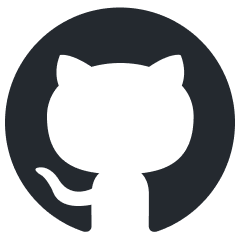
maiar-ai
Maiar: A Composable, Plugin-Based AI Agent Framework
Stars: 63
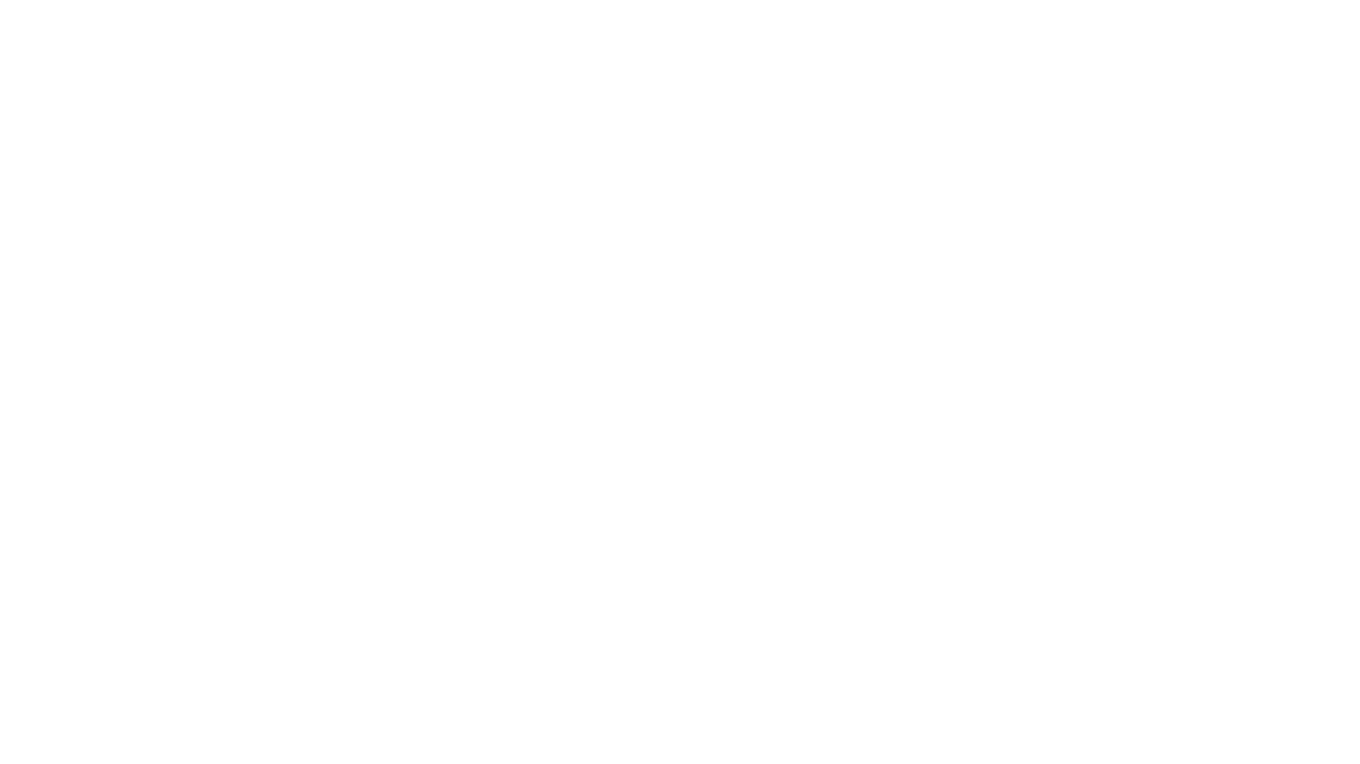
MAIAR is a composable, plugin-based AI agent framework designed to abstract data ingestion, decision-making, and action execution into modular plugins. It enables developers to define triggers and actions as standalone plugins, while the core runtime handles decision-making dynamically. This framework offers extensibility, composability, and model-driven behavior, allowing seamless addition of new functionality. MAIAR's architecture is influenced by Unix pipes, ensuring highly composable plugins, dynamic execution pipelines, and transparent debugging. It remains declarative and extensible, allowing developers to build complex AI workflows without rigid architectures.
README:
MAIAR is designed around the thesis that AI agents, in their current iteration, primarily consist of three major steps:
- Data Ingestion & Triggers – What causes the AI to act.
- Decision-Making – How the AI determines the appropriate action.
- Action Execution – Carrying out the selected operation.
Instead of rigid workflows or monolithic agent logic, Maiar abstracts these steps into a modular, plugin-based system. Developers define triggers and actions as standalone plugins, while the core runtime dynamically handles decision-making. This enables a highly extensible, composable, and model driven framework where new functionality can be added seamlessly.
- Getting Started Running MAIAR
- Getting Started Contributing to MAIAR
- How It Works
- Pipes & Context Chains
- Extensibility & Modularity
- Design Principles
Welcome to MAIAR! This guide will help you set up and run your own AI agent using the MAIAR framework.
Before you begin, make sure you have the following installed:
nvm install 22.13.1
nvm use 22.13.1
- Create a new directory for your project and initialize it:
mkdir my-maiar-agent
cd my-maiar-agent
pnpm init
- Install the core MAIAR packages, providers, and some starter plugins:
pnpm add @maiar-ai/core @maiar-ai/model-openai @maiar-ai/memory-sqlite @maiar-ai/plugin-terminal @maiar-ai/plugin-text dotenv
- Create a new directory called
src
in your project root and create a new file calledindex.ts
in it:
import "dotenv/config";
import path from "path";
import { createRuntime } from "@maiar-ai/core";
import { OpenAIProvider } from "@maiar-ai/model-openai";
import { SQLiteProvider } from "@maiar-ai/memory-sqlite";
import { ConsoleMonitorProvider } from "@maiar-ai/monitor-console";
import { PluginTerminal } from "@maiar-ai/plugin-terminal";
import { PluginTextGeneration } from "@maiar-ai/plugin-text";
const runtime = createRuntime({
models: [
new OpenAIProvider({
apiKey: process.env.OPENAI_API_KEY as string,
model: "gpt-3.5-turbo"
})
],
memory: new SQLiteProvider({
dbPath: path.join(process.cwd(), "data", "conversations.db")
}),
monitors: [new ConsoleMonitorProvider()],
plugins: [
new PluginTextGeneration(),
new PluginTerminal({ user: "test", agentName: "maiar-starter" })
]
});
// Start the runtime
console.log("Starting agent...");
runtime.start().catch((error) => {
console.error("Failed to start agent:", error);
process.exit(1);
});
// Handle shutdown gracefully
process.on("SIGINT", async () => {
console.log("Shutting down agent...");
await runtime.stop();
process.exit(0);
});
- Create a
.env
file in your project root. For our example, we'll use the OpenAI API key:
[!INFO] Since we're using OpenAI's API for this quickstart, you'll need to:
- Create an OpenAI account at platform.openai.com
- Generate an API key in your account settings
- Add funds to your account to use the API
- Create a
.env
file with your API key as shown below
OPENAI_API_KEY=your_api_key_here
- Add TypeScript configuration. Create a
tsconfig.json
:
{
"compilerOptions": {
"target": "ES2020",
"module": "CommonJS",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist"
},
"include": ["src/**/*.ts"],
"exclude": ["node_modules"]
}
- Add build and start scripts to your
package.json
under thescripts
section:
"build": "tsc",
"start": "node dist/index.js"
- Build and start your agent:
pnpm build
pnpm start
- Test your agent by starting
maiar-chat
:
pnpm maiar-chat
You should see a terminal prompt to chat with your agent.
[!IMPORTANT]
Please make sure to checkout the contributing guide first and foremost
- Make sure you have the following installed:
nvm install 22.13.1
nvm use 22.13.1
- The pnpm package manager explicitly - Required for managing the monorepo workspace and its dependencies efficiently
- Install the project dependencies:
# From the root of the repository
pnpm install
- Start the development environment. You'll need two terminal windows:
Terminal 1 - Core Packages:
# From the root of the repository
pnpm dev
This command watches for changes in the core packages (packages/**/*.ts
) and automatically rebuilds them. It:
- Cleans any previous build state
- Builds all core packages
- Updates the
.build-complete
marker file with current timestamp to indicate the core packages build is finished as a state file to communicate with the development project - Watches for changes and repeats the process
Terminal 2 - Development Project:
# From the root of the repository
cd maiar-starter # or your own development project
pnpm dev
This command runs the starter project in development mode. It:
- Watches for changes in both the starter project's source files and the core packages through the
.build-complete
marker file - Rebuilds the starter project
- Restarts the application automatically and handles exiting gracefully so orphaned processes are cleaned up
This setup ensures that changes to either the core packages or the starter project are automatically rebuilt and reflected in your running application, providing a seamless development experience.
[!NOTE]
The
maiar-starter
project serves as a reference implementation demonstrating how to develop against the core MAIAR packages. You can apply this same development setup to any project that depends on MAIAR packages - simply mirror the dev script configuration and.build-complete
marker file handling shown in the starter project's package.json. The key focus of this repository is the core packages inpackages/*
, withmaiar-starter
serving as an example consumer.
At its core, MAIAR builds execution pipelines dynamically. When an event or request is received, the runtime:
- Processes triggers to determine when and how the AI should act.
- Uses model assisted reasoning to construct an execution pipeline.
- Runs plugins in sequence, modifying a structured context chain as it progresses.
Rather than hardcoding client logic, MAIAR produces emergent behavior by selecting the most relevant plugins and actions based on context. This enables adaptability and ensures that agents can evolve without rewriting core logic.
MAIAR's architecture is influenced by Unix pipes, where structured input flows through a sequence of operations, using a standard in and out data interface. Each plugin acts as an independent unit:
- Receives input (context) from prior steps
- Performs a specific operation
- Outputs a structured result to the next step
This structured context chain ensures:
- Highly composable plugins – New functionality can be added without modifying existing logic.
- Dynamic execution pipelines – Workflows are built on-the-fly rather than being hardcoded.
- Transparent debugging & monitoring – Each step in the chain is tracked and can be audited.
This design enables MAIAR to remain declarative and extensible, allowing developers to build complex AI workflows without locking themselves into rigid architectures.
MAIAR is intentionally unopinionated about external dependencies, ensuring developers have full control over their infrastructure. The framework avoids enforcing specific technologies, making it easy to integrate with:
- Database Adapters – Works with any database system.
- Model Providers – Supports OpenAI, local models, or custom integrations.
- Logging & Monitoring – Custom logging systems can be plugged in without modifying core logic.
- Future Expansions – As needs evolve, new capabilities can be added without disrupting existing workflows.
By maintaining a flexible core, MAIAR ensures that AI agents can adapt to different environments and use cases without unnecessary constraints.
- Plugin-First – Every capability, from event ingestion to action execution, is encapsulated in a plugin.
- Modular & Composable – No rigid loops, no hardcoded workflows. The agent dynamically assembles execution pipelines.
- Model Driven Behavior – Instead of pre-defined workflows, the AI evaluates its available tools and selects the best course of action.
- Declarative Plugin Interface – Plugins declare their triggers and actions, while the runtime orchestrates them.
- Pipes & Context Chains – Input flows through plugins in a structured sequence, mirroring Unix pipes.
- Extensibility & Flexibility – The core library avoids enforcing specific tools or integrations. It's designed around interfaces and providers that allow you to plug in your own tools and integrations.
- Effortless Development – Define a plugin, specify its triggers & actions, and the agent handles the rest.
- Dynamic AI Workflows – Pipelines are built on-the-fly, allowing flexible and emergent behavior.
- Composability-First – Standardized context chains make plugins reusable and easily integrable.
- Unopinionated & Extensible – Developers have full control over databases, models, and infrastructure choices.
MAIAR isn't just another AI agent framework—it's a declarative, extensible, and composable way to build intelligent applications. Whether you're adding new capabilities or integrating with existing platforms, MAIAR makes it simple.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for maiar-ai
Similar Open Source Tools
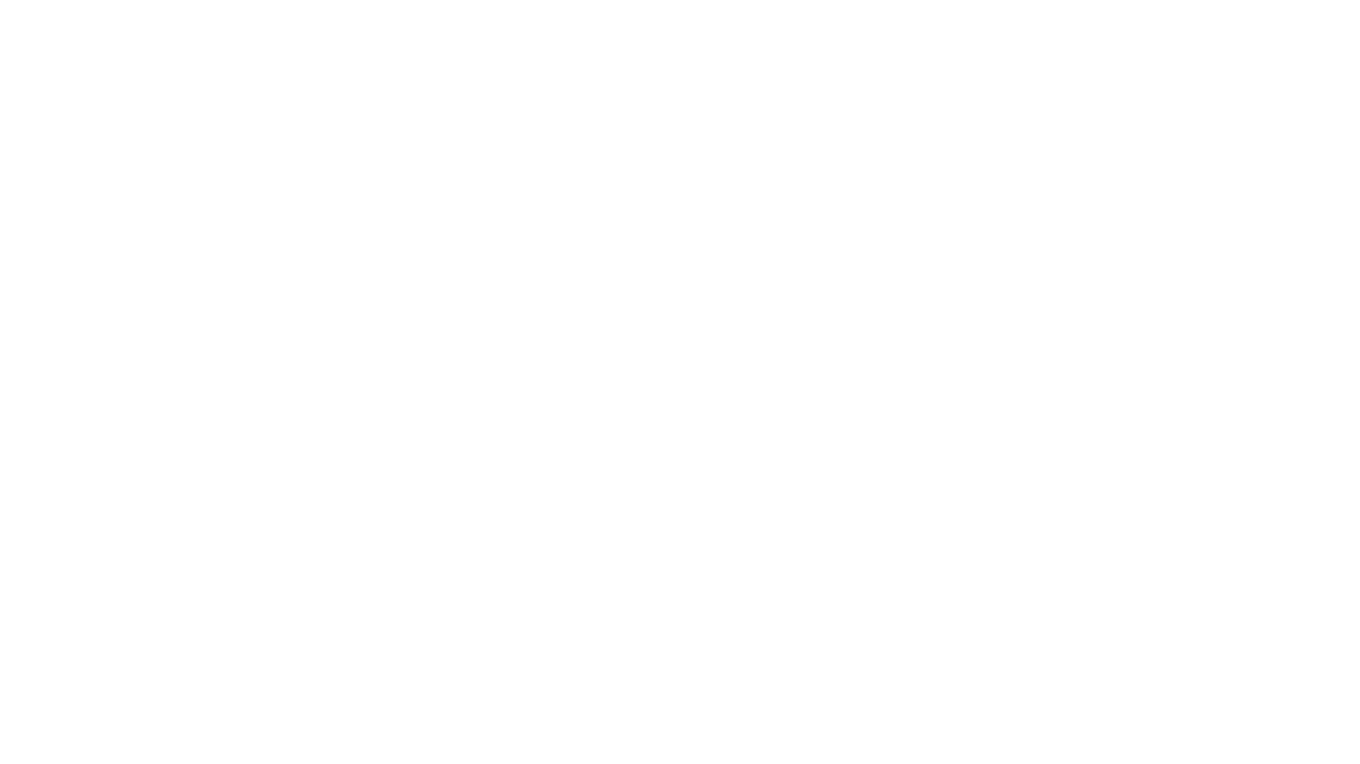
maiar-ai
MAIAR is a composable, plugin-based AI agent framework designed to abstract data ingestion, decision-making, and action execution into modular plugins. It enables developers to define triggers and actions as standalone plugins, while the core runtime handles decision-making dynamically. This framework offers extensibility, composability, and model-driven behavior, allowing seamless addition of new functionality. MAIAR's architecture is influenced by Unix pipes, ensuring highly composable plugins, dynamic execution pipelines, and transparent debugging. It remains declarative and extensible, allowing developers to build complex AI workflows without rigid architectures.
