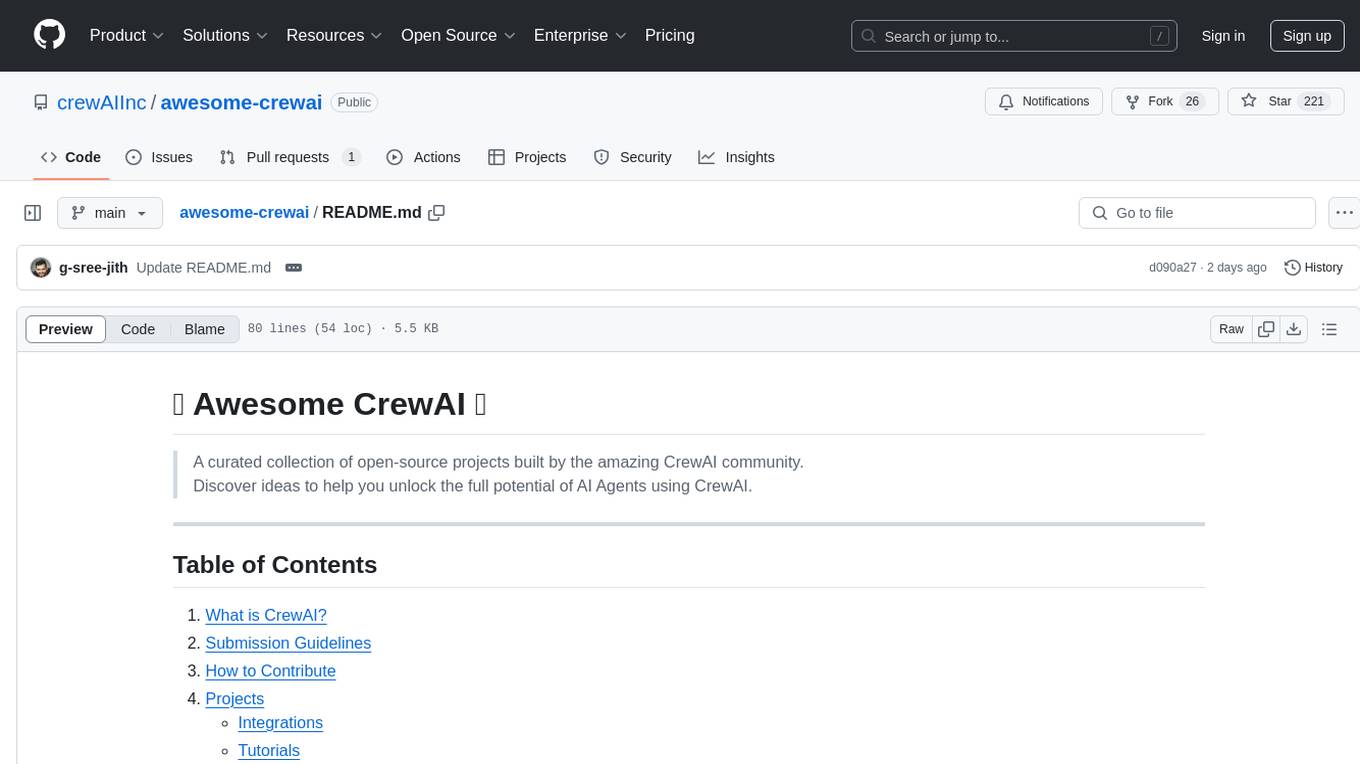
awesome-crewai
Awesome CrewAI is a curated collection of open-source projects built by the CrewAI community, aimed at unlocking the full potential of AI agents for supercharging business processes and decision-making. It includes integrations, tutorials, and tools that showcase the capabilities of CrewAI in various domains.
