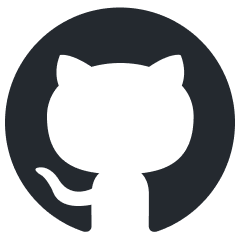
aichildedu
A microservice-based AI education platform for children that integrates LLMs, image generation, and speech synthesis to provide personalized storybook creation, intelligent conversational learning, and multimedia content generation.
Stars: 162
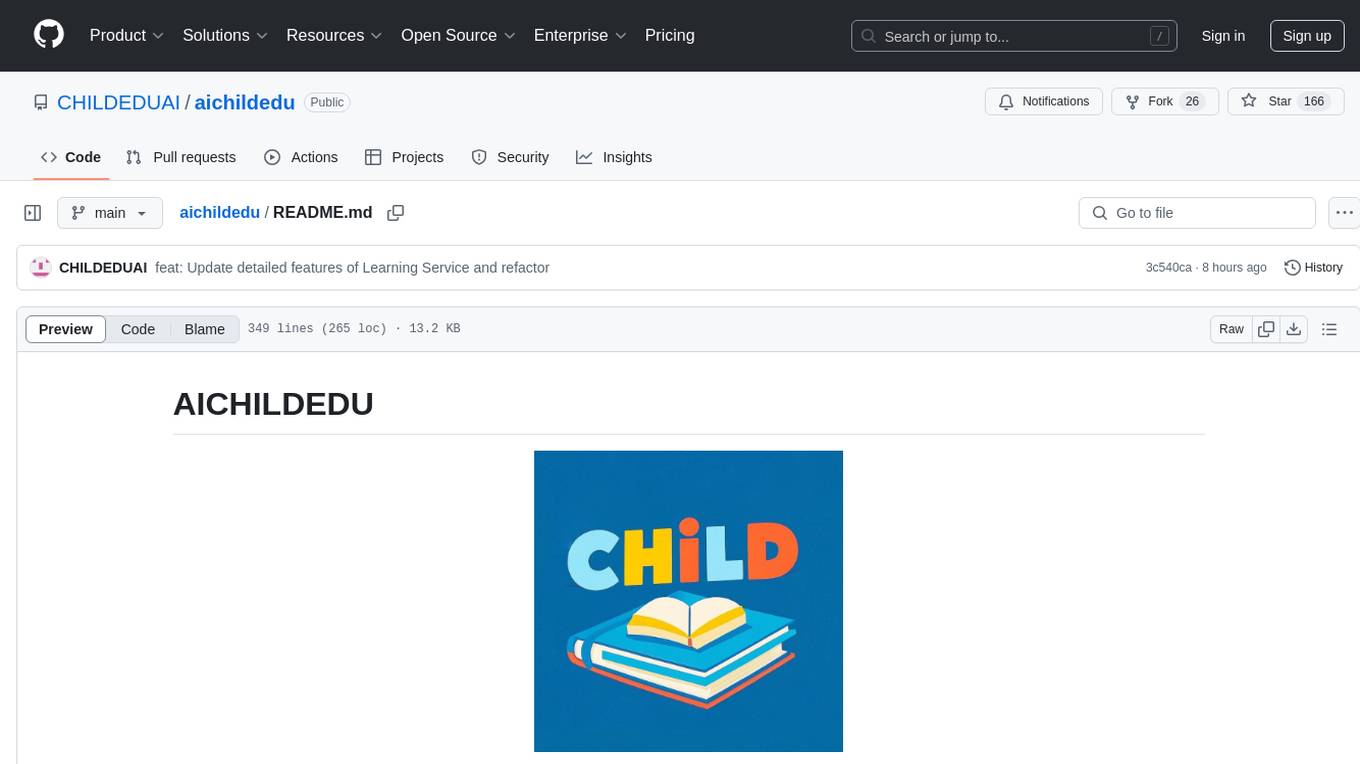
README:
A microservice-based AI education platform for children that integrates LLMs, image generation, and speech synthesis to provide personalized storybook creation, intelligent conversational learning, and multimedia content generation.
AICHILDEDU is an innovative educational platform designed specifically for children, leveraging the power of AI to create engaging, personalized, and educational content. The platform uses a microservice architecture to deliver a variety of AI-powered educational experiences, allowing for flexible scaling and feature expansion.
- Personalized Story Generation: Create custom educational stories tailored to specific age groups, themes, and educational focuses
- Educational Quiz Creation: Generate engaging quizzes and questions that reinforce learning objectives
- Multimedia Integration: Combine text, images, voice, and video into comprehensive educational materials
- Age-Appropriate Content: Content customization based on age groups and developmental stages
- Multi-Language Support: Support for content generation in multiple languages
- User Management: Comprehensive user system with parent, teacher, and admin roles
- Parental Controls: Robust parental control features to ensure child-appropriate content
- Asynchronous Processing: Non-blocking task execution for resource-intensive AI operations
AICHILDEDU follows a microservice architecture with the following key components:
┌─────────────────┐
│ API Gateway │
└───────┬─────────┘
│
┌────────┬────────┬────────────────┼────────────────┬────────────────┐
│ │ │ │ │ │
▼ ▼ ▼ ▼ ▼ ▼
┌──────────┐ ┌─────────┐ ┌──────────────┐ ┌──────────────┐ ┌──────────────┐
│ User │ │ Content │ │ Learning │ │Recommendation│ │ Analytics │
│ Service │ │ Service │ │ Service │ │ Service │ │ Service │
└──────────┘ └─────────┘ └──────────────┘ └──────────────┘ └──────────────┘
┌──────────────────────────┐
│ AI Services │
│ │
│ ┌─────────┐ ┌─────────┐ │
│ │ Text │ │ Image │ │
│ │Generator│ │Generator│ │
│ └─────────┘ └─────────┘ │
│ ┌─────────┐ ┌─────────┐ │
│ │ Voice │ │ Video │ │
│ │Generator│ │Generator│ │
│ └─────────┘ └─────────┘ │
└──────────────────────────┘
- Backend: Python, FastAPI, Uvicorn
- Databases: PostgreSQL, MongoDB, Redis
- AI & ML: LangChain, OpenAI GPT models, TensorFlow, PyTorch, Transformers
- Storage: MinIO (Object Storage)
- Search: Elasticsearch
- Containerization: Docker, Docker Compose
- Authentication: JWT-based authentication
- Docker and Docker Compose
- Python 3.10 or higher (for local development)
- OpenAI API key
-
Clone the repository:
git clone https://github.com/CHILDEDUAI/aichildedu.git cd aichildedu
-
Create an
.env
file in the root directory with the following variables:# Database POSTGRES_USER=postgres POSTGRES_PASSWORD=postgres MONGODB_URI=mongodb://mongodb:27017/ MONGODB_DB=aiedu # Authentication SECRET_KEY=your_secret_key_change_in_production # OpenAI OPENAI_API_KEY=your_openai_api_key
-
Start the services using Docker Compose:
docker-compose up -d
-
The API Gateway will be available at
http://localhost:8000
-
Create a virtual environment:
python -m venv venv source venv/bin/activate # On Windows, use `venv\Scripts\activate`
-
Install dependencies:
pip install -r requirements.txt
-
Run the desired service:
uvicorn aichildedu.user_service.main:app --reload --port 8001
import requests
api_url = "http://localhost:8000/api/v1/ai/text/story"
story_request = {
"title": "The Curious Robot",
"theme": "Technology and Friendship",
"age_group": "6-8",
"characters": [
{
"name": "Robo",
"description": "A curious and friendly robot who wants to learn about the world"
},
{
"name": "Mia",
"description": "A smart girl who loves technology and building things"
}
],
"educational_focus": "Introduction to robotics and programming concepts",
"length": "medium",
"language": "en"
}
response = requests.post(api_url, json=story_request)
task = response.json()
print(f"Story generation task created: {task['task_id']}")
print(f"Check status at: {task['status_check_url']}")
import requests
task_id = "task_12345"
status_url = f"http://localhost:8000/api/v1/ai/text/tasks/{task_id}"
response = requests.get(status_url)
status = response.json()
print(f"Task status: {status['status']}")
print(f"Progress: {status['progress']}%")
import requests
task_id = "task_12345"
result_url = f"http://localhost:8000/api/v1/ai/text/tasks/{task_id}/result"
response = requests.get(result_url)
story = response.json()
print(f"Story Title: {story['title']}")
print(f"Summary: {story['summary']}")
print("\nContent:")
print(story['content'])
The API documentation is available at:
- API Gateway:
http://localhost:8000/docs
- User Service:
http://localhost:8001/docs
- Content Service:
http://localhost:8002/docs
- Learning Service:
http://localhost:8003/docs
- AI Text Generator:
http://localhost:8010/docs
- AI Image Generator:
http://localhost:8011/docs
- AI Voice Generator:
http://localhost:8012/docs
- AI Video Generator:
http://localhost:8013/docs
Handles user management, authentication, and authorization. Manages user profiles, child accounts, and parental controls.
