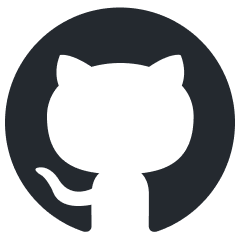
agency
A fast and minimal framework for building agent-integrated systems
Stars: 378
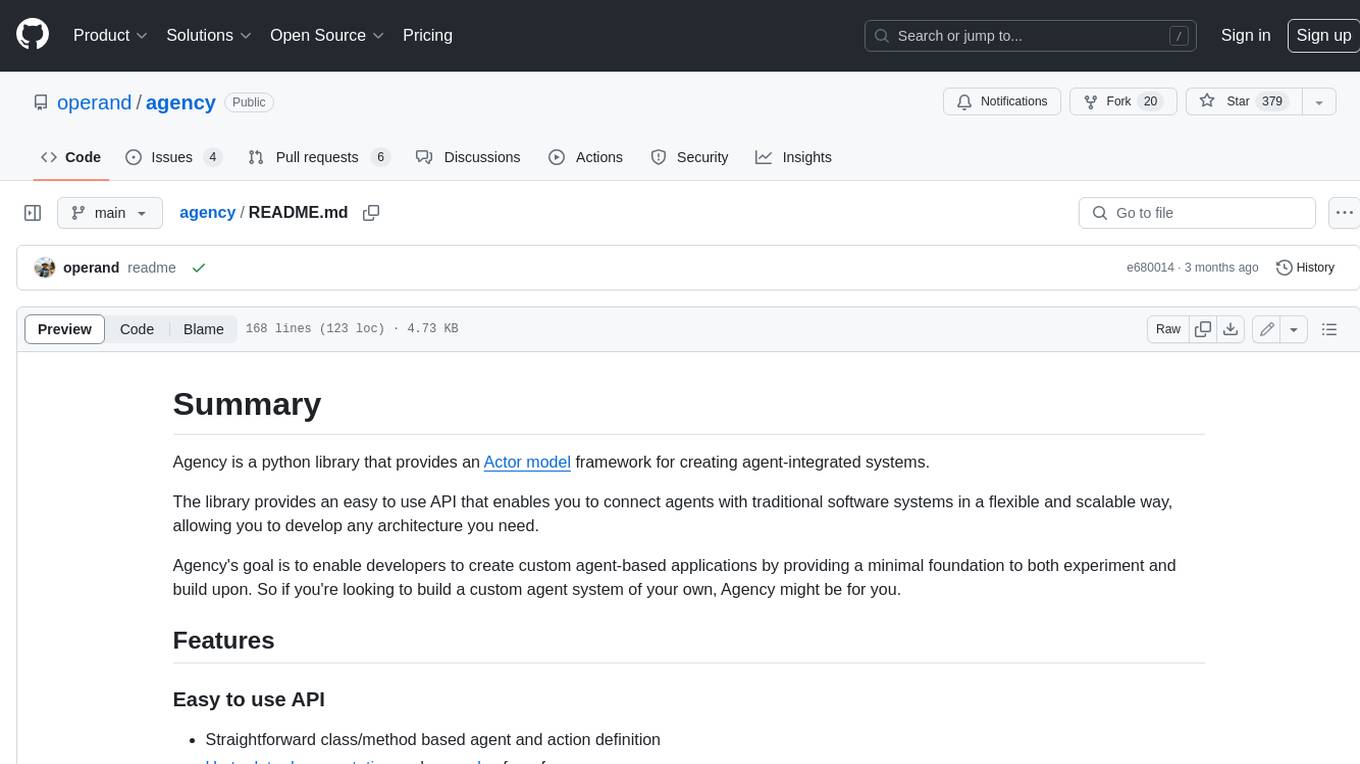
Agency is a python library that provides an Actor model framework for creating agent-integrated systems. It offers an easy-to-use API for connecting agents with traditional software systems, enabling flexible and scalable architectures. Agency aims to empower developers in creating custom agent-based applications by providing a foundation for experimentation and development. Key features include an intuitive API, performance and scalability through multiprocessing and AMQP support, observability and control with action and lifecycle callbacks, access policies, and detailed logging. The library also includes a demo application with multiple agent examples, OpenAI agent examples, HuggingFace transformers agent example, operating system access, Gradio UI, and Docker configuration for reference and development.
README:
Agency is a python library that provides an Actor model framework for creating agent-integrated systems.
The library provides an easy to use API that enables you to connect agents with traditional software systems in a flexible and scalable way, allowing you to develop any architecture you need.
Agency's goal is to enable developers to create custom agent-based applications by providing a minimal foundation to both experiment and build upon. So if you're looking to build a custom agent system of your own, Agency might be for you.
- Straightforward class/method based agent and action definition
- Up to date documentation and examples for reference
- Supports multiprocessing and multithreading for concurrency
- AMQP support for networked agent systems
- Action and lifecycle callbacks
- Access policies and permission callbacks
- Detailed logging
Demo application available at examples/demo
- Multiple agent examples for experimentation
- Two OpenAI agent examples
- HuggingFace transformers agent example
- Operating system access
- Includes Gradio UI
- Docker configuration for reference and development
In Agency, all entities are represented as instances of the Agent
class. This
includes all AI-driven agents, software interfaces, or human users that may
communicate as part of your application.
All agents may expose "actions" that other agents can discover and invoke at run time. An example of a simple agent could be:
class CalculatorAgent(Agent):
@action
def add(a, b):
return a + b
This defines an agent with a single action: add
. Other agents will be able
to call this method by sending a message to an instance of CalculatorAgent
and
specifying the add
action. For example:
other_agent.send({
'to': 'CalcAgent',
'action': {
'name': 'add',
'args': {
'a': 1,
'b': 2,
}
},
})
Actions may specify an access policy, allowing you to control access for safety.
@action(access_policy=ACCESS_PERMITTED) # This allows the action at any time
def add(a, b):
...
@action(access_policy=ACCESS_REQUESTED) # This requires review before the action
def add(a, b):
...
Agents may also define callbacks for various purposes:
class CalculatorAgent(Agent):
...
def before_action(self, message: dict):
"""Called before an action is attempted"""
def after_action(self, message: dict, return_value: str, error: str):
"""Called after an action is attempted"""
def after_add(self):
"""Called after the agent is added to a space and may begin communicating"""
def before_remove(self):
"""Called before the agent is removed from the space"""
A Space
is how you connect your agents together. An agent cannot communicate
with others until it is added to a common Space
.
There are two included Space
implementations to choose from:
-
LocalSpace
- which connects agents within the same application. -
AMQPSpace
- which connects agents across a network using an AMQP server like RabbitMQ.
Finally, here is a simple example of creating a LocalSpace
and adding two
agents to it.
space = LocalSpace()
space.add(CalculatorAgent, "CalcAgent")
space.add(MyAgent, "MyAgent")
# The agents above can now communicate
These are just the basic features that Agency provides. For more information please see the help site.
pip install agency
or
poetry add agency
The demo application is maintained as an experimental development environment and a showcase for library features. It includes multiple agent examples which may communicate with eachother and supports a "slash" syntax for invoking actions as an agent yourself.
To run the demo, please follow the directions at examples/demo.
The following is a screenshot of the Gradio UI that demonstrates the example
OpenAIFunctionAgent
following orders and interacting with the Host
agent.
Please do!
If you're considering a contribution, please check out the contributing guide.
If you have any suggestions or otherwise, feel free to add an issue or open a discussion.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for agency
Similar Open Source Tools
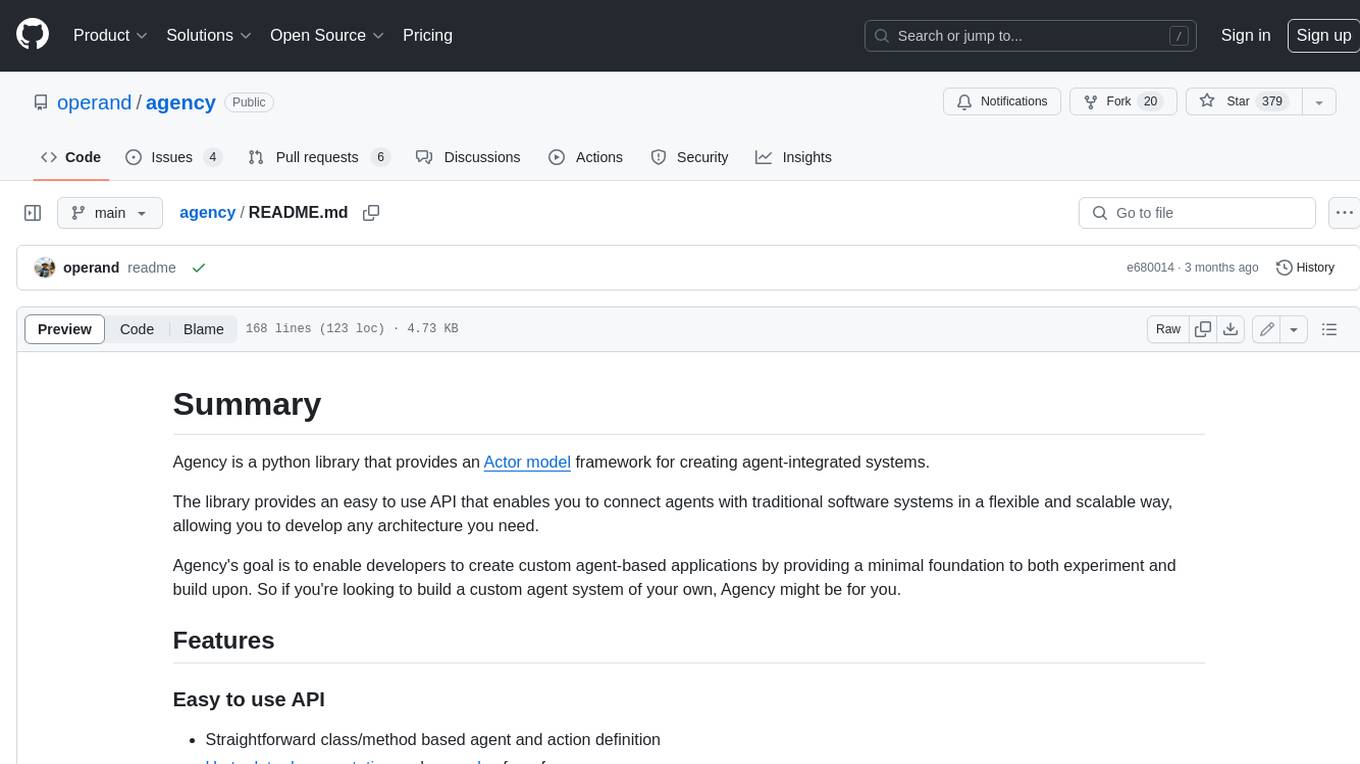
agency
Agency is a python library that provides an Actor model framework for creating agent-integrated systems. It offers an easy-to-use API for connecting agents with traditional software systems, enabling flexible and scalable architectures. Agency aims to empower developers in creating custom agent-based applications by providing a foundation for experimentation and development. Key features include an intuitive API, performance and scalability through multiprocessing and AMQP support, observability and control with action and lifecycle callbacks, access policies, and detailed logging. The library also includes a demo application with multiple agent examples, OpenAI agent examples, HuggingFace transformers agent example, operating system access, Gradio UI, and Docker configuration for reference and development.
