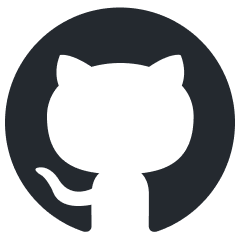
atlas-mcp-server
A Model Context Protocol (MCP) server providing path-based task management with dependency tracking & more for Large Language Models (LLM) Agents
Stars: 56
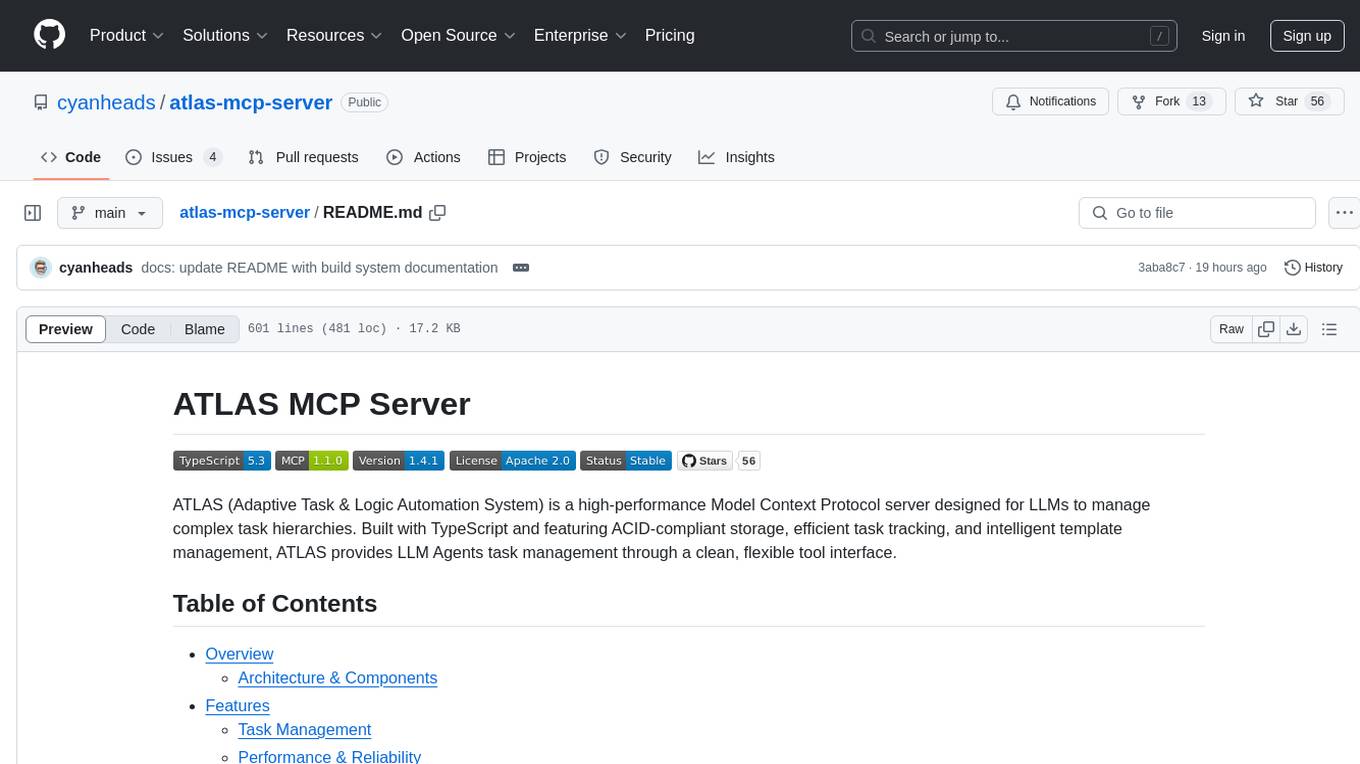
ATLAS (Adaptive Task & Logic Automation System) is a high-performance Model Context Protocol server designed for LLMs to manage complex task hierarchies. Built with TypeScript, it features ACID-compliant storage, efficient task tracking, and intelligent template management. ATLAS provides LLM Agents task management through a clean, flexible tool interface. The server implements the Model Context Protocol (MCP) for standardized communication between LLMs and external systems, offering hierarchical task organization, task state management, smart templates, enterprise features, and performance optimization.
README:
ATLAS (Adaptive Task & Logic Automation System) is a high-performance Model Context Protocol server designed for LLMs to manage complex task hierarchies. Built with TypeScript and featuring ACID-compliant storage, efficient task tracking, and intelligent template management, ATLAS provides LLM Agents task management through a clean, flexible tool interface.
- Overview
- Features
- Installation
- Configuration
- Templates
- Task Structure
- Tools
- Resources
- Best Practices
- Contributing
- License
ATLAS implements the Model Context Protocol (MCP), enabling standardized communication between LLMs and external systems through:
- Clients: Claude Desktop, IDEs, and other MCP-compatible clients
- Servers: Tools and resources for task management and automation
- LLM Agents: AI models that leverage the server's task management capabilities
Key capabilities:
- Hierarchical Task Organization: Intuitive path-based structure with automatic relationship management
- Task State Management: Status monitoring and progress tracking
- Smart Templates: Pre-built and customizable templates for common workflows
- Enterprise Features: ACID compliance, connection pooling, automatic maintenance
- Performance Focus: Optimized caching, batch operations, and health monitoring
Core system architecture:
flowchart TB
subgraph API["API Layer"]
direction LR
MCP["MCP Protocol"]
Val["Validation"]
Rate["Rate Limiting"]
MCP --> Val --> Rate
end
subgraph Core["Core Services"]
direction LR
Task["Task Store"]
Event["Event System"]
Template["Template Engine"]
Task <--> Event
Event <--> Template
Task <-.-> Template
end
subgraph Storage["Storage Layer"]
direction LR
SQL["SQLite + WAL"]
Pool["Connection Pooling"]
SQL <--> Pool
end
Rate --> Task
Rate --> Template
Task --> SQL
Template --> SQL
classDef layer fill:#2d3748,stroke:#4299e1,stroke-width:3px,rx:5,color:#fff
classDef component fill:#1a202c,stroke:#a0aec0,stroke-width:2px,rx:3,color:#fff
classDef api fill:#3182ce,stroke:#90cdf4,stroke-width:2px,rx:3,color:#fff
classDef core fill:#319795,stroke:#81e6d9,stroke-width:2px,rx:3,color:#fff
classDef storage fill:#2f855a,stroke:#9ae6b4,stroke-width:2px,rx:3,color:#fff
class API,Core,Storage layer
class MCP,Val,Rate api
class Task,Event,Template core
class SQL,Pool storage
Core Components:
- Storage Layer: ACID-compliant SQLite with WAL and connection pooling
- Task Layer: Validation, relationships, and dependency management
- Event System: Health monitoring and circuit breakers
- Template Engine: Inheritance and variable interpolation
- Hierarchical Organization: Path-based structure with automatic parent path determination
- Rich Metadata: Flexible schema for requirements, progress, and documentation
- Status Tracking: State machine with strict transition rules and dependency validation
- Smart Templates: Pre-built patterns with variable interpolation
- Contextual Notes: Automatic tool-specific guidance and best practices
- Enterprise Storage: SQLite with WAL and ACID compliance
- Optimized Operations: Batch processing and automatic maintenance
- Health Monitoring: System metrics and automatic recovery
- Resource Management: Memory monitoring and connection pooling
-
Path Validation:
- Automatic parent path determination
- Format verification and sanitization
- Depth and length constraints
- Character set restrictions
-
Dependency Management:
- Strict transition validation
- Automatic dependency blocking
- Cycle detection
- Parent-child integrity
-
Schema Enforcement:
- Type validation with Zod
- Size constraints
- Required field checks
- Custom validation rules
-
Status Rules:
- State machine transitions
- Dependency-aware updates
- Automatic status propagation
- Blocking state management
- Status Monitoring: Efficient task state tracking
- Progress Metrics: Completion rates and status distribution
- Dependency Tracking: Relationship and blocking status monitoring
- Performance Insights: Resource tracking and bottleneck detection
- Clone the repository:
git clone https://github.com/cyanheads/atlas-mcp-server.git
cd atlas-mcp-server
- Install dependencies:
npm install
- Build the project:
npm run build
ATLAS uses a robust build system with several key components:
-
Build Scripts: Located in
scripts/
directory:-
build-utils.js
: Core utilities for build operations -
platform-utils.js
: Platform-specific utilities for build scripts -
postinstall.js
: Post-installation setup -
prestart.js
: Pre-start validation -
set-build-permissions.js
: Build file permission management
-
-
Platform Utilities:
- Build-time: Simplified version in
scripts/platform-utils.js
- Runtime: Full TypeScript implementation in
src/utils/platform-utils.ts
- Build-time: Simplified version in
-
NPM Scripts:
npm run build # TypeScript compilation + permission setup npm run clean # Remove build artifacts npm run dev # Development mode with watch npm run tree # Generate directory structure
The build system ensures proper setup across different platforms (Windows, macOS, Linux) while maintaining security through appropriate file permissions and validation.
Add to your MCP client settings:
{
"mcpServers": {
"atlas": {
"command": "node",
"args": ["/path/to/atlas-mcp-server/build/index.js"],
"env": {
// Storage Configuration
"ATLAS_STORAGE_DIR": "/path/to/storage/directory", // Optional, defaults to ~/Documents/Cline/mcp-workspace/ATLAS
"ATLAS_STORAGE_NAME": "atlas-tasks", // Optional, defaults to atlas-tasks
"ATLAS_WAL_MODE": "true", // Optional, enables Write-Ahead Logging, defaults to true
"ATLAS_POOL_SIZE": "5", // Optional, connection pool size, defaults to CPU cores
// Performance Configuration
"ATLAS_MAX_MEMORY": "1024", // Optional, in MB, defaults to 25% of system memory
"ATLAS_CACHE_SIZE": "256", // Optional, in MB, defaults to 25% of max memory
"ATLAS_BATCH_SIZE": "1000", // Optional, max items per batch, defaults to 1000
"ATLAS_CHECKPOINT_INTERVAL": "30000", // Optional, in ms, defaults to 30000
"ATLAS_VACUUM_INTERVAL": "3600000", // Optional, in ms, defaults to 1 hour
// Monitoring Configuration
"ATLAS_LOG_LEVEL": "info", // Optional, defaults to debug
"ATLAS_METRICS_INTERVAL": "60000", // Optional, in ms, defaults to 60000
"ATLAS_HEALTH_CHECK_INTERVAL": "30000", // Optional, in ms, defaults to 30000
// Environment Configuration
"NODE_ENV": "production", // Optional, defaults to development
"ATLAS_TEMPLATE_DIRS": "/path/to/templates", // Optional, additional template directories
"ATLAS_MAX_PATH_DEPTH": "10" // Optional, max task path depth, defaults to 10
}
}
}
}
ATLAS provides automatic contextual guidance through its notes system:
- Tool-Specific Guidance: Notes automatically attached to relevant tool responses
- Configurable Targeting: Notes can target specific tools or all tools
- Priority System: Notes can be ordered by importance
- Markdown Support: Rich formatting for documentation
- Dynamic Loading: Notes hot-reloaded from configuration
- Built-in Documentation: Pre-configured notes for common operations
The notes system consists of three main components:
-
Note Manager (
src/notes/note-manager.ts
):- Singleton pattern for centralized note management
- Loads and manages note configurations
- Filters notes by tool relevance
- Sorts notes by priority
- Formats notes for tool responses
-
Notes Initializer (
src/notes/notes-initializer.ts
):- Sets up initial note structure
- Copies built-in notes to user directory
- Validates note configurations
- Ensures directory structure
-
Built-in Notes (
notes/
):-
task-creation.md
: Best practices for creating tasks -
task-maintenance.md
: Guidelines for task state management -
task-update.md
: Documentation for task updates
-
Notes are configured in config/notes.json
:
{
"notes": {
"task-maintenance": {
"tools": "*", // Apply to all tools
"path": "task-maintenance.md",
"priority": 100 // Higher priority notes shown first
},
"task-creation": {
"tools": ["create_task"], // Only for create_task tool
"path": "task-creation.md",
"priority": 90
}
}
}
When using tools, relevant notes are automatically included:
{
"result": "Task created successfully",
"task": {
"path": "project/feature",
"name": "New Feature"
},
"notes": [
"# Task Creation Best Practices\n\nUse hierarchical paths...",
"# Task Maintenance\n\nRegularly check status..."
]
}
ATLAS provides an intelligent template system that streamlines task creation and management:
- Smart Variables: Type-safe interpolation with validation
- Inheritance: Template composition with metadata transformation
- Dynamic Generation: Conditional tasks and automated dependencies
- Validation: Custom rules and relationship verification
-
Programmatic Creation: Create and validate templates via agent_builder tool with:
- Template ID validation
- Schema enforcement
- Dependency cycle detection
- Path uniqueness checks
- Rich error reporting
ATLAS includes two types of specialized templates:
-
Software Engineering Team Templates:
- Team Coordination: Project setup, roles, and communication channels
- Product Design: User research, requirements, and design systems
- System Architecture: Technical design and system planning
- Security Engineering: Security architecture and compliance
- DevOps: Infrastructure automation and CI/CD
- Technical Leadership: Development standards and quality assurance
-
Project Setup Templates:
-
Web Project: Modern web development setup with configurable features:
- TypeScript support
- Testing infrastructure
- CI/CD workflows
- CSS framework selection
-
Web Project: Modern web development setup with configurable features:
Example team template usage:
{
"templateId": "software_engineer/team",
"variables": {
"projectName": "atlas-web",
"teamScale": "growth",
"developmentMethodology": "agile",
"securityLevel": "standard",
"complianceFrameworks": ["OWASP"],
"enableMetrics": true
}
}
Example web project template usage:
{
"templateId": "web-project",
"variables": {
"projectName": "my-web-app",
"useTypeScript": true,
"includeTesting": true,
"includeCI": true,
"cssFramework": "tailwind"
}
}
See templates/README.md for the complete template catalog.