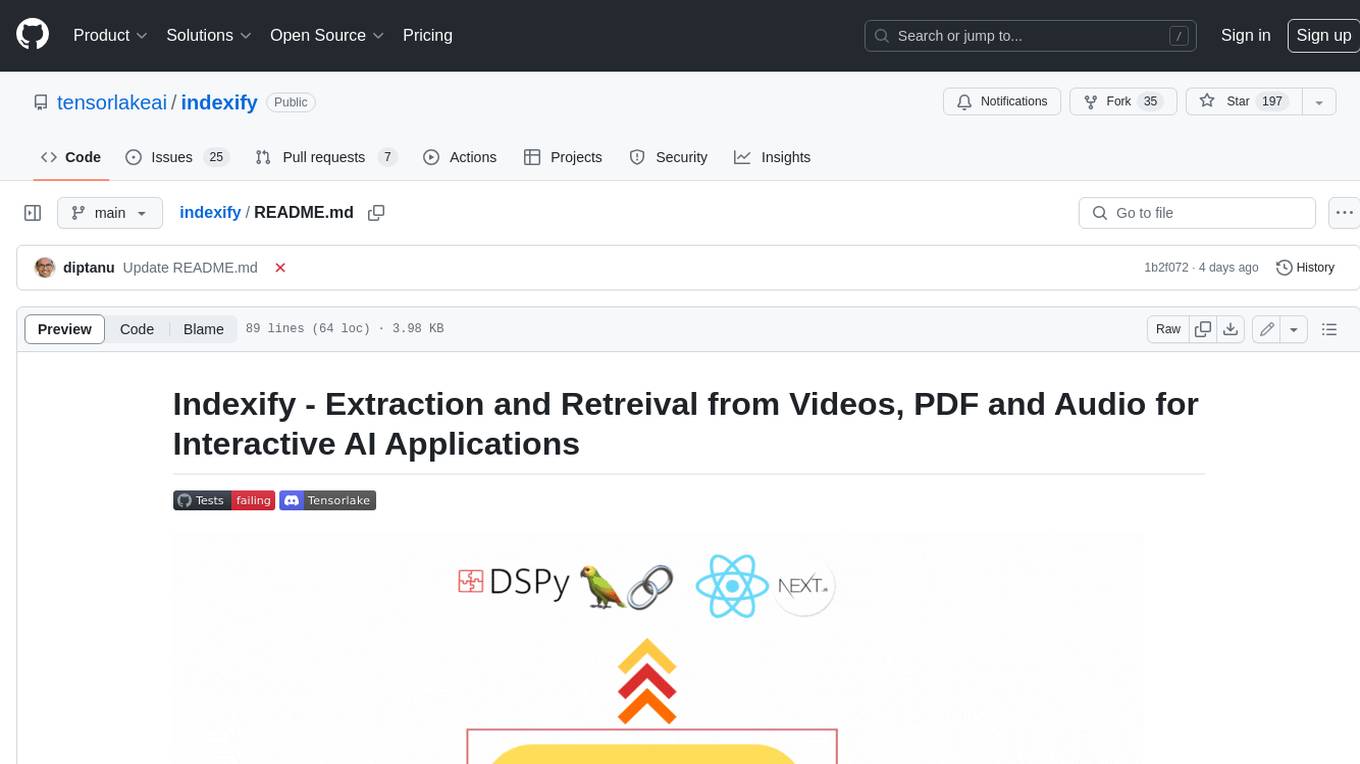
indexify
Indexify is an open-source engine for building fast data pipelines for unstructured data (video, audio, images, and documents) using reusable extractors for embedding, transformation, and feature extraction. LLM Applications can query transformed content friendly to LLMs by semantic search and SQL queries. Indexify keeps vector databases and structured databases (PostgreSQL) updated by automatically invoking the pipelines as new data is ingested into the system from external data sources. **Why use Indexify** * Makes Unstructured Data **Queryable** with **SQL** and **Semantic Search** * **Real-Time** Extraction Engine to keep indexes **automatically** updated as new data is ingested. * Create **Extraction Graph** to describe **data transformation** and extraction of **embedding** and **structured extraction**. * **Incremental Extraction** and **Selective Deletion** when content is deleted or updated. * **Extractor SDK** allows adding new extraction capabilities, and many readily available extractors for **PDF**, **Image**, and **Video** indexing and extraction. * Works with **any LLM Framework** including **Langchain**, **DSPy**, etc. * Runs on your laptop during **prototyping** and also scales to **1000s of machines** on the cloud. * Works with many **Blob Stores**, **Vector Stores**, and **Structured Databases** * We have even **Open Sourced Automation** to deploy to Kubernetes in production.
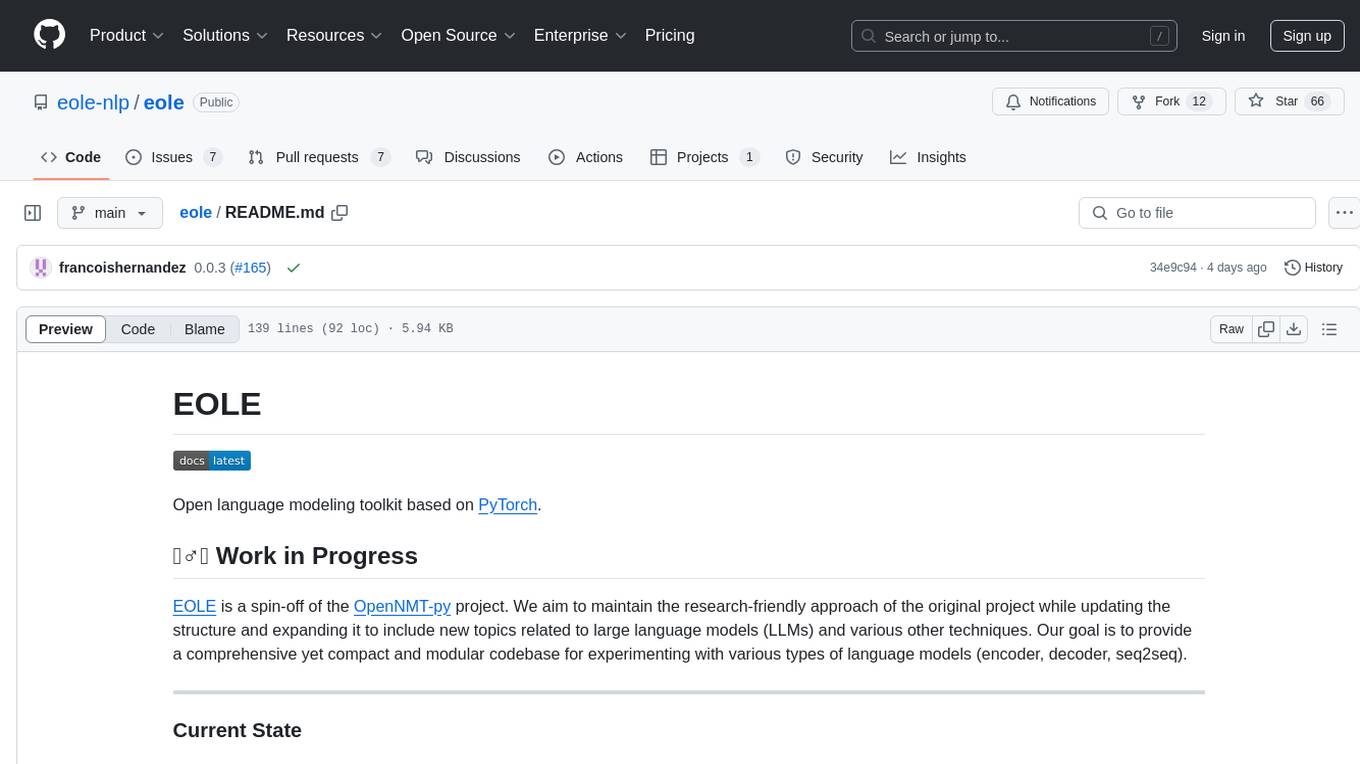
eole
EOLE is an open language modeling toolkit based on PyTorch. It aims to provide a research-friendly approach with a comprehensive yet compact and modular codebase for experimenting with various types of language models. The toolkit includes features such as versatile training and inference, dynamic data transforms, comprehensive large language model support, advanced quantization, efficient finetuning, flexible inference, and tensor parallelism. EOLE is a work in progress with ongoing enhancements in configuration management, command line entry points, reproducible recipes, core API simplification, and plans for further simplification, refactoring, inference server development, additional recipes, documentation enhancement, test coverage improvement, logging enhancements, and broader model support.
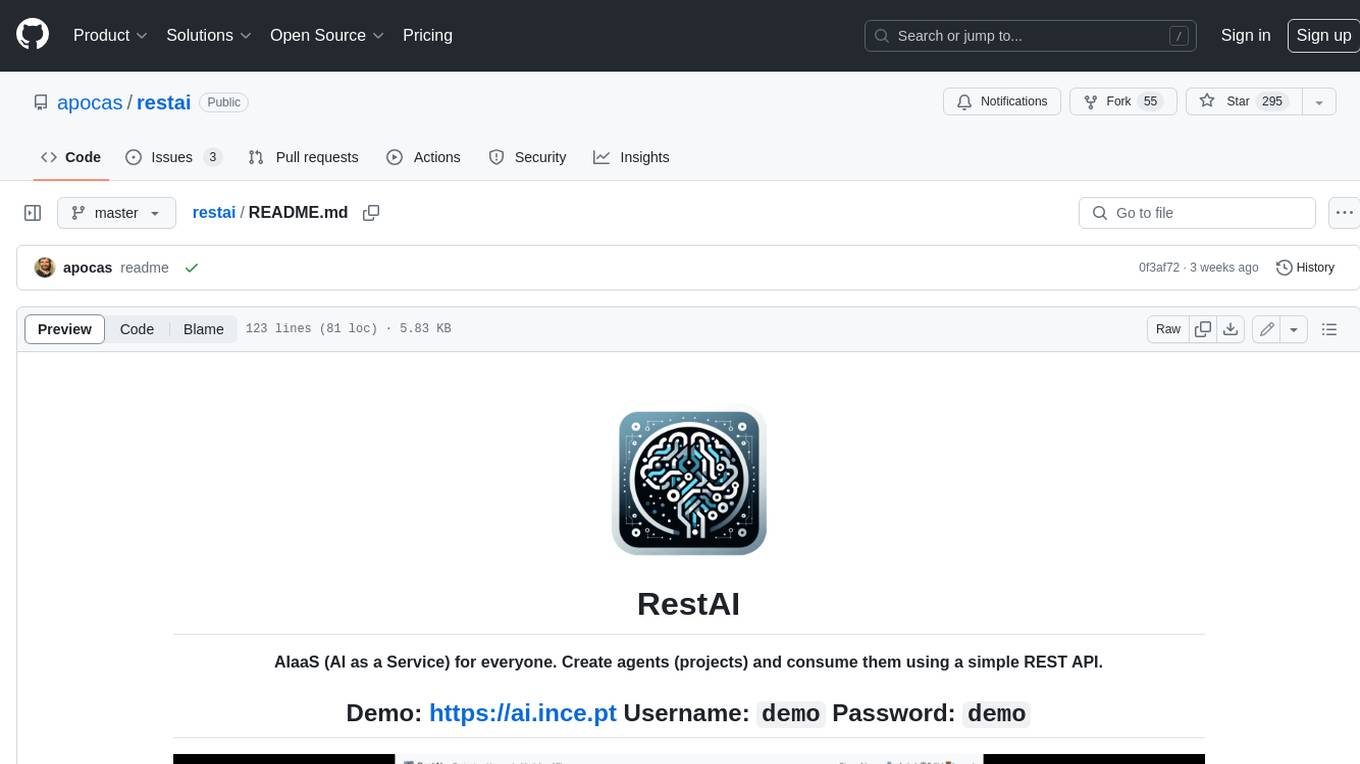
restai
RestAI is an AIaaS (AI as a Service) platform that allows users to create and consume AI agents (projects) using a simple REST API. It supports various types of agents, including RAG (Retrieval-Augmented Generation), RAGSQL (RAG for SQL), inference, vision, and router. RestAI features automatic VRAM management, support for any public LLM supported by LlamaIndex or any local LLM supported by Ollama, a user-friendly API with Swagger documentation, and a frontend for easy access. It also provides evaluation capabilities for RAG agents using deepeval.
