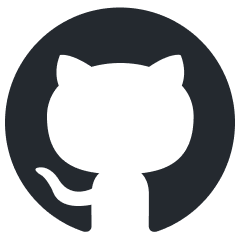
100days_AI
None
Stars: 226
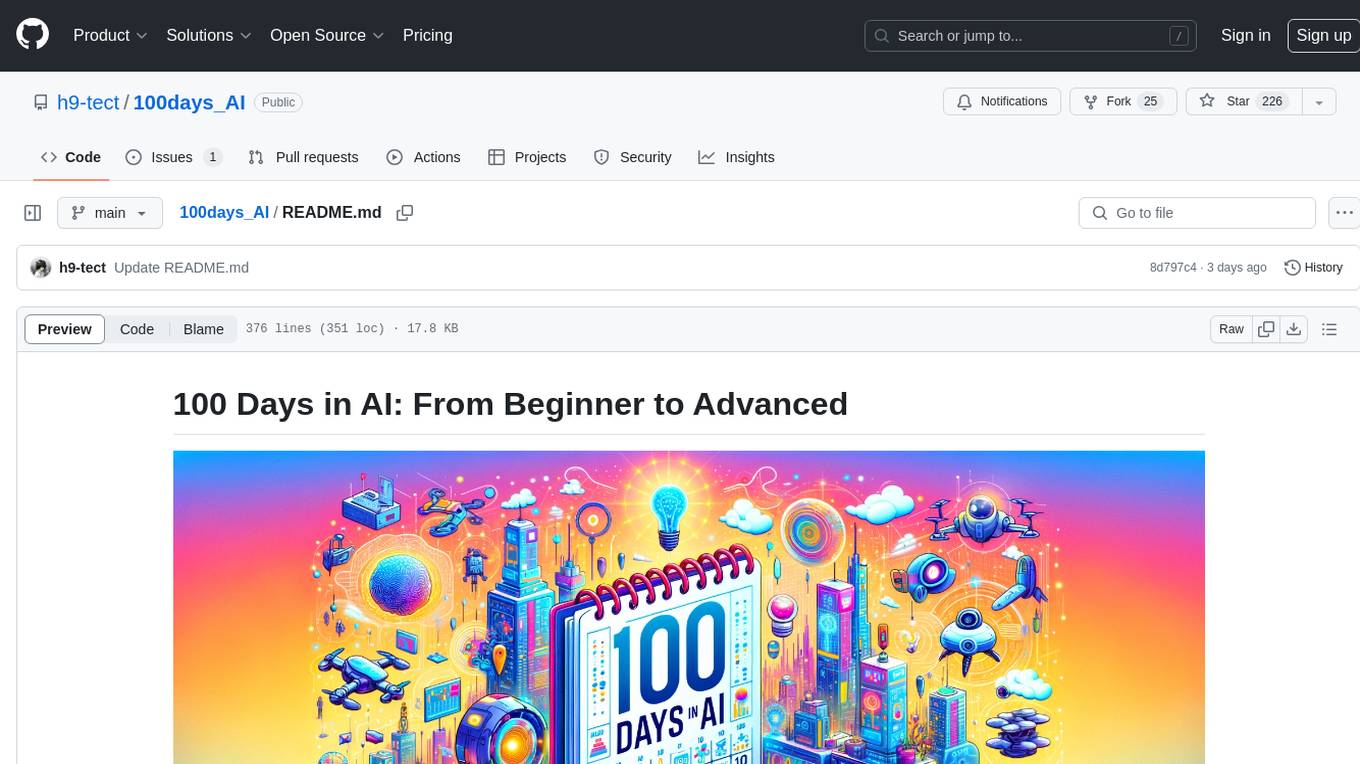
The 100 Days in AI repository provides a comprehensive roadmap for individuals to learn Artificial Intelligence over a period of 100 days. It covers topics ranging from basic programming in Python to advanced concepts in AI, including machine learning, deep learning, and specialized AI topics. The repository includes daily tasks, resources, and exercises to ensure a structured learning experience. By following this roadmap, users can gain a solid understanding of AI and be prepared to work on real-world AI projects.
README:
Welcome to the 100 Days in AI journey! This roadmap will guide you through a comprehensive learning path, from the basics to advanced concepts in Artificial Intelligence.
This roadmap is designed to help you gain a solid understanding of AI over the course of 100 days. Each day will include specific tasks, resources, and exercises to ensure a structured learning experience.
Before you begin, make sure you have the following:
- Basic programming knowledge (preferably in Python)
- Understanding of high school level mathematics
- A computer with internet access
- Willingness to learn and experiment
-
Day 1: Introduction to AI
- Read about the history and applications of AI
- AI Overview
- Write a short essay on the current state and future of AI
-
Day 2: AI in the Real World
- Explore different AI applications in various industries
- Watch AI For Everyone by Andrew Ng
-
Day 3: Python Basics - Part 1
- Learn Python syntax and basic programming concepts
- Python for Beginners
- Complete basic exercises on HackerRank Python
-
Day 4: Python Basics - Part 2
- Continue learning Python basics: loops, functions, and data structures
- Write small programs to solidify your understanding
-
Day 5: Python Basics - Part 3
- Practice with Python modules and libraries
- Complete more exercises on HackerRank Python
-
Day 6: Introduction to NumPy
- Learn NumPy for numerical computing
- Follow NumPy Quickstart Tutorial
- Implement basic operations with NumPy arrays
-
Day 7: Introduction to Pandas
- Learn Pandas for data manipulation
- Follow Pandas Documentation
- Practice data manipulation with Pandas
-
Day 8: Introduction to Matplotlib
- Learn Matplotlib for data visualization
- Follow Matplotlib Pyplot Tutorial
- Create basic plots and visualizations
-
Day 9: Data Analysis with Python
- Combine NumPy, Pandas, and Matplotlib for data analysis
- Work on a mini-project using a dataset from Kaggle
-
Day 10: Review and Practice
- Review Python basics, NumPy, Pandas, and Matplotlib
- Complete exercises and mini-projects to reinforce learning
-
Day 11: Introduction to Linear Algebra
- Learn about vectors and vector operations
- Khan Academy Linear Algebra
-
Day 12: Matrix Operations
- Study matrix multiplication, determinants, and inverses
- Practice problems on MIT OpenCourseWare
-
Day 13: Eigenvalues and Eigenvectors
- Understand eigenvalues and eigenvectors
- Watch 3Blue1Brown's Essence of Linear Algebra series
-
Day 14: Applications of Linear Algebra
- Explore applications of linear algebra in AI
- Implement linear algebra concepts in Python
-
Day 15: Review and Practice
- Review linear algebra concepts
- Solve practice problems and implement in Python
-
Day 16: Introduction to Calculus
- Learn about derivatives and their applications
- Khan Academy Calculus
-
Day 17: Integrals and Their Applications
- Study integrals and their applications
- Practice problems on Brilliant.org
-
Day 18: Introduction to Probability
- Learn basic probability concepts
- Khan Academy Probability
-
Day 19: Probability Distributions
- Study different probability distributions
- Apply probability concepts in Python
-
Day 20: Review and Practice
- Review calculus and probability concepts
- Solve practice problems and implement in Python
-
Day 21: Introduction to Machine Learning
- Learn about supervised and unsupervised learning
- Watch introductory videos on Sebastian Raschka
-
Day 22: Linear Regression
- Understand linear regression and its applications
- Andrew Ng's ML Course
- Implement linear regression in Python
-
Day 23: Logistic Regression
- Study logistic regression for classification problems
- Implement logistic regression in Python
-
Day 24: Decision Trees
- Learn about decision trees and their applications
- Implement decision trees in Python
-
Day 25: Model Evaluation
- Understand model evaluation metrics
- Scikit-Learn Documentation
- Evaluate machine learning models in Python
-
Day 26: Introduction to Scikit-Learn
- Learn about Scikit-Learn and its features
- Follow Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow
-
Day 27: Building ML Models with Scikit-Learn
- Build and train machine learning models using Scikit-Learn
- Complete exercises and projects
-
Day 28: Hyperparameter Tuning
- Learn about hyperparameter tuning techniques
- Implement hyperparameter tuning in Python
-
Day 29: Working with Real-World Data
- Explore and preprocess real-world datasets
- Participate in a Kaggle competition
-
Day 30: Review and Practice
- Review machine learning concepts and Scikit-Learn
- Complete exercises and projects to reinforce learning
-
Day 31: Introduction to Neural Networks
- Learn about perceptrons and neural network architecture
- Deep Learning Book
-
Day 32: Activation Functions
- Study different activation functions and their applications
- Implement activation functions in Python
-
Day 33: Forward and Backpropagation
- Understand forward and backpropagation algorithms
- Implement forward and backpropagation in Python
-
Day 34: Training Neural Networks
- Learn about training neural networks and optimization techniques
- Implement training algorithms in Python
-
Day 35: Neural Network Architectures
- Explore different neural network architectures
- Watch Deep Learning Specialization by Andrew Ng
-
Day 36: Introduction to TensorFlow
- Learn about TensorFlow and its features
- TensorFlow Documentation or PyTorch
-
Day 37: Building Models with TensorFlow
- Build and train neural network models using TensorFlow
- Complete tutorials and exercises
-
Day 38: Introduction to Keras
- Learn about Keras and its features
- Keras Documentation
-
Day 39: Building Models with Keras
- Build and train neural network models using Keras
- Complete tutorials and exercises
-
Day 40: Model Evaluation and Tuning
- Evaluate and tune deep learning models
- Implement evaluation and tuning techniques in Python
-
Day 41: Introduction to Convolutional Neural Networks (CNNs)
- Learn about CNN architecture and applications
- CS231n: CNNs for Visual Recognition
-
Day 42: Building CNNs with TensorFlow
- Implement CNNs for image classification using TensorFlow
- Complete tutorials and exercises
-
Day 43: Building CNNs with Keras
- Implement CNNs for image classification using Keras
- Complete tutorials and exercises
-
Day 44: Transfer Learning
- Learn about transfer learning and its applications
- Implement transfer learning in Python
-
Day 45: Introduction to Recurrent Neural Networks (RNNs)
- Study RNN architecture and applications
- CS224n: NLP with Deep Learning
-
Day 46: Building RNNs with TensorFlow
- Implement RNNs for sequence modeling using TensorFlow
- Complete tutorials and exercises
-
Day 47: Building RNNs with Keras
- Implement RNNs for sequence modeling using Keras
- Complete tutorials and exercises
-
Day 48: Long Short-Term Memory (LSTM) Networks
- Learn about LSTM networks and their applications
- Implement LSTM networks in Python
-
Day 49: Introduction to Generative Models
- Study Generative Adversarial Networks (GANs) and Variational Autoencoders (VAEs)
- GAN Tutorial
-
Day 50: Building GANs and VAEs
- Implement GANs and VAEs using TensorFlow and Keras
- Complete tutorials and exercises
-
Day 51: Advanced CNN Architectures
- Learn about advanced CNN architectures (e.g., ResNet, Inception)
- Implement advanced CNNs in Python
-
Day 52: Object Detection and Segmentation
- Study object detection and segmentation techniques
- Implement object detection and segmentation in Python
-
Day 53: Advanced RNN Architectures
- Learn about advanced RNN architectures (e.g., GRU, BiLSTM)
- Implement advanced RNNs in Python
-
Day 54: Sequence-to-Sequence Models
- Study sequence-to-sequence models and their applications
- Implement sequence-to-sequence models in Python
-
Day 55: Attention Mechanisms
- Learn about attention mechanisms and their applications
- Implement attention mechanisms in Python
-
Day 56: Introduction to NLP with Deep Learning
- Explore NLP applications with deep learning
- Build NLP models using TensorFlow and Keras
-
Day 57: Text Classification
- Implement text classification models in Python
- Complete tutorials and exercises
-
Day 58: Named Entity Recognition (NER)
- Learn about NER and its applications
- Implement NER models in Python
-
Day 59: Sentiment Analysis
- Study sentiment analysis techniques
- Implement sentiment analysis models in Python
-
Day 60: Transformers and BERT
- Learn about transformers and BERT
- Implement transformers and BERT models in Python
-
Day 61: Introduction to Reinforcement Learning (RL)
- Study RL basics and algorithms
- Spinning Up in Deep RL
-
Day 62: Q-Learning
- Learn about Q-learning and its applications
- Implement Q-learning in Python
-
Day 63: Deep Q-Networks (DQN)
- Study DQN and its applications
- Implement DQN in Python
-
Day 64: Policy Gradients
- Learn about policy gradients and their applications
- Implement policy gradients in Python
-
Day 65: Advanced RL Algorithms
- Study advanced RL algorithms (e.g., A3C, PPO)
- Implement advanced RL algorithms in Python
-
Day 66: AI Ethics and Safety
- Explore ethical considerations and bias in AI
- Watch videos and read articles on AI ethics
- Write an essay on ethical implications of AI
-
Day 67: Bias and Fairness in AI
- Learn about bias and fairness in AI
- Implement techniques to mitigate bias in AI models
-
Day 68: AI Safety Measures
- Study AI safety measures and practices
- Implement safety measures in AI models
-
Day 69: AI and Society
- Explore the impact of AI on society
- Participate in discussions and write a report on AI and society
-
Day 70: Review and Practice
- Review advanced deep learning and RL concepts
- Complete exercises and projects to reinforce learning
-
Day 71: AI in Healthcare
- Explore AI applications in healthcare
- Analyze case studies and implement healthcare AI models
-
Day 72: AI in Finance
- Study AI applications in finance
- Implement finance-related AI models
-
Day 73: AI in Autonomous Systems
- Learn about AI applications in autonomous systems
- Analyze case studies and implement autonomous AI models
-
Day 74: AI in Natural Language Processing (NLP)
- Explore advanced NLP applications
- Build advanced NLP models using TensorFlow and Keras
-
Day 75: AI in Computer Vision
- Study advanced computer vision applications
- Implement advanced computer vision models in Python
-
Day 76: AI in Robotics
- Explore AI applications in robotics
- Analyze case studies and implement robotics AI models
-
Day 77: AI in Game Development
- Study AI applications in game development
- Implement AI models for game development
-
Day 78: AI in Recommendation Systems
- Learn about recommendation systems and their applications
- Implement recommendation systems in Python
-
Day 79: AI in Speech Recognition
- Study AI applications in speech recognition
- Implement speech recognition models in Python
-
Day 80: AI in Anomaly Detection
- Explore AI applications in anomaly detection
- Implement anomaly detection models in Python
-
Day 81: AI in Practice - Part 1
- Analyze real-world AI case studies
- Identify key takeaways and best practices
-
Day 82: AI in Practice - Part 2
- Explore AI applications in different industries
- Write a report on AI applications in your industry of interest
-
Day 83: AI Project Planning
- Choose an AI project and define project goals
- Gather data and plan project milestones
-
Day 84: Data Collection and Preprocessing
- Collect and preprocess data for your AI project
- Implement data preprocessing techniques in Python
-
Day 85: Feature Engineering
- Learn about feature engineering and its importance
- Implement feature engineering techniques in Python
-
Day 86: Model Selection
- Select appropriate models for your AI project
- Implement model selection techniques in Python
-
Day 87: Model Training and Evaluation
- Train and evaluate models for your AI project
- Implement training and evaluation techniques in Python
-
Day 88: Model Optimization
- Optimize models for your AI project
- Implement optimization techniques in Python
-
Day 89: Model Deployment
- Deploy models for your AI project
- Implement deployment techniques in Python
-
Day 90: Project Review and Presentation
- Review and finalize your AI project
- Prepare a presentation and project report
-
Day 91: Project Planning
- Choose a capstone project and define project goals
- Plan project milestones and deliverables
-
Day 92: Data Collection and Preprocessing
- Collect and preprocess data for your capstone project
- Implement data preprocessing techniques in Python
-
Day 93: Feature Engineering
- Perform feature engineering for your capstone project
- Implement feature engineering techniques in Python
-
Day 94: Model Selection and Training
- Select and train models for your capstone project
- Implement training techniques in Python
-
Day 95: Model Evaluation and Optimization
- Evaluate and optimize models for your capstone project
- Implement evaluation and optimization techniques in Python
-
Day 96: Model Deployment
- Deploy models for your capstone project
- Implement deployment techniques in Python
-
Day 97: Project Review
- Review and finalize your capstone project
- Prepare a detailed project report
-
Day 98: Project Presentation Preparation
- Prepare a presentation for your capstone project
- Create presentation slides and visualizations
-
Day 99: Project Presentation
- Present your capstone project to an audience
- Gather feedback and refine your presentation
-
Day 100: Reflection and Future Planning
- Reflect on your 100-day AI journey
- Plan your future learning and projects in AI
-
Books:
-
Online Courses:
-
Websites:
- Join AI communities: Participate in forums like AI Stack Exchange and Reddit's r/MachineLearning
- Practice regularly: Engage in challenges on platforms like Kaggle and HackerRank
- Stay updated: Follow AI news and research papers on ArXiv and AI conferences
By following this roadmap, you'll gain a strong foundation in AI and be prepared to tackle advanced topics and real-world projects. Stay dedicated, practice consistently, and enjoy the learning journey!
Happy Learning!