Manages educational content creation, storage, retrieval, and organization with the following features:
-
Content Types Management:
- Stories: Interactive educational narratives with character development, themes, and moral lessons
- Quizzes: Customizable question sets with answers, difficulty levels, and scoring mechanisms
- Lessons: Structured learning materials with educational objectives and prerequisites
-
Content Organization:
- Categories: Hierarchical organization of content with parent-child relationships
- Tags: Flexible content labeling for improved discoverability and filtering
- Collections: User-created or curated sets of content (custom, curriculum, featured)
-
Content Filtering and Search:
- Age-appropriate filtering based on min/max age ranges
- Difficulty level filtering (beginner, intermediate, advanced)
- Content rating filtering (G, PG, educational)
- Multi-language support
- Full-text search capabilities
-
Content Metadata:
- Educational value tracking
- Subjects and themes classification
- Reading time estimation
- Word count metrics
-
User Interaction:
- Content reactions (like, favorite, helpful)
- Content sharing and permissions
- User-specific content collections
-
Multimedia Asset Management:
- Storage and retrieval of images, audio, and video assets
- Asset association with educational content
Tracks learning progress, personalized learning paths, and educational achievements with the following features:
-
Learning Path Management:
- Curriculum Design: Structured learning paths based on age groups and subjects
- Prerequisites: Dependency management for learning materials
- Progress Tracking: Real-time monitoring of learning milestones
- Adaptive Learning: Dynamic adjustment of content difficulty based on performance
-
Assessment and Evaluation:
- Progress Assessment: Regular evaluation of learning outcomes
- Skill Level Analysis: Tracking of subject-specific competencies
- Performance Metrics: Detailed analytics of learning activities
- Achievement System: Gamified rewards and badges for accomplishments
-
Personalization Features:
- Learning Style Detection: Identification of individual learning preferences
- Content Recommendations: AI-powered suggestions for next learning steps
- Pace Adjustment: Flexible learning speed based on individual progress
- Interest-Based Learning: Content tailoring based on demonstrated interests
-
Progress Reporting:
- Detailed Analytics: Comprehensive learning progress visualization
- Parent/Teacher Dashboard: Monitoring tools for guardians and educators
- Progress Reports: Regular automated assessment summaries
- Learning Insights: AI-generated recommendations for improvement
-
Collaborative Learning:
- Peer Learning: Facilitated group learning activities
- Social Interaction: Safe, moderated peer communication
- Group Projects: Collaborative educational tasks
- Shared Achievements: Community learning milestones
-
Learning Support:
- AI Tutoring: Intelligent assistance for difficult concepts
- Homework Help: Guided problem-solving support
- Review Sessions: Automated review of challenging materials
- Learning Resources: Additional materials for deeper understanding
Creates educational stories and quizzes with the following features:
- Personalized story generation based on age, theme, and educational focus
- Quiz generation with customizable difficulty levels
- Asynchronous task processing for better resource management
- Template-based content customization
Creates illustrations to accompany educational content:
- Story illustration generation
- Character visualization
- Educational diagrams and charts
Provides audio narration for educational content:
- Story narration with different character voices
- Multi-language support
- Age-appropriate voice adaptation
Creates educational videos and animations:
- Story animations
- Educational concept visualizations
- Interactive learning content
- Fork the repository
- Create a feature branch
- Implement your changes
- Write tests for new features
- Submit a pull request
This project follows PEP 8 style guidelines. Please ensure your code adheres to these standards before submitting PRs.
This project is licensed under the Apache License 2.0 - see the LICENSE file for details.
