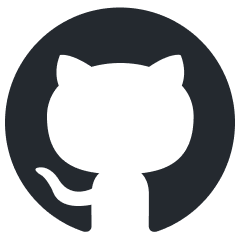
MCPSharp
MCPSharp is a .NET library that helps you build Model Context Protocol (MCP) servers and clients - the standardized API protocol used by AI assistants and models.
Stars: 142
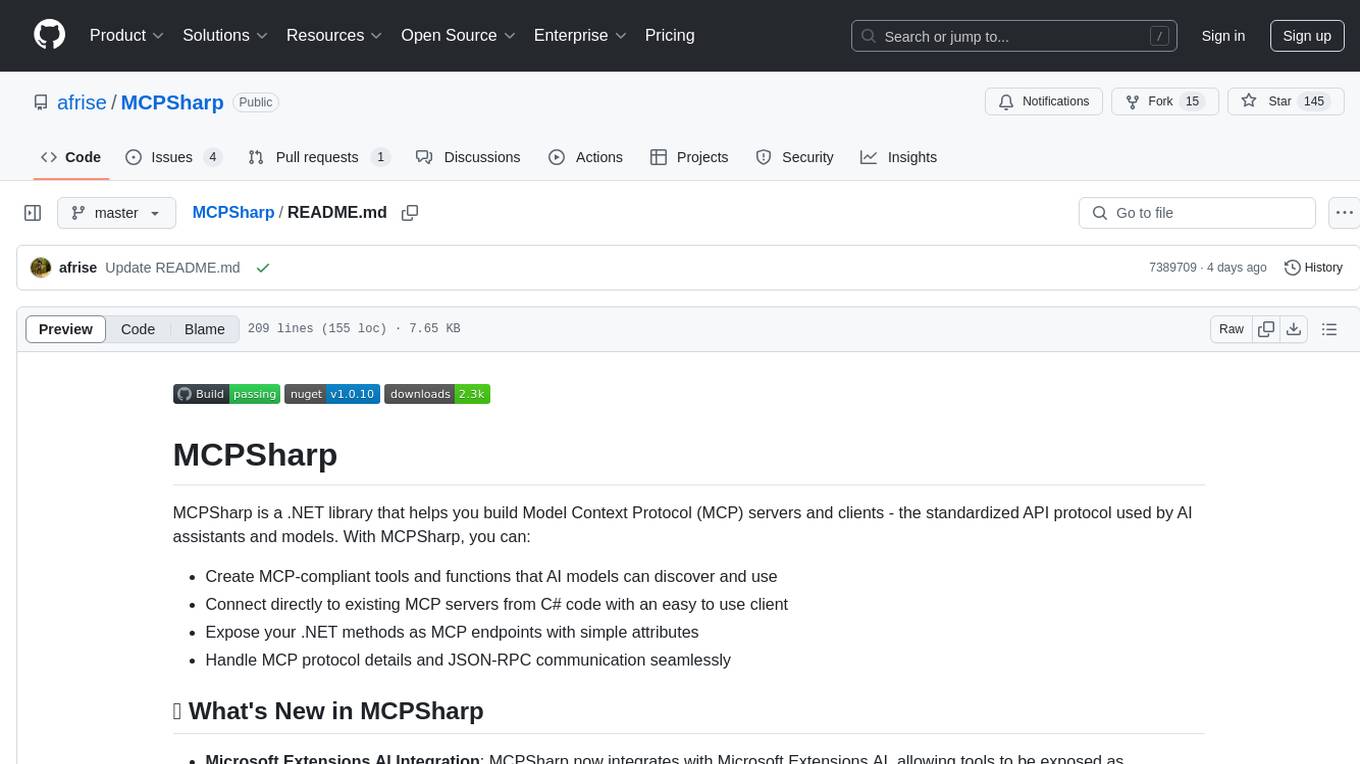
MCPSharp is a .NET library that helps build Model Context Protocol (MCP) servers and clients for AI assistants and models. It allows creating MCP-compliant tools, connecting to existing MCP servers, exposing .NET methods as MCP endpoints, and handling MCP protocol details seamlessly. With features like attribute-based API, JSON-RPC support, parameter validation, and type conversion, MCPSharp simplifies the development of AI capabilities in applications through standardized interfaces.
README:
MCPSharp is a .NET library that helps you build Model Context Protocol (MCP) servers and clients - the standardized API protocol used by AI assistants and models. With MCPSharp, you can:
- Create MCP-compliant tools and functions that AI models can discover and use
- Connect directly to existing MCP servers from C# code with an easy to use client
- Expose your .NET methods as MCP endpoints with simple attributes
- Handle MCP protocol details and JSON-RPC communication seamlessly
- Microsoft.Extensions.AI Integration: MCPSharp now integrates with Microsoft.Extensions.AI, allowing tools to be exposed as AIFunctions
- Semantic Kernel Support: Add tools using Semantic Kernel's KernelFunctionAttribute
- Dynamic Tool Registration: Register tools on-the-fly with custom implementation logic
- Tool Change Notifications: Server now notifies clients when tools are added, updated, or removed
- Complex Object Parameter Support: Better handling of complex objects in tool parameters
- Better Error Handling: Improved error handling with detailed stack traces
Use MCPSharp when you want to:
- Create tools that AI assistants like Anthropic's Claude Desktop can use
- Build MCP-compliant APIs without dealing with the protocol details
- Expose existing .NET code as MCP endpoints
- Add AI capabilities to your applications through standardized interfaces
- Integrate with Microsoft.Extensions.AI and/or Semantic Kernel without locking into a single vendor
- Easy-to-use attribute-based API (
[McpTool]
,[McpResource]
) - Built-in JSON-RPC support with automatic request/response handling
- Automatic parameter validation and type conversion
- Rich documentation support through XML comments
- Near zero configuration required for basic usage
- Any version of .NET that supports standard 2.0
dotnet add package MCPSharp
Create a class and mark your method(s) with the [McpTool]
attribute:
using MCPSharp;
public class Calculator
{
[McpTool("add", "Adds two numbers")] // Note: [McpFunction] is deprecated, use [McpTool] instead
public static int Add([McpParameter(true)] int a, [McpParameter(true)] int b)
{
return a + b;
}
}
await MCPServer.StartAsync("CalculatorServer", "1.0.0");
The StartAsync() method will automatically find any methods in the base assembly that are marked with the McpTool attribute. In order to add any methods that are in a referenced library, you can manually register them by calling MCPServer.Register<T>();
with T
being the class containing the desired methods. If your methods are marked with Semantic Kernel attributes, this will work as well. If the client supports list changed notifications, it will be notified when additional tools are registered.
Register tools dynamically with custom implementation:
MCPServer.AddToolHandler(new Tool()
{
Name = "dynamicTool",
Description = "A dynamic tool",
InputSchema = new InputSchema {
Type = "object",
Required = ["input"],
Properties = new Dictionary<string, ParameterSchema>{
{"input", new ParameterSchema{Type="string", Description="Input value"}}
}
}
}, (string input) => { return $"You provided: {input}"; });
// Client-side integration
MCPClient client = new("AIClient", "1.0", "path/to/mcp/server");
IList<AIFunction> functions = await client.GetFunctionsAsync();
This list can be plugged into the ChatOptions.Tools property for an IChatClient, Allowing MCP servers to be used seamlessly with Any IChatClient Implementation.
using Microsoft.SemanticKernel;
public class MySkillClass
{
[KernelFunction("MyFunction")]
[Description("Description of my function")]
public string MyFunction(string input) => $"Processed: {input}";
}
// Register with MCPServer
MCPServer.Register<MySkillClass>();
Currently, This is the only way to make a Semantic kernel method registerable with the MCP server. If you have a use case that is not covered here, please reach out!
-
[McpTool]
- Marks a class or method as an MCP tool- Optional parameters:
-
Name
- The tool name (default: class/method name) -
Description
- Description of the tool
-
- Optional parameters:
-
[McpParameter]
- Provides metadata for function parameters- Optional parameters:
-
Description
- Parameter description -
Required
- Whether the parameter is required (default: false)
-
- Optional parameters:
-
[McpResource]
- Marks a property or method as an MCP resource- Parameters:
-
Name
- Resource name -
Uri
- Resource URI (can include templates) -
MimeType
- MIME type of the resource -
Description
- Resource description
-
- Parameters:
-
MCPServer.StartAsync(string serverName, string version)
- Starts the MCP server -
MCPServer.Register<T>()
- Registers a class containing tools or resources -
MCPServer.AddToolHandler(Tool tool, Delegate func)
- Registers a dynamic tool
-
new MCPClient(string name, string version, string server, string args = null, IDictionary<string, string> env = null)
- Create a client instance -
client.GetToolsAsync()
- Get available tools -
client.CallToolAsync(string name, Dictionary<string, object> parameters)
- Call a tool -
client.GetResourcesAsync()
- Get available resources -
client.GetFunctionsAsync()
- Get tools as AIFunctions
MCPSharp automatically extracts documentation from XML comments:
/// <summary>
/// Provides mathematical operations
/// </summary>
public class Calculator
{
/// <summary>
/// Adds two numbers together
/// </summary>
/// <param name="a">The first number to add</param>
/// <param name="b">The second number to add</param>
/// <returns>The sum of the two numbers</returns>
[McpTool]
public static int Add(
[McpParameter(true)] int a,
[McpParameter(true)] int b)
{
return a + b;
}
}
Enable XML documentation in your project file:
<PropertyGroup>
<GenerateDocumentationFile>true</GenerateDocumentationFile>
<NoWarn>$(NoWarn);1591</NoWarn>
</PropertyGroup>
This allows you to be able to quickly change the names and descriptions of your MCP tools without having to recompile. For example, if you find the model is having trouble understanding how to use it correctly.
-
[McpFunction]
is deprecated and replaced with[McpTool]
for better alignment with MCP standards - Use
MCPServer.Register<T>()
instead ofMCPServer.RegisterTool<T>()
for consistency (old method still works but is deprecated)
We welcome contributions! Please feel free to submit a Pull Request.
This project is licensed under the MIT License.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for MCPSharp
Similar Open Source Tools
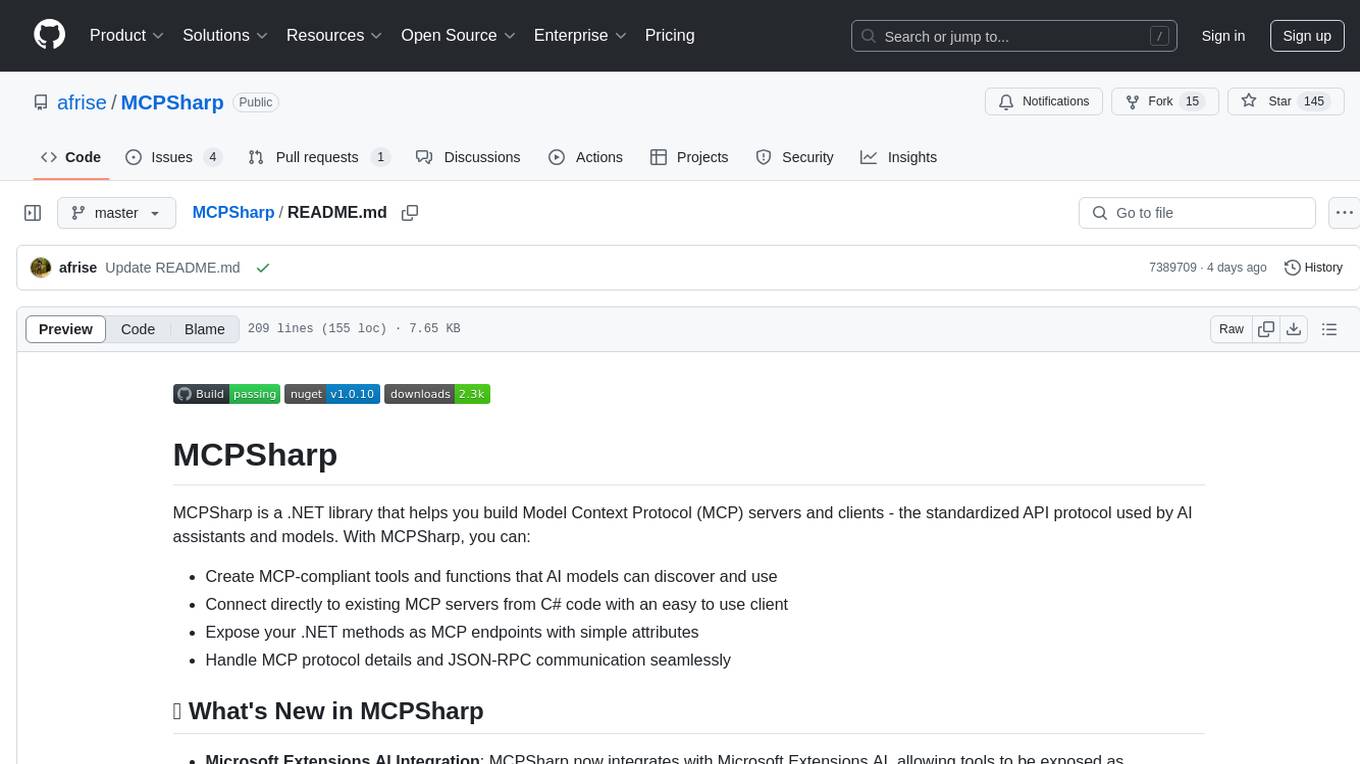
MCPSharp
MCPSharp is a .NET library that helps build Model Context Protocol (MCP) servers and clients for AI assistants and models. It allows creating MCP-compliant tools, connecting to existing MCP servers, exposing .NET methods as MCP endpoints, and handling MCP protocol details seamlessly. With features like attribute-based API, JSON-RPC support, parameter validation, and type conversion, MCPSharp simplifies the development of AI capabilities in applications through standardized interfaces.