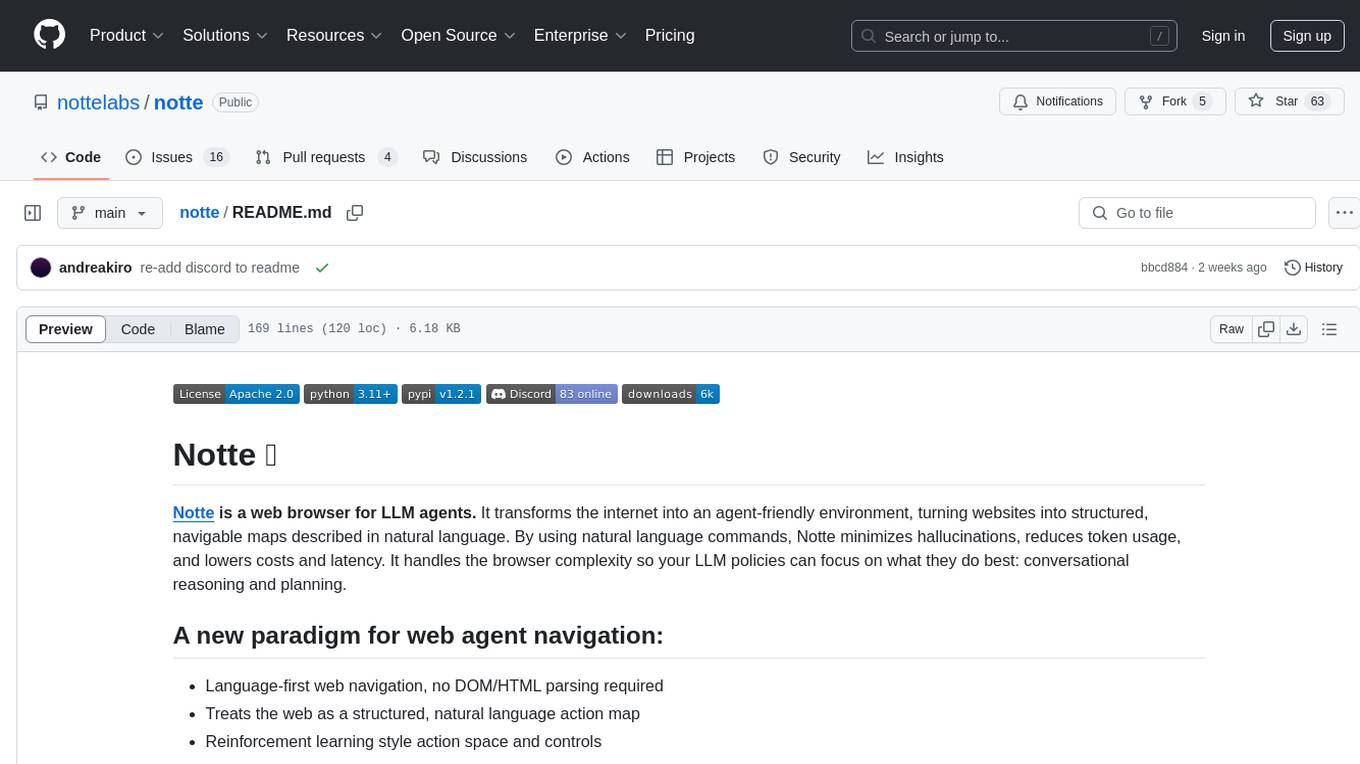
notte
Notte is a web browser designed specifically for LLM agents, providing a language-first web navigation experience without the need for DOM/HTML parsing. It transforms websites into structured, navigable maps described in natural language, enabling users to interact with the web using natural language commands. By simplifying browser complexity, Notte allows LLM policies to focus on conversational reasoning and planning, reducing token usage, costs, and latency. The tool supports various language model providers and offers a reinforcement learning style action space and controls for full navigation control.
For similar jobs
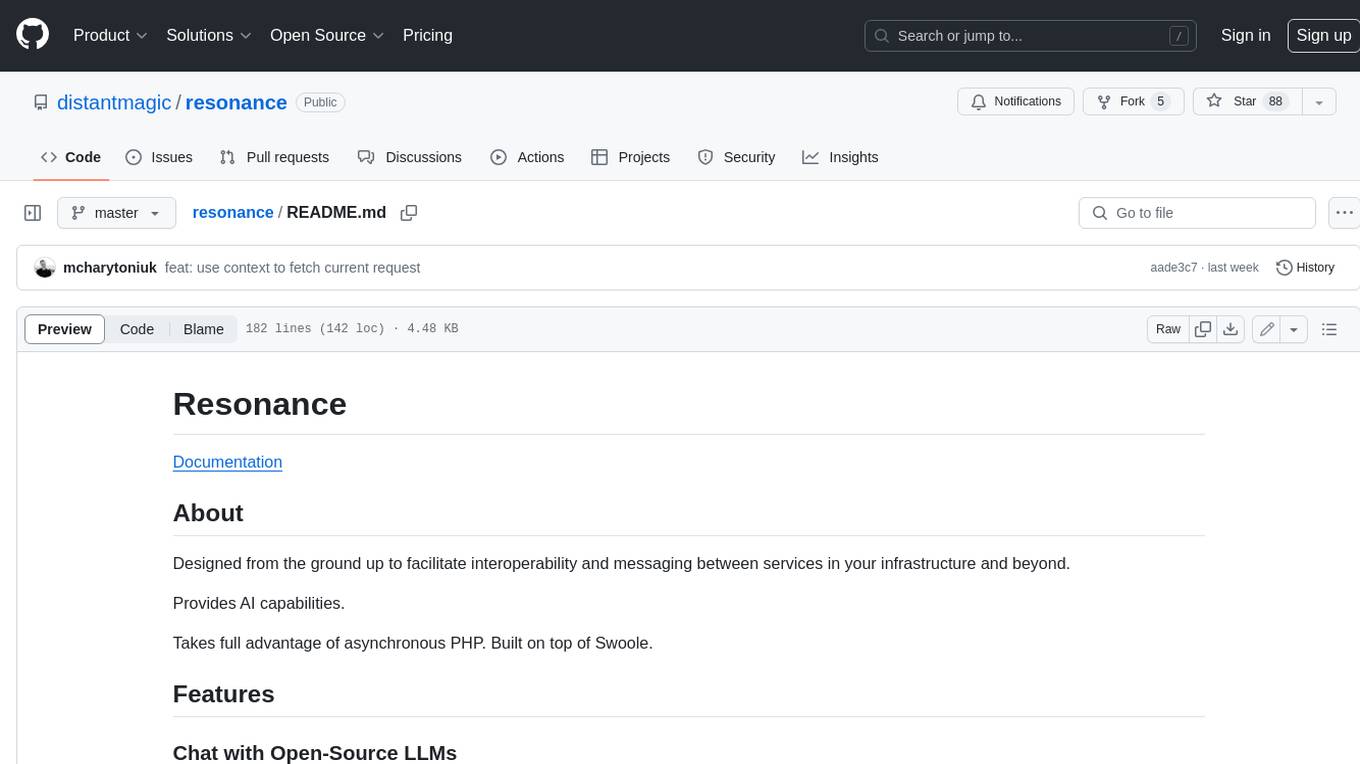
resonance
Resonance is a framework designed to facilitate interoperability and messaging between services in your infrastructure and beyond. It provides AI capabilities and takes full advantage of asynchronous PHP, built on top of Swoole. With Resonance, you can: * Chat with Open-Source LLMs: Create prompt controllers to directly answer user's prompts. LLM takes care of determining user's intention, so you can focus on taking appropriate action. * Asynchronous Where it Matters: Respond asynchronously to incoming RPC or WebSocket