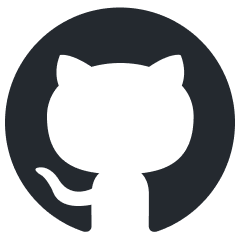
amadeus-python
Python library for the Amadeus Self-Service travel APIs
Stars: 212
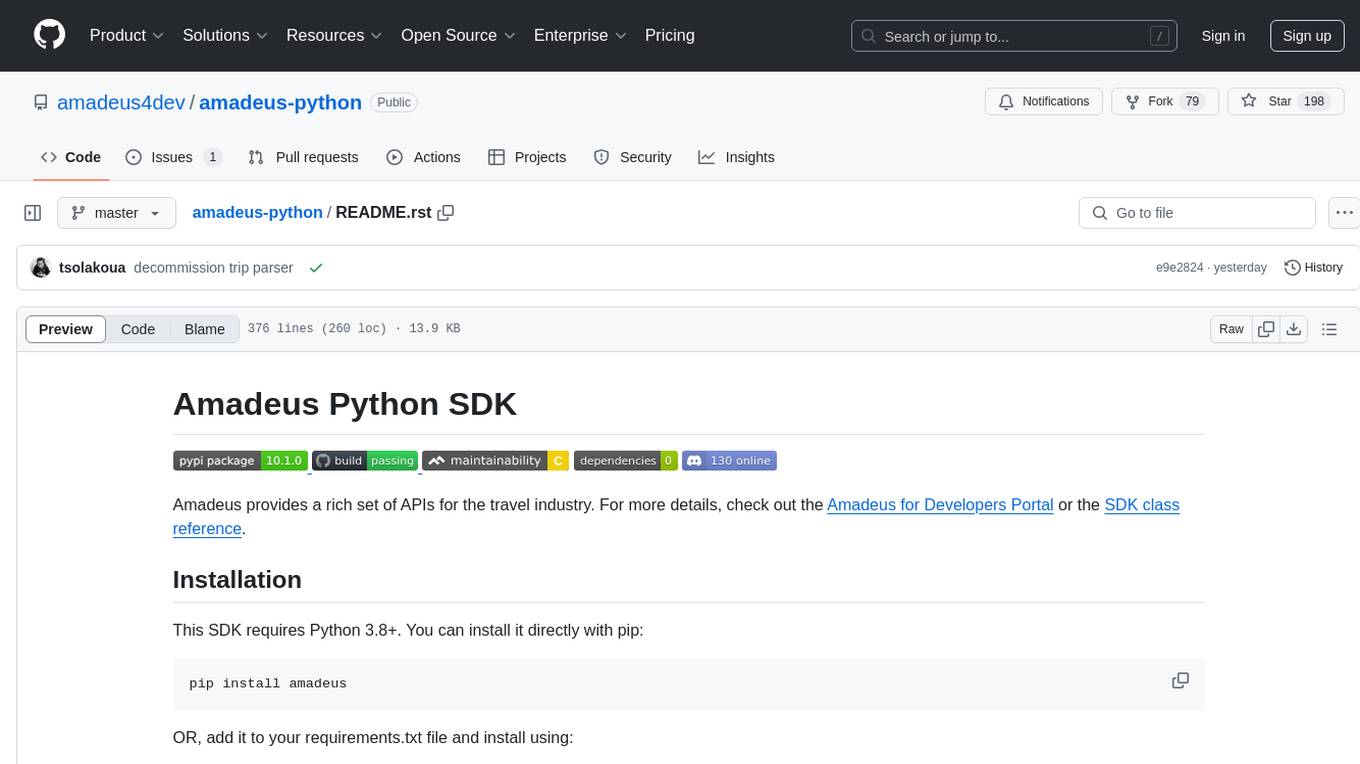
Amadeus Python SDK provides a rich set of APIs for the travel industry. It allows users to make API calls for various travel-related tasks such as flight offers search, hotel bookings, trip purpose prediction, flight delay prediction, airport on-time performance, travel recommendations, and more. The SDK conveniently maps API paths to similar paths, making it easy to interact with the Amadeus APIs. Users can initialize the client with their API key and secret, make API calls, handle responses, and enable logging for debugging purposes. The SDK documentation includes detailed information about each SDK method, arguments, and return types.
README:
|Module Version| |Build Status| |Maintainability| |Dependencies| |Discord|
Amadeus provides a rich set of APIs for the travel industry. For more details, check out the Amadeus for Developers Portal <https://developers.amadeus.com>
__ or the SDK class reference <https://amadeus4dev.github.io/amadeus-python>
__.
This SDK requires Python 3.8+. You can install it directly with pip:
.. code:: sh
pip install amadeus
OR, add it to your requirements.txt
file and install using:
.. code:: sh
pip install -r requirements.txt
To make your first API call, you will need to register <https://developers.amadeus.com/register>
__ for an Amadeus Developer Account and set up your first application <https://developers.amadeus.com/my-apps/>
__.
.. code:: py
from amadeus import Client, ResponseError
amadeus = Client(
client_id='REPLACE_BY_YOUR_API_KEY',
client_secret='REPLACE_BY_YOUR_API_SECRET'
)
try:
response = amadeus.shopping.flight_offers_search.get(
originLocationCode='MAD',
destinationLocationCode='ATH',
departureDate='2024-11-01',
adults=1)
print(response.data)
except ResponseError as error:
print(error)
You can find all the endpoints in self-contained code examples <https://github.com/amadeus4dev/amadeus-code-examples>
_.
The client can be initialized directly.
.. code:: py
# Initialize using parameters
amadeus = Client(client_id='REPLACE_BY_YOUR_API_KEY', client_secret='REPLACE_BY_YOUR_API_SECRET')
Alternatively, it can be initialized without any parameters if the environment variables AMADEUS_CLIENT_ID
and AMADEUS_CLIENT_SECRET
are present.
.. code:: py
amadeus = Client()
Your credentials can be found on the Amadeus dashboard <https://developers.amadeus.com/my-apps/>
__.
By default, the SDK environment is set to test
environment. To switch to a production (pay-as-you-go) environment, please switch the hostname as follows:
.. code:: py
amadeus = Client(hostname='production')
Amadeus has a large set of APIs, and our documentation is here to get you started today. Head over to our reference documentation <https://amadeus4dev.github.io/amadeus-python/>
__ for in-depth information about every SDK method, as well as its arguments and return types.
-
Initialize the SDK <https://amadeus4dev.github.io/amadeus-python/#/client>
__ -
Find an Airport <https://amadeus4dev.github.io/amadeus-python/#amadeus.reference_data.locations.Airports>
__ -
Find a Flight <https://amadeus4dev.github.io/amadeus-python/#amadeus.shopping.FlightOffersSearch>
__ -
Get Flight Inspiration <https://amadeus4dev.github.io/amadeus-python/#shopping-flights>
__
This library conveniently maps every API path to a similar path.
For example, GET /v2/reference-data/urls/checkin-links?airlineCode=BA
would be:
.. code:: py
amadeus.reference_data.urls.checkin_links.get(airlineCode='BA')
Similarly, to select a resource by ID, you can pass in the ID to the singular path.
For example, GET /v2/shopping/hotel-offers/XZY
would be:
.. code:: py
amadeus.shopping.hotel_offer('XYZ').get()
You can make any arbitrary API call directly with the .get
method as well:
.. code:: py
amadeus.get('/v2/reference-data/urls/checkin-links', airlineCode='BA')
Or, with POST
method:
.. code:: py
amadeus.post('/v1/shopping/flight-offers/pricing', body)
Every API call returns a Response
object. If the API call contained a JSON response it will parse the JSON into the .result
attribute. If this data also contains a data
key, it will make that available as the .data
attribute. The raw body of the response is always available as the .body
attribute.
.. code:: py
from amadeus import Location
response = amadeus.reference_data.locations.get(
keyword='LON',
subType=Location.ANY
)
print(response.body) #=> The raw response, as a string
print(response.result) #=> The body parsed as JSON, if the result was parsable
print(response.data) #=> The list of locations, extracted from the JSON
If an API endpoint supports pagination, the other pages are available under the .next
, .previous
, .last
and .first
methods.
.. code:: py
from amadeus import Location
response = amadeus.reference_data.locations.get(
keyword='LON',
subType=Location.ANY
)
amadeus.next(response) #=> returns a new response for the next page
If a page is not available, the method will return None
.
The SDK makes it easy to add your own logger.
.. code:: py
import logging
logger = logging.getLogger('your_logger')
logger.setLevel(logging.DEBUG)
amadeus = Client(
client_id='REPLACE_BY_YOUR_API_KEY',
client_secret='REPLACE_BY_YOUR_API_SECRET',
logger=logger
)
Additionally, to enable more verbose logging, you can set the appropriate level on your own logger. The easiest way would be to enable debugging via a parameter during initialization, or using the AMADEUS_LOG_LEVEL
environment variable.
.. code:: py
amadeus = Client(
client_id='REPLACE_BY_YOUR_API_KEY',
client_secret='REPLACE_BY_YOUR_API_SECRET',
log_level='debug'
)
.. code:: py
# Flight Inspiration Search
amadeus.shopping.flight_destinations.get(origin='MAD')
# Flight Cheapest Date Search
amadeus.shopping.flight_dates.get(origin='MAD', destination='MUC')
# Flight Offers Search GET
amadeus.shopping.flight_offers_search.get(originLocationCode='SYD', destinationLocationCode='BKK', departureDate='2022-11-01', adults=1)
# Flight Offers Search POST
amadeus.shopping.flight_offers_search.post(body)
# Flight Offers Price
flights = amadeus.shopping.flight_offers_search.get(originLocationCode='SYD', destinationLocationCode='BKK', departureDate='2022-11-01', adults=1).data
amadeus.shopping.flight_offers.pricing.post(flights[0])
amadeus.shopping.flight_offers.pricing.post(flights[0:2], include='credit-card-fees,other-services')
# Flight Create Orders
amadeus.booking.flight_orders.post(flights[0], traveler)
# Flight Order Management
# The flight ID comes from the Flight Create Orders (in test environment it's temporary)
# Retrieve the order based on it's ID
flight_booking = amadeus.booking.flight_orders.post(body).data
amadeus.booking.flight_order(flight_booking['id']).get()
# Delete the order based on it's ID
amadeus.booking.flight_order(flight_booking['id']).delete()
# Flight SeatMap Display GET
amadeus.shopping.seatmaps.get(**{"flight-orderId": "orderid"})
# Flight SeatMap Display POST
amadeus.shopping.seatmaps.post(body)
# Flight Availabilities POST
amadeus.shopping.availability.flight_availabilities.post(body)
# Branded Fares Upsell
amadeus.shopping.flight_offers.upselling.post(body)
# Flight Choice Prediction
body = amadeus.shopping.flight_offers_search.get(
originLocationCode='MAD',
destinationLocationCode='NYC',
departureDate='2022-11-01',
adults=1).result
amadeus.shopping.flight_offers.prediction.post(body)
# Flight Checkin Links
amadeus.reference_data.urls.checkin_links.get(airlineCode='BA')
# Airline Code Lookup
amadeus.reference_data.airlines.get(airlineCodes='U2')
# Airport and City Search (autocomplete)
# Find all the cities and airports starting by 'LON'
amadeus.reference_data.locations.get(keyword='LON', subType=Location.ANY)
# Get a specific city or airport based on its id
amadeus.reference_data.location('ALHR').get()
# City Search
amadeus.reference_data.locations.cities.get(keyword='PAR')
# Airport Nearest Relevant Airport (for London)
amadeus.reference_data.locations.airports.get(longitude=0.1278, latitude=51.5074)
# Flight Most Booked Destinations
amadeus.travel.analytics.air_traffic.booked.get(originCityCode='MAD', period='2017-08')
# Flight Most Traveled Destinations
amadeus.travel.analytics.air_traffic.traveled.get(originCityCode='MAD', period='2017-01')
# Flight Busiest Travel Period
amadeus.travel.analytics.air_traffic.busiest_period.get(cityCode='MAD', period='2017', direction='ARRIVING')
# Hotel Search v3
# Get list of available offers by hotel ids
amadeus.shopping.hotel_offers_search.get(hotelIds='RTPAR001', adults='2')
# Check conditions of a specific offer
amadeus.shopping.hotel_offer_search('XXX').get()
# Hotel List
# Get list of hotels by hotel id
amadeus.reference_data.locations.hotels.by_hotels.get(hotelIds='ADPAR001')
# Get list of hotels by city code
amadeus.reference_data.locations.hotels.by_city.get(cityCode='PAR')
# Get list of hotels by a geocode
amadeus.reference_data.locations.hotels.by_geocode.get(longitude=2.160873,latitude=41.397158)
# Hotel Name Autocomplete
amadeus.reference_data.locations.hotel.get(keyword='PARI', subType=[Hotel.HOTEL_GDS, Hotel.HOTEL_LEISURE])
# Hotel Booking v2
# The offerId comes from the hotel_offer above
amadeus.booking.hotel_orders.post(
guests=guests,
travel_agent=travel_agent,
room_associations=room_associations,
payment=payment)
# Hotel Booking v1
# The offerId comes from the hotel_offer above
amadeus.booking.hotel_bookings.post(offerId, guests, payments)
# Hotel Ratings
# What travelers think about this hotel?
amadeus.e_reputation.hotel_sentiments.get(hotelIds = 'ADNYCCTB')
# Location Score
amadeus.location.analytics.category_rated_areas.get(latitude=41.397158, longitude=2.160873)
# Trip Purpose Prediction
amadeus.travel.predictions.trip_purpose.get(originLocationCode='ATH', destinationLocationCode='MAD', departureDate='2022-11-01', returnDate='2022-11-08')
# Flight Delay Prediction
amadeus.travel.predictions.flight_delay.get(originLocationCode='NCE', destinationLocationCode='IST', departureDate='2022-08-01', \
departureTime='18:20:00', arrivalDate='2022-08-01', arrivalTime='22:15:00', aircraftCode='321', carrierCode='TK', flightNumber='1816', duration='PT31H10M')
# Airport On-Time Performance
amadeus.airport.predictions.on_time.get(airportCode='JFK', date='2022-11-01')
# Airport Routes
amadeus.airport.direct_destinations.get(departureAirportCode='BLR')
# Travel Recommendations
amadeus.reference_data.recommended_locations.get(cityCodes='PAR', travelerCountryCode='FR')
# Retrieve status of a given flight
amadeus.schedule.flights.get(carrierCode='AZ', flightNumber='319', scheduledDepartureDate='2022-09-13')
# Tours and Activities
# What are the popular activities in Madrid (based a geo location and a radius)
amadeus.shopping.activities.get(latitude=40.41436995, longitude=-3.69170868)
# What are the popular activities in Barcelona? (based on a square)
amadeus.shopping.activities.by_square.get(north=41.397158, west=2.160873,
south=41.394582, east=2.177181)
# Returns a single activity from a given id
amadeus.shopping.activity('4615').get()
# Returns itinerary price metrics
amadeus.analytics.itinerary_price_metrics.get(originIataCode='MAD', destinationIataCode='CDG',
departureDate='2021-03-21')
# Airline Routes
amadeus.airline.destinations.get(airlineCode='BA')
# Transfer Search
amadeus.shopping.transfer_offers.post(body)
# Transfer Booking
amadeus.ordering.transfer_orders.post(body, offerId='1000000000')
# Transfer Management
amadeus.ordering.transfer_order('ABC').transfers.cancellation.post(body, confirmNbr=123)
Want to contribute? Read our Contributors Guide <.github/CONTRIBUTING.md>
__ for guidance on installing and
running this code in a development environment.