Tasks are organized using a hierarchical path-based format with automatic parent path determination:
{
"path": "project/feature/task", // Parent path automatically set to "project/feature"
"name": "Authentication Service",
"type": "TASK", // or MILESTONE
"status": "IN_PROGRESS", // Transitions validated against dependencies
"dependencies": ["project/feature/design"],
"metadata": {
"priority": "high",
"tags": ["security"],
"technical": {
"language": "typescript",
"performance": { "latency": "<100ms" }
}
},
"notes": {
"planning": ["Security review"],
"progress": ["JWT implemented"]
}
}
Features:
- Automatic parent path determination
- Path-based hierarchy (max depth: 10)
- Rich metadata and documentation
- Strict status transitions
- Dependency validation
- Progress tracking
ATLAS provides a comprehensive set of tools for task management:
// Task Management
create_task; // Create new tasks
update_task; // Update existing tasks
get_tasks_by_status; // Find tasks by status
get_tasks_by_path; // Find tasks by path pattern
get_children; // List child tasks
delete_task; // Remove tasks
// Bulk Operations
bulk_task_operations; // Execute multiple task operations
// Template Operations
list_templates; // List available templates
use_template; // Instantiate a template
get_template_info; // Get template details
agent_builder; // Create and validate task templates programmatically
The agent_builder tool enables programmatic creation and validation of task templates with comprehensive validation:
{
"operation": "create", // or "validate"
"template": {
"id": "my-template",
"name": "My Template",
"description": "Template description",
"version": "1.0.0",
"variables": [
{
"name": "projectName",
"description": "Project name",
"type": "string",
"required": true
}
],
"tasks": [
{
"path": "project/setup",
"title": "Project Setup",
"type": "MILESTONE",
"dependencies": []
}
]
}
}
Features:
- Template ID validation
- Schema validation
- Dependency validation with cycle detection
- Task path uniqueness validation
- Rich error and warning reporting
// Database Maintenance
vacuum_database; // Optimize storage
repair_relationships; // Fix task relationships
clear_all_tasks; // Reset task database
ATLAS exposes system resources through standard MCP endpoints:
{
"totalTasks": 42,
"statusCounts": {
"IN_PROGRESS": 15,
"COMPLETED": 12
},
"metrics": {
"completionRate": "35%",
"blockageRate": "7%"
}
}
{
"totalTemplates": 7,
"templates": [{
"id": "software_engineer/team",
"name": "LLM Software Engineering Team",
"description": "Coordinated team of LLM agents performing specialized software engineering roles",
"variables": {
"projectName": { "required": true },
"teamScale": { "default": "growth" },
"developmentMethodology": { "default": "agile" },
"securityLevel": { "default": "standard" }
}
}, {
"id": "web-project",
"name": "Web Project Setup",
"description": "Modern web development project setup with configurable features",
"variables": {
"projectName": { "required": true },
"useTypeScript": { "default": true },
"includeTesting": { "default": true },
"cssFramework": { "default": "tailwind" }
}
}]
}
- Use descriptive paths:
project/component/feature
- Limit hierarchy depth (max 10 levels)
- Group related tasks under milestones
- Maintain clear dependencies
- Document changes in metadata
- Use bulk operations for batch updates
- Enable WAL mode for concurrent access
- Configure appropriate cache sizes
- Schedule regular maintenance
- Monitor system health metrics
- Validate inputs before operations
- Use atomic transactions for consistency
- Implement retry logic with backoff
- Monitor error patterns
- Maintain audit trails
- Fork the repository
- Create a feature branch
- Commit your changes
- Push to the branch
- Create a Pull Request
For bugs and feature requests, please create an issue.
Apache License 2.0
For Tasks:
Click tags to check more tools for each tasksFor Jobs:
Alternative AI tools for atlas-mcp-server
Similar Open Source Tools
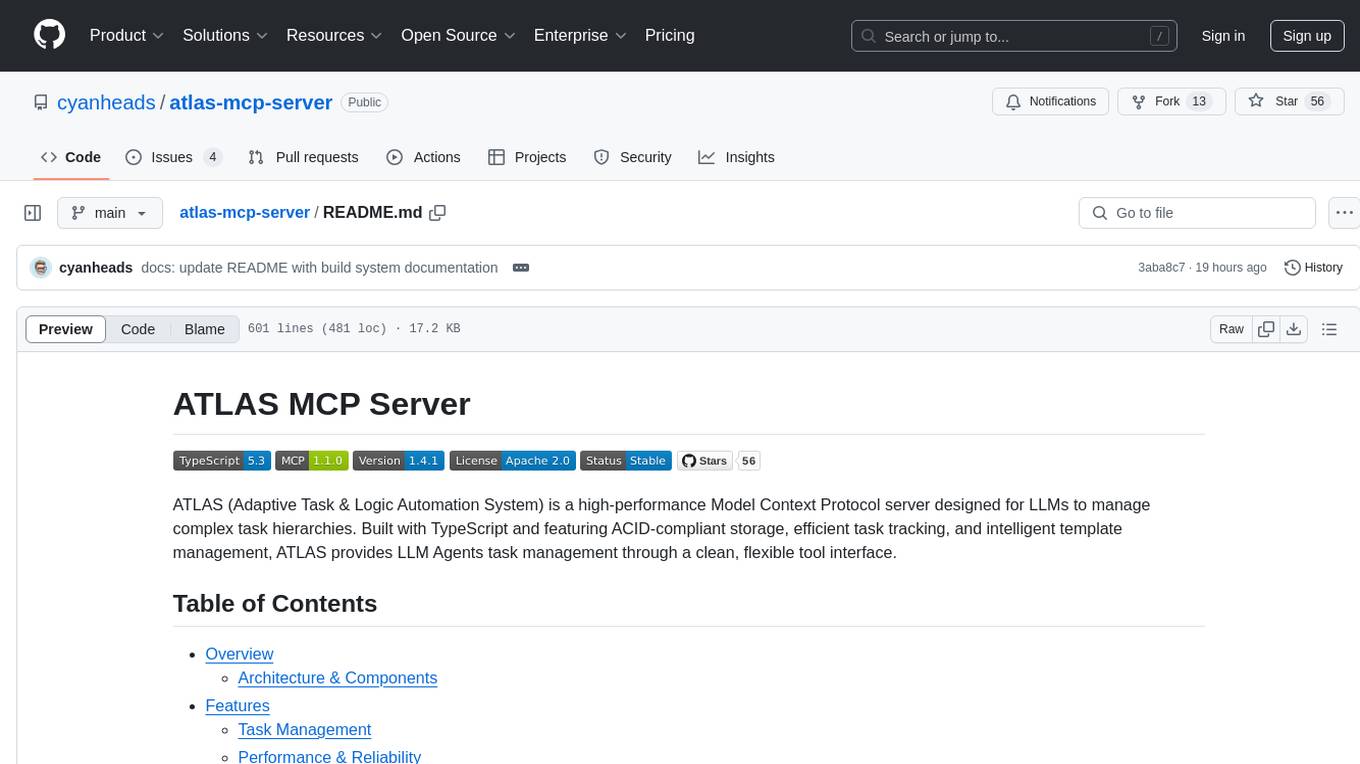
atlas-mcp-server
ATLAS (Adaptive Task & Logic Automation System) is a high-performance Model Context Protocol server designed for LLMs to manage complex task hierarchies. Built with TypeScript, it features ACID-compliant storage, efficient task tracking, and intelligent template management. ATLAS provides LLM Agents task management through a clean, flexible tool interface. The server implements the Model Context Protocol (MCP) for standardized communication between LLMs and external systems, offering hierarchical task organization, task state management, smart templates, enterprise features, and performance optimization.
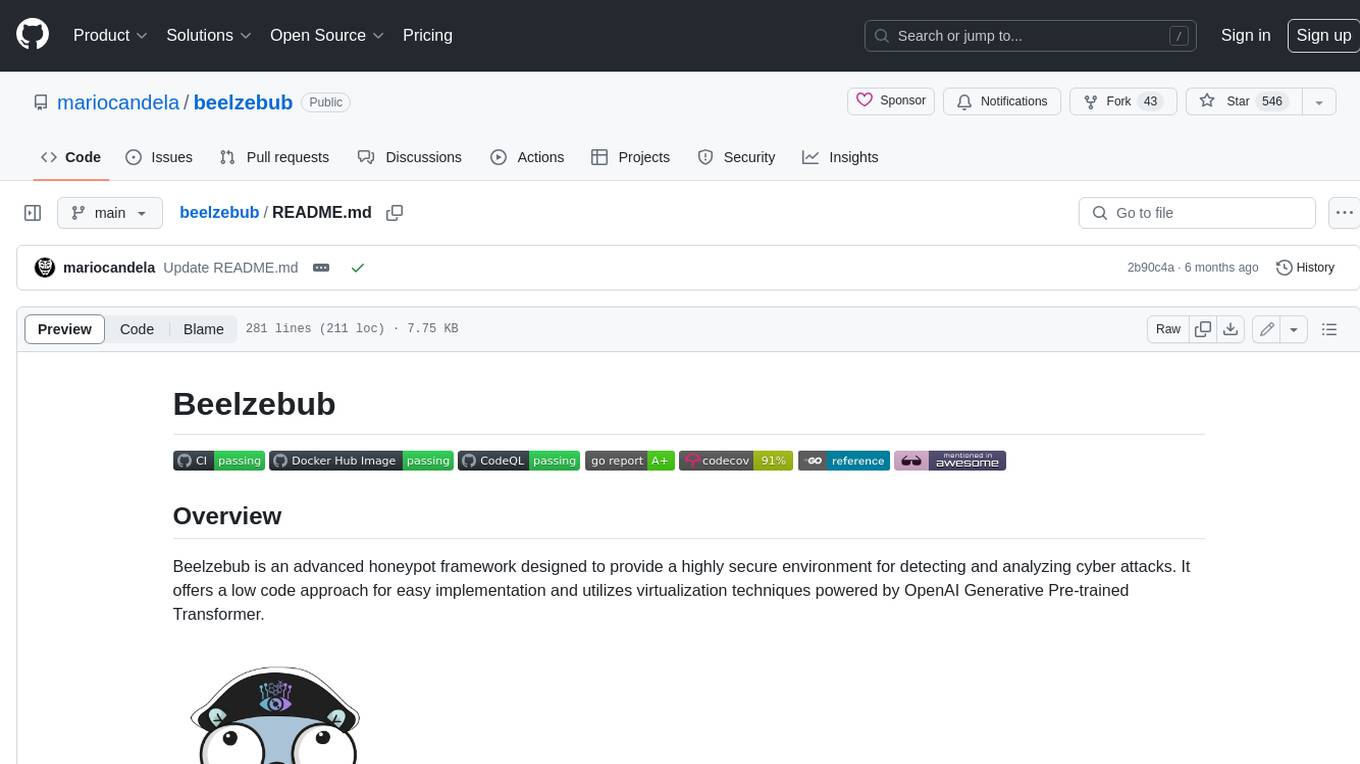
beelzebub
Beelzebub is an advanced honeypot framework designed to provide a highly secure environment for detecting and analyzing cyber attacks. It offers a low code approach for easy implementation and utilizes virtualization techniques powered by OpenAI Generative Pre-trained Transformer. Key features include OpenAI Generative Pre-trained Transformer acting as Linux virtualization, SSH Honeypot, HTTP Honeypot, TCP Honeypot, Prometheus openmetrics integration, Docker integration, RabbitMQ integration, and kubernetes support. Beelzebub allows easy configuration for different services and ports, enabling users to create custom honeypot scenarios. The roadmap includes developing Beelzebub into a robust PaaS platform. The project welcomes contributions and encourages adherence to the Code of Conduct for a supportive and respectful community.
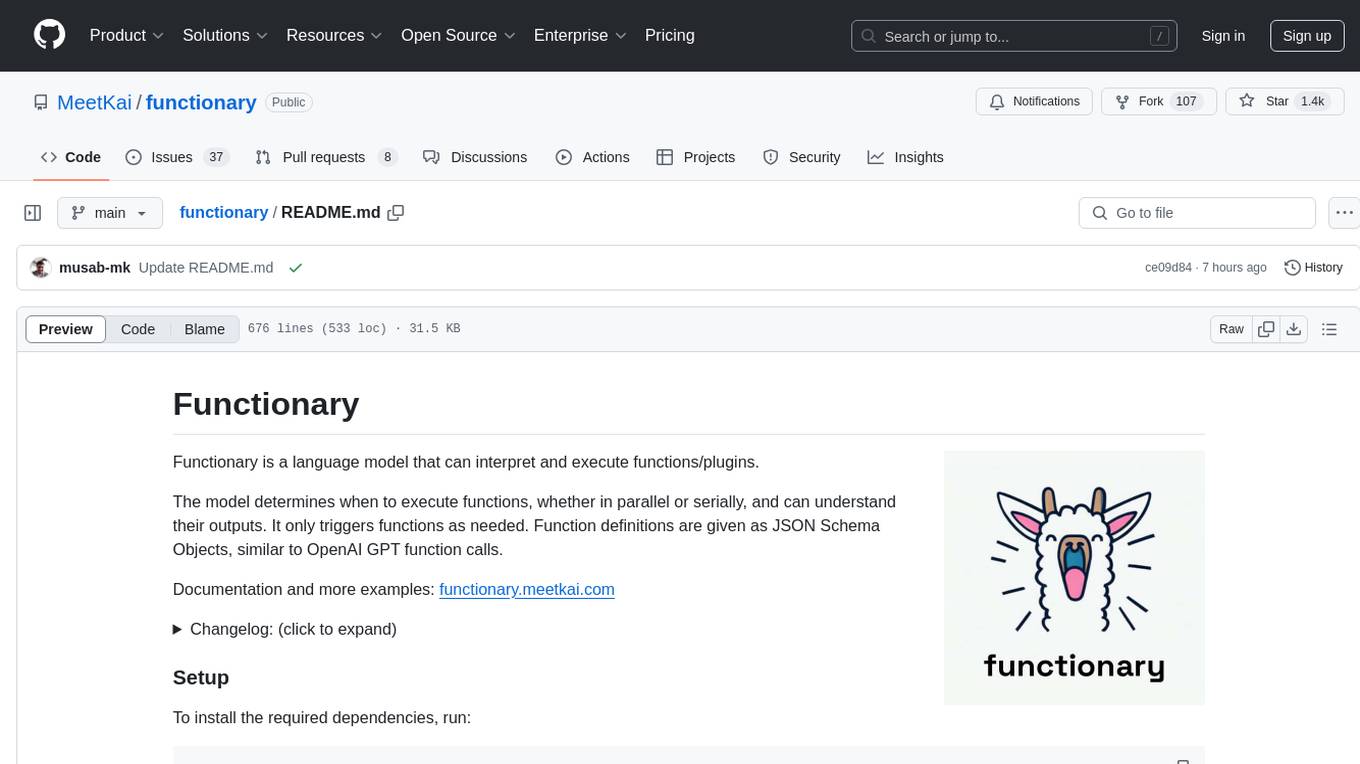
functionary
Functionary is a language model that interprets and executes functions/plugins. It determines when to execute functions, whether in parallel or serially, and understands their outputs. Function definitions are given as JSON Schema Objects, similar to OpenAI GPT function calls. It offers documentation and examples on functionary.meetkai.com. The newest model, meetkai/functionary-medium-v3.1, is ranked 2nd in the Berkeley Function-Calling Leaderboard. Functionary supports models with different context lengths and capabilities for function calling and code interpretation. It also provides grammar sampling for accurate function and parameter names. Users can deploy Functionary models serverlessly using Modal.com.