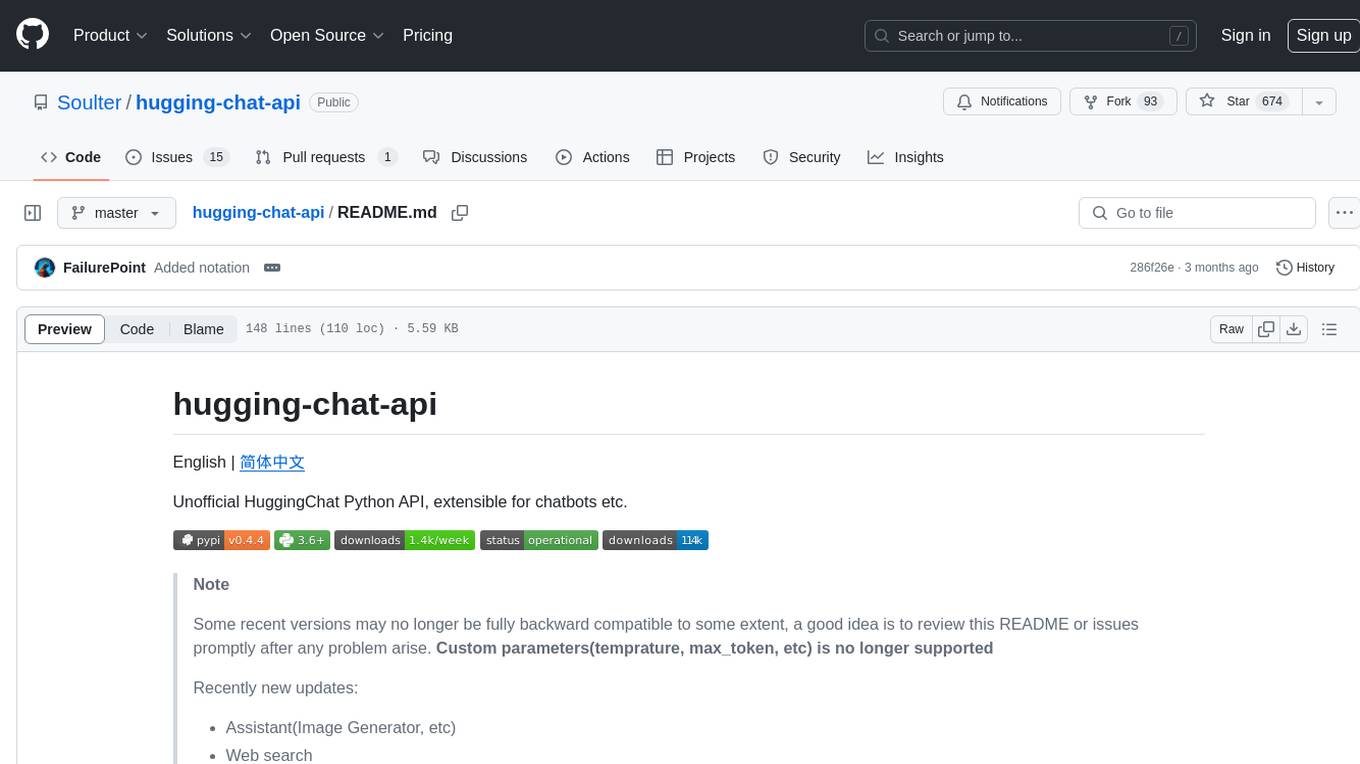
hugging-chat-api
Unofficial HuggingChat Python API for creating chatbots, supporting features like image generation, web search, memorizing context, and changing LLMs. Users can log in, chat with the ChatBot, perform web searches, create new conversations, manage conversations, switch models, get conversation info, use assistants, and delete conversations. The API also includes a CLI mode with various commands for interacting with the tool. Users are advised not to use the application for high-stakes decisions or advice and to avoid high-frequency requests to preserve server resources.
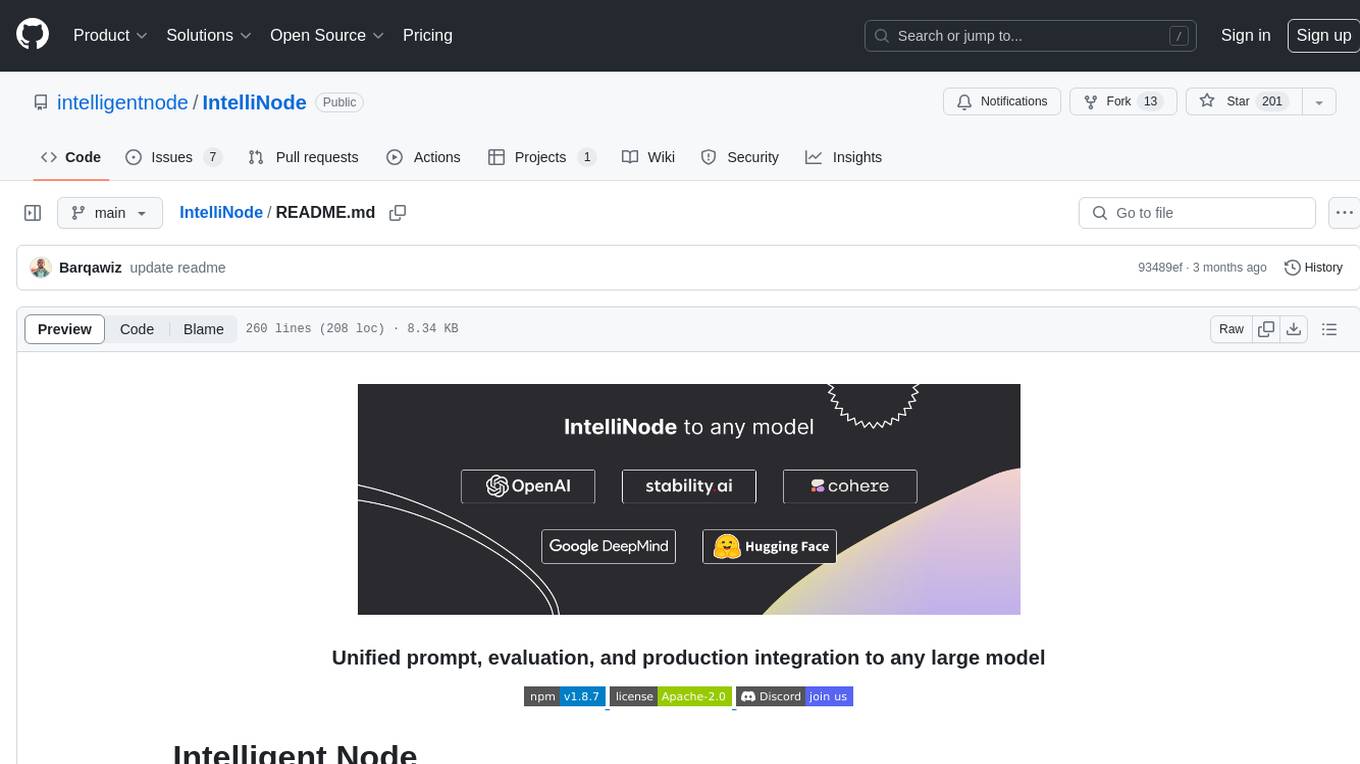
IntelliNode
IntelliNode is a javascript module that integrates cutting-edge AI models like ChatGPT, LLaMA, WaveNet, Gemini, and Stable diffusion into projects. It offers functions for generating text, speech, and images, as well as semantic search, multi-model evaluation, and chatbot capabilities. The module provides a wrapper layer for low-level model access, a controller layer for unified input handling, and a function layer for abstract functionality tailored to various use cases.
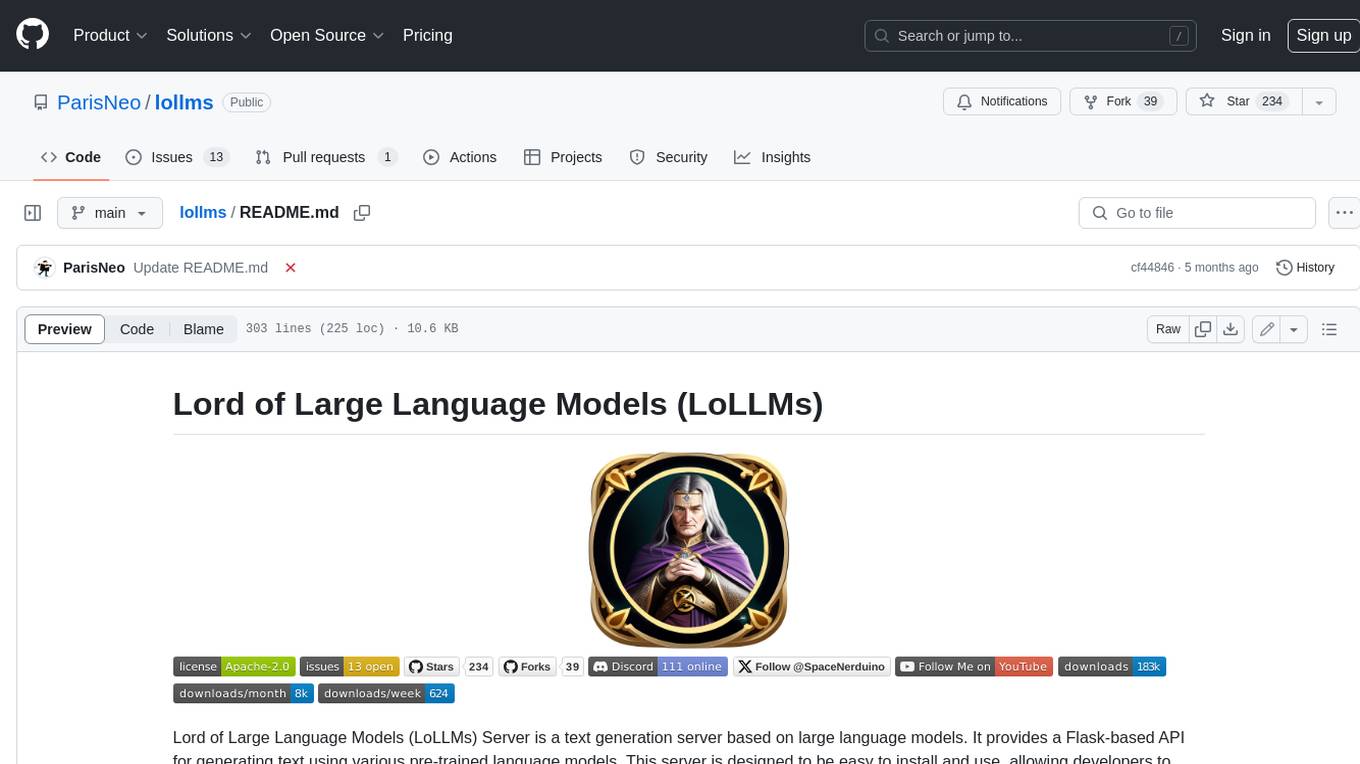
lollms
LoLLMs Server is a text generation server based on large language models. It provides a Flask-based API for generating text using various pre-trained language models. This server is designed to be easy to install and use, allowing developers to integrate powerful text generation capabilities into their applications.