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for aichildedu
Similar Open Source Tools
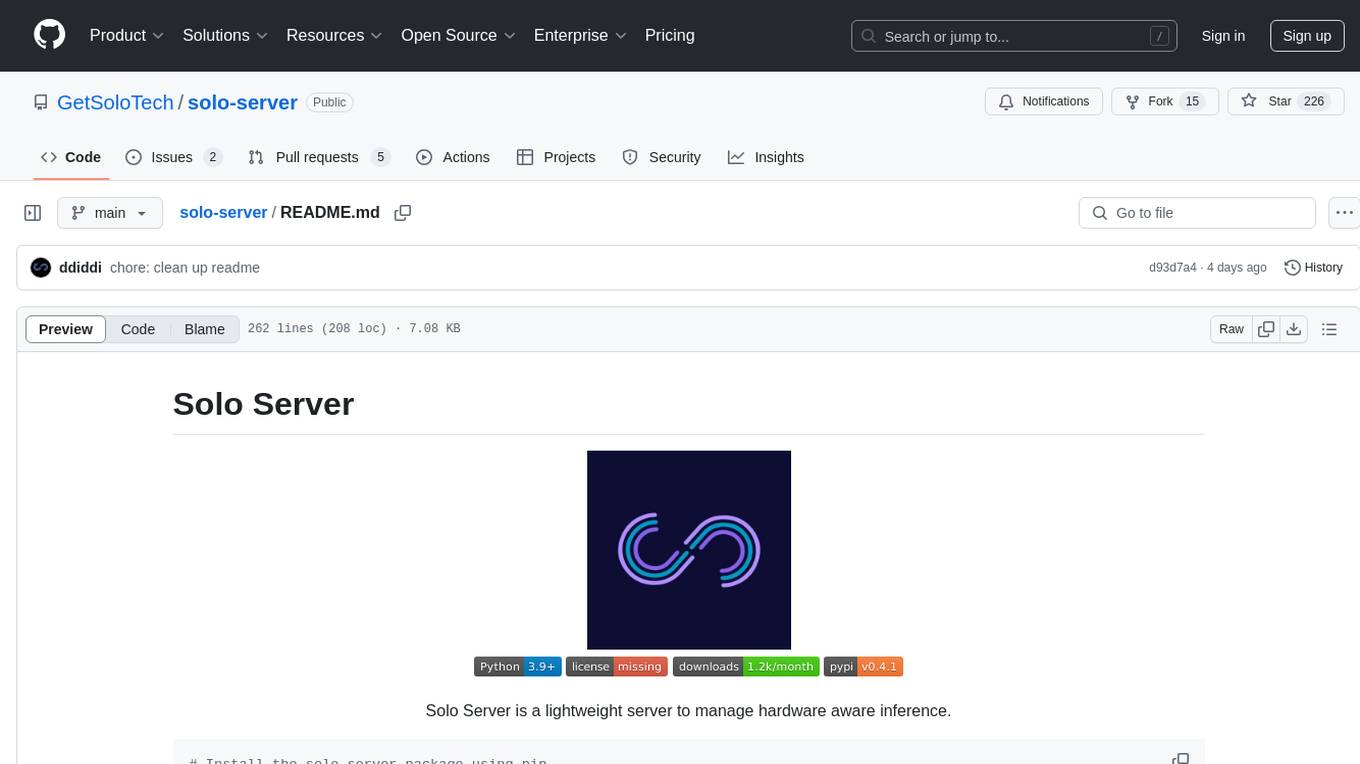
solo-server
Solo Server is a lightweight server designed for managing hardware-aware inference. It provides seamless setup through a simple CLI and HTTP servers, an open model registry for pulling models from platforms like Ollama and Hugging Face, cross-platform compatibility for effortless deployment of AI models on hardware, and a configurable framework that auto-detects hardware components (CPU, GPU, RAM) and sets optimal configurations.
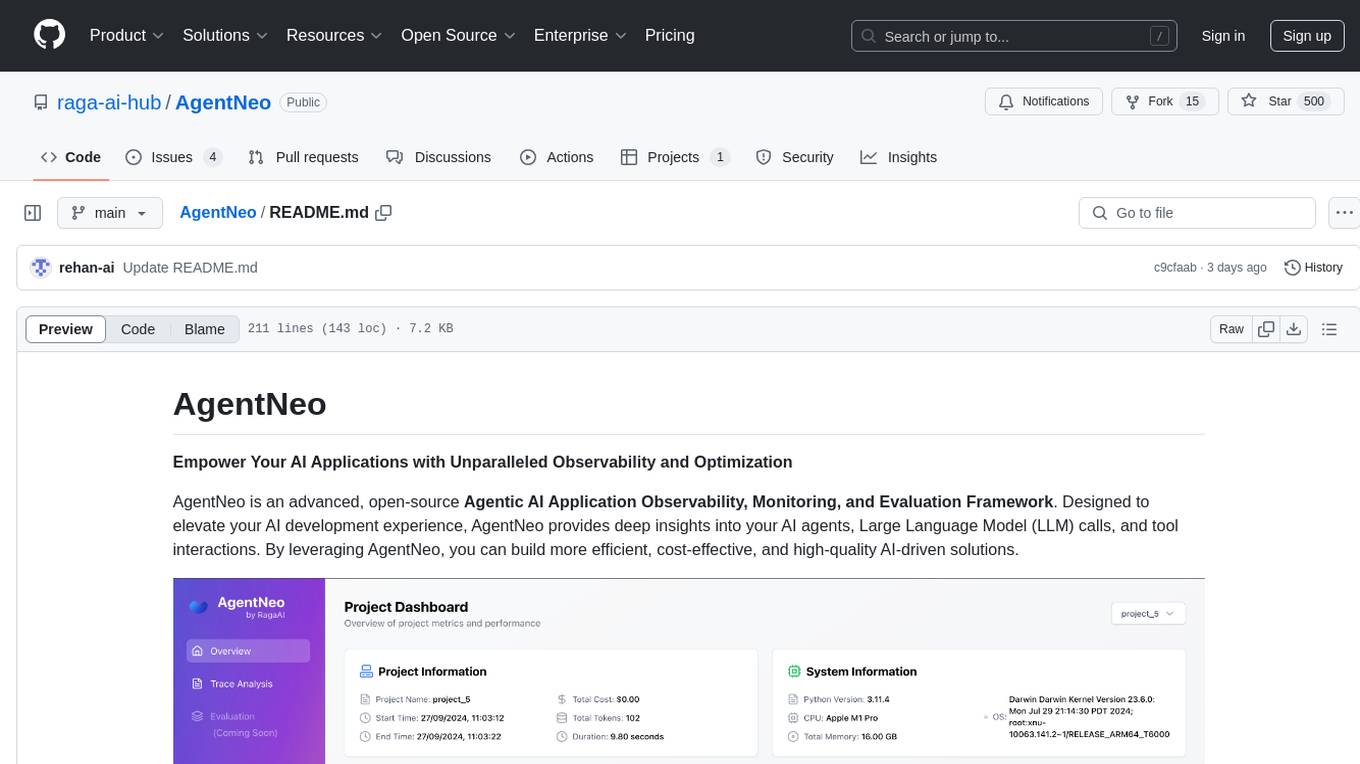
AgentNeo
AgentNeo is an advanced, open-source Agentic AI Application Observability, Monitoring, and Evaluation Framework designed to provide deep insights into AI agents, Large Language Model (LLM) calls, and tool interactions. It offers robust logging, visualization, and evaluation capabilities to help debug and optimize AI applications with ease. With features like tracing LLM calls, monitoring agents and tools, tracking interactions, detailed metrics collection, flexible data storage, simple instrumentation, interactive dashboard, project management, execution graph visualization, and evaluation tools, AgentNeo empowers users to build efficient, cost-effective, and high-quality AI-driven solutions.