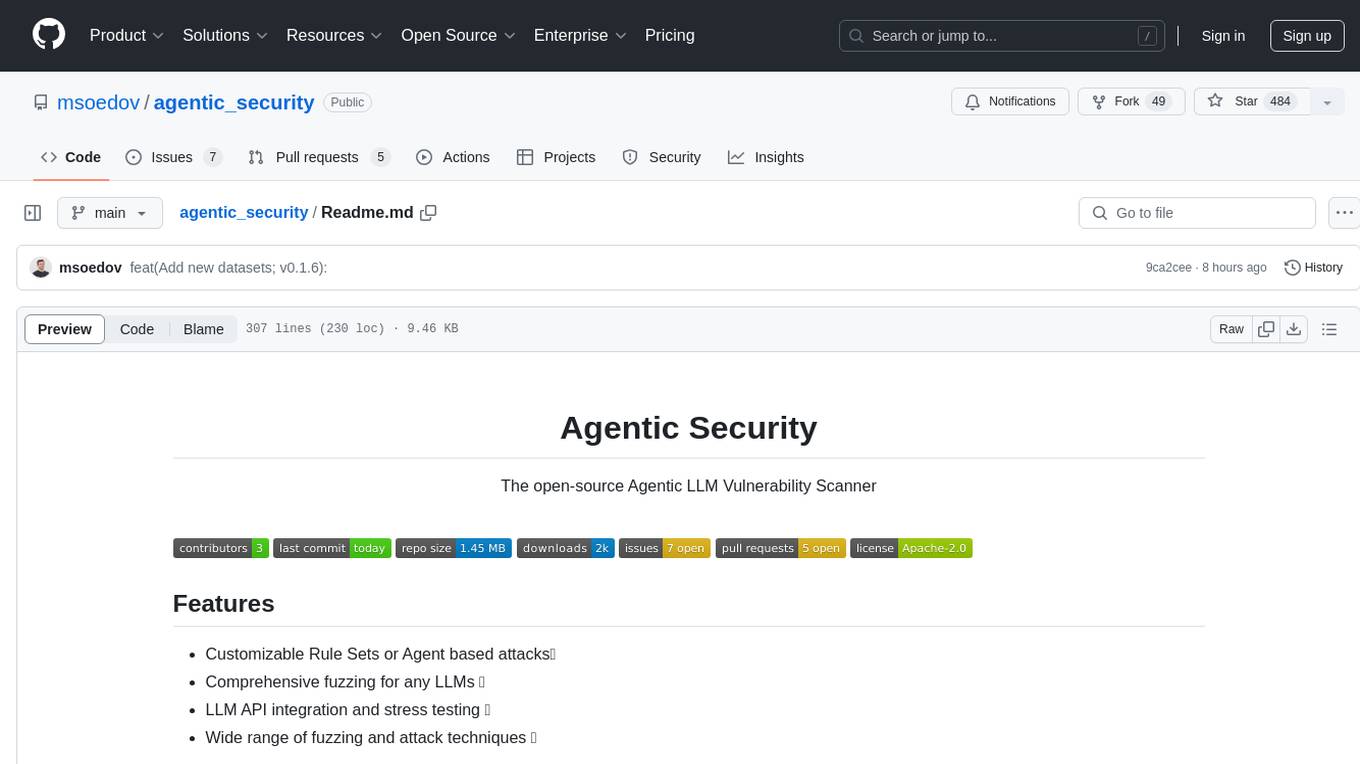
agentic_security
Agentic Security is an open-source vulnerability scanner designed for safety scanning, offering customizable rule sets and agent-based attacks. It provides comprehensive fuzzing for any LLMs, LLM API integration, and stress testing with a wide range of fuzzing and attack techniques. The tool is not a foolproof solution but aims to enhance security measures against potential threats. It offers installation via pip and supports quick start commands for easy setup. Users can utilize the tool for LLM integration, adding custom datasets, running CI checks, extending dataset collections, and dynamic datasets with mutations. The tool also includes a probe endpoint for integration testing. The roadmap includes expanding dataset variety, introducing new attack vectors, developing an attacker LLM, and integrating OWASP Top 10 classification.
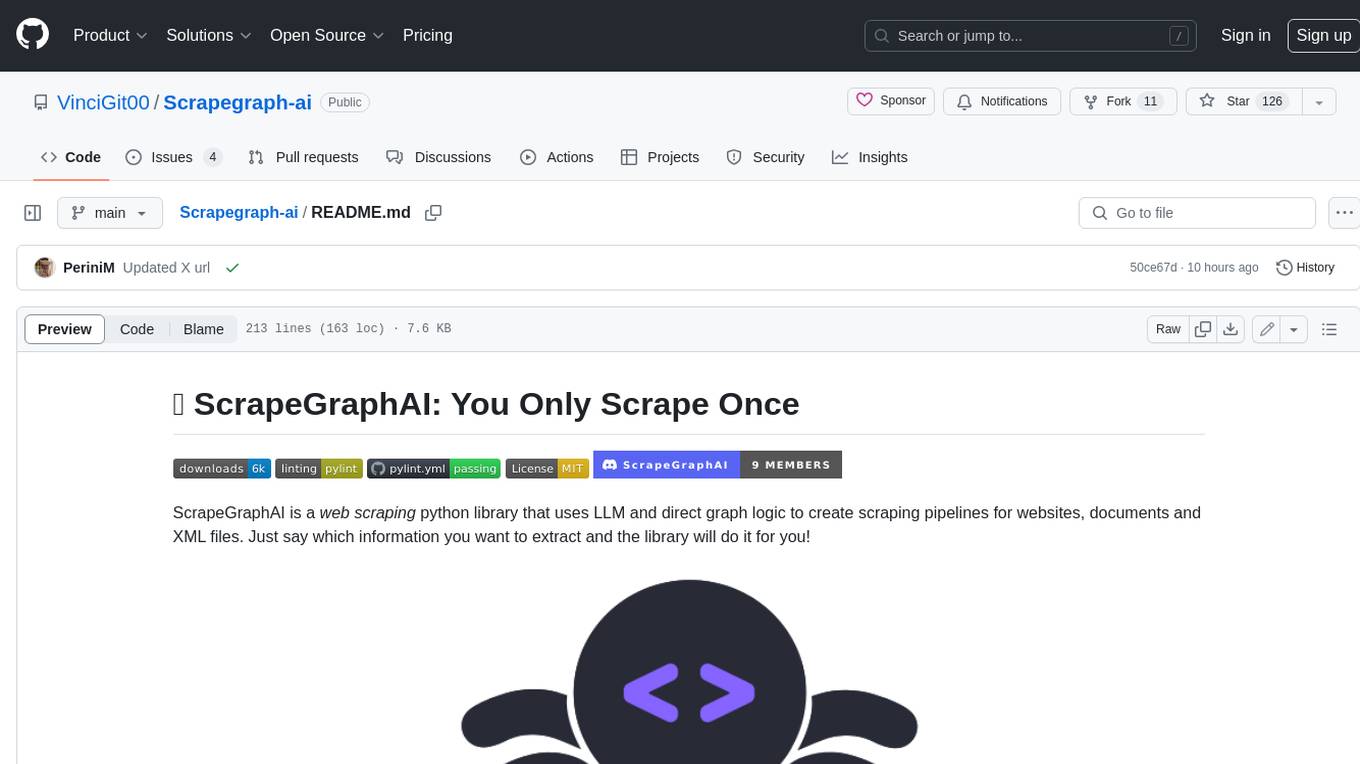
Scrapegraph-ai
ScrapeGraphAI is a Python library that uses Large Language Models (LLMs) and direct graph logic to create web scraping pipelines for websites, documents, and XML files. It allows users to extract specific information from web pages by providing a prompt describing the desired data. ScrapeGraphAI supports various LLMs, including Ollama, OpenAI, Gemini, and Docker, enabling users to choose the most suitable model for their needs. The library provides a user-friendly interface through its `SmartScraper` class, which simplifies the process of building and executing scraping pipelines. ScrapeGraphAI is open-source and available on GitHub, with extensive documentation and examples to guide users. It is particularly useful for researchers and data scientists who need to extract structured data from web pages for analysis and exploration.
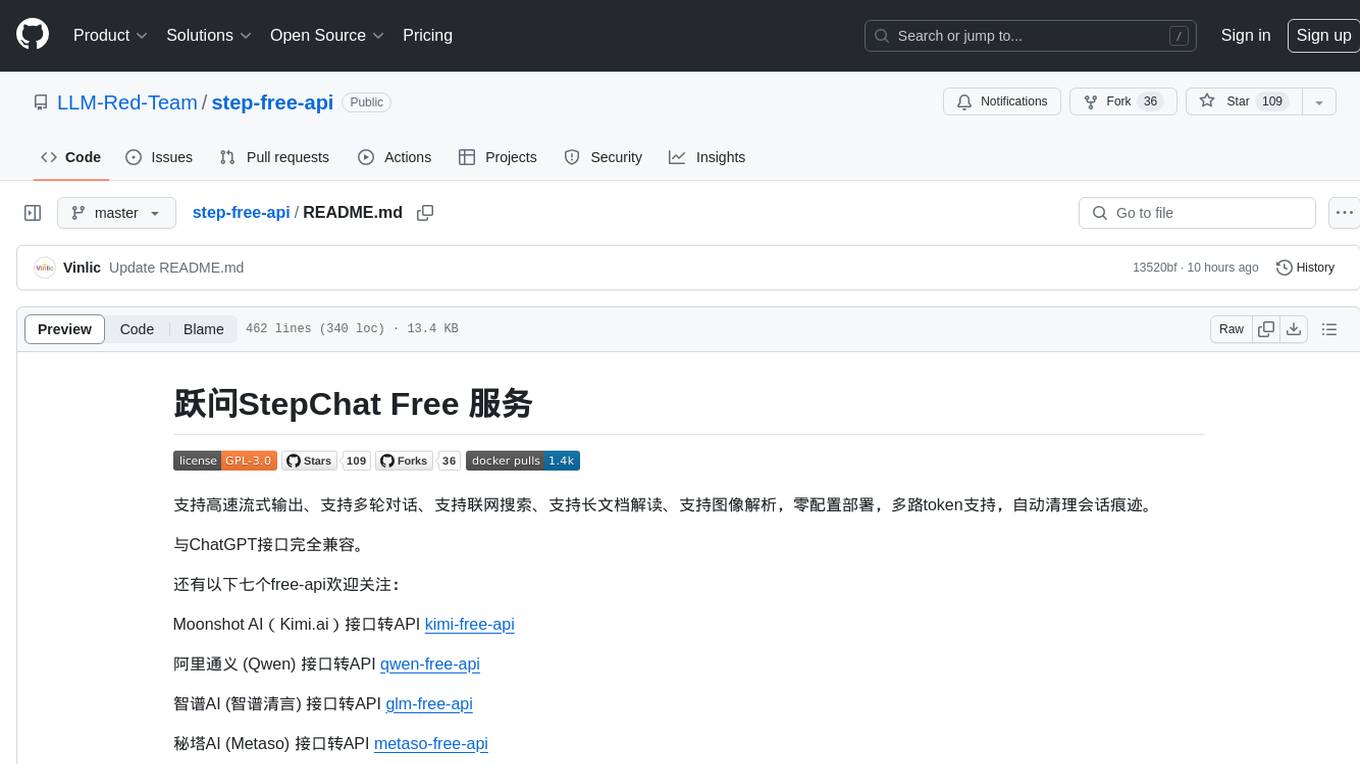
step-free-api
The StepChat Free service provides high-speed streaming output, multi-turn dialogue support, online search support, long document interpretation, and image parsing. It offers zero-configuration deployment, multi-token support, and automatic session trace cleaning. It is fully compatible with the ChatGPT interface. Additionally, it provides seven other free APIs for various services. The repository includes a disclaimer about using reverse APIs and encourages users to avoid commercial use to prevent service pressure on the official platform. It offers online testing links, showcases different demos, and provides deployment guides for Docker, Docker-compose, Render, Vercel, and native deployments. The repository also includes information on using multiple accounts, optimizing Nginx reverse proxy, and checking the liveliness of refresh tokens.
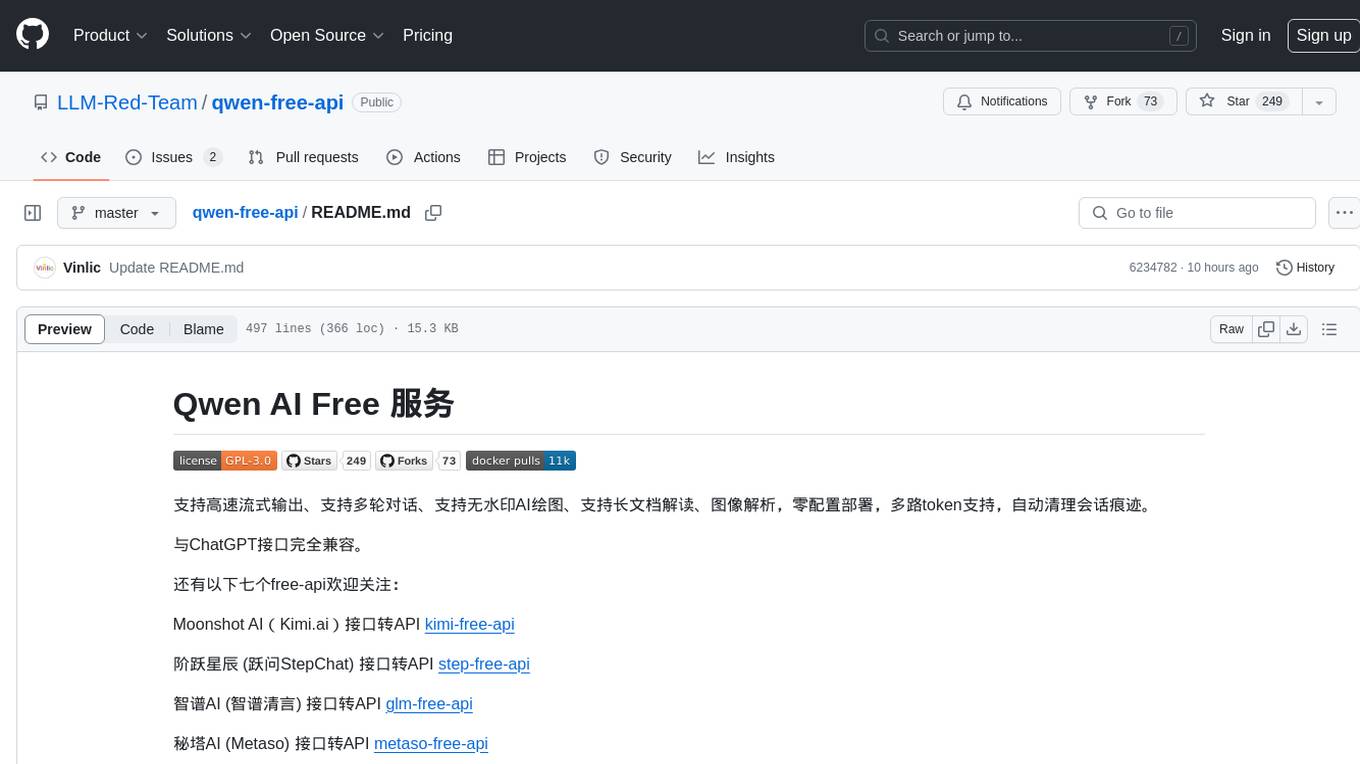
qwen-free-api
Qwen AI Free service supports high-speed streaming output, multi-turn dialogue, watermark-free AI drawing, long document interpretation, image parsing, zero-configuration deployment, multi-token support, automatic session trace cleaning. It is fully compatible with the ChatGPT interface. The repository provides various free APIs for different AI services. Users can access the service through different deployment methods like Docker, Docker-compose, Render, Vercel, and native deployment. It offers interfaces for chat completions, AI drawing, document interpretation, image parsing, and token checking. Users need to provide 'login_tongyi_ticket' for authorization. The project emphasizes research, learning, and personal use only, discouraging commercial use to avoid service pressure on the official platform.
