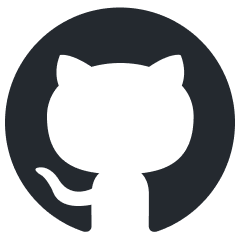
ailab
None
Stars: 136
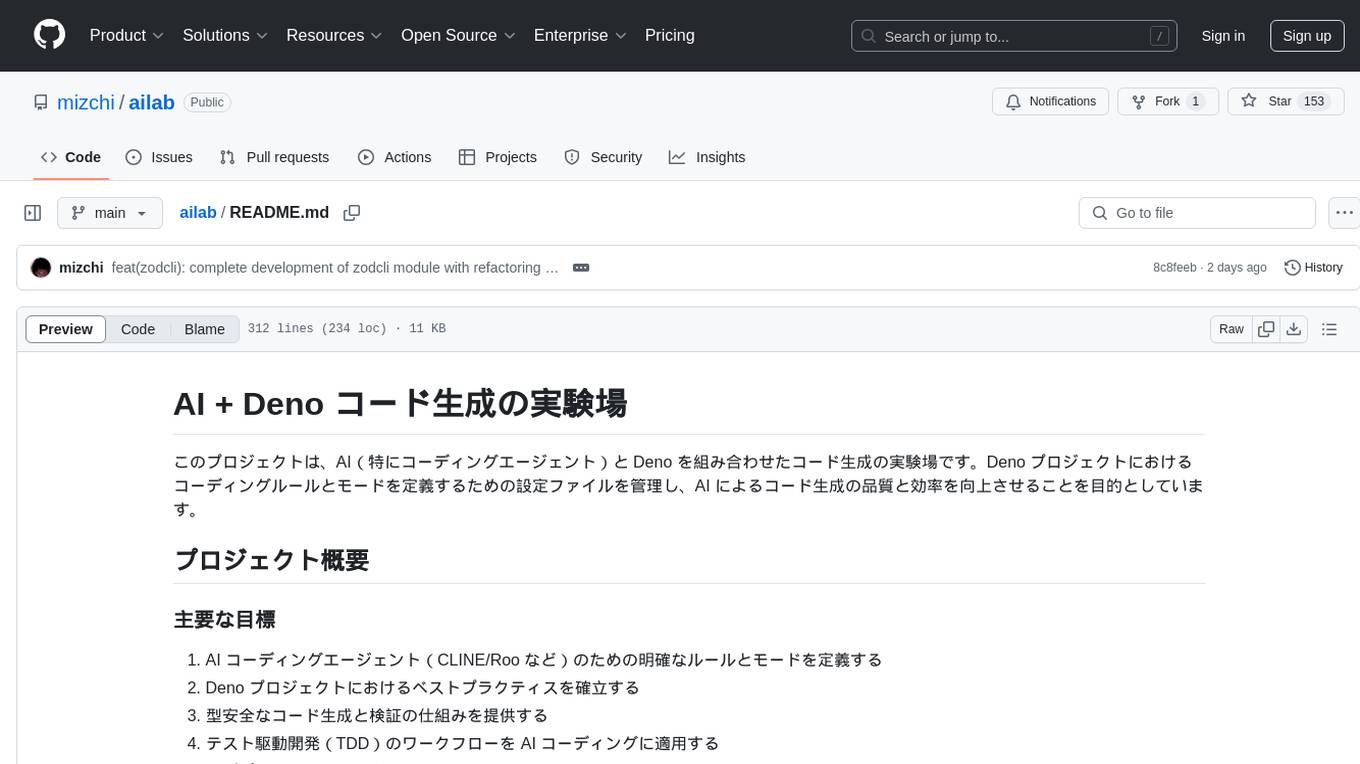
The 'ailab' project is an experimental ground for code generation combining AI (especially coding agents) and Deno. It aims to manage configuration files defining coding rules and modes in Deno projects, enhancing the quality and efficiency of code generation by AI. The project focuses on defining clear rules and modes for AI coding agents, establishing best practices in Deno projects, providing mechanisms for type-safe code generation and validation, applying test-driven development (TDD) workflow to AI coding, and offering implementation examples utilizing design patterns like adapter pattern.
README:
このプロジェクトは、AI(特にコーディングエージェント)と Deno を組み合わせたコード生成の実験場です。Deno プロジェクトにおけるコーディングルールとモードを定義するための設定ファイルを管理し、AI によるコード生成の品質と効率を向上させることを目的としています。
- AI コーディングエージェント(CLINE/Roo など)のための明確なルールとモードを定義する
- Deno プロジェクトにおけるベストプラクティスを確立する
- 型安全なコード生成と検証の仕組みを提供する
- テスト駆動開発(TDD)のワークフローを AI コーディングに適用する
- アダプターパターンなどの設計パターンを活用した実装例を提供する
-
コーディングルール定義
- 基本ルール(型と関数インターフェース設計、コードコメント、実装パターン選択)
- Deno 固有のルール(テスト、モジュール依存関係、コード品質監視)
- Git ワークフロー(コミット、プルリクエスト作成)
- TypeScript ベストプラクティス(型使用方針、エラー処理、実装パターン)
-
実装モード
- スクリプトモード: 一つのファイルに完結した実装
- テストファーストモード: 型シグネチャとテストを先に書く実装
- モジュールモード: 複数のファイルで構成される実装
-
ユーティリティモジュール
- type-predictor: JSON データから型を予測し、zod スキーマを生成するモジュール
- アダプターパターン実装例: 外部 API との通信を抽象化する実装
- Deno: TypeScript のネイティブサポート、セキュリティ機能、標準ライブラリ
- TypeScript: 静的型付け、型推論、インターフェース
- Zod: スキーマ検証、ランタイム型チェック
- Neverthrow: Result 型によるエラー処理
-
Deno 標準テストライブラリ:
@std/expect
、@std/testing/bdd
- テストカバレッジ計測
-
Deno タスクランナー:
deno.json
での定義 - GitHub Actions: CI/CD パイプライン
JSONデータから型を予測し、zodスキーマを生成するモジュール。
特徴:
- JSONデータの構造を解析し、型を予測
- 配列、オブジェクト、Record型、列挙型などの高度な型を検出
- zodスキーマの自動生成
- 詳細な型情報の分析機能
使用例:
import { TypePredictor } from "./mod.ts";
// インスタンスを作成
const predictor = new TypePredictor();
// JSONデータから型を予測してスキーマを生成
const data = {
name: "John",
age: 30,
scores: [85, 92, 78],
};
// スキーマを生成
const schema = predictor.predict(data);
// スキーマを使用してバリデーション
const result = schema.safeParse(data);
Zod を使用した型安全なコマンドラインパーサーモジュールです。
特徴:
- 型安全: Zodスキーマに基づいた型安全なCLIパーサー
- 自動ヘルプ生成: コマンド構造から自動的にヘルプテキストを生成
- 位置引数とオプションのサポート: 位置引数と名前付き引数の両方をサポート
- サブコマンドのサポート: gitのようなサブコマンド構造をサポート
- デフォルト値: Zodの機能を活用したデフォルト値の設定
使用例:
import { createCliCommand, runCommand } from "@mizchi/zodcli";
import { z } from "npm:zod";
const cli = createCliCommand({
name: "myapp",
description: "My CLI application",
args: {
file: {
type: z.string().describe("input file"),
positional: true,
},
verbose: {
type: z.boolean().default(false),
short: "v",
}
}
});
const result = cli.parse(Deno.args);
runCommand(result, (data) => {
console.log(`Processing ${data.file}, verbose: ${data.verbose}`);
});
TypeScriptでのAdapterパターンは、外部依存を抽象化し、テスト可能なコードを実現するためのパターンです。
実装パターン:
- 関数ベース: 内部状態を持たない単純な操作の場合
- classベース: 設定やキャッシュなどの内部状態を管理する必要がある場合
- 高階関数とコンストラクタインジェクション: 外部APIとの通信など、モックが必要な場合
ベストプラクティス:
- インターフェースはシンプルに保つ
- 基本的には関数ベースを優先する
- 内部状態が必要な場合のみclassを使用する
- エラー処理はResult型で表現し、例外を使わない
コンポーネント | ステータス | 進捗率 | 優先度 |
---|---|---|---|
ルールとモード定義 | 安定 | 90% | 低 |
type-predictor | 開発中 | 60% | 高 |
zodcli | 安定 | 100% | 中 |
アダプターパターン例 | 安定 | 80% | 中 |
テストインフラ | 安定 | 70% | 中 |
CI/CD パイプライン | 開発中 | 50% | 中 |
メモリバンク | 初期段階 | 30% | 高 |
ドキュメント | 初期段階 | 40% | 高 |
-
type-predictor の基本機能完成(現在)
- 基本的な型検出と予測
- 基本的な zod スキーマ生成
- 基本的なテストケース
-
type-predictor の拡張機能(次のフェーズ)
- Record 型、列挙型、ユニオン型の検出
- エッジケースのテスト追加
- パフォーマンスの最適化
-
実装例の充実(将来)
- 新しい設計パターンの実装例
- モジュールモードの詳細な実装例
- ユースケースの例の追加
-
完全なドキュメントとテスト(最終フェーズ)
- API ドキュメントの完成
- テストカバレッジ目標の達成
- チュートリアルとガイドラインの完成
- CI/CD パイプラインの完全自動化
このリポジトリの .cline
ディレクトリは、Deno プロジェクトにおけるコーディングルールとモードを定義するための設定ファイルを管理しています。
.cline/
├── build.ts - プロンプトファイルを結合して .clinerules と .roomodes を生成するスクリプト
├── rules/ - コーディングルールを定義するマークダウンファイル
│ ├── 01_basic.md - 基本的なルールと AI Coding with Deno の概要
│ ├── deno_rules.md - Deno に関するルール(テスト、モジュール依存関係など)
│ ├── git_workflow.md - Git ワークフローに関するルール
│ └── ts_bestpractice.md - TypeScript のコーディングベストプラクティス
└── roomodes/ - 実装モードを定義するマークダウンファイル
├── deno-script.md - スクリプトモードの定義
├── deno-module.md - モジュールモードの定義
└── deno-tdd.md - テストファーストモードの定義
.cline/build.ts
スクリプトを実行すると、以下のファイルが生成されます:
-
.clinerules
-rules
ディレクトリ内のマークダウンファイルを結合したファイル -
.roomodes
-roomodes
ディレクトリ内のマークダウンファイルから生成された JSON ファイル
-
.cline/rules
ディレクトリにコーディングルールを定義するマークダウンファイルを追加または編集します。 -
.cline/roomodes
ディレクトリに実装モードを定義するマークダウンファイルを追加または編集します。 -
.cline/build.ts
スクリプトを実行して、.clinerules
と.roomodes
ファイルを生成します。
deno run --allow-read --allow-write .cline/build.ts
- 生成された
.clinerules
と.roomodes
ファイルは、AI コーディングアシスタント(CLINE/Roo など)によって読み込まれ、プロジェクトのルールとモードが適用されます。
プロジェクトで定義されているモードは以下の通りです:
-
deno-script
(Deno:ScriptMode) - スクリプトモード -
deno-module
(Deno:Module) - モジュールモード -
deno-tdd
(Deno:TestFirstMode) - テストファーストモード
モードを切り替えるには、AI コーディングアシスタントに対して以下のように指示します:
モードを deno-script に切り替えてください。
または、ファイルの冒頭に特定のマーカーを含めることでモードを指定することもできます:
- スクリプトモード:
@script
- テストファーストモード:
@tdd
例:
// @script @tdd
// このファイルはスクリプトモードとテストファーストモードの両方で実装されます
-
Deno のインストール
# Unix (macOS, Linux) curl -fsSL https://deno.land/x/install/install.sh | sh # Windows (PowerShell) iwr https://deno.land/x/install/install.ps1 -useb | iex
-
エディタ設定
- VSCode + Deno 拡張機能
- 設定例:
{ "deno.enable": true, "deno.lint": true, "deno.unstable": false, "editor.formatOnSave": true, "editor.defaultFormatter": "denoland.vscode-deno" }
-
プロジェクトのセットアップ
# リポジトリのクローン git clone <repository-url> cd <repository-directory> # 依存関係のキャッシュ deno cache --reload deps.ts # ルールとモードの生成 deno run --allow-read --allow-write .cline/build.ts
-
新しいスクリプトの作成
# スクリプトモードでの開発 touch scripts/new-script.ts # ファイル冒頭に `@script` を追加
-
テストの実行
# 単一ファイルのテスト deno test scripts/new-script.ts # すべてのテストの実行 deno test # カバレッジの計測 deno test --coverage=coverage && deno coverage coverage
-
リントとフォーマット
# リント deno lint # フォーマット deno fmt
-
依存関係の検証
deno task check:deps
MIT
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for ailab
Similar Open Source Tools
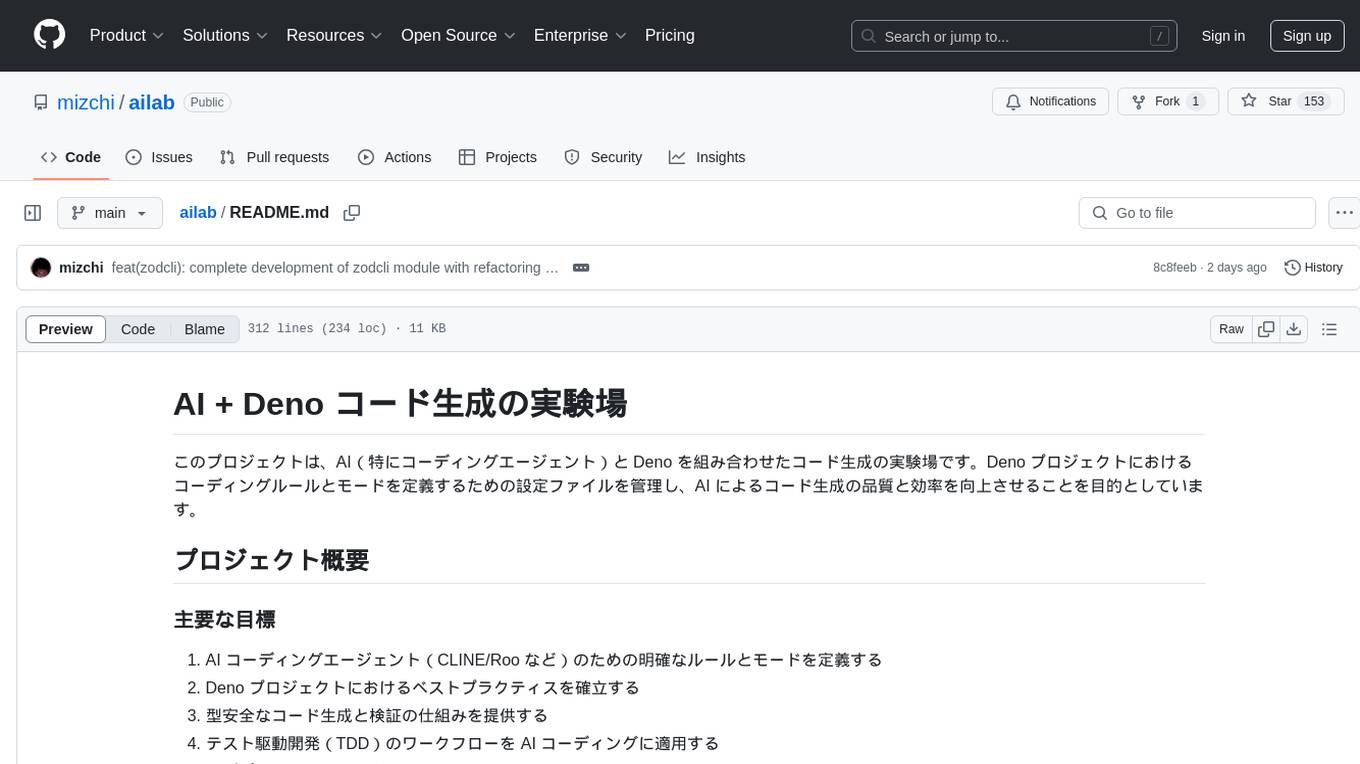
ailab
The 'ailab' project is an experimental ground for code generation combining AI (especially coding agents) and Deno. It aims to manage configuration files defining coding rules and modes in Deno projects, enhancing the quality and efficiency of code generation by AI. The project focuses on defining clear rules and modes for AI coding agents, establishing best practices in Deno projects, providing mechanisms for type-safe code generation and validation, applying test-driven development (TDD) workflow to AI coding, and offering implementation examples utilizing design patterns like adapter pattern.