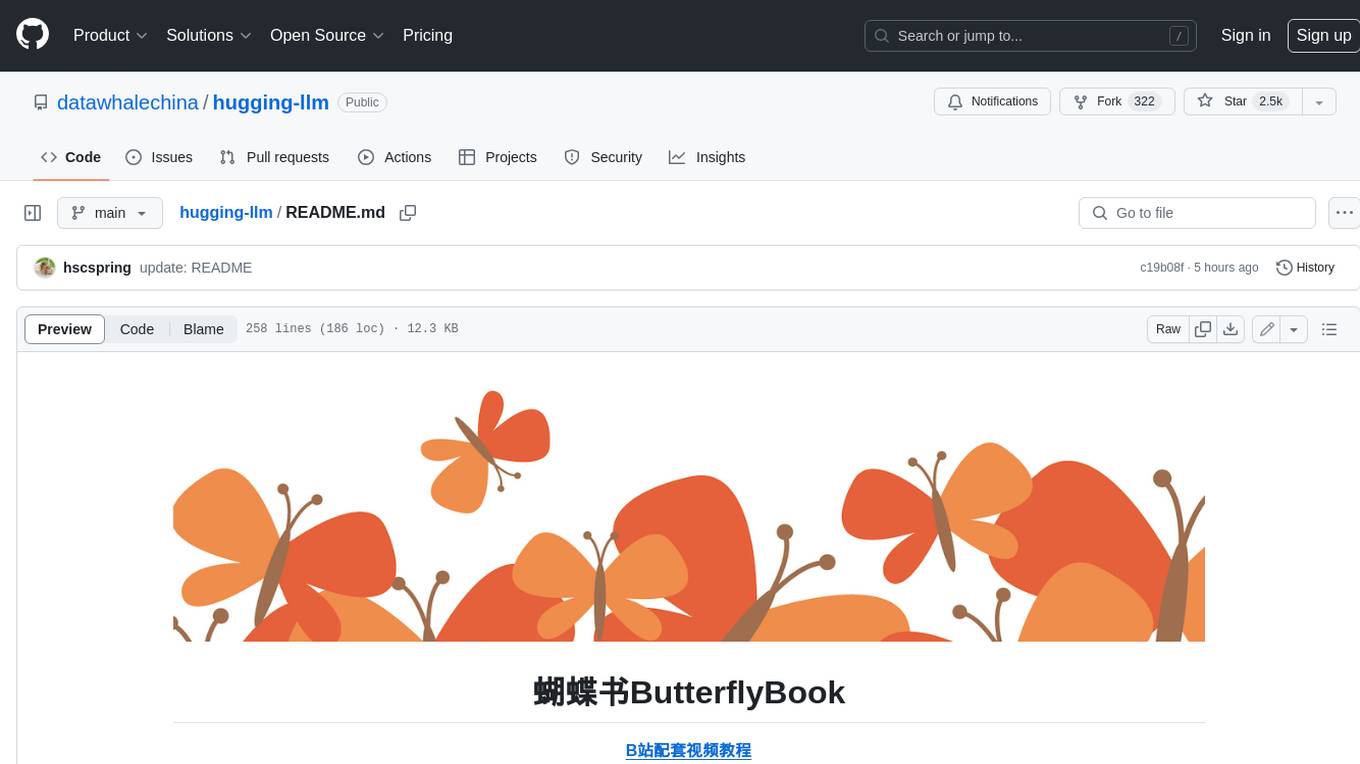
hugging-llm
HuggingLLM is a project that aims to introduce ChatGPT to a wider audience, particularly those interested in using the technology to create new products or applications. The project focuses on providing practical guidance on how to use ChatGPT-related APIs to create new features and applications. It also includes detailed background information and system design introductions for relevant tasks, as well as example code and implementation processes. The project is designed for individuals with some programming experience who are interested in using ChatGPT for practical applications, and it encourages users to experiment and create their own applications and demos.
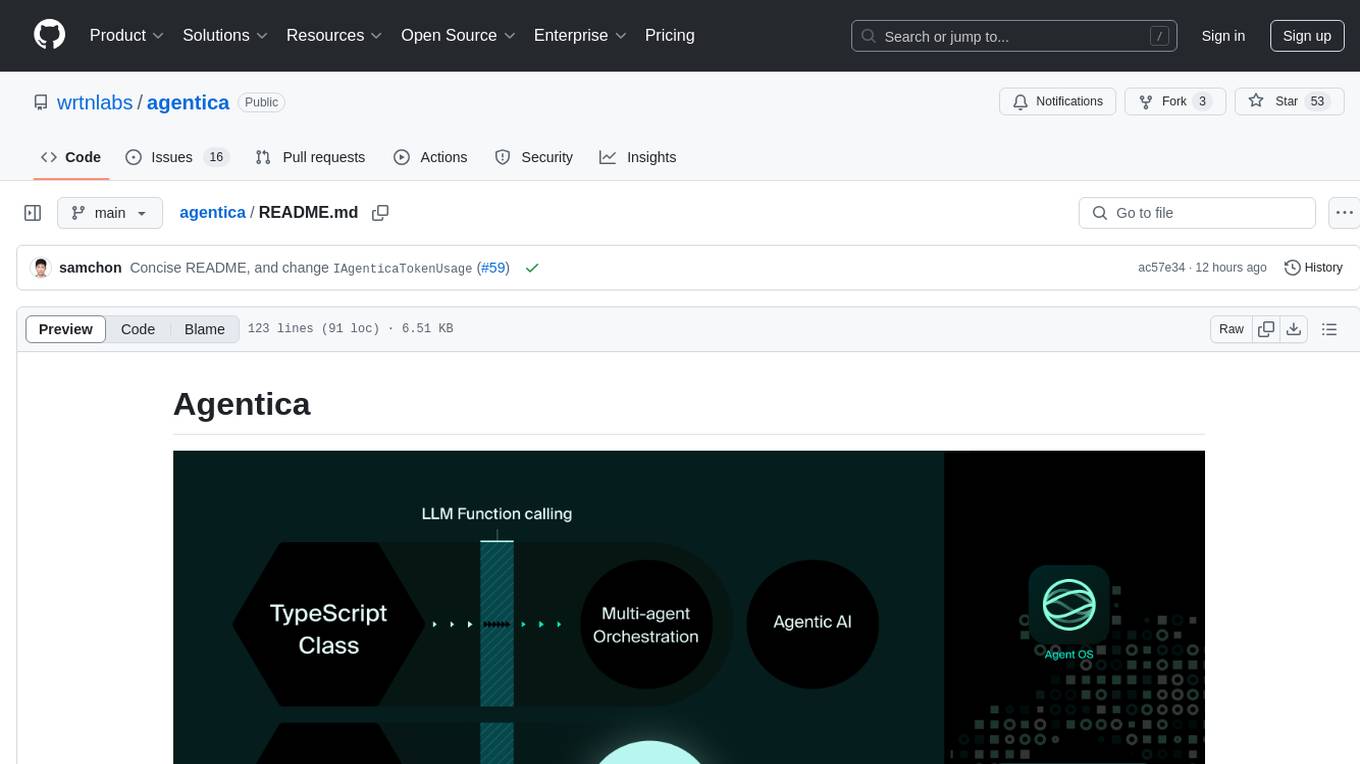
agentica
Agentica is a specialized Agentic AI library focused on LLM Function Calling. Users can provide Swagger/OpenAPI documents or TypeScript class types to Agentica for seamless functionality. The library simplifies AI development by handling various tasks effortlessly.