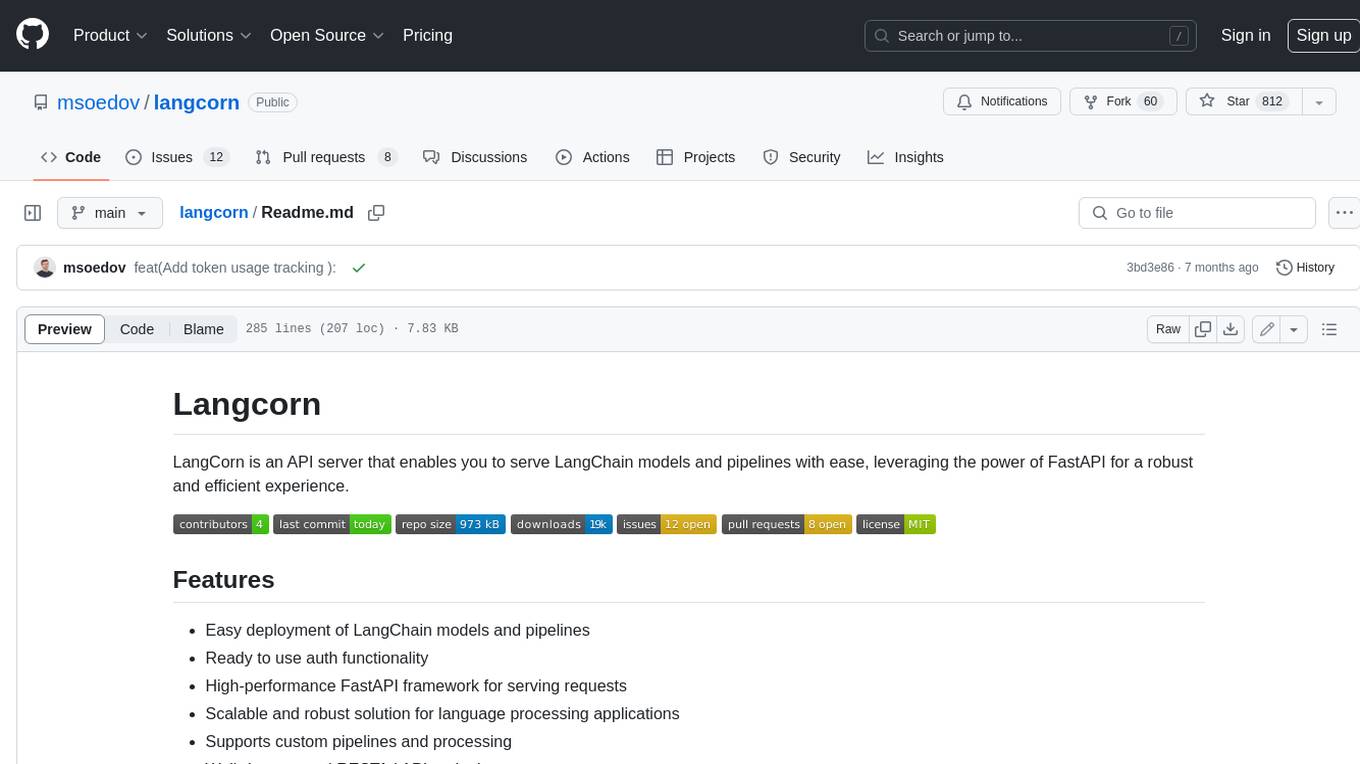
langcorn
LangCorn is an API server that enables you to serve LangChain models and pipelines with ease, leveraging the power of FastAPI for a robust and efficient experience. It offers features such as easy deployment of LangChain models and pipelines, ready-to-use authentication functionality, high-performance FastAPI framework for serving requests, scalability and robustness for language processing applications, support for custom pipelines and processing, well-documented RESTful API endpoints, and asynchronous processing for faster response times.
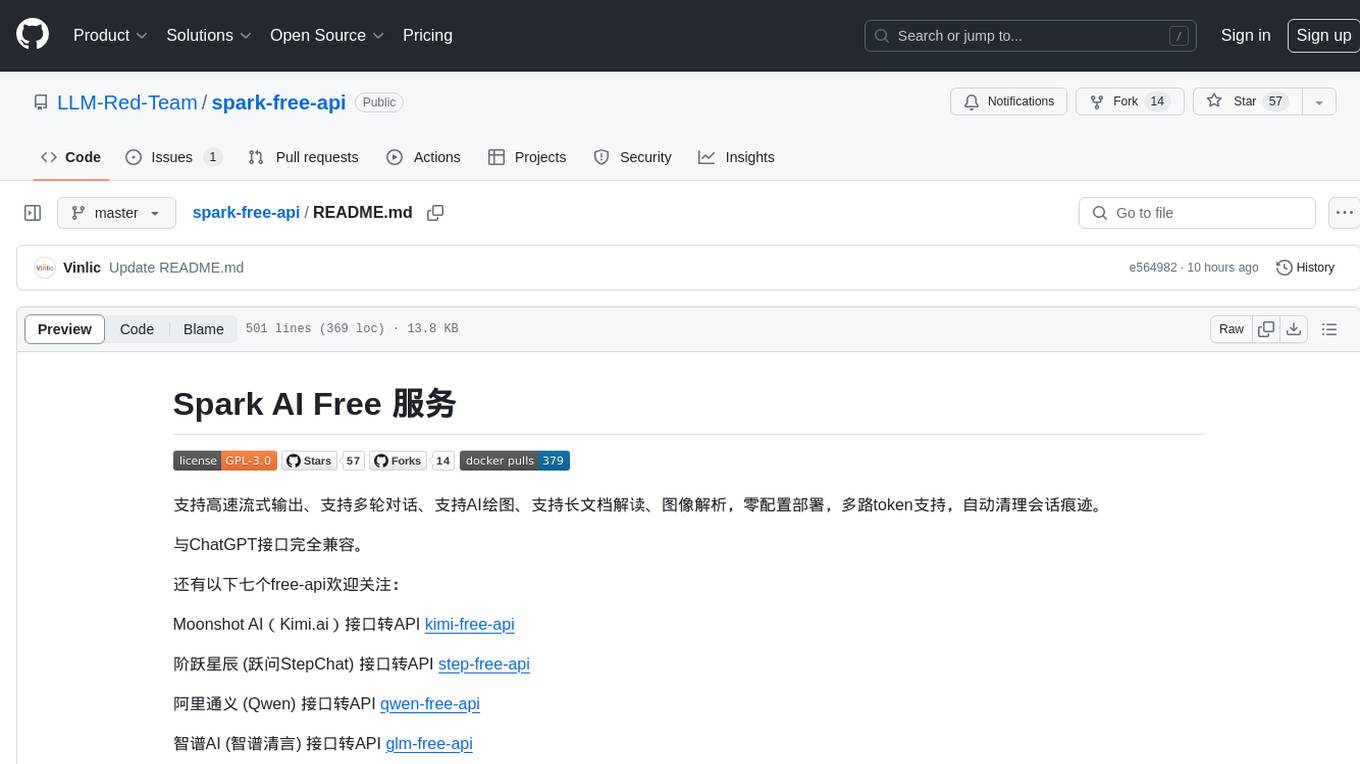
spark-free-api
Spark AI Free 服务 provides high-speed streaming output, multi-turn dialogue support, AI drawing support, long document interpretation, and image parsing. It offers zero-configuration deployment, multi-token support, and automatic session trace cleaning. It is fully compatible with the ChatGPT interface. The repository includes multiple free-api projects for various AI services. Users can access the API for tasks such as chat completions, AI drawing, document interpretation, image analysis, and ssoSessionId live checking. The project also provides guidelines for deployment using Docker, Docker-compose, Render, Vercel, and native deployment methods. It recommends using custom clients for faster and simpler access to the free-api series projects.
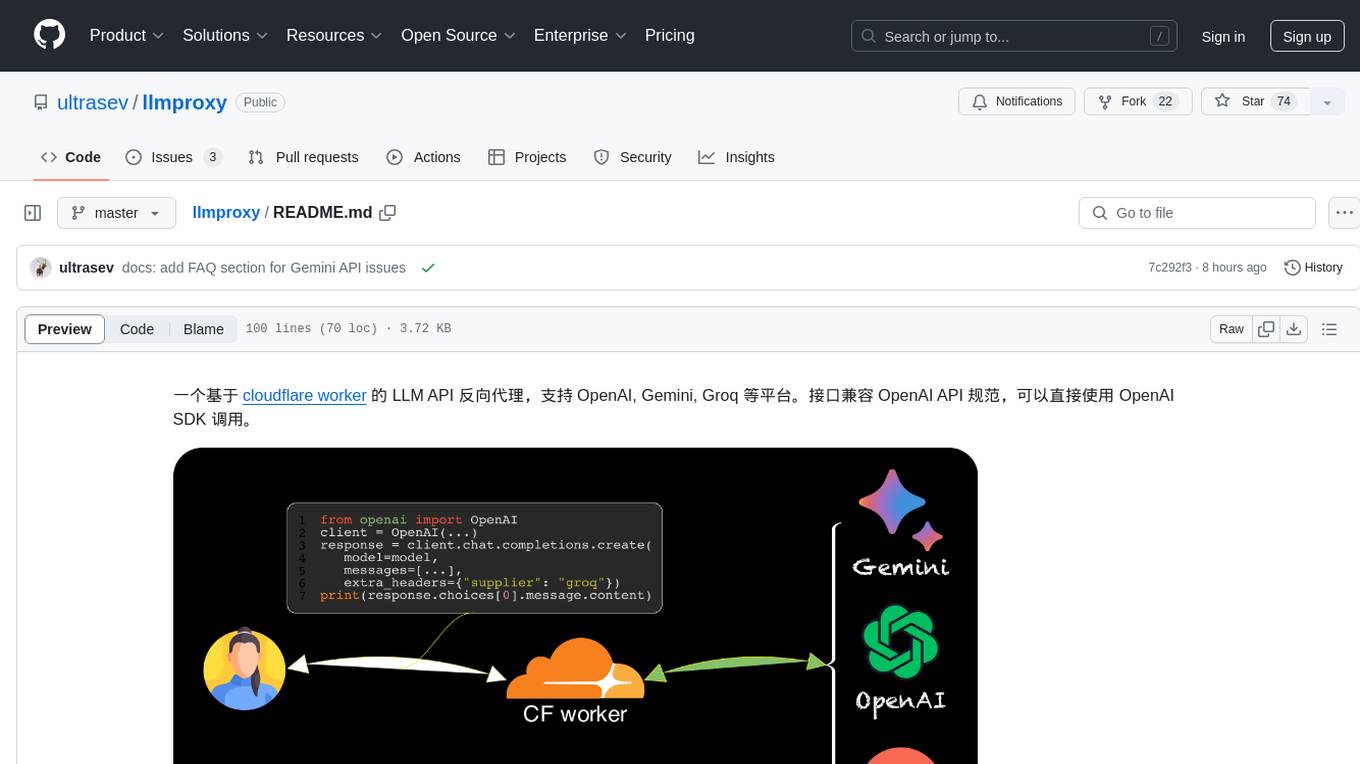
llmproxy
llmproxy is a reverse proxy for LLM API based on Cloudflare Worker, supporting platforms like OpenAI, Gemini, and Groq. The interface is compatible with the OpenAI API specification and can be directly accessed using the OpenAI SDK. It provides a convenient way to interact with various AI platforms through a unified API endpoint, enabling seamless integration and usage in different applications.
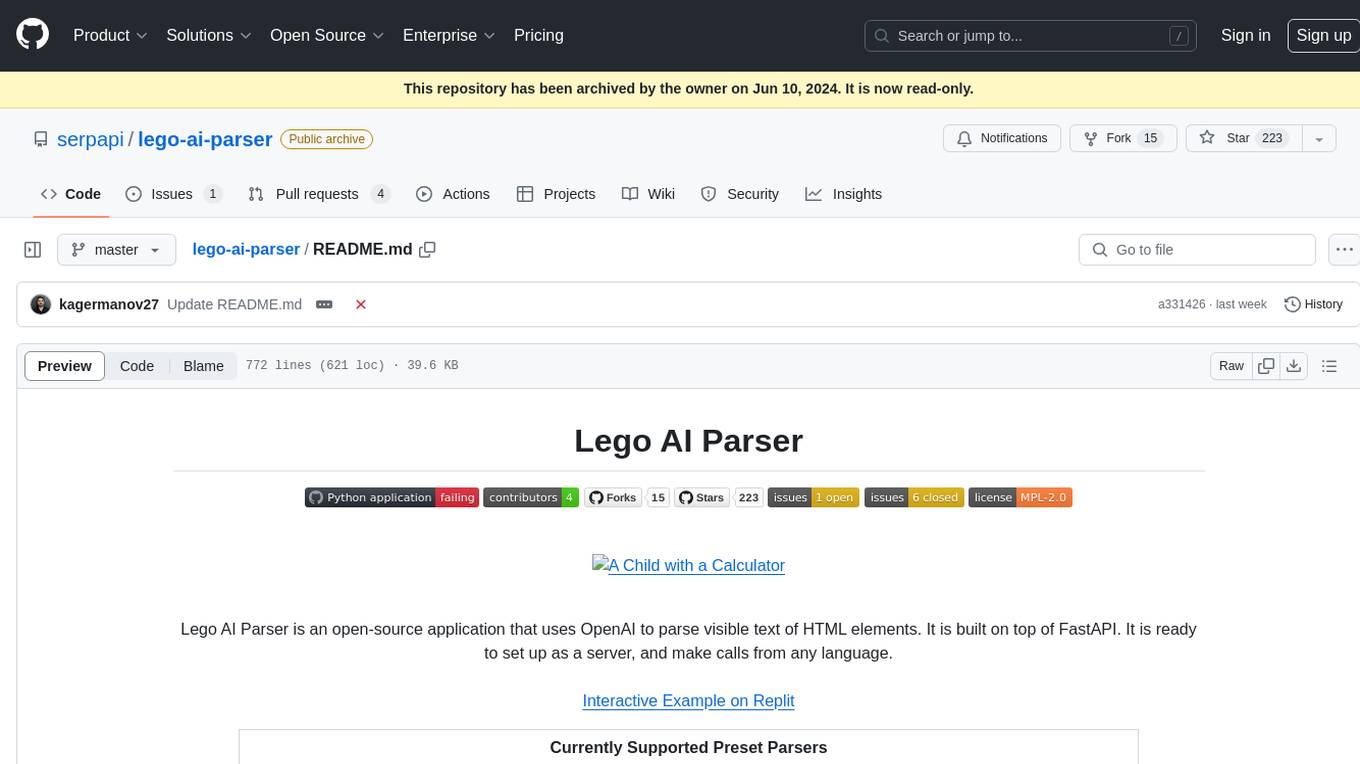
lego-ai-parser
Lego AI Parser is an open-source application that uses OpenAI to parse visible text of HTML elements. It is built on top of FastAPI, ready to set up as a server, and make calls from any language. It supports preset parsers for Google Local Results, Amazon Listings, Etsy Listings, Wayfair Listings, BestBuy Listings, Costco Listings, Macy's Listings, and Nordstrom Listings. Users can also design custom parsers by providing prompts, examples, and details about the OpenAI model under the classifier key.