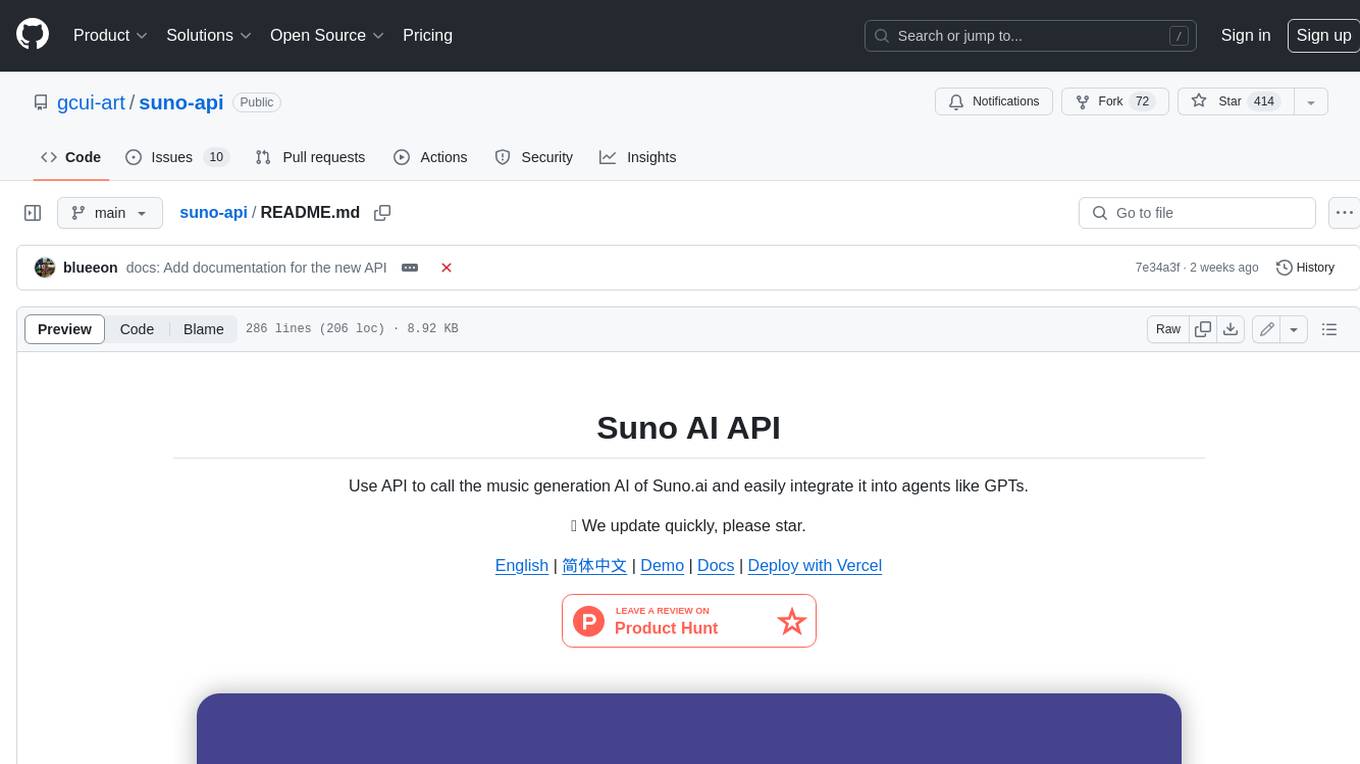
suno-api
Suno AI API is an open-source project that allows developers to integrate the music generation capabilities of Suno.ai into their own applications. The API provides a simple and convenient way to generate music, lyrics, and other audio content using Suno.ai's powerful AI models. With Suno AI API, developers can easily add music generation functionality to their apps, websites, and other projects.
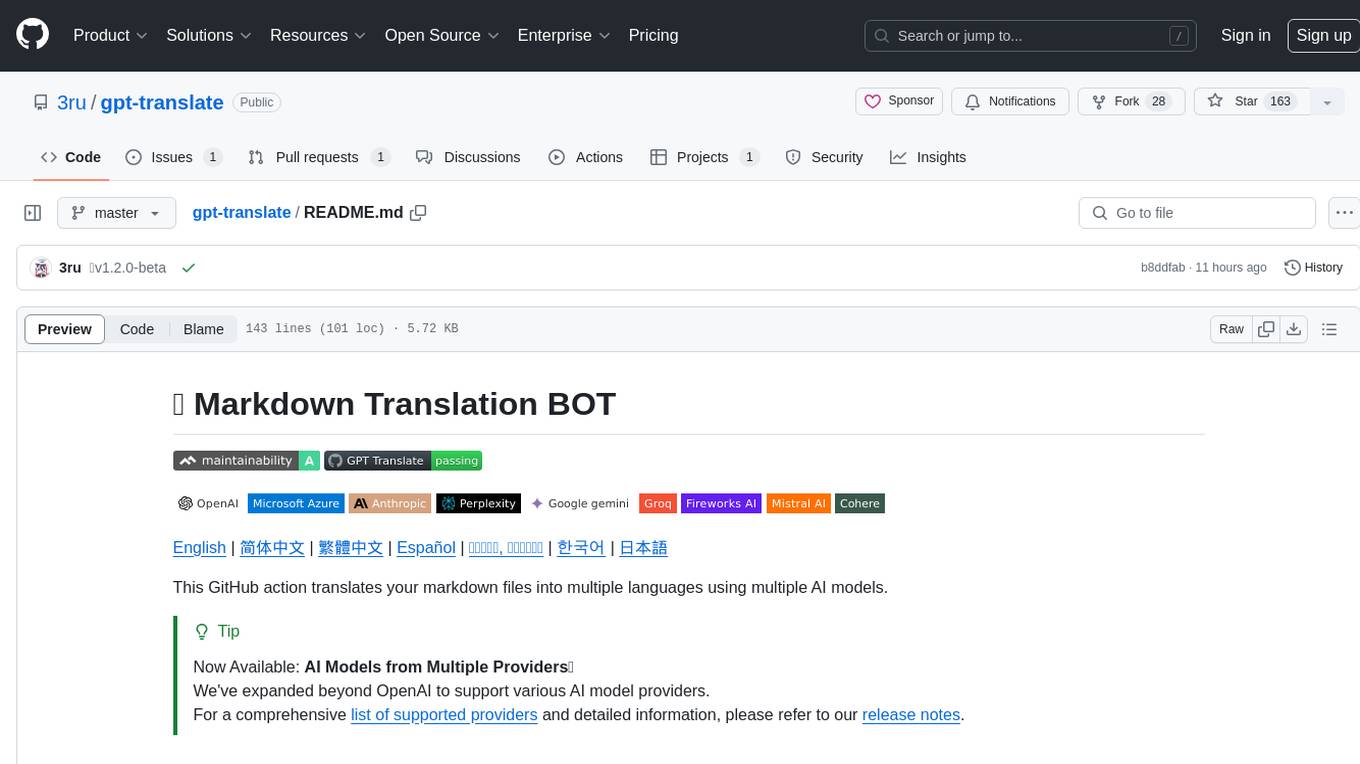
gpt-translate
Markdown Translation BOT is a GitHub action that translates markdown files into multiple languages using various AI models. It supports markdown, markdown-jsx, and json files only. The action can be executed by individuals with write permissions to the repository, preventing API abuse by non-trusted parties. Users can set up the action by providing their API key and configuring the workflow settings. The tool allows users to create comments with specific commands to trigger translations and automatically generate pull requests or add translated files to existing pull requests. It supports multiple file translations and can interpret any language supported by GPT-4 or GPT-3.5.
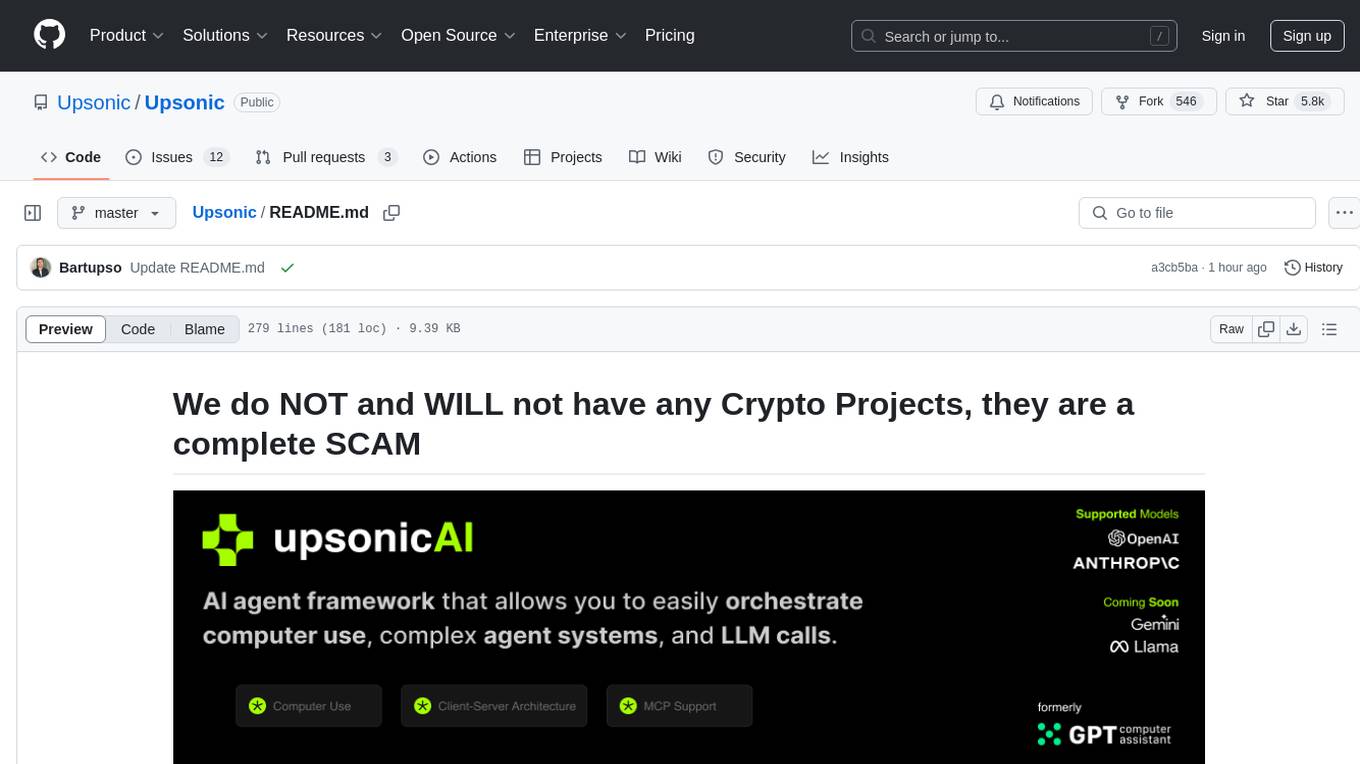
gpt-computer-assistant
GPT Computer Assistant (GCA) is an open-source framework designed to build vertical AI agents that can automate tasks on Windows, macOS, and Ubuntu systems. It leverages the Model Context Protocol (MCP) and its own modules to mimic human-like actions and achieve advanced capabilities. With GCA, users can empower themselves to accomplish more in less time by automating tasks like updating dependencies, analyzing databases, and configuring cloud security settings.
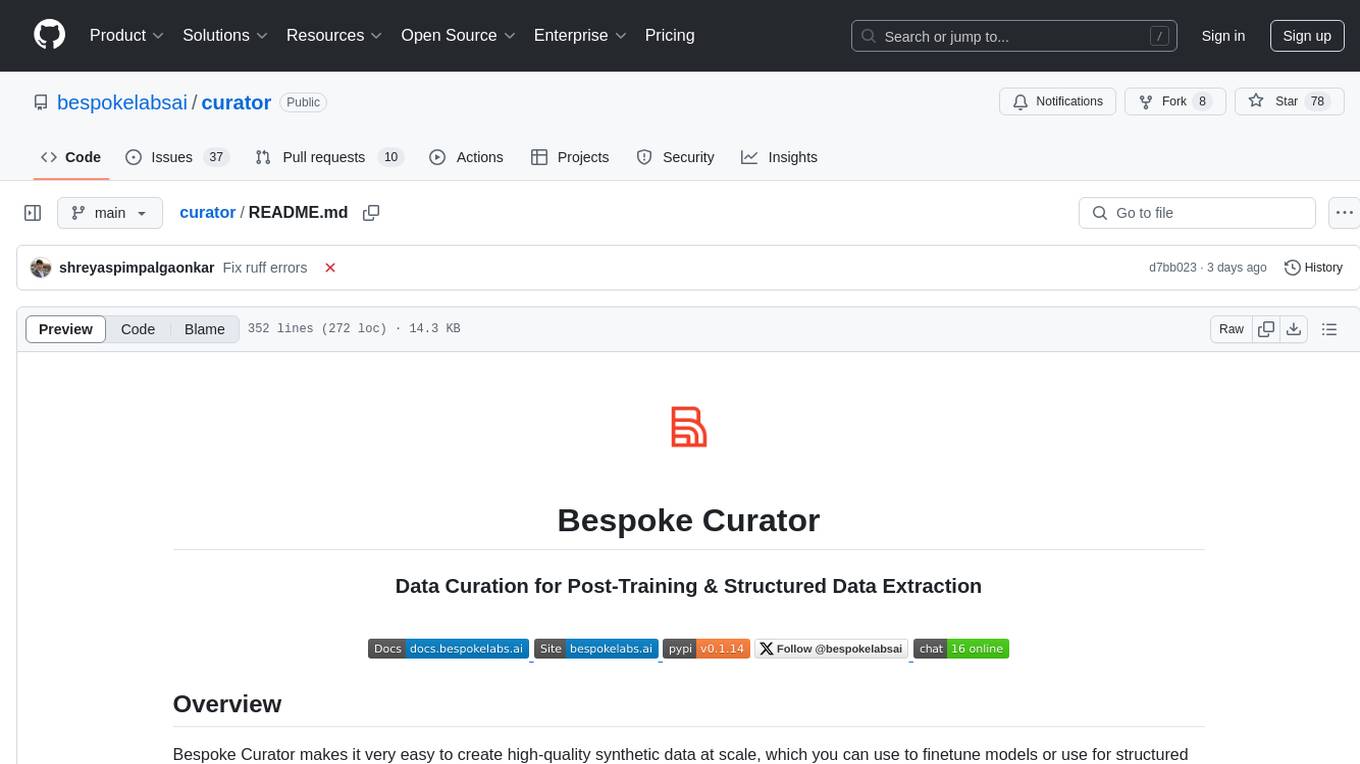
curator
Bespoke Curator is an open-source tool for data curation and structured data extraction. It provides a Python library for generating synthetic data at scale, with features like programmability, performance optimization, caching, and integration with HuggingFace Datasets. The tool includes a Curator Viewer for dataset visualization and offers a rich set of functionalities for creating and refining data generation strategies.