messages (or both combined) with little overhead. You can set up all the asynchronous features using attributes. No elaborate configuration is needed. * Simple Things Remain Simple: Writing HTTP controllers is similar to how it's done in the synchronous code. Controllers have new exciting features that take advantage of the asynchronous environment. * Consistency is Key: You can keep the same approach to writing software no matter the size of your project. There are no growing central configuration files or service dependencies registries. Every relation between code modules is local to those modules. * Promises in PHP: Resonance provides a partial implementation of Promise/A+ spec to handle various asynchronous tasks. * GraphQL Out of the Box: You can build elaborate GraphQL schemas by using just the PHP attributes. Resonance takes care of reusing SQL queries and optimizing the resources' usage. All fields can be resolved asynchronously.
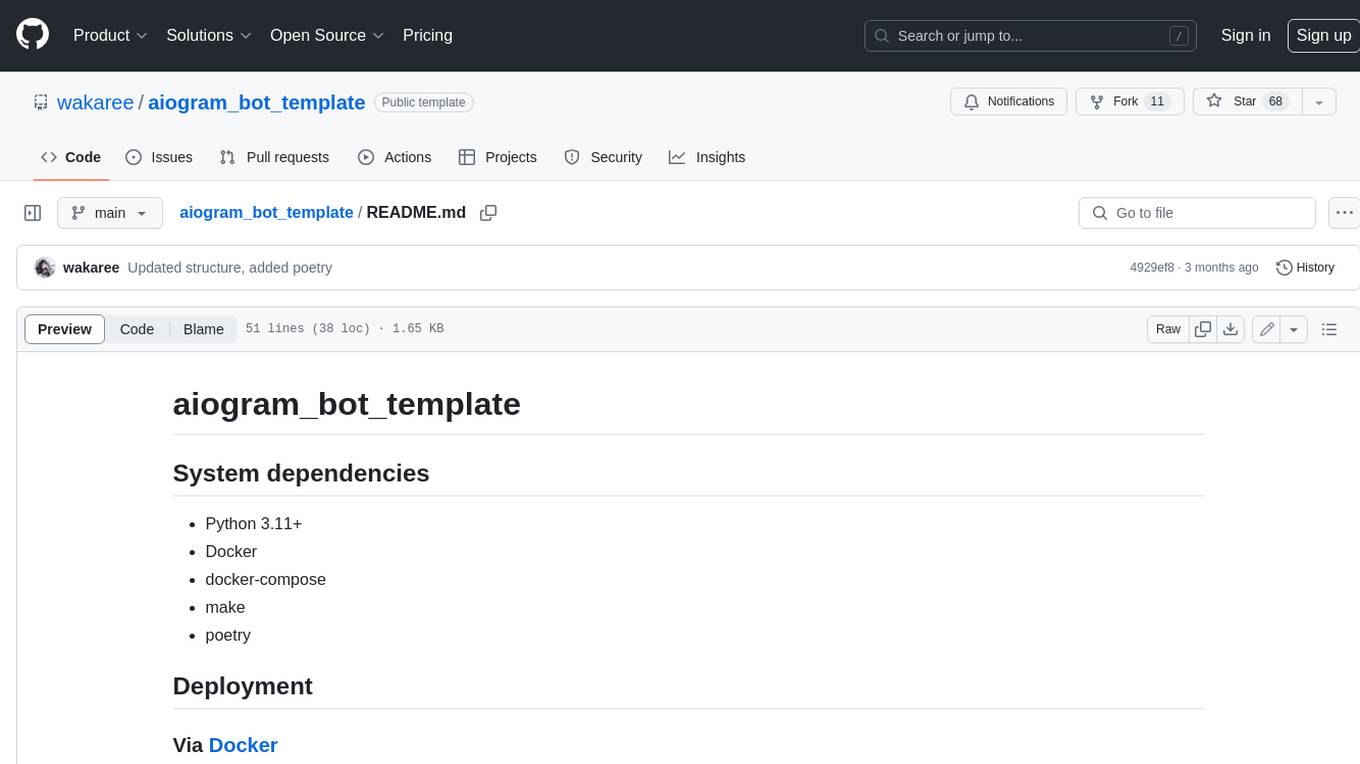
aiogram_bot_template
Aiogram bot template is a boilerplate for creating Telegram bots using Aiogram framework. It provides a solid foundation for building robust and scalable bots with a focus on code organization, database integration, and localization.
