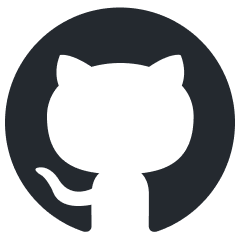
strictjson
A Strict JSON Framework for LLM Outputs
Stars: 214
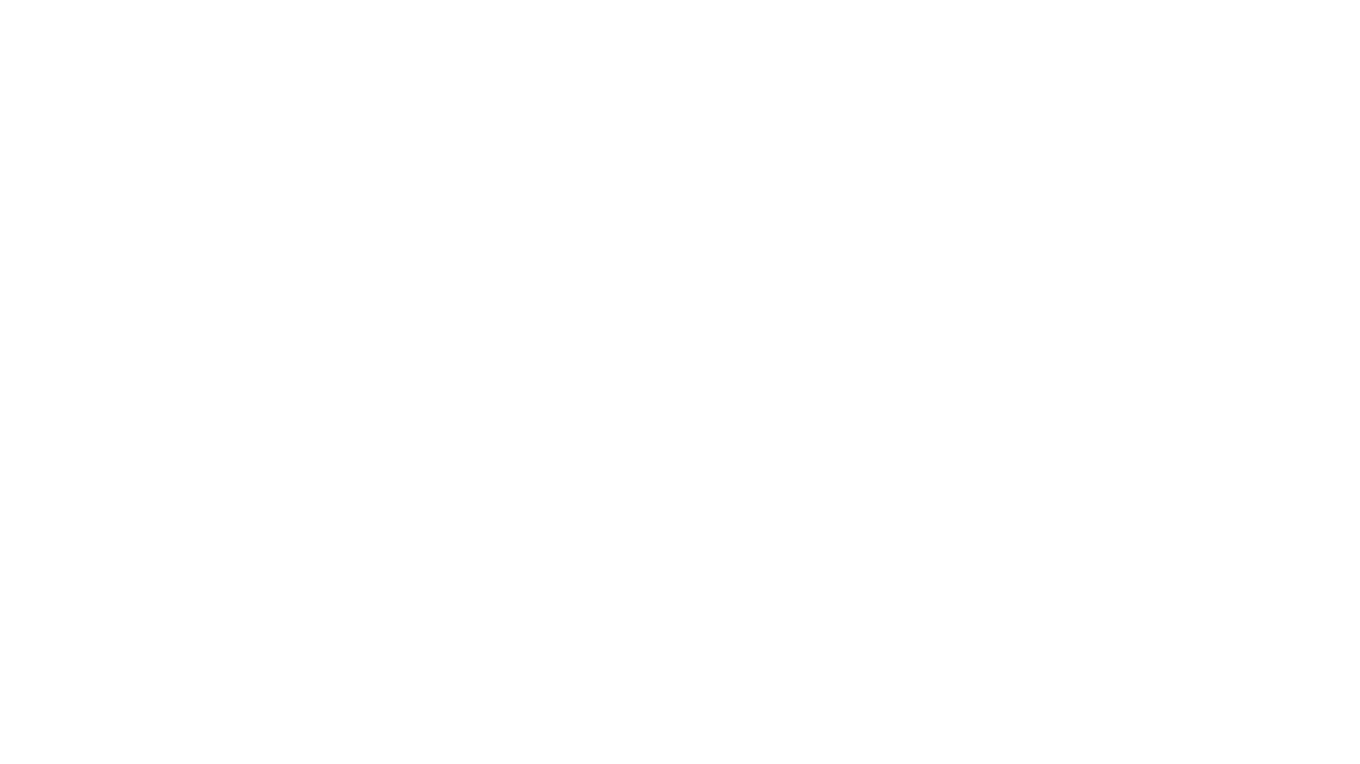
Strict JSON is a framework designed to handle JSON outputs with complex structures, fixing issues that standard json.loads() cannot resolve. It provides functionalities for parsing LLM outputs into dictionaries, supporting various data types, type forcing, and error correction. The tool allows easy integration with OpenAI JSON Mode and offers community support through tutorials and discussions. Users can download the package via pip, set up API keys, and import functions for usage. The tool works by extracting JSON values using regex, matching output values to literals, and ensuring all JSON fields are output by LLM with optional type checking. It also supports LLM-based checks for type enforcement and error correction loops.
README:
[UPDATE]: For Agentic Framework, do check out TaskGen (the official Agentic Framework building on StrictJSON). This will make the StrictJSON repo neater and this github will focus on using StrictJSON for LLM Output Parsing
- Works for JSON outputs with multiple ' or " or { or } or \ or unmatched braces/brackets that may break a json.loads()
- Ensures LLM outputs into a dictionary based on a JSON format (HUGE: Nested lists and dictionaries now supported)
- Supports
int
,float
,str
,dict
,list
,array
,code
,Dict[]
,List[]
,Enum[]
,bool
type forcing with LLM-based error correction, as well as LLM-based error correction usingtype: ensure <restriction>
, and (advanced) custom user checks usingcustom_checks
- Easy construction of LLM-based functions using
Function
(Note: renamed fromstrict_function
to keep in line with naming convention of capitalised class groups.strict_function
still works for legacy support.) - Easy integration with OpenAI JSON Mode by setting
openai_json_mode = True
- Exposing of llm variable for
strict_json
andFunction
for easy use of self-defined LLMs -
AsyncFunction
andstrict_json_async
for async (and faster) processing
- Created: 7 Apr 2023
- Collaborators welcome
- Video tutorial (Ask Me Anything): https://www.youtube.com/watch?v=L4aytve5v1Q
- Video tutorial: https://www.youtube.com/watch?v=IjTUKAciTCg
- Discussion Channel (my discord - John's AI Group): discord.gg/bzp87AHJy5
- Download package via command line
pip install strictjson
- Import the required functions from
strictjson
- Set up the relevant API Keys for your LLM if needed. Refer to
Tutorial.ipynb
for how to do it for Jupyter Notebooks.
- Extract JSON values as a string using a special regex (add delimiters to
key
to make###key###
) to split keys and values. (New!) Also works for nested datatypes by splitting recursively. - Uses
ast.literal_eval
to best match the extracted output value to a literal (e.g. int, string, dict). - Ensures that all JSON fields are output by LLM, with optional type checking, if not it will feed in error message to LLM to iteratively correct its generation (default: 3 tries)
- system_prompt: Write in whatever you want the LLM to become. "You are a <purpose in life>"
- user_prompt: The user input. Later, when we use it as a function, this is the function input
-
output_format: JSON of output variables in a dictionary, with the key as the output key, and the value as the output description
- The output keys will be preserved exactly, while the LLM will generate content to match the description of the value as best as possible
-
llm: The llm you want to use. Takes in
system_prompt
anduser_prompt
and outputs the LLM-generated string
def llm(system_prompt: str, user_prompt: str) -> str:
''' Here, we use OpenAI for illustration, you can change it to your own LLM '''
# ensure your LLM imports are all within this function
from openai import OpenAI
# define your own LLM here
client = OpenAI()
response = client.chat.completions.create(
model='gpt-4o-mini',
temperature = 0,
messages=[
{"role": "system", "content": system_prompt},
{"role": "user", "content": user_prompt}
]
)
return response.choices[0].message.content
res = strict_json(system_prompt = 'You are a classifier',
user_prompt = 'It is a beautiful and sunny day',
output_format = {'Sentiment': 'Type of Sentiment',
'Adjectives': 'Array of adjectives',
'Words': 'Number of words'},
llm = llm)
print(res)
{'Sentiment': 'Positive', 'Adjectives': ['beautiful', 'sunny'], 'Words': 7}
- More advanced demonstration involving code that would typically break
json.loads()
res = strict_json(system_prompt = 'You are a code generator, generating code to fulfil a task',
user_prompt = 'Given array p, output a function named func_sum to return its sum',
output_format = {'Elaboration': 'How you would do it',
'C': 'Code',
'Python': 'Code'},
llm = llm)
print(res)
{'Elaboration': 'Use a loop to iterate through each element in the array and add it to a running total.',
'C': 'int func_sum(int p[], int size) {\n int sum = 0;\n for (int i = 0; i < size; i++) {\n sum += p[i];\n }\n return sum;\n}',
'Python': 'def func_sum(p):\n sum = 0\n for num in p:\n sum += num\n return sum'}
- Generally,
strict_json
will infer the data type automatically for you for the output fields - However, if you would like very specific data types, you can do data forcing using
type: <data_type>
at the last part of the output field description -
<data_type>
must be of the formint
,float
,str
,dict
,list
,array
,code
,Dict[]
,List[]
,Array[]
,Enum[]
,bool
for type checking to work -
code
removes all unicode escape characters that might interfere with normal code running - The
Enum
andList
are not case sensitive, soenum
andlist
works just as well - For
Enum[list_of_category_names]
, it is best to give an "Other" category in case the LLM fails to classify correctly with the other options. - If
list
orList[]
is not formatted correctly in LLM's output, we will correct it by asking the LLM to list out the elements line by line - For
dict
, we can further check whether keys are present usingDict[list_of_key_names]
- Other types will first be forced by rule-based conversion, any further errors will be fed into LLM's error feedback mechanism
- If
<data_type>
is not the specified data types, it can still be useful to shape the output for the LLM. However, no type checking will be done. - Note: LLM understands the word
Array
better thanList
sinceArray
is the official JSON object type, so in the backend, any type with the wordList
will be converted toArray
.
- If you would like the LLM to ensure that the type is being met, use
type: ensure <requirement>
- This will run a LLM to check if the requirement is met. If requirement is not met, the LLM will generate what needs to be done to meet the requirement, which will be fed into the error-correcting loop of
strict_json
res = strict_json(system_prompt = 'You are a classifier',
user_prompt = 'It is a beautiful and sunny day',
output_format = {'Sentiment': 'Type of Sentiment, type: Enum["Pos", "Neg", "Other"]',
'Adjectives': 'Array of adjectives, type: List[str]',
'Words': 'Number of words, type: int',
'In English': 'Whether sentence is in English, type: bool'},
llm = llm)
print(res)
{'Sentiment': 'Pos', 'Adjectives': ['beautiful', 'sunny'], 'Words': 7, 'In English': True}
res = strict_json(system_prompt = 'You are an expert at organising birthday parties',
user_prompt = 'Give me some information on how to organise a birthday',
output_format = {'Famous Quote about Age': 'type: ensure quote contains the word age',
'Lucky draw numbers': '3 numbers from 1-50, type: List[int]',
'Sample venues': 'Describe two venues, type: List[Dict["Venue", "Description"]]'},
llm = llm)
print(res)
Using LLM to check "The secret of staying young is to live honestly, eat slowly, and lie about your age. - Lucille Ball" to see if it adheres to "quote contains the word age" Requirement Met: True
{'Famous Quote about Age': 'The secret of staying young is to live honestly, eat slowly, and lie about your age. - Lucille Ball',
'Lucky draw numbers': [7, 21, 35],
'Sample venues': [{'Venue': 'Beachside Resort', 'Description': 'A beautiful resort with stunning views of the beach. Perfect for a summer birthday party.'}, {'Venue': 'Indoor Trampoline Park', 'Description': 'An exciting venue with trampolines and fun activities. Ideal for an active and energetic birthday celebration.'}]}
-
Enhances
strict_json()
with a function-like interface for repeated use of modular LLM-based functions (or wraps external functions) -
Use angle brackets <> to enclose input variable names. First input variable name to appear in
fn_description
will be first input variable and second to appear will be second input variable. For example,fn_description = 'Adds up two numbers, <var1> and <var2>'
will result in a function with first input variablevar1
and second input variablevar2
-
(Optional) If you would like greater specificity in your function's input, you can describe the variable after the : in the input variable name, e.g.
<var1: an integer from 10 to 30>
. Here,var1
is the input variable andan integer from 10 to 30
is the description. -
(Optional) If your description of the variable is one of
int
,float
,str
,dict
,list
,array
,code
,Dict[]
,List[]
,Array[]
,Enum[]
,bool
, we will enforce type checking when generating the function inputs inget_next_subtask
method of theAgent
class. Example:<var1: int>
. Refer to Section 3. Type Forcing Output Variables for details. -
Inputs (primary):
-
fn_description: String. Function description to describe process of transforming input variables to output variables. Variables must be enclosed in <> and listed in order of appearance in function input.
- New feature: If
external_fn
is provided and nofn_description
is provided, then we will automatically parse out the fn_description based on docstring ofexternal_fn
. The docstring should contain the names of all compulsory input variables - New feature: If
external_fn
is provided and nooutput_format
is provided, then we will automatically derive theoutput_format
from the function signature
- New feature: If
- output_format: Dict. Dictionary containing output variables names and description for each variable.
-
fn_description: String. Function description to describe process of transforming input variables to output variables. Variables must be enclosed in <> and listed in order of appearance in function input.
-
Inputs (optional):
- examples - Dict or List[Dict]. Examples in Dictionary form with the input and output variables (list if more than one)
-
external_fn - Python Function. If defined, instead of using LLM to process the function, we will run the external function.