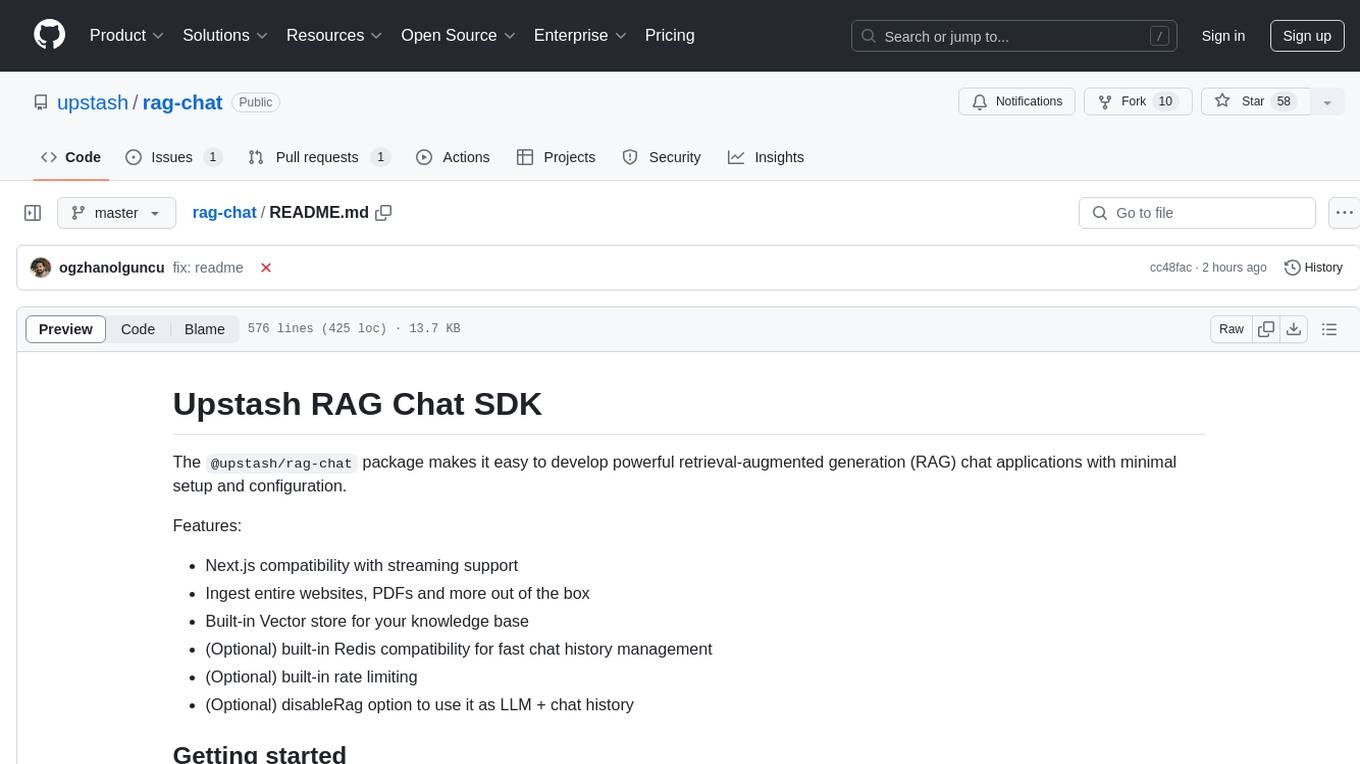
rag-chat
The `@upstash/rag-chat` package simplifies the development of retrieval-augmented generation (RAG) chat applications by providing Next.js compatibility with streaming support, built-in vector store, optional Redis compatibility for fast chat history management, rate limiting, and disableRag option. Users can easily set up the environment variables and initialize RAGChat to interact with AI models, manage knowledge base, chat history, and enable debugging features. Advanced configuration options allow customization of RAGChat instance with built-in rate limiting, observability via Helicone, and integration with Next.js route handlers and Vercel AI SDK. The package supports OpenAI models, Upstash-hosted models, and custom providers like TogetherAi and Replicate.
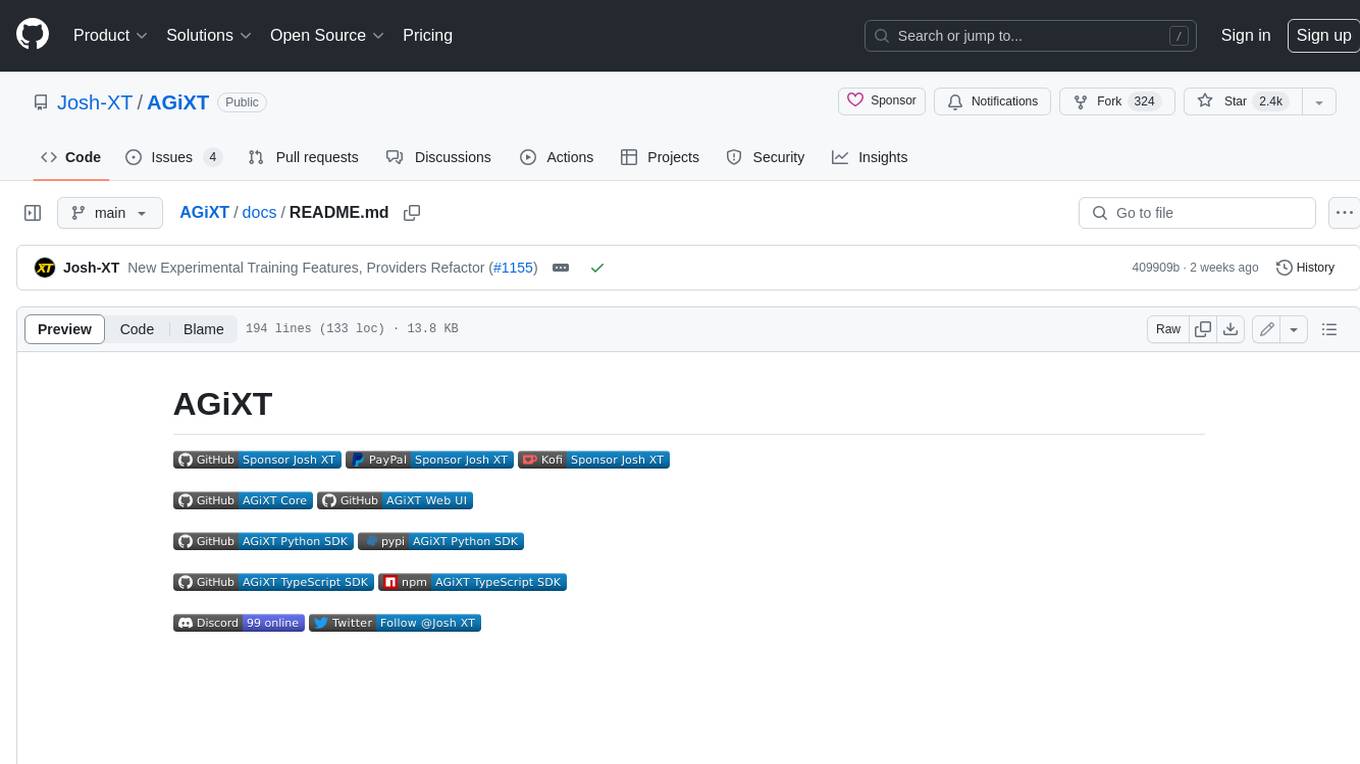
AGiXT
AGiXT is a dynamic Artificial Intelligence Automation Platform engineered to orchestrate efficient AI instruction management and task execution across a multitude of providers. Our solution infuses adaptive memory handling with a broad spectrum of commands to enhance AI's understanding and responsiveness, leading to improved task completion. The platform's smart features, like Smart Instruct and Smart Chat, seamlessly integrate web search, planning strategies, and conversation continuity, transforming the interaction between users and AI. By leveraging a powerful plugin system that includes web browsing and command execution, AGiXT stands as a versatile bridge between AI models and users. With an expanding roster of AI providers, code evaluation capabilities, comprehensive chain management, and platform interoperability, AGiXT is consistently evolving to drive a multitude of applications, affirming its place at the forefront of AI technology.
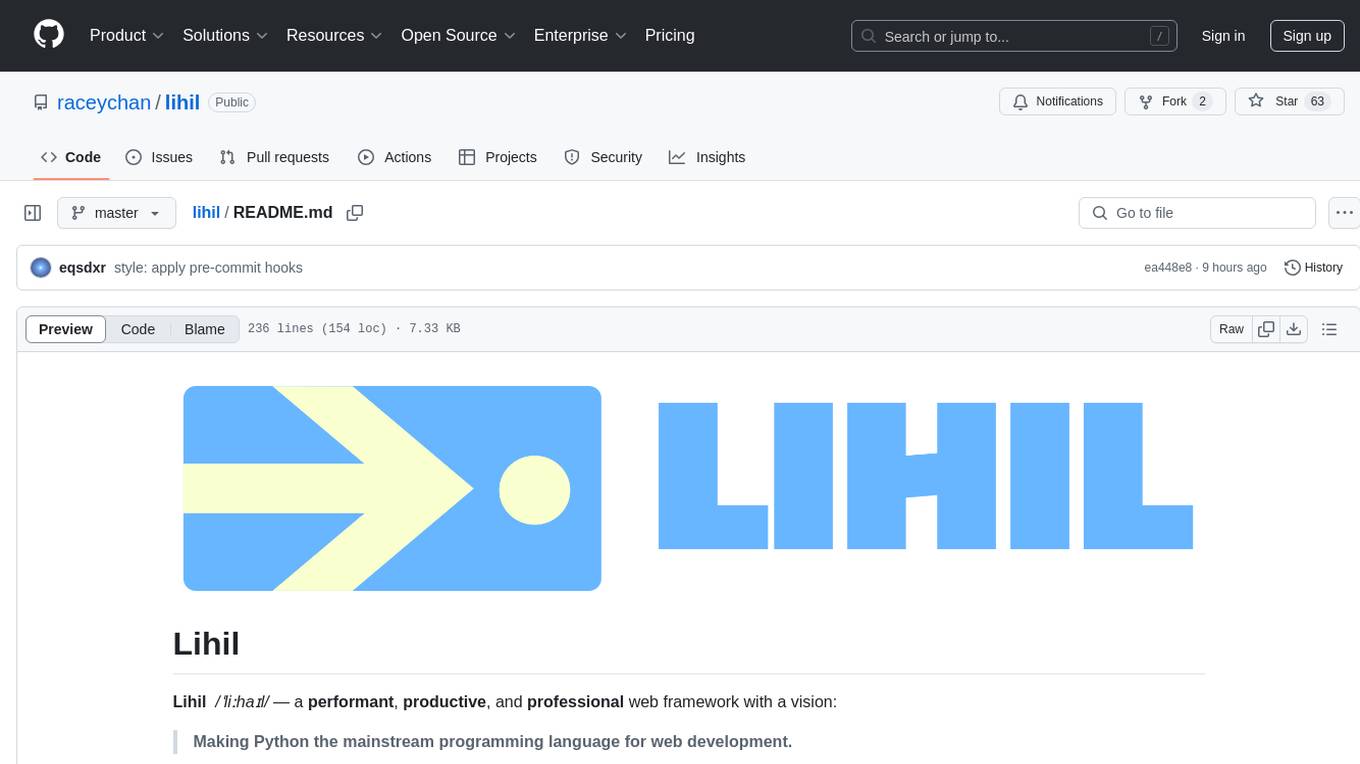
lihil
Lihil is a performant, productive, and professional web framework designed to make Python the mainstream programming language for web development. It is 100% test covered and strictly typed, offering fast performance, ergonomic API, and built-in solutions for common problems. Lihil is suitable for enterprise web development, delivering robust and scalable solutions with best practices in microservice architecture and related patterns. It features dependency injection, OpenAPI docs generation, error response generation, data validation, message system, testability, and strong support for AI features. Lihil is ASGI compatible and uses starlette as its ASGI toolkit, ensuring compatibility with starlette classes and middlewares. The framework follows semantic versioning and has a roadmap for future enhancements and features.