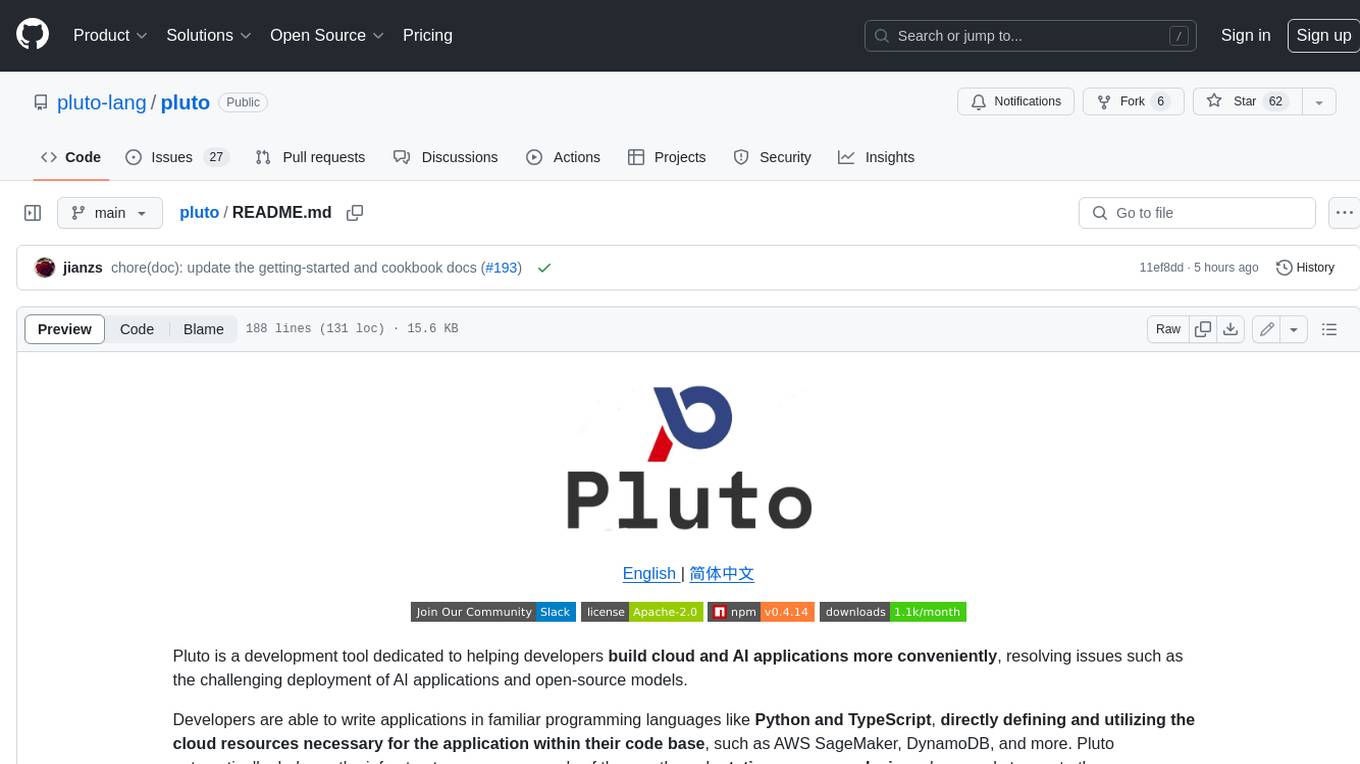
pluto
Pluto is a development tool dedicated to helping developers **build cloud and AI applications more conveniently** , resolving issues such as the challenging deployment of AI applications and open-source models. Developers are able to write applications in familiar programming languages like **Python and TypeScript** , **directly defining and utilizing the cloud resources necessary for the application within their code base** , such as AWS SageMaker, DynamoDB, and more. Pluto automatically deduces the infrastructure resource needs of the app through **static program analysis** and proceeds to create these resources on the specified cloud platform, **simplifying the resources creation and application deployment process**.