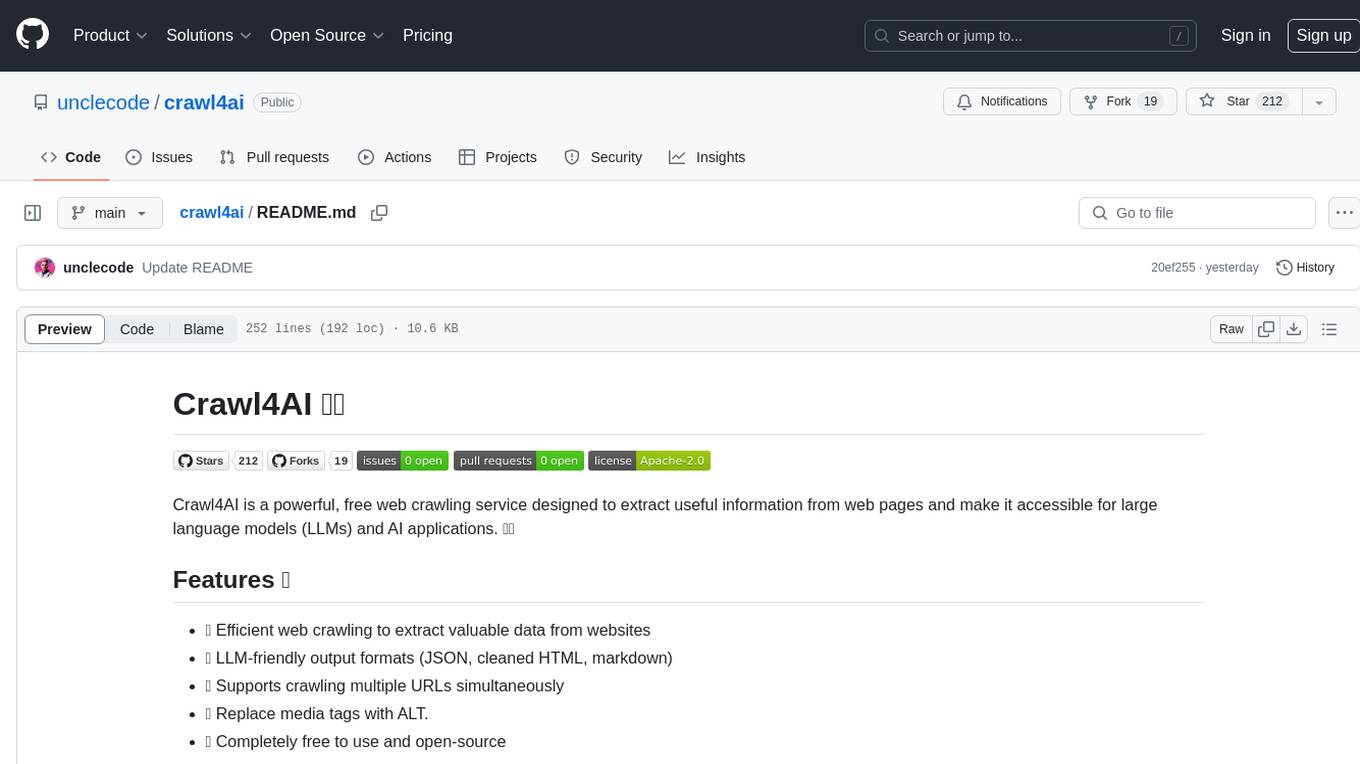
crawl4ai
Crawl4AI is a powerful and free web crawling service that extracts valuable data from websites and provides LLM-friendly output formats. It supports crawling multiple URLs simultaneously, replaces media tags with ALT, and is completely free to use and open-source. Users can integrate Crawl4AI into Python projects as a library or run it as a standalone local server. The tool allows users to crawl and extract data from specified URLs using different providers and models, with options to include raw HTML content, force fresh crawls, and extract meaningful text blocks. Configuration settings can be adjusted in the `crawler/config.py` file to customize providers, API keys, chunk processing, and word thresholds. Contributions to Crawl4AI are welcome from the open-source community to enhance its value for AI enthusiasts and developers.
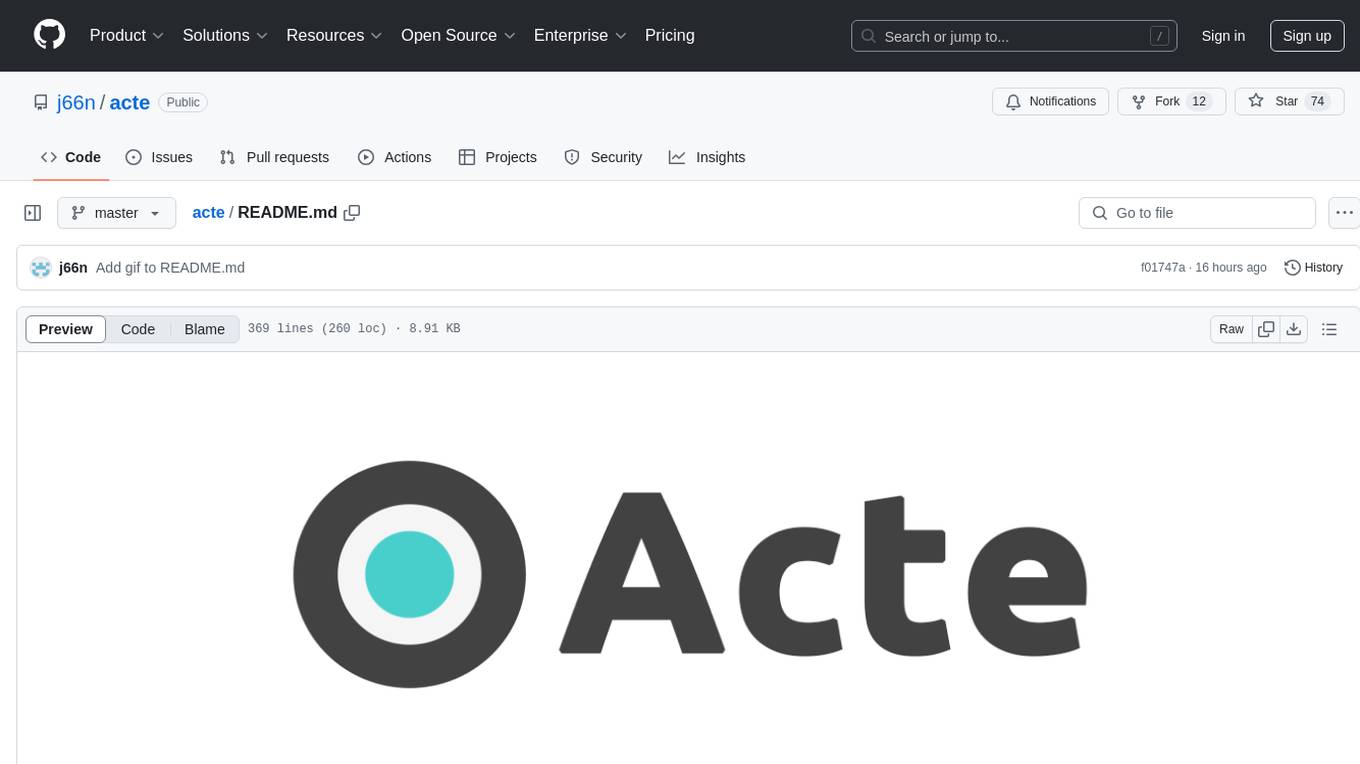
acte
Acte is a framework designed to build GUI-like tools for AI Agents. It aims to address the issues of cognitive load and freedom degrees when interacting with multiple APIs in complex scenarios. By providing a graphical user interface (GUI) for Agents, Acte helps reduce cognitive load and constraints interaction, similar to how humans interact with computers through GUIs. The tool offers APIs for starting new sessions, executing actions, and displaying screens, accessible via HTTP requests or the SessionManager class.
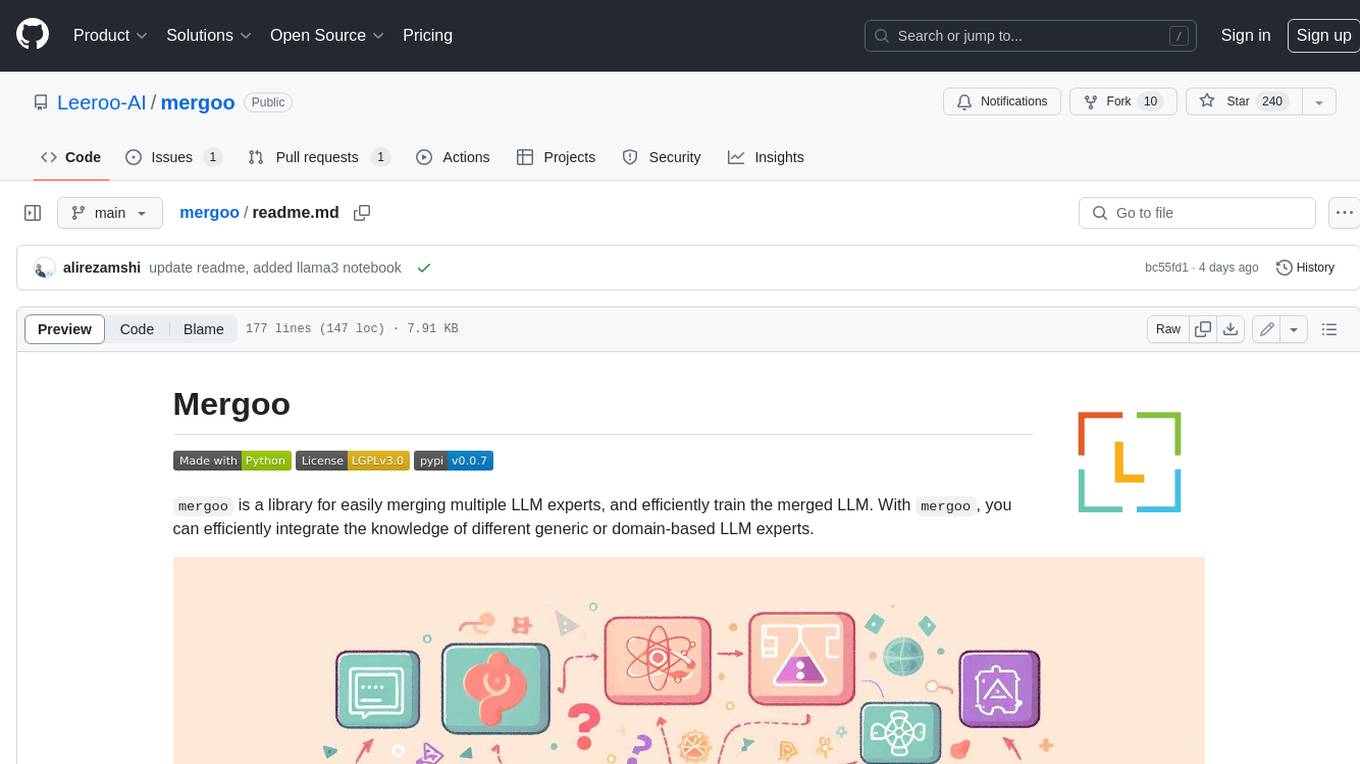
mergoo
Mergoo is a library for easily merging multiple LLM experts and efficiently training the merged LLM. With Mergoo, you can efficiently integrate the knowledge of different generic or domain-based LLM experts. Mergoo supports several merging methods, including Mixture-of-Experts, Mixture-of-Adapters, and Layer-wise merging. It also supports various base models, including LLaMa, Mistral, and BERT, and trainers, including Hugging Face Trainer, SFTrainer, and PEFT. Mergoo provides flexible merging for each layer and supports training choices such as only routing MoE layers or fully fine-tuning the merged LLM.