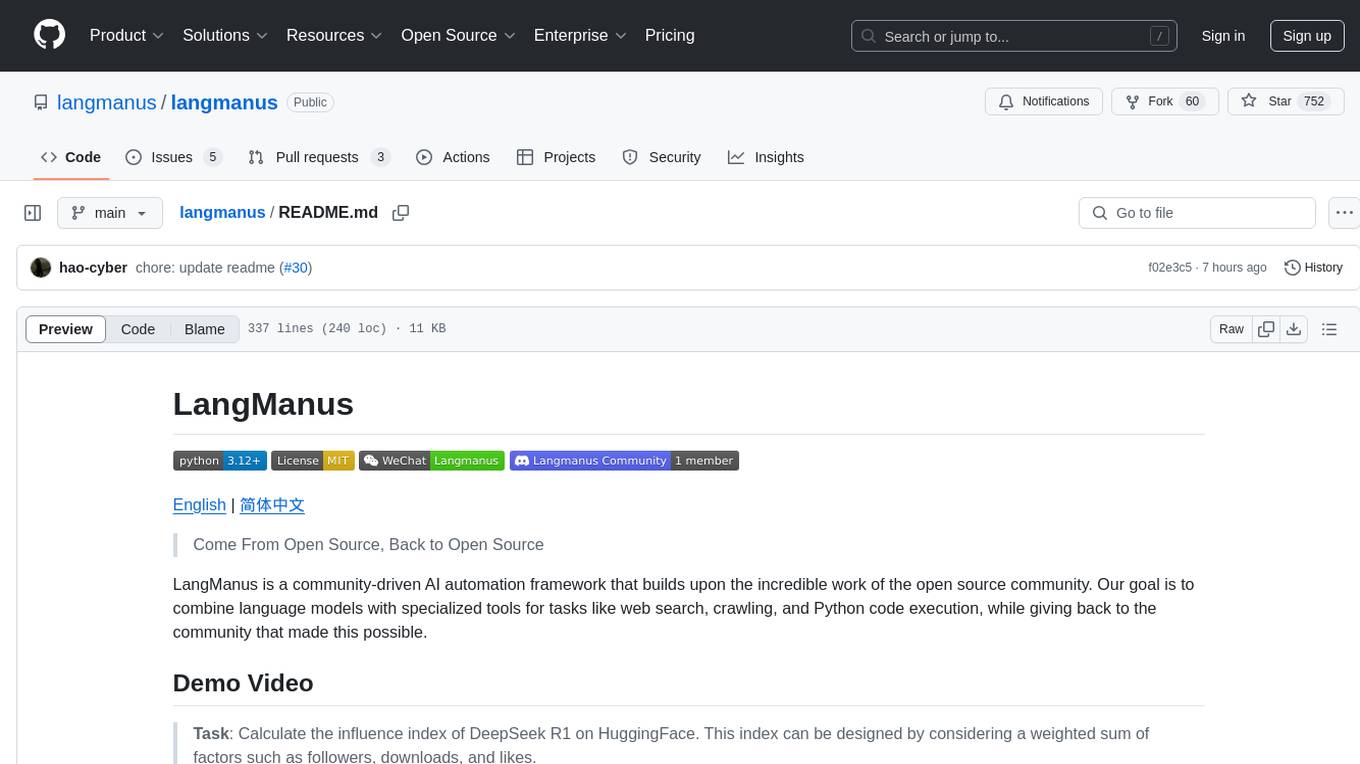
langmanus
LangManus is a community-driven AI automation framework that combines language models with specialized tools for tasks like web search, crawling, and Python code execution. It implements a hierarchical multi-agent system with agents like Coordinator, Planner, Supervisor, Researcher, Coder, Browser, and Reporter. The framework supports LLM integration, search and retrieval tools, Python integration, workflow management, and visualization. LangManus aims to give back to the open-source community and welcomes contributions in various forms.
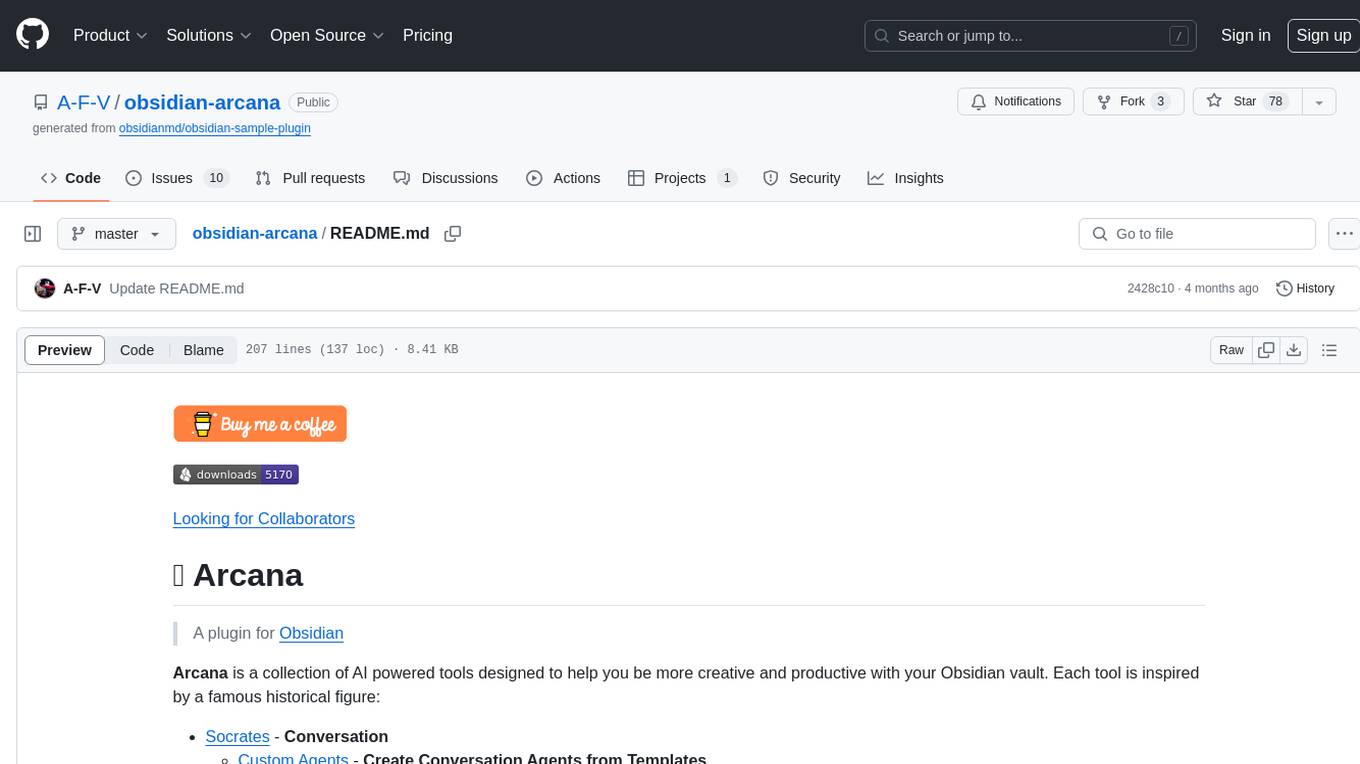
obsidian-arcana
Arcana is a plugin for Obsidian that offers a collection of AI-powered tools inspired by famous historical figures to enhance creativity and productivity. It includes tools for conversation, text-to-speech transcription, speech-to-text replies, metadata markup, text generation, file moving, flashcard generation, auto tagging, and note naming. Users can interact with these tools using the command palette and sidebar views, with an OpenAI API key required for usage. The plugin aims to assist users in various note-taking and knowledge management tasks within the Obsidian vault environment.
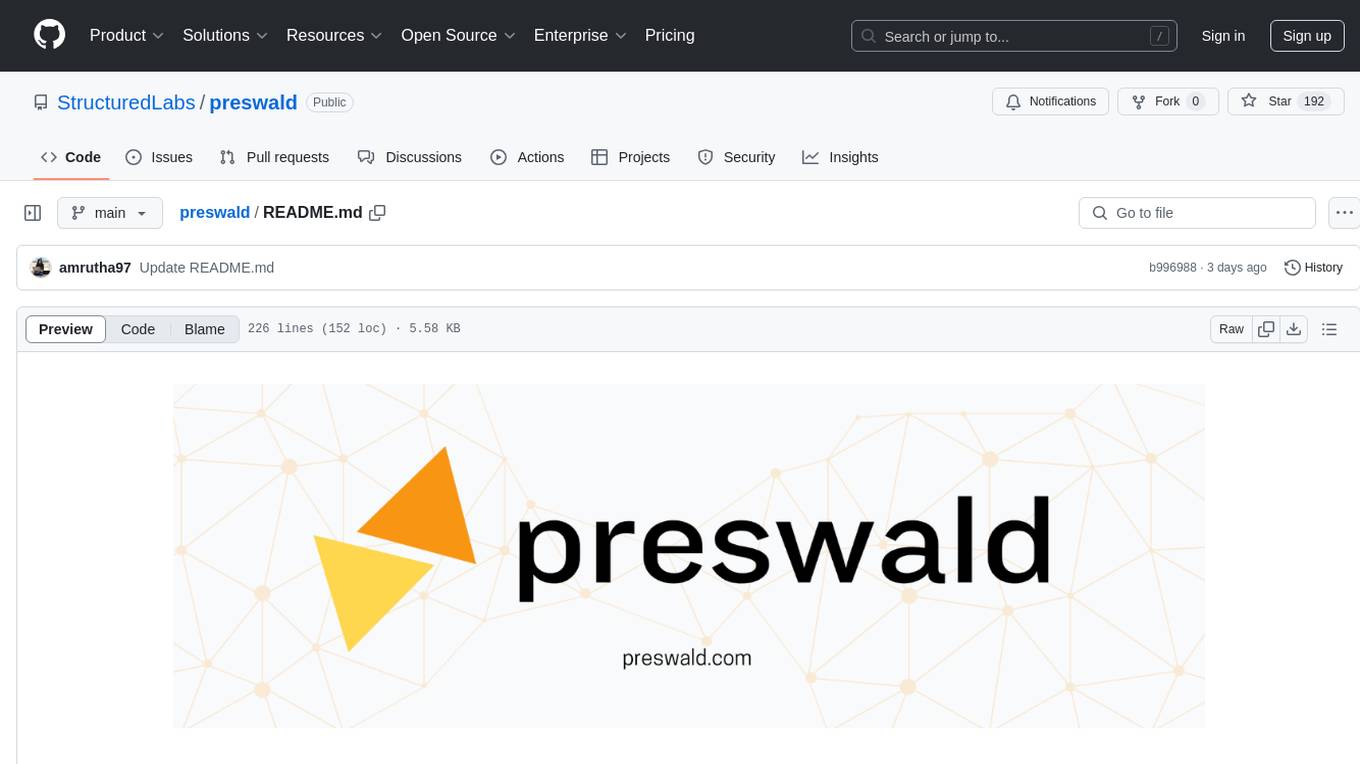
preswald
Preswald is a full-stack platform for building, deploying, and managing interactive data applications in Python. It simplifies the process by combining ingestion, storage, transformation, and visualization into one lightweight SDK. With Preswald, users can connect to various data sources, customize app themes, and easily deploy apps locally. The platform focuses on code-first simplicity, end-to-end coverage, and efficiency by design, making it suitable for prototyping internal tools or deploying production-grade apps with reduced complexity and cost.
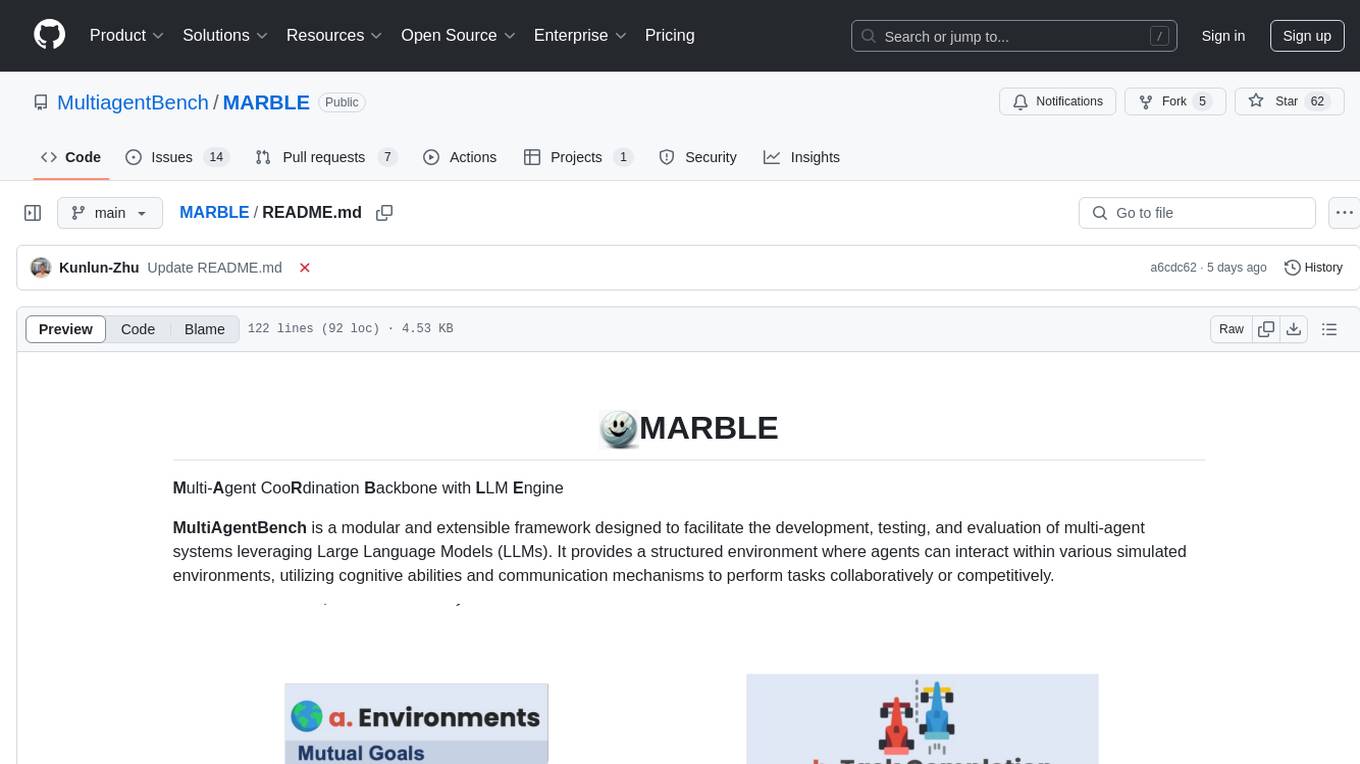
MARBLE
MARBLE (Multi-Agent Coordination Backbone with LLM Engine) is a modular framework for developing, testing, and evaluating multi-agent systems leveraging Large Language Models. It provides a structured environment for agents to interact in simulated environments, utilizing cognitive abilities and communication mechanisms for collaborative or competitive tasks. The framework features modular design, multi-agent support, LLM integration, shared memory, flexible environments, metrics and evaluation, industrial coding standards, and Docker support.