If there are multiple outputs of this function, we will map it to the keys of
output_format
in a one-to-one fashion -
fn_name - String. If provided, this will be the name of the function. Otherwise, if
external_fn
is provided, it will be the name ofexternal_fn
. Otherwise, we will use LLM to generate a function name from thefn_description
- kwargs - Dict. Additional arguments you would like to pass on to the strict_json function
-
Outputs: JSON of output variables in a dictionary (similar to
strict_json
)
# basic configuration with variable names (in order of appearance in fn_description)
fn = Function(fn_description = 'Output a sentence with <obj> and <entity> in the style of <emotion>',
output_format = {'output': 'sentence'},
llm = llm)
# Use the function
fn('ball', 'dog', 'happy') #obj, entity, emotion
{'output': 'The happy dog chased the ball.'}
# Construct the function: infer pattern from just examples without description (here it is multiplication)
fn = Function(fn_description = 'Map <var1> and <var2> to output based on examples',
output_format = {'output': 'final answer'},
examples = [{'var1': 3, 'var2': 2, 'output': 6},
{'var1': 5, 'var2': 3, 'output': 15},
{'var1': 7, 'var2': 4, 'output': 28}],
llm = llm)
# Use the function
fn(2, 10) #var1, var2
{'output': 20}
# Construct the function: description and examples with variable names
# variable names will be referenced in order of appearance in fn_description
fn = Function(fn_description = 'Output the sum and difference of <num1> and <num2>',
output_format = {'sum': 'sum of two numbers',
'difference': 'absolute difference of two numbers'},
examples = {'num1': 2, 'num2': 4, 'sum': 6, 'difference': 2},
llm = llm)
# Use the function
fn(3, 4) #num1, num2
{'sum': 7, 'difference': 1}
Example Usage 4 (External Function with automatic inference of fn_description and output_format - Preferred)
# Docstring should provide all input variables, otherwise we will add it in automatically
# We will ignore shared_variables, *args and **kwargs
# No need to define llm in Function for External Functions
from typing import List
def add_number_to_list(num1: int, num_list: List[int], *args, **kwargs) -> List[int]:
'''Adds num1 to num_list'''
num_list.append(num1)
return num_list
fn = Function(external_fn = add_number_to_list)
# Show the processed function docstring
print(str(fn))
# Use the function
fn(3, [2, 4, 5])
Description: Adds <num1: int> to <num_list: list>
Input: ['num1', 'num_list']
Output: {'num_list': 'Array of numbers'}
{'num_list': [2, 4, 5, 3]}
Example Usage 5 (External Function with manually defined fn_description and output_format - Legacy Approach)
def binary_to_decimal(x):
return int(str(x), 2)
# an external function with a single output variable, with an expressive variable description
fn = Function(fn_description = 'Convert input <x: a binary number in base 2> to base 10',
output_format = {'output1': 'x in base 10'},
external_fn = binary_to_decimal,
llm = llm)
# Use the function
fn(10) #x
{'output1': 2}
- If you want to use the OpenAI JSON Mode, you can simply add in
openai_json_mode = True
and setmodel = 'gpt-4-1106-preview'
ormodel = 'gpt-3.5-turbo-1106'
instrict_json
orFunction
- We will set model to
gpt-3.5-turbo-1106
by default if you provide an invalid model - This does not work with the
llm
variable - Note that type checking does not work with OpenAI JSON Mode
res = strict_json(system_prompt = 'You are a classifier',
user_prompt = 'It is a beautiful and sunny day',
output_format = {'Sentiment': 'Type of Sentiment',
'Adjectives': 'Array of adjectives',
'Words': 'Number of words'},
model = 'gpt-3.5-turbo-1106' # Set the model
openai_json_mode = True) # Toggle this to True
print(res)
{'Sentiment': 'positive', 'Adjectives': ['beautiful', 'sunny'], 'Words': 6}
- StrictJSON supports nested outputs like nested lists and dictionaries
res = strict_json(system_prompt = 'You are a classifier',
user_prompt = 'It is a beautiful and sunny day',
output_format = {'Sentiment': ['Type of Sentiment',
'Strength of Sentiment, type: Enum[1, 2, 3, 4, 5]'],
'Adjectives': "Name and Description as separate keys, type: List[Dict['Name', 'Description']]",
'Words': {
'Number of words': 'Word count',
'Language': {
'English': 'Whether it is English, type: bool',
'Chinese': 'Whether it is Chinese, type: bool'
},
'Proper Words': 'Whether the words are proper in the native language, type: bool'
}
},
llm = llm)
print(res)
{'Sentiment': ['Positive', 3],
'Adjectives': [{'Name': 'beautiful', 'Description': 'pleasing to the senses'}, {'Name': 'sunny', 'Description': 'filled with sunshine'}],
'Words':
{'Number of words': 6,
'Language': {'English': True, 'Chinese': False},
'Proper Words': True}
}
- By default,
strict_json
returns a Python Dictionary - If needed to parse as JSON, simply set
return_as_json=True
- By default, this is set to
False
in order to return a Python Dictionry
-
AsyncFunction
andstrict_json_async
- These are the async equivalents of
Function
andstrict_json
- You will need to define an LLM that can operate in async mode
- Everything is the same as the sync version of the functions, except you use the
await
keyword when callingAsyncFunction
andstrict_json_async
- These are the async equivalents of
-
Using Async can help do parallel processes simulataneously, resulting in a much faster workflow
async def llm_async(system_prompt: str, user_prompt: str):
''' Here, we use OpenAI for illustration, you can change it to your own LLM '''
# ensure your LLM imports are all within this function
from openai import AsyncOpenAI
# define your own LLM here
client = AsyncOpenAI()
response = await client.chat.completions.create(
model='gpt-4o-mini',
temperature = 0,
messages=[
{"role": "system", "content": system_prompt},
{"role": "user", "content": user_prompt}
]
)
return response.choices[0].message.content
res = await strict_json_async(system_prompt = 'You are a classifier',
user_prompt = 'It is a beautiful and sunny day',
output_format = {'Sentiment': 'Type of Sentiment',
'Adjectives': 'Array of adjectives',
'Words': 'Number of words'},
llm = llm_async) # set this to your own LLM
print(res)
{'Sentiment': 'Positive', 'Adjectives': ['beautiful', 'sunny'], 'Words': 7}
fn = AsyncFunction(fn_description = 'Output a sentence with <obj> and <entity> in the style of <emotion>',
output_format = {'output': 'sentence'},
llm = llm_async) # set this to your own LLM
res = await fn('ball', 'dog', 'happy') #obj, entity, emotion
print(res)
{'output': 'The dog happily chased the ball.'}
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for strictjson
Similar Open Source Tools
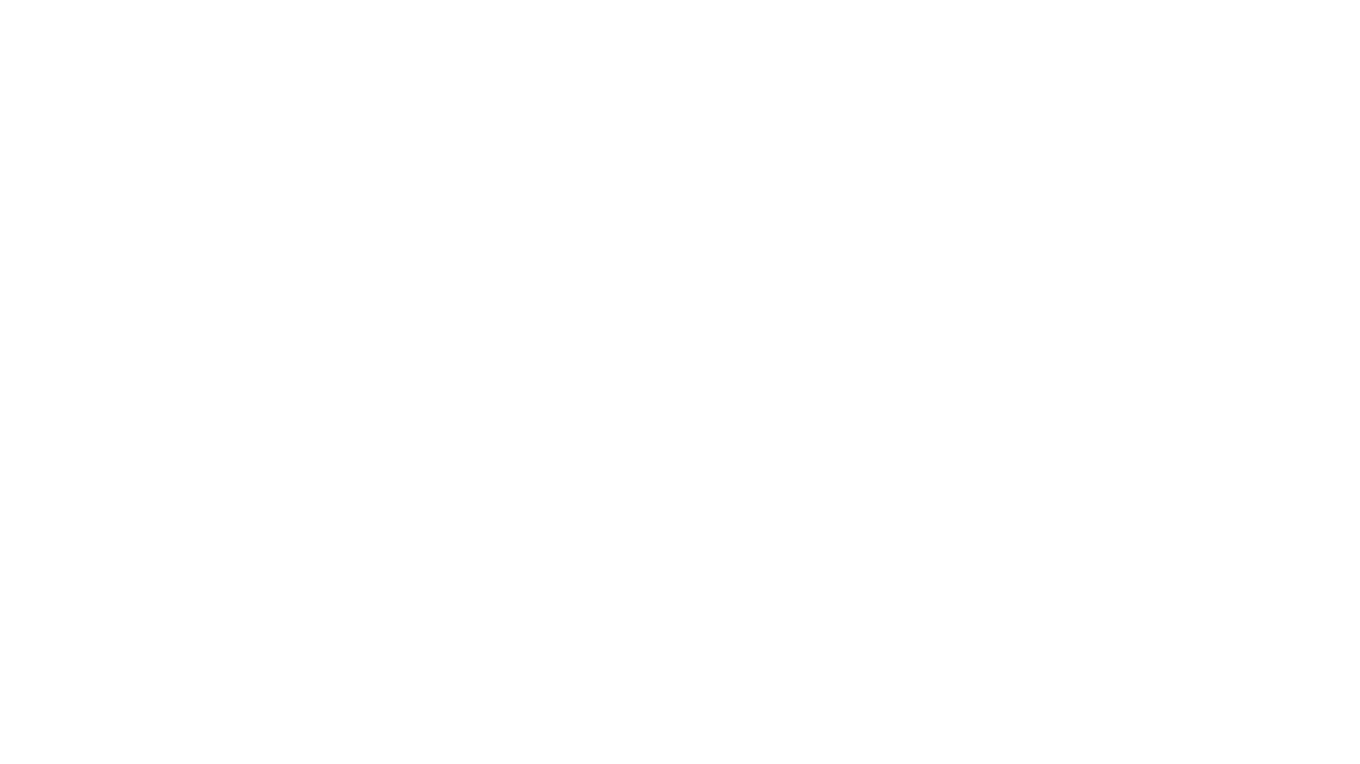
strictjson
Strict JSON is a framework designed to handle JSON outputs with complex structures, fixing issues that standard json.loads() cannot resolve. It provides functionalities for parsing LLM outputs into dictionaries, supporting various data types, type forcing, and error correction. The tool allows easy integration with OpenAI JSON Mode and offers community support through tutorials and discussions. Users can download the package via pip, set up API keys, and import functions for usage. The tool works by extracting JSON values using regex, matching output values to literals, and ensuring all JSON fields are output by LLM with optional type checking. It also supports LLM-based checks for type enforcement and error correction loops.
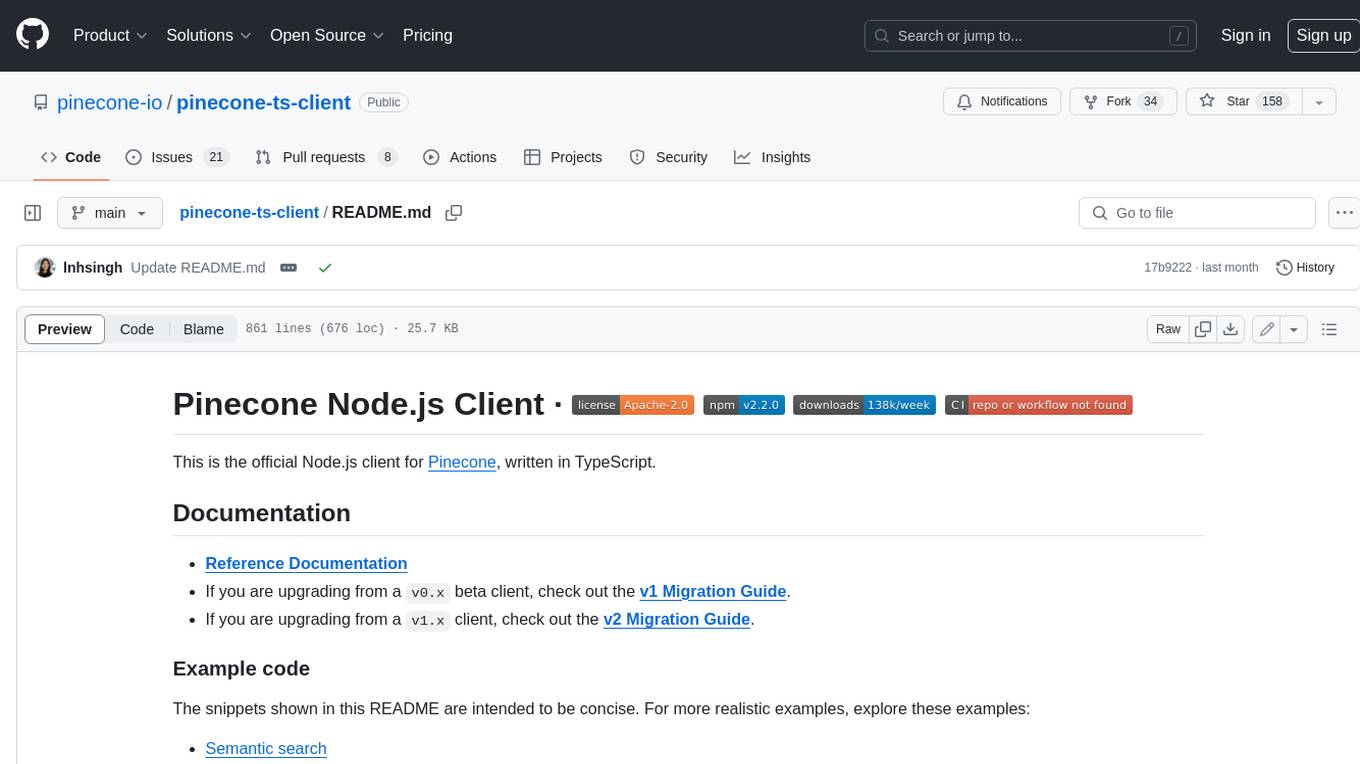
pinecone-ts-client
The official Node.js client for Pinecone, written in TypeScript. This client library provides a high-level interface for interacting with the Pinecone vector database service. With this client, you can create and manage indexes, upsert and query vector data, and perform other operations related to vector search and retrieval. The client is designed to be easy to use and provides a consistent and idiomatic experience for Node.js developers. It supports all the features and functionality of the Pinecone API, making it a comprehensive solution for building vector-powered applications in Node.js.