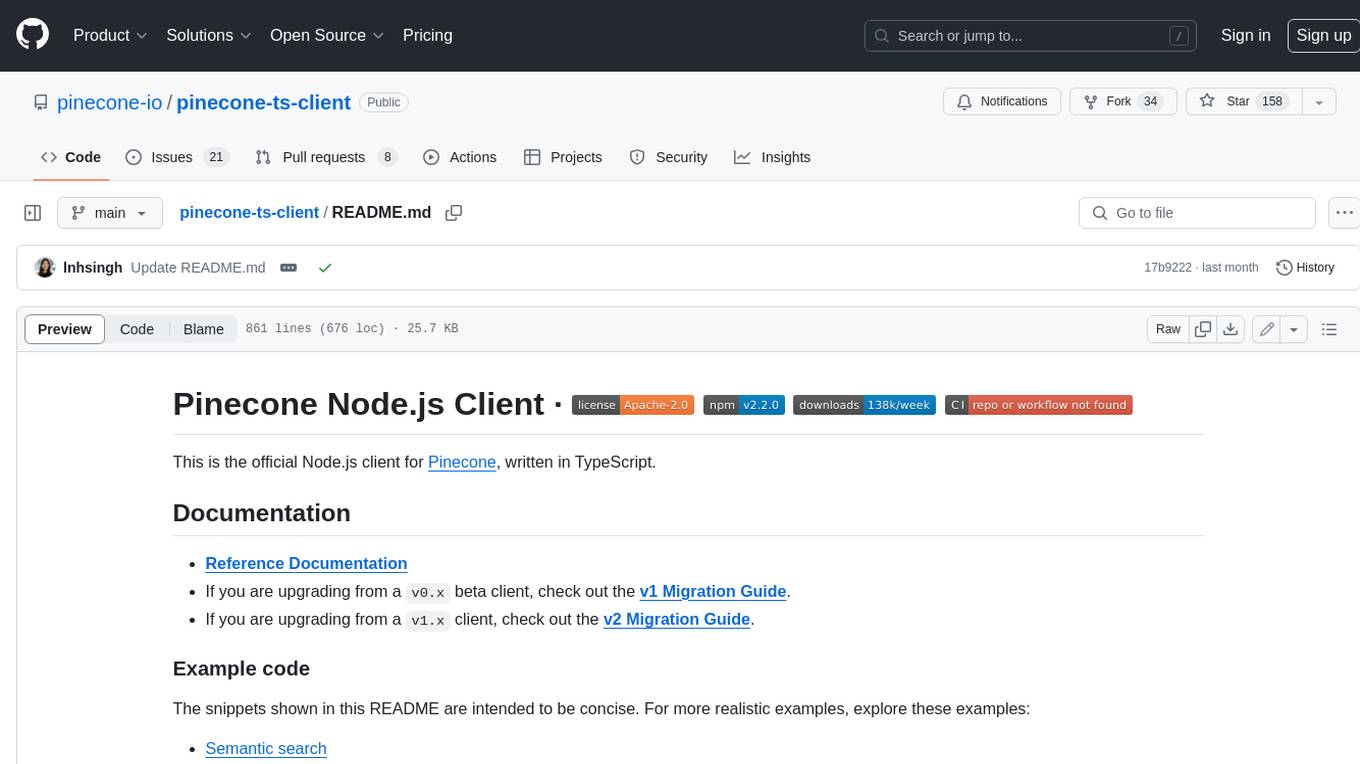
pinecone-ts-client
The official Node.js client for Pinecone, written in TypeScript. This client library provides a high-level interface for interacting with the Pinecone vector database service. With this client, you can create and manage indexes, upsert and query vector data, and perform other operations related to vector search and retrieval. The client is designed to be easy to use and provides a consistent and idiomatic experience for Node.js developers. It supports all the features and functionality of the Pinecone API, making it a comprehensive solution for building vector-powered applications in Node.js.