This library is released under the MIT License <LICENSE>
__.
You can find us on StackOverflow <https://stackoverflow.com/questions/tagged/amadeus>
__ or join our developer community on Discord <https://discord.gg/cVrFBqx>
__.
.. |Module Version| image:: https://badge.fury.io/py/amadeus.svg :target: https://pypi.org/project/amadeus/ .. |Build Status| image:: https://github.com/amadeus4dev/amadeus-python/actions/workflows/build.yml/badge.svg :target: https://github.com/amadeus4dev/amadeus-python/actions/workflows/build.yml .. |Maintainability| image:: https://api.codeclimate.com/v1/badges/c2e19cf9628d6f4aece2/maintainability :target: https://codeclimate.com/github/amadeus4dev/amadeus-python/maintainability .. |Dependencies| image:: https://raw.githubusercontent.com/amadeus4dev/amadeus-python/master/.github/images/dependencies.svg?sanitize=true :target: https://badge.fury.io/py/amadeus .. |Discord| image:: https://img.shields.io/discord/696822960023011329?label=&logo=discord&logoColor=ffffff&color=7389D8&labelColor=6A7EC2 :target: https://discord.gg/cVrFBqx
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for amadeus-python
Similar Open Source Tools
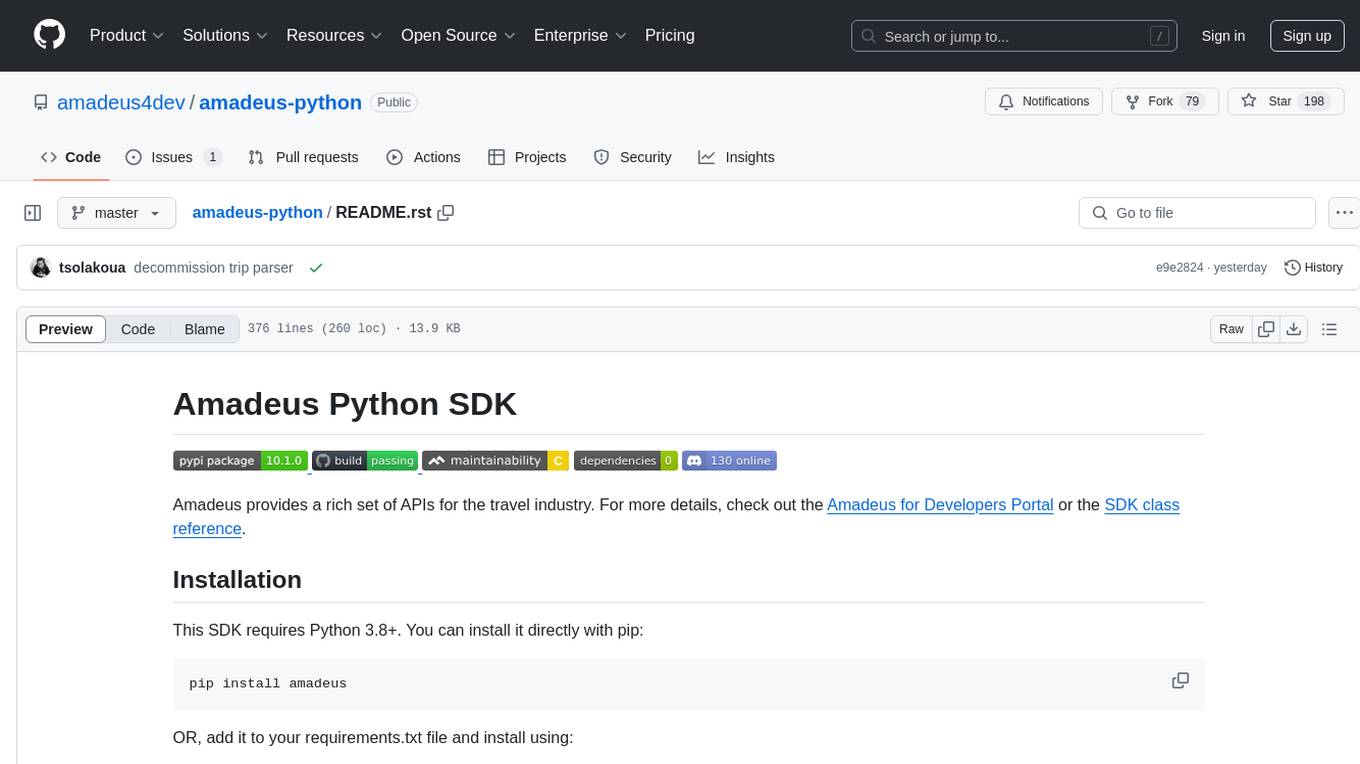
amadeus-python
Amadeus Python SDK provides a rich set of APIs for the travel industry. It allows users to make API calls for various travel-related tasks such as flight offers search, hotel bookings, trip purpose prediction, flight delay prediction, airport on-time performance, travel recommendations, and more. The SDK conveniently maps API paths to similar paths, making it easy to interact with the Amadeus APIs. Users can initialize the client with their API key and secret, make API calls, handle responses, and enable logging for debugging purposes. The SDK documentation includes detailed information about each SDK method, arguments, and return types.
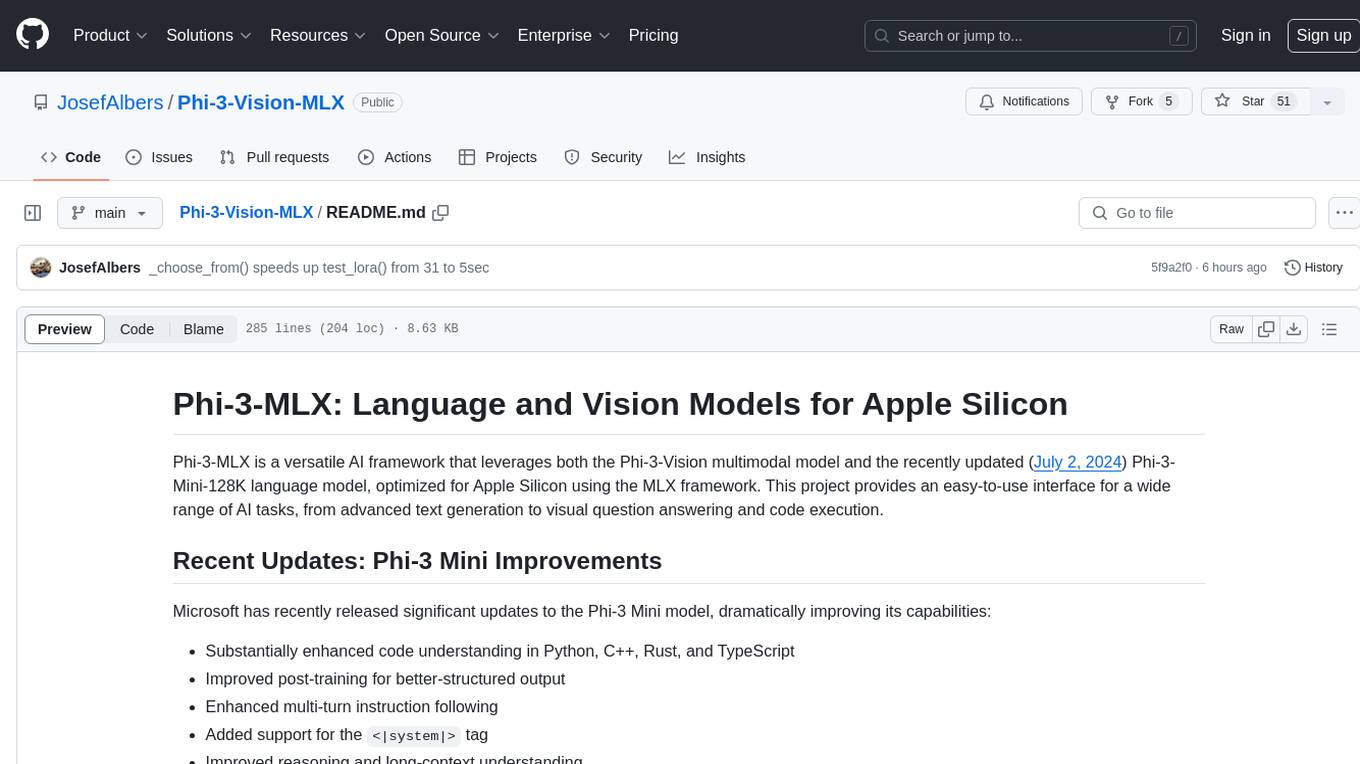
Phi-3-Vision-MLX
Phi-3-MLX is a versatile AI framework that leverages both the Phi-3-Vision multimodal model and the Phi-3-Mini-128K language model optimized for Apple Silicon using the MLX framework. It provides an easy-to-use interface for a wide range of AI tasks, from advanced text generation to visual question answering and code execution. The project features support for batched generation, flexible agent system, custom toolchains, model quantization, LoRA fine-tuning capabilities, and API integration for extended functionality.
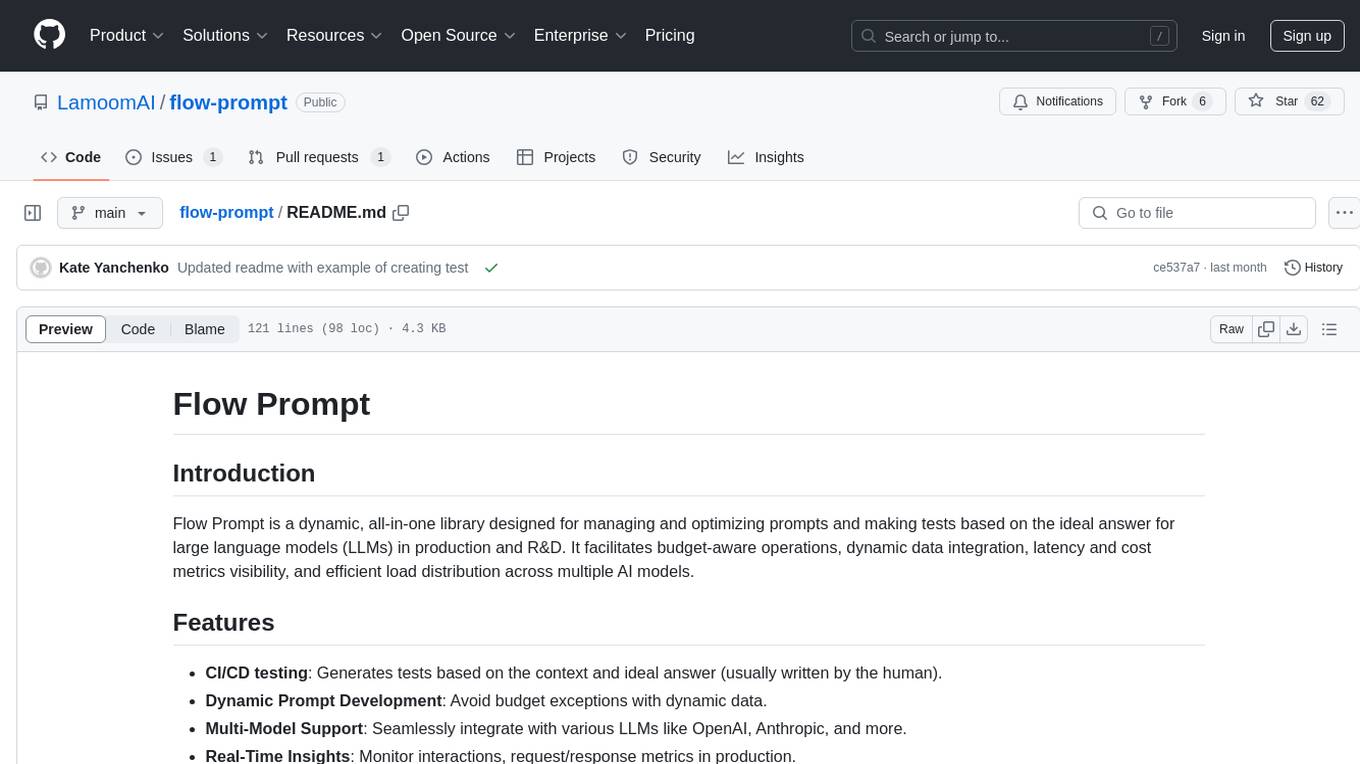
flow-prompt
Flow Prompt is a dynamic library for managing and optimizing prompts for large language models. It facilitates budget-aware operations, dynamic data integration, and efficient load distribution. Features include CI/CD testing, dynamic prompt development, multi-model support, real-time insights, and prompt testing and evolution.