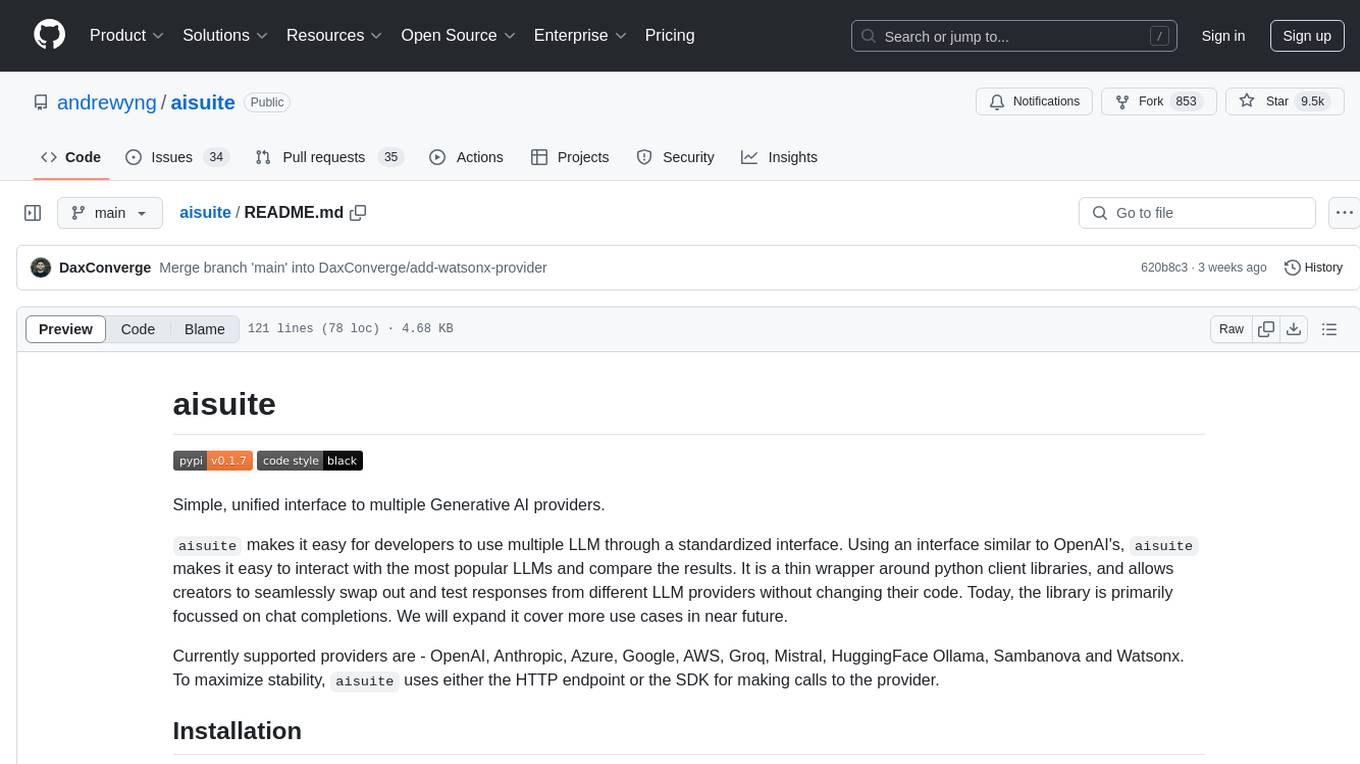
aisuite
Aisuite is a simple, unified interface to multiple Generative AI providers. It allows developers to easily interact with various Language Model (LLM) providers like OpenAI, Anthropic, Azure, Google, AWS, and more through a standardized interface. The library focuses on chat completions and provides a thin wrapper around python client libraries, enabling creators to test responses from different LLM providers without changing their code. Aisuite maximizes stability by using HTTP endpoints or SDKs for making calls to the providers. Users can install the base package or specific provider packages, set up API keys, and utilize the library to generate chat completion responses from different models.
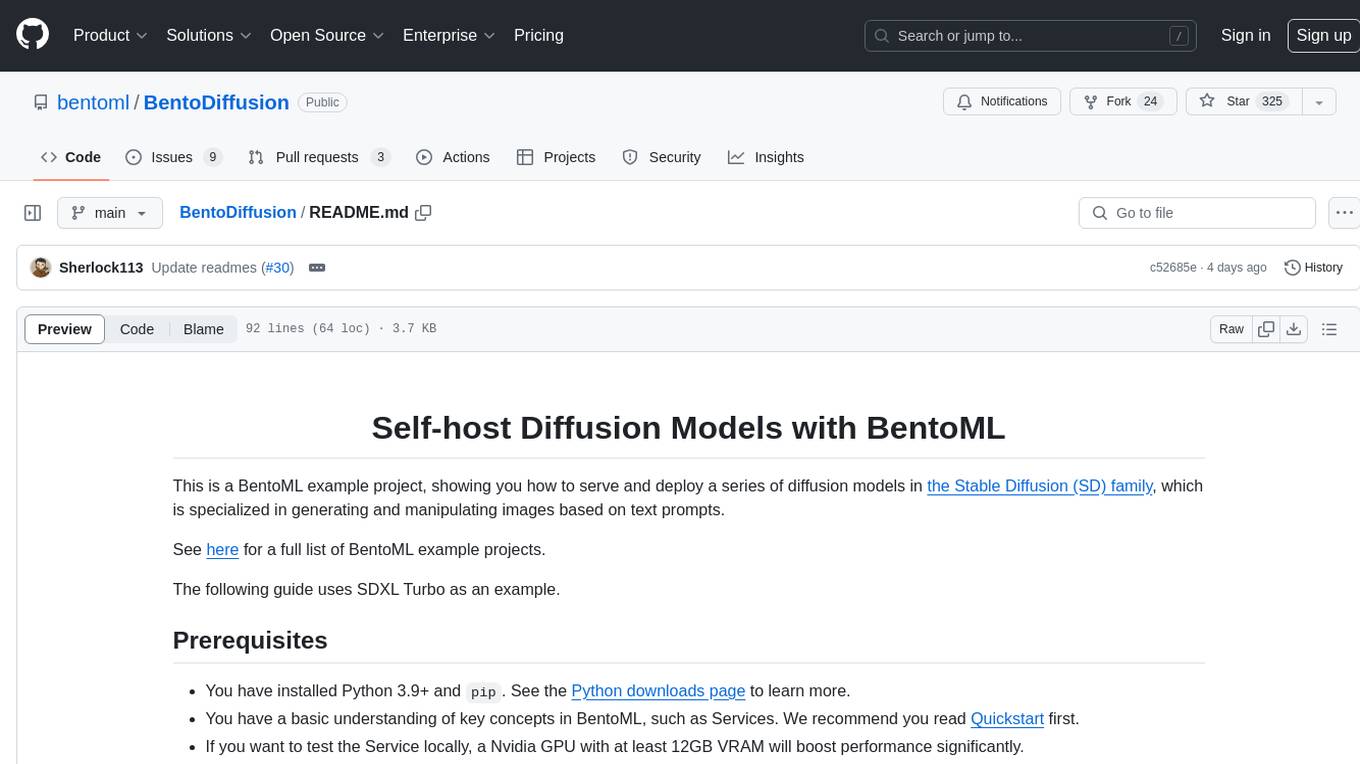
BentoDiffusion
BentoDiffusion is a BentoML example project that demonstrates how to serve and deploy diffusion models in the Stable Diffusion (SD) family. These models are specialized in generating and manipulating images based on text prompts. The project provides a guide on using SDXL Turbo as an example, along with instructions on prerequisites, installing dependencies, running the BentoML service, and deploying to BentoCloud. Users can interact with the deployed service using Swagger UI or other methods. Additionally, the project offers the option to choose from various diffusion models available in the repository for deployment.
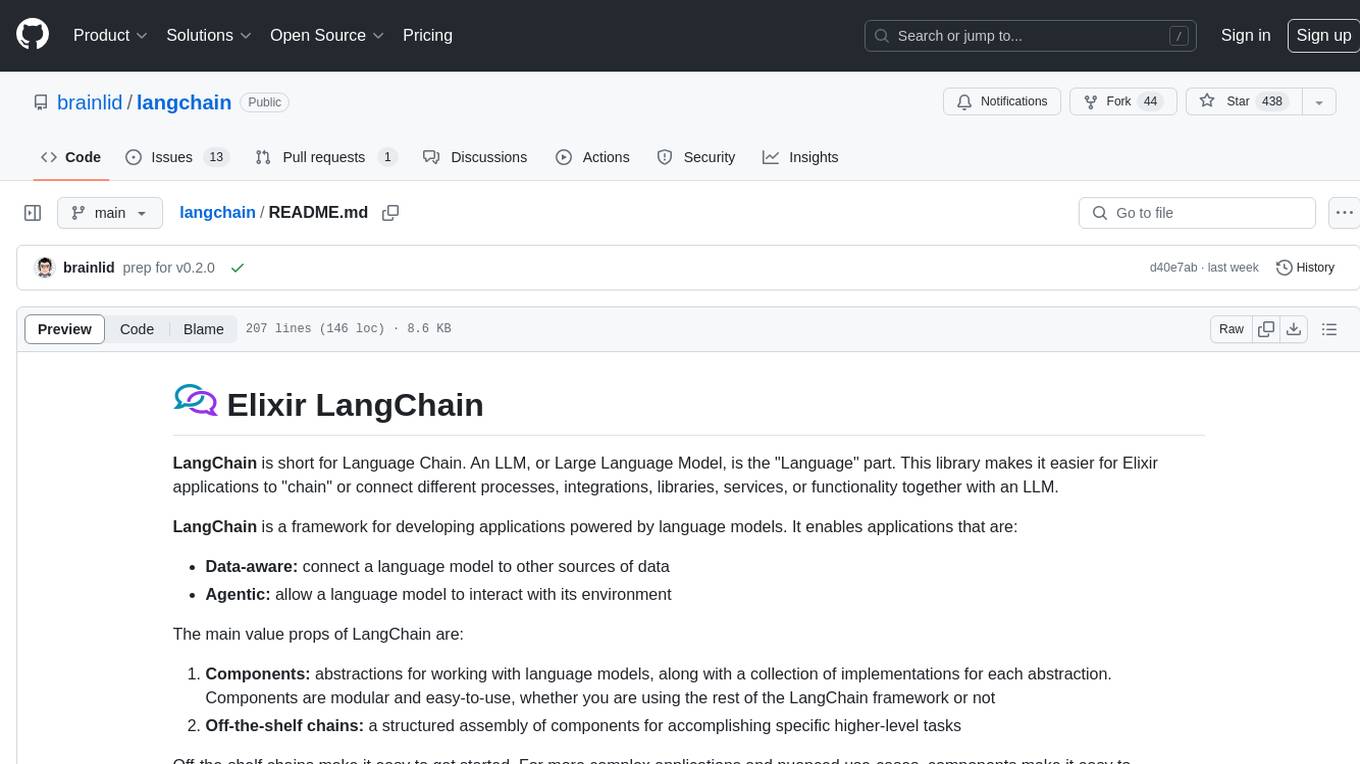
langchain
LangChain is a framework for developing Elixir applications powered by language models. It enables applications to connect language models to other data sources and interact with the environment. The library provides components for working with language models and off-the-shelf chains for specific tasks. It aims to assist in building applications that combine large language models with other sources of computation or knowledge. LangChain is written in Elixir and is not aimed for parity with the JavaScript and Python versions due to differences in programming paradigms and design choices. The library is designed to make it easy to integrate language models into applications and expose features, data, and functionality to the models.
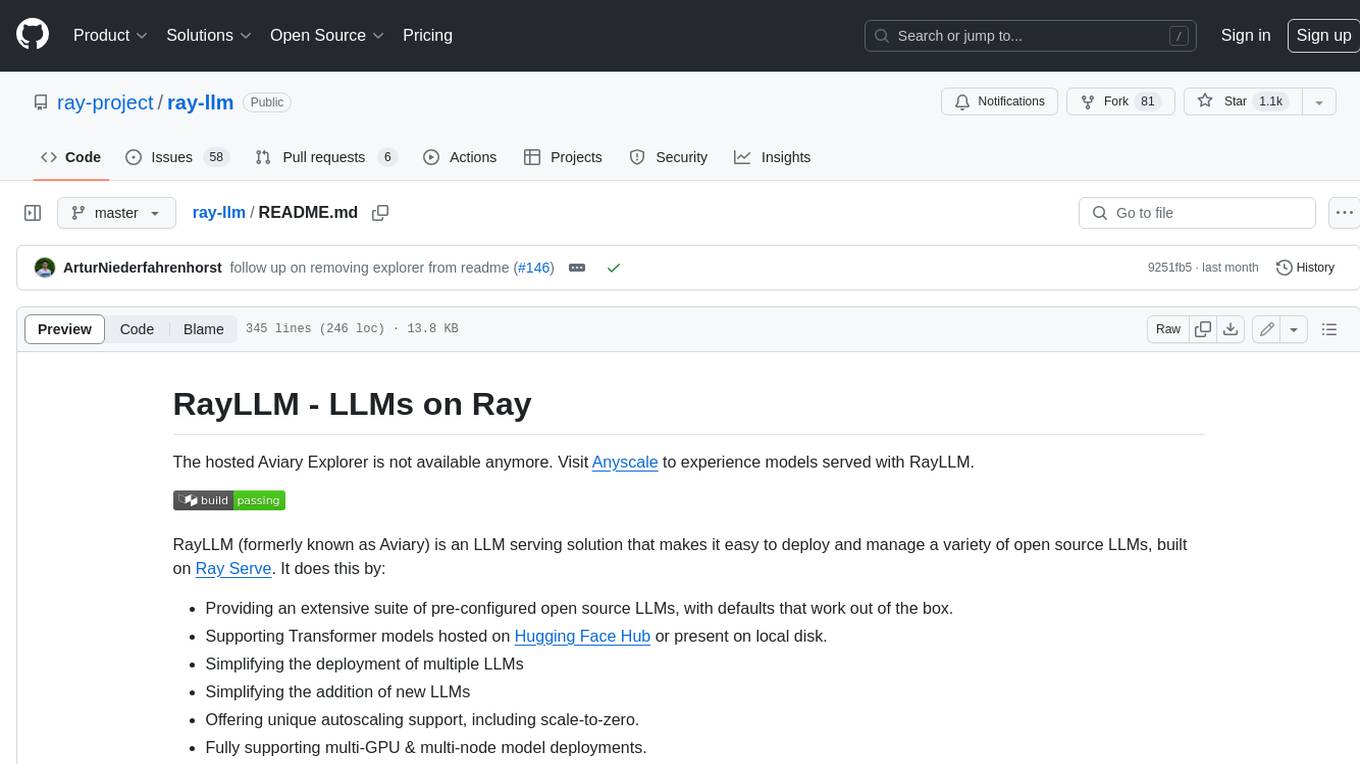
ray-llm
RayLLM (formerly known as Aviary) is an LLM serving solution that makes it easy to deploy and manage a variety of open source LLMs, built on Ray Serve. It provides an extensive suite of pre-configured open source LLMs, with defaults that work out of the box. RayLLM supports Transformer models hosted on Hugging Face Hub or present on local disk. It simplifies the deployment of multiple LLMs, the addition of new LLMs, and offers unique autoscaling support, including scale-to-zero. RayLLM fully supports multi-GPU & multi-node model deployments and offers high performance features like continuous batching, quantization and streaming. It provides a REST API that is similar to OpenAI's to make it easy to migrate and cross test them. RayLLM supports multiple LLM backends out of the box, including vLLM and TensorRT-LLM.