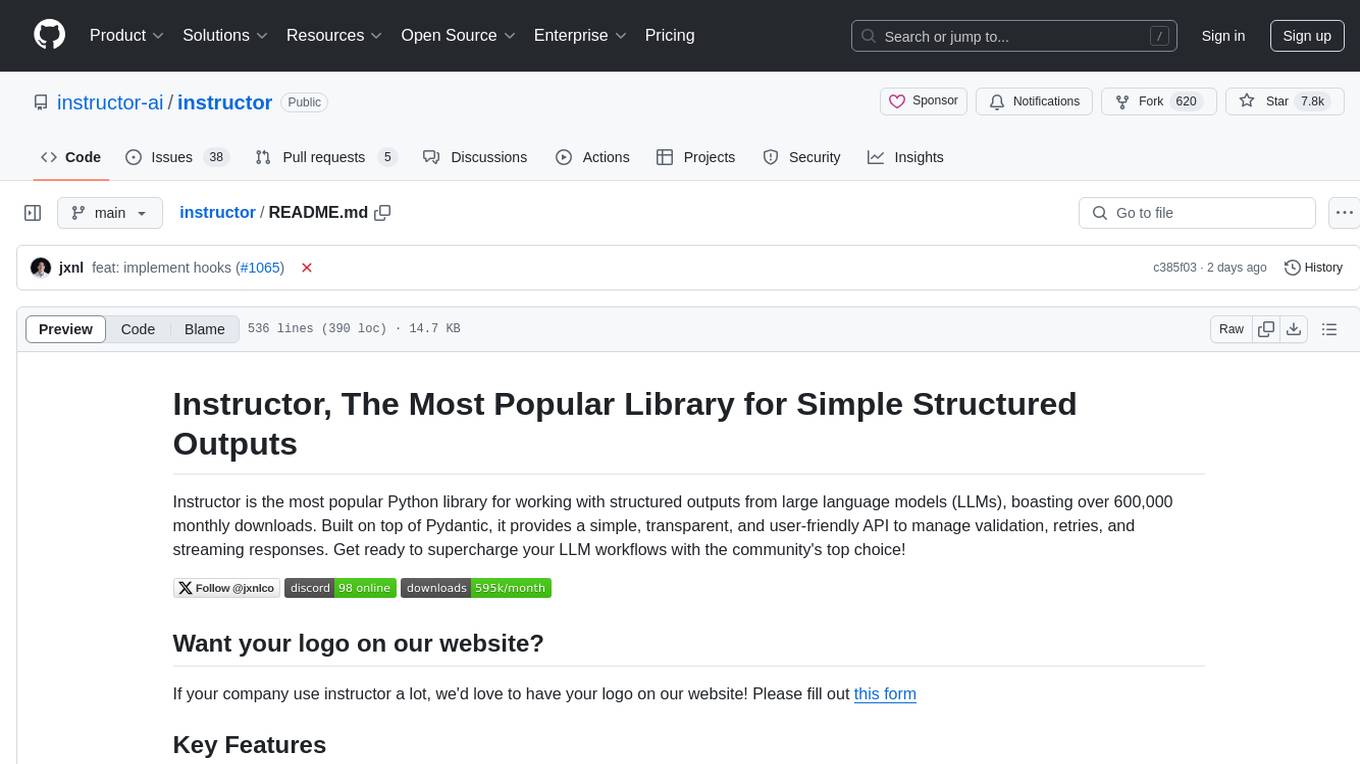
instructor
Instructor is a popular Python library for managing structured outputs from large language models (LLMs). It offers a user-friendly API for validation, retries, and streaming responses. With support for various LLM providers and multiple languages, Instructor simplifies working with LLM outputs. The library includes features like response models, retry management, validation, streaming support, and flexible backends. It also provides hooks for logging and monitoring LLM interactions, and supports integration with Anthropic, Cohere, Gemini, Litellm, and Google AI models. Instructor facilitates tasks such as extracting user data from natural language, creating fine-tuned models, managing uploaded files, and monitoring usage of OpenAI models.
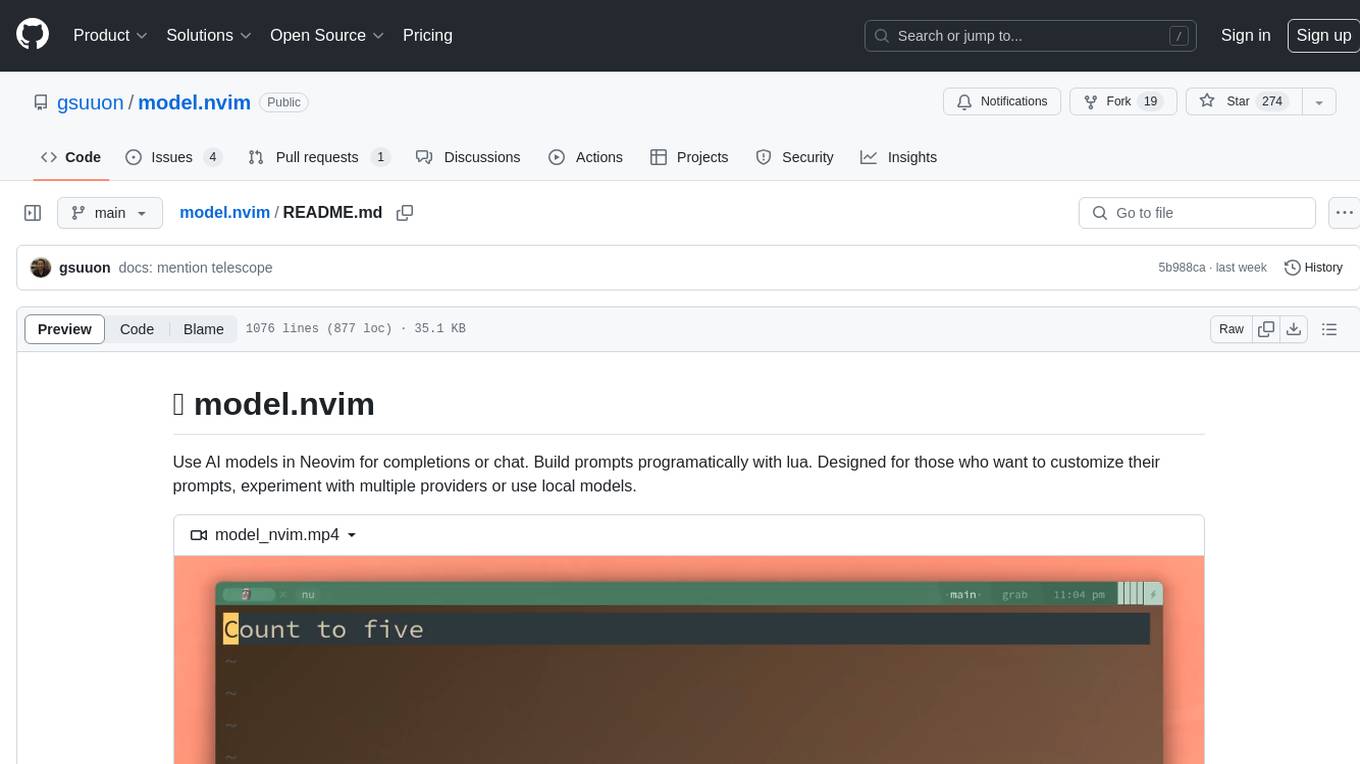
model.nvim
model.nvim is a tool designed for Neovim users who want to utilize AI models for completions or chat within their text editor. It allows users to build prompts programmatically with Lua, customize prompts, experiment with multiple providers, and use both hosted and local models. The tool supports features like provider agnosticism, programmatic prompts in Lua, async and multistep prompts, streaming completions, and chat functionality in 'mchat' filetype buffer. Users can customize prompts, manage responses, and context, and utilize various providers like OpenAI ChatGPT, Google PaLM, llama.cpp, ollama, and more. The tool also supports treesitter highlights and folds for chat buffers.
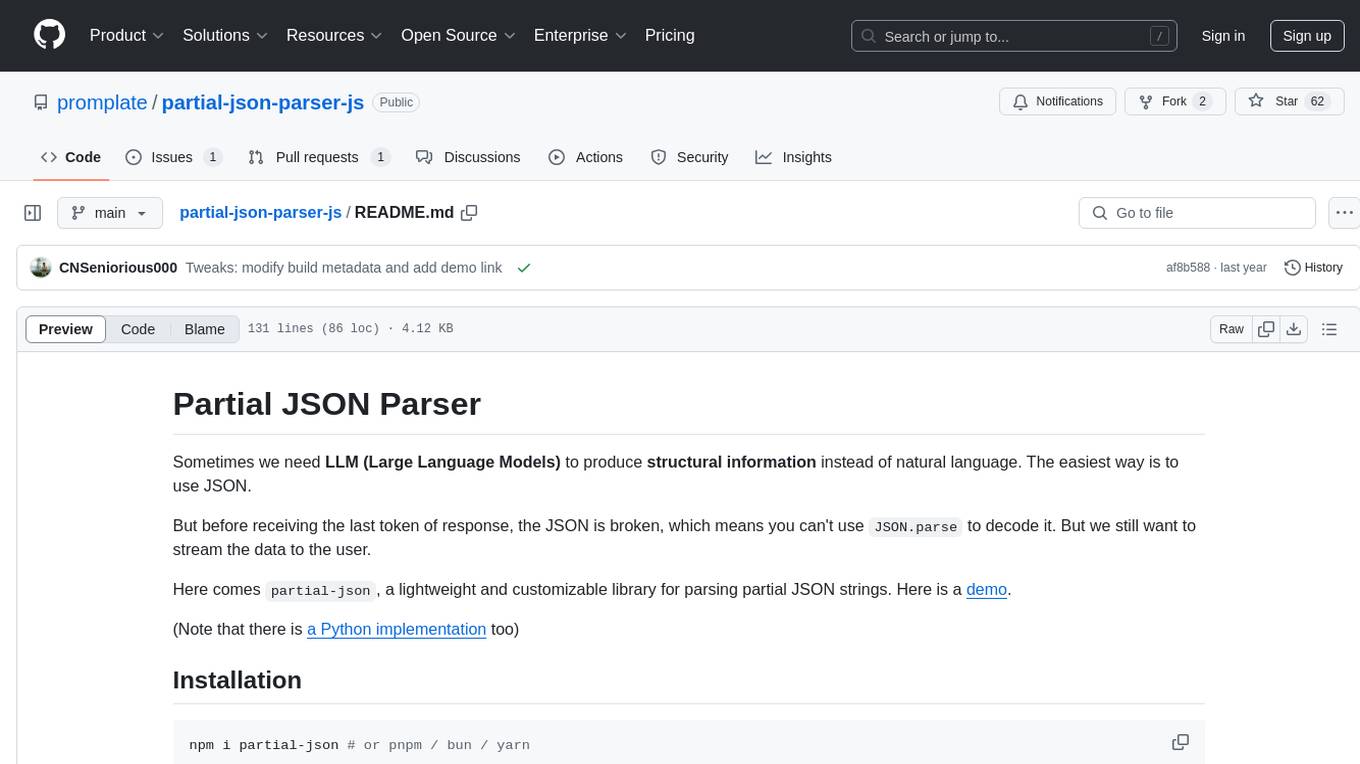
partial-json-parser-js
Partial JSON Parser is a lightweight and customizable library for parsing partial JSON strings. It allows users to parse incomplete JSON data and stream it to the user. The library provides options to specify what types of partialness are allowed during parsing, such as strings, objects, arrays, special values, and more. It helps handle malformed JSON and returns the parsed JavaScript value. Partial JSON Parser is implemented purely in JavaScript and offers both commonjs and esm builds.
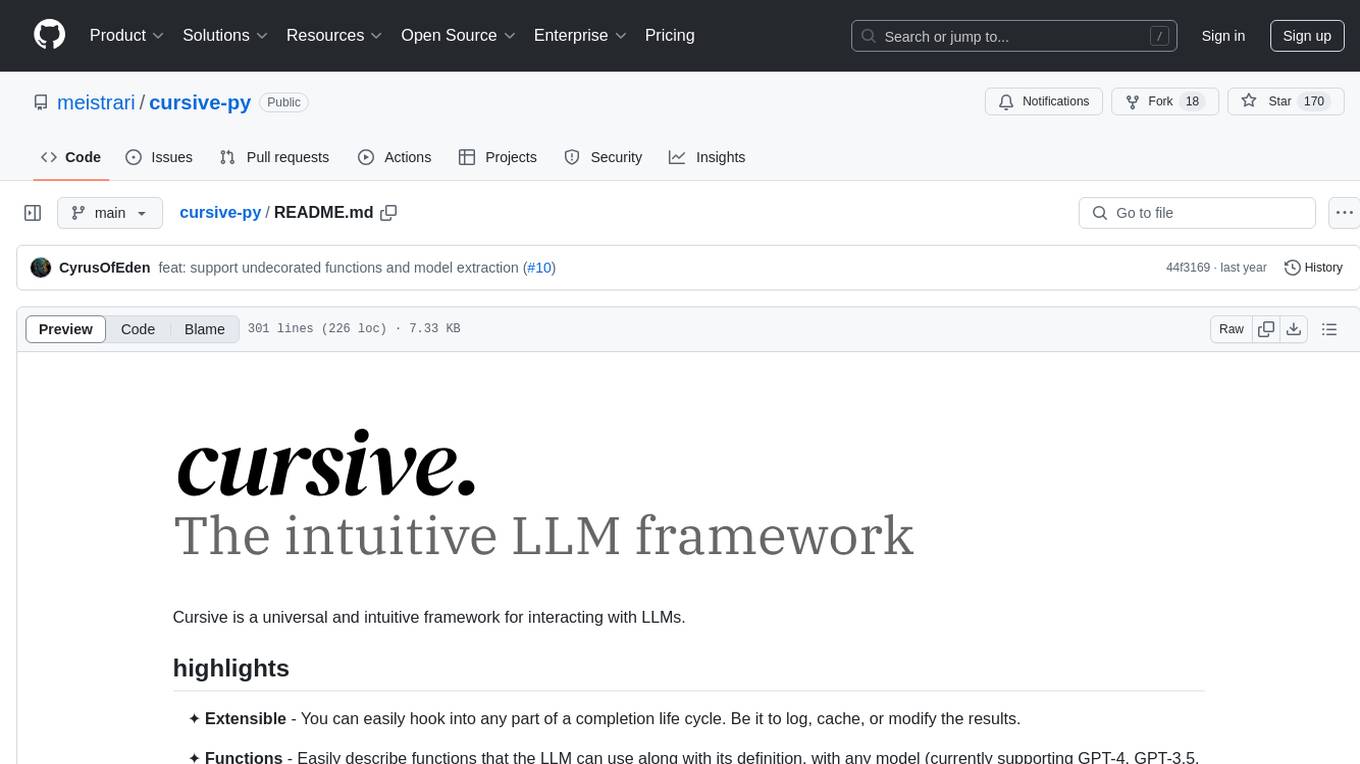
cursive-py
Cursive is a universal and intuitive framework for interacting with LLMs. It is extensible, allowing users to hook into any part of a completion life cycle. Users can easily describe functions that LLMs can use with any supported model. Cursive aims to bridge capabilities between different models, providing a single interface for users to choose any model. It comes with built-in token usage and costs calculations, automatic retry, and model expanding features. Users can define and describe functions, generate Pydantic BaseModels, hook into completion life cycle, create embeddings, and configure retry and model expanding behavior. Cursive supports various models from OpenAI, Anthropic, OpenRouter, Cohere, and Replicate, with options to pass API keys for authentication.