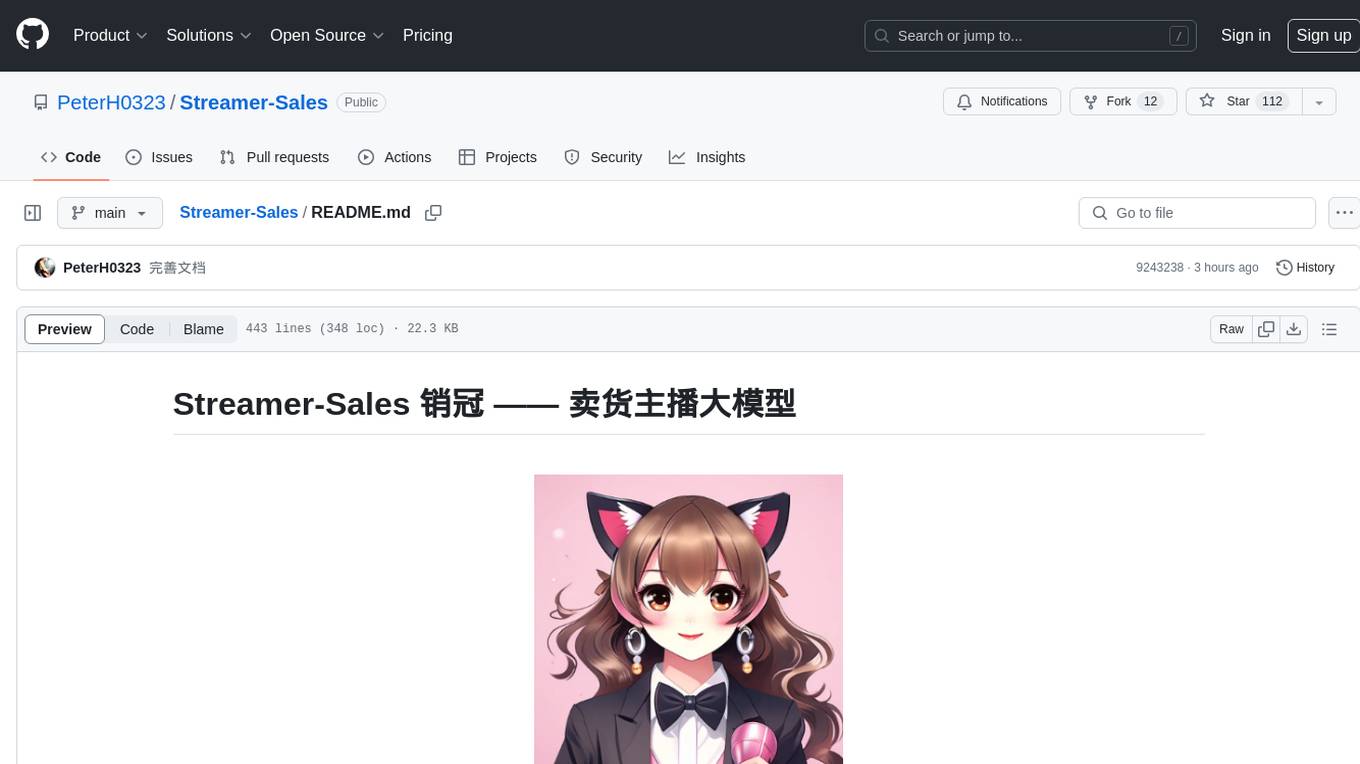
Streamer-Sales
Streamer-Sales is a large model for live streamers that can explain products based on their characteristics and inspire users to make purchases. It is designed to enhance sales efficiency and user experience, whether for online live sales or offline store promotions. The model can deeply understand product features and create tailored explanations in vivid and precise language, sparking user's desire to purchase. It aims to revolutionize the shopping experience by providing detailed and unique product descriptions to engage users effectively.
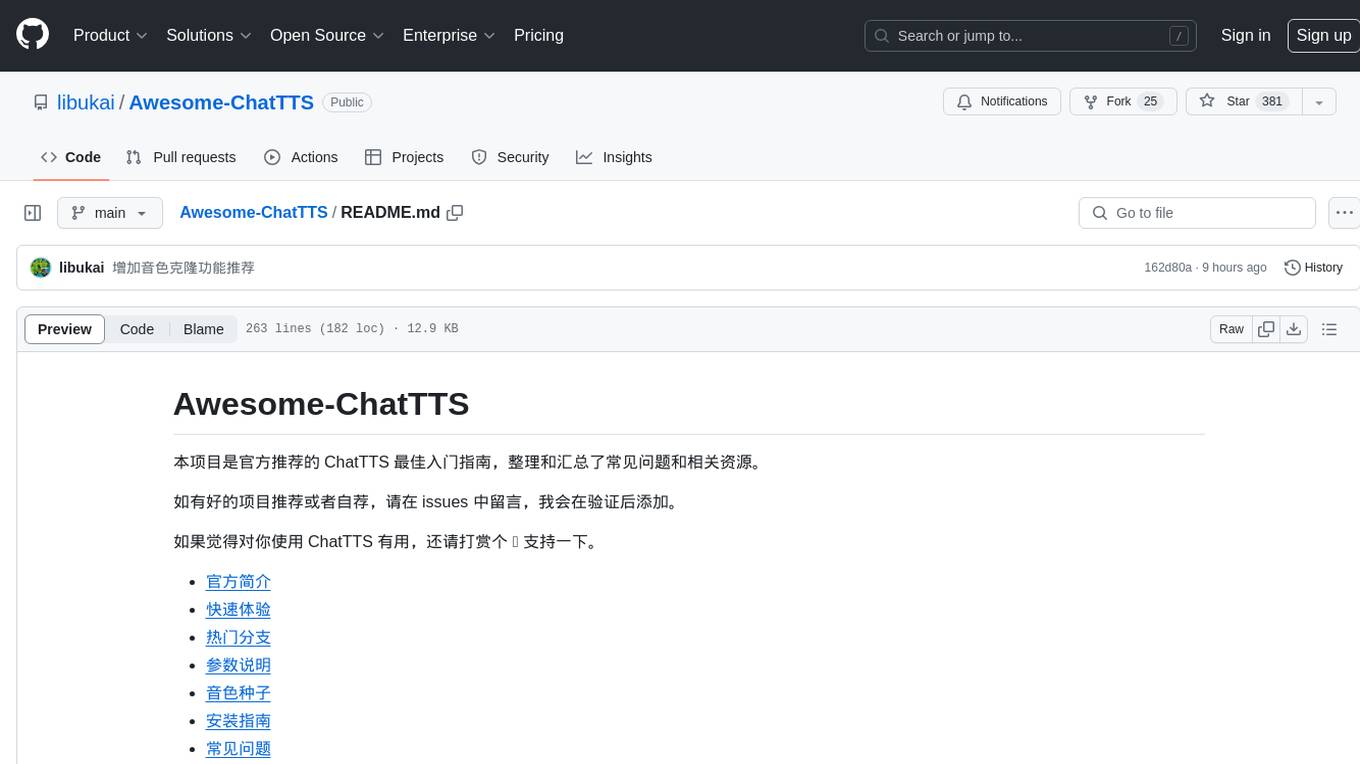
Awesome-ChatTTS
Awesome-ChatTTS is an official recommended guide for ChatTTS beginners, compiling common questions and related resources. It provides a comprehensive overview of the project, including official introduction, quick experience options, popular branches, parameter explanations, voice seed details, installation guides, FAQs, and error troubleshooting. The repository also includes video tutorials, discussion community links, and project trends analysis. Users can explore various branches for different functionalities and enhancements related to ChatTTS.
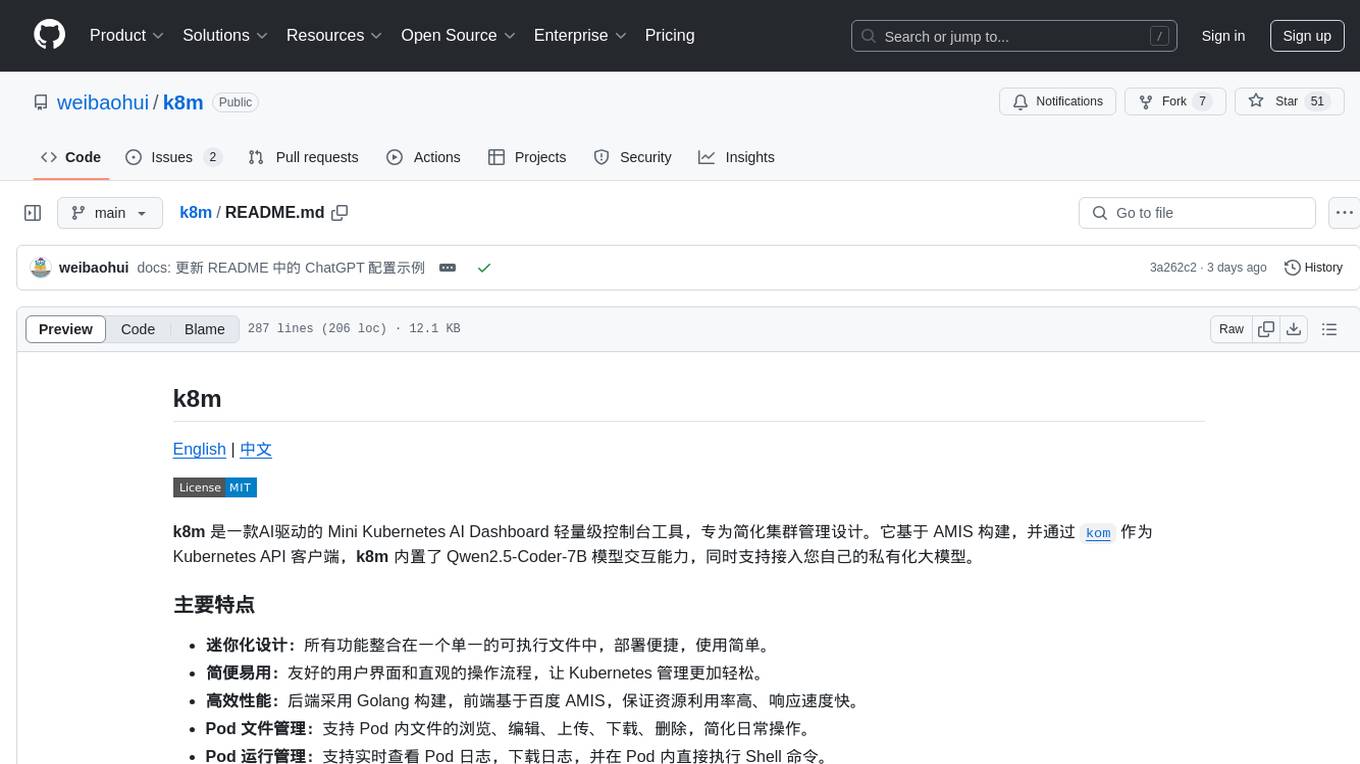
k8m
k8m is an AI-driven Mini Kubernetes AI Dashboard lightweight console tool designed to simplify cluster management. It is built on AMIS and uses 'kom' as the Kubernetes API client. k8m has built-in Qwen2.5-Coder-7B model interaction capabilities and supports integration with your own private large models. Its key features include miniaturized design for easy deployment, user-friendly interface for intuitive operation, efficient performance with backend in Golang and frontend based on Baidu AMIS, pod file management for browsing, editing, uploading, downloading, and deleting files, pod runtime management for real-time log viewing, log downloading, and executing shell commands within pods, CRD management for automatic discovery and management of CRD resources, and intelligent translation and diagnosis based on ChatGPT for YAML property translation, Describe information interpretation, AI log diagnosis, and command recommendations, providing intelligent support for managing k8s. It is cross-platform compatible with Linux, macOS, and Windows, supporting multiple architectures like x86 and ARM for seamless operation. k8m's design philosophy is 'AI-driven, lightweight and efficient, simplifying complexity,' helping developers and operators quickly get started and easily manage Kubernetes clusters.