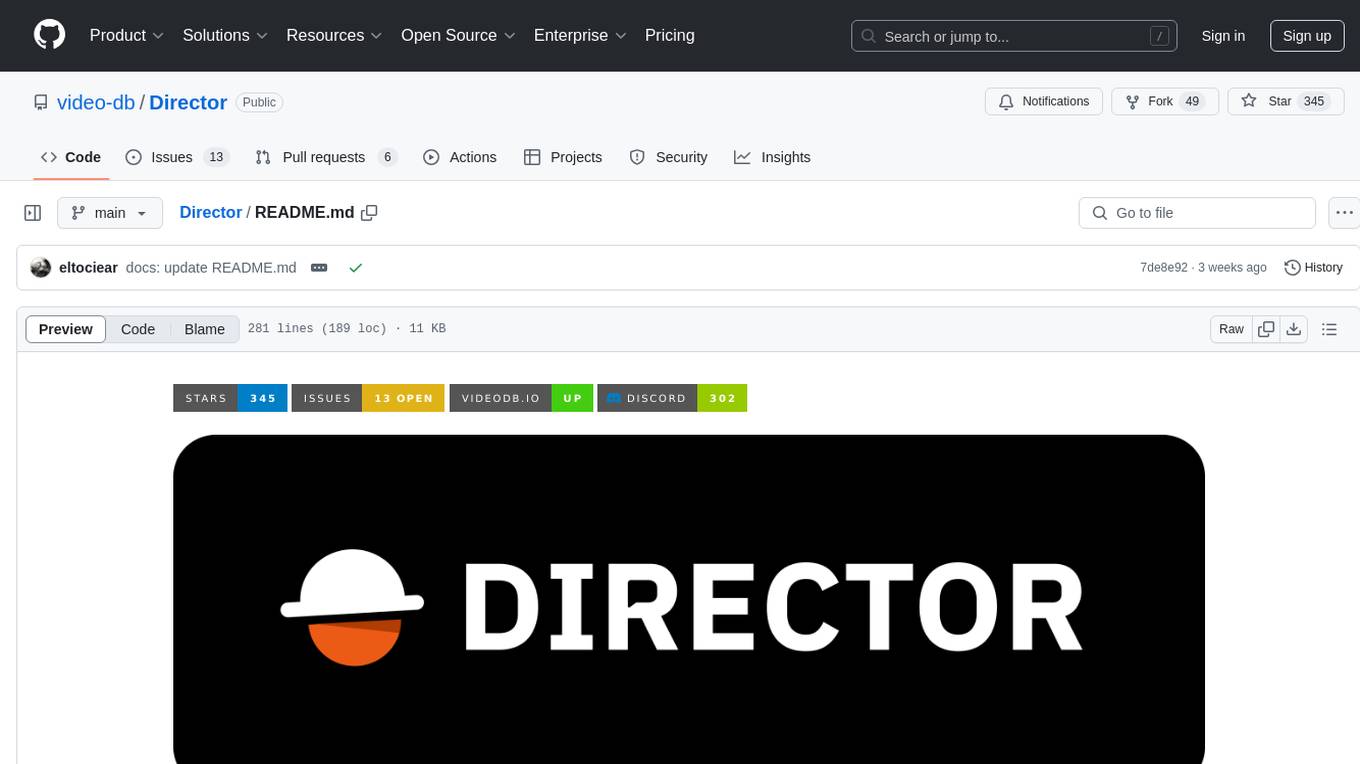
Director
Director is a framework to build video agents that can reason through complex video tasks like search, editing, compilation, generation, etc. It enables users to summarize videos, search for specific moments, create clips instantly, integrate GenAI projects and APIs, add overlays, generate thumbnails, and more. Built on VideoDB's 'video-as-data' infrastructure, Director is perfect for developers, creators, and teams looking to simplify media workflows and unlock new possibilities.
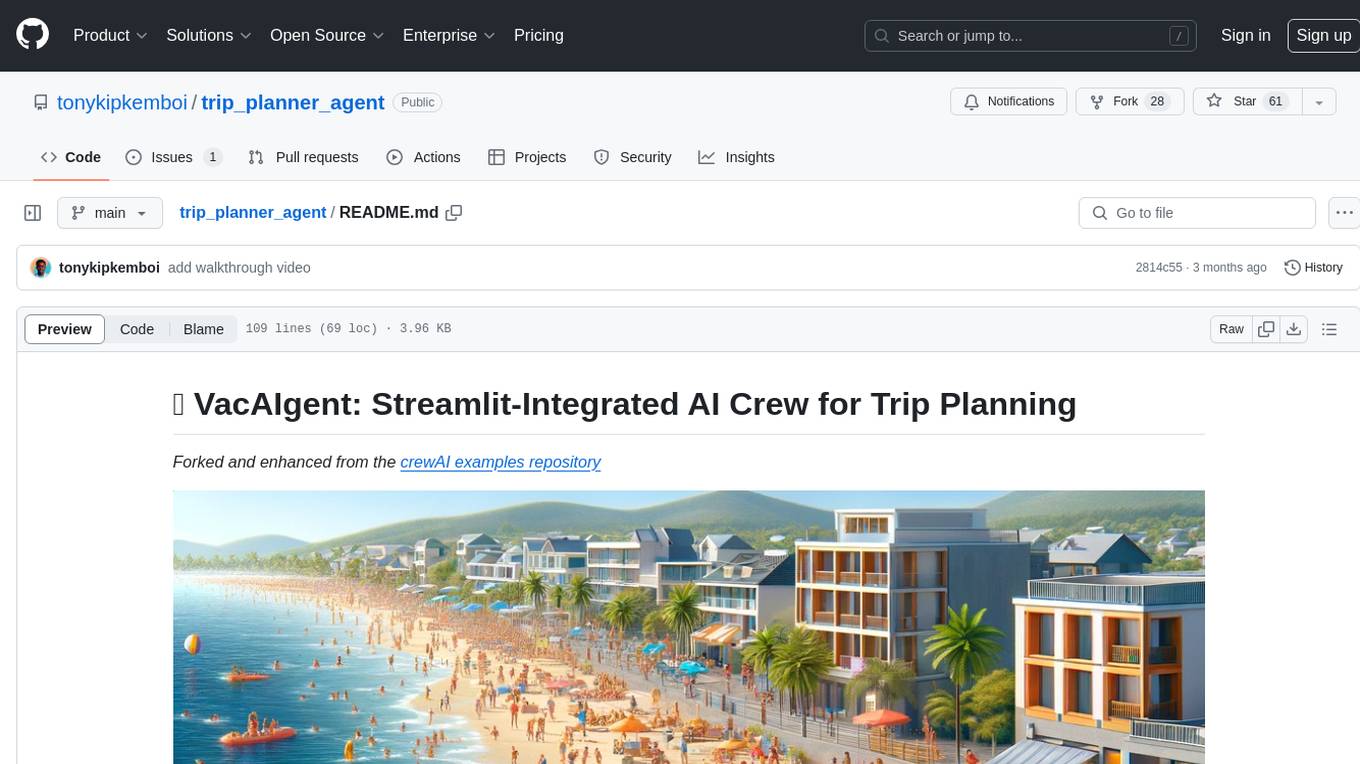
trip_planner_agent
VacAIgent is an AI tool that automates and enhances trip planning by leveraging the CrewAI framework. It integrates a user-friendly Streamlit interface for interactive travel planning. Users can input preferences and receive tailored travel plans with the help of autonomous AI agents. The tool allows for collaborative decision-making on cities and crafting complete itineraries based on specified preferences, all accessible via a streamlined Streamlit user interface. VacAIgent can be customized to use different AI models like GPT-3.5 or local models like Ollama for enhanced privacy and customization.
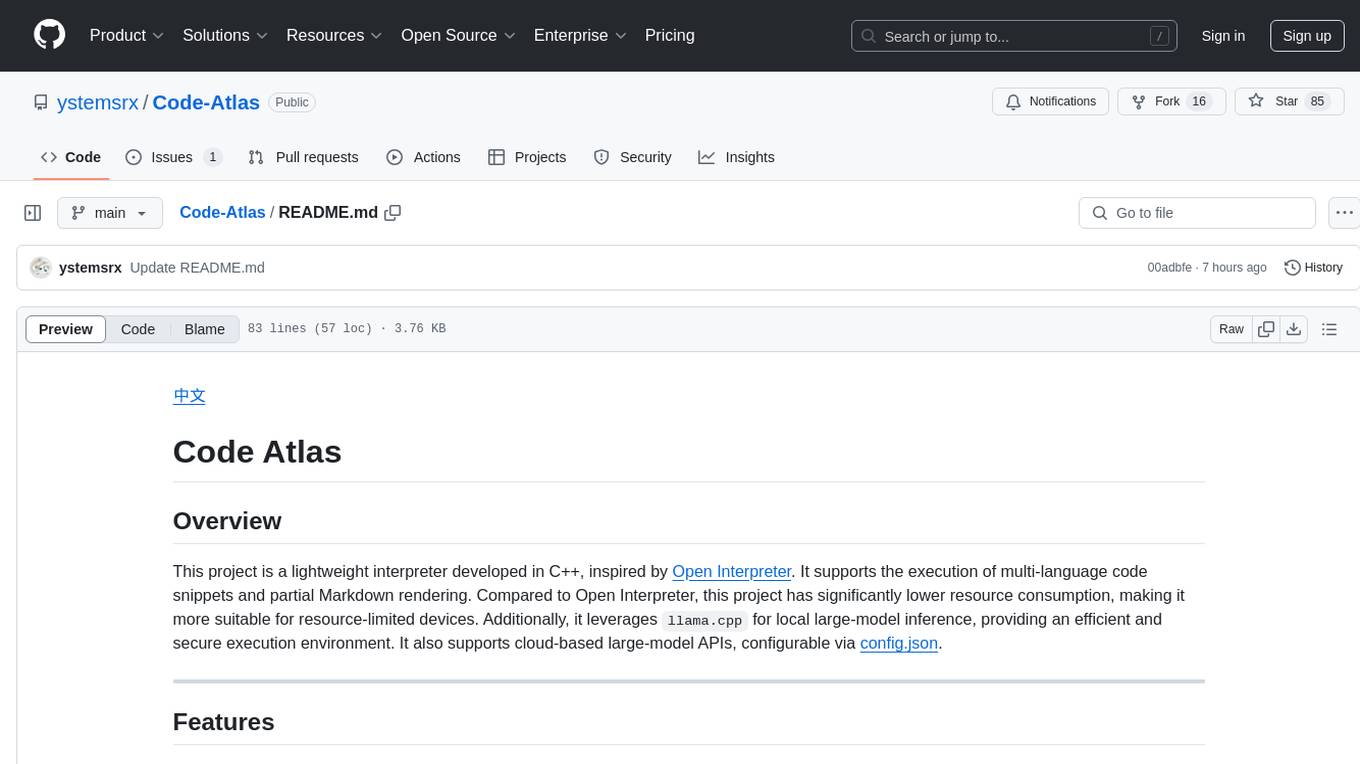
Code-Atlas
Code Atlas is a lightweight interpreter developed in C++ that supports the execution of multi-language code snippets and partial Markdown rendering. It consumes significantly lower resources compared to similar tools, making it suitable for resource-limited devices. It leverages llama.cpp for local large-model inference and supports cloud-based large-model APIs. The tool provides features for code execution, Markdown rendering, local AI inference, and resource efficiency.
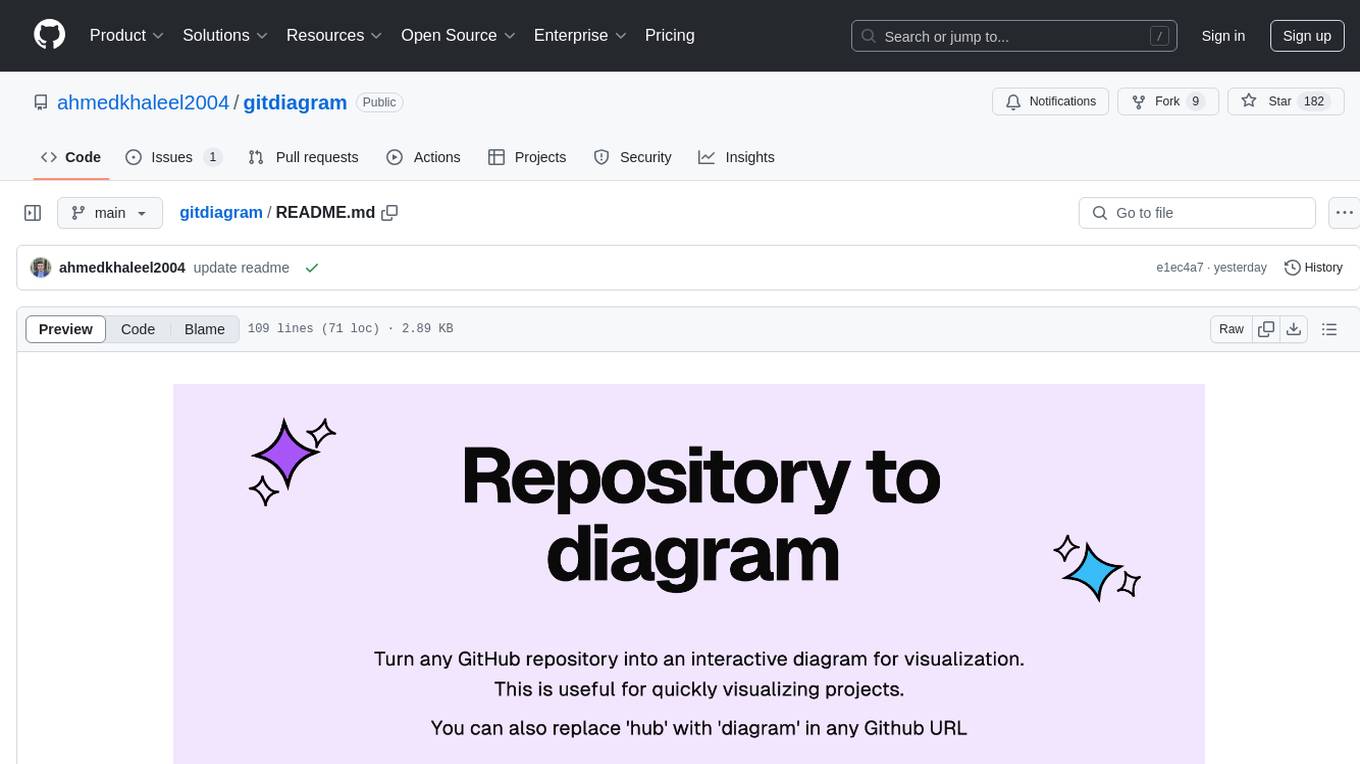
gitdiagram
GitDiagram is a tool that turns any GitHub repository into an interactive diagram for visualization in seconds. It offers instant visualization, interactivity, fast generation, customization, and API access. The tool utilizes a tech stack including Next.js, FastAPI, PostgreSQL, Claude 3.5 Sonnet, Vercel, EC2, GitHub Actions, PostHog, and Api-Analytics. Users can self-host the tool for local development and contribute to its development. GitDiagram is inspired by Gitingest and has future plans to use larger context models, allow user API key input, implement RAG with Mermaid.js docs, and include font-awesome icons in diagrams.