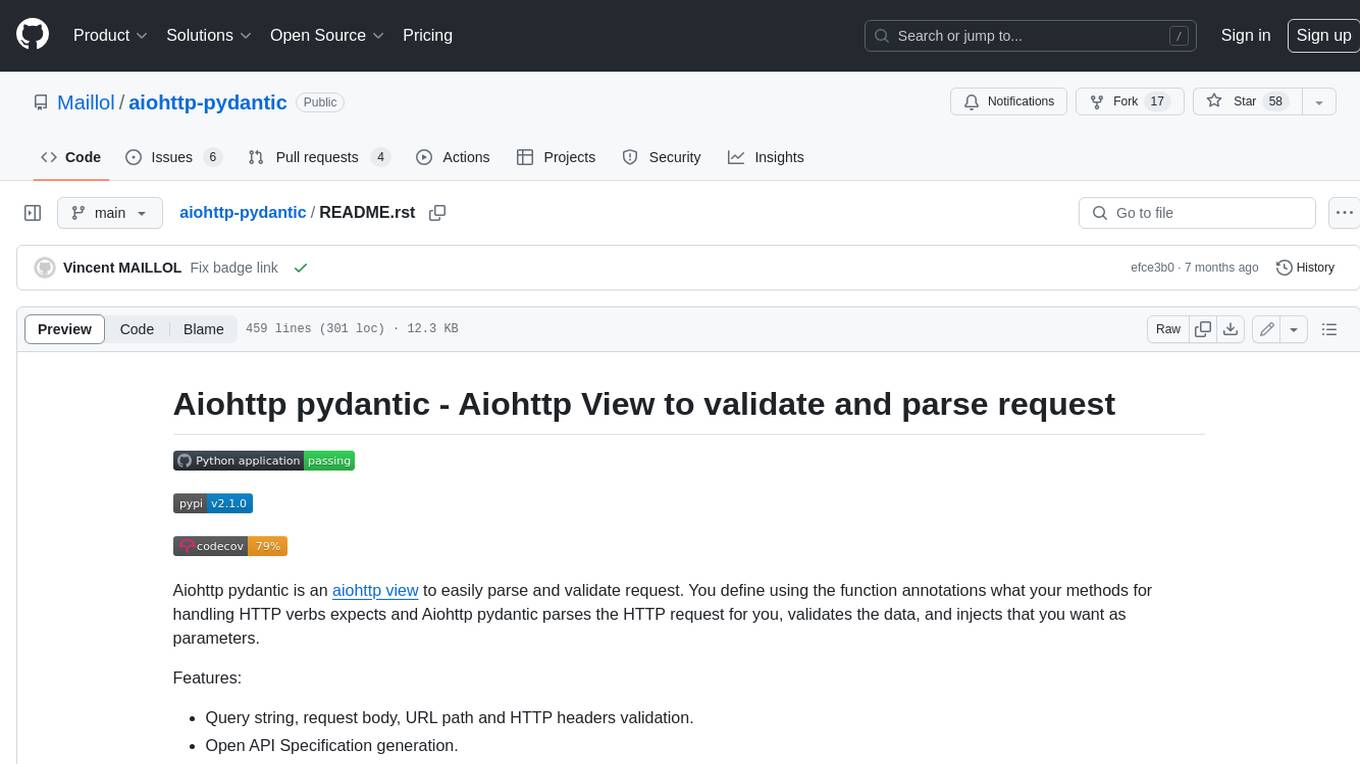
aiohttp-pydantic
Aiohttp pydantic is an aiohttp view to easily parse and validate requests. You define using function annotations what your methods for handling HTTP verbs expect, and Aiohttp pydantic parses the HTTP request for you, validates the data, and injects the parameters you want. It provides features like query string, request body, URL path, and HTTP headers validation, as well as Open API Specification generation.
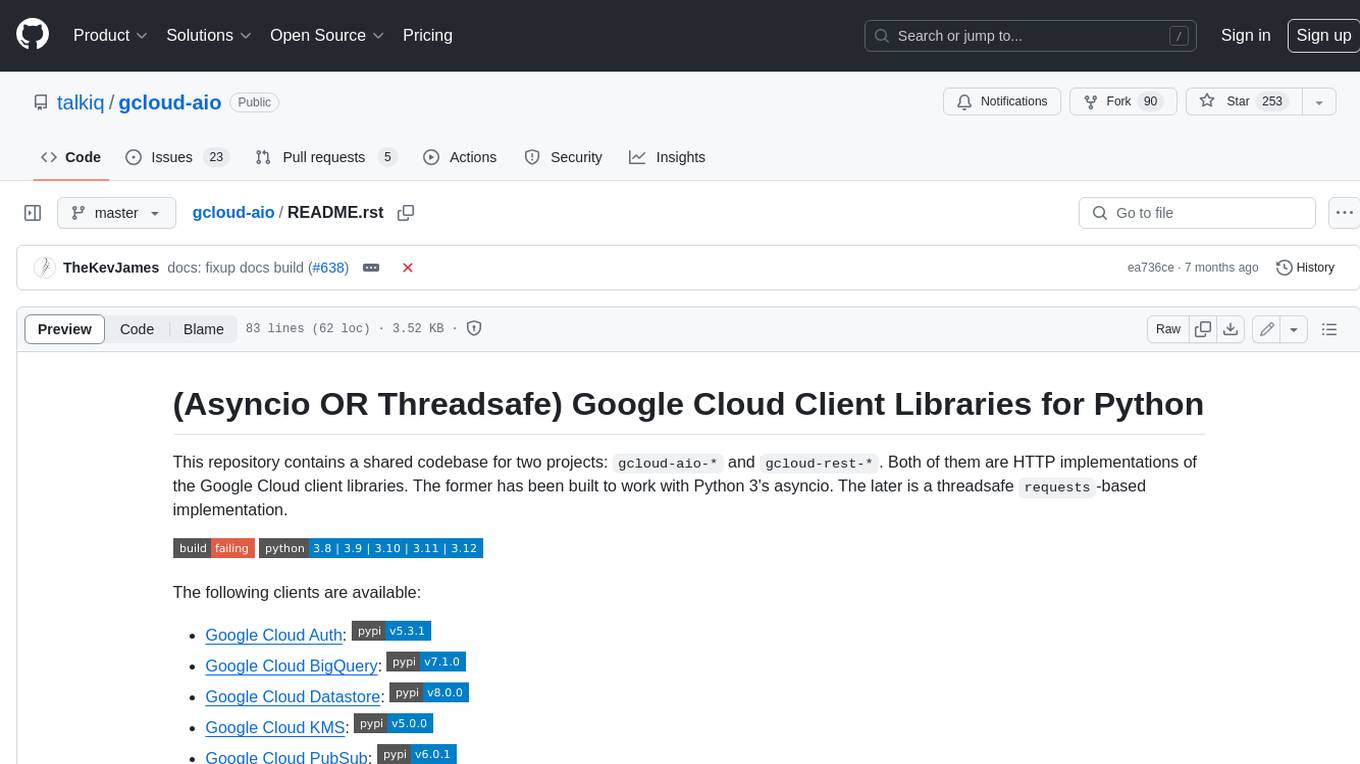
gcloud-aio
This repository contains shared codebase for two projects: gcloud-aio and gcloud-rest. gcloud-aio is built for Python 3's asyncio, while gcloud-rest is a threadsafe requests-based implementation. It provides clients for Google Cloud services like Auth, BigQuery, Datastore, KMS, PubSub, Storage, and Task Queue. Users can install the library using pip and refer to the documentation for usage details. Developers can contribute to the project by following the contribution guide.