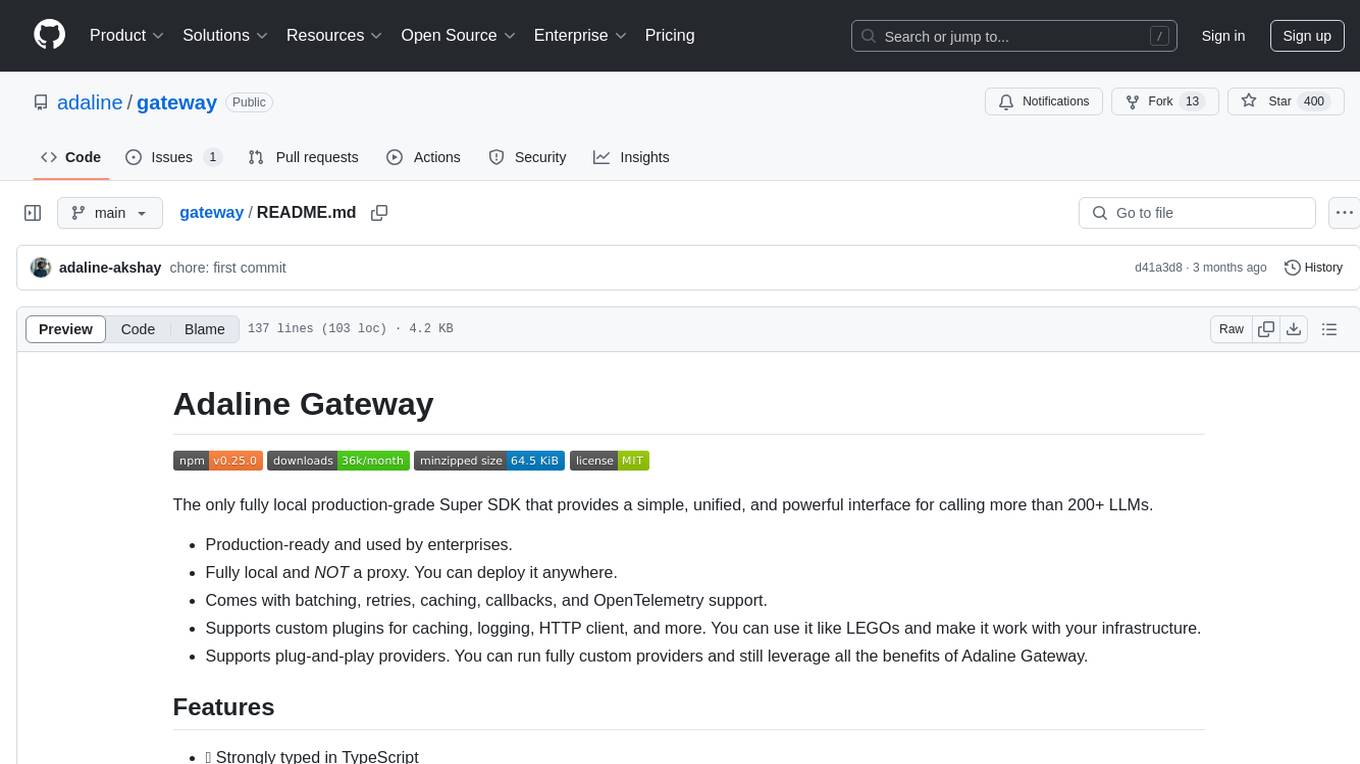
gateway
Adaline Gateway is a fully local production-grade Super SDK that offers a unified interface for calling over 200+ LLMs. It is production-ready, supports batching, retries, caching, callbacks, and OpenTelemetry. Users can create custom plugins and providers for seamless integration with their infrastructure.
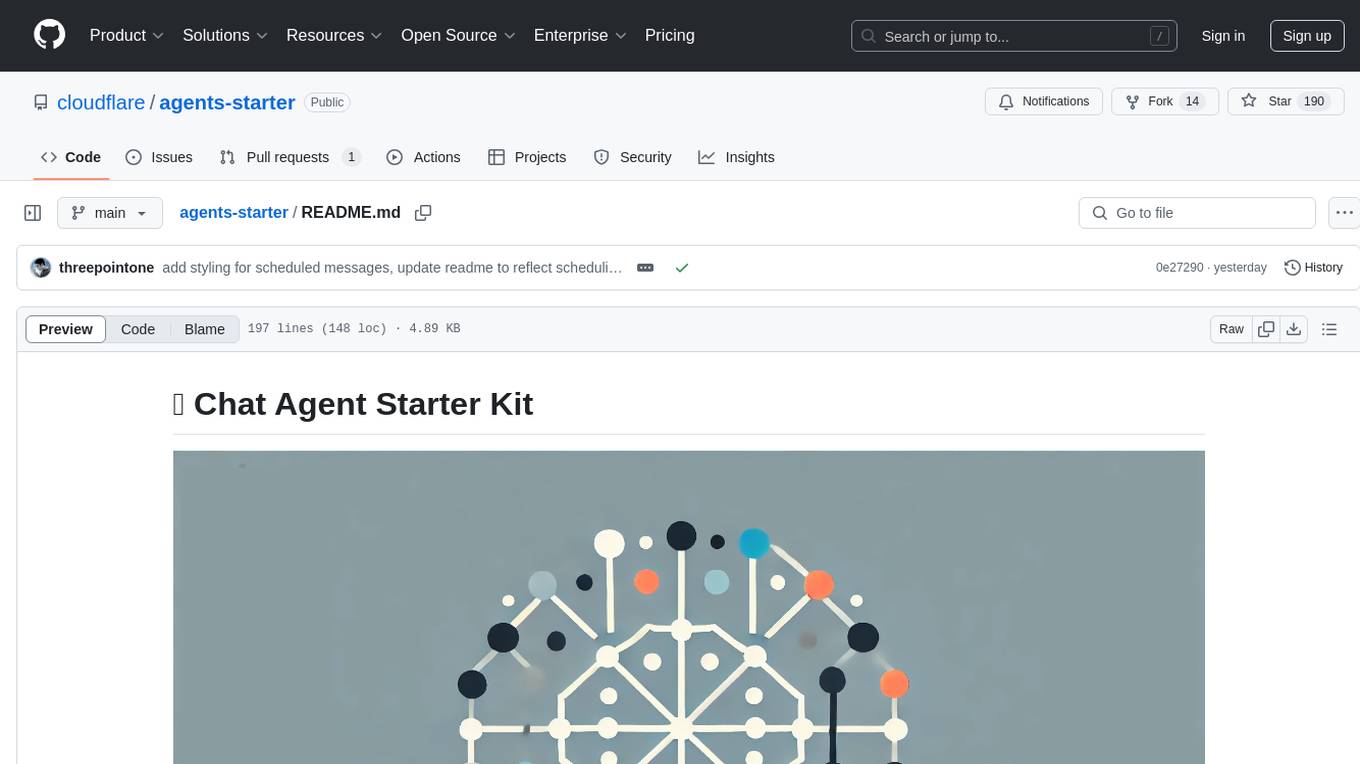
agents-starter
A starter template for building AI-powered chat agents using Cloudflare's Agent platform, powered by agents-sdk. It provides a foundation for creating interactive chat experiences with AI, complete with a modern UI and tool integration capabilities. Features include interactive chat interface with AI, built-in tool system with human-in-the-loop confirmation, advanced task scheduling, dark/light theme support, real-time streaming responses, state management, and chat history. Prerequisites include a Cloudflare account and OpenAI API key. The project structure includes components for chat UI implementation, chat agent logic, tool definitions, and helper functions. Customization guide covers adding new tools, modifying the UI, and example use cases for customer support, development assistant, data analysis assistant, personal productivity assistant, and scheduling assistant.
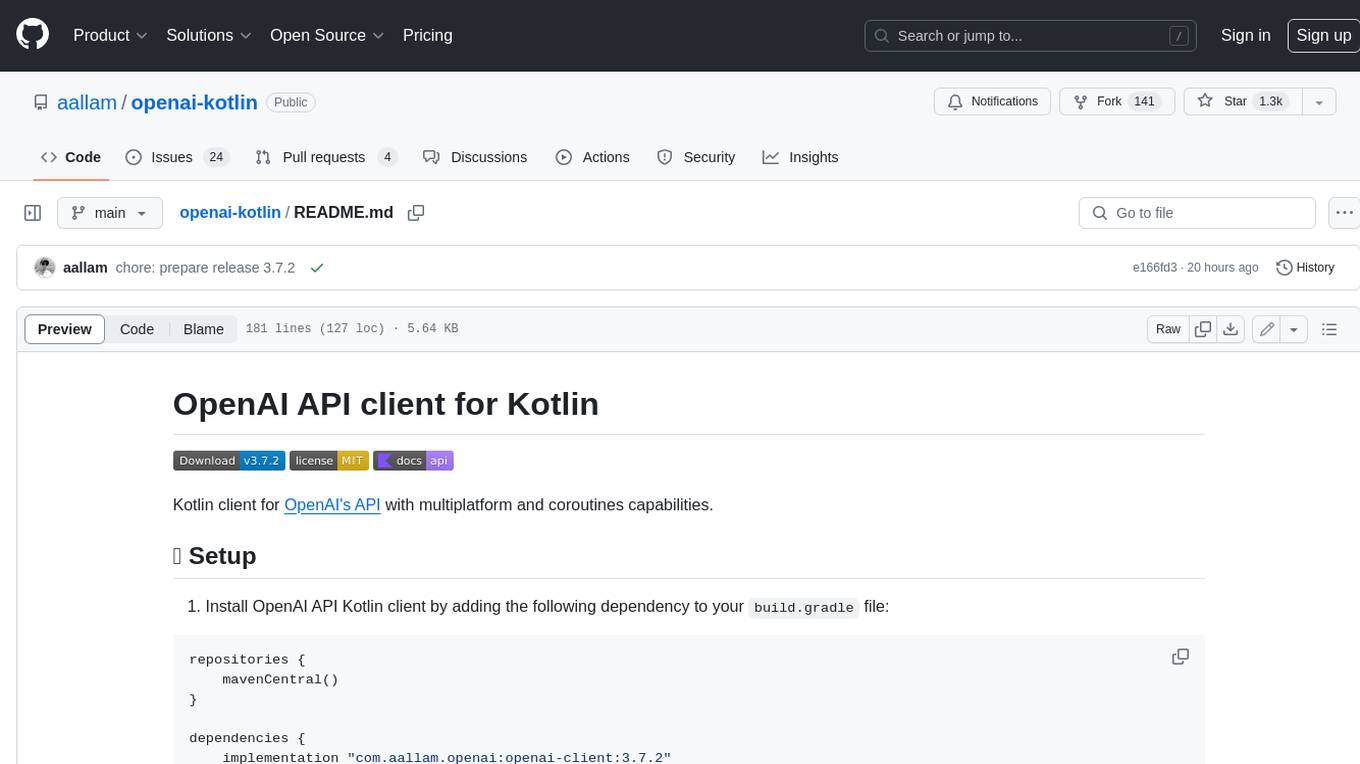
openai-kotlin
OpenAI Kotlin API client is a Kotlin client for OpenAI's API with multiplatform and coroutines capabilities. It allows users to interact with OpenAI's API using Kotlin programming language. The client supports various features such as models, chat, images, embeddings, files, fine-tuning, moderations, audio, assistants, threads, messages, and runs. It also provides guides on getting started, chat & function call, file source guide, and assistants. Sample apps are available for reference, and troubleshooting guides are provided for common issues. The project is open-source and licensed under the MIT license, allowing contributions from the community.
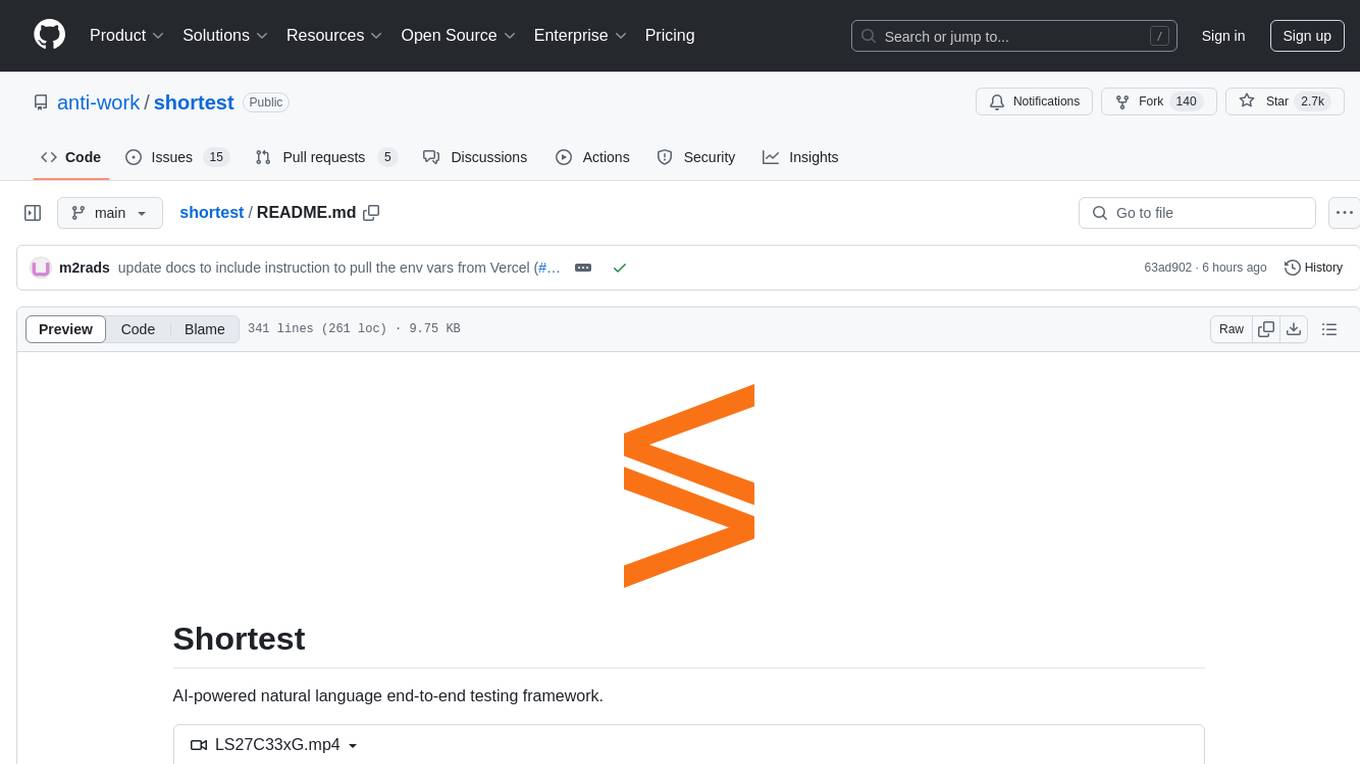
shortest
Shortest is an AI-powered natural language end-to-end testing framework built on Playwright. It provides a seamless testing experience by allowing users to write tests in natural language and execute them using Anthropic Claude API. The framework also offers GitHub integration with 2FA support, making it suitable for testing web applications with complex authentication flows. Shortest simplifies the testing process by enabling users to run tests locally or in CI/CD pipelines, ensuring the reliability and efficiency of web applications.