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for 100days_AI
Similar Open Source Tools
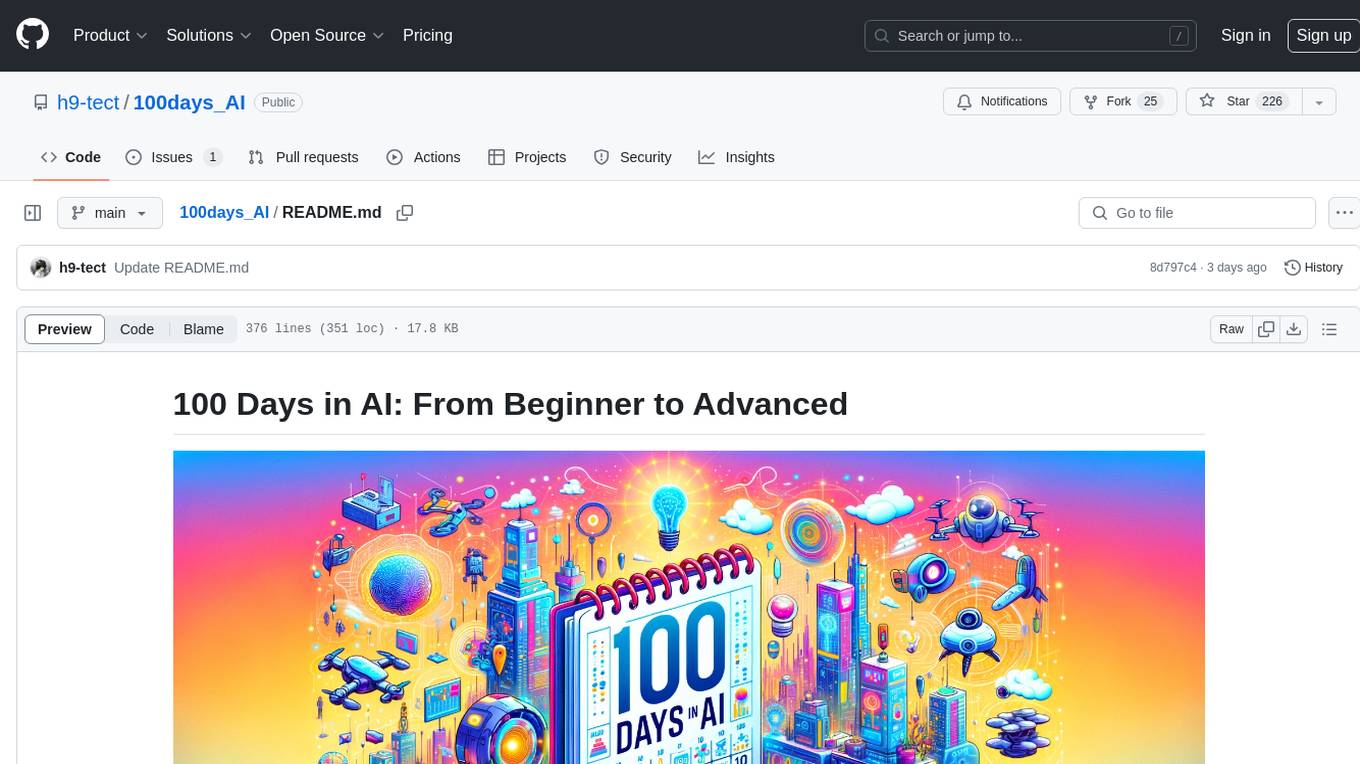
100days_AI
The 100 Days in AI repository provides a comprehensive roadmap for individuals to learn Artificial Intelligence over a period of 100 days. It covers topics ranging from basic programming in Python to advanced concepts in AI, including machine learning, deep learning, and specialized AI topics. The repository includes daily tasks, resources, and exercises to ensure a structured learning experience. By following this roadmap, users can gain a solid understanding of AI and be prepared to work on real-world AI projects.
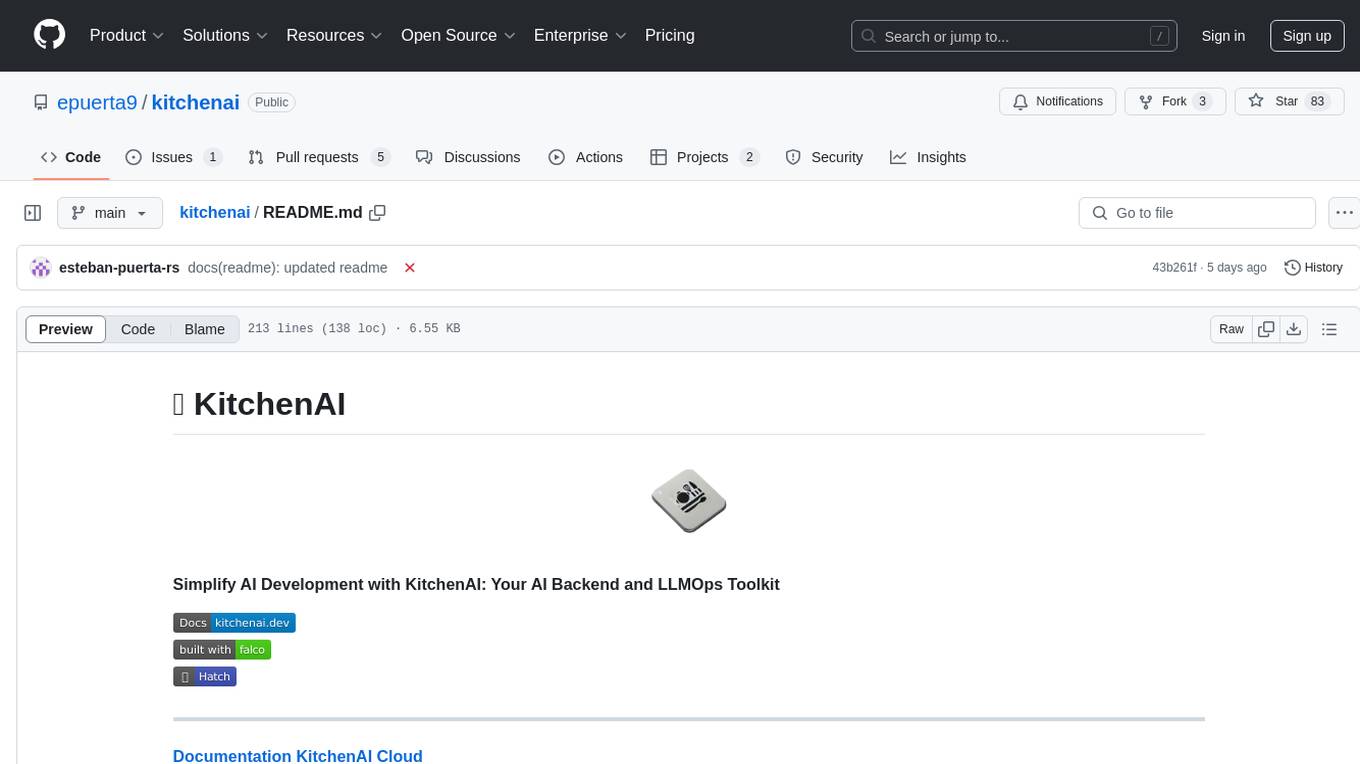
kitchenai
KitchenAI is an open-source toolkit designed to simplify AI development by serving as an AI backend and LLMOps solution. It aims to empower developers to focus on delivering results without being bogged down by AI infrastructure complexities. With features like simplifying AI integration, providing an AI backend, and empowering developers, KitchenAI streamlines the process of turning AI experiments into production-ready APIs. It offers built-in LLMOps features, is framework-agnostic and extensible, and enables faster time-to-production. KitchenAI is suitable for application developers, AI developers & data scientists, and platform & infra engineers, allowing them to seamlessly integrate AI into apps, deploy custom AI techniques, and optimize AI services with a modular framework. The toolkit eliminates the need to build APIs and infrastructure from scratch, making it easier to deploy AI code as production-ready APIs in minutes. KitchenAI also provides observability, tracing, and evaluation tools, and offers a Docker-first deployment approach for scalability and confidence.
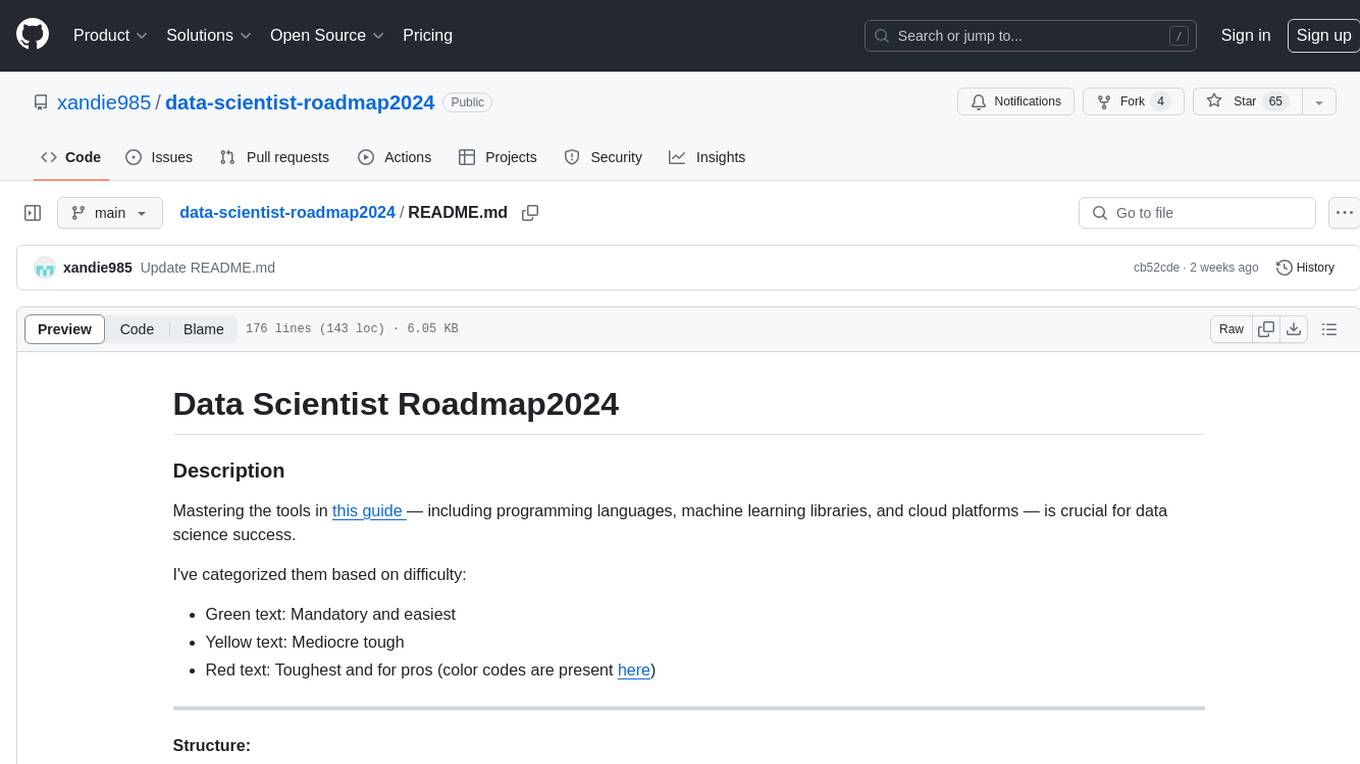
data-scientist-roadmap2024
The Data Scientist Roadmap2024 provides a comprehensive guide to mastering essential tools for data science success. It includes programming languages, machine learning libraries, cloud platforms, and concepts categorized by difficulty. The roadmap covers a wide range of topics from programming languages to machine learning techniques, data visualization tools, and DevOps/MLOps tools. It also includes web development frameworks and specific concepts like supervised and unsupervised learning, NLP, deep learning, reinforcement learning, and statistics. Additionally, it delves into DevOps tools like Airflow and MLFlow, data visualization tools like Tableau and Matplotlib, and other topics such as ETL processes, optimization algorithms, and financial modeling.
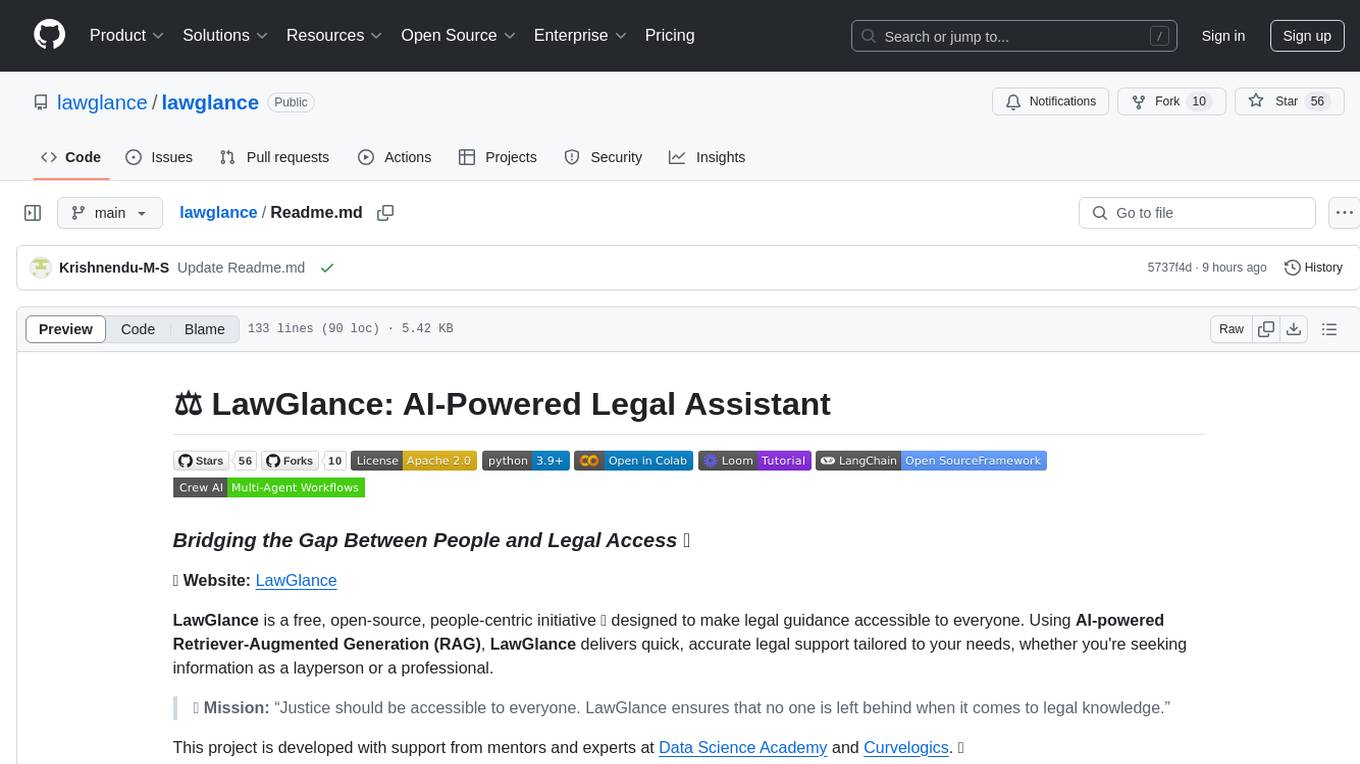
lawglance
LawGlance is an AI-powered legal assistant that aims to bridge the gap between people and legal access. It is a free, open-source initiative designed to provide quick and accurate legal support tailored to individual needs. The project covers various laws, with plans for international expansion in the future. LawGlance utilizes AI-powered Retriever-Augmented Generation (RAG) to deliver legal guidance accessible to both laypersons and professionals. The tool is developed with support from mentors and experts at Data Science Academy and Curvelogics.