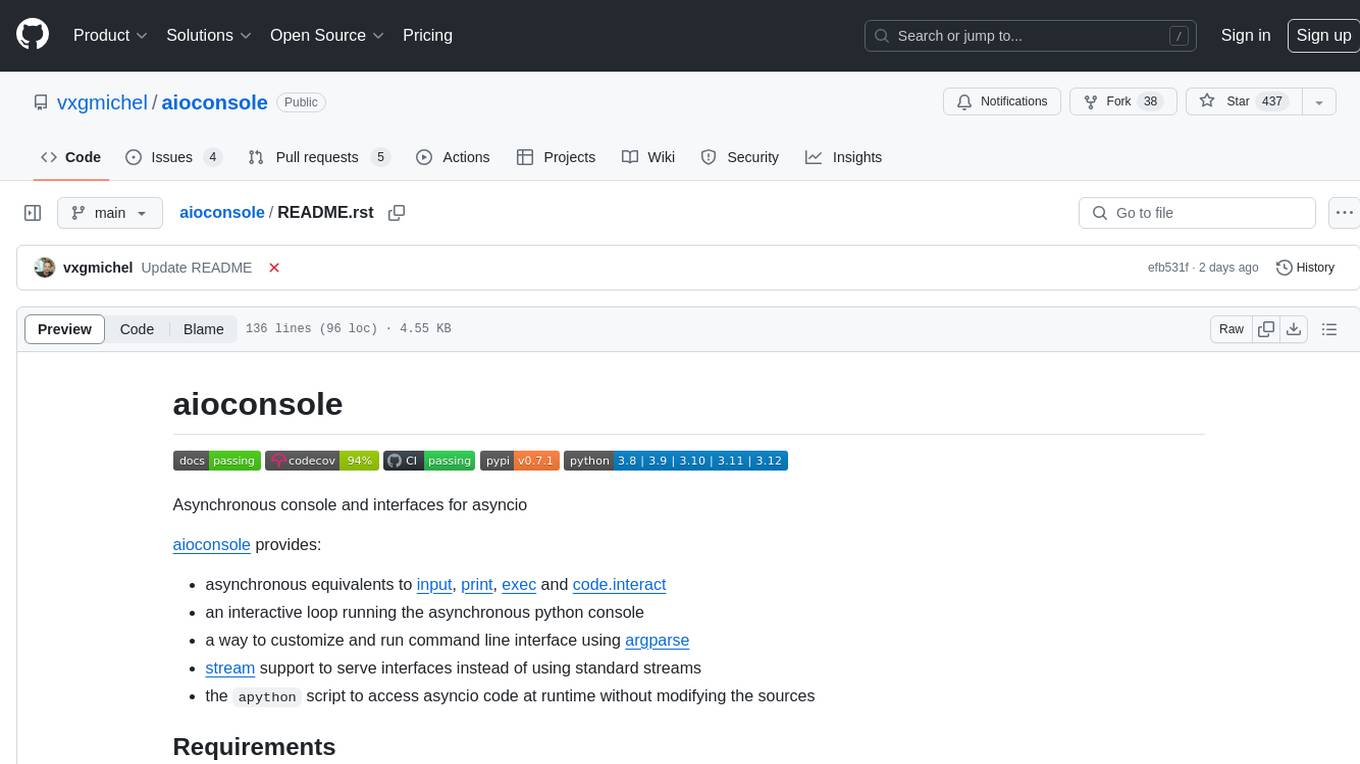
aioconsole
aioconsole is a Python package that provides asynchronous console and interfaces for asyncio. It offers asynchronous equivalents to input, print, exec, and code.interact, an interactive loop running the asynchronous Python console, customization and running of command line interfaces using argparse, stream support to serve interfaces instead of using standard streams, and the apython script to access asyncio code at runtime without modifying the sources. The package requires Python version 3.8 or higher and can be installed from PyPI or GitHub. It allows users to run Python files or modules with a modified asyncio policy, replacing the default event loop with an interactive loop. aioconsole is useful for scenarios where users need to interact with asyncio code in a console environment.