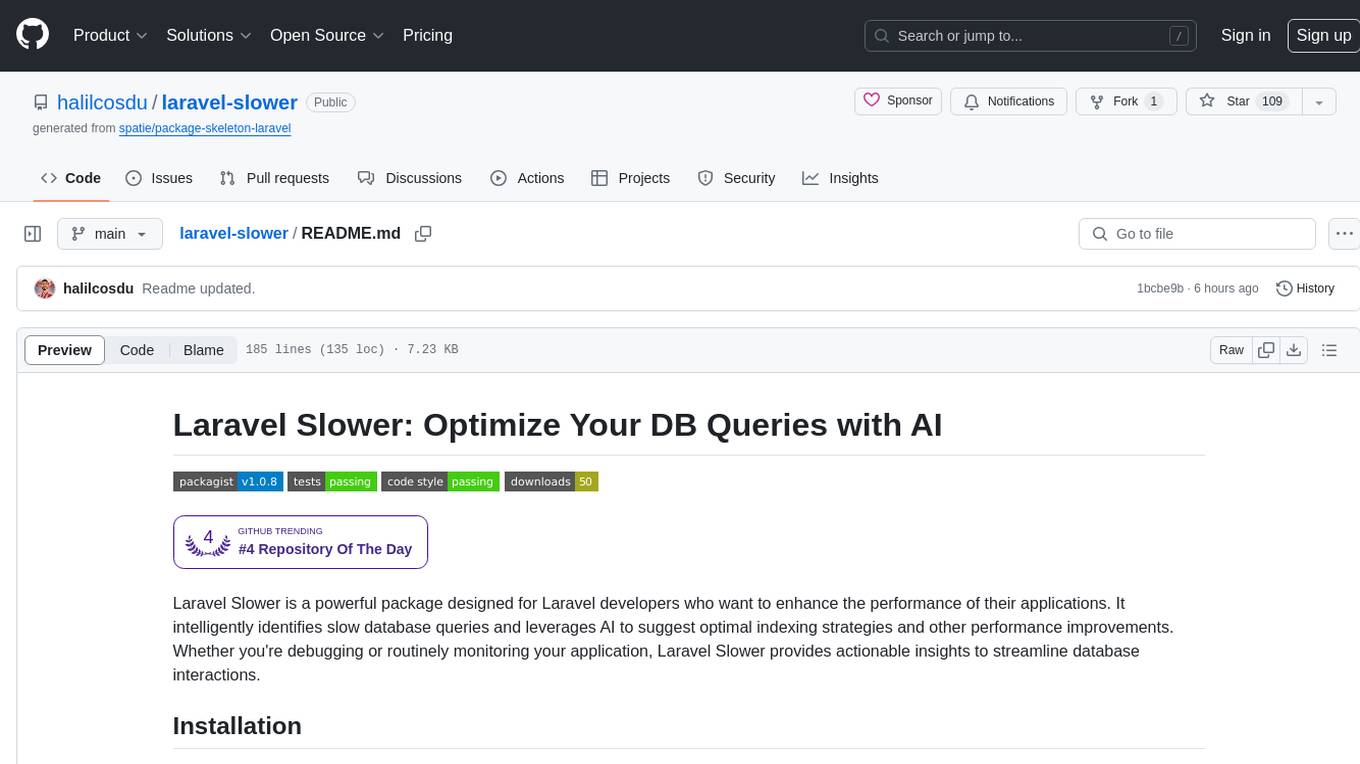
laravel-slower
Laravel Slower is a powerful package designed for Laravel developers to optimize the performance of their applications by identifying slow database queries and providing AI-driven suggestions for optimal indexing strategies and performance improvements. It offers actionable insights for debugging and monitoring database interactions, enhancing efficiency and scalability.
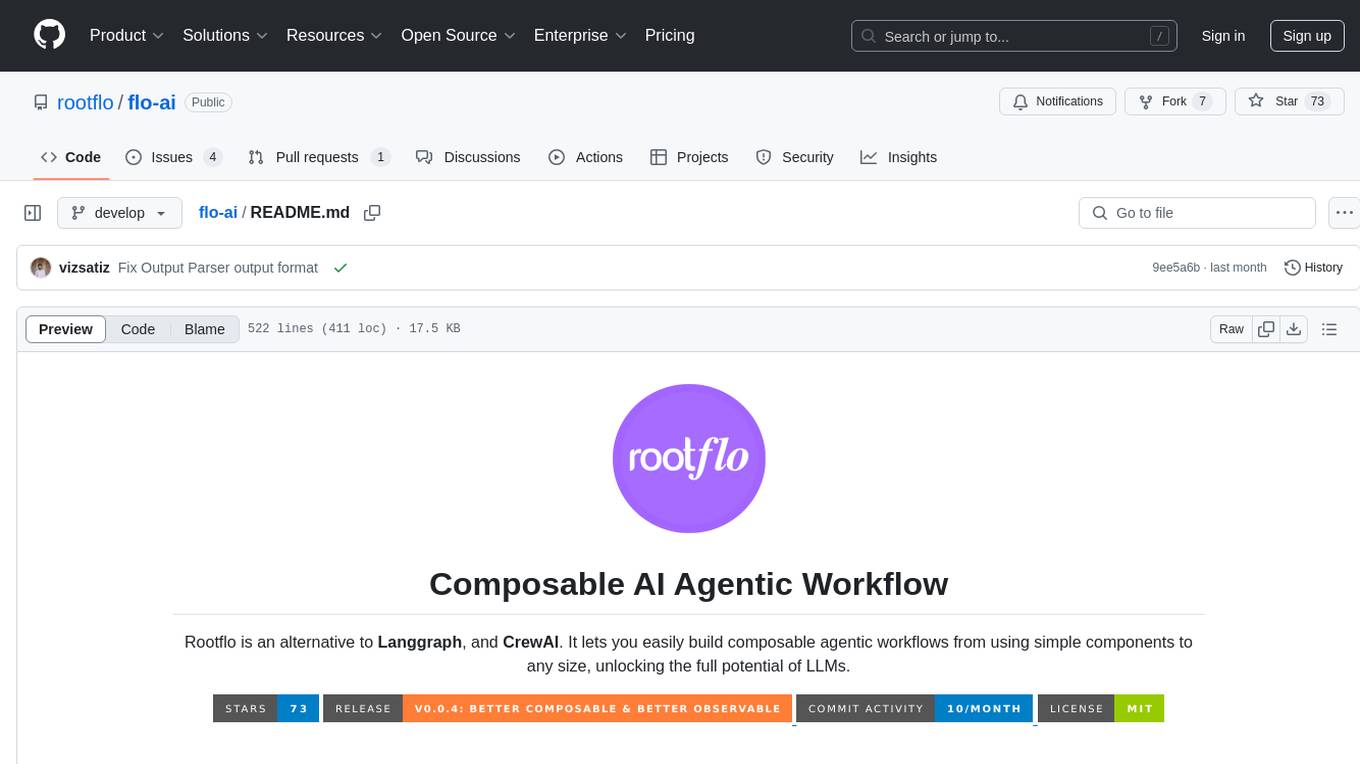
flo-ai
Flo AI is a Python framework that enables users to build production-ready AI agents and teams with minimal code. It allows users to compose complex AI architectures using pre-built components while maintaining the flexibility to create custom components. The framework supports composable, production-ready, YAML-first, and flexible AI systems. Users can easily create AI agents and teams, manage teams of AI agents working together, and utilize built-in support for Retrieval-Augmented Generation (RAG) and compatibility with Langchain tools. Flo AI also provides tools for output parsing and formatting, tool logging, data collection, and JSON output collection. It is MIT Licensed and offers detailed documentation, tutorials, and examples for AI engineers and teams to accelerate development, maintainability, scalability, and testability of AI systems.
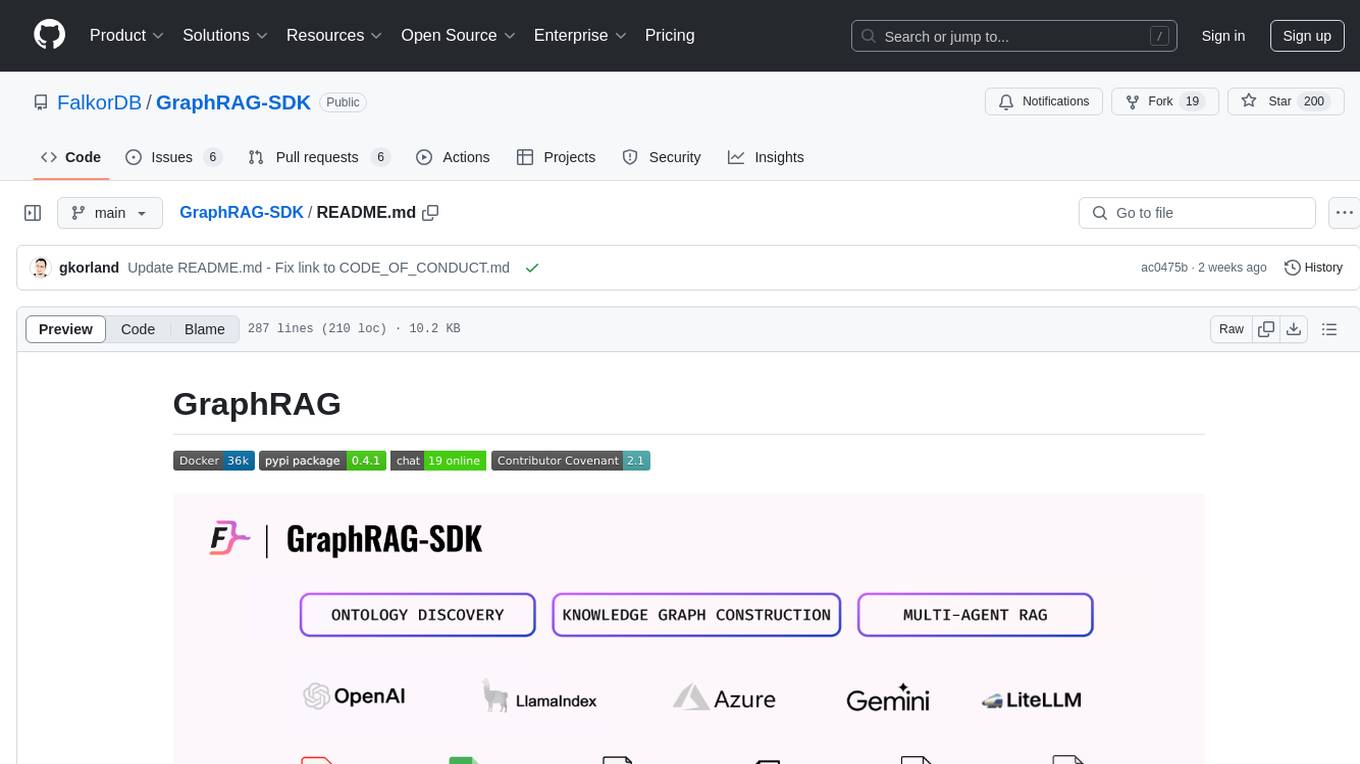
GraphRAG-SDK
Build fast and accurate GenAI applications with GraphRAG SDK, a specialized toolkit for building Graph Retrieval-Augmented Generation (GraphRAG) systems. It integrates knowledge graphs, ontology management, and state-of-the-art LLMs to deliver accurate, efficient, and customizable RAG workflows. The SDK simplifies the development process by automating ontology creation, knowledge graph agent creation, and query handling, enabling users to interact and query their knowledge graphs effectively. It supports multi-agent systems and orchestrates agents specialized in different domains. The SDK is optimized for FalkorDB, ensuring high performance and scalability for large-scale applications. By leveraging knowledge graphs, it enables semantic relationships and ontology-driven queries that go beyond standard vector similarity, enhancing retrieval-augmented generation capabilities.
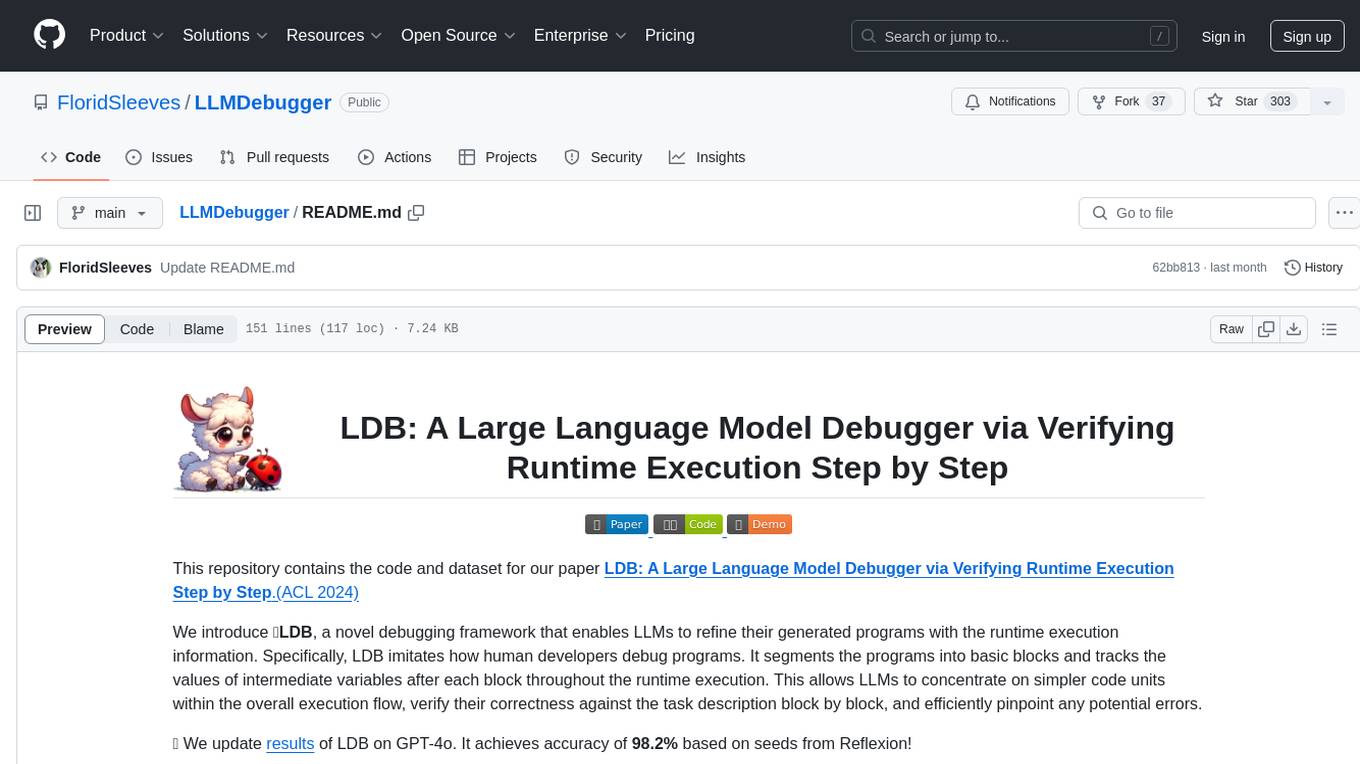
LLMDebugger
This repository contains the code and dataset for LDB, a novel debugging framework that enables Large Language Models (LLMs) to refine their generated programs by tracking the values of intermediate variables throughout the runtime execution. LDB segments programs into basic blocks, allowing LLMs to concentrate on simpler code units, verify correctness block by block, and pinpoint errors efficiently. The tool provides APIs for debugging and generating code with debugging messages, mimicking how human developers debug programs.