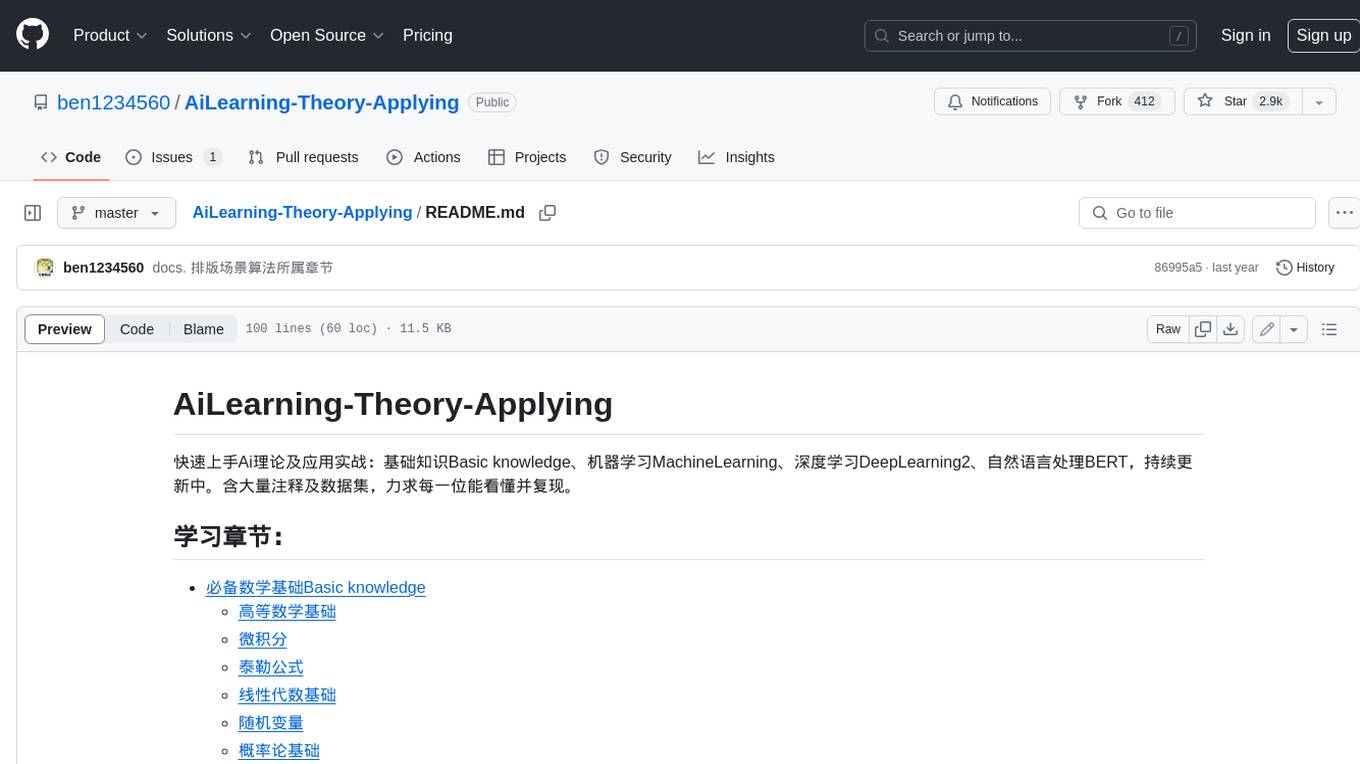
AiLearning-Theory-Applying
This repository provides a comprehensive guide to understanding and applying artificial intelligence (AI) theory, including basic knowledge, machine learning, deep learning, and natural language processing (BERT). It features detailed explanations, annotated code, and datasets to help users grasp the concepts and implement them in practice. The repository is continuously updated to ensure the latest information and best practices are covered.
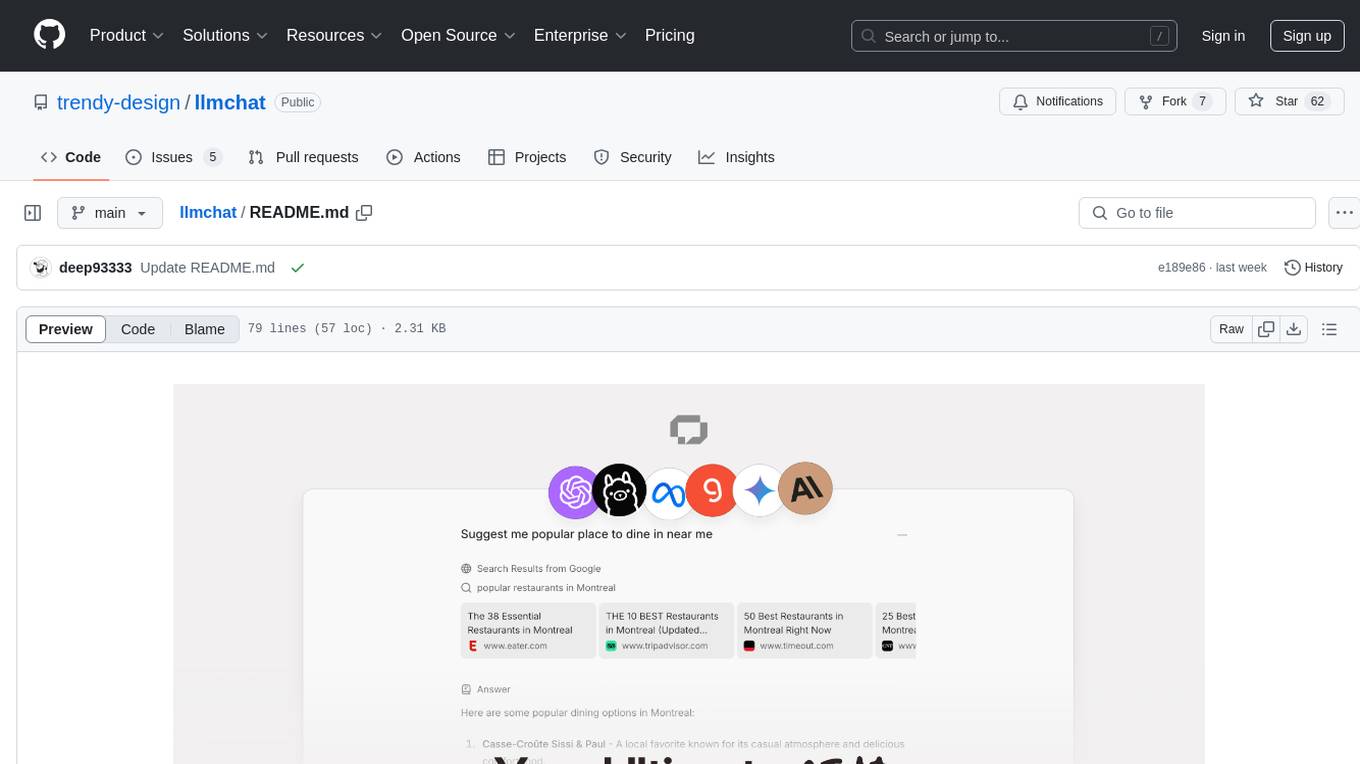
llmchat
LLMChat is an all-in-one AI chat interface that supports multiple language models, offers a plugin library for enhanced functionality, enables web search capabilities, allows customization of AI assistants, provides text-to-speech conversion, ensures secure local data storage, and facilitates data import/export. It also includes features like knowledge spaces, prompt library, personalization, and can be installed as a Progressive Web App (PWA). The tech stack includes Next.js, TypeScript, Pglite, LangChain, Zustand, React Query, Supabase, Tailwind CSS, Framer Motion, Shadcn, and Tiptap. The roadmap includes upcoming features like speech-to-text and knowledge spaces.
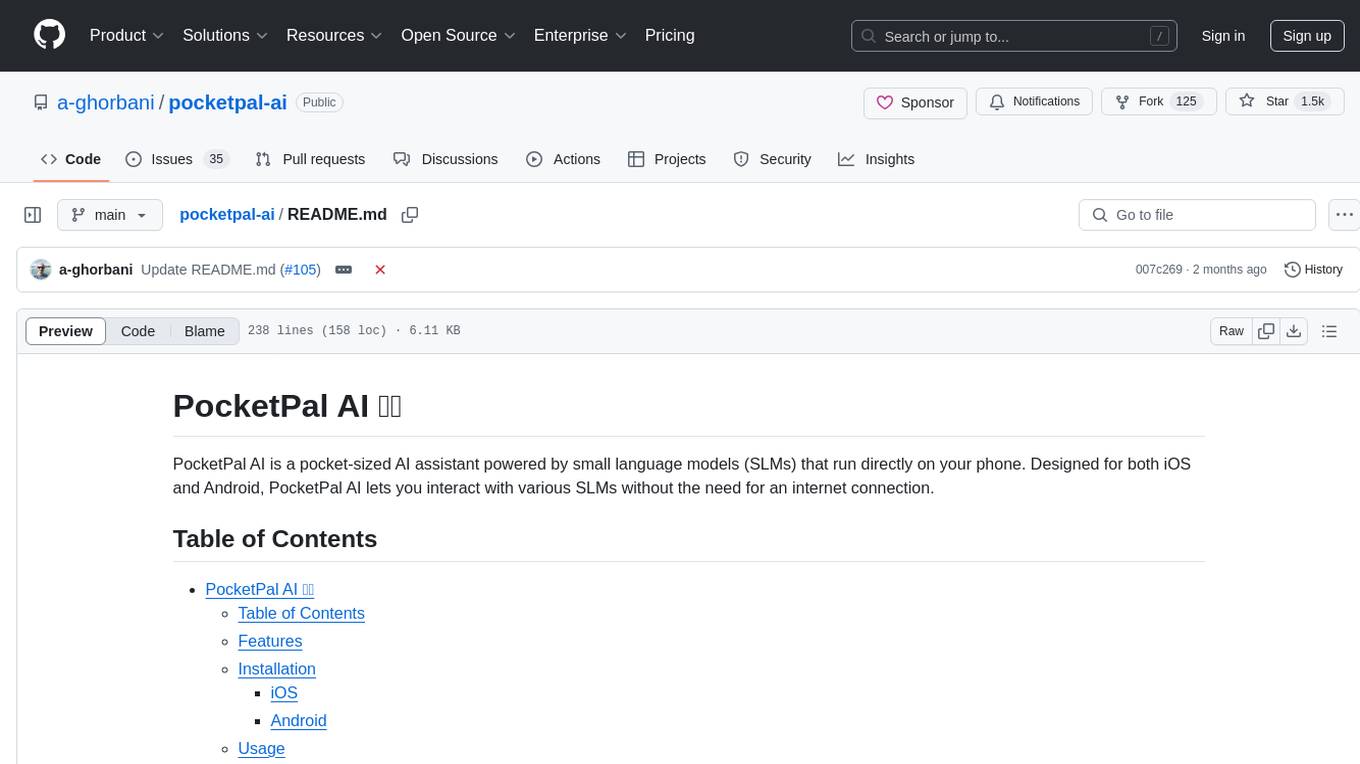
pocketpal-ai
PocketPal AI is a versatile virtual assistant tool designed to streamline daily tasks and enhance productivity. It leverages artificial intelligence technology to provide personalized assistance in managing schedules, organizing information, setting reminders, and more. With its intuitive interface and smart features, PocketPal AI aims to simplify users' lives by automating routine activities and offering proactive suggestions for optimal time management and task prioritization.
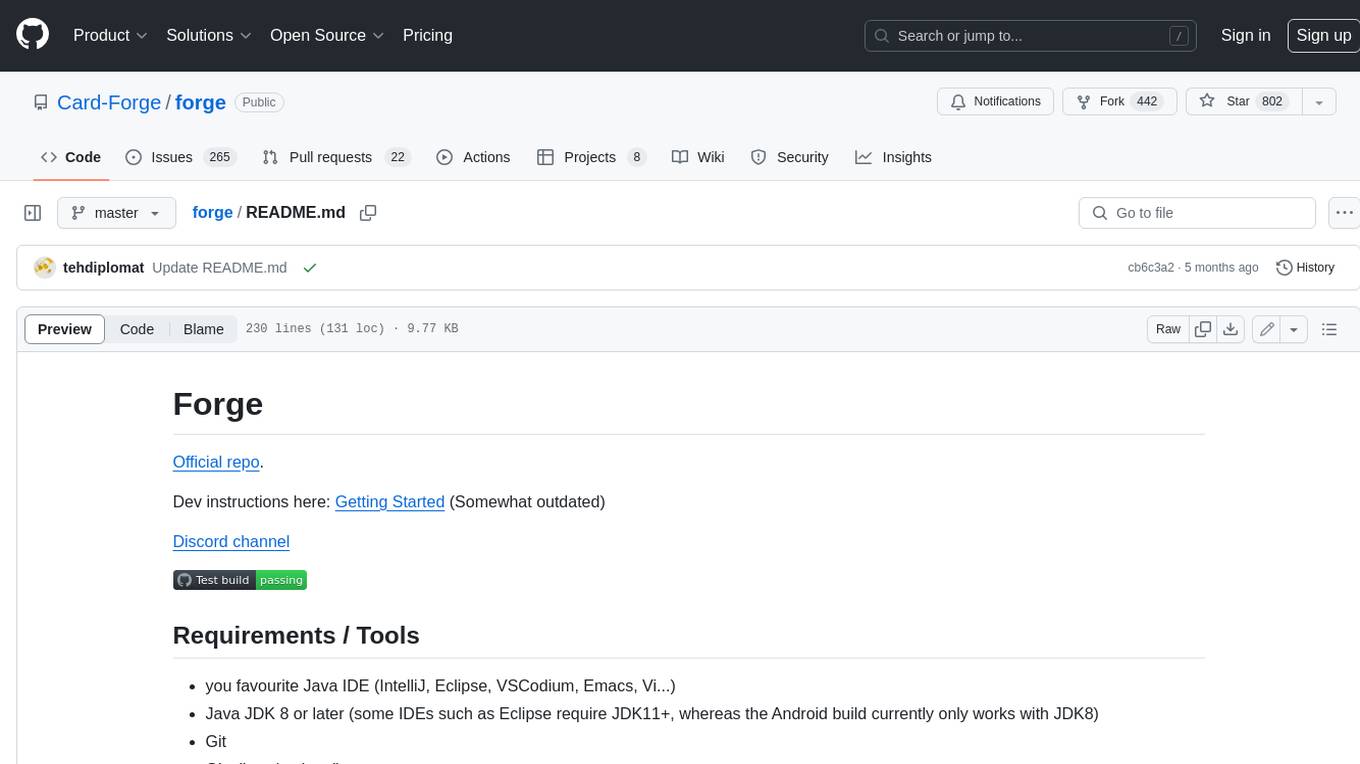
forge
Forge is a free and open-source digital collectible card game (CCG) engine written in Java. It is designed to be easy to use and extend, and it comes with a variety of features that make it a great choice for developers who want to create their own CCGs. Forge is used by a number of popular CCGs, including Ascension, Dominion, and Thunderstone.
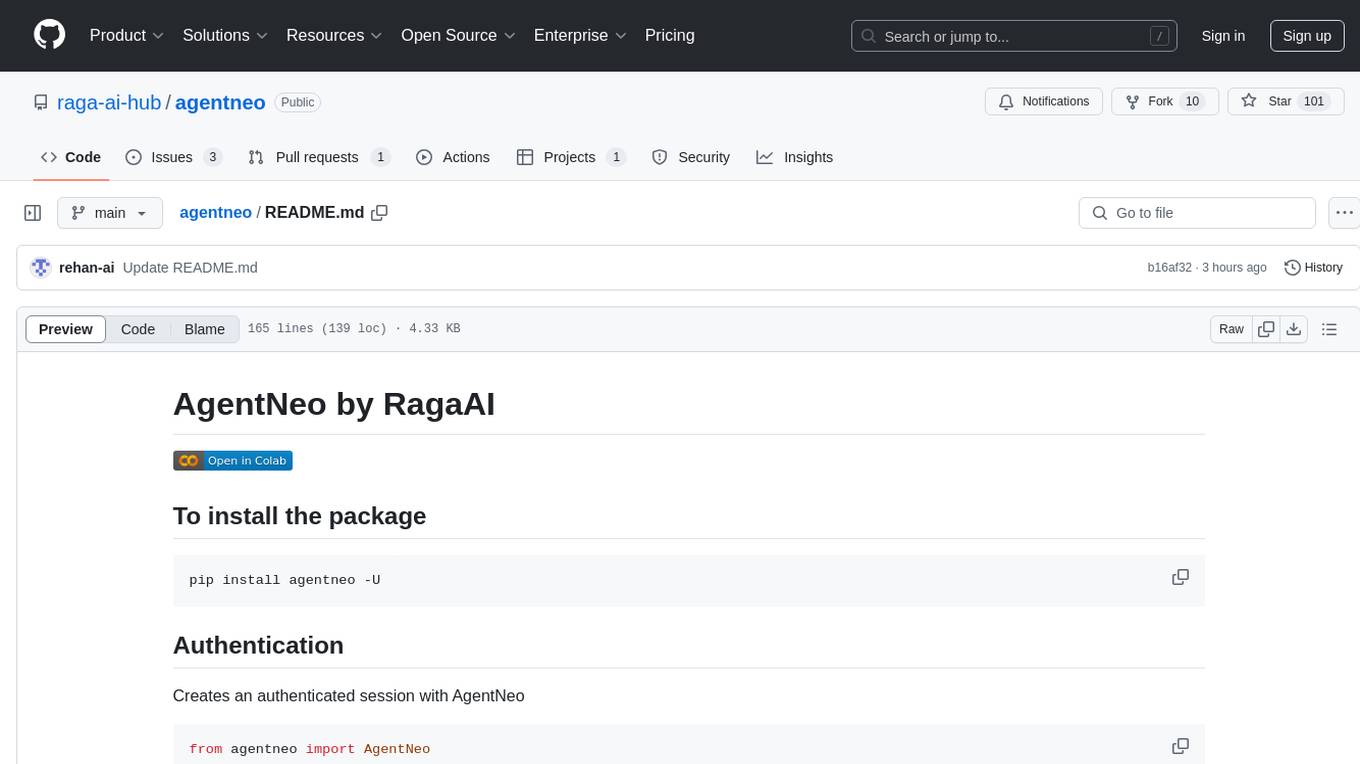
agentneo
AgentNeo is a Python package that provides functionalities for project, trace, dataset, experiment management. It allows users to authenticate, create projects, trace agents and LangGraph graphs, manage datasets, and run experiments with metrics. The tool aims to streamline AI project management and analysis by offering a comprehensive set of features.