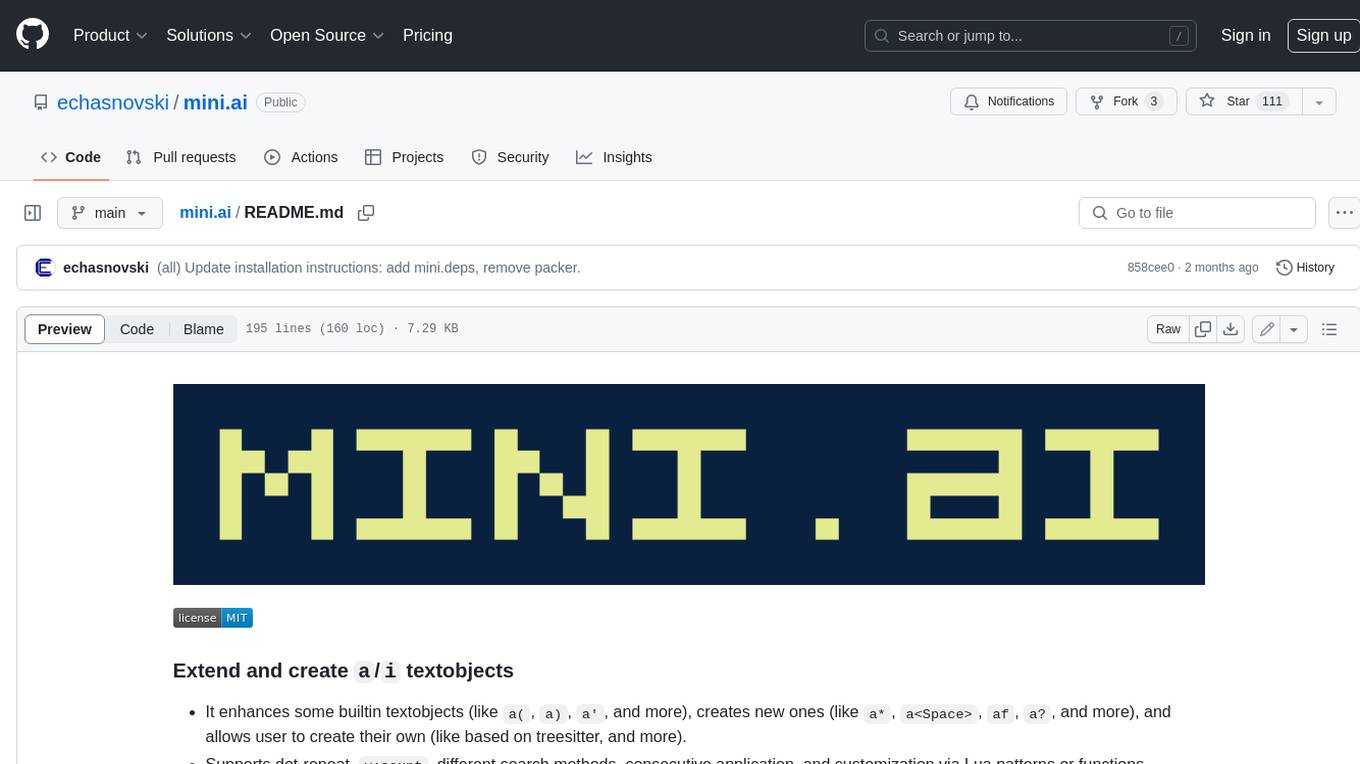
mini.ai
This plugin extends and creates `a`/`i` textobjects in Neovim. It enhances some builtin textobjects (like `a(`, `a)`, `a'`, and more), creates new ones (like `a*`, `a
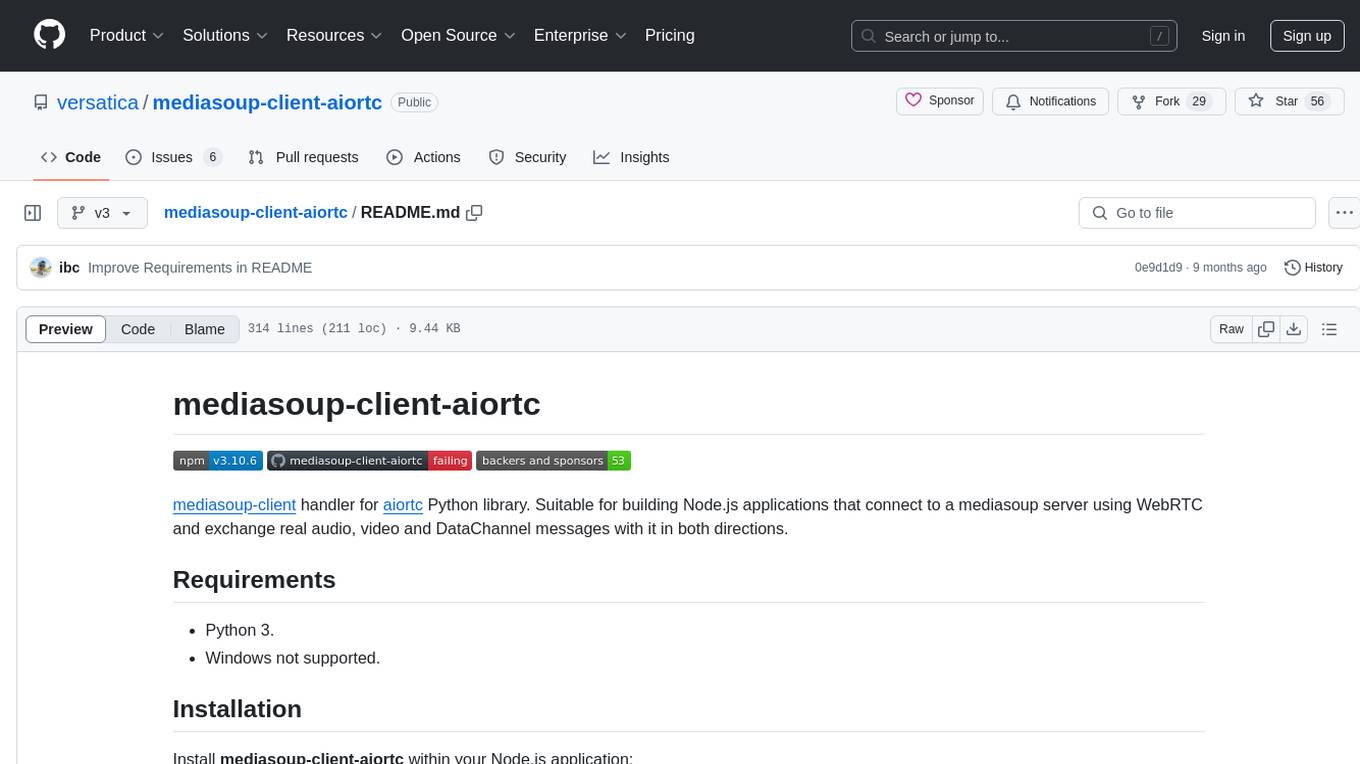
mediasoup-client-aiortc
mediasoup-client-aiortc is a handler for the aiortc Python library, allowing Node.js applications to connect to a mediasoup server using WebRTC for real-time audio, video, and DataChannel communication. It facilitates the creation of Worker instances to manage Python subprocesses, obtain audio/video tracks, and create mediasoup-client handlers. The tool supports features like getUserMedia, handlerFactory creation, and event handling for subprocess closure and unexpected termination. It provides custom classes for media stream and track constraints, enabling diverse audio/video sources like devices, files, or URLs. The tool enhances WebRTC capabilities in Node.js applications through seamless Python subprocess communication.
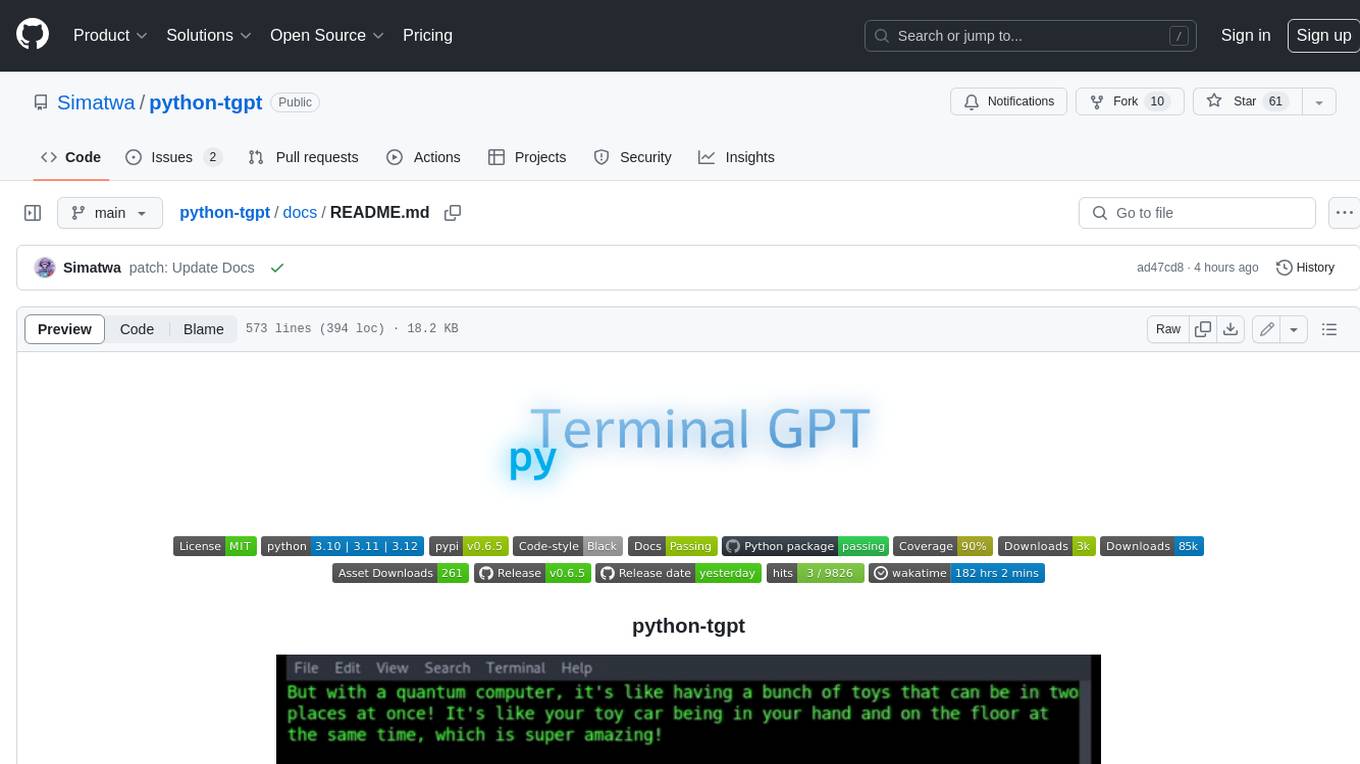
python-tgpt
Python-tgpt is a Python package that enables seamless interaction with over 45 free LLM providers without requiring an API key. It also provides image generation capabilities. The name _python-tgpt_ draws inspiration from its parent project tgpt, which operates on Golang. Through this Python adaptation, users can effortlessly engage with a number of free LLMs available, fostering a smoother AI interaction experience.
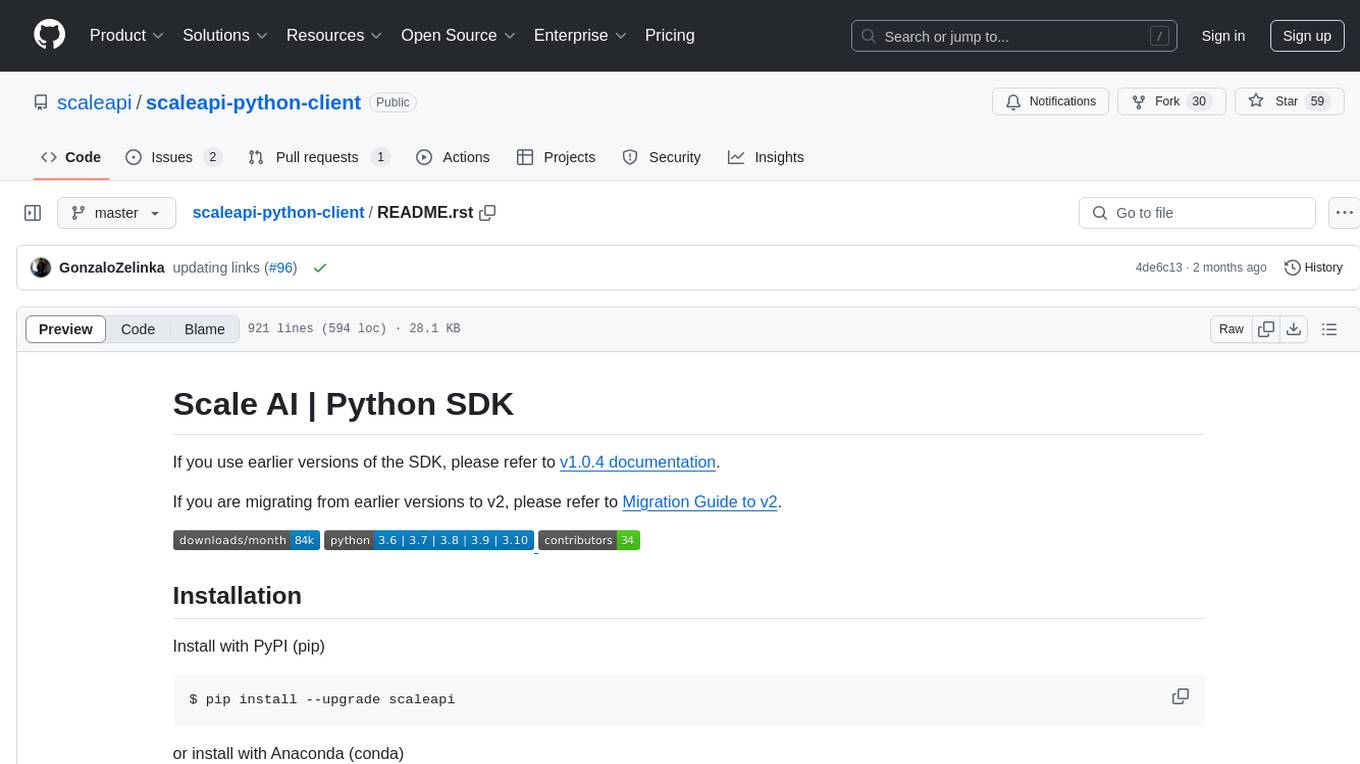
scaleapi-python-client
The Scale AI Python SDK is a tool that provides a Python interface for interacting with the Scale API. It allows users to easily create tasks, manage projects, upload files, and work with evaluation tasks, training tasks, and Studio assignments. The SDK handles error handling and provides detailed documentation for each method. Users can also manage teammates, project groups, and batches within the Scale Studio environment. The SDK supports various functionalities such as creating tasks, retrieving tasks, canceling tasks, auditing tasks, updating task attributes, managing files, managing team members, and working with evaluation and training tasks.