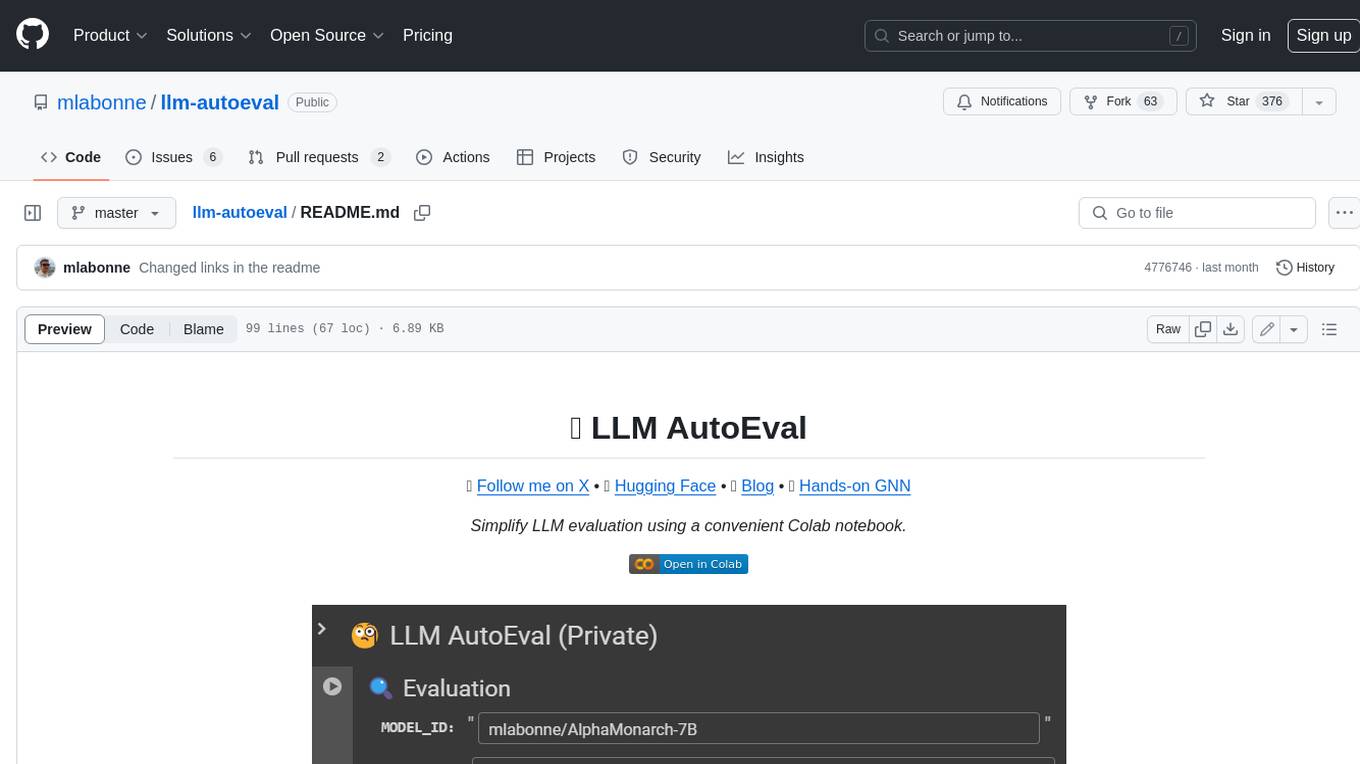
llm-autoeval
LLM AutoEval is a tool that simplifies the process of evaluating Large Language Models (LLMs) using a convenient Colab notebook. It automates the setup and execution of evaluations using RunPod, allowing users to customize evaluation parameters and generate summaries that can be uploaded to GitHub Gist for easy sharing and reference. LLM AutoEval supports various benchmark suites, including Nous, Lighteval, and Open LLM, enabling users to compare their results with existing models and leaderboards.
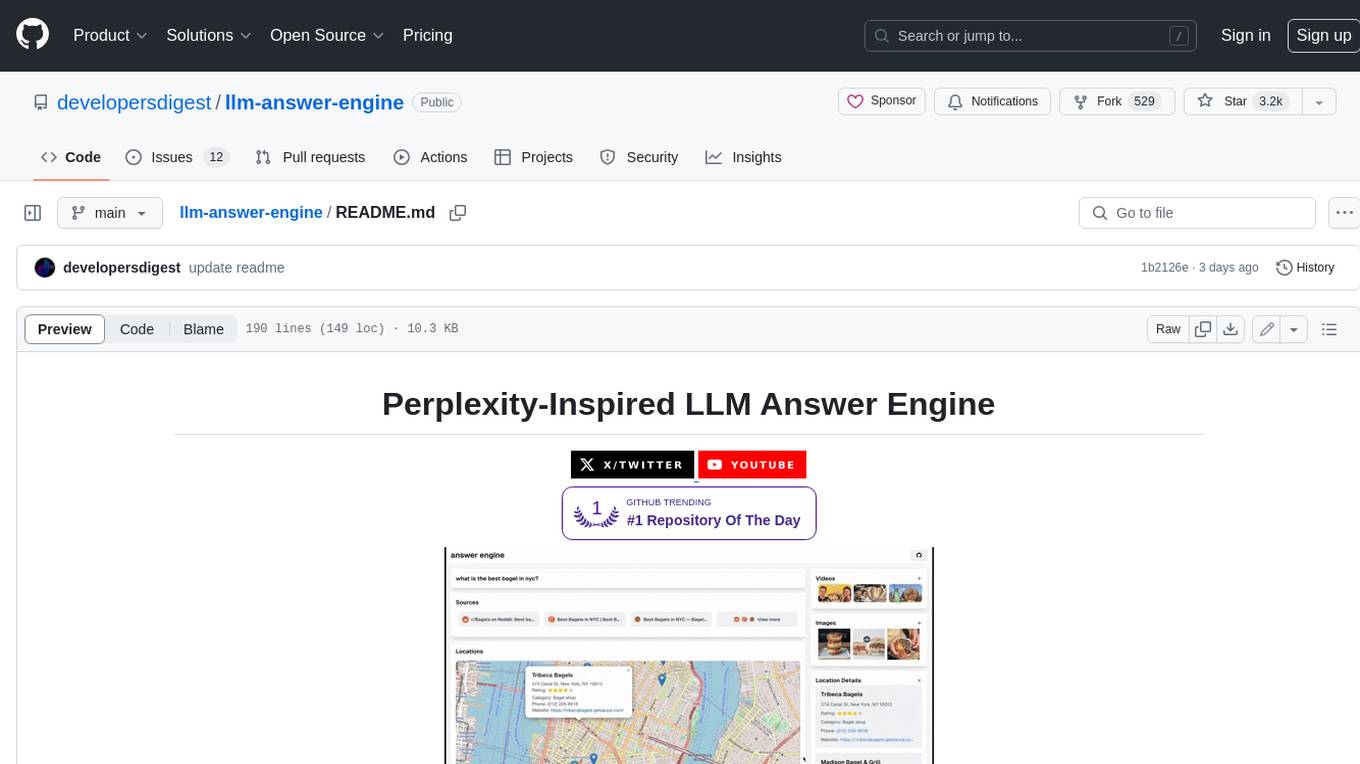
llm-answer-engine
This repository contains the code and instructions needed to build a sophisticated answer engine that leverages the capabilities of Groq, Mistral AI's Mixtral, Langchain.JS, Brave Search, Serper API, and OpenAI. Designed to efficiently return sources, answers, images, videos, and follow-up questions based on user queries, this project is an ideal starting point for developers interested in natural language processing and search technologies.
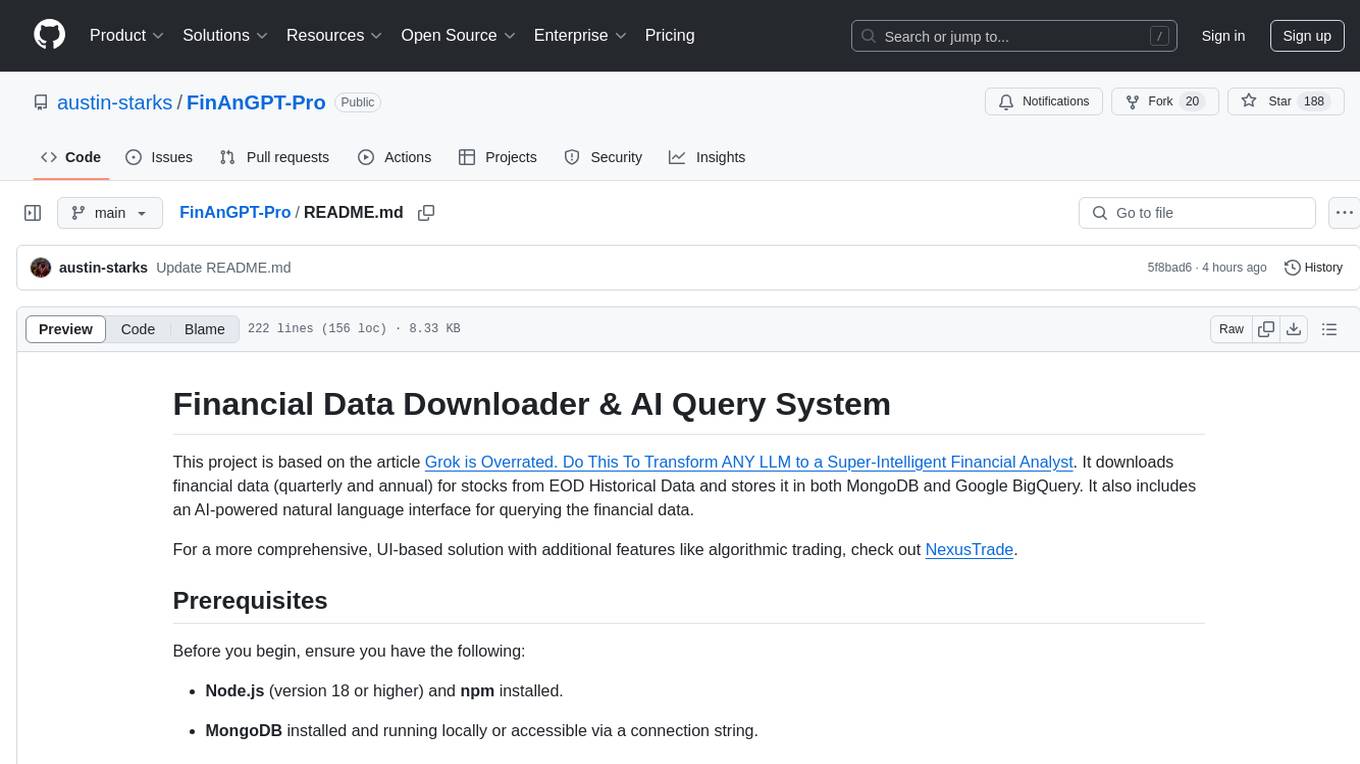
FinAnGPT-Pro
FinAnGPT-Pro is a financial data downloader and AI query system that downloads quarterly and annual financial data for stocks from EOD Historical Data, storing it in MongoDB and Google BigQuery. It includes an AI-powered natural language interface for querying financial data. Users can set up the tool by following the prerequisites and setup instructions provided in the README. The tool allows users to download financial data for all stocks in a watchlist or for a single stock, query financial data using a natural language interface, and receive responses in a structured format. Important considerations include error handling, rate limiting, data validation, BigQuery costs, MongoDB connection, and security measures for API keys and credentials.
For similar tasks
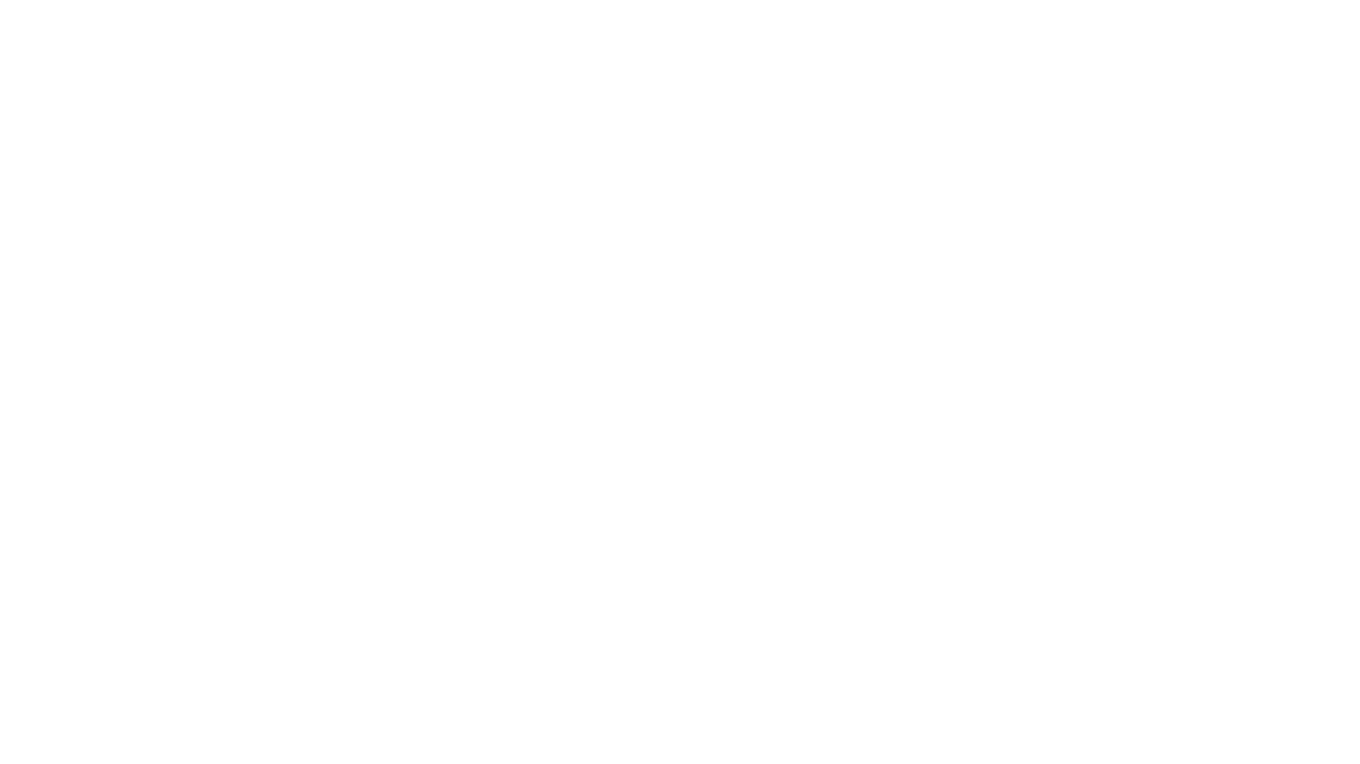
maiar-ai
MAIAR is a composable, plugin-based AI agent framework designed to abstract data ingestion, decision-making, and action execution into modular plugins. It enables developers to define triggers and actions as standalone plugins, while the core runtime handles decision-making dynamically. This framework offers extensibility, composability, and model-driven behavior, allowing seamless addition of new functionality. MAIAR's architecture is influenced by Unix pipes, ensuring highly composable plugins, dynamic execution pipelines, and transparent debugging. It remains declarative and extensible, allowing developers to build complex AI workflows without rigid architectures.