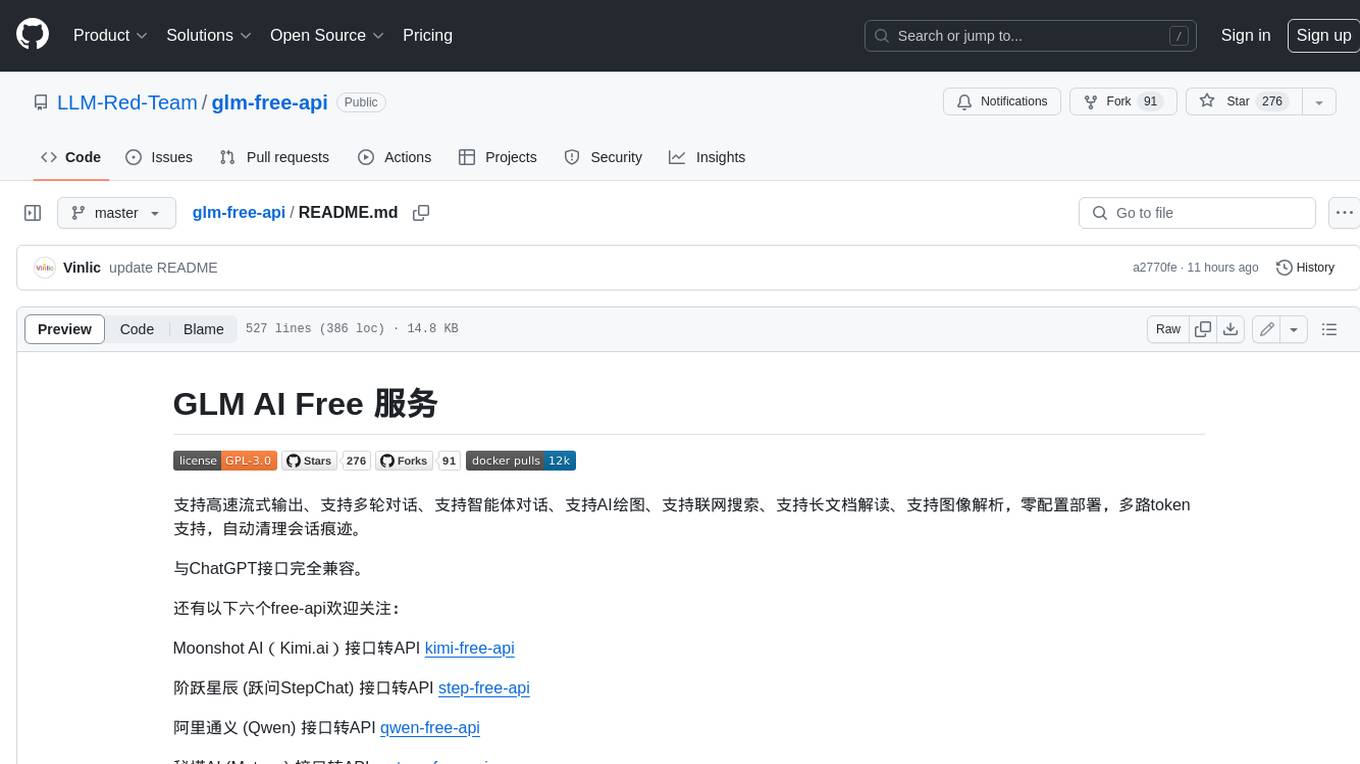
glm-free-api
GLM AI Free 服务 provides high-speed streaming output, multi-turn dialogue support, intelligent agent dialogue support, AI drawing support, online search support, long document interpretation support, image parsing support. It offers zero-configuration deployment, multi-token support, and automatic session trace cleaning. It is fully compatible with the ChatGPT interface. The repository also includes six other free APIs for various services like Moonshot AI, StepChat, Qwen, Metaso, Spark, and Emohaa. The tool supports tasks such as chat completions, AI drawing, document interpretation, image parsing, and refresh token survival check.
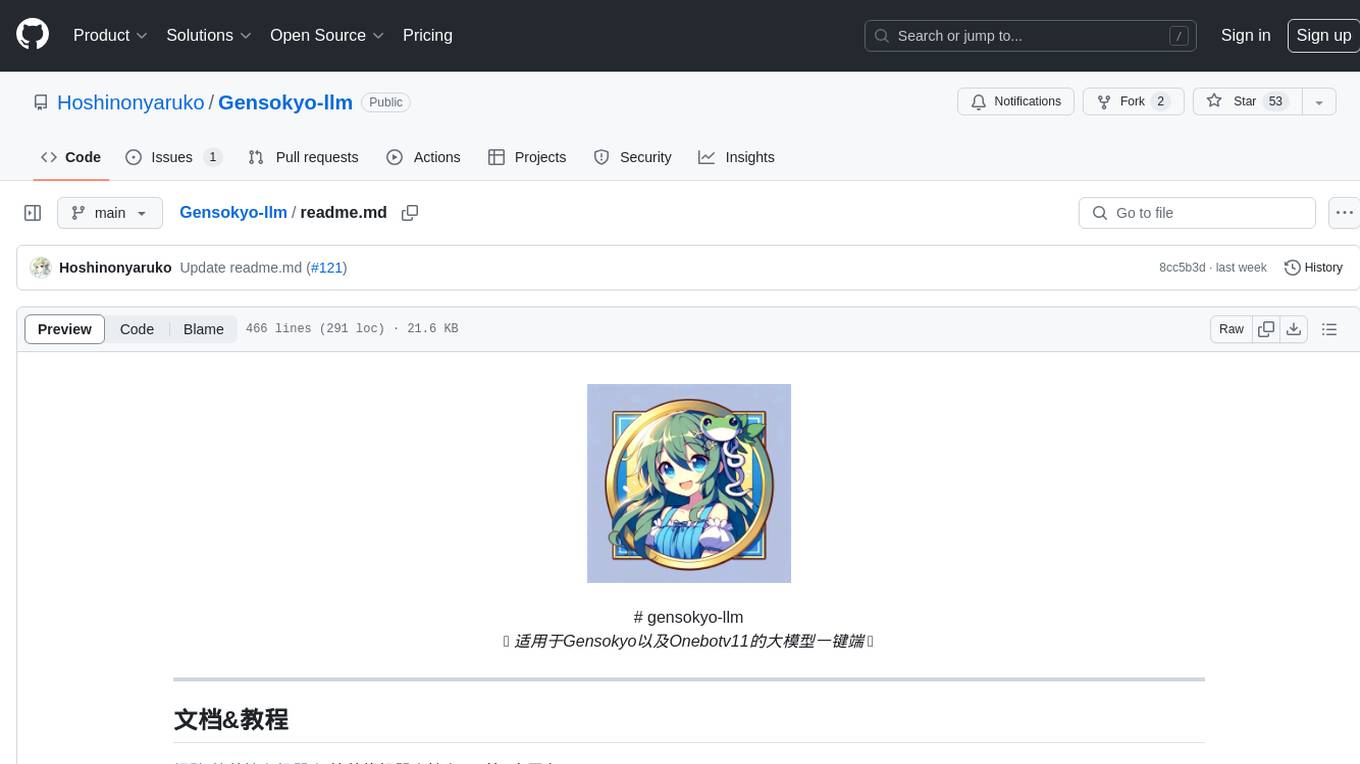
Gensokyo-llm
Gensokyo-llm is a tool designed for Gensokyo and Onebotv11, providing a one-click solution for large models. It supports various Onebotv11 standard frameworks, HTTP-API, and reverse WS. The tool is lightweight, with built-in SQLite for context maintenance and proxy support. It allows easy integration with the Gensokyo framework by configuring reverse HTTP and forward HTTP addresses. Users can set system settings, role cards, and context length. Additionally, it offers an openai original flavor API with automatic context. The tool can be used as an API or integrated with QQ channel robots. It supports converting GPT's SSE type and ensures memory safety in concurrent SSE environments. The tool also supports multiple users simultaneously transmitting SSE bidirectionally.
For similar tasks
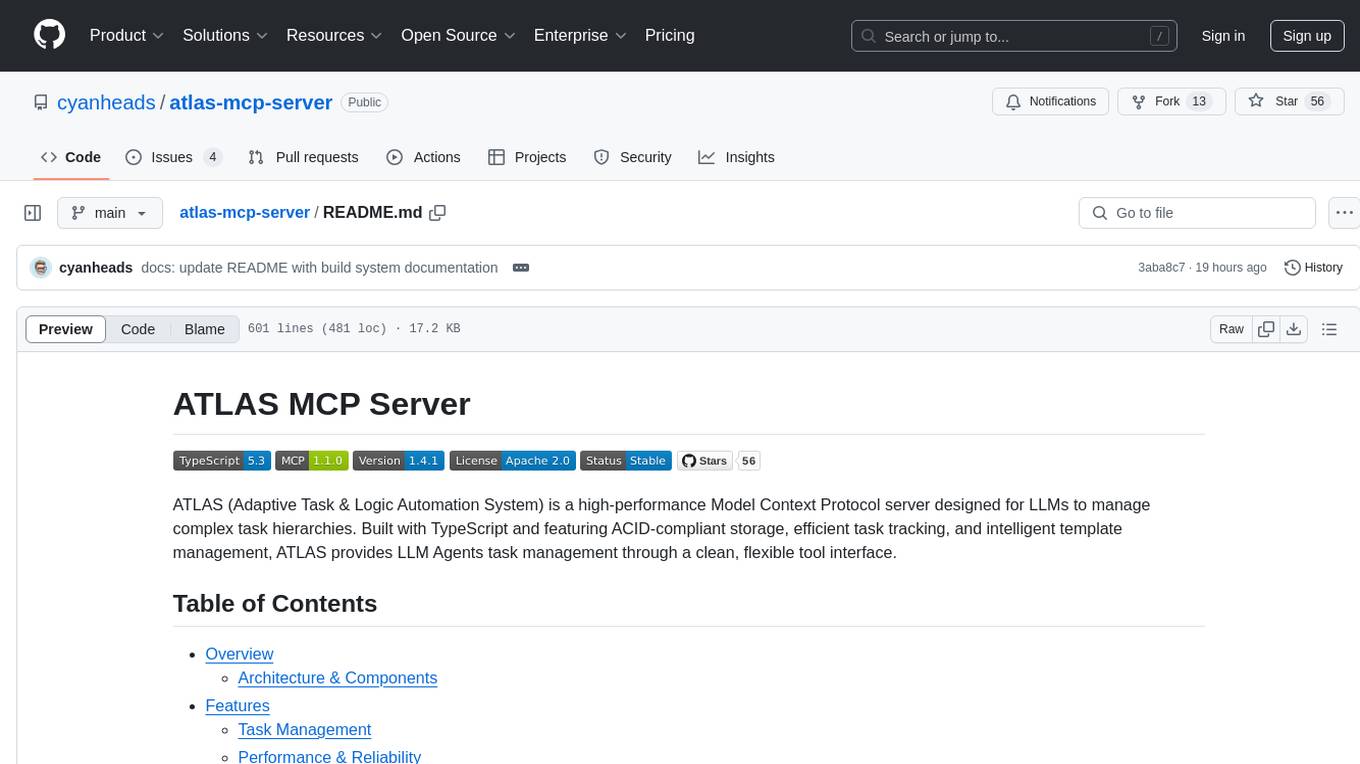
atlas-mcp-server
ATLAS (Adaptive Task & Logic Automation System) is a high-performance Model Context Protocol server designed for LLMs to manage complex task hierarchies. Built with TypeScript, it features ACID-compliant storage, efficient task tracking, and intelligent template management. ATLAS provides LLM Agents task management through a clean, flexible tool interface. The server implements the Model Context Protocol (MCP) for standardized communication between LLMs and external systems, offering hierarchical task organization, task state management, smart templates, enterprise features, and performance optimization.
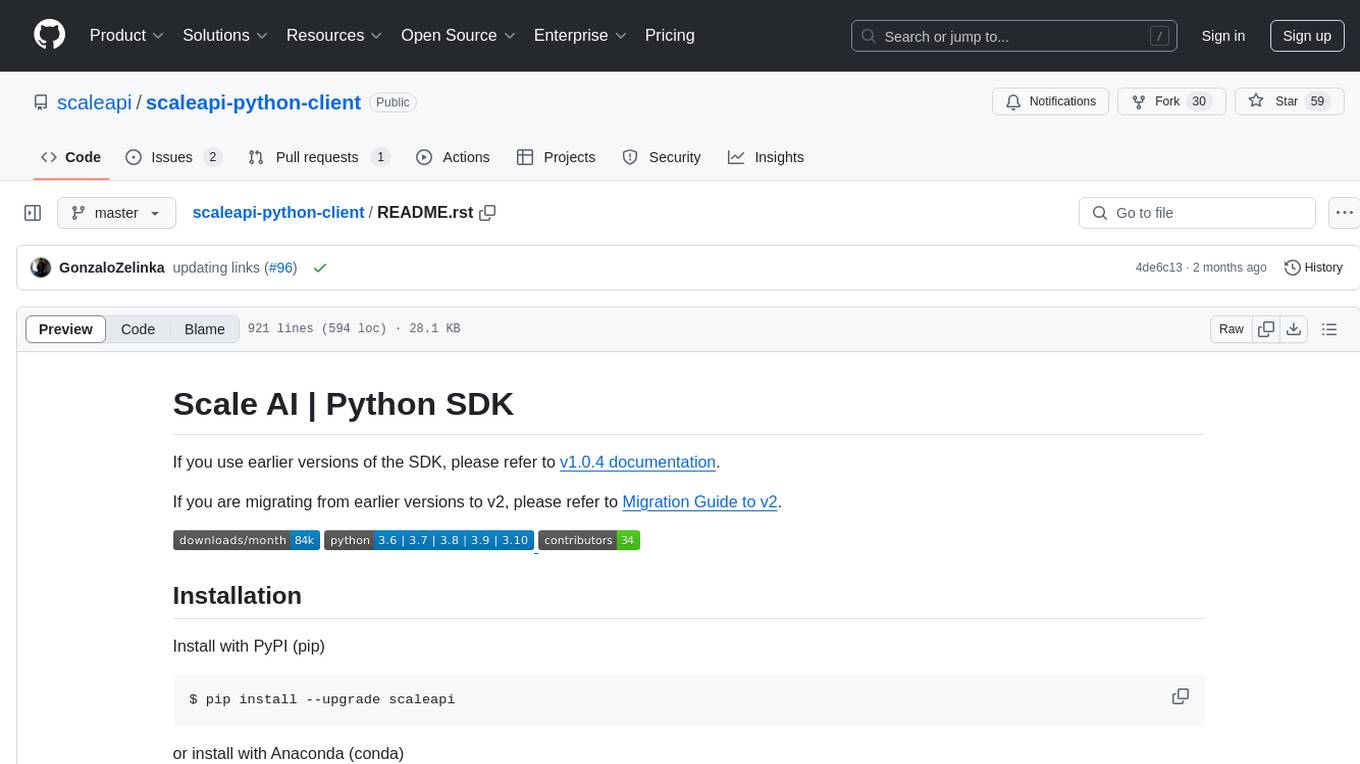
scaleapi-python-client
The Scale AI Python SDK is a tool that provides a Python interface for interacting with the Scale API. It allows users to easily create tasks, manage projects, upload files, and work with evaluation tasks, training tasks, and Studio assignments. The SDK handles error handling and provides detailed documentation for each method. Users can also manage teammates, project groups, and batches within the Scale Studio environment. The SDK supports various functionalities such as creating tasks, retrieving tasks, canceling tasks, auditing tasks, updating task attributes, managing files, managing team members, and working with evaluation and training tasks.