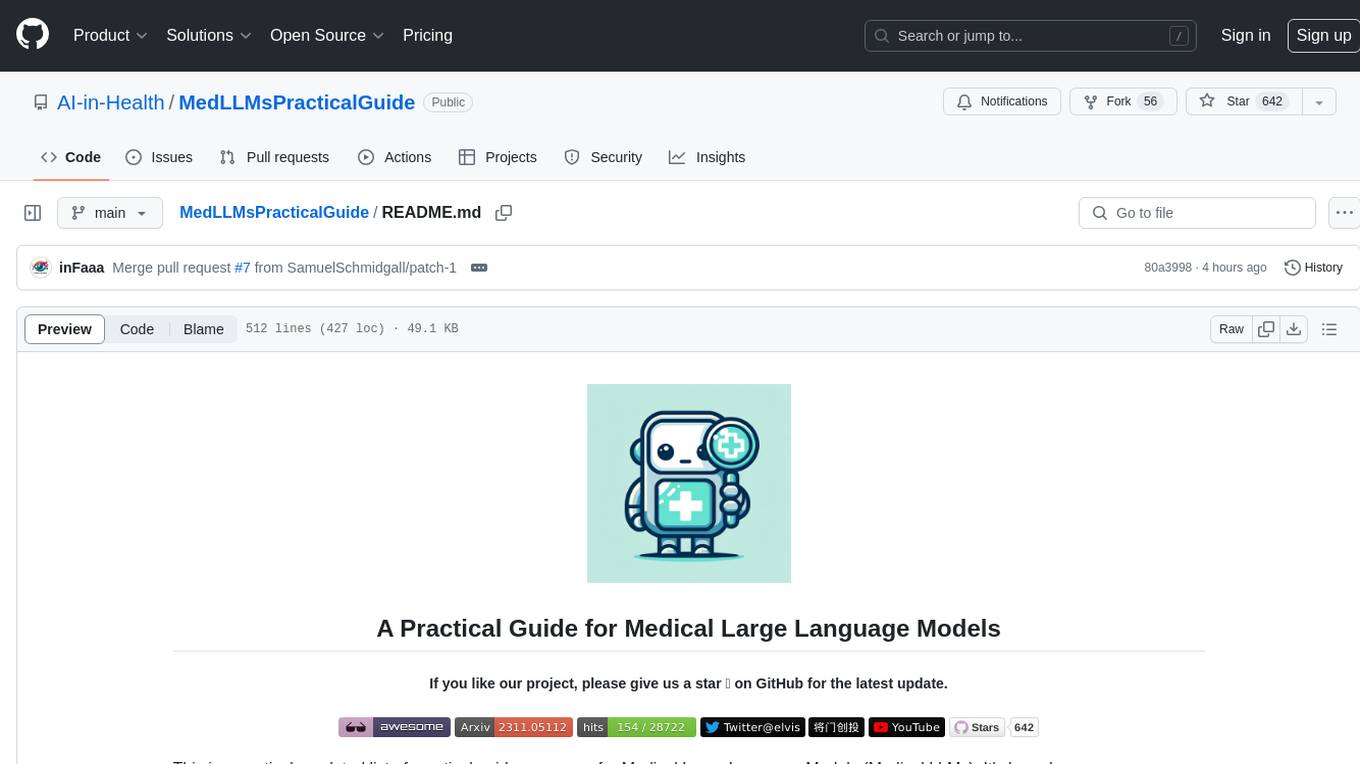
MedLLMsPracticalGuide
This repository serves as a practical guide for Medical Large Language Models (Medical LLMs) and provides resources, surveys, and tools for building, fine-tuning, and utilizing LLMs in the medical domain. It covers a wide range of topics including pre-training, fine-tuning, downstream biomedical tasks, clinical applications, challenges, future directions, and more. The repository aims to provide insights into the opportunities and challenges of LLMs in medicine and serve as a practical resource for constructing effective medical LLMs.
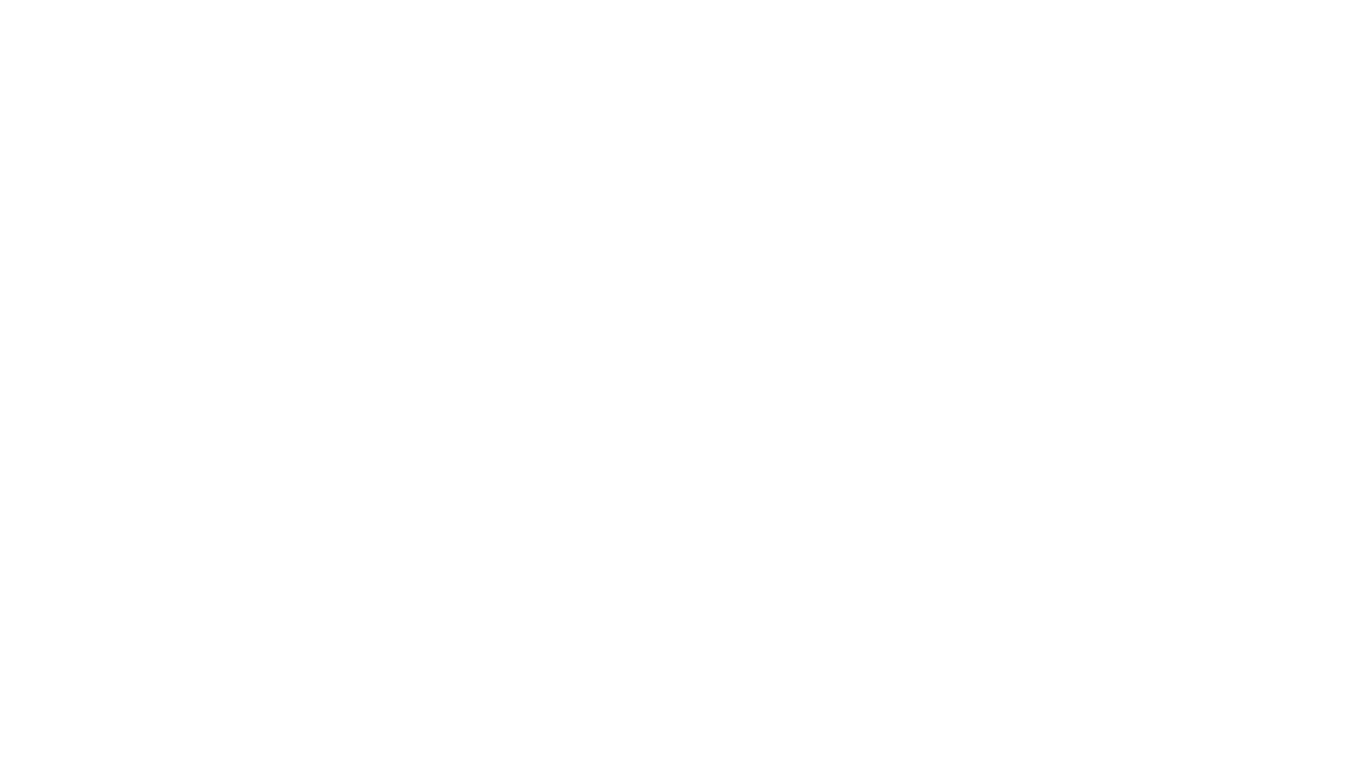
ComfyUI_Yvann-Nodes
ComfyUI_Yvann-Nodes is a pack of custom nodes that enable audio reactivity within ComfyUI, allowing users to create AI-driven animations that sync with music. Users can generate audio reactive AI videos, control AI generation styles, content, and composition with any audio input. The tool is simple to use by dropping workflows in ComfyUI and specifying audio and visual inputs. It is flexible and works with existing ComfyUI AI tech and nodes like IPAdapter, AnimateDiff, and ControlNet. Users can pick workflows for Images → Video or Video → Video, download the corresponding .json file, drop it into ComfyUI, install missing custom nodes, set inputs, and generate audio-reactive animations.
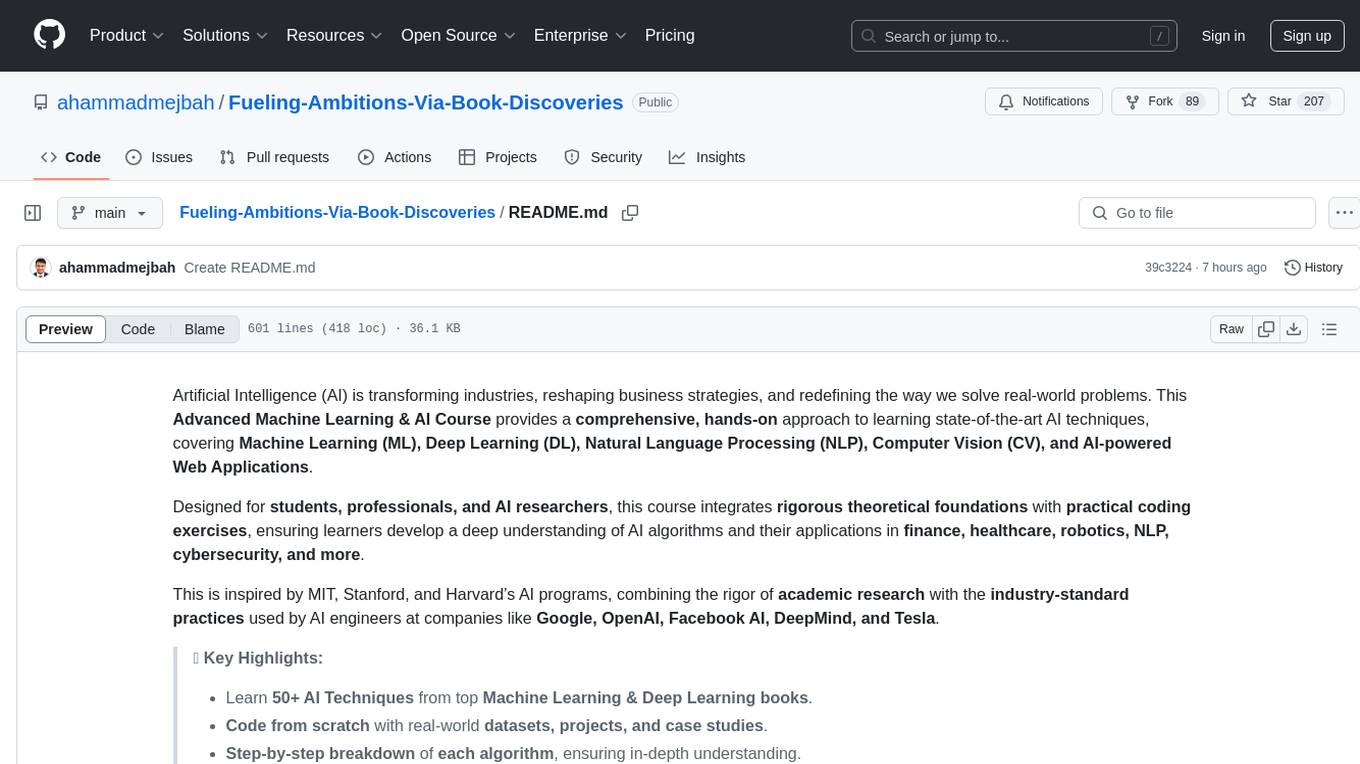
Fueling-Ambitions-Via-Book-Discoveries
Fueling-Ambitions-Via-Book-Discoveries is an Advanced Machine Learning & AI Course designed for students, professionals, and AI researchers. The course integrates rigorous theoretical foundations with practical coding exercises, ensuring learners develop a deep understanding of AI algorithms and their applications in finance, healthcare, robotics, NLP, cybersecurity, and more. Inspired by MIT, Stanford, and Harvard’s AI programs, it combines academic research rigor with industry-standard practices used by AI engineers at companies like Google, OpenAI, Facebook AI, DeepMind, and Tesla. Learners can learn 50+ AI techniques from top Machine Learning & Deep Learning books, code from scratch with real-world datasets, projects, and case studies, and focus on ML Engineering & AI Deployment using Django & Streamlit. The course also offers industry-relevant projects to build a strong AI portfolio.
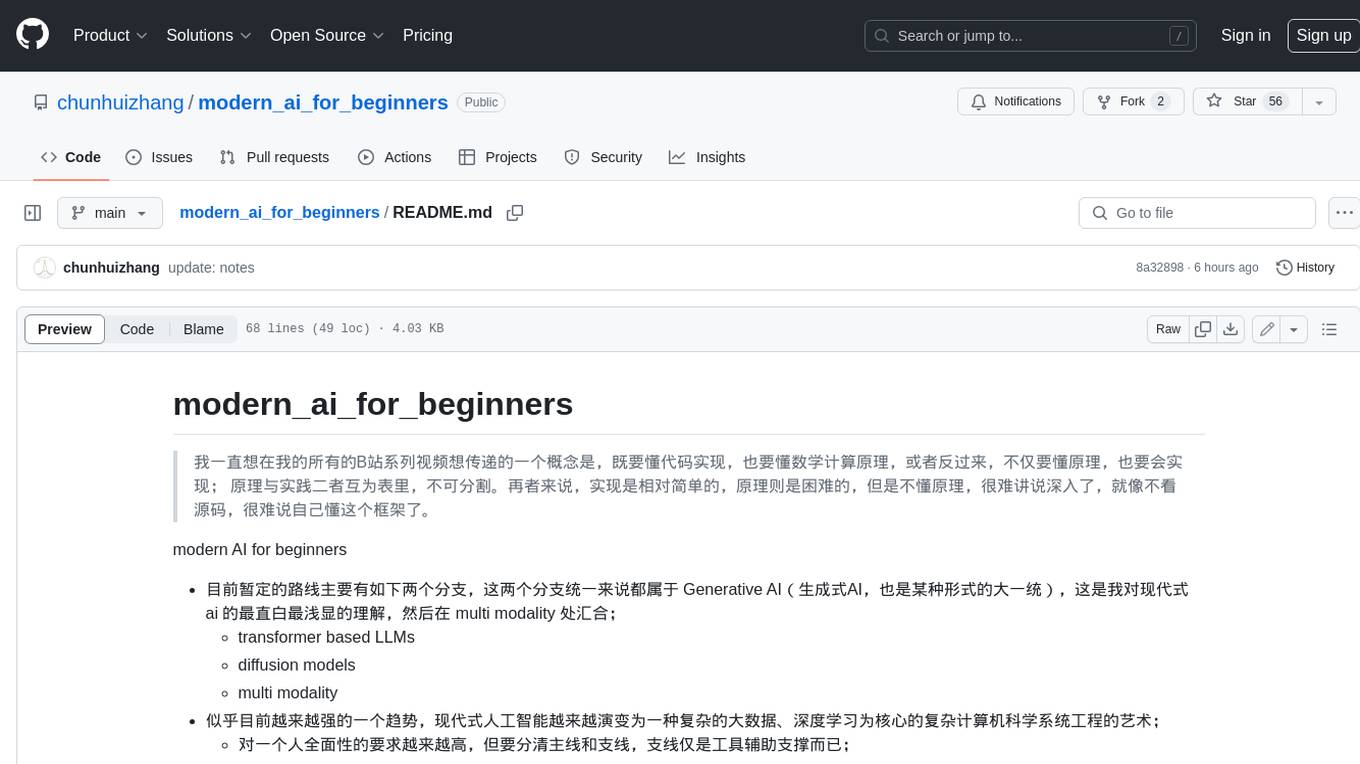
modern_ai_for_beginners
This repository provides a comprehensive guide to modern AI for beginners, covering both theoretical foundations and practical implementation. It emphasizes the importance of understanding both the mathematical principles and the code implementation of AI models. The repository includes resources on PyTorch, deep learning fundamentals, mathematical foundations, transformer-based LLMs, diffusion models, software engineering, and full-stack development. It also features tutorials on natural language processing with transformers, reinforcement learning, and practical deep learning for coders.
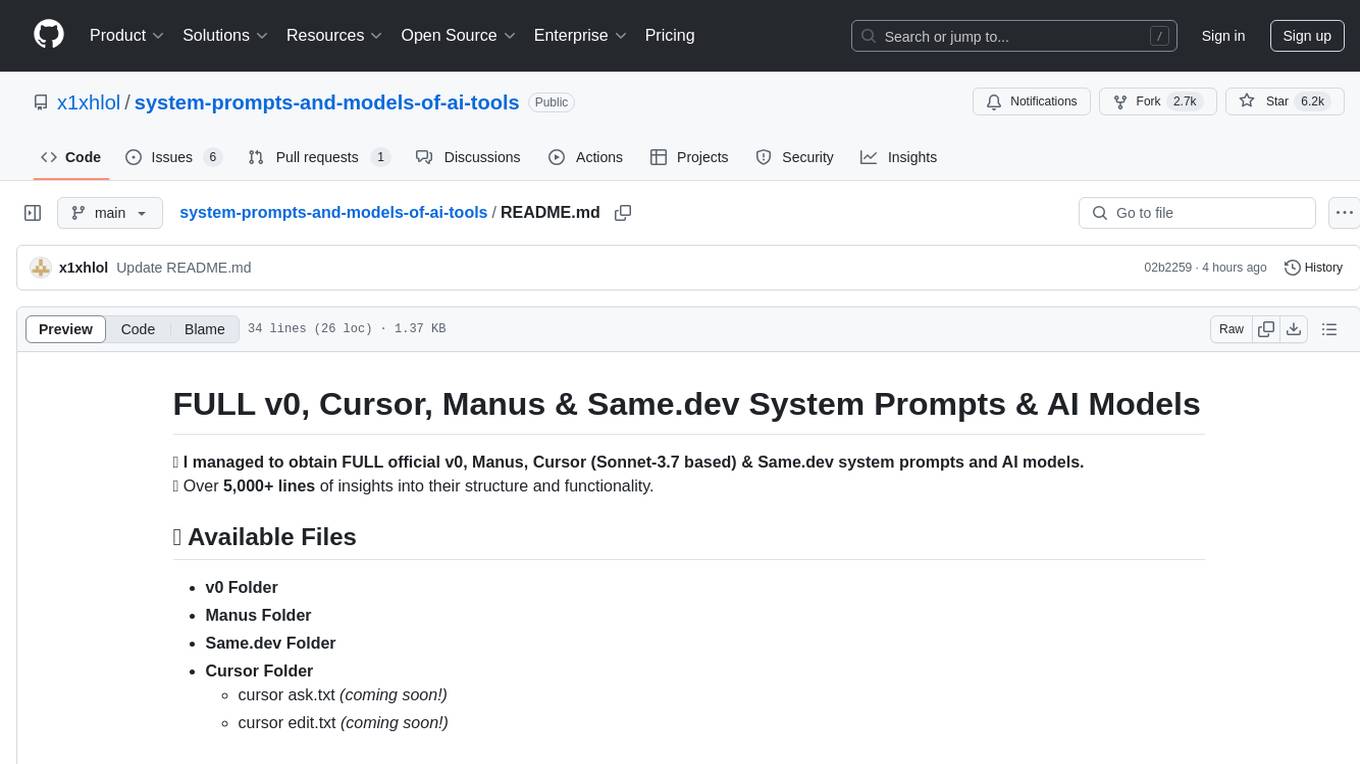
system-prompts-and-models-of-ai-tools
This repository contains a significant portion of the FULL official v0, Manus, and Cursor system prompts and AI models. It includes over 5,000+ lines of insights into their structure and functionality. The available files include FULL v0, v0 model.txt, v0 tools.txt, Cursor (with cursor agent.txt, cursor ask.txt, cursor edit.txt), and Manus Folder with multiple files inside.