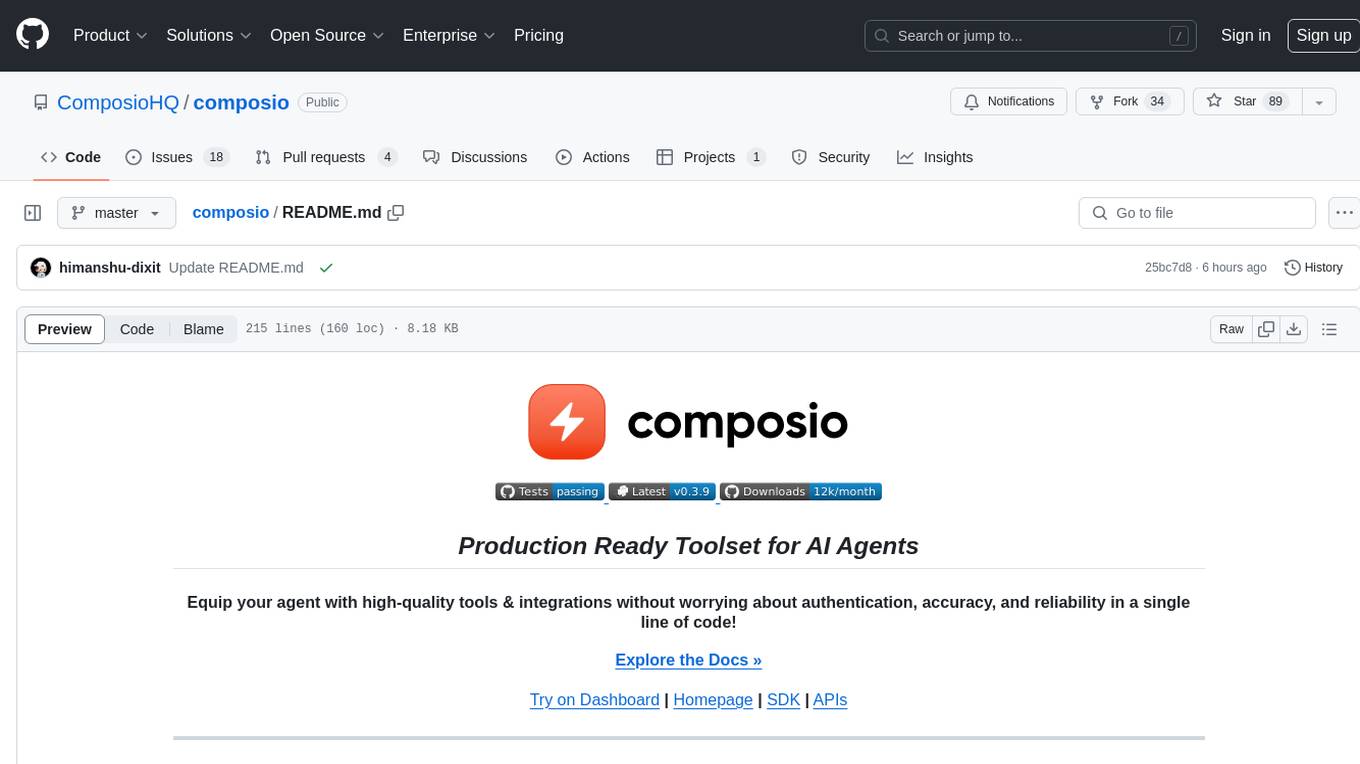
composio
Composio is a production-ready toolset for AI agents that enables users to integrate AI agents with various agentic tools effortlessly. It provides support for over 100 tools across different categories, including popular softwares like GitHub, Notion, Linear, Gmail, Slack, and more. Composio ensures managed authorization with support for six different authentication protocols, offering better agentic accuracy and ease of use. Users can easily extend Composio with additional tools, frameworks, and authorization protocols. The toolset is designed to be embeddable and pluggable, allowing for seamless integration and consistent user experience.
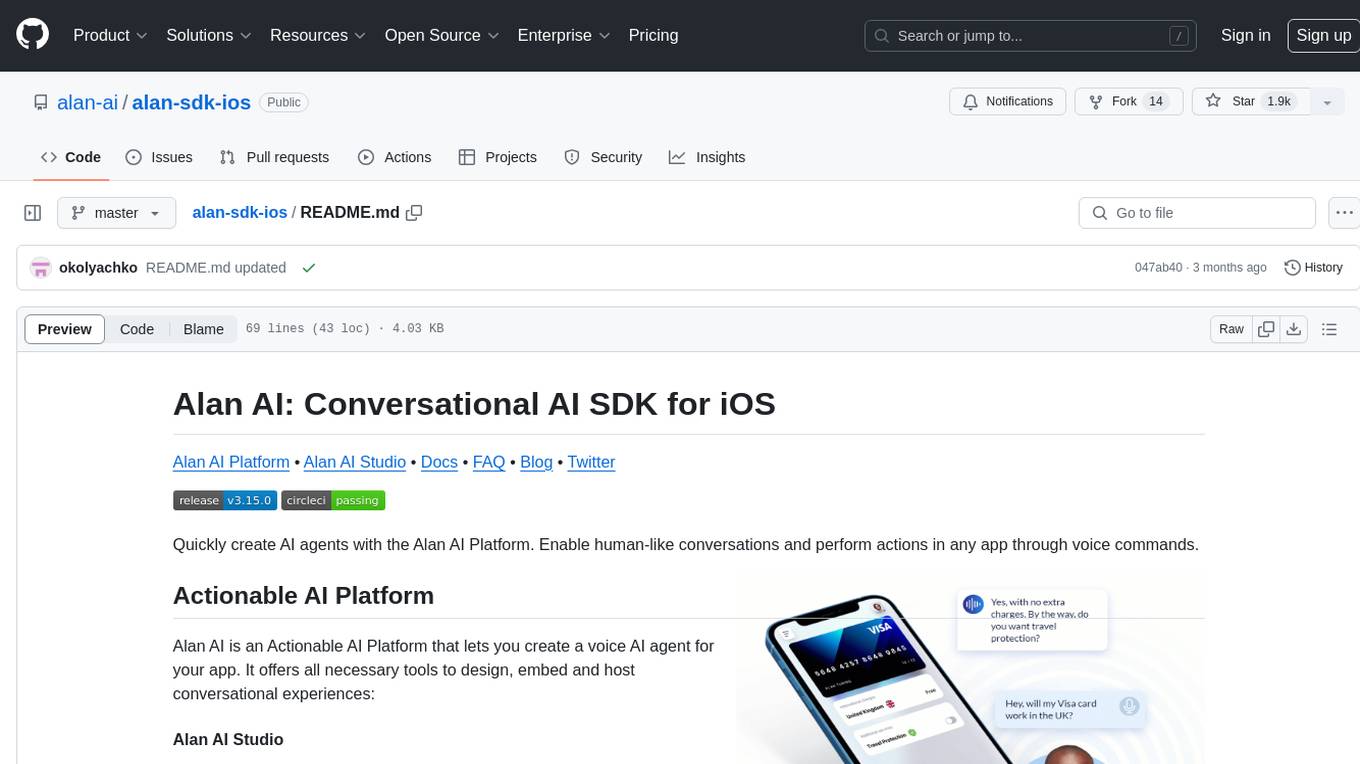
alan-sdk-ios
Alan AI SDK for iOS is a powerful tool that allows developers to quickly create AI agents for their iOS apps. With Alan AI Platform, users can easily design, embed, and host conversational experiences in their applications. The platform offers a web-based IDE called Alan AI Studio for creating dialog scenarios, lightweight SDKs for embedding AI agents, and a backend powered by top-notch speech recognition and natural language understanding technologies. Alan AI enables human-like conversations and actions through voice commands, with features like on-the-fly updates, dialog flow testing, and analytics.
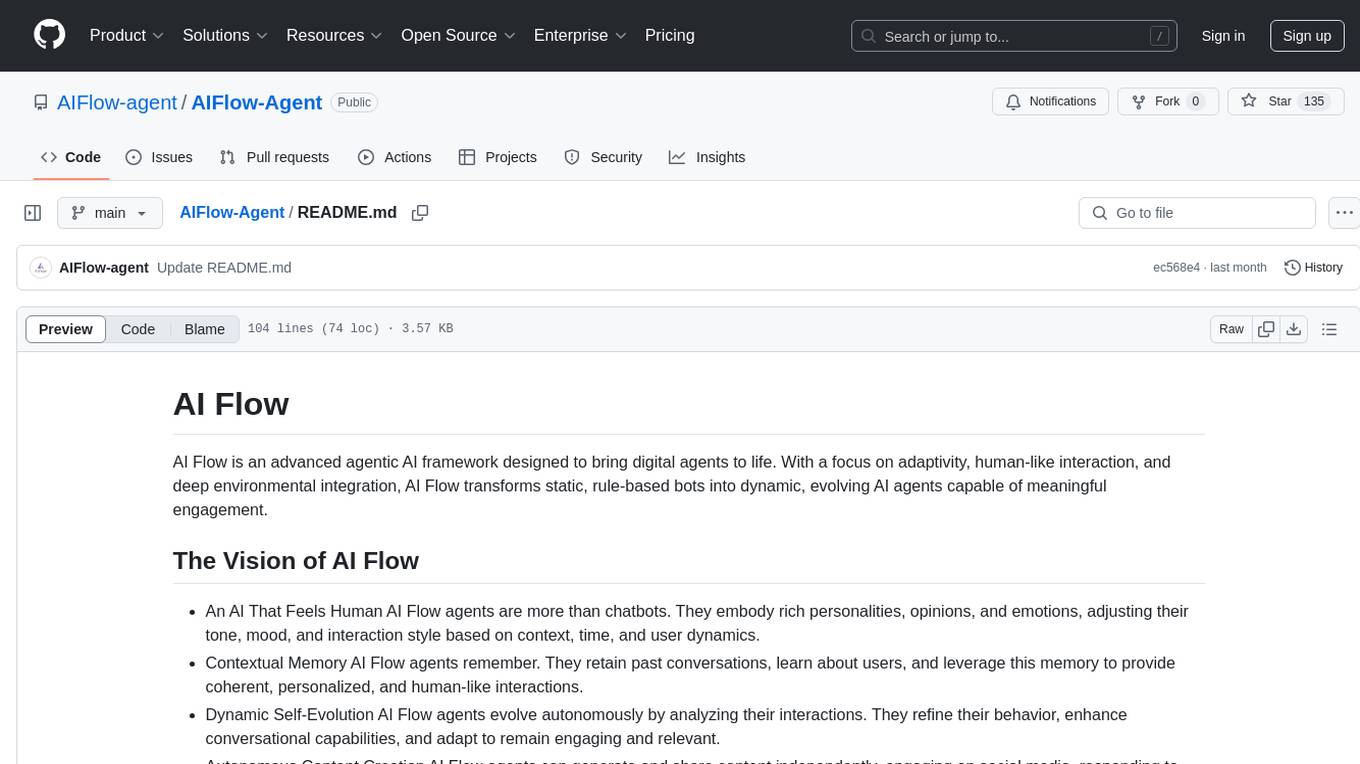
AIFlow-Agent
AI Flow is an advanced agentic AI framework that transforms static, rule-based bots into dynamic AI agents capable of meaningful engagement. It focuses on adaptivity, human-like interaction, and deep environmental integration. AI Flow agents embody rich personalities, opinions, and emotions, remember past conversations, evolve autonomously, create content independently, collaborate with other agents, and anticipate user needs without explicit prompts.
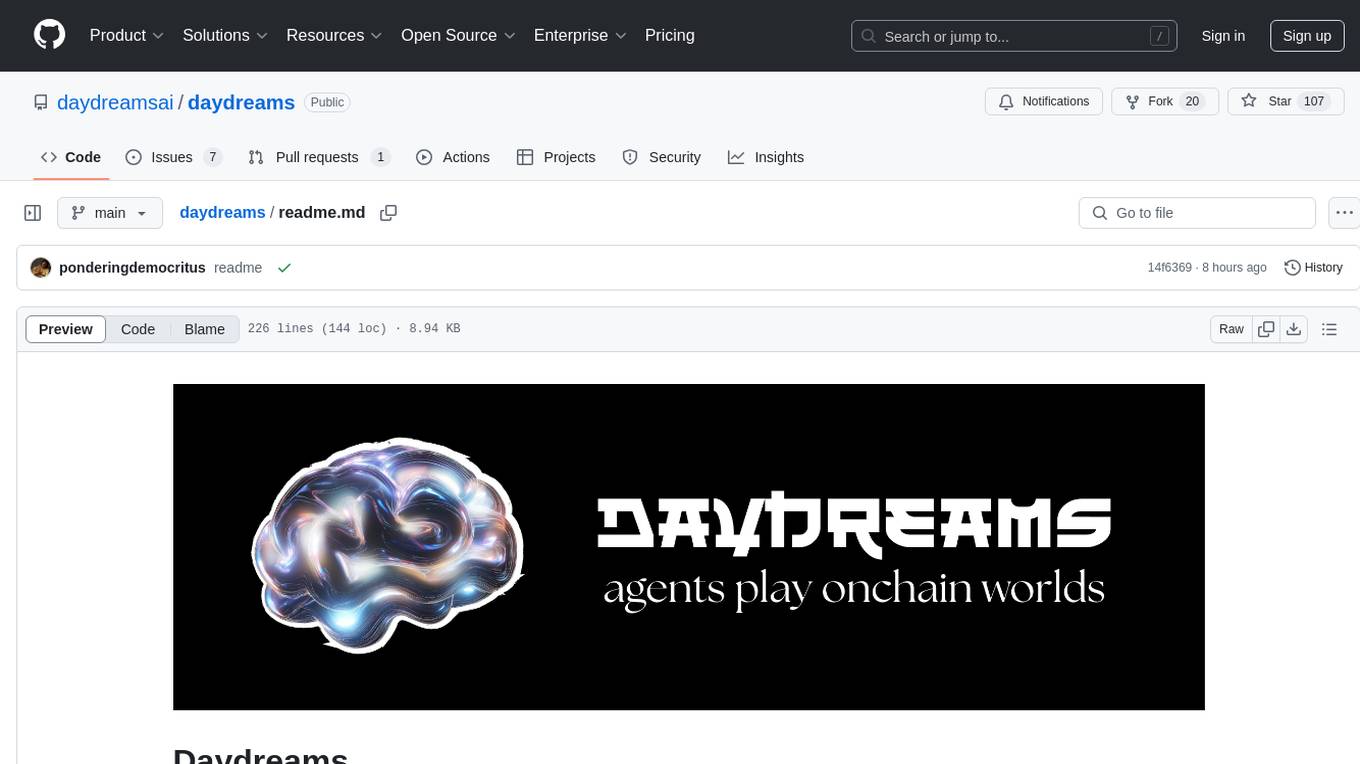
daydreams
Daydreams is a generative agent library designed for playing onchain games by injecting context. It is chain agnostic and allows users to perform onchain tasks, including playing any onchain game. The tool is lightweight and powerful, enabling users to define game context, register actions, set goals, monitor progress, and integrate with external agents. Daydreams aims to be 'lite' and 'composable', dynamically generating code needed to play games. It is currently in pre-alpha stage, seeking feedback and collaboration for further development.