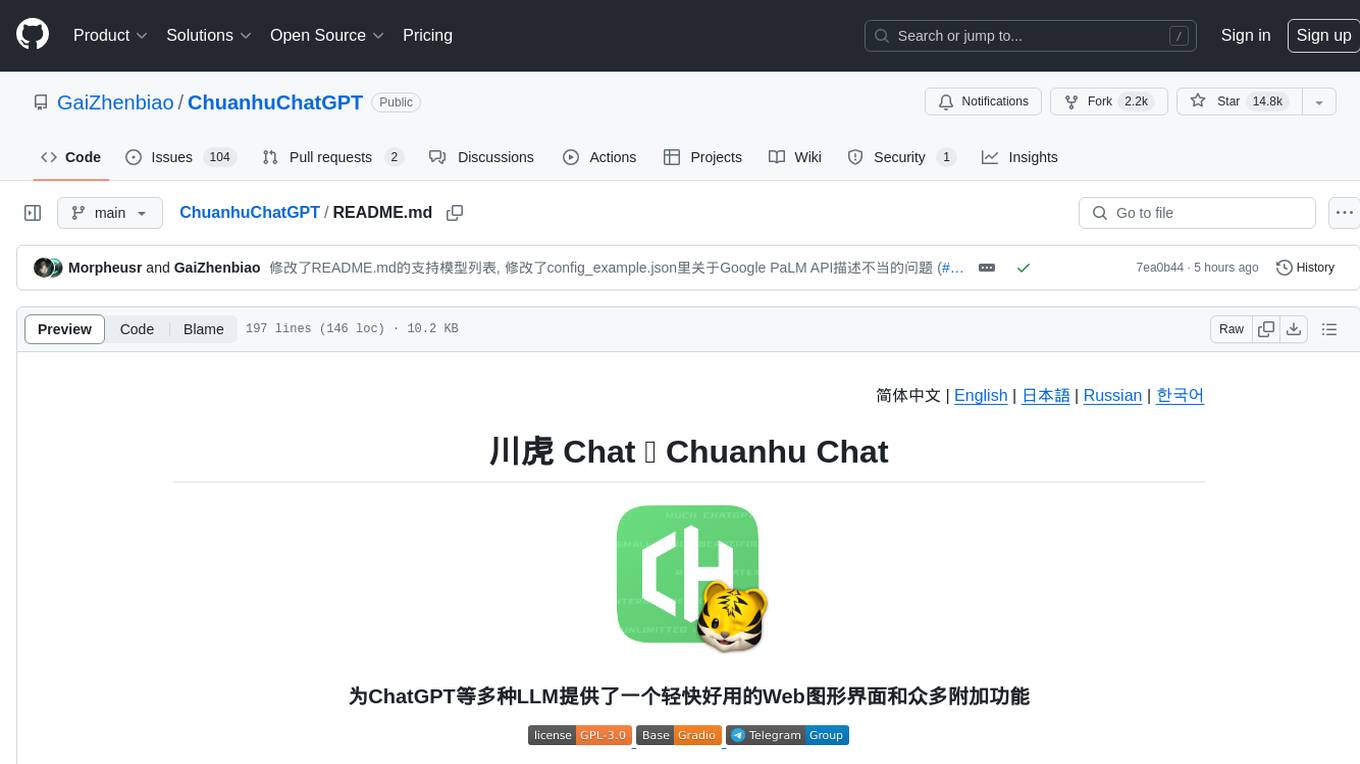
ChuanhuChatGPT
Chuanhu Chat is a user-friendly web graphical interface that provides various additional features for ChatGPT and other language models. It supports GPT-4, file-based question answering, local deployment of language models, online search, agent assistant, and fine-tuning. The tool offers a range of functionalities including auto-solving questions, online searching with network support, knowledge base for quick reading, local deployment of language models, GPT 3.5 fine-tuning, and custom model integration. It also features system prompts for effective role-playing, basic conversation capabilities with options to regenerate or delete dialogues, conversation history management with auto-saving and search functionalities, and a visually appealing user experience with themes, dark mode, LaTeX rendering, and PWA application support.
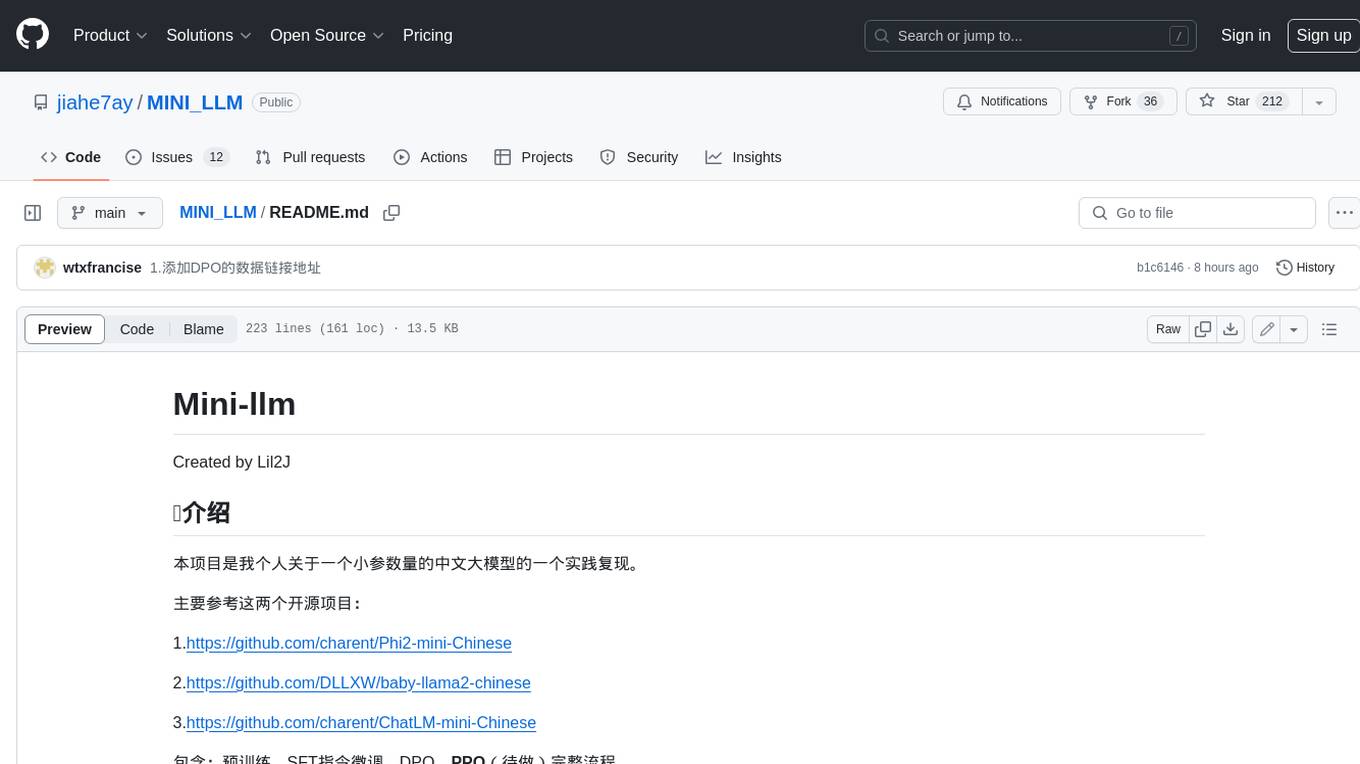
MINI_LLM
This project is a personal implementation and reproduction of a small-parameter Chinese LLM. It mainly refers to these two open source projects: https://github.com/charent/Phi2-mini-Chinese and https://github.com/DLLXW/baby-llama2-chinese. It includes the complete process of pre-training, SFT instruction fine-tuning, DPO, and PPO (to be done). I hope to share it with everyone and hope that everyone can work together to improve it!
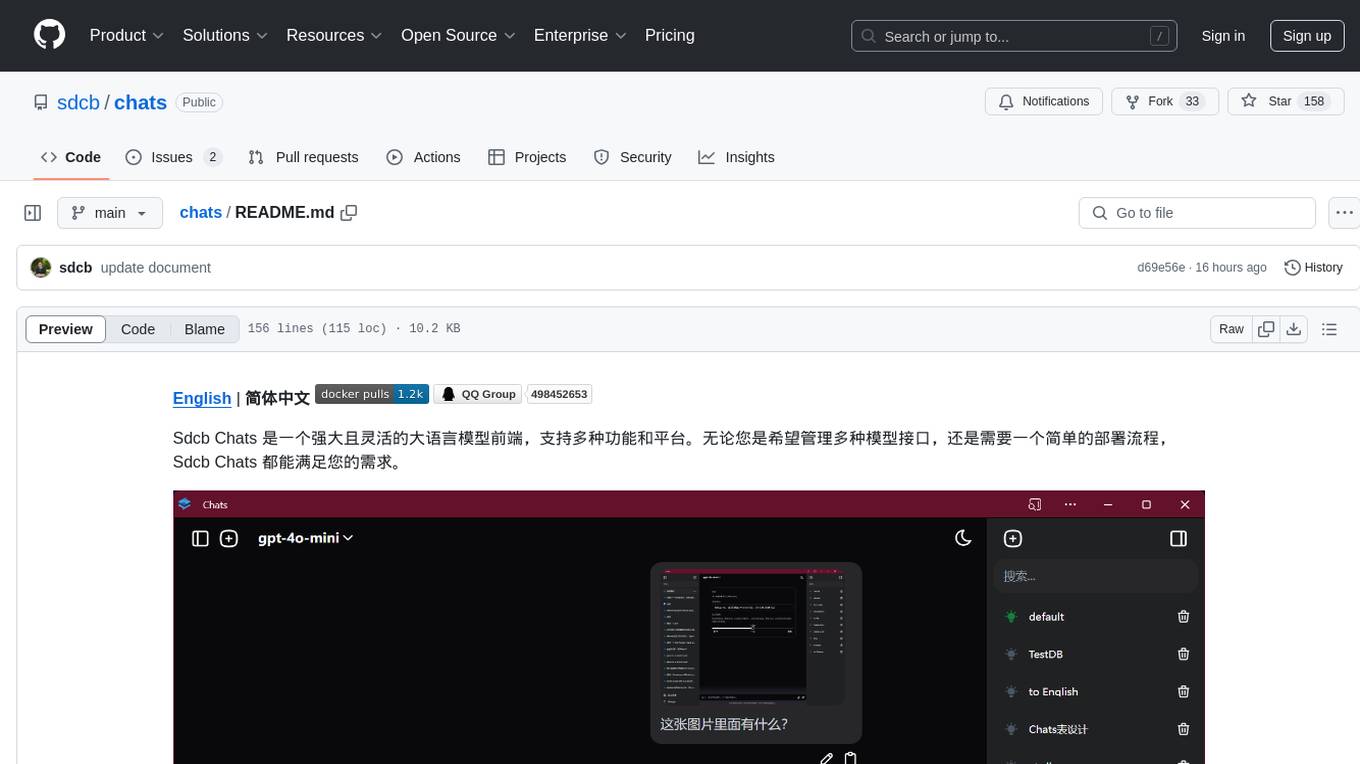
chats
Sdcb Chats is a powerful and flexible frontend for large language models, supporting multiple functions and platforms. Whether you want to manage multiple model interfaces or need a simple deployment process, Sdcb Chats can meet your needs. It supports dynamic management of multiple large language model interfaces, integrates visual models to enhance user interaction experience, provides fine-grained user permission settings for security, real-time tracking and management of user account balances, easy addition, deletion, and configuration of models, transparently forwards user chat requests based on the OpenAI protocol, supports multiple databases including SQLite, SQL Server, and PostgreSQL, compatible with various file services such as local files, AWS S3, Minio, Aliyun OSS, Azure Blob Storage, and supports multiple login methods including Keycloak SSO and phone SMS verification.