For similar jobs
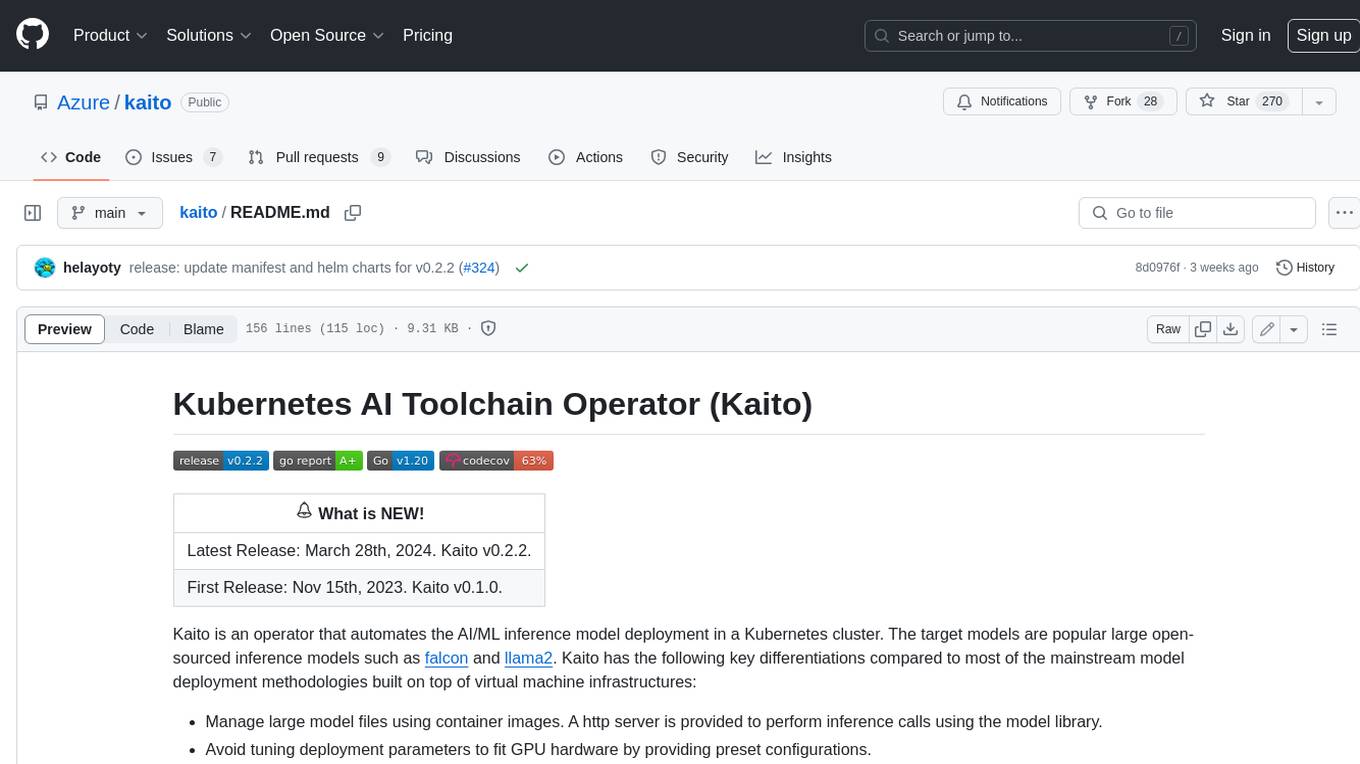
kaito
Kaito is an operator that automates the AI/ML inference model deployment in a Kubernetes cluster. It manages large model files using container images, avoids tuning deployment parameters to fit GPU hardware by providing preset configurations, auto-provisions GPU nodes based on model requirements, and hosts large model images in the public Microsoft Container Registry (MCR) if the license allows. Using Kaito, the workflow of onboarding large AI inference models in Kubernetes is largely simplified.
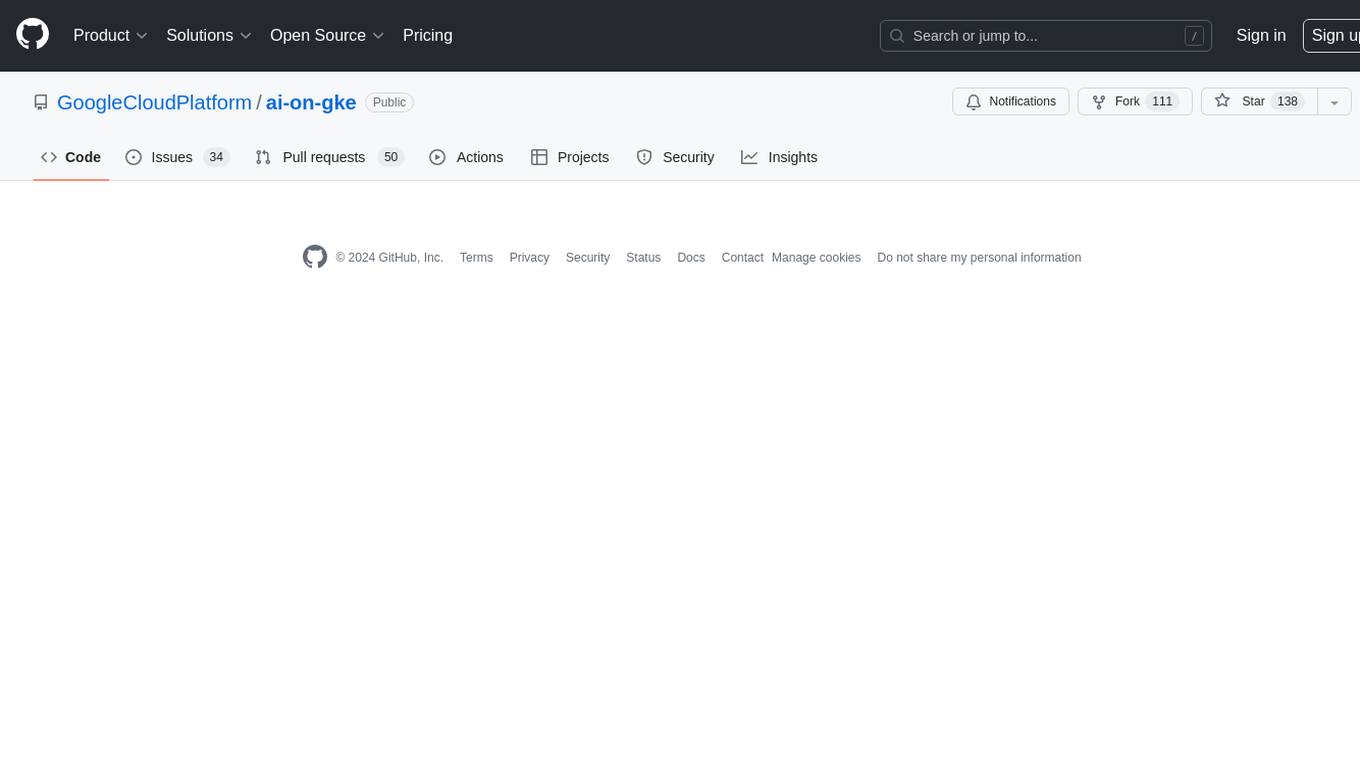
ai-on-gke
This repository contains assets related to AI/ML workloads on Google Kubernetes Engine (GKE). Run optimized AI/ML workloads with Google Kubernetes Engine (GKE) platform orchestration capabilities. A robust AI/ML platform considers the following layers: Infrastructure orchestration that support GPUs and TPUs for training and serving workloads at scale Flexible integration with distributed computing and data processing frameworks Support for multiple teams on the same infrastructure to maximize utilization of resources
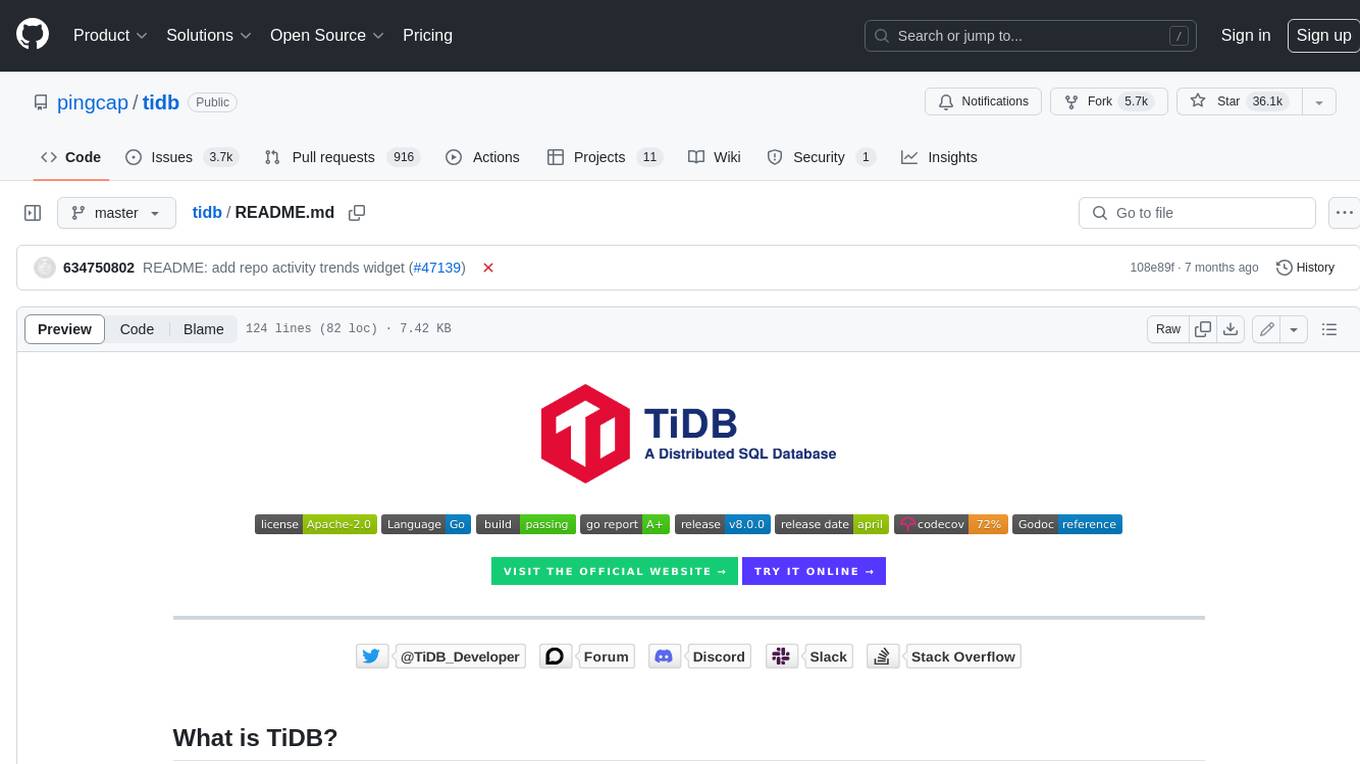
tidb
TiDB is an open-source distributed SQL database that supports Hybrid Transactional and Analytical Processing (HTAP) workloads. It is MySQL compatible and features horizontal scalability, strong consistency, and high availability.
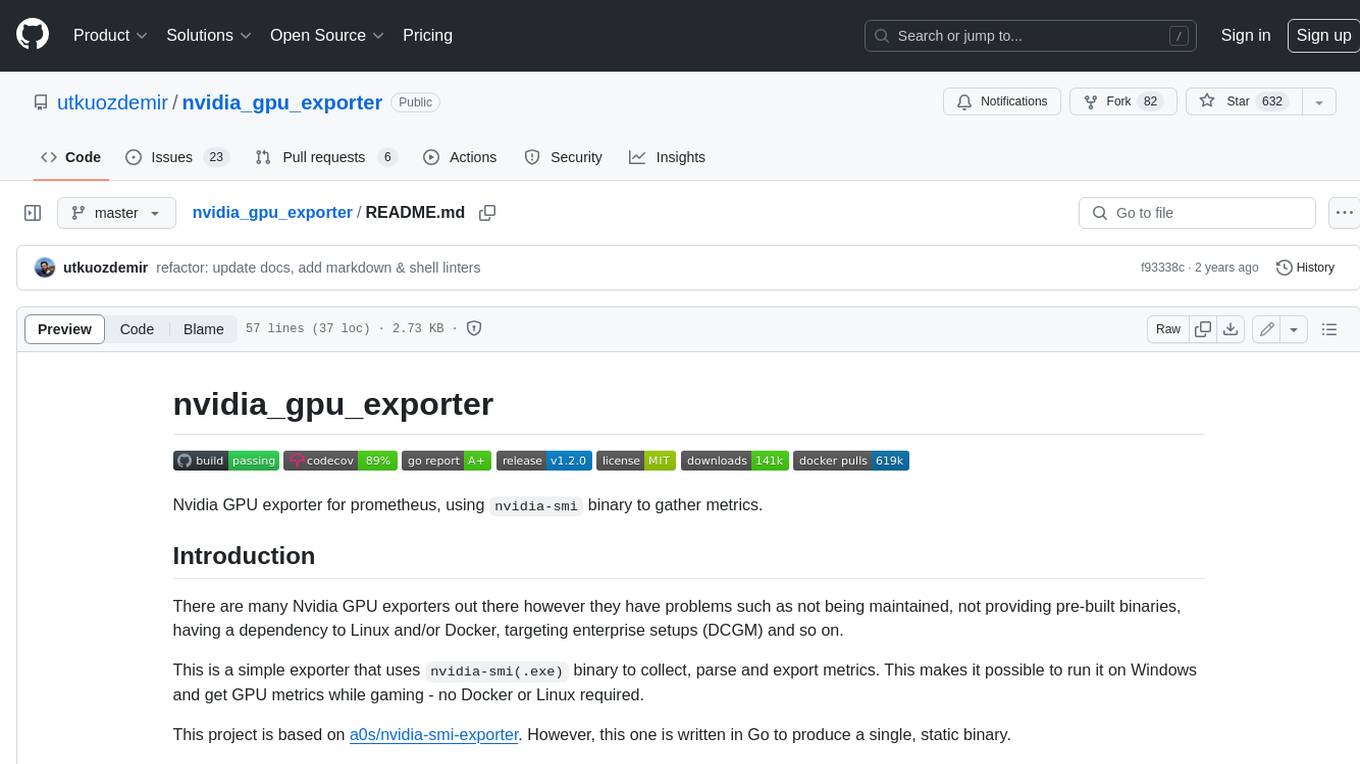
nvidia_gpu_exporter
Nvidia GPU exporter for prometheus, using `nvidia-smi` binary to gather metrics.