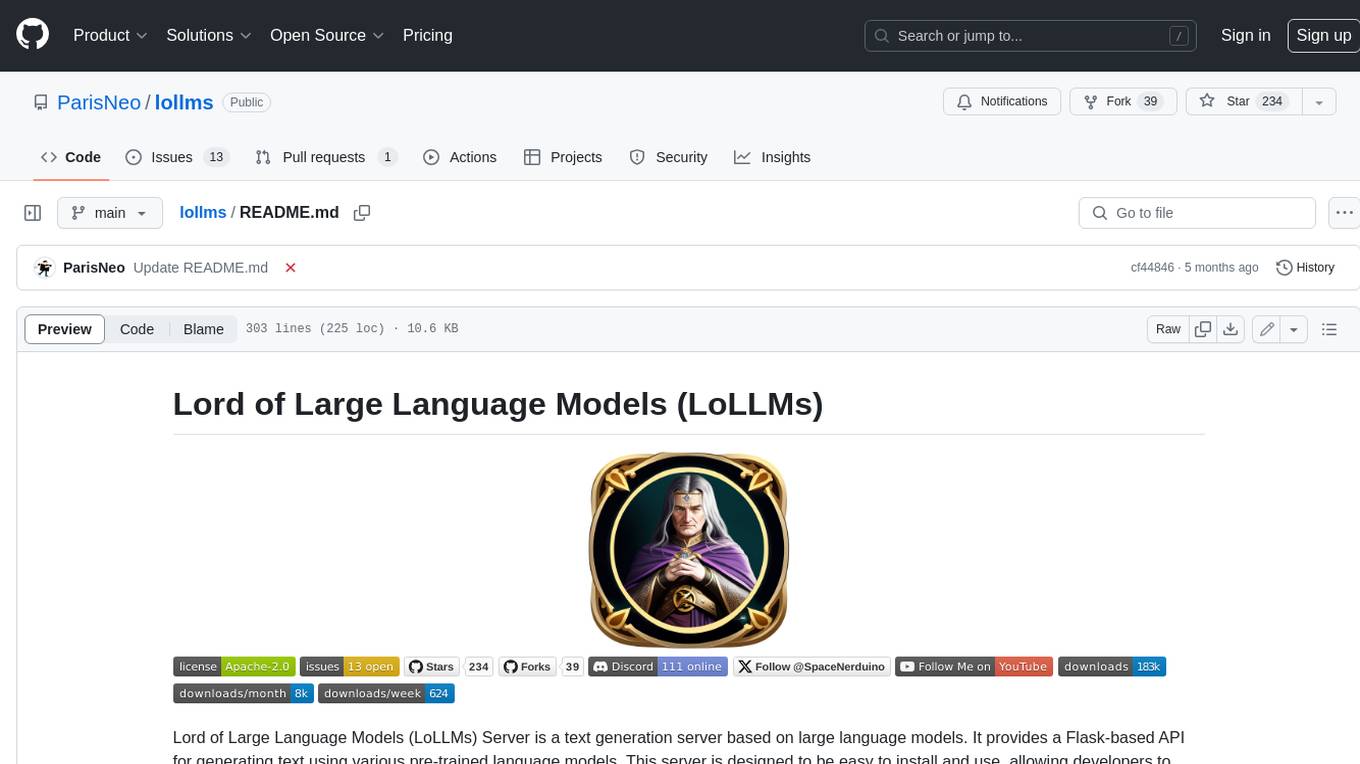
lollms
LoLLMs Server is a text generation server based on large language models. It provides a Flask-based API for generating text using various pre-trained language models. This server is designed to be easy to install and use, allowing developers to integrate powerful text generation capabilities into their applications.
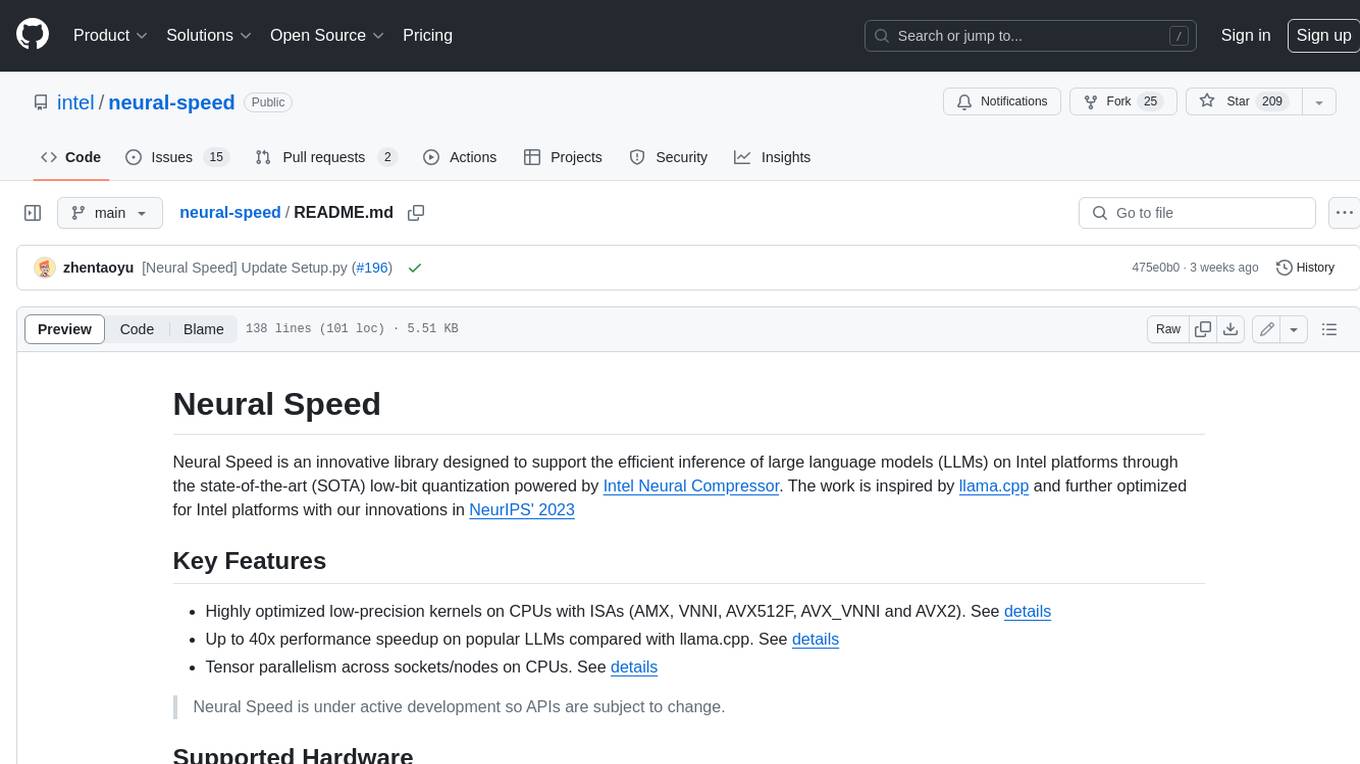
neural-speed
Neural Speed is an innovative library designed to support the efficient inference of large language models (LLMs) on Intel platforms through the state-of-the-art (SOTA) low-bit quantization powered by Intel Neural Compressor. The work is inspired by llama.cpp and further optimized for Intel platforms with our innovations in NeurIPS' 2023
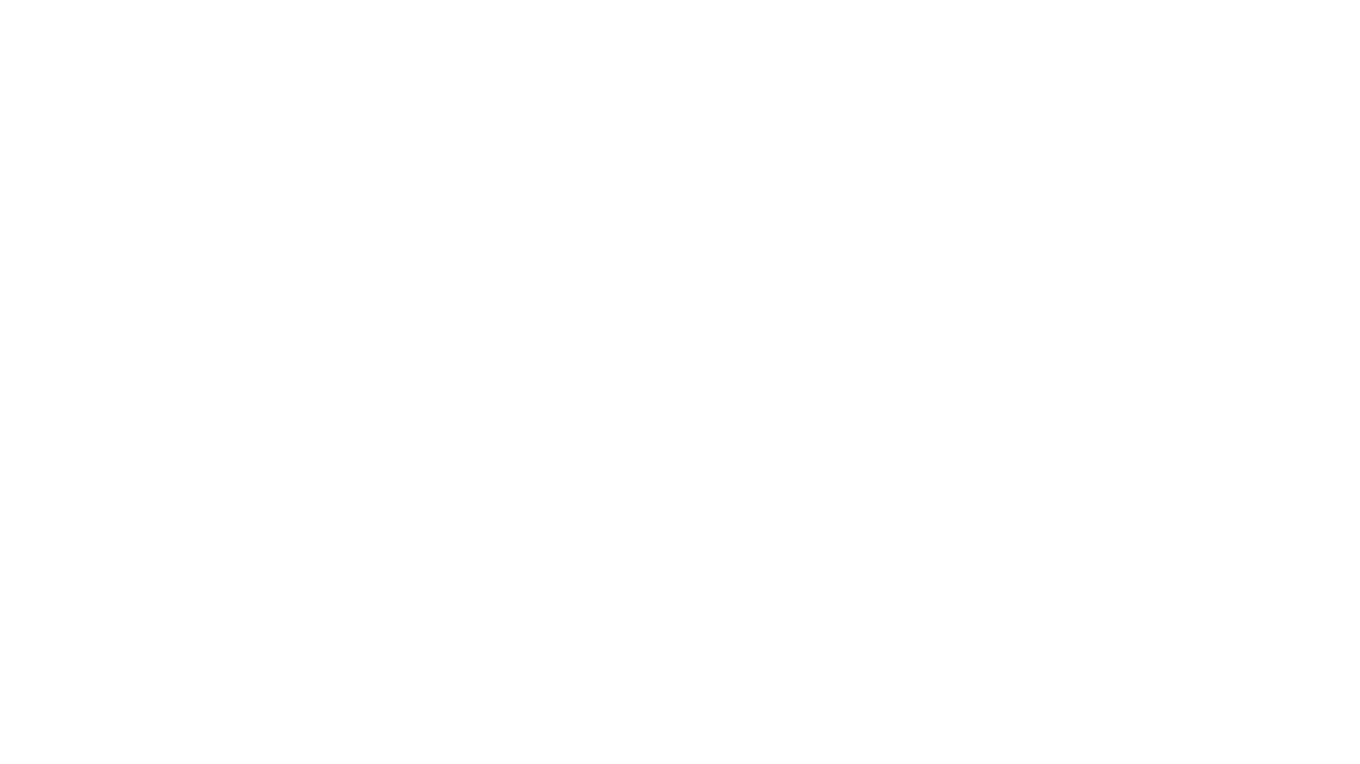
fastrtc
FastRTC is a real-time communication library for Python that allows users to turn any Python function into a real-time audio and video stream over WebRTC or WebSockets. It provides features like automatic voice detection, UI launching, WebRTC support, WebSocket support, telephone support, and customizable backend for production applications. The library offers various examples and usage scenarios for audio and video streaming, object detection, voice APIs, chat applications, and more.
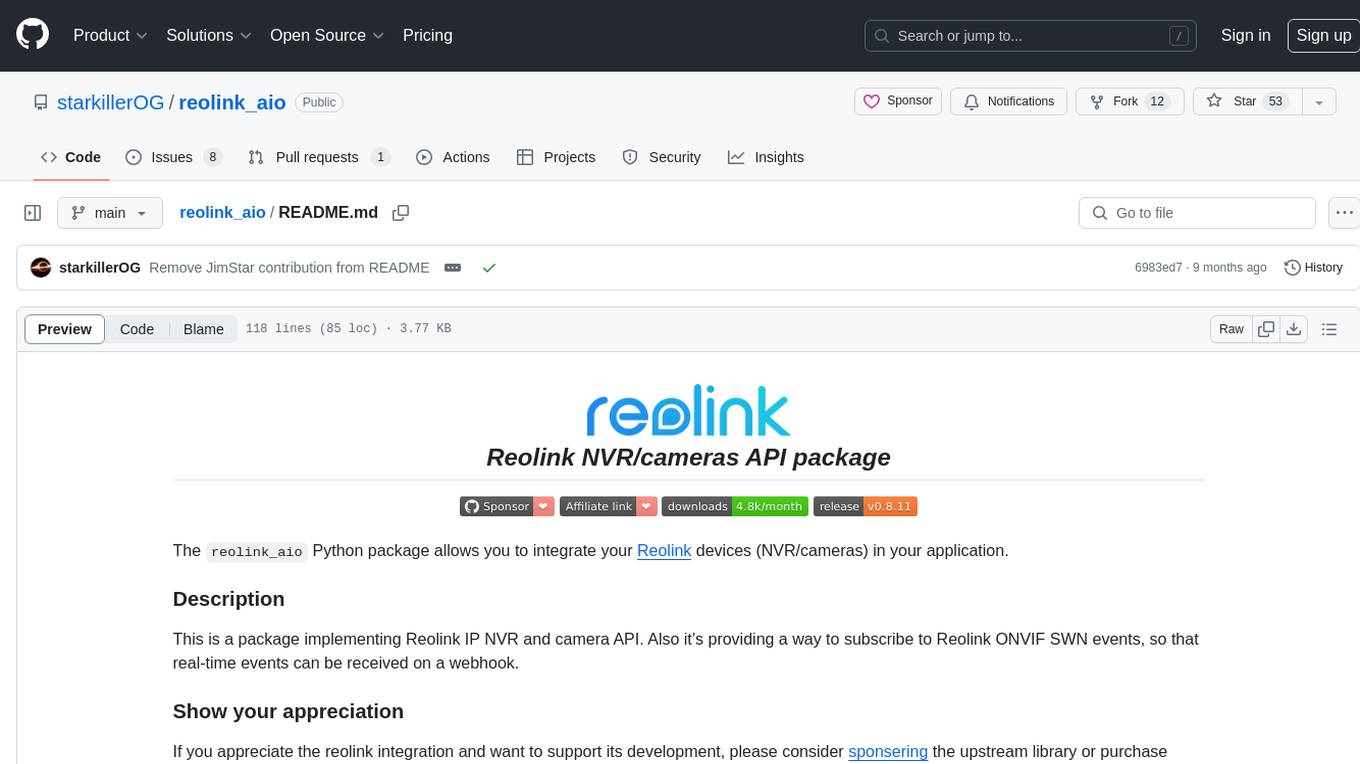
reolink_aio
The 'reolink_aio' Python package is designed to integrate Reolink devices (NVR/cameras) into your application. It implements Reolink IP NVR and camera API, allowing users to subscribe to Reolink ONVIF SWN events for real-time event notifications via webhook. The package provides functionalities to obtain and cache NVR or camera settings, capabilities, and states, as well as enable features like infrared lights, spotlight, and siren. Users can also subscribe to events, renew timers, and disconnect from the host device.