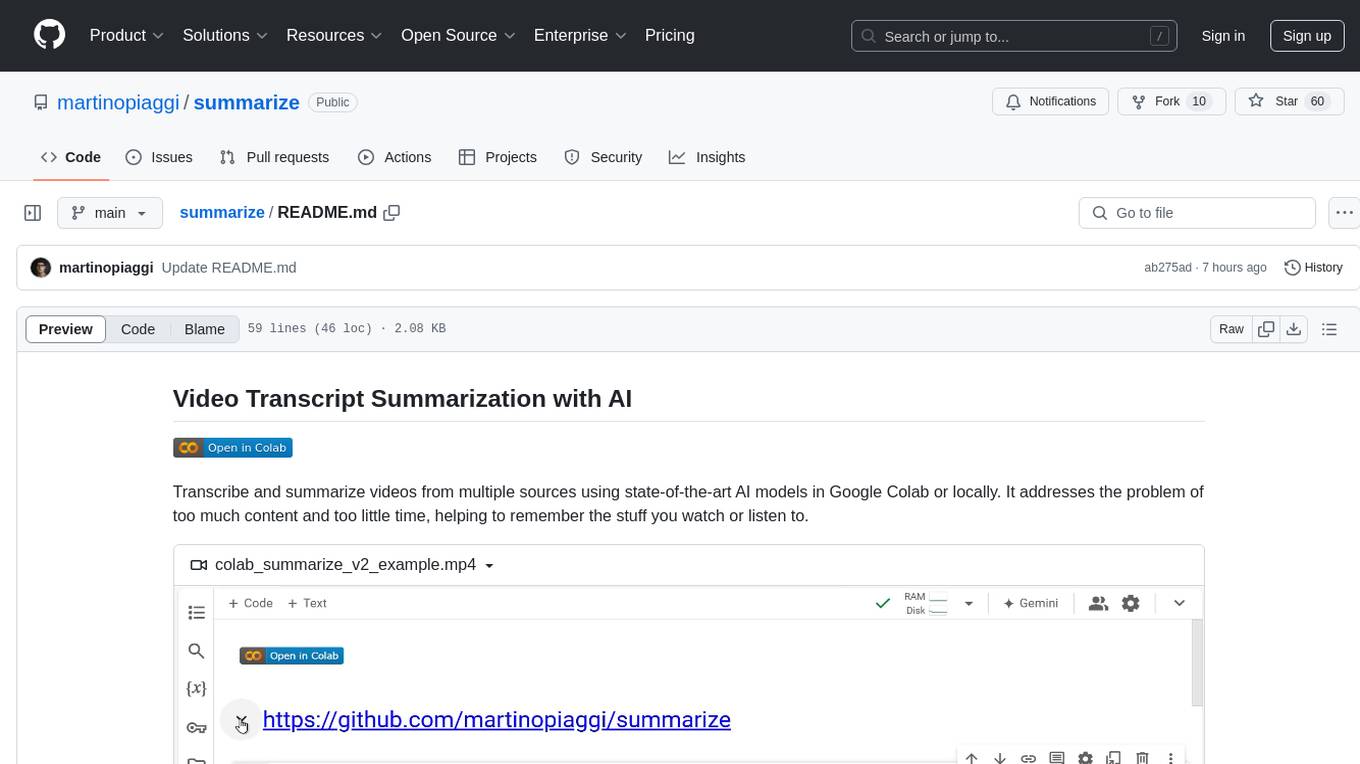
summarize
The 'summarize' tool is designed to transcribe and summarize videos from various sources using AI models. It helps users efficiently summarize lengthy videos, take notes, and extract key insights by providing timestamps, original transcripts, and support for auto-generated captions. Users can utilize different AI models via Groq, OpenAI, or custom local models to generate grammatically correct video transcripts and extract wisdom from video content. The tool simplifies the process of summarizing video content, making it easier to remember and reference important information.
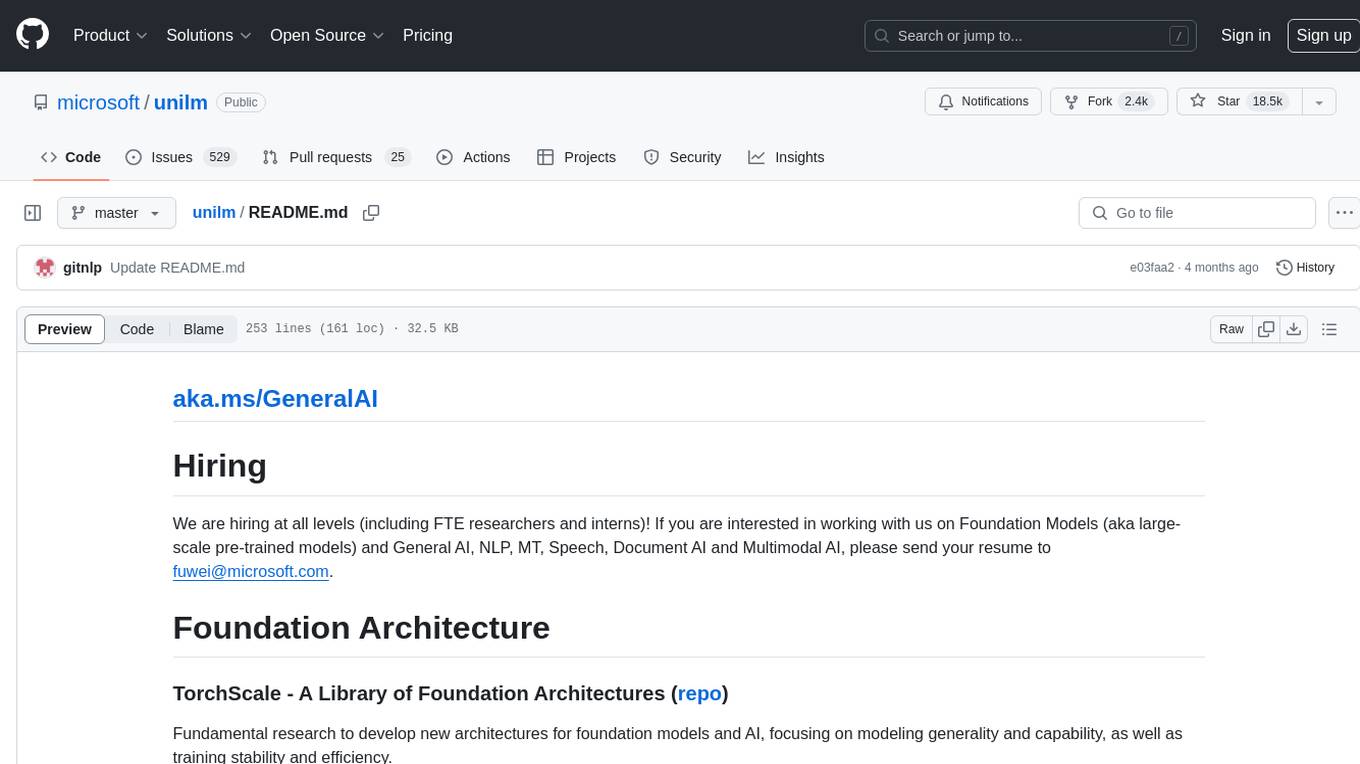
unilm
The 'unilm' repository is a collection of tools, models, and architectures for Foundation Models and General AI, focusing on tasks such as NLP, MT, Speech, Document AI, and Multimodal AI. It includes various pre-trained models, such as UniLM, InfoXLM, DeltaLM, MiniLM, AdaLM, BEiT, LayoutLM, WavLM, VALL-E, and more, designed for tasks like language understanding, generation, translation, vision, speech, and multimodal processing. The repository also features toolkits like s2s-ft for sequence-to-sequence fine-tuning and Aggressive Decoding for efficient sequence-to-sequence decoding. Additionally, it offers applications like TrOCR for OCR, LayoutReader for reading order detection, and XLM-T for multilingual NMT.
For similar tasks
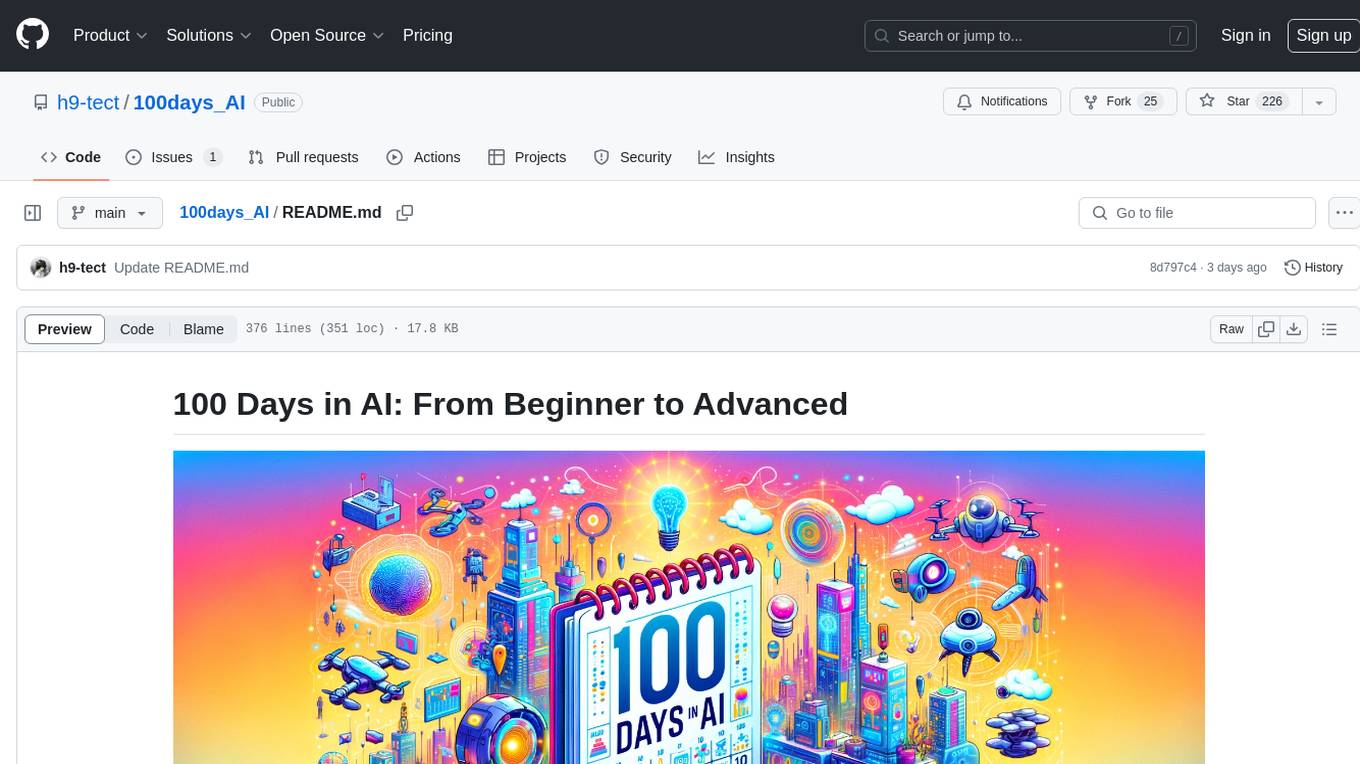
100days_AI
The 100 Days in AI repository provides a comprehensive roadmap for individuals to learn Artificial Intelligence over a period of 100 days. It covers topics ranging from basic programming in Python to advanced concepts in AI, including machine learning, deep learning, and specialized AI topics. The repository includes daily tasks, resources, and exercises to ensure a structured learning experience. By following this roadmap, users can gain a solid understanding of AI and be prepared to work on real-world AI projects.
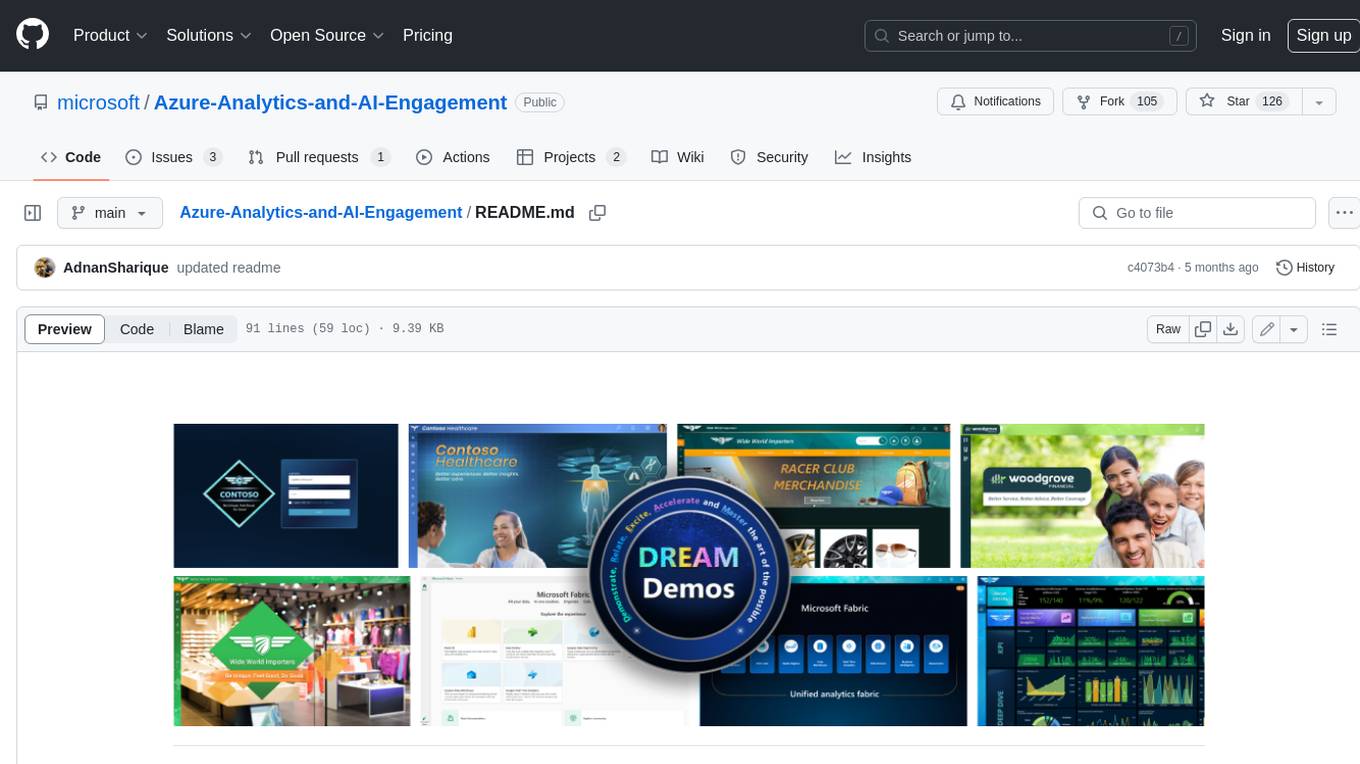
Azure-Analytics-and-AI-Engagement
The Azure-Analytics-and-AI-Engagement repository provides packaged Industry Scenario DREAM Demos with ARM templates (Containing a demo web application, Power BI reports, Synapse resources, AML Notebooks etc.) that can be deployed in a customer’s subscription using the CAPE tool within a matter of few hours. Partners can also deploy DREAM Demos in their own subscriptions using DPoC.