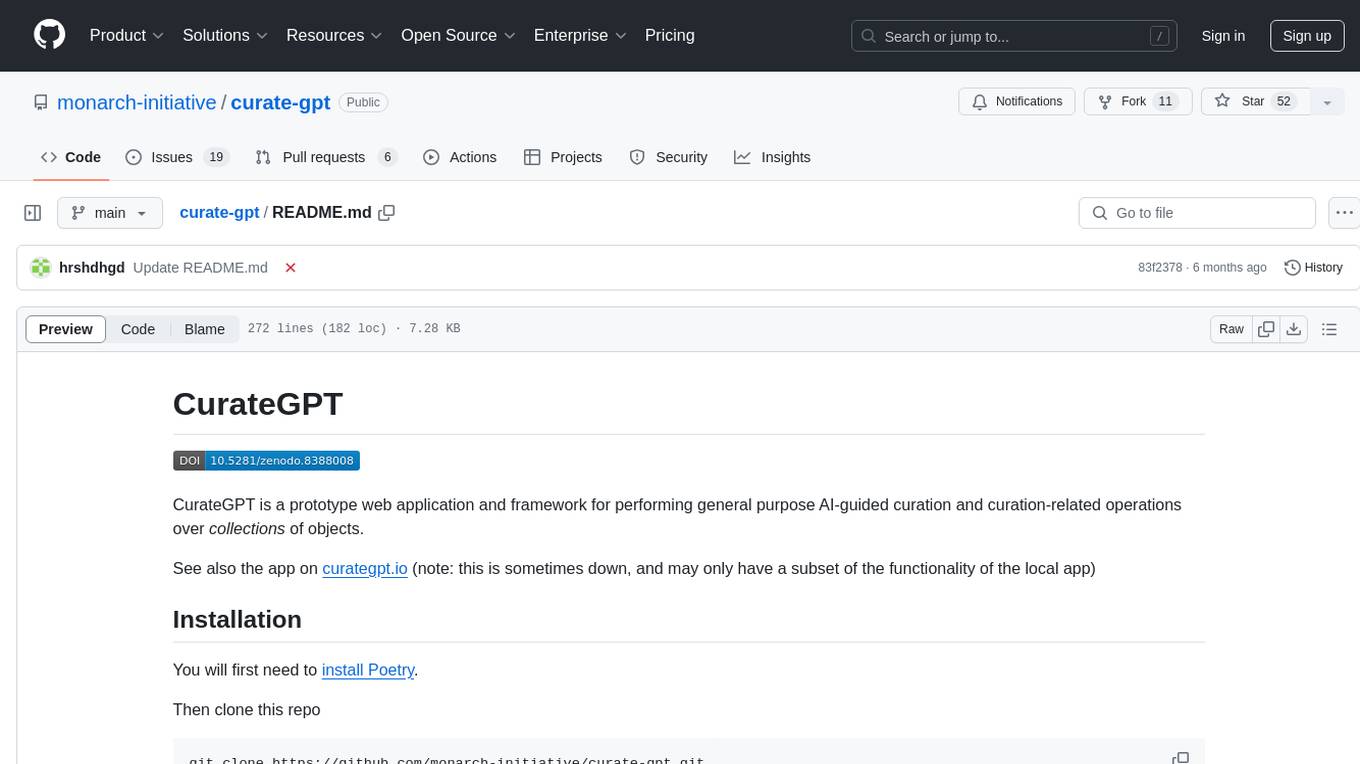
curate-gpt
CurateGPT is a prototype web application and framework for performing general purpose AI-guided curation and curation-related operations over collections of objects. It allows users to load JSON, YAML, or CSV data, build vector database indexes for ontologies, and interact with various data sources like GitHub, Google Drives, Google Sheets, and more. The tool supports ontology curation, knowledge base querying, term autocompletion, and all-by-all comparisons for objects in a collection.
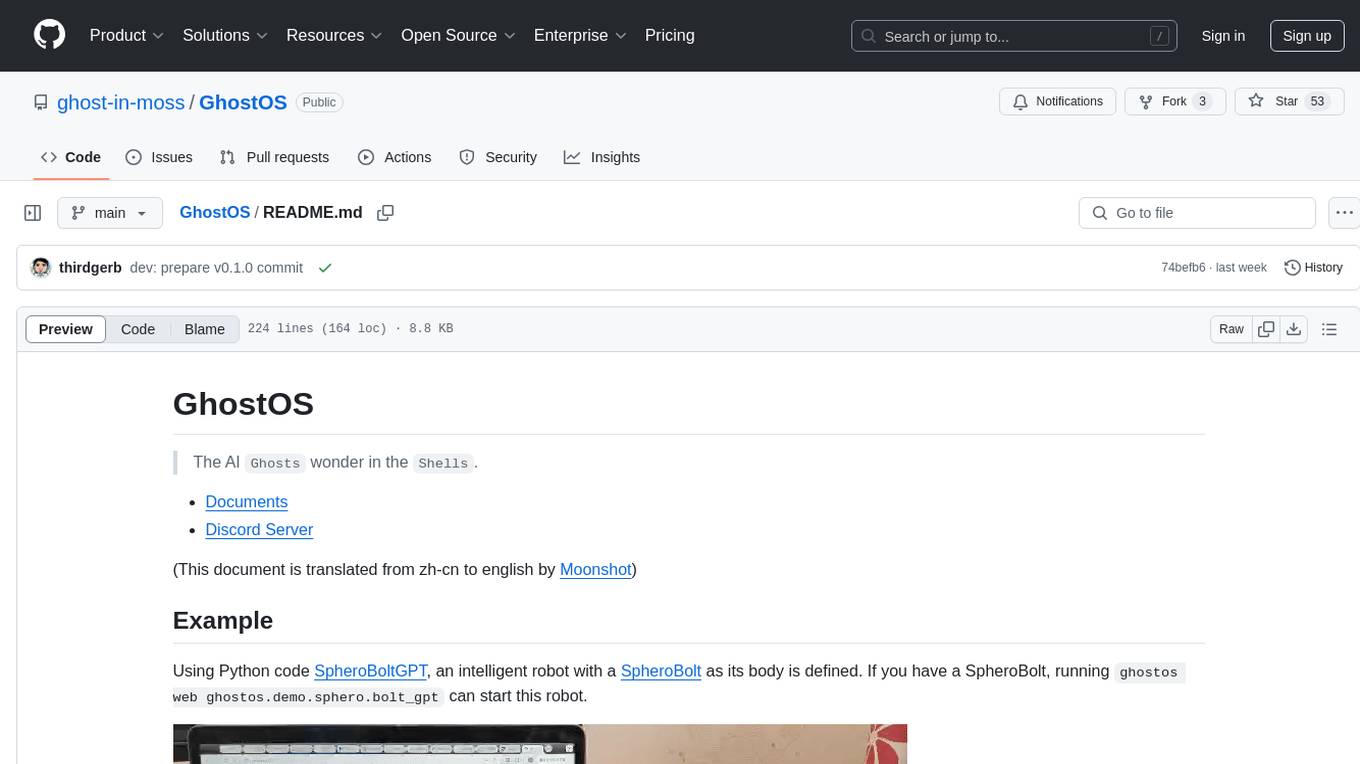
GhostOS
GhostOS is an AI Agent framework designed to replace JSON Schema with a Turing-complete code interaction interface (Moss Protocol). It aims to create intelligent entities capable of continuous learning and growth through code generation and project management. The framework supports various capabilities such as turning Python files into web agents, real-time voice conversation, body movements control, and emotion expression. GhostOS is still in early experimental development and focuses on out-of-the-box capabilities for AI agents.
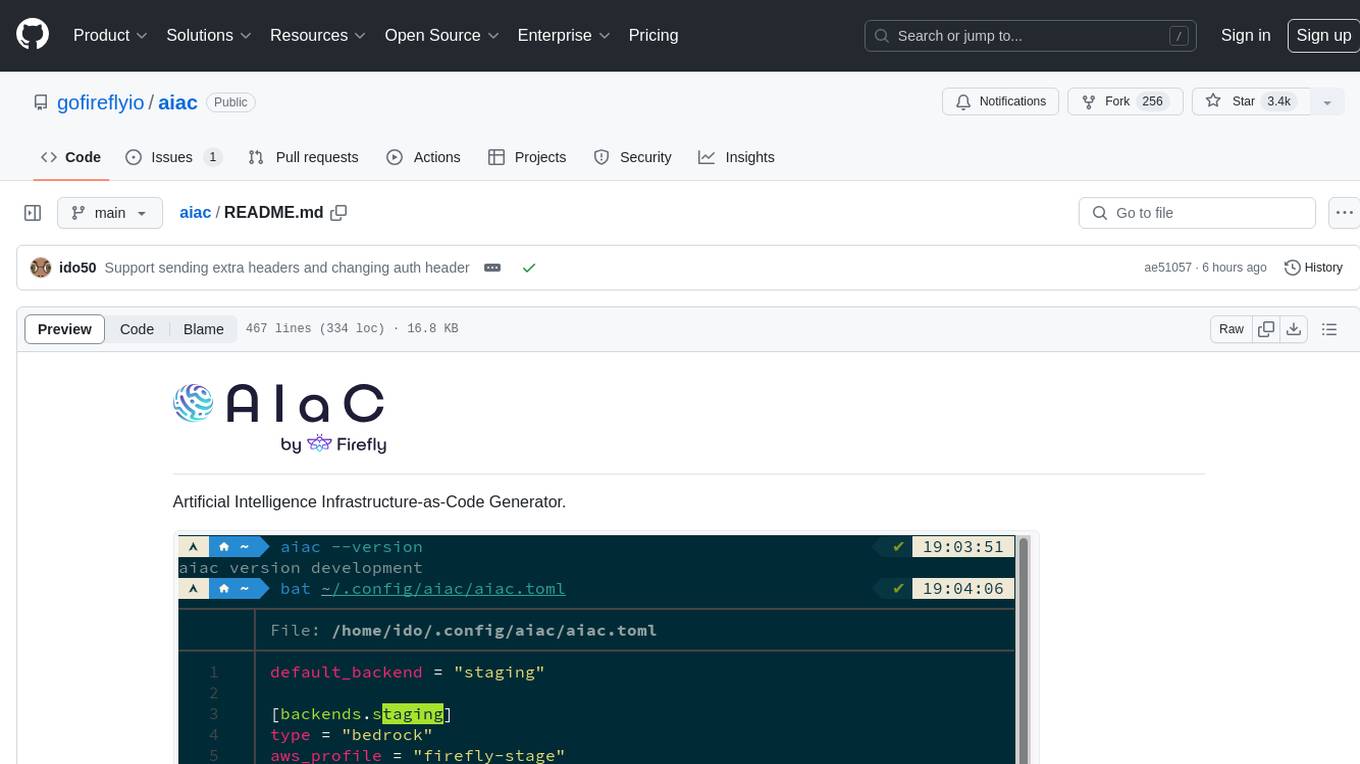
aiac
AIAC is a library and command line tool to generate Infrastructure as Code (IaC) templates, configurations, utilities, queries, and more via LLM providers such as OpenAI, Amazon Bedrock, and Ollama. Users can define multiple 'backends' targeting different LLM providers and environments using a simple configuration file. The tool allows users to ask a model to generate templates for different scenarios and composes an appropriate request to the selected provider, storing the resulting code to a file and/or printing it to standard output.