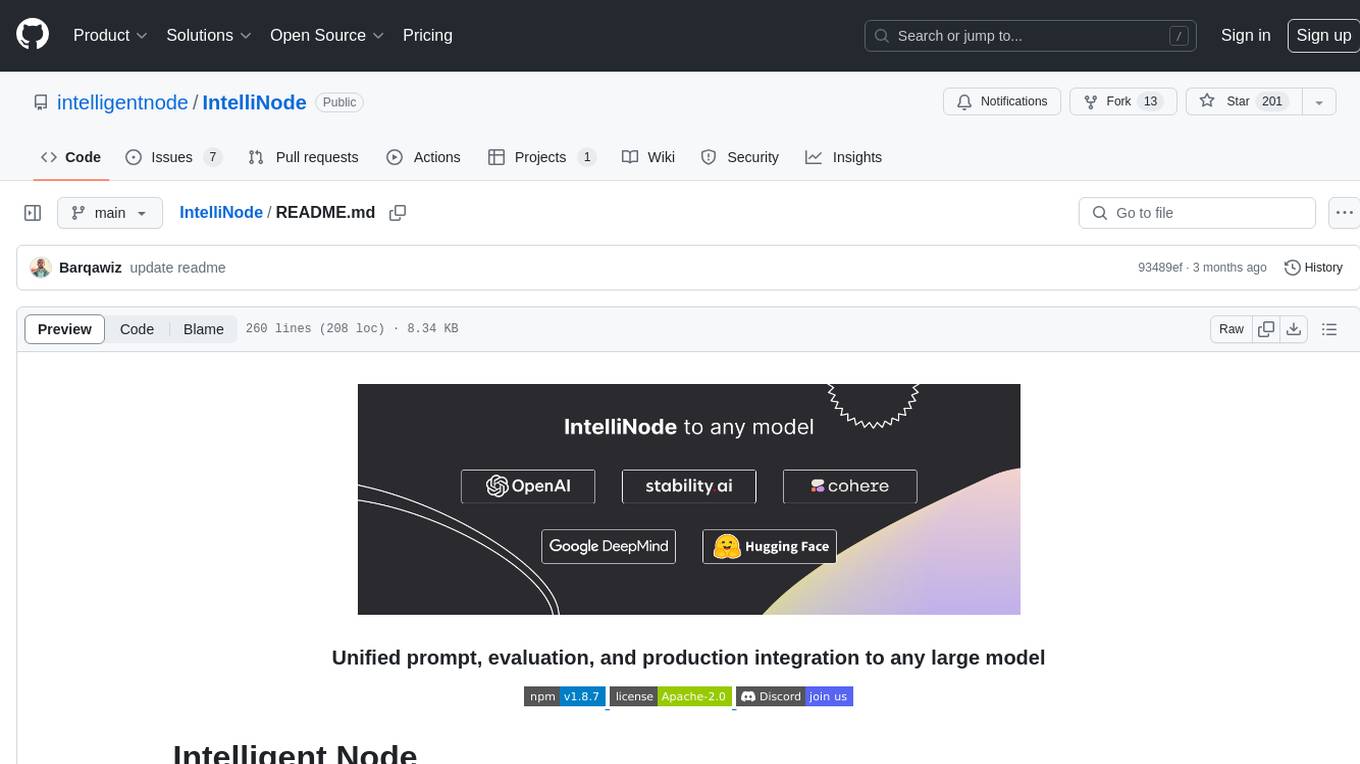
IntelliNode
IntelliNode is a javascript module that integrates cutting-edge AI models like ChatGPT, LLaMA, WaveNet, Gemini, and Stable diffusion into projects. It offers functions for generating text, speech, and images, as well as semantic search, multi-model evaluation, and chatbot capabilities. The module provides a wrapper layer for low-level model access, a controller layer for unified input handling, and a function layer for abstract functionality tailored to various use cases.
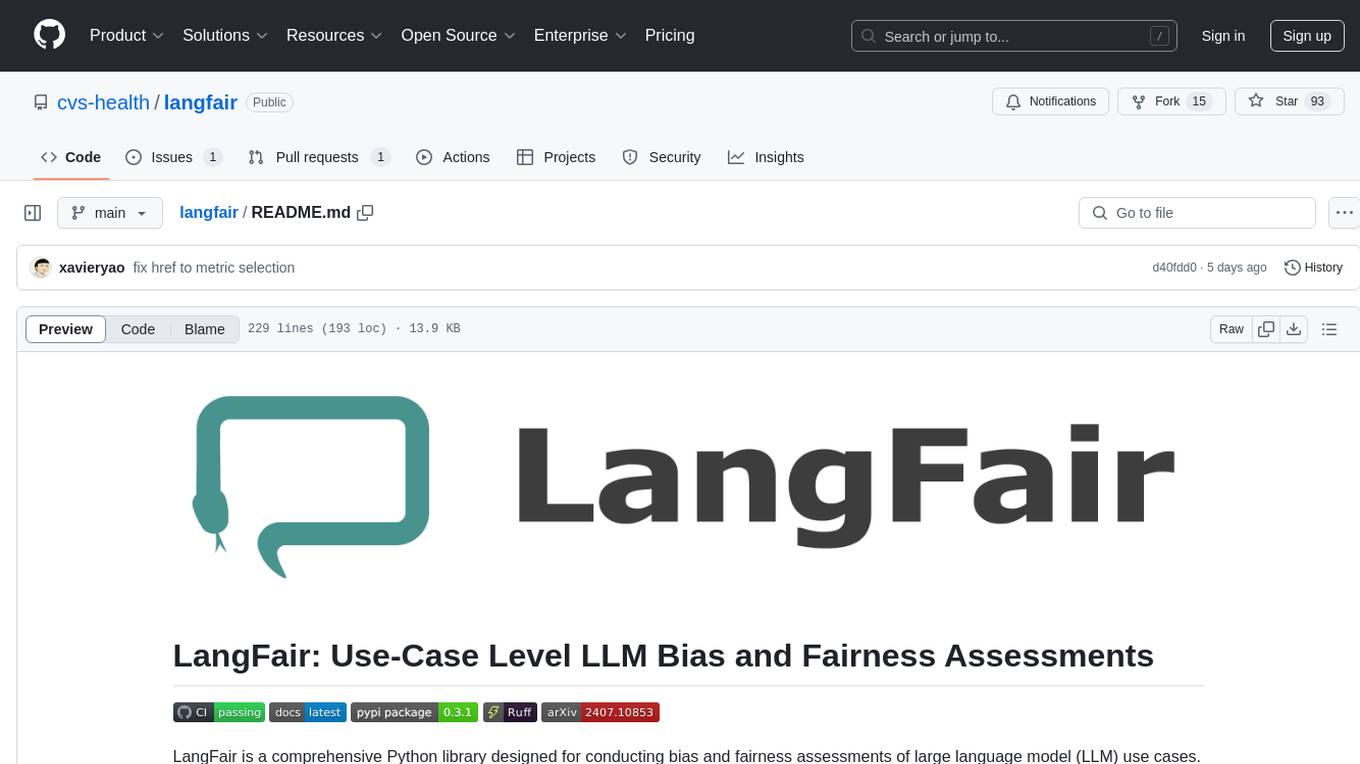
langfair
LangFair is a Python library for bias and fairness assessments of large language models (LLMs). It offers a comprehensive framework for choosing bias and fairness metrics, demo notebooks, and a technical playbook. Users can tailor evaluations to their use cases with a Bring Your Own Prompts approach. The focus is on output-based metrics practical for governance audits and real-world testing.
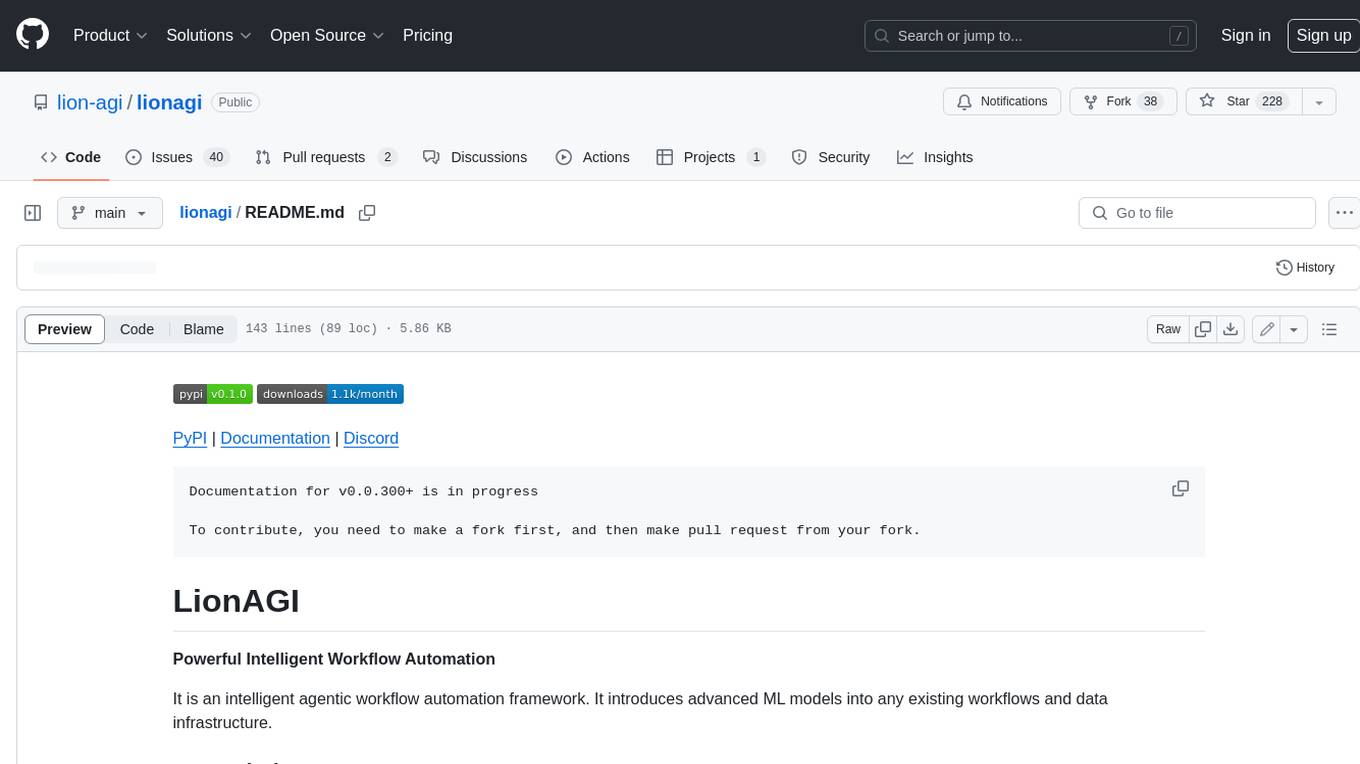
lionagi
LionAGI is a powerful intelligent workflow automation framework that introduces advanced ML models into any existing workflows and data infrastructure. It can interact with almost any model, run interactions in parallel for most models, produce structured pydantic outputs with flexible usage, automate workflow via graph based agents, use advanced prompting techniques, and more. LionAGI aims to provide a centralized agent-managed framework for "ML-powered tools coordination" and to dramatically lower the barrier of entries for creating use-case/domain specific tools. It is designed to be asynchronous only and requires Python 3.10 or higher.
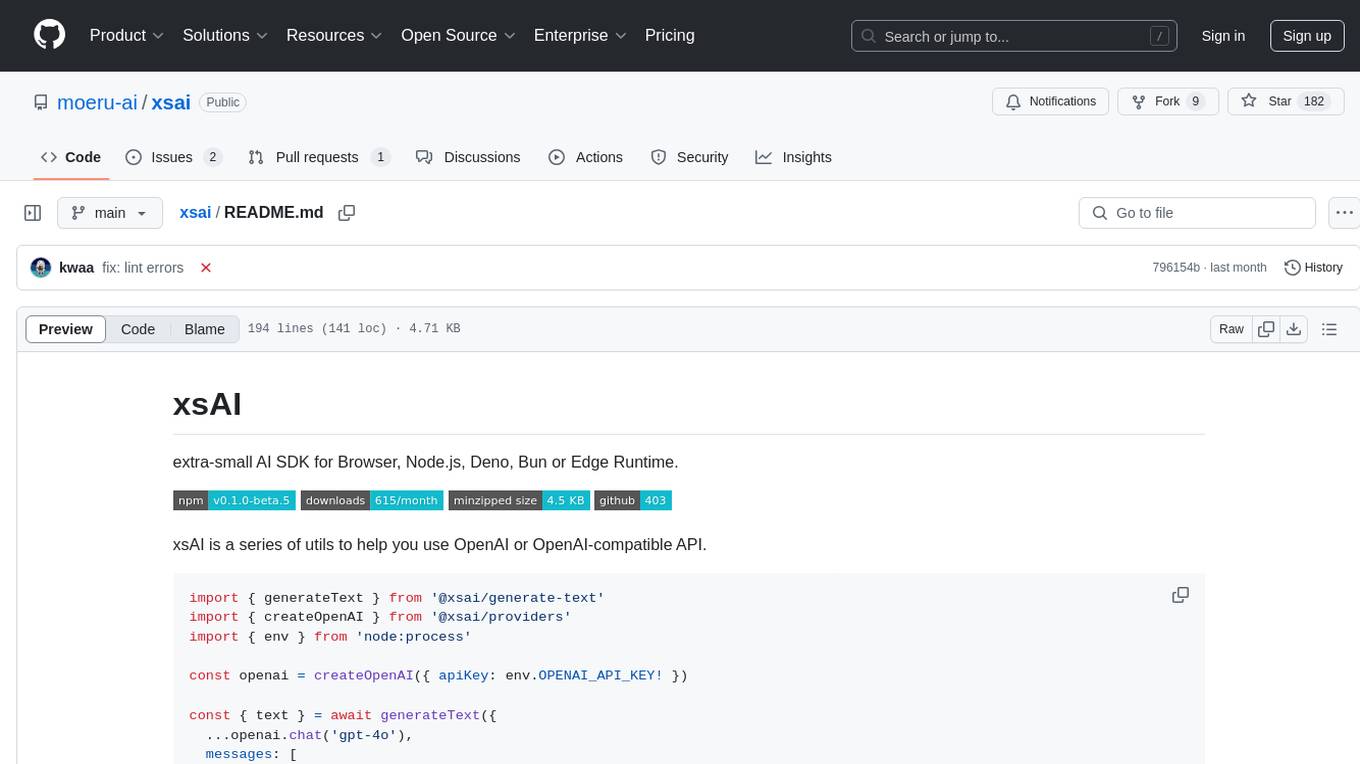
xsai
xsAI is an extra-small AI SDK designed for Browser, Node.js, Deno, Bun, or Edge Runtime. It provides a series of utils to help users utilize OpenAI or OpenAI-compatible APIs. The SDK is lightweight and efficient, using a variety of methods to minimize its size. It is runtime-agnostic, working seamlessly across different environments without depending on Node.js Built-in Modules. Users can easily install specific utils like generateText or streamText, and leverage tools like weather to perform tasks such as getting the weather in a location.