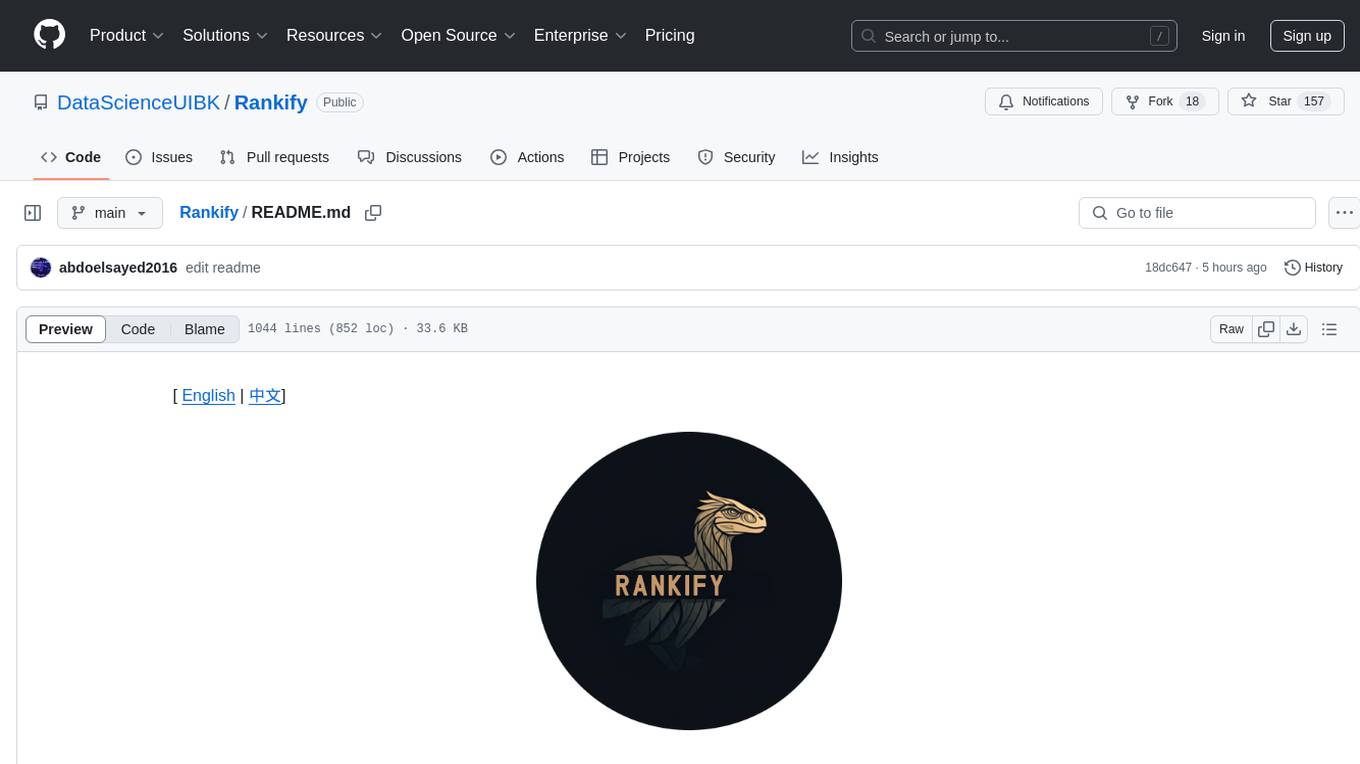
Rankify
Rankify is a Python toolkit designed for unified retrieval, re-ranking, and retrieval-augmented generation (RAG) research. It integrates 40 pre-retrieved benchmark datasets and supports 7 retrieval techniques, 24 state-of-the-art re-ranking models, and multiple RAG methods. Rankify provides a modular and extensible framework, enabling seamless experimentation and benchmarking across retrieval pipelines. It offers comprehensive documentation, open-source implementation, and pre-built evaluation tools, making it a powerful resource for researchers and practitioners in the field.
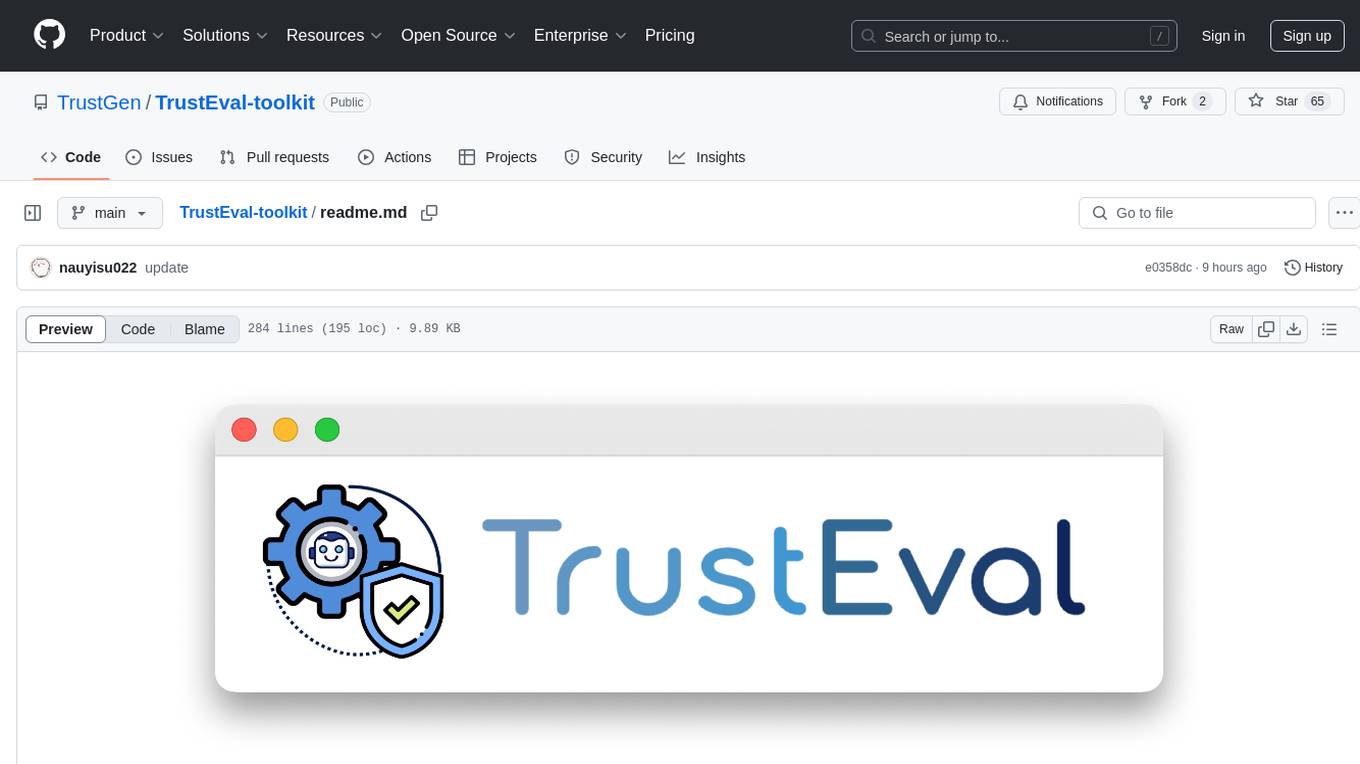
TrustEval-toolkit
TrustEval-toolkit is a dynamic and comprehensive framework for evaluating the trustworthiness of Generative Foundation Models (GenFMs) across dimensions such as safety, fairness, robustness, privacy, and more. It offers features like dynamic dataset generation, multi-model compatibility, customizable metrics, metadata-driven pipelines, comprehensive evaluation dimensions, optimized inference, and detailed reports.