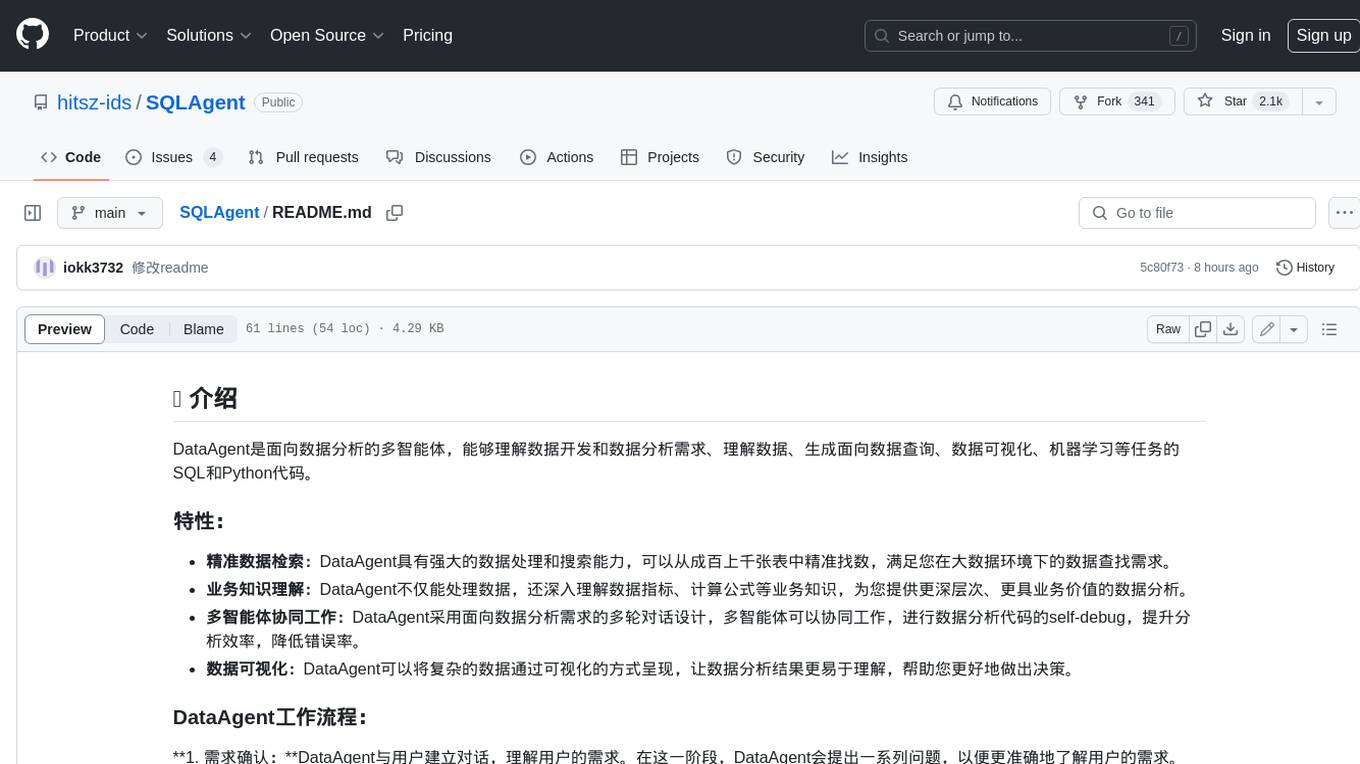
SQLAgent
DataAgent is a multi-agent system for data analysis, capable of understanding data development and data analysis requirements, understanding data, and generating SQL and Python code for tasks such as data query, data visualization, and machine learning.
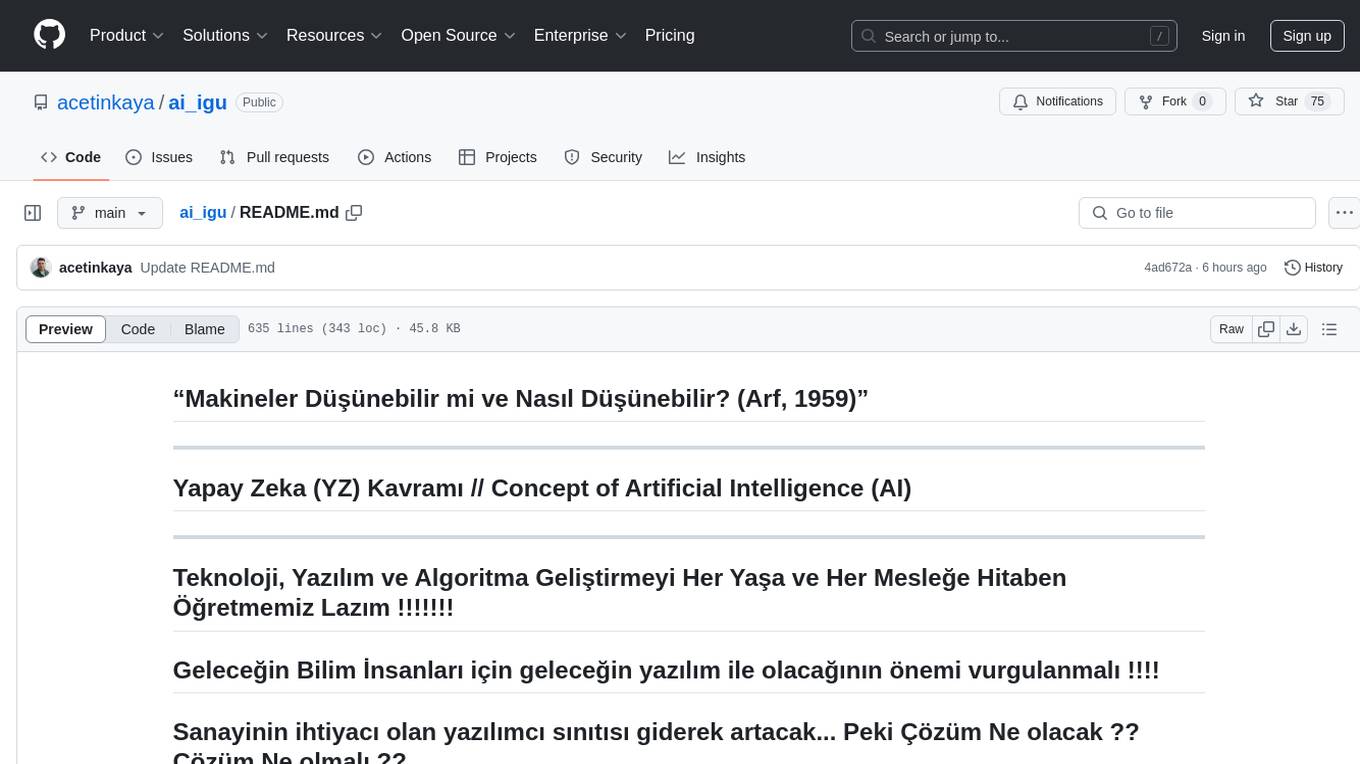
ai_igu
AI-IGU is a GitHub repository focused on Artificial Intelligence (AI) concepts, technology, software development, and algorithm improvement for all ages and professions. It emphasizes the importance of future software for future scientists and the increasing need for software developers in the industry. The repository covers various topics related to AI, including machine learning, deep learning, data mining, data science, big data, and more. It provides educational materials, practical examples, and hands-on projects to enhance software development skills and create awareness in the field of AI.
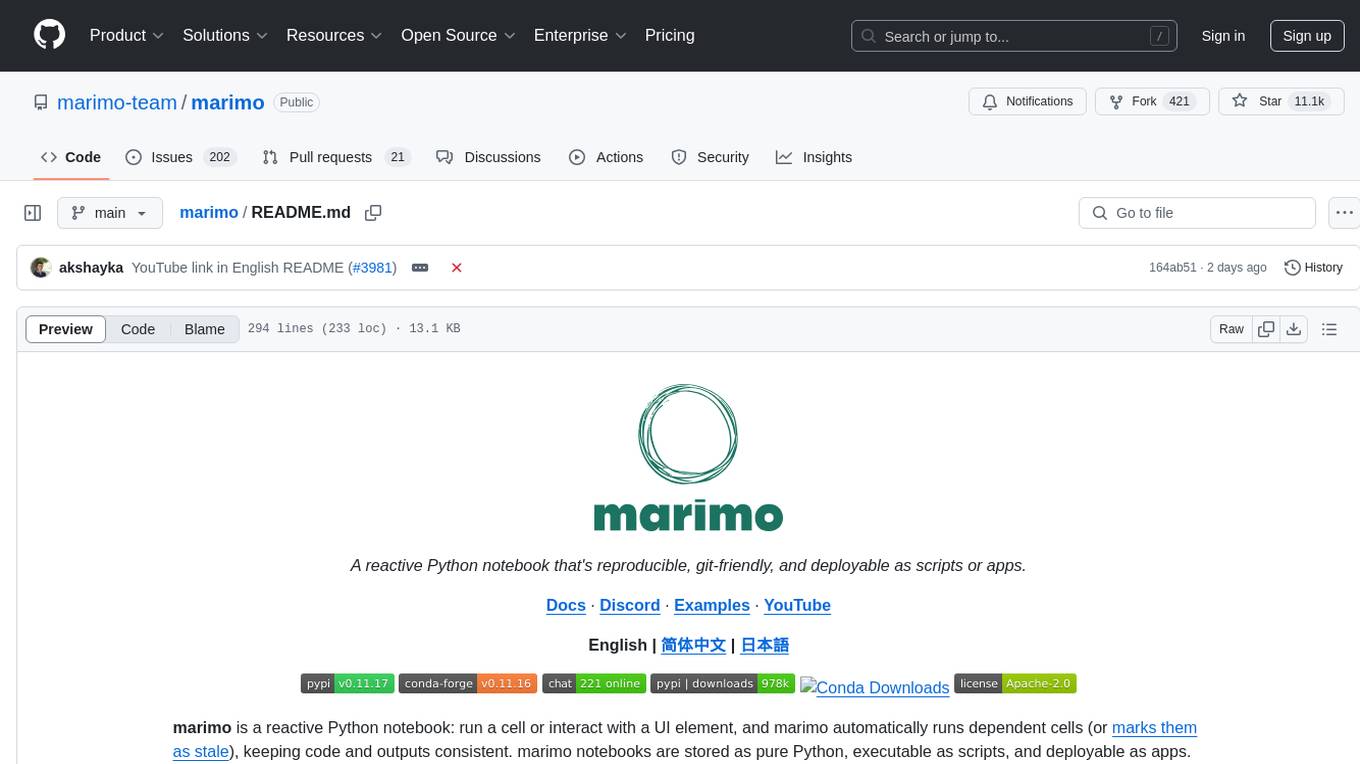
marimo
Marimo is a reactive Python notebook that ensures code and outputs consistency by automatically running dependent cells or marking them as stale. It replaces various tools like Jupyter, streamlit, and more, offering an interactive environment with features like binding UI elements to Python, reproducibility, executability as scripts or apps, shareability, and designed for data tasks. It is git-friendly, offers a modern editor with AI assistants, and comes with built-in package management. Marimo provides deterministic execution order, dynamic markdown and SQL capabilities, and a performant runtime. It is easy to get started with and suitable for both beginners and power users.
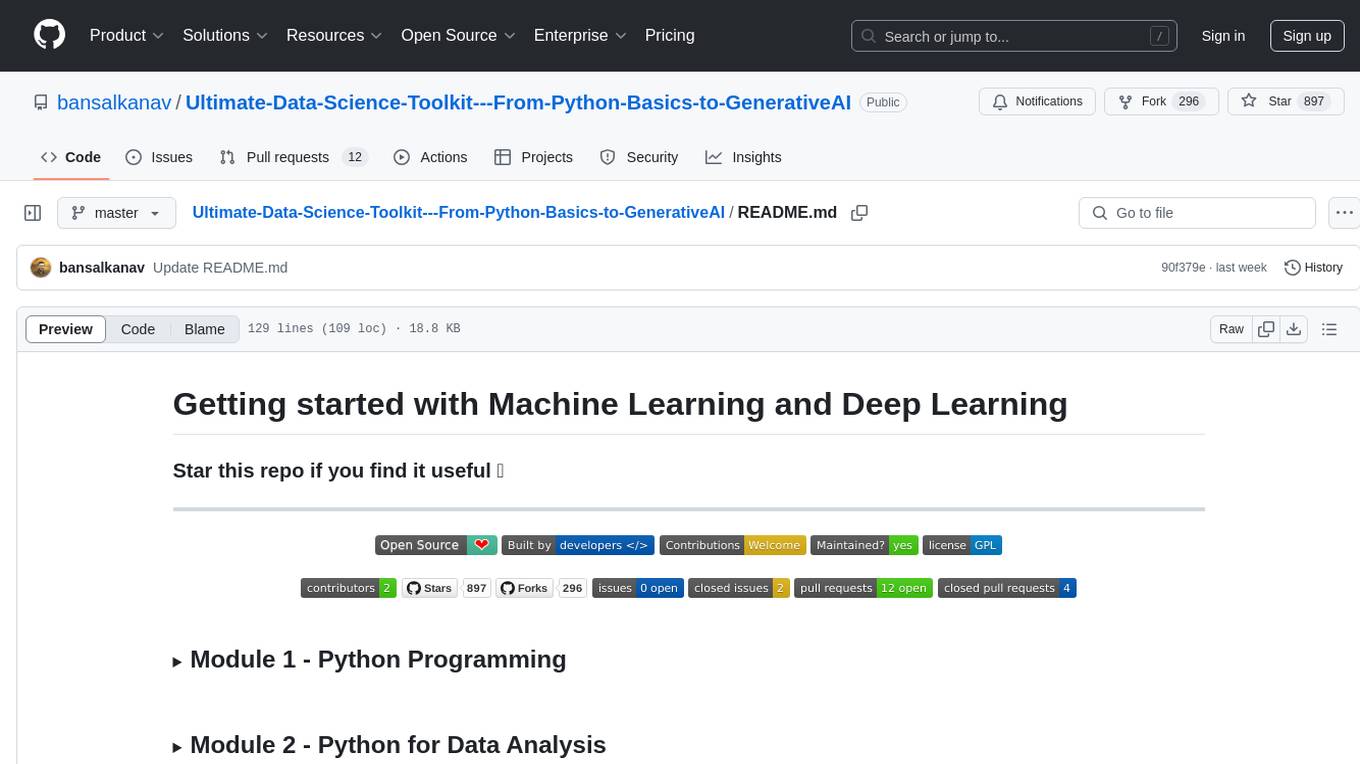
Ultimate-Data-Science-Toolkit---From-Python-Basics-to-GenerativeAI
Ultimate Data Science Toolkit is a comprehensive repository covering Python basics to Generative AI. It includes modules on Python programming, data analysis, statistics, machine learning, MLOps, case studies, and deep learning. The repository provides detailed tutorials on various topics such as Python data structures, control statements, functions, modules, object-oriented programming, exception handling, file handling, web API, databases, list comprehension, lambda functions, Pandas, Numpy, data visualization, statistical analysis, supervised and unsupervised machine learning algorithms, model serialization, ML pipeline orchestration, case studies, and deep learning concepts like neural networks and autoencoders.