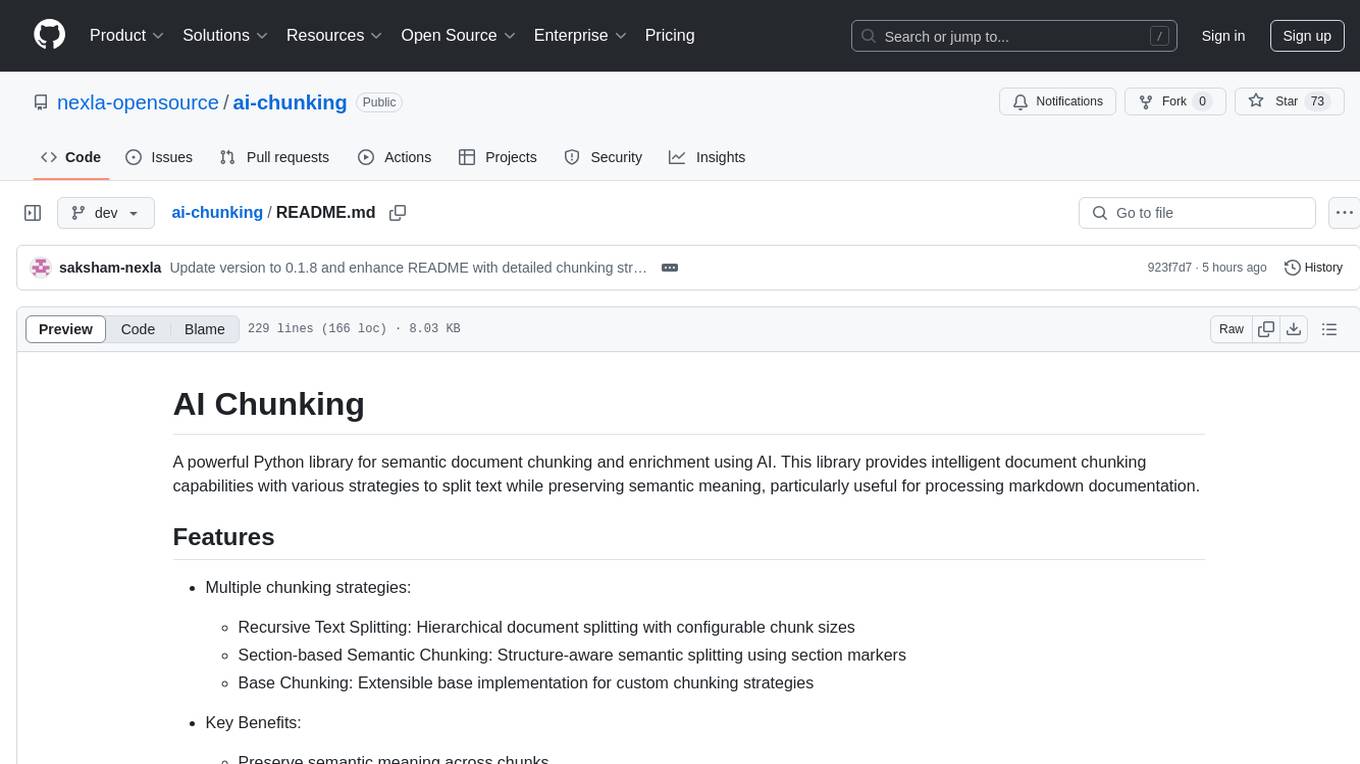
ai-chunking
AI Chunking is a powerful Python library for semantic document chunking and enrichment using AI. It provides intelligent document chunking capabilities with various strategies to split text while preserving semantic meaning, particularly useful for processing markdown documentation. The library offers multiple chunking strategies such as Recursive Text Splitting, Section-based Semantic Chunking, and Base Chunking. Users can configure chunk sizes, overlap, and support various text formats. The tool is easy to extend with custom chunking strategies, making it versatile for different document processing needs.
For similar tasks
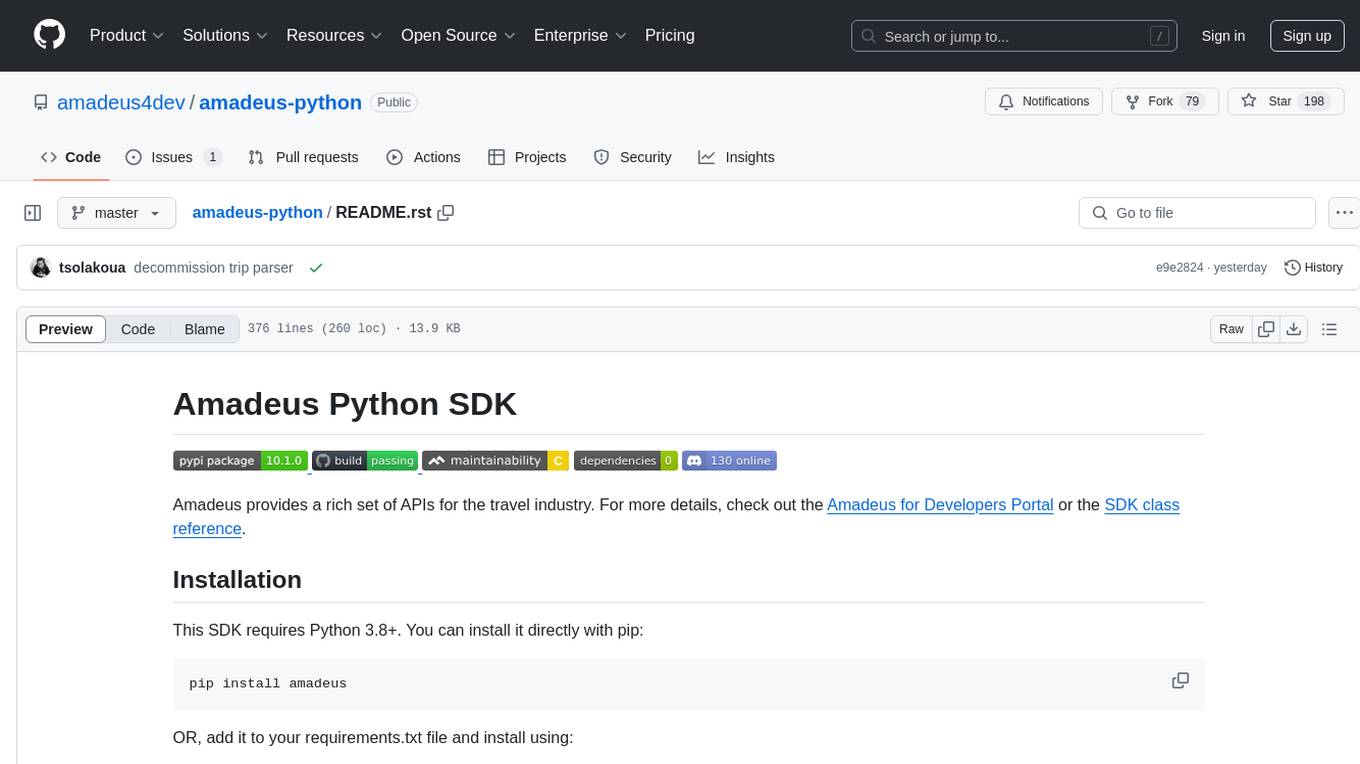
amadeus-python
Amadeus Python SDK provides a rich set of APIs for the travel industry. It allows users to make API calls for various travel-related tasks such as flight offers search, hotel bookings, trip purpose prediction, flight delay prediction, airport on-time performance, travel recommendations, and more. The SDK conveniently maps API paths to similar paths, making it easy to interact with the Amadeus APIs. Users can initialize the client with their API key and secret, make API calls, handle responses, and enable logging for debugging purposes. The SDK documentation includes detailed information about each SDK method, arguments, and return types.
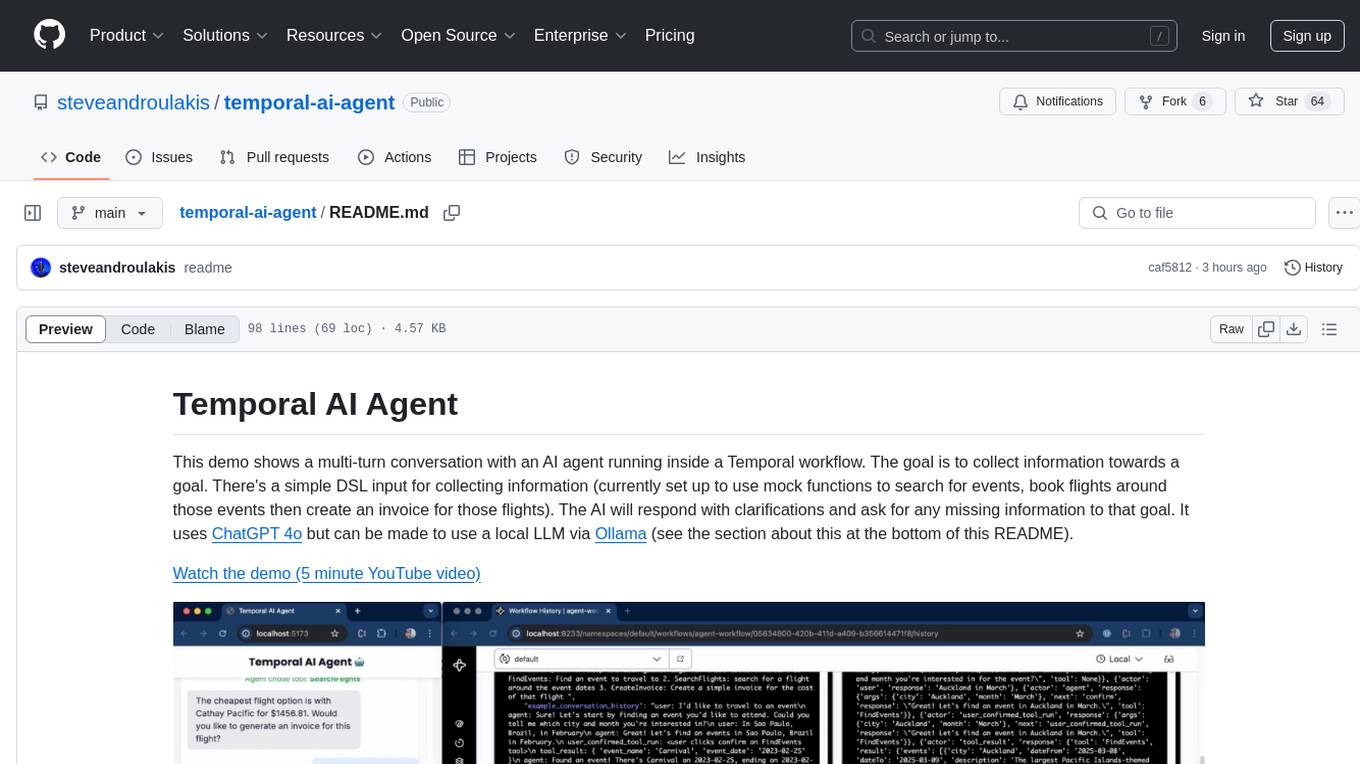
temporal-ai-agent
Temporal AI Agent is a demo showcasing a multi-turn conversation with an AI agent running inside a Temporal workflow. The agent collects information towards a goal using a simple DSL input. It is currently set up to search for events, book flights around those events, and create an invoice for those flights. The AI agent responds with clarifications and prompts for missing information. Users can configure the agent to use ChatGPT 4o or a local LLM via Ollama. The tool requires Rapidapi key for sky-scrapper to find flights and a Stripe key for creating invoices. Users can customize the agent by modifying tool and goal definitions in the codebase.
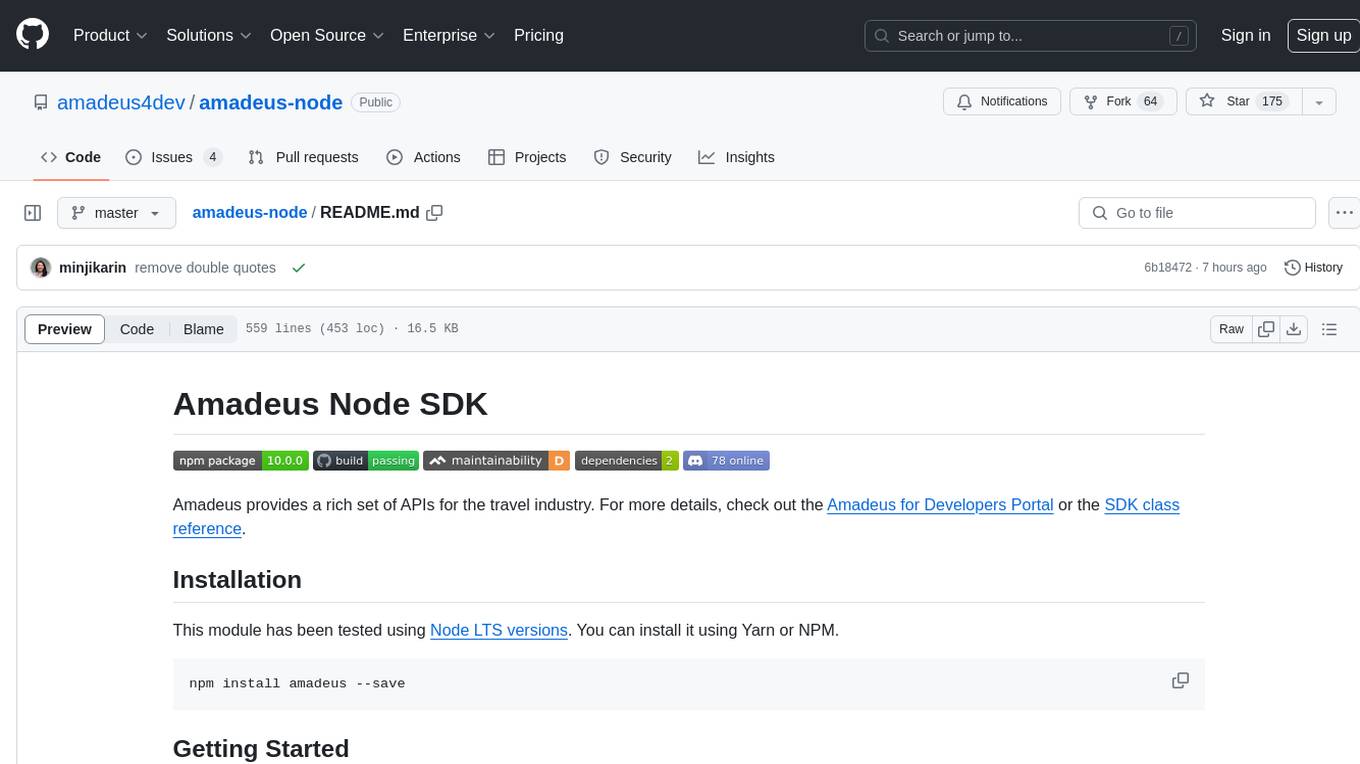
amadeus-node
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
For similar jobs
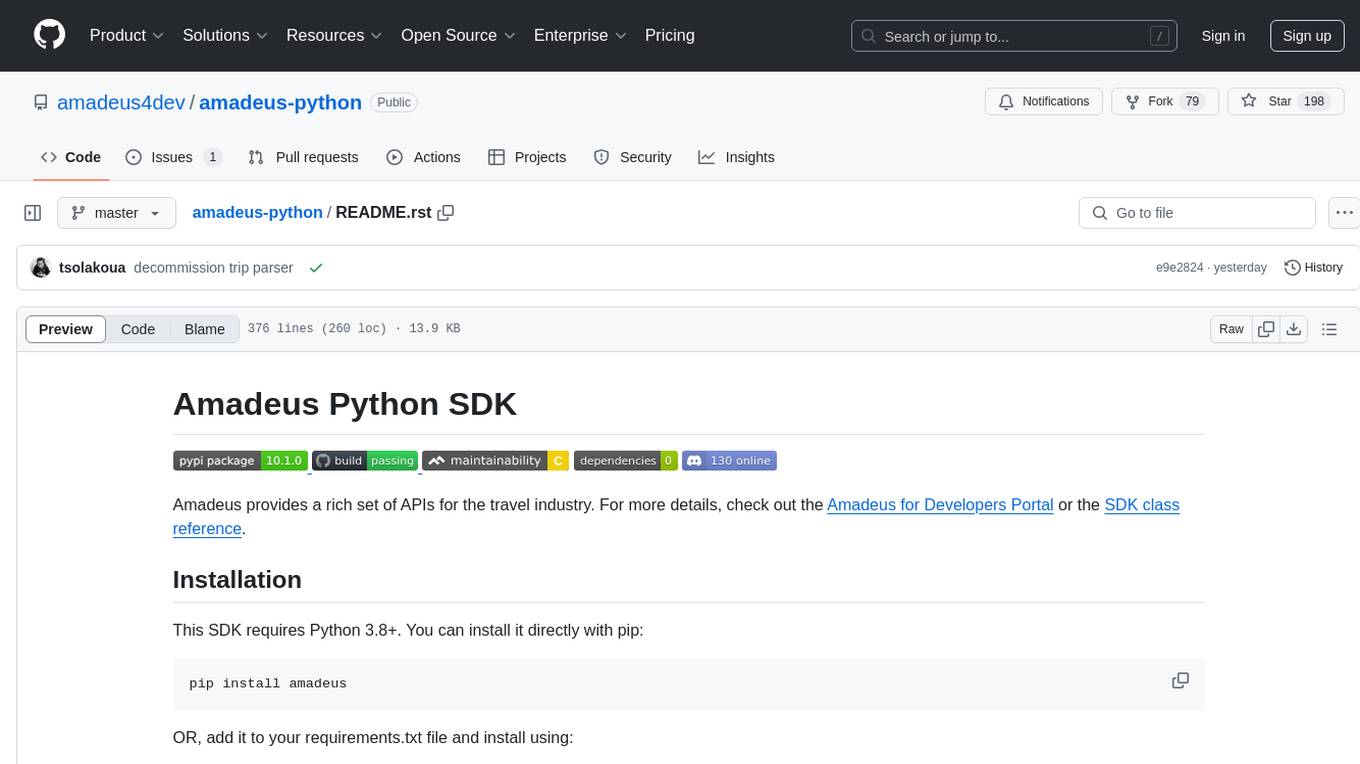
amadeus-python
Amadeus Python SDK provides a rich set of APIs for the travel industry. It allows users to make API calls for various travel-related tasks such as flight offers search, hotel bookings, trip purpose prediction, flight delay prediction, airport on-time performance, travel recommendations, and more. The SDK conveniently maps API paths to similar paths, making it easy to interact with the Amadeus APIs. Users can initialize the client with their API key and secret, make API calls, handle responses, and enable logging for debugging purposes. The SDK documentation includes detailed information about each SDK method, arguments, and return types.