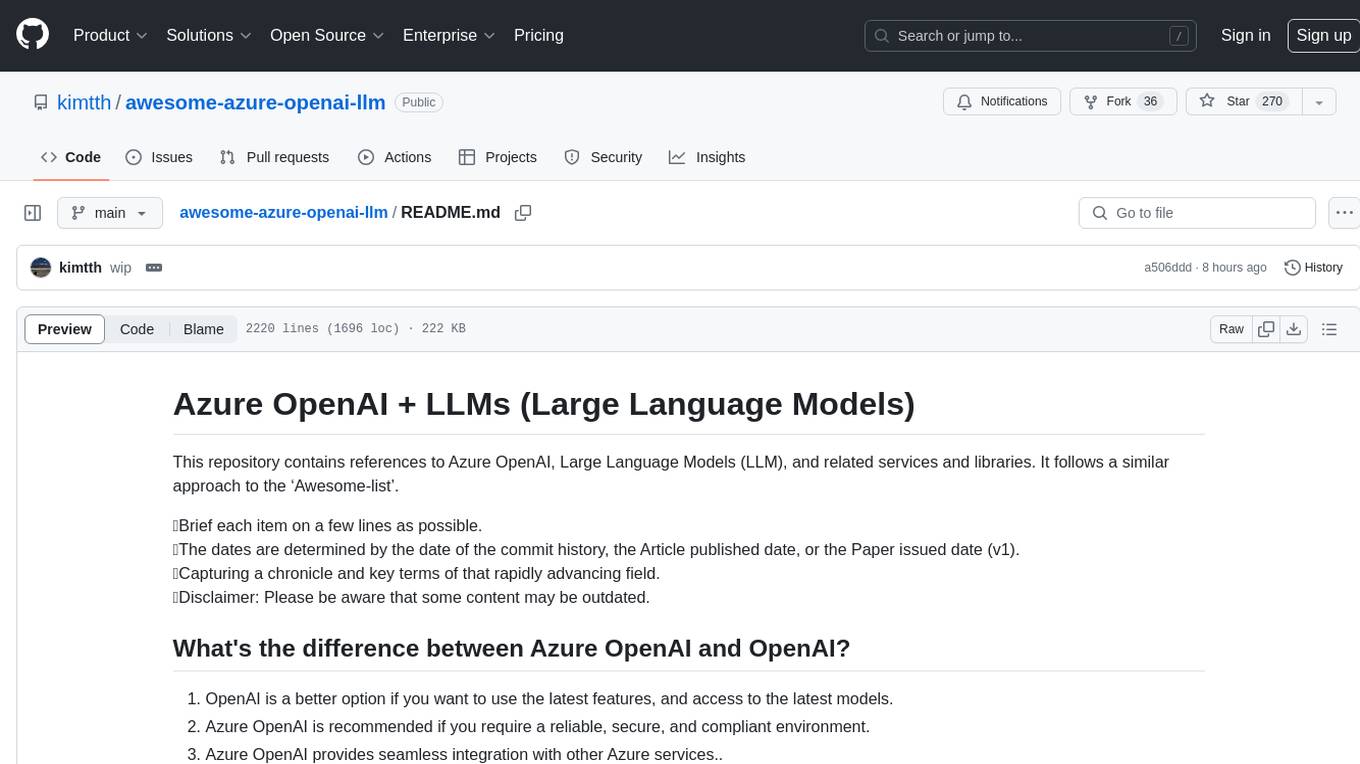
awesome-azure-openai-llm
This repository is a collection of references to Azure OpenAI, Large Language Models (LLM), and related services and libraries. It provides information on various topics such as RAG, Azure OpenAI, LLM applications, agent design patterns, semantic kernel, prompting, finetuning, challenges & abilities, LLM landscape, surveys & references, AI tools & extensions, datasets, and evaluations. The content covers a wide range of topics related to AI, machine learning, and natural language processing, offering insights into the latest advancements in the field.
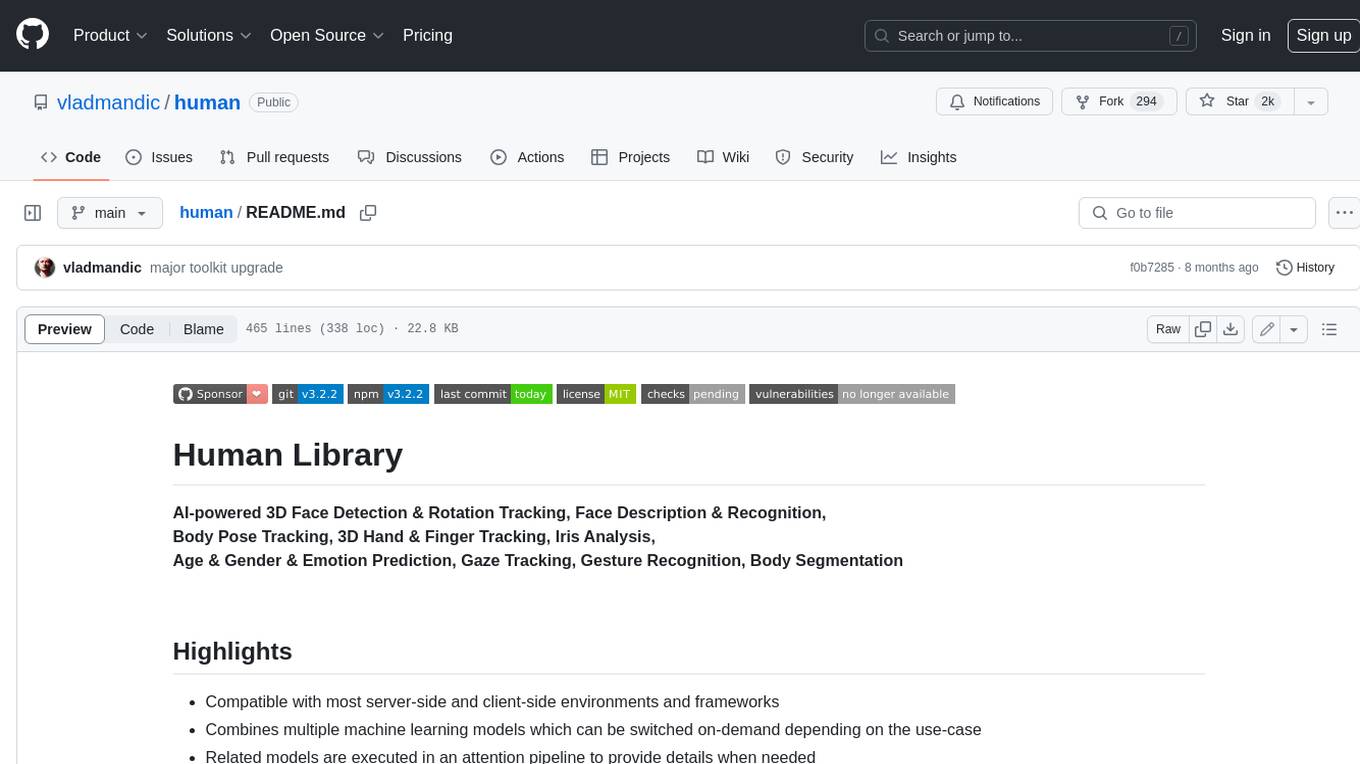
human
AI-powered 3D Face Detection & Rotation Tracking, Face Description & Recognition, Body Pose Tracking, 3D Hand & Finger Tracking, Iris Analysis, Age & Gender & Emotion Prediction, Gaze Tracking, Gesture Recognition, Body Segmentation
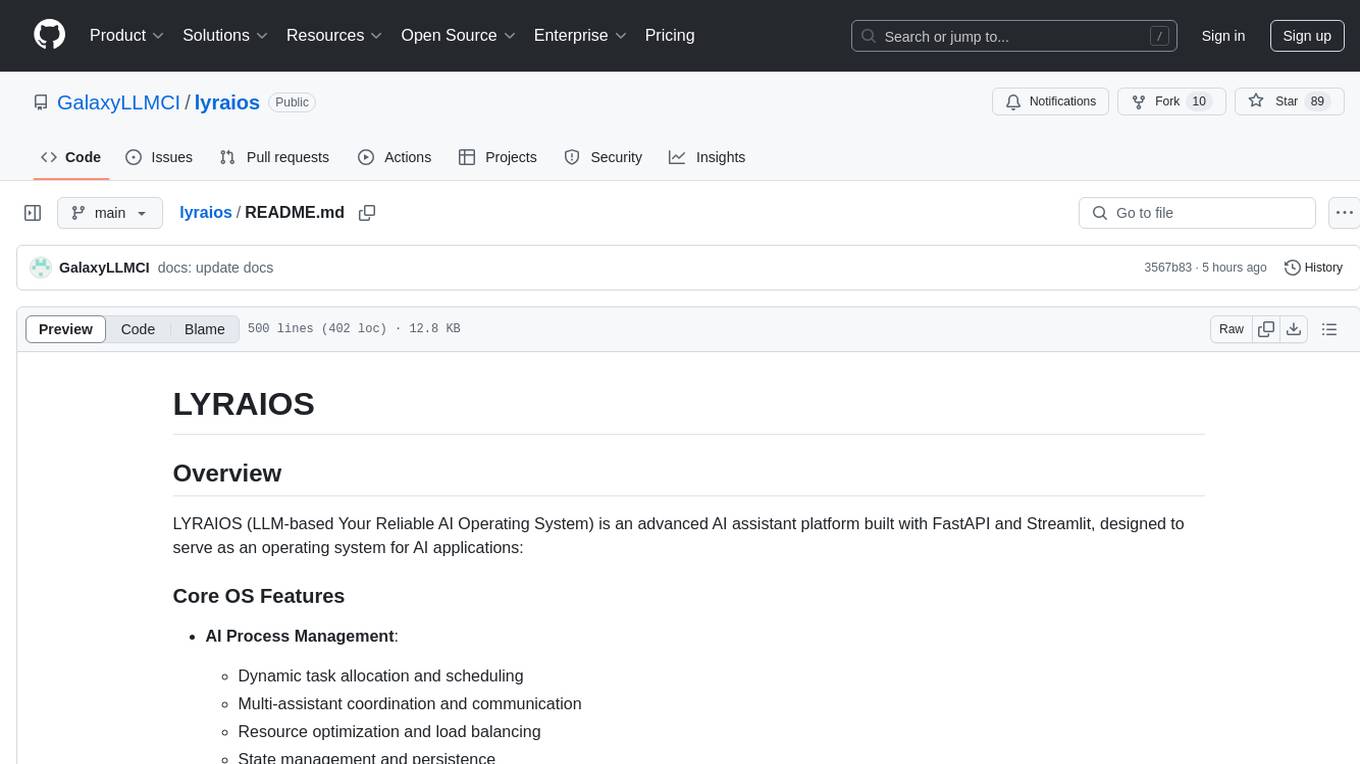
lyraios
LYRAIOS (LLM-based Your Reliable AI Operating System) is an advanced AI assistant platform built with FastAPI and Streamlit, designed to serve as an operating system for AI applications. It offers core features such as AI process management, memory system, and I/O system. The platform includes built-in tools like Calculator, Web Search, Financial Analysis, File Management, and Research Tools. It also provides specialized assistant teams for Python and research tasks. LYRAIOS is built on a technical architecture comprising FastAPI backend, Streamlit frontend, Vector Database, PostgreSQL storage, and Docker support. It offers features like knowledge management, process control, and security & access control. The roadmap includes enhancements in core platform, AI process management, memory system, tools & integrations, security & access control, open protocol architecture, multi-agent collaboration, and cross-platform support.