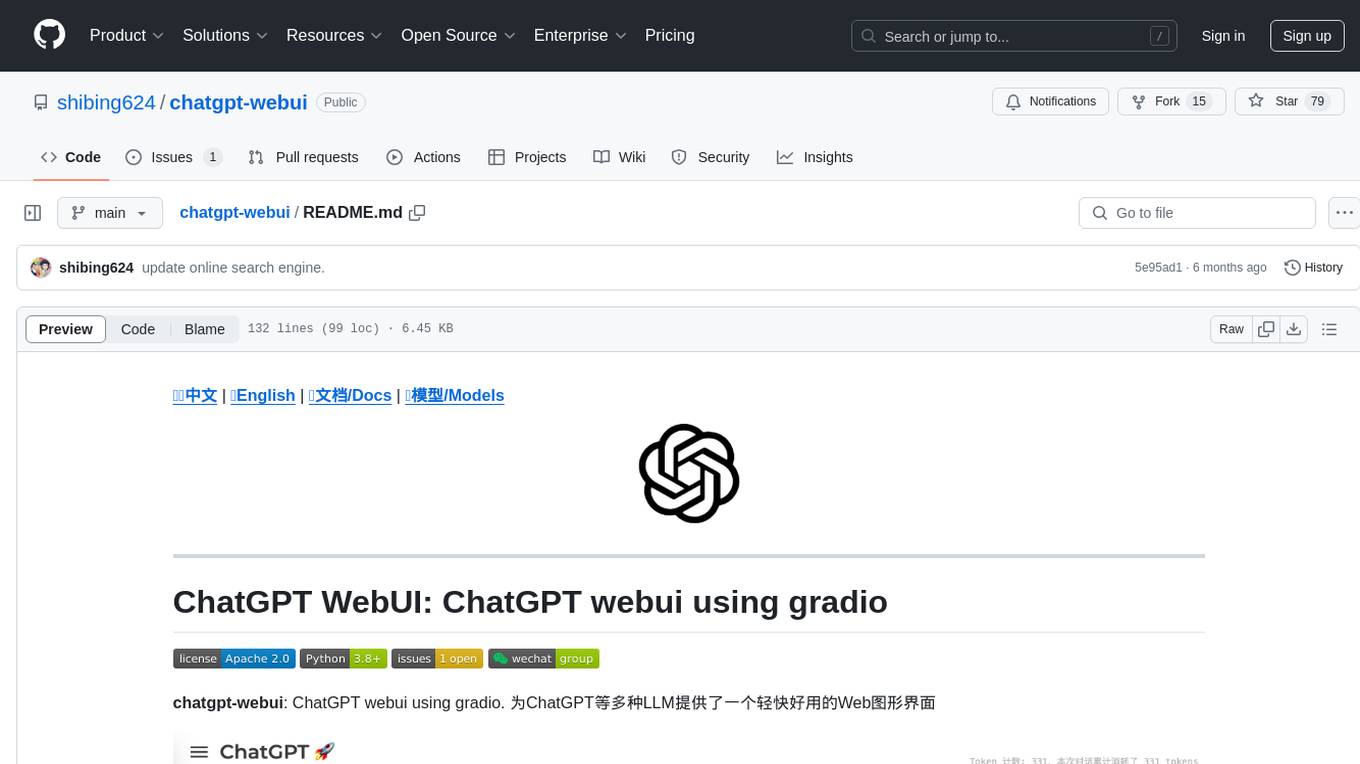
chatgpt-webui
ChatGPT WebUI is a user-friendly web graphical interface for various LLMs like ChatGPT, providing simplified features such as core ChatGPT conversation and document retrieval dialogues. It has been optimized for better RAG retrieval accuracy and supports various search engines. Users can deploy local language models easily and interact with different LLMs like GPT-4, Azure OpenAI, and more. The tool offers powerful functionalities like GPT4 API configuration, system prompt setup for role-playing, and basic conversation features. It also provides a history of conversations, customization options, and a seamless user experience with themes, dark mode, and PWA installation support.
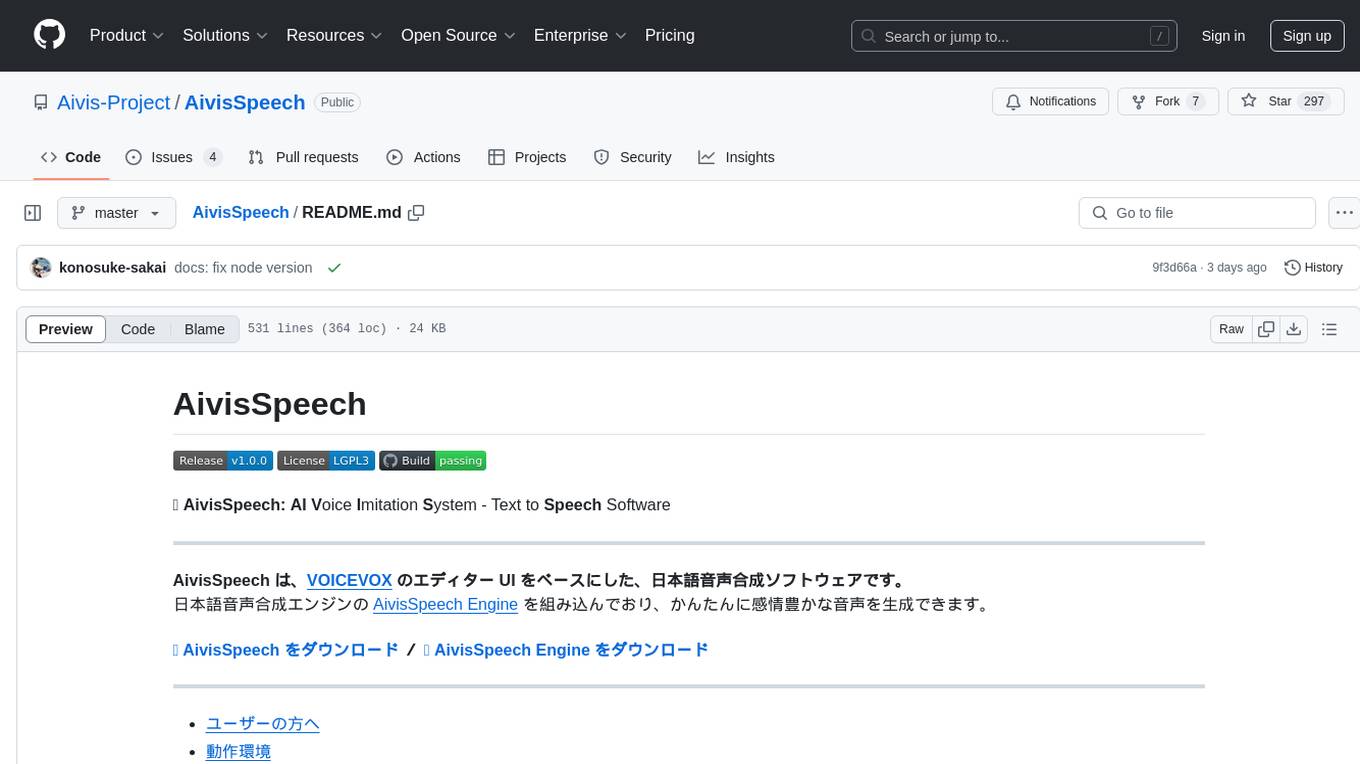
AivisSpeech
AivisSpeech is a Japanese text-to-speech software based on the VOICEVOX editor UI. It incorporates the AivisSpeech Engine for generating emotionally rich voices easily. It supports AIVMX format voice synthesis model files and specific model architectures like Style-Bert-VITS2. Users can download AivisSpeech and AivisSpeech Engine for Windows and macOS PCs, with minimum memory requirements specified. The development follows the latest version of VOICEVOX, focusing on minimal modifications, rebranding only where necessary, and avoiding refactoring. The project does not update documentation, maintain test code, or refactor unused features to prevent conflicts with VOICEVOX.
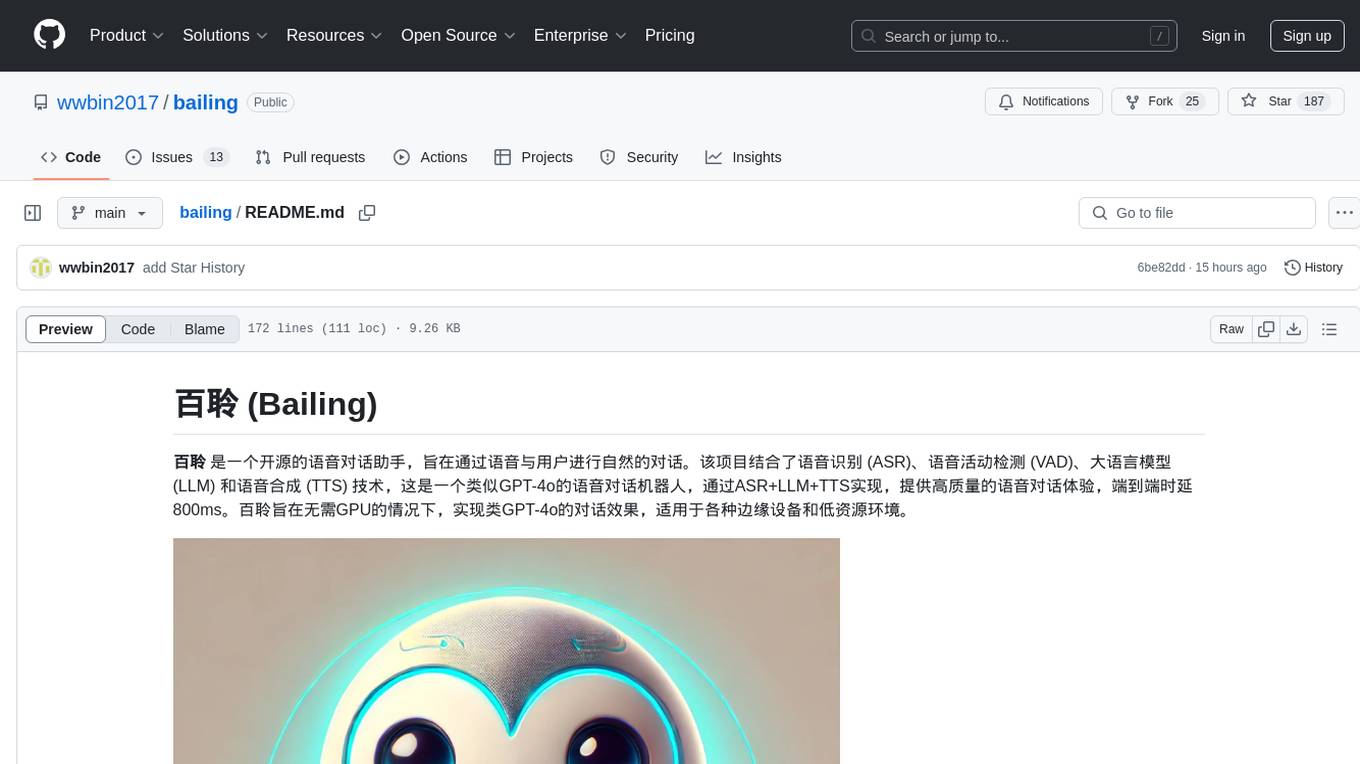
bailing
Bailing is an open-source voice assistant designed for natural conversations with users. It combines Automatic Speech Recognition (ASR), Voice Activity Detection (VAD), Large Language Model (LLM), and Text-to-Speech (TTS) technologies to provide a high-quality voice interaction experience similar to GPT-4o. Bailing aims to achieve GPT-4o-like conversation effects without the need for GPU, making it suitable for various edge devices and low-resource environments. The project features efficient open-source models, modular design allowing for module replacement and upgrades, support for memory function, tool integration for information retrieval and task execution via voice commands, and efficient task management with progress tracking and reminders.
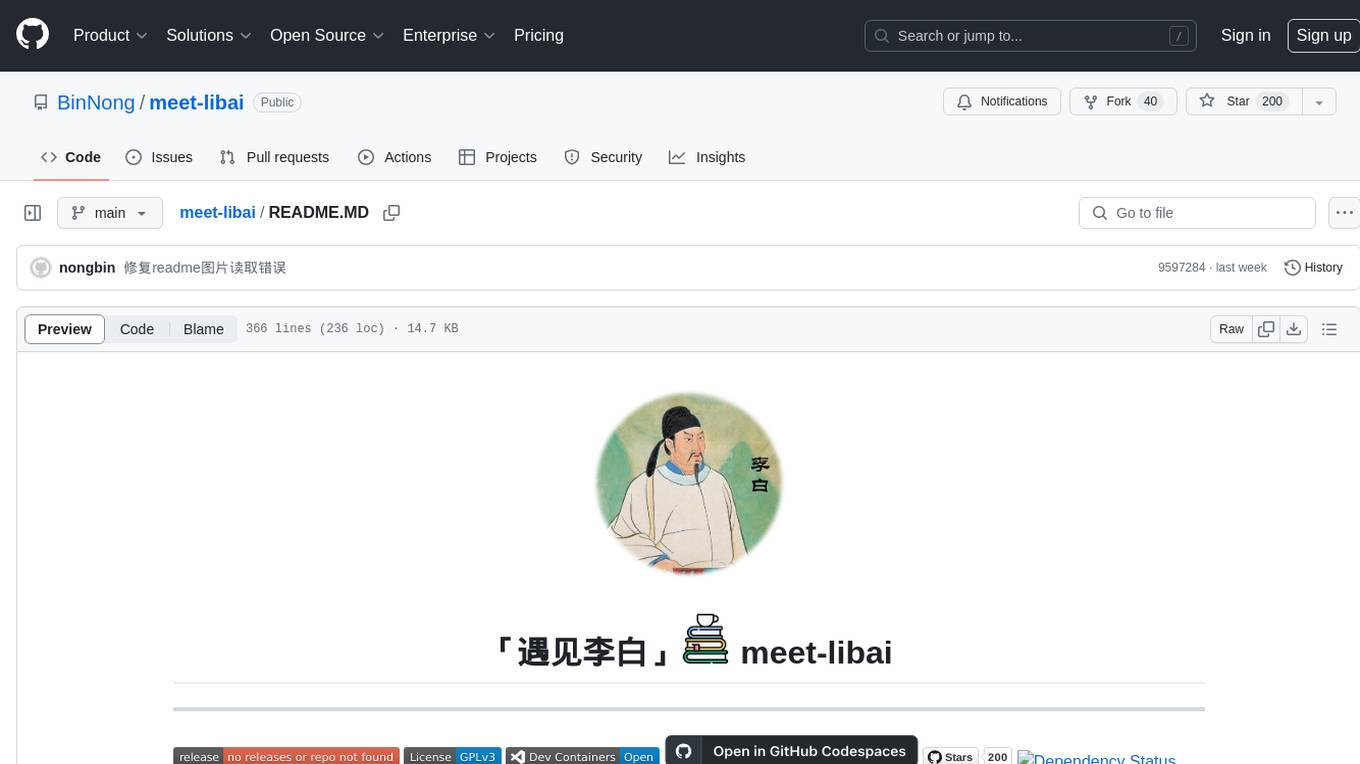
meet-libai
The 'meet-libai' project aims to promote and popularize the cultural heritage of the Chinese poet Li Bai by constructing a knowledge graph of Li Bai and training a professional AI intelligent body using large models. The project includes features such as data preprocessing, knowledge graph construction, question-answering system development, and visualization exploration of the graph structure. It also provides code implementations for large models and RAG retrieval enhancement.