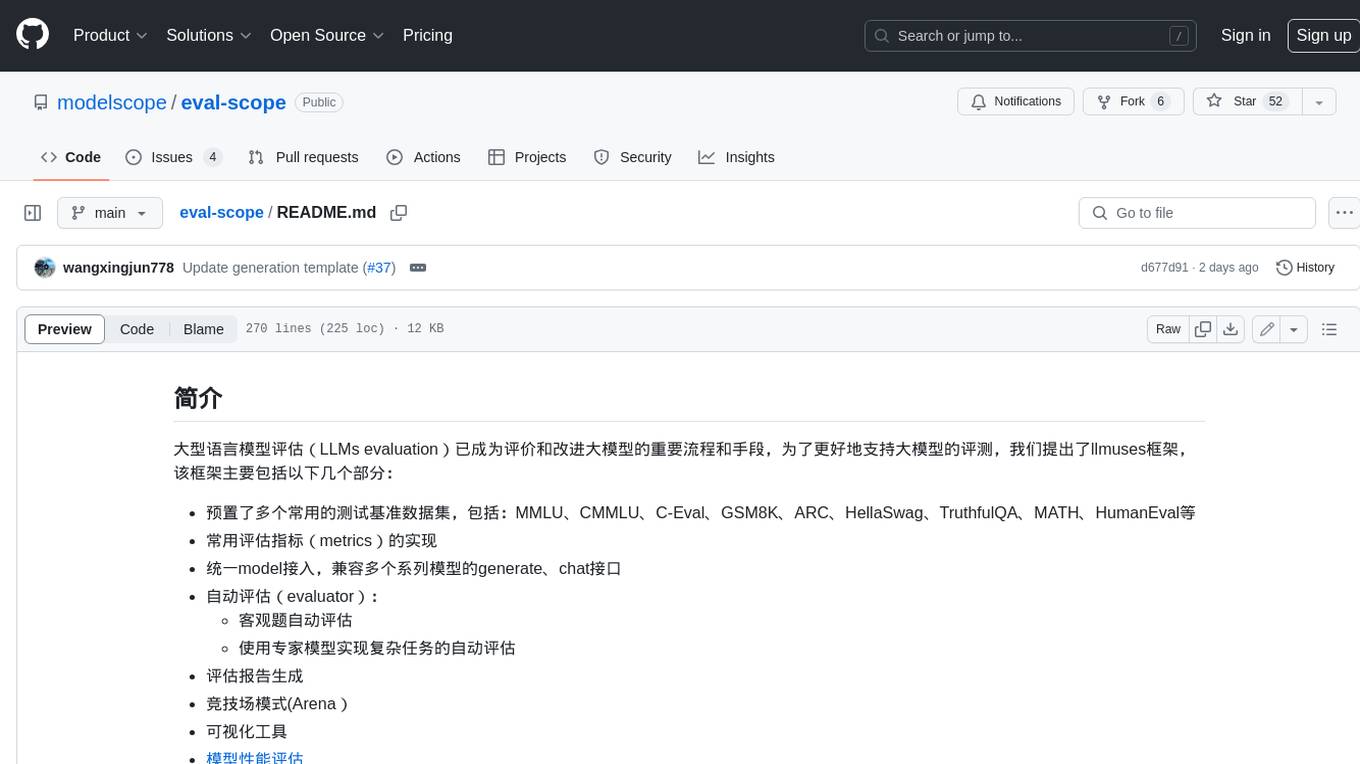
eval-scope
Eval-Scope is a framework for evaluating and improving large language models (LLMs). It provides a set of commonly used test datasets, metrics, and a unified model interface for generating and evaluating LLM responses. Eval-Scope also includes an automatic evaluator that can score objective questions and use expert models to evaluate complex tasks. Additionally, it offers a visual report generator, an arena mode for comparing multiple models, and a variety of other features to support LLM evaluation and development.
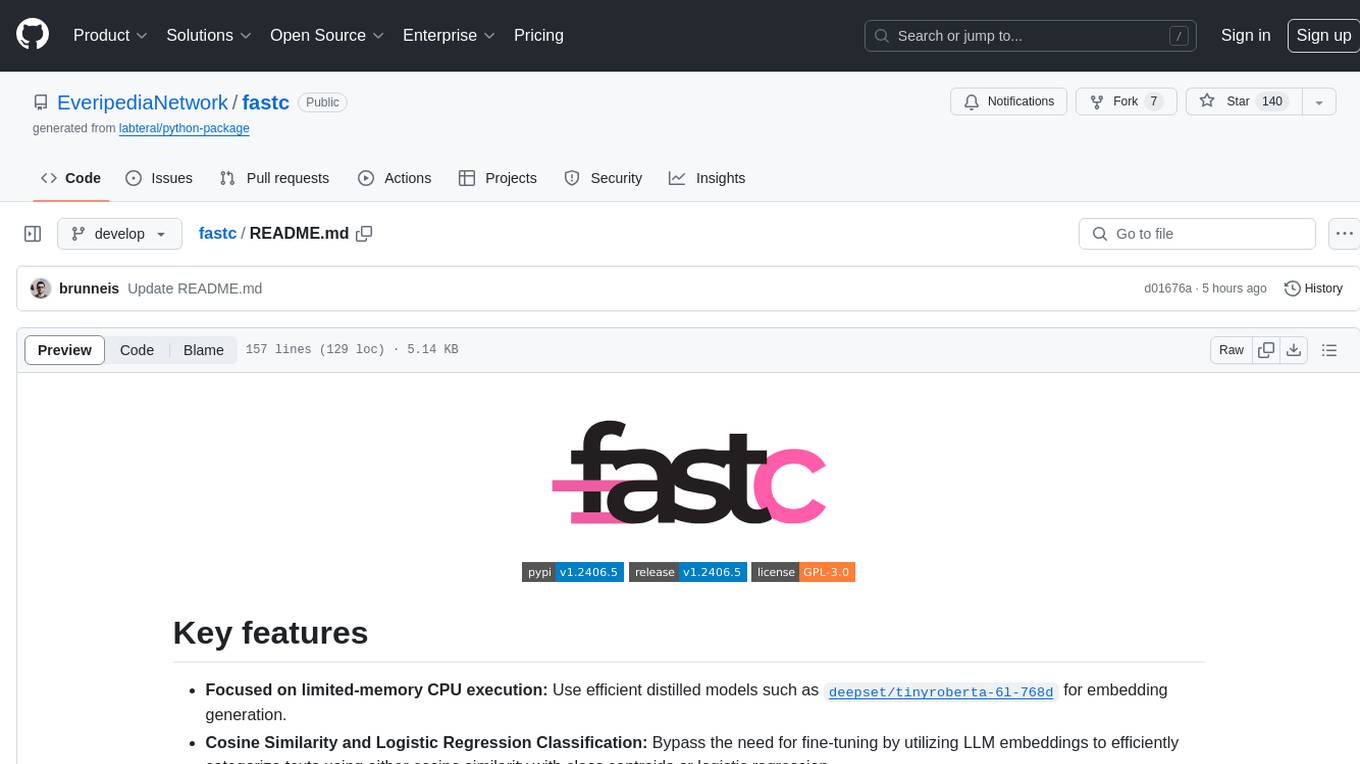
fastc
Fastc is a tool focused on CPU execution, using efficient models for embedding generation and cosine similarity classification. It allows for efficient multi-classifier execution without extra overhead. Users can easily train text classifiers, export models, publish to HuggingFace, load existing models, make class predictions, use instruct templates, and launch an inference server. The tool provides an HTTP API for text classification with JSON payloads and supports multiple languages for language identification.
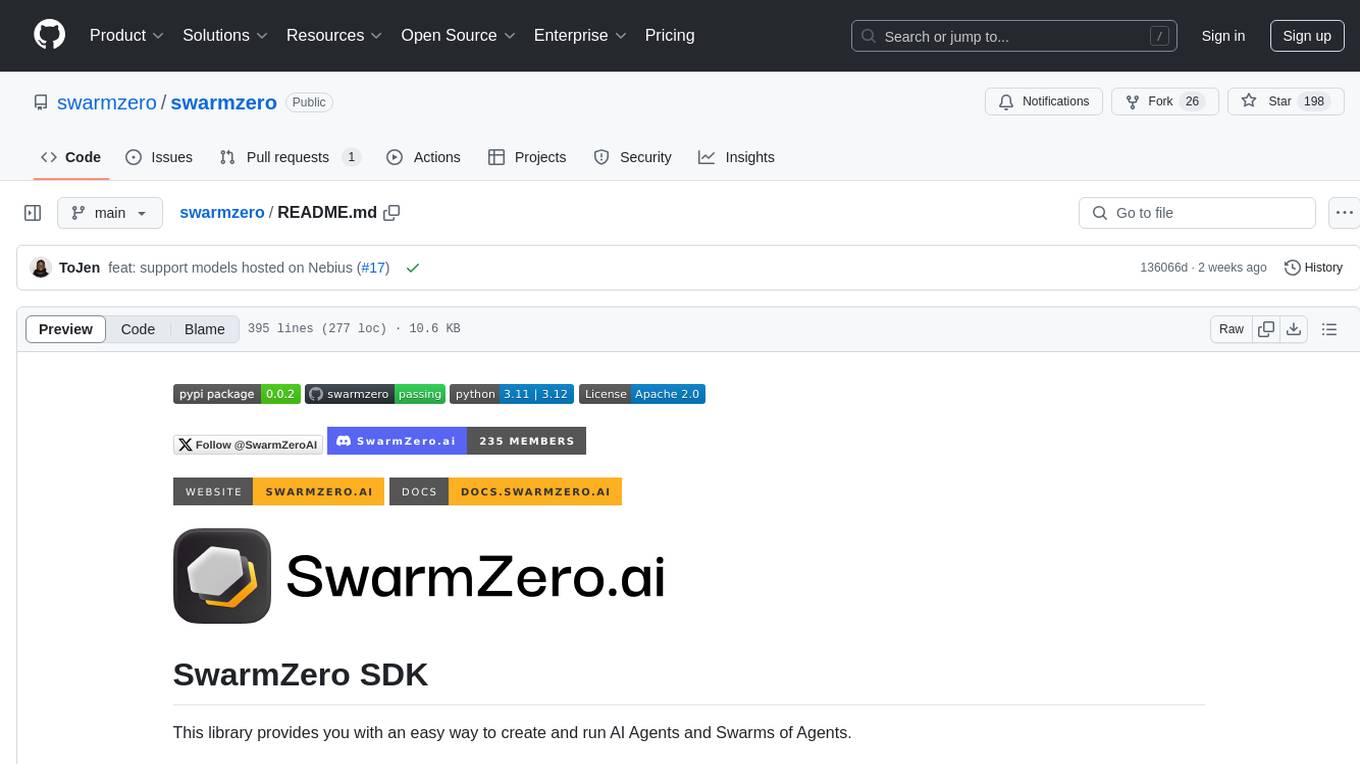
swarmzero
SwarmZero SDK is a library that simplifies the creation and execution of AI Agents and Swarms of Agents. It supports various LLM Providers such as OpenAI, Azure OpenAI, Anthropic, MistralAI, Gemini, Nebius, and Ollama. Users can easily install the library using pip or poetry, set up the environment and configuration, create and run Agents, collaborate with Swarms, add tools for complex tasks, and utilize retriever tools for semantic information retrieval. Sample prompts are provided to help users explore the capabilities of the agents and swarms. The SDK also includes detailed examples and documentation for reference.