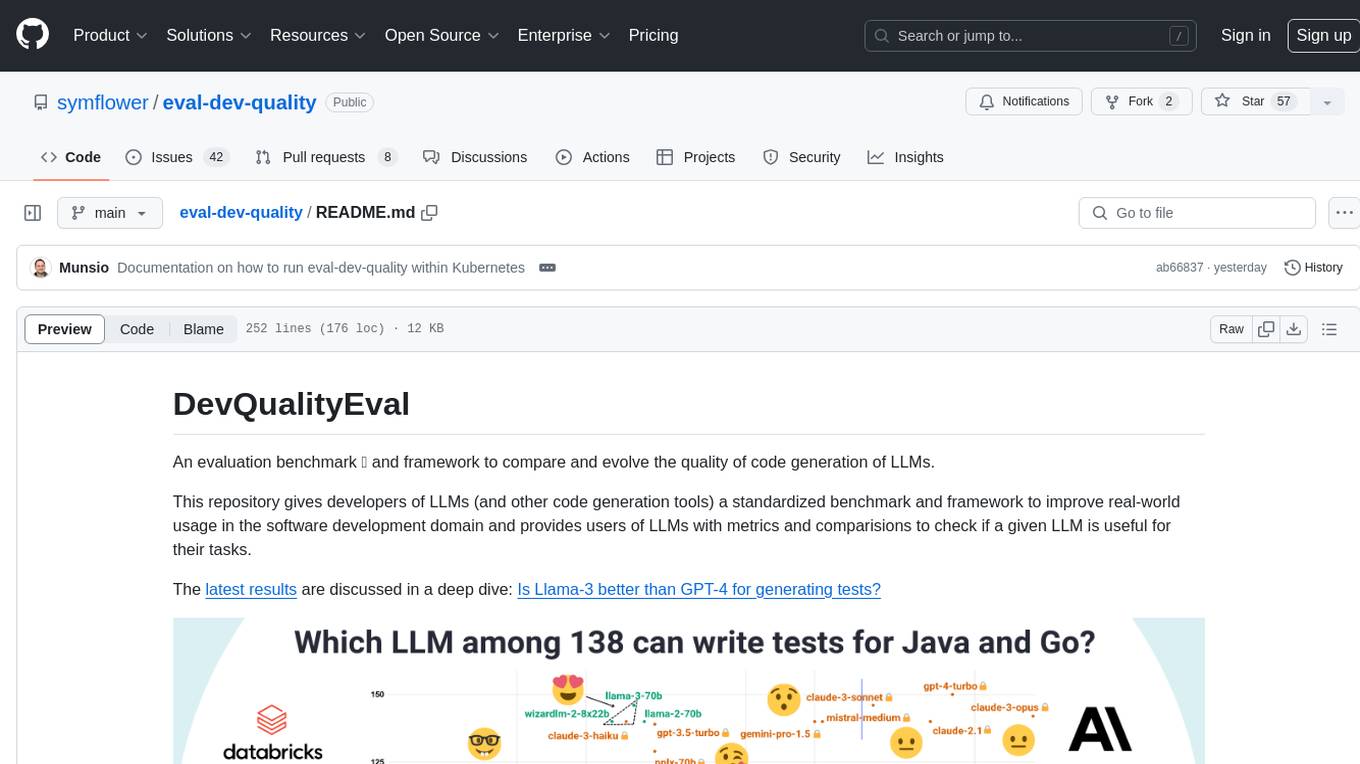
eval-dev-quality
DevQualityEval is an evaluation benchmark and framework designed to compare and improve the quality of code generation of Language Model Models (LLMs). It provides developers with a standardized benchmark to enhance real-world usage in software development and offers users metrics and comparisons to assess the usefulness of LLMs for their tasks. The tool evaluates LLMs' performance in solving software development tasks and measures the quality of their results through a point-based system. Users can run specific tasks, such as test generation, across different programming languages to evaluate LLMs' language understanding and code generation capabilities.
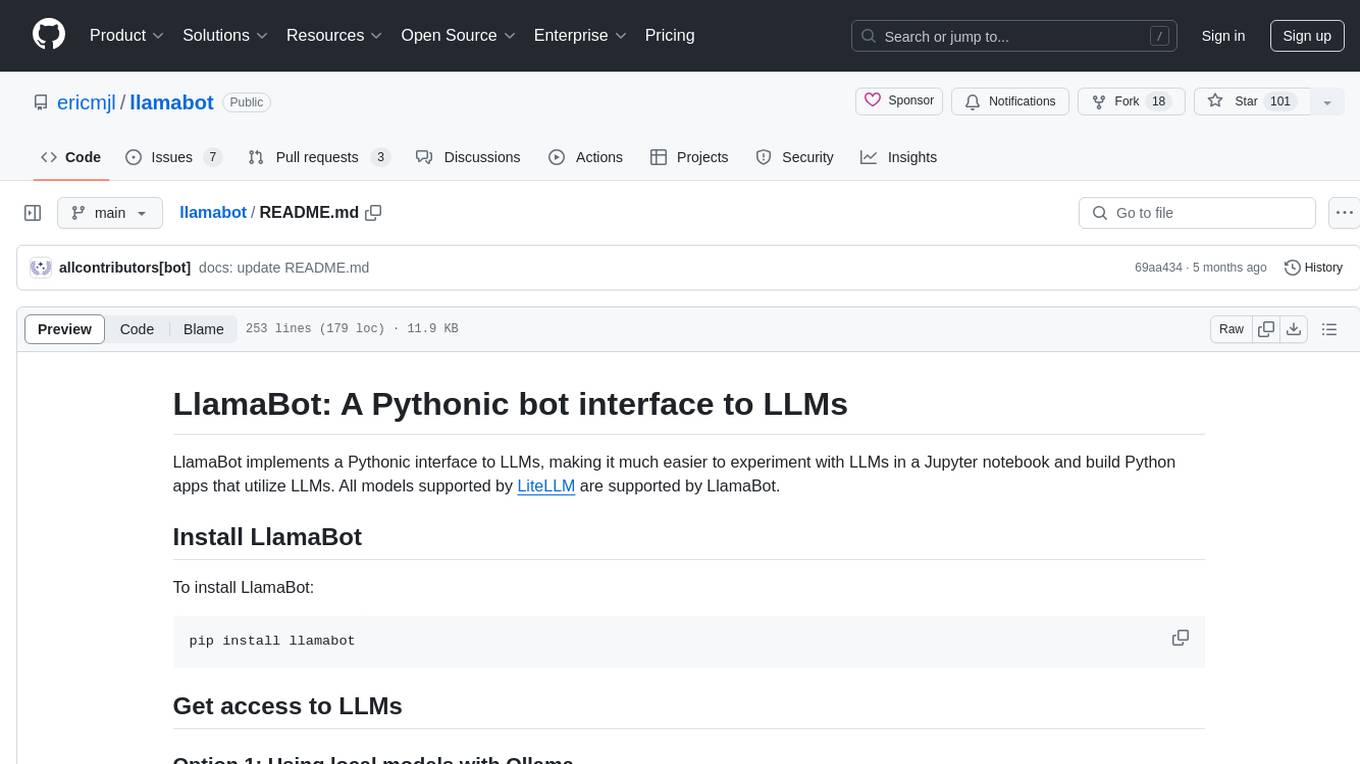
llamabot
LlamaBot is a Pythonic bot interface to Large Language Models (LLMs), providing an easy way to experiment with LLMs in Jupyter notebooks and build Python apps utilizing LLMs. It supports all models available in LiteLLM. Users can access LLMs either through local models with Ollama or by using API providers like OpenAI and Mistral. LlamaBot offers different bot interfaces like SimpleBot, ChatBot, QueryBot, and ImageBot for various tasks such as rephrasing text, maintaining chat history, querying documents, and generating images. The tool also includes CLI demos showcasing its capabilities and supports contributions for new features and bug reports from the community.
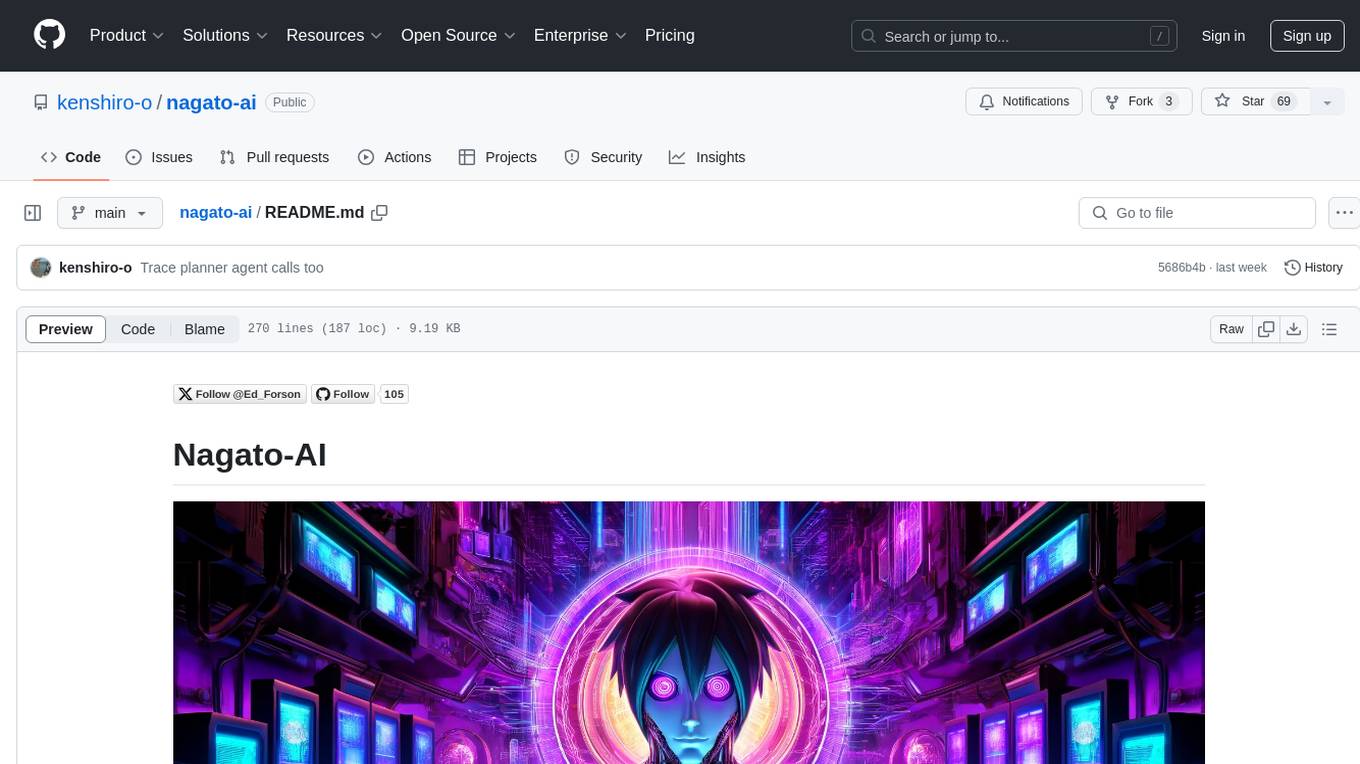
nagato-ai
Nagato-AI is an intuitive AI Agent library that supports multiple LLMs including OpenAI's GPT, Anthropic's Claude, Google's Gemini, and Groq LLMs. Users can create agents from these models and combine them to build an effective AI Agent system. The library is named after the powerful ninja Nagato from the anime Naruto, who can control multiple bodies with different abilities. Nagato-AI acts as a linchpin to summon and coordinate AI Agents for specific missions. It provides flexibility in programming and supports tools like Coordinator, Researcher, Critic agents, and HumanConfirmInputTool.