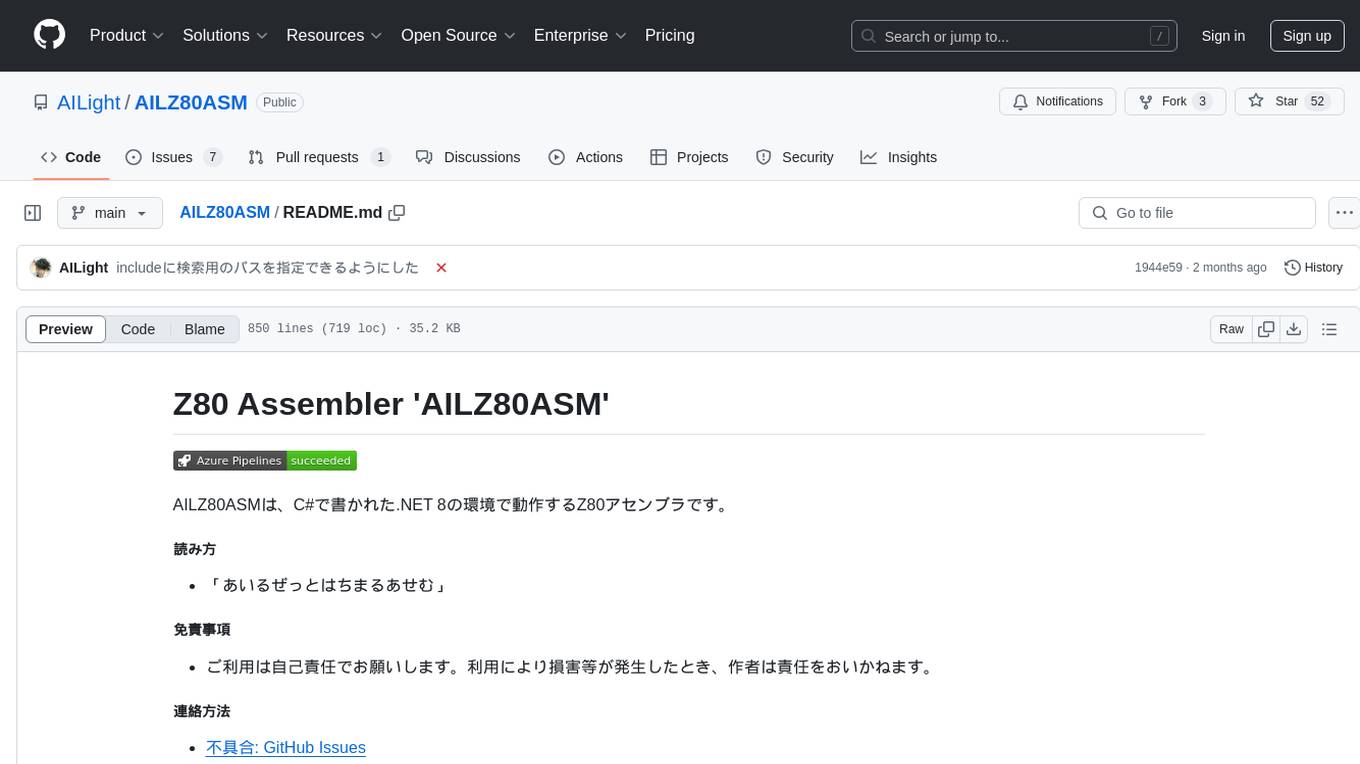
AILZ80ASM
AILZ80ASM is a Z80 assembler that runs in a .NET 8 environment written in C#. It can be used to assemble Z80 assembly code and generate output files in various formats. The tool supports various command-line options for customization and provides features like macros, conditional assembly, and error checking. AILZ80ASM offers good performance metrics with fast assembly times and efficient output file sizes. It also includes support for handling different file encodings and provides a range of built-in functions for working with labels, expressions, and data types.
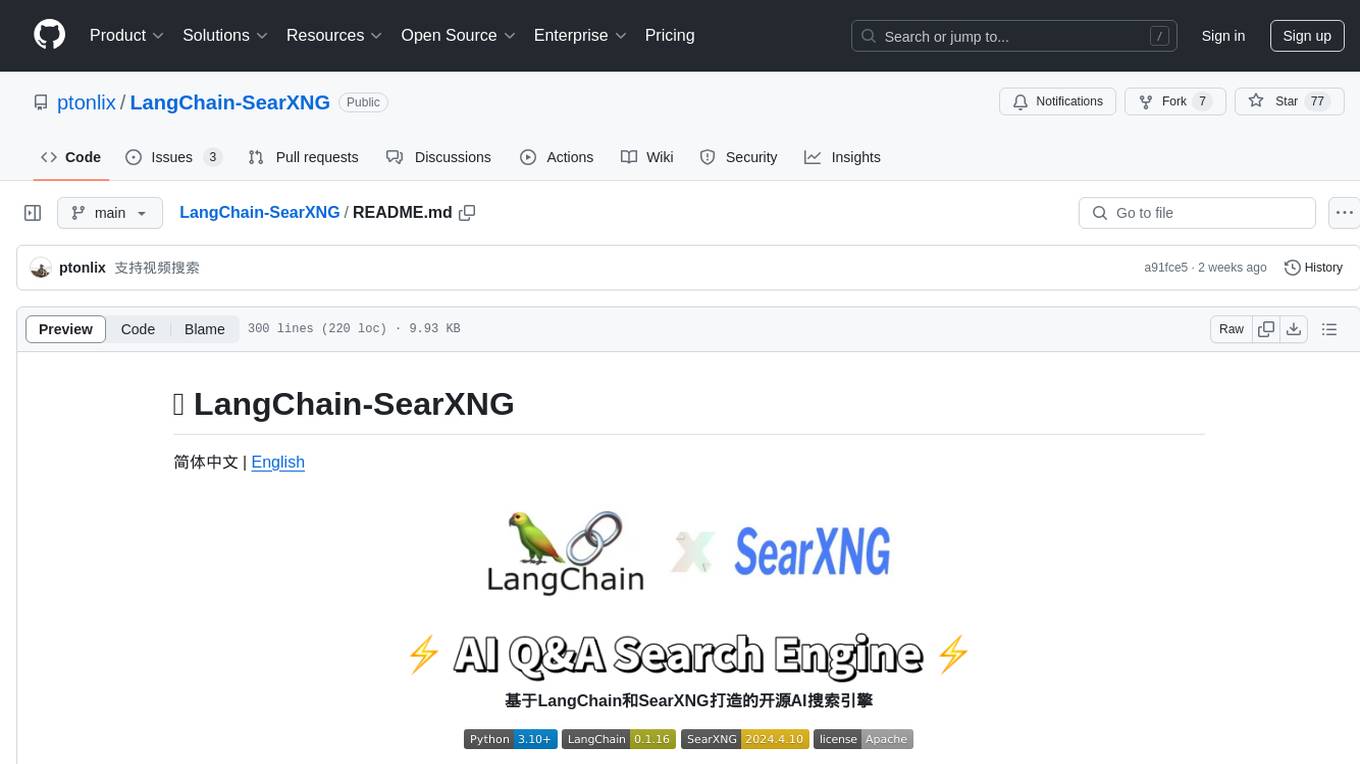
LangChain-SearXNG
LangChain-SearXNG is an open-source AI search engine built on LangChain and SearXNG. It supports faster and more accurate search and question-answering functionalities. Users can deploy SearXNG and set up Python environment to run LangChain-SearXNG. The tool integrates AI models like OpenAI and ZhipuAI for search queries. It offers two search modes: Searxng and ZhipuWebSearch, allowing users to control the search workflow based on input parameters. LangChain-SearXNG v2 version enhances response speed and content quality compared to the previous version, providing a detailed configuration guide and showcasing the effectiveness of different search modes through comparisons.
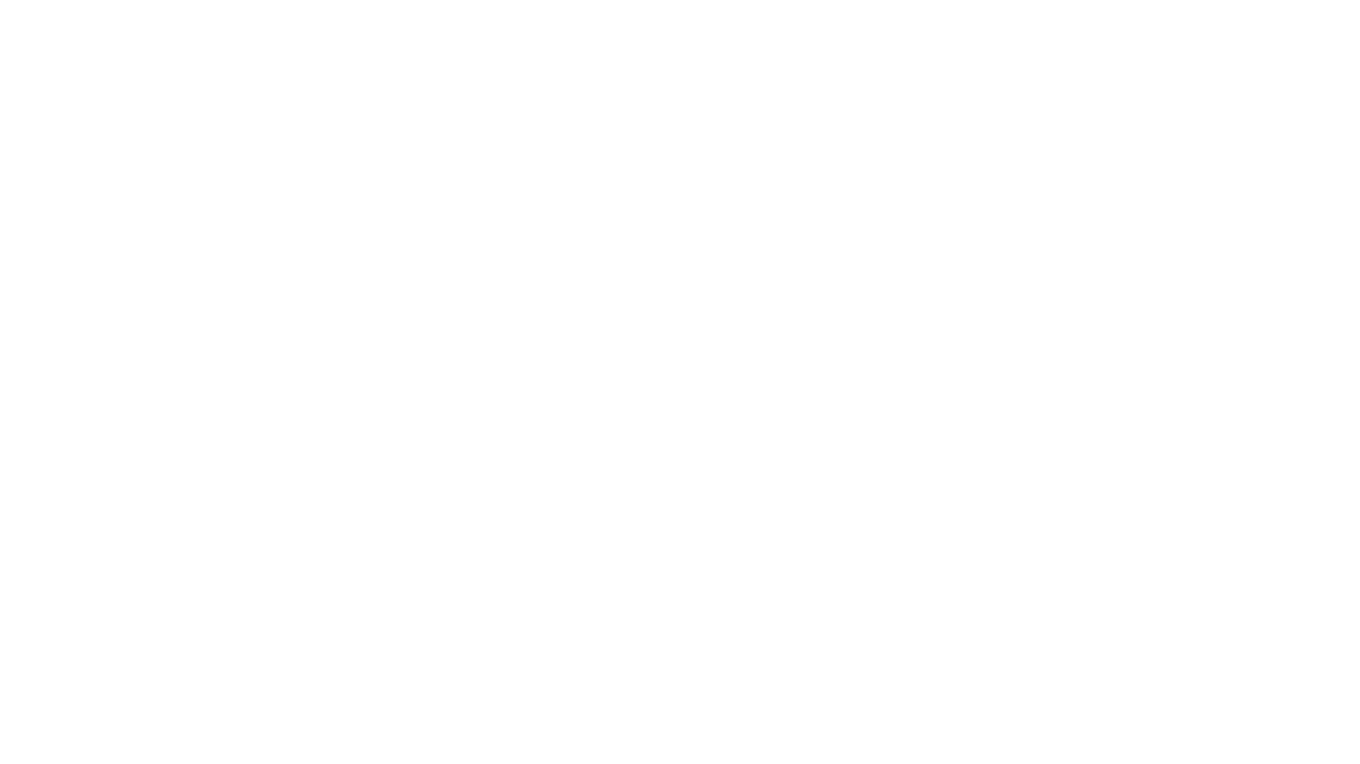
xlings
Xlings is a developer tool for programming learning, development, and course building. It provides features such as software installation, one-click environment setup, project dependency management, and cross-platform language package management. Additionally, it offers real-time compilation and running, AI code suggestions, tutorial project creation, automatic code checking for practice, and demo examples collection.