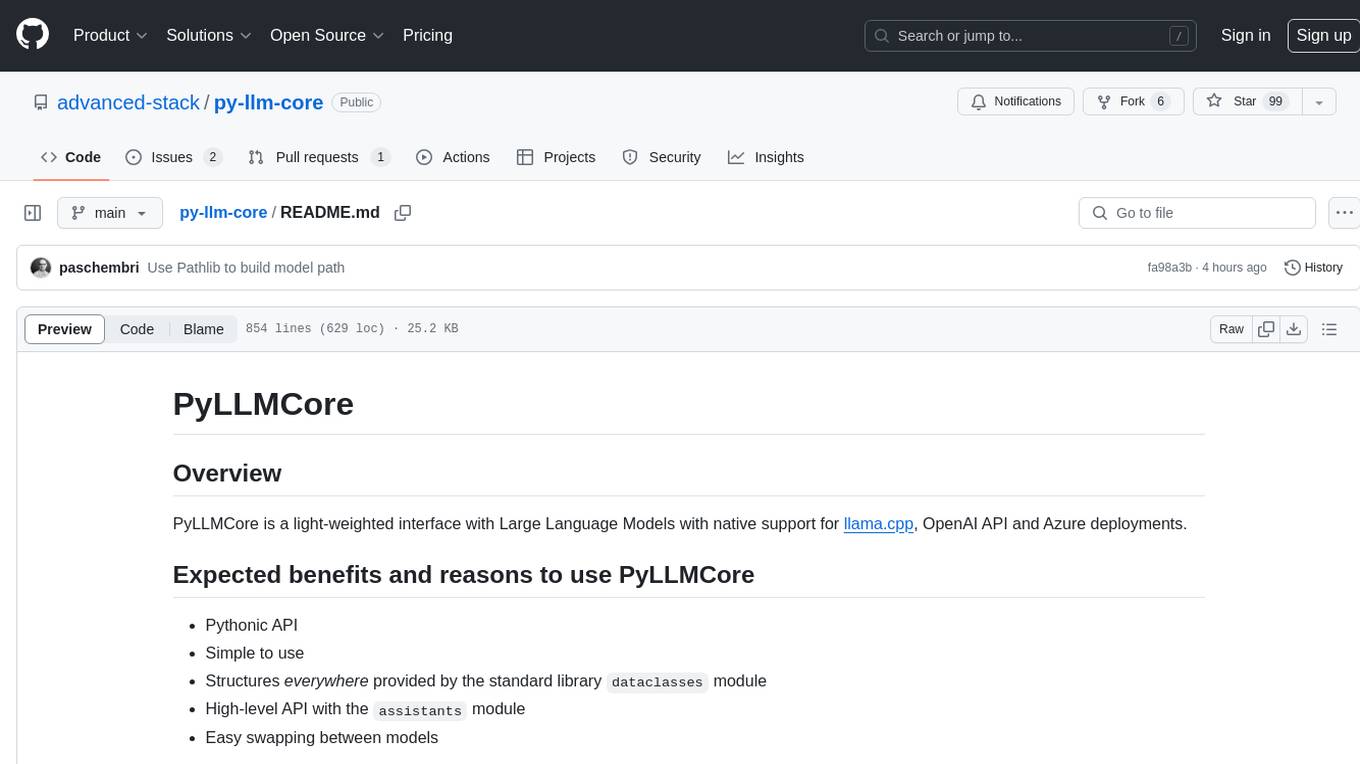
py-llm-core
PyLLMCore is a light-weighted interface with Large Language Models with native support for llama.cpp, OpenAI API, and Azure deployments. It offers a Pythonic API that is simple to use, with structures provided by the standard library dataclasses module. The high-level API includes the assistants module for easy swapping between models. PyLLMCore supports various models including those compatible with llama.cpp, OpenAI, and Azure APIs. It covers use cases such as parsing, summarizing, question answering, hallucinations reduction, context size management, and tokenizing. The tool allows users to interact with language models for tasks like parsing text, summarizing content, answering questions, reducing hallucinations, managing context size, and tokenizing text.
For similar tasks
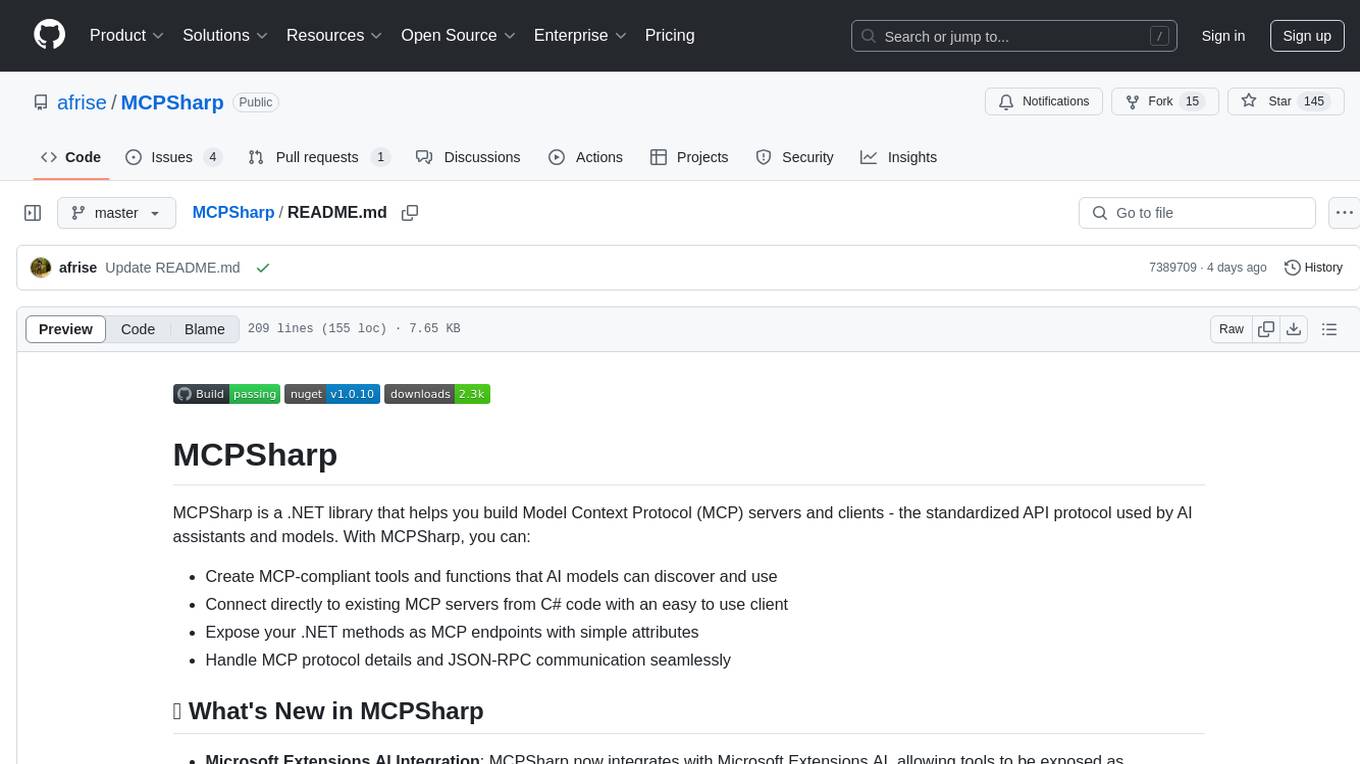
MCPSharp
MCPSharp is a .NET library that helps build Model Context Protocol (MCP) servers and clients for AI assistants and models. It allows creating MCP-compliant tools, connecting to existing MCP servers, exposing .NET methods as MCP endpoints, and handling MCP protocol details seamlessly. With features like attribute-based API, JSON-RPC support, parameter validation, and type conversion, MCPSharp simplifies the development of AI capabilities in applications through standardized interfaces.
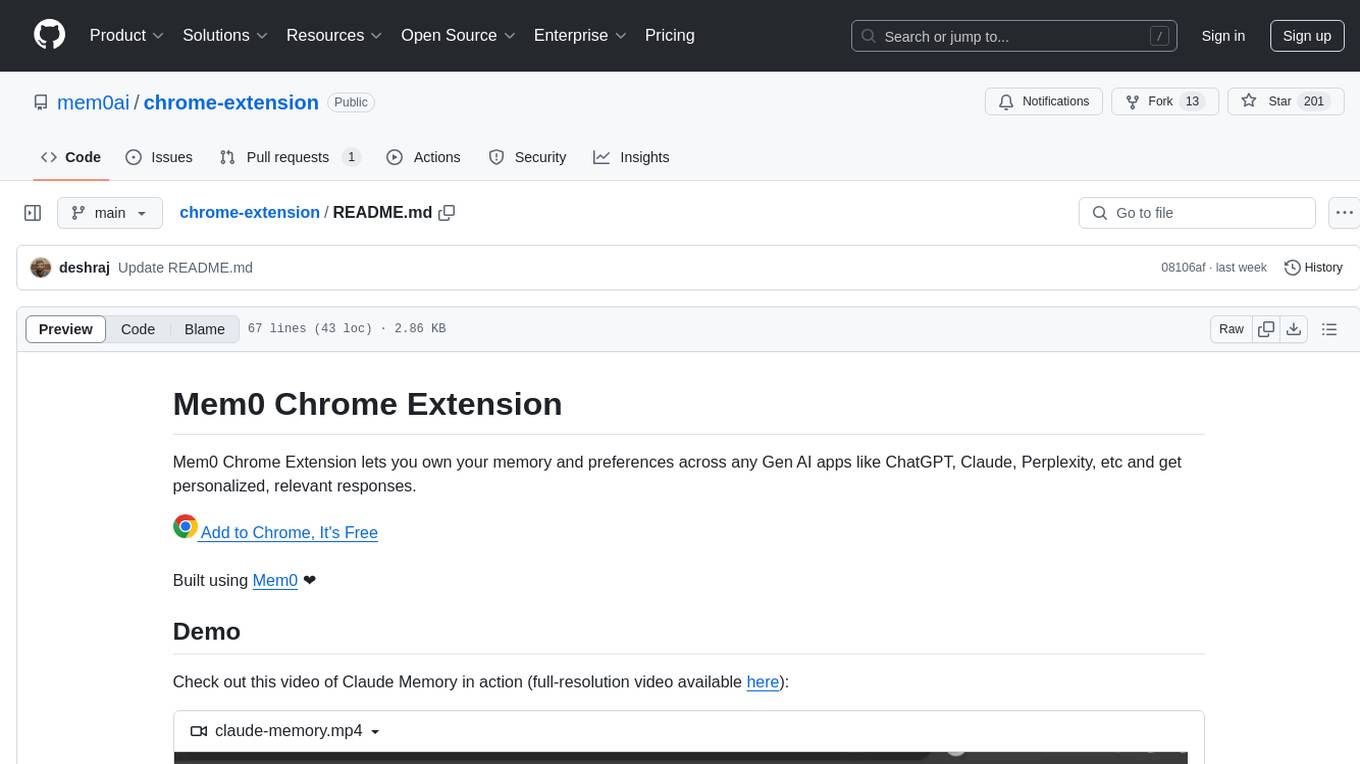
chrome-extension
Mem0 Chrome Extension lets you own your memory and preferences across any Gen AI apps like ChatGPT, Claude, Perplexity, etc and get personalized, relevant responses. It allows users to store memories from conversations, retrieve relevant memories during chats, manage and organize stored information, and seamlessly integrate with the Claude AI interface. The extension requires an API key and user ID for connecting to the Mem0 API, and it stores this information locally in the browser. Users can troubleshoot common issues, and contributions to improve the extension are welcome under the MIT License.