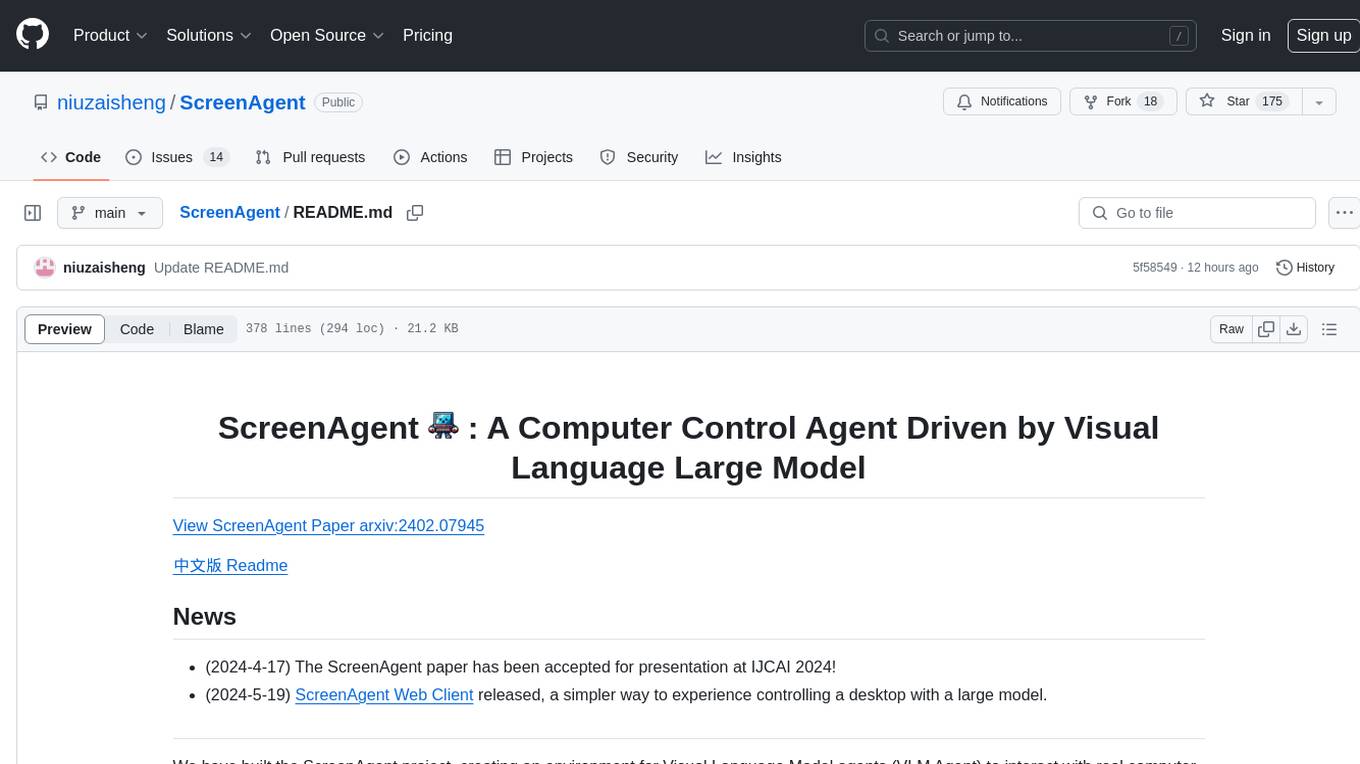
ScreenAgent
ScreenAgent is a project focused on creating an environment for Visual Language Model agents (VLM Agent) to interact with real computer screens. The project includes designing an automatic control process for agents to interact with the environment and complete multi-step tasks. It also involves building the ScreenAgent dataset, which collects screenshots and action sequences for various daily computer tasks. The project provides a controller client code, configuration files, and model training code to enable users to control a desktop with a large model.
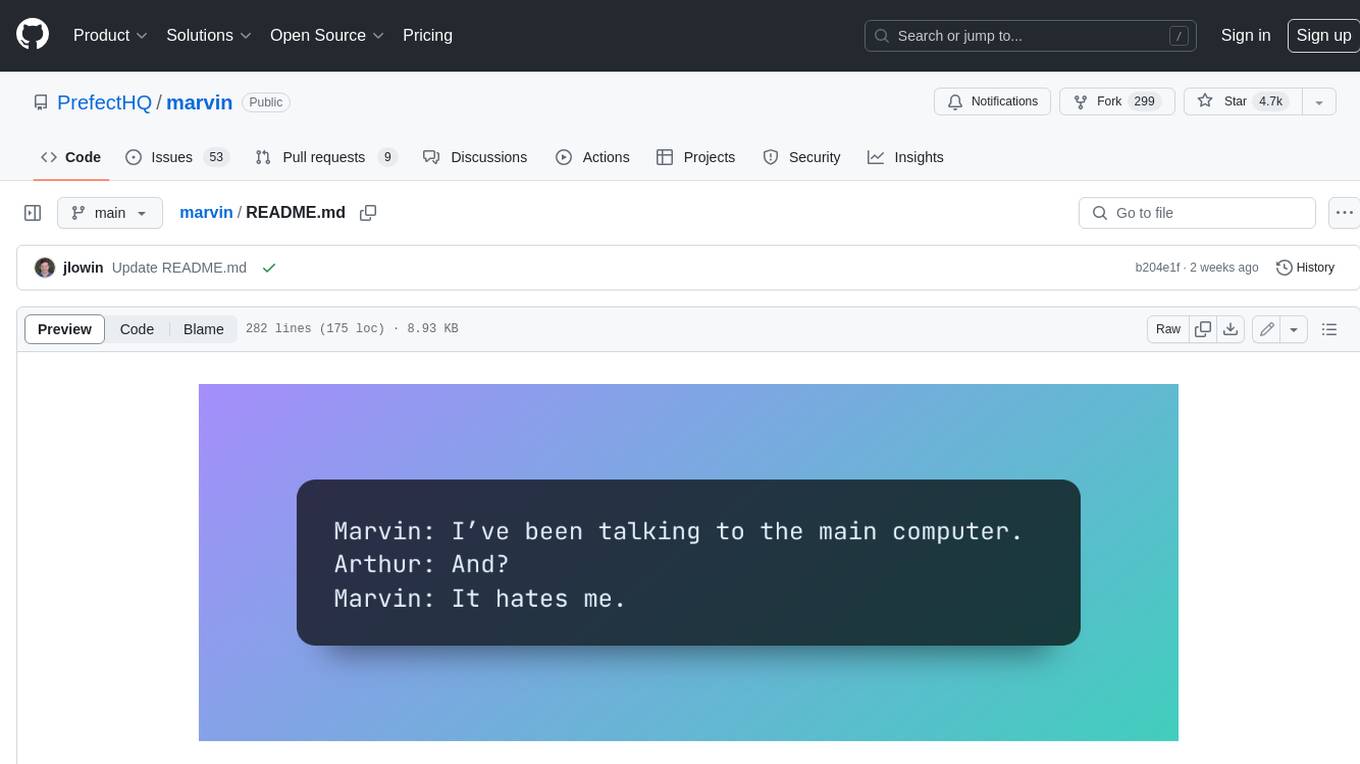
marvin
Marvin is a lightweight AI toolkit for building natural language interfaces that are reliable, scalable, and easy to trust. Each of Marvin's tools is simple and self-documenting, using AI to solve common but complex challenges like entity extraction, classification, and generating synthetic data. Each tool is independent and incrementally adoptable, so you can use them on their own or in combination with any other library. Marvin is also multi-modal, supporting both image and audio generation as well using images as inputs for extraction and classification. Marvin is for developers who care more about _using_ AI than _building_ AI, and we are focused on creating an exceptional developer experience. Marvin users should feel empowered to bring tightly-scoped "AI magic" into any traditional software project with just a few extra lines of code. Marvin aims to merge the best practices for building dependable, observable software with the best practices for building with generative AI into a single, easy-to-use library. It's a serious tool, but we hope you have fun with it. Marvin is open-source, free to use, and made with 💙 by the team at Prefect.
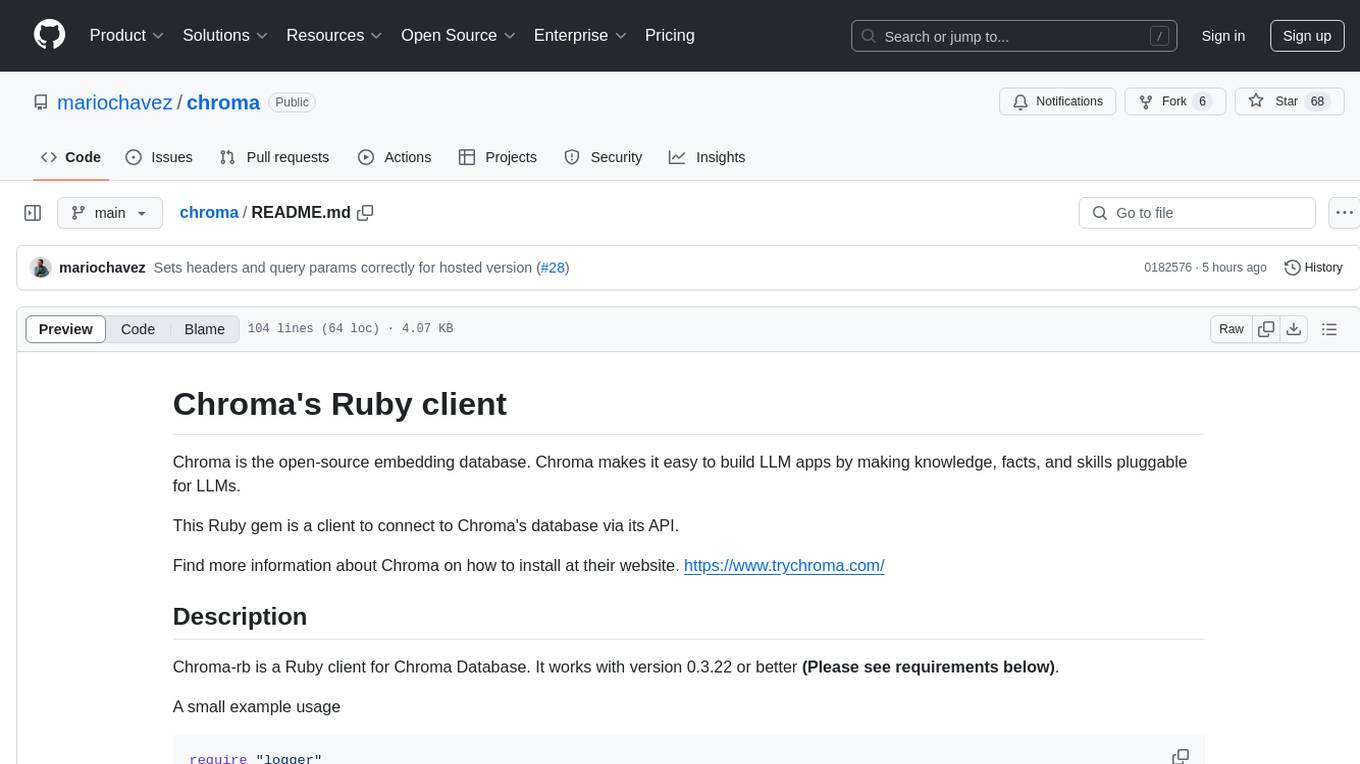
chroma
Chroma is an open-source embedding database that simplifies building LLM apps by enabling the integration of knowledge, facts, and skills for LLMs. The Ruby client for Chroma Database, chroma-rb, facilitates connecting to Chroma's database via its API. Users can configure the host, check server version, create collections, and add embeddings. The gem supports Chroma Database version 0.3.22 or newer, requiring Ruby 3.1.4 or later. It can be used with the hosted Chroma service at trychroma.com by setting configuration options like api_key, tenant, and database. Additionally, the gem provides integration with Jupyter Notebook for creating embeddings using Ollama and Nomic embed text with a Ruby HTTP client.