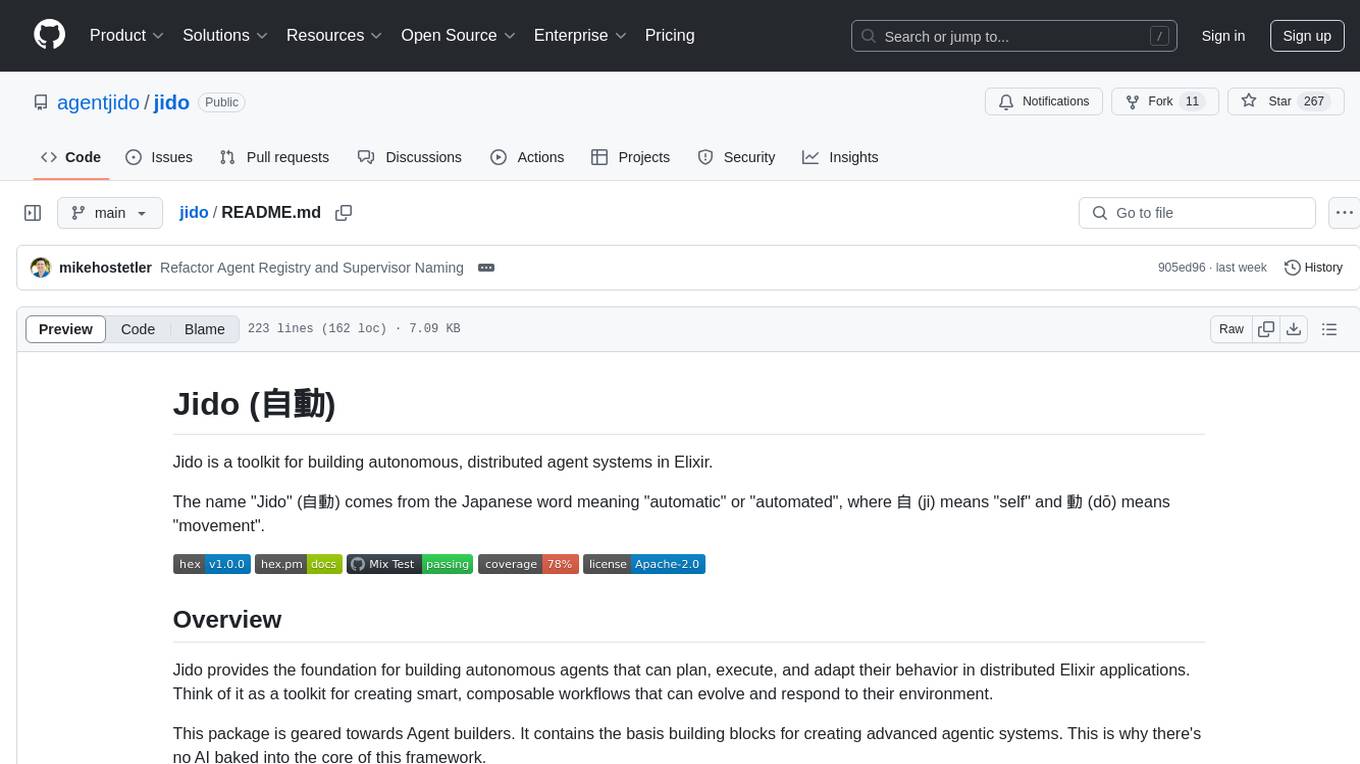
jido
Jido is a toolkit for building autonomous, distributed agent systems in Elixir. It provides the foundation for creating smart, composable workflows that can evolve and respond to their environment. Geared towards Agent builders, it contains core state primitives, composable actions, agent data structures, real-time sensors, signal system, skills, and testing tools. Jido is designed for multi-node Elixir clusters and offers rich helpers for unit and property-based testing.
For similar jobs
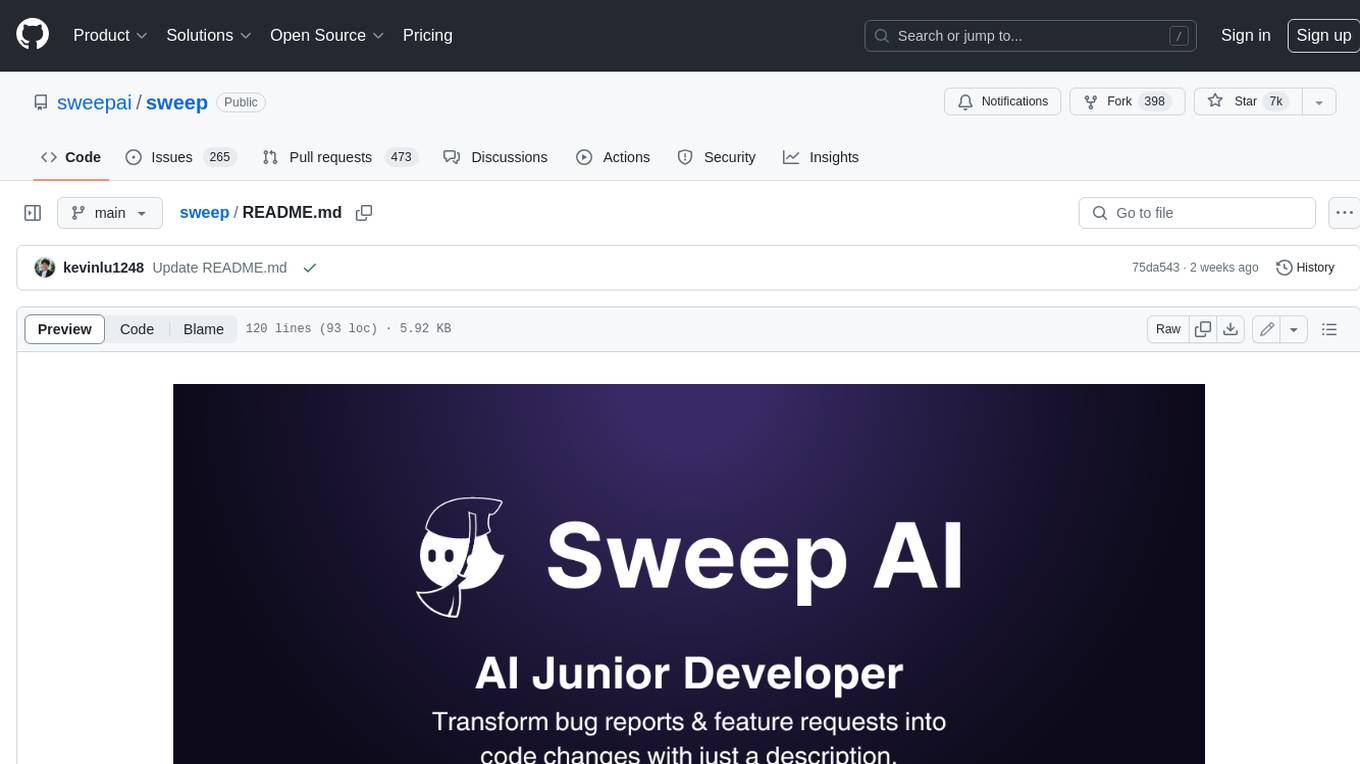
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