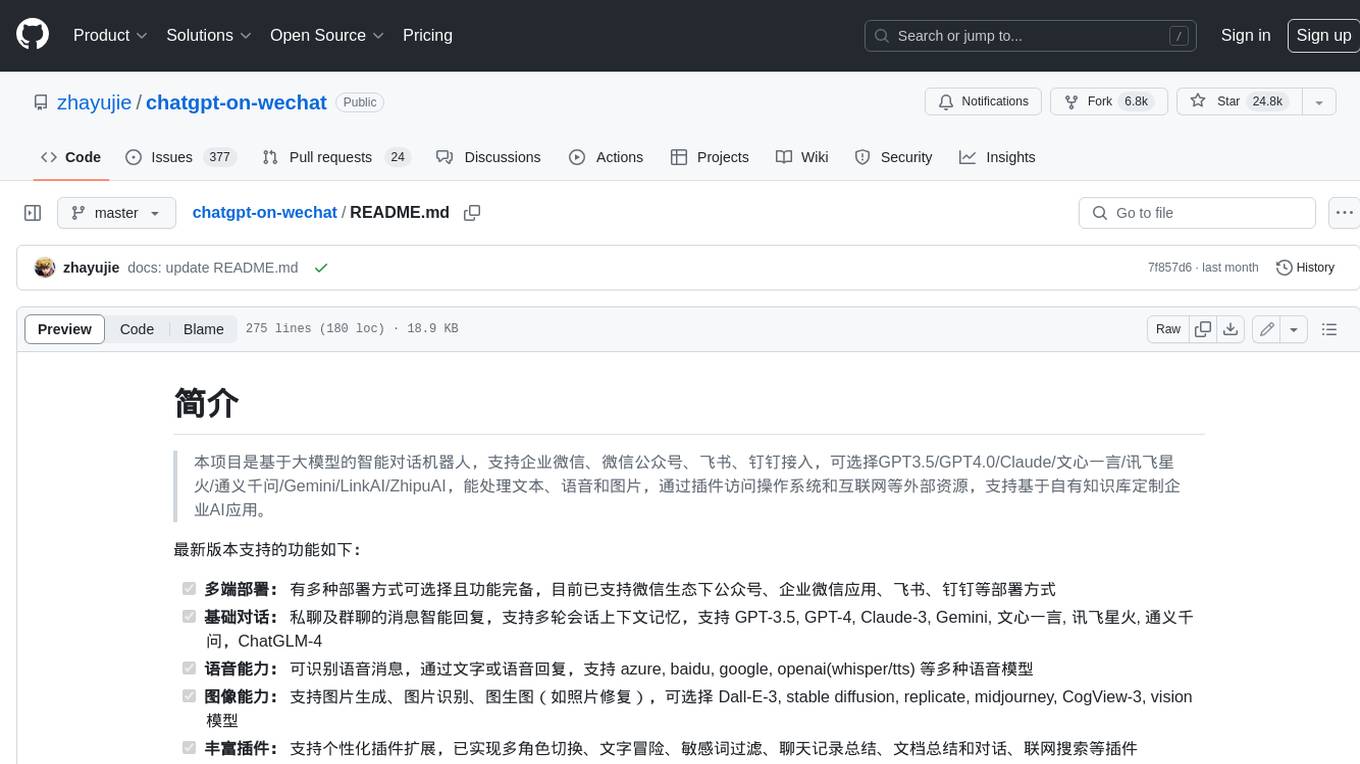
chatgpt-on-wechat
This project is a smart chatbot based on a large model, supporting WeChat, WeChat Official Account, Feishu, and DingTalk access. You can choose from GPT3.5/GPT4.0/Claude/Wenxin Yanyi/Xunfei Xinghuo/Tongyi Qianwen/Gemini/LinkAI/ZhipuAI, which can process text, voice, and images, and access external resources such as operating systems and the Internet through plugins, supporting the development of enterprise AI applications based on proprietary knowledge bases.
For similar tasks
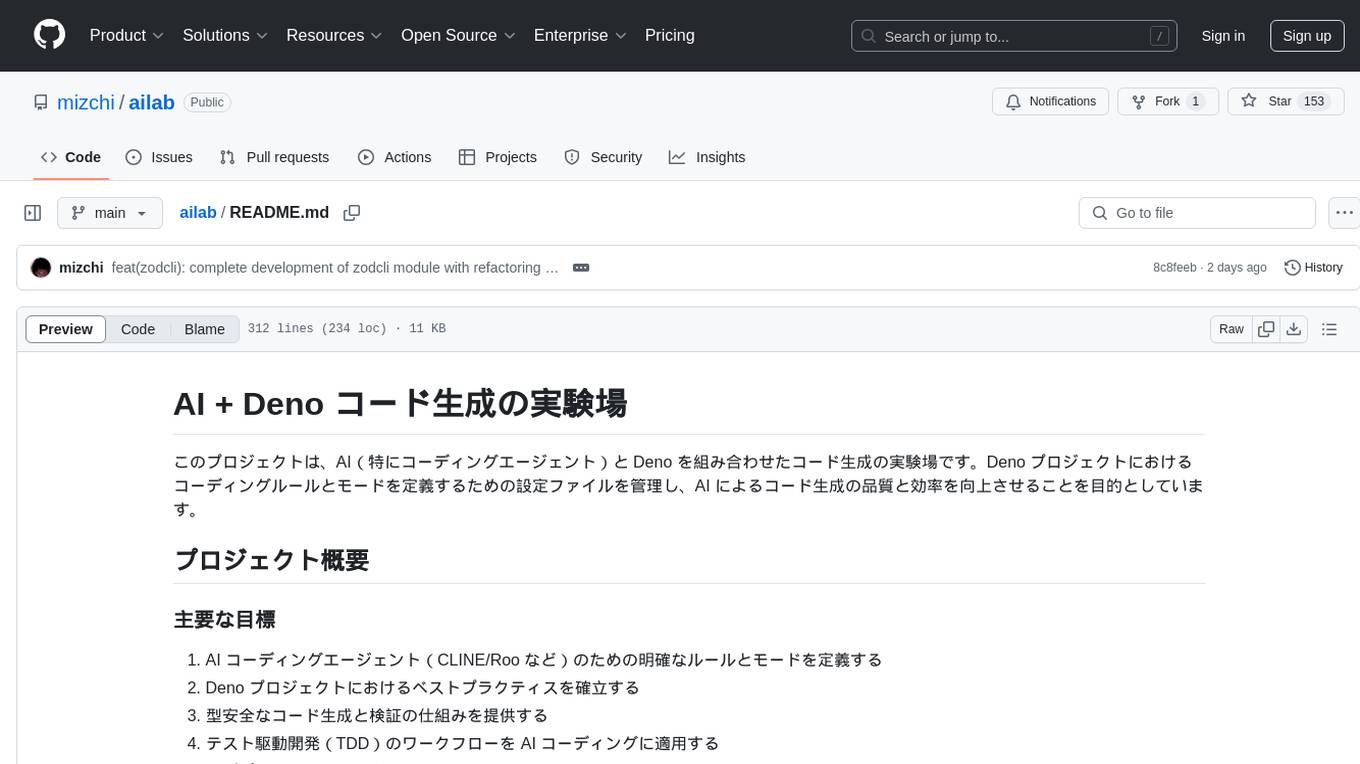
ailab
The 'ailab' project is an experimental ground for code generation combining AI (especially coding agents) and Deno. It aims to manage configuration files defining coding rules and modes in Deno projects, enhancing the quality and efficiency of code generation by AI. The project focuses on defining clear rules and modes for AI coding agents, establishing best practices in Deno projects, providing mechanisms for type-safe code generation and validation, applying test-driven development (TDD) workflow to AI coding, and offering implementation examples utilizing design patterns like adapter pattern.
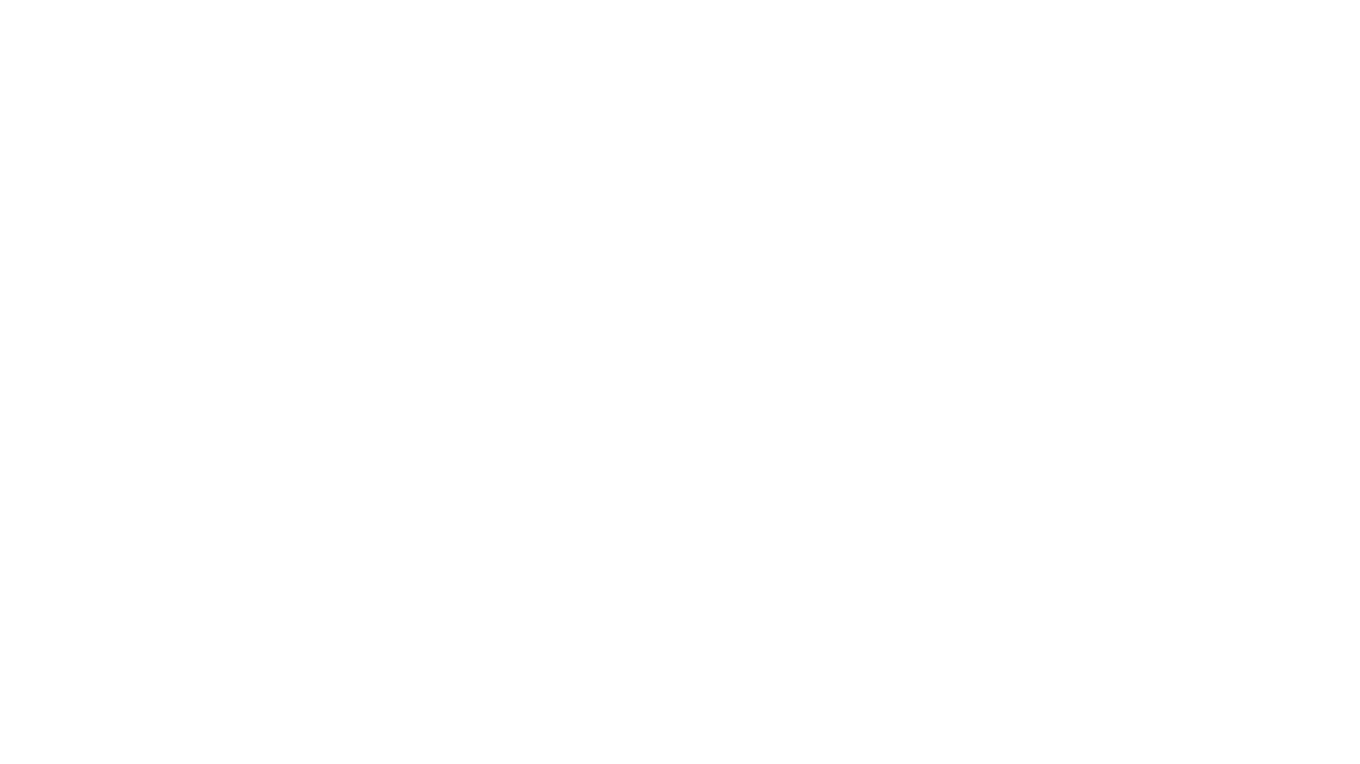
strictjson
Strict JSON is a framework designed to handle JSON outputs with complex structures, fixing issues that standard json.loads() cannot resolve. It provides functionalities for parsing LLM outputs into dictionaries, supporting various data types, type forcing, and error correction. The tool allows easy integration with OpenAI JSON Mode and offers community support through tutorials and discussions. Users can download the package via pip, set up API keys, and import functions for usage. The tool works by extracting JSON values using regex, matching output values to literals, and ensuring all JSON fields are output by LLM with optional type checking. It also supports LLM-based checks for type enforcement and error correction loops.
For similar jobs
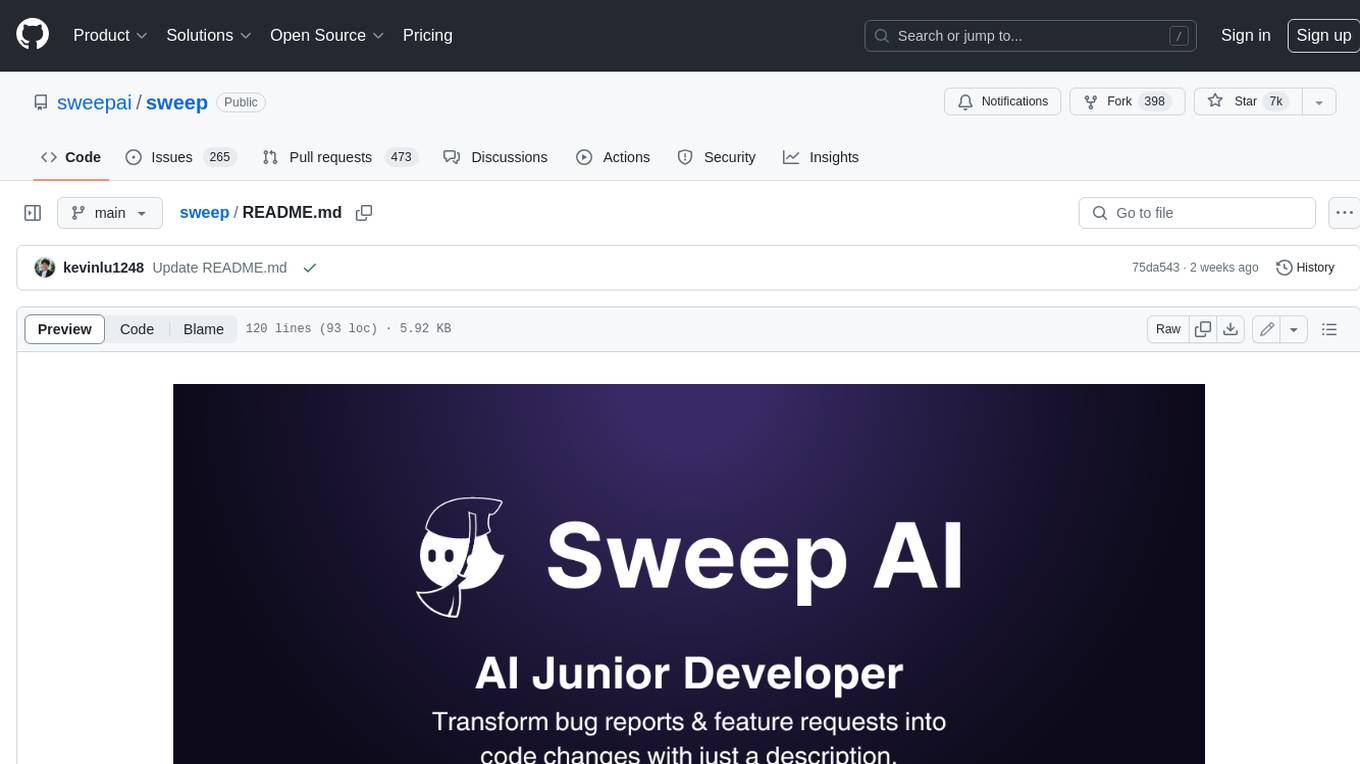
sweep
Sweep is an AI junior developer that turns bugs and feature requests into code changes. It automatically handles developer experience improvements like adding type hints and improving test coverage.