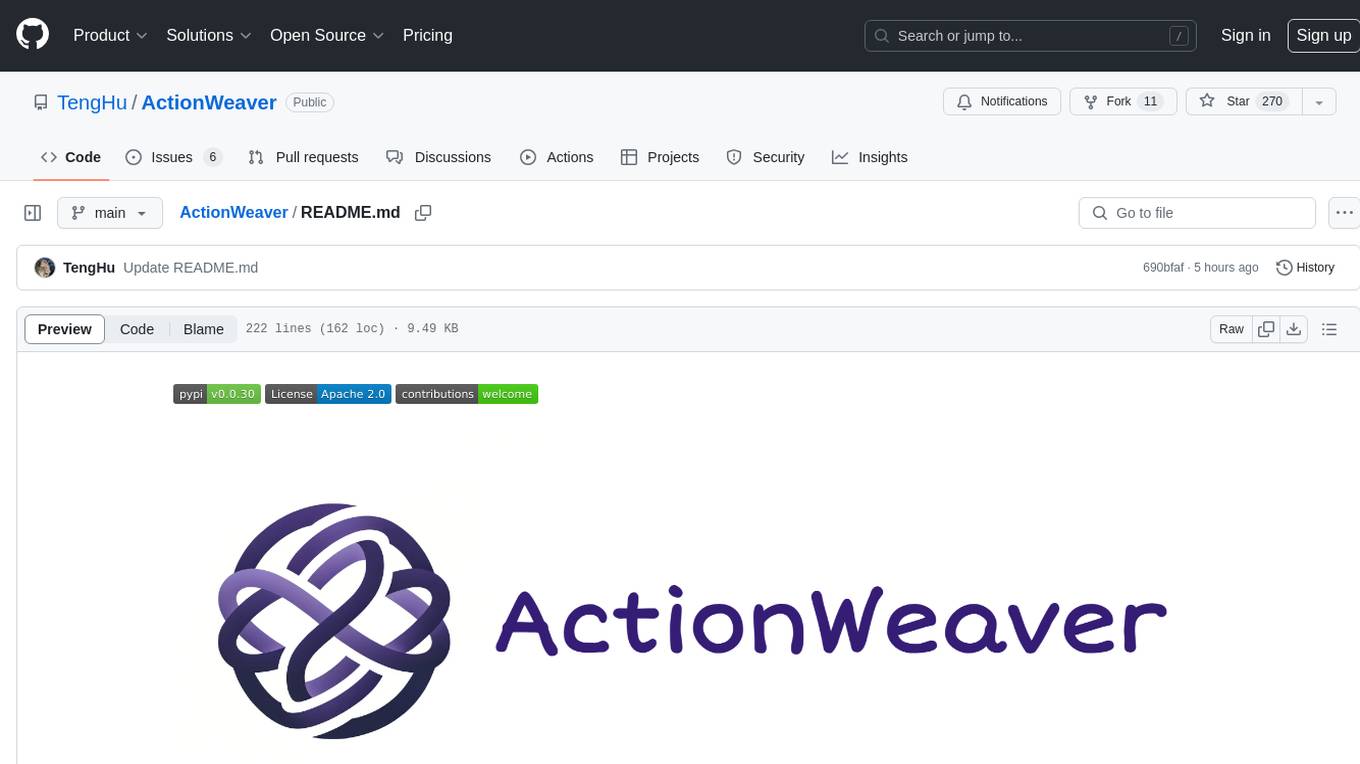
ActionWeaver
ActionWeaver is an AI application framework designed for simplicity, relying on OpenAI and Pydantic. It supports both OpenAI API and Azure OpenAI service. The framework allows for function calling as a core feature, extensibility to integrate any Python code, function orchestration for building complex call hierarchies, and telemetry and observability integration. Users can easily install ActionWeaver using pip and leverage its capabilities to create, invoke, and orchestrate actions with the language model. The framework also provides structured extraction using Pydantic models and allows for exception handling customization. Contributions to the project are welcome, and users are encouraged to cite ActionWeaver if found useful.
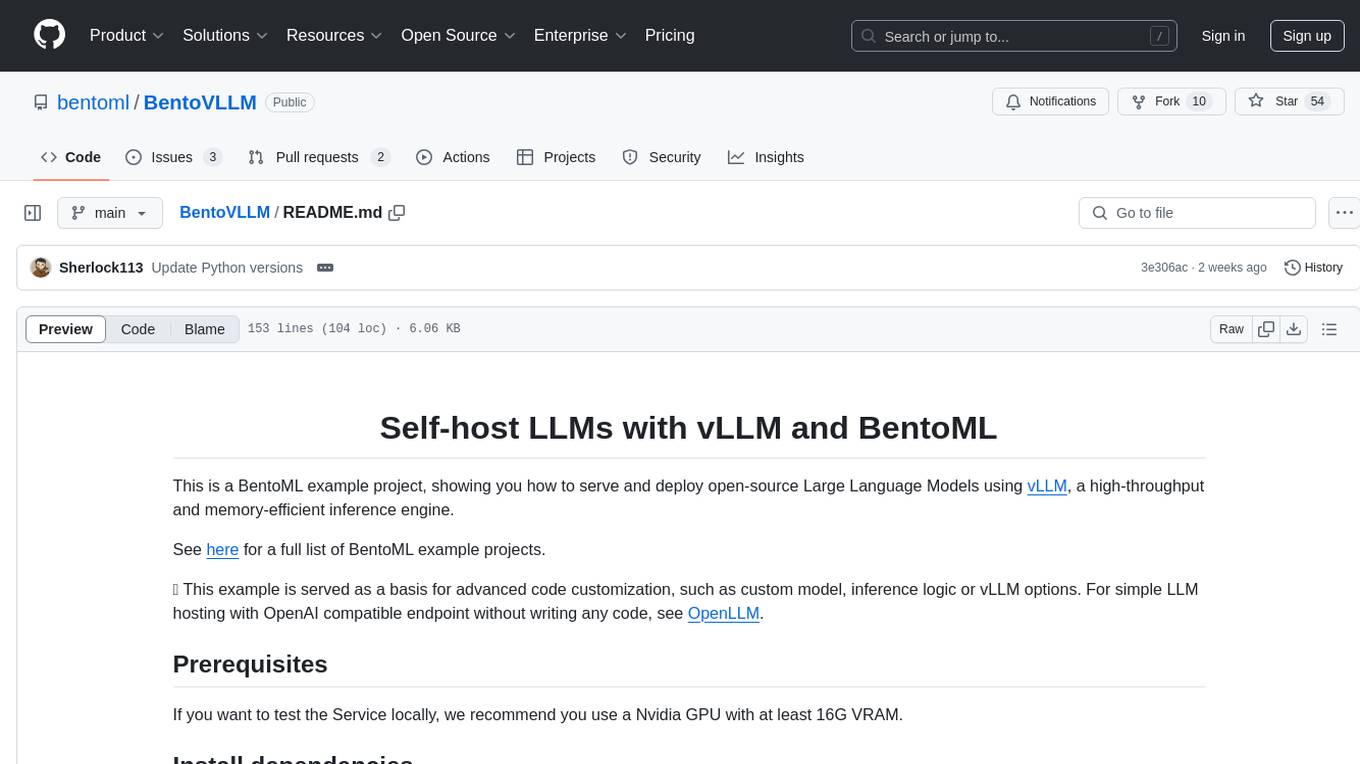
BentoVLLM
BentoVLLM is an example project demonstrating how to serve and deploy open-source Large Language Models using vLLM, a high-throughput and memory-efficient inference engine. It provides a basis for advanced code customization, such as custom models, inference logic, or vLLM options. The project allows for simple LLM hosting with OpenAI compatible endpoints without the need to write any code. Users can interact with the server using Swagger UI or other methods, and the service can be deployed to BentoCloud for better management and scalability. Additionally, the repository includes integration examples for different LLM models and tools.
For similar tasks
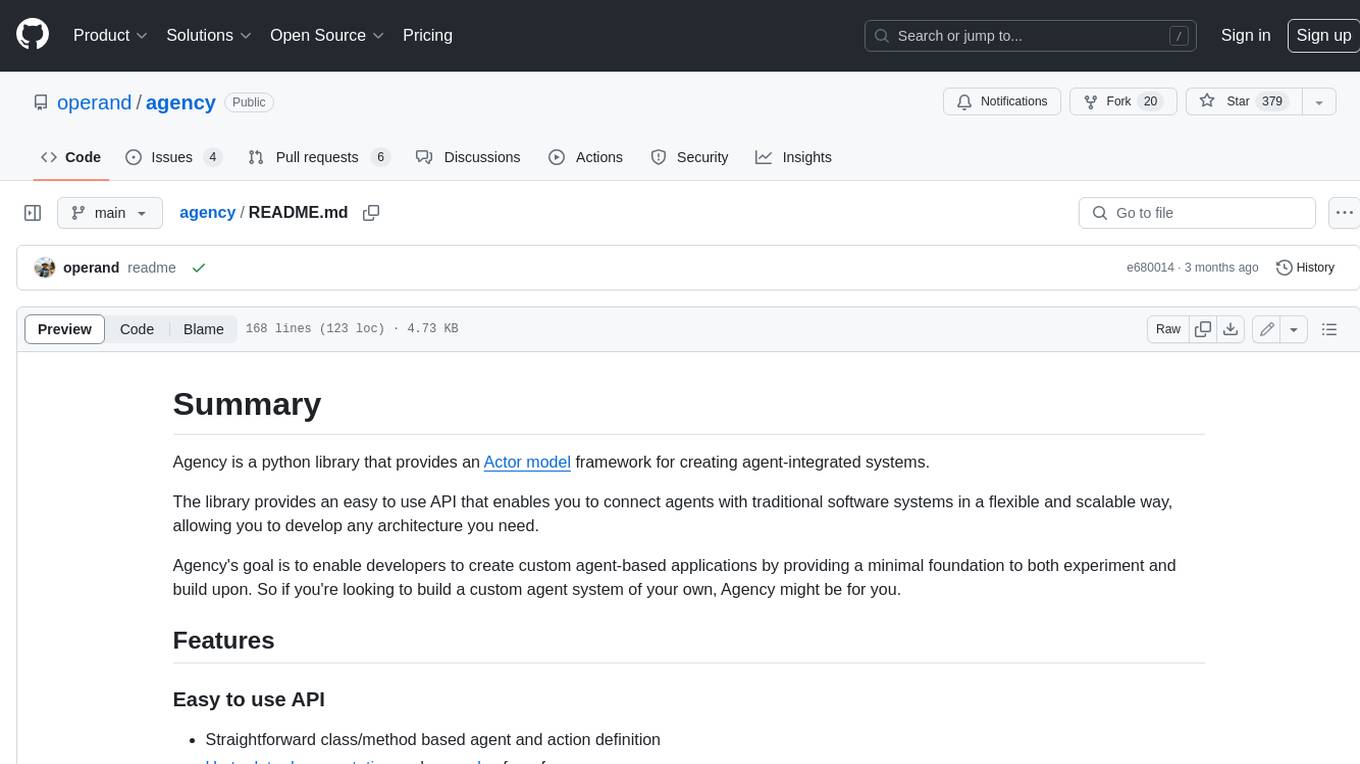
agency
Agency is a python library that provides an Actor model framework for creating agent-integrated systems. It offers an easy-to-use API for connecting agents with traditional software systems, enabling flexible and scalable architectures. Agency aims to empower developers in creating custom agent-based applications by providing a foundation for experimentation and development. Key features include an intuitive API, performance and scalability through multiprocessing and AMQP support, observability and control with action and lifecycle callbacks, access policies, and detailed logging. The library also includes a demo application with multiple agent examples, OpenAI agent examples, HuggingFace transformers agent example, operating system access, Gradio UI, and Docker configuration for reference and development.