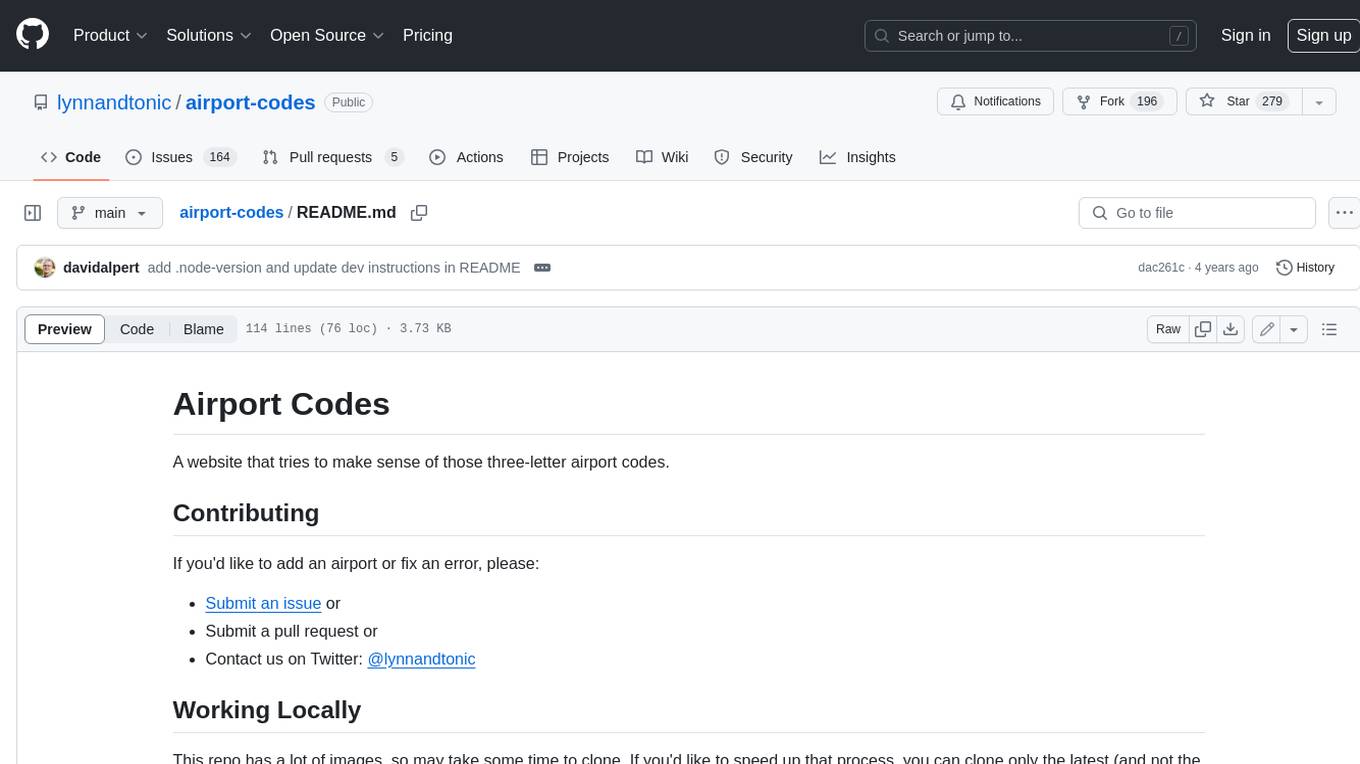
airport-codes
A website that tries to make sense of those three-letter airport codes. It provides detailed information about each airport, including its name, location, and a description. The site also includes a search function that allows users to find airports by name, city, or country. Airport content can be found in `/data` in individual files. Use the three-letter airport code as the filename (e.g. `phx.json`). Content in each `json` file: `id` = three-letter code (e.g. phx), `name` = airport name (Sky Harbor International Airport), `city` = primary city name (Phoenix), `state` = state name, if applicable (Arizona), `stateShort` = state abbreviation, if applicable (AZ), `country` = country name (USA), `description` = description, accepts markdown, use * for emphasis on letters, `imageCredit` = name of photographer, `imageCreditLink` = URL of photographer's Flickr page. You can also optionally add for aid in searching: `city2` = another city or country the airport may be known for. Adding a `json` file to `/data` will automatically render it. You do not need to manually add the path anywhere.
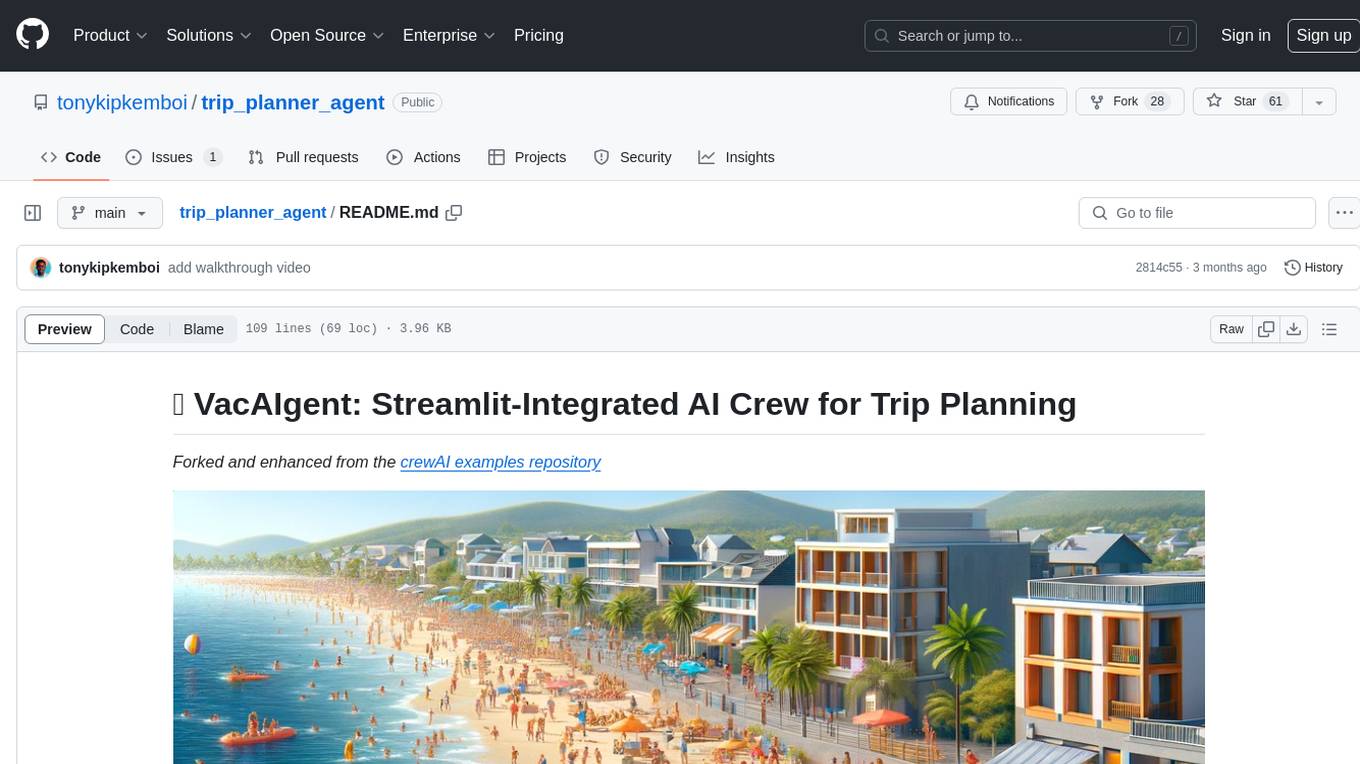
trip_planner_agent
VacAIgent is an AI tool that automates and enhances trip planning by leveraging the CrewAI framework. It integrates a user-friendly Streamlit interface for interactive travel planning. Users can input preferences and receive tailored travel plans with the help of autonomous AI agents. The tool allows for collaborative decision-making on cities and crafting complete itineraries based on specified preferences, all accessible via a streamlined Streamlit user interface. VacAIgent can be customized to use different AI models like GPT-3.5 or local models like Ollama for enhanced privacy and customization.
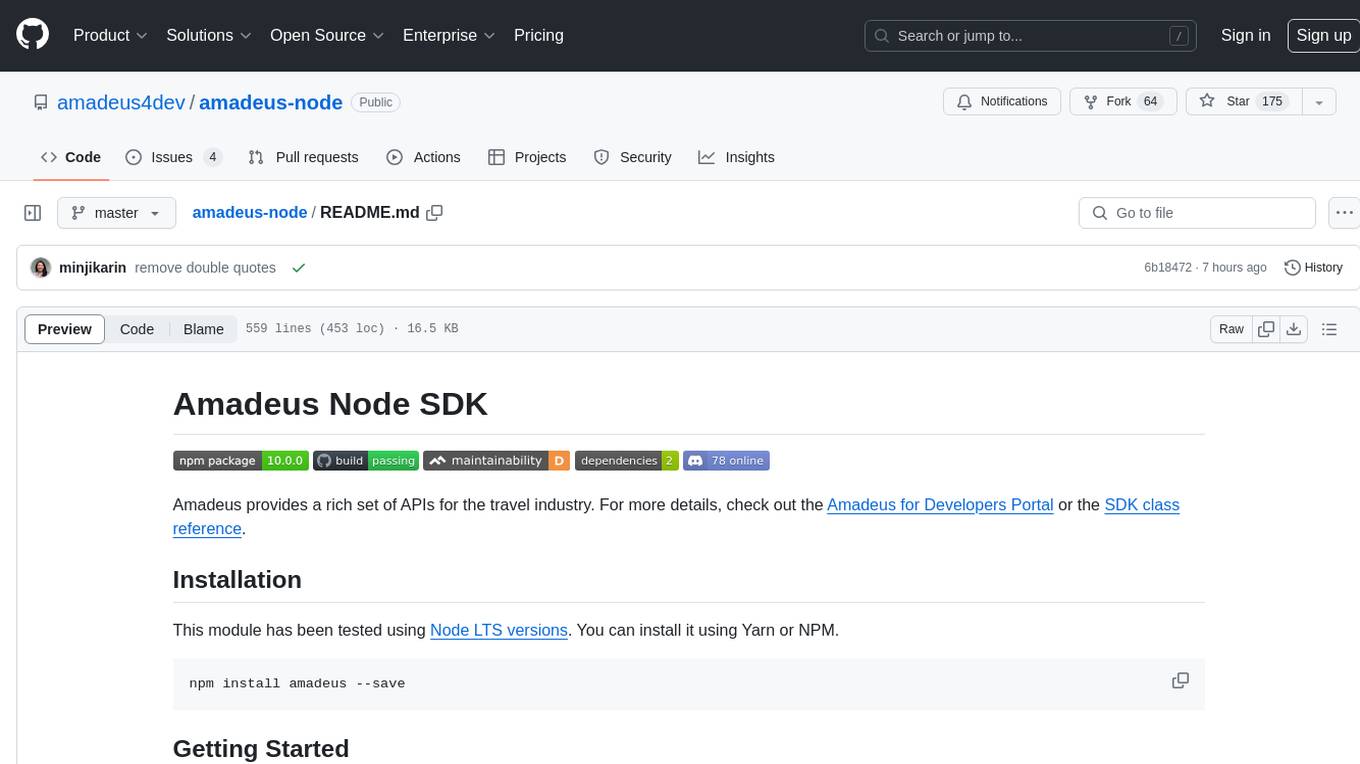
amadeus-node
Amadeus Node SDK provides a rich set of APIs for the travel industry. It allows developers to interact with various endpoints related to flights, hotels, activities, and more. The SDK simplifies making API calls, handling promises, pagination, logging, and debugging. It supports a wide range of functionalities such as flight search, booking, seat maps, flight status, points of interest, hotel search, sentiment analysis, trip predictions, and more. Developers can easily integrate the SDK into their Node.js applications to access Amadeus APIs and build travel-related applications.