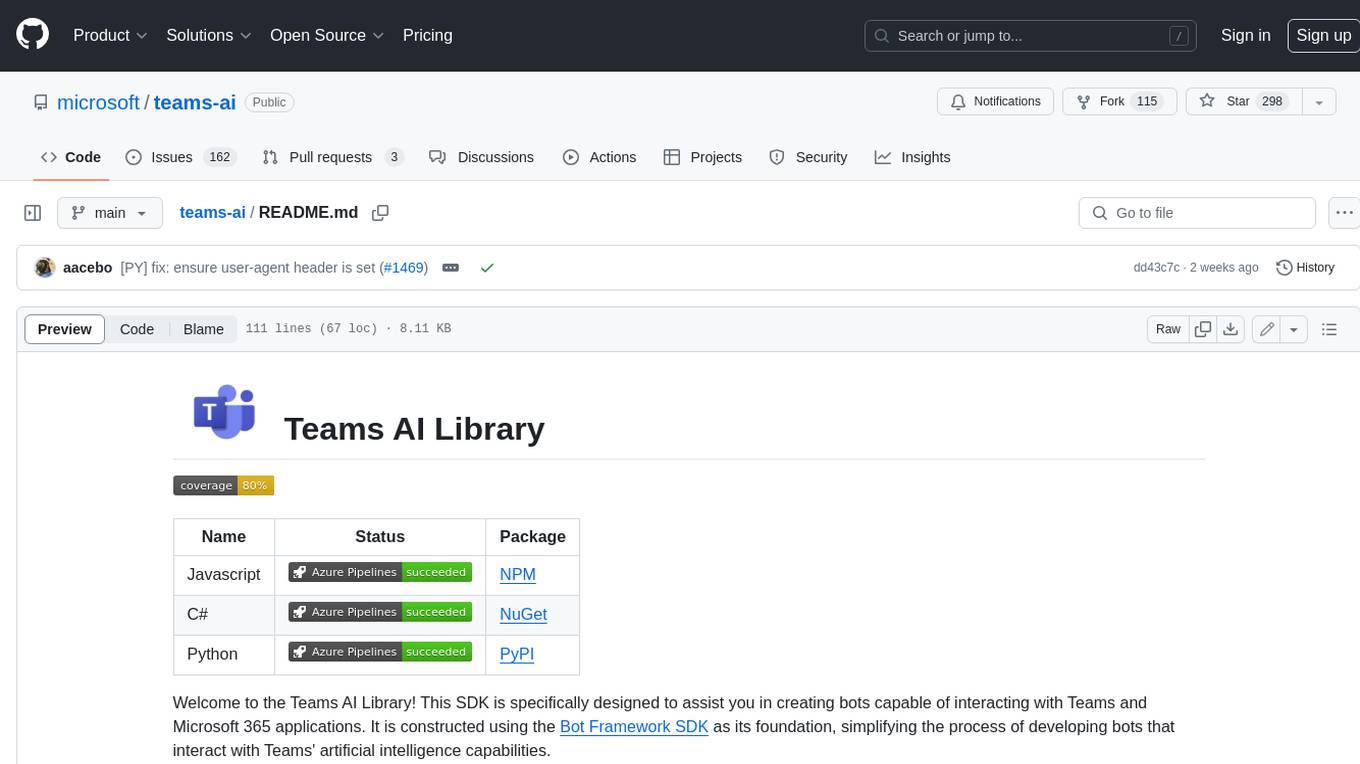
teams-ai
The Teams AI Library is a software development kit (SDK) that helps developers create bots that can interact with Teams and Microsoft 365 applications. It is built on top of the Bot Framework SDK and simplifies the process of developing bots that interact with Teams' artificial intelligence capabilities. The SDK is available for JavaScript/TypeScript, .NET, and Python.
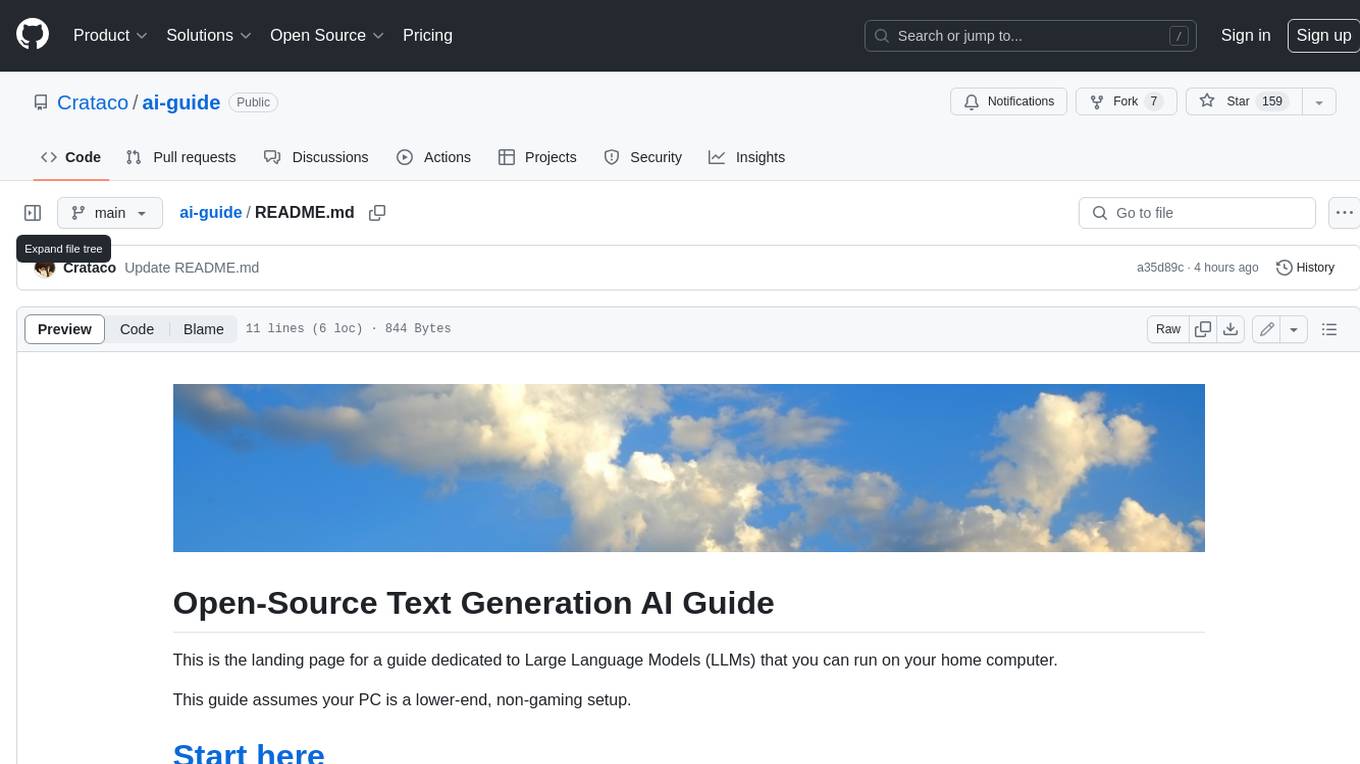
ai-guide
This guide is dedicated to Large Language Models (LLMs) that you can run on your home computer. It assumes your PC is a lower-end, non-gaming setup.
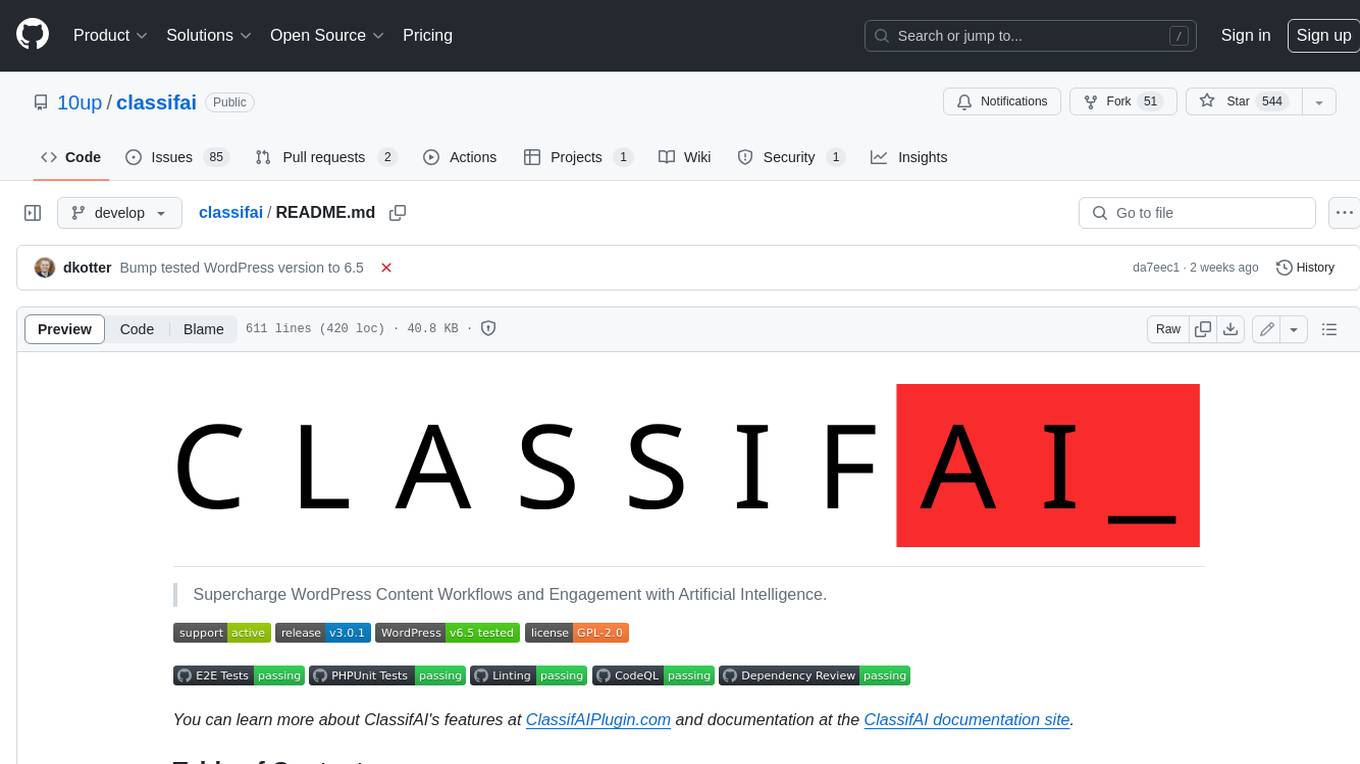
classifai
Supercharge WordPress Content Workflows and Engagement with Artificial Intelligence. Tap into leading cloud-based services like OpenAI, Microsoft Azure AI, Google Gemini and IBM Watson to augment your WordPress-powered websites. Publish content faster while improving SEO performance and increasing audience engagement. ClassifAI integrates Artificial Intelligence and Machine Learning technologies to lighten your workload and eliminate tedious tasks, giving you more time to create original content that matters.