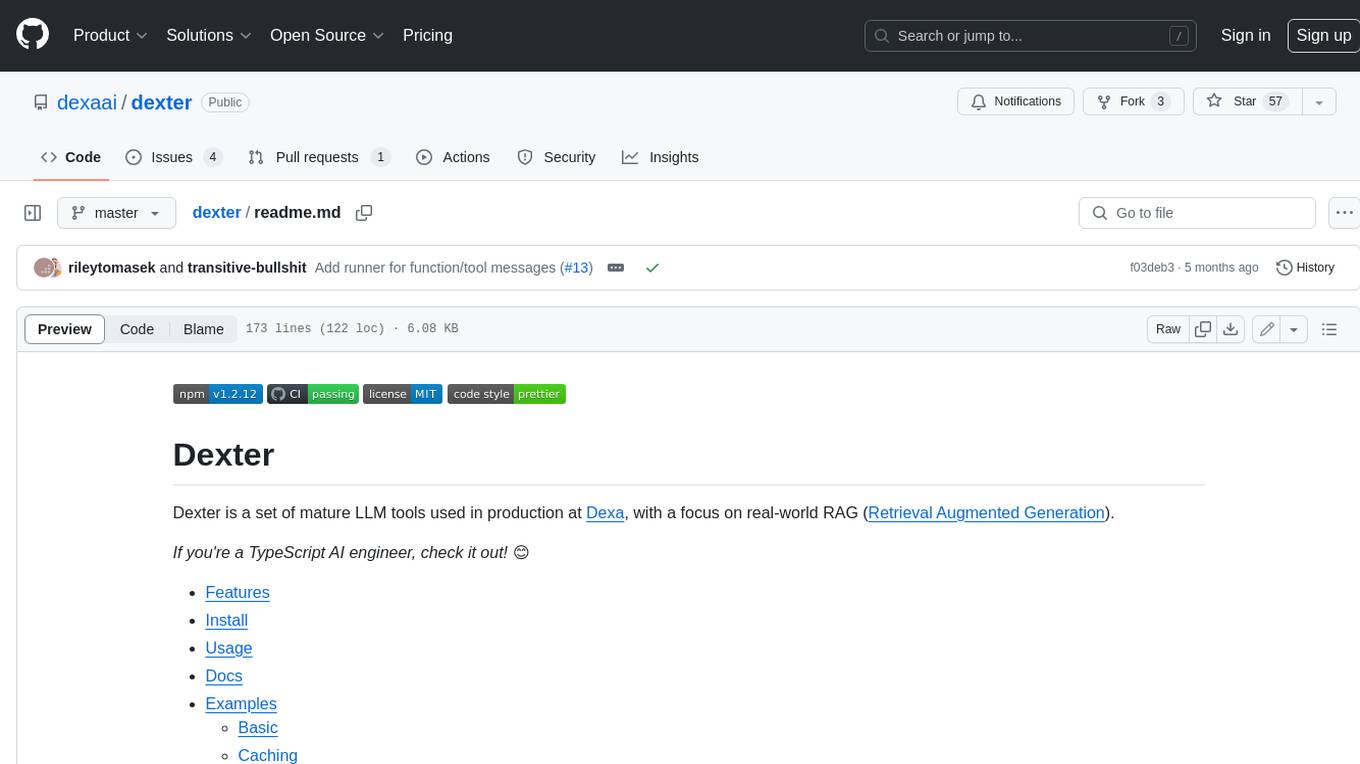
dexter
Dexter is a set of mature LLM tools used in production at Dexa, with a focus on real-world RAG (Retrieval Augmented Generation). It is a production-quality RAG that is extremely fast and minimal, and handles caching, throttling, and batching for ingesting large datasets. It also supports optional hybrid search with SPLADE embeddings, and is a minimal TS package with full typing that uses `fetch` everywhere and supports Node.js 18+, Deno, Cloudflare Workers, Vercel edge functions, etc. Dexter has full docs and includes examples for basic usage, caching, Redis caching, AI function, AI runner, and chatbot.
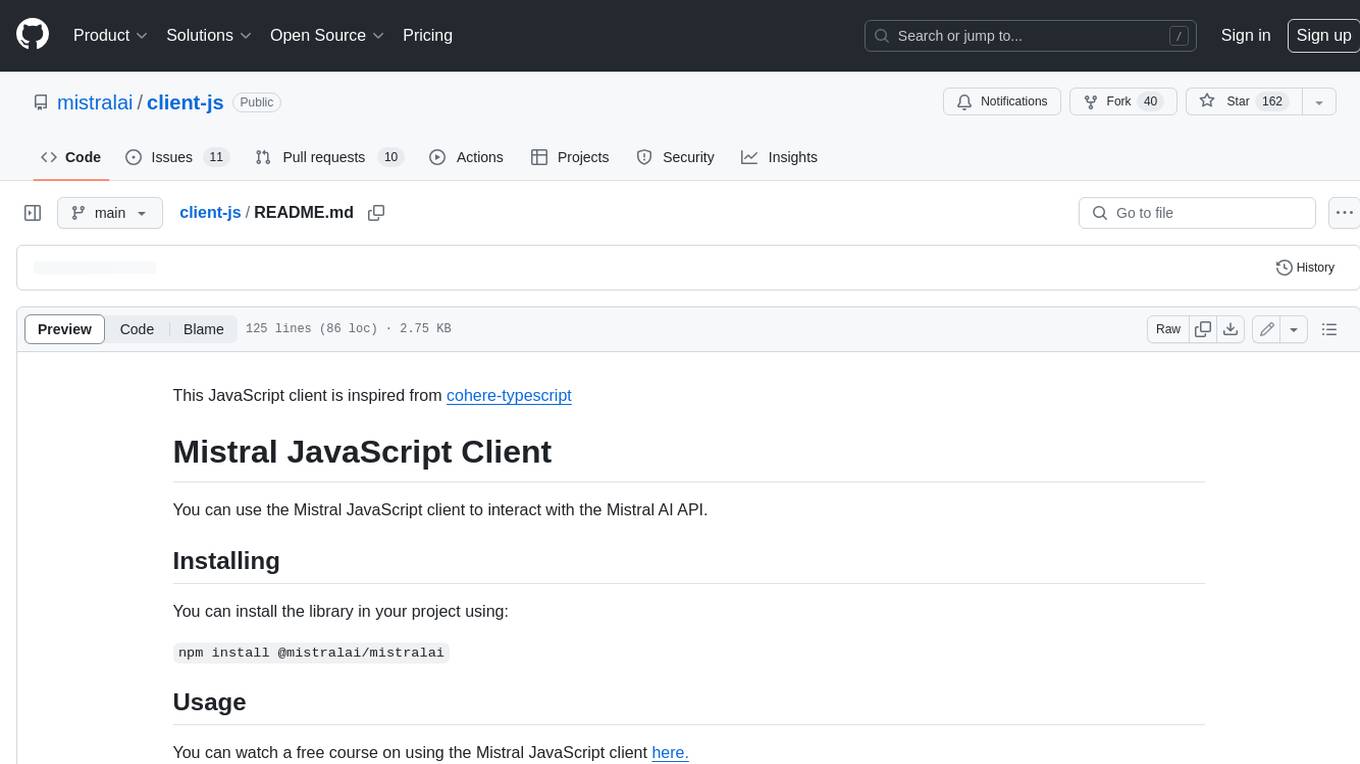
client-js
The Mistral JavaScript client is a library that allows you to interact with the Mistral AI API. With this client, you can perform various tasks such as listing models, chatting with streaming, chatting without streaming, and generating embeddings. To use the client, you can install it in your project using npm and then set up the client with your API key. Once the client is set up, you can use it to perform the desired tasks. For example, you can use the client to chat with a model by providing a list of messages. The client will then return the response from the model. You can also use the client to generate embeddings for a given input. The embeddings can then be used for various downstream tasks such as clustering or classification.
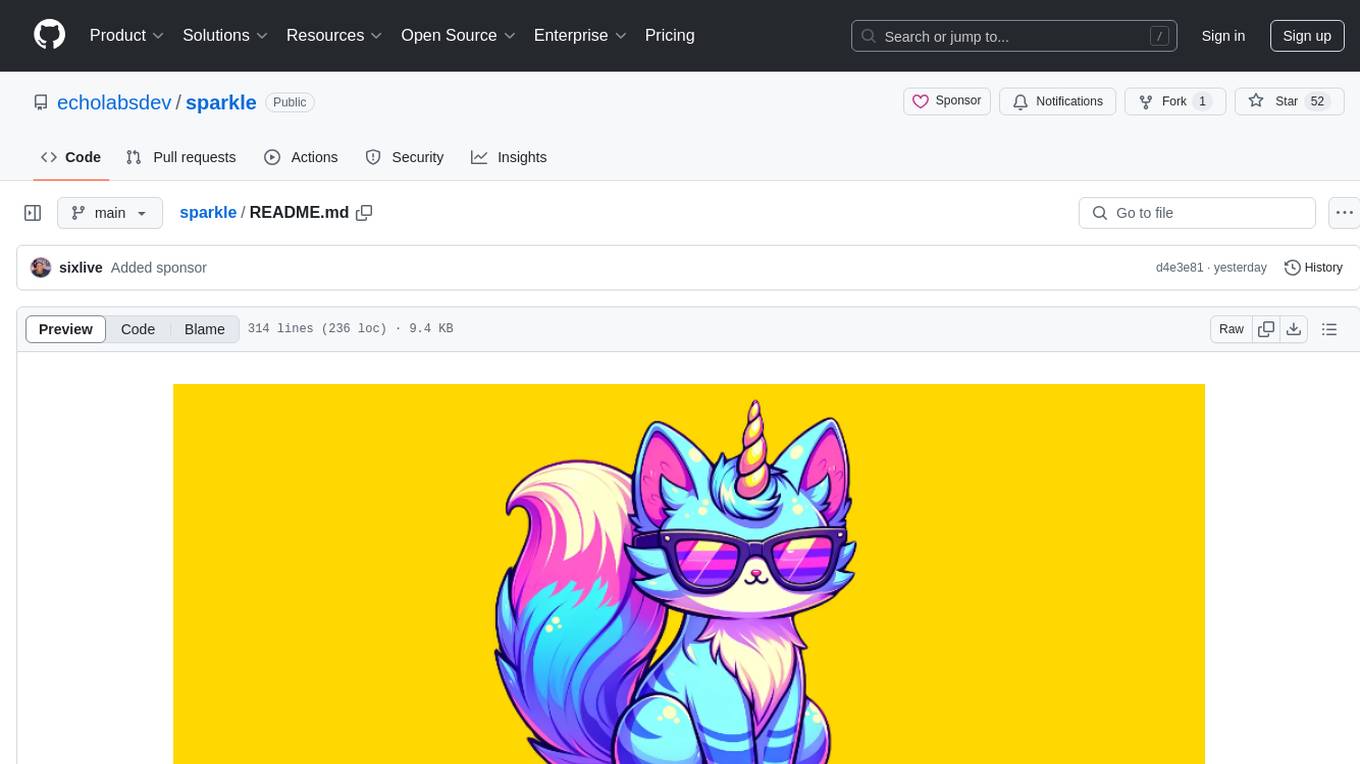
sparkle
Sparkle is a tool that streamlines the process of building AI-driven features in applications using Large Language Models (LLMs). It guides users through creating and managing agents, defining tools, and interacting with LLM providers like OpenAI. Sparkle allows customization of LLM provider settings, model configurations, and provides a seamless integration with Sparkle Server for exposing agents via an OpenAI-compatible chat API endpoint.
For similar tasks
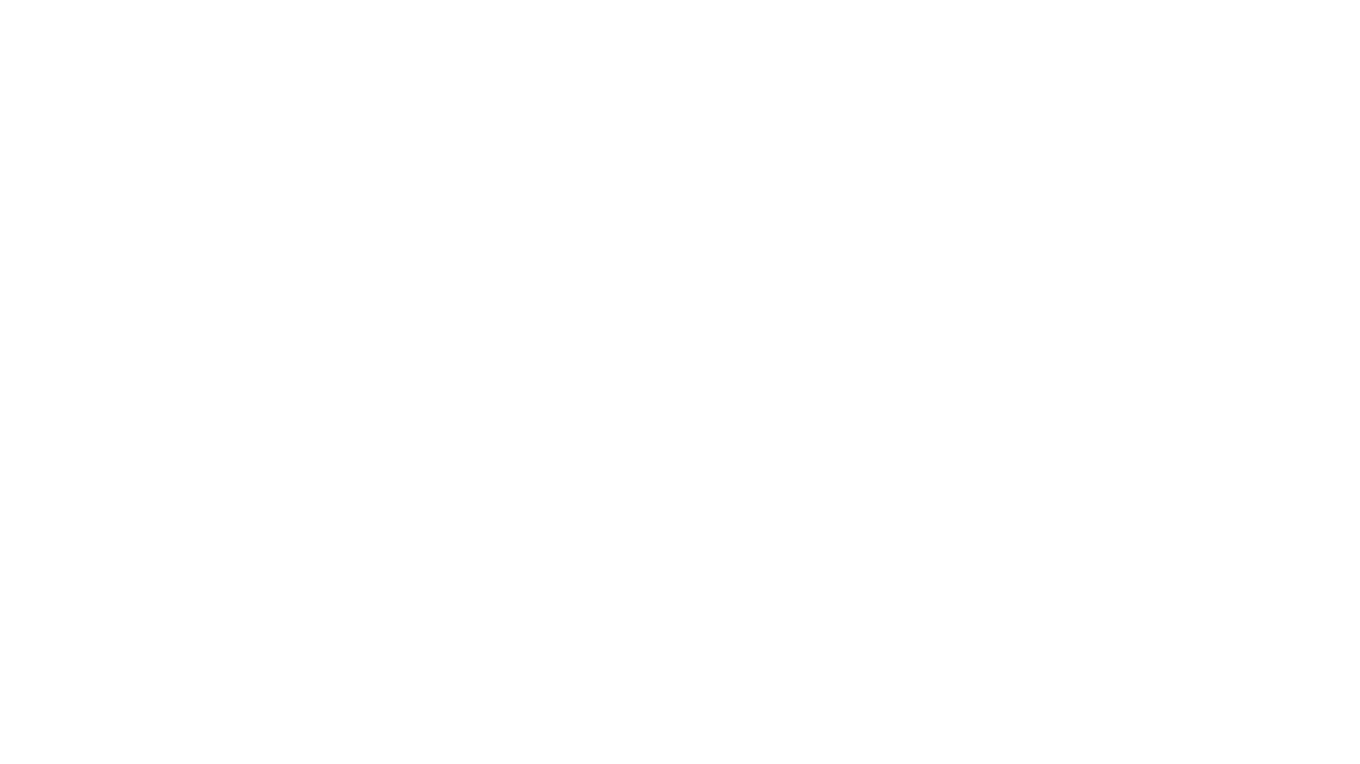
strictjson
Strict JSON is a framework designed to handle JSON outputs with complex structures, fixing issues that standard json.loads() cannot resolve. It provides functionalities for parsing LLM outputs into dictionaries, supporting various data types, type forcing, and error correction. The tool allows easy integration with OpenAI JSON Mode and offers community support through tutorials and discussions. Users can download the package via pip, set up API keys, and import functions for usage. The tool works by extracting JSON values using regex, matching output values to literals, and ensuring all JSON fields are output by LLM with optional type checking. It also supports LLM-based checks for type enforcement and error correction loops.