For similar jobs
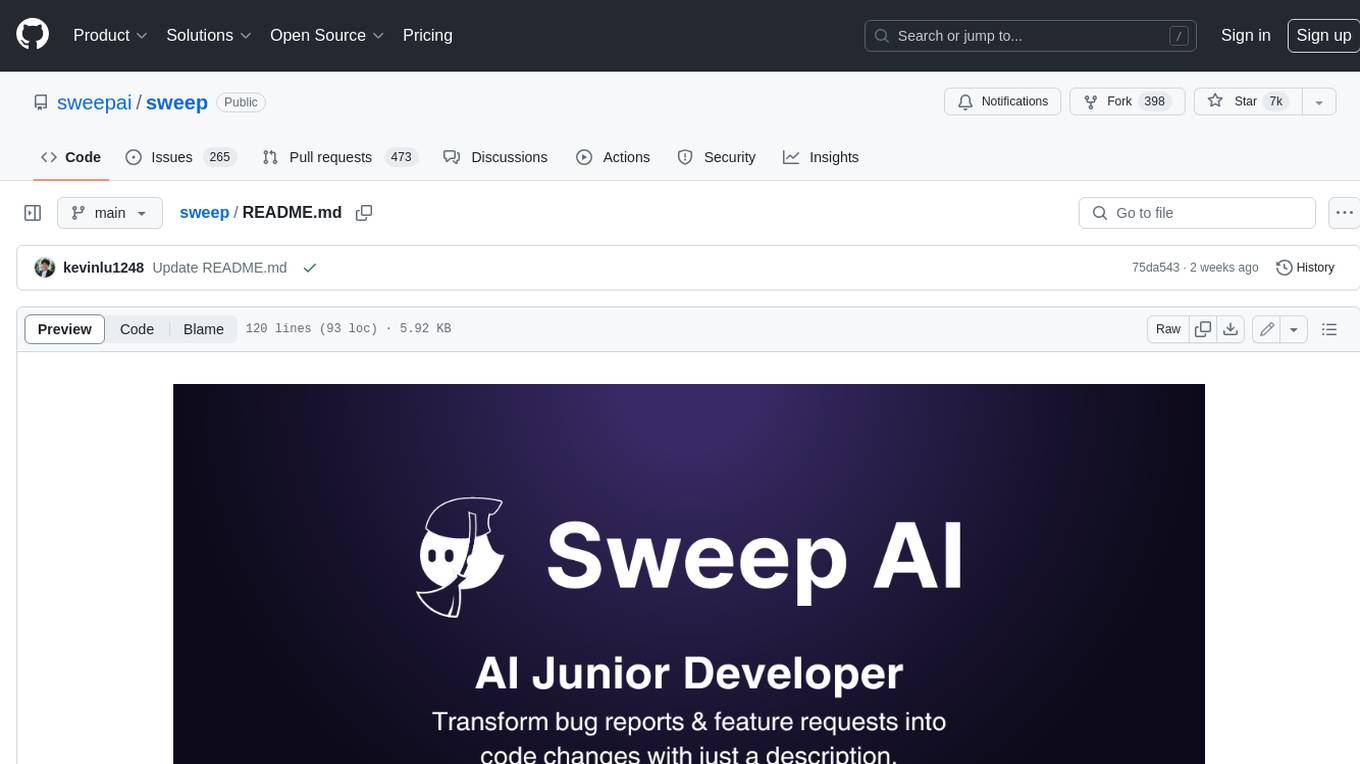
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
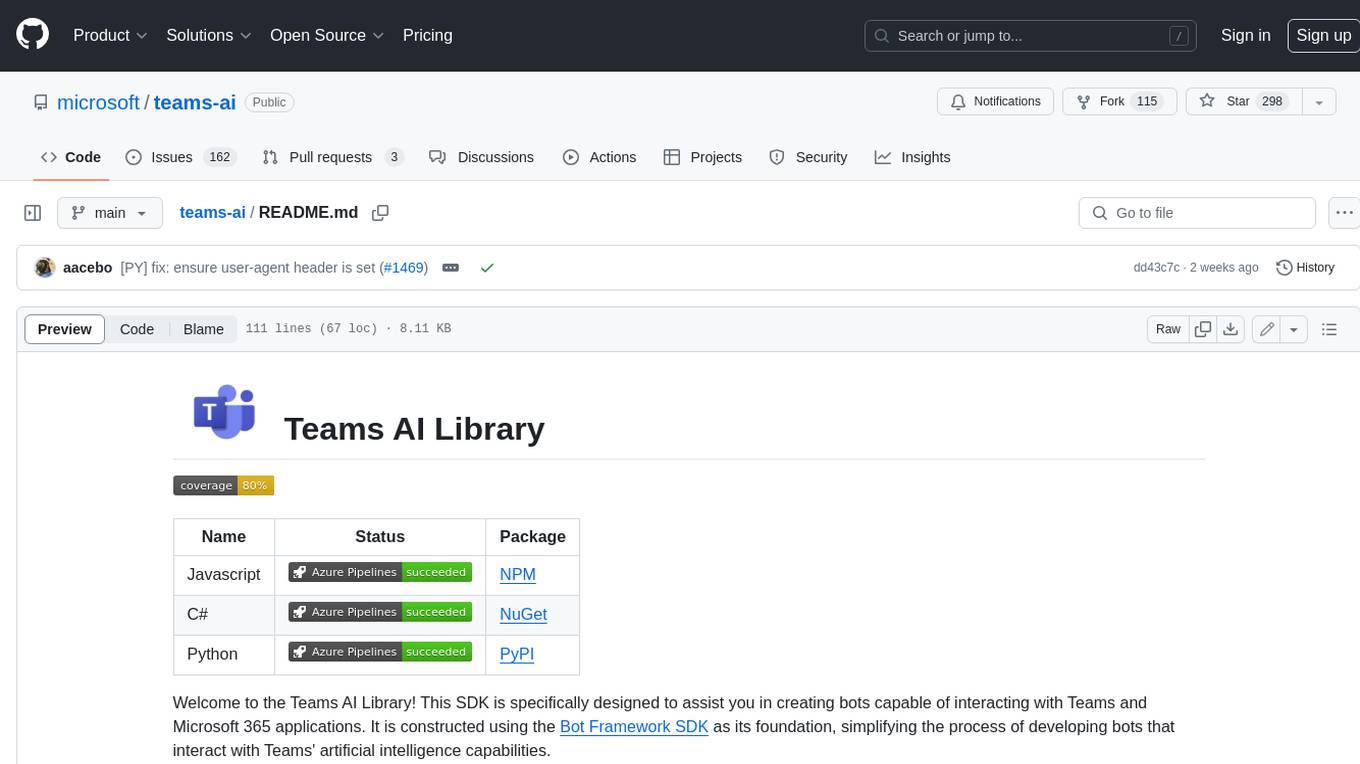
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
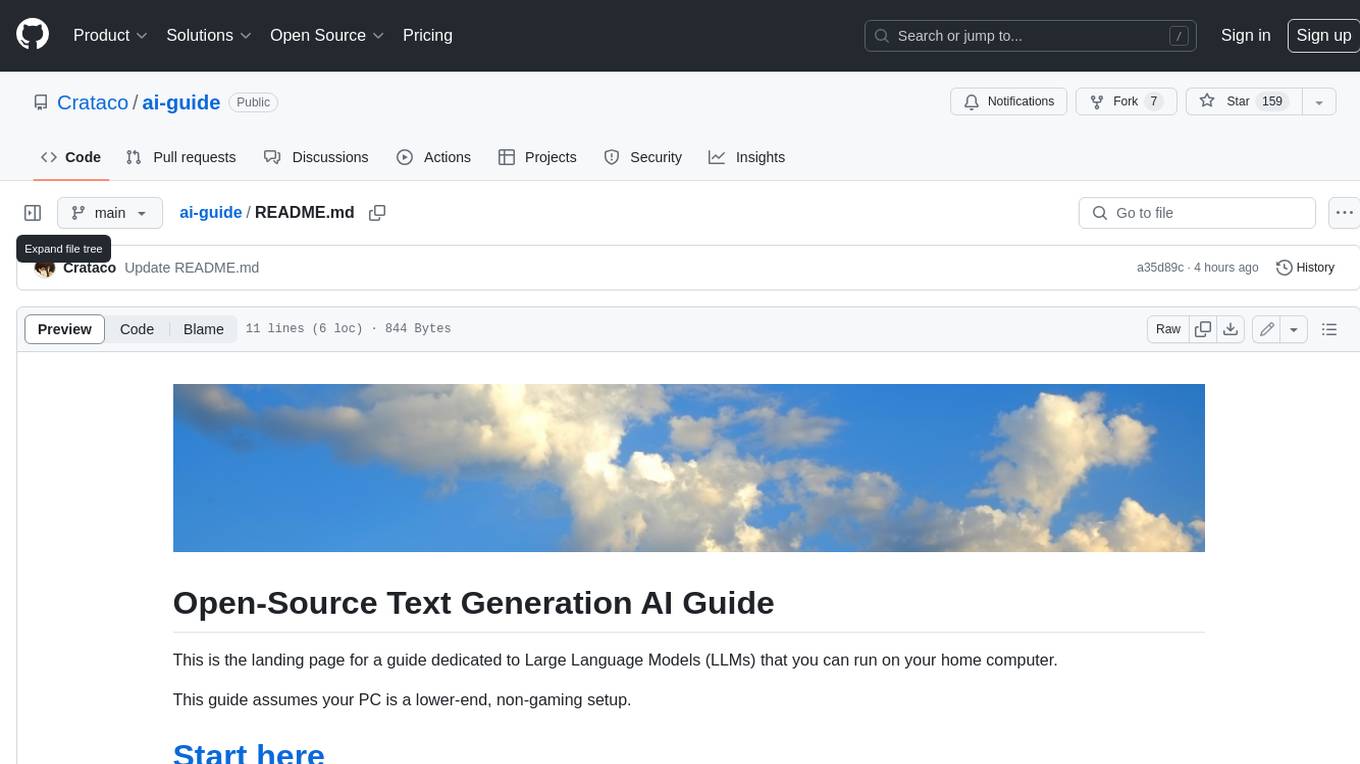
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
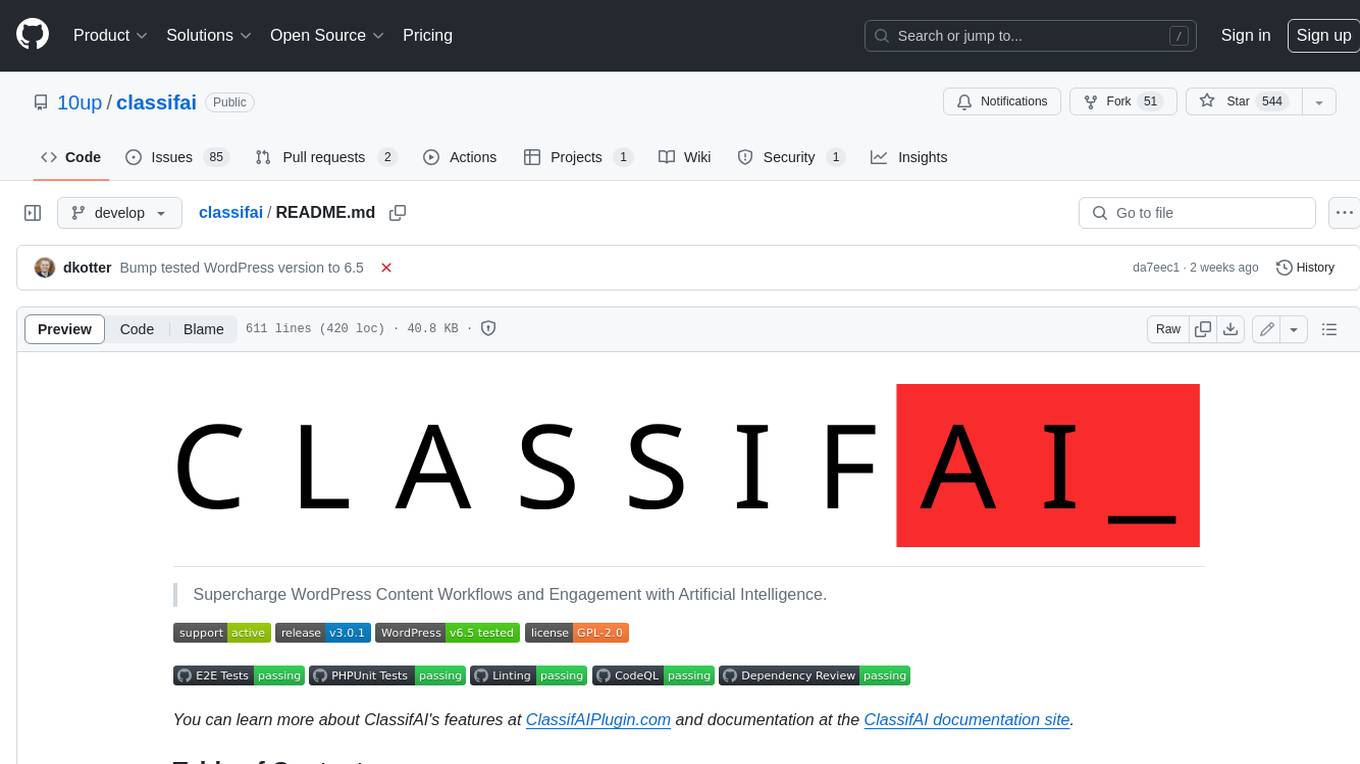
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