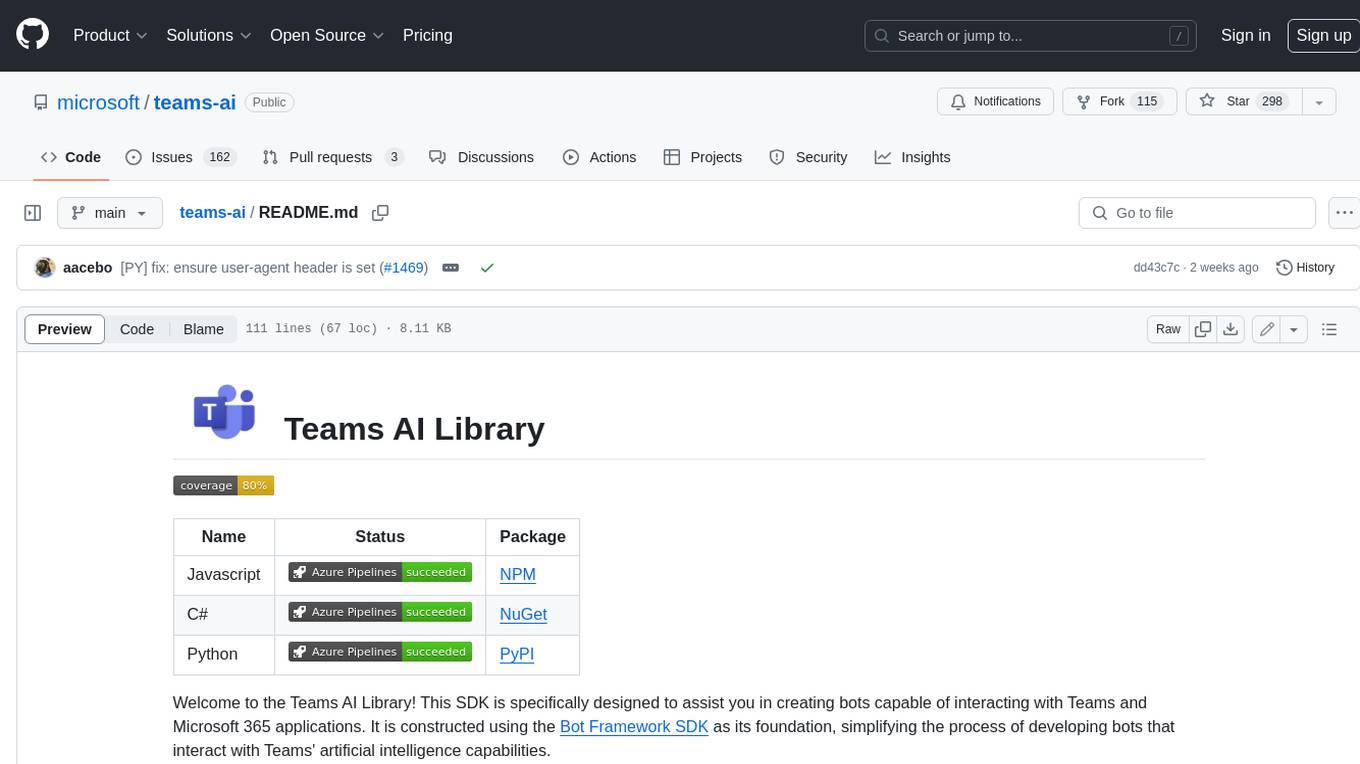
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
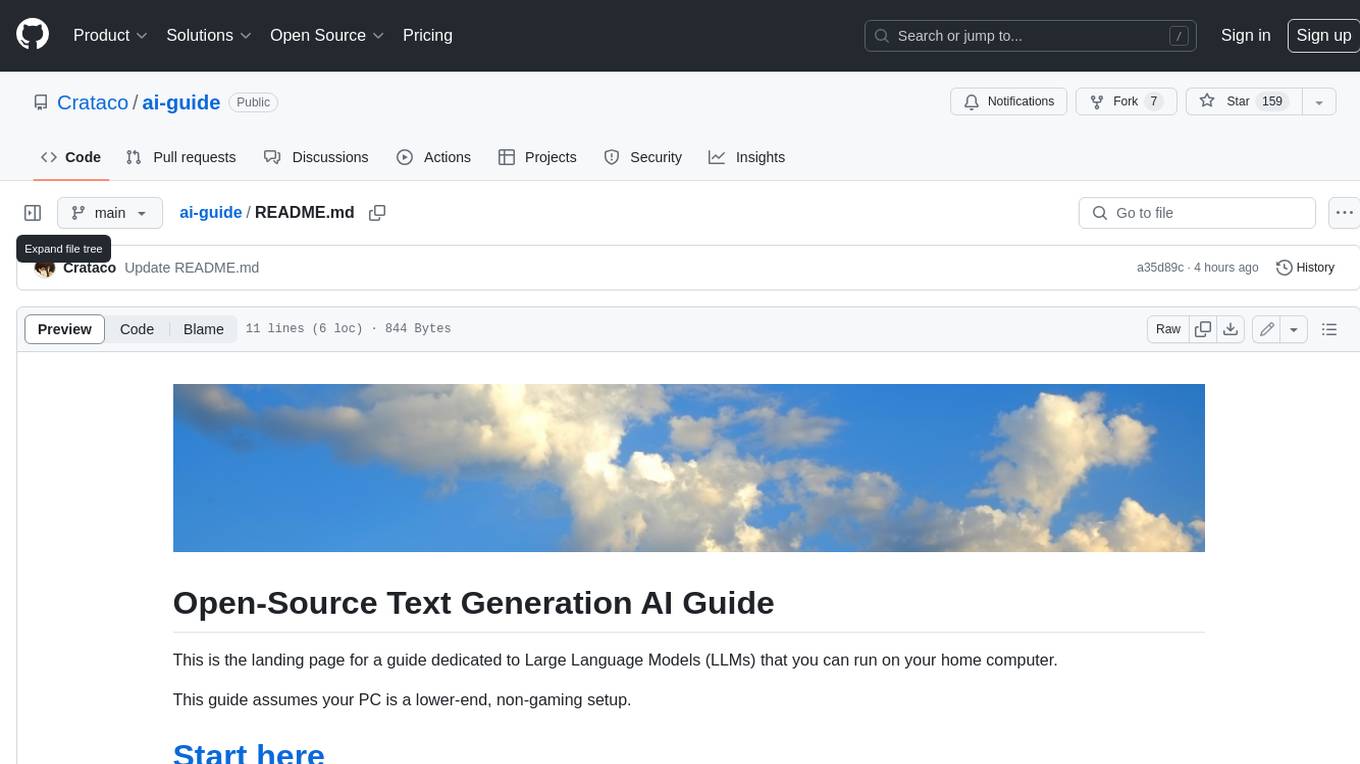
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
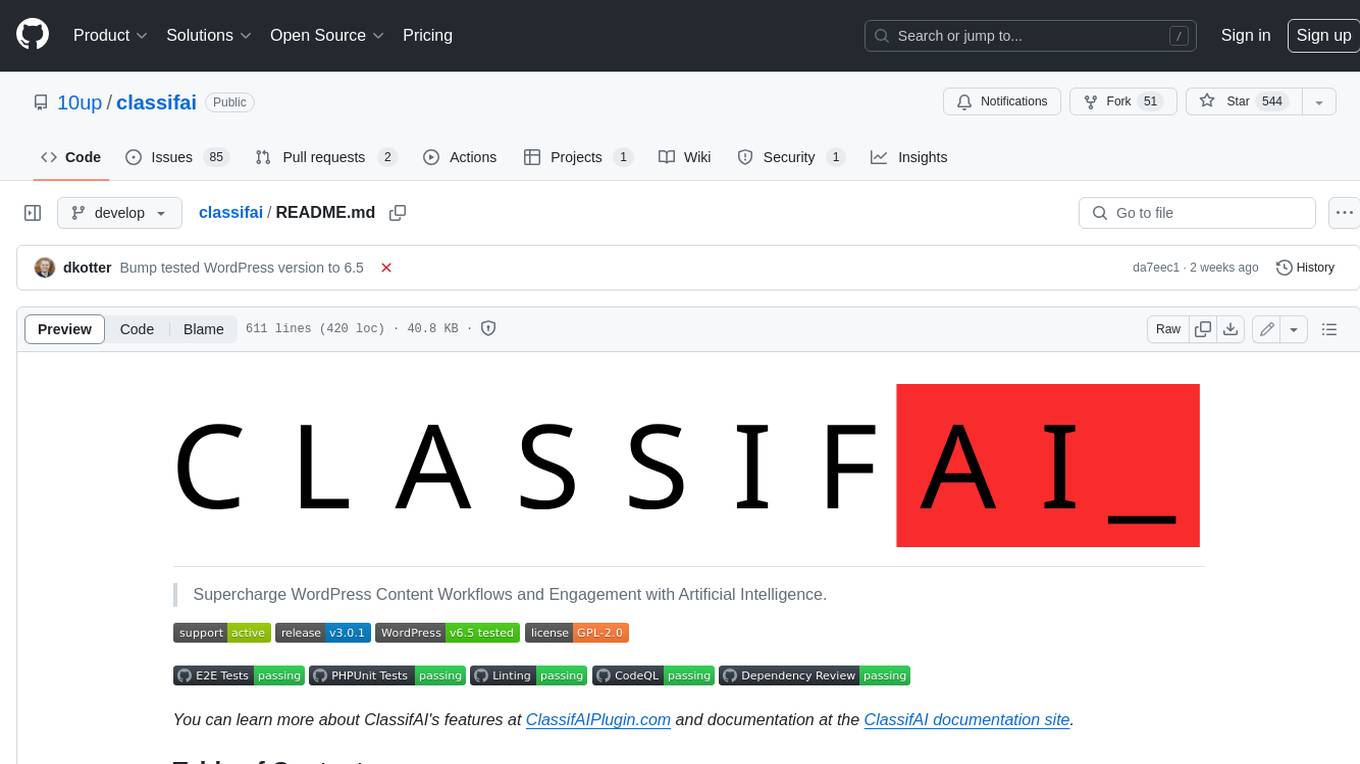
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
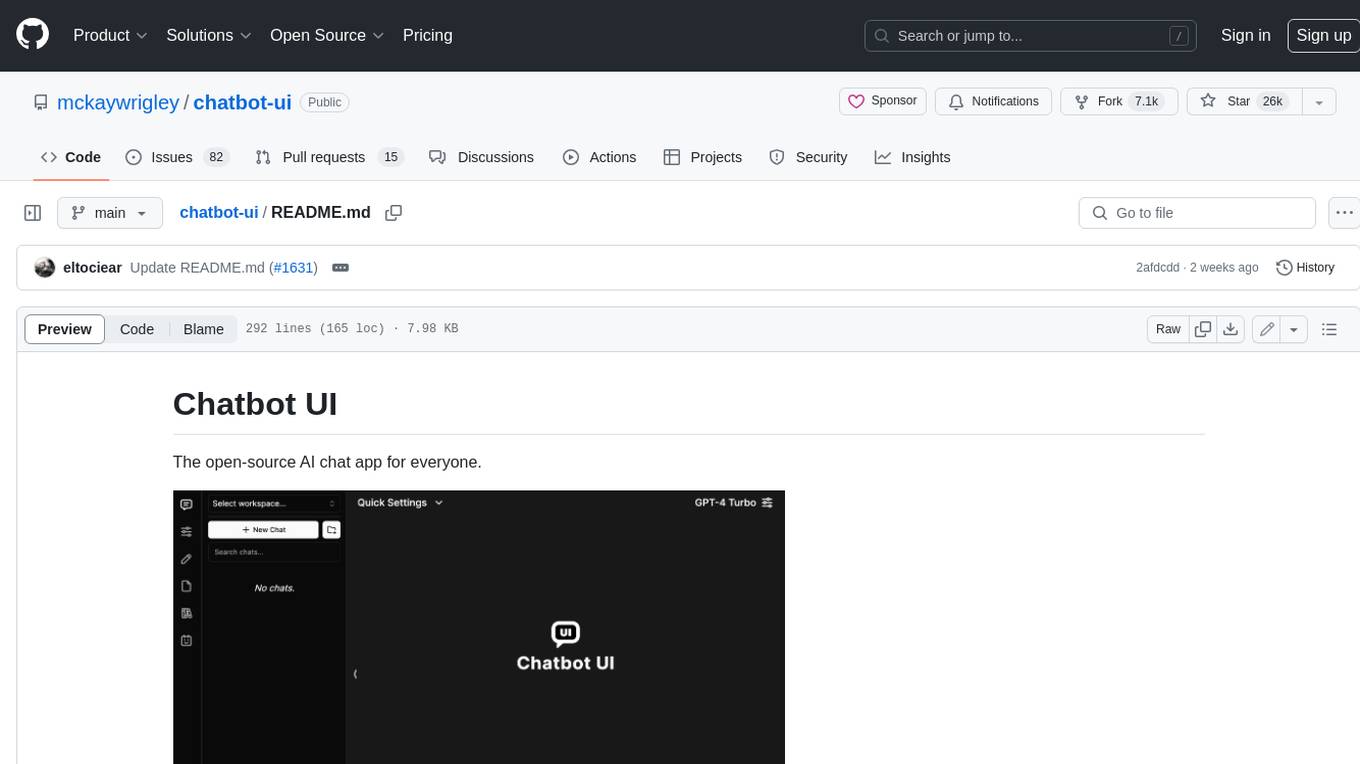
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
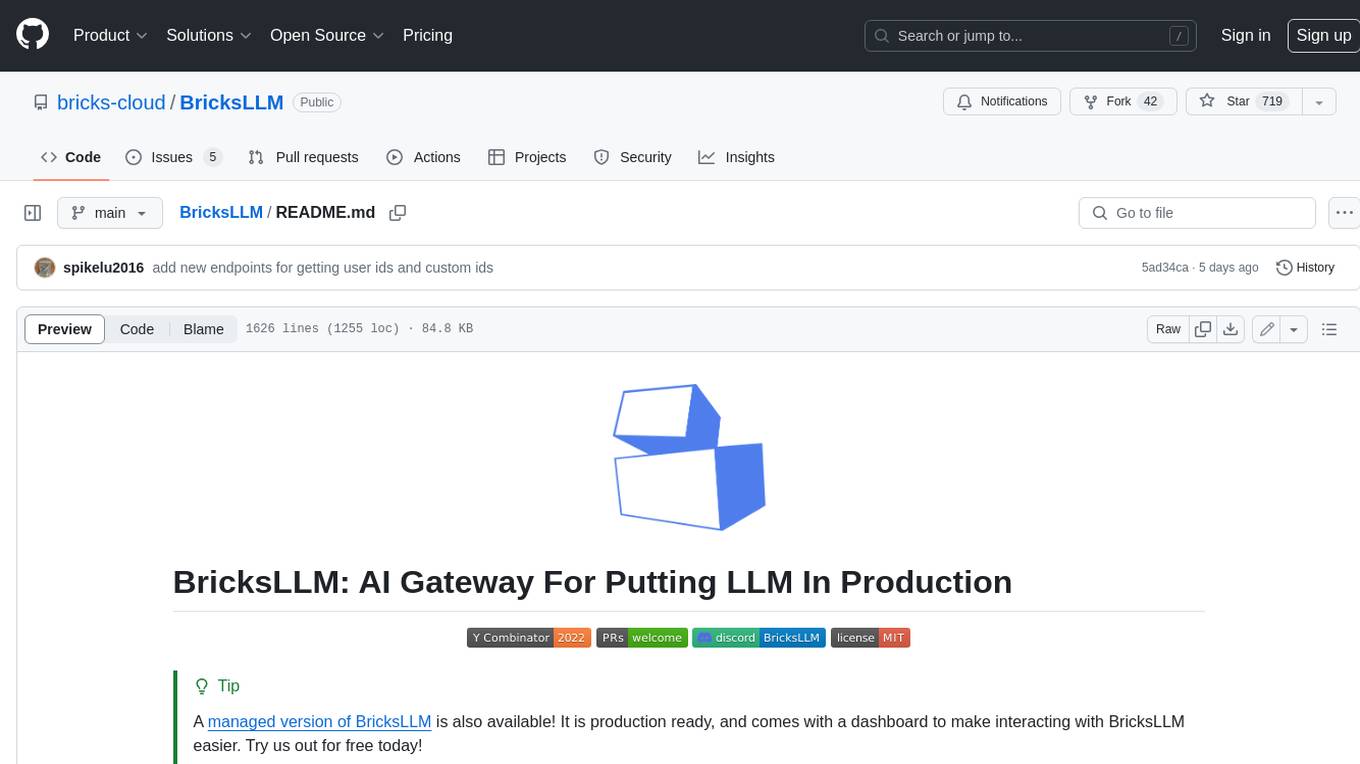
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
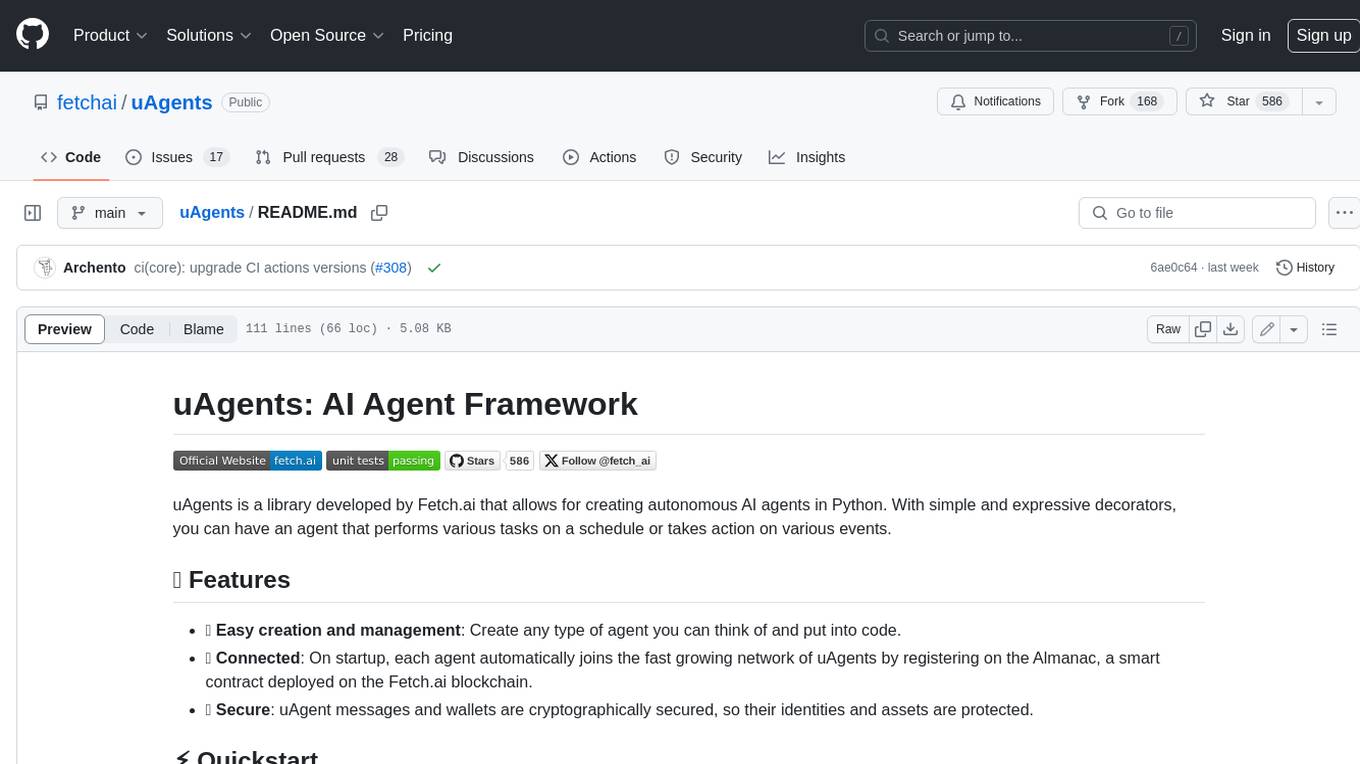
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
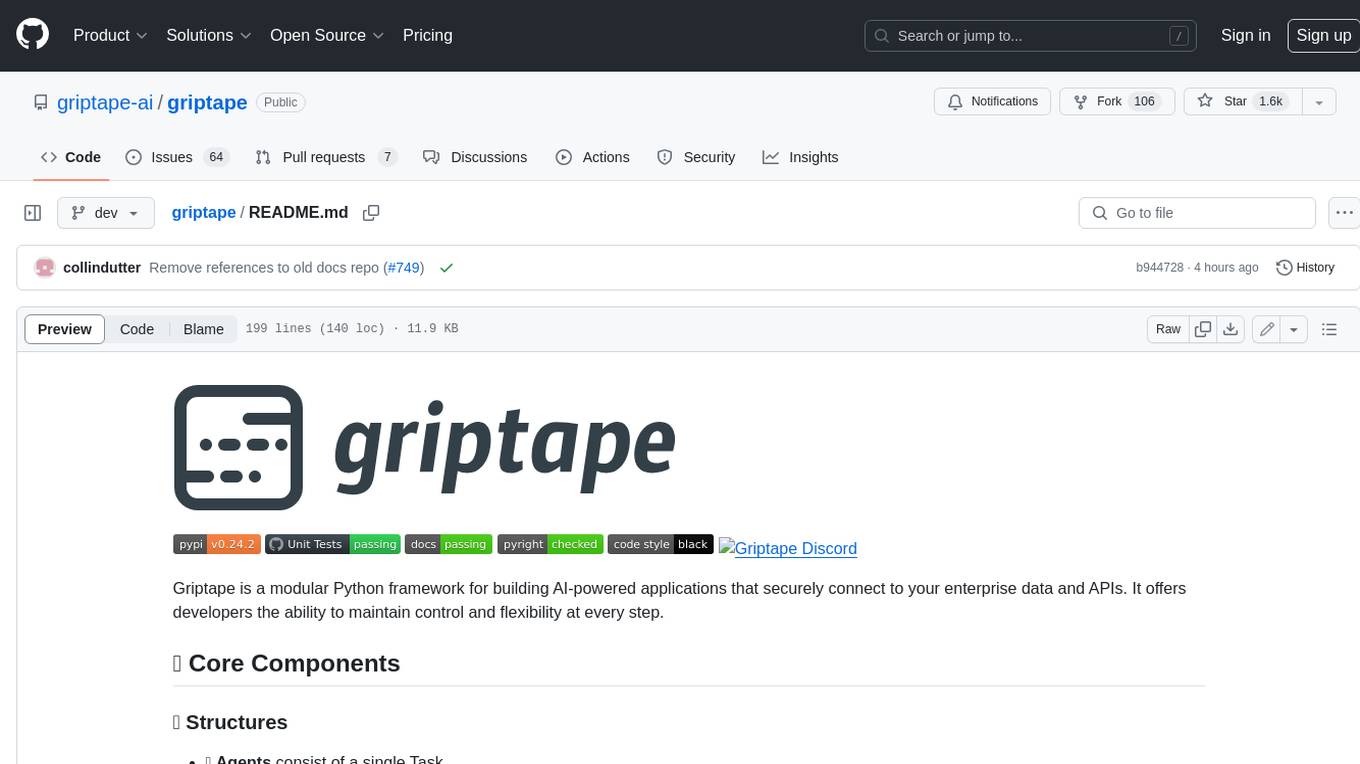
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.