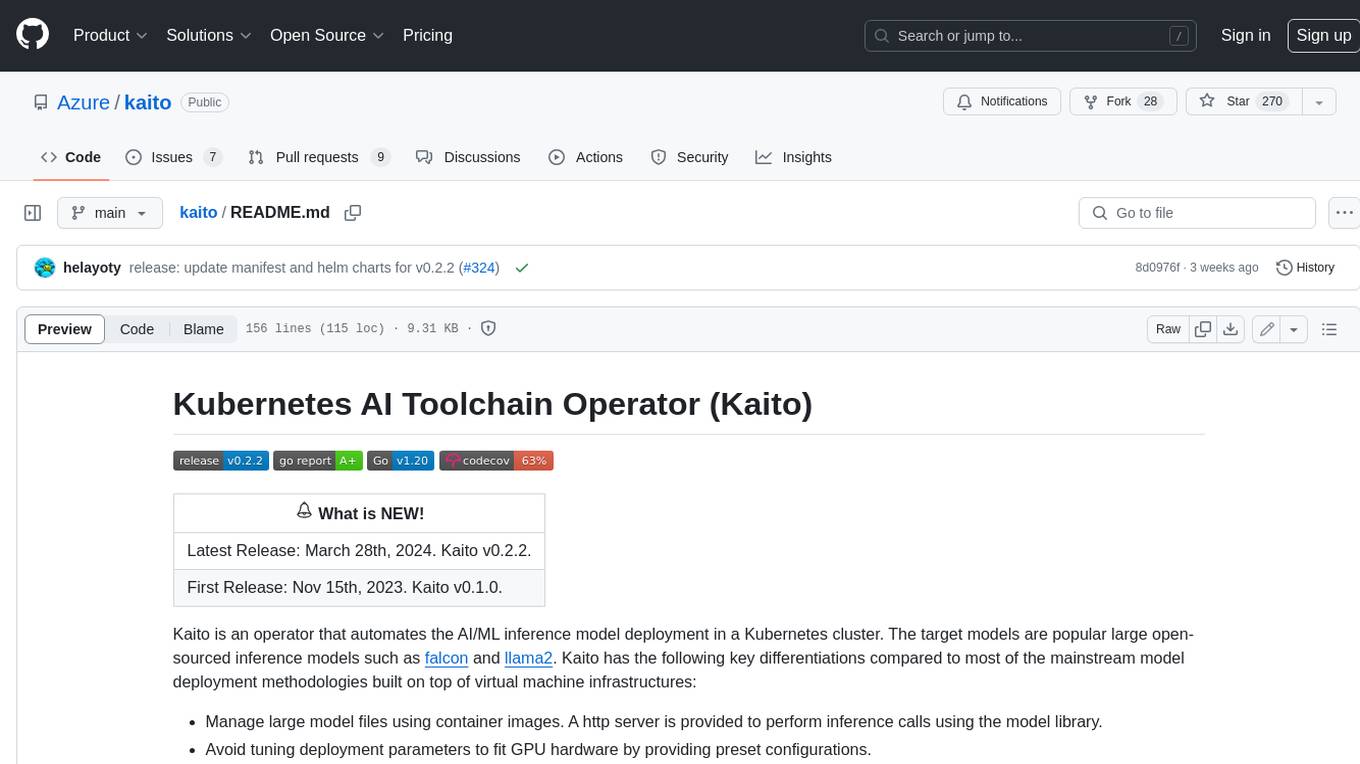
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
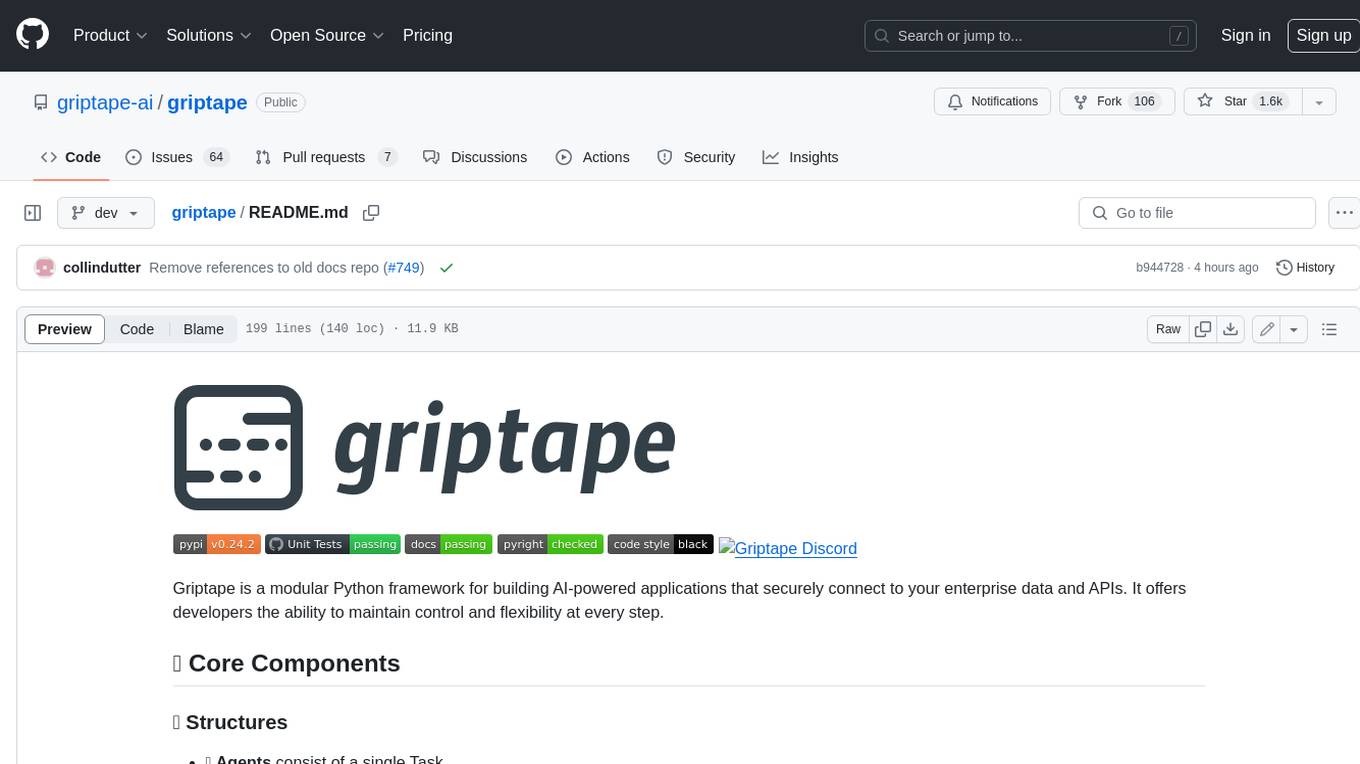
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.
For similar jobs
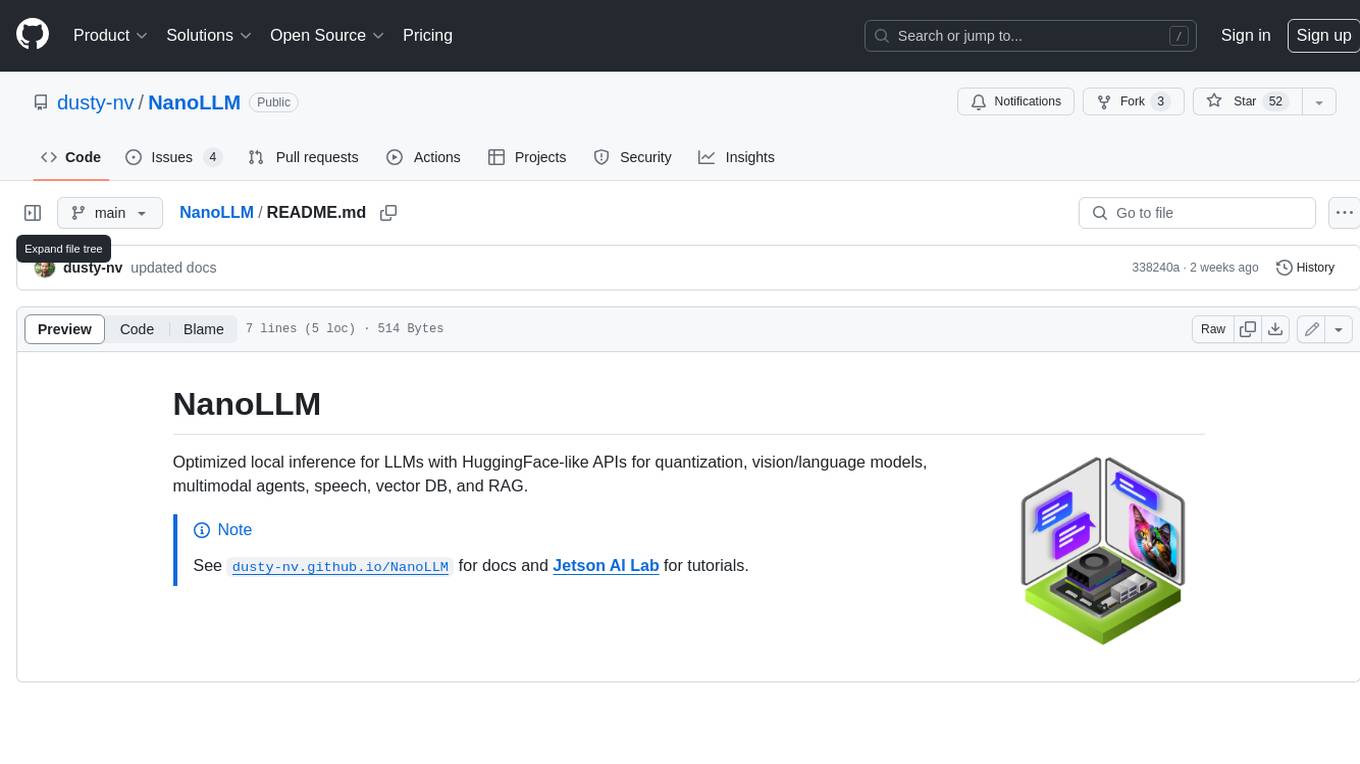
NanoLLM
NanoLLM is a tool designed for optimized local inference for Large Language Models (LLMs) using HuggingFace-like APIs. It supports quantization, vision/language models, multimodal agents, speech, vector DB, and RAG. The tool aims to provide efficient and effective processing for LLMs on local devices, enhancing performance and usability for various AI applications.
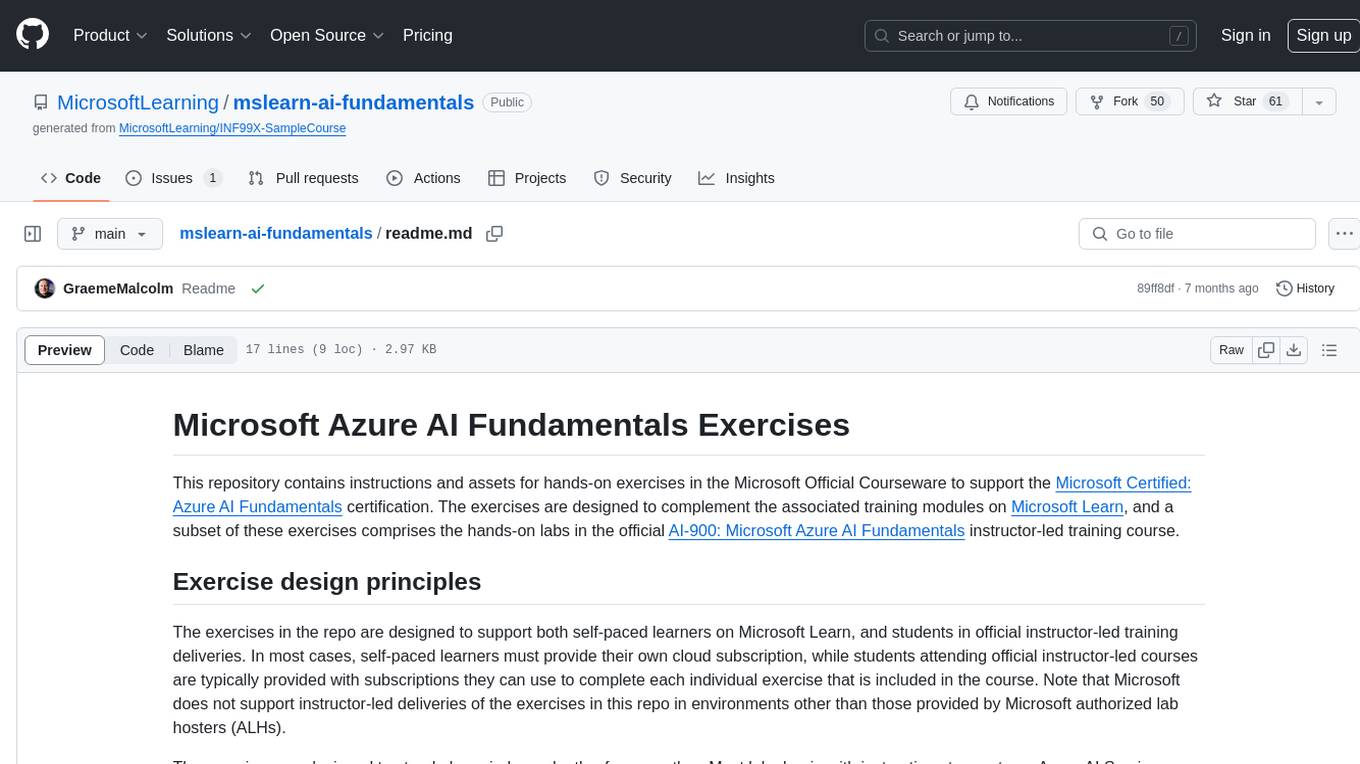
mslearn-ai-fundamentals
This repository contains materials for the Microsoft Learn AI Fundamentals module. It covers the basics of artificial intelligence, machine learning, and data science. The content includes hands-on labs, interactive learning modules, and assessments to help learners understand key concepts and techniques in AI. Whether you are new to AI or looking to expand your knowledge, this module provides a comprehensive introduction to the fundamentals of AI.
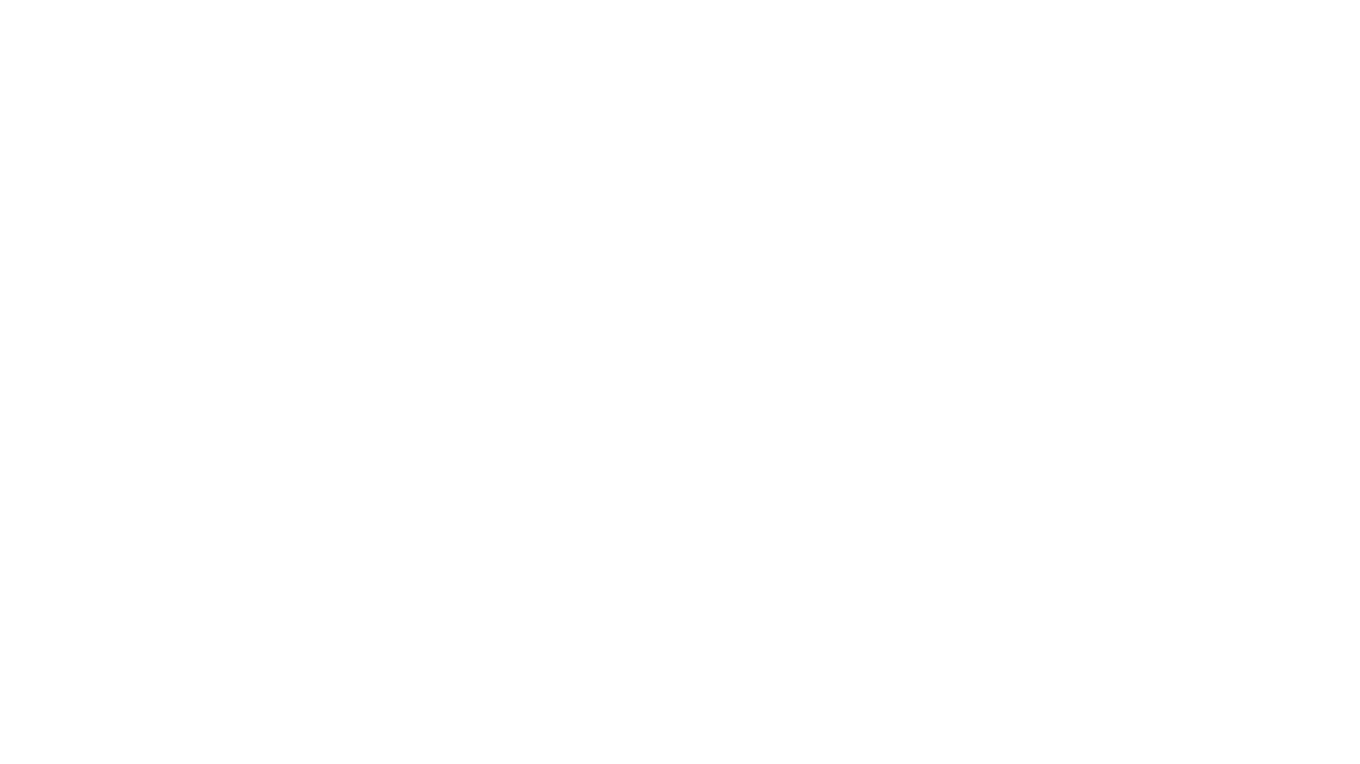
awesome-ai-tools
Awesome AI Tools is a curated list of popular tools and resources for artificial intelligence enthusiasts. It includes a wide range of tools such as machine learning libraries, deep learning frameworks, data visualization tools, and natural language processing resources. Whether you are a beginner or an experienced AI practitioner, this repository aims to provide you with a comprehensive collection of tools to enhance your AI projects and research. Explore the list to discover new tools, stay updated with the latest advancements in AI technology, and find the right resources to support your AI endeavors.