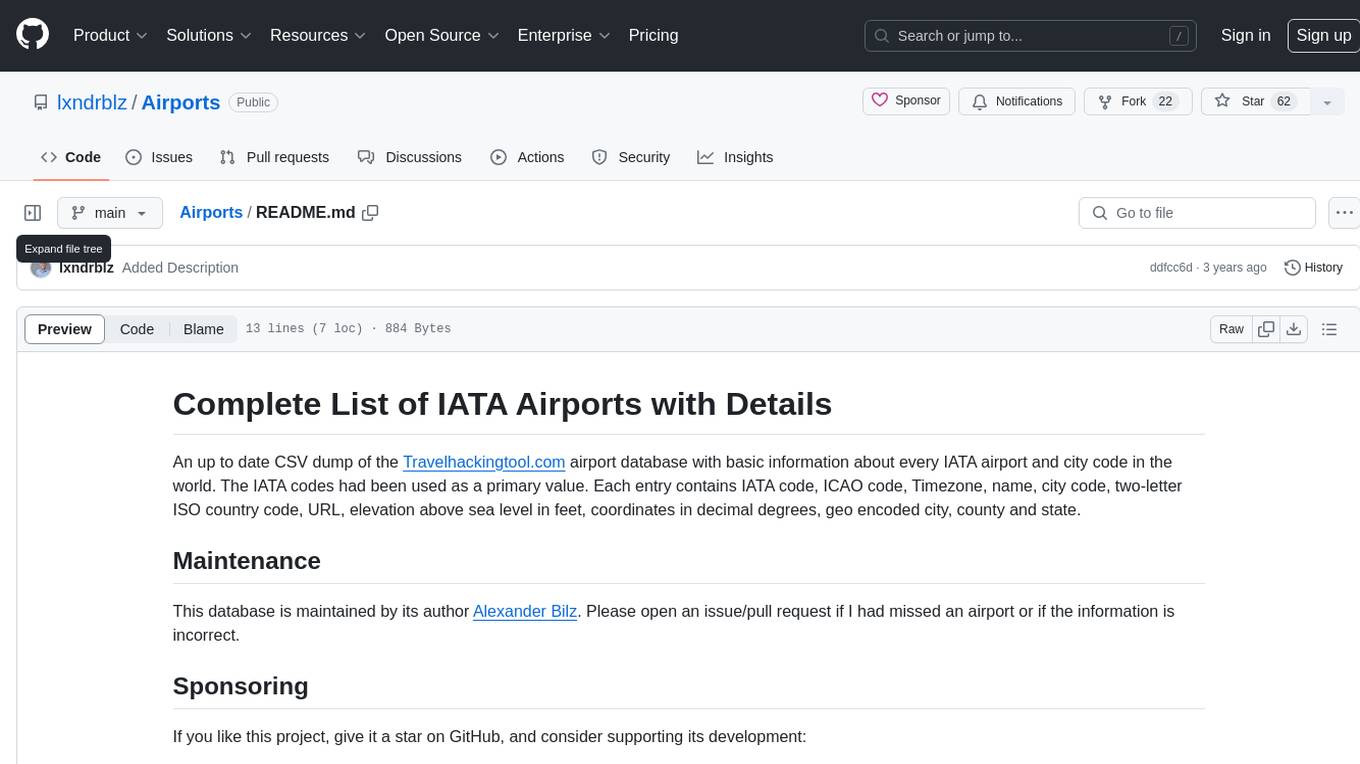
Airports
Airports is a repository containing an up-to-date CSV dump of the Travelhackingtool.com airport database. It provides basic information about every IATA airport and city code worldwide, including IATA code, ICAO code, timezone, name, city code, country code, URL, elevation, coordinates, and geo-encoded city, county, and state.
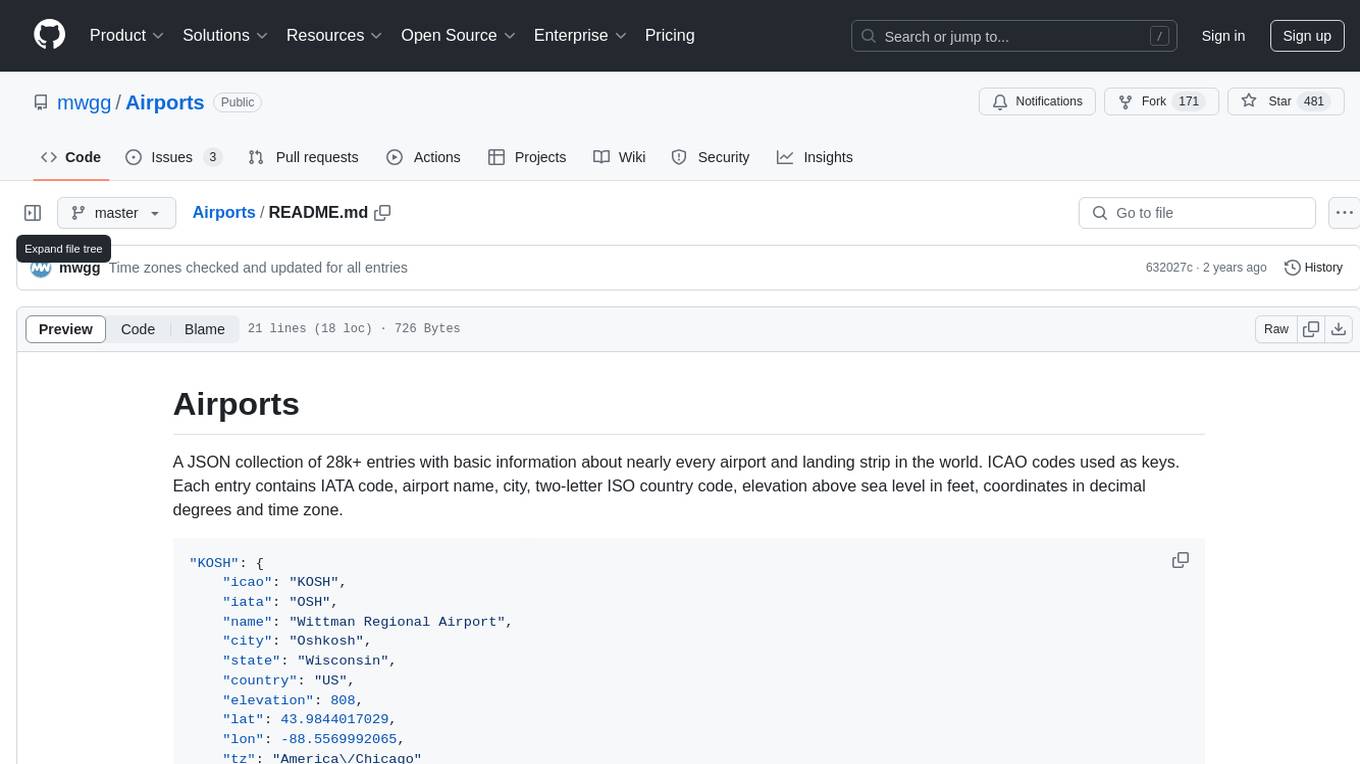
Airports
Airports is a JSON collection with detailed information about over 28,000 airports and landing strips worldwide. Each entry includes IATA code, airport name, city, country code, elevation, coordinates, and time zone.
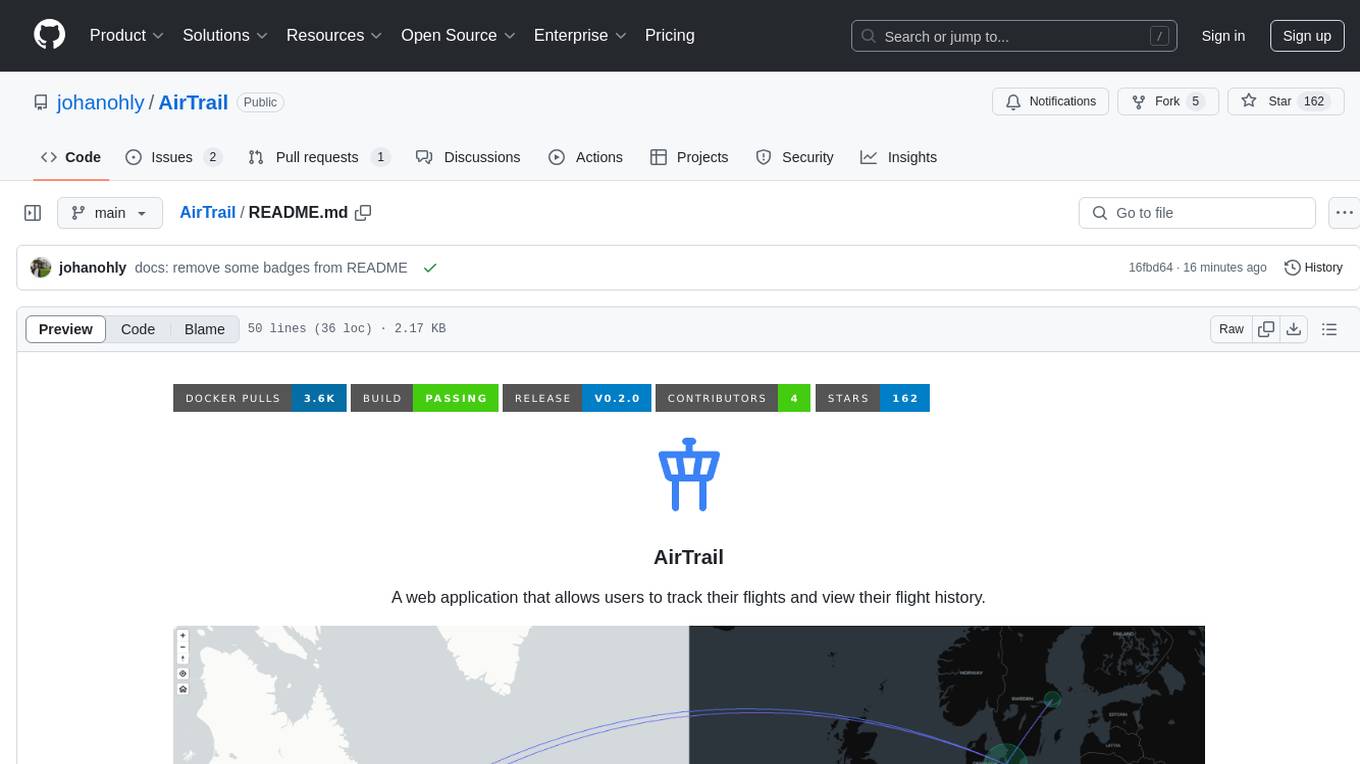
AirTrail
AirTrail is a web application that allows users to track their flights and view their flight history. It features an interactive world map to view flights, flight history tracking, statistics insights, multiple user management with user authentication, responsive design, dark mode, and flight import from various sources.
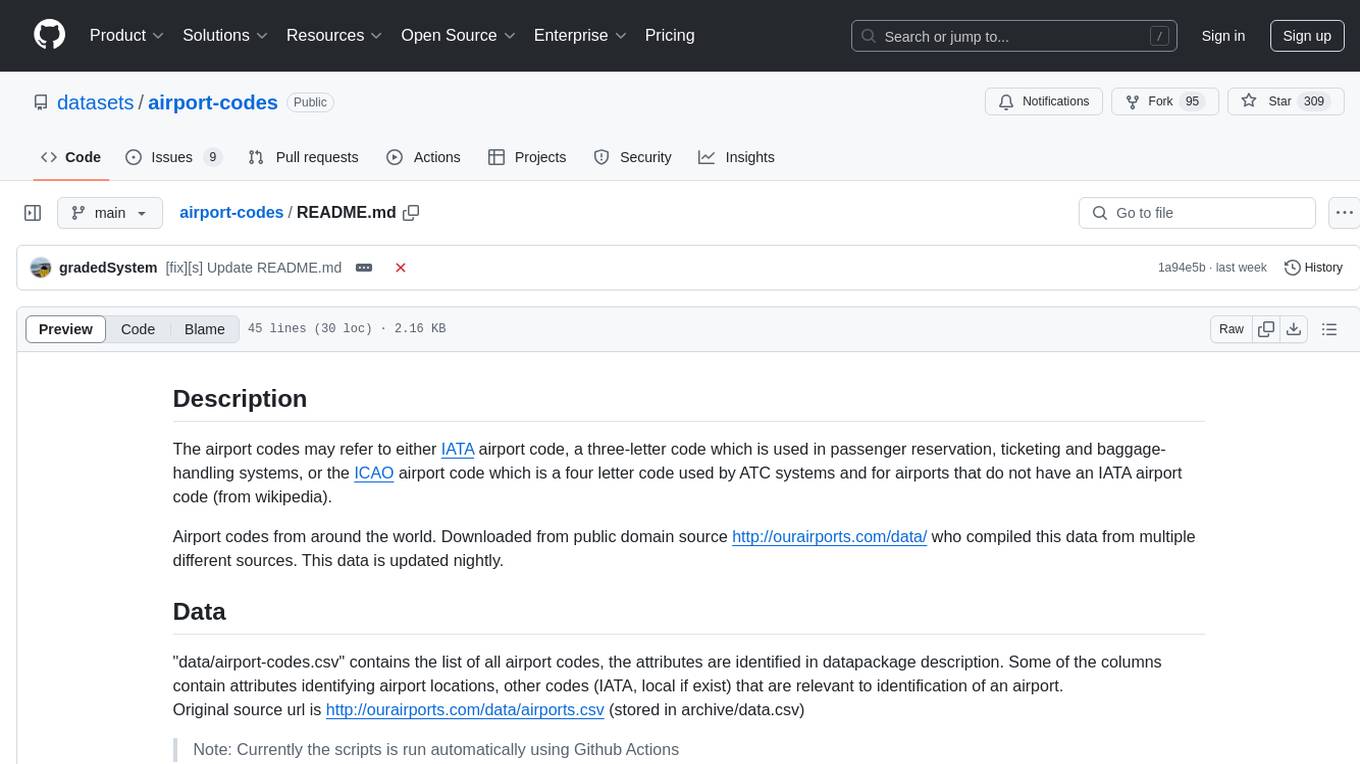
airport-codes
The airport-codes repository contains a list of airport codes from around the world, including IATA and ICAO codes. The data is sourced from multiple different sources and is updated nightly. The repository provides a script to process the data and merge location coordinates. The data can be used for various purposes such as passenger reservation, ticketing, and ATC systems.