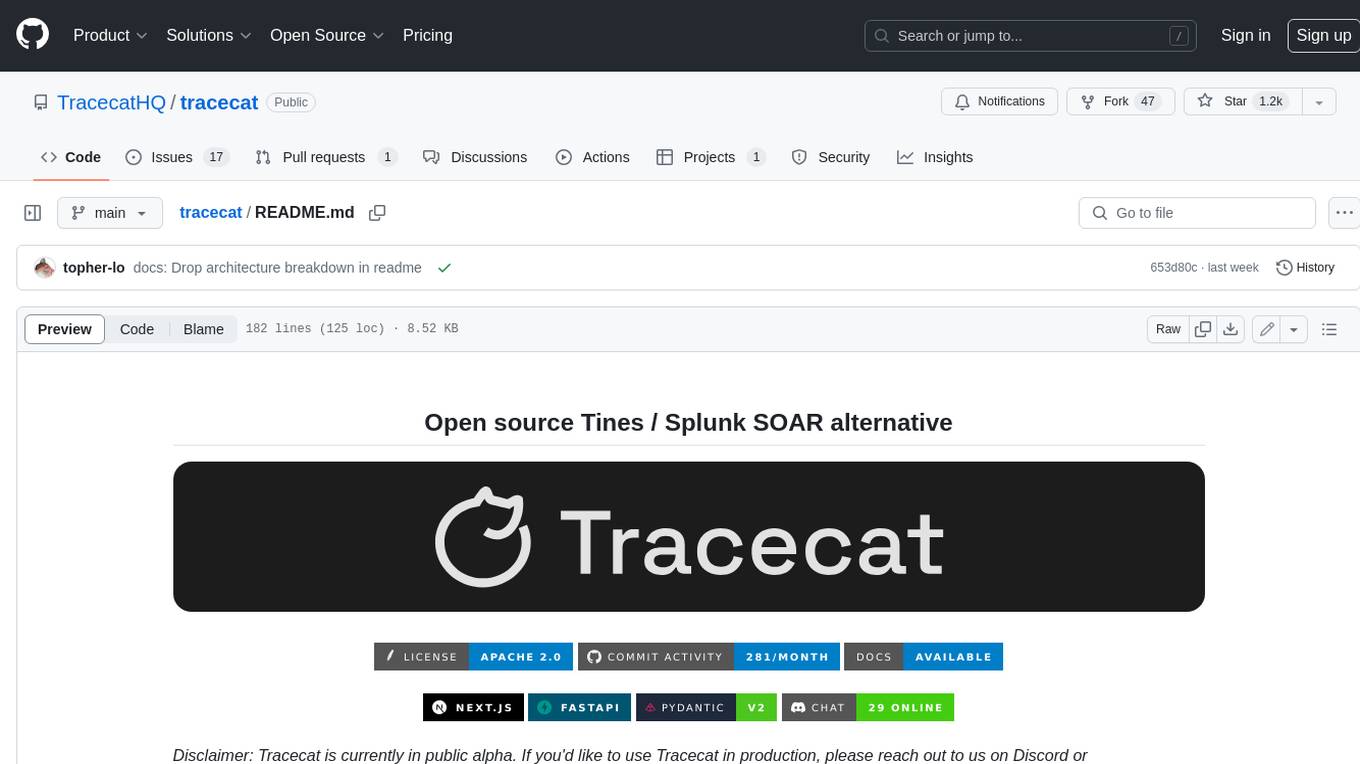
tracecat
Tracecat is an open-source automation platform for security teams. It's designed to be simple but powerful, with a focus on AI features and a practitioner-obsessed UI/UX. Tracecat can be used to automate a variety of tasks, including phishing email investigation, evidence collection, and remediation plan generation.
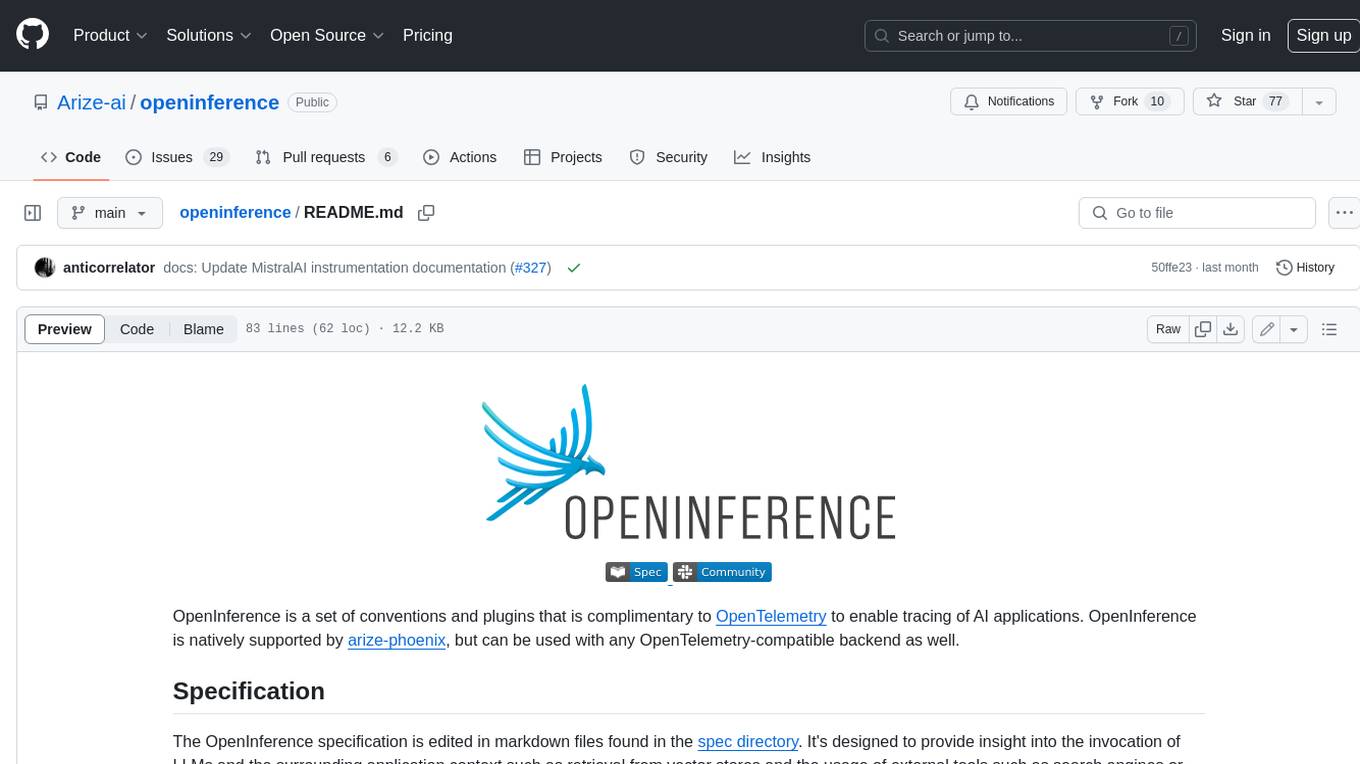
openinference
OpenInference is a set of conventions and plugins that complement OpenTelemetry to enable tracing of AI applications. It provides a way to capture and analyze the performance and behavior of AI models, including their interactions with other components of the application. OpenInference is designed to be language-agnostic and can be used with any OpenTelemetry-compatible backend. It includes a set of instrumentations for popular machine learning SDKs and frameworks, making it easy to add tracing to your AI applications.
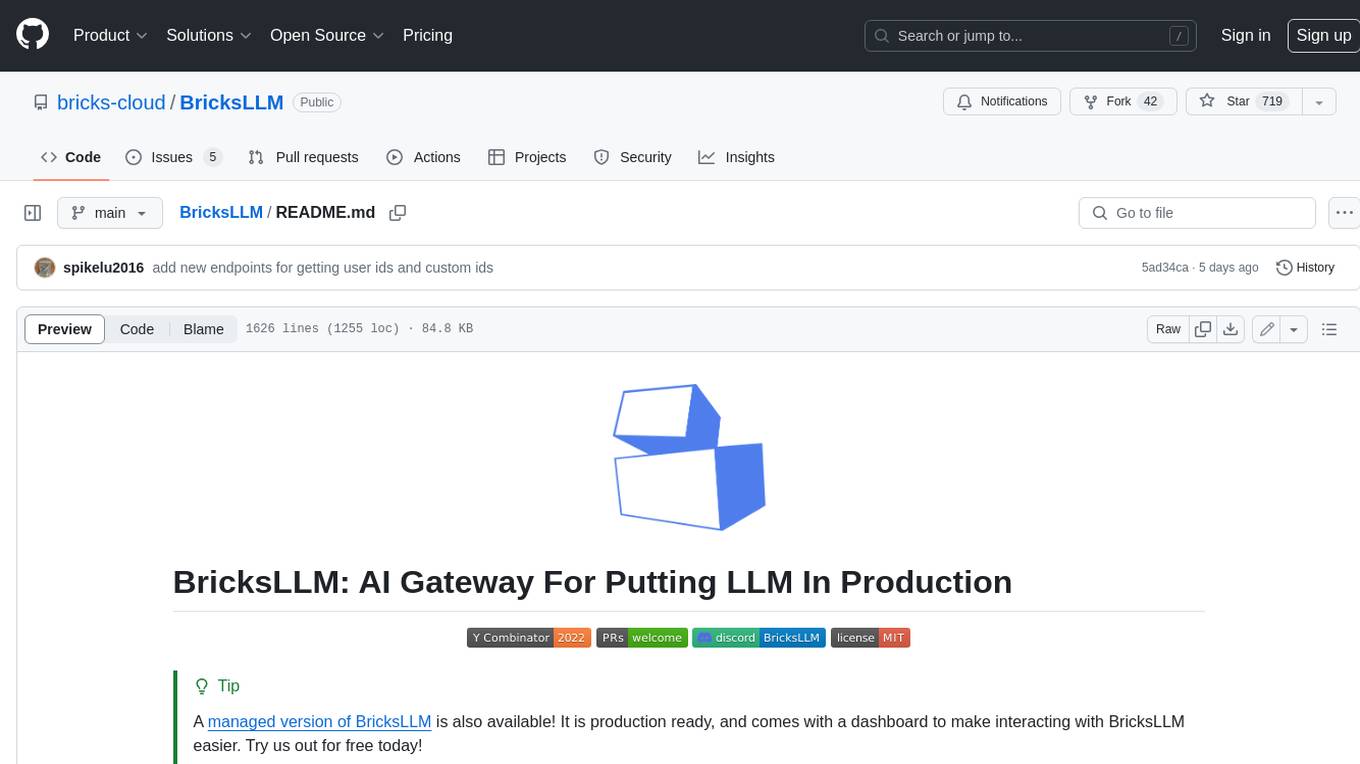
BricksLLM
BricksLLM is a cloud native AI gateway written in Go. Currently, it provides native support for OpenAI, Anthropic, Azure OpenAI and vLLM. BricksLLM aims to provide enterprise level infrastructure that can power any LLM production use cases. Here are some use cases for BricksLLM: * Set LLM usage limits for users on different pricing tiers * Track LLM usage on a per user and per organization basis * Block or redact requests containing PIIs * Improve LLM reliability with failovers, retries and caching * Distribute API keys with rate limits and cost limits for internal development/production use cases * Distribute API keys with rate limits and cost limits for students
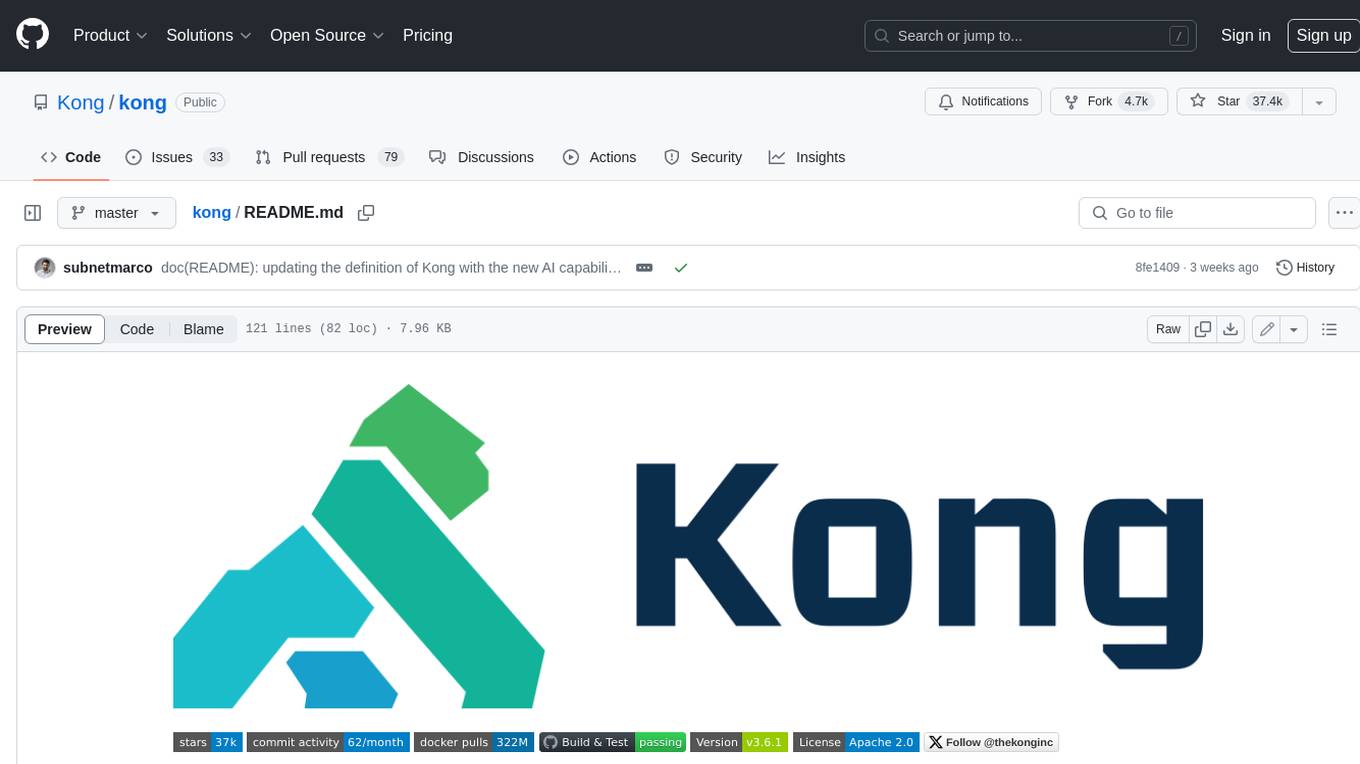
kong
Kong, or Kong API Gateway, is a cloud-native, platform-agnostic, scalable API Gateway distinguished for its high performance and extensibility via plugins. It also provides advanced AI capabilities with multi-LLM support. By providing functionality for proxying, routing, load balancing, health checking, authentication (and more), Kong serves as the central layer for orchestrating microservices or conventional API traffic with ease. Kong runs natively on Kubernetes thanks to its official Kubernetes Ingress Controller.