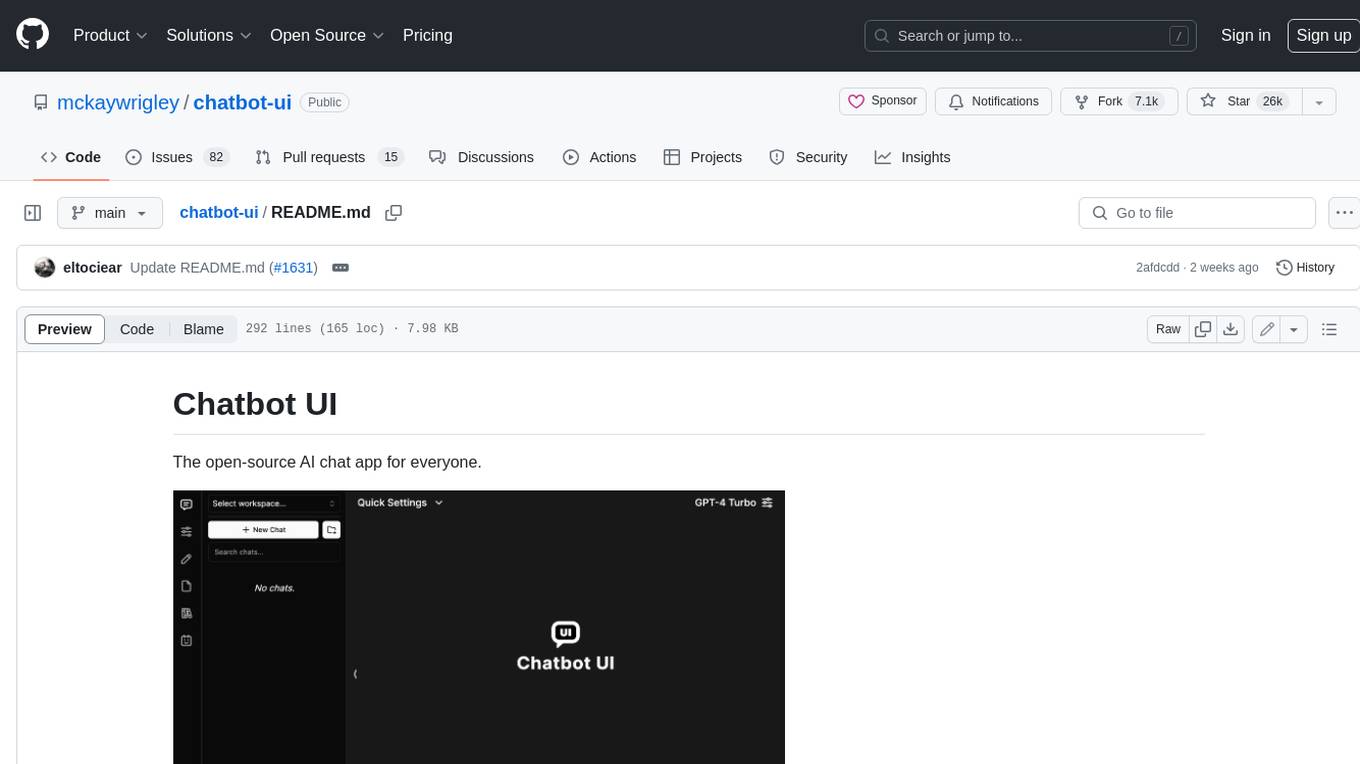
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
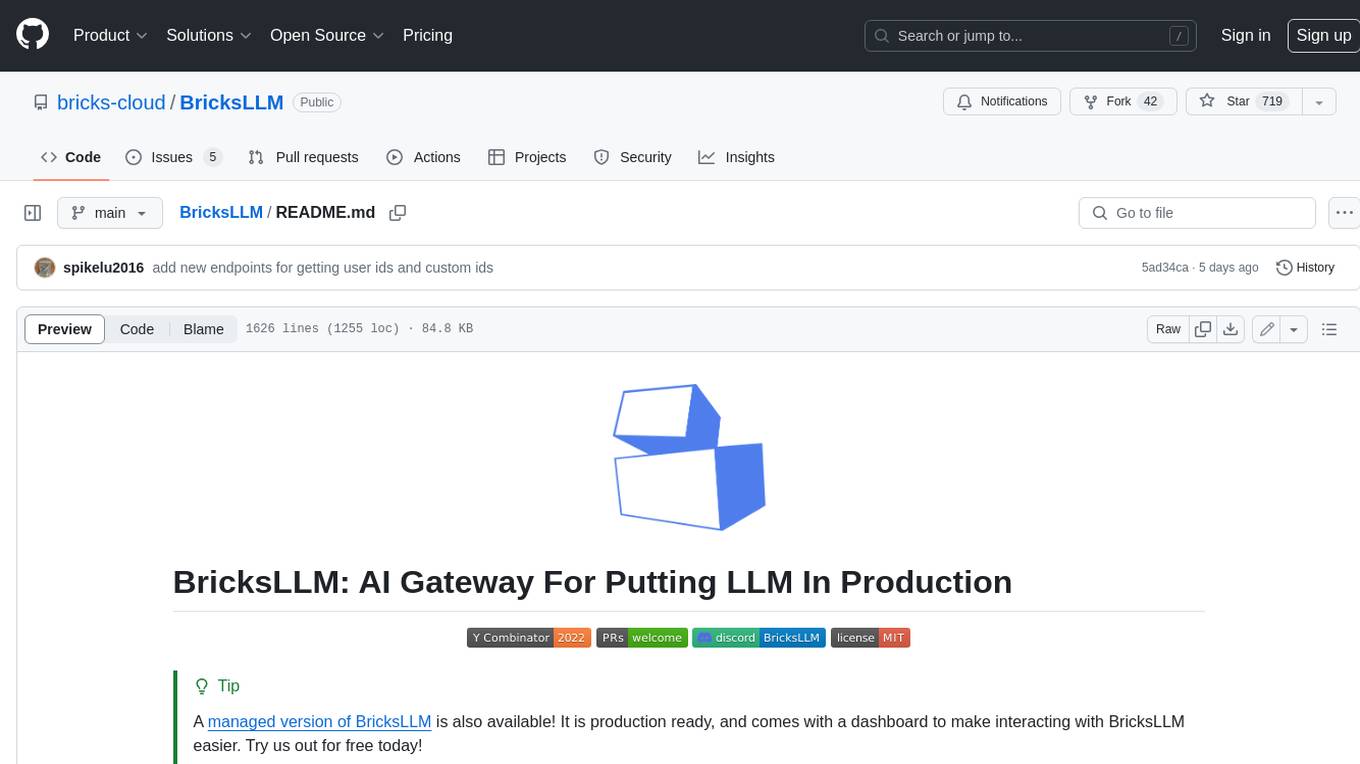
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
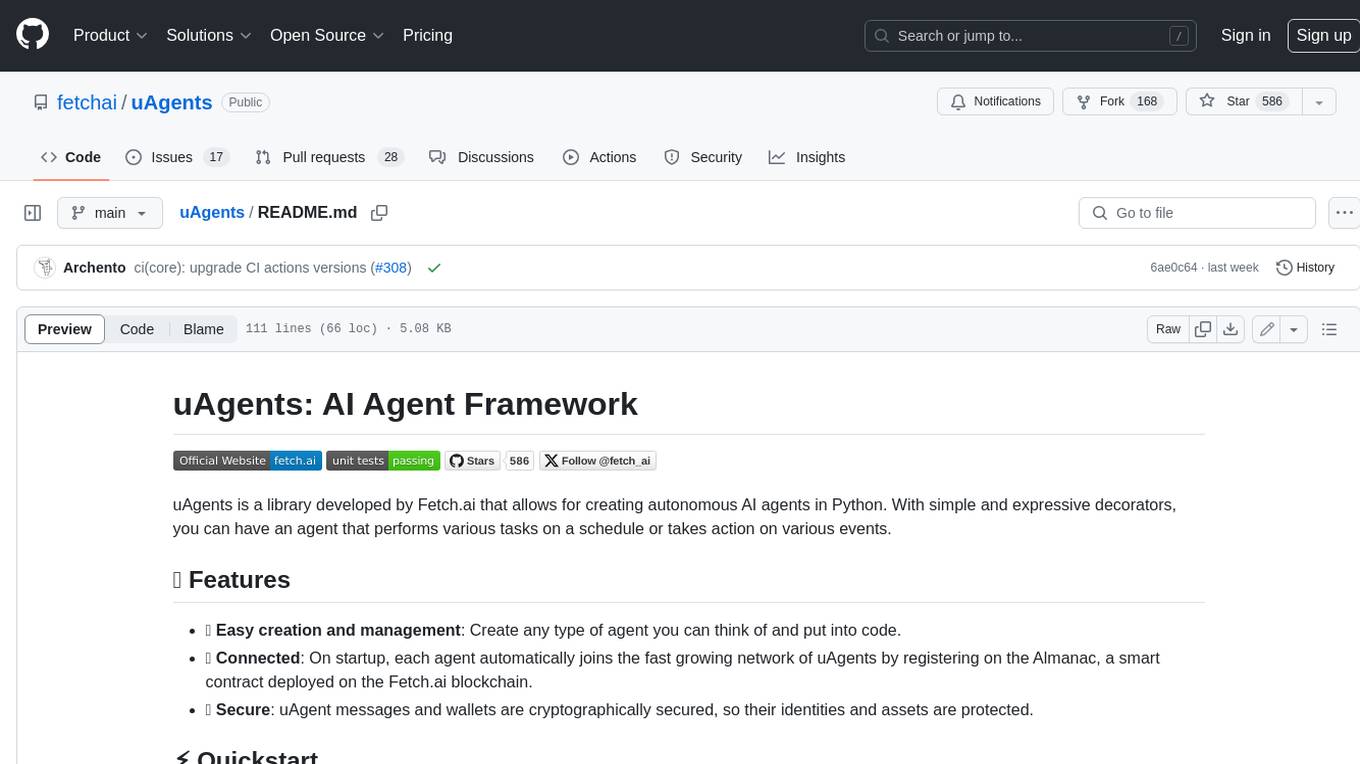
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
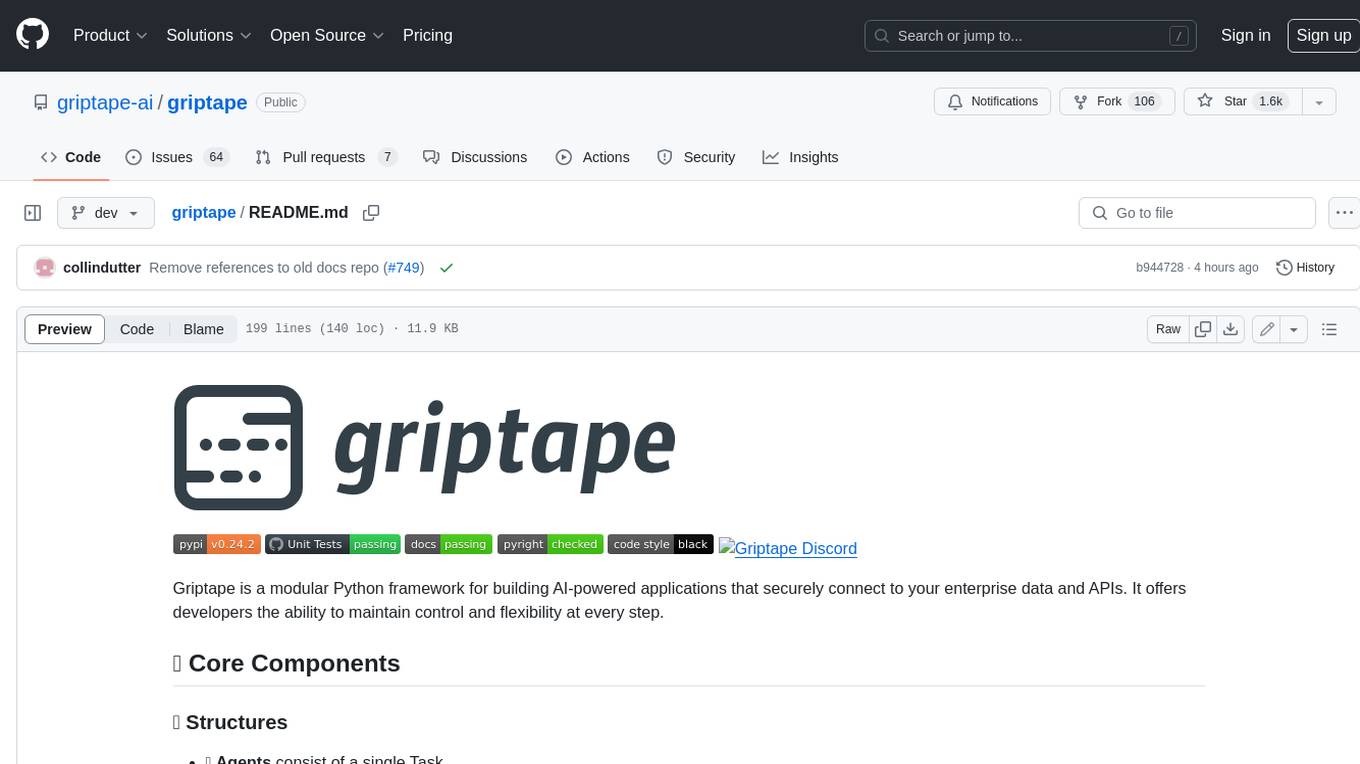
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.