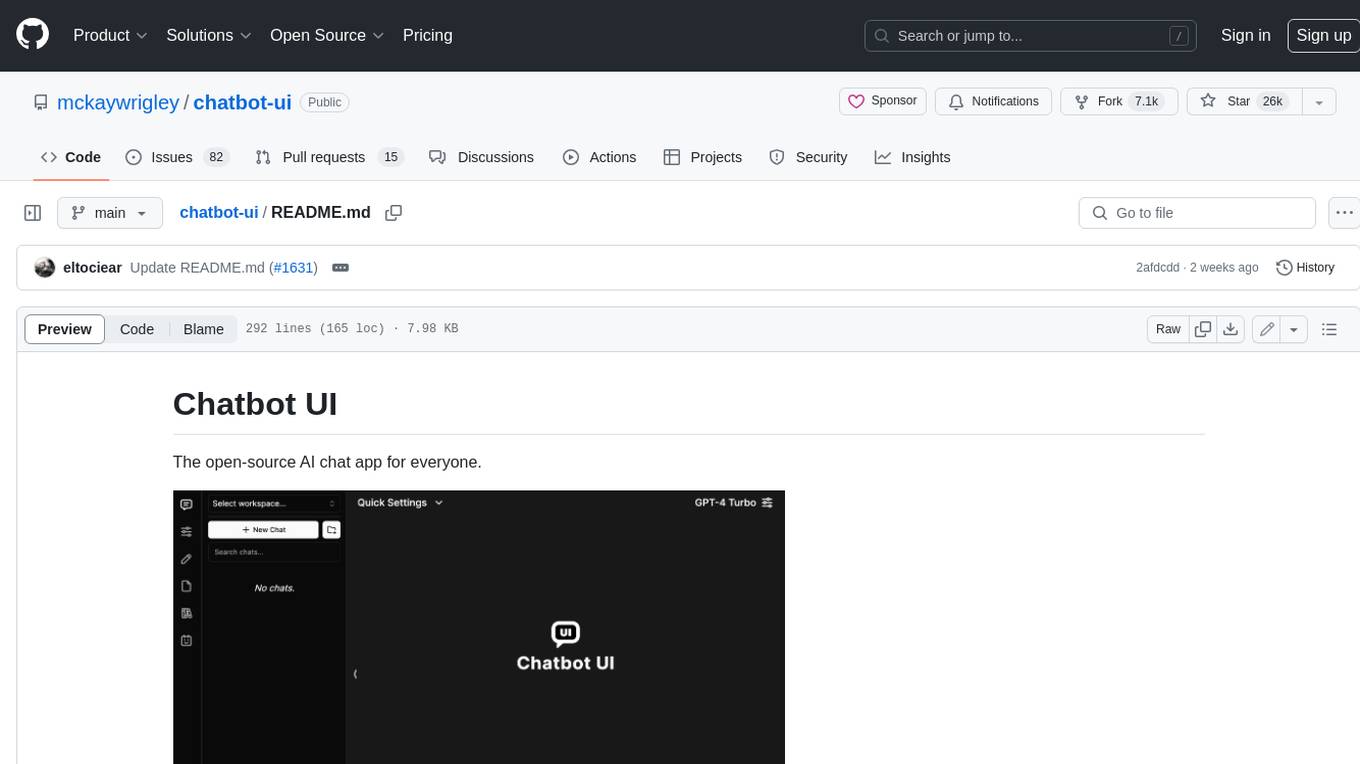
chatbot-ui
Chatbot UI is an open-source AI chat app that allows users to create and deploy their own AI chatbots. It is easy to use and can be customized to fit any need. Chatbot UI is perfect for businesses, developers, and anyone who wants to create a chatbot.
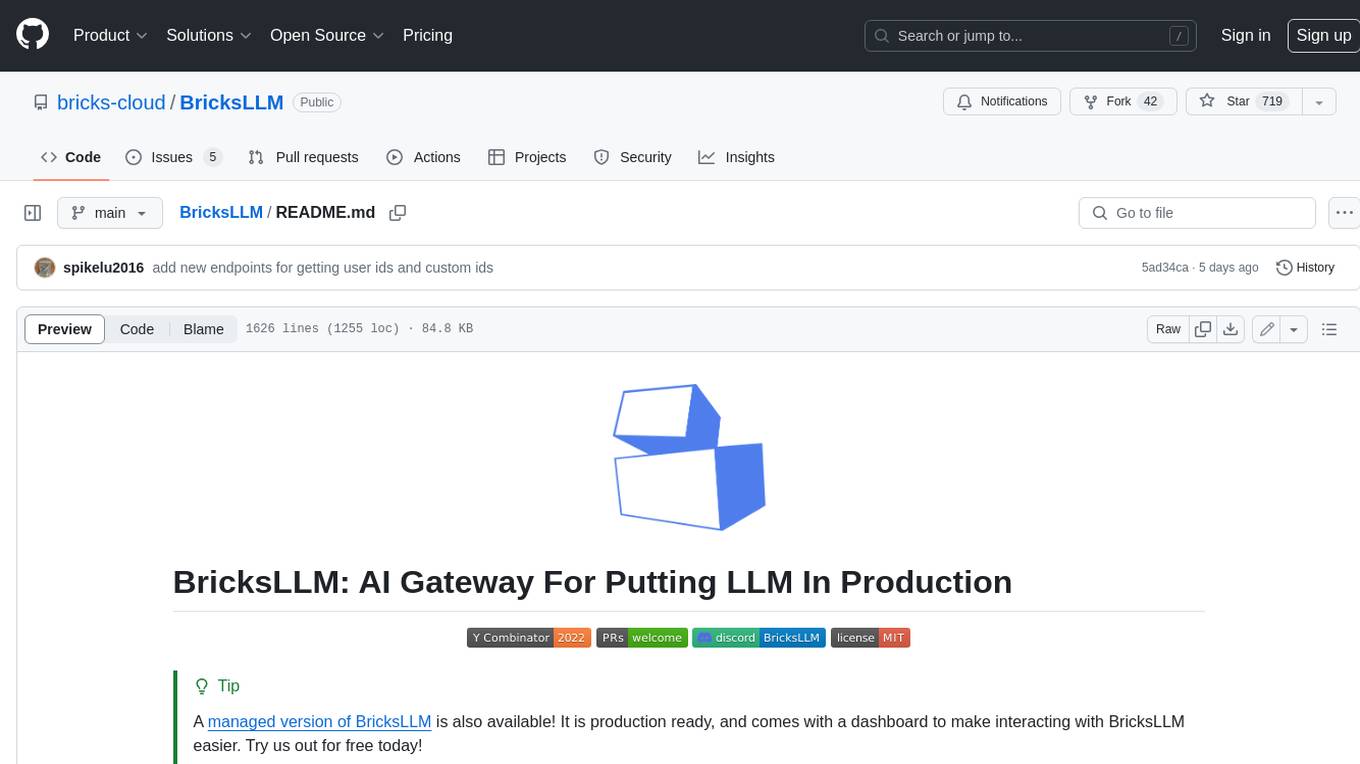
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
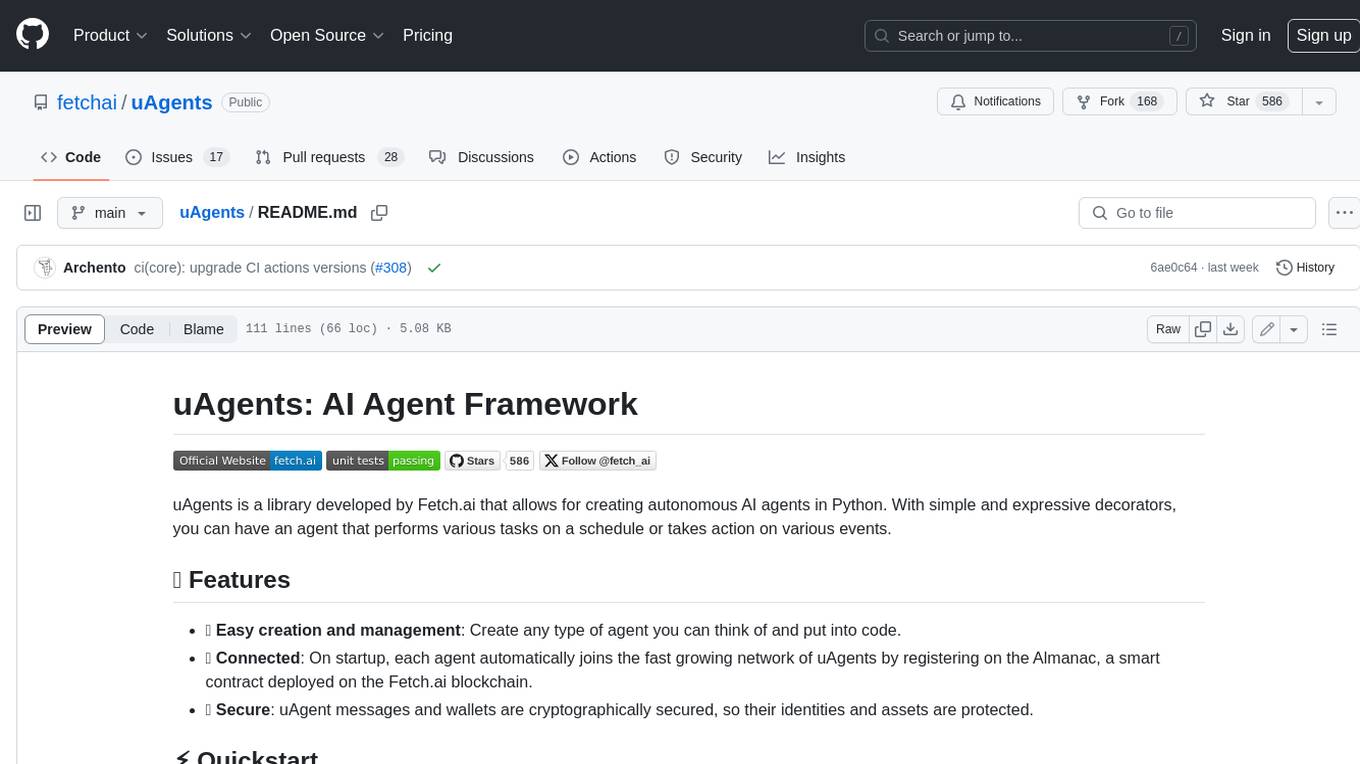
uAgents
uAgents is a Python library developed by Fetch.ai that allows for the creation of autonomous AI agents. These agents can perform various tasks on a schedule or take action on various events. uAgents are easy to create and manage, and they are connected to a fast-growing network of other uAgents. They are also secure, with cryptographically secured messages and wallets.
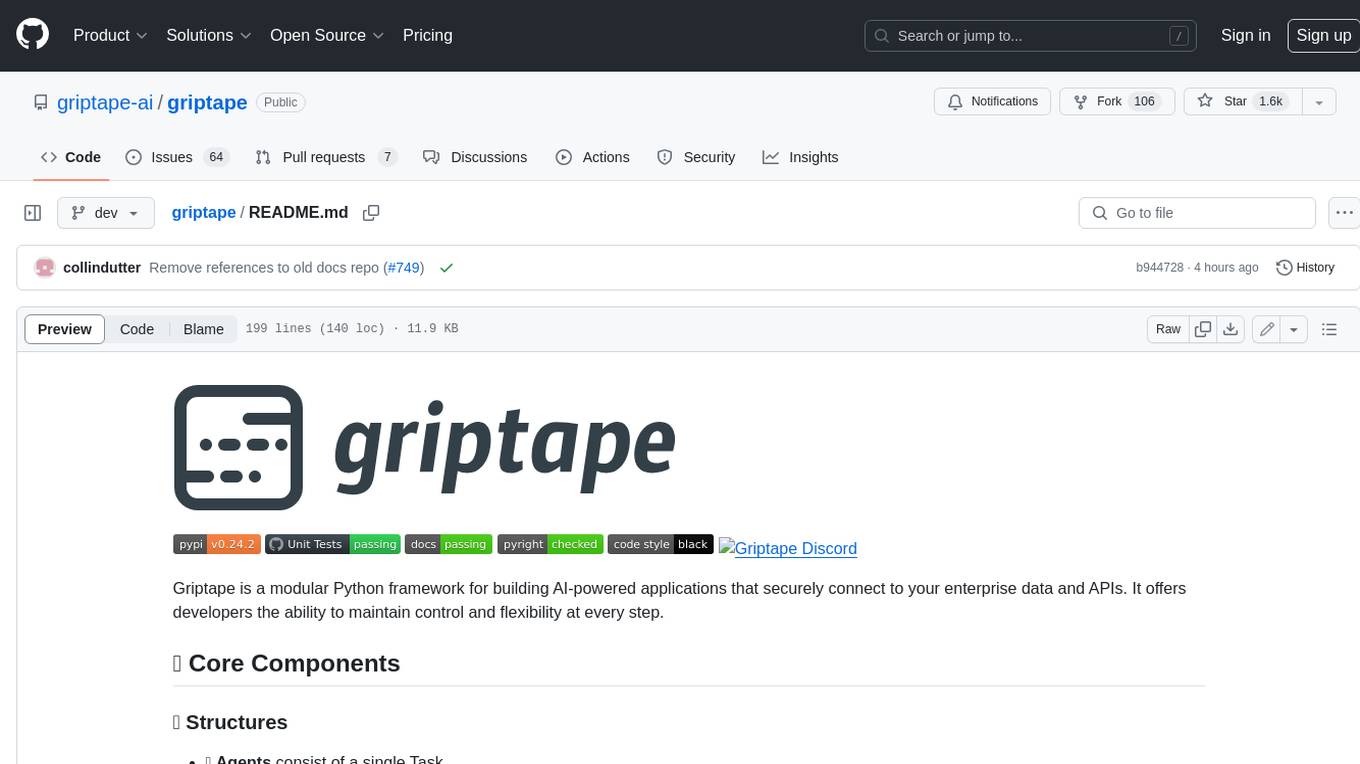
griptape
Griptape is a modular Python framework for building AI-powered applications that securely connect to your enterprise data and APIs. It offers developers the ability to maintain control and flexibility at every step. Griptape's core components include Structures (Agents, Pipelines, and Workflows), Tasks, Tools, Memory (Conversation Memory, Task Memory, and Meta Memory), Drivers (Prompt and Embedding Drivers, Vector Store Drivers, Image Generation Drivers, Image Query Drivers, SQL Drivers, Web Scraper Drivers, and Conversation Memory Drivers), Engines (Query Engines, Extraction Engines, Summary Engines, Image Generation Engines, and Image Query Engines), and additional components (Rulesets, Loaders, Artifacts, Chunkers, and Tokenizers). Griptape enables developers to create AI-powered applications with ease and efficiency.