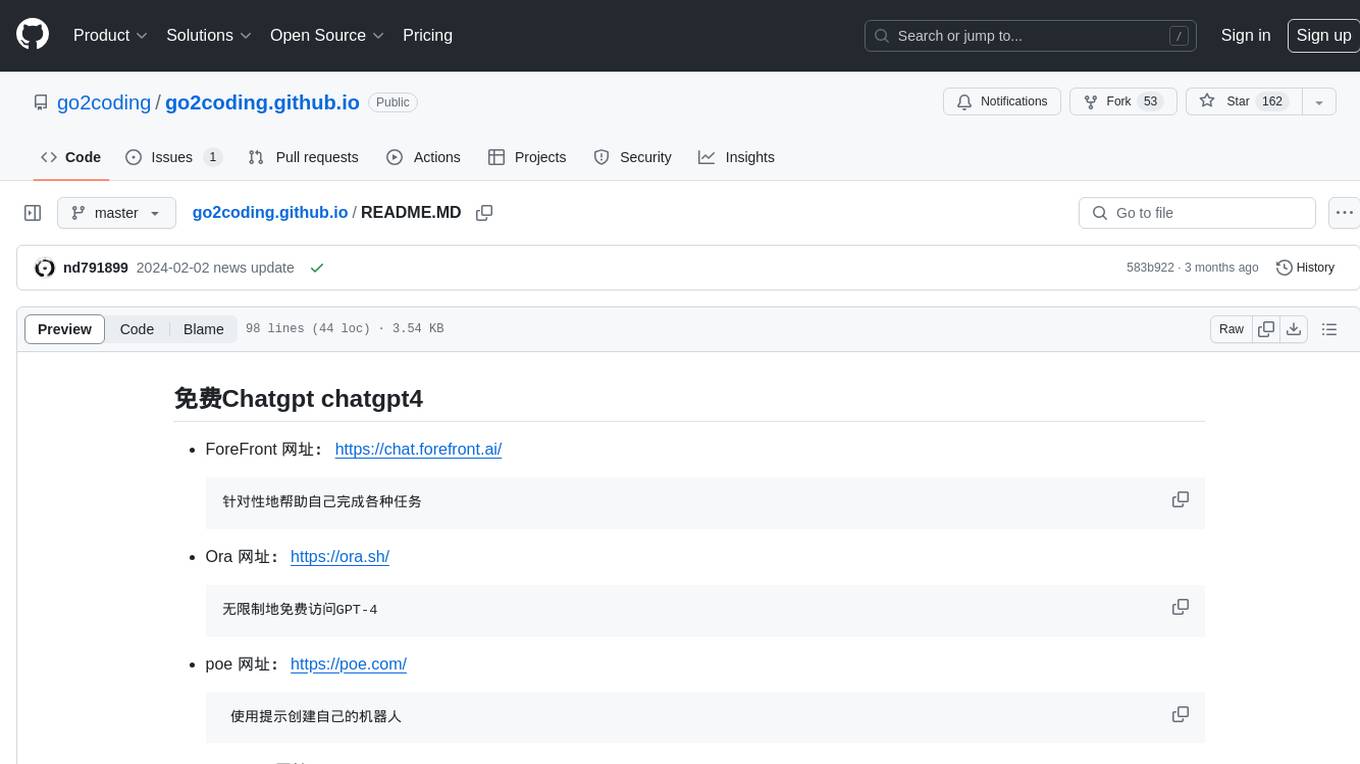
go2coding.github.io
The go2coding.github.io repository is a collection of resources for AI enthusiasts, providing information on AI products, open-source projects, AI learning websites, and AI learning frameworks. It aims to help users stay updated on industry trends, learn from community projects, access learning resources, and understand and choose AI frameworks. The repository also includes instructions for local and external deployment of the project as a static website, with details on domain registration, hosting services, uploading static web pages, configuring domain resolution, and a visual guide to the AI tool navigation website. Additionally, it offers a platform for AI knowledge exchange through a QQ group and promotes AI tools through a WeChat public account.
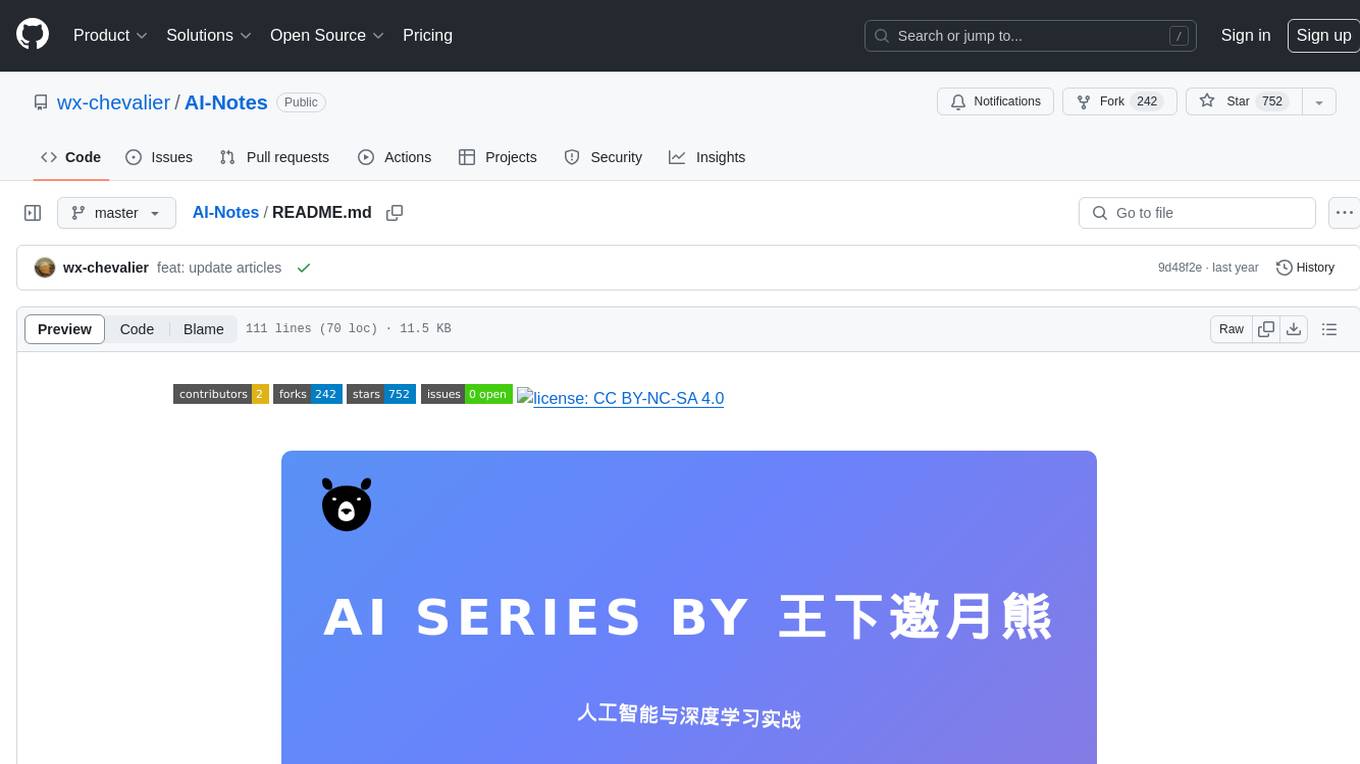
AI-Notes
AI-Notes is a repository dedicated to practical applications of artificial intelligence and deep learning. It covers concepts such as data mining, machine learning, natural language processing, and AI. The repository contains Jupyter Notebook examples for hands-on learning and experimentation. It explores the development stages of AI, from narrow artificial intelligence to general artificial intelligence and superintelligence. The content delves into machine learning algorithms, deep learning techniques, and the impact of AI on various industries like autonomous driving and healthcare. The repository aims to provide a comprehensive understanding of AI technologies and their real-world applications.
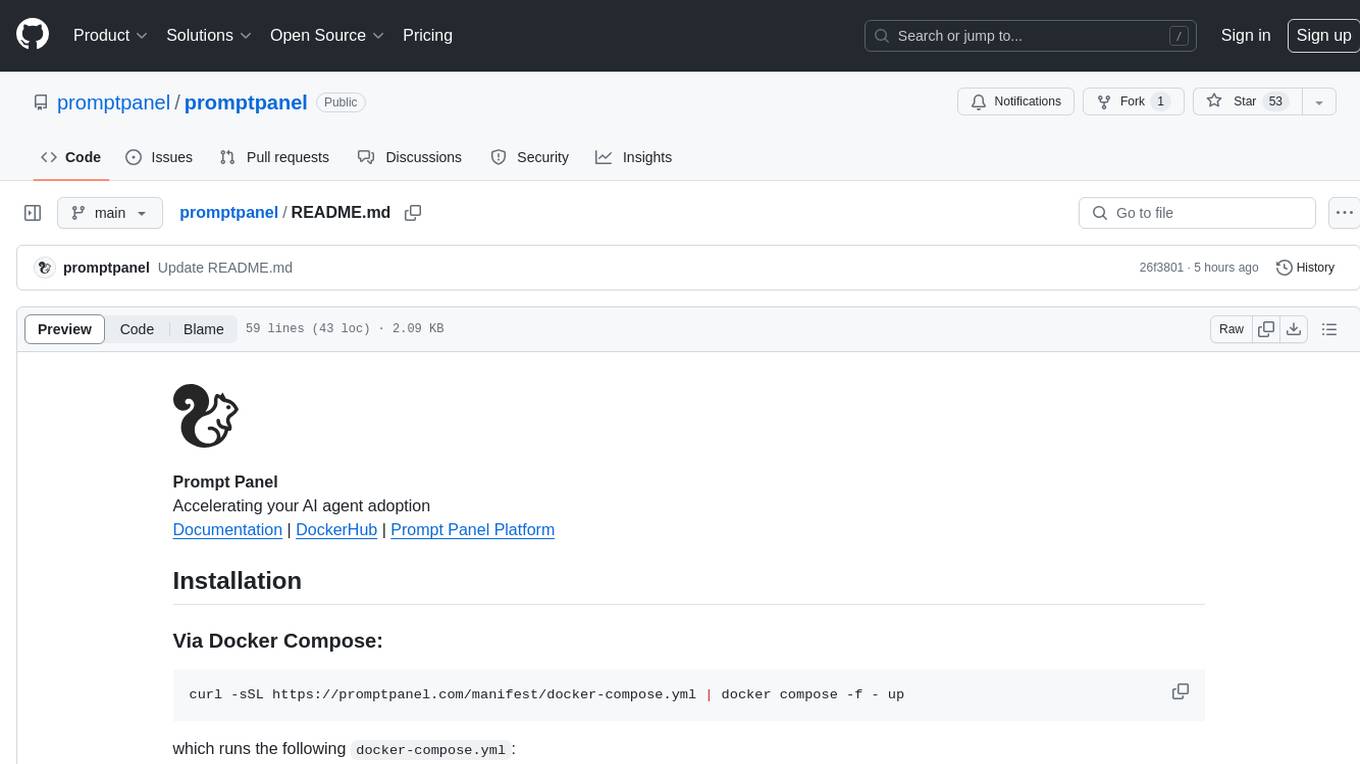
promptpanel
Prompt Panel is a tool designed to accelerate the adoption of AI agents by providing a platform where users can run large language models across any inference provider, create custom agent plugins, and use their own data safely. The tool allows users to break free from walled-gardens and have full control over their models, conversations, and logic. With Prompt Panel, users can pair their data with any language model, online or offline, and customize the system to meet their unique business needs without any restrictions.
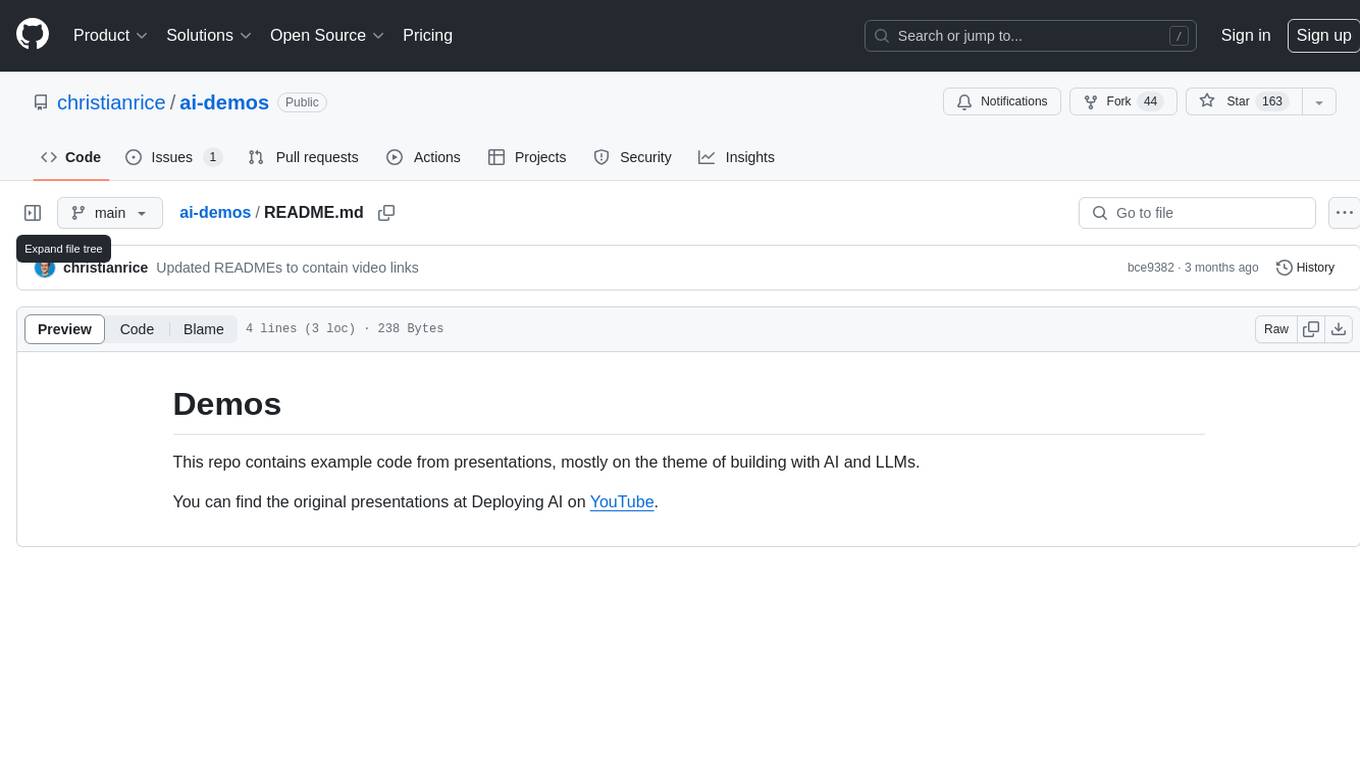
ai-demos
The 'ai-demos' repository is a collection of example code from presentations focusing on building with AI and LLMs. It serves as a resource for developers looking to explore practical applications of artificial intelligence in their projects. The code snippets showcase various techniques and approaches to leverage AI technologies effectively. The repository aims to inspire and educate developers on integrating AI solutions into their applications.
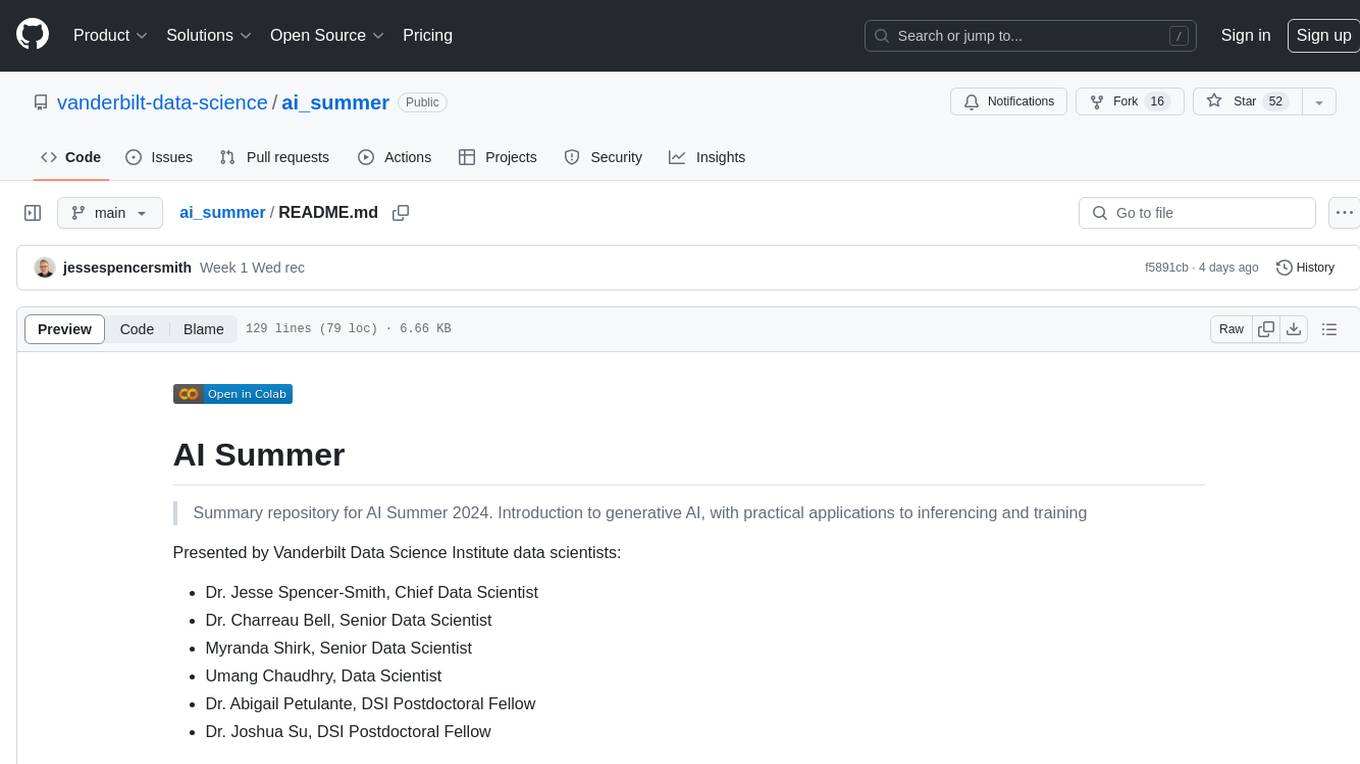
ai_summer
AI Summer is a repository focused on providing workshops and resources for developing foundational skills in generative AI models and transformer models. The repository offers practical applications for inferencing and training, with a specific emphasis on understanding and utilizing advanced AI chat models like BingGPT. Participants are encouraged to engage in interactive programming environments, decide on projects to work on, and actively participate in discussions and breakout rooms. The workshops cover topics such as generative AI models, retrieval-augmented generation, building AI solutions, and fine-tuning models. The goal is to equip individuals with the necessary skills to work with AI technologies effectively and securely, both locally and in the cloud.