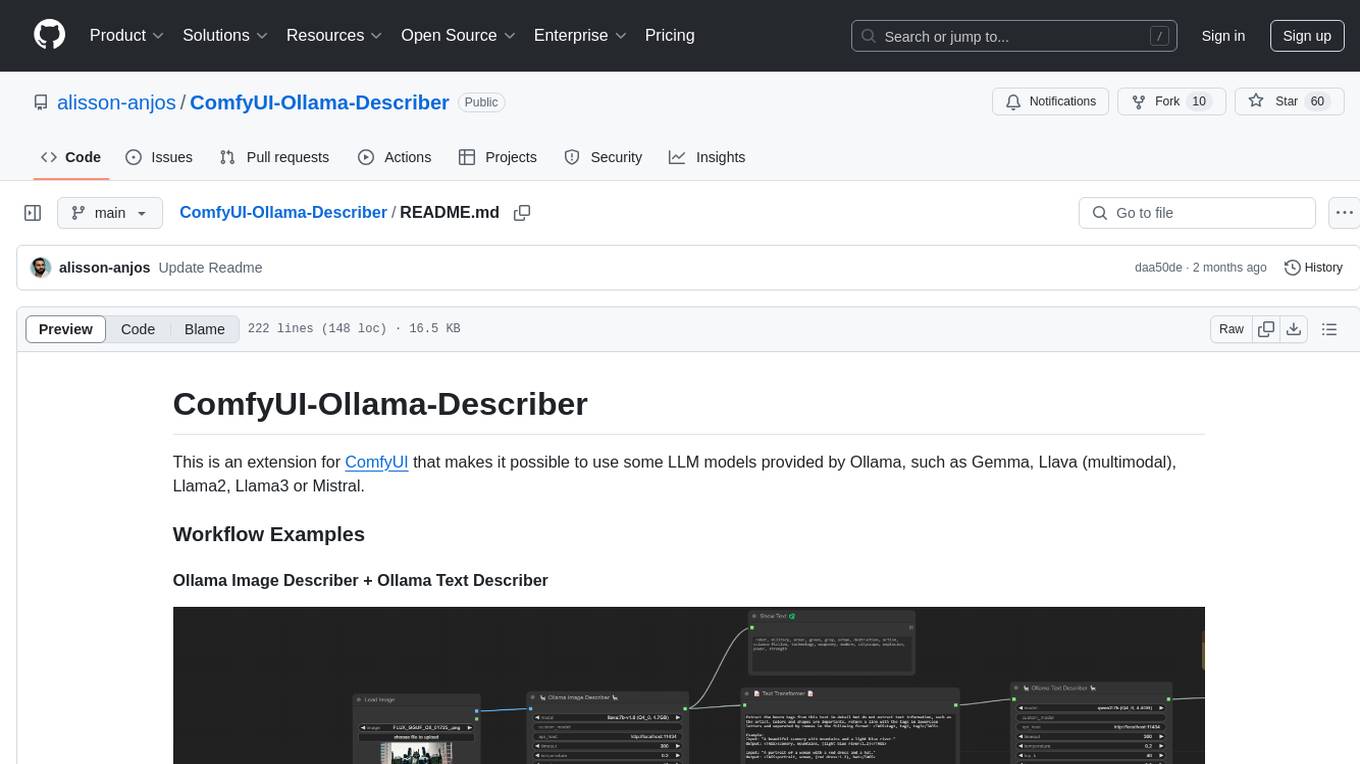
ComfyUI-Ollama-Describer
ComfyUI-Ollama-Describer is an extension for ComfyUI that enables the use of LLM models provided by Ollama, such as Gemma, Llava (multimodal), Llama2, Llama3, or Mistral. It requires the Ollama library for interacting with large-scale language models, supporting GPUs using CUDA and AMD GPUs on Windows, Linux, and Mac. The extension allows users to run Ollama through Docker and utilize NVIDIA GPUs for faster processing. It provides nodes for image description, text description, image captioning, and text transformation, with various customizable parameters for model selection, API communication, response generation, and model memory management.
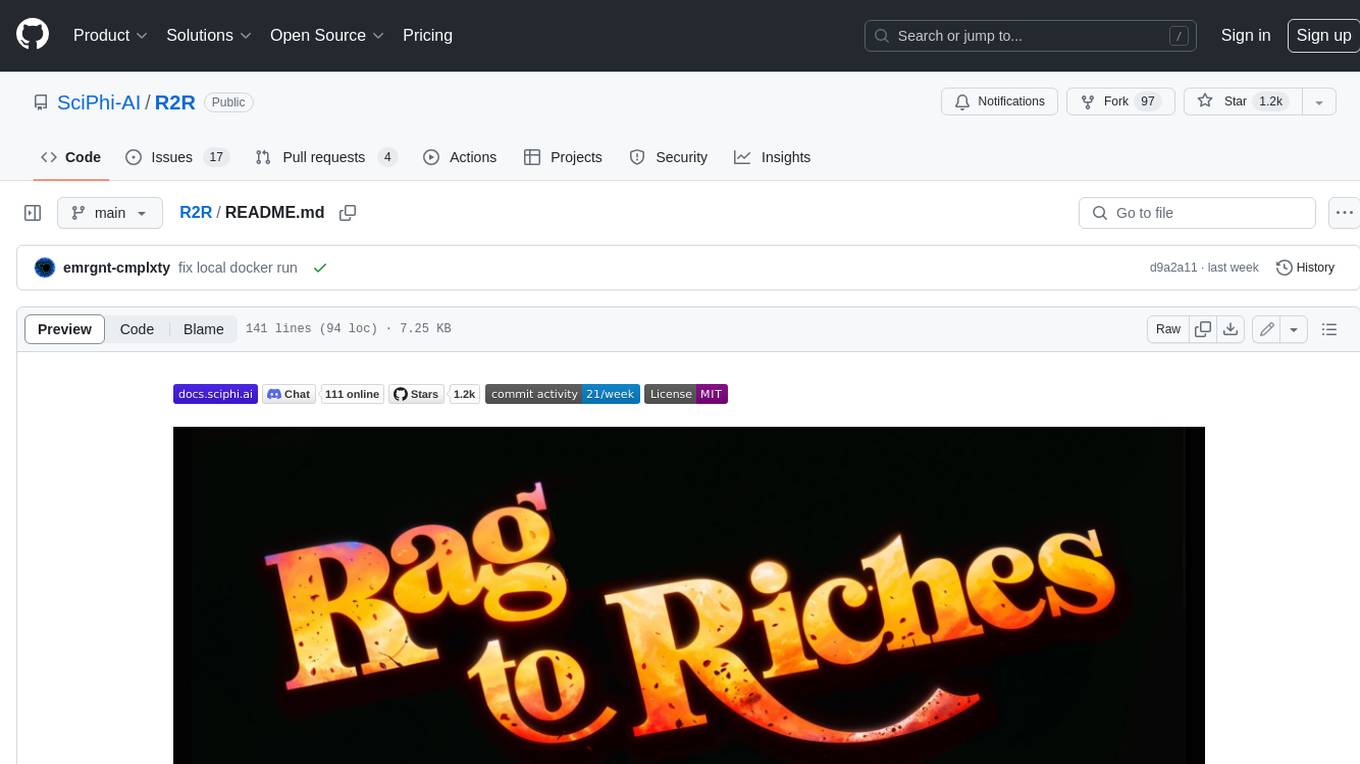
R2R
R2R (RAG to Riches) is a fast and efficient framework for serving high-quality Retrieval-Augmented Generation (RAG) to end users. The framework is designed with customizable pipelines and a feature-rich FastAPI implementation, enabling developers to quickly deploy and scale RAG-based applications. R2R was conceived to bridge the gap between local LLM experimentation and scalable production solutions. **R2R is to LangChain/LlamaIndex what NextJS is to React**. A JavaScript client for R2R deployments can be found here. ### Key Features * **🚀 Deploy** : Instantly launch production-ready RAG pipelines with streaming capabilities. * **🧩 Customize** : Tailor your pipeline with intuitive configuration files. * **🔌 Extend** : Enhance your pipeline with custom code integrations. * **⚖️ Autoscale** : Scale your pipeline effortlessly in the cloud using SciPhi. * **🤖 OSS** : Benefit from a framework developed by the open-source community, designed to simplify RAG deployment.