For similar jobs
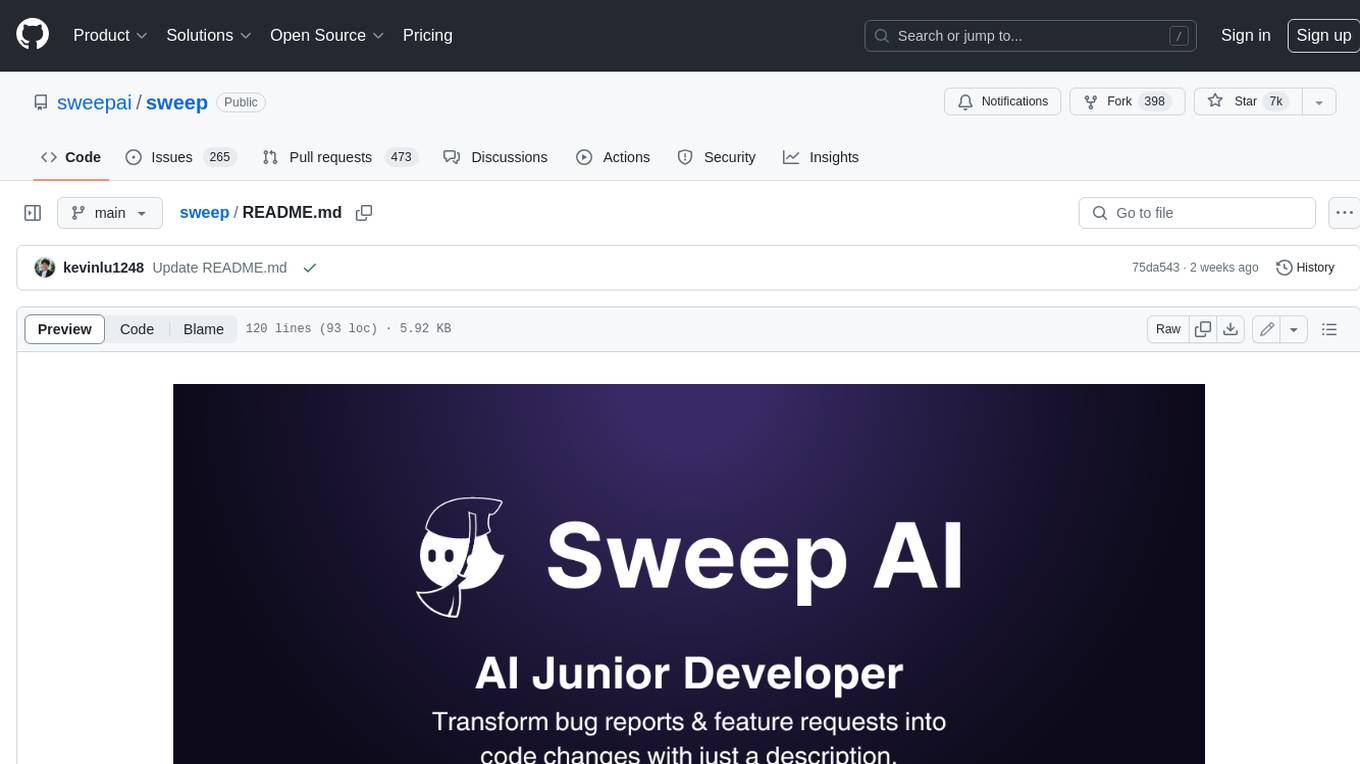
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
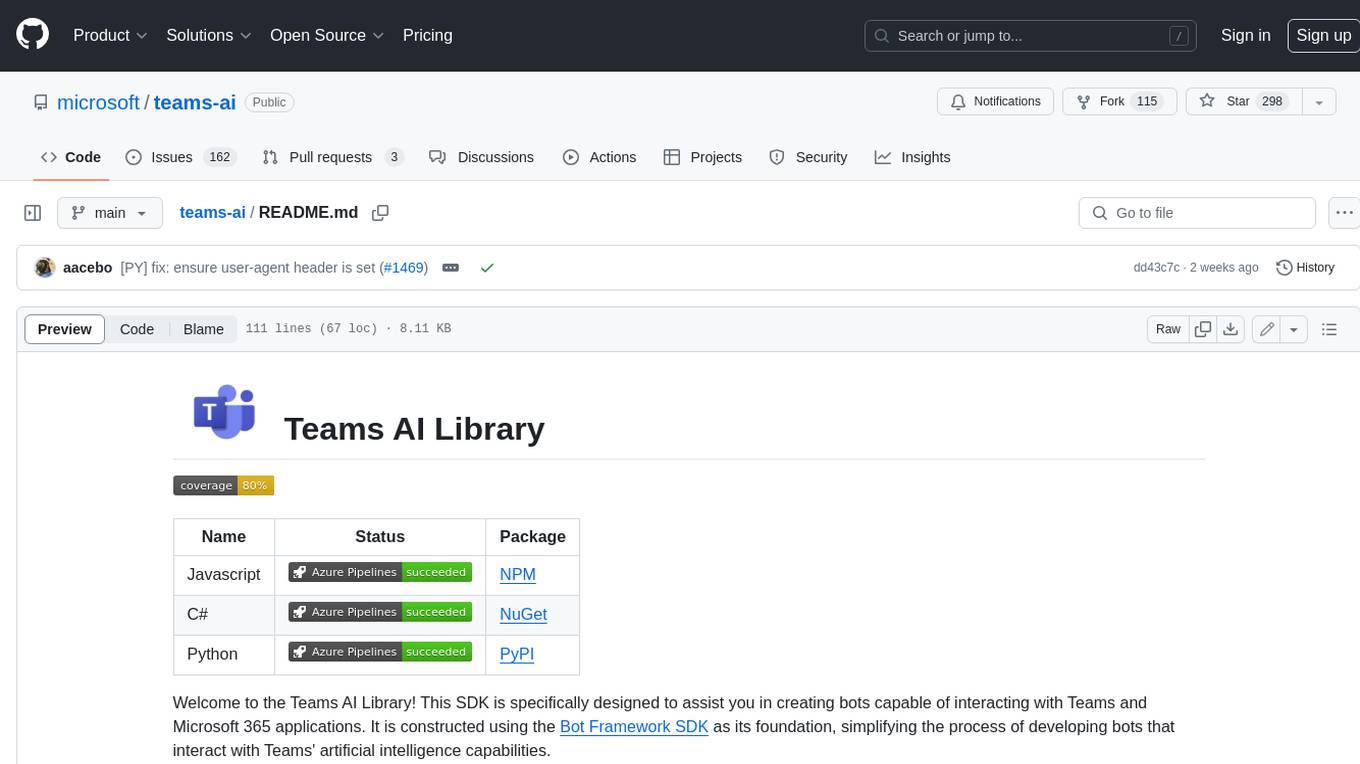
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
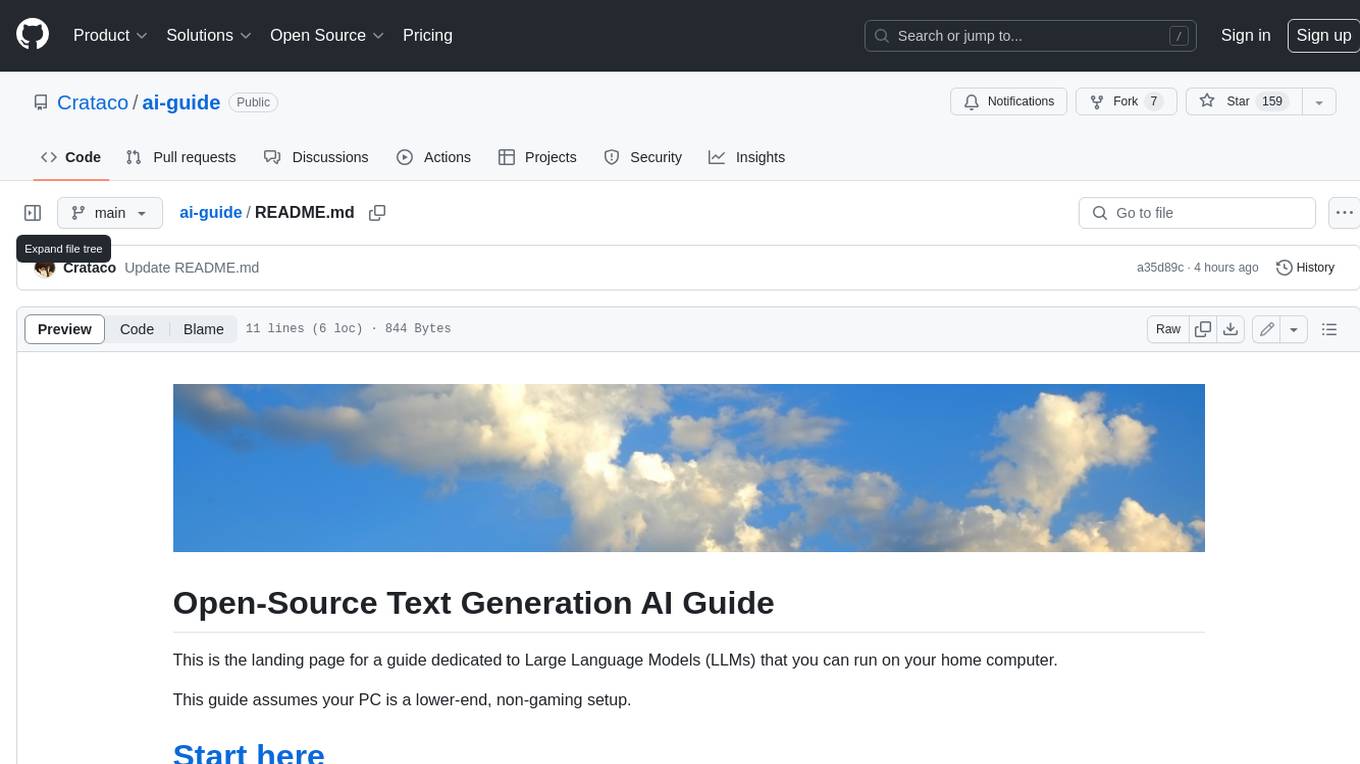
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
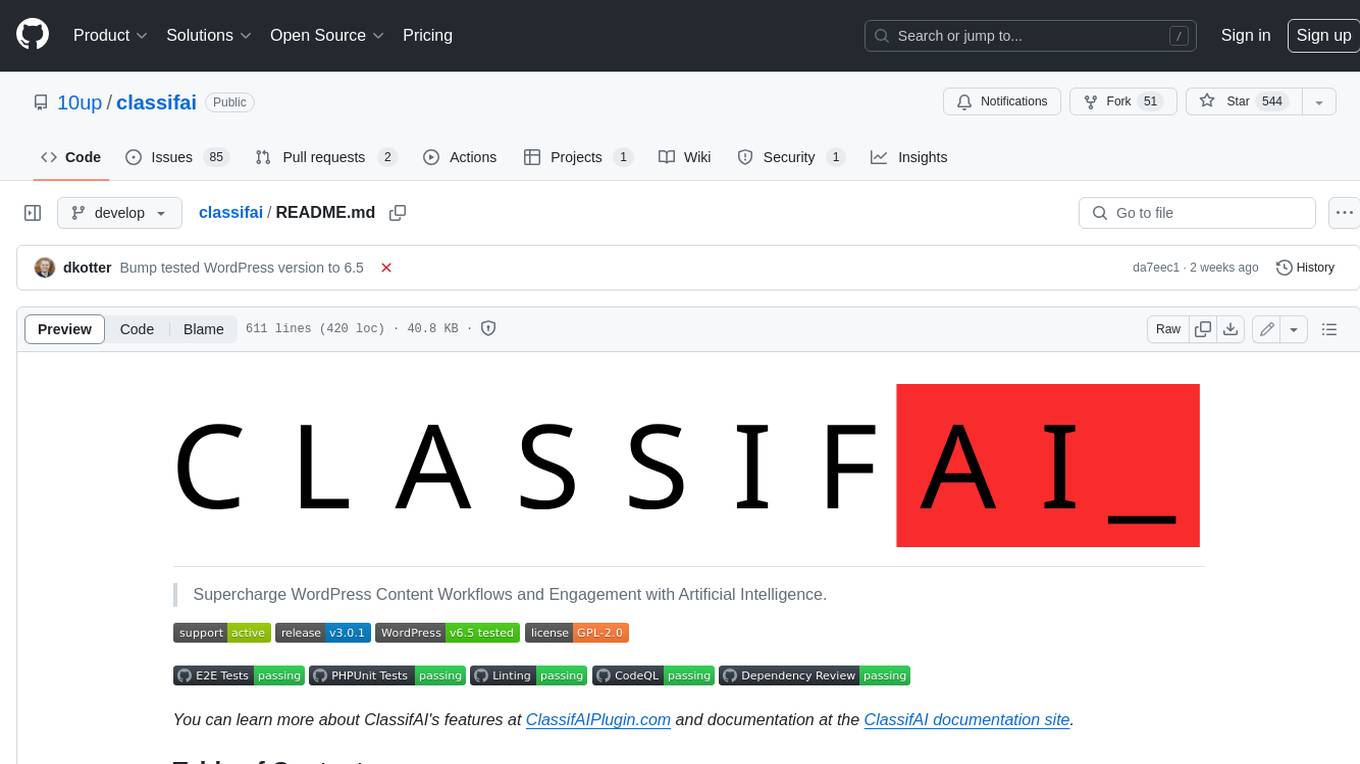
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