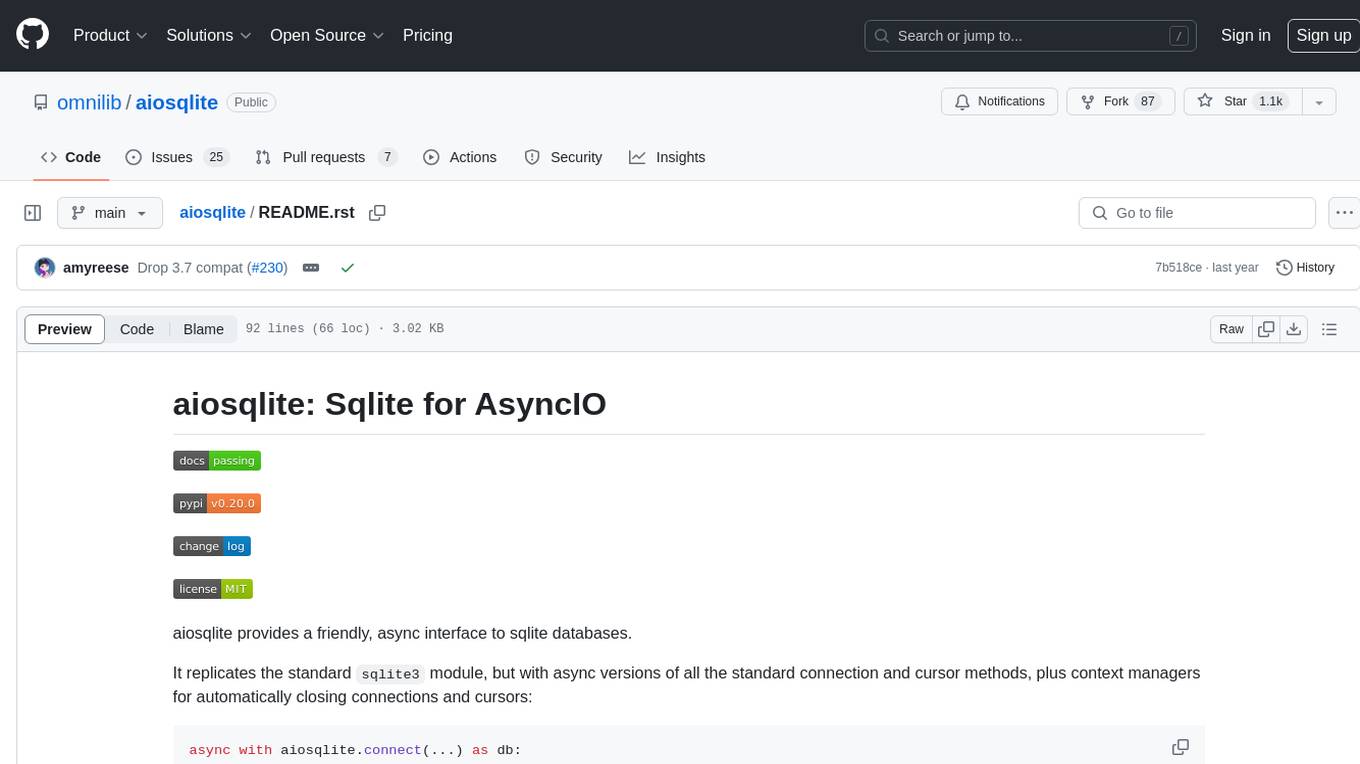
aiosqlite
aiosqlite is a Python library that provides a friendly, async interface to SQLite databases. It replicates the standard sqlite3 module but with async versions of all the standard connection and cursor methods, along with context managers for automatically closing connections and cursors. It allows interaction with SQLite databases on the main AsyncIO event loop without blocking execution of other coroutines while waiting for queries or data fetches. The library also replicates most of the advanced features of sqlite3, such as row factories and total changes tracking.