For similar jobs
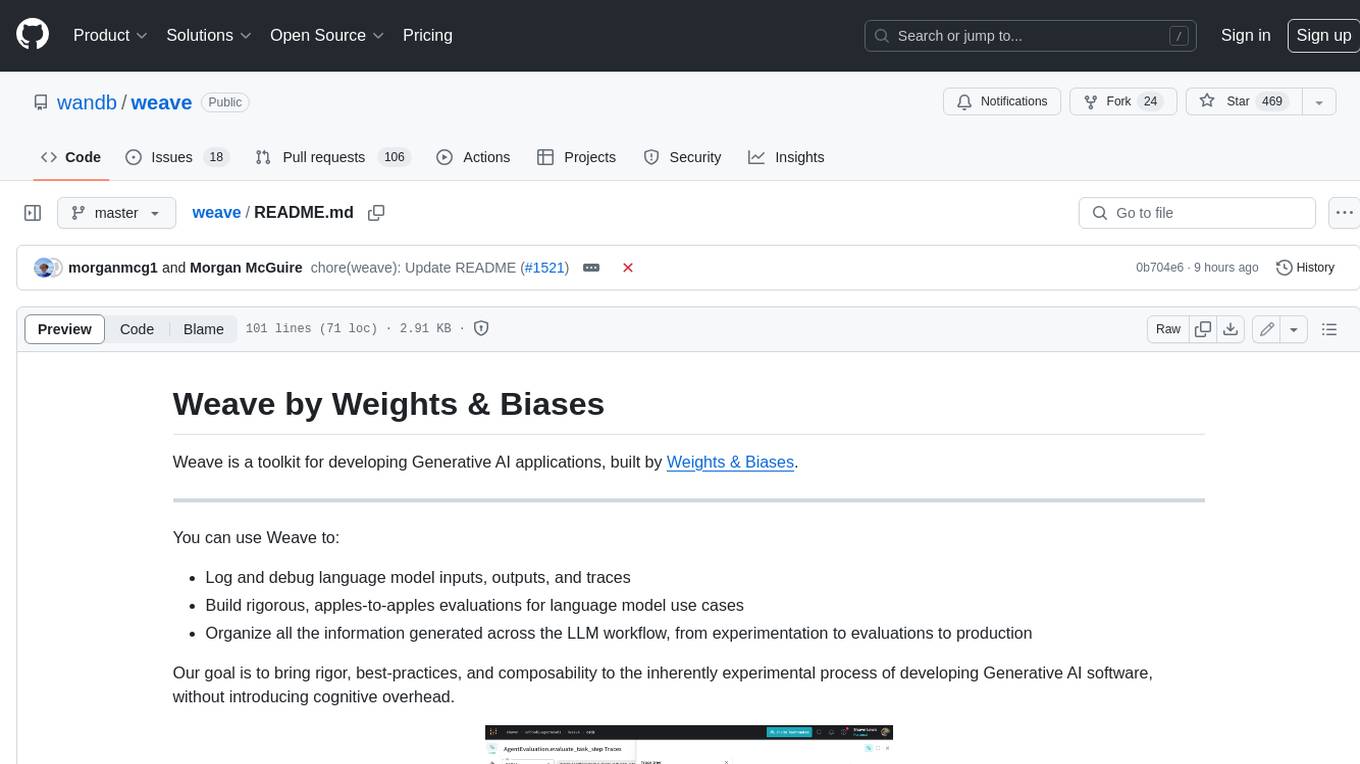
weave
Weave is a toolkit for developing Generative AI applications, built by Weights & Biases. With Weave, you can log and debug language model inputs, outputs, and traces; build rigorous, apples-to-apples evaluations for language model use cases; and organize all the information generated across the LLM workflow, from experimentation to evaluations to production. Weave aims to bring rigor, best-practices, and composability to the inherently experimental process of developing Generative AI software, without introducing cognitive overhead.
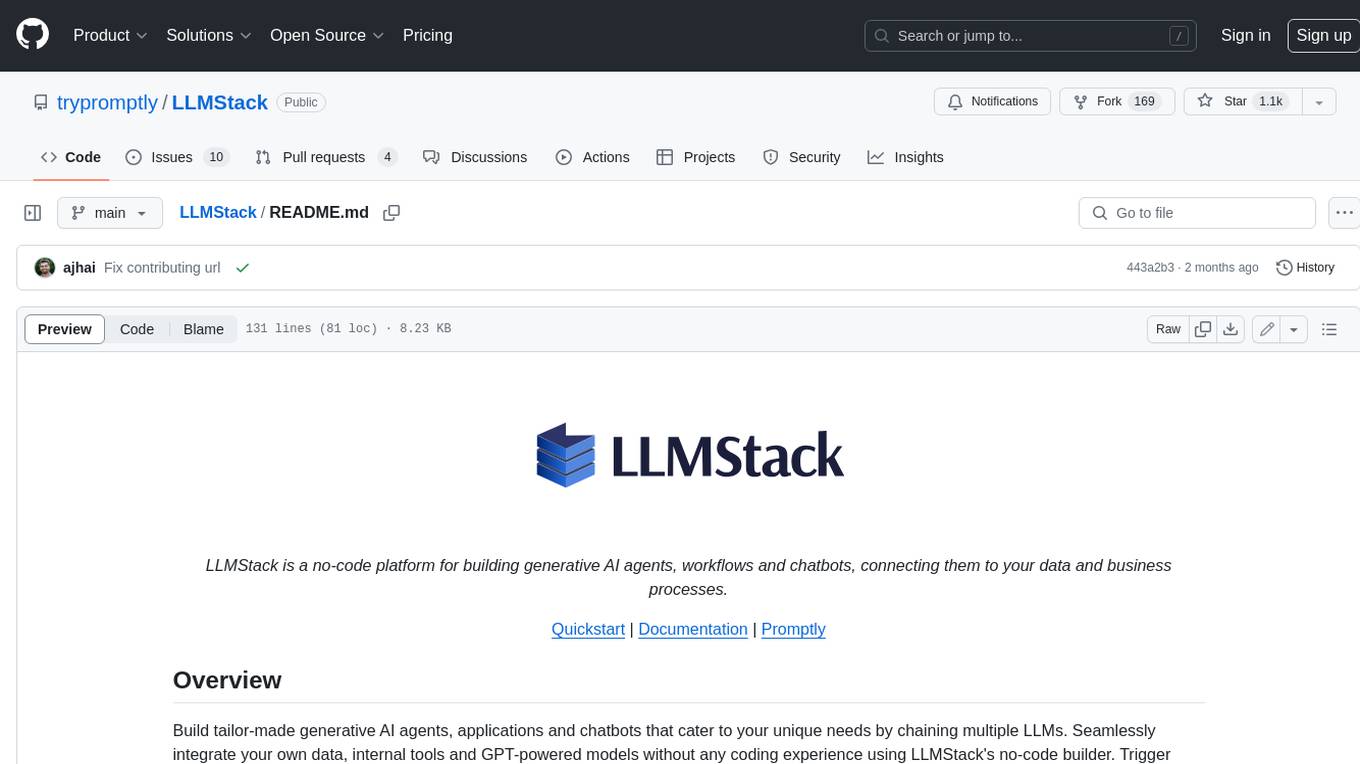
LLMStack
LLMStack is a no-code platform for building generative AI agents, workflows, and chatbots. It allows users to connect their own data, internal tools, and GPT-powered models without any coding experience. LLMStack can be deployed to the cloud or on-premise and can be accessed via HTTP API or triggered from Slack or Discord.
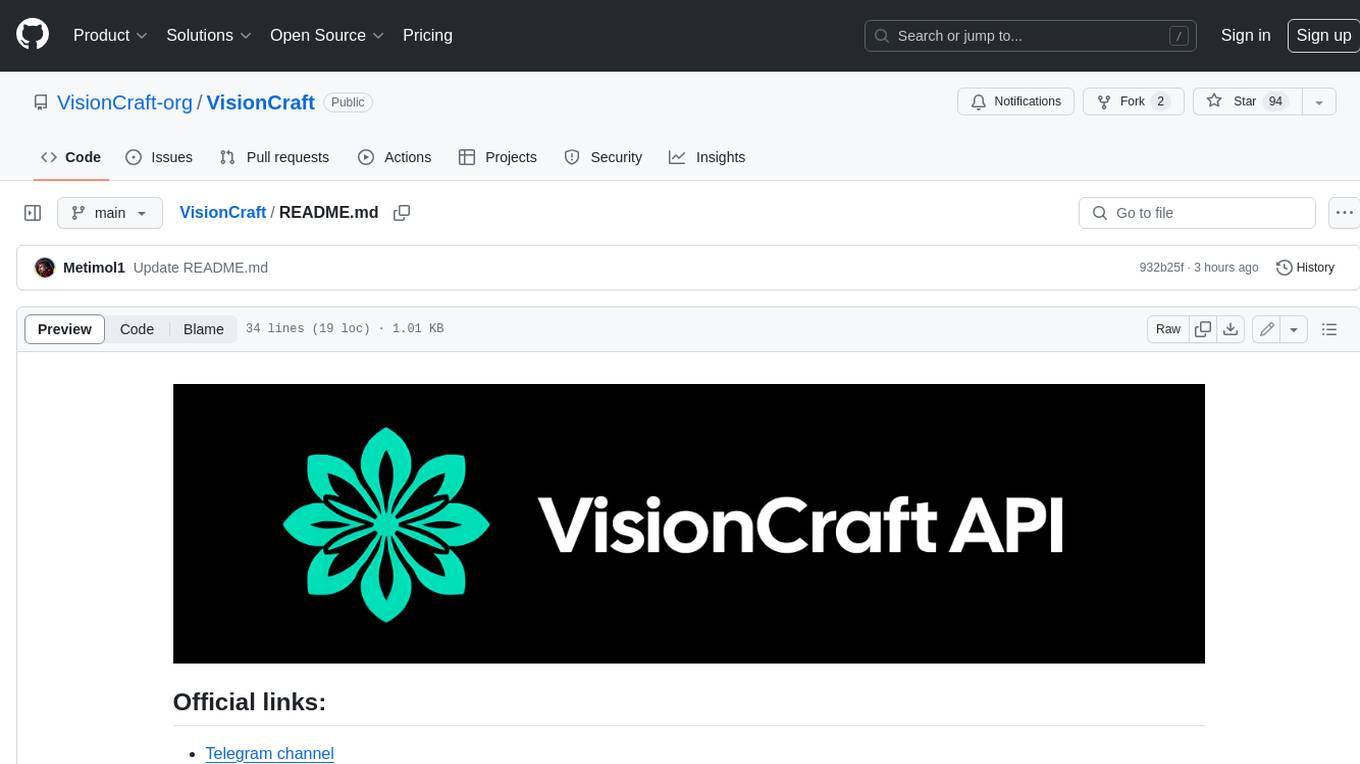
VisionCraft
The VisionCraft API is a free API for using over 100 different AI models. From images to sound.
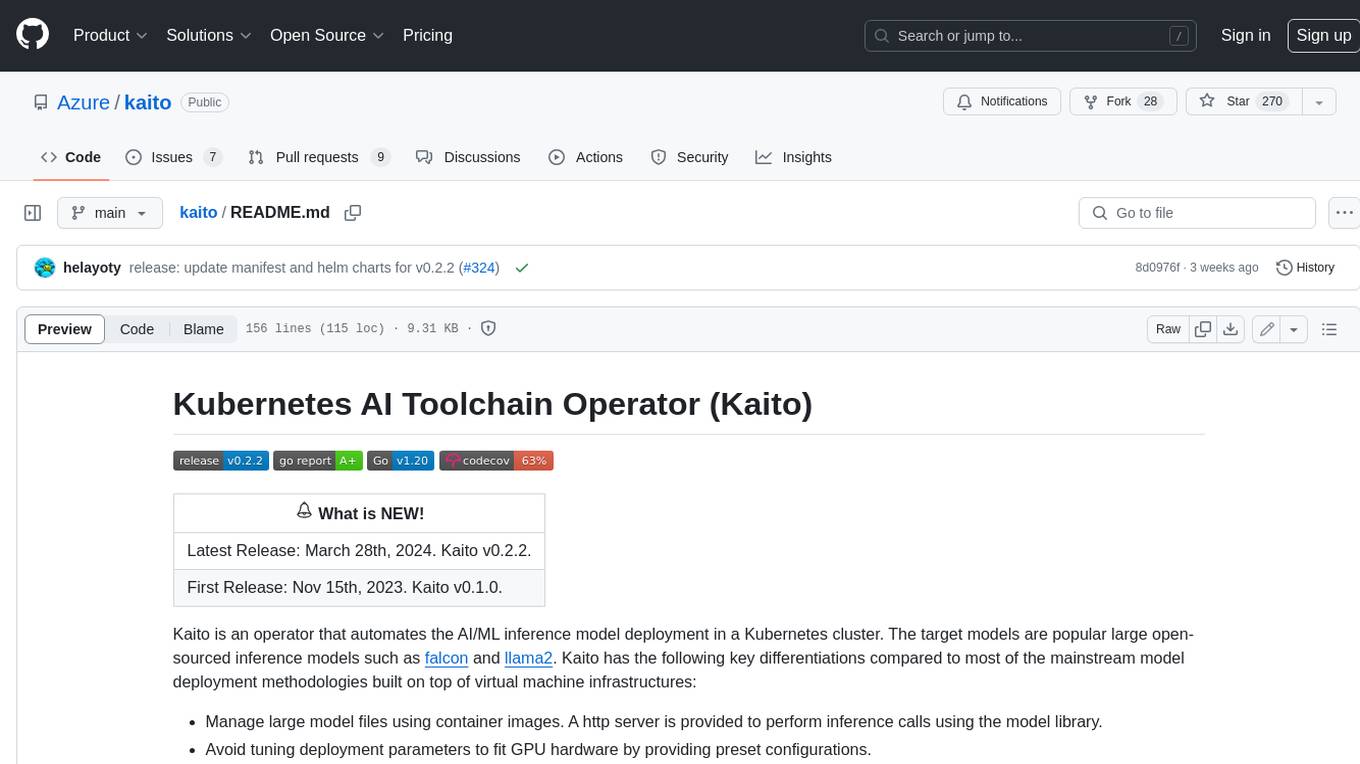
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
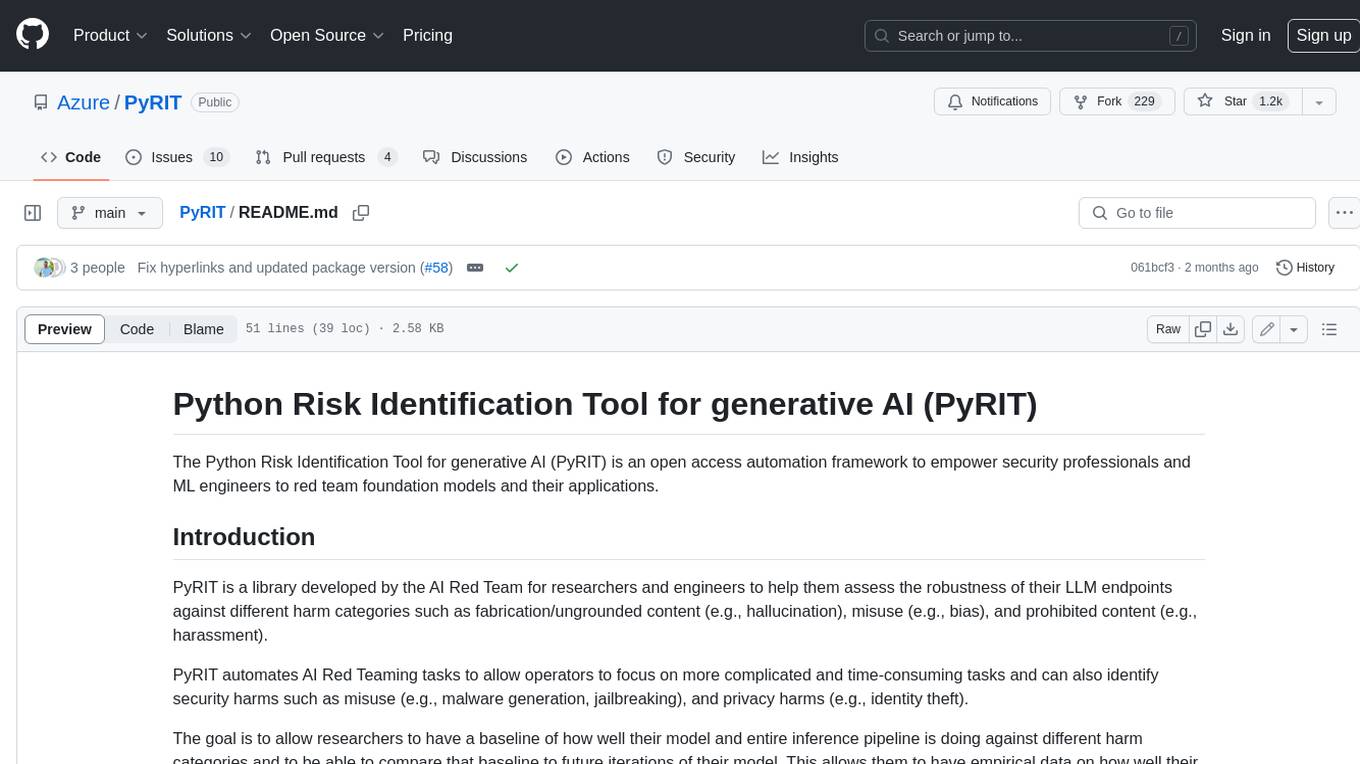
PyRIT
PyRIT is an open access automation framework designed to empower security professionals and ML engineers to red team foundation models and their applications. It automates AI Red Teaming tasks to allow operators to focus on more complicated and time-consuming tasks and can also identify security harms such as misuse (e.g., malware generation, jailbreaking), and privacy harms (e.g., identity theft). The goal is to allow researchers to have a baseline of how well their model and entire inference pipeline is doing against different harm categories and to be able to compare that baseline to future iterations of their model. This allows them to have empirical data on how well their model is doing today, and detect any degradation of performance based on future improvements.