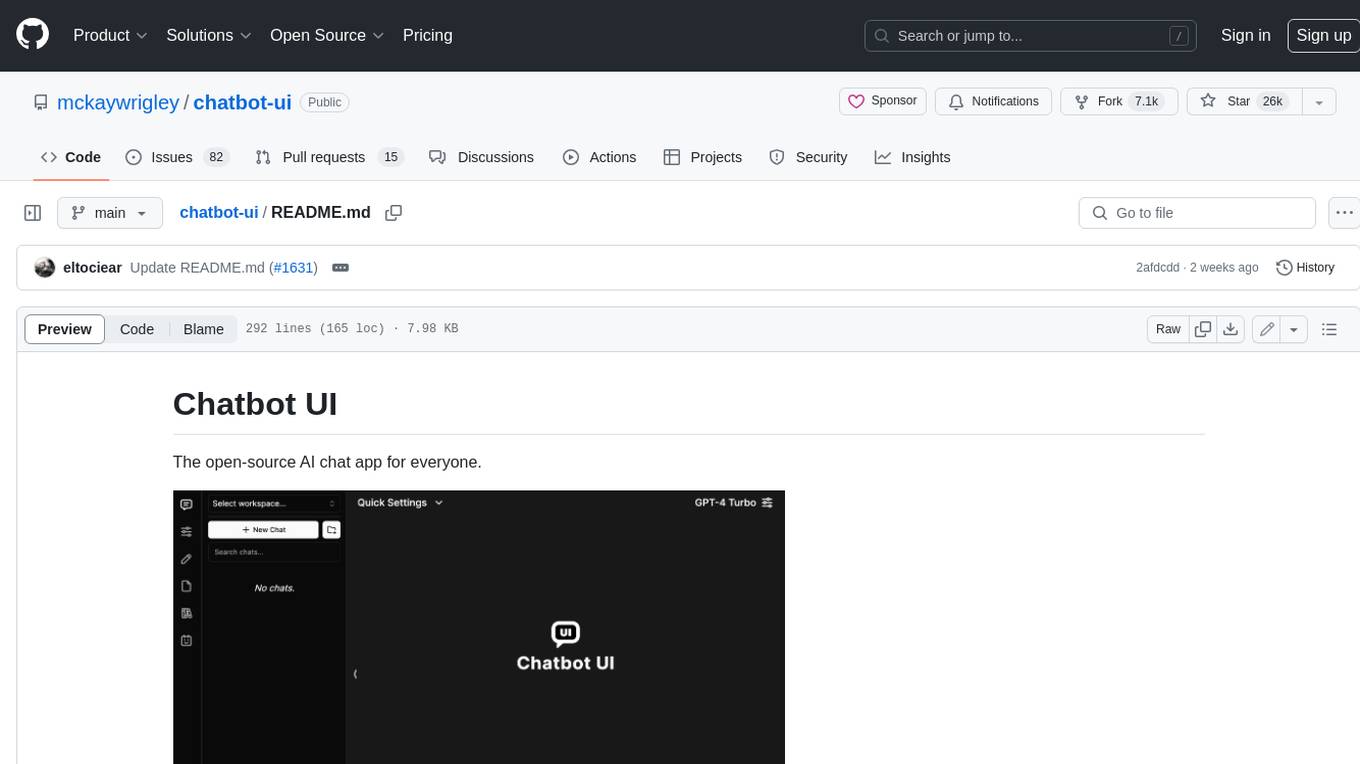
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
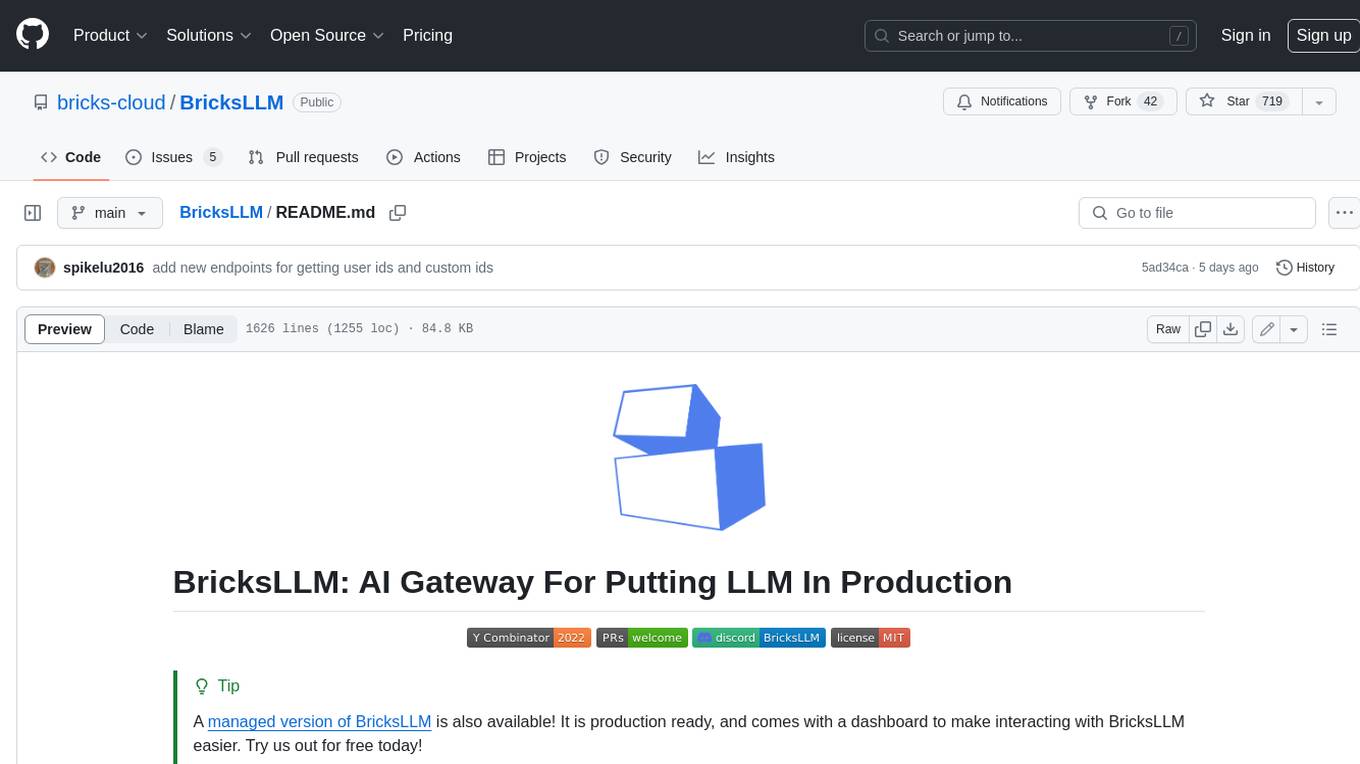
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
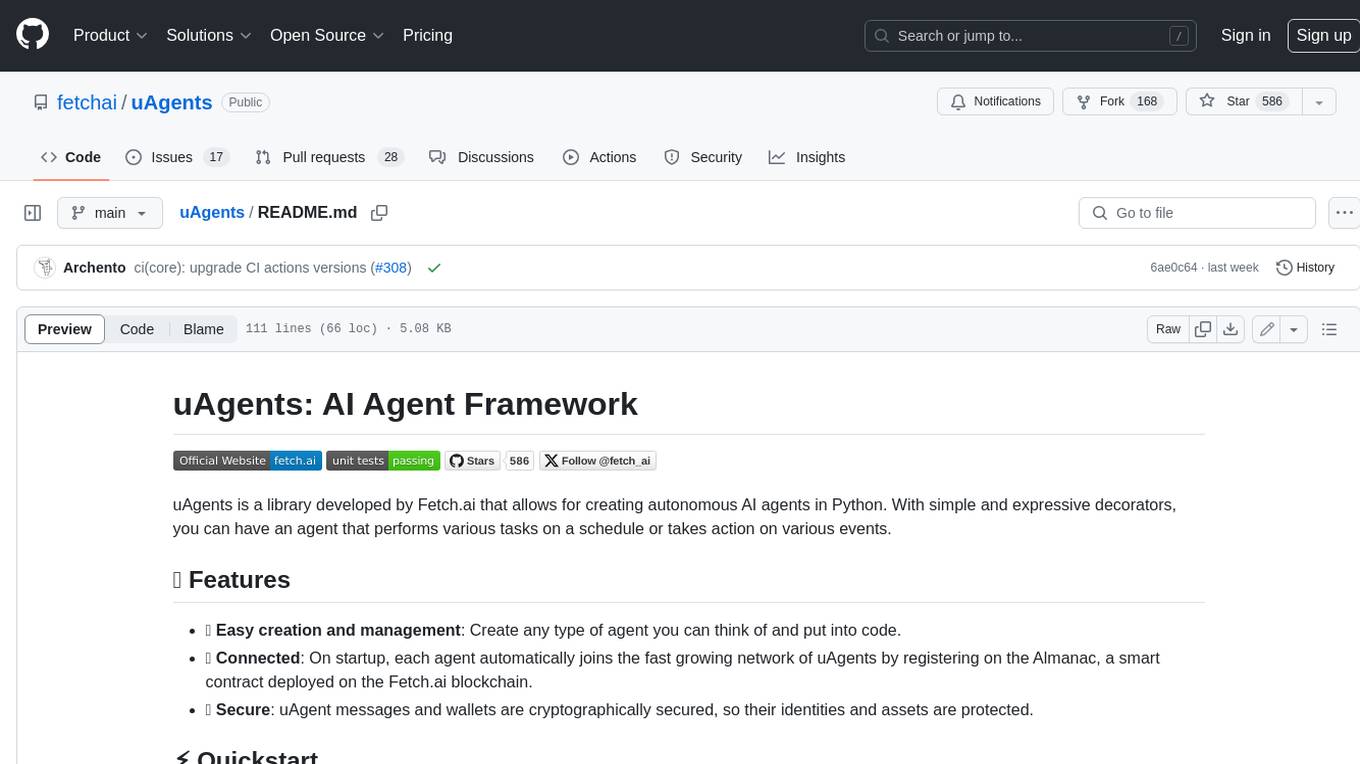
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
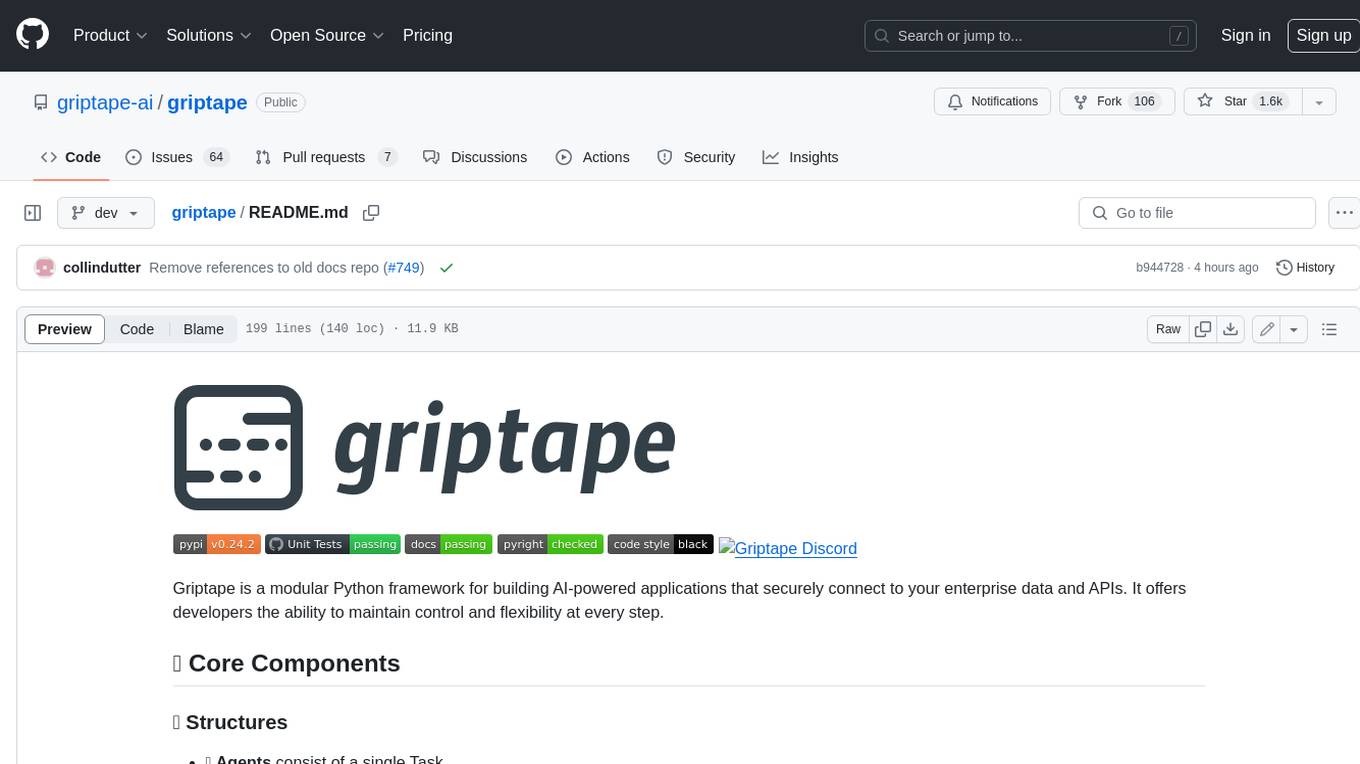
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.