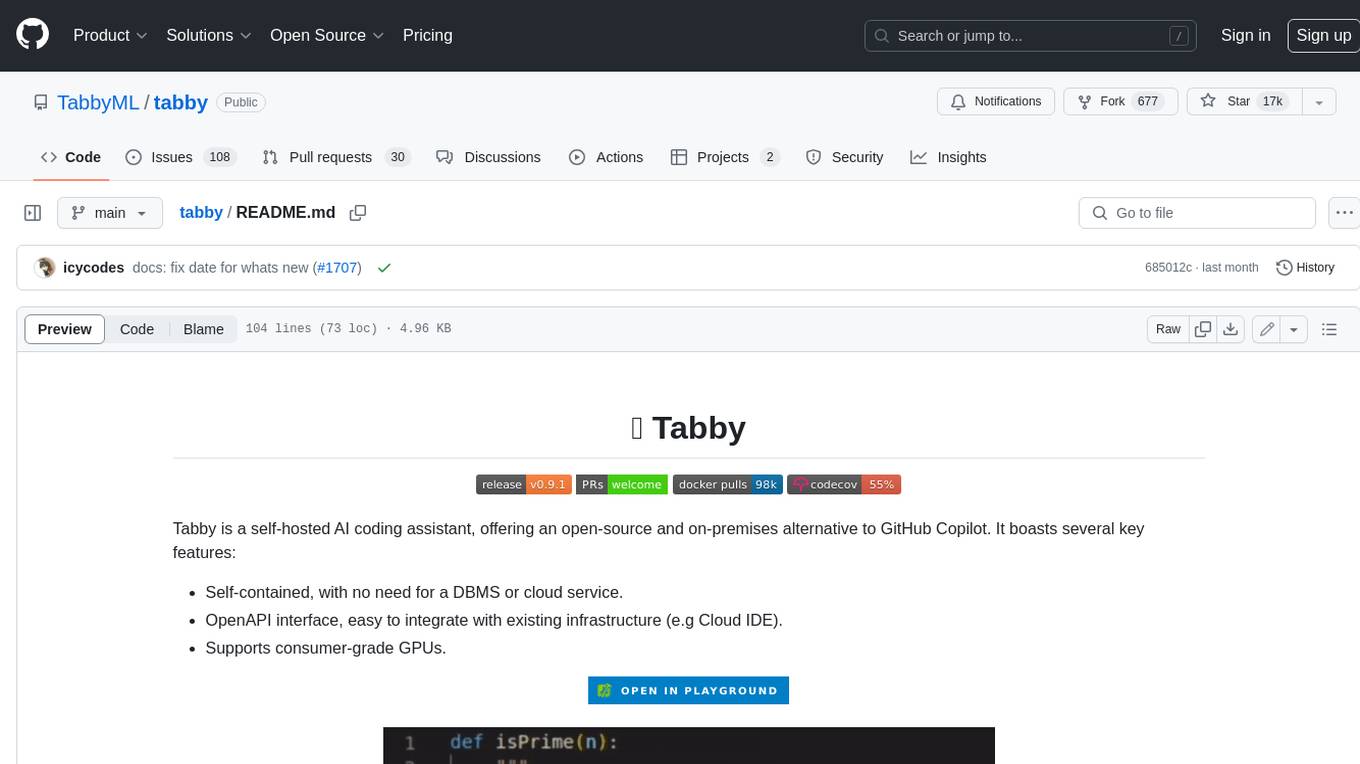
tabby
Tabby is a self-hosted AI coding assistant, offering an open-source and on-premises alternative to GitHub Copilot. It boasts several key features: * Self-contained, with no need for a DBMS or cloud service. * OpenAPI interface, easy to integrate with existing infrastructure (e.g Cloud IDE). * Supports consumer-grade GPUs.
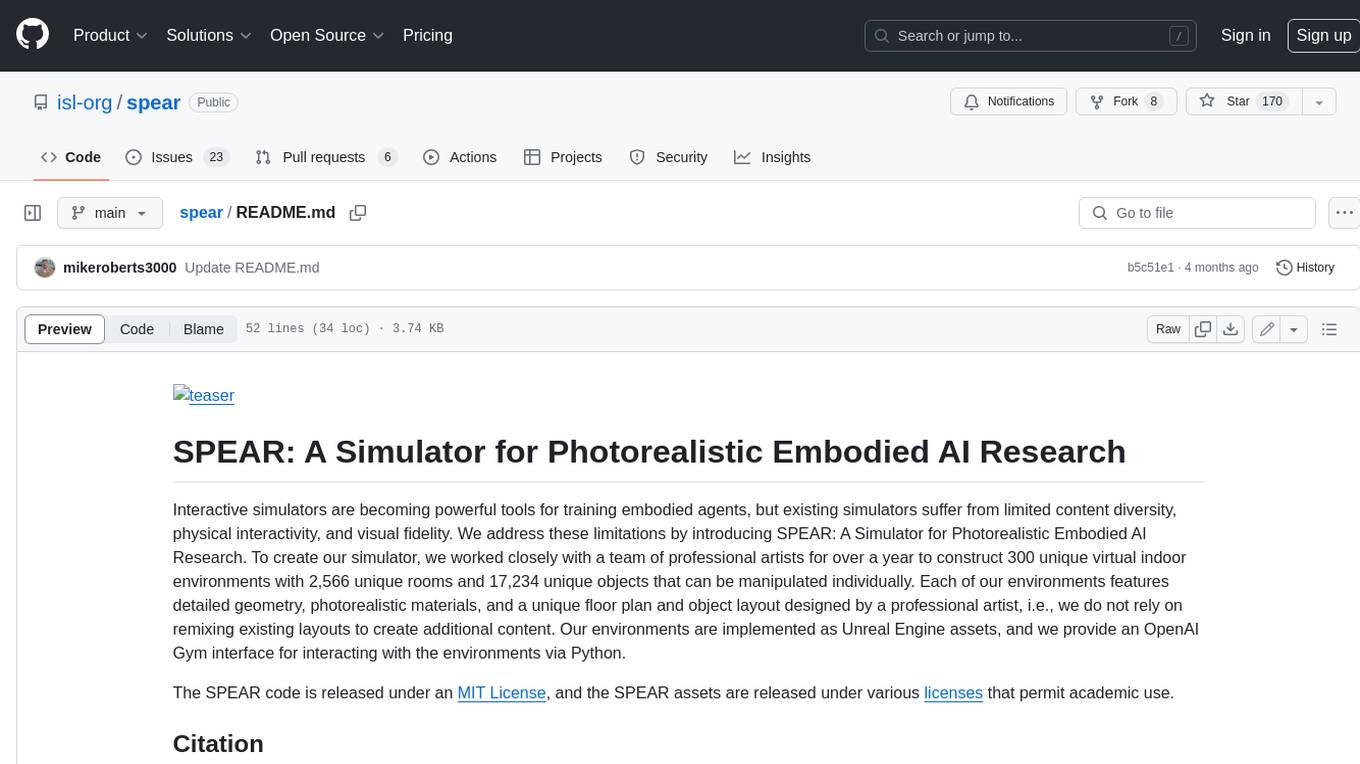
spear
SPEAR (Simulator for Photorealistic Embodied AI Research) is a powerful tool for training embodied agents. It features 300 unique virtual indoor environments with 2,566 unique rooms and 17,234 unique objects that can be manipulated individually. Each environment is designed by a professional artist and features detailed geometry, photorealistic materials, and a unique floor plan and object layout. SPEAR is implemented as Unreal Engine assets and provides an OpenAI Gym interface for interacting with the environments via Python.
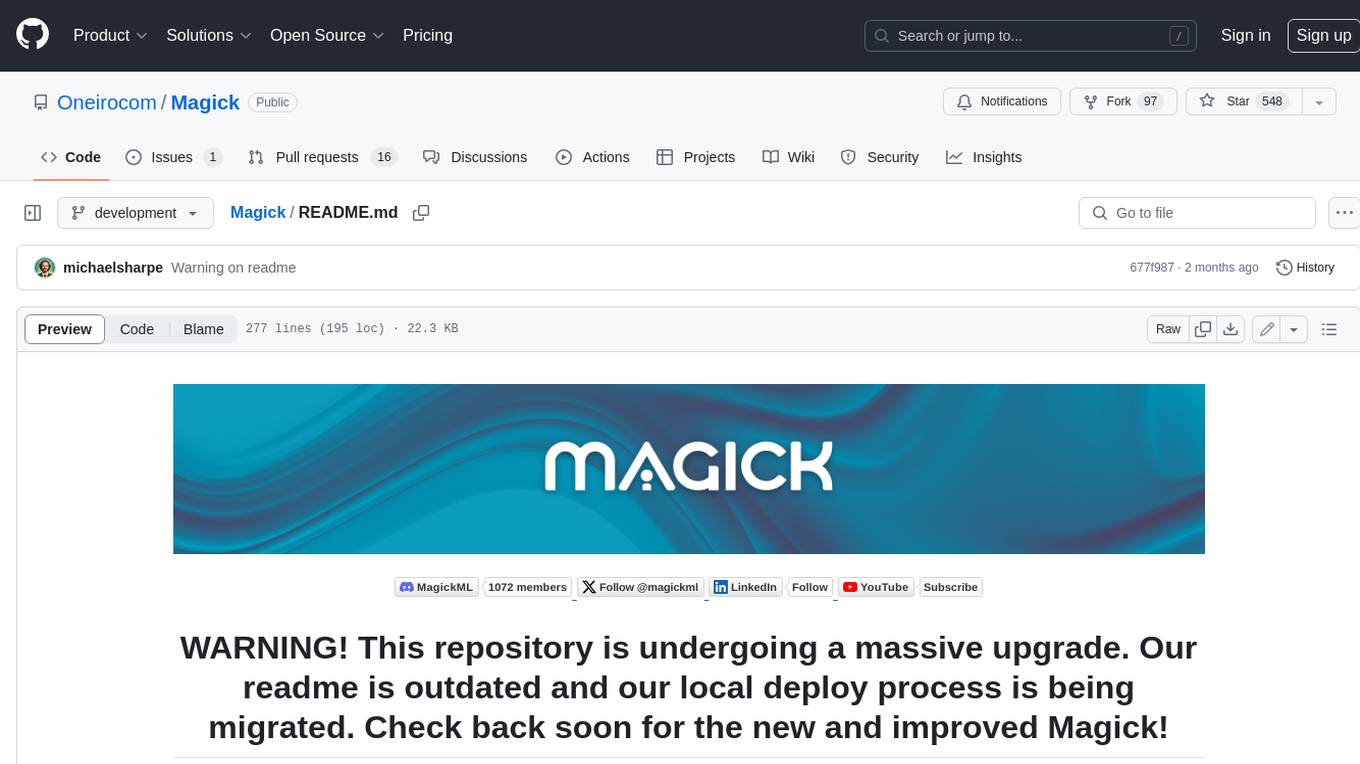
Magick
Magick is a groundbreaking visual AIDE (Artificial Intelligence Development Environment) for no-code data pipelines and multimodal agents. Magick can connect to other services and comes with nodes and templates well-suited for intelligent agents, chatbots, complex reasoning